apollo-server-demo/ 0000755 0001750 0000144 00000000000 14067647700 013765 5 ustar andreh users apollo-server-demo/src/ 0000755 0001750 0000144 00000000000 14056722463 014552 5 ustar andreh users apollo-server-demo/src/03.js 0000644 0001750 0000144 00000001032 14056722463 015326 0 ustar andreh users const { ApolloServer, gql } = require("apollo-server"); const schema = ` type Query { hello: String goodbye: String } `; const typeDefs = gql(schema); const resolvers = { Query: () => ({ hello: () => "hi", }), }; const server = new ApolloServer({ typeDefs, mocks: resolvers, }); server.listen({ port: 5000 }).then(({ url }) => { console.log(`Listening on ${url} Now the mocking + overrides are working again. Try querying for both attributes. What changed in the response? What changed in the code?`); }); apollo-server-demo/src/07.js 0000644 0001750 0000144 00000002553 14056722463 015343 0 ustar andreh users const express = require("express"); const { ApolloServer, gql } = require("apollo-server-express"); const { buildClientSchema, printSchema } = require("graphql/utilities"); const { makeExecutableSchema } = require("graphql-tools"); const fs = require("fs"); let schemaJSON; try { schemaJSON = JSON.parse(fs.readFileSync("src/server-schema.json", "UTF-8")); } catch (e) { if (e.code === "ENOENT") { console.log(`File src/server-schema.json is missing. Try filling it with the schema of the server running in production.`); } else { console.error(e); } process.exit(1); } const schemaTXT = printSchema(buildClientSchema(schemaJSON.data)); const typeDefs = gql(schemaTXT); const resolvers = { FIXME: () => ({ FIXME: (_a, _b, context) => { console.log(context); if (context.authorization === "USER-A") { return null; } else { return null; } }, }), }; const server = new ApolloServer({ typeDefs, mocks: resolvers, playground: {}, context: ({ req }) => ({ authorization: (req.headers["authorization"] || "").replace(/Bearer /, "") }), }); const app = express(); server.applyMiddleware({ app }); const port = 5000; app.listen({ port }, () => { console.log(`Listening on http://localhost:${port}${server.graphqlPath} Now we'll have to use custom headers that will be available when overriding.`); }); apollo-server-demo/src/06.js 0000644 0001750 0000144 00000002162 14056722463 015336 0 ustar andreh users const express = require("express"); const { ApolloServer, gql } = require("apollo-server-express"); const { buildClientSchema, printSchema } = require("graphql/utilities"); const { makeExecutableSchema } = require("graphql-tools"); const fs = require("fs"); let schemaJSON; try { schemaJSON = JSON.parse(fs.readFileSync("src/server-schema.json", "UTF-8")); } catch (e) { if (e.code === "ENOENT") { console.log(`File src/server-schema.json is missing. Try filling it with the schema of the server running in production.`); } else { console.error(e); } process.exit(1); } const schemaTXT = printSchema(buildClientSchema(schemaJSON.data)); const typeDefs = gql(schemaTXT); const resolvers = { FIXME: () => ({ FIXME: () => "FIXME", }), }; const server = new ApolloServer({ typeDefs, mocks: resolvers, playground: {}, }); const app = express(); server.applyMiddleware({ app }); const port = 5000; app.listen({ port }, () => { console.log(`Listening on http://localhost:${port}${server.graphqlPath} Now try using the UI and making queries. How to add custom overrides to the "resolvers" object?`); }); apollo-server-demo/src/04.js 0000644 0001750 0000144 00000001131 14056722463 015327 0 ustar andreh users const express = require("express"); const { ApolloServer, gql } = require("apollo-server-express"); const typeDefs = gql` type Query { hello: String goodbye: String } `; const resolvers = { Query: () => ({ hello: () => "hi still", }), }; const server = new ApolloServer({ typeDefs, mocks: resolvers, }); const app = express(); server.applyMiddleware({ app }); const port = 5000; app.listen({ port }, () => { console.log(`Listening on http://localhost:${port}${server.graphqlPath} The code is refactored, but behaves the same. What changed? What is a middleware?`); }); apollo-server-demo/src/00.js 0000644 0001750 0000144 00000001004 14056722463 015322 0 ustar andreh users const { ApolloServer, gql } = require("apollo-server"); const schema = ` type Query { hello: String } `; const typeDefs = gql(schema); const server = new ApolloServer({ typeDefs, }); server.listen({ port: 5000 }).then(({ url }) => { console.log(`Listening on ${url} Given the above URL and the following GraphQL schema: ${schema} What is the curl command to query it? The expected response is: {"data":{"hello":null}} After getting the correct response, move to the next sequential file.`); }); apollo-server-demo/src/02.js 0000644 0001750 0000144 00000001155 14056722463 015333 0 ustar andreh users const { ApolloServer, gql } = require("apollo-server"); const schema = ` type Query { hello: String goodbye: String } `; const typeDefs = gql(schema); const resolvers = { Query: { hello: () => "hi", }, }; const server = new ApolloServer({ typeDefs, resolvers, mocks: true, }); server.listen({ port: 5000 }).then(({ url }) => { console.log(`Listening on ${url} We have a new schema: ${schema} Let's query the new field. 1. Where does the value of the new field comes from? It isn't null, neither added to the resolvers. 2. Also, what happened to the value of the "hello" field?`); }); apollo-server-demo/src/05.js 0000644 0001750 0000144 00000001343 14056722463 015335 0 ustar andreh users const express = require("express"); const { ApolloServer, gql } = require("apollo-server-express"); const typeDefs = gql` type Query { hello: String goodbye: String } `; const resolvers = { Query: () => ({ hello: () => "hi still", }), }; const server = new ApolloServer({ typeDefs, mocks: resolvers, playground: {}, }); const app = express(); server.applyMiddleware({ app }); const port = 5000; app.listen({ port }, () => { console.log(`Listening on http://localhost:${port}${server.graphqlPath} We now have a "/ui"! Test it on the browser: http://localhost:${port}${server.graphqlPath}/ui How can it possibly do completion on the schema? What is an InstrospectionQuery? What changed in the code?`); }); apollo-server-demo/src/01.js 0000644 0001750 0000144 00000000720 14056722463 015327 0 ustar andreh users const { ApolloServer, gql } = require("apollo-server"); const typeDefs = gql` type Query { hello: String } `; const resolvers = { Query: { hello: () => "hi", }, }; const server = new ApolloServer({ typeDefs, resolvers, }); server.listen({ port: 5000 }).then(({ url }) => { console.log(`Listening on ${url} With the same curl as the previous step, the response is different. What changed in the response? What changed in the code?`); }); apollo-server-demo/description 0000644 0001750 0000144 00000000077 14056722463 016235 0 ustar andreh users Tutorial on creating a GraphQL mock server with apollo-server. apollo-server-demo/README.md 0000644 0001750 0000144 00000000304 14056722463 015237 0 ustar andreh users # apollo-server-demo Step-by-step tutorial building an Apollo GraphQL mock server. Install the dependencies with: ```shell $ npm ci ``` Start the first step with: ```shell $ node src/00.js ``` apollo-server-demo/package.json 0000644 0001750 0000144 00000000141 14056722463 016245 0 ustar andreh users { "dependencies": { "apollo-server": "2.19.2", "apollo-server-express": "2.19.2" } } apollo-server-demo/node_modules/ 0000755 0001750 0000144 00000000000 14067647701 016443 5 ustar andreh users apollo-server-demo/node_modules/combined-stream/ 0000755 0001750 0000144 00000000000 14067647701 021514 5 ustar andreh users apollo-server-demo/node_modules/combined-stream/Readme.md 0000644 0001750 0000144 00000010707 03560116604 023225 0 ustar andreh users # combined-stream A stream that emits multiple other streams one after another. **NB** Currently `combined-stream` works with streams version 1 only. There is ongoing effort to switch this library to streams version 2. Any help is welcome. :) Meanwhile you can explore other libraries that provide streams2 support with more or less compatibility with `combined-stream`. - [combined-stream2](https://www.npmjs.com/package/combined-stream2): A drop-in streams2-compatible replacement for the combined-stream module. - [multistream](https://www.npmjs.com/package/multistream): A stream that emits multiple other streams one after another. ## Installation ``` bash npm install combined-stream ``` ## Usage Here is a simple example that shows how you can use combined-stream to combine two files into one: ``` javascript var CombinedStream = require('combined-stream'); var fs = require('fs'); var combinedStream = CombinedStream.create(); combinedStream.append(fs.createReadStream('file1.txt')); combinedStream.append(fs.createReadStream('file2.txt')); combinedStream.pipe(fs.createWriteStream('combined.txt')); ``` While the example above works great, it will pause all source streams until they are needed. If you don't want that to happen, you can set `pauseStreams` to `false`: ``` javascript var CombinedStream = require('combined-stream'); var fs = require('fs'); var combinedStream = CombinedStream.create({pauseStreams: false}); combinedStream.append(fs.createReadStream('file1.txt')); combinedStream.append(fs.createReadStream('file2.txt')); combinedStream.pipe(fs.createWriteStream('combined.txt')); ``` However, what if you don't have all the source streams yet, or you don't want to allocate the resources (file descriptors, memory, etc.) for them right away? Well, in that case you can simply provide a callback that supplies the stream by calling a `next()` function: ``` javascript var CombinedStream = require('combined-stream'); var fs = require('fs'); var combinedStream = CombinedStream.create(); combinedStream.append(function(next) { next(fs.createReadStream('file1.txt')); }); combinedStream.append(function(next) { next(fs.createReadStream('file2.txt')); }); combinedStream.pipe(fs.createWriteStream('combined.txt')); ``` ## API ### CombinedStream.create([options]) Returns a new combined stream object. Available options are: * `maxDataSize` * `pauseStreams` The effect of those options is described below. ### combinedStream.pauseStreams = `true` Whether to apply back pressure to the underlaying streams. If set to `false`, the underlaying streams will never be paused. If set to `true`, the underlaying streams will be paused right after being appended, as well as when `delayedStream.pipe()` wants to throttle. ### combinedStream.maxDataSize = `2 * 1024 * 1024` The maximum amount of bytes (or characters) to buffer for all source streams. If this value is exceeded, `combinedStream` emits an `'error'` event. ### combinedStream.dataSize = `0` The amount of bytes (or characters) currently buffered by `combinedStream`. ### combinedStream.append(stream) Appends the given `stream` to the combinedStream object. If `pauseStreams` is set to `true, this stream will also be paused right away. `streams` can also be a function that takes one parameter called `next`. `next` is a function that must be invoked in order to provide the `next` stream, see example above. Regardless of how the `stream` is appended, combined-stream always attaches an `'error'` listener to it, so you don't have to do that manually. Special case: `stream` can also be a String or Buffer. ### combinedStream.write(data) You should not call this, `combinedStream` takes care of piping the appended streams into itself for you. ### combinedStream.resume() Causes `combinedStream` to start drain the streams it manages. The function is idempotent, and also emits a `'resume'` event each time which usually goes to the stream that is currently being drained. ### combinedStream.pause(); If `combinedStream.pauseStreams` is set to `false`, this does nothing. Otherwise a `'pause'` event is emitted, this goes to the stream that is currently being drained, so you can use it to apply back pressure. ### combinedStream.end(); Sets `combinedStream.writable` to false, emits an `'end'` event, and removes all streams from the queue. ### combinedStream.destroy(); Same as `combinedStream.end()`, except it emits a `'close'` event instead of `'end'`. ## License combined-stream is licensed under the MIT license. apollo-server-demo/node_modules/combined-stream/package.json 0000644 0001750 0000144 00000001552 03560116604 023772 0 ustar andreh users { "author": "Felix Geisendörfer <felix@debuggable.com> (http://debuggable.com/)", "name": "combined-stream", "description": "A stream that emits multiple other streams one after another.", "version": "1.0.8", "homepage": "https://github.com/felixge/node-combined-stream", "repository": { "type": "git", "url": "git://github.com/felixge/node-combined-stream.git" }, "main": "./lib/combined_stream", "scripts": { "test": "node test/run.js" }, "engines": { "node": ">= 0.8" }, "dependencies": { "delayed-stream": "~1.0.0" }, "devDependencies": { "far": "~0.0.7" }, "license": "MIT" ,"_resolved": "https://registry.npmjs.org/combined-stream/-/combined-stream-1.0.8.tgz" ,"_integrity": "sha512-FQN4MRfuJeHf7cBbBMJFXhKSDq+2kAArBlmRBvcvFE5BB1HZKXtSFASDhdlz9zOYwxh8lDdnvmMOe/+5cdoEdg==" ,"_from": "combined-stream@1.0.8" } apollo-server-demo/node_modules/combined-stream/License 0000644 0001750 0000144 00000002075 03560116604 023012 0 ustar andreh users Copyright (c) 2011 Debuggable Limited <felix@debuggable.com> Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/combined-stream/lib/ 0000755 0001750 0000144 00000000000 14067647701 022262 5 ustar andreh users apollo-server-demo/node_modules/combined-stream/lib/combined_stream.js 0000644 0001750 0000144 00000011117 03560116604 025741 0 ustar andreh users var util = require('util'); var Stream = require('stream').Stream; var DelayedStream = require('delayed-stream'); module.exports = CombinedStream; function CombinedStream() { this.writable = false; this.readable = true; this.dataSize = 0; this.maxDataSize = 2 * 1024 * 1024; this.pauseStreams = true; this._released = false; this._streams = []; this._currentStream = null; this._insideLoop = false; this._pendingNext = false; } util.inherits(CombinedStream, Stream); CombinedStream.create = function(options) { var combinedStream = new this(); options = options || {}; for (var option in options) { combinedStream[option] = options[option]; } return combinedStream; }; CombinedStream.isStreamLike = function(stream) { return (typeof stream !== 'function') && (typeof stream !== 'string') && (typeof stream !== 'boolean') && (typeof stream !== 'number') && (!Buffer.isBuffer(stream)); }; CombinedStream.prototype.append = function(stream) { var isStreamLike = CombinedStream.isStreamLike(stream); if (isStreamLike) { if (!(stream instanceof DelayedStream)) { var newStream = DelayedStream.create(stream, { maxDataSize: Infinity, pauseStream: this.pauseStreams, }); stream.on('data', this._checkDataSize.bind(this)); stream = newStream; } this._handleErrors(stream); if (this.pauseStreams) { stream.pause(); } } this._streams.push(stream); return this; }; CombinedStream.prototype.pipe = function(dest, options) { Stream.prototype.pipe.call(this, dest, options); this.resume(); return dest; }; CombinedStream.prototype._getNext = function() { this._currentStream = null; if (this._insideLoop) { this._pendingNext = true; return; // defer call } this._insideLoop = true; try { do { this._pendingNext = false; this._realGetNext(); } while (this._pendingNext); } finally { this._insideLoop = false; } }; CombinedStream.prototype._realGetNext = function() { var stream = this._streams.shift(); if (typeof stream == 'undefined') { this.end(); return; } if (typeof stream !== 'function') { this._pipeNext(stream); return; } var getStream = stream; getStream(function(stream) { var isStreamLike = CombinedStream.isStreamLike(stream); if (isStreamLike) { stream.on('data', this._checkDataSize.bind(this)); this._handleErrors(stream); } this._pipeNext(stream); }.bind(this)); }; CombinedStream.prototype._pipeNext = function(stream) { this._currentStream = stream; var isStreamLike = CombinedStream.isStreamLike(stream); if (isStreamLike) { stream.on('end', this._getNext.bind(this)); stream.pipe(this, {end: false}); return; } var value = stream; this.write(value); this._getNext(); }; CombinedStream.prototype._handleErrors = function(stream) { var self = this; stream.on('error', function(err) { self._emitError(err); }); }; CombinedStream.prototype.write = function(data) { this.emit('data', data); }; CombinedStream.prototype.pause = function() { if (!this.pauseStreams) { return; } if(this.pauseStreams && this._currentStream && typeof(this._currentStream.pause) == 'function') this._currentStream.pause(); this.emit('pause'); }; CombinedStream.prototype.resume = function() { if (!this._released) { this._released = true; this.writable = true; this._getNext(); } if(this.pauseStreams && this._currentStream && typeof(this._currentStream.resume) == 'function') this._currentStream.resume(); this.emit('resume'); }; CombinedStream.prototype.end = function() { this._reset(); this.emit('end'); }; CombinedStream.prototype.destroy = function() { this._reset(); this.emit('close'); }; CombinedStream.prototype._reset = function() { this.writable = false; this._streams = []; this._currentStream = null; }; CombinedStream.prototype._checkDataSize = function() { this._updateDataSize(); if (this.dataSize <= this.maxDataSize) { return; } var message = 'DelayedStream#maxDataSize of ' + this.maxDataSize + ' bytes exceeded.'; this._emitError(new Error(message)); }; CombinedStream.prototype._updateDataSize = function() { this.dataSize = 0; var self = this; this._streams.forEach(function(stream) { if (!stream.dataSize) { return; } self.dataSize += stream.dataSize; }); if (this._currentStream && this._currentStream.dataSize) { this.dataSize += this._currentStream.dataSize; } }; CombinedStream.prototype._emitError = function(err) { this._reset(); this.emit('error', err); }; apollo-server-demo/node_modules/combined-stream/yarn.lock 0000644 0001750 0000144 00000001047 03560116604 023326 0 ustar andreh users # THIS IS AN AUTOGENERATED FILE. DO NOT EDIT THIS FILE DIRECTLY. # yarn lockfile v1 delayed-stream@~1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/delayed-stream/-/delayed-stream-1.0.0.tgz#df3ae199acadfb7d440aaae0b29e2272b24ec619" far@~0.0.7: version "0.0.7" resolved "https://registry.yarnpkg.com/far/-/far-0.0.7.tgz#01c1fd362bcd26ce9cf161af3938aa34619f79a7" dependencies: oop "0.0.3" oop@0.0.3: version "0.0.3" resolved "https://registry.yarnpkg.com/oop/-/oop-0.0.3.tgz#70fa405a5650891a194fdc82ca68dad6dabf4401" apollo-server-demo/node_modules/object.getownpropertydescriptors/ 0000755 0001750 0000144 00000000000 14067647700 025301 5 ustar andreh users apollo-server-demo/node_modules/object.getownpropertydescriptors/index.js 0000644 0001750 0000144 00000000575 03560116604 026743 0 ustar andreh users 'use strict'; var define = require('define-properties'); var callBind = require('call-bind'); var implementation = require('./implementation'); var getPolyfill = require('./polyfill'); var shim = require('./shim'); var bound = callBind(getPolyfill(), Object); define(bound, { getPolyfill: getPolyfill, implementation: implementation, shim: shim }); module.exports = bound; apollo-server-demo/node_modules/object.getownpropertydescriptors/LICENSE 0000644 0001750 0000144 00000002072 03560116604 026275 0 ustar andreh users The MIT License (MIT) Copyright (c) 2015 Jordan Harband Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/object.getownpropertydescriptors/.nyc_output/ 0000755 0001750 0000144 00000000000 14067647700 027570 5 ustar andreh users ././@LongLink 0000644 0000000 0000000 00000000163 00000000000 011603 L ustar root root apollo-server-demo/node_modules/object.getownpropertydescriptors/.nyc_output/d854d13f5dfcddafaac1f326fdc04e6f.json apollo-server-demo/node_modules/object.getownpropertydescriptors/.nyc_output/d854d13f5dfcddafaac1f320000644 0001750 0000144 00000014643 03560116604 033275 0 ustar andreh users {"/Users/ljharb/Dropbox/git/Object.getOwnPropertyDescriptors.git/auto.js":{"path":"/Users/ljharb/Dropbox/git/Object.getOwnPropertyDescriptors.git/auto.js","statementMap":{"0":{"start":{"line":3,"column":0},"end":{"line":3,"column":20}}},"fnMap":{},"branchMap":{},"s":{"0":0},"f":{},"b":{}},"/Users/ljharb/Dropbox/git/Object.getOwnPropertyDescriptors.git/implementation.js":{"path":"/Users/ljharb/Dropbox/git/Object.getOwnPropertyDescriptors.git/implementation.js","statementMap":{"0":{"start":{"line":3,"column":25},"end":{"line":3,"column":71}},"1":{"start":{"line":4,"column":17},"end":{"line":4,"column":55}},"2":{"start":{"line":5,"column":29},"end":{"line":5,"column":79}},"3":{"start":{"line":6,"column":15},"end":{"line":6,"column":51}},"4":{"start":{"line":7,"column":16},"end":{"line":7,"column":46}},"5":{"start":{"line":9,"column":12},"end":{"line":9,"column":43}},"6":{"start":{"line":10,"column":19},"end":{"line":10,"column":45}},"7":{"start":{"line":11,"column":18},"end":{"line":11,"column":46}},"8":{"start":{"line":12,"column":14},"end":{"line":12,"column":49}},"9":{"start":{"line":13,"column":14},"end":{"line":13,"column":49}},"10":{"start":{"line":14,"column":13},"end":{"line":16,"column":16}},"11":{"start":{"line":15,"column":1},"end":{"line":15,"column":53}},"12":{"start":{"line":18,"column":12},"end":{"line":18,"column":57}},"13":{"start":{"line":20,"column":0},"end":{"line":38,"column":2}},"14":{"start":{"line":21,"column":1},"end":{"line":21,"column":31}},"15":{"start":{"line":22,"column":1},"end":{"line":24,"column":2}},"16":{"start":{"line":23,"column":2},"end":{"line":23,"column":92}},"17":{"start":{"line":26,"column":9},"end":{"line":26,"column":24}},"18":{"start":{"line":27,"column":1},"end":{"line":37,"column":3}},"19":{"start":{"line":30,"column":20},"end":{"line":30,"column":33}},"20":{"start":{"line":31,"column":3},"end":{"line":33,"column":4}},"21":{"start":{"line":32,"column":4},"end":{"line":32,"column":45}},"22":{"start":{"line":34,"column":3},"end":{"line":34,"column":14}}},"fnMap":{"0":{"name":"(anonymous_0)","decl":{"start":{"line":14,"column":27},"end":{"line":14,"column":28}},"loc":{"start":{"line":14,"column":42},"end":{"line":16,"column":1}},"line":14},"1":{"name":"getOwnPropertyDescriptors","decl":{"start":{"line":20,"column":26},"end":{"line":20,"column":51}},"loc":{"start":{"line":20,"column":59},"end":{"line":38,"column":1}},"line":20},"2":{"name":"(anonymous_2)","decl":{"start":{"line":29,"column":2},"end":{"line":29,"column":3}},"loc":{"start":{"line":29,"column":22},"end":{"line":35,"column":3}},"line":29}},"branchMap":{"0":{"loc":{"start":{"line":14,"column":13},"end":{"line":16,"column":16}},"type":"cond-expr","locations":[{"start":{"line":14,"column":27},"end":{"line":16,"column":1}},{"start":{"line":16,"column":4},"end":{"line":16,"column":16}}],"line":14},"1":{"loc":{"start":{"line":18,"column":12},"end":{"line":18,"column":57}},"type":"binary-expr","locations":[{"start":{"line":18,"column":12},"end":{"line":18,"column":29}},{"start":{"line":18,"column":33},"end":{"line":18,"column":57}}],"line":18},"2":{"loc":{"start":{"line":22,"column":1},"end":{"line":24,"column":2}},"type":"if","locations":[{"start":{"line":22,"column":1},"end":{"line":24,"column":2}},{"start":{"line":22,"column":1},"end":{"line":24,"column":2}}],"line":22},"3":{"loc":{"start":{"line":31,"column":3},"end":{"line":33,"column":4}},"type":"if","locations":[{"start":{"line":31,"column":3},"end":{"line":33,"column":4}},{"start":{"line":31,"column":3},"end":{"line":33,"column":4}}],"line":31}},"s":{"0":0,"1":0,"2":0,"3":0,"4":0,"5":0,"6":0,"7":0,"8":0,"9":0,"10":0,"11":0,"12":0,"13":0,"14":0,"15":0,"16":0,"17":0,"18":0,"19":0,"20":0,"21":0,"22":0},"f":{"0":0,"1":0,"2":0},"b":{"0":[0,0],"1":[0,0],"2":[0,0],"3":[0,0]}},"/Users/ljharb/Dropbox/git/Object.getOwnPropertyDescriptors.git/index.js":{"path":"/Users/ljharb/Dropbox/git/Object.getOwnPropertyDescriptors.git/index.js","statementMap":{"0":{"start":{"line":3,"column":13},"end":{"line":3,"column":41}},"1":{"start":{"line":4,"column":15},"end":{"line":4,"column":35}},"2":{"start":{"line":6,"column":21},"end":{"line":6,"column":48}},"3":{"start":{"line":7,"column":18},"end":{"line":7,"column":39}},"4":{"start":{"line":8,"column":11},"end":{"line":8,"column":28}},"5":{"start":{"line":10,"column":12},"end":{"line":10,"column":43}},"6":{"start":{"line":12,"column":0},"end":{"line":16,"column":3}},"7":{"start":{"line":18,"column":0},"end":{"line":18,"column":23}}},"fnMap":{},"branchMap":{},"s":{"0":0,"1":0,"2":0,"3":0,"4":0,"5":0,"6":0,"7":0},"f":{},"b":{}},"/Users/ljharb/Dropbox/git/Object.getOwnPropertyDescriptors.git/polyfill.js":{"path":"/Users/ljharb/Dropbox/git/Object.getOwnPropertyDescriptors.git/polyfill.js","statementMap":{"0":{"start":{"line":3,"column":21},"end":{"line":3,"column":48}},"1":{"start":{"line":5,"column":0},"end":{"line":7,"column":2}},"2":{"start":{"line":6,"column":1},"end":{"line":6,"column":115}}},"fnMap":{"0":{"name":"getPolyfill","decl":{"start":{"line":5,"column":26},"end":{"line":5,"column":37}},"loc":{"start":{"line":5,"column":40},"end":{"line":7,"column":1}},"line":5}},"branchMap":{"0":{"loc":{"start":{"line":6,"column":8},"end":{"line":6,"column":114}},"type":"cond-expr","locations":[{"start":{"line":6,"column":65},"end":{"line":6,"column":97}},{"start":{"line":6,"column":100},"end":{"line":6,"column":114}}],"line":6}},"s":{"0":0,"1":0,"2":0},"f":{"0":0},"b":{"0":[0,0]}},"/Users/ljharb/Dropbox/git/Object.getOwnPropertyDescriptors.git/shim.js":{"path":"/Users/ljharb/Dropbox/git/Object.getOwnPropertyDescriptors.git/shim.js","statementMap":{"0":{"start":{"line":3,"column":18},"end":{"line":3,"column":39}},"1":{"start":{"line":4,"column":13},"end":{"line":4,"column":41}},"2":{"start":{"line":6,"column":0},"end":{"line":14,"column":2}},"3":{"start":{"line":7,"column":16},"end":{"line":7,"column":29}},"4":{"start":{"line":8,"column":1},"end":{"line":12,"column":3}},"5":{"start":{"line":11,"column":45},"end":{"line":11,"column":98}},"6":{"start":{"line":13,"column":1},"end":{"line":13,"column":17}}},"fnMap":{"0":{"name":"shimGetOwnPropertyDescriptors","decl":{"start":{"line":6,"column":26},"end":{"line":6,"column":55}},"loc":{"start":{"line":6,"column":58},"end":{"line":14,"column":1}},"line":6},"1":{"name":"(anonymous_1)","decl":{"start":{"line":11,"column":31},"end":{"line":11,"column":32}},"loc":{"start":{"line":11,"column":43},"end":{"line":11,"column":100}},"line":11}},"branchMap":{},"s":{"0":0,"1":0,"2":0,"3":0,"4":0,"5":0,"6":0},"f":{"0":0,"1":0},"b":{}}} ././@LongLink 0000644 0000000 0000000 00000000163 00000000000 011603 L ustar root root apollo-server-demo/node_modules/object.getownpropertydescriptors/.nyc_output/fefd413c1f8369199b55c2067a0b6486.json apollo-server-demo/node_modules/object.getownpropertydescriptors/.nyc_output/fefd413c1f8369199b55c200000644 0001750 0000144 00000016352 03560116604 032654 0 ustar andreh users {"/Users/ljharb/Dropbox/git/Object.getOwnPropertyDescriptors.git/implementation.js":{"path":"/Users/ljharb/Dropbox/git/Object.getOwnPropertyDescriptors.git/implementation.js","statementMap":{"0":{"start":{"line":3,"column":25},"end":{"line":3,"column":71}},"1":{"start":{"line":4,"column":17},"end":{"line":4,"column":55}},"2":{"start":{"line":5,"column":29},"end":{"line":5,"column":79}},"3":{"start":{"line":6,"column":15},"end":{"line":6,"column":51}},"4":{"start":{"line":7,"column":16},"end":{"line":7,"column":46}},"5":{"start":{"line":9,"column":12},"end":{"line":9,"column":43}},"6":{"start":{"line":10,"column":19},"end":{"line":10,"column":45}},"7":{"start":{"line":11,"column":18},"end":{"line":11,"column":46}},"8":{"start":{"line":12,"column":14},"end":{"line":12,"column":49}},"9":{"start":{"line":13,"column":14},"end":{"line":13,"column":49}},"10":{"start":{"line":14,"column":13},"end":{"line":16,"column":16}},"11":{"start":{"line":15,"column":1},"end":{"line":15,"column":53}},"12":{"start":{"line":18,"column":12},"end":{"line":18,"column":57}},"13":{"start":{"line":20,"column":0},"end":{"line":38,"column":2}},"14":{"start":{"line":21,"column":1},"end":{"line":21,"column":31}},"15":{"start":{"line":22,"column":1},"end":{"line":24,"column":2}},"16":{"start":{"line":23,"column":2},"end":{"line":23,"column":92}},"17":{"start":{"line":26,"column":9},"end":{"line":26,"column":24}},"18":{"start":{"line":27,"column":1},"end":{"line":37,"column":3}},"19":{"start":{"line":30,"column":20},"end":{"line":30,"column":33}},"20":{"start":{"line":31,"column":3},"end":{"line":33,"column":4}},"21":{"start":{"line":32,"column":4},"end":{"line":32,"column":45}},"22":{"start":{"line":34,"column":3},"end":{"line":34,"column":14}}},"fnMap":{"0":{"name":"(anonymous_0)","decl":{"start":{"line":14,"column":27},"end":{"line":14,"column":28}},"loc":{"start":{"line":14,"column":42},"end":{"line":16,"column":1}},"line":14},"1":{"name":"getOwnPropertyDescriptors","decl":{"start":{"line":20,"column":26},"end":{"line":20,"column":51}},"loc":{"start":{"line":20,"column":59},"end":{"line":38,"column":1}},"line":20},"2":{"name":"(anonymous_2)","decl":{"start":{"line":29,"column":2},"end":{"line":29,"column":3}},"loc":{"start":{"line":29,"column":22},"end":{"line":35,"column":3}},"line":29}},"branchMap":{"0":{"loc":{"start":{"line":14,"column":13},"end":{"line":16,"column":16}},"type":"cond-expr","locations":[{"start":{"line":14,"column":27},"end":{"line":16,"column":1}},{"start":{"line":16,"column":4},"end":{"line":16,"column":16}}],"line":14},"1":{"loc":{"start":{"line":18,"column":12},"end":{"line":18,"column":57}},"type":"binary-expr","locations":[{"start":{"line":18,"column":12},"end":{"line":18,"column":29}},{"start":{"line":18,"column":33},"end":{"line":18,"column":57}}],"line":18},"2":{"loc":{"start":{"line":22,"column":1},"end":{"line":24,"column":2}},"type":"if","locations":[{"start":{"line":22,"column":1},"end":{"line":24,"column":2}},{"start":{"line":22,"column":1},"end":{"line":24,"column":2}}],"line":22},"3":{"loc":{"start":{"line":31,"column":3},"end":{"line":33,"column":4}},"type":"if","locations":[{"start":{"line":31,"column":3},"end":{"line":33,"column":4}},{"start":{"line":31,"column":3},"end":{"line":33,"column":4}}],"line":31}},"s":{"0":1,"1":1,"2":1,"3":1,"4":1,"5":1,"6":1,"7":1,"8":1,"9":1,"10":1,"11":4,"12":1,"13":1,"14":6,"15":4,"16":0,"17":4,"18":4,"19":10,"20":10,"21":9,"22":10},"f":{"0":4,"1":6,"2":10},"b":{"0":[1,0],"1":[1,1],"2":[0,4],"3":[9,1]},"_coverageSchema":"332fd63041d2c1bcb487cc26dd0d5f7d97098a6c","hash":"e25ff1a994096a20f45cc01afb83058447fdf87f","contentHash":"2c66926545d34c0612a21b1d178c92ed_10.3.2"},"/Users/ljharb/Dropbox/git/Object.getOwnPropertyDescriptors.git/index.js":{"path":"/Users/ljharb/Dropbox/git/Object.getOwnPropertyDescriptors.git/index.js","statementMap":{"0":{"start":{"line":3,"column":13},"end":{"line":3,"column":41}},"1":{"start":{"line":4,"column":15},"end":{"line":4,"column":35}},"2":{"start":{"line":6,"column":21},"end":{"line":6,"column":48}},"3":{"start":{"line":7,"column":18},"end":{"line":7,"column":39}},"4":{"start":{"line":8,"column":11},"end":{"line":8,"column":28}},"5":{"start":{"line":10,"column":12},"end":{"line":10,"column":43}},"6":{"start":{"line":12,"column":0},"end":{"line":16,"column":3}},"7":{"start":{"line":18,"column":0},"end":{"line":18,"column":23}}},"fnMap":{},"branchMap":{},"s":{"0":1,"1":1,"2":1,"3":1,"4":1,"5":1,"6":1,"7":1},"f":{},"b":{},"_coverageSchema":"332fd63041d2c1bcb487cc26dd0d5f7d97098a6c","hash":"1a5bc0a5149328df10c65aa337bbf364c8f93945","contentHash":"8456eb36042cc4a40644cd2cbc40235a_10.3.2"},"/Users/ljharb/Dropbox/git/Object.getOwnPropertyDescriptors.git/polyfill.js":{"path":"/Users/ljharb/Dropbox/git/Object.getOwnPropertyDescriptors.git/polyfill.js","statementMap":{"0":{"start":{"line":3,"column":21},"end":{"line":3,"column":48}},"1":{"start":{"line":5,"column":0},"end":{"line":7,"column":2}},"2":{"start":{"line":6,"column":1},"end":{"line":6,"column":115}}},"fnMap":{"0":{"name":"getPolyfill","decl":{"start":{"line":5,"column":26},"end":{"line":5,"column":37}},"loc":{"start":{"line":5,"column":40},"end":{"line":7,"column":1}},"line":5}},"branchMap":{"0":{"loc":{"start":{"line":6,"column":8},"end":{"line":6,"column":114}},"type":"cond-expr","locations":[{"start":{"line":6,"column":65},"end":{"line":6,"column":97}},{"start":{"line":6,"column":100},"end":{"line":6,"column":114}}],"line":6}},"s":{"0":1,"1":1,"2":2},"f":{"0":2},"b":{"0":[2,0]},"_coverageSchema":"332fd63041d2c1bcb487cc26dd0d5f7d97098a6c","hash":"9bff677cbd1701e0172fc883bc0d0abdbb192843","contentHash":"22dd276ee90738de42383470acf2e7b4_10.3.2"},"/Users/ljharb/Dropbox/git/Object.getOwnPropertyDescriptors.git/shim.js":{"path":"/Users/ljharb/Dropbox/git/Object.getOwnPropertyDescriptors.git/shim.js","statementMap":{"0":{"start":{"line":3,"column":18},"end":{"line":3,"column":39}},"1":{"start":{"line":4,"column":13},"end":{"line":4,"column":41}},"2":{"start":{"line":6,"column":0},"end":{"line":14,"column":2}},"3":{"start":{"line":7,"column":16},"end":{"line":7,"column":29}},"4":{"start":{"line":8,"column":1},"end":{"line":12,"column":3}},"5":{"start":{"line":11,"column":45},"end":{"line":11,"column":98}},"6":{"start":{"line":13,"column":1},"end":{"line":13,"column":17}}},"fnMap":{"0":{"name":"shimGetOwnPropertyDescriptors","decl":{"start":{"line":6,"column":26},"end":{"line":6,"column":55}},"loc":{"start":{"line":6,"column":58},"end":{"line":14,"column":1}},"line":6},"1":{"name":"(anonymous_1)","decl":{"start":{"line":11,"column":31},"end":{"line":11,"column":32}},"loc":{"start":{"line":11,"column":43},"end":{"line":11,"column":100}},"line":11}},"branchMap":{},"s":{"0":1,"1":1,"2":1,"3":1,"4":1,"5":1,"6":1},"f":{"0":1,"1":1},"b":{},"_coverageSchema":"332fd63041d2c1bcb487cc26dd0d5f7d97098a6c","hash":"2b930d1ab6e39a7820edd3d42a285e87a63f39f3","contentHash":"109ca2b0674cc82fcca9f971c5c9d5a2_10.3.2"},"/Users/ljharb/Dropbox/git/Object.getOwnPropertyDescriptors.git/auto.js":{"path":"/Users/ljharb/Dropbox/git/Object.getOwnPropertyDescriptors.git/auto.js","statementMap":{"0":{"start":{"line":3,"column":0},"end":{"line":3,"column":20}}},"fnMap":{},"branchMap":{},"s":{"0":1},"f":{},"b":{},"_coverageSchema":"332fd63041d2c1bcb487cc26dd0d5f7d97098a6c","hash":"42cf295bbf4757d31f51de2fa21d204fb10ce99d","contentHash":"1a297464974cdaae7c39553ec63eb285_10.3.2"}} apollo-server-demo/node_modules/object.getownpropertydescriptors/.eslintrc 0000644 0001750 0000144 00000000543 03560116604 027115 0 ustar andreh users { "root": true, "extends": "@ljharb", "rules": { "id-length": [2, { "min": 1, "max": 30 }], "new-cap": [2, { "capIsNewExceptions": ["CreateDataProperty", "IsCallable", "RequireObjectCoercible", "ToObject"] }] }, "overrides": [ { "files": "test/**", "rules": { "max-lines-per-function": 0, "no-invalid-this": 1 }, }, ], } apollo-server-demo/node_modules/object.getownpropertydescriptors/.github/ 0000755 0001750 0000144 00000000000 14067647700 026641 5 ustar andreh users apollo-server-demo/node_modules/object.getownpropertydescriptors/.github/workflows/ 0000755 0001750 0000144 00000000000 14067647700 030676 5 ustar andreh users apollo-server-demo/node_modules/object.getownpropertydescriptors/.github/workflows/node-zero.yml 0000644 0001750 0000144 00000003040 03560116604 033306 0 ustar andreh users name: 'Tests: node.js (0.x)' on: [pull_request, push] jobs: matrix: runs-on: ubuntu-latest outputs: stable: ${{ steps.set-matrix.outputs.requireds }} unstable: ${{ steps.set-matrix.outputs.optionals }} steps: - uses: ljharb/actions/node/matrix@main id: set-matrix with: preset: '0.x' stable: needs: [matrix] name: 'stable minors' runs-on: ubuntu-latest strategy: matrix: ${{ fromJson(needs.matrix.outputs.stable) }} steps: - uses: actions/checkout@v2 - uses: ljharb/actions/node/run@main with: node-version: ${{ matrix.node-version }} command: 'tests-only' cache-node-modules-key: node_modules-${{ github.workflow }}-${{ github.action }}-${{ github.run_id }} skip-ls-check: true unstable: needs: [matrix, stable] name: 'unstable minors' continue-on-error: true if: ${{ !github.head_ref || !startsWith(github.head_ref, 'renovate') }} runs-on: ubuntu-latest strategy: matrix: ${{ fromJson(needs.matrix.outputs.unstable) }} steps: - uses: actions/checkout@v2 - uses: ljharb/actions/node/run@main with: node-version: ${{ matrix.node-version }} command: 'tests-only' cache-node-modules-key: node_modules-${{ github.workflow }}-${{ github.action }}-${{ github.run_id }} skip-ls-check: true node: name: 'node 0.x' needs: [stable, unstable] runs-on: ubuntu-latest steps: - run: 'echo tests completed' ././@LongLink 0000644 0000000 0000000 00000000153 00000000000 011602 L ustar root root apollo-server-demo/node_modules/object.getownpropertydescriptors/.github/workflows/require-allow-edits.yml apollo-server-demo/node_modules/object.getownpropertydescriptors/.github/workflows/require-allow-edi0000644 0001750 0000144 00000000376 03560116604 034144 0 ustar andreh users name: Require “Allow Edits†on: [pull_request_target] jobs: _: name: "Require “Allow Editsâ€" runs-on: ubuntu-latest steps: - uses: ljharb/require-allow-edits@main env: GITHUB_TOKEN: ${{ secrets.GITHUB_TOKEN }} apollo-server-demo/node_modules/object.getownpropertydescriptors/.github/workflows/rebase.yml 0000644 0001750 0000144 00000000401 03560116604 032643 0 ustar andreh users name: Automatic Rebase on: [pull_request_target] jobs: _: name: "Automatic Rebase" runs-on: ubuntu-latest steps: - uses: actions/checkout@v1 - uses: ljharb/rebase@master env: GITHUB_TOKEN: ${{ secrets.GITHUB_TOKEN }} apollo-server-demo/node_modules/object.getownpropertydescriptors/.github/workflows/node-4+.yml 0000644 0001750 0000144 00000002450 03560116604 032551 0 ustar andreh users name: 'Tests: node.js' on: [pull_request, push] jobs: matrix: runs-on: ubuntu-latest outputs: latest: ${{ steps.set-matrix.outputs.requireds }} minors: ${{ steps.set-matrix.outputs.optionals }} steps: - uses: ljharb/actions/node/matrix@main id: set-matrix with: preset: '>=4' latest: needs: [matrix] name: 'latest minors' runs-on: ubuntu-latest strategy: matrix: ${{ fromJson(needs.matrix.outputs.latest) }} steps: - uses: actions/checkout@v2 - uses: ljharb/actions/node/run@main name: 'npm install && npm run tests-only' with: node-version: ${{ matrix.node-version }} command: 'tests-only' minors: needs: [matrix, latest] name: 'non-latest minors' continue-on-error: true if: ${{ !github.head_ref || !startsWith(github.head_ref, 'renovate') }} runs-on: ubuntu-latest strategy: matrix: ${{ fromJson(needs.matrix.outputs.minors) }} steps: - uses: actions/checkout@v2 - uses: ljharb/actions/node/run@main with: node-version: ${{ matrix.node-version }} command: 'tests-only' node: name: 'node 4+' needs: [latest, minors] runs-on: ubuntu-latest steps: - run: 'echo tests completed' apollo-server-demo/node_modules/object.getownpropertydescriptors/.github/workflows/node-iojs.yml 0000644 0001750 0000144 00000002636 03560116604 033305 0 ustar andreh users name: 'Tests: node.js (io.js)' on: [pull_request, push] jobs: matrix: runs-on: ubuntu-latest outputs: latest: ${{ steps.set-matrix.outputs.requireds }} minors: ${{ steps.set-matrix.outputs.optionals }} steps: - uses: ljharb/actions/node/matrix@main id: set-matrix with: preset: 'iojs' latest: needs: [matrix] name: 'latest minors' runs-on: ubuntu-latest strategy: matrix: ${{ fromJson(needs.matrix.outputs.latest) }} steps: - uses: actions/checkout@v2 - uses: ljharb/actions/node/run@main name: 'npm install && npm run tests-only' with: node-version: ${{ matrix.node-version }} command: 'tests-only' skip-ls-check: true minors: needs: [matrix, latest] name: 'non-latest minors' continue-on-error: true if: ${{ !github.head_ref || !startsWith(github.head_ref, 'renovate') }} runs-on: ubuntu-latest strategy: matrix: ${{ fromJson(needs.matrix.outputs.minors) }} steps: - uses: actions/checkout@v2 - uses: ljharb/actions/node/run@main name: 'npm install && npm run tests-only' with: node-version: ${{ matrix.node-version }} command: 'tests-only' skip-ls-check: true node: name: 'io.js' needs: [latest, minors] runs-on: ubuntu-latest steps: - run: 'echo tests completed' apollo-server-demo/node_modules/object.getownpropertydescriptors/.github/workflows/node-pretest.yml 0000644 0001750 0000144 00000001067 03560116604 034024 0 ustar andreh users name: 'Tests: pretest/posttest' on: [pull_request, push] jobs: pretest: runs-on: ubuntu-latest steps: - uses: actions/checkout@v2 - uses: ljharb/actions/node/run@main name: 'npm install && npm run pretest' with: node-version: 'lts/*' command: 'pretest' posttest: runs-on: ubuntu-latest steps: - uses: actions/checkout@v2 - uses: ljharb/actions/node/run@main name: 'npm install && npm run posttest' with: node-version: 'lts/*' command: 'posttest' apollo-server-demo/node_modules/object.getownpropertydescriptors/README.md 0000644 0001750 0000144 00000006675 03560116604 026564 0 ustar andreh users # Object.getOwnPropertyDescriptors <sup>[![Version Badge][npm-version-svg]][package-url]</sup> [![Build Status][travis-svg]][travis-url] [![dependency status][deps-svg]][deps-url] [![dev dependency status][dev-deps-svg]][dev-deps-url] [![License][license-image]][license-url] [![Downloads][downloads-image]][downloads-url] [![npm badge][npm-badge-png]][package-url] An ES2017 spec-compliant shim for `Object.getOwnPropertyDescriptors` that works in ES5. Invoke its "shim" method to shim `Object.getOwnPropertyDescriptors` if it is unavailable, and if `Object.getOwnPropertyDescriptor` is available. This package implements the [es-shim API](https://github.com/es-shims/api) interface. It works in an ES3-supported environment and complies with the [spec](https://github.com/tc39/ecma262/pull/582). ## Example ```js var getDescriptors = require('object.getownpropertydescriptors'); var assert = require('assert'); var obj = { normal: Infinity }; var enumDescriptor = { enumerable: false, writable: false, configurable: true, value: true }; var writableDescriptor = { enumerable: true, writable: true, configurable: true, value: 42 }; var symbol = Symbol(); var symDescriptor = { enumerable: true, writable: true, configurable: false, value: [symbol] }; Object.defineProperty(obj, 'enumerable', enumDescriptor); Object.defineProperty(obj, 'writable', writableDescriptor); Object.defineProperty(obj, 'symbol', symDescriptor); var descriptors = getDescriptors(obj); assert.deepEqual(descriptors, { normal: { enumerable: true, writable: true, configurable: true, value: Infinity }, enumerable: enumDescriptor, writable: writableDescriptor, symbol: symDescriptor }); ``` ```js var getDescriptors = require('object.getownpropertydescriptors'); var assert = require('assert'); /* when Object.getOwnPropertyDescriptors is not present */ delete Object.getOwnPropertyDescriptors; var shimmedDescriptors = getDescriptors.shim(); assert.equal(shimmedDescriptors, getDescriptors); assert.deepEqual(shimmedDescriptors(obj), getDescriptors(obj)); ``` ```js var getDescriptors = require('object.getownpropertydescriptors'); var assert = require('assert'); /* when Object.getOwnPropertyDescriptors is present */ var shimmedDescriptors = getDescriptors.shim(); assert.notEqual(shimmedDescriptors, getDescriptors); assert.deepEqual(shimmedDescriptors(obj), getDescriptors(obj)); ``` ## Tests Simply clone the repo, `npm install`, and run `npm test` [package-url]: https://npmjs.org/package/object.getownpropertydescriptors [npm-version-svg]: http://versionbadg.es/es-shims/object.getownpropertydescriptors.svg [travis-svg]: https://travis-ci.org/es-shims/Object.getOwnPropertyDescriptors.svg [travis-url]: https://travis-ci.org/es-shims/Object.getOwnPropertyDescriptors [deps-svg]: https://david-dm.org/es-shims/object.getownpropertydescriptors.svg [deps-url]: https://david-dm.org/es-shims/object.getownpropertydescriptors [dev-deps-svg]: https://david-dm.org/es-shims/object.getownpropertydescriptors/dev-status.svg [dev-deps-url]: https://david-dm.org/es-shims/object.getownpropertydescriptors#info=devDependencies [npm-badge-png]: https://nodei.co/npm/object.getownpropertydescriptors.png?downloads=true&stars=true [license-image]: http://img.shields.io/npm/l/object.getownpropertydescriptors.svg [license-url]: LICENSE [downloads-image]: http://img.shields.io/npm/dm/object.getownpropertydescriptors.svg [downloads-url]: http://npm-stat.com/charts.html?package=object.getownpropertydescriptors apollo-server-demo/node_modules/object.getownpropertydescriptors/.editorconfig 0000644 0001750 0000144 00000000424 03560116604 027744 0 ustar andreh users root = true [*] indent_style = tab; insert_final_newline = true; quote_type = auto; space_after_anonymous_functions = true; space_after_control_statements = true; spaces_around_operators = true; trim_trailing_whitespace = true; spaces_in_brackets = false; end_of_line = lf; apollo-server-demo/node_modules/object.getownpropertydescriptors/package.json 0000644 0001750 0000144 00000003743 03560116604 027564 0 ustar andreh users { "name": "object.getownpropertydescriptors", "version": "2.1.1", "author": "Jordan Harband <ljharb@gmail.com>", "funding": { "url": "https://github.com/sponsors/ljharb" }, "description": "ES2017 spec-compliant shim for `Object.getOwnPropertyDescriptors` that works in ES5.", "license": "MIT", "main": "index.js", "scripts": { "prepublish": "safe-publish-latest", "pretest": "npm run --silent lint", "test": "npm run --silent tests-only", "posttest": "npx aud --production", "tests-only": "nyc tape 'test/**/*.js'", "lint": "eslint .", "postlint": "es-shim-api --bound" }, "repository": { "type": "git", "url": "git://github.com/es-shims/object.getownpropertydescriptors.git" }, "keywords": [ "Object.getOwnPropertyDescriptors", "descriptor", "property descriptor", "ES8", "ES2017", "shim", "polyfill", "getOwnPropertyDescriptor", "es-shim API" ], "dependencies": { "call-bind": "^1.0.0", "define-properties": "^1.1.3", "es-abstract": "^1.18.0-next.1" }, "devDependencies": { "@es-shims/api": "^2.1.2", "@ljharb/eslint-config": "^17.2.0", "aud": "^1.1.2", "eslint": "^7.8.1", "functions-have-names": "^1.2.1", "has-strict-mode": "^1.0.0", "nyc": "^10.3.2", "safe-publish-latest": "^1.1.4", "tape": "^5.0.1" }, "testling": { "files": [ "test/index.js", "test/shimmed.js" ], "browsers": [ "iexplore/9.0..latest", "firefox/4.0..6.0", "firefox/15.0..latest", "firefox/nightly", "chrome/5.0..10.0", "chrome/20.0..latest", "chrome/canary", "opera/12.0..latest", "opera/next", "safari/5.0..latest", "ipad/6.0..latest", "iphone/6.0..latest", "android-browser/4.2" ] }, "engines": { "node": ">= 0.8" } ,"_resolved": "https://registry.npmjs.org/object.getownpropertydescriptors/-/object.getownpropertydescriptors-2.1.1.tgz" ,"_integrity": "sha512-6DtXgZ/lIZ9hqx4GtZETobXLR/ZLaa0aqV0kzbn80Rf8Z2e/XFnhA0I7p07N2wH8bBBltr2xQPi6sbKWAY2Eng==" ,"_from": "object.getownpropertydescriptors@2.1.1" } apollo-server-demo/node_modules/object.getownpropertydescriptors/auto.js 0000644 0001750 0000144 00000000044 03560116604 026573 0 ustar andreh users 'use strict'; require('./shim')(); apollo-server-demo/node_modules/object.getownpropertydescriptors/shim.js 0000644 0001750 0000144 00000000575 03560116604 026574 0 ustar andreh users 'use strict'; var getPolyfill = require('./polyfill'); var define = require('define-properties'); module.exports = function shimGetOwnPropertyDescriptors() { var polyfill = getPolyfill(); define( Object, { getOwnPropertyDescriptors: polyfill }, { getOwnPropertyDescriptors: function () { return Object.getOwnPropertyDescriptors !== polyfill; } } ); return polyfill; }; apollo-server-demo/node_modules/object.getownpropertydescriptors/implementation.js 0000644 0001750 0000144 00000002252 03560116604 030653 0 ustar andreh users 'use strict'; var CreateDataProperty = require('es-abstract/2020/CreateDataProperty'); var IsCallable = require('es-abstract/2020/IsCallable'); var RequireObjectCoercible = require('es-abstract/2020/RequireObjectCoercible'); var ToObject = require('es-abstract/2020/ToObject'); var callBound = require('call-bind/callBound'); var $gOPD = Object.getOwnPropertyDescriptor; var $getOwnNames = Object.getOwnPropertyNames; var $getSymbols = Object.getOwnPropertySymbols; var $concat = callBound('Array.prototype.concat'); var $reduce = callBound('Array.prototype.reduce'); var getAll = $getSymbols ? function (obj) { return $concat($getOwnNames(obj), $getSymbols(obj)); } : $getOwnNames; var isES5 = IsCallable($gOPD) && IsCallable($getOwnNames); module.exports = function getOwnPropertyDescriptors(value) { RequireObjectCoercible(value); if (!isES5) { throw new TypeError('getOwnPropertyDescriptors requires Object.getOwnPropertyDescriptor'); } var O = ToObject(value); return $reduce( getAll(O), function (acc, key) { var descriptor = $gOPD(O, key); if (typeof descriptor !== 'undefined') { CreateDataProperty(acc, key, descriptor); } return acc; }, {} ); }; apollo-server-demo/node_modules/object.getownpropertydescriptors/.nycrc 0000644 0001750 0000144 00000000330 03560116604 026402 0 ustar andreh users { "all": true, "check-coverage": false, "reporter": ["text-summary", "text", "html", "json"], "lines": 86, "statements": 85.93, "functions": 82.43, "branches": 76.06, "exclude": [ "coverage", "test" ] } apollo-server-demo/node_modules/object.getownpropertydescriptors/test/ 0000755 0001750 0000144 00000000000 14067647700 026260 5 ustar andreh users apollo-server-demo/node_modules/object.getownpropertydescriptors/test/index.js 0000644 0001750 0000144 00000000727 03560116604 027721 0 ustar andreh users 'use strict'; var getDescriptors = require('../'); var test = require('tape'); var runTests = require('./tests'); test('as a function', function (t) { t.test('bad object/this value', function (st) { st['throws'](function () { return getDescriptors(undefined); }, TypeError, 'undefined is not an object'); st['throws'](function () { return getDescriptors(null); }, TypeError, 'null is not an object'); st.end(); }); runTests(getDescriptors, t); t.end(); }); apollo-server-demo/node_modules/object.getownpropertydescriptors/test/tests.js 0000644 0001750 0000144 00000006172 03560116604 027754 0 ustar andreh users 'use strict'; module.exports = function (getDescriptors, t) { var enumDescriptor = { configurable: true, enumerable: false, value: true, writable: false }; var writableDescriptor = { configurable: true, enumerable: true, value: 42, writable: true }; t.test('works with Object.prototype poisoned setter', { skip: !Object.defineProperty }, function (st) { var key = 'foo'; var obj = {}; obj[key] = 42; var expected = {}; expected[key] = { configurable: true, enumerable: true, value: 42, writable: true }; /* eslint-disable no-extend-native, accessor-pairs */ Object.defineProperty(Object.prototype, key, { configurable: true, set: function (v) { throw new Error(v); } }); /* eslint-enable no-extend-native, accessor-pairs */ var hasOwnNamesBug = false; try { Object.getOwnPropertyNames(obj); } catch (e) { // v8 in node 0.6 - 0.12 has a bug :-( hasOwnNamesBug = true; st.comment('SKIP: this engine has a bug with Object.getOwnPropertyNames: it can not handle a throwing setter on Object.prototype.'); } if (!hasOwnNamesBug) { st.doesNotThrow(function () { var result = getDescriptors(obj); st.deepEqual(result, expected, 'got expected descriptors'); }); } delete Object.prototype[key]; st.end(); }); t.test('gets all expected non-Symbol descriptors', function (st) { var obj = { normal: Infinity }; Object.defineProperty(obj, 'enumerable', enumDescriptor); Object.defineProperty(obj, 'writable', writableDescriptor); var descriptors = getDescriptors(obj); st.deepEqual(descriptors, { enumerable: enumDescriptor, normal: { configurable: true, enumerable: true, value: Infinity, writable: true }, writable: writableDescriptor }); st.end(); }); var supportsSymbols = typeof Symbol === 'function' && typeof Symbol('foo') === 'symbol'; t.test('gets Symbol descriptors too', { skip: !supportsSymbols }, function (st) { var symbol = Symbol('sym'); var symDescriptor = { configurable: false, enumerable: true, value: [symbol], writable: true }; var obj = { normal: Infinity }; Object.defineProperty(obj, 'enumerable', enumDescriptor); Object.defineProperty(obj, 'writable', writableDescriptor); Object.defineProperty(obj, 'symbol', symDescriptor); var descriptors = getDescriptors(obj); st.deepEqual(descriptors, { enumerable: enumDescriptor, normal: { configurable: true, enumerable: true, value: Infinity, writable: true }, symbol: symDescriptor, writable: writableDescriptor }); st.end(); }); /* global Proxy */ var supportsProxy = typeof Proxy === 'function'; t.test('Proxies that return an undefined descriptor', { skip: !supportsProxy }, function (st) { var obj = { foo: true }; var fooDescriptor = Object.getOwnPropertyDescriptor(obj, 'foo'); var proxy = new Proxy(obj, { getOwnPropertyDescriptor: function (target, key) { return Object.getOwnPropertyDescriptor(target, key); }, ownKeys: function () { return ['foo', 'bar']; } }); st.deepEqual(getDescriptors(proxy), { foo: fooDescriptor }, 'object has no descriptors'); st.end(); }); }; apollo-server-demo/node_modules/object.getownpropertydescriptors/test/shimmed.js 0000644 0001750 0000144 00000002555 03560116604 030241 0 ustar andreh users 'use strict'; require('../auto'); var getDescriptors = require('../'); var test = require('tape'); var defineProperties = require('define-properties'); var runTests = require('./tests'); var isEnumerable = Object.prototype.propertyIsEnumerable; var functionsHaveNames = require('functions-have-names')(); test('shimmed', function (t) { t.equal(Object.getOwnPropertyDescriptors.length, 1, 'Object.getOwnPropertyDescriptors has a length of 1'); t.test('Function name', { skip: !functionsHaveNames }, function (st) { st.equal(Object.getOwnPropertyDescriptors.name, 'getOwnPropertyDescriptors', 'Object.getOwnPropertyDescriptors has name "getOwnPropertyDescriptors"'); st.end(); }); t.test('enumerability', { skip: !defineProperties.supportsDescriptors }, function (et) { et.equal(false, isEnumerable.call(Object, 'getOwnPropertyDescriptors'), 'Object.getOwnPropertyDescriptors is not enumerable'); et.end(); }); var supportsStrictMode = (function () { return typeof this === 'undefined'; }()); t.test('bad object/this value', { skip: !supportsStrictMode }, function (st) { st['throws'](function () { return getDescriptors(undefined, 'a'); }, TypeError, 'undefined is not an object'); st['throws'](function () { return getDescriptors(null, 'a'); }, TypeError, 'null is not an object'); st.end(); }); runTests(Object.getOwnPropertyDescriptors, t); t.end(); }); apollo-server-demo/node_modules/object.getownpropertydescriptors/test/implementation.js 0000644 0001750 0000144 00000001205 03560116604 031627 0 ustar andreh users 'use strict'; var implementation = require('../implementation'); var callBind = require('call-bind'); var test = require('tape'); var hasStrictMode = require('has-strict-mode')(); var runTests = require('./tests'); test('as a function', function (t) { t.test('bad array/this value', { skip: !hasStrictMode }, function (st) { /* eslint no-useless-call: 0 */ st['throws'](function () { implementation.call(undefined); }, TypeError, 'undefined is not an object'); st['throws'](function () { implementation.call(null); }, TypeError, 'null is not an object'); st.end(); }); runTests(callBind(implementation, Object), t); t.end(); }); apollo-server-demo/node_modules/object.getownpropertydescriptors/.eslintignore 0000644 0001750 0000144 00000000012 03560116604 027763 0 ustar andreh users coverage/ apollo-server-demo/node_modules/object.getownpropertydescriptors/CHANGELOG.md 0000644 0001750 0000144 00000006072 03560116604 027105 0 ustar andreh users 2.1.1 / 2020-11-26 ================= * [Fix] do not mutate the native function when present * [Deps] update `es-abstract`; use `call-bind` where applicable * [meta] remove unused Makefile and associated utilities * [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `tape`, `functions-have-names`; add `aud` * [actions] add Require Allow Edits workflow * [actions] switch Automatic Rebase workflow to `pull_request_target` event * [Tests] migrate tests to Github Actions * [Tests] run `nyc` on all tests * [Tests] add `implementation` test; run `es-shim-api` in postlint; use `tape` runner * [Tests] only audit prod deps 2.1.0 / 2019-12-12 ================= * [New] add auto entry point * [Refactor] use split-up `es-abstract` (78% bundle size decrease) * [readme] fix repo URLs, remove testling * [Docs] Fix formatting in the README (#30) * [Deps] update `define-properties`, `es-abstract` * [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `functions-have-names`, `covert`, `replace`, `semver`, `tape`, `@es-shims/api`; add `safe-publish-latest` * [meta] add `funding` field * [meta] Only apps should have lockfiles. * [Tests] use shared travis-ci configs * [Tests] use `functions-have-names` * [Tests] use `npx aud` instead of `nsp` or `npm audit` with hoops * [Tests] remove `jscs` * [actions] add automatic rebasing / merge commit blocking 2.0.3 / 2016-07-26 ================= * [Fix] Update implementation to not return `undefined` descriptors * [Deps] update `es-abstract` * [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `@es-shims/api`, `jscs`, `nsp`, `tape`, `semver` * [Dev Deps] remove unused eccheck script + dep * [Tests] up to `node` `v6.3`, `v5.12`, `v4.4` * [Tests] use pretest/posttest for linting/security * Update to stage 4 2.0.2 / 2016-01-27 ================= * [Fix] ensure that `Object.getOwnPropertyDescriptors` does not fail when `Object.prototype` has a poisoned setter (#1, #2) 2.0.1 / 2016-01-27 ================= * [Deps] move `@es-shims/api` to dev deps 2.0.0 / 2016-01-27 ================= * [Breaking] implement the es-shims API * [Deps] update `define-properties`, `es-abstract` * [Dev Deps] update `tape`, `jscs`, `nsp`, `eslint`, `@ljharb/eslint-config`, `semver` * [Tests] fix npm upgrades in older nodes * [Tests] up to `node` `v5.5` * [Docs] Switch from vb.teelaun.ch to versionbadg.es for the npm version badge SVG 1.0.4 / 2015-07-20 ================= * [Tests] Test on `io.js` `v2.4` * [Deps, Dev Deps] Update `define-properties`, `tape`, `eslint`, `semver` 1.0.3 / 2015-06-28 ================= * Increase robustness by caching `Array#{concat, reduce}` * [Deps] Update `define_properties` * [Dev Deps] Update `eslint`, `semver`, `nsp` * [Tests] Test up to `io.js` `v2.3` 1.0.2 / 2015-05-23 ================= * Update `es-abstract`, `tape`, `eslint`, `jscs`, `semver`, `covert` * Test up to `io.js` `v2.0` 1.0.1 / 2015-03-20 ================= * Update `es-abstract`, `editorconfig-tools`, `nsp`, `eslint`, `semver`, `replace` 1.0.0 / 2015-02-17 ================= * v1.0.0 apollo-server-demo/node_modules/object.getownpropertydescriptors/polyfill.js 0000644 0001750 0000144 00000000343 03560116604 027457 0 ustar andreh users 'use strict'; var implementation = require('./implementation'); module.exports = function getPolyfill() { return typeof Object.getOwnPropertyDescriptors === 'function' ? Object.getOwnPropertyDescriptors : implementation; }; apollo-server-demo/node_modules/es-to-primitive/ 0000755 0001750 0000144 00000000000 14067647701 021500 5 ustar andreh users apollo-server-demo/node_modules/es-to-primitive/index.js 0000644 0001750 0000144 00000000706 03560116604 023135 0 ustar andreh users 'use strict'; var ES5 = require('./es5'); var ES6 = require('./es6'); var ES2015 = require('./es2015'); if (Object.defineProperty) { Object.defineProperty(ES2015, 'ES5', { enumerable: false, value: ES5 }); Object.defineProperty(ES2015, 'ES6', { enumerable: false, value: ES6 }); Object.defineProperty(ES2015, 'ES2015', { enumerable: false, value: ES2015 }); } else { ES6.ES5 = ES5; ES6.ES6 = ES6; ES6.ES2015 = ES2015; } module.exports = ES2015; apollo-server-demo/node_modules/es-to-primitive/LICENSE 0000644 0001750 0000144 00000002072 03560116604 022473 0 ustar andreh users The MIT License (MIT) Copyright (c) 2015 Jordan Harband Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/es-to-primitive/.eslintrc 0000644 0001750 0000144 00000000605 03560116604 023312 0 ustar andreh users { "root": true, "extends": "@ljharb", "rules": { "complexity": [2, 14], "func-name-matching": 0, "id-length": [2, { "min": 1, "max": 24, "properties": "never" }], "max-statements": [2, 20], "new-cap": [2, { "capIsNewExceptions": ["GetMethod"] }] }, "overrides": [ { "files": "test/**", "rules": { "max-lines-per-function": [2, { "max": 68 }], }, } ], } apollo-server-demo/node_modules/es-to-primitive/.github/ 0000755 0001750 0000144 00000000000 14067647701 023040 5 ustar andreh users apollo-server-demo/node_modules/es-to-primitive/.github/FUNDING.yml 0000644 0001750 0000144 00000001112 03560116604 024635 0 ustar andreh users # These are supported funding model platforms github: [ljharb] patreon: # Replace with a single Patreon username open_collective: # Replace with a single Open Collective username ko_fi: # Replace with a single Ko-fi username tidelift: npm/es-to-primitive community_bridge: # Replace with a single Community Bridge project-name e.g., cloud-foundry liberapay: # Replace with a single Liberapay username issuehunt: # Replace with a single IssueHunt username otechie: # Replace with a single Otechie username custom: # Replace with up to 4 custom sponsorship URLs e.g., ['link1', 'link2'] apollo-server-demo/node_modules/es-to-primitive/README.md 0000644 0001750 0000144 00000003712 03560116604 022747 0 ustar andreh users # es-to-primitive <sup>[![Version Badge][npm-version-svg]][package-url]</sup> [![Build Status][travis-svg]][travis-url] [![dependency status][deps-svg]][deps-url] [![dev dependency status][dev-deps-svg]][dev-deps-url] [![License][license-image]][license-url] [![Downloads][downloads-image]][downloads-url] [![npm badge][npm-badge-png]][package-url] ECMAScript “ToPrimitive†algorithm. Provides ES5 and ES2015 versions. When different versions of the spec conflict, the default export will be the latest version of the abstract operation. Alternative versions will also be available under an `es5`/`es2015` exported property if you require a specific version. ## Example ```js var toPrimitive = require('es-to-primitive'); var assert = require('assert'); assert(toPrimitive(function () {}) === String(function () {})); var date = new Date(); assert(toPrimitive(date) === String(date)); assert(toPrimitive({ valueOf: function () { return 3; } }) === 3); assert(toPrimitive(['a', 'b', 3]) === String(['a', 'b', 3])); var sym = Symbol(); assert(toPrimitive(Object(sym)) === sym); ``` ## Tests Simply clone the repo, `npm install`, and run `npm test` [package-url]: https://npmjs.org/package/es-to-primitive [npm-version-svg]: http://versionbadg.es/ljharb/es-to-primitive.svg [travis-svg]: https://travis-ci.org/ljharb/es-to-primitive.svg [travis-url]: https://travis-ci.org/ljharb/es-to-primitive [deps-svg]: https://david-dm.org/ljharb/es-to-primitive.svg [deps-url]: https://david-dm.org/ljharb/es-to-primitive [dev-deps-svg]: https://david-dm.org/ljharb/es-to-primitive/dev-status.svg [dev-deps-url]: https://david-dm.org/ljharb/es-to-primitive#info=devDependencies [npm-badge-png]: https://nodei.co/npm/es-to-primitive.png?downloads=true&stars=true [license-image]: http://img.shields.io/npm/l/es-to-primitive.svg [license-url]: LICENSE [downloads-image]: http://img.shields.io/npm/dm/es-to-primitive.svg [downloads-url]: http://npm-stat.com/charts.html?package=es-to-primitive apollo-server-demo/node_modules/es-to-primitive/es5.js 0000644 0001750 0000144 00000002257 03560116604 022525 0 ustar andreh users 'use strict'; var toStr = Object.prototype.toString; var isPrimitive = require('./helpers/isPrimitive'); var isCallable = require('is-callable'); // http://ecma-international.org/ecma-262/5.1/#sec-8.12.8 var ES5internalSlots = { '[[DefaultValue]]': function (O) { var actualHint; if (arguments.length > 1) { actualHint = arguments[1]; } else { actualHint = toStr.call(O) === '[object Date]' ? String : Number; } if (actualHint === String || actualHint === Number) { var methods = actualHint === String ? ['toString', 'valueOf'] : ['valueOf', 'toString']; var value, i; for (i = 0; i < methods.length; ++i) { if (isCallable(O[methods[i]])) { value = O[methods[i]](); if (isPrimitive(value)) { return value; } } } throw new TypeError('No default value'); } throw new TypeError('invalid [[DefaultValue]] hint supplied'); } }; // http://ecma-international.org/ecma-262/5.1/#sec-9.1 module.exports = function ToPrimitive(input) { if (isPrimitive(input)) { return input; } if (arguments.length > 1) { return ES5internalSlots['[[DefaultValue]]'](input, arguments[1]); } return ES5internalSlots['[[DefaultValue]]'](input); }; apollo-server-demo/node_modules/es-to-primitive/package.json 0000644 0001750 0000144 00000003615 03560116604 023760 0 ustar andreh users { "name": "es-to-primitive", "version": "1.2.1", "author": "Jordan Harband <ljharb@gmail.com>", "funding": { "url": "https://github.com/sponsors/ljharb" }, "description": "ECMAScript “ToPrimitive†algorithm. Provides ES5 and ES2015 versions.", "license": "MIT", "main": "index.js", "scripts": { "pretest": "npm run --silent lint", "test": "npm run --silent tests-only", "posttest": "npx aud", "tests-only": "node --es-staging test", "coverage": "covert test/*.js", "coverage-quiet": "covert test/*.js --quiet", "lint": "eslint ." }, "repository": { "type": "git", "url": "git://github.com/ljharb/es-to-primitive.git" }, "keywords": [ "primitive", "abstract", "ecmascript", "es5", "es6", "es2015", "toPrimitive", "coerce", "type", "object", "string", "number", "boolean", "symbol", "null", "undefined" ], "dependencies": { "is-callable": "^1.1.4", "is-date-object": "^1.0.1", "is-symbol": "^1.0.2" }, "devDependencies": { "@ljharb/eslint-config": "^15.0.0", "covert": "^1.1.1", "eslint": "^6.6.0", "foreach": "^2.0.5", "function.prototype.name": "^1.1.1", "has-symbols": "^1.0.0", "object-inspect": "^1.6.0", "object-is": "^1.0.1", "replace": "^1.1.1", "semver": "^6.3.0", "tape": "^4.11.0" }, "testling": { "files": "test", "browsers": [ "iexplore/6.0..latest", "firefox/3.0..6.0", "firefox/15.0..latest", "firefox/nightly", "chrome/4.0..10.0", "chrome/20.0..latest", "chrome/canary", "opera/10.0..latest", "opera/next", "safari/4.0..latest", "ipad/6.0..latest", "iphone/6.0..latest", "android-browser/4.2" ] }, "engines": { "node": ">= 0.4" } ,"_resolved": "https://registry.npmjs.org/es-to-primitive/-/es-to-primitive-1.2.1.tgz" ,"_integrity": "sha512-QCOllgZJtaUo9miYBcLChTUaHNjJF3PYs1VidD7AwiEj1kYxKeQTctLAezAOH5ZKRH0g2IgPn6KwB4IT8iRpvA==" ,"_from": "es-to-primitive@1.2.1" } apollo-server-demo/node_modules/es-to-primitive/Makefile 0000644 0001750 0000144 00000007372 03560116604 023136 0 ustar andreh users # Since we rely on paths relative to the makefile location, abort if make isn't being run from there. $(if $(findstring /,$(MAKEFILE_LIST)),$(error Please only invoke this makefile from the directory it resides in)) # The files that need updating when incrementing the version number. VERSIONED_FILES := *.js *.json README* # Add the local npm packages' bin folder to the PATH, so that `make` can find them, when invoked directly. # Note that rather than using `$(npm bin)` the 'node_modules/.bin' path component is hard-coded, so that invocation works even from an environment # where npm is (temporarily) unavailable due to having deactivated an nvm instance loaded into the calling shell in order to avoid interference with tests. export PATH := $(shell printf '%s' "$$PWD/node_modules/.bin:$$PATH") UTILS := semver # Make sure that all required utilities can be located. UTIL_CHECK := $(or $(shell PATH="$(PATH)" which $(UTILS) >/dev/null && echo 'ok'),$(error Did you forget to run `npm install` after cloning the repo? At least one of the required supporting utilities not found: $(UTILS))) # Default target (by virtue of being the first non '.'-prefixed in the file). .PHONY: _no-target-specified _no-target-specified: $(error Please specify the target to make - `make list` shows targets. Alternatively, use `npm test` to run the default tests; `npm run` shows all tests) # Lists all targets defined in this makefile. .PHONY: list list: @$(MAKE) -pRrn : -f $(MAKEFILE_LIST) 2>/dev/null | awk -v RS= -F: '/^# File/,/^# Finished Make data base/ {if ($$1 !~ "^[#.]") {print $$1}}' | command grep -v -e '^[^[:alnum:]]' -e '^$@$$command ' | sort # All-tests target: invokes the specified test suites for ALL shells defined in $(SHELLS). .PHONY: test test: @npm test .PHONY: _ensure-tag _ensure-tag: ifndef TAG $(error Please invoke with `make TAG=<new-version> release`, where <new-version> is either an increment specifier (patch, minor, major, prepatch, preminor, premajor, prerelease), or an explicit major.minor.patch version number) endif CHANGELOG_ERROR = $(error No CHANGELOG specified) .PHONY: _ensure-changelog _ensure-changelog: @ (git status -sb --porcelain | command grep -E '^( M|[MA] ) CHANGELOG.md' > /dev/null) || (echo no CHANGELOG.md specified && exit 2) # Ensures that the git workspace is clean. .PHONY: _ensure-clean _ensure-clean: @[ -z "$$((git status --porcelain --untracked-files=no || echo err) | command grep -v 'CHANGELOG.md')" ] || { echo "Workspace is not clean; please commit changes first." >&2; exit 2; } # Makes a release; invoke with `make TAG=<versionOrIncrementSpec> release`. .PHONY: release release: _ensure-tag _ensure-changelog _ensure-clean @old_ver=`git describe --abbrev=0 --tags --match 'v[0-9]*.[0-9]*.[0-9]*'` || { echo "Failed to determine current version." >&2; exit 1; }; old_ver=$${old_ver#v}; \ new_ver=`echo "$(TAG)" | sed 's/^v//'`; new_ver=$${new_ver:-patch}; \ if printf "$$new_ver" | command grep -q '^[0-9]'; then \ semver "$$new_ver" >/dev/null || { echo 'Invalid version number specified: $(TAG) - must be major.minor.patch' >&2; exit 2; }; \ semver -r "> $$old_ver" "$$new_ver" >/dev/null || { echo 'Invalid version number specified: $(TAG) - must be HIGHER than current one.' >&2; exit 2; } \ else \ new_ver=`semver -i "$$new_ver" "$$old_ver"` || { echo 'Invalid version-increment specifier: $(TAG)' >&2; exit 2; } \ fi; \ printf "=== Bumping version **$$old_ver** to **$$new_ver** before committing and tagging:\n=== TYPE 'proceed' TO PROCEED, anything else to abort: " && read response && [ "$$response" = 'proceed' ] || { echo 'Aborted.' >&2; exit 2; }; \ replace "$$old_ver" "$$new_ver" -- $(VERSIONED_FILES) && \ git commit -m "v$$new_ver" $(VERSIONED_FILES) CHANGELOG.md && \ git tag -a -m "v$$new_ver" "v$$new_ver" apollo-server-demo/node_modules/es-to-primitive/test/ 0000755 0001750 0000144 00000000000 14067647701 022457 5 ustar andreh users apollo-server-demo/node_modules/es-to-primitive/test/index.js 0000644 0001750 0000144 00000001036 03560116604 024111 0 ustar andreh users 'use strict'; var toPrimitive = require('../'); var ES5 = require('../es5'); var ES6 = require('../es6'); var ES2015 = require('../es2015'); var test = require('tape'); test('default export', function (t) { t.equal(toPrimitive, ES2015, 'default export is ES2015'); t.equal(toPrimitive.ES5, ES5, 'ES5 property has ES5 method'); t.equal(toPrimitive.ES6, ES6, 'ES6 property has ES6 method'); t.equal(toPrimitive.ES2015, ES2015, 'ES2015 property has ES2015 method'); t.end(); }); require('./es5'); require('./es6'); require('./es2015'); apollo-server-demo/node_modules/es-to-primitive/test/es5.js 0000644 0001750 0000144 00000014523 03560116604 023503 0 ustar andreh users 'use strict'; var test = require('tape'); var toPrimitive = require('../es5'); var is = require('object-is'); var forEach = require('foreach'); var functionName = require('function.prototype.name'); var debug = require('object-inspect'); var hasSymbols = require('has-symbols')(); test('function properties', function (t) { t.equal(toPrimitive.length, 1, 'length is 1'); t.equal(functionName(toPrimitive), 'ToPrimitive', 'name is ToPrimitive'); t.end(); }); var primitives = [null, undefined, true, false, 0, -0, 42, NaN, Infinity, -Infinity, '', 'abc']; test('primitives', function (t) { forEach(primitives, function (i) { t.ok(is(toPrimitive(i), i), 'toPrimitive(' + debug(i) + ') returns the same value'); t.ok(is(toPrimitive(i, String), i), 'toPrimitive(' + debug(i) + ', String) returns the same value'); t.ok(is(toPrimitive(i, Number), i), 'toPrimitive(' + debug(i) + ', Number) returns the same value'); }); t.end(); }); test('Symbols', { skip: !hasSymbols }, function (t) { var symbols = [ Symbol('foo'), Symbol.iterator, Symbol['for']('foo') // eslint-disable-line no-restricted-properties ]; forEach(symbols, function (sym) { t.equal(toPrimitive(sym), sym, 'toPrimitive(' + debug(sym) + ') returns the same value'); t.equal(toPrimitive(sym, String), sym, 'toPrimitive(' + debug(sym) + ', String) returns the same value'); t.equal(toPrimitive(sym, Number), sym, 'toPrimitive(' + debug(sym) + ', Number) returns the same value'); }); var primitiveSym = Symbol('primitiveSym'); var stringSym = Symbol.prototype.toString.call(primitiveSym); var objectSym = Object(primitiveSym); t.equal(toPrimitive(objectSym), primitiveSym, 'toPrimitive(' + debug(objectSym) + ') returns ' + debug(primitiveSym)); // This is different from ES2015, as the ES5 algorithm doesn't account for the existence of Symbols: t.equal(toPrimitive(objectSym, String), stringSym, 'toPrimitive(' + debug(objectSym) + ', String) returns ' + debug(stringSym)); t.equal(toPrimitive(objectSym, Number), primitiveSym, 'toPrimitive(' + debug(objectSym) + ', Number) returns ' + debug(primitiveSym)); t.end(); }); test('Arrays', function (t) { var arrays = [[], ['a', 'b'], [1, 2]]; forEach(arrays, function (arr) { t.ok(is(toPrimitive(arr), arr.toString()), 'toPrimitive(' + debug(arr) + ') returns toString of the array'); t.equal(toPrimitive(arr, String), arr.toString(), 'toPrimitive(' + debug(arr) + ') returns toString of the array'); t.ok(is(toPrimitive(arr, Number), arr.toString()), 'toPrimitive(' + debug(arr) + ') returns toString of the array'); }); t.end(); }); test('Dates', function (t) { var dates = [new Date(), new Date(0), new Date(NaN)]; forEach(dates, function (date) { t.equal(toPrimitive(date), date.toString(), 'toPrimitive(' + debug(date) + ') returns toString of the date'); t.equal(toPrimitive(date, String), date.toString(), 'toPrimitive(' + debug(date) + ') returns toString of the date'); t.ok(is(toPrimitive(date, Number), date.valueOf()), 'toPrimitive(' + debug(date) + ') returns valueOf of the date'); }); t.end(); }); var coercibleObject = { valueOf: function () { return 3; }, toString: function () { return 42; } }; var valueOfOnlyObject = { valueOf: function () { return 4; }, toString: function () { return {}; } }; var toStringOnlyObject = { valueOf: function () { return {}; }, toString: function () { return 7; } }; var coercibleFnObject = { valueOf: function () { return function valueOfFn() {}; }, toString: function () { return 42; } }; var uncoercibleObject = { valueOf: function () { return {}; }, toString: function () { return {}; } }; var uncoercibleFnObject = { valueOf: function () { return function valueOfFn() {}; }, toString: function () { return function toStrFn() {}; } }; test('Objects', function (t) { t.equal(toPrimitive(coercibleObject), coercibleObject.valueOf(), 'coercibleObject with no hint coerces to valueOf'); t.equal(toPrimitive(coercibleObject, String), coercibleObject.toString(), 'coercibleObject with hint String coerces to toString'); t.equal(toPrimitive(coercibleObject, Number), coercibleObject.valueOf(), 'coercibleObject with hint Number coerces to valueOf'); t.equal(toPrimitive(coercibleFnObject), coercibleFnObject.toString(), 'coercibleFnObject coerces to toString'); t.equal(toPrimitive(coercibleFnObject, String), coercibleFnObject.toString(), 'coercibleFnObject with hint String coerces to toString'); t.equal(toPrimitive(coercibleFnObject, Number), coercibleFnObject.toString(), 'coercibleFnObject with hint Number coerces to toString'); t.ok(is(toPrimitive({}), '[object Object]'), '{} with no hint coerces to Object#toString'); t.equal(toPrimitive({}, String), '[object Object]', '{} with hint String coerces to Object#toString'); t.ok(is(toPrimitive({}, Number), '[object Object]'), '{} with hint Number coerces to Object#toString'); t.equal(toPrimitive(toStringOnlyObject), toStringOnlyObject.toString(), 'toStringOnlyObject returns toString'); t.equal(toPrimitive(toStringOnlyObject, String), toStringOnlyObject.toString(), 'toStringOnlyObject with hint String returns toString'); t.equal(toPrimitive(toStringOnlyObject, Number), toStringOnlyObject.toString(), 'toStringOnlyObject with hint Number returns toString'); t.equal(toPrimitive(valueOfOnlyObject), valueOfOnlyObject.valueOf(), 'valueOfOnlyObject returns valueOf'); t.equal(toPrimitive(valueOfOnlyObject, String), valueOfOnlyObject.valueOf(), 'valueOfOnlyObject with hint String returns valueOf'); t.equal(toPrimitive(valueOfOnlyObject, Number), valueOfOnlyObject.valueOf(), 'valueOfOnlyObject with hint Number returns valueOf'); t.test('exceptions', function (st) { st['throws'](toPrimitive.bind(null, uncoercibleObject), TypeError, 'uncoercibleObject throws a TypeError'); st['throws'](toPrimitive.bind(null, uncoercibleObject, String), TypeError, 'uncoercibleObject with hint String throws a TypeError'); st['throws'](toPrimitive.bind(null, uncoercibleObject, Number), TypeError, 'uncoercibleObject with hint Number throws a TypeError'); st['throws'](toPrimitive.bind(null, uncoercibleFnObject), TypeError, 'uncoercibleFnObject throws a TypeError'); st['throws'](toPrimitive.bind(null, uncoercibleFnObject, String), TypeError, 'uncoercibleFnObject with hint String throws a TypeError'); st['throws'](toPrimitive.bind(null, uncoercibleFnObject, Number), TypeError, 'uncoercibleFnObject with hint Number throws a TypeError'); st.end(); }); t.end(); }); apollo-server-demo/node_modules/es-to-primitive/test/es6.js 0000644 0001750 0000144 00000020756 03560116604 023511 0 ustar andreh users 'use strict'; var test = require('tape'); var toPrimitive = require('../es6'); var is = require('object-is'); var forEach = require('foreach'); var functionName = require('function.prototype.name'); var debug = require('object-inspect'); var hasSymbols = require('has-symbols')(); var hasSymbolToPrimitive = hasSymbols && typeof Symbol.toPrimitive === 'symbol'; test('function properties', function (t) { t.equal(toPrimitive.length, 1, 'length is 1'); t.equal(functionName(toPrimitive), 'ToPrimitive', 'name is ToPrimitive'); t.end(); }); var primitives = [null, undefined, true, false, 0, -0, 42, NaN, Infinity, -Infinity, '', 'abc']; test('primitives', function (t) { forEach(primitives, function (i) { t.ok(is(toPrimitive(i), i), 'toPrimitive(' + debug(i) + ') returns the same value'); t.ok(is(toPrimitive(i, String), i), 'toPrimitive(' + debug(i) + ', String) returns the same value'); t.ok(is(toPrimitive(i, Number), i), 'toPrimitive(' + debug(i) + ', Number) returns the same value'); }); t.end(); }); test('Symbols', { skip: !hasSymbols }, function (t) { var symbols = [ Symbol('foo'), Symbol.iterator, Symbol['for']('foo') // eslint-disable-line no-restricted-properties ]; forEach(symbols, function (sym) { t.equal(toPrimitive(sym), sym, 'toPrimitive(' + debug(sym) + ') returns the same value'); t.equal(toPrimitive(sym, String), sym, 'toPrimitive(' + debug(sym) + ', String) returns the same value'); t.equal(toPrimitive(sym, Number), sym, 'toPrimitive(' + debug(sym) + ', Number) returns the same value'); }); var primitiveSym = Symbol('primitiveSym'); var objectSym = Object(primitiveSym); t.equal(toPrimitive(objectSym), primitiveSym, 'toPrimitive(' + debug(objectSym) + ') returns ' + debug(primitiveSym)); t.equal(toPrimitive(objectSym, String), primitiveSym, 'toPrimitive(' + debug(objectSym) + ', String) returns ' + debug(primitiveSym)); t.equal(toPrimitive(objectSym, Number), primitiveSym, 'toPrimitive(' + debug(objectSym) + ', Number) returns ' + debug(primitiveSym)); t.end(); }); test('Arrays', function (t) { var arrays = [[], ['a', 'b'], [1, 2]]; forEach(arrays, function (arr) { t.equal(toPrimitive(arr), String(arr), 'toPrimitive(' + debug(arr) + ') returns the string version of the array'); t.equal(toPrimitive(arr, String), String(arr), 'toPrimitive(' + debug(arr) + ') returns the string version of the array'); t.equal(toPrimitive(arr, Number), String(arr), 'toPrimitive(' + debug(arr) + ') returns the string version of the array'); }); t.end(); }); test('Dates', function (t) { var dates = [new Date(), new Date(0), new Date(NaN)]; forEach(dates, function (date) { t.equal(toPrimitive(date), String(date), 'toPrimitive(' + debug(date) + ') returns the string version of the date'); t.equal(toPrimitive(date, String), String(date), 'toPrimitive(' + debug(date) + ') returns the string version of the date'); t.ok(is(toPrimitive(date, Number), Number(date)), 'toPrimitive(' + debug(date) + ') returns the number version of the date'); }); t.end(); }); var coercibleObject = { valueOf: function () { return 3; }, toString: function () { return 42; } }; var valueOfOnlyObject = { valueOf: function () { return 4; }, toString: function () { return {}; } }; var toStringOnlyObject = { valueOf: function () { return {}; }, toString: function () { return 7; } }; var coercibleFnObject = { valueOf: function () { return function valueOfFn() {}; }, toString: function () { return 42; } }; var uncoercibleObject = { valueOf: function () { return {}; }, toString: function () { return {}; } }; var uncoercibleFnObject = { valueOf: function () { return function valueOfFn() {}; }, toString: function () { return function toStrFn() {}; } }; test('Objects', function (t) { t.equal(toPrimitive(coercibleObject), coercibleObject.valueOf(), 'coercibleObject with no hint coerces to valueOf'); t.equal(toPrimitive(coercibleObject, Number), coercibleObject.valueOf(), 'coercibleObject with hint Number coerces to valueOf'); t.equal(toPrimitive(coercibleObject, String), coercibleObject.toString(), 'coercibleObject with hint String coerces to non-stringified toString'); t.equal(toPrimitive(coercibleFnObject), coercibleFnObject.toString(), 'coercibleFnObject coerces to non-stringified toString'); t.equal(toPrimitive(coercibleFnObject, Number), coercibleFnObject.toString(), 'coercibleFnObject with hint Number coerces to non-stringified toString'); t.equal(toPrimitive(coercibleFnObject, String), coercibleFnObject.toString(), 'coercibleFnObject with hint String coerces to non-stringified toString'); t.equal(toPrimitive({}), '[object Object]', '{} with no hint coerces to Object#toString'); t.equal(toPrimitive({}, Number), '[object Object]', '{} with hint Number coerces to Object#toString'); t.equal(toPrimitive({}, String), '[object Object]', '{} with hint String coerces to Object#toString'); t.equal(toPrimitive(toStringOnlyObject), toStringOnlyObject.toString(), 'toStringOnlyObject returns non-stringified toString'); t.equal(toPrimitive(toStringOnlyObject, Number), toStringOnlyObject.toString(), 'toStringOnlyObject with hint Number returns non-stringified toString'); t.equal(toPrimitive(toStringOnlyObject, String), toStringOnlyObject.toString(), 'toStringOnlyObject with hint String returns non-stringified toString'); t.equal(toPrimitive(valueOfOnlyObject), valueOfOnlyObject.valueOf(), 'valueOfOnlyObject returns valueOf'); t.equal(toPrimitive(valueOfOnlyObject, Number), valueOfOnlyObject.valueOf(), 'valueOfOnlyObject with hint Number returns valueOf'); t.equal(toPrimitive(valueOfOnlyObject, String), valueOfOnlyObject.valueOf(), 'valueOfOnlyObject with hint String returns non-stringified valueOf'); t.test('Symbol.toPrimitive', { skip: !hasSymbolToPrimitive }, function (st) { var overriddenObject = { toString: st.fail, valueOf: st.fail }; overriddenObject[Symbol.toPrimitive] = function (hint) { return String(hint); }; st.equal(toPrimitive(overriddenObject), 'default', 'object with Symbol.toPrimitive + no hint invokes that'); st.equal(toPrimitive(overriddenObject, Number), 'number', 'object with Symbol.toPrimitive + hint Number invokes that'); st.equal(toPrimitive(overriddenObject, String), 'string', 'object with Symbol.toPrimitive + hint String invokes that'); var nullToPrimitive = { toString: coercibleObject.toString, valueOf: coercibleObject.valueOf }; nullToPrimitive[Symbol.toPrimitive] = null; st.equal(toPrimitive(nullToPrimitive), toPrimitive(coercibleObject), 'object with no hint + null Symbol.toPrimitive ignores it'); st.equal(toPrimitive(nullToPrimitive, Number), toPrimitive(coercibleObject, Number), 'object with hint Number + null Symbol.toPrimitive ignores it'); st.equal(toPrimitive(nullToPrimitive, String), toPrimitive(coercibleObject, String), 'object with hint String + null Symbol.toPrimitive ignores it'); st.test('exceptions', function (sst) { var nonFunctionToPrimitive = { toString: sst.fail, valueOf: sst.fail }; nonFunctionToPrimitive[Symbol.toPrimitive] = {}; sst['throws'](toPrimitive.bind(null, nonFunctionToPrimitive), TypeError, 'Symbol.toPrimitive returning a non-function throws'); var uncoercibleToPrimitive = { toString: sst.fail, valueOf: sst.fail }; uncoercibleToPrimitive[Symbol.toPrimitive] = function (hint) { return { toString: function () { return hint; } }; }; sst['throws'](toPrimitive.bind(null, uncoercibleToPrimitive), TypeError, 'Symbol.toPrimitive returning an object throws'); var throwingToPrimitive = { toString: sst.fail, valueOf: sst.fail }; throwingToPrimitive[Symbol.toPrimitive] = function (hint) { throw new RangeError(hint); }; sst['throws'](toPrimitive.bind(null, throwingToPrimitive), RangeError, 'Symbol.toPrimitive throwing throws'); sst.end(); }); st.end(); }); t.test('exceptions', function (st) { st['throws'](toPrimitive.bind(null, uncoercibleObject), TypeError, 'uncoercibleObject throws a TypeError'); st['throws'](toPrimitive.bind(null, uncoercibleObject, Number), TypeError, 'uncoercibleObject with hint Number throws a TypeError'); st['throws'](toPrimitive.bind(null, uncoercibleObject, String), TypeError, 'uncoercibleObject with hint String throws a TypeError'); st['throws'](toPrimitive.bind(null, uncoercibleFnObject), TypeError, 'uncoercibleFnObject throws a TypeError'); st['throws'](toPrimitive.bind(null, uncoercibleFnObject, Number), TypeError, 'uncoercibleFnObject with hint Number throws a TypeError'); st['throws'](toPrimitive.bind(null, uncoercibleFnObject, String), TypeError, 'uncoercibleFnObject with hint String throws a TypeError'); st.end(); }); t.end(); }); apollo-server-demo/node_modules/es-to-primitive/test/es2015.js 0000644 0001750 0000144 00000021034 03560116604 023721 0 ustar andreh users 'use strict'; var test = require('tape'); var toPrimitive = require('../es2015'); var is = require('object-is'); var forEach = require('foreach'); var functionName = require('function.prototype.name'); var debug = require('object-inspect'); var hasSymbols = typeof Symbol === 'function' && typeof Symbol.iterator === 'symbol'; var hasSymbolToPrimitive = hasSymbols && typeof Symbol.toPrimitive === 'symbol'; test('function properties', function (t) { t.equal(toPrimitive.length, 1, 'length is 1'); t.equal(functionName(toPrimitive), 'ToPrimitive', 'name is ToPrimitive'); t.end(); }); var primitives = [null, undefined, true, false, 0, -0, 42, NaN, Infinity, -Infinity, '', 'abc']; test('primitives', function (t) { forEach(primitives, function (i) { t.ok(is(toPrimitive(i), i), 'toPrimitive(' + debug(i) + ') returns the same value'); t.ok(is(toPrimitive(i, String), i), 'toPrimitive(' + debug(i) + ', String) returns the same value'); t.ok(is(toPrimitive(i, Number), i), 'toPrimitive(' + debug(i) + ', Number) returns the same value'); }); t.end(); }); test('Symbols', { skip: !hasSymbols }, function (t) { var symbols = [ Symbol('foo'), Symbol.iterator, Symbol['for']('foo') // eslint-disable-line no-restricted-properties ]; forEach(symbols, function (sym) { t.equal(toPrimitive(sym), sym, 'toPrimitive(' + debug(sym) + ') returns the same value'); t.equal(toPrimitive(sym, String), sym, 'toPrimitive(' + debug(sym) + ', String) returns the same value'); t.equal(toPrimitive(sym, Number), sym, 'toPrimitive(' + debug(sym) + ', Number) returns the same value'); }); var primitiveSym = Symbol('primitiveSym'); var objectSym = Object(primitiveSym); t.equal(toPrimitive(objectSym), primitiveSym, 'toPrimitive(' + debug(objectSym) + ') returns ' + debug(primitiveSym)); t.equal(toPrimitive(objectSym, String), primitiveSym, 'toPrimitive(' + debug(objectSym) + ', String) returns ' + debug(primitiveSym)); t.equal(toPrimitive(objectSym, Number), primitiveSym, 'toPrimitive(' + debug(objectSym) + ', Number) returns ' + debug(primitiveSym)); t.end(); }); test('Arrays', function (t) { var arrays = [[], ['a', 'b'], [1, 2]]; forEach(arrays, function (arr) { t.equal(toPrimitive(arr), String(arr), 'toPrimitive(' + debug(arr) + ') returns the string version of the array'); t.equal(toPrimitive(arr, String), String(arr), 'toPrimitive(' + debug(arr) + ') returns the string version of the array'); t.equal(toPrimitive(arr, Number), String(arr), 'toPrimitive(' + debug(arr) + ') returns the string version of the array'); }); t.end(); }); test('Dates', function (t) { var dates = [new Date(), new Date(0), new Date(NaN)]; forEach(dates, function (date) { t.equal(toPrimitive(date), String(date), 'toPrimitive(' + debug(date) + ') returns the string version of the date'); t.equal(toPrimitive(date, String), String(date), 'toPrimitive(' + debug(date) + ') returns the string version of the date'); t.ok(is(toPrimitive(date, Number), Number(date)), 'toPrimitive(' + debug(date) + ') returns the number version of the date'); }); t.end(); }); var coercibleObject = { valueOf: function () { return 3; }, toString: function () { return 42; } }; var valueOfOnlyObject = { valueOf: function () { return 4; }, toString: function () { return {}; } }; var toStringOnlyObject = { valueOf: function () { return {}; }, toString: function () { return 7; } }; var coercibleFnObject = { valueOf: function () { return function valueOfFn() {}; }, toString: function () { return 42; } }; var uncoercibleObject = { valueOf: function () { return {}; }, toString: function () { return {}; } }; var uncoercibleFnObject = { valueOf: function () { return function valueOfFn() {}; }, toString: function () { return function toStrFn() {}; } }; test('Objects', function (t) { t.equal(toPrimitive(coercibleObject), coercibleObject.valueOf(), 'coercibleObject with no hint coerces to valueOf'); t.equal(toPrimitive(coercibleObject, Number), coercibleObject.valueOf(), 'coercibleObject with hint Number coerces to valueOf'); t.equal(toPrimitive(coercibleObject, String), coercibleObject.toString(), 'coercibleObject with hint String coerces to non-stringified toString'); t.equal(toPrimitive(coercibleFnObject), coercibleFnObject.toString(), 'coercibleFnObject coerces to non-stringified toString'); t.equal(toPrimitive(coercibleFnObject, Number), coercibleFnObject.toString(), 'coercibleFnObject with hint Number coerces to non-stringified toString'); t.equal(toPrimitive(coercibleFnObject, String), coercibleFnObject.toString(), 'coercibleFnObject with hint String coerces to non-stringified toString'); t.equal(toPrimitive({}), '[object Object]', '{} with no hint coerces to Object#toString'); t.equal(toPrimitive({}, Number), '[object Object]', '{} with hint Number coerces to Object#toString'); t.equal(toPrimitive({}, String), '[object Object]', '{} with hint String coerces to Object#toString'); t.equal(toPrimitive(toStringOnlyObject), toStringOnlyObject.toString(), 'toStringOnlyObject returns non-stringified toString'); t.equal(toPrimitive(toStringOnlyObject, Number), toStringOnlyObject.toString(), 'toStringOnlyObject with hint Number returns non-stringified toString'); t.equal(toPrimitive(toStringOnlyObject, String), toStringOnlyObject.toString(), 'toStringOnlyObject with hint String returns non-stringified toString'); t.equal(toPrimitive(valueOfOnlyObject), valueOfOnlyObject.valueOf(), 'valueOfOnlyObject returns valueOf'); t.equal(toPrimitive(valueOfOnlyObject, Number), valueOfOnlyObject.valueOf(), 'valueOfOnlyObject with hint Number returns valueOf'); t.equal(toPrimitive(valueOfOnlyObject, String), valueOfOnlyObject.valueOf(), 'valueOfOnlyObject with hint String returns non-stringified valueOf'); t.test('Symbol.toPrimitive', { skip: !hasSymbolToPrimitive }, function (st) { var overriddenObject = { toString: st.fail, valueOf: st.fail }; overriddenObject[Symbol.toPrimitive] = function (hint) { return String(hint); }; st.equal(toPrimitive(overriddenObject), 'default', 'object with Symbol.toPrimitive + no hint invokes that'); st.equal(toPrimitive(overriddenObject, Number), 'number', 'object with Symbol.toPrimitive + hint Number invokes that'); st.equal(toPrimitive(overriddenObject, String), 'string', 'object with Symbol.toPrimitive + hint String invokes that'); var nullToPrimitive = { toString: coercibleObject.toString, valueOf: coercibleObject.valueOf }; nullToPrimitive[Symbol.toPrimitive] = null; st.equal(toPrimitive(nullToPrimitive), toPrimitive(coercibleObject), 'object with no hint + null Symbol.toPrimitive ignores it'); st.equal(toPrimitive(nullToPrimitive, Number), toPrimitive(coercibleObject, Number), 'object with hint Number + null Symbol.toPrimitive ignores it'); st.equal(toPrimitive(nullToPrimitive, String), toPrimitive(coercibleObject, String), 'object with hint String + null Symbol.toPrimitive ignores it'); st.test('exceptions', function (sst) { var nonFunctionToPrimitive = { toString: sst.fail, valueOf: sst.fail }; nonFunctionToPrimitive[Symbol.toPrimitive] = {}; sst['throws'](toPrimitive.bind(null, nonFunctionToPrimitive), TypeError, 'Symbol.toPrimitive returning a non-function throws'); var uncoercibleToPrimitive = { toString: sst.fail, valueOf: sst.fail }; uncoercibleToPrimitive[Symbol.toPrimitive] = function (hint) { return { toString: function () { return hint; } }; }; sst['throws'](toPrimitive.bind(null, uncoercibleToPrimitive), TypeError, 'Symbol.toPrimitive returning an object throws'); var throwingToPrimitive = { toString: sst.fail, valueOf: sst.fail }; throwingToPrimitive[Symbol.toPrimitive] = function (hint) { throw new RangeError(hint); }; sst['throws'](toPrimitive.bind(null, throwingToPrimitive), RangeError, 'Symbol.toPrimitive throwing throws'); sst.end(); }); st.end(); }); t.test('exceptions', function (st) { st['throws'](toPrimitive.bind(null, uncoercibleObject), TypeError, 'uncoercibleObject throws a TypeError'); st['throws'](toPrimitive.bind(null, uncoercibleObject, Number), TypeError, 'uncoercibleObject with hint Number throws a TypeError'); st['throws'](toPrimitive.bind(null, uncoercibleObject, String), TypeError, 'uncoercibleObject with hint String throws a TypeError'); st['throws'](toPrimitive.bind(null, uncoercibleFnObject), TypeError, 'uncoercibleFnObject throws a TypeError'); st['throws'](toPrimitive.bind(null, uncoercibleFnObject, Number), TypeError, 'uncoercibleFnObject with hint Number throws a TypeError'); st['throws'](toPrimitive.bind(null, uncoercibleFnObject, String), TypeError, 'uncoercibleFnObject with hint String throws a TypeError'); st.end(); }); t.end(); }); apollo-server-demo/node_modules/es-to-primitive/es6.js 0000644 0001750 0000144 00000000065 03560116604 022521 0 ustar andreh users 'use strict'; module.exports = require('./es2015'); apollo-server-demo/node_modules/es-to-primitive/helpers/ 0000755 0001750 0000144 00000000000 14067647701 023142 5 ustar andreh users apollo-server-demo/node_modules/es-to-primitive/helpers/isPrimitive.js 0000644 0001750 0000144 00000000227 03560116604 025772 0 ustar andreh users 'use strict'; module.exports = function isPrimitive(value) { return value === null || (typeof value !== 'function' && typeof value !== 'object'); }; apollo-server-demo/node_modules/es-to-primitive/CHANGELOG.md 0000644 0001750 0000144 00000004100 03560116604 023271 0 ustar andreh users 1.2.1 / 2019-11-08 ================= * [readme] remove testling URLs * [meta] add `funding` field * [meta] create FUNDING.yml * [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `covert`, `replace`, `semver`, `tape`, `function.prototype.name` * [Tests] use shared travis-ci configs * [Tests] Add es5 tests for `symbol` types (#45) * [Tests] use `npx aud` instead of `nsp` or `npm audit` with hoops * [Tests] remove `jscs` 1.2.0 / 2018-09-27 ================= * [New] create ES2015 entry point/property, to replace ES6 * [Fix] Ensure optional arguments are not part of the length (#29) * [Deps] update `is-callable` * [Dev Deps] update `tape`, `jscs`, `nsp`, `eslint`, `@ljharb/eslint-config`, `semver`, `object-inspect`, `replace` * [Tests] avoid util.inspect bug with `new Date(NaN)` on node v6.0 and v6.1. * [Tests] up to `node` `v10.11`, `v9.11`, `v8.12`, `v6.14`, `v4.9` 1.1.1 / 2016-01-03 ================= * [Fix: ES5] fix coercion logic: ES5’s ToPrimitive does not coerce any primitive value, regardless of hint (#2) 1.1.0 / 2015-12-27 ================= * [New] add `Symbol.toPrimitive` support * [Deps] update `is-callable`, `is-date-object` * [Dev Deps] update `eslint`, `tape`, `semver`, `jscs`, `covert`, `nsp`, `@ljharb/eslint-config` * [Dev Deps] remove unused deps * [Tests] up to `node` `v5.3` * [Tests] fix npm upgrades on older node versions * [Tests] fix testling * [Docs] Switch from vb.teelaun.ch to versionbadg.es for the npm version badge SVG 1.0.1 / 2016-01-03 ================= * [Fix: ES5] fix coercion logic: ES5’s ToPrimitive does not coerce any primitive value, regardless of hint (#2) * [Deps] update `is-callable`, `is-date-object` * [Dev Deps] update `eslint`, `tape`, `semver`, `jscs`, `covert`, `nsp`, `@ljharb/eslint-config` * [Dev Deps] remove unused deps * [Tests] up to `node` `v5.3` * [Tests] fix npm upgrades on older node versions * [Tests] fix testling * [Docs] Switch from vb.teelaun.ch to versionbadg.es for the npm version badge SVG 1.0.0 / 2015-03-19 ================= * Initial release. apollo-server-demo/node_modules/es-to-primitive/.travis.yml 0000644 0001750 0000144 00000000453 03560116604 023600 0 ustar andreh users version: ~> 1.0 language: node_js cache: directories: - "$(nvm cache dir)" os: - linux import: - ljharb/travis-ci:node/all.yml - ljharb/travis-ci:node/pretest.yml - ljharb/travis-ci:node/posttest.yml - ljharb/travis-ci:node/coverage.yml matrix: allow_failures: - env: COVERAGE=true apollo-server-demo/node_modules/es-to-primitive/es2015.js 0000644 0001750 0000144 00000004133 03560116604 022743 0 ustar andreh users 'use strict'; var hasSymbols = typeof Symbol === 'function' && typeof Symbol.iterator === 'symbol'; var isPrimitive = require('./helpers/isPrimitive'); var isCallable = require('is-callable'); var isDate = require('is-date-object'); var isSymbol = require('is-symbol'); var ordinaryToPrimitive = function OrdinaryToPrimitive(O, hint) { if (typeof O === 'undefined' || O === null) { throw new TypeError('Cannot call method on ' + O); } if (typeof hint !== 'string' || (hint !== 'number' && hint !== 'string')) { throw new TypeError('hint must be "string" or "number"'); } var methodNames = hint === 'string' ? ['toString', 'valueOf'] : ['valueOf', 'toString']; var method, result, i; for (i = 0; i < methodNames.length; ++i) { method = O[methodNames[i]]; if (isCallable(method)) { result = method.call(O); if (isPrimitive(result)) { return result; } } } throw new TypeError('No default value'); }; var GetMethod = function GetMethod(O, P) { var func = O[P]; if (func !== null && typeof func !== 'undefined') { if (!isCallable(func)) { throw new TypeError(func + ' returned for property ' + P + ' of object ' + O + ' is not a function'); } return func; } return void 0; }; // http://www.ecma-international.org/ecma-262/6.0/#sec-toprimitive module.exports = function ToPrimitive(input) { if (isPrimitive(input)) { return input; } var hint = 'default'; if (arguments.length > 1) { if (arguments[1] === String) { hint = 'string'; } else if (arguments[1] === Number) { hint = 'number'; } } var exoticToPrim; if (hasSymbols) { if (Symbol.toPrimitive) { exoticToPrim = GetMethod(input, Symbol.toPrimitive); } else if (isSymbol(input)) { exoticToPrim = Symbol.prototype.valueOf; } } if (typeof exoticToPrim !== 'undefined') { var result = exoticToPrim.call(input, hint); if (isPrimitive(result)) { return result; } throw new TypeError('unable to convert exotic object to primitive'); } if (hint === 'default' && (isDate(input) || isSymbol(input))) { hint = 'string'; } return ordinaryToPrimitive(input, hint === 'default' ? 'number' : hint); }; apollo-server-demo/node_modules/util.promisify/ 0000755 0001750 0000144 00000000000 14067647700 021437 5 ustar andreh users apollo-server-demo/node_modules/util.promisify/index.js 0000644 0001750 0000144 00000001147 03560116604 023075 0 ustar andreh users 'use strict'; var define = require('define-properties'); var util = require('util'); var implementation = require('./implementation'); var getPolyfill = require('./polyfill'); var polyfill = getPolyfill(); var shim = require('./shim'); /* eslint-disable no-unused-vars */ var boundPromisify = function promisify(orig) { /* eslint-enable no-unused-vars */ return polyfill.apply(util, arguments); }; define(boundPromisify, { custom: polyfill.custom, customPromisifyArgs: polyfill.customPromisifyArgs, getPolyfill: getPolyfill, implementation: implementation, shim: shim }); module.exports = boundPromisify; apollo-server-demo/node_modules/util.promisify/LICENSE 0000644 0001750 0000144 00000002057 03560116604 022436 0 ustar andreh users MIT License Copyright (c) 2017 Jordan Harband Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/util.promisify/.eslintrc 0000644 0001750 0000144 00000000477 03560116604 023261 0 ustar andreh users { "root": true, "extends": "@ljharb", "env": { "es6": true }, "rules": { "id-length": [2, { "min": 1, "max": 30 }], "max-lines": [2, 100], "max-lines-per-function": [2, 70], "max-statements": [2, 18], "multiline-comment-style": 0, "no-magic-numbers": 0, "operator-linebreak": [2, "before"] } } apollo-server-demo/node_modules/util.promisify/.github/ 0000755 0001750 0000144 00000000000 14067647700 022777 5 ustar andreh users apollo-server-demo/node_modules/util.promisify/.github/FUNDING.yml 0000644 0001750 0000144 00000001111 03560116604 024574 0 ustar andreh users # These are supported funding model platforms github: [ljharb] patreon: # Replace with a single Patreon username open_collective: # Replace with a single Open Collective username ko_fi: # Replace with a single Ko-fi username tidelift: npm/util.promisify community_bridge: # Replace with a single Community Bridge project-name e.g., cloud-foundry liberapay: # Replace with a single Liberapay username issuehunt: # Replace with a single IssueHunt username otechie: # Replace with a single Otechie username custom: # Replace with up to 4 custom sponsorship URLs e.g., ['link1', 'link2'] apollo-server-demo/node_modules/util.promisify/README.md 0000644 0001750 0000144 00000001610 03560116604 022702 0 ustar andreh users # util.promisify Polyfill for util.promisify in node versions < v8 node v8.0.0 added support for a built-in `util.promisify`: https://github.com/nodejs/node/pull/12442/ This package provides the built-in `util.promisify` in node v8.0.0 and later, and a replacement in other environments. ## Usage **Direct** ```js const promisify = require('util.promisify'); // Use `promisify` just like the built-in method on `util` ``` **Shim** ```js require('util.promisify/shim')(); // `util.promisify` is now defined const util = require('util'); // Use `util.promisify` ``` Note: this package requires a native ES5 environment, and for `Promise` to be globally available. It will throw upon requiring it if these are not present. ## Promisifying modules If you want to promisify a whole module, like the `fs` module, you can use [`util.promisify-all`](https://www.npmjs.com/package/util.promisify-all). apollo-server-demo/node_modules/util.promisify/package.json 0000644 0001750 0000144 00000003601 03560116604 023713 0 ustar andreh users { "name": "util.promisify", "version": "1.1.1", "description": "Polyfill/shim for util.promisify in node versions < v8", "main": "index.js", "dependencies": { "call-bind": "^1.0.0", "define-properties": "^1.1.3", "for-each": "^0.3.3", "has-symbols": "^1.0.1", "object.getownpropertydescriptors": "^2.1.1" }, "devDependencies": { "@es-shims/api": "^2.1.2", "@ljharb/eslint-config": "^17.3.0", "aud": "^1.1.3", "auto-changelog": "^2.2.1", "eslint": "^7.17.0", "nyc": "^10.3.2", "safe-publish-latest": "^1.1.4", "tape": "^5.1.1" }, "scripts": { "prepublish": "safe-publish-latest", "lint": "eslint .", "postlint": "es-shim-api --bound", "pretest": "npm run lint", "tests-only": "nyc tape 'test/**/*.js'", "test": "npm run tests-only", "posttest": "aud --production", "version": "auto-changelog && git add CHANGELOG.md", "postversion": "auto-changelog && git add CHANGELOG.md && git commit --no-edit --amend && git tag -f \"v$(node -e \"console.log(require('./package.json').version)\")\"" }, "repository": { "type": "git", "url": "git+https://github.com/ljharb/util.promisify.git" }, "keywords": [ "promisify", "promise", "util", "polyfill", "shim", "util.promisify" ], "author": "Jordan Harband <ljharb@gmail.com>", "funding": { "url": "https://github.com/sponsors/ljharb" }, "license": "MIT", "bugs": { "url": "https://github.com/ljharb/util.promisify/issues" }, "homepage": "https://github.com/ljharb/util.promisify#readme", "auto-changelog": { "output": "CHANGELOG.md", "template": "keepachangelog", "unreleased": false, "commitLimit": false, "backfillLimit": false, "hideCredit": true } ,"_resolved": "https://registry.npmjs.org/util.promisify/-/util.promisify-1.1.1.tgz" ,"_integrity": "sha512-/s3UsZUrIfa6xDhr7zZhnE9SLQ5RIXyYfiVnMMyMDzOc8WhWN4Nbh36H842OyurKbCDAesZOJaVyvmSl6fhGQw==" ,"_from": "util.promisify@1.1.1" } apollo-server-demo/node_modules/util.promisify/auto.js 0000644 0001750 0000144 00000000044 03560116604 022731 0 ustar andreh users 'use strict'; require('./shim')(); apollo-server-demo/node_modules/util.promisify/shim.js 0000644 0001750 0000144 00000000545 03560116604 022727 0 ustar andreh users 'use strict'; var util = require('util'); var getPolyfill = require('./polyfill'); module.exports = function shimUtilPromisify() { var polyfill = getPolyfill(); if (polyfill !== util.promisify) { Object.defineProperty(util, 'promisify', { configurable: true, enumerable: true, value: polyfill, writable: true }); } return polyfill; }; apollo-server-demo/node_modules/util.promisify/implementation.js 0000644 0001750 0000144 00000006032 03560116604 025011 0 ustar andreh users 'use strict'; var forEach = require('for-each'); var isES5 = typeof Object.defineProperty === 'function'; var hasProto = [].__proto__ === Array.prototype; // eslint-disable-line no-proto if (!isES5 || !hasProto) { throw new TypeError('util.promisify requires a true ES5 environment, that also supports `__proto__`'); } var getOwnPropertyDescriptors = require('object.getownpropertydescriptors'); if (typeof Promise !== 'function') { throw new TypeError('`Promise` must be globally available for util.promisify to work.'); } var callBound = require('call-bind/callBound'); var $slice = callBound('Array.prototype.slice'); var $concat = callBound('Array.prototype.concat'); var $forEach = callBound('Array.prototype.forEach'); var hasSymbols = require('has-symbols')(); // eslint-disable-next-line no-restricted-properties var kCustomPromisifiedSymbol = hasSymbols ? Symbol['for']('nodejs.util.promisify.custom') : null; var kCustomPromisifyArgsSymbol = hasSymbols ? Symbol('customPromisifyArgs') : null; module.exports = function promisify(orig) { if (typeof orig !== 'function') { var error = new TypeError('The "original" argument must be of type function'); error.name = 'TypeError [ERR_INVALID_ARG_TYPE]'; error.code = 'ERR_INVALID_ARG_TYPE'; throw error; } if (hasSymbols && orig[kCustomPromisifiedSymbol]) { var customFunction = orig[kCustomPromisifiedSymbol]; if (typeof customFunction !== 'function') { throw new TypeError('The [util.promisify.custom] property must be a function'); } Object.defineProperty(customFunction, kCustomPromisifiedSymbol, { configurable: true, enumerable: false, value: customFunction, writable: false }); return customFunction; } // Names to create an object from in case the callback receives multiple // arguments, e.g. ['stdout', 'stderr'] for child_process.exec. var argumentNames = orig[kCustomPromisifyArgsSymbol]; var promisified = function fn() { var args = $slice(arguments); var self = this; // eslint-disable-line no-invalid-this return new Promise(function (resolve, reject) { orig.apply(self, $concat(args, function (err) { var values = arguments.length > 1 ? $slice(arguments, 1) : []; if (err) { reject(err); } else if (typeof argumentNames !== 'undefined' && values.length > 1) { var obj = {}; $forEach(argumentNames, function (name, index) { obj[name] = values[index]; }); resolve(obj); } else { resolve(values[0]); } })); }); }; promisified.__proto__ = orig.__proto__; // eslint-disable-line no-proto Object.defineProperty(promisified, kCustomPromisifiedSymbol, { configurable: true, enumerable: false, value: promisified, writable: false }); var descriptors = getOwnPropertyDescriptors(orig); forEach(descriptors, function (k, v) { try { Object.defineProperty(promisified, k, v); } catch (e) { // handle nonconfigurable function properties } }); return promisified; }; module.exports.custom = kCustomPromisifiedSymbol; module.exports.customPromisifyArgs = kCustomPromisifyArgsSymbol; apollo-server-demo/node_modules/util.promisify/.nycrc 0000644 0001750 0000144 00000000330 03560116604 022540 0 ustar andreh users { "all": true, "check-coverage": false, "reporter": ["text-summary", "text", "html", "json"], "lines": 86, "statements": 85.93, "functions": 82.43, "branches": 76.06, "exclude": [ "coverage", "test" ] } apollo-server-demo/node_modules/util.promisify/test/ 0000755 0001750 0000144 00000000000 14067647700 022416 5 ustar andreh users apollo-server-demo/node_modules/util.promisify/test/index.js 0000644 0001750 0000144 00000000577 03560116604 024062 0 ustar andreh users 'use strict'; var test = require('tape'); var runTests = require('./tests'); test('as a function', function (t) { /* eslint global-require: 1 */ if (typeof Promise === 'function') { var promisify = require('../'); runTests(promisify, t); } else { t['throws']( function () { require('../'); }, TypeError, 'throws when no Promise available' ); } t.end(); }); apollo-server-demo/node_modules/util.promisify/test/tests.js 0000644 0001750 0000144 00000003051 03560116604 024103 0 ustar andreh users 'use strict'; var hasSymbols = require('has-symbols')(); module.exports = function runTests(promisify, t) { t.equal(typeof promisify, 'function', 'promisify is a function'); t['throws']( function () { promisify(null); }, TypeError, 'throws on non-functions' ); var yes = function () { var cb = arguments[arguments.length - 1]; cb(null, Array.prototype.slice.call(arguments, 0, -1)); }; var no = function () { var cb = arguments[arguments.length - 1]; cb(Array.prototype.slice.call(arguments, 0, -1)); }; var pYes = promisify(yes); var pNo = promisify(no); t.equal(typeof pYes, 'function', 'pYes is a function'); t.equal(typeof pNo, 'function', 'pNo is a function'); t.test('pYes is properly promisified', { skip: typeof Promise !== 'function' }, function (st) { st.plan(1); var p = pYes(1, 2, 3); return p.then(function (result) { st.deepEqual(result, [1, 2, 3], 'fulfillment: arguments are preserved'); }); }); t.test('pNo is properly promisified', { skip: typeof Promise !== 'function' }, function (st) { st.plan(1); var p = pNo(1, 2, 3); return p.then(null, function (args) { st.deepEqual(args, [1, 2, 3], 'rejection: arguments are preserved'); }); }); t.test('custom symbol', { skip: !hasSymbols }, function (st) { st.equal(Symbol.keyFor(promisify.custom), 'nodejs.util.promisify.custom', 'is a global symbol with the right key'); // eslint-disable-next-line no-restricted-properties st.equal(Symbol['for']('nodejs.util.promisify.custom'), promisify.custom, 'is the global symbol'); st.end(); }); }; apollo-server-demo/node_modules/util.promisify/test/shimmed.js 0000644 0001750 0000144 00000000435 03560116604 024372 0 ustar andreh users 'use strict'; var test = require('tape'); var util = require('util'); var runTests = require('./tests'); test('shimmed', { skip: typeof Promise !== 'function' }, function (t) { require('../auto'); // eslint-disable-line global-require runTests(util.promisify, t); t.end(); }); apollo-server-demo/node_modules/util.promisify/test/implementation.js 0000644 0001750 0000144 00000000634 03560116604 025772 0 ustar andreh users 'use strict'; var test = require('tape'); var runTests = require('./tests'); test('implementation', function (t) { /* eslint global-require: 1 */ if (typeof Promise === 'function') { var promisify = require('../implementation'); runTests(promisify, t); } else { t['throws']( function () { require('../implementation'); }, TypeError, 'throws when no Promise available' ); } t.end(); }); apollo-server-demo/node_modules/util.promisify/.eslintignore 0000644 0001750 0000144 00000000012 03560116604 024121 0 ustar andreh users coverage/ apollo-server-demo/node_modules/util.promisify/CHANGELOG.md 0000644 0001750 0000144 00000020756 03560116604 023250 0 ustar andreh users # Changelog All notable changes to this project will be documented in this file. The format is based on [Keep a Changelog](https://keepachangelog.com/en/1.0.0/) and this project adheres to [Semantic Versioning](https://semver.org/spec/v2.0.0.html). ## [v1.1.1](https://github.com/ljharb/util.promisify/compare/v1.1.0...v1.1.1) - 2021-01-08 ### Commits - [Fix] add missing runtime dependency `has-symbols` [`9b45a3b`](https://github.com/ljharb/util.promisify/commit/9b45a3bfbc0bcf5e474e1d045aacca3dc9609e54) ## [v1.1.0](https://github.com/ljharb/util.promisify/compare/v1.0.1...v1.1.0) - 2021-01-06 ### Commits - [Tests] migrate tests to Github Actions [`a09e2f5`](https://github.com/ljharb/util.promisify/commit/a09e2f5cc3590c3098681c98b08dcb15b5c0877b) - [Tests] add tests [`5162b64`](https://github.com/ljharb/util.promisify/commit/5162b642805030b7d83e978e73392213d0b2431a) - [meta] do not publish github action workflow files [`4b5a39e`](https://github.com/ljharb/util.promisify/commit/4b5a39ed1df1c6ce86fb687f7494882fd29099ba) - [Fix] handle nonconfigurable own function properties, in older engines [`07693ae`](https://github.com/ljharb/util.promisify/commit/07693ae63cdc71d88c2203d62aca53623fba4815) - [New] use a global symbol for `util.promisify.custom` [`8f8631b`](https://github.com/ljharb/util.promisify/commit/8f8631b04c3f2cf1bd082837c8d73431e356eb2f) - [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `aud`, `auto-changelog` [`83e7267`](https://github.com/ljharb/util.promisify/commit/83e7267f27e38a9abcba6803f945c71a68255ff9) - [actions] add "Allow Edits" workflow [`e2a92ae`](https://github.com/ljharb/util.promisify/commit/e2a92ae988554713f89e62fcbf0ac602f76976f6) - [Tests] move `es-shim-api` to `postlint` [`7b93efa`](https://github.com/ljharb/util.promisify/commit/7b93efacd4c978b76d02be9b33b94b61ee366e65) - [Deps] use `call-bind` instead of `es-abstract` [`e68f500`](https://github.com/ljharb/util.promisify/commit/e68f500d9dd0cdd0563d72b758e34bdf1bed0d6c) - [actions] switch Automatic Rebase workflow to `pull_request_target` event [`7da936c`](https://github.com/ljharb/util.promisify/commit/7da936c0681062c5eb812185ebc9ccf4d86851c5) - [Dev Deps] update `aud`, `auto-changelog` [`88465d4`](https://github.com/ljharb/util.promisify/commit/88465d4202969895123e3113db3e8b45972ca2f6) - [Tests] only audit prod deps [`8a13dc5`](https://github.com/ljharb/util.promisify/commit/8a13dc5192ab899034e1f78151324ea06fb381b1) - [Deps] update `object.getownpropertydescriptors` [`899d30b`](https://github.com/ljharb/util.promisify/commit/899d30b3389b033b3964dd0e7faa0469db8b3ba4) - [Deps] update `es-abstract` [`552d18b`](https://github.com/ljharb/util.promisify/commit/552d18b34ebc0eda0d0bc33a84ca1827aa86aaf9) - [Dev Deps] update `auto-changelog` [`dd61917`](https://github.com/ljharb/util.promisify/commit/dd61917fabad7c8c4c52807ca4b5b40611a14e62) - [Deps] update `es-abstract` [`40a839a`](https://github.com/ljharb/util.promisify/commit/40a839a8db3d79699688d27f6613a827056428c8) - [Dev Deps] update `@ljharb/eslint-config` [`07c3b39`](https://github.com/ljharb/util.promisify/commit/07c3b3952682e9c4d58b6bfb9404049827b5c523) ## [v1.0.1](https://github.com/ljharb/util.promisify/compare/v1.0.0...v1.0.1) - 2020-01-16 ### Fixed - [Refactor] remove unnecessary duplication. Fixes #3. [`#3`](https://github.com/ljharb/util.promisify/issues/3) ### Commits - [Tests] use shared travis-ci configs [`f1b5e43`](https://github.com/ljharb/util.promisify/commit/f1b5e43359e74a30f35bd10a33be765de73917c6) - [Tests] up to `node` `v10.0`, `v9.11`, `v8.11`, `v6.14`, `4.9`; use `nvm install-latest-npm`; pin included builds to LTS [`e89390f`](https://github.com/ljharb/util.promisify/commit/e89390f498f7eb5111188fff5260cbb9f5216cd3) - [meta] add `auto-changelog` [`fe8e751`](https://github.com/ljharb/util.promisify/commit/fe8e751819a1318d3c929b086c70308aed50715d) - [Tests] up to `node` `v11.0`, `v10.12`, `v8.12` [`e09b894`](https://github.com/ljharb/util.promisify/commit/e09b894291aef2991e5c553f0b64968e03b58262) - [Refactor] use `callBound` helper from `es-abstract` for robustness [`baa0cf6`](https://github.com/ljharb/util.promisify/commit/baa0cf697068573cbe650e01aa6774154dd3f454) - [actions] add automatic rebasing / merge commit blocking [`24912f4`](https://github.com/ljharb/util.promisify/commit/24912f41b30d88b8984fb07307f737de6f576873) - [Docs] Add usage information for the shim/monkey-patch [`38b1ee5`](https://github.com/ljharb/util.promisify/commit/38b1ee56b558019213a6fdc2553796e8cdaf773e) - [Refactor] use `__proto__` instead of ES6’s `Object.setPrototypeOf` [`02ec7e2`](https://github.com/ljharb/util.promisify/commit/02ec7e241caf8848c1e141c801f98ed31325b59a) - [meta] create FUNDING.yml [`076b8b5`](https://github.com/ljharb/util.promisify/commit/076b8b5d19783a0e4c932e41782846e431deeb7d) - [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `safe-publish-latest` [`4cedaa9`](https://github.com/ljharb/util.promisify/commit/4cedaa9c6b0a77a0416b69d480b3b806c00dec6e) - Adds usage information to the README [`ddb4556`](https://github.com/ljharb/util.promisify/commit/ddb45562320ab8aea93dc0364640ea21ab68bfbb) - [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `safe-publish-latest` [`95362c0`](https://github.com/ljharb/util.promisify/commit/95362c0e93186a30ede6333430ddfa0606a769b4) - [Dev Deps] update `@es-shims/api`, `@ljharb/eslint-config`, `eslint` [`fd79a58`](https://github.com/ljharb/util.promisify/commit/fd79a58573186c83d81777fa0b1ad293b2f475e3) - [Dev Deps] update `eslint`, `@ljharb/eslint-config` [`2cf792b`](https://github.com/ljharb/util.promisify/commit/2cf792b9dcaab24b642ef1de8239ceb089fc5d38) - [Docs] Link to util.promisify-all [`032ff5c`](https://github.com/ljharb/util.promisify/commit/032ff5c6ee2958a02f56c770337441c3a587b88c) - [Tests] allow node 0.10 and 0.8 to fail again [`c2f8418`](https://github.com/ljharb/util.promisify/commit/c2f8418dfc36b83cd8a18b86a735c2936c6f5f9e) - [Tests] remove mistakenly added travis jobs [`13a242f`](https://github.com/ljharb/util.promisify/commit/13a242fb33dcbd4e2872436f2e430e62526fb147) - [Tests] on `node` `v10.1` [`8244578`](https://github.com/ljharb/util.promisify/commit/82445786197fd3e54aeffaa2fe0f1da38bcafec4) - [meta] add `funding` field [`e1645ca`](https://github.com/ljharb/util.promisify/commit/e1645ca10648d1ae917e3f5ae954b37de338dc20) - [New] add `auto` entry point [`2c48047`](https://github.com/ljharb/util.promisify/commit/2c480479d67646fb2bfb92a4e5d50ff14bcdca3c) - [Fix] use `has-symbols` package to ensure we support Symbol shams too. [`75135c8`](https://github.com/ljharb/util.promisify/commit/75135c8a48ea4e1be1cfe7a95af11905818303e7) - [Deps] update `es-abstract` [`32aa5cc`](https://github.com/ljharb/util.promisify/commit/32aa5ccd3ee7513edef99ed7d516d6c0f4901883) - [Dev Deps] update `eslint` [`c3043e6`](https://github.com/ljharb/util.promisify/commit/c3043e6e562847102e9136479268777bc07e9b26) - [Deps] update `object.getownpropertydescriptors` [`521ed25`](https://github.com/ljharb/util.promisify/commit/521ed25d40dc230b38ac3755036219fbaf94694c) - [Deps] update `has-symbol` [`16d91ec`](https://github.com/ljharb/util.promisify/commit/16d91ecc0016c31e49b7c3da938c19132c243732) - [Deps] update `define-properties` [`532915e`](https://github.com/ljharb/util.promisify/commit/532915ed58fe6f0edc3670837b510e09fb39b99a) - [Tests] `npm` v5+ breaks on node < v4 [`0647c63`](https://github.com/ljharb/util.promisify/commit/0647c63d932451c043c3e8f3b003c636057f035a) ## v1.0.0 - 2017-05-30 ### Commits - Dotfiles. [`02c20cb`](https://github.com/ljharb/util.promisify/commit/02c20cb4eb01cf656102f57f71635785114f1d09) - Initial implementation. [`05ff048`](https://github.com/ljharb/util.promisify/commit/05ff0480448f019a85675ce81ecc4e9bdc099286) - Initial commit [`9472155`](https://github.com/ljharb/util.promisify/commit/947215502491bb1b3238aa0ac5c67258e41db3a8) - package.json [`e0302c0`](https://github.com/ljharb/util.promisify/commit/e0302c01e5e3b1dd78647303f9a4337b5bb63196) - Initial readme. [`5df78e1`](https://github.com/ljharb/util.promisify/commit/5df78e16e89e8328c61d6bbac85409a36560fe3b) - [Dev Deps] add `safe-publish-latest` [`596b6b4`](https://github.com/ljharb/util.promisify/commit/596b6b4fbce79dbaf5fff366454ab5b31d2eb993) - [Tests] add `npm run lint` [`54c2ccb`](https://github.com/ljharb/util.promisify/commit/54c2ccb85db682fc293b30a0bfece76d0a5c7c60) - [Dev Deps] add `@es-shims/api` [`d9014f1`](https://github.com/ljharb/util.promisify/commit/d9014f12add2fb3fe743647df614c69ed305a824) - [Tests] allow 0.10 and 0.8 to fail, for now. [`c5c7b61`](https://github.com/ljharb/util.promisify/commit/c5c7b619b88878fc715d1768b48bd45378c9f807) apollo-server-demo/node_modules/util.promisify/polyfill.js 0000644 0001750 0000144 00000000437 03560116604 023621 0 ustar andreh users 'use strict'; var util = require('util'); var implementation = require('./implementation'); module.exports = function getPolyfill() { if (typeof util.promisify === 'function' && util.promisify.custom === implementation.custom) { return util.promisify; } return implementation; }; apollo-server-demo/node_modules/proxy-addr/ 0000755 0001750 0000144 00000000000 14067647700 020533 5 ustar andreh users apollo-server-demo/node_modules/proxy-addr/index.js 0000644 0001750 0000144 00000013560 03560116604 022173 0 ustar andreh users /*! * proxy-addr * Copyright(c) 2014-2016 Douglas Christopher Wilson * MIT Licensed */ 'use strict' /** * Module exports. * @public */ module.exports = proxyaddr module.exports.all = alladdrs module.exports.compile = compile /** * Module dependencies. * @private */ var forwarded = require('forwarded') var ipaddr = require('ipaddr.js') /** * Variables. * @private */ var DIGIT_REGEXP = /^[0-9]+$/ var isip = ipaddr.isValid var parseip = ipaddr.parse /** * Pre-defined IP ranges. * @private */ var IP_RANGES = { linklocal: ['169.254.0.0/16', 'fe80::/10'], loopback: ['127.0.0.1/8', '::1/128'], uniquelocal: ['10.0.0.0/8', '172.16.0.0/12', '192.168.0.0/16', 'fc00::/7'] } /** * Get all addresses in the request, optionally stopping * at the first untrusted. * * @param {Object} request * @param {Function|Array|String} [trust] * @public */ function alladdrs (req, trust) { // get addresses var addrs = forwarded(req) if (!trust) { // Return all addresses return addrs } if (typeof trust !== 'function') { trust = compile(trust) } for (var i = 0; i < addrs.length - 1; i++) { if (trust(addrs[i], i)) continue addrs.length = i + 1 } return addrs } /** * Compile argument into trust function. * * @param {Array|String} val * @private */ function compile (val) { if (!val) { throw new TypeError('argument is required') } var trust if (typeof val === 'string') { trust = [val] } else if (Array.isArray(val)) { trust = val.slice() } else { throw new TypeError('unsupported trust argument') } for (var i = 0; i < trust.length; i++) { val = trust[i] if (!Object.prototype.hasOwnProperty.call(IP_RANGES, val)) { continue } // Splice in pre-defined range val = IP_RANGES[val] trust.splice.apply(trust, [i, 1].concat(val)) i += val.length - 1 } return compileTrust(compileRangeSubnets(trust)) } /** * Compile `arr` elements into range subnets. * * @param {Array} arr * @private */ function compileRangeSubnets (arr) { var rangeSubnets = new Array(arr.length) for (var i = 0; i < arr.length; i++) { rangeSubnets[i] = parseipNotation(arr[i]) } return rangeSubnets } /** * Compile range subnet array into trust function. * * @param {Array} rangeSubnets * @private */ function compileTrust (rangeSubnets) { // Return optimized function based on length var len = rangeSubnets.length return len === 0 ? trustNone : len === 1 ? trustSingle(rangeSubnets[0]) : trustMulti(rangeSubnets) } /** * Parse IP notation string into range subnet. * * @param {String} note * @private */ function parseipNotation (note) { var pos = note.lastIndexOf('/') var str = pos !== -1 ? note.substring(0, pos) : note if (!isip(str)) { throw new TypeError('invalid IP address: ' + str) } var ip = parseip(str) if (pos === -1 && ip.kind() === 'ipv6' && ip.isIPv4MappedAddress()) { // Store as IPv4 ip = ip.toIPv4Address() } var max = ip.kind() === 'ipv6' ? 128 : 32 var range = pos !== -1 ? note.substring(pos + 1, note.length) : null if (range === null) { range = max } else if (DIGIT_REGEXP.test(range)) { range = parseInt(range, 10) } else if (ip.kind() === 'ipv4' && isip(range)) { range = parseNetmask(range) } else { range = null } if (range <= 0 || range > max) { throw new TypeError('invalid range on address: ' + note) } return [ip, range] } /** * Parse netmask string into CIDR range. * * @param {String} netmask * @private */ function parseNetmask (netmask) { var ip = parseip(netmask) var kind = ip.kind() return kind === 'ipv4' ? ip.prefixLengthFromSubnetMask() : null } /** * Determine address of proxied request. * * @param {Object} request * @param {Function|Array|String} trust * @public */ function proxyaddr (req, trust) { if (!req) { throw new TypeError('req argument is required') } if (!trust) { throw new TypeError('trust argument is required') } var addrs = alladdrs(req, trust) var addr = addrs[addrs.length - 1] return addr } /** * Static trust function to trust nothing. * * @private */ function trustNone () { return false } /** * Compile trust function for multiple subnets. * * @param {Array} subnets * @private */ function trustMulti (subnets) { return function trust (addr) { if (!isip(addr)) return false var ip = parseip(addr) var ipconv var kind = ip.kind() for (var i = 0; i < subnets.length; i++) { var subnet = subnets[i] var subnetip = subnet[0] var subnetkind = subnetip.kind() var subnetrange = subnet[1] var trusted = ip if (kind !== subnetkind) { if (subnetkind === 'ipv4' && !ip.isIPv4MappedAddress()) { // Incompatible IP addresses continue } if (!ipconv) { // Convert IP to match subnet IP kind ipconv = subnetkind === 'ipv4' ? ip.toIPv4Address() : ip.toIPv4MappedAddress() } trusted = ipconv } if (trusted.match(subnetip, subnetrange)) { return true } } return false } } /** * Compile trust function for single subnet. * * @param {Object} subnet * @private */ function trustSingle (subnet) { var subnetip = subnet[0] var subnetkind = subnetip.kind() var subnetisipv4 = subnetkind === 'ipv4' var subnetrange = subnet[1] return function trust (addr) { if (!isip(addr)) return false var ip = parseip(addr) var kind = ip.kind() if (kind !== subnetkind) { if (subnetisipv4 && !ip.isIPv4MappedAddress()) { // Incompatible IP addresses return false } // Convert IP to match subnet IP kind ip = subnetisipv4 ? ip.toIPv4Address() : ip.toIPv4MappedAddress() } return ip.match(subnetip, subnetrange) } } apollo-server-demo/node_modules/proxy-addr/LICENSE 0000644 0001750 0000144 00000002106 03560116604 021525 0 ustar andreh users (The MIT License) Copyright (c) 2014-2016 Douglas Christopher Wilson Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the 'Software'), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/proxy-addr/HISTORY.md 0000644 0001750 0000144 00000005465 03560116604 022216 0 ustar andreh users 2.0.6 / 2020-02-24 ================== * deps: ipaddr.js@1.9.1 2.0.5 / 2019-04-16 ================== * deps: ipaddr.js@1.9.0 2.0.4 / 2018-07-26 ================== * deps: ipaddr.js@1.8.0 2.0.3 / 2018-02-19 ================== * deps: ipaddr.js@1.6.0 2.0.2 / 2017-09-24 ================== * deps: forwarded@~0.1.2 - perf: improve header parsing - perf: reduce overhead when no `X-Forwarded-For` header 2.0.1 / 2017-09-10 ================== * deps: forwarded@~0.1.1 - Fix trimming leading / trailing OWS - perf: hoist regular expression * deps: ipaddr.js@1.5.2 2.0.0 / 2017-08-08 ================== * Drop support for Node.js below 0.10 1.1.5 / 2017-07-25 ================== * Fix array argument being altered * deps: ipaddr.js@1.4.0 1.1.4 / 2017-03-24 ================== * deps: ipaddr.js@1.3.0 1.1.3 / 2017-01-14 ================== * deps: ipaddr.js@1.2.0 1.1.2 / 2016-05-29 ================== * deps: ipaddr.js@1.1.1 - Fix IPv6-mapped IPv4 validation edge cases 1.1.1 / 2016-05-03 ================== * Fix regression matching mixed versions against multiple subnets 1.1.0 / 2016-05-01 ================== * Fix accepting various invalid netmasks - IPv4 netmasks must be contingous - IPv6 addresses cannot be used as a netmask * deps: ipaddr.js@1.1.0 1.0.10 / 2015-12-09 =================== * deps: ipaddr.js@1.0.5 - Fix regression in `isValid` with non-string arguments 1.0.9 / 2015-12-01 ================== * deps: ipaddr.js@1.0.4 - Fix accepting some invalid IPv6 addresses - Reject CIDRs with negative or overlong masks * perf: enable strict mode 1.0.8 / 2015-05-10 ================== * deps: ipaddr.js@1.0.1 1.0.7 / 2015-03-16 ================== * deps: ipaddr.js@0.1.9 - Fix OOM on certain inputs to `isValid` 1.0.6 / 2015-02-01 ================== * deps: ipaddr.js@0.1.8 1.0.5 / 2015-01-08 ================== * deps: ipaddr.js@0.1.6 1.0.4 / 2014-11-23 ================== * deps: ipaddr.js@0.1.5 - Fix edge cases with `isValid` 1.0.3 / 2014-09-21 ================== * Use `forwarded` npm module 1.0.2 / 2014-09-18 ================== * Fix a global leak when multiple subnets are trusted * Support Node.js 0.6 * deps: ipaddr.js@0.1.3 1.0.1 / 2014-06-03 ================== * Fix links in npm package 1.0.0 / 2014-05-08 ================== * Add `trust` argument to determine proxy trust on * Accepts custom function * Accepts IPv4/IPv6 address(es) * Accepts subnets * Accepts pre-defined names * Add optional `trust` argument to `proxyaddr.all` to stop at first untrusted * Add `proxyaddr.compile` to pre-compile `trust` function to make subsequent calls faster 0.0.1 / 2014-05-04 ================== * Fix bad npm publish 0.0.0 / 2014-05-04 ================== * Initial release apollo-server-demo/node_modules/proxy-addr/README.md 0000644 0001750 0000144 00000010460 03560116604 022001 0 ustar andreh users # proxy-addr [![NPM Version][npm-version-image]][npm-url] [![NPM Downloads][npm-downloads-image]][npm-url] [![Node.js Version][node-image]][node-url] [![Build Status][travis-image]][travis-url] [![Test Coverage][coveralls-image]][coveralls-url] Determine address of proxied request ## Install This is a [Node.js](https://nodejs.org/en/) module available through the [npm registry](https://www.npmjs.com/). Installation is done using the [`npm install` command](https://docs.npmjs.com/getting-started/installing-npm-packages-locally): ```sh $ npm install proxy-addr ``` ## API <!-- eslint-disable no-unused-vars --> ```js var proxyaddr = require('proxy-addr') ``` ### proxyaddr(req, trust) Return the address of the request, using the given `trust` parameter. The `trust` argument is a function that returns `true` if you trust the address, `false` if you don't. The closest untrusted address is returned. <!-- eslint-disable no-undef --> ```js proxyaddr(req, function (addr) { return addr === '127.0.0.1' }) proxyaddr(req, function (addr, i) { return i < 1 }) ``` The `trust` arugment may also be a single IP address string or an array of trusted addresses, as plain IP addresses, CIDR-formatted strings, or IP/netmask strings. <!-- eslint-disable no-undef --> ```js proxyaddr(req, '127.0.0.1') proxyaddr(req, ['127.0.0.0/8', '10.0.0.0/8']) proxyaddr(req, ['127.0.0.0/255.0.0.0', '192.168.0.0/255.255.0.0']) ``` This module also supports IPv6. Your IPv6 addresses will be normalized automatically (i.e. `fe80::00ed:1` equals `fe80:0:0:0:0:0:ed:1`). <!-- eslint-disable no-undef --> ```js proxyaddr(req, '::1') proxyaddr(req, ['::1/128', 'fe80::/10']) ``` This module will automatically work with IPv4-mapped IPv6 addresses as well to support node.js in IPv6-only mode. This means that you do not have to specify both `::ffff:a00:1` and `10.0.0.1`. As a convenience, this module also takes certain pre-defined names in addition to IP addresses, which expand into IP addresses: <!-- eslint-disable no-undef --> ```js proxyaddr(req, 'loopback') proxyaddr(req, ['loopback', 'fc00:ac:1ab5:fff::1/64']) ``` * `loopback`: IPv4 and IPv6 loopback addresses (like `::1` and `127.0.0.1`). * `linklocal`: IPv4 and IPv6 link-local addresses (like `fe80::1:1:1:1` and `169.254.0.1`). * `uniquelocal`: IPv4 private addresses and IPv6 unique-local addresses (like `fc00:ac:1ab5:fff::1` and `192.168.0.1`). When `trust` is specified as a function, it will be called for each address to determine if it is a trusted address. The function is given two arguments: `addr` and `i`, where `addr` is a string of the address to check and `i` is a number that represents the distance from the socket address. ### proxyaddr.all(req, [trust]) Return all the addresses of the request, optionally stopping at the first untrusted. This array is ordered from closest to furthest (i.e. `arr[0] === req.connection.remoteAddress`). <!-- eslint-disable no-undef --> ```js proxyaddr.all(req) ``` The optional `trust` argument takes the same arguments as `trust` does in `proxyaddr(req, trust)`. <!-- eslint-disable no-undef --> ```js proxyaddr.all(req, 'loopback') ``` ### proxyaddr.compile(val) Compiles argument `val` into a `trust` function. This function takes the same arguments as `trust` does in `proxyaddr(req, trust)` and returns a function suitable for `proxyaddr(req, trust)`. <!-- eslint-disable no-undef, no-unused-vars --> ```js var trust = proxyaddr.compile('loopback') var addr = proxyaddr(req, trust) ``` This function is meant to be optimized for use against every request. It is recommend to compile a trust function up-front for the trusted configuration and pass that to `proxyaddr(req, trust)` for each request. ## Testing ```sh $ npm test ``` ## Benchmarks ```sh $ npm run-script bench ``` ## License [MIT](LICENSE) [coveralls-image]: https://badgen.net/coveralls/c/github/jshttp/proxy-addr/master [coveralls-url]: https://coveralls.io/r/jshttp/proxy-addr?branch=master [node-image]: https://badgen.net/npm/node/proxy-addr [node-url]: https://nodejs.org/en/download [npm-downloads-image]: https://badgen.net/npm/dm/proxy-addr [npm-url]: https://npmjs.org/package/proxy-addr [npm-version-image]: https://badgen.net/npm/v/proxy-addr [travis-image]: https://badgen.net/travis/jshttp/proxy-addr/master [travis-url]: https://travis-ci.org/jshttp/proxy-addr apollo-server-demo/node_modules/proxy-addr/package.json 0000644 0001750 0000144 00000002614 03560116604 023012 0 ustar andreh users { "name": "proxy-addr", "description": "Determine address of proxied request", "version": "2.0.6", "author": "Douglas Christopher Wilson <doug@somethingdoug.com>", "license": "MIT", "keywords": [ "ip", "proxy", "x-forwarded-for" ], "repository": "jshttp/proxy-addr", "dependencies": { "forwarded": "~0.1.2", "ipaddr.js": "1.9.1" }, "devDependencies": { "benchmark": "2.1.4", "beautify-benchmark": "0.2.4", "deep-equal": "1.0.1", "eslint": "6.8.0", "eslint-config-standard": "14.1.0", "eslint-plugin-import": "2.20.1", "eslint-plugin-markdown": "1.0.1", "eslint-plugin-node": "11.0.0", "eslint-plugin-promise": "4.2.1", "eslint-plugin-standard": "4.0.1", "mocha": "7.0.1", "nyc": "15.0.0" }, "files": [ "LICENSE", "HISTORY.md", "README.md", "index.js" ], "engines": { "node": ">= 0.10" }, "scripts": { "bench": "node benchmark/index.js", "lint": "eslint --plugin markdown --ext js,md .", "test": "mocha --reporter spec --bail --check-leaks test/", "test-cov": "nyc --reporter=text npm test", "test-travis": "nyc --reporter=html --reporter=text npm test" } ,"_resolved": "https://registry.npmjs.org/proxy-addr/-/proxy-addr-2.0.6.tgz" ,"_integrity": "sha512-dh/frvCBVmSsDYzw6n926jv974gddhkFPfiN8hPOi30Wax25QZyZEGveluCgliBnqmuM+UJmBErbAUFIoDbjOw==" ,"_from": "proxy-addr@2.0.6" } apollo-server-demo/node_modules/eventemitter3/ 0000755 0001750 0000144 00000000000 14067647700 021240 5 ustar andreh users apollo-server-demo/node_modules/eventemitter3/index.js 0000644 0001750 0000144 00000021665 03560116604 022705 0 ustar andreh users 'use strict'; var has = Object.prototype.hasOwnProperty , prefix = '~'; /** * Constructor to create a storage for our `EE` objects. * An `Events` instance is a plain object whose properties are event names. * * @constructor * @private */ function Events() {} // // We try to not inherit from `Object.prototype`. In some engines creating an // instance in this way is faster than calling `Object.create(null)` directly. // If `Object.create(null)` is not supported we prefix the event names with a // character to make sure that the built-in object properties are not // overridden or used as an attack vector. // if (Object.create) { Events.prototype = Object.create(null); // // This hack is needed because the `__proto__` property is still inherited in // some old browsers like Android 4, iPhone 5.1, Opera 11 and Safari 5. // if (!new Events().__proto__) prefix = false; } /** * Representation of a single event listener. * * @param {Function} fn The listener function. * @param {*} context The context to invoke the listener with. * @param {Boolean} [once=false] Specify if the listener is a one-time listener. * @constructor * @private */ function EE(fn, context, once) { this.fn = fn; this.context = context; this.once = once || false; } /** * Add a listener for a given event. * * @param {EventEmitter} emitter Reference to the `EventEmitter` instance. * @param {(String|Symbol)} event The event name. * @param {Function} fn The listener function. * @param {*} context The context to invoke the listener with. * @param {Boolean} once Specify if the listener is a one-time listener. * @returns {EventEmitter} * @private */ function addListener(emitter, event, fn, context, once) { if (typeof fn !== 'function') { throw new TypeError('The listener must be a function'); } var listener = new EE(fn, context || emitter, once) , evt = prefix ? prefix + event : event; if (!emitter._events[evt]) emitter._events[evt] = listener, emitter._eventsCount++; else if (!emitter._events[evt].fn) emitter._events[evt].push(listener); else emitter._events[evt] = [emitter._events[evt], listener]; return emitter; } /** * Clear event by name. * * @param {EventEmitter} emitter Reference to the `EventEmitter` instance. * @param {(String|Symbol)} evt The Event name. * @private */ function clearEvent(emitter, evt) { if (--emitter._eventsCount === 0) emitter._events = new Events(); else delete emitter._events[evt]; } /** * Minimal `EventEmitter` interface that is molded against the Node.js * `EventEmitter` interface. * * @constructor * @public */ function EventEmitter() { this._events = new Events(); this._eventsCount = 0; } /** * Return an array listing the events for which the emitter has registered * listeners. * * @returns {Array} * @public */ EventEmitter.prototype.eventNames = function eventNames() { var names = [] , events , name; if (this._eventsCount === 0) return names; for (name in (events = this._events)) { if (has.call(events, name)) names.push(prefix ? name.slice(1) : name); } if (Object.getOwnPropertySymbols) { return names.concat(Object.getOwnPropertySymbols(events)); } return names; }; /** * Return the listeners registered for a given event. * * @param {(String|Symbol)} event The event name. * @returns {Array} The registered listeners. * @public */ EventEmitter.prototype.listeners = function listeners(event) { var evt = prefix ? prefix + event : event , handlers = this._events[evt]; if (!handlers) return []; if (handlers.fn) return [handlers.fn]; for (var i = 0, l = handlers.length, ee = new Array(l); i < l; i++) { ee[i] = handlers[i].fn; } return ee; }; /** * Return the number of listeners listening to a given event. * * @param {(String|Symbol)} event The event name. * @returns {Number} The number of listeners. * @public */ EventEmitter.prototype.listenerCount = function listenerCount(event) { var evt = prefix ? prefix + event : event , listeners = this._events[evt]; if (!listeners) return 0; if (listeners.fn) return 1; return listeners.length; }; /** * Calls each of the listeners registered for a given event. * * @param {(String|Symbol)} event The event name. * @returns {Boolean} `true` if the event had listeners, else `false`. * @public */ EventEmitter.prototype.emit = function emit(event, a1, a2, a3, a4, a5) { var evt = prefix ? prefix + event : event; if (!this._events[evt]) return false; var listeners = this._events[evt] , len = arguments.length , args , i; if (listeners.fn) { if (listeners.once) this.removeListener(event, listeners.fn, undefined, true); switch (len) { case 1: return listeners.fn.call(listeners.context), true; case 2: return listeners.fn.call(listeners.context, a1), true; case 3: return listeners.fn.call(listeners.context, a1, a2), true; case 4: return listeners.fn.call(listeners.context, a1, a2, a3), true; case 5: return listeners.fn.call(listeners.context, a1, a2, a3, a4), true; case 6: return listeners.fn.call(listeners.context, a1, a2, a3, a4, a5), true; } for (i = 1, args = new Array(len -1); i < len; i++) { args[i - 1] = arguments[i]; } listeners.fn.apply(listeners.context, args); } else { var length = listeners.length , j; for (i = 0; i < length; i++) { if (listeners[i].once) this.removeListener(event, listeners[i].fn, undefined, true); switch (len) { case 1: listeners[i].fn.call(listeners[i].context); break; case 2: listeners[i].fn.call(listeners[i].context, a1); break; case 3: listeners[i].fn.call(listeners[i].context, a1, a2); break; case 4: listeners[i].fn.call(listeners[i].context, a1, a2, a3); break; default: if (!args) for (j = 1, args = new Array(len -1); j < len; j++) { args[j - 1] = arguments[j]; } listeners[i].fn.apply(listeners[i].context, args); } } } return true; }; /** * Add a listener for a given event. * * @param {(String|Symbol)} event The event name. * @param {Function} fn The listener function. * @param {*} [context=this] The context to invoke the listener with. * @returns {EventEmitter} `this`. * @public */ EventEmitter.prototype.on = function on(event, fn, context) { return addListener(this, event, fn, context, false); }; /** * Add a one-time listener for a given event. * * @param {(String|Symbol)} event The event name. * @param {Function} fn The listener function. * @param {*} [context=this] The context to invoke the listener with. * @returns {EventEmitter} `this`. * @public */ EventEmitter.prototype.once = function once(event, fn, context) { return addListener(this, event, fn, context, true); }; /** * Remove the listeners of a given event. * * @param {(String|Symbol)} event The event name. * @param {Function} fn Only remove the listeners that match this function. * @param {*} context Only remove the listeners that have this context. * @param {Boolean} once Only remove one-time listeners. * @returns {EventEmitter} `this`. * @public */ EventEmitter.prototype.removeListener = function removeListener(event, fn, context, once) { var evt = prefix ? prefix + event : event; if (!this._events[evt]) return this; if (!fn) { clearEvent(this, evt); return this; } var listeners = this._events[evt]; if (listeners.fn) { if ( listeners.fn === fn && (!once || listeners.once) && (!context || listeners.context === context) ) { clearEvent(this, evt); } } else { for (var i = 0, events = [], length = listeners.length; i < length; i++) { if ( listeners[i].fn !== fn || (once && !listeners[i].once) || (context && listeners[i].context !== context) ) { events.push(listeners[i]); } } // // Reset the array, or remove it completely if we have no more listeners. // if (events.length) this._events[evt] = events.length === 1 ? events[0] : events; else clearEvent(this, evt); } return this; }; /** * Remove all listeners, or those of the specified event. * * @param {(String|Symbol)} [event] The event name. * @returns {EventEmitter} `this`. * @public */ EventEmitter.prototype.removeAllListeners = function removeAllListeners(event) { var evt; if (event) { evt = prefix ? prefix + event : event; if (this._events[evt]) clearEvent(this, evt); } else { this._events = new Events(); this._eventsCount = 0; } return this; }; // // Alias methods names because people roll like that. // EventEmitter.prototype.off = EventEmitter.prototype.removeListener; EventEmitter.prototype.addListener = EventEmitter.prototype.on; // // Expose the prefix. // EventEmitter.prefixed = prefix; // // Allow `EventEmitter` to be imported as module namespace. // EventEmitter.EventEmitter = EventEmitter; // // Expose the module. // if ('undefined' !== typeof module) { module.exports = EventEmitter; } apollo-server-demo/node_modules/eventemitter3/umd/ 0000755 0001750 0000144 00000000000 14067647700 022025 5 ustar andreh users apollo-server-demo/node_modules/eventemitter3/umd/eventemitter3.min.js.map 0000644 0001750 0000144 00000013017 03560116604 026507 0 ustar andreh users {"version":3,"sources":["umd/eventemitter3.js"],"names":["f","exports","module","define","amd","window","global","self","this","EventEmitter3","r","e","n","t","o","i","c","require","u","a","Error","code","p","call","length","1","has","Object","prototype","hasOwnProperty","prefix","Events","EE","fn","context","once","addListener","emitter","event","TypeError","listener","evt","_events","push","_eventsCount","clearEvent","EventEmitter","create","__proto__","eventNames","events","name","names","slice","getOwnPropertySymbols","concat","listeners","handlers","l","ee","Array","listenerCount","emit","a1","a2","a3","a4","a5","args","len","arguments","removeListener","undefined","apply","j","on","removeAllListeners","off","prefixed"],"mappings":"CAAA,SAAUA,GAAG,GAAoB,iBAAVC,SAAoC,oBAATC,OAAsBA,OAAOD,QAAQD,SAAS,GAAmB,mBAATG,QAAqBA,OAAOC,IAAKD,OAAO,GAAGH,OAAO,EAA0B,oBAATK,OAAwBA,OAA+B,oBAATC,OAAwBA,OAA6B,oBAAPC,KAAsBA,KAAYC,MAAOC,cAAgBT,KAAlU,CAAyU,WAAqC,OAAmB,SAASU,EAAEC,EAAEC,EAAEC,GAAG,SAASC,EAAEC,EAAEf,GAAG,IAAIY,EAAEG,GAAG,CAAC,IAAIJ,EAAEI,GAAG,CAAC,IAAIC,EAAE,mBAAmBC,SAASA,QAAQ,IAAIjB,GAAGgB,EAAE,OAAOA,EAAED,GAAE,GAAI,GAAGG,EAAE,OAAOA,EAAEH,GAAE,GAAI,IAAII,EAAE,IAAIC,MAAM,uBAAuBL,EAAE,KAAK,MAAMI,EAAEE,KAAK,mBAAmBF,EAAE,IAAIG,EAAEV,EAAEG,GAAG,CAACd,QAAQ,IAAIU,EAAEI,GAAG,GAAGQ,KAAKD,EAAErB,QAAQ,SAASS,GAAoB,OAAOI,EAAlBH,EAAEI,GAAG,GAAGL,IAAeA,IAAIY,EAAEA,EAAErB,QAAQS,EAAEC,EAAEC,EAAEC,GAAG,OAAOD,EAAEG,GAAGd,QAAQ,IAAI,IAAIiB,EAAE,mBAAmBD,SAASA,QAAQF,EAAE,EAAEA,EAAEF,EAAEW,OAAOT,IAAID,EAAED,EAAEE,IAAI,OAAOD,EAA7b,CAA4c,CAACW,EAAE,CAAC,SAASR,EAAQf,EAAOD,GAC71B,aAEA,IAAIyB,EAAMC,OAAOC,UAAUC,eACvBC,EAAS,IASb,SAASC,KA4BT,SAASC,EAAGC,EAAIC,EAASC,GACvB3B,KAAKyB,GAAKA,EACVzB,KAAK0B,QAAUA,EACf1B,KAAK2B,KAAOA,IAAQ,EActB,SAASC,EAAYC,EAASC,EAAOL,EAAIC,EAASC,GAChD,GAAkB,mBAAPF,EACT,MAAM,IAAIM,UAAU,mCAGtB,IAAIC,EAAW,IAAIR,EAAGC,EAAIC,GAAWG,EAASF,GAC1CM,EAAMX,EAASA,EAASQ,EAAQA,EAMpC,OAJKD,EAAQK,QAAQD,GACXJ,EAAQK,QAAQD,GAAKR,GAC1BI,EAAQK,QAAQD,GAAO,CAACJ,EAAQK,QAAQD,GAAMD,GADhBH,EAAQK,QAAQD,GAAKE,KAAKH,IADlCH,EAAQK,QAAQD,GAAOD,EAAUH,EAAQO,gBAI7DP,EAUT,SAASQ,EAAWR,EAASI,GACI,KAAzBJ,EAAQO,aAAoBP,EAAQK,QAAU,IAAIX,SAC5CM,EAAQK,QAAQD,GAU9B,SAASK,IACPtC,KAAKkC,QAAU,IAAIX,EACnBvB,KAAKoC,aAAe,EAxElBjB,OAAOoB,SACThB,EAAOH,UAAYD,OAAOoB,OAAO,OAM5B,IAAIhB,GAASiB,YAAWlB,GAAS,IA2ExCgB,EAAalB,UAAUqB,WAAa,WAClC,IACIC,EACAC,EAFAC,EAAQ,GAIZ,GAA0B,IAAtB5C,KAAKoC,aAAoB,OAAOQ,EAEpC,IAAKD,KAASD,EAAS1C,KAAKkC,QACtBhB,EAAIH,KAAK2B,EAAQC,IAAOC,EAAMT,KAAKb,EAASqB,EAAKE,MAAM,GAAKF,GAGlE,OAAIxB,OAAO2B,sBACFF,EAAMG,OAAO5B,OAAO2B,sBAAsBJ,IAG5CE,GAUTN,EAAalB,UAAU4B,UAAY,SAAmBlB,GACpD,IAAIG,EAAMX,EAASA,EAASQ,EAAQA,EAChCmB,EAAWjD,KAAKkC,QAAQD,GAE5B,IAAKgB,EAAU,MAAO,GACtB,GAAIA,EAASxB,GAAI,MAAO,CAACwB,EAASxB,IAElC,IAAK,IAAIlB,EAAI,EAAG2C,EAAID,EAASjC,OAAQmC,EAAK,IAAIC,MAAMF,GAAI3C,EAAI2C,EAAG3C,IAC7D4C,EAAG5C,GAAK0C,EAAS1C,GAAGkB,GAGtB,OAAO0B,GAUTb,EAAalB,UAAUiC,cAAgB,SAAuBvB,GAC5D,IAAIG,EAAMX,EAASA,EAASQ,EAAQA,EAChCkB,EAAYhD,KAAKkC,QAAQD,GAE7B,OAAKe,EACDA,EAAUvB,GAAW,EAClBuB,EAAUhC,OAFM,GAYzBsB,EAAalB,UAAUkC,KAAO,SAAcxB,EAAOyB,EAAIC,EAAIC,EAAIC,EAAIC,GACjE,IAAI1B,EAAMX,EAASA,EAASQ,EAAQA,EAEpC,IAAK9B,KAAKkC,QAAQD,GAAM,OAAO,EAE/B,IAEI2B,EACArD,EAHAyC,EAAYhD,KAAKkC,QAAQD,GACzB4B,EAAMC,UAAU9C,OAIpB,GAAIgC,EAAUvB,GAAI,CAGhB,OAFIuB,EAAUrB,MAAM3B,KAAK+D,eAAejC,EAAOkB,EAAUvB,QAAIuC,GAAW,GAEhEH,GACN,KAAK,EAAG,OAAOb,EAAUvB,GAAGV,KAAKiC,EAAUtB,UAAU,EACrD,KAAK,EAAG,OAAOsB,EAAUvB,GAAGV,KAAKiC,EAAUtB,QAAS6B,IAAK,EACzD,KAAK,EAAG,OAAOP,EAAUvB,GAAGV,KAAKiC,EAAUtB,QAAS6B,EAAIC,IAAK,EAC7D,KAAK,EAAG,OAAOR,EAAUvB,GAAGV,KAAKiC,EAAUtB,QAAS6B,EAAIC,EAAIC,IAAK,EACjE,KAAK,EAAG,OAAOT,EAAUvB,GAAGV,KAAKiC,EAAUtB,QAAS6B,EAAIC,EAAIC,EAAIC,IAAK,EACrE,KAAK,EAAG,OAAOV,EAAUvB,GAAGV,KAAKiC,EAAUtB,QAAS6B,EAAIC,EAAIC,EAAIC,EAAIC,IAAK,EAG3E,IAAKpD,EAAI,EAAGqD,EAAO,IAAIR,MAAMS,EAAK,GAAItD,EAAIsD,EAAKtD,IAC7CqD,EAAKrD,EAAI,GAAKuD,UAAUvD,GAG1ByC,EAAUvB,GAAGwC,MAAMjB,EAAUtB,QAASkC,OACjC,CACL,IACIM,EADAlD,EAASgC,EAAUhC,OAGvB,IAAKT,EAAI,EAAGA,EAAIS,EAAQT,IAGtB,OAFIyC,EAAUzC,GAAGoB,MAAM3B,KAAK+D,eAAejC,EAAOkB,EAAUzC,GAAGkB,QAAIuC,GAAW,GAEtEH,GACN,KAAK,EAAGb,EAAUzC,GAAGkB,GAAGV,KAAKiC,EAAUzC,GAAGmB,SAAU,MACpD,KAAK,EAAGsB,EAAUzC,GAAGkB,GAAGV,KAAKiC,EAAUzC,GAAGmB,QAAS6B,GAAK,MACxD,KAAK,EAAGP,EAAUzC,GAAGkB,GAAGV,KAAKiC,EAAUzC,GAAGmB,QAAS6B,EAAIC,GAAK,MAC5D,KAAK,EAAGR,EAAUzC,GAAGkB,GAAGV,KAAKiC,EAAUzC,GAAGmB,QAAS6B,EAAIC,EAAIC,GAAK,MAChE,QACE,IAAKG,EAAM,IAAKM,EAAI,EAAGN,EAAO,IAAIR,MAAMS,EAAK,GAAIK,EAAIL,EAAKK,IACxDN,EAAKM,EAAI,GAAKJ,UAAUI,GAG1BlB,EAAUzC,GAAGkB,GAAGwC,MAAMjB,EAAUzC,GAAGmB,QAASkC,IAKpD,OAAO,GAYTtB,EAAalB,UAAU+C,GAAK,SAAYrC,EAAOL,EAAIC,GACjD,OAAOE,EAAY5B,KAAM8B,EAAOL,EAAIC,GAAS,IAY/CY,EAAalB,UAAUO,KAAO,SAAcG,EAAOL,EAAIC,GACrD,OAAOE,EAAY5B,KAAM8B,EAAOL,EAAIC,GAAS,IAa/CY,EAAalB,UAAU2C,eAAiB,SAAwBjC,EAAOL,EAAIC,EAASC,GAClF,IAAIM,EAAMX,EAASA,EAASQ,EAAQA,EAEpC,IAAK9B,KAAKkC,QAAQD,GAAM,OAAOjC,KAC/B,IAAKyB,EAEH,OADAY,EAAWrC,KAAMiC,GACVjC,KAGT,IAAIgD,EAAYhD,KAAKkC,QAAQD,GAE7B,GAAIe,EAAUvB,GAEVuB,EAAUvB,KAAOA,GACfE,IAAQqB,EAAUrB,MAClBD,GAAWsB,EAAUtB,UAAYA,GAEnCW,EAAWrC,KAAMiC,OAEd,CACL,IAAK,IAAI1B,EAAI,EAAGmC,EAAS,GAAI1B,EAASgC,EAAUhC,OAAQT,EAAIS,EAAQT,KAEhEyC,EAAUzC,GAAGkB,KAAOA,GACnBE,IAASqB,EAAUzC,GAAGoB,MACtBD,GAAWsB,EAAUzC,GAAGmB,UAAYA,IAErCgB,EAAOP,KAAKa,EAAUzC,IAOtBmC,EAAO1B,OAAQhB,KAAKkC,QAAQD,GAAyB,IAAlBS,EAAO1B,OAAe0B,EAAO,GAAKA,EACpEL,EAAWrC,KAAMiC,GAGxB,OAAOjC,MAUTsC,EAAalB,UAAUgD,mBAAqB,SAA4BtC,GACtE,IAAIG,EAUJ,OARIH,GACFG,EAAMX,EAASA,EAASQ,EAAQA,EAC5B9B,KAAKkC,QAAQD,IAAMI,EAAWrC,KAAMiC,KAExCjC,KAAKkC,QAAU,IAAIX,EACnBvB,KAAKoC,aAAe,GAGfpC,MAMTsC,EAAalB,UAAUiD,IAAM/B,EAAalB,UAAU2C,eACpDzB,EAAalB,UAAUQ,YAAcU,EAAalB,UAAU+C,GAK5D7B,EAAagC,SAAWhD,EAKxBgB,EAAaA,aAAeA,OAKxB,IAAuB5C,IACzBA,EAAOD,QAAU6C,IAGjB,KAAK,GAAG,CAAC,GAlV0W,CAkVtW"} apollo-server-demo/node_modules/eventemitter3/umd/eventemitter3.js 0000644 0001750 0000144 00000023463 03560116604 025157 0 ustar andreh users (function(f){if(typeof exports==="object"&&typeof module!=="undefined"){module.exports=f()}else if(typeof define==="function"&&define.amd){define([],f)}else{var g;if(typeof window!=="undefined"){g=window}else if(typeof global!=="undefined"){g=global}else if(typeof self!=="undefined"){g=self}else{g=this}g.EventEmitter3 = f()}})(function(){var define,module,exports;return (function(){function r(e,n,t){function o(i,f){if(!n[i]){if(!e[i]){var c="function"==typeof require&&require;if(!f&&c)return c(i,!0);if(u)return u(i,!0);var a=new Error("Cannot find module '"+i+"'");throw a.code="MODULE_NOT_FOUND",a}var p=n[i]={exports:{}};e[i][0].call(p.exports,function(r){var n=e[i][1][r];return o(n||r)},p,p.exports,r,e,n,t)}return n[i].exports}for(var u="function"==typeof require&&require,i=0;i<t.length;i++)o(t[i]);return o}return r})()({1:[function(require,module,exports){ 'use strict'; var has = Object.prototype.hasOwnProperty , prefix = '~'; /** * Constructor to create a storage for our `EE` objects. * An `Events` instance is a plain object whose properties are event names. * * @constructor * @private */ function Events() {} // // We try to not inherit from `Object.prototype`. In some engines creating an // instance in this way is faster than calling `Object.create(null)` directly. // If `Object.create(null)` is not supported we prefix the event names with a // character to make sure that the built-in object properties are not // overridden or used as an attack vector. // if (Object.create) { Events.prototype = Object.create(null); // // This hack is needed because the `__proto__` property is still inherited in // some old browsers like Android 4, iPhone 5.1, Opera 11 and Safari 5. // if (!new Events().__proto__) prefix = false; } /** * Representation of a single event listener. * * @param {Function} fn The listener function. * @param {*} context The context to invoke the listener with. * @param {Boolean} [once=false] Specify if the listener is a one-time listener. * @constructor * @private */ function EE(fn, context, once) { this.fn = fn; this.context = context; this.once = once || false; } /** * Add a listener for a given event. * * @param {EventEmitter} emitter Reference to the `EventEmitter` instance. * @param {(String|Symbol)} event The event name. * @param {Function} fn The listener function. * @param {*} context The context to invoke the listener with. * @param {Boolean} once Specify if the listener is a one-time listener. * @returns {EventEmitter} * @private */ function addListener(emitter, event, fn, context, once) { if (typeof fn !== 'function') { throw new TypeError('The listener must be a function'); } var listener = new EE(fn, context || emitter, once) , evt = prefix ? prefix + event : event; if (!emitter._events[evt]) emitter._events[evt] = listener, emitter._eventsCount++; else if (!emitter._events[evt].fn) emitter._events[evt].push(listener); else emitter._events[evt] = [emitter._events[evt], listener]; return emitter; } /** * Clear event by name. * * @param {EventEmitter} emitter Reference to the `EventEmitter` instance. * @param {(String|Symbol)} evt The Event name. * @private */ function clearEvent(emitter, evt) { if (--emitter._eventsCount === 0) emitter._events = new Events(); else delete emitter._events[evt]; } /** * Minimal `EventEmitter` interface that is molded against the Node.js * `EventEmitter` interface. * * @constructor * @public */ function EventEmitter() { this._events = new Events(); this._eventsCount = 0; } /** * Return an array listing the events for which the emitter has registered * listeners. * * @returns {Array} * @public */ EventEmitter.prototype.eventNames = function eventNames() { var names = [] , events , name; if (this._eventsCount === 0) return names; for (name in (events = this._events)) { if (has.call(events, name)) names.push(prefix ? name.slice(1) : name); } if (Object.getOwnPropertySymbols) { return names.concat(Object.getOwnPropertySymbols(events)); } return names; }; /** * Return the listeners registered for a given event. * * @param {(String|Symbol)} event The event name. * @returns {Array} The registered listeners. * @public */ EventEmitter.prototype.listeners = function listeners(event) { var evt = prefix ? prefix + event : event , handlers = this._events[evt]; if (!handlers) return []; if (handlers.fn) return [handlers.fn]; for (var i = 0, l = handlers.length, ee = new Array(l); i < l; i++) { ee[i] = handlers[i].fn; } return ee; }; /** * Return the number of listeners listening to a given event. * * @param {(String|Symbol)} event The event name. * @returns {Number} The number of listeners. * @public */ EventEmitter.prototype.listenerCount = function listenerCount(event) { var evt = prefix ? prefix + event : event , listeners = this._events[evt]; if (!listeners) return 0; if (listeners.fn) return 1; return listeners.length; }; /** * Calls each of the listeners registered for a given event. * * @param {(String|Symbol)} event The event name. * @returns {Boolean} `true` if the event had listeners, else `false`. * @public */ EventEmitter.prototype.emit = function emit(event, a1, a2, a3, a4, a5) { var evt = prefix ? prefix + event : event; if (!this._events[evt]) return false; var listeners = this._events[evt] , len = arguments.length , args , i; if (listeners.fn) { if (listeners.once) this.removeListener(event, listeners.fn, undefined, true); switch (len) { case 1: return listeners.fn.call(listeners.context), true; case 2: return listeners.fn.call(listeners.context, a1), true; case 3: return listeners.fn.call(listeners.context, a1, a2), true; case 4: return listeners.fn.call(listeners.context, a1, a2, a3), true; case 5: return listeners.fn.call(listeners.context, a1, a2, a3, a4), true; case 6: return listeners.fn.call(listeners.context, a1, a2, a3, a4, a5), true; } for (i = 1, args = new Array(len -1); i < len; i++) { args[i - 1] = arguments[i]; } listeners.fn.apply(listeners.context, args); } else { var length = listeners.length , j; for (i = 0; i < length; i++) { if (listeners[i].once) this.removeListener(event, listeners[i].fn, undefined, true); switch (len) { case 1: listeners[i].fn.call(listeners[i].context); break; case 2: listeners[i].fn.call(listeners[i].context, a1); break; case 3: listeners[i].fn.call(listeners[i].context, a1, a2); break; case 4: listeners[i].fn.call(listeners[i].context, a1, a2, a3); break; default: if (!args) for (j = 1, args = new Array(len -1); j < len; j++) { args[j - 1] = arguments[j]; } listeners[i].fn.apply(listeners[i].context, args); } } } return true; }; /** * Add a listener for a given event. * * @param {(String|Symbol)} event The event name. * @param {Function} fn The listener function. * @param {*} [context=this] The context to invoke the listener with. * @returns {EventEmitter} `this`. * @public */ EventEmitter.prototype.on = function on(event, fn, context) { return addListener(this, event, fn, context, false); }; /** * Add a one-time listener for a given event. * * @param {(String|Symbol)} event The event name. * @param {Function} fn The listener function. * @param {*} [context=this] The context to invoke the listener with. * @returns {EventEmitter} `this`. * @public */ EventEmitter.prototype.once = function once(event, fn, context) { return addListener(this, event, fn, context, true); }; /** * Remove the listeners of a given event. * * @param {(String|Symbol)} event The event name. * @param {Function} fn Only remove the listeners that match this function. * @param {*} context Only remove the listeners that have this context. * @param {Boolean} once Only remove one-time listeners. * @returns {EventEmitter} `this`. * @public */ EventEmitter.prototype.removeListener = function removeListener(event, fn, context, once) { var evt = prefix ? prefix + event : event; if (!this._events[evt]) return this; if (!fn) { clearEvent(this, evt); return this; } var listeners = this._events[evt]; if (listeners.fn) { if ( listeners.fn === fn && (!once || listeners.once) && (!context || listeners.context === context) ) { clearEvent(this, evt); } } else { for (var i = 0, events = [], length = listeners.length; i < length; i++) { if ( listeners[i].fn !== fn || (once && !listeners[i].once) || (context && listeners[i].context !== context) ) { events.push(listeners[i]); } } // // Reset the array, or remove it completely if we have no more listeners. // if (events.length) this._events[evt] = events.length === 1 ? events[0] : events; else clearEvent(this, evt); } return this; }; /** * Remove all listeners, or those of the specified event. * * @param {(String|Symbol)} [event] The event name. * @returns {EventEmitter} `this`. * @public */ EventEmitter.prototype.removeAllListeners = function removeAllListeners(event) { var evt; if (event) { evt = prefix ? prefix + event : event; if (this._events[evt]) clearEvent(this, evt); } else { this._events = new Events(); this._eventsCount = 0; } return this; }; // // Alias methods names because people roll like that. // EventEmitter.prototype.off = EventEmitter.prototype.removeListener; EventEmitter.prototype.addListener = EventEmitter.prototype.on; // // Expose the prefix. // EventEmitter.prefixed = prefix; // // Allow `EventEmitter` to be imported as module namespace. // EventEmitter.EventEmitter = EventEmitter; // // Expose the module. // if ('undefined' !== typeof module) { module.exports = EventEmitter; } },{}]},{},[1])(1) }); apollo-server-demo/node_modules/eventemitter3/umd/eventemitter3.min.js 0000644 0001750 0000144 00000006671 03560116604 025743 0 ustar andreh users !function(e){if("object"==typeof exports&&"undefined"!=typeof module)module.exports=e();else if("function"==typeof define&&define.amd)define([],e);else{("undefined"!=typeof window?window:"undefined"!=typeof global?global:"undefined"!=typeof self?self:this).EventEmitter3=e()}}(function(){return function i(s,f,c){function u(t,e){if(!f[t]){if(!s[t]){var n="function"==typeof require&&require;if(!e&&n)return n(t,!0);if(a)return a(t,!0);var r=new Error("Cannot find module '"+t+"'");throw r.code="MODULE_NOT_FOUND",r}var o=f[t]={exports:{}};s[t][0].call(o.exports,function(e){return u(s[t][1][e]||e)},o,o.exports,i,s,f,c)}return f[t].exports}for(var a="function"==typeof require&&require,e=0;e<c.length;e++)u(c[e]);return u}({1:[function(e,t,n){"use strict";var r=Object.prototype.hasOwnProperty,v="~";function o(){}function f(e,t,n){this.fn=e,this.context=t,this.once=n||!1}function i(e,t,n,r,o){if("function"!=typeof n)throw new TypeError("The listener must be a function");var i=new f(n,r||e,o),s=v?v+t:t;return e._events[s]?e._events[s].fn?e._events[s]=[e._events[s],i]:e._events[s].push(i):(e._events[s]=i,e._eventsCount++),e}function u(e,t){0==--e._eventsCount?e._events=new o:delete e._events[t]}function s(){this._events=new o,this._eventsCount=0}Object.create&&(o.prototype=Object.create(null),(new o).__proto__||(v=!1)),s.prototype.eventNames=function(){var e,t,n=[];if(0===this._eventsCount)return n;for(t in e=this._events)r.call(e,t)&&n.push(v?t.slice(1):t);return Object.getOwnPropertySymbols?n.concat(Object.getOwnPropertySymbols(e)):n},s.prototype.listeners=function(e){var t=v?v+e:e,n=this._events[t];if(!n)return[];if(n.fn)return[n.fn];for(var r=0,o=n.length,i=new Array(o);r<o;r++)i[r]=n[r].fn;return i},s.prototype.listenerCount=function(e){var t=v?v+e:e,n=this._events[t];return n?n.fn?1:n.length:0},s.prototype.emit=function(e,t,n,r,o,i){var s=v?v+e:e;if(!this._events[s])return!1;var f,c,u=this._events[s],a=arguments.length;if(u.fn){switch(u.once&&this.removeListener(e,u.fn,void 0,!0),a){case 1:return u.fn.call(u.context),!0;case 2:return u.fn.call(u.context,t),!0;case 3:return u.fn.call(u.context,t,n),!0;case 4:return u.fn.call(u.context,t,n,r),!0;case 5:return u.fn.call(u.context,t,n,r,o),!0;case 6:return u.fn.call(u.context,t,n,r,o,i),!0}for(c=1,f=new Array(a-1);c<a;c++)f[c-1]=arguments[c];u.fn.apply(u.context,f)}else{var l,p=u.length;for(c=0;c<p;c++)switch(u[c].once&&this.removeListener(e,u[c].fn,void 0,!0),a){case 1:u[c].fn.call(u[c].context);break;case 2:u[c].fn.call(u[c].context,t);break;case 3:u[c].fn.call(u[c].context,t,n);break;case 4:u[c].fn.call(u[c].context,t,n,r);break;default:if(!f)for(l=1,f=new Array(a-1);l<a;l++)f[l-1]=arguments[l];u[c].fn.apply(u[c].context,f)}}return!0},s.prototype.on=function(e,t,n){return i(this,e,t,n,!1)},s.prototype.once=function(e,t,n){return i(this,e,t,n,!0)},s.prototype.removeListener=function(e,t,n,r){var o=v?v+e:e;if(!this._events[o])return this;if(!t)return u(this,o),this;var i=this._events[o];if(i.fn)i.fn!==t||r&&!i.once||n&&i.context!==n||u(this,o);else{for(var s=0,f=[],c=i.length;s<c;s++)(i[s].fn!==t||r&&!i[s].once||n&&i[s].context!==n)&&f.push(i[s]);f.length?this._events[o]=1===f.length?f[0]:f:u(this,o)}return this},s.prototype.removeAllListeners=function(e){var t;return e?(t=v?v+e:e,this._events[t]&&u(this,t)):(this._events=new o,this._eventsCount=0),this},s.prototype.off=s.prototype.removeListener,s.prototype.addListener=s.prototype.on,s.prefixed=v,s.EventEmitter=s,void 0!==t&&(t.exports=s)},{}]},{},[1])(1)}); apollo-server-demo/node_modules/eventemitter3/LICENSE 0000644 0001750 0000144 00000002072 03560116604 022234 0 ustar andreh users The MIT License (MIT) Copyright (c) 2014 Arnout Kazemier Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/eventemitter3/index.d.ts 0000644 0001750 0000144 00000003405 03560116604 023131 0 ustar andreh users /** * Minimal `EventEmitter` interface that is molded against the Node.js * `EventEmitter` interface. */ declare class EventEmitter<EventTypes extends string | symbol = string | symbol> { static prefixed: string | boolean; /** * Return an array listing the events for which the emitter has registered * listeners. */ eventNames(): Array<EventTypes>; /** * Return the listeners registered for a given event. */ listeners(event: EventTypes): Array<EventEmitter.ListenerFn>; /** * Return the number of listeners listening to a given event. */ listenerCount(event: EventTypes): number; /** * Calls each of the listeners registered for a given event. */ emit(event: EventTypes, ...args: Array<any>): boolean; /** * Add a listener for a given event. */ on(event: EventTypes, fn: EventEmitter.ListenerFn, context?: any): this; addListener(event: EventTypes, fn: EventEmitter.ListenerFn, context?: any): this; /** * Add a one-time listener for a given event. */ once(event: EventTypes, fn: EventEmitter.ListenerFn, context?: any): this; /** * Remove the listeners of a given event. */ removeListener(event: EventTypes, fn?: EventEmitter.ListenerFn, context?: any, once?: boolean): this; off(event: EventTypes, fn?: EventEmitter.ListenerFn, context?: any, once?: boolean): this; /** * Remove all listeners, or those of the specified event. */ removeAllListeners(event?: EventTypes): this; } declare namespace EventEmitter { export interface ListenerFn { (...args: Array<any>): void; } export interface EventEmitterStatic { new<EventTypes extends string | symbol = string | symbol>(): EventEmitter<EventTypes>; } export const EventEmitter: EventEmitterStatic; } export = EventEmitter; apollo-server-demo/node_modules/eventemitter3/README.md 0000644 0001750 0000144 00000006712 03560116604 022513 0 ustar andreh users # EventEmitter3 [](https://www.npmjs.com/package/eventemitter3)[](https://travis-ci.org/primus/eventemitter3)[](https://david-dm.org/primus/eventemitter3)[](https://coveralls.io/r/primus/eventemitter3?branch=master)[](https://webchat.freenode.net/?channels=primus) [](https://saucelabs.com/u/eventemitter3) EventEmitter3 is a high performance EventEmitter. It has been micro-optimized for various of code paths making this, one of, if not the fastest EventEmitter available for Node.js and browsers. The module is API compatible with the EventEmitter that ships by default with Node.js but there are some slight differences: - Domain support has been removed. - We do not `throw` an error when you emit an `error` event and nobody is listening. - The `newListener` and `removeListener` events have been removed as they are useful only in some uncommon use-cases. - The `setMaxListeners`, `getMaxListeners`, `prependListener` and `prependOnceListener` methods are not available. - Support for custom context for events so there is no need to use `fn.bind`. - The `removeListener` method removes all matching listeners, not only the first. It's a drop in replacement for existing EventEmitters, but just faster. Free performance, who wouldn't want that? The EventEmitter is written in EcmaScript 3 so it will work in the oldest browsers and node versions that you need to support. ## Installation ```bash $ npm install --save eventemitter3 ``` ## CDN Recommended CDN: ```text https://unpkg.com/eventemitter3@latest/umd/eventemitter3.min.js ``` ## Usage After installation the only thing you need to do is require the module: ```js var EventEmitter = require('eventemitter3'); ``` And you're ready to create your own EventEmitter instances. For the API documentation, please follow the official Node.js documentation: http://nodejs.org/api/events.html ### Contextual emits We've upgraded the API of the `EventEmitter.on`, `EventEmitter.once` and `EventEmitter.removeListener` to accept an extra argument which is the `context` or `this` value that should be set for the emitted events. This means you no longer have the overhead of an event that required `fn.bind` in order to get a custom `this` value. ```js var EE = new EventEmitter() , context = { foo: 'bar' }; function emitted() { console.log(this === context); // true } EE.once('event-name', emitted, context); EE.on('another-event', emitted, context); EE.removeListener('another-event', emitted, context); ``` ### Tests and benchmarks This module is well tested. You can run: - `npm test` to run the tests under Node.js. - `npm run test-browser` to run the tests in real browsers via Sauce Labs. We also have a set of benchmarks to compare EventEmitter3 with some available alternatives. To run the benchmarks run `npm run benchmark`. Tests and benchmarks are not included in the npm package. If you want to play with them you have to clone the GitHub repository. ## License [MIT](LICENSE) apollo-server-demo/node_modules/eventemitter3/package.json 0000644 0001750 0000144 00000003273 03560116604 023521 0 ustar andreh users { "name": "eventemitter3", "version": "3.1.2", "description": "EventEmitter3 focuses on performance while maintaining a Node.js AND browser compatible interface.", "main": "index.js", "typings": "index.d.ts", "scripts": { "browserify": "rm -rf umd && mkdir umd && browserify index.js -s EventEmitter3 -o umd/eventemitter3.js", "minify": "uglifyjs umd/eventemitter3.js --source-map -cm -o umd/eventemitter3.min.js", "benchmark": "find benchmarks/run -name '*.js' -exec benchmarks/start.sh {} \\;", "test": "nyc --reporter=html --reporter=text mocha test/test.js", "prepublishOnly": "npm run browserify && npm run minify", "test-browser": "node test/browser.js" }, "files": [ "index.js", "index.d.ts", "umd" ], "repository": { "type": "git", "url": "git://github.com/primus/eventemitter3.git" }, "keywords": [ "EventEmitter", "EventEmitter2", "EventEmitter3", "Events", "addEventListener", "addListener", "emit", "emits", "emitter", "event", "once", "pub/sub", "publish", "reactor", "subscribe" ], "author": "Arnout Kazemier", "license": "MIT", "bugs": { "url": "https://github.com/primus/eventemitter3/issues" }, "devDependencies": { "assume": "~2.2.0", "browserify": "~16.2.0", "mocha": "~6.1.0", "nyc": "~14.0.0", "pre-commit": "~1.2.0", "sauce-browsers": "~2.0.0", "sauce-test": "~1.3.3", "uglify-js": "~3.5.0" } ,"_resolved": "https://registry.npmjs.org/eventemitter3/-/eventemitter3-3.1.2.tgz" ,"_integrity": "sha512-tvtQIeLVHjDkJYnzf2dgVMxfuSGJeM/7UCG17TT4EumTfNtF+0nebF/4zWOIkCreAbtNqhGEboB6BWrwqNaw4Q==" ,"_from": "eventemitter3@3.1.2" } apollo-server-demo/node_modules/media-typer/ 0000755 0001750 0000144 00000000000 14067647700 020662 5 ustar andreh users apollo-server-demo/node_modules/media-typer/index.js 0000644 0001750 0000144 00000014347 12403227667 022336 0 ustar andreh users /*! * media-typer * Copyright(c) 2014 Douglas Christopher Wilson * MIT Licensed */ /** * RegExp to match *( ";" parameter ) in RFC 2616 sec 3.7 * * parameter = token "=" ( token | quoted-string ) * token = 1*<any CHAR except CTLs or separators> * separators = "(" | ")" | "<" | ">" | "@" * | "," | ";" | ":" | "\" | <"> * | "/" | "[" | "]" | "?" | "=" * | "{" | "}" | SP | HT * quoted-string = ( <"> *(qdtext | quoted-pair ) <"> ) * qdtext = <any TEXT except <">> * quoted-pair = "\" CHAR * CHAR = <any US-ASCII character (octets 0 - 127)> * TEXT = <any OCTET except CTLs, but including LWS> * LWS = [CRLF] 1*( SP | HT ) * CRLF = CR LF * CR = <US-ASCII CR, carriage return (13)> * LF = <US-ASCII LF, linefeed (10)> * SP = <US-ASCII SP, space (32)> * SHT = <US-ASCII HT, horizontal-tab (9)> * CTL = <any US-ASCII control character (octets 0 - 31) and DEL (127)> * OCTET = <any 8-bit sequence of data> */ var paramRegExp = /; *([!#$%&'\*\+\-\.0-9A-Z\^_`a-z\|~]+) *= *("(?:[ !\u0023-\u005b\u005d-\u007e\u0080-\u00ff]|\\[\u0020-\u007e])*"|[!#$%&'\*\+\-\.0-9A-Z\^_`a-z\|~]+) */g; var textRegExp = /^[\u0020-\u007e\u0080-\u00ff]+$/ var tokenRegExp = /^[!#$%&'\*\+\-\.0-9A-Z\^_`a-z\|~]+$/ /** * RegExp to match quoted-pair in RFC 2616 * * quoted-pair = "\" CHAR * CHAR = <any US-ASCII character (octets 0 - 127)> */ var qescRegExp = /\\([\u0000-\u007f])/g; /** * RegExp to match chars that must be quoted-pair in RFC 2616 */ var quoteRegExp = /([\\"])/g; /** * RegExp to match type in RFC 6838 * * type-name = restricted-name * subtype-name = restricted-name * restricted-name = restricted-name-first *126restricted-name-chars * restricted-name-first = ALPHA / DIGIT * restricted-name-chars = ALPHA / DIGIT / "!" / "#" / * "$" / "&" / "-" / "^" / "_" * restricted-name-chars =/ "." ; Characters before first dot always * ; specify a facet name * restricted-name-chars =/ "+" ; Characters after last plus always * ; specify a structured syntax suffix * ALPHA = %x41-5A / %x61-7A ; A-Z / a-z * DIGIT = %x30-39 ; 0-9 */ var subtypeNameRegExp = /^[A-Za-z0-9][A-Za-z0-9!#$&^_.-]{0,126}$/ var typeNameRegExp = /^[A-Za-z0-9][A-Za-z0-9!#$&^_-]{0,126}$/ var typeRegExp = /^ *([A-Za-z0-9][A-Za-z0-9!#$&^_-]{0,126})\/([A-Za-z0-9][A-Za-z0-9!#$&^_.+-]{0,126}) *$/; /** * Module exports. */ exports.format = format exports.parse = parse /** * Format object to media type. * * @param {object} obj * @return {string} * @api public */ function format(obj) { if (!obj || typeof obj !== 'object') { throw new TypeError('argument obj is required') } var parameters = obj.parameters var subtype = obj.subtype var suffix = obj.suffix var type = obj.type if (!type || !typeNameRegExp.test(type)) { throw new TypeError('invalid type') } if (!subtype || !subtypeNameRegExp.test(subtype)) { throw new TypeError('invalid subtype') } // format as type/subtype var string = type + '/' + subtype // append +suffix if (suffix) { if (!typeNameRegExp.test(suffix)) { throw new TypeError('invalid suffix') } string += '+' + suffix } // append parameters if (parameters && typeof parameters === 'object') { var param var params = Object.keys(parameters).sort() for (var i = 0; i < params.length; i++) { param = params[i] if (!tokenRegExp.test(param)) { throw new TypeError('invalid parameter name') } string += '; ' + param + '=' + qstring(parameters[param]) } } return string } /** * Parse media type to object. * * @param {string|object} string * @return {Object} * @api public */ function parse(string) { if (!string) { throw new TypeError('argument string is required') } // support req/res-like objects as argument if (typeof string === 'object') { string = getcontenttype(string) } if (typeof string !== 'string') { throw new TypeError('argument string is required to be a string') } var index = string.indexOf(';') var type = index !== -1 ? string.substr(0, index) : string var key var match var obj = splitType(type) var params = {} var value paramRegExp.lastIndex = index while (match = paramRegExp.exec(string)) { if (match.index !== index) { throw new TypeError('invalid parameter format') } index += match[0].length key = match[1].toLowerCase() value = match[2] if (value[0] === '"') { // remove quotes and escapes value = value .substr(1, value.length - 2) .replace(qescRegExp, '$1') } params[key] = value } if (index !== -1 && index !== string.length) { throw new TypeError('invalid parameter format') } obj.parameters = params return obj } /** * Get content-type from req/res objects. * * @param {object} * @return {Object} * @api private */ function getcontenttype(obj) { if (typeof obj.getHeader === 'function') { // res-like return obj.getHeader('content-type') } if (typeof obj.headers === 'object') { // req-like return obj.headers && obj.headers['content-type'] } } /** * Quote a string if necessary. * * @param {string} val * @return {string} * @api private */ function qstring(val) { var str = String(val) // no need to quote tokens if (tokenRegExp.test(str)) { return str } if (str.length > 0 && !textRegExp.test(str)) { throw new TypeError('invalid parameter value') } return '"' + str.replace(quoteRegExp, '\\$1') + '"' } /** * Simply "type/subtype+siffx" into parts. * * @param {string} string * @return {Object} * @api private */ function splitType(string) { var match = typeRegExp.exec(string.toLowerCase()) if (!match) { throw new TypeError('invalid media type') } var type = match[1] var subtype = match[2] var suffix // suffix after last + var index = subtype.lastIndexOf('+') if (index !== -1) { suffix = subtype.substr(index + 1) subtype = subtype.substr(0, index) } var obj = { type: type, subtype: subtype, suffix: suffix } return obj } apollo-server-demo/node_modules/media-typer/LICENSE 0000644 0001750 0000144 00000002101 12403225242 021642 0 ustar andreh users (The MIT License) Copyright (c) 2014 Douglas Christopher Wilson Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the 'Software'), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/media-typer/HISTORY.md 0000644 0001750 0000144 00000000715 12403230352 022327 0 ustar andreh users 0.3.0 / 2014-09-07 ================== * Support Node.js 0.6 * Throw error when parameter format invalid on parse 0.2.0 / 2014-06-18 ================== * Add `typer.format()` to format media types 0.1.0 / 2014-06-17 ================== * Accept `req` as argument to `parse` * Accept `res` as argument to `parse` * Parse media type with extra LWS between type and first parameter 0.0.0 / 2014-06-13 ================== * Initial implementation apollo-server-demo/node_modules/media-typer/README.md 0000644 0001750 0000144 00000004503 12403225460 022126 0 ustar andreh users # media-typer [![NPM Version][npm-image]][npm-url] [![NPM Downloads][downloads-image]][downloads-url] [![Node.js Version][node-version-image]][node-version-url] [![Build Status][travis-image]][travis-url] [![Test Coverage][coveralls-image]][coveralls-url] Simple RFC 6838 media type parser ## Installation ```sh $ npm install media-typer ``` ## API ```js var typer = require('media-typer') ``` ### typer.parse(string) ```js var obj = typer.parse('image/svg+xml; charset=utf-8') ``` Parse a media type string. This will return an object with the following properties (examples are shown for the string `'image/svg+xml; charset=utf-8'`): - `type`: The type of the media type (always lower case). Example: `'image'` - `subtype`: The subtype of the media type (always lower case). Example: `'svg'` - `suffix`: The suffix of the media type (always lower case). Example: `'xml'` - `parameters`: An object of the parameters in the media type (name of parameter always lower case). Example: `{charset: 'utf-8'}` ### typer.parse(req) ```js var obj = typer.parse(req) ``` Parse the `content-type` header from the given `req`. Short-cut for `typer.parse(req.headers['content-type'])`. ### typer.parse(res) ```js var obj = typer.parse(res) ``` Parse the `content-type` header set on the given `res`. Short-cut for `typer.parse(res.getHeader('content-type'))`. ### typer.format(obj) ```js var obj = typer.format({type: 'image', subtype: 'svg', suffix: 'xml'}) ``` Format an object into a media type string. This will return a string of the mime type for the given object. For the properties of the object, see the documentation for `typer.parse(string)`. ## License [MIT](LICENSE) [npm-image]: https://img.shields.io/npm/v/media-typer.svg?style=flat [npm-url]: https://npmjs.org/package/media-typer [node-version-image]: https://img.shields.io/badge/node.js-%3E%3D_0.6-brightgreen.svg?style=flat [node-version-url]: http://nodejs.org/download/ [travis-image]: https://img.shields.io/travis/jshttp/media-typer.svg?style=flat [travis-url]: https://travis-ci.org/jshttp/media-typer [coveralls-image]: https://img.shields.io/coveralls/jshttp/media-typer.svg?style=flat [coveralls-url]: https://coveralls.io/r/jshttp/media-typer [downloads-image]: https://img.shields.io/npm/dm/media-typer.svg?style=flat [downloads-url]: https://npmjs.org/package/media-typer apollo-server-demo/node_modules/media-typer/package.json 0000644 0001750 0000144 00000001627 12403230206 023133 0 ustar andreh users { "name": "media-typer", "description": "Simple RFC 6838 media type parser and formatter", "version": "0.3.0", "author": "Douglas Christopher Wilson <doug@somethingdoug.com>", "license": "MIT", "repository": "jshttp/media-typer", "devDependencies": { "istanbul": "0.3.2", "mocha": "~1.21.4", "should": "~4.0.4" }, "files": [ "LICENSE", "HISTORY.md", "index.js" ], "engines": { "node": ">= 0.6" }, "scripts": { "test": "mocha --reporter spec --check-leaks --bail test/", "test-cov": "istanbul cover node_modules/mocha/bin/_mocha -- --reporter dot --check-leaks test/", "test-travis": "istanbul cover node_modules/mocha/bin/_mocha --report lcovonly -- --reporter spec --check-leaks test/" } ,"_resolved": "https://registry.npmjs.org/media-typer/-/media-typer-0.3.0.tgz" ,"_integrity": "sha1-hxDXrwqmJvj/+hzgAWhUUmMlV0g=" ,"_from": "media-typer@0.3.0" } apollo-server-demo/node_modules/utils-merge/ 0000755 0001750 0000144 00000000000 14067647700 020677 5 ustar andreh users apollo-server-demo/node_modules/utils-merge/index.js 0000644 0001750 0000144 00000000575 13160327632 022343 0 ustar andreh users /** * Merge object b with object a. * * var a = { foo: 'bar' } * , b = { bar: 'baz' }; * * merge(a, b); * // => { foo: 'bar', bar: 'baz' } * * @param {Object} a * @param {Object} b * @return {Object} * @api public */ exports = module.exports = function(a, b){ if (a && b) { for (var key in b) { a[key] = b[key]; } } return a; }; apollo-server-demo/node_modules/utils-merge/LICENSE 0000644 0001750 0000144 00000002074 13160330552 021672 0 ustar andreh users The MIT License (MIT) Copyright (c) 2013-2017 Jared Hanson Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/utils-merge/README.md 0000644 0001750 0000144 00000002447 13160331250 022144 0 ustar andreh users # utils-merge [](https://www.npmjs.com/package/utils-merge) [](https://travis-ci.org/jaredhanson/utils-merge) [](https://codeclimate.com/github/jaredhanson/utils-merge) [](https://coveralls.io/r/jaredhanson/utils-merge) [](https://david-dm.org/jaredhanson/utils-merge) Merges the properties from a source object into a destination object. ## Install ```bash $ npm install utils-merge ``` ## Usage ```javascript var a = { foo: 'bar' } , b = { bar: 'baz' }; merge(a, b); // => { foo: 'bar', bar: 'baz' } ``` ## License [The MIT License](http://opensource.org/licenses/MIT) Copyright (c) 2013-2017 Jared Hanson <[http://jaredhanson.net/](http://jaredhanson.net/)> <a target='_blank' rel='nofollow' href='https://app.codesponsor.io/link/vK9dyjRnnWsMzzJTQ57fRJpH/jaredhanson/utils-merge'> <img alt='Sponsor' width='888' height='68' src='https://app.codesponsor.io/embed/vK9dyjRnnWsMzzJTQ57fRJpH/jaredhanson/utils-merge.svg' /></a> apollo-server-demo/node_modules/utils-merge/package.json 0000644 0001750 0000144 00000001771 13160331727 023163 0 ustar andreh users { "name": "utils-merge", "version": "1.0.1", "description": "merge() utility function", "keywords": [ "util" ], "author": { "name": "Jared Hanson", "email": "jaredhanson@gmail.com", "url": "http://www.jaredhanson.net/" }, "repository": { "type": "git", "url": "git://github.com/jaredhanson/utils-merge.git" }, "bugs": { "url": "http://github.com/jaredhanson/utils-merge/issues" }, "license": "MIT", "licenses": [ { "type": "MIT", "url": "http://opensource.org/licenses/MIT" } ], "main": "./index", "dependencies": {}, "devDependencies": { "make-node": "0.3.x", "mocha": "1.x.x", "chai": "1.x.x" }, "engines": { "node": ">= 0.4.0" }, "scripts": { "test": "node_modules/.bin/mocha --reporter spec --require test/bootstrap/node test/*.test.js" } ,"_resolved": "https://registry.npmjs.org/utils-merge/-/utils-merge-1.0.1.tgz" ,"_integrity": "sha1-n5VxD1CiZ5R7LMwSR0HBAoQn5xM=" ,"_from": "utils-merge@1.0.1" } apollo-server-demo/node_modules/utils-merge/.npmignore 0000644 0001750 0000144 00000000117 13160331341 022655 0 ustar andreh users CONTRIBUTING.md Makefile docs/ examples/ reports/ test/ .jshintrc .travis.yml apollo-server-demo/node_modules/body-parser/ 0000755 0001750 0000144 00000000000 14067647700 020671 5 ustar andreh users apollo-server-demo/node_modules/body-parser/index.js 0000644 0001750 0000144 00000005140 03560116604 022324 0 ustar andreh users /*! * body-parser * Copyright(c) 2014-2015 Douglas Christopher Wilson * MIT Licensed */ 'use strict' /** * Module dependencies. * @private */ var deprecate = require('depd')('body-parser') /** * Cache of loaded parsers. * @private */ var parsers = Object.create(null) /** * @typedef Parsers * @type {function} * @property {function} json * @property {function} raw * @property {function} text * @property {function} urlencoded */ /** * Module exports. * @type {Parsers} */ exports = module.exports = deprecate.function(bodyParser, 'bodyParser: use individual json/urlencoded middlewares') /** * JSON parser. * @public */ Object.defineProperty(exports, 'json', { configurable: true, enumerable: true, get: createParserGetter('json') }) /** * Raw parser. * @public */ Object.defineProperty(exports, 'raw', { configurable: true, enumerable: true, get: createParserGetter('raw') }) /** * Text parser. * @public */ Object.defineProperty(exports, 'text', { configurable: true, enumerable: true, get: createParserGetter('text') }) /** * URL-encoded parser. * @public */ Object.defineProperty(exports, 'urlencoded', { configurable: true, enumerable: true, get: createParserGetter('urlencoded') }) /** * Create a middleware to parse json and urlencoded bodies. * * @param {object} [options] * @return {function} * @deprecated * @public */ function bodyParser (options) { var opts = {} // exclude type option if (options) { for (var prop in options) { if (prop !== 'type') { opts[prop] = options[prop] } } } var _urlencoded = exports.urlencoded(opts) var _json = exports.json(opts) return function bodyParser (req, res, next) { _json(req, res, function (err) { if (err) return next(err) _urlencoded(req, res, next) }) } } /** * Create a getter for loading a parser. * @private */ function createParserGetter (name) { return function get () { return loadParser(name) } } /** * Load a parser module. * @private */ function loadParser (parserName) { var parser = parsers[parserName] if (parser !== undefined) { return parser } // this uses a switch for static require analysis switch (parserName) { case 'json': parser = require('./lib/types/json') break case 'raw': parser = require('./lib/types/raw') break case 'text': parser = require('./lib/types/text') break case 'urlencoded': parser = require('./lib/types/urlencoded') break } // store to prevent invoking require() return (parsers[parserName] = parser) } apollo-server-demo/node_modules/body-parser/LICENSE 0000644 0001750 0000144 00000002224 03560116604 021664 0 ustar andreh users (The MIT License) Copyright (c) 2014 Jonathan Ong <me@jongleberry.com> Copyright (c) 2014-2015 Douglas Christopher Wilson <doug@somethingdoug.com> Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the 'Software'), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/body-parser/HISTORY.md 0000644 0001750 0000144 00000035411 03560116604 022346 0 ustar andreh users 1.19.0 / 2019-04-25 =================== * deps: bytes@3.1.0 - Add petabyte (`pb`) support * deps: http-errors@1.7.2 - Set constructor name when possible - deps: setprototypeof@1.1.1 - deps: statuses@'>= 1.5.0 < 2' * deps: iconv-lite@0.4.24 - Added encoding MIK * deps: qs@6.7.0 - Fix parsing array brackets after index * deps: raw-body@2.4.0 - deps: bytes@3.1.0 - deps: http-errors@1.7.2 - deps: iconv-lite@0.4.24 * deps: type-is@~1.6.17 - deps: mime-types@~2.1.24 - perf: prevent internal `throw` on invalid type 1.18.3 / 2018-05-14 =================== * Fix stack trace for strict json parse error * deps: depd@~1.1.2 - perf: remove argument reassignment * deps: http-errors@~1.6.3 - deps: depd@~1.1.2 - deps: setprototypeof@1.1.0 - deps: statuses@'>= 1.3.1 < 2' * deps: iconv-lite@0.4.23 - Fix loading encoding with year appended - Fix deprecation warnings on Node.js 10+ * deps: qs@6.5.2 * deps: raw-body@2.3.3 - deps: http-errors@1.6.3 - deps: iconv-lite@0.4.23 * deps: type-is@~1.6.16 - deps: mime-types@~2.1.18 1.18.2 / 2017-09-22 =================== * deps: debug@2.6.9 * perf: remove argument reassignment 1.18.1 / 2017-09-12 =================== * deps: content-type@~1.0.4 - perf: remove argument reassignment - perf: skip parameter parsing when no parameters * deps: iconv-lite@0.4.19 - Fix ISO-8859-1 regression - Update Windows-1255 * deps: qs@6.5.1 - Fix parsing & compacting very deep objects * deps: raw-body@2.3.2 - deps: iconv-lite@0.4.19 1.18.0 / 2017-09-08 =================== * Fix JSON strict violation error to match native parse error * Include the `body` property on verify errors * Include the `type` property on all generated errors * Use `http-errors` to set status code on errors * deps: bytes@3.0.0 * deps: debug@2.6.8 * deps: depd@~1.1.1 - Remove unnecessary `Buffer` loading * deps: http-errors@~1.6.2 - deps: depd@1.1.1 * deps: iconv-lite@0.4.18 - Add support for React Native - Add a warning if not loaded as utf-8 - Fix CESU-8 decoding in Node.js 8 - Improve speed of ISO-8859-1 encoding * deps: qs@6.5.0 * deps: raw-body@2.3.1 - Use `http-errors` for standard emitted errors - deps: bytes@3.0.0 - deps: iconv-lite@0.4.18 - perf: skip buffer decoding on overage chunk * perf: prevent internal `throw` when missing charset 1.17.2 / 2017-05-17 =================== * deps: debug@2.6.7 - Fix `DEBUG_MAX_ARRAY_LENGTH` - deps: ms@2.0.0 * deps: type-is@~1.6.15 - deps: mime-types@~2.1.15 1.17.1 / 2017-03-06 =================== * deps: qs@6.4.0 - Fix regression parsing keys starting with `[` 1.17.0 / 2017-03-01 =================== * deps: http-errors@~1.6.1 - Make `message` property enumerable for `HttpError`s - deps: setprototypeof@1.0.3 * deps: qs@6.3.1 - Fix compacting nested arrays 1.16.1 / 2017-02-10 =================== * deps: debug@2.6.1 - Fix deprecation messages in WebStorm and other editors - Undeprecate `DEBUG_FD` set to `1` or `2` 1.16.0 / 2017-01-17 =================== * deps: debug@2.6.0 - Allow colors in workers - Deprecated `DEBUG_FD` environment variable - Fix error when running under React Native - Use same color for same namespace - deps: ms@0.7.2 * deps: http-errors@~1.5.1 - deps: inherits@2.0.3 - deps: setprototypeof@1.0.2 - deps: statuses@'>= 1.3.1 < 2' * deps: iconv-lite@0.4.15 - Added encoding MS-31J - Added encoding MS-932 - Added encoding MS-936 - Added encoding MS-949 - Added encoding MS-950 - Fix GBK/GB18030 handling of Euro character * deps: qs@6.2.1 - Fix array parsing from skipping empty values * deps: raw-body@~2.2.0 - deps: iconv-lite@0.4.15 * deps: type-is@~1.6.14 - deps: mime-types@~2.1.13 1.15.2 / 2016-06-19 =================== * deps: bytes@2.4.0 * deps: content-type@~1.0.2 - perf: enable strict mode * deps: http-errors@~1.5.0 - Use `setprototypeof` module to replace `__proto__` setting - deps: statuses@'>= 1.3.0 < 2' - perf: enable strict mode * deps: qs@6.2.0 * deps: raw-body@~2.1.7 - deps: bytes@2.4.0 - perf: remove double-cleanup on happy path * deps: type-is@~1.6.13 - deps: mime-types@~2.1.11 1.15.1 / 2016-05-05 =================== * deps: bytes@2.3.0 - Drop partial bytes on all parsed units - Fix parsing byte string that looks like hex * deps: raw-body@~2.1.6 - deps: bytes@2.3.0 * deps: type-is@~1.6.12 - deps: mime-types@~2.1.10 1.15.0 / 2016-02-10 =================== * deps: http-errors@~1.4.0 - Add `HttpError` export, for `err instanceof createError.HttpError` - deps: inherits@2.0.1 - deps: statuses@'>= 1.2.1 < 2' * deps: qs@6.1.0 * deps: type-is@~1.6.11 - deps: mime-types@~2.1.9 1.14.2 / 2015-12-16 =================== * deps: bytes@2.2.0 * deps: iconv-lite@0.4.13 * deps: qs@5.2.0 * deps: raw-body@~2.1.5 - deps: bytes@2.2.0 - deps: iconv-lite@0.4.13 * deps: type-is@~1.6.10 - deps: mime-types@~2.1.8 1.14.1 / 2015-09-27 =================== * Fix issue where invalid charset results in 400 when `verify` used * deps: iconv-lite@0.4.12 - Fix CESU-8 decoding in Node.js 4.x * deps: raw-body@~2.1.4 - Fix masking critical errors from `iconv-lite` - deps: iconv-lite@0.4.12 * deps: type-is@~1.6.9 - deps: mime-types@~2.1.7 1.14.0 / 2015-09-16 =================== * Fix JSON strict parse error to match syntax errors * Provide static `require` analysis in `urlencoded` parser * deps: depd@~1.1.0 - Support web browser loading * deps: qs@5.1.0 * deps: raw-body@~2.1.3 - Fix sync callback when attaching data listener causes sync read * deps: type-is@~1.6.8 - Fix type error when given invalid type to match against - deps: mime-types@~2.1.6 1.13.3 / 2015-07-31 =================== * deps: type-is@~1.6.6 - deps: mime-types@~2.1.4 1.13.2 / 2015-07-05 =================== * deps: iconv-lite@0.4.11 * deps: qs@4.0.0 - Fix dropping parameters like `hasOwnProperty` - Fix user-visible incompatibilities from 3.1.0 - Fix various parsing edge cases * deps: raw-body@~2.1.2 - Fix error stack traces to skip `makeError` - deps: iconv-lite@0.4.11 * deps: type-is@~1.6.4 - deps: mime-types@~2.1.2 - perf: enable strict mode - perf: remove argument reassignment 1.13.1 / 2015-06-16 =================== * deps: qs@2.4.2 - Downgraded from 3.1.0 because of user-visible incompatibilities 1.13.0 / 2015-06-14 =================== * Add `statusCode` property on `Error`s, in addition to `status` * Change `type` default to `application/json` for JSON parser * Change `type` default to `application/x-www-form-urlencoded` for urlencoded parser * Provide static `require` analysis * Use the `http-errors` module to generate errors * deps: bytes@2.1.0 - Slight optimizations * deps: iconv-lite@0.4.10 - The encoding UTF-16 without BOM now defaults to UTF-16LE when detection fails - Leading BOM is now removed when decoding * deps: on-finished@~2.3.0 - Add defined behavior for HTTP `CONNECT` requests - Add defined behavior for HTTP `Upgrade` requests - deps: ee-first@1.1.1 * deps: qs@3.1.0 - Fix dropping parameters like `hasOwnProperty` - Fix various parsing edge cases - Parsed object now has `null` prototype * deps: raw-body@~2.1.1 - Use `unpipe` module for unpiping requests - deps: iconv-lite@0.4.10 * deps: type-is@~1.6.3 - deps: mime-types@~2.1.1 - perf: reduce try block size - perf: remove bitwise operations * perf: enable strict mode * perf: remove argument reassignment * perf: remove delete call 1.12.4 / 2015-05-10 =================== * deps: debug@~2.2.0 * deps: qs@2.4.2 - Fix allowing parameters like `constructor` * deps: on-finished@~2.2.1 * deps: raw-body@~2.0.1 - Fix a false-positive when unpiping in Node.js 0.8 - deps: bytes@2.0.1 * deps: type-is@~1.6.2 - deps: mime-types@~2.0.11 1.12.3 / 2015-04-15 =================== * Slight efficiency improvement when not debugging * deps: depd@~1.0.1 * deps: iconv-lite@0.4.8 - Add encoding alias UNICODE-1-1-UTF-7 * deps: raw-body@1.3.4 - Fix hanging callback if request aborts during read - deps: iconv-lite@0.4.8 1.12.2 / 2015-03-16 =================== * deps: qs@2.4.1 - Fix error when parameter `hasOwnProperty` is present 1.12.1 / 2015-03-15 =================== * deps: debug@~2.1.3 - Fix high intensity foreground color for bold - deps: ms@0.7.0 * deps: type-is@~1.6.1 - deps: mime-types@~2.0.10 1.12.0 / 2015-02-13 =================== * add `debug` messages * accept a function for the `type` option * use `content-type` to parse `Content-Type` headers * deps: iconv-lite@0.4.7 - Gracefully support enumerables on `Object.prototype` * deps: raw-body@1.3.3 - deps: iconv-lite@0.4.7 * deps: type-is@~1.6.0 - fix argument reassignment - fix false-positives in `hasBody` `Transfer-Encoding` check - support wildcard for both type and subtype (`*/*`) - deps: mime-types@~2.0.9 1.11.0 / 2015-01-30 =================== * make internal `extended: true` depth limit infinity * deps: type-is@~1.5.6 - deps: mime-types@~2.0.8 1.10.2 / 2015-01-20 =================== * deps: iconv-lite@0.4.6 - Fix rare aliases of single-byte encodings * deps: raw-body@1.3.2 - deps: iconv-lite@0.4.6 1.10.1 / 2015-01-01 =================== * deps: on-finished@~2.2.0 * deps: type-is@~1.5.5 - deps: mime-types@~2.0.7 1.10.0 / 2014-12-02 =================== * make internal `extended: true` array limit dynamic 1.9.3 / 2014-11-21 ================== * deps: iconv-lite@0.4.5 - Fix Windows-31J and X-SJIS encoding support * deps: qs@2.3.3 - Fix `arrayLimit` behavior * deps: raw-body@1.3.1 - deps: iconv-lite@0.4.5 * deps: type-is@~1.5.3 - deps: mime-types@~2.0.3 1.9.2 / 2014-10-27 ================== * deps: qs@2.3.2 - Fix parsing of mixed objects and values 1.9.1 / 2014-10-22 ================== * deps: on-finished@~2.1.1 - Fix handling of pipelined requests * deps: qs@2.3.0 - Fix parsing of mixed implicit and explicit arrays * deps: type-is@~1.5.2 - deps: mime-types@~2.0.2 1.9.0 / 2014-09-24 ================== * include the charset in "unsupported charset" error message * include the encoding in "unsupported content encoding" error message * deps: depd@~1.0.0 1.8.4 / 2014-09-23 ================== * fix content encoding to be case-insensitive 1.8.3 / 2014-09-19 ================== * deps: qs@2.2.4 - Fix issue with object keys starting with numbers truncated 1.8.2 / 2014-09-15 ================== * deps: depd@0.4.5 1.8.1 / 2014-09-07 ================== * deps: media-typer@0.3.0 * deps: type-is@~1.5.1 1.8.0 / 2014-09-05 ================== * make empty-body-handling consistent between chunked requests - empty `json` produces `{}` - empty `raw` produces `new Buffer(0)` - empty `text` produces `''` - empty `urlencoded` produces `{}` * deps: qs@2.2.3 - Fix issue where first empty value in array is discarded * deps: type-is@~1.5.0 - fix `hasbody` to be true for `content-length: 0` 1.7.0 / 2014-09-01 ================== * add `parameterLimit` option to `urlencoded` parser * change `urlencoded` extended array limit to 100 * respond with 413 when over `parameterLimit` in `urlencoded` 1.6.7 / 2014-08-29 ================== * deps: qs@2.2.2 - Remove unnecessary cloning 1.6.6 / 2014-08-27 ================== * deps: qs@2.2.0 - Array parsing fix - Performance improvements 1.6.5 / 2014-08-16 ================== * deps: on-finished@2.1.0 1.6.4 / 2014-08-14 ================== * deps: qs@1.2.2 1.6.3 / 2014-08-10 ================== * deps: qs@1.2.1 1.6.2 / 2014-08-07 ================== * deps: qs@1.2.0 - Fix parsing array of objects 1.6.1 / 2014-08-06 ================== * deps: qs@1.1.0 - Accept urlencoded square brackets - Accept empty values in implicit array notation 1.6.0 / 2014-08-05 ================== * deps: qs@1.0.2 - Complete rewrite - Limits array length to 20 - Limits object depth to 5 - Limits parameters to 1,000 1.5.2 / 2014-07-27 ================== * deps: depd@0.4.4 - Work-around v8 generating empty stack traces 1.5.1 / 2014-07-26 ================== * deps: depd@0.4.3 - Fix exception when global `Error.stackTraceLimit` is too low 1.5.0 / 2014-07-20 ================== * deps: depd@0.4.2 - Add `TRACE_DEPRECATION` environment variable - Remove non-standard grey color from color output - Support `--no-deprecation` argument - Support `--trace-deprecation` argument * deps: iconv-lite@0.4.4 - Added encoding UTF-7 * deps: raw-body@1.3.0 - deps: iconv-lite@0.4.4 - Added encoding UTF-7 - Fix `Cannot switch to old mode now` error on Node.js 0.10+ * deps: type-is@~1.3.2 1.4.3 / 2014-06-19 ================== * deps: type-is@1.3.1 - fix global variable leak 1.4.2 / 2014-06-19 ================== * deps: type-is@1.3.0 - improve type parsing 1.4.1 / 2014-06-19 ================== * fix urlencoded extended deprecation message 1.4.0 / 2014-06-19 ================== * add `text` parser * add `raw` parser * check accepted charset in content-type (accepts utf-8) * check accepted encoding in content-encoding (accepts identity) * deprecate `bodyParser()` middleware; use `.json()` and `.urlencoded()` as needed * deprecate `urlencoded()` without provided `extended` option * lazy-load urlencoded parsers * parsers split into files for reduced mem usage * support gzip and deflate bodies - set `inflate: false` to turn off * deps: raw-body@1.2.2 - Support all encodings from `iconv-lite` 1.3.1 / 2014-06-11 ================== * deps: type-is@1.2.1 - Switch dependency from mime to mime-types@1.0.0 1.3.0 / 2014-05-31 ================== * add `extended` option to urlencoded parser 1.2.2 / 2014-05-27 ================== * deps: raw-body@1.1.6 - assert stream encoding on node.js 0.8 - assert stream encoding on node.js < 0.10.6 - deps: bytes@1 1.2.1 / 2014-05-26 ================== * invoke `next(err)` after request fully read - prevents hung responses and socket hang ups 1.2.0 / 2014-05-11 ================== * add `verify` option * deps: type-is@1.2.0 - support suffix matching 1.1.2 / 2014-05-11 ================== * improve json parser speed 1.1.1 / 2014-05-11 ================== * fix repeated limit parsing with every request 1.1.0 / 2014-05-10 ================== * add `type` option * deps: pin for safety and consistency 1.0.2 / 2014-04-14 ================== * use `type-is` module 1.0.1 / 2014-03-20 ================== * lower default limits to 100kb apollo-server-demo/node_modules/body-parser/README.md 0000644 0001750 0000144 00000041314 03560116604 022141 0 ustar andreh users # body-parser [![NPM Version][npm-image]][npm-url] [![NPM Downloads][downloads-image]][downloads-url] [![Build Status][travis-image]][travis-url] [![Test Coverage][coveralls-image]][coveralls-url] Node.js body parsing middleware. Parse incoming request bodies in a middleware before your handlers, available under the `req.body` property. **Note** As `req.body`'s shape is based on user-controlled input, all properties and values in this object are untrusted and should be validated before trusting. For example, `req.body.foo.toString()` may fail in multiple ways, for example the `foo` property may not be there or may not be a string, and `toString` may not be a function and instead a string or other user input. [Learn about the anatomy of an HTTP transaction in Node.js](https://nodejs.org/en/docs/guides/anatomy-of-an-http-transaction/). _This does not handle multipart bodies_, due to their complex and typically large nature. For multipart bodies, you may be interested in the following modules: * [busboy](https://www.npmjs.org/package/busboy#readme) and [connect-busboy](https://www.npmjs.org/package/connect-busboy#readme) * [multiparty](https://www.npmjs.org/package/multiparty#readme) and [connect-multiparty](https://www.npmjs.org/package/connect-multiparty#readme) * [formidable](https://www.npmjs.org/package/formidable#readme) * [multer](https://www.npmjs.org/package/multer#readme) This module provides the following parsers: * [JSON body parser](#bodyparserjsonoptions) * [Raw body parser](#bodyparserrawoptions) * [Text body parser](#bodyparsertextoptions) * [URL-encoded form body parser](#bodyparserurlencodedoptions) Other body parsers you might be interested in: - [body](https://www.npmjs.org/package/body#readme) - [co-body](https://www.npmjs.org/package/co-body#readme) ## Installation ```sh $ npm install body-parser ``` ## API <!-- eslint-disable no-unused-vars --> ```js var bodyParser = require('body-parser') ``` The `bodyParser` object exposes various factories to create middlewares. All middlewares will populate the `req.body` property with the parsed body when the `Content-Type` request header matches the `type` option, or an empty object (`{}`) if there was no body to parse, the `Content-Type` was not matched, or an error occurred. The various errors returned by this module are described in the [errors section](#errors). ### bodyParser.json([options]) Returns middleware that only parses `json` and only looks at requests where the `Content-Type` header matches the `type` option. This parser accepts any Unicode encoding of the body and supports automatic inflation of `gzip` and `deflate` encodings. A new `body` object containing the parsed data is populated on the `request` object after the middleware (i.e. `req.body`). #### Options The `json` function takes an optional `options` object that may contain any of the following keys: ##### inflate When set to `true`, then deflated (compressed) bodies will be inflated; when `false`, deflated bodies are rejected. Defaults to `true`. ##### limit Controls the maximum request body size. If this is a number, then the value specifies the number of bytes; if it is a string, the value is passed to the [bytes](https://www.npmjs.com/package/bytes) library for parsing. Defaults to `'100kb'`. ##### reviver The `reviver` option is passed directly to `JSON.parse` as the second argument. You can find more information on this argument [in the MDN documentation about JSON.parse](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/JSON/parse#Example.3A_Using_the_reviver_parameter). ##### strict When set to `true`, will only accept arrays and objects; when `false` will accept anything `JSON.parse` accepts. Defaults to `true`. ##### type The `type` option is used to determine what media type the middleware will parse. This option can be a string, array of strings, or a function. If not a function, `type` option is passed directly to the [type-is](https://www.npmjs.org/package/type-is#readme) library and this can be an extension name (like `json`), a mime type (like `application/json`), or a mime type with a wildcard (like `*/*` or `*/json`). If a function, the `type` option is called as `fn(req)` and the request is parsed if it returns a truthy value. Defaults to `application/json`. ##### verify The `verify` option, if supplied, is called as `verify(req, res, buf, encoding)`, where `buf` is a `Buffer` of the raw request body and `encoding` is the encoding of the request. The parsing can be aborted by throwing an error. ### bodyParser.raw([options]) Returns middleware that parses all bodies as a `Buffer` and only looks at requests where the `Content-Type` header matches the `type` option. This parser supports automatic inflation of `gzip` and `deflate` encodings. A new `body` object containing the parsed data is populated on the `request` object after the middleware (i.e. `req.body`). This will be a `Buffer` object of the body. #### Options The `raw` function takes an optional `options` object that may contain any of the following keys: ##### inflate When set to `true`, then deflated (compressed) bodies will be inflated; when `false`, deflated bodies are rejected. Defaults to `true`. ##### limit Controls the maximum request body size. If this is a number, then the value specifies the number of bytes; if it is a string, the value is passed to the [bytes](https://www.npmjs.com/package/bytes) library for parsing. Defaults to `'100kb'`. ##### type The `type` option is used to determine what media type the middleware will parse. This option can be a string, array of strings, or a function. If not a function, `type` option is passed directly to the [type-is](https://www.npmjs.org/package/type-is#readme) library and this can be an extension name (like `bin`), a mime type (like `application/octet-stream`), or a mime type with a wildcard (like `*/*` or `application/*`). If a function, the `type` option is called as `fn(req)` and the request is parsed if it returns a truthy value. Defaults to `application/octet-stream`. ##### verify The `verify` option, if supplied, is called as `verify(req, res, buf, encoding)`, where `buf` is a `Buffer` of the raw request body and `encoding` is the encoding of the request. The parsing can be aborted by throwing an error. ### bodyParser.text([options]) Returns middleware that parses all bodies as a string and only looks at requests where the `Content-Type` header matches the `type` option. This parser supports automatic inflation of `gzip` and `deflate` encodings. A new `body` string containing the parsed data is populated on the `request` object after the middleware (i.e. `req.body`). This will be a string of the body. #### Options The `text` function takes an optional `options` object that may contain any of the following keys: ##### defaultCharset Specify the default character set for the text content if the charset is not specified in the `Content-Type` header of the request. Defaults to `utf-8`. ##### inflate When set to `true`, then deflated (compressed) bodies will be inflated; when `false`, deflated bodies are rejected. Defaults to `true`. ##### limit Controls the maximum request body size. If this is a number, then the value specifies the number of bytes; if it is a string, the value is passed to the [bytes](https://www.npmjs.com/package/bytes) library for parsing. Defaults to `'100kb'`. ##### type The `type` option is used to determine what media type the middleware will parse. This option can be a string, array of strings, or a function. If not a function, `type` option is passed directly to the [type-is](https://www.npmjs.org/package/type-is#readme) library and this can be an extension name (like `txt`), a mime type (like `text/plain`), or a mime type with a wildcard (like `*/*` or `text/*`). If a function, the `type` option is called as `fn(req)` and the request is parsed if it returns a truthy value. Defaults to `text/plain`. ##### verify The `verify` option, if supplied, is called as `verify(req, res, buf, encoding)`, where `buf` is a `Buffer` of the raw request body and `encoding` is the encoding of the request. The parsing can be aborted by throwing an error. ### bodyParser.urlencoded([options]) Returns middleware that only parses `urlencoded` bodies and only looks at requests where the `Content-Type` header matches the `type` option. This parser accepts only UTF-8 encoding of the body and supports automatic inflation of `gzip` and `deflate` encodings. A new `body` object containing the parsed data is populated on the `request` object after the middleware (i.e. `req.body`). This object will contain key-value pairs, where the value can be a string or array (when `extended` is `false`), or any type (when `extended` is `true`). #### Options The `urlencoded` function takes an optional `options` object that may contain any of the following keys: ##### extended The `extended` option allows to choose between parsing the URL-encoded data with the `querystring` library (when `false`) or the `qs` library (when `true`). The "extended" syntax allows for rich objects and arrays to be encoded into the URL-encoded format, allowing for a JSON-like experience with URL-encoded. For more information, please [see the qs library](https://www.npmjs.org/package/qs#readme). Defaults to `true`, but using the default has been deprecated. Please research into the difference between `qs` and `querystring` and choose the appropriate setting. ##### inflate When set to `true`, then deflated (compressed) bodies will be inflated; when `false`, deflated bodies are rejected. Defaults to `true`. ##### limit Controls the maximum request body size. If this is a number, then the value specifies the number of bytes; if it is a string, the value is passed to the [bytes](https://www.npmjs.com/package/bytes) library for parsing. Defaults to `'100kb'`. ##### parameterLimit The `parameterLimit` option controls the maximum number of parameters that are allowed in the URL-encoded data. If a request contains more parameters than this value, a 413 will be returned to the client. Defaults to `1000`. ##### type The `type` option is used to determine what media type the middleware will parse. This option can be a string, array of strings, or a function. If not a function, `type` option is passed directly to the [type-is](https://www.npmjs.org/package/type-is#readme) library and this can be an extension name (like `urlencoded`), a mime type (like `application/x-www-form-urlencoded`), or a mime type with a wildcard (like `*/x-www-form-urlencoded`). If a function, the `type` option is called as `fn(req)` and the request is parsed if it returns a truthy value. Defaults to `application/x-www-form-urlencoded`. ##### verify The `verify` option, if supplied, is called as `verify(req, res, buf, encoding)`, where `buf` is a `Buffer` of the raw request body and `encoding` is the encoding of the request. The parsing can be aborted by throwing an error. ## Errors The middlewares provided by this module create errors depending on the error condition during parsing. The errors will typically have a `status`/`statusCode` property that contains the suggested HTTP response code, an `expose` property to determine if the `message` property should be displayed to the client, a `type` property to determine the type of error without matching against the `message`, and a `body` property containing the read body, if available. The following are the common errors emitted, though any error can come through for various reasons. ### content encoding unsupported This error will occur when the request had a `Content-Encoding` header that contained an encoding but the "inflation" option was set to `false`. The `status` property is set to `415`, the `type` property is set to `'encoding.unsupported'`, and the `charset` property will be set to the encoding that is unsupported. ### request aborted This error will occur when the request is aborted by the client before reading the body has finished. The `received` property will be set to the number of bytes received before the request was aborted and the `expected` property is set to the number of expected bytes. The `status` property is set to `400` and `type` property is set to `'request.aborted'`. ### request entity too large This error will occur when the request body's size is larger than the "limit" option. The `limit` property will be set to the byte limit and the `length` property will be set to the request body's length. The `status` property is set to `413` and the `type` property is set to `'entity.too.large'`. ### request size did not match content length This error will occur when the request's length did not match the length from the `Content-Length` header. This typically occurs when the request is malformed, typically when the `Content-Length` header was calculated based on characters instead of bytes. The `status` property is set to `400` and the `type` property is set to `'request.size.invalid'`. ### stream encoding should not be set This error will occur when something called the `req.setEncoding` method prior to this middleware. This module operates directly on bytes only and you cannot call `req.setEncoding` when using this module. The `status` property is set to `500` and the `type` property is set to `'stream.encoding.set'`. ### too many parameters This error will occur when the content of the request exceeds the configured `parameterLimit` for the `urlencoded` parser. The `status` property is set to `413` and the `type` property is set to `'parameters.too.many'`. ### unsupported charset "BOGUS" This error will occur when the request had a charset parameter in the `Content-Type` header, but the `iconv-lite` module does not support it OR the parser does not support it. The charset is contained in the message as well as in the `charset` property. The `status` property is set to `415`, the `type` property is set to `'charset.unsupported'`, and the `charset` property is set to the charset that is unsupported. ### unsupported content encoding "bogus" This error will occur when the request had a `Content-Encoding` header that contained an unsupported encoding. The encoding is contained in the message as well as in the `encoding` property. The `status` property is set to `415`, the `type` property is set to `'encoding.unsupported'`, and the `encoding` property is set to the encoding that is unsupported. ## Examples ### Express/Connect top-level generic This example demonstrates adding a generic JSON and URL-encoded parser as a top-level middleware, which will parse the bodies of all incoming requests. This is the simplest setup. ```js var express = require('express') var bodyParser = require('body-parser') var app = express() // parse application/x-www-form-urlencoded app.use(bodyParser.urlencoded({ extended: false })) // parse application/json app.use(bodyParser.json()) app.use(function (req, res) { res.setHeader('Content-Type', 'text/plain') res.write('you posted:\n') res.end(JSON.stringify(req.body, null, 2)) }) ``` ### Express route-specific This example demonstrates adding body parsers specifically to the routes that need them. In general, this is the most recommended way to use body-parser with Express. ```js var express = require('express') var bodyParser = require('body-parser') var app = express() // create application/json parser var jsonParser = bodyParser.json() // create application/x-www-form-urlencoded parser var urlencodedParser = bodyParser.urlencoded({ extended: false }) // POST /login gets urlencoded bodies app.post('/login', urlencodedParser, function (req, res) { res.send('welcome, ' + req.body.username) }) // POST /api/users gets JSON bodies app.post('/api/users', jsonParser, function (req, res) { // create user in req.body }) ``` ### Change accepted type for parsers All the parsers accept a `type` option which allows you to change the `Content-Type` that the middleware will parse. ```js var express = require('express') var bodyParser = require('body-parser') var app = express() // parse various different custom JSON types as JSON app.use(bodyParser.json({ type: 'application/*+json' })) // parse some custom thing into a Buffer app.use(bodyParser.raw({ type: 'application/vnd.custom-type' })) // parse an HTML body into a string app.use(bodyParser.text({ type: 'text/html' })) ``` ## License [MIT](LICENSE) [npm-image]: https://img.shields.io/npm/v/body-parser.svg [npm-url]: https://npmjs.org/package/body-parser [travis-image]: https://img.shields.io/travis/expressjs/body-parser/master.svg [travis-url]: https://travis-ci.org/expressjs/body-parser [coveralls-image]: https://img.shields.io/coveralls/expressjs/body-parser/master.svg [coveralls-url]: https://coveralls.io/r/expressjs/body-parser?branch=master [downloads-image]: https://img.shields.io/npm/dm/body-parser.svg [downloads-url]: https://npmjs.org/package/body-parser apollo-server-demo/node_modules/body-parser/package.json 0000644 0001750 0000144 00000003364 03560116604 023153 0 ustar andreh users { "name": "body-parser", "description": "Node.js body parsing middleware", "version": "1.19.0", "contributors": [ "Douglas Christopher Wilson <doug@somethingdoug.com>", "Jonathan Ong <me@jongleberry.com> (http://jongleberry.com)" ], "license": "MIT", "repository": "expressjs/body-parser", "dependencies": { "bytes": "3.1.0", "content-type": "~1.0.4", "debug": "2.6.9", "depd": "~1.1.2", "http-errors": "1.7.2", "iconv-lite": "0.4.24", "on-finished": "~2.3.0", "qs": "6.7.0", "raw-body": "2.4.0", "type-is": "~1.6.17" }, "devDependencies": { "eslint": "5.16.0", "eslint-config-standard": "12.0.0", "eslint-plugin-import": "2.17.2", "eslint-plugin-markdown": "1.0.0", "eslint-plugin-node": "8.0.1", "eslint-plugin-promise": "4.1.1", "eslint-plugin-standard": "4.0.0", "istanbul": "0.4.5", "methods": "1.1.2", "mocha": "6.1.4", "safe-buffer": "5.1.2", "supertest": "4.0.2" }, "files": [ "lib/", "LICENSE", "HISTORY.md", "index.js" ], "engines": { "node": ">= 0.8" }, "scripts": { "lint": "eslint --plugin markdown --ext js,md .", "test": "mocha --require test/support/env --reporter spec --check-leaks --bail test/", "test-cov": "istanbul cover node_modules/mocha/bin/_mocha -- --require test/support/env --reporter dot --check-leaks test/", "test-travis": "istanbul cover node_modules/mocha/bin/_mocha --report lcovonly -- --require test/support/env --reporter spec --check-leaks test/" } ,"_resolved": "https://registry.npmjs.org/body-parser/-/body-parser-1.19.0.tgz" ,"_integrity": "sha512-dhEPs72UPbDnAQJ9ZKMNTP6ptJaionhP5cBb541nXPlW60Jepo9RV/a4fX4XWW9CuFNK22krhrj1+rgzifNCsw==" ,"_from": "body-parser@1.19.0" } apollo-server-demo/node_modules/body-parser/lib/ 0000755 0001750 0000144 00000000000 14067647700 021437 5 ustar andreh users apollo-server-demo/node_modules/body-parser/lib/types/ 0000755 0001750 0000144 00000000000 14067647700 022603 5 ustar andreh users apollo-server-demo/node_modules/body-parser/lib/types/text.js 0000644 0001750 0000144 00000004355 03560116604 024122 0 ustar andreh users /*! * body-parser * Copyright(c) 2014-2015 Douglas Christopher Wilson * MIT Licensed */ 'use strict' /** * Module dependencies. */ var bytes = require('bytes') var contentType = require('content-type') var debug = require('debug')('body-parser:text') var read = require('../read') var typeis = require('type-is') /** * Module exports. */ module.exports = text /** * Create a middleware to parse text bodies. * * @param {object} [options] * @return {function} * @api public */ function text (options) { var opts = options || {} var defaultCharset = opts.defaultCharset || 'utf-8' var inflate = opts.inflate !== false var limit = typeof opts.limit !== 'number' ? bytes.parse(opts.limit || '100kb') : opts.limit var type = opts.type || 'text/plain' var verify = opts.verify || false if (verify !== false && typeof verify !== 'function') { throw new TypeError('option verify must be function') } // create the appropriate type checking function var shouldParse = typeof type !== 'function' ? typeChecker(type) : type function parse (buf) { return buf } return function textParser (req, res, next) { if (req._body) { debug('body already parsed') next() return } req.body = req.body || {} // skip requests without bodies if (!typeis.hasBody(req)) { debug('skip empty body') next() return } debug('content-type %j', req.headers['content-type']) // determine if request should be parsed if (!shouldParse(req)) { debug('skip parsing') next() return } // get charset var charset = getCharset(req) || defaultCharset // read read(req, res, next, parse, debug, { encoding: charset, inflate: inflate, limit: limit, verify: verify }) } } /** * Get the charset of a request. * * @param {object} req * @api private */ function getCharset (req) { try { return (contentType.parse(req).parameters.charset || '').toLowerCase() } catch (e) { return undefined } } /** * Get the simple type checker. * * @param {string} type * @return {function} */ function typeChecker (type) { return function checkType (req) { return Boolean(typeis(req, type)) } } apollo-server-demo/node_modules/body-parser/lib/types/urlencoded.js 0000644 0001750 0000144 00000013245 03560116604 025260 0 ustar andreh users /*! * body-parser * Copyright(c) 2014 Jonathan Ong * Copyright(c) 2014-2015 Douglas Christopher Wilson * MIT Licensed */ 'use strict' /** * Module dependencies. * @private */ var bytes = require('bytes') var contentType = require('content-type') var createError = require('http-errors') var debug = require('debug')('body-parser:urlencoded') var deprecate = require('depd')('body-parser') var read = require('../read') var typeis = require('type-is') /** * Module exports. */ module.exports = urlencoded /** * Cache of parser modules. */ var parsers = Object.create(null) /** * Create a middleware to parse urlencoded bodies. * * @param {object} [options] * @return {function} * @public */ function urlencoded (options) { var opts = options || {} // notice because option default will flip in next major if (opts.extended === undefined) { deprecate('undefined extended: provide extended option') } var extended = opts.extended !== false var inflate = opts.inflate !== false var limit = typeof opts.limit !== 'number' ? bytes.parse(opts.limit || '100kb') : opts.limit var type = opts.type || 'application/x-www-form-urlencoded' var verify = opts.verify || false if (verify !== false && typeof verify !== 'function') { throw new TypeError('option verify must be function') } // create the appropriate query parser var queryparse = extended ? extendedparser(opts) : simpleparser(opts) // create the appropriate type checking function var shouldParse = typeof type !== 'function' ? typeChecker(type) : type function parse (body) { return body.length ? queryparse(body) : {} } return function urlencodedParser (req, res, next) { if (req._body) { debug('body already parsed') next() return } req.body = req.body || {} // skip requests without bodies if (!typeis.hasBody(req)) { debug('skip empty body') next() return } debug('content-type %j', req.headers['content-type']) // determine if request should be parsed if (!shouldParse(req)) { debug('skip parsing') next() return } // assert charset var charset = getCharset(req) || 'utf-8' if (charset !== 'utf-8') { debug('invalid charset') next(createError(415, 'unsupported charset "' + charset.toUpperCase() + '"', { charset: charset, type: 'charset.unsupported' })) return } // read read(req, res, next, parse, debug, { debug: debug, encoding: charset, inflate: inflate, limit: limit, verify: verify }) } } /** * Get the extended query parser. * * @param {object} options */ function extendedparser (options) { var parameterLimit = options.parameterLimit !== undefined ? options.parameterLimit : 1000 var parse = parser('qs') if (isNaN(parameterLimit) || parameterLimit < 1) { throw new TypeError('option parameterLimit must be a positive number') } if (isFinite(parameterLimit)) { parameterLimit = parameterLimit | 0 } return function queryparse (body) { var paramCount = parameterCount(body, parameterLimit) if (paramCount === undefined) { debug('too many parameters') throw createError(413, 'too many parameters', { type: 'parameters.too.many' }) } var arrayLimit = Math.max(100, paramCount) debug('parse extended urlencoding') return parse(body, { allowPrototypes: true, arrayLimit: arrayLimit, depth: Infinity, parameterLimit: parameterLimit }) } } /** * Get the charset of a request. * * @param {object} req * @api private */ function getCharset (req) { try { return (contentType.parse(req).parameters.charset || '').toLowerCase() } catch (e) { return undefined } } /** * Count the number of parameters, stopping once limit reached * * @param {string} body * @param {number} limit * @api private */ function parameterCount (body, limit) { var count = 0 var index = 0 while ((index = body.indexOf('&', index)) !== -1) { count++ index++ if (count === limit) { return undefined } } return count } /** * Get parser for module name dynamically. * * @param {string} name * @return {function} * @api private */ function parser (name) { var mod = parsers[name] if (mod !== undefined) { return mod.parse } // this uses a switch for static require analysis switch (name) { case 'qs': mod = require('qs') break case 'querystring': mod = require('querystring') break } // store to prevent invoking require() parsers[name] = mod return mod.parse } /** * Get the simple query parser. * * @param {object} options */ function simpleparser (options) { var parameterLimit = options.parameterLimit !== undefined ? options.parameterLimit : 1000 var parse = parser('querystring') if (isNaN(parameterLimit) || parameterLimit < 1) { throw new TypeError('option parameterLimit must be a positive number') } if (isFinite(parameterLimit)) { parameterLimit = parameterLimit | 0 } return function queryparse (body) { var paramCount = parameterCount(body, parameterLimit) if (paramCount === undefined) { debug('too many parameters') throw createError(413, 'too many parameters', { type: 'parameters.too.many' }) } debug('parse urlencoding') return parse(body, undefined, undefined, { maxKeys: parameterLimit }) } } /** * Get the simple type checker. * * @param {string} type * @return {function} */ function typeChecker (type) { return function checkType (req) { return Boolean(typeis(req, type)) } } apollo-server-demo/node_modules/body-parser/lib/types/json.js 0000644 0001750 0000144 00000011466 03560116604 024110 0 ustar andreh users /*! * body-parser * Copyright(c) 2014 Jonathan Ong * Copyright(c) 2014-2015 Douglas Christopher Wilson * MIT Licensed */ 'use strict' /** * Module dependencies. * @private */ var bytes = require('bytes') var contentType = require('content-type') var createError = require('http-errors') var debug = require('debug')('body-parser:json') var read = require('../read') var typeis = require('type-is') /** * Module exports. */ module.exports = json /** * RegExp to match the first non-space in a string. * * Allowed whitespace is defined in RFC 7159: * * ws = *( * %x20 / ; Space * %x09 / ; Horizontal tab * %x0A / ; Line feed or New line * %x0D ) ; Carriage return */ var FIRST_CHAR_REGEXP = /^[\x20\x09\x0a\x0d]*(.)/ // eslint-disable-line no-control-regex /** * Create a middleware to parse JSON bodies. * * @param {object} [options] * @return {function} * @public */ function json (options) { var opts = options || {} var limit = typeof opts.limit !== 'number' ? bytes.parse(opts.limit || '100kb') : opts.limit var inflate = opts.inflate !== false var reviver = opts.reviver var strict = opts.strict !== false var type = opts.type || 'application/json' var verify = opts.verify || false if (verify !== false && typeof verify !== 'function') { throw new TypeError('option verify must be function') } // create the appropriate type checking function var shouldParse = typeof type !== 'function' ? typeChecker(type) : type function parse (body) { if (body.length === 0) { // special-case empty json body, as it's a common client-side mistake // TODO: maybe make this configurable or part of "strict" option return {} } if (strict) { var first = firstchar(body) if (first !== '{' && first !== '[') { debug('strict violation') throw createStrictSyntaxError(body, first) } } try { debug('parse json') return JSON.parse(body, reviver) } catch (e) { throw normalizeJsonSyntaxError(e, { message: e.message, stack: e.stack }) } } return function jsonParser (req, res, next) { if (req._body) { debug('body already parsed') next() return } req.body = req.body || {} // skip requests without bodies if (!typeis.hasBody(req)) { debug('skip empty body') next() return } debug('content-type %j', req.headers['content-type']) // determine if request should be parsed if (!shouldParse(req)) { debug('skip parsing') next() return } // assert charset per RFC 7159 sec 8.1 var charset = getCharset(req) || 'utf-8' if (charset.substr(0, 4) !== 'utf-') { debug('invalid charset') next(createError(415, 'unsupported charset "' + charset.toUpperCase() + '"', { charset: charset, type: 'charset.unsupported' })) return } // read read(req, res, next, parse, debug, { encoding: charset, inflate: inflate, limit: limit, verify: verify }) } } /** * Create strict violation syntax error matching native error. * * @param {string} str * @param {string} char * @return {Error} * @private */ function createStrictSyntaxError (str, char) { var index = str.indexOf(char) var partial = str.substring(0, index) + '#' try { JSON.parse(partial); /* istanbul ignore next */ throw new SyntaxError('strict violation') } catch (e) { return normalizeJsonSyntaxError(e, { message: e.message.replace('#', char), stack: e.stack }) } } /** * Get the first non-whitespace character in a string. * * @param {string} str * @return {function} * @private */ function firstchar (str) { return FIRST_CHAR_REGEXP.exec(str)[1] } /** * Get the charset of a request. * * @param {object} req * @api private */ function getCharset (req) { try { return (contentType.parse(req).parameters.charset || '').toLowerCase() } catch (e) { return undefined } } /** * Normalize a SyntaxError for JSON.parse. * * @param {SyntaxError} error * @param {object} obj * @return {SyntaxError} */ function normalizeJsonSyntaxError (error, obj) { var keys = Object.getOwnPropertyNames(error) for (var i = 0; i < keys.length; i++) { var key = keys[i] if (key !== 'stack' && key !== 'message') { delete error[key] } } // replace stack before message for Node.js 0.10 and below error.stack = obj.stack.replace(error.message, obj.message) error.message = obj.message return error } /** * Get the simple type checker. * * @param {string} type * @return {function} */ function typeChecker (type) { return function checkType (req) { return Boolean(typeis(req, type)) } } apollo-server-demo/node_modules/body-parser/lib/types/raw.js 0000644 0001750 0000144 00000003534 03560116604 023725 0 ustar andreh users /*! * body-parser * Copyright(c) 2014-2015 Douglas Christopher Wilson * MIT Licensed */ 'use strict' /** * Module dependencies. */ var bytes = require('bytes') var debug = require('debug')('body-parser:raw') var read = require('../read') var typeis = require('type-is') /** * Module exports. */ module.exports = raw /** * Create a middleware to parse raw bodies. * * @param {object} [options] * @return {function} * @api public */ function raw (options) { var opts = options || {} var inflate = opts.inflate !== false var limit = typeof opts.limit !== 'number' ? bytes.parse(opts.limit || '100kb') : opts.limit var type = opts.type || 'application/octet-stream' var verify = opts.verify || false if (verify !== false && typeof verify !== 'function') { throw new TypeError('option verify must be function') } // create the appropriate type checking function var shouldParse = typeof type !== 'function' ? typeChecker(type) : type function parse (buf) { return buf } return function rawParser (req, res, next) { if (req._body) { debug('body already parsed') next() return } req.body = req.body || {} // skip requests without bodies if (!typeis.hasBody(req)) { debug('skip empty body') next() return } debug('content-type %j', req.headers['content-type']) // determine if request should be parsed if (!shouldParse(req)) { debug('skip parsing') next() return } // read read(req, res, next, parse, debug, { encoding: null, inflate: inflate, limit: limit, verify: verify }) } } /** * Get the simple type checker. * * @param {string} type * @return {function} */ function typeChecker (type) { return function checkType (req) { return Boolean(typeis(req, type)) } } apollo-server-demo/node_modules/body-parser/lib/read.js 0000644 0001750 0000144 00000007466 03560116604 022713 0 ustar andreh users /*! * body-parser * Copyright(c) 2014-2015 Douglas Christopher Wilson * MIT Licensed */ 'use strict' /** * Module dependencies. * @private */ var createError = require('http-errors') var getBody = require('raw-body') var iconv = require('iconv-lite') var onFinished = require('on-finished') var zlib = require('zlib') /** * Module exports. */ module.exports = read /** * Read a request into a buffer and parse. * * @param {object} req * @param {object} res * @param {function} next * @param {function} parse * @param {function} debug * @param {object} options * @private */ function read (req, res, next, parse, debug, options) { var length var opts = options var stream // flag as parsed req._body = true // read options var encoding = opts.encoding !== null ? opts.encoding : null var verify = opts.verify try { // get the content stream stream = contentstream(req, debug, opts.inflate) length = stream.length stream.length = undefined } catch (err) { return next(err) } // set raw-body options opts.length = length opts.encoding = verify ? null : encoding // assert charset is supported if (opts.encoding === null && encoding !== null && !iconv.encodingExists(encoding)) { return next(createError(415, 'unsupported charset "' + encoding.toUpperCase() + '"', { charset: encoding.toLowerCase(), type: 'charset.unsupported' })) } // read body debug('read body') getBody(stream, opts, function (error, body) { if (error) { var _error if (error.type === 'encoding.unsupported') { // echo back charset _error = createError(415, 'unsupported charset "' + encoding.toUpperCase() + '"', { charset: encoding.toLowerCase(), type: 'charset.unsupported' }) } else { // set status code on error _error = createError(400, error) } // read off entire request stream.resume() onFinished(req, function onfinished () { next(createError(400, _error)) }) return } // verify if (verify) { try { debug('verify body') verify(req, res, body, encoding) } catch (err) { next(createError(403, err, { body: body, type: err.type || 'entity.verify.failed' })) return } } // parse var str = body try { debug('parse body') str = typeof body !== 'string' && encoding !== null ? iconv.decode(body, encoding) : body req.body = parse(str) } catch (err) { next(createError(400, err, { body: str, type: err.type || 'entity.parse.failed' })) return } next() }) } /** * Get the content stream of the request. * * @param {object} req * @param {function} debug * @param {boolean} [inflate=true] * @return {object} * @api private */ function contentstream (req, debug, inflate) { var encoding = (req.headers['content-encoding'] || 'identity').toLowerCase() var length = req.headers['content-length'] var stream debug('content-encoding "%s"', encoding) if (inflate === false && encoding !== 'identity') { throw createError(415, 'content encoding unsupported', { encoding: encoding, type: 'encoding.unsupported' }) } switch (encoding) { case 'deflate': stream = zlib.createInflate() debug('inflate body') req.pipe(stream) break case 'gzip': stream = zlib.createGunzip() debug('gunzip body') req.pipe(stream) break case 'identity': stream = req stream.length = length break default: throw createError(415, 'unsupported content encoding "' + encoding + '"', { encoding: encoding, type: 'encoding.unsupported' }) } return stream } apollo-server-demo/node_modules/get-intrinsic/ 0000755 0001750 0000144 00000000000 14067647700 021221 5 ustar andreh users apollo-server-demo/node_modules/get-intrinsic/index.js 0000644 0001750 0000144 00000027765 03560116604 022675 0 ustar andreh users 'use strict'; /* globals AggregateError, Atomics, FinalizationRegistry, SharedArrayBuffer, WeakRef, */ var undefined; var $SyntaxError = SyntaxError; var $Function = Function; var $TypeError = TypeError; // eslint-disable-next-line consistent-return var getEvalledConstructor = function (expressionSyntax) { try { // eslint-disable-next-line no-new-func return Function('"use strict"; return (' + expressionSyntax + ').constructor;')(); } catch (e) {} }; var $gOPD = Object.getOwnPropertyDescriptor; if ($gOPD) { try { $gOPD({}, ''); } catch (e) { $gOPD = null; // this is IE 8, which has a broken gOPD } } var throwTypeError = function () { throw new $TypeError(); }; var ThrowTypeError = $gOPD ? (function () { try { // eslint-disable-next-line no-unused-expressions, no-caller, no-restricted-properties arguments.callee; // IE 8 does not throw here return throwTypeError; } catch (calleeThrows) { try { // IE 8 throws on Object.getOwnPropertyDescriptor(arguments, '') return $gOPD(arguments, 'callee').get; } catch (gOPDthrows) { return throwTypeError; } } }()) : throwTypeError; var hasSymbols = require('has-symbols')(); var getProto = Object.getPrototypeOf || function (x) { return x.__proto__; }; // eslint-disable-line no-proto var asyncGenFunction = getEvalledConstructor('async function* () {}'); var asyncGenFunctionPrototype = asyncGenFunction ? asyncGenFunction.prototype : undefined; var asyncGenPrototype = asyncGenFunctionPrototype ? asyncGenFunctionPrototype.prototype : undefined; var TypedArray = typeof Uint8Array === 'undefined' ? undefined : getProto(Uint8Array); var INTRINSICS = { '%AggregateError%': typeof AggregateError === 'undefined' ? undefined : AggregateError, '%Array%': Array, '%ArrayBuffer%': typeof ArrayBuffer === 'undefined' ? undefined : ArrayBuffer, '%ArrayIteratorPrototype%': hasSymbols ? getProto([][Symbol.iterator]()) : undefined, '%AsyncFromSyncIteratorPrototype%': undefined, '%AsyncFunction%': getEvalledConstructor('async function () {}'), '%AsyncGenerator%': asyncGenFunctionPrototype, '%AsyncGeneratorFunction%': asyncGenFunction, '%AsyncIteratorPrototype%': asyncGenPrototype ? getProto(asyncGenPrototype) : undefined, '%Atomics%': typeof Atomics === 'undefined' ? undefined : Atomics, '%BigInt%': typeof BigInt === 'undefined' ? undefined : BigInt, '%Boolean%': Boolean, '%DataView%': typeof DataView === 'undefined' ? undefined : DataView, '%Date%': Date, '%decodeURI%': decodeURI, '%decodeURIComponent%': decodeURIComponent, '%encodeURI%': encodeURI, '%encodeURIComponent%': encodeURIComponent, '%Error%': Error, '%eval%': eval, // eslint-disable-line no-eval '%EvalError%': EvalError, '%Float32Array%': typeof Float32Array === 'undefined' ? undefined : Float32Array, '%Float64Array%': typeof Float64Array === 'undefined' ? undefined : Float64Array, '%FinalizationRegistry%': typeof FinalizationRegistry === 'undefined' ? undefined : FinalizationRegistry, '%Function%': $Function, '%GeneratorFunction%': getEvalledConstructor('function* () {}'), '%Int8Array%': typeof Int8Array === 'undefined' ? undefined : Int8Array, '%Int16Array%': typeof Int16Array === 'undefined' ? undefined : Int16Array, '%Int32Array%': typeof Int32Array === 'undefined' ? undefined : Int32Array, '%isFinite%': isFinite, '%isNaN%': isNaN, '%IteratorPrototype%': hasSymbols ? getProto(getProto([][Symbol.iterator]())) : undefined, '%JSON%': typeof JSON === 'object' ? JSON : undefined, '%Map%': typeof Map === 'undefined' ? undefined : Map, '%MapIteratorPrototype%': typeof Map === 'undefined' || !hasSymbols ? undefined : getProto(new Map()[Symbol.iterator]()), '%Math%': Math, '%Number%': Number, '%Object%': Object, '%parseFloat%': parseFloat, '%parseInt%': parseInt, '%Promise%': typeof Promise === 'undefined' ? undefined : Promise, '%Proxy%': typeof Proxy === 'undefined' ? undefined : Proxy, '%RangeError%': RangeError, '%ReferenceError%': ReferenceError, '%Reflect%': typeof Reflect === 'undefined' ? undefined : Reflect, '%RegExp%': RegExp, '%Set%': typeof Set === 'undefined' ? undefined : Set, '%SetIteratorPrototype%': typeof Set === 'undefined' || !hasSymbols ? undefined : getProto(new Set()[Symbol.iterator]()), '%SharedArrayBuffer%': typeof SharedArrayBuffer === 'undefined' ? undefined : SharedArrayBuffer, '%String%': String, '%StringIteratorPrototype%': hasSymbols ? getProto(''[Symbol.iterator]()) : undefined, '%Symbol%': hasSymbols ? Symbol : undefined, '%SyntaxError%': $SyntaxError, '%ThrowTypeError%': ThrowTypeError, '%TypedArray%': TypedArray, '%TypeError%': $TypeError, '%Uint8Array%': typeof Uint8Array === 'undefined' ? undefined : Uint8Array, '%Uint8ClampedArray%': typeof Uint8ClampedArray === 'undefined' ? undefined : Uint8ClampedArray, '%Uint16Array%': typeof Uint16Array === 'undefined' ? undefined : Uint16Array, '%Uint32Array%': typeof Uint32Array === 'undefined' ? undefined : Uint32Array, '%URIError%': URIError, '%WeakMap%': typeof WeakMap === 'undefined' ? undefined : WeakMap, '%WeakRef%': typeof WeakRef === 'undefined' ? undefined : WeakRef, '%WeakSet%': typeof WeakSet === 'undefined' ? undefined : WeakSet }; var LEGACY_ALIASES = { '%ArrayBufferPrototype%': ['ArrayBuffer', 'prototype'], '%ArrayPrototype%': ['Array', 'prototype'], '%ArrayProto_entries%': ['Array', 'prototype', 'entries'], '%ArrayProto_forEach%': ['Array', 'prototype', 'forEach'], '%ArrayProto_keys%': ['Array', 'prototype', 'keys'], '%ArrayProto_values%': ['Array', 'prototype', 'values'], '%AsyncFunctionPrototype%': ['AsyncFunction', 'prototype'], '%AsyncGenerator%': ['AsyncGeneratorFunction', 'prototype'], '%AsyncGeneratorPrototype%': ['AsyncGeneratorFunction', 'prototype', 'prototype'], '%BooleanPrototype%': ['Boolean', 'prototype'], '%DataViewPrototype%': ['DataView', 'prototype'], '%DatePrototype%': ['Date', 'prototype'], '%ErrorPrototype%': ['Error', 'prototype'], '%EvalErrorPrototype%': ['EvalError', 'prototype'], '%Float32ArrayPrototype%': ['Float32Array', 'prototype'], '%Float64ArrayPrototype%': ['Float64Array', 'prototype'], '%FunctionPrototype%': ['Function', 'prototype'], '%Generator%': ['GeneratorFunction', 'prototype'], '%GeneratorPrototype%': ['GeneratorFunction', 'prototype', 'prototype'], '%Int8ArrayPrototype%': ['Int8Array', 'prototype'], '%Int16ArrayPrototype%': ['Int16Array', 'prototype'], '%Int32ArrayPrototype%': ['Int32Array', 'prototype'], '%JSONParse%': ['JSON', 'parse'], '%JSONStringify%': ['JSON', 'stringify'], '%MapPrototype%': ['Map', 'prototype'], '%NumberPrototype%': ['Number', 'prototype'], '%ObjectPrototype%': ['Object', 'prototype'], '%ObjProto_toString%': ['Object', 'prototype', 'toString'], '%ObjProto_valueOf%': ['Object', 'prototype', 'valueOf'], '%PromisePrototype%': ['Promise', 'prototype'], '%PromiseProto_then%': ['Promise', 'prototype', 'then'], '%Promise_all%': ['Promise', 'all'], '%Promise_reject%': ['Promise', 'reject'], '%Promise_resolve%': ['Promise', 'resolve'], '%RangeErrorPrototype%': ['RangeError', 'prototype'], '%ReferenceErrorPrototype%': ['ReferenceError', 'prototype'], '%RegExpPrototype%': ['RegExp', 'prototype'], '%SetPrototype%': ['Set', 'prototype'], '%SharedArrayBufferPrototype%': ['SharedArrayBuffer', 'prototype'], '%StringPrototype%': ['String', 'prototype'], '%SymbolPrototype%': ['Symbol', 'prototype'], '%SyntaxErrorPrototype%': ['SyntaxError', 'prototype'], '%TypedArrayPrototype%': ['TypedArray', 'prototype'], '%TypeErrorPrototype%': ['TypeError', 'prototype'], '%Uint8ArrayPrototype%': ['Uint8Array', 'prototype'], '%Uint8ClampedArrayPrototype%': ['Uint8ClampedArray', 'prototype'], '%Uint16ArrayPrototype%': ['Uint16Array', 'prototype'], '%Uint32ArrayPrototype%': ['Uint32Array', 'prototype'], '%URIErrorPrototype%': ['URIError', 'prototype'], '%WeakMapPrototype%': ['WeakMap', 'prototype'], '%WeakSetPrototype%': ['WeakSet', 'prototype'] }; var bind = require('function-bind'); var hasOwn = require('has'); var $concat = bind.call(Function.call, Array.prototype.concat); var $spliceApply = bind.call(Function.apply, Array.prototype.splice); var $replace = bind.call(Function.call, String.prototype.replace); var $strSlice = bind.call(Function.call, String.prototype.slice); /* adapted from https://github.com/lodash/lodash/blob/4.17.15/dist/lodash.js#L6735-L6744 */ var rePropName = /[^%.[\]]+|\[(?:(-?\d+(?:\.\d+)?)|(["'])((?:(?!\2)[^\\]|\\.)*?)\2)\]|(?=(?:\.|\[\])(?:\.|\[\]|%$))/g; var reEscapeChar = /\\(\\)?/g; /** Used to match backslashes in property paths. */ var stringToPath = function stringToPath(string) { var first = $strSlice(string, 0, 1); var last = $strSlice(string, -1); if (first === '%' && last !== '%') { throw new $SyntaxError('invalid intrinsic syntax, expected closing `%`'); } else if (last === '%' && first !== '%') { throw new $SyntaxError('invalid intrinsic syntax, expected opening `%`'); } var result = []; $replace(string, rePropName, function (match, number, quote, subString) { result[result.length] = quote ? $replace(subString, reEscapeChar, '$1') : number || match; }); return result; }; /* end adaptation */ var getBaseIntrinsic = function getBaseIntrinsic(name, allowMissing) { var intrinsicName = name; var alias; if (hasOwn(LEGACY_ALIASES, intrinsicName)) { alias = LEGACY_ALIASES[intrinsicName]; intrinsicName = '%' + alias[0] + '%'; } if (hasOwn(INTRINSICS, intrinsicName)) { var value = INTRINSICS[intrinsicName]; if (typeof value === 'undefined' && !allowMissing) { throw new $TypeError('intrinsic ' + name + ' exists, but is not available. Please file an issue!'); } return { alias: alias, name: intrinsicName, value: value }; } throw new $SyntaxError('intrinsic ' + name + ' does not exist!'); }; module.exports = function GetIntrinsic(name, allowMissing) { if (typeof name !== 'string' || name.length === 0) { throw new $TypeError('intrinsic name must be a non-empty string'); } if (arguments.length > 1 && typeof allowMissing !== 'boolean') { throw new $TypeError('"allowMissing" argument must be a boolean'); } var parts = stringToPath(name); var intrinsicBaseName = parts.length > 0 ? parts[0] : ''; var intrinsic = getBaseIntrinsic('%' + intrinsicBaseName + '%', allowMissing); var intrinsicRealName = intrinsic.name; var value = intrinsic.value; var skipFurtherCaching = false; var alias = intrinsic.alias; if (alias) { intrinsicBaseName = alias[0]; $spliceApply(parts, $concat([0, 1], alias)); } for (var i = 1, isOwn = true; i < parts.length; i += 1) { var part = parts[i]; var first = $strSlice(part, 0, 1); var last = $strSlice(part, -1); if ( ( (first === '"' || first === "'" || first === '`') || (last === '"' || last === "'" || last === '`') ) && first !== last ) { throw new $SyntaxError('property names with quotes must have matching quotes'); } if (part === 'constructor' || !isOwn) { skipFurtherCaching = true; } intrinsicBaseName += '.' + part; intrinsicRealName = '%' + intrinsicBaseName + '%'; if (hasOwn(INTRINSICS, intrinsicRealName)) { value = INTRINSICS[intrinsicRealName]; } else if (value != null) { if (!(part in value)) { if (!allowMissing) { throw new $TypeError('base intrinsic for ' + name + ' exists, but the property is not available.'); } return void undefined; } if ($gOPD && (i + 1) >= parts.length) { var desc = $gOPD(value, part); isOwn = !!desc; // By convention, when a data property is converted to an accessor // property to emulate a data property that does not suffer from // the override mistake, that accessor's getter is marked with // an `originalValue` property. Here, when we detect this, we // uphold the illusion by pretending to see that original data // property, i.e., returning the value rather than the getter // itself. if (isOwn && 'get' in desc && !('originalValue' in desc.get)) { value = desc.get; } else { value = value[part]; } } else { isOwn = hasOwn(value, part); value = value[part]; } if (isOwn && !skipFurtherCaching) { INTRINSICS[intrinsicRealName] = value; } } } return value; }; apollo-server-demo/node_modules/get-intrinsic/LICENSE 0000644 0001750 0000144 00000002057 03560116604 022220 0 ustar andreh users MIT License Copyright (c) 2020 Jordan Harband Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/get-intrinsic/.eslintrc 0000644 0001750 0000144 00000001147 03560116604 023036 0 ustar andreh users { "root": true, "extends": "@ljharb", "env": { "es6": true, }, "rules": { "array-bracket-newline": 0, "array-element-newline": 0, "complexity": 0, "eqeqeq": [2, "allow-null"], "func-name-matching": 0, "id-length": 0, "max-lines-per-function": [2, 80], "max-params": [2, 4], "max-statements": 0, "max-statements-per-line": [2, { "max": 2 }], "multiline-comment-style": 0, "no-magic-numbers": 0, "operator-linebreak": [2, "before"], "sort-keys": 0, }, "overrides": [ { "files": "test/**", "rules": { "max-lines-per-function": 0, "new-cap": 0, }, }, ], } apollo-server-demo/node_modules/get-intrinsic/.github/ 0000755 0001750 0000144 00000000000 14067647700 022561 5 ustar andreh users apollo-server-demo/node_modules/get-intrinsic/.github/FUNDING.yml 0000644 0001750 0000144 00000001110 03560116604 024355 0 ustar andreh users # These are supported funding model platforms github: [ljharb] patreon: # Replace with a single Patreon username open_collective: # Replace with a single Open Collective username ko_fi: # Replace with a single Ko-fi username tidelift: npm/get-intrinsic community_bridge: # Replace with a single Community Bridge project-name e.g., cloud-foundry liberapay: # Replace with a single Liberapay username issuehunt: # Replace with a single IssueHunt username otechie: # Replace with a single Otechie username custom: # Replace with up to 4 custom sponsorship URLs e.g., ['link1', 'link2'] apollo-server-demo/node_modules/get-intrinsic/.github/require-allow-edits.yml 0000644 0001750 0000144 00000000301 03560116604 027162 0 ustar andreh users name: Require “Allow Edits†on: [pull_request_target] jobs: _: name: "Require “Allow Editsâ€" runs-on: ubuntu-latest steps: - uses: ljharb/require-allow-edits@main apollo-server-demo/node_modules/get-intrinsic/.github/rebase.yml 0000644 0001750 0000144 00000000401 03560116604 024526 0 ustar andreh users name: Automatic Rebase on: [pull_request_target] jobs: _: name: "Automatic Rebase" runs-on: ubuntu-latest steps: - uses: actions/checkout@v2 - uses: ljharb/rebase@master env: GITHUB_TOKEN: ${{ secrets.GITHUB_TOKEN }} apollo-server-demo/node_modules/get-intrinsic/README.md 0000644 0001750 0000144 00000000137 03560116604 022467 0 ustar andreh users # get-intrinsic Get and robustly cache all JS language-level intrinsics at first require time. apollo-server-demo/node_modules/get-intrinsic/package.json 0000644 0001750 0000144 00000004055 03560116604 023501 0 ustar andreh users { "name": "get-intrinsic", "version": "1.0.2", "description": "Get and robustly cache all JS language-level intrinsics at first require time", "main": "index.js", "exports": { ".": [ { "default": "./index.js" }, "./index.js" ] }, "scripts": { "lint": "eslint --ext=.js,.mjs .", "pretest": "npm run lint", "tests-only": "nyc tape 'test/*'", "test": "npm run tests-only", "posttest": "aud --production", "version": "auto-changelog && git add CHANGELOG.md", "postversion": "auto-changelog && git add CHANGELOG.md && git commit --no-edit --amend && git tag -f \"v$(node -e \"console.log(require('./package.json').version)\")\"" }, "repository": { "type": "git", "url": "git+https://github.com/ljharb/get-intrinsic.git" }, "keywords": [ "javascript", "ecmascript", "es", "js", "intrinsic", "getintrinsic", "es-abstract" ], "author": "Jordan Harband <ljharb@gmail.com>", "funding": { "url": "https://github.com/sponsors/ljharb" }, "license": "MIT", "bugs": { "url": "https://github.com/ljharb/get-intrinsic/issues" }, "homepage": "https://github.com/ljharb/get-intrinsic#readme", "devDependencies": { "@ljharb/eslint-config": "^17.3.0", "aud": "^1.1.3", "auto-changelog": "^2.2.1", "es-abstract": "^1.18.0-next.1", "es-value-fixtures": "^1.0.0", "eslint": "^7.15.0", "foreach": "^2.0.5", "has-bigints": "^1.0.1", "make-async-function": "^1.0.0", "make-async-generator-function": "^1.0.0", "make-generator-function": "^2.0.0", "nyc": "^10.3.2", "object-inspect": "^1.9.0", "tape": "^5.0.1" }, "auto-changelog": { "output": "CHANGELOG.md", "template": "keepachangelog", "unreleased": false, "commitLimit": false, "backfillLimit": false, "hideCredit": true }, "dependencies": { "function-bind": "^1.1.1", "has": "^1.0.3", "has-symbols": "^1.0.1" } ,"_resolved": "https://registry.npmjs.org/get-intrinsic/-/get-intrinsic-1.0.2.tgz" ,"_integrity": "sha512-aeX0vrFm21ILl3+JpFFRNe9aUvp6VFZb2/CTbgLb8j75kOhvoNYjt9d8KA/tJG4gSo8nzEDedRl0h7vDmBYRVg==" ,"_from": "get-intrinsic@1.0.2" } apollo-server-demo/node_modules/get-intrinsic/.nycrc 0000644 0001750 0000144 00000000350 03560116604 022324 0 ustar andreh users { "all": true, "check-coverage": false, "reporter": ["text-summary", "text", "html", "json"], "lines": 86, "statements": 85.93, "functions": 82.43, "branches": 76.06, "exclude": [ "coverage", "operations", "test" ] } apollo-server-demo/node_modules/get-intrinsic/test/ 0000755 0001750 0000144 00000000000 14067647700 022200 5 ustar andreh users apollo-server-demo/node_modules/get-intrinsic/test/GetIntrinsic.js 0000644 0001750 0000144 00000017402 03560116604 025132 0 ustar andreh users 'use strict'; var GetIntrinsic = require('../'); var test = require('tape'); var forEach = require('foreach'); var debug = require('object-inspect'); var generatorFns = require('make-generator-function')(); var asyncFns = require('make-async-function').list(); var asyncGenFns = require('make-async-generator-function')(); var callBound = require('es-abstract/helpers/callBound'); var v = require('es-value-fixtures'); var $gOPD = require('es-abstract/helpers/getOwnPropertyDescriptor'); var defineProperty = require('es-abstract/test/helpers/defineProperty'); var $isProto = callBound('%Object.prototype.isPrototypeOf%'); test('export', function (t) { t.equal(typeof GetIntrinsic, 'function', 'it is a function'); t.equal(GetIntrinsic.length, 2, 'function has length of 2'); t.end(); }); test('throws', function (t) { t['throws']( function () { GetIntrinsic('not an intrinsic'); }, SyntaxError, 'nonexistent intrinsic throws a syntax error' ); t['throws']( function () { GetIntrinsic(''); }, TypeError, 'empty string intrinsic throws a type error' ); t['throws']( function () { GetIntrinsic('.'); }, SyntaxError, '"just a dot" intrinsic throws a syntax error' ); t['throws']( function () { GetIntrinsic('%String'); }, SyntaxError, 'Leading % without trailing % throws a syntax error' ); t['throws']( function () { GetIntrinsic('String%'); }, SyntaxError, 'Trailing % without leading % throws a syntax error' ); t['throws']( function () { GetIntrinsic("String['prototype]"); }, SyntaxError, 'Dynamic property access is disallowed for intrinsics (unterminated string)' ); t['throws']( function () { GetIntrinsic('%Proxy.prototype.undefined%'); }, TypeError, "Throws when middle part doesn't exist (%Proxy.prototype.undefined%)" ); forEach(v.nonStrings, function (nonString) { t['throws']( function () { GetIntrinsic(nonString); }, TypeError, debug(nonString) + ' is not a String' ); }); forEach(v.nonBooleans, function (nonBoolean) { t['throws']( function () { GetIntrinsic('%', nonBoolean); }, TypeError, debug(nonBoolean) + ' is not a Boolean' ); }); forEach([ 'toString', 'propertyIsEnumerable', 'hasOwnProperty' ], function (objectProtoMember) { t['throws']( function () { GetIntrinsic(objectProtoMember); }, SyntaxError, debug(objectProtoMember) + ' is not an intrinsic' ); }); t.end(); }); test('base intrinsics', function (t) { t.equal(GetIntrinsic('%Object%'), Object, '%Object% yields Object'); t.equal(GetIntrinsic('Object'), Object, 'Object yields Object'); t.equal(GetIntrinsic('%Array%'), Array, '%Array% yields Array'); t.equal(GetIntrinsic('Array'), Array, 'Array yields Array'); t.end(); }); test('dotted paths', function (t) { t.equal(GetIntrinsic('%Object.prototype.toString%'), Object.prototype.toString, '%Object.prototype.toString% yields Object.prototype.toString'); t.equal(GetIntrinsic('Object.prototype.toString'), Object.prototype.toString, 'Object.prototype.toString yields Object.prototype.toString'); t.equal(GetIntrinsic('%Array.prototype.push%'), Array.prototype.push, '%Array.prototype.push% yields Array.prototype.push'); t.equal(GetIntrinsic('Array.prototype.push'), Array.prototype.push, 'Array.prototype.push yields Array.prototype.push'); test('underscore paths are aliases for dotted paths', { skip: !Object.isFrozen || Object.isFrozen(Object.prototype) }, function (st) { var original = GetIntrinsic('%ObjProto_toString%'); forEach([ '%Object.prototype.toString%', 'Object.prototype.toString', '%ObjectPrototype.toString%', 'ObjectPrototype.toString', '%ObjProto_toString%', 'ObjProto_toString' ], function (name) { defineProperty(Object.prototype, 'toString', { value: function toString() { return original.apply(this, arguments); } }); st.equal(GetIntrinsic(name), original, name + ' yields original Object.prototype.toString'); }); defineProperty(Object.prototype, 'toString', { value: original }); st.end(); }); test('dotted paths cache', { skip: !Object.isFrozen || Object.isFrozen(Object.prototype) }, function (st) { var original = GetIntrinsic('%Object.prototype.propertyIsEnumerable%'); forEach([ '%Object.prototype.propertyIsEnumerable%', 'Object.prototype.propertyIsEnumerable', '%ObjectPrototype.propertyIsEnumerable%', 'ObjectPrototype.propertyIsEnumerable' ], function (name) { // eslint-disable-next-line no-extend-native Object.prototype.propertyIsEnumerable = function propertyIsEnumerable() { return original.apply(this, arguments); }; st.equal(GetIntrinsic(name), original, name + ' yields cached Object.prototype.propertyIsEnumerable'); }); // eslint-disable-next-line no-extend-native Object.prototype.propertyIsEnumerable = original; st.end(); }); test('dotted path reports correct error', function (st) { st['throws'](function () { GetIntrinsic('%NonExistentIntrinsic.prototype.property%'); }, /%NonExistentIntrinsic%/, 'The base intrinsic of %NonExistentIntrinsic.prototype.property% is %NonExistentIntrinsic%'); st['throws'](function () { GetIntrinsic('%NonExistentIntrinsicPrototype.property%'); }, /%NonExistentIntrinsicPrototype%/, 'The base intrinsic of %NonExistentIntrinsicPrototype.property% is %NonExistentIntrinsicPrototype%'); st.end(); }); t.end(); }); test('accessors', { skip: !$gOPD || typeof Map !== 'function' }, function (t) { var actual = $gOPD(Map.prototype, 'size'); t.ok(actual, 'Map.prototype.size has a descriptor'); t.equal(typeof actual.get, 'function', 'Map.prototype.size has a getter function'); t.equal(GetIntrinsic('%Map.prototype.size%'), actual.get, '%Map.prototype.size% yields the getter for it'); t.equal(GetIntrinsic('Map.prototype.size'), actual.get, 'Map.prototype.size yields the getter for it'); t.end(); }); test('generator functions', { skip: !generatorFns.length }, function (t) { var $GeneratorFunction = GetIntrinsic('%GeneratorFunction%'); var $GeneratorFunctionPrototype = GetIntrinsic('%Generator%'); var $GeneratorPrototype = GetIntrinsic('%GeneratorPrototype%'); forEach(generatorFns, function (genFn) { var fnName = genFn.name; fnName = fnName ? "'" + fnName + "'" : 'genFn'; t.ok(genFn instanceof $GeneratorFunction, fnName + ' instanceof %GeneratorFunction%'); t.ok($isProto($GeneratorFunctionPrototype, genFn), '%Generator% is prototype of ' + fnName); t.ok($isProto($GeneratorPrototype, genFn.prototype), '%GeneratorPrototype% is prototype of ' + fnName + '.prototype'); }); t.end(); }); test('async functions', { skip: !asyncFns.length }, function (t) { var $AsyncFunction = GetIntrinsic('%AsyncFunction%'); var $AsyncFunctionPrototype = GetIntrinsic('%AsyncFunctionPrototype%'); forEach(asyncFns, function (asyncFn) { var fnName = asyncFn.name; fnName = fnName ? "'" + fnName + "'" : 'asyncFn'; t.ok(asyncFn instanceof $AsyncFunction, fnName + ' instanceof %AsyncFunction%'); t.ok($isProto($AsyncFunctionPrototype, asyncFn), '%AsyncFunctionPrototype% is prototype of ' + fnName); }); t.end(); }); test('async generator functions', { skip: !asyncGenFns.length }, function (t) { var $AsyncGeneratorFunction = GetIntrinsic('%AsyncGeneratorFunction%'); var $AsyncGeneratorFunctionPrototype = GetIntrinsic('%AsyncGenerator%'); var $AsyncGeneratorPrototype = GetIntrinsic('%AsyncGeneratorPrototype%'); forEach(asyncGenFns, function (asyncGenFn) { var fnName = asyncGenFn.name; fnName = fnName ? "'" + fnName + "'" : 'asyncGenFn'; t.ok(asyncGenFn instanceof $AsyncGeneratorFunction, fnName + ' instanceof %AsyncGeneratorFunction%'); t.ok($isProto($AsyncGeneratorFunctionPrototype, asyncGenFn), '%AsyncGenerator% is prototype of ' + fnName); t.ok($isProto($AsyncGeneratorPrototype, asyncGenFn.prototype), '%AsyncGeneratorPrototype% is prototype of ' + fnName + '.prototype'); }); t.end(); }); apollo-server-demo/node_modules/get-intrinsic/.eslintignore 0000644 0001750 0000144 00000000012 03560116604 023703 0 ustar andreh users coverage/ apollo-server-demo/node_modules/get-intrinsic/CHANGELOG.md 0000644 0001750 0000144 00000203624 03560116604 023027 0 ustar andreh users # Changelog All notable changes to this project will be documented in this file. The format is based on [Keep a Changelog](https://keepachangelog.com/en/1.0.0/) and this project adheres to [Semantic Versioning](https://semver.org/spec/v2.0.0.html). ## [v1.18.0-next.1](https://github.com/ljharb/get-intrinsic/compare/v1.18.0-next.0...v1.18.0-next.1) - 2020-09-30 ### Fixed - [patch] `GetIntrinsic`: Adapt to override-mistake-fix pattern [`#79`](https://github.com/ljharb/object.assign/issues/79) - [Fix] `ES2020`: `ToInteger`: `-0` should always be normalized to `+0` [`#116`](https://github.com/ljharb/get-intrinsic/issues/116) ### Commits - [Tests] ses-compat - initialize module after ses lockdown [`311ff25`](https://github.com/ljharb/get-intrinsic/commit/311ff2536571c76d06e0ea5a48b835dbd8345537) - [Tests] [Refactor] use defineProperty helper rather than assignment [`e957788`](https://github.com/ljharb/get-intrinsic/commit/e957788019cab11e64a7afd5582b22c8a14a5ca7) - [Tests] [Refactor] clean up defineProperty test helper [`4e74e41`](https://github.com/ljharb/get-intrinsic/commit/4e74e4157594d835dfe122239a115f0b84a6b5b9) - [Fix] `callBind`: ensure compatibility with SES [`e3d956a`](https://github.com/ljharb/get-intrinsic/commit/e3d956a221c4f4314928acc71da27533aa5e7c6b) - [Deps] update `is-callable`, `object.assign` [`e094224`](https://github.com/ljharb/get-intrinsic/commit/e0942249ca82c4f9729e5049c5e40ca819804c6e) - [Dev Deps] update `eslint`, `@ljharb/eslint-config` [`7677020`](https://github.com/ljharb/get-intrinsic/commit/7677020ec03e7608e860e318ac896431d18112bf) - [Tests] temporarily allow SES tests to fail [`a2d744b`](https://github.com/ljharb/get-intrinsic/commit/a2d744b26cb9bb7730d21267fe702ee2a11f2008) - [eslint] fix warning [`6547ecc`](https://github.com/ljharb/get-intrinsic/commit/6547eccb1cd566c6149cd038d7ea2bfd76a1f7de) ## [v1.18.0-next.0](https://github.com/ljharb/get-intrinsic/compare/v1.17.7...v1.18.0-next.0) - 2020-09-09 ### Fixed - [Fix] `ES5`+: `ToPropertyDescriptor`: use intrinsic TypeError [`#107`](https://github.com/ljharb/get-intrinsic/issues/107) - [Fix] `ES2018+`: `CopyDataProperties`/`NumberToString`: use intrinsic TypeError [`#107`](https://github.com/ljharb/get-intrinsic/issues/107) ### Commits - [New] add `ES2020` [`67a1a94`](https://github.com/ljharb/get-intrinsic/commit/67a1a94ee9c9b36d7505c0eac0e2b95a2811e5e4) - [New] `ES5`+: add `abs`, `floor`; use `modulo` consistently [`d253fe0`](https://github.com/ljharb/get-intrinsic/commit/d253fe0f78ec0af9636083d6a7f9ebc55ec69412) - [New] `ES2015`+: add `QuoteJSONString`, `OrdinaryCreateFromConstructor` [`4e8d479`](https://github.com/ljharb/get-intrinsic/commit/4e8d4797dd9353ff33bd6b748d8b0ed63d1fbbdb) - [New] `GetIntrinsic`: Cache accessed intrinsics [`5999619`](https://github.com/ljharb/get-intrinsic/commit/599961920d3b977df94527601772e19cee1474fe) - [New] `ES2018`+: add `SetFunctionLength`, `UnicodeEscape` [`343db0e`](https://github.com/ljharb/get-intrinsic/commit/343db0e0f761b99657d4da7f5c1a05cdcb5fcbeb) - [New] `ES2017`+: add `StringGetOwnProperty` [`bcef4b2`](https://github.com/ljharb/get-intrinsic/commit/bcef4b2d03031cd4bbb9d417a03495d5b8c06ab6) - [New] `ES2016`+: add `UTF16Encoding` [`a4340d8`](https://github.com/ljharb/get-intrinsic/commit/a4340d8d145d47928bb6ca1593f9c07d4e6c1204) - [New] `GetIntrinsic`: Add ES201x function intrinsics [`1f8ad9b`](https://github.com/ljharb/get-intrinsic/commit/1f8ad9b85ef15ead22ccb6af78d335e5167c4026) - [New] add `isLeadingSurrogate`/`isTrailingSurrogate` helpers [`7ae6aae`](https://github.com/ljharb/get-intrinsic/commit/7ae6aaeabe308e67a7e6eae94aa0bf51dd72e127) - [Dev Deps] update `eslint` [`7e6ccd7`](https://github.com/ljharb/get-intrinsic/commit/7e6ccd7c78a35fcfdb593cbde7aefd30c94d80be) - [New] `GetIntrinsic`: add `%AggregateError%`, `%FinalizationRegistry%`, and `%WeakRef%` [`249621e`](https://github.com/ljharb/get-intrinsic/commit/249621ed013ed62cb67fd8bfc99fed52b688d64f) - [Dev Deps] update `eslint` [`f63d0a2`](https://github.com/ljharb/get-intrinsic/commit/f63d0a290c1aef446fa277f499dbb6a1942f8608) - [Deps] update `is-regex` [`c2d4586`](https://github.com/ljharb/get-intrinsic/commit/c2d4586472c0385ad800ed8af9862b1f90defbaa) - [Dev Deps] update `eslint` [`3f88447`](https://github.com/ljharb/get-intrinsic/commit/3f884471a5c373a3e69479edb32f64cb9a1e1f60) - [Deps] update `object-inspect` [`bb82b41`](https://github.com/ljharb/get-intrinsic/commit/bb82b415015fa7810425dc2ed973cea9d27d7348) ## [v1.17.7](https://github.com/ljharb/get-intrinsic/compare/v1.17.6...v1.17.7) - 2020-09-30 ### Fixed - [patch] `GetIntrinsic`: Adapt to override-mistake-fix pattern [`#79`](https://github.com/ljharb/object.assign/issues/79) - [Fix] `ES5`+: `ToPropertyDescriptor`: use intrinsic TypeError [`#107`](https://github.com/ljharb/get-intrinsic/issues/107) - [Fix] `ES2018+`: `CopyDataProperties`/`NumberToString`: use intrinsic TypeError [`#107`](https://github.com/ljharb/get-intrinsic/issues/107) ### Commits - [Fix] `callBind`: ensure compatibility with SES [`af46f9f`](https://github.com/ljharb/get-intrinsic/commit/af46f9fd55ec42f776b249150eac40a80f848b21) - [Deps] update `is-callable`, `is-regex`, `object-inspect`, `object.assign` [`864f71d`](https://github.com/ljharb/get-intrinsic/commit/864f71dac9a812171eb1fc2975fcf5b166704f68) - [Dev Deps] update `eslint`, `@ljharb/eslint-config` [`af450a8`](https://github.com/ljharb/get-intrinsic/commit/af450a8f3e82ccf266f85f58ab580f04bd47d300) ## [v1.17.6](https://github.com/ljharb/get-intrinsic/compare/v1.17.5...v1.17.6) - 2020-06-13 ### Commits - [meta] mark spackled files as autogenerated [`286a24b`](https://github.com/ljharb/get-intrinsic/commit/286a24b26aefd6463ebe0a41e43fdd67257851c0) - [Tests] reformat expected missing ops [`8a9cf6a`](https://github.com/ljharb/get-intrinsic/commit/8a9cf6ab4016ac388549b54961f48ad1417cdac4) - [meta] `ES2015`: complete ops list [`c98e703`](https://github.com/ljharb/get-intrinsic/commit/c98e7031b6618b93c633d537bb5541df20b13734) - [Fix] `ES2015+`: `IsConstructor`: when `Reflect.construct` is available, be spec-accurate [`d959e6d`](https://github.com/ljharb/get-intrinsic/commit/d959e6d039b4a186f4e3f13f7968d9a44bce9022) - [Fix] `ES2015+`: `Set`: Always return boolean value [`24c2ac0`](https://github.com/ljharb/get-intrinsic/commit/24c2ac073c13685f67ff68a21d06a57ced6eabe9) - [Fix]: Use `Reflect.apply(…)` if available [`606a752`](https://github.com/ljharb/get-intrinsic/commit/606a752c1d32ff37df59e21f2ed8e465ec20319c) - [Fix] `2016`: Use `getIteratorMethod` in `IterableToArrayLike` [`9464824`](https://github.com/ljharb/get-intrinsic/commit/94648247357afe6f0c5feb8766c3eb05948f46b7) - [Tests] try out CodeQL analysis [`f0c185b`](https://github.com/ljharb/get-intrinsic/commit/f0c185b9145553d16b67eaae1b7af99f98d57981) - [Fix] `ES2015+`: `Set`: ensure exceptions are thrown in IE 9 when requested [`7a963e3`](https://github.com/ljharb/get-intrinsic/commit/7a963e3939da431e994d2181875f95b1e8ab239e) - [Test]: Run tests with `undefined` this [`5322bde`](https://github.com/ljharb/get-intrinsic/commit/5322bdef67de6d179d09900422fe95a14485ba30) - [Fix] `helpers/getSymbolDescription`: use the global Symbol registry when available [`9e1c00d`](https://github.com/ljharb/get-intrinsic/commit/9e1c00dd2eb4f2202661bba69274f9cd9e4b660a) - [Fix] `2018+`: Fix `CopyDataProperties` depending on `this` [`8a05dc9`](https://github.com/ljharb/get-intrinsic/commit/8a05dc9aeba1d1f603d986dc6ac43f81f16d8c52) - [Tests] `helpers/getSymbolDescription`: add test cases [`e468cbe`](https://github.com/ljharb/get-intrinsic/commit/e468cbed7ceb07454d8fd8d86bdfd5c46f18b66f) - [Tests] some envs have `Symbol.for` but can not infer a name [`2ab5e6d`](https://github.com/ljharb/get-intrinsic/commit/2ab5e6d15404999ac4fee8050c653d863c0e04c4) - [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `in-publish`, `object-is`, `tape`; add `aud` [`752669e`](https://github.com/ljharb/get-intrinsic/commit/752669e7a87aa289c98f35a33d0554fdcaaa54b9) - [meta] `Type`: fix spec URL [`965b68b`](https://github.com/ljharb/get-intrinsic/commit/965b68bce27d500ca520ec91e15d9cf4633ff9f7) - [Deps] switch from `string.prototype.trimleft`/`string.prototype.trimright` to `string.prototype.trimstart`/`string.prototype.trimend` [`80dc848`](https://github.com/ljharb/get-intrinsic/commit/80dc8485f1db88d927cc006d0ad9da1daa26e777) - [Deps] update `is‑callable`, `is‑regex` [`e280a27`](https://github.com/ljharb/get-intrinsic/commit/e280a2785f86f33ed730cfa74f2b8a2de86793fe) - [Dev Deps] update `eslint`, `tape` [`5a1188f`](https://github.com/ljharb/get-intrinsic/commit/5a1188fe6bee6e4a57fb9ab4146f4ebc52fc4bbe) - [Fix] `helpers/floor`: module-cache `Math.floor` [`fddd8e6`](https://github.com/ljharb/get-intrinsic/commit/fddd8e6457876e3df1fd41b396a10f14d59a19f4) - [Fix] `helpers/OwnPropertyKeys`: Use `Reflect.ownKeys(…)` if available [`65068e7`](https://github.com/ljharb/get-intrinsic/commit/65068e7f81fda0956f56b6cb8fba115596fccd37) - [Fix] `helpers/getSymbolDescription`: Prefer bound `description` getter when present [`537d8d5`](https://github.com/ljharb/get-intrinsic/commit/537d8d5a1cc3c2a046edf693a984685b09998e44) - [Dev Deps] update `eslint` [`c2440d9`](https://github.com/ljharb/get-intrinsic/commit/c2440d9a4779671dc97009a9a8f10dd569d5e5ea) - [eslint] `helpers/isPropertyDescriptor`: fix indentation [`e438539`](https://github.com/ljharb/get-intrinsic/commit/e43853995f2a13e3b715ce6cd7b263ab6b422d36) ## [v1.17.5](https://github.com/ljharb/get-intrinsic/compare/v1.17.4...v1.17.5) - 2020-03-22 ### Commits - [Fix] `CreateDataProperty`: update an existing property [`bdd77b5`](https://github.com/ljharb/get-intrinsic/commit/bdd77b507eb23bce9d6b3c1961435afd41660f43) - [Dev Deps] update `@ljharb/eslint-config` [`9f1690f`](https://github.com/ljharb/get-intrinsic/commit/9f1690f0e4d4a88e044ccd128de7c838951ac7af) - [Dev Deps] update `make-arrow-function`, `tape` [`920a682`](https://github.com/ljharb/get-intrinsic/commit/920a6827c7d2c7d2b1260f008270734eb3a6fe6c) - [Fix] run missing spackle from cd7504701879ddea0f5981e99cbcf93bfea9171d [`b9069ac`](https://github.com/ljharb/get-intrinsic/commit/b9069ac46060732a0b8bbc36479851ee272cf463) ## [v1.17.4](https://github.com/ljharb/get-intrinsic/compare/v1.17.3...v1.17.4) - 2020-01-21 ### Commits - [Fix] `2015+`: add code to handle IE 8’s problems: [`cd75047`](https://github.com/ljharb/get-intrinsic/commit/cd7504701879ddea0f5981e99cbcf93bfea9171d) - [Tests] fix tests for IE 8 [`c625ee1`](https://github.com/ljharb/get-intrinsic/commit/c625ee169c52e1a0f573ba3015c9bc8497acd366) ## [v1.17.3](https://github.com/ljharb/get-intrinsic/compare/v1.17.2...v1.17.3) - 2020-01-19 ### Commits - [Fix] `ObjectCreate` `2015+`: Fall back to `__proto__` and normal `new` in older browsers [`71772e2`](https://github.com/ljharb/get-intrinsic/commit/71772e252bbe7bbdc2ba6bc819896ba9eeb213a0) - [Fix] `GetIntrinsic`: ensure the `allowMissing` property actually works on dotted intrinsics [`05a2883`](https://github.com/ljharb/get-intrinsic/commit/05a288305f01e6fb51e4e4126fd85c1f496c6691) ## [v1.17.2](https://github.com/ljharb/get-intrinsic/compare/v1.17.1...v1.17.2) - 2020-01-14 ### Commits - [Fix] `helpers/OwnPropertyKeys`: include non-enumerables too [`810b305`](https://github.com/ljharb/get-intrinsic/commit/810b30522b85fb6203194894c5068528c041b602) ## [v1.17.1](https://github.com/ljharb/get-intrinsic/compare/v1.17.0...v1.17.1) - 2020-01-14 ### Commits - [Refactor] add `OwnPropertyKeys` helper, use it in `CopyDataProperties` [`406775c`](https://github.com/ljharb/get-intrinsic/commit/406775c5e155c560e017207b743025577eb89756) - [Refactor] `IteratorClose`: remove useless assignment [`e0e74ce`](https://github.com/ljharb/get-intrinsic/commit/e0e74ce25e4b182f05033f0ca5241c932baf4ba8) - [Dev Deps] update `eslint`, `tape` [`7fcb8ad`](https://github.com/ljharb/get-intrinsic/commit/7fcb8adfa71ee7ae79352b0e09d4b26b2278fd1b) - [Dev Deps] update `diff` [`8645d63`](https://github.com/ljharb/get-intrinsic/commit/8645d635e5ce060900d4d365e05c878d97b0973e) ## [v1.17.0](https://github.com/ljharb/get-intrinsic/compare/v1.17.0-next.1...v1.17.0) - 2019-12-20 ### Commits - [Refactor] `GetIntrinsic`: remove the internal property salts, since % already handles that [`3567ae9`](https://github.com/ljharb/get-intrinsic/commit/3567ae92c6e6041bbbca9fb688b6eb7cd55d824e) - [meta] remove unused Makefile and associated utils [`f0b1083`](https://github.com/ljharb/get-intrinsic/commit/f0b1083130078c988e5704ea6bfaeb7a560a0ff0) - [Refactor] `GetIntrinsic`: further simplification [`9be0385`](https://github.com/ljharb/get-intrinsic/commit/9be038535161ae13a1cb33c2c3f9eda989e65779) - [Fix] `GetIntrinsic`: IE 8 has a broken `Object.getOwnPropertyDescriptor` [`c52fa59`](https://github.com/ljharb/get-intrinsic/commit/c52fa59eb8cccc9f2911f17f0e1d9877290ed6c7) - [Deps] update `is-callable`, `string.prototype.trimleft`, `string.prototype.trimright` [`fb308ec`](https://github.com/ljharb/get-intrinsic/commit/fb308ec1bac3a0abb771df2dbf8019077e3a8edd) - [Dev Deps] update `@ljharb/eslint-config` [`96719b9`](https://github.com/ljharb/get-intrinsic/commit/96719b933e487088cf4a94be05805a7ed779001d) - [Dev Deps] update `tape` [`b84552d`](https://github.com/ljharb/get-intrinsic/commit/b84552d1985eadf604fa91c839254c7ed530fb9b) - [Dev Deps] update `object-is` [`e2df4de`](https://github.com/ljharb/get-intrinsic/commit/e2df4de34c18f3cd544c0b5a8d06ee05f7b7c36a) - [Deps] update `is-regex` [`158ed34`](https://github.com/ljharb/get-intrinsic/commit/158ed3491fe3fb19f54d43a2ece2baf37201aefa) - [Dev Deps] update `object.fromentries` [`84c50fb`](https://github.com/ljharb/get-intrinsic/commit/84c50fb95c736579f5113911d82da45032f04e43) - [Tests] add `.eslintignore` [`0c7f99a`](https://github.com/ljharb/get-intrinsic/commit/0c7f99a0a3d36298654a6808f878c991c13e6f2d) ## [v1.17.0-next.1](https://github.com/ljharb/get-intrinsic/compare/v1.17.0-next.0...v1.17.0-next.1) - 2019-12-11 ### Commits - [Meta] only run spackle script in publish [`4bc91b8`](https://github.com/ljharb/get-intrinsic/commit/4bc91b8b2564afa8a88783a88e2bab143f59bd10) - [Fix] `object.assign` is a runtime dep [`71b8d22`](https://github.com/ljharb/get-intrinsic/commit/71b8d22b2e753181bc8697798be65fc728aa50fa) ## [v1.17.0-next.0](https://github.com/ljharb/get-intrinsic/compare/v1.16.3...v1.17.0-next.0) - 2019-12-11 ### Merged - [New] Split up each operation into its own file [`#77`](https://github.com/ljharb/get-intrinsic/pull/77) ### Commits - [meta] spackle! [`0deb443`](https://github.com/ljharb/get-intrinsic/commit/0deb443a31412962f52174c7f1b333e688669066) - [New] split up each operation into its own file [`990c8be`](https://github.com/ljharb/get-intrinsic/commit/990c8be643203ac20bc6c6fc5f027dacc62834a9) - [meta] add `spackle` script to fill in holes of operations that inherit from previous years [`e5ee0ba`](https://github.com/ljharb/get-intrinsic/commit/e5ee0baf59e41d2bd00ae980f867bf52ec251e38) ## [v1.16.3](https://github.com/ljharb/get-intrinsic/compare/v1.16.2...v1.16.3) - 2019-12-04 ### Commits - [Fix] `GetIntrinsic`: when given a path to a getter, return the actual getter [`0c000ee`](https://github.com/ljharb/get-intrinsic/commit/0c000ee62bedac8c2be38613492f5492088043df) - [Dev Deps] update `eslint` [`f2d1a86`](https://github.com/ljharb/get-intrinsic/commit/f2d1a8654b8be01576f6add586d1cd363885bb79) ## [v1.16.2](https://github.com/ljharb/get-intrinsic/compare/v1.16.1...v1.16.2) - 2019-11-24 ### Commits - [Fix] IE 6-8 strings can’t use array slice, they need string slice [`fa5f0cc`](https://github.com/ljharb/get-intrinsic/commit/fa5f0cc5c9d734c0355bd51f26da76d255f74232) - [Fix] IE 6-7 lack JSON [`e529b4b`](https://github.com/ljharb/get-intrinsic/commit/e529b4bf20be76bff3ff392699692af4b9297884) - [Dev Deps] update `eslint` [`bf52fa4`](https://github.com/ljharb/get-intrinsic/commit/bf52fa48f7d40dae2f53d6d09d00f107d1305d20) ## [v1.16.1](https://github.com/ljharb/get-intrinsic/compare/v1.16.0...v1.16.1) - 2019-11-24 ### Fixed - [meta] re-include year files inside `operations` [`#62`](https://github.com/ljharb/get-intrinsic/issues/62) ### Commits - [Tests] use shared travis-ci config [`17fb792`](https://github.com/ljharb/get-intrinsic/commit/17fb792b654a4fc866f9a59379f5b621462159e9) - [Dev Deps] update `eslint` [`11096ee`](https://github.com/ljharb/get-intrinsic/commit/11096ee9f0179f0eda79d71974190761ddd28e8d) - [Fix] `GetIntrinsics`: turns out IE 8 throws when `Object.getOwnPropertyDescriptor(arguments);`, and does not throw on `callee` anyways [`14e0115`](https://github.com/ljharb/get-intrinsic/commit/14e0115afd53b9d81fc2739c67cee02b7d682121) - [Tests] add Automatic Rebase github action [`37ae5a5`](https://github.com/ljharb/get-intrinsic/commit/37ae5a52a4e3ef6137fe04eb678842bd3d273a19) - [Dev Deps] update `@ljharb/eslint-config` [`dc500f2`](https://github.com/ljharb/get-intrinsic/commit/dc500f2623604a87b8e5cb490e8a369e2d7f720c) - [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `safe-publish-latest` [`51805a6`](https://github.com/ljharb/get-intrinsic/commit/51805a68a83fb21824961c7a35f77921681f39f3) - [Deps] update `es-to-primitive`, `has-symbols`, `object-inspect` [`114c0a8`](https://github.com/ljharb/get-intrinsic/commit/114c0a86a7814f6f9962ffb73d09610bde4409a0) - [meta] add `funding` field [`466f48f`](https://github.com/ljharb/get-intrinsic/commit/466f48fafd9227eb585989e59de359bea864eb0a) - [Tests] disable `check-coverage`, and let codecov do it [`941d75b`](https://github.com/ljharb/get-intrinsic/commit/941d75b08c3b5d061866913d7047f1bed3b97791) - [actions] fix rebase action to use master [`d3a597a`](https://github.com/ljharb/get-intrinsic/commit/d3a597a9fc9927e7e76da1818fcdc334476cc512) - [meta] name the rebase action [`bbc9331`](https://github.com/ljharb/get-intrinsic/commit/bbc93318d834cd5e53494a6248c101a0f08359dd) ## [v1.16.0](https://github.com/ljharb/get-intrinsic/compare/v1.15.0...v1.16.0) - 2019-10-18 ### Commits - [Fix] `GetIterator`: add fallback for pre-Symbol environments, tests [`1891885`](https://github.com/ljharb/get-intrinsic/commit/1891885f8ccead8e212f574cf7c1ef68715e3c72) - [New] `ES2015+`: add `SetFunctionName` [`d171aea`](https://github.com/ljharb/get-intrinsic/commit/d171aea0d90048e787e7cff9f4aaf3fe5248a1d5) - [New] add `getSymbolDescription` and `getInferredName` helpers [`f721f34`](https://github.com/ljharb/get-intrinsic/commit/f721f34e19025767851822c9328ca17270b05578) - [New] `ES2016+`: add `OrdinarySetPrototypeOf` [`0fd1234`](https://github.com/ljharb/get-intrinsic/commit/0fd12343f3e02386c385413fa9c2ff4bd7780e0b) - [New] `ES2015+`: add `CreateListFromArrayLike` [`b11432a`](https://github.com/ljharb/get-intrinsic/commit/b11432aec3852850ae39afafd43c5e36950dcc22) - [New] `ES2015+`: add `GetPrototypeFromConstructor`, with caveats [`f1d05e0`](https://github.com/ljharb/get-intrinsic/commit/f1d05e0f91f7053a925f8f3c634671c65fab37a2) - [New] `ES2016+`: add `OrdinaryGetPrototypeOf` [`1e43409`](https://github.com/ljharb/get-intrinsic/commit/1e4340974db457ded9b5c375aa326b13e038e145) - [Tests] add `node` `v12.2` [`8fc2556`](https://github.com/ljharb/get-intrinsic/commit/8fc25561cbfed1cba3d1db94e573ed6b799578f1) - [Tests] drop statement threshold [`ef4b0df`](https://github.com/ljharb/get-intrinsic/commit/ef4b0dfff3f79f52767973efaae181a74ca5f4be) - [Dev Deps] update `object.fromentries` [`26830be`](https://github.com/ljharb/get-intrinsic/commit/26830bebe02a2a06d8965ee7b206575bfd4709dc) ## [v1.15.0](https://github.com/ljharb/get-intrinsic/compare/v1.14.2...v1.15.0) - 2019-10-02 ### Commits - [New] `ES5`+: add `msFromTime`, `SecFromTime`, `MinFromTime`, `HourFromTime`, `TimeWithinDay`, `Day`, `DayFromYear`, `TimeFromYear`, `YearFromTime`, `WeekDay`, `DaysInYear`, `InLeapYear`, `DayWithinYear`, `MonthFromTime`, `DateFromTime`, `MakeDay`, `MakeDate`, `MakeTime`, `TimeClip`, `modulo` [`2722e96`](https://github.com/ljharb/get-intrinsic/commit/2722e968af42af259fb4c4cec408ce1b33e6de66) - [New] add ES2020’s intrinsic dot notation [`0be1213`](https://github.com/ljharb/get-intrinsic/commit/0be1213284bede338f60bf96a9621d6eab4883eb) - [New] add `callBound` helper [`4ea63aa`](https://github.com/ljharb/get-intrinsic/commit/4ea63aab6d6b9f8b2e50aca47c5d4b4b9dacd4ef) - [New] `ES2018`+: add `DateString`, `TimeString` [`9fdeaf5`](https://github.com/ljharb/get-intrinsic/commit/9fdeaf558bafd42d1521ce9de8db198d887cf3fc) - [meta] npmignore operations scripts; add "deltas" [`a71d377`](https://github.com/ljharb/get-intrinsic/commit/a71d377045d5026875bae4f9cc06f770b59c5181) - [New] add `isPrefixOf` helper [`8230a5e`](https://github.com/ljharb/get-intrinsic/commit/8230a5e0f268bc9548c616c96e63614f07c997b0) - [New] `ES2015`+: add `ToDateString` [`b215d86`](https://github.com/ljharb/get-intrinsic/commit/b215d8600ebbc1b26e86ff821545dda8e03fab46) - [New] add `regexTester` helper [`bf462c6`](https://github.com/ljharb/get-intrinsic/commit/bf462c63ce0dcc902c267379cc3480a913c0dc67) - [New] add `maxSafeInteger` helper [`c15a612`](https://github.com/ljharb/get-intrinsic/commit/c15a612b86d56d1794d6ef84b93d9e7721b1096b) - [Tests] on `node` `v12.11` [`9538b51`](https://github.com/ljharb/get-intrinsic/commit/9538b51cc1ccaf1c517df48e29fd8fa8f50b238d) - [Deps] update `string.prototype.trimleft`, `string.prototype.trimright` [`ba00f56`](https://github.com/ljharb/get-intrinsic/commit/ba00f56d4eb0ed6d7bcbc2b483cf273c686d249b) - [Dev Deps] update `eslint` [`d7ea1b8`](https://github.com/ljharb/get-intrinsic/commit/d7ea1b8fc75e1a552692552778e388d300d275ff) ## [v1.14.2](https://github.com/ljharb/get-intrinsic/compare/v1.14.1...v1.14.2) - 2019-09-08 ### Commits - [Fix] `ES2016`: `IterableToArrayLike`: add proper fallback for strings, pre-Symbols [`a6b5b30`](https://github.com/ljharb/get-intrinsic/commit/a6b5b30f322be791c9b979a98875212491a8581a) - [Tests] on `node` `v12.10` [`ce0f82b`](https://github.com/ljharb/get-intrinsic/commit/ce0f82b2b81588f2f5e9bd2efc812b7625667315) ## [v1.14.1](https://github.com/ljharb/get-intrinsic/compare/v1.14.0...v1.14.1) - 2019-09-03 ## [v1.14.0](https://github.com/ljharb/get-intrinsic/compare/v1.13.0...v1.14.0) - 2019-09-02 ### Commits - [New] add ES2019 [`3bacba8`](https://github.com/ljharb/get-intrinsic/commit/3bacba857f9096cba328dbddd2879546495afc72) - [New] `ES2015+`: add `ValidateAndApplyPropertyDescriptor` [`338bc63`](https://github.com/ljharb/get-intrinsic/commit/338bc63bfadd683970931ab1cef8e36024487391) - [New] `ES2015+`: add `GetSubstitution` [`f350165`](https://github.com/ljharb/get-intrinsic/commit/f35016589a223f7e74182c5ab33e3398420a0f9a) - [New] ES5+: add `Abstract Equality Comparison`, `Strict Equality Comparison` [`bb0aaaf`](https://github.com/ljharb/get-intrinsic/commit/bb0aaafd0c30b9b1dabc6e6d299cba75a79de077) - [Tests] fix linting to apply to all files [`dda7421`](https://github.com/ljharb/get-intrinsic/commit/dda742178ebce166e4f1ada7684ac045032fcd42) - [New] ES5+: add `Abstract Relational Comparison` [`96eb298`](https://github.com/ljharb/get-intrinsic/commit/96eb298be0c8c41d8ed922bda2b3762ce3cb708d) - [Tests] add some missing ES2015 ops [`1efe5de`](https://github.com/ljharb/get-intrinsic/commit/1efe5de0f98e6912c25baa006eaa0cce7a9eb543) - [Dev Deps] update `eslint`, `@ljharb/eslint-config` [`138143e`](https://github.com/ljharb/get-intrinsic/commit/138143e0f724720ac7316bacb161b4d32c0a0fdc) - [New] `ES2015+`: add `OrdinaryGetOwnProperty` [`0609672`](https://github.com/ljharb/get-intrinsic/commit/0609672ca9b48ea387013a4c3ea4a975d8e774cd) - [New] add `callBind` helper, and use it [`9518775`](https://github.com/ljharb/get-intrinsic/commit/9518775eba69c2645daaabae44e8249b3d73f0a6) - [New] `ES2015+`: add `ArraySetLength` [`799302e`](https://github.com/ljharb/get-intrinsic/commit/799302e3cf662c614fe3b434d6581fa17f5f5eba) - [Tests] use the values helper more in es5 tests [`1a6337f`](https://github.com/ljharb/get-intrinsic/commit/1a6337f7689a8657d65d1acf365532c973edeab2) - [Tests] migrate es5 tests to use values helper [`95cadbb`](https://github.com/ljharb/get-intrinsic/commit/95cadbbdddbf66af637ffec9d138473eeb237352) - [New] `ES2016`: add `IterableToArrayLike` [`06b9be9`](https://github.com/ljharb/get-intrinsic/commit/06b9be96b132ef9b8b085c772d0215866917b73a) - [New] ES2015+: add `TestIntegrityLevel` [`e0cd84d`](https://github.com/ljharb/get-intrinsic/commit/e0cd84dc8f5e3e5605962851575e6a897e9b4a93) - [New] ES2015+: add `SetIntegrityLevel` [`658bd05`](https://github.com/ljharb/get-intrinsic/commit/658bd05a385fd4552a41f7726518e035959c0481) - [New] `ES2015+`: add `GetOwnPropertyKeys` [`6e57098`](https://github.com/ljharb/get-intrinsic/commit/6e570987a3c70b03574293b98479d733821a3092) - [Fix] `ES2015+`: `FromPropertyDescriptor`: no longer requires a fully complete Property Descriptor [`bac1b26`](https://github.com/ljharb/get-intrinsic/commit/bac1b26bd303d9ec0de0bde7ef200bbfaf99492a) - [New] `ES2015+`: add `ArrayCreate` [`ccb47e4`](https://github.com/ljharb/get-intrinsic/commit/ccb47e4938b1aca4e766e2915969a8256f3bd51f) - [Fix] `ES2015+`: `CreateDataProperty`, `DefinePropertyOrThrow`, `ValidateAndApplyPropertyDescriptor`: add fallbacks for ES3 [`c538dd8`](https://github.com/ljharb/get-intrinsic/commit/c538dd87442bde76cdd1a50be8a30ae52cf9cd9a) - [meta] change http URLs to https [`d8b1e87`](https://github.com/ljharb/get-intrinsic/commit/d8b1e874e5606fe15904e11ee1bae047df40d97f) - [New] `ES2015+`: add `InstanceofOperator` [`6a431b9`](https://github.com/ljharb/get-intrinsic/commit/6a431b90b9807d644a509374a272ec8ab5abf8b0) - [New] `ES2015+`: add `OrdinaryDefineOwnProperty` [`f5ae698`](https://github.com/ljharb/get-intrinsic/commit/f5ae698f3d1148233b91e60995ac78666cf0912d) - [New] `ES2017+`: add `IterableToList` [`2a99268`](https://github.com/ljharb/get-intrinsic/commit/2a992680b552fb54532da6a976c1921baab83a0b) - [New] `ES2015+`: add `CreateHTML` [`06750b2`](https://github.com/ljharb/get-intrinsic/commit/06750b2bc1141129b9c9fdb929bc5c989e1266cc) - [Tests] add v.descriptors helpers [`f229347`](https://github.com/ljharb/get-intrinsic/commit/f2293479002f02ca946c7ca33f1defcc1a149fcc) - [New] add `isPropertyDescriptor` helper [`c801cef`](https://github.com/ljharb/get-intrinsic/commit/c801cef62c7c60a80771199115ed2d84615e11cf) - [New] ES2015+: add `OrdinaryHasInstance` [`ea69a84`](https://github.com/ljharb/get-intrinsic/commit/ea69a84ca8c4a6a2702a708caf01d8c262a350ab) - [New] `ES2015+`: add `OrdinaryHasProperty` [`979fd9e`](https://github.com/ljharb/get-intrinsic/commit/979fd9eda18442c9b8adbf9c434331a8fde0eb4a) - [New] `ES2015+`: add `SymbolDescriptiveString` [`2bcde98`](https://github.com/ljharb/get-intrinsic/commit/2bcde98310b680146c76b58aaae799c73a4198b6) - [New] ES2015+: add `IsPromise` [`cbdd387`](https://github.com/ljharb/get-intrinsic/commit/cbdd3872f81ed490c104cdbfb8dd16aa4dc3d285) - [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `safe-publish-latest`, `semver`, `replace` [`ce4d3c4`](https://github.com/ljharb/get-intrinsic/commit/ce4d3c42c5fb2408156c3dc768162f3561e95413) - [Tests] some intrinsic cleanup [`6f0f437`](https://github.com/ljharb/get-intrinsic/commit/6f0f437394f1e234c542150e06df8dae8af5b4b9) - [Tests] up to `node` `v12.4`, `v11.15`, `v6.17` [`48e2dbb`](https://github.com/ljharb/get-intrinsic/commit/48e2dbbe12f6b5b4cb9b93fbfd822ef1b0087301) - [Fix] `ES2015+`: `ValidateAndApplyPropertyDescriptor`: use ES2017 logic to bypass spec bugs [`3ca93d3`](https://github.com/ljharb/get-intrinsic/commit/3ca93d313cd9d0744295ce961d3e177b183d4411) - [Tests] up to `node` `v12.6`, `v10.16`, `v8.16` [`fe18201`](https://github.com/ljharb/get-intrinsic/commit/fe182015152bb2fe83662659f9111c886d81624c) - add FUNDING.yml [`16ffa72`](https://github.com/ljharb/get-intrinsic/commit/16ffa725ebe0a64611550e10ccd7b4155806b718) - [Fix] `ES5`: `IsPropertyDescriptor`: call into `IsDataDescriptor` and `IsAccessorDescriptor` [`0af0e31`](https://github.com/ljharb/get-intrinsic/commit/0af0e31329b123a29307cb6e52059ebc82ab87a7) - [New] add `every` helper [`1fd013c`](https://github.com/ljharb/get-intrinsic/commit/1fd013c1a94f16a2323c603277c60b446371228c) - [Tests] use `npx aud` instead of `npm audit` with hoops [`6a5a357`](https://github.com/ljharb/get-intrinsic/commit/6a5a357eae7461c02f666eb45d77b8193d31d11d) - [Tests] up to `node` `v12.9` [`7eb3080`](https://github.com/ljharb/get-intrinsic/commit/7eb3080e2568eb9c30d68977d7691bb60bf00e63) - [Dev Deps] update `cheerio`, `eslint`, `semver`, `tape` [`8028280`](https://github.com/ljharb/get-intrinsic/commit/802828064b8340c2777fd288ed9b2d54757473ef) - [Fix] `ES2015+`: `GetIterator`: only require native Symbols when `method` is omitted [`35c96a5`](https://github.com/ljharb/get-intrinsic/commit/35c96a52a4af5da6a1d1caabd302eb8dd44f47ae) - readme: add security note [`2f59799`](https://github.com/ljharb/get-intrinsic/commit/2f59799f988985e8f88f359ebc7b3f5db1a9d8c3) - [Dev Deps] update `eslint`, `replace`, `tape` [`10875c9`](https://github.com/ljharb/get-intrinsic/commit/10875c9360ac7546aa8c20cc2319eff4f847b9f9) - [Fix] `ES2015`: `Call`: error message now properly displays Symbols using `object-inspect` [`14b298a`](https://github.com/ljharb/get-intrinsic/commit/14b298a82ac3603e2c098e17107c07b4bdddc299) - [Refactor] use `has-symbols` for Symbol detection [`35c6730`](https://github.com/ljharb/get-intrinsic/commit/35c673011f132cd02f450bd701e22b4846f3e68a) - [Tests] use `eclint` instead of `editorconfig-tools` [`bffa735`](https://github.com/ljharb/get-intrinsic/commit/bffa7355ea17e191bd5097d52177848c082a87da) - [Tests] run `npx aud` only on prod deps [`ba56593`](https://github.com/ljharb/get-intrinsic/commit/ba56593083cbc75fc9428a4b468cfbd264751379) - [Dev Deps] update `eslint` [`1a42780`](https://github.com/ljharb/get-intrinsic/commit/1a427806791e3ac11622661bb6bc0b381ae652d3) - [meta] linter cleanup [`4ac4f62`](https://github.com/ljharb/get-intrinsic/commit/4ac4f62f3ecb9c4de47f12cc9a8626eaac23110a) - [Dev deps] update `semver` [`2bb88e9`](https://github.com/ljharb/get-intrinsic/commit/2bb88e9a62117e1cc54f87e22292a58812585ba3) - [meta] fix getOps script [`af6f7d2`](https://github.com/ljharb/get-intrinsic/commit/af6f7d2693a85c3b042a21574730357e3ccb6a2f) - [meta] fix FUNDING.yml [`a5e6289`](https://github.com/ljharb/get-intrinsic/commit/a5e6289cef30ab21f1ba27653750b26daf731ff4) - [meta] add github sponsorship [`13ff759`](https://github.com/ljharb/get-intrinsic/commit/13ff75943f5a59df6b06aff60c179af632baeb6e) - [Deps] update `object-keys` [`195d439`](https://github.com/ljharb/get-intrinsic/commit/195d439a49ef9517c3ca1863a36ba471efac660f) - [meta] fix getOps script [`b6d6434`](https://github.com/ljharb/get-intrinsic/commit/b6d643444471d6bc06d975ad65f2f65dcf2fda2a) - [Deps] update `object-keys` [`6af6a10`](https://github.com/ljharb/get-intrinsic/commit/6af6a102446c420d7f8b2fd7b2e950440037391f) - [Tests] temporarily allow node 0.6 to fail; segfaulting in travis [`d454a7a`](https://github.com/ljharb/get-intrinsic/commit/d454a7a38c55cee707dba5dd224c17b7a789d965) - [Fix] `helpers/assertRecord`: remove `console.log` [`470a7ce`](https://github.com/ljharb/get-intrinsic/commit/470a7ce79b9c322db901cc0ac56fed93d8bfc4fd) ## [v1.13.0](https://github.com/ljharb/get-intrinsic/compare/v1.12.0...v1.13.0) - 2019-01-02 ### Commits - [Tests] add `getOps` to programmatically fetch abstract operation names [`586a35e`](https://github.com/ljharb/get-intrinsic/commit/586a35ed559db3571fb7525d4dfcb19d9a689ecd) - [New] add ES2018 [`e7fd676`](https://github.com/ljharb/get-intrinsic/commit/e7fd6763ff7d45918b9ad8befcb794ee70d3bf49) - [Tests] remove `jscs` [`2f7ce40`](https://github.com/ljharb/get-intrinsic/commit/2f7ce401b117118df4c0f00bdf41db5d549da4d2) - [New] add ES2015/ES2016: EnumerableOwnNames; ES2017: EnumerableOwnProperties; ES2018: EnumerableOwnPropertyNames [`a8153d3`](https://github.com/ljharb/get-intrinsic/commit/a8153d3db64a02a021a9d385f0fc7e8b4b677bef) - [New] add `assertRecord` helper [`3a2826d`](https://github.com/ljharb/get-intrinsic/commit/3a2826d6a927966178f4c5de5cedccae7c2ea350) - [Tests] move descriptor factories to `values` helper [`7dcee9b`](https://github.com/ljharb/get-intrinsic/commit/7dcee9b927eebc3d8ecb13f18b2eed08c3e60d4a) - [New] ES2015+: add `thisBooleanValue`, `thisNumberValue`, `thisStringValue`, `thisTimeValue` [`aea0e44`](https://github.com/ljharb/get-intrinsic/commit/aea0e44e4926438087f0a526f9dac783460544ea) - [New] `ES2015+`: add `DefinePropertyOrThrow` [`be3cf5d`](https://github.com/ljharb/get-intrinsic/commit/be3cf5def49058ea8db5f7f303a366e2513a4d70) - [New] `ES2015+`: add `DeletePropertyOrThrow` [`5cf4887`](https://github.com/ljharb/get-intrinsic/commit/5cf488703cbf9240f319dc158230734950d56de5) - [Fix] add tests and a fix for `CreateMethodProperty` [`8f9c068`](https://github.com/ljharb/get-intrinsic/commit/8f9c068fcf9455f7b70351284c66fc7a0b2fffdf) - [Tests] up to `node` `v11.6`, `v10.15`, `v8.15`, `v6.16` [`e6fb553`](https://github.com/ljharb/get-intrinsic/commit/e6fb55301078fabb711f9e99649f9a83b6bbece1) - [New] `ES2015`+: Add `CreateMethodProperty` [`5e8d6ca`](https://github.com/ljharb/get-intrinsic/commit/5e8d6ca228af1888790a27fe62ae9bcd2404ef97) - [Tests] ensure missing ops list is correct [`c12262d`](https://github.com/ljharb/get-intrinsic/commit/c12262d0d3c6ae54c2f9feffbdef198b1d385136) - [Tests] up to `node` `v11.0`, `v10.12`, `v8.12` [`8f91211`](https://github.com/ljharb/get-intrinsic/commit/8f91211bc097d78af4b980fa1454c46155c855de) - [Tests] remove unneeded jscs overrides [`bede79e`](https://github.com/ljharb/get-intrinsic/commit/bede79e06f6ccdcb34fad93bff51092c242a5c27) - [Tests] up to `node` `v10.7` [`3218b61`](https://github.com/ljharb/get-intrinsic/commit/3218b61e3709a7c7ee862686530e0eb999d6fcae) - [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `tape` [`5944e17`](https://github.com/ljharb/get-intrinsic/commit/5944e175e0ec0b2a895eaad782e55079e0d64385) - [patch] ES2018: remove unreleased `IsPropertyDescriptor` [`06dbc11`](https://github.com/ljharb/get-intrinsic/commit/06dbc117b9ab83db792a1128ef856625ddf89b0b) - [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `replace`, `tape` [`a093b0d`](https://github.com/ljharb/get-intrinsic/commit/a093b0de254b631c69a589a9285da4d9560e6917) - [Tests] use `npm audit` instead of `nsp` [`d082818`](https://github.com/ljharb/get-intrinsic/commit/d082818dba85a93d2be3617458dc9a5a7f4ce6a5) - [Dev Deps] update `eslint`, `safe-publish-latest`, `semver` [`9d6a36a`](https://github.com/ljharb/get-intrinsic/commit/9d6a36ad187d5c64567133f38e3e974d6bdbcf5e) - [Deps] update `is-callable`, `has`, `object-keys` [`4695a34`](https://github.com/ljharb/get-intrinsic/commit/4695a34d87b793d28c46b9e5d680f5b873d479cc) - [Dev Deps] update `semver`, `eslint` [`25944c5`](https://github.com/ljharb/get-intrinsic/commit/25944c5061cc5bcf790cfca6085fadeab546ac7a) - [Deps] update `es-to-primitive` [`80bfd94`](https://github.com/ljharb/get-intrinsic/commit/80bfd946398a15f1722b25f1cf3d0d1a67145e72) - [Dev Deps] update `eslint` [`bcb7dad`](https://github.com/ljharb/get-intrinsic/commit/bcb7dad289fa35c65f6c1a9ad11d59a33508bfd0) - [Fix] remove duplicate abstract operation [`f42ce4c`](https://github.com/ljharb/get-intrinsic/commit/f42ce4c14a4be83e00d96eb1a57264338fcd9011) ## [v1.12.0](https://github.com/ljharb/get-intrinsic/compare/v1.11.0...v1.12.0) - 2018-05-31 ### Commits - [Docs] convert URLs to https [`ca86456`](https://github.com/ljharb/get-intrinsic/commit/ca864563ca263ee98457c510e90b4fc6f66a9014) - [Dev Deps] update `eslint`, `nsp`, `object.assign`, `semver`, `tape` [`5eb3c9a`](https://github.com/ljharb/get-intrinsic/commit/5eb3c9a9cf7be687c90f0778f065028aa76d44a9) - [New] add `GetIntrinsic` entry point [`10c9f99`](https://github.com/ljharb/get-intrinsic/commit/10c9f991b9be944f3263081c85fae397a934348b) - Use GetIntrinsic [`cad40fa`](https://github.com/ljharb/get-intrinsic/commit/cad40fa7d03e9645726074bb497151929f415f7a) - Reverting bad changes from 5eb3c9a9cf7be687c90f0778f065028aa76d44a9 [`c4657a5`](https://github.com/ljharb/get-intrinsic/commit/c4657a5d8751e4aeb2b2e74bac4b645c3fab8d38) - [New] `ES2015`+: add `AdvanceStringIndex` [`4041660`](https://github.com/ljharb/get-intrinsic/commit/40416600db0778beda32f3afa8029bfb8f059a11) - [New] `ES2015`+: add `ObjectCreate` [`e976362`](https://github.com/ljharb/get-intrinsic/commit/e976362f74ff283347cb5a766d2fd7b26e5a1001) - [Tests] up to `node` `v10.0`, `v9.11`, `v8.11`, `v6.14`, `v4.9`; use `nvm install-latest-npm` [`20aae84`](https://github.com/ljharb/get-intrinsic/commit/20aae842fc8ec6218f9eb9e3419e0f6f682300f3) - [Robustness]: `ES2015+`: ensure `Math.{abs,floor}` and `Function.call` are cached. [`2a1bc18`](https://github.com/ljharb/get-intrinsic/commit/2a1bc189d905cab3663e79be8acf484423dbd7e2) - [Tests] add missing `NormalCompletion` abstract op [`5a263ed`](https://github.com/ljharb/get-intrinsic/commit/5a263ed8c4c51af246d4b7fd6957faba08bd4cae) - [Tests] `GetIntrinsic`: increase coverage [`089eafd`](https://github.com/ljharb/get-intrinsic/commit/089eafd078226f2ce75cd397d9de2c71b8a2b6fe) - [Robustness] `helpers/assign`: call-bind "has" [`8f5fae0`](https://github.com/ljharb/get-intrinsic/commit/8f5fae08422ce2de9177dcc65da20e5c38af8374) - [Tests] fix the tests on node 10+, where match objects have "groups" [`1084499`](https://github.com/ljharb/get-intrinsic/commit/1084499ff0f7a2f91e77196277b1b55e548ae823) - [Tests] improve error message for missing ops [`8c3c532`](https://github.com/ljharb/get-intrinsic/commit/8c3c532b96b0efe25a704c7e10252380d50a0b5d) - [Dev Deps] update `replace` [`7fd0054`](https://github.com/ljharb/get-intrinsic/commit/7fd00541c871304c7860fbf368c4dc3ca1569d1f) - [Tests] fix coverage thresholds [`5bcd3a0`](https://github.com/ljharb/get-intrinsic/commit/5bcd3a07ba48d8ee55b38647bc97af9abdc5ec9d) - [Tests] add travis cache [`55a58b5`](https://github.com/ljharb/get-intrinsic/commit/55a58b5bd6bc6c3f696b143c25628e5b75b20d3d) - [Dev Deps] update `eslint`; ignore `nyc` on greenkeeper since v11+ drops support for older nodes [`f0506b5`](https://github.com/ljharb/get-intrinsic/commit/f0506b5d526485635a973a185830b942e566e65f) - [Tests] `ES2016`+: add `OrdinarySet` [`f2fa168`](https://github.com/ljharb/get-intrinsic/commit/f2fa16820a341b6684d3f09b43c7b888a3840246) - [Tests] lowering coverage thresholds for individual runs [`7956878`](https://github.com/ljharb/get-intrinsic/commit/7956878619663d1d9fed16d12b8d789c10326375) - [Tests] `ES2017`: add `IsSharedArrayBuffer` to list [`56b462e`](https://github.com/ljharb/get-intrinsic/commit/56b462e71682610ecdfa63ad4a06c7935482cc5e) - [Tests] lowering coverage thresholds for individual runs [`929e5d1`](https://github.com/ljharb/get-intrinsic/commit/929e5d15982cd58677ffa20b7f386ea9db0358c3) - [Tests] on `node` `v10.2` [`1f80100`](https://github.com/ljharb/get-intrinsic/commit/1f80100333d42fde0836ef44f5411612937d8a57) - [Tests] on `node` `v10.1` [`9ee6ffa`](https://github.com/ljharb/get-intrinsic/commit/9ee6ffabf99126420dc5326dfc0a1dc18da83359) - [Tests] use `object-inspect` instead of `util.format` for debug info [`c0cce8e`](https://github.com/ljharb/get-intrinsic/commit/c0cce8e41980f33bbe60926e3e7b1124146afca9) - [Tests] make `node` `v0.6` required [`8eaf4cd`](https://github.com/ljharb/get-intrinsic/commit/8eaf4cd5b73dae50cbfbe4387e05122a661994da) - [Tests] fix tests to preserve "groups" property [`f885332`](https://github.com/ljharb/get-intrinsic/commit/f8853324a630b58cd6f36311a52b179fdd712a29) ## [v1.11.0](https://github.com/ljharb/get-intrinsic/compare/v1.10.0...v1.11.0) - 2018-03-21 ### Commits - [New] `ES2015+`: add iterator abstract ops: [`2588b6b`](https://github.com/ljharb/get-intrinsic/commit/2588b6b416c805cf8ce2a2919ec767fdc5e353b3) - [Tests] up to `node` `v9.8`, `v8.10`, `v6.13` [`225d552`](https://github.com/ljharb/get-intrinsic/commit/225d552af24840520c7176123fa619c39903ccec) - [Dev Deps] update `eslint`, `nsp`, `object.assign`, `semver`, `tape` [`7f6db81`](https://github.com/ljharb/get-intrinsic/commit/7f6db81a26d8f54f0bb4f3e5ce820a82359b750d) ## [v1.10.0](https://github.com/ljharb/get-intrinsic/compare/v1.9.0...v1.10.0) - 2017-11-24 ### Commits - [New] ES2015+: `AdvanceStringIndex` [`5aa27f0`](https://github.com/ljharb/get-intrinsic/commit/5aa27f0e169ffaf7d22604de20a28759f51c9b68) - [Tests] up to `node` `v9`, `v8.9`; use `nvm install-latest-npm`; pin included builds to LTS [`717aea6`](https://github.com/ljharb/get-intrinsic/commit/717aea68e2c88ad35fc8284b53c291d9a2c3551e) - [Tests] up to `node` `v9.2`, `v6.12` [`052918d`](https://github.com/ljharb/get-intrinsic/commit/052918de7cd285b0078cab733ed0cfe6c33df4c4) - [Dev Deps] update `eslint`, `nsp` [`d1887db`](https://github.com/ljharb/get-intrinsic/commit/d1887dbbde52b35749dc1f3012565705ab72db2a) - [Tests] require node 0.6 to pass again [`b76fb1d`](https://github.com/ljharb/get-intrinsic/commit/b76fb1db56ed7797f79b438db6b3b222b602890a) - [Dev Deps] update `eslint` [`be164d3`](https://github.com/ljharb/get-intrinsic/commit/be164d33866ca53c10399e524cfab6bd46d47cb3) ## [v1.9.0](https://github.com/ljharb/get-intrinsic/compare/v1.8.2...v1.9.0) - 2017-09-30 ### Commits - [Tests] consolidate duplicated tests. [`f2baca3`](https://github.com/ljharb/get-intrinsic/commit/f2baca3fb29d896aac6db57b66ef6b3808e96a8d) - [New] add `ArraySpeciesCreate` [`8256b1b`](https://github.com/ljharb/get-intrinsic/commit/8256b1b5c62d950fb35eba92e07a90947f5bf7a1) - [Tests] increase coverage [`d585ee3`](https://github.com/ljharb/get-intrinsic/commit/d585ee3e841a1322a323ae1c42e39c7c6b189d8d) - [New] ES2015+: add `CreateDataProperty` and `CreateDataPropertyOrThrow` [`1003754`](https://github.com/ljharb/get-intrinsic/commit/10037548bea58cb3f53c4dd8313ce67bbea006af) - [Fix] ES6+ ArraySpeciesCreate: properly handle non-array `originalArray`s. [`5dd1065`](https://github.com/ljharb/get-intrinsic/commit/5dd10658f783aac051a19147f62760a4985e7c76) - [Dev Deps] update `nsp`, `eslint` [`9382bfa`](https://github.com/ljharb/get-intrinsic/commit/9382bfaf78fdd03e6795121c3352df89aecff331) ## [v1.8.2](https://github.com/ljharb/get-intrinsic/compare/v1.8.1...v1.8.2) - 2017-09-03 ### Fixed - [Fix] `es2015`+: `ToNumber`: provide the proper hint for Date objects. [`#27`](https://github.com/ljharb/get-intrinsic/issues/27) ### Commits - [Dev Deps] update `eslint` [`cf4e870`](https://github.com/ljharb/get-intrinsic/commit/cf4e87065642b7d58871bdc85b4e6503c77cacc3) ## [v1.8.1](https://github.com/ljharb/get-intrinsic/compare/v1.8.0...v1.8.1) - 2017-08-30 ### Fixed - [Fix] ES2015+: `ToPropertyKey`: should return a symbol for Symbols. [`#26`](https://github.com/ljharb/get-intrinsic/issues/26) ### Commits - [Dev Deps] update `eslint`, `@ljharb/eslint-config` [`9ae67a5`](https://github.com/ljharb/get-intrinsic/commit/9ae67a506d1482245177a961f06d88a97525f419) - [Docs] github broke markdown parsing [`80a7af5`](https://github.com/ljharb/get-intrinsic/commit/80a7af5a1681280043bdfcda175b6895e8346666) - [Deps] update `function-bind` [`1588dab`](https://github.com/ljharb/get-intrinsic/commit/1588dab2522d78ab9673448c998cac59f94cda15) ## [v1.8.0](https://github.com/ljharb/get-intrinsic/compare/v1.7.0...v1.8.0) - 2017-08-04 ### Commits - [New] move es6+ to es2015+; leave es6/es7 as aliases. [`99d9096`](https://github.com/ljharb/get-intrinsic/commit/99d9096f971192ad2b5b5a81a6eefd7ef1630415) - [New] ES2015+: add `CompletePropertyDescriptor`, `Set`, `HasOwnProperty`, `HasProperty`, `IsConcatSpreadable`, `Invoke`, `CreateIterResultObject`, `RegExpExec` [`d53852e`](https://github.com/ljharb/get-intrinsic/commit/d53852e45fab6690ca1f42164dcc48b4208a09d3) - [New] ES5+: add `IsPropertyDescriptor`, `IsAccessorDescriptor`, `IsDataDescriptor`, `IsGenericDescriptor`, `FromPropertyDescriptor`, `ToPropertyDescriptor` [`caa62da`](https://github.com/ljharb/get-intrinsic/commit/caa62dabfa9794584575160fdabf098b04c5589b) - [New] add ES2017 [`ade044d`](https://github.com/ljharb/get-intrinsic/commit/ade044dd59e997e0ef138b990d5cb853409705cf) - [Tests] use same lists of value types across tests; ensure tests are the same when ops are the same. [`047f761`](https://github.com/ljharb/get-intrinsic/commit/047f761cf30c946af0487ce13958eed9d5790bda) - [New] add abstract operations data, by year (starting at 2015) [`55d610f`](https://github.com/ljharb/get-intrinsic/commit/55d610fb6c1bf9f1acf38d0241ce3214c8f39091) - [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `nsp`, `semver`, `tape` [`37c5272`](https://github.com/ljharb/get-intrinsic/commit/37c5272826c83430df99e79696c6f40f228f96a0) - [Tests] add tests for missing and excess operations [`93efd66`](https://github.com/ljharb/get-intrinsic/commit/93efd669546bed6130fcc43b901d1764e726227b) - [Tests] up to `node` `v8.2`, `v7.10`, `v6.11`, `v4.8`; newer npm breaks on older node [`ff32a32`](https://github.com/ljharb/get-intrinsic/commit/ff32a326c5518e58ab7f2f9640baa3f124938cd7) - [Tests] add codecov [`311c416`](https://github.com/ljharb/get-intrinsic/commit/311c4163ca5dac913d27366fa7c6020d220ab2b3) - [Tests] make IsRegExp tests consistent across editions. [`e48bcb7`](https://github.com/ljharb/get-intrinsic/commit/e48bcb7a1f8769aa4a930b695d474d1afc9451d1) - [Tests] switch to `nyc` for code coverage [`2e97841`](https://github.com/ljharb/get-intrinsic/commit/2e9784171820b174c030bdcb91f0e114975769d1) - [Tests] fix coverage [`60d5305`](https://github.com/ljharb/get-intrinsic/commit/60d53055cf24452c80afee4e5288219a175ed445) - [Tests] ES2015: add ToNumber symbol tests [`6549464`](https://github.com/ljharb/get-intrinsic/commit/65494646a25b1817f85a79e4de35f097e6796f93) - [Fix] assign helper only supports one source [`b397fb3`](https://github.com/ljharb/get-intrinsic/commit/b397fb3bea4765a9b325c156cdcfc3e5a11742ec) - Only apps should have lockfiles. [`5c28e72`](https://github.com/ljharb/get-intrinsic/commit/5c28e723778f3327c459dc239a961ba7a897d9a3) - [Dev Deps] update `nsp`, `eslint`, `@ljharb/eslint-config` [`5f09a50`](https://github.com/ljharb/get-intrinsic/commit/5f09a50f86e246575080227d87e9c96c139ef407) - [Dev Deps] update `tape` [`2f0fc3c`](https://github.com/ljharb/get-intrinsic/commit/2f0fc3cd7569e258411522a294ed36d4cfd8674d) - [Fix] es7/es2016: do not mutate ES6. [`c69b8a3`](https://github.com/ljharb/get-intrinsic/commit/c69b8a3d4cde726bf581b44fdc3cae3818f94e11) - [Deps] update `is-regex` [`0600ae5`](https://github.com/ljharb/get-intrinsic/commit/0600ae5bf7fb58fd5c3034aeb28cd1ae3ec76a80) ## [v1.7.0](https://github.com/ljharb/get-intrinsic/compare/v1.6.1...v1.7.0) - 2017-01-22 ### Commits - [Tests] up to `node` `v7.4`; improve test matrix [`fe20c5b`](https://github.com/ljharb/get-intrinsic/commit/fe20c5b26c2d377f3ae1701283b38f016d139788) - [New] `ES6`: Add `GetMethod` [`2edc976`](https://github.com/ljharb/get-intrinsic/commit/2edc976b3d4024b9be44da8f316413a1674d50fe) - [New] ES6: Add `Get` [`3b375c5`](https://github.com/ljharb/get-intrinsic/commit/3b375c573a321318d17f117d3e62ced6b9d9e3d7) - [New] `ES6`: Add `GetV` [`d72527e`](https://github.com/ljharb/get-intrinsic/commit/d72527e9d8f09e7c8b7aada0aa78e8da81caa673) - [Dev Deps] update `tape`, `eslint`, `@ljharb/eslint-config` [`949ff34`](https://github.com/ljharb/get-intrinsic/commit/949ff344d78c3ae1cbe5d137a69df3bd0c94fbec) - [Tests] up to `node` `v7.0`, `v6.9`, `v4.6`; improve test matrix [`31bf7e1`](https://github.com/ljharb/get-intrinsic/commit/31bf7e184a51624d88885bbec52cd7b1e1126b3f) - [Dev Deps] update `tape`, `nsp`, `eslint`, `@ljharb/eslint-config`, `safe-publish-latest` [`0351537`](https://github.com/ljharb/get-intrinsic/commit/035153777213981e0e24f6cf007ffbd279384130) - [Dev Deps] update `eslint`, `@ljharb/eslint-config` [`fce5110`](https://github.com/ljharb/get-intrinsic/commit/fce511086990f07e0febe0f3f2ae79215565399a) - [Tests] up to `node` `v7.2` [`cca76e3`](https://github.com/ljharb/get-intrinsic/commit/cca76e388691b9f66774172cafb62fe6b5deccb2) ## [v1.6.1](https://github.com/ljharb/get-intrinsic/compare/v1.6.0...v1.6.1) - 2016-08-21 ### Commits - [Fix] IsConstructor should return true for `class` constructors. [`8fd9281`](https://github.com/ljharb/get-intrinsic/commit/8fd9281a87ae96f4292b7f2da5d7f162e8c65505) ## [v1.6.0](https://github.com/ljharb/get-intrinsic/compare/v1.5.1...v1.6.0) - 2016-08-20 ### Commits - [New] ES6: `SpeciesConstructor` [`f15a7f3`](https://github.com/ljharb/get-intrinsic/commit/f15a7f37ac8bd2c60039d37615afc1187a28cf2f) - [New] ES5 / ES6: add `Type` [`2fae9c6`](https://github.com/ljharb/get-intrinsic/commit/2fae9c60b7dbccd45b478a54bcac455a774fc8e1) - [Dev Deps] update `jscs`, `nsp`, `eslint`, `@ljharb/eslint-config`, `semver` [`bd992af`](https://github.com/ljharb/get-intrinsic/commit/bd992af6e97a7cab4c11167626b9aefea1dc093e) - [Dev Deps] update `tape`, `jscs`, `nsp`, `eslint`, `@ljharb/eslint-config`, `semver` [`b783e29`](https://github.com/ljharb/get-intrinsic/commit/b783e299100b1dd12e2ac19c0da55f2ce68256c4) - [Tests] up to `node` `v6.4`, `v4.5` [`e217b69`](https://github.com/ljharb/get-intrinsic/commit/e217b690f6f259f002104a8d3255b0dd352e0189) - [Dev Deps] add `safe-publish-latest` [`b469ab3`](https://github.com/ljharb/get-intrinsic/commit/b469ab34ef0b16eefa15a908cd2ef2cd06539200) - [Test] on `node` `v5.12` [`a1fa32f`](https://github.com/ljharb/get-intrinsic/commit/a1fa32f0de4134867c3f854544ecab095279fe8f) ## [v1.5.1](https://github.com/ljharb/get-intrinsic/compare/v1.5.0...v1.5.1) - 2016-05-30 ### Fixed - [Deps] update `es-to-primitive`, fix ES5 tests. [`#6`](https://github.com/ljharb/get-intrinsic/issues/6) ### Commits - [Dev Deps] update `tape`, `jscs`, `nsp`, `eslint`, `@ljharb/eslint-config` [`4a0c1c3`](https://github.com/ljharb/get-intrinsic/commit/4a0c1c3ecac89f07970cbee7e029202848e862a4) - [Dev Deps] update `jscs`, `nsp`, `eslint`, `@ljharb/eslint-config` [`f0f379a`](https://github.com/ljharb/get-intrinsic/commit/f0f379a596a2193cc20cb882ef4deb800503b6eb) - [Dev Deps] update `jscs`, `nsp`, `eslint` [`2eec6cd`](https://github.com/ljharb/get-intrinsic/commit/2eec6cd5b51df7ac053808bfdfdc5ca0409cbe97) - `s/ /\t/g` [`efe1104`](https://github.com/ljharb/get-intrinsic/commit/efe1104274a819b79a9f9584b66c5d80434ec8b7) - [Dev Deps] update `jscs`, `eslint`, `@ljharb/eslint-config` [`e6738f6`](https://github.com/ljharb/get-intrinsic/commit/e6738f6a40c05ddcf032aa3f46a8ebfef697e3c8) - [Dev Deps] update `jscs`, `eslint`, `@ljharb/eslint-config` [`5320c76`](https://github.com/ljharb/get-intrinsic/commit/5320c7604041ce28f98141e7cb362a00d65fdb6b) - [Tests] up to `node` `v5.6`, `v4.3` [`67cb32b`](https://github.com/ljharb/get-intrinsic/commit/67cb32b07a91f0b5c2b9d418f99b9839b4072759) - [Tests] up to `node` `v5.9`, `v4.4` [`3b86e4a`](https://github.com/ljharb/get-intrinsic/commit/3b86e4af1325dfb00d21a4307b842979faf8f104) - [Refactor] create `isNaN` helper. [`dca4e0e`](https://github.com/ljharb/get-intrinsic/commit/dca4e0e2233d359eab713daaa25189ae61961dd9) - [Tests] up to `node` `v6.2` [`6b3dab1`](https://github.com/ljharb/get-intrinsic/commit/6b3dab1ff7d2add734ee21d0b90547cfc0a415ea) - [Tests] use pretest/posttest for linting/security [`a2b6a25`](https://github.com/ljharb/get-intrinsic/commit/a2b6a2557a9aab198ecae352e733d967f7bb543c) - [Dev Deps] update `jscs`, `@ljharb/eslint-config` [`7b66c31`](https://github.com/ljharb/get-intrinsic/commit/7b66c31747e086ec28b3be3b6dee93931d3b68bf) - [Fix] `ES.IsRegExp`: actually look up `Symbol.match` on the argument. [`8c7df66`](https://github.com/ljharb/get-intrinsic/commit/8c7df665ea7d9420f6496c4380e5577b1a9205d5) - [Tests] on `node` `v5.10` [`9ca82a5`](https://github.com/ljharb/get-intrinsic/commit/9ca82a5cec4d6a197b2f147571e3f58f0d5e6978) - [Deps] update `is-callable` [`c9be39b`](https://github.com/ljharb/get-intrinsic/commit/c9be39bf2b4426916516946dbe8801baceddb002) - [Dev Deps] update `eslint` [`1bc8fc9`](https://github.com/ljharb/get-intrinsic/commit/1bc8fc94ed798f081e38dfee9081c4fc6ae68729) - [Tests] on `node` `v5.7` [`78b08fb`](https://github.com/ljharb/get-intrinsic/commit/78b08fb0fe90423ccf0fa453e9ac315bdd329f99) - [Deps] update `function-bind` [`e657bcb`](https://github.com/ljharb/get-intrinsic/commit/e657bcba7e0ba482c5795c099ad487cb0796d9ac) - [Deps] update `is-callable` [`0a3fbb3`](https://github.com/ljharb/get-intrinsic/commit/0a3fbb368cc6fbedb22a6c2972314d063991fad8) ## [v1.5.0](https://github.com/ljharb/get-intrinsic/compare/v1.4.3...v1.5.0) - 2015-12-27 ### Commits - [Dev Deps] update `jscs`, `eslint`, `semver` [`8545989`](https://github.com/ljharb/get-intrinsic/commit/854598960fb8939dad45e9f432980f6a17ede598) - [Dev Deps] update `jscs`, `nsp`, `eslint` [`ff2f1d8`](https://github.com/ljharb/get-intrinsic/commit/ff2f1d8ab7fa79b869425068b1c3203dde08f8e9) - [Dev Deps] update `jscs`, `nsp`, `eslint`, `@ljharb/eslint-config` [`6ad543f`](https://github.com/ljharb/get-intrinsic/commit/6ad543ff06367954d2602cfdbf8fbc51f45cc6e1) - [Dev Deps] update `tape`, `nsp` [`43394e1`](https://github.com/ljharb/get-intrinsic/commit/43394e1c689fc2d4c2f1fc289d5d6ccc881c1df5) - [Tests] up to `node` `v5.3` [`2a1d7fe`](https://github.com/ljharb/get-intrinsic/commit/2a1d7fee4179b439ff63870a7eece404618f45b8) - [Deps] update `es-to-primitive` [`80cd4d3`](https://github.com/ljharb/get-intrinsic/commit/80cd4d38cc86e329e43aa2ce93937da2642b759e) - [Deps] update `is-callable` [`e65039f`](https://github.com/ljharb/get-intrinsic/commit/e65039fb7d18bfe2670fdd871f297716c5d72b40) - [Tests] on `node` `v5.1` [`5687653`](https://github.com/ljharb/get-intrinsic/commit/5687653c6c073681ddf74eb1f7f0e4b165eb67d7) ## [v1.4.3](https://github.com/ljharb/get-intrinsic/compare/v1.4.2...v1.4.3) - 2015-11-04 ### Fixed - [Fix] `ES6.ToNumber`: should give `NaN` for explicitly signed hex strings. [`#4`](https://github.com/ljharb/get-intrinsic/issues/4) ### Commits - [Refactor] group tests better. [`e8d8758`](https://github.com/ljharb/get-intrinsic/commit/e8d875826d8b0f5aab5b67a116fa607816e8d5b8) - [Refactor] `ES6.ToNumber`: No need to double-trim. [`2538ea7`](https://github.com/ljharb/get-intrinsic/commit/2538ea7c14560a5a49146390023f3900812cdac1) - [Tests] should still pass on `node` `v0.8` [`2555593`](https://github.com/ljharb/get-intrinsic/commit/2555593bf55a18f9a2db4a1ef6d1894dd26ae51d) ## [v1.4.2](https://github.com/ljharb/get-intrinsic/compare/v1.4.1...v1.4.2) - 2015-11-02 ### Fixed - [Fix] ensure `ES.ToNumber` trims whitespace, and does not trim non-whitespace. [`#3`](https://github.com/ljharb/get-intrinsic/issues/3) ## [v1.4.1](https://github.com/ljharb/get-intrinsic/compare/v1.4.0...v1.4.1) - 2015-10-31 ### Fixed - [Fix] ensure only 0-1 are valid binary and 0-7 are valid octal digits. [`#2`](https://github.com/ljharb/get-intrinsic/issues/2) ### Commits - [Dev Deps] update `tape`, `jscs`, `nsp`, `eslint`, `@ljharb/eslint-config` [`576d57f`](https://github.com/ljharb/get-intrinsic/commit/576d57f47cc2e2be2393ba44dbd9f49df22ff53a) - package.json: use object form of "authors", add "contributors" [`799bfef`](https://github.com/ljharb/get-intrinsic/commit/799bfef43be7743672add3bc79b559437ab4b890) - [Tests] fix npm upgrades for older node versions [`ba2a70e`](https://github.com/ljharb/get-intrinsic/commit/ba2a70ed8346bbeccb413a4004456b91ffedb7d5) - [Tests] on `node` `v5.0` [`eaf17a8`](https://github.com/ljharb/get-intrinsic/commit/eaf17a81244d595cb86ee50aa93503232bb5f52d) ## [v1.4.0](https://github.com/ljharb/get-intrinsic/compare/v1.3.2...v1.4.0) - 2015-10-17 ### Commits - Add `SameValueNonNumber` to ES7. [`095c0c9`](https://github.com/ljharb/get-intrinsic/commit/095c0c9696c59545d1ac9f470528da0b09e5b697) - [Dev Deps] update `tape`, `jscs`, `eslint`, `@ljharb/eslint-config` [`58a67a3`](https://github.com/ljharb/get-intrinsic/commit/58a67a30bd7121c1932c254653e127dcd0458ef9) - [Dev Deps] update `jscs`, `eslint`, `@ljharb/eslint-config` [`96050f2`](https://github.com/ljharb/get-intrinsic/commit/96050f2c9ed3513a91ced725eddcfced7116ec6e) - [Tests] on `node` `v4.2` [`ee16fbe`](https://github.com/ljharb/get-intrinsic/commit/ee16fbe166682f843dc58ce85590bd1343d19061) - [Deps] update `is-callable` [`785f0bf`](https://github.com/ljharb/get-intrinsic/commit/785f0bfad5f700020df8271b33a9377a3296d58c) ## [v1.3.2](https://github.com/ljharb/get-intrinsic/compare/v1.3.1...v1.3.2) - 2015-09-26 ### Commits - Fix `IsRegExp` to properly handle `Symbol.match`, per spec. [`ab96c1c`](https://github.com/ljharb/get-intrinsic/commit/ab96c1c31ba975b89eb9e6f62c98eeb0270c0cac) - [Dev Deps] update `jscs`, `nsp`, `eslint`, `@ljharb/eslint-config`, `semver` [`490a8ba`](https://github.com/ljharb/get-intrinsic/commit/490a8bad458fee8f2ebb46821571ae17d71fe5ee) - [Tests] up to `io.js` `v3.3`, `node` `v4.1` [`922af35`](https://github.com/ljharb/get-intrinsic/commit/922af3599fc302b7c5fefff5262b2c4809c8b868) - [Dev Deps] update `eslint`, `@ljharb/eslint-config` [`7e1186a`](https://github.com/ljharb/get-intrinsic/commit/7e1186a9e88f9baf8c475959cbaa5028a9a0e3e0) - [Dev Deps] update `tape` [`d3f4f33`](https://github.com/ljharb/get-intrinsic/commit/d3f4f33f891927173ac716ddf24c125c2aece65e) ## [v1.3.1](https://github.com/ljharb/get-intrinsic/compare/v1.3.0...v1.3.1) - 2015-08-15 ### Commits - Ensure that objects that `toString` to a binary or octal literal also convert properly. [`34d0f5b`](https://github.com/ljharb/get-intrinsic/commit/34d0f5b30f09189fed2dbf3324c4729355f7407b) ## [v1.3.0](https://github.com/ljharb/get-intrinsic/compare/v1.2.2...v1.3.0) - 2015-08-15 ### Commits - Update `jscs`, `eslint`, `@ljharb/eslint-config` [`da1eb8c`](https://github.com/ljharb/get-intrinsic/commit/da1eb8c2637c4003570e515471680549bba8d5a8) - [New] ES6’s ToNumber now supports binary and octal literals. [`c81b8ec`](https://github.com/ljharb/get-intrinsic/commit/c81b8ec7873fb55a55a835675dd7db1aeb39484b) - [Dev Deps] update `jscs` [`b351a07`](https://github.com/ljharb/get-intrinsic/commit/b351a07ac2022ca0a2bc185a91b606ec6e5653c7) - Update `tape`, `eslint` [`64ddee9`](https://github.com/ljharb/get-intrinsic/commit/64ddee9211d11b86d35893d10e5ed01d52bce544) - [Dev Deps] update `tape` [`4d93933`](https://github.com/ljharb/get-intrinsic/commit/4d9393323bc6cc5df198ca9c9ff4be9fb296268e) - Switch from vb.teelaun.ch to versionbadg.es for the npm version badge SVG. [`164831e`](https://github.com/ljharb/get-intrinsic/commit/164831e97c8f39645830c69951ce459bf195be86) - Update `tape` [`6704daa`](https://github.com/ljharb/get-intrinsic/commit/6704daad3673f16be5c30f3bd4f274383458c609) - Test on `io.js` `v2.5` [`d846f8f`](https://github.com/ljharb/get-intrinsic/commit/d846f8fbf90785c204dd5e815716c1cc1d0f2d77) - Test on `io.js` `v3.0` [`84d008e`](https://github.com/ljharb/get-intrinsic/commit/84d008ec77bb157ef7bde9949850316655c01ab9) ## [v1.2.2](https://github.com/ljharb/get-intrinsic/compare/v1.2.1...v1.2.2) - 2015-07-28 ### Commits - Use my personal shared `eslint` config. [`8ce5117`](https://github.com/ljharb/get-intrinsic/commit/8ce511774378309dd13837e6473d2e39e8c81428) - Update `eslint` [`9bdef0e`](https://github.com/ljharb/get-intrinsic/commit/9bdef0e57b33b8f43c873dded948d9ee2453cf58) - Update `eslint`, `jscs`, `tape`, `semver` [`4166e79`](https://github.com/ljharb/get-intrinsic/commit/4166e7945a2009157ce6a40002a4b0ccef2471c4) - Update `eslint`, `nsp`, `semver` [`edfbec0`](https://github.com/ljharb/get-intrinsic/commit/edfbec00d53647f683516e141ade99087e6c1f77) - Update `jscs`, `eslint`, `covert`, `semver` [`dedefc3`](https://github.com/ljharb/get-intrinsic/commit/dedefc341e425cae9a4d7adcf1f05d295c2d55de) - Test up to `io.js` `v2.3` [`f720287`](https://github.com/ljharb/get-intrinsic/commit/f7202878d35af09f313755b1503ce9a5982e226d) - Add some more ES6.ToString tests. [`1199a5e`](https://github.com/ljharb/get-intrinsic/commit/1199a5e480008c50281af96172e6c2d981991b42) - Update `tape`, `eslint`, `semver` [`e0ac913`](https://github.com/ljharb/get-intrinsic/commit/e0ac913b7a3dbfd57847abf7f9c591c0380c6ca0) - Test on latest `io.js` versions. [`e018b38`](https://github.com/ljharb/get-intrinsic/commit/e018b3844d2715b0231b18265dec68f52a98bb34) - Test on `io.js` `v2.4` [`4cdd2cb`](https://github.com/ljharb/get-intrinsic/commit/4cdd2cbda257e2f281c423e5b1cb7666dde3b073) - Update `eslint` [`fa07aec`](https://github.com/ljharb/get-intrinsic/commit/fa07aec6ae2803f080971152206d5dd8e02449c3) - Test on `io.js` `v2.1` [`edfc1fd`](https://github.com/ljharb/get-intrinsic/commit/edfc1fdbfb8ba59a8450b4fe3e75508c2734281a) - Test up to `io.js` `v2.0` [`4b73b2a`](https://github.com/ljharb/get-intrinsic/commit/4b73b2a7e4fd8dd7d48140c8bb0f698b655fc839) - Both `ES5.CheckObjectCoercible` and `ES6.RequireObjectCoercible` return the value if they don't throw. [`d72e869`](https://github.com/ljharb/get-intrinsic/commit/d72e869f16f0ae5603192632658efd0bdc336ad0) ## [v1.2.1](https://github.com/ljharb/get-intrinsic/compare/v1.2.0...v1.2.1) - 2015-03-20 ### Commits - Fix isFinite helper. [`0d4f914`](https://github.com/ljharb/get-intrinsic/commit/0d4f914f87bfefb04fb7a4650eb51da58c00c354) ## [v1.2.0](https://github.com/ljharb/get-intrinsic/compare/v1.1.1...v1.2.0) - 2015-03-19 ### Commits - Use `es-to-primitive` [`c554cf5`](https://github.com/ljharb/get-intrinsic/commit/c554cf5bcd7f41ffee8637b9109f9f9cb651acab) - Test on latest `io.js` versions; allow failures on all but 2 latest `node`/`io.js` versions. [`7941eba`](https://github.com/ljharb/get-intrinsic/commit/7941ebaf01f5cf2ccdca17c5131535a7583e04e0) ## [v1.1.1](https://github.com/ljharb/get-intrinsic/compare/v1.1.0...v1.1.1) - 2015-03-19 ### Commits - Update `eslint`, `editorconfig-tools`, `semver` [`84554ec`](https://github.com/ljharb/get-intrinsic/commit/84554eccb4b57d5c6da94203d439b0fc8200b1f3) - Update `eslint`, `nsp` [`b9308e7`](https://github.com/ljharb/get-intrinsic/commit/b9308e75b335d91e1d65b2d7b30cd26274b8cbbc) - Fixing isPrimitive check. [`5affc7d`](https://github.com/ljharb/get-intrinsic/commit/5affc7d416329e1eed574c818879a31f2fb547c7) - Fixing `make release` [`73d9f1f`](https://github.com/ljharb/get-intrinsic/commit/73d9f1f21af3b282becd6b62a9223e1c1789bccc) - Update `eslint` [`0c60789`](https://github.com/ljharb/get-intrinsic/commit/0c607890e0b02496a19a0a1ff23f6f4731c309dd) ## [v1.1.0](https://github.com/ljharb/get-intrinsic/compare/v1.0.2...v1.1.0) - 2015-02-17 ### Commits - Moving the ES6 methods to their own internal module. [`01d7e1b`](https://github.com/ljharb/get-intrinsic/commit/01d7e1b06e6829273f50b89584d6bddeca91a9af) - Add ES7 export (non-default). [`e1f4455`](https://github.com/ljharb/get-intrinsic/commit/e1f4455762b63a3a0c66b785b1af9018d1b24222) - Add ES6 tests [`eea6300`](https://github.com/ljharb/get-intrinsic/commit/eea63002b5d29399060f75b44653fd530f3c9011) - Implementation. [`0a64fb8`](https://github.com/ljharb/get-intrinsic/commit/0a64fb86e98dc5ed130358d69c657c8aaaf0b79a) - Dotfiles. [`fd70ce7`](https://github.com/ljharb/get-intrinsic/commit/fd70ce7800a2bec48d1273cf5bfeaeae5d689b79) - Moving the ES5 methods to their own internal module. [`5ee4426`](https://github.com/ljharb/get-intrinsic/commit/5ee44265ed3704f2f68d35c58b02e5bec9dee746) - Add ES5 tests [`2bff2bd`](https://github.com/ljharb/get-intrinsic/commit/2bff2bd3ecee99be72603dbfd0d3867d4b0fe95e) - Creating a bunch of internal helper modules. [`1969d6f`](https://github.com/ljharb/get-intrinsic/commit/1969d6f9a1e6f8cc095482c8bd17a86f1e44ca91) - package.json [`4d59162`](https://github.com/ljharb/get-intrinsic/commit/4d59162ff8dff9847fee58c1237ebfc3a7e53fdb) - Add `make release`, `make list`, `make test`. [`aa2bc63`](https://github.com/ljharb/get-intrinsic/commit/aa2bc635b3ec283ec63d95fc58b76094fbc5b2d8) - README. [`3a856b2`](https://github.com/ljharb/get-intrinsic/commit/3a856b2a7e95bf5e23471d9db63aae25450944b4) - LICENSE [`007e224`](https://github.com/ljharb/get-intrinsic/commit/007e2246f7a0edf4086c90f095e0ca4482169977) - All grade A-supported `node`/`iojs` versions now ship with an `npm` that understands `^`. [`b22c912`](https://github.com/ljharb/get-intrinsic/commit/b22c912070775d96dbaf6716553a48e3bcff53bb) - Tests for main ES object. [`2b85940`](https://github.com/ljharb/get-intrinsic/commit/2b85940c00747827877a15cbaa38b7f21fb809ae) - Update `tape`, `jscs`, `nsp`, `eslint` [`f83ada2`](https://github.com/ljharb/get-intrinsic/commit/f83ada228ec0f685538a888bf23f096bfed8a6f3) - Use `is-callable` instead of this internal function. [`b8b2d51`](https://github.com/ljharb/get-intrinsic/commit/b8b2d51056a93e73925ff6ade79e176a973ceda2) - Run `travis-ci` tests on `iojs` and `node` v0.12; allow 0.8 failures. [`91dfb1a`](https://github.com/ljharb/get-intrinsic/commit/91dfb1abd6aa287acae7dc6375e76bd5b2d7741d) - Update `tape`, `jscs` [`c2e81bd`](https://github.com/ljharb/get-intrinsic/commit/c2e81bd61d36d88ad289580fcf5efc69b0b13108) - Update `eslint` [`adf41d8`](https://github.com/ljharb/get-intrinsic/commit/adf41d8e68c640dd4f161bc647e16440bf714d9f) - Test on `iojs-v1.2`. [`5911eef`](https://github.com/ljharb/get-intrinsic/commit/5911eef8b9dc4a332bccc3f1aae728790dc9c9ab) - Initial commit [`8721dea`](https://github.com/ljharb/get-intrinsic/commit/8721dea87fb406a307c770c9747b4b5a1cbfe236) ## [v1.0.2](https://github.com/ljharb/get-intrinsic/compare/v1.0.1...v1.0.2) - 2020-12-17 ### Commits - [Fix] Throw for non‑existent intrinsics [`68f873b`](https://github.com/ljharb/get-intrinsic/commit/68f873b013c732a05ad6f5fc54f697e55515461b) - [Fix] Throw for non‑existent segments in the intrinsic path [`8325dee`](https://github.com/ljharb/get-intrinsic/commit/8325deee43128f3654d3399aa9591741ebe17b21) - [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `aud`, `has-bigints`, `object-inspect` [`0c227a7`](https://github.com/ljharb/get-intrinsic/commit/0c227a7d8b629166f25715fd242553892e458525) - [meta] do not lint coverage output [`70d2419`](https://github.com/ljharb/get-intrinsic/commit/70d24199b620043cd9110fc5f426d214ebe21dc9) ## [v1.0.1](https://github.com/ljharb/get-intrinsic/compare/v1.0.0...v1.0.1) - 2020-10-30 ### Commits - [Tests] gather coverage data on every job [`d1d280d`](https://github.com/ljharb/get-intrinsic/commit/d1d280dec714e3f0519cc877dbcb193057d9cac6) - [Fix] add missing dependencies [`5031771`](https://github.com/ljharb/get-intrinsic/commit/5031771bb1095b38be88ce7c41d5de88718e432e) - [Tests] use `es-value-fixtures` [`af48765`](https://github.com/ljharb/get-intrinsic/commit/af48765a23c5323fb0b6b38dbf00eb5099c7bebc) ## v1.0.0 - 2020-10-29 ### Commits - Implementation [`bbce57c`](https://github.com/ljharb/get-intrinsic/commit/bbce57c6f33d05b2d8d3efa273ceeb3ee01127bb) - Tests [`17b4f0d`](https://github.com/ljharb/get-intrinsic/commit/17b4f0d56dea6b4059b56fc30ef3ee4d9500ebc2) - Initial commit [`3153294`](https://github.com/ljharb/get-intrinsic/commit/31532948de363b0a27dd9fd4649e7b7028ec4b44) - npm init [`fb326c4`](https://github.com/ljharb/get-intrinsic/commit/fb326c4d2817c8419ec31de1295f06bb268a7902) - [meta] add Automatic Rebase and Require Allow Edits workflows [`48862fb`](https://github.com/ljharb/get-intrinsic/commit/48862fb2508c8f6a57968e6d08b7c883afc9d550) - [meta] add `auto-changelog` [`5f28ad0`](https://github.com/ljharb/get-intrinsic/commit/5f28ad019e060a353d8028f9f2591a9cc93074a1) - [meta] add "funding"; create `FUNDING.yml` [`c2bbdde`](https://github.com/ljharb/get-intrinsic/commit/c2bbddeba73a875be61484ee4680b129a6d4e0a1) - [Tests] add `npm run lint` [`0a84b98`](https://github.com/ljharb/get-intrinsic/commit/0a84b98b22b7cf7a748666f705b0003a493c35fd) - Only apps should have lockfiles [`9586c75`](https://github.com/ljharb/get-intrinsic/commit/9586c75866c1ee678e4d5d4dbbdef6997e511b05) apollo-server-demo/node_modules/get-intrinsic/.travis.yml 0000644 0001750 0000144 00000000426 03560116604 023322 0 ustar andreh users version: ~> 1.0 language: node_js os: - linux import: - ljharb/travis-ci:node/all.yml - ljharb/travis-ci:node/pretest.yml - ljharb/travis-ci:node/posttest.yml after_success: - 'if [ -f coverage/*.json ]; then bash <(curl -s https://codecov.io/bash) -f coverage/*.json; fi' apollo-server-demo/node_modules/object.assign/ 0000755 0001750 0000144 00000000000 14067647701 021174 5 ustar andreh users apollo-server-demo/node_modules/object.assign/index.js 0000644 0001750 0000144 00000001024 03560116604 022623 0 ustar andreh users 'use strict'; var defineProperties = require('define-properties'); var callBind = require('call-bind'); var implementation = require('./implementation'); var getPolyfill = require('./polyfill'); var shim = require('./shim'); var polyfill = callBind.apply(getPolyfill()); // eslint-disable-next-line no-unused-vars var bound = function assign(target, source1) { return polyfill(Object, arguments); }; defineProperties(bound, { getPolyfill: getPolyfill, implementation: implementation, shim: shim }); module.exports = bound; apollo-server-demo/node_modules/object.assign/dist/ 0000755 0001750 0000144 00000000000 14067647701 022137 5 ustar andreh users apollo-server-demo/node_modules/object.assign/dist/browser.js 0000644 0001750 0000144 00000067063 03560116604 024161 0 ustar andreh users (function(){function r(e,n,t){function o(i,f){if(!n[i]){if(!e[i]){var c="function"==typeof require&&require;if(!f&&c)return c(i,!0);if(u)return u(i,!0);var a=new Error("Cannot find module '"+i+"'");throw a.code="MODULE_NOT_FOUND",a}var p=n[i]={exports:{}};e[i][0].call(p.exports,function(r){var n=e[i][1][r];return o(n||r)},p,p.exports,r,e,n,t)}return n[i].exports}for(var u="function"==typeof require&&require,i=0;i<t.length;i++)o(t[i]);return o}return r})()({1:[function(require,module,exports){ 'use strict'; var keys = require('object-keys').shim(); delete keys.shim; var assign = require('./'); module.exports = assign.shim(); delete assign.shim; },{"./":3,"object-keys":14}],2:[function(require,module,exports){ 'use strict'; // modified from https://github.com/es-shims/es6-shim var keys = require('object-keys'); var canBeObject = function (obj) { return typeof obj !== 'undefined' && obj !== null; }; var hasSymbols = require('has-symbols/shams')(); var callBound = require('call-bind/callBound'); var toObject = Object; var $push = callBound('Array.prototype.push'); var $propIsEnumerable = callBound('Object.prototype.propertyIsEnumerable'); var originalGetSymbols = hasSymbols ? Object.getOwnPropertySymbols : null; // eslint-disable-next-line no-unused-vars module.exports = function assign(target, source1) { if (!canBeObject(target)) { throw new TypeError('target must be an object'); } var objTarget = toObject(target); var s, source, i, props, syms, value, key; for (s = 1; s < arguments.length; ++s) { source = toObject(arguments[s]); props = keys(source); var getSymbols = hasSymbols && (Object.getOwnPropertySymbols || originalGetSymbols); if (getSymbols) { syms = getSymbols(source); for (i = 0; i < syms.length; ++i) { key = syms[i]; if ($propIsEnumerable(source, key)) { $push(props, key); } } } for (i = 0; i < props.length; ++i) { key = props[i]; value = source[key]; if ($propIsEnumerable(source, key)) { objTarget[key] = value; } } } return objTarget; }; },{"call-bind/callBound":4,"has-symbols/shams":11,"object-keys":14}],3:[function(require,module,exports){ 'use strict'; var defineProperties = require('define-properties'); var callBind = require('call-bind'); var implementation = require('./implementation'); var getPolyfill = require('./polyfill'); var shim = require('./shim'); var polyfill = callBind.apply(getPolyfill()); // eslint-disable-next-line no-unused-vars var bound = function assign(target, source1) { return polyfill(Object, arguments); }; defineProperties(bound, { getPolyfill: getPolyfill, implementation: implementation, shim: shim }); module.exports = bound; },{"./implementation":2,"./polyfill":16,"./shim":17,"call-bind":5,"define-properties":6}],4:[function(require,module,exports){ 'use strict'; var GetIntrinsic = require('get-intrinsic'); var callBind = require('./'); var $indexOf = callBind(GetIntrinsic('String.prototype.indexOf')); module.exports = function callBoundIntrinsic(name, allowMissing) { var intrinsic = GetIntrinsic(name, !!allowMissing); if (typeof intrinsic === 'function' && $indexOf(name, '.prototype.') > -1) { return callBind(intrinsic); } return intrinsic; }; },{"./":5,"get-intrinsic":9}],5:[function(require,module,exports){ 'use strict'; var bind = require('function-bind'); var GetIntrinsic = require('get-intrinsic'); var $apply = GetIntrinsic('%Function.prototype.apply%'); var $call = GetIntrinsic('%Function.prototype.call%'); var $reflectApply = GetIntrinsic('%Reflect.apply%', true) || bind.call($call, $apply); var $defineProperty = GetIntrinsic('%Object.defineProperty%', true); if ($defineProperty) { try { $defineProperty({}, 'a', { value: 1 }); } catch (e) { // IE 8 has a broken defineProperty $defineProperty = null; } } module.exports = function callBind() { return $reflectApply(bind, $call, arguments); }; var applyBind = function applyBind() { return $reflectApply(bind, $apply, arguments); }; if ($defineProperty) { $defineProperty(module.exports, 'apply', { value: applyBind }); } else { module.exports.apply = applyBind; } },{"function-bind":8,"get-intrinsic":9}],6:[function(require,module,exports){ 'use strict'; var keys = require('object-keys'); var hasSymbols = typeof Symbol === 'function' && typeof Symbol('foo') === 'symbol'; var toStr = Object.prototype.toString; var concat = Array.prototype.concat; var origDefineProperty = Object.defineProperty; var isFunction = function (fn) { return typeof fn === 'function' && toStr.call(fn) === '[object Function]'; }; var arePropertyDescriptorsSupported = function () { var obj = {}; try { origDefineProperty(obj, 'x', { enumerable: false, value: obj }); // eslint-disable-next-line no-unused-vars, no-restricted-syntax for (var _ in obj) { // jscs:ignore disallowUnusedVariables return false; } return obj.x === obj; } catch (e) { /* this is IE 8. */ return false; } }; var supportsDescriptors = origDefineProperty && arePropertyDescriptorsSupported(); var defineProperty = function (object, name, value, predicate) { if (name in object && (!isFunction(predicate) || !predicate())) { return; } if (supportsDescriptors) { origDefineProperty(object, name, { configurable: true, enumerable: false, value: value, writable: true }); } else { object[name] = value; } }; var defineProperties = function (object, map) { var predicates = arguments.length > 2 ? arguments[2] : {}; var props = keys(map); if (hasSymbols) { props = concat.call(props, Object.getOwnPropertySymbols(map)); } for (var i = 0; i < props.length; i += 1) { defineProperty(object, props[i], map[props[i]], predicates[props[i]]); } }; defineProperties.supportsDescriptors = !!supportsDescriptors; module.exports = defineProperties; },{"object-keys":14}],7:[function(require,module,exports){ 'use strict'; /* eslint no-invalid-this: 1 */ var ERROR_MESSAGE = 'Function.prototype.bind called on incompatible '; var slice = Array.prototype.slice; var toStr = Object.prototype.toString; var funcType = '[object Function]'; module.exports = function bind(that) { var target = this; if (typeof target !== 'function' || toStr.call(target) !== funcType) { throw new TypeError(ERROR_MESSAGE + target); } var args = slice.call(arguments, 1); var bound; var binder = function () { if (this instanceof bound) { var result = target.apply( this, args.concat(slice.call(arguments)) ); if (Object(result) === result) { return result; } return this; } else { return target.apply( that, args.concat(slice.call(arguments)) ); } }; var boundLength = Math.max(0, target.length - args.length); var boundArgs = []; for (var i = 0; i < boundLength; i++) { boundArgs.push('$' + i); } bound = Function('binder', 'return function (' + boundArgs.join(',') + '){ return binder.apply(this,arguments); }')(binder); if (target.prototype) { var Empty = function Empty() {}; Empty.prototype = target.prototype; bound.prototype = new Empty(); Empty.prototype = null; } return bound; }; },{}],8:[function(require,module,exports){ 'use strict'; var implementation = require('./implementation'); module.exports = Function.prototype.bind || implementation; },{"./implementation":7}],9:[function(require,module,exports){ 'use strict'; /* globals AggregateError, Atomics, FinalizationRegistry, SharedArrayBuffer, WeakRef, */ var undefined; var $SyntaxError = SyntaxError; var $Function = Function; var $TypeError = TypeError; // eslint-disable-next-line consistent-return var getEvalledConstructor = function (expressionSyntax) { try { // eslint-disable-next-line no-new-func return Function('"use strict"; return (' + expressionSyntax + ').constructor;')(); } catch (e) {} }; var $gOPD = Object.getOwnPropertyDescriptor; if ($gOPD) { try { $gOPD({}, ''); } catch (e) { $gOPD = null; // this is IE 8, which has a broken gOPD } } var throwTypeError = function () { throw new $TypeError(); }; var ThrowTypeError = $gOPD ? (function () { try { // eslint-disable-next-line no-unused-expressions, no-caller, no-restricted-properties arguments.callee; // IE 8 does not throw here return throwTypeError; } catch (calleeThrows) { try { // IE 8 throws on Object.getOwnPropertyDescriptor(arguments, '') return $gOPD(arguments, 'callee').get; } catch (gOPDthrows) { return throwTypeError; } } }()) : throwTypeError; var hasSymbols = require('has-symbols')(); var getProto = Object.getPrototypeOf || function (x) { return x.__proto__; }; // eslint-disable-line no-proto var asyncGenFunction = getEvalledConstructor('async function* () {}'); var asyncGenFunctionPrototype = asyncGenFunction ? asyncGenFunction.prototype : undefined; var asyncGenPrototype = asyncGenFunctionPrototype ? asyncGenFunctionPrototype.prototype : undefined; var TypedArray = typeof Uint8Array === 'undefined' ? undefined : getProto(Uint8Array); var INTRINSICS = { '%AggregateError%': typeof AggregateError === 'undefined' ? undefined : AggregateError, '%Array%': Array, '%ArrayBuffer%': typeof ArrayBuffer === 'undefined' ? undefined : ArrayBuffer, '%ArrayIteratorPrototype%': hasSymbols ? getProto([][Symbol.iterator]()) : undefined, '%AsyncFromSyncIteratorPrototype%': undefined, '%AsyncFunction%': getEvalledConstructor('async function () {}'), '%AsyncGenerator%': asyncGenFunctionPrototype, '%AsyncGeneratorFunction%': asyncGenFunction, '%AsyncIteratorPrototype%': asyncGenPrototype ? getProto(asyncGenPrototype) : undefined, '%Atomics%': typeof Atomics === 'undefined' ? undefined : Atomics, '%BigInt%': typeof BigInt === 'undefined' ? undefined : BigInt, '%Boolean%': Boolean, '%DataView%': typeof DataView === 'undefined' ? undefined : DataView, '%Date%': Date, '%decodeURI%': decodeURI, '%decodeURIComponent%': decodeURIComponent, '%encodeURI%': encodeURI, '%encodeURIComponent%': encodeURIComponent, '%Error%': Error, '%eval%': eval, // eslint-disable-line no-eval '%EvalError%': EvalError, '%Float32Array%': typeof Float32Array === 'undefined' ? undefined : Float32Array, '%Float64Array%': typeof Float64Array === 'undefined' ? undefined : Float64Array, '%FinalizationRegistry%': typeof FinalizationRegistry === 'undefined' ? undefined : FinalizationRegistry, '%Function%': $Function, '%GeneratorFunction%': getEvalledConstructor('function* () {}'), '%Int8Array%': typeof Int8Array === 'undefined' ? undefined : Int8Array, '%Int16Array%': typeof Int16Array === 'undefined' ? undefined : Int16Array, '%Int32Array%': typeof Int32Array === 'undefined' ? undefined : Int32Array, '%isFinite%': isFinite, '%isNaN%': isNaN, '%IteratorPrototype%': hasSymbols ? getProto(getProto([][Symbol.iterator]())) : undefined, '%JSON%': typeof JSON === 'object' ? JSON : undefined, '%Map%': typeof Map === 'undefined' ? undefined : Map, '%MapIteratorPrototype%': typeof Map === 'undefined' || !hasSymbols ? undefined : getProto(new Map()[Symbol.iterator]()), '%Math%': Math, '%Number%': Number, '%Object%': Object, '%parseFloat%': parseFloat, '%parseInt%': parseInt, '%Promise%': typeof Promise === 'undefined' ? undefined : Promise, '%Proxy%': typeof Proxy === 'undefined' ? undefined : Proxy, '%RangeError%': RangeError, '%ReferenceError%': ReferenceError, '%Reflect%': typeof Reflect === 'undefined' ? undefined : Reflect, '%RegExp%': RegExp, '%Set%': typeof Set === 'undefined' ? undefined : Set, '%SetIteratorPrototype%': typeof Set === 'undefined' || !hasSymbols ? undefined : getProto(new Set()[Symbol.iterator]()), '%SharedArrayBuffer%': typeof SharedArrayBuffer === 'undefined' ? undefined : SharedArrayBuffer, '%String%': String, '%StringIteratorPrototype%': hasSymbols ? getProto(''[Symbol.iterator]()) : undefined, '%Symbol%': hasSymbols ? Symbol : undefined, '%SyntaxError%': $SyntaxError, '%ThrowTypeError%': ThrowTypeError, '%TypedArray%': TypedArray, '%TypeError%': $TypeError, '%Uint8Array%': typeof Uint8Array === 'undefined' ? undefined : Uint8Array, '%Uint8ClampedArray%': typeof Uint8ClampedArray === 'undefined' ? undefined : Uint8ClampedArray, '%Uint16Array%': typeof Uint16Array === 'undefined' ? undefined : Uint16Array, '%Uint32Array%': typeof Uint32Array === 'undefined' ? undefined : Uint32Array, '%URIError%': URIError, '%WeakMap%': typeof WeakMap === 'undefined' ? undefined : WeakMap, '%WeakRef%': typeof WeakRef === 'undefined' ? undefined : WeakRef, '%WeakSet%': typeof WeakSet === 'undefined' ? undefined : WeakSet }; var LEGACY_ALIASES = { '%ArrayBufferPrototype%': ['ArrayBuffer', 'prototype'], '%ArrayPrototype%': ['Array', 'prototype'], '%ArrayProto_entries%': ['Array', 'prototype', 'entries'], '%ArrayProto_forEach%': ['Array', 'prototype', 'forEach'], '%ArrayProto_keys%': ['Array', 'prototype', 'keys'], '%ArrayProto_values%': ['Array', 'prototype', 'values'], '%AsyncFunctionPrototype%': ['AsyncFunction', 'prototype'], '%AsyncGenerator%': ['AsyncGeneratorFunction', 'prototype'], '%AsyncGeneratorPrototype%': ['AsyncGeneratorFunction', 'prototype', 'prototype'], '%BooleanPrototype%': ['Boolean', 'prototype'], '%DataViewPrototype%': ['DataView', 'prototype'], '%DatePrototype%': ['Date', 'prototype'], '%ErrorPrototype%': ['Error', 'prototype'], '%EvalErrorPrototype%': ['EvalError', 'prototype'], '%Float32ArrayPrototype%': ['Float32Array', 'prototype'], '%Float64ArrayPrototype%': ['Float64Array', 'prototype'], '%FunctionPrototype%': ['Function', 'prototype'], '%Generator%': ['GeneratorFunction', 'prototype'], '%GeneratorPrototype%': ['GeneratorFunction', 'prototype', 'prototype'], '%Int8ArrayPrototype%': ['Int8Array', 'prototype'], '%Int16ArrayPrototype%': ['Int16Array', 'prototype'], '%Int32ArrayPrototype%': ['Int32Array', 'prototype'], '%JSONParse%': ['JSON', 'parse'], '%JSONStringify%': ['JSON', 'stringify'], '%MapPrototype%': ['Map', 'prototype'], '%NumberPrototype%': ['Number', 'prototype'], '%ObjectPrototype%': ['Object', 'prototype'], '%ObjProto_toString%': ['Object', 'prototype', 'toString'], '%ObjProto_valueOf%': ['Object', 'prototype', 'valueOf'], '%PromisePrototype%': ['Promise', 'prototype'], '%PromiseProto_then%': ['Promise', 'prototype', 'then'], '%Promise_all%': ['Promise', 'all'], '%Promise_reject%': ['Promise', 'reject'], '%Promise_resolve%': ['Promise', 'resolve'], '%RangeErrorPrototype%': ['RangeError', 'prototype'], '%ReferenceErrorPrototype%': ['ReferenceError', 'prototype'], '%RegExpPrototype%': ['RegExp', 'prototype'], '%SetPrototype%': ['Set', 'prototype'], '%SharedArrayBufferPrototype%': ['SharedArrayBuffer', 'prototype'], '%StringPrototype%': ['String', 'prototype'], '%SymbolPrototype%': ['Symbol', 'prototype'], '%SyntaxErrorPrototype%': ['SyntaxError', 'prototype'], '%TypedArrayPrototype%': ['TypedArray', 'prototype'], '%TypeErrorPrototype%': ['TypeError', 'prototype'], '%Uint8ArrayPrototype%': ['Uint8Array', 'prototype'], '%Uint8ClampedArrayPrototype%': ['Uint8ClampedArray', 'prototype'], '%Uint16ArrayPrototype%': ['Uint16Array', 'prototype'], '%Uint32ArrayPrototype%': ['Uint32Array', 'prototype'], '%URIErrorPrototype%': ['URIError', 'prototype'], '%WeakMapPrototype%': ['WeakMap', 'prototype'], '%WeakSetPrototype%': ['WeakSet', 'prototype'] }; var bind = require('function-bind'); var hasOwn = require('has'); var $concat = bind.call(Function.call, Array.prototype.concat); var $spliceApply = bind.call(Function.apply, Array.prototype.splice); var $replace = bind.call(Function.call, String.prototype.replace); /* adapted from https://github.com/lodash/lodash/blob/4.17.15/dist/lodash.js#L6735-L6744 */ var rePropName = /[^%.[\]]+|\[(?:(-?\d+(?:\.\d+)?)|(["'])((?:(?!\2)[^\\]|\\.)*?)\2)\]|(?=(?:\.|\[\])(?:\.|\[\]|%$))/g; var reEscapeChar = /\\(\\)?/g; /** Used to match backslashes in property paths. */ var stringToPath = function stringToPath(string) { var result = []; $replace(string, rePropName, function (match, number, quote, subString) { result[result.length] = quote ? $replace(subString, reEscapeChar, '$1') : number || match; }); return result; }; /* end adaptation */ var getBaseIntrinsic = function getBaseIntrinsic(name, allowMissing) { var intrinsicName = name; var alias; if (hasOwn(LEGACY_ALIASES, intrinsicName)) { alias = LEGACY_ALIASES[intrinsicName]; intrinsicName = '%' + alias[0] + '%'; } if (hasOwn(INTRINSICS, intrinsicName)) { var value = INTRINSICS[intrinsicName]; if (typeof value === 'undefined' && !allowMissing) { throw new $TypeError('intrinsic ' + name + ' exists, but is not available. Please file an issue!'); } return { alias: alias, name: intrinsicName, value: value }; } throw new $SyntaxError('intrinsic ' + name + ' does not exist!'); }; module.exports = function GetIntrinsic(name, allowMissing) { if (typeof name !== 'string' || name.length === 0) { throw new $TypeError('intrinsic name must be a non-empty string'); } if (arguments.length > 1 && typeof allowMissing !== 'boolean') { throw new $TypeError('"allowMissing" argument must be a boolean'); } var parts = stringToPath(name); var intrinsicBaseName = parts.length > 0 ? parts[0] : ''; var intrinsic = getBaseIntrinsic('%' + intrinsicBaseName + '%', allowMissing); var intrinsicRealName = intrinsic.name; var value = intrinsic.value; var skipFurtherCaching = false; var alias = intrinsic.alias; if (alias) { intrinsicBaseName = alias[0]; $spliceApply(parts, $concat([0, 1], alias)); } for (var i = 1, isOwn = true; i < parts.length; i += 1) { var part = parts[i]; if (part === 'constructor' || !isOwn) { skipFurtherCaching = true; } intrinsicBaseName += '.' + part; intrinsicRealName = '%' + intrinsicBaseName + '%'; if (hasOwn(INTRINSICS, intrinsicRealName)) { value = INTRINSICS[intrinsicRealName]; } else if (value != null) { if ($gOPD && (i + 1) >= parts.length) { var desc = $gOPD(value, part); isOwn = !!desc; if (!allowMissing && !(part in value)) { throw new $TypeError('base intrinsic for ' + name + ' exists, but the property is not available.'); } // By convention, when a data property is converted to an accessor // property to emulate a data property that does not suffer from // the override mistake, that accessor's getter is marked with // an `originalValue` property. Here, when we detect this, we // uphold the illusion by pretending to see that original data // property, i.e., returning the value rather than the getter // itself. if (isOwn && 'get' in desc && !('originalValue' in desc.get)) { value = desc.get; } else { value = value[part]; } } else { isOwn = hasOwn(value, part); value = value[part]; } if (isOwn && !skipFurtherCaching) { INTRINSICS[intrinsicRealName] = value; } } } return value; }; },{"function-bind":8,"has":12,"has-symbols":10}],10:[function(require,module,exports){ (function (global){(function (){ 'use strict'; var origSymbol = global.Symbol; var hasSymbolSham = require('./shams'); module.exports = function hasNativeSymbols() { if (typeof origSymbol !== 'function') { return false; } if (typeof Symbol !== 'function') { return false; } if (typeof origSymbol('foo') !== 'symbol') { return false; } if (typeof Symbol('bar') !== 'symbol') { return false; } return hasSymbolSham(); }; }).call(this)}).call(this,typeof global !== "undefined" ? global : typeof self !== "undefined" ? self : typeof window !== "undefined" ? window : {}) },{"./shams":11}],11:[function(require,module,exports){ 'use strict'; /* eslint complexity: [2, 18], max-statements: [2, 33] */ module.exports = function hasSymbols() { if (typeof Symbol !== 'function' || typeof Object.getOwnPropertySymbols !== 'function') { return false; } if (typeof Symbol.iterator === 'symbol') { return true; } var obj = {}; var sym = Symbol('test'); var symObj = Object(sym); if (typeof sym === 'string') { return false; } if (Object.prototype.toString.call(sym) !== '[object Symbol]') { return false; } if (Object.prototype.toString.call(symObj) !== '[object Symbol]') { return false; } // temp disabled per https://github.com/ljharb/object.assign/issues/17 // if (sym instanceof Symbol) { return false; } // temp disabled per https://github.com/WebReflection/get-own-property-symbols/issues/4 // if (!(symObj instanceof Symbol)) { return false; } // if (typeof Symbol.prototype.toString !== 'function') { return false; } // if (String(sym) !== Symbol.prototype.toString.call(sym)) { return false; } var symVal = 42; obj[sym] = symVal; for (sym in obj) { return false; } // eslint-disable-line no-restricted-syntax if (typeof Object.keys === 'function' && Object.keys(obj).length !== 0) { return false; } if (typeof Object.getOwnPropertyNames === 'function' && Object.getOwnPropertyNames(obj).length !== 0) { return false; } var syms = Object.getOwnPropertySymbols(obj); if (syms.length !== 1 || syms[0] !== sym) { return false; } if (!Object.prototype.propertyIsEnumerable.call(obj, sym)) { return false; } if (typeof Object.getOwnPropertyDescriptor === 'function') { var descriptor = Object.getOwnPropertyDescriptor(obj, sym); if (descriptor.value !== symVal || descriptor.enumerable !== true) { return false; } } return true; }; },{}],12:[function(require,module,exports){ 'use strict'; var bind = require('function-bind'); module.exports = bind.call(Function.call, Object.prototype.hasOwnProperty); },{"function-bind":8}],13:[function(require,module,exports){ 'use strict'; var keysShim; if (!Object.keys) { // modified from https://github.com/es-shims/es5-shim var has = Object.prototype.hasOwnProperty; var toStr = Object.prototype.toString; var isArgs = require('./isArguments'); // eslint-disable-line global-require var isEnumerable = Object.prototype.propertyIsEnumerable; var hasDontEnumBug = !isEnumerable.call({ toString: null }, 'toString'); var hasProtoEnumBug = isEnumerable.call(function () {}, 'prototype'); var dontEnums = [ 'toString', 'toLocaleString', 'valueOf', 'hasOwnProperty', 'isPrototypeOf', 'propertyIsEnumerable', 'constructor' ]; var equalsConstructorPrototype = function (o) { var ctor = o.constructor; return ctor && ctor.prototype === o; }; var excludedKeys = { $applicationCache: true, $console: true, $external: true, $frame: true, $frameElement: true, $frames: true, $innerHeight: true, $innerWidth: true, $onmozfullscreenchange: true, $onmozfullscreenerror: true, $outerHeight: true, $outerWidth: true, $pageXOffset: true, $pageYOffset: true, $parent: true, $scrollLeft: true, $scrollTop: true, $scrollX: true, $scrollY: true, $self: true, $webkitIndexedDB: true, $webkitStorageInfo: true, $window: true }; var hasAutomationEqualityBug = (function () { /* global window */ if (typeof window === 'undefined') { return false; } for (var k in window) { try { if (!excludedKeys['$' + k] && has.call(window, k) && window[k] !== null && typeof window[k] === 'object') { try { equalsConstructorPrototype(window[k]); } catch (e) { return true; } } } catch (e) { return true; } } return false; }()); var equalsConstructorPrototypeIfNotBuggy = function (o) { /* global window */ if (typeof window === 'undefined' || !hasAutomationEqualityBug) { return equalsConstructorPrototype(o); } try { return equalsConstructorPrototype(o); } catch (e) { return false; } }; keysShim = function keys(object) { var isObject = object !== null && typeof object === 'object'; var isFunction = toStr.call(object) === '[object Function]'; var isArguments = isArgs(object); var isString = isObject && toStr.call(object) === '[object String]'; var theKeys = []; if (!isObject && !isFunction && !isArguments) { throw new TypeError('Object.keys called on a non-object'); } var skipProto = hasProtoEnumBug && isFunction; if (isString && object.length > 0 && !has.call(object, 0)) { for (var i = 0; i < object.length; ++i) { theKeys.push(String(i)); } } if (isArguments && object.length > 0) { for (var j = 0; j < object.length; ++j) { theKeys.push(String(j)); } } else { for (var name in object) { if (!(skipProto && name === 'prototype') && has.call(object, name)) { theKeys.push(String(name)); } } } if (hasDontEnumBug) { var skipConstructor = equalsConstructorPrototypeIfNotBuggy(object); for (var k = 0; k < dontEnums.length; ++k) { if (!(skipConstructor && dontEnums[k] === 'constructor') && has.call(object, dontEnums[k])) { theKeys.push(dontEnums[k]); } } } return theKeys; }; } module.exports = keysShim; },{"./isArguments":15}],14:[function(require,module,exports){ 'use strict'; var slice = Array.prototype.slice; var isArgs = require('./isArguments'); var origKeys = Object.keys; var keysShim = origKeys ? function keys(o) { return origKeys(o); } : require('./implementation'); var originalKeys = Object.keys; keysShim.shim = function shimObjectKeys() { if (Object.keys) { var keysWorksWithArguments = (function () { // Safari 5.0 bug var args = Object.keys(arguments); return args && args.length === arguments.length; }(1, 2)); if (!keysWorksWithArguments) { Object.keys = function keys(object) { // eslint-disable-line func-name-matching if (isArgs(object)) { return originalKeys(slice.call(object)); } return originalKeys(object); }; } } else { Object.keys = keysShim; } return Object.keys || keysShim; }; module.exports = keysShim; },{"./implementation":13,"./isArguments":15}],15:[function(require,module,exports){ 'use strict'; var toStr = Object.prototype.toString; module.exports = function isArguments(value) { var str = toStr.call(value); var isArgs = str === '[object Arguments]'; if (!isArgs) { isArgs = str !== '[object Array]' && value !== null && typeof value === 'object' && typeof value.length === 'number' && value.length >= 0 && toStr.call(value.callee) === '[object Function]'; } return isArgs; }; },{}],16:[function(require,module,exports){ 'use strict'; var implementation = require('./implementation'); var lacksProperEnumerationOrder = function () { if (!Object.assign) { return false; } /* * v8, specifically in node 4.x, has a bug with incorrect property enumeration order * note: this does not detect the bug unless there's 20 characters */ var str = 'abcdefghijklmnopqrst'; var letters = str.split(''); var map = {}; for (var i = 0; i < letters.length; ++i) { map[letters[i]] = letters[i]; } var obj = Object.assign({}, map); var actual = ''; for (var k in obj) { actual += k; } return str !== actual; }; var assignHasPendingExceptions = function () { if (!Object.assign || !Object.preventExtensions) { return false; } /* * Firefox 37 still has "pending exception" logic in its Object.assign implementation, * which is 72% slower than our shim, and Firefox 40's native implementation. */ var thrower = Object.preventExtensions({ 1: 2 }); try { Object.assign(thrower, 'xy'); } catch (e) { return thrower[1] === 'y'; } return false; }; module.exports = function getPolyfill() { if (!Object.assign) { return implementation; } if (lacksProperEnumerationOrder()) { return implementation; } if (assignHasPendingExceptions()) { return implementation; } return Object.assign; }; },{"./implementation":2}],17:[function(require,module,exports){ 'use strict'; var define = require('define-properties'); var getPolyfill = require('./polyfill'); module.exports = function shimAssign() { var polyfill = getPolyfill(); define( Object, { assign: polyfill }, { assign: function () { return Object.assign !== polyfill; } } ); return polyfill; }; },{"./polyfill":16,"define-properties":6}]},{},[1]); apollo-server-demo/node_modules/object.assign/LICENSE 0000644 0001750 0000144 00000002070 03560116604 022165 0 ustar andreh users The MIT License (MIT) Copyright (c) 2014 Jordan Harband Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/object.assign/.eslintrc 0000644 0001750 0000144 00000001116 03560116604 023004 0 ustar andreh users { "root": true, "extends": "@ljharb", "rules": { "complexity": [2, 19], "id-length": [2, { "min": 1, "max": 30 }], "max-statements": [2, 33], "max-statements-per-line": [2, { "max": 2 }], "no-magic-numbers": [1, { "ignore": [0] }], "no-restricted-syntax": [2, "BreakStatement", "ContinueStatement", "DebuggerStatement", "LabeledStatement", "WithStatement"], }, "overrides": [ { "files": "test/**", "rules": { "no-invalid-this": 1, "max-lines-per-function": 0, "max-statements-per-line": [2, { "max": 3 }], "no-magic-numbers": 0, }, }, ], } apollo-server-demo/node_modules/object.assign/.github/ 0000755 0001750 0000144 00000000000 14067647701 022534 5 ustar andreh users apollo-server-demo/node_modules/object.assign/.github/FUNDING.yml 0000644 0001750 0000144 00000001110 03560116604 024327 0 ustar andreh users # These are supported funding model platforms github: [ljharb] patreon: # Replace with a single Patreon username open_collective: # Replace with a single Open Collective username ko_fi: # Replace with a single Ko-fi username tidelift: npm/object.assign community_bridge: # Replace with a single Community Bridge project-name e.g., cloud-foundry liberapay: # Replace with a single Liberapay username issuehunt: # Replace with a single IssueHunt username otechie: # Replace with a single Otechie username custom: # Replace with up to 4 custom sponsorship URLs e.g., ['link1', 'link2'] apollo-server-demo/node_modules/object.assign/.github/workflows/ 0000755 0001750 0000144 00000000000 14067647701 024571 5 ustar andreh users apollo-server-demo/node_modules/object.assign/.github/workflows/require-allow-edits.yml 0000644 0001750 0000144 00000000376 03560116604 031205 0 ustar andreh users name: Require “Allow Edits†on: [pull_request_target] jobs: _: name: "Require “Allow Editsâ€" runs-on: ubuntu-latest steps: - uses: ljharb/require-allow-edits@main env: GITHUB_TOKEN: ${{ secrets.GITHUB_TOKEN }} apollo-server-demo/node_modules/object.assign/.github/workflows/rebase.yml 0000644 0001750 0000144 00000000401 03560116604 026535 0 ustar andreh users name: Automatic Rebase on: [pull_request_target] jobs: _: name: "Automatic Rebase" runs-on: ubuntu-latest steps: - uses: actions/checkout@v1 - uses: ljharb/rebase@master env: GITHUB_TOKEN: ${{ secrets.GITHUB_TOKEN }} apollo-server-demo/node_modules/object.assign/README.md 0000644 0001750 0000144 00000007077 03560116604 022453 0 ustar andreh users #object.assign <sup>[![Version Badge][npm-version-svg]][npm-url]</sup> [![Build Status][travis-svg]][travis-url] [![dependency status][deps-svg]][deps-url] [![dev dependency status][dev-deps-svg]][dev-deps-url] [![License][license-image]][license-url] [![Downloads][downloads-image]][downloads-url] [![npm badge][npm-badge-png]][npm-url] [![browser support][testling-png]][testling-url] An Object.assign shim. Invoke its "shim" method to shim Object.assign if it is unavailable. This package implements the [es-shim API](https://github.com/es-shims/api) interface. It works in an ES3-supported environment and complies with the [spec](http://www.ecma-international.org/ecma-262/6.0/#sec-object.assign). In an ES6 environment, it will also work properly with `Symbol`s. Takes a minimum of 2 arguments: `target` and `source`. Takes a variable sized list of source arguments - at least 1, as many as you want. Throws a TypeError if the `target` argument is `null` or `undefined`. Most common usage: ```js var assign = require('object.assign').getPolyfill(); // returns native method if compliant /* or */ var assign = require('object.assign/polyfill')(); // returns native method if compliant ``` ## Example ```js var assert = require('assert'); // Multiple sources! var target = { a: true }; var source1 = { b: true }; var source2 = { c: true }; var sourceN = { n: true }; var expected = { a: true, b: true, c: true, n: true }; assign(target, source1, source2, sourceN); assert.deepEqual(target, expected); // AWESOME! ``` ```js var target = { a: true, b: true, c: true }; var source1 = { c: false, d: false }; var sourceN = { e: false }; var assigned = assign(target, source1, sourceN); assert.equal(target, assigned); // returns the target object assert.deepEqual(assigned, { a: true, b: true, c: false, d: false, e: false }); ``` ```js /* when Object.assign is not present */ delete Object.assign; var shimmedAssign = require('object.assign').shim(); /* or */ var shimmedAssign = require('object.assign/shim')(); assert.equal(shimmedAssign, assign); var target = { a: true, b: true, c: true }; var source = { c: false, d: false, e: false }; var assigned = assign(target, source); assert.deepEqual(Object.assign(target, source), assign(target, source)); ``` ```js /* when Object.assign is present */ var shimmedAssign = require('object.assign').shim(); assert.equal(shimmedAssign, Object.assign); var target = { a: true, b: true, c: true }; var source = { c: false, d: false, e: false }; assert.deepEqual(Object.assign(target, source), assign(target, source)); ``` ## Tests Simply clone the repo, `npm install`, and run `npm test` [npm-url]: https://npmjs.org/package/object.assign [npm-version-svg]: http://versionbadg.es/ljharb/object.assign.svg [travis-svg]: https://travis-ci.org/ljharb/object.assign.svg [travis-url]: https://travis-ci.org/ljharb/object.assign [deps-svg]: https://david-dm.org/ljharb/object.assign.svg?theme=shields.io [deps-url]: https://david-dm.org/ljharb/object.assign [dev-deps-svg]: https://david-dm.org/ljharb/object.assign/dev-status.svg?theme=shields.io [dev-deps-url]: https://david-dm.org/ljharb/object.assign#info=devDependencies [testling-png]: https://ci.testling.com/ljharb/object.assign.png [testling-url]: https://ci.testling.com/ljharb/object.assign [npm-badge-png]: https://nodei.co/npm/object.assign.png?downloads=true&stars=true [license-image]: http://img.shields.io/npm/l/object.assign.svg [license-url]: LICENSE [downloads-image]: http://img.shields.io/npm/dm/object.assign.svg [downloads-url]: http://npm-stat.com/charts.html?package=object.assign apollo-server-demo/node_modules/object.assign/.editorconfig 0000644 0001750 0000144 00000000436 03560116604 023641 0 ustar andreh users root = true [*] indent_style = tab indent_size = 4 end_of_line = lf charset = utf-8 trim_trailing_whitespace = true insert_final_newline = true max_line_length = 150 [CHANGELOG.md] indent_style = space indent_size = 2 [*.json] max_line_length = off [Makefile] max_line_length = off apollo-server-demo/node_modules/object.assign/package.json 0000644 0001750 0000144 00000004235 03560116604 023453 0 ustar andreh users { "name": "object.assign", "version": "4.1.2", "author": "Jordan Harband", "funding": { "url": "https://github.com/sponsors/ljharb" }, "description": "ES6 spec-compliant Object.assign shim. From https://github.com/es-shims/es6-shim", "license": "MIT", "main": "index.js", "scripts": { "pretest": "npm run lint && es-shim-api --bound", "test": "npm run tests-only && npm run test:ses", "posttest": "aud --production", "tests-only": "npm run test:implementation && npm run test:shim", "test:native": "nyc node test/native", "test:shim": "nyc node test/shimmed", "test:implementation": "nyc node test", "test:ses": "node test/ses-compat", "lint": "eslint .", "build": "mkdir -p dist && browserify browserShim.js > dist/browser.js", "prepublish": "safe-publish-latest && npm run build" }, "repository": { "type": "git", "url": "git://github.com/ljharb/object.assign.git" }, "keywords": [ "Object.assign", "assign", "ES6", "extend", "$.extend", "jQuery", "_.extend", "Underscore", "es-shim API", "polyfill", "shim" ], "dependencies": { "call-bind": "^1.0.0", "define-properties": "^1.1.3", "has-symbols": "^1.0.1", "object-keys": "^1.1.1" }, "devDependencies": { "@es-shims/api": "^2.1.2", "@ljharb/eslint-config": "^17.2.0", "aud": "^1.1.2", "browserify": "^16.5.2", "eslint": "^7.12.1", "for-each": "^0.3.3", "functions-have-names": "^1.2.1", "has": "^1.0.3", "is": "^3.3.0", "nyc": "^10.3.2", "safe-publish-latest": "^1.1.4", "ses": "^0.10.4", "tape": "^5.0.1" }, "testling": { "files": "test/index.js", "browsers": [ "iexplore/6.0..latest", "firefox/3.0..6.0", "firefox/15.0..latest", "firefox/nightly", "chrome/4.0..10.0", "chrome/20.0..latest", "chrome/canary", "opera/10.0..latest", "opera/next", "safari/4.0..latest", "ipad/6.0..latest", "iphone/6.0..latest", "android-browser/4.2" ] }, "engines": { "node": ">= 0.4" } ,"_resolved": "https://registry.npmjs.org/object.assign/-/object.assign-4.1.2.tgz" ,"_integrity": "sha512-ixT2L5THXsApyiUPYKmW+2EHpXXe5Ii3M+f4e+aJFAHao5amFRW6J0OO6c/LU8Be47utCx2GL89hxGB6XSmKuQ==" ,"_from": "object.assign@4.1.2" } apollo-server-demo/node_modules/object.assign/auto.js 0000644 0001750 0000144 00000000044 03560116604 022465 0 ustar andreh users 'use strict'; require('./shim')(); apollo-server-demo/node_modules/object.assign/shim.js 0000644 0001750 0000144 00000000461 03560116604 022460 0 ustar andreh users 'use strict'; var define = require('define-properties'); var getPolyfill = require('./polyfill'); module.exports = function shimAssign() { var polyfill = getPolyfill(); define( Object, { assign: polyfill }, { assign: function () { return Object.assign !== polyfill; } } ); return polyfill; }; apollo-server-demo/node_modules/object.assign/implementation.js 0000644 0001750 0000144 00000002461 03560116604 024547 0 ustar andreh users 'use strict'; // modified from https://github.com/es-shims/es6-shim var keys = require('object-keys'); var canBeObject = function (obj) { return typeof obj !== 'undefined' && obj !== null; }; var hasSymbols = require('has-symbols/shams')(); var callBound = require('call-bind/callBound'); var toObject = Object; var $push = callBound('Array.prototype.push'); var $propIsEnumerable = callBound('Object.prototype.propertyIsEnumerable'); var originalGetSymbols = hasSymbols ? Object.getOwnPropertySymbols : null; // eslint-disable-next-line no-unused-vars module.exports = function assign(target, source1) { if (!canBeObject(target)) { throw new TypeError('target must be an object'); } var objTarget = toObject(target); var s, source, i, props, syms, value, key; for (s = 1; s < arguments.length; ++s) { source = toObject(arguments[s]); props = keys(source); var getSymbols = hasSymbols && (Object.getOwnPropertySymbols || originalGetSymbols); if (getSymbols) { syms = getSymbols(source); for (i = 0; i < syms.length; ++i) { key = syms[i]; if ($propIsEnumerable(source, key)) { $push(props, key); } } } for (i = 0; i < props.length; ++i) { key = props[i]; value = source[key]; if ($propIsEnumerable(source, key)) { objTarget[key] = value; } } } return objTarget; }; apollo-server-demo/node_modules/object.assign/.nycrc 0000644 0001750 0000144 00000000350 03560116604 022276 0 ustar andreh users { "all": true, "check-coverage": false, "reporter": ["text-summary", "text", "html", "json"], "lines": 86, "statements": 85.93, "functions": 82.43, "branches": 76.06, "exclude": [ "coverage", "operations", "test" ] } apollo-server-demo/node_modules/object.assign/test/ 0000755 0001750 0000144 00000000000 14067647701 022153 5 ustar andreh users apollo-server-demo/node_modules/object.assign/test/index.js 0000644 0001750 0000144 00000000650 03560116604 023606 0 ustar andreh users 'use strict'; var assign = require('../'); var test = require('tape'); var runTests = require('./tests'); test('as a function', function (t) { t.test('bad array/this value', function (st) { st['throws'](function () { assign(undefined); }, TypeError, 'undefined is not an object'); st['throws'](function () { assign(null); }, TypeError, 'null is not an object'); st.end(); }); runTests(assign, t); t.end(); }); apollo-server-demo/node_modules/object.assign/test/tests.js 0000644 0001750 0000144 00000017313 03560116604 023645 0 ustar andreh users 'use strict'; var hasSymbols = require('has-symbols/shams')(); var forEach = require('for-each'); var has = require('has'); module.exports = function (assign, t) { t.test('error cases', function (st) { st['throws'](function () { assign(null); }, TypeError, 'target must be an object'); st['throws'](function () { assign(undefined); }, TypeError, 'target must be an object'); st['throws'](function () { assign(null, {}); }, TypeError, 'target must be an object'); st['throws'](function () { assign(undefined, {}); }, TypeError, 'target must be an object'); st.end(); }); t.test('non-object target, no sources', function (st) { var bool = assign(true); st.equal(typeof bool, 'object', 'bool is object'); st.equal(Boolean.prototype.valueOf.call(bool), true, 'bool coerces to `true`'); var number = assign(1); st.equal(typeof number, 'object', 'number is object'); st.equal(Number.prototype.valueOf.call(number), 1, 'number coerces to `1`'); var string = assign('1'); st.equal(typeof string, 'object', 'number is object'); st.equal(String.prototype.valueOf.call(string), '1', 'number coerces to `"1"`'); st.end(); }); t.test('non-object target, with sources', function (st) { var signal = {}; st.test('boolean', function (st2) { var bool = assign(true, { a: signal }); st2.equal(typeof bool, 'object', 'bool is object'); st2.equal(Boolean.prototype.valueOf.call(bool), true, 'bool coerces to `true`'); st2.equal(bool.a, signal, 'source properties copied'); st2.end(); }); st.test('number', function (st2) { var number = assign(1, { a: signal }); st2.equal(typeof number, 'object', 'number is object'); st2.equal(Number.prototype.valueOf.call(number), 1, 'number coerces to `1`'); st2.equal(number.a, signal, 'source properties copied'); st2.end(); }); st.test('string', function (st2) { var string = assign('1', { a: signal }); st2.equal(typeof string, 'object', 'number is object'); st2.equal(String.prototype.valueOf.call(string), '1', 'number coerces to `"1"`'); st2.equal(string.a, signal, 'source properties copied'); st2.end(); }); st.end(); }); t.test('non-object sources', function (st) { st.deepEqual(assign({ a: 1 }, null, { b: 2 }), { a: 1, b: 2 }, 'ignores null source'); st.deepEqual(assign({ a: 1 }, { b: 2 }, undefined), { a: 1, b: 2 }, 'ignores undefined source'); st.end(); }); t.test('returns the modified target object', function (st) { var target = {}; var returned = assign(target, { a: 1 }); st.equal(returned, target, 'returned object is the same reference as the target object'); st.end(); }); t.test('has the right length', function (st) { st.equal(assign.length, 2, 'length is 2 => 2 required arguments'); st.end(); }); t.test('merge two objects', function (st) { var target = { a: 1 }; var returned = assign(target, { b: 2 }); st.deepEqual(returned, { a: 1, b: 2 }, 'returned object has properties from both'); st.end(); }); t.test('works with functions', function (st) { var target = function () {}; target.a = 1; var returned = assign(target, { b: 2 }); st.equal(target, returned, 'returned object is target'); st.equal(returned.a, 1); st.equal(returned.b, 2); st.end(); }); t.test('works with primitives', function (st) { var target = 2; var source = { b: 42 }; var returned = assign(target, source); st.equal(Object.prototype.toString.call(returned), '[object Number]', 'returned is object form of number primitive'); st.equal(Number(returned), target, 'returned and target have same valueOf'); st.equal(returned.b, source.b); st.end(); }); /* globals window */ t.test('works with window.location', { skip: typeof window === 'undefined' }, function (st) { var target = {}; assign(target, window.location); for (var prop in window.location) { if (has(window.location, prop)) { st.deepEqual(target[prop], window.location[prop], prop + ' is copied'); } } st.end(); }); t.test('merge N objects', function (st) { var target = { a: 1 }; var source1 = { b: 2 }; var source2 = { c: 3 }; var returned = assign(target, source1, source2); st.deepEqual(returned, { a: 1, b: 2, c: 3 }, 'returned object has properties from all sources'); st.end(); }); t.test('only iterates over own keys', function (st) { var Foo = function () {}; Foo.prototype.bar = true; var foo = new Foo(); foo.baz = true; var target = { a: 1 }; var returned = assign(target, foo); st.equal(returned, target, 'returned object is the same reference as the target object'); st.deepEqual(target, { a: 1, baz: true }, 'returned object has only own properties from both'); st.end(); }); t.test('includes enumerable symbols, after keys', { skip: !hasSymbols }, function (st) { var visited = []; var obj = {}; Object.defineProperty(obj, 'a', { enumerable: true, get: function () { visited.push('a'); return 42; } }); var symbol = Symbol('enumerable'); Object.defineProperty(obj, symbol, { enumerable: true, get: function () { visited.push(symbol); return Infinity; } }); var nonEnumSymbol = Symbol('non-enumerable'); Object.defineProperty(obj, nonEnumSymbol, { enumerable: false, get: function () { visited.push(nonEnumSymbol); return -Infinity; } }); var target = assign({}, obj); st.deepEqual(visited, ['a', symbol], 'key is visited first, then symbol'); st.equal(target.a, 42, 'target.a is 42'); st.equal(target[symbol], Infinity, 'target[symbol] is Infinity'); st.notEqual(target[nonEnumSymbol], -Infinity, 'target[nonEnumSymbol] is not -Infinity'); st.end(); }); t.test('does not fail when symbols are not present', { skip: !Object.isFrozen || Object.isFrozen(Object) }, function (st) { var getSyms; if (hasSymbols) { getSyms = Object.getOwnPropertySymbols; delete Object.getOwnPropertySymbols; } var visited = []; var obj = {}; Object.defineProperty(obj, 'a', { enumerable: true, get: function () { visited.push('a'); return 42; } }); var keys = ['a']; if (hasSymbols) { var symbol = Symbol('sym'); Object.defineProperty(obj, symbol, { enumerable: true, get: function () { visited.push(symbol); return Infinity; } }); keys.push(symbol); } var target = assign({}, obj); st.deepEqual(visited, keys, 'assign visits expected keys'); st.equal(target.a, 42, 'target.a is 42'); if (hasSymbols) { st.equal(target[symbol], Infinity); Object.getOwnPropertySymbols = getSyms; } st.end(); }); t.test('preserves correct property enumeration order', function (st) { var str = 'abcdefghijklmnopqrst'; var letters = {}; forEach(str.split(''), function (letter) { letters[letter] = letter; }); var n = 5; st.comment('run the next test ' + n + ' times'); var object = assign({}, letters); var actual = ''; for (var k in object) { actual += k; } for (var i = 0; i < n; ++i) { st.equal(actual, str, 'property enumeration order should be followed'); } st.end(); }); t.test('checks enumerability and existence, in case of modification during [[Get]]', { skip: !Object.defineProperty }, function (st) { var targetBvalue = {}; var targetCvalue = {}; var target = { b: targetBvalue, c: targetCvalue }; var source = {}; Object.defineProperty(source, 'a', { enumerable: true, get: function () { delete this.b; Object.defineProperty(this, 'c', { enumerable: false }); return 'a'; } }); var sourceBvalue = {}; var sourceCvalue = {}; source.b = sourceBvalue; source.c = sourceCvalue; var result = assign(target, source); st.equal(result, target, 'sanity check: result is === target'); st.equal(result.b, targetBvalue, 'target key not overwritten by deleted source key'); st.equal(result.c, targetCvalue, 'target key not overwritten by non-enumerable source key'); st.end(); }); }; apollo-server-demo/node_modules/object.assign/test/shimmed.js 0000644 0001750 0000144 00000003572 03560116604 024133 0 ustar andreh users 'use strict'; var assign = require('../'); assign.shim(); var test = require('tape'); var defineProperties = require('define-properties'); var isEnumerable = Object.prototype.propertyIsEnumerable; var functionsHaveNames = require('functions-have-names')(); var runTests = require('./tests'); test('shimmed', function (t) { t.equal(Object.assign.length, 2, 'Object.assign has a length of 2'); t.test('Function name', { skip: !functionsHaveNames }, function (st) { st.equal(Object.assign.name, 'assign', 'Object.assign has name "assign"'); st.end(); }); t.test('enumerability', { skip: !defineProperties.supportsDescriptors }, function (et) { et.equal(false, isEnumerable.call(Object, 'assign'), 'Object.assign is not enumerable'); et.end(); }); var supportsStrictMode = (function () { return typeof this === 'undefined'; }()); t.test('bad object value', { skip: !supportsStrictMode }, function (st) { st['throws'](function () { return Object.assign(undefined); }, TypeError, 'undefined is not an object'); st['throws'](function () { return Object.assign(null); }, TypeError, 'null is not an object'); st.end(); }); // v8 in node 0.8 and 0.10 have non-enumerable string properties var stringCharsAreEnumerable = isEnumerable.call('xy', 0); t.test('when Object.assign is present and has pending exceptions', { skip: !stringCharsAreEnumerable || !Object.preventExtensions }, function (st) { /* * Firefox 37 still has "pending exception" logic in its Object.assign implementation, * which is 72% slower than our shim, and Firefox 40's native implementation. */ var thrower = Object.preventExtensions({ 1: '2' }); var error; try { Object.assign(thrower, 'xy'); } catch (e) { error = e; } st.equal(error instanceof TypeError, true, 'error is TypeError'); st.equal(thrower[1], '2', 'thrower[1] === "2"'); st.end(); }); runTests(Object.assign, t); t.end(); }); apollo-server-demo/node_modules/object.assign/test/ses-compat.js 0000644 0001750 0000144 00000000334 03560116604 024551 0 ustar andreh users 'use strict'; /* globals lockdown */ // requiring ses exposes "lockdown" on the global require('ses'); // lockdown freezes the primordials lockdown({ errorTaming: 'unsafe' }); // initialize the module require('./'); apollo-server-demo/node_modules/object.assign/test/native.js 0000644 0001750 0000144 00000003514 03560116604 023767 0 ustar andreh users 'use strict'; var test = require('tape'); var defineProperties = require('define-properties'); var isEnumerable = Object.prototype.propertyIsEnumerable; var functionsHaveNames = require('functions-have-names')(); var runTests = require('./tests'); test('native', function (t) { t.equal(Object.assign.length, 2, 'Object.assign has a length of 2'); t.test('Function name', { skip: !functionsHaveNames }, function (st) { st.equal(Object.assign.name, 'assign', 'Object.assign has name "assign"'); st.end(); }); t.test('enumerability', { skip: !defineProperties.supportsDescriptors }, function (et) { et.equal(false, isEnumerable.call(Object, 'assign'), 'Object.assign is not enumerable'); et.end(); }); var supportsStrictMode = (function () { return typeof this === 'undefined'; }()); t.test('bad object value', { skip: !supportsStrictMode }, function (st) { st['throws'](function () { return Object.assign(undefined); }, TypeError, 'undefined is not an object'); st['throws'](function () { return Object.assign(null); }, TypeError, 'null is not an object'); st.end(); }); // v8 in node 0.8 and 0.10 have non-enumerable string properties var stringCharsAreEnumerable = isEnumerable.call('xy', 0); t.test('when Object.assign is present and has pending exceptions', { skip: !stringCharsAreEnumerable || !Object.preventExtensions }, function (st) { /* * Firefox 37 still has "pending exception" logic in its Object.assign implementation, * which is 72% slower than our shim, and Firefox 40's native implementation. */ var thrower = Object.preventExtensions({ 1: '2' }); var error; try { Object.assign(thrower, 'xy'); } catch (e) { error = e; } st.equal(error instanceof TypeError, true, 'error is TypeError'); st.equal(thrower[1], '2', 'thrower[1] === "2"'); st.end(); }); runTests(Object.assign, t); t.end(); }); apollo-server-demo/node_modules/object.assign/.eslintignore 0000644 0001750 0000144 00000000006 03560116604 023660 0 ustar andreh users dist/ apollo-server-demo/node_modules/object.assign/CHANGELOG.md 0000644 0001750 0000144 00000017210 03560116604 022773 0 ustar andreh users 4.1.2 / 2020-10-30 ================== * [Refactor] use extracted `call-bind` instead of full `es-abstract` * [Dev Deps] update `eslint`, `ses`, `browserify` * [Tests] run tests in SES * [Tests] ses-compat: show error stacks 4.1.1 / 2020-09-11 ================== * [Fix] avoid mutating `Object.assign` in modern engines * [Refactor] use `callBind` from `es-abstract` instead of `function-bind` * [Deps] update `has-symbols`, `object-keys`, `define-properties` * [meta] add `funding` field, FUNDING.yml * [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `@es-shims/api`, `browserify`, `covert`, `for-each`, `is`, `tape`, `functions-have-names`; add `aud`, `safe-publish-latest`; remove `jscs` * [actions] add Require Allow Edits workflow * [actions] add automatic rebasing / merge commit blocking * [Tests] ses-compat - add test to ensure package initializes correctly after ses lockdown (#77) * [Tests] Add passing test for a source of `window.location` (#68) * [Tests] use shared travis-ci config * [Tests] use `npx aud` instead of `npm audit` with hoops or `nsp` * [Tests] use `functions-have-names` 4.1.0 / 2017-12-21 ================== * [New] add `auto` entry point (#52) * [Refactor] Use `has-symbols` module * [Deps] update `function-bind`, `object-keys` * [Dev Deps] update `@es-shims/api`, `browserify`, `nsp`, `eslint`, `@ljharb/eslint-config`, `is` * [Tests] up to `node` `v9.3`, `v8.9`, `v6.12`; use `nvm install-latest-npm`; pin included builds to LTS 4.0.4 / 2016-07-04 ================== * [Fix] Cache original `getOwnPropertySymbols`, and use that when `Object.getOwnPropertySymbols` is unavailable * [Deps] update `object-keys` * [Dev Deps] update `eslint`, `get-own-property-symbols`, `core-js`, `jscs`, `nsp`, `browserify`, `@ljharb/eslint-config`, `tape`, `@es-shims/api` * [Tests] up to `node` `v6.2`, `v5.10`, `v4.4` * [Tests] run sham tests on node 0.10 * [Tests] use pretest/posttest for linting/security 4.0.3 / 2015-10-21 ================== * [Fix] Support core-js's Symbol sham (#17) * [Fix] Ensure that properties removed or made non-enumerable during enumeration are not assigned (#16) * [Fix] Avoid looking up keys and values more than once * [Tests] Avoid using `reduce` so `npm run test:shams:corejs` passes in `node` `v0.8` ([core-js#122](https://github.com/zloirock/core-js/issues/122)) * [Tests] Refactor to use my conventional structure that separates shimmed, implementation, and common tests * [Tests] Create `npm run test:shams` and better organize tests for symbol shams * [Tests] Remove `nsp` in favor of `requiresafe` 4.0.2 / 2015-10-20 ================== * [Fix] Ensure correct property enumeration order, particularly in v8 (#15) * [Deps] update `object-keys`, `define-properties` * [Dev Deps] update `browserify`, `is`, `tape`, `jscs`, `eslint`, `@ljharb/eslint-config` * [Tests] up to `io.js` `v3.3`, `node` `v4.2` 4.0.1 / 2015-08-16 ================== * [Docs] Add `Symbol` note to readme 4.0.0 / 2015-08-15 ================== * [Breaking] Implement the [es-shim API](es-shims/api). * [Robustness] Make implementation robust against later modification of environment methods. * [Refactor] Move implementation to `implementation.js` * [Docs] Switch from vb.teelaun.ch to versionbadg.es for the npm version badge SVG * [Deps] update `object-keys`, `define-properties` * [Dev Deps] update `browserify`, `tape`, `eslint`, `jscs`, `browserify` * [Tests] Add `npm run tests-only` * [Tests] use my personal shared `eslint` config. * [Tests] up to `io.js` `v3.0` 3.0.1 / 2015-06-28 ================== * Cache `Object` and `Array#push` to make the shim more robust. * [Fix] Remove use of `Array#filter`, which isn't in ES3. * [Deps] Update `object-keys`, `define-properties` * [Dev Deps] Update `get-own-property-symbols`, `browserify`, `eslint`, `nsp` * [Tests] Test up to `io.js` `v2.3` * [Tests] Adding `Object.assign` tests for non-object targets, per https://github.com/paulmillr/es6-shim/issues/348 3.0.0 / 2015-05-20 ================== * Attempt to feature-detect Symbols, even if `typeof Symbol() !== 'symbol'` (#12) * Make a separate `hasSymbols` internal module * Update `browserify`, `eslint` 2.0.3 / 2015-06-28 ================== * Cache `Object` and `Array#push` to make the shim more robust. * [Fix] Remove use of `Array#filter`, which isn't in ES3 * [Deps] Update `object-keys`, `define-properties` * [Dev Deps] Update `browserify`, `nsp`, `eslint` * [Tests] Test up to `io.js` `v2.3` 2.0.2 / 2015-05-20 ================== * Make sure `.shim` is non-enumerable. * Refactor `.shim` implementation to use `define-properties` predicates, rather than `delete`ing the original. * Update docs to match spec/implementation. (#11) * Add `npm run eslint` * Test up to `io.js` `v2.0` * Update `jscs`, `browserify`, `covert` 2.0.1 / 2015-04-12 ================== * Make sure non-enumerable Symbols are excluded. 2.0.0 / 2015-04-12 ================== * Make sure the shim function overwrites a broken implementation with pending exceptions. * Ensure shim is not enumerable using `define-properties` * Ensure `Object.assign` includes symbols. * All grade A-supported `node`/`iojs` versions now ship with an `npm` that understands `^`. * Run `travis-ci` tests on `iojs` and `node` v0.12; speed up builds; allow 0.8 failures. * Add `npm run security` via `nsp` * Update `browserify`, `jscs`, `tape`, `object-keys`, `is` 1.1.1 / 2014-12-14 ================== * Actually include the browser build in `npm` 1.1.0 / 2014-12-14 ================== * Add `npm run build`, and build an automatic-shimming browser distribution as part of the npm publish process. * Update `is`, `jscs` 1.0.3 / 2014-11-29 ================== * Revert "optimize --production installs" 1.0.2 / 2014-11-27 ================== * Update `jscs`, `is`, `object-keys`, `tape` * Add badges to README * Name URLs in README * Lock `covert` to `v1.0.0` * Optimize --production installs 1.0.1 / 2014-08-26 ================== * Update `is`, `covert` 1.0.0 / 2014-08-07 ================== * Update `object-keys`, `tape` 0.5.0 / 2014-07-31 ================== * Object.assign no longer throws on null or undefined sources, per https://bugs.ecmascript.org/show_bug.cgi?id=3096 0.4.3 / 2014-07-30 ================== * Don’t modify vars in the function signature, since it deoptimizes v8 0.4.2 / 2014-07-30 ================== * Fixing the version number: v0.4.2 0.4.1 / 2014-07-19 ================== * Revert "Use the native Object.keys if it’s available." 0.4.0 / 2014-07-19 ================== * Use the native Object.keys if it’s available. * Fixes [#2](https://github.com/ljharb/object.assign/issues/2). * Adding failing tests for [#2](https://github.com/ljharb/object.assign/issues/2). * Fix indentation. * Adding `npm run lint` * Update `tape`, `covert` * README: Use SVG badge for Travis [#1](https://github.com/ljharb/object.assign/issues/1) from mathiasbynens/patch-1 0.3.1 / 2014-04-10 ================== * Object.assign does partially modify objects if it throws, per https://twitter.com/awbjs/status/454320863093862400 0.3.0 / 2014-04-10 ================== * Update with newest ES6 behavior - Object.assign now takes a variable number of source objects. * Update `tape` * Make sure old and unstable nodes don’t fail Travis 0.2.1 / 2014-03-16 ================== * Let object-keys handle the fallback * Update dependency badges * Adding bower.json 0.2.0 / 2014-03-16 ================== * Use a for loop, because ES3 browsers don’t have "reduce" 0.1.1 / 2014-03-14 ================== * Updating readme 0.1.0 / 2014-03-14 ================== * Initial release. apollo-server-demo/node_modules/object.assign/hasSymbols.js 0000644 0001750 0000144 00000003122 03560116604 023641 0 ustar andreh users 'use strict'; var keys = require('object-keys'); module.exports = function hasSymbols() { if (typeof Symbol !== 'function' || typeof Object.getOwnPropertySymbols !== 'function') { return false; } if (typeof Symbol.iterator === 'symbol') { return true; } var obj = {}; var sym = Symbol('test'); var symObj = Object(sym); if (typeof sym === 'string') { return false; } if (Object.prototype.toString.call(sym) !== '[object Symbol]') { return false; } if (Object.prototype.toString.call(symObj) !== '[object Symbol]') { return false; } /* * temp disabled per https://github.com/ljharb/object.assign/issues/17 * if (sym instanceof Symbol) { return false; } * temp disabled per https://github.com/WebReflection/get-own-property-symbols/issues/4 * if (!(symObj instanceof Symbol)) { return false; } */ var symVal = 42; obj[sym] = symVal; for (sym in obj) { return false; } // eslint-disable-line no-unreachable-loop if (keys(obj).length !== 0) { return false; } if (typeof Object.keys === 'function' && Object.keys(obj).length !== 0) { return false; } if (typeof Object.getOwnPropertyNames === 'function' && Object.getOwnPropertyNames(obj).length !== 0) { return false; } var syms = Object.getOwnPropertySymbols(obj); if (syms.length !== 1 || syms[0] !== sym) { return false; } if (!Object.prototype.propertyIsEnumerable.call(obj, sym)) { return false; } if (typeof Object.getOwnPropertyDescriptor === 'function') { var descriptor = Object.getOwnPropertyDescriptor(obj, sym); if (descriptor.value !== symVal || descriptor.enumerable !== true) { return false; } } return true; }; apollo-server-demo/node_modules/object.assign/polyfill.js 0000644 0001750 0000144 00000002423 03560116604 023352 0 ustar andreh users 'use strict'; var implementation = require('./implementation'); var lacksProperEnumerationOrder = function () { if (!Object.assign) { return false; } /* * v8, specifically in node 4.x, has a bug with incorrect property enumeration order * note: this does not detect the bug unless there's 20 characters */ var str = 'abcdefghijklmnopqrst'; var letters = str.split(''); var map = {}; for (var i = 0; i < letters.length; ++i) { map[letters[i]] = letters[i]; } var obj = Object.assign({}, map); var actual = ''; for (var k in obj) { actual += k; } return str !== actual; }; var assignHasPendingExceptions = function () { if (!Object.assign || !Object.preventExtensions) { return false; } /* * Firefox 37 still has "pending exception" logic in its Object.assign implementation, * which is 72% slower than our shim, and Firefox 40's native implementation. */ var thrower = Object.preventExtensions({ 1: 2 }); try { Object.assign(thrower, 'xy'); } catch (e) { return thrower[1] === 'y'; } return false; }; module.exports = function getPolyfill() { if (!Object.assign) { return implementation; } if (lacksProperEnumerationOrder()) { return implementation; } if (assignHasPendingExceptions()) { return implementation; } return Object.assign; }; apollo-server-demo/node_modules/streamsearch/ 0000755 0001750 0000144 00000000000 14067647701 021124 5 ustar andreh users apollo-server-demo/node_modules/streamsearch/LICENSE 0000644 0001750 0000144 00000002053 12132565775 022132 0 ustar andreh users Copyright Brian White. All rights reserved. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/streamsearch/README.md 0000644 0001750 0000144 00000004404 12132565775 022406 0 ustar andreh users Description =========== streamsearch is a module for [node.js](http://nodejs.org/) that allows searching a stream using the Boyer-Moore-Horspool algorithm. This module is based heavily on the Streaming Boyer-Moore-Horspool C++ implementation by Hongli Lai [here](https://github.com/FooBarWidget/boyer-moore-horspool). Requirements ============ * [node.js](http://nodejs.org/) -- v0.8.0 or newer Installation ============ npm install streamsearch Example ======= ```javascript var StreamSearch = require('streamsearch'), inspect = require('util').inspect; var needle = new Buffer([13, 10]), // CRLF s = new StreamSearch(needle), chunks = [ new Buffer('foo'), new Buffer(' bar'), new Buffer('\r'), new Buffer('\n'), new Buffer('baz, hello\r'), new Buffer('\n world.'), new Buffer('\r\n Node.JS rules!!\r\n\r\n') ]; s.on('info', function(isMatch, data, start, end) { if (data) console.log('data: ' + inspect(data.toString('ascii', start, end))); if (isMatch) console.log('match!'); }); for (var i = 0, len = chunks.length; i < len; ++i) s.push(chunks[i]); // output: // // data: 'foo' // data: ' bar' // match! // data: 'baz, hello' // match! // data: ' world.' // match! // data: ' Node.JS rules!!' // match! // data: '' // match! ``` API === Events ------ * **info**(< _boolean_ >isMatch[, < _Buffer_ >chunk, < _integer_ >start, < _integer_ >end]) - A match _may_ or _may not_ have been made. In either case, a preceding `chunk` of data _may_ be available that did not match the needle. Data (if available) is in `chunk` between `start` (inclusive) and `end` (exclusive). Properties ---------- * **maxMatches** - < _integer_ > - The maximum number of matches. Defaults to Infinity. * **matches** - < _integer_ > - The current match count. Functions --------- * **(constructor)**(< _mixed_ >needle) - Creates and returns a new instance for searching for a _Buffer_ or _string_ `needle`. * **push**(< _Buffer_ >chunk) - _integer_ - Processes `chunk`. The return value is the last processed index in `chunk` + 1. * **reset**() - _(void)_ - Resets internal state. Useful for when you wish to start searching a new/different stream for example. apollo-server-demo/node_modules/streamsearch/package.json 0000644 0001750 0000144 00000001241 12132565775 023411 0 ustar andreh users { "name": "streamsearch", "version": "0.1.2", "author": "Brian White <mscdex@mscdex.net>", "description": "Streaming Boyer-Moore-Horspool searching for node.js", "main": "./lib/sbmh", "engines": { "node" : ">=0.8.0" }, "keywords": [ "stream", "horspool", "boyer-moore-horspool", "boyer-moore", "search" ], "licenses": [ { "type": "MIT", "url": "http://github.com/mscdex/streamsearch/raw/master/LICENSE" } ], "repository": { "type": "git", "url": "http://github.com/mscdex/streamsearch.git" } ,"_resolved": "https://registry.npmjs.org/streamsearch/-/streamsearch-0.1.2.tgz" ,"_integrity": "sha1-gIudDlb8Jz2Am6VzOOkpkZoanxo=" ,"_from": "streamsearch@0.1.2" } apollo-server-demo/node_modules/streamsearch/lib/ 0000755 0001750 0000144 00000000000 14067647701 021672 5 ustar andreh users apollo-server-demo/node_modules/streamsearch/lib/sbmh.js 0000644 0001750 0000144 00000014162 12132565775 023166 0 ustar andreh users /* Based heavily on the Streaming Boyer-Moore-Horspool C++ implementation by Hongli Lai at: https://github.com/FooBarWidget/boyer-moore-horspool */ var EventEmitter = require('events').EventEmitter, inherits = require('util').inherits; function jsmemcmp(buf1, pos1, buf2, pos2, num) { for (var i = 0; i < num; ++i, ++pos1, ++pos2) if (buf1[pos1] !== buf2[pos2]) return false; return true; } function SBMH(needle) { if (typeof needle === 'string') needle = new Buffer(needle); var i, j, needle_len = needle.length; this.maxMatches = Infinity; this.matches = 0; this._occ = new Array(256); this._lookbehind_size = 0; this._needle = needle; this._bufpos = 0; this._lookbehind = new Buffer(needle_len); // Initialize occurrence table. for (j = 0; j < 256; ++j) this._occ[j] = needle_len; // Populate occurrence table with analysis of the needle, // ignoring last letter. if (needle_len >= 1) { for (i = 0; i < needle_len - 1; ++i) this._occ[needle[i]] = needle_len - 1 - i; } } inherits(SBMH, EventEmitter); SBMH.prototype.reset = function() { this._lookbehind_size = 0; this.matches = 0; this._bufpos = 0; }; SBMH.prototype.push = function(chunk, pos) { var r, chlen; if (!Buffer.isBuffer(chunk)) chunk = new Buffer(chunk, 'binary'); chlen = chunk.length; this._bufpos = pos || 0; while (r !== chlen && this.matches < this.maxMatches) r = this._sbmh_feed(chunk); return r; }; SBMH.prototype._sbmh_feed = function(data) { var len = data.length, needle = this._needle, needle_len = needle.length; // Positive: points to a position in `data` // pos == 3 points to data[3] // Negative: points to a position in the lookbehind buffer // pos == -2 points to lookbehind[lookbehind_size - 2] var pos = -this._lookbehind_size, last_needle_char = needle[needle_len - 1], occ = this._occ, lookbehind = this._lookbehind; if (pos < 0) { // Lookbehind buffer is not empty. Perform Boyer-Moore-Horspool // search with character lookup code that considers both the // lookbehind buffer and the current round's haystack data. // // Loop until // there is a match. // or until // we've moved past the position that requires the // lookbehind buffer. In this case we switch to the // optimized loop. // or until // the character to look at lies outside the haystack. while (pos < 0 && pos <= len - needle_len) { var ch = this._sbmh_lookup_char(data, pos + needle_len - 1); if (ch === last_needle_char && this._sbmh_memcmp(data, pos, needle_len - 1)) { this._lookbehind_size = 0; ++this.matches; if (pos > -this._lookbehind_size) this.emit('info', true, lookbehind, 0, this._lookbehind_size + pos); else this.emit('info', true); this._bufpos = pos + needle_len; return pos + needle_len; } else pos += occ[ch]; } // No match. if (pos < 0) { // There's too few data for Boyer-Moore-Horspool to run, // so let's use a different algorithm to skip as much as // we can. // Forward pos until // the trailing part of lookbehind + data // looks like the beginning of the needle // or until // pos == 0 while (pos < 0 && !this._sbmh_memcmp(data, pos, len - pos)) pos++; } if (pos >= 0) { // Discard lookbehind buffer. this.emit('info', false, lookbehind, 0, this._lookbehind_size); this._lookbehind_size = 0; } else { // Cut off part of the lookbehind buffer that has // been processed and append the entire haystack // into it. var bytesToCutOff = this._lookbehind_size + pos; if (bytesToCutOff > 0) { // The cut off data is guaranteed not to contain the needle. this.emit('info', false, lookbehind, 0, bytesToCutOff); } lookbehind.copy(lookbehind, 0, bytesToCutOff, this._lookbehind_size - bytesToCutOff); this._lookbehind_size -= bytesToCutOff; data.copy(lookbehind, this._lookbehind_size); this._lookbehind_size += len; this._bufpos = len; return len; } } if (pos >= 0) pos += this._bufpos; // Lookbehind buffer is now empty. Perform Boyer-Moore-Horspool // search with optimized character lookup code that only considers // the current round's haystack data. while (pos <= len - needle_len) { var ch = data[pos + needle_len - 1]; if (ch === last_needle_char && data[pos] === needle[0] && jsmemcmp(needle, 0, data, pos, needle_len - 1)) { ++this.matches; if (pos > 0) this.emit('info', true, data, this._bufpos, pos); else this.emit('info', true); this._bufpos = pos + needle_len; return pos + needle_len; } else pos += occ[ch]; } // There was no match. If there's trailing haystack data that we cannot // match yet using the Boyer-Moore-Horspool algorithm (because the trailing // data is less than the needle size) then match using a modified // algorithm that starts matching from the beginning instead of the end. // Whatever trailing data is left after running this algorithm is added to // the lookbehind buffer. if (pos < len) { while (pos < len && (data[pos] !== needle[0] || !jsmemcmp(data, pos, needle, 0, len - pos))) { ++pos; } if (pos < len) { data.copy(lookbehind, 0, pos, pos + (len - pos)); this._lookbehind_size = len - pos; } } // Everything until pos is guaranteed not to contain needle data. if (pos > 0) this.emit('info', false, data, this._bufpos, pos < len ? pos : len); this._bufpos = len; return len; }; SBMH.prototype._sbmh_lookup_char = function(data, pos) { if (pos < 0) return this._lookbehind[this._lookbehind_size + pos]; else return data[pos]; } SBMH.prototype._sbmh_memcmp = function(data, pos, len) { var i = 0; while (i < len) { if (this._sbmh_lookup_char(data, pos + i) === this._needle[i]) ++i; else return false; } return true; } module.exports = SBMH; apollo-server-demo/node_modules/apollo-server-env/ 0000755 0001750 0000144 00000000000 14067647700 022022 5 ustar andreh users apollo-server-demo/node_modules/apollo-server-env/dist/ 0000755 0001750 0000144 00000000000 14067647700 022765 5 ustar andreh users apollo-server-demo/node_modules/apollo-server-env/dist/fetch.d.ts 0000644 0001750 0000144 00000005712 03560116604 024643 0 ustar andreh users import { Agent as HttpAgent } from 'http'; import { Agent as HttpsAgent } from 'https'; export declare function fetch( input: RequestInfo, init?: RequestInit, ): Promise<Response>; export type RequestAgent = HttpAgent | HttpsAgent; export type RequestInfo = Request | string; export declare class Headers implements Iterable<[string, string]> { constructor(init?: HeadersInit); append(name: string, value: string): void; delete(name: string): void; get(name: string): string | null; has(name: string): boolean; set(name: string, value: string): void; entries(): Iterator<[string, string]>; keys(): Iterator<string>; values(): Iterator<[string]>; [Symbol.iterator](): Iterator<[string, string]>; } export type HeadersInit = Headers | string[][] | { [name: string]: string }; export declare class Body { readonly bodyUsed: boolean; arrayBuffer(): Promise<ArrayBuffer>; json(): Promise<any>; text(): Promise<string>; } export declare class Request extends Body { constructor(input: Request | string, init?: RequestInit); readonly method: string; readonly url: string; readonly headers: Headers; clone(): Request; } export interface RequestInit { method?: string; headers?: HeadersInit; body?: BodyInit; mode?: RequestMode; credentials?: RequestCredentials; cache?: RequestCache; redirect?: RequestRedirect; referrer?: string; referrerPolicy?: ReferrerPolicy; integrity?: string; // The following properties are node-fetch extensions follow?: number; timeout?: number; compress?: boolean; size?: number; agent?: RequestAgent | false; // Cloudflare Workers accept a `cf` property to control Cloudflare features // See https://developers.cloudflare.com/workers/reference/cloudflare-features/ cf?: { [key: string]: any; }; } export type RequestMode = 'navigate' | 'same-origin' | 'no-cors' | 'cors'; export type RequestCredentials = 'omit' | 'same-origin' | 'include'; export type RequestCache = | 'default' | 'no-store' | 'reload' | 'no-cache' | 'force-cache' | 'only-if-cached'; export type RequestRedirect = 'follow' | 'error' | 'manual'; export type ReferrerPolicy = | '' | 'no-referrer' | 'no-referrer-when-downgrade' | 'same-origin' | 'origin' | 'strict-origin' | 'origin-when-cross-origin' | 'strict-origin-when-cross-origin' | 'unsafe-url'; export declare class Response extends Body { constructor(body?: BodyInit, init?: ResponseInit); static error(): Response; static redirect(url: string, status?: number): Response; readonly url: string; readonly redirected: boolean; readonly status: number; readonly ok: boolean; readonly statusText: string; readonly headers: Headers; clone(): Response; } export interface ResponseInit { headers?: HeadersInit; status?: number; statusText?: string; // Although this isn't part of the spec, `node-fetch` accepts a `url` property url?: string; } export type BodyInit = ArrayBuffer | ArrayBufferView | string; apollo-server-demo/node_modules/apollo-server-env/dist/index.js 0000644 0001750 0000144 00000002113 03560116604 024415 0 ustar andreh users "use strict"; var __createBinding = (this && this.__createBinding) || (Object.create ? (function(o, m, k, k2) { if (k2 === undefined) k2 = k; Object.defineProperty(o, k2, { enumerable: true, get: function() { return m[k]; } }); }) : (function(o, m, k, k2) { if (k2 === undefined) k2 = k; o[k2] = m[k]; })); var __exportStar = (this && this.__exportStar) || function(m, exports) { for (var p in m) if (p !== "default" && !exports.hasOwnProperty(p)) __createBinding(exports, m, p); }; var __importDefault = (this && this.__importDefault) || function (mod) { return (mod && mod.__esModule) ? mod : { "default": mod }; }; Object.defineProperty(exports, "__esModule", { value: true }); require("./polyfills/Object.values"); require("./polyfills/Object.entries"); const runtimeSupportsPromisify_1 = __importDefault(require("./utils/runtimeSupportsPromisify")); if (!runtimeSupportsPromisify_1.default) { require('util.promisify').shim(); } __exportStar(require("./polyfills/fetch"), exports); __exportStar(require("./polyfills/url"), exports); //# sourceMappingURL=index.js.map apollo-server-demo/node_modules/apollo-server-env/dist/polyfills/ 0000755 0001750 0000144 00000000000 14067647700 025002 5 ustar andreh users apollo-server-demo/node_modules/apollo-server-env/dist/polyfills/Object.entries.js 0000644 0001750 0000144 00000000322 03560116604 030201 0 ustar andreh users "use strict"; if (!global.Object.entries) { global.Object.entries = function (object) { return Object.keys(object).map(key => [key, object[key]]); }; } //# sourceMappingURL=Object.entries.js.map apollo-server-demo/node_modules/apollo-server-env/dist/polyfills/Object.values.js.map 0000644 0001750 0000144 00000000535 03560116604 030611 0 ustar andreh users {"version":3,"file":"Object.values.js","sourceRoot":"","sources":["../../src/polyfills/Object.values.ts"],"names":[],"mappings":";AAAA,IAAI,CAAC,MAAM,CAAC,MAAM,CAAC,MAAM,EAAE;IACzB,MAAM,CAAC,MAAM,CAAC,MAAM,GAAG,UAAS,MAAW;QACzC,OAAO,MAAM,CAAC,IAAI,CAAC,MAAM,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,EAAE,CAAC,MAAM,CAAC,GAAG,CAAC,CAAC,CAAC;IACrD,CAAC,CAAC;CACH"} apollo-server-demo/node_modules/apollo-server-env/dist/polyfills/Object.values.js 0000644 0001750 0000144 00000000310 03560116604 030024 0 ustar andreh users "use strict"; if (!global.Object.values) { global.Object.values = function (object) { return Object.keys(object).map(key => object[key]); }; } //# sourceMappingURL=Object.values.js.map apollo-server-demo/node_modules/apollo-server-env/dist/polyfills/Object.entries.js.map 0000644 0001750 0000144 00000000564 03560116604 030765 0 ustar andreh users {"version":3,"file":"Object.entries.js","sourceRoot":"","sources":["../../src/polyfills/Object.entries.ts"],"names":[],"mappings":";AAAA,IAAI,CAAC,MAAM,CAAC,MAAM,CAAC,OAAO,EAAE;IAC1B,MAAM,CAAC,MAAM,CAAC,OAAO,GAAG,UAAS,MAAW;QAC1C,OAAO,MAAM,CAAC,IAAI,CAAC,MAAM,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,EAAE,CAAC,CAAC,GAAG,EAAE,MAAM,CAAC,GAAG,CAAC,CAAkB,CAAC,CAAC;IAC7E,CAAC,CAAC;CACH"} apollo-server-demo/node_modules/apollo-server-env/dist/polyfills/url.js.map 0000644 0001750 0000144 00000000247 03560116604 026707 0 ustar andreh users {"version":3,"file":"url.js","sourceRoot":"","sources":["../../src/polyfills/url.js"],"names":[],"mappings":";;AAAA,2BAA2C;AAAlC,0FAAA,GAAG,OAAA;AAAE,sGAAA,eAAe,OAAA"} apollo-server-demo/node_modules/apollo-server-env/dist/polyfills/fetch.js 0000644 0001750 0000144 00000001150 03560116604 026414 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); var node_fetch_1 = require("node-fetch"); Object.defineProperty(exports, "fetch", { enumerable: true, get: function () { return node_fetch_1.default; } }); Object.defineProperty(exports, "Request", { enumerable: true, get: function () { return node_fetch_1.Request; } }); Object.defineProperty(exports, "Response", { enumerable: true, get: function () { return node_fetch_1.Response; } }); Object.defineProperty(exports, "Headers", { enumerable: true, get: function () { return node_fetch_1.Headers; } }); //# sourceMappingURL=fetch.js.map apollo-server-demo/node_modules/apollo-server-env/dist/polyfills/url.js 0000644 0001750 0000144 00000000552 03560116604 026132 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); var url_1 = require("url"); Object.defineProperty(exports, "URL", { enumerable: true, get: function () { return url_1.URL; } }); Object.defineProperty(exports, "URLSearchParams", { enumerable: true, get: function () { return url_1.URLSearchParams; } }); //# sourceMappingURL=url.js.map apollo-server-demo/node_modules/apollo-server-env/dist/polyfills/fetch.js.map 0000644 0001750 0000144 00000000325 03560116604 027173 0 ustar andreh users {"version":3,"file":"fetch.js","sourceRoot":"","sources":["../../src/polyfills/fetch.js"],"names":[],"mappings":";;AAAA,yCAA0E;AAAjE,mGAAA,OAAO,OAAS;AAAE,qGAAA,OAAO,OAAA;AAAE,sGAAA,QAAQ,OAAA;AAAE,qGAAA,OAAO,OAAA"} apollo-server-demo/node_modules/apollo-server-env/dist/index.d.ts 0000644 0001750 0000144 00000000134 03560116604 024652 0 ustar andreh users export * from './fetch'; export * from './url'; export * from './typescript-utility-types'; apollo-server-demo/node_modules/apollo-server-env/dist/index.browser.js.map 0000644 0001750 0000144 00000002574 03560116604 026666 0 ustar andreh users {"version":3,"file":"index.browser.js","sourceRoot":"","sources":["../src/index.browser.js"],"names":[],"mappings":";;;AAAA,IAAI,CAAC,MAAM,EAAE;IACX,MAAM,GAAG,IAAI,CAAC;CACf;AAED,IAAI,EAAE,KAAK,EAAE,OAAO,EAAE,QAAQ,EAAE,OAAO,EAAE,GAAG,EAAE,eAAe,EAAE,GAAG,MAAM,CAAC;AAEhE,sBAAK;AAAE,0BAAO;AAAE,4BAAQ;AAAE,0BAAO;AAAE,kBAAG;AAAE,0CAAe;AADhE,gBAAA,KAAK,GAAG,KAAK,CAAC,IAAI,CAAC,MAAM,CAAC,CAAC;AAG3B,IAAI,CAAC,MAAM,CAAC,OAAO,EAAE;IACnB,MAAM,CAAC,OAAO,GAAG,EAAE,CAAC;CACrB;AAED,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC,GAAG,EAAE;IACvB,MAAM,CAAC,OAAO,CAAC,GAAG,GAAG;QAEnB,QAAQ,EAAE,OAAO,GAAG,KAAK,WAAW,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC,CAAC,YAAY;KAC9D,CAAC;CACH;AAED,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC,OAAO,EAAE;IAC3B,MAAM,CAAC,OAAO,CAAC,OAAO,GAAG,EAAE,CAAC;CAC7B;AAED,IAAI,CAAC,MAAM,CAAC,OAAO,CAAC,MAAM,EAAE;IAE1B,MAAM,CAAC,OAAO,CAAC,MAAM,GAAG,SAAS,MAAM,CAAC,iBAAiB;QACvD,IAAI,SAAS,GAAG,IAAI,CAAC,GAAG,EAAE,GAAG,IAAI,CAAC;QAClC,IAAI,OAAO,GAAG,IAAI,CAAC,KAAK,CAAC,SAAS,CAAC,CAAC;QACpC,IAAI,WAAW,GAAG,IAAI,CAAC,KAAK,CAAC,CAAC,SAAS,GAAG,CAAC,CAAC,GAAG,GAAG,CAAC,CAAC;QACpD,IAAI,iBAAiB,EAAE;YACrB,OAAO,GAAG,OAAO,GAAG,iBAAiB,CAAC,CAAC,CAAC,CAAC;YACzC,WAAW,GAAG,WAAW,GAAG,iBAAiB,CAAC,CAAC,CAAC,CAAC;YACjD,IAAI,WAAW,GAAG,CAAC,EAAE;gBACnB,OAAO,EAAE,CAAC;gBACV,WAAW,IAAI,GAAG,CAAC;aACpB;SACF;QACD,OAAO,CAAC,OAAO,EAAE,WAAW,CAAC,CAAC;IAChC,CAAC,CAAC;CACH;AAED,IAAI,CAAC,MAAM,CAAC,EAAE,EAAE;IAEd,MAAM,CAAC,EAAE,GAAG,EAAE,CAAC;CAChB"} apollo-server-demo/node_modules/apollo-server-env/dist/utils/ 0000755 0001750 0000144 00000000000 14067647700 024125 5 ustar andreh users apollo-server-demo/node_modules/apollo-server-env/dist/utils/runtimeSupportsPromisify.js.map 0000644 0001750 0000144 00000001214 03560116604 032370 0 ustar andreh users {"version":3,"file":"runtimeSupportsPromisify.js","sourceRoot":"","sources":["../../src/utils/runtimeSupportsPromisify.ts"],"names":[],"mappings":";;AAAA,MAAM,wBAAwB,GAAG,CAAC,GAAG,EAAE;IACrC,IACE,OAAO;QACP,OAAO,CAAC,OAAO;QACf,OAAO,CAAC,OAAO,CAAC,IAAI,KAAK,MAAM;QAC/B,OAAO,CAAC,QAAQ;QAChB,OAAO,OAAO,CAAC,QAAQ,CAAC,IAAI,KAAK,QAAQ,EACzC;QACA,MAAM,CAAC,SAAS,CAAC,GAAG,OAAO,CAAC,QAAQ,CAAC,IAAI;aACtC,KAAK,CAAC,GAAG,EAAE,CAAC,CAAC;aACb,GAAG,CAAC,OAAO,CAAC,EAAE,CAAC,QAAQ,CAAC,OAAO,EAAE,EAAE,CAAC,CAAC,CAAC;QAEzC,IAAI,SAAS,IAAI,CAAC,EAAE;YAClB,OAAO,IAAI,CAAC;SACb;QACD,OAAO,KAAK,CAAC;KACd;IAID,OAAO,KAAK,CAAC;AACf,CAAC,CAAC,EAAE,CAAC;AAEL,kBAAe,wBAAwB,CAAC"} apollo-server-demo/node_modules/apollo-server-env/dist/utils/runtimeSupportsPromisify.js 0000644 0001750 0000144 00000001167 03560116604 031623 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); const runtimeSupportsPromisify = (() => { if (process && process.release && process.release.name === 'node' && process.versions && typeof process.versions.node === 'string') { const [nodeMajor] = process.versions.node .split('.', 1) .map(segment => parseInt(segment, 10)); if (nodeMajor >= 8) { return true; } return false; } return false; })(); exports.default = runtimeSupportsPromisify; //# sourceMappingURL=runtimeSupportsPromisify.js.map apollo-server-demo/node_modules/apollo-server-env/dist/url.d.ts 0000644 0001750 0000144 00000002232 03560116604 024346 0 ustar andreh users export declare class URL { constructor(input: string, base?: string | URL); hash: string; host: string; hostname: string; href: string; readonly origin: string; password: string; pathname: string; port: string; protocol: string; search: string; readonly searchParams: URLSearchParams; username: string; toString(): string; toJSON(): string; } export declare class URLSearchParams implements Iterable<[string, string]> { constructor(init?: URLSearchParamsInit); append(name: string, value: string): void; delete(name: string): void; entries(): IterableIterator<[string, string]>; forEach(callback: (value: string, name: string) => void): void; get(name: string): string | null; getAll(name: string): string[]; has(name: string): boolean; keys(): IterableIterator<string>; set(name: string, value: string): void; sort(): void; toString(): string; values(): IterableIterator<string>; [Symbol.iterator](): IterableIterator<[string, string]>; } export type URLSearchParamsInit = | URLSearchParams | string | { [key: string]: Object | Object[] | undefined } | Iterable<[string, Object]> | Array<[string, Object]>; apollo-server-demo/node_modules/apollo-server-env/dist/global.d.ts 0000644 0001750 0000144 00000002040 03560116604 025001 0 ustar andreh users declare function fetch( input: RequestInfo, init?: RequestInit, ): Promise<Response>; declare interface GlobalFetch { fetch(input: RequestInfo, init?: RequestInit): Promise<Response>; } type RequestInfo = import('./fetch').RequestInfo; type Headers = import('./fetch').Headers; type HeadersInit = import('./fetch').HeadersInit; type Body = import('./fetch').Body; type Request = import('./fetch').Request; type RequestAgent = import('./fetch').RequestAgent; type RequestInit = import('./fetch').RequestInit; type RequestMode = import('./fetch').RequestMode; type RequestCredentials = import('./fetch').RequestCredentials; type RequestCache = import('./fetch').RequestCache; type RequestRedirect = import('./fetch').RequestRedirect; type ReferrerPolicy = import('./fetch').ReferrerPolicy; type Response = import('./fetch').Response; type ResponseInit = import('./fetch').ResponseInit; type BodyInit = import('./fetch').BodyInit; type URLSearchParams = import('./url').URLSearchParams; type URLSearchParamsInit = import('./url').URLSearchParamsInit; apollo-server-demo/node_modules/apollo-server-env/dist/typescript-utility-types.d.ts 0000644 0001750 0000144 00000000173 03560116604 030617 0 ustar andreh users export type ValueOrPromise<T> = T | Promise<T>; export type WithRequired<T, K extends keyof T> = T & Required<Pick<T, K>>; apollo-server-demo/node_modules/apollo-server-env/dist/index.browser.js 0000644 0001750 0000144 00000002604 03560116604 026104 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); exports.URLSearchParams = exports.URL = exports.Headers = exports.Response = exports.Request = exports.fetch = void 0; if (!global) { global = self; } let { fetch, Request, Response, Headers, URL, URLSearchParams } = global; exports.fetch = fetch; exports.Request = Request; exports.Response = Response; exports.Headers = Headers; exports.URL = URL; exports.URLSearchParams = URLSearchParams; exports.fetch = fetch = fetch.bind(global); if (!global.process) { global.process = {}; } if (!global.process.env) { global.process.env = { NODE_ENV: typeof app !== 'undefined' ? app.env : 'production', }; } if (!global.process.version) { global.process.version = ''; } if (!global.process.hrtime) { global.process.hrtime = function hrtime(previousTimestamp) { var clocktime = Date.now() * 1e-3; var seconds = Math.floor(clocktime); var nanoseconds = Math.floor((clocktime % 1) * 1e9); if (previousTimestamp) { seconds = seconds - previousTimestamp[0]; nanoseconds = nanoseconds - previousTimestamp[1]; if (nanoseconds < 0) { seconds--; nanoseconds += 1e9; } } return [seconds, nanoseconds]; }; } if (!global.os) { global.os = {}; } //# sourceMappingURL=index.browser.js.map apollo-server-demo/node_modules/apollo-server-env/dist/index.js.map 0000644 0001750 0000144 00000000405 03560116604 025173 0 ustar andreh users {"version":3,"file":"index.js","sourceRoot":"","sources":["../src/index.ts"],"names":[],"mappings":";;;;;;;;;;;;;;;AAAA,qCAAmC;AACnC,sCAAoC;AAEpC,gGAAwE;AAExE,IAAI,CAAC,kCAAwB,EAAE;IAC7B,OAAO,CAAC,gBAAgB,CAAC,CAAC,IAAI,EAAE,CAAC;CAClC;AAED,oDAAkC;AAClC,kDAAgC"} apollo-server-demo/node_modules/apollo-server-env/LICENSE 0000644 0001750 0000144 00000002154 03560116604 023017 0 ustar andreh users The MIT License (MIT) Copyright (c) 2016-2020 Apollo Graph, Inc. (Formerly Meteor Development Group, Inc.) Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/apollo-server-env/src/ 0000755 0001750 0000144 00000000000 14067647700 022611 5 ustar andreh users apollo-server-demo/node_modules/apollo-server-env/src/fetch.d.ts 0000644 0001750 0000144 00000005712 03560116604 024467 0 ustar andreh users import { Agent as HttpAgent } from 'http'; import { Agent as HttpsAgent } from 'https'; export declare function fetch( input: RequestInfo, init?: RequestInit, ): Promise<Response>; export type RequestAgent = HttpAgent | HttpsAgent; export type RequestInfo = Request | string; export declare class Headers implements Iterable<[string, string]> { constructor(init?: HeadersInit); append(name: string, value: string): void; delete(name: string): void; get(name: string): string | null; has(name: string): boolean; set(name: string, value: string): void; entries(): Iterator<[string, string]>; keys(): Iterator<string>; values(): Iterator<[string]>; [Symbol.iterator](): Iterator<[string, string]>; } export type HeadersInit = Headers | string[][] | { [name: string]: string }; export declare class Body { readonly bodyUsed: boolean; arrayBuffer(): Promise<ArrayBuffer>; json(): Promise<any>; text(): Promise<string>; } export declare class Request extends Body { constructor(input: Request | string, init?: RequestInit); readonly method: string; readonly url: string; readonly headers: Headers; clone(): Request; } export interface RequestInit { method?: string; headers?: HeadersInit; body?: BodyInit; mode?: RequestMode; credentials?: RequestCredentials; cache?: RequestCache; redirect?: RequestRedirect; referrer?: string; referrerPolicy?: ReferrerPolicy; integrity?: string; // The following properties are node-fetch extensions follow?: number; timeout?: number; compress?: boolean; size?: number; agent?: RequestAgent | false; // Cloudflare Workers accept a `cf` property to control Cloudflare features // See https://developers.cloudflare.com/workers/reference/cloudflare-features/ cf?: { [key: string]: any; }; } export type RequestMode = 'navigate' | 'same-origin' | 'no-cors' | 'cors'; export type RequestCredentials = 'omit' | 'same-origin' | 'include'; export type RequestCache = | 'default' | 'no-store' | 'reload' | 'no-cache' | 'force-cache' | 'only-if-cached'; export type RequestRedirect = 'follow' | 'error' | 'manual'; export type ReferrerPolicy = | '' | 'no-referrer' | 'no-referrer-when-downgrade' | 'same-origin' | 'origin' | 'strict-origin' | 'origin-when-cross-origin' | 'strict-origin-when-cross-origin' | 'unsafe-url'; export declare class Response extends Body { constructor(body?: BodyInit, init?: ResponseInit); static error(): Response; static redirect(url: string, status?: number): Response; readonly url: string; readonly redirected: boolean; readonly status: number; readonly ok: boolean; readonly statusText: string; readonly headers: Headers; clone(): Response; } export interface ResponseInit { headers?: HeadersInit; status?: number; statusText?: string; // Although this isn't part of the spec, `node-fetch` accepts a `url` property url?: string; } export type BodyInit = ArrayBuffer | ArrayBufferView | string; apollo-server-demo/node_modules/apollo-server-env/src/polyfills/ 0000755 0001750 0000144 00000000000 14067647700 024626 5 ustar andreh users apollo-server-demo/node_modules/apollo-server-env/src/polyfills/fetch.js 0000644 0001750 0000144 00000000113 03560116604 026236 0 ustar andreh users export { default as fetch, Request, Response, Headers } from 'node-fetch'; apollo-server-demo/node_modules/apollo-server-env/src/polyfills/url.js 0000644 0001750 0000144 00000000054 03560116604 025753 0 ustar andreh users export { URL, URLSearchParams } from 'url'; apollo-server-demo/node_modules/apollo-server-env/src/polyfills/Object.entries.ts 0000644 0001750 0000144 00000000247 03560116604 030045 0 ustar andreh users if (!global.Object.entries) { global.Object.entries = function(object: any) { return Object.keys(object).map(key => [key, object[key]] as [string, any]); }; } apollo-server-demo/node_modules/apollo-server-env/src/polyfills/Object.values.ts 0000644 0001750 0000144 00000000215 03560116604 027666 0 ustar andreh users if (!global.Object.values) { global.Object.values = function(object: any) { return Object.keys(object).map(key => object[key]); }; } apollo-server-demo/node_modules/apollo-server-env/src/index.d.ts 0000644 0001750 0000144 00000000134 03560116604 024476 0 ustar andreh users export * from './fetch'; export * from './url'; export * from './typescript-utility-types'; apollo-server-demo/node_modules/apollo-server-env/src/utils/ 0000755 0001750 0000144 00000000000 14067647700 023751 5 ustar andreh users apollo-server-demo/node_modules/apollo-server-env/src/utils/runtimeSupportsPromisify.ts 0000644 0001750 0000144 00000001144 03560116604 031454 0 ustar andreh users const runtimeSupportsPromisify = (() => { if ( process && process.release && process.release.name === 'node' && process.versions && typeof process.versions.node === 'string' ) { const [nodeMajor] = process.versions.node .split('.', 1) .map(segment => parseInt(segment, 10)); if (nodeMajor >= 8) { return true; } return false; } // If we haven't matched any of the above criteria, we'll remain unsupported // for this mysterious environment until a pull-request proves us otherwise. return false; })(); export default runtimeSupportsPromisify; apollo-server-demo/node_modules/apollo-server-env/src/url.d.ts 0000644 0001750 0000144 00000002232 03560116604 024172 0 ustar andreh users export declare class URL { constructor(input: string, base?: string | URL); hash: string; host: string; hostname: string; href: string; readonly origin: string; password: string; pathname: string; port: string; protocol: string; search: string; readonly searchParams: URLSearchParams; username: string; toString(): string; toJSON(): string; } export declare class URLSearchParams implements Iterable<[string, string]> { constructor(init?: URLSearchParamsInit); append(name: string, value: string): void; delete(name: string): void; entries(): IterableIterator<[string, string]>; forEach(callback: (value: string, name: string) => void): void; get(name: string): string | null; getAll(name: string): string[]; has(name: string): boolean; keys(): IterableIterator<string>; set(name: string, value: string): void; sort(): void; toString(): string; values(): IterableIterator<string>; [Symbol.iterator](): IterableIterator<[string, string]>; } export type URLSearchParamsInit = | URLSearchParams | string | { [key: string]: Object | Object[] | undefined } | Iterable<[string, Object]> | Array<[string, Object]>; apollo-server-demo/node_modules/apollo-server-env/src/global.d.ts 0000644 0001750 0000144 00000002040 03560116604 024625 0 ustar andreh users declare function fetch( input: RequestInfo, init?: RequestInit, ): Promise<Response>; declare interface GlobalFetch { fetch(input: RequestInfo, init?: RequestInit): Promise<Response>; } type RequestInfo = import('./fetch').RequestInfo; type Headers = import('./fetch').Headers; type HeadersInit = import('./fetch').HeadersInit; type Body = import('./fetch').Body; type Request = import('./fetch').Request; type RequestAgent = import('./fetch').RequestAgent; type RequestInit = import('./fetch').RequestInit; type RequestMode = import('./fetch').RequestMode; type RequestCredentials = import('./fetch').RequestCredentials; type RequestCache = import('./fetch').RequestCache; type RequestRedirect = import('./fetch').RequestRedirect; type ReferrerPolicy = import('./fetch').ReferrerPolicy; type Response = import('./fetch').Response; type ResponseInit = import('./fetch').ResponseInit; type BodyInit = import('./fetch').BodyInit; type URLSearchParams = import('./url').URLSearchParams; type URLSearchParamsInit = import('./url').URLSearchParamsInit; apollo-server-demo/node_modules/apollo-server-env/src/typescript-utility-types.d.ts 0000644 0001750 0000144 00000000173 03560116604 030443 0 ustar andreh users export type ValueOrPromise<T> = T | Promise<T>; export type WithRequired<T, K extends keyof T> = T & Required<Pick<T, K>>; apollo-server-demo/node_modules/apollo-server-env/src/index.browser.js 0000644 0001750 0000144 00000002151 03560116604 025725 0 ustar andreh users if (!global) { global = self; } let { fetch, Request, Response, Headers, URL, URLSearchParams } = global; fetch = fetch.bind(global); export { fetch, Request, Response, Headers, URL, URLSearchParams }; if (!global.process) { global.process = {}; } if (!global.process.env) { global.process.env = { // app is a global available on fly.io NODE_ENV: typeof app !== 'undefined' ? app.env : 'production', }; } if (!global.process.version) { global.process.version = ''; } if (!global.process.hrtime) { // Adapted from https://github.com/kumavis/browser-process-hrtime global.process.hrtime = function hrtime(previousTimestamp) { var clocktime = Date.now() * 1e-3; var seconds = Math.floor(clocktime); var nanoseconds = Math.floor((clocktime % 1) * 1e9); if (previousTimestamp) { seconds = seconds - previousTimestamp[0]; nanoseconds = nanoseconds - previousTimestamp[1]; if (nanoseconds < 0) { seconds--; nanoseconds += 1e9; } } return [seconds, nanoseconds]; }; } if (!global.os) { // FIXME: Add some sensible values global.os = {}; } apollo-server-demo/node_modules/apollo-server-env/src/index.ts 0000644 0001750 0000144 00000000440 03560116604 024254 0 ustar andreh users import './polyfills/Object.values'; import './polyfills/Object.entries'; import runtimeSupportsPromisify from './utils/runtimeSupportsPromisify'; if (!runtimeSupportsPromisify) { require('util.promisify').shim(); } export * from './polyfills/fetch'; export * from './polyfills/url'; apollo-server-demo/node_modules/apollo-server-env/README.md 0000644 0001750 0000144 00000000562 03560116604 023272 0 ustar andreh users # `apollo-server-env` This package is used internally by Apollo Server and not meant to be consumed directly. Its primary function is to provide polyfills (e.g. via [`core-js`](https://npm.im/core-js)) for newer language features which might not be available in the underlying JavaScript Engine (i.e. more bare-bones V8 environments, older versions of Node.js, etc.). apollo-server-demo/node_modules/apollo-server-env/package.json 0000644 0001750 0000144 00000002114 03560116604 024274 0 ustar andreh users { "name": "apollo-server-env", "version": "3.0.0", "author": "Apollo <opensource@apollographql.com>", "license": "MIT", "repository": { "type": "git", "url": "https://github.com/apollographql/apollo-server", "directory": "packages/apollo-server-env" }, "homepage": "https://github.com/apollographql/apollo-server#readme", "bugs": { "url": "https://github.com/apollographql/apollo-server/issues" }, "main": "dist/index.js", "browser": "dist/index.browser.js", "types": "dist/index.d.ts", "scripts": { "clean": "git clean -fdX -- dist", "compile": "tsc && cp src/*.d.ts dist", "prepare": "npm run clean && npm run compile" }, "engines": { "node": ">=6" }, "dependencies": { "node-fetch": "^2.1.2", "util.promisify": "^1.0.0" }, "gitHead": "c212627be591cd2c2469321b58b15f1b7909778d" ,"_resolved": "https://registry.npmjs.org/apollo-server-env/-/apollo-server-env-3.0.0.tgz" ,"_integrity": "sha512-tPSN+VttnPsoQAl/SBVUpGbLA97MXG990XIwq6YUnJyAixrrsjW1xYG7RlaOqetxm80y5mBZKLrRDiiSsW/vog==" ,"_from": "apollo-server-env@3.0.0" } apollo-server-demo/node_modules/express/ 0000755 0001750 0000144 00000000000 14067647700 020133 5 ustar andreh users apollo-server-demo/node_modules/express/index.js 0000644 0001750 0000144 00000000340 03560116604 021563 0 ustar andreh users /*! * express * Copyright(c) 2009-2013 TJ Holowaychuk * Copyright(c) 2013 Roman Shtylman * Copyright(c) 2014-2015 Douglas Christopher Wilson * MIT Licensed */ 'use strict'; module.exports = require('./lib/express'); apollo-server-demo/node_modules/express/LICENSE 0000644 0001750 0000144 00000002341 03560116604 021126 0 ustar andreh users (The MIT License) Copyright (c) 2009-2014 TJ Holowaychuk <tj@vision-media.ca> Copyright (c) 2013-2014 Roman Shtylman <shtylman+expressjs@gmail.com> Copyright (c) 2014-2015 Douglas Christopher Wilson <doug@somethingdoug.com> Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the 'Software'), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/express/Readme.md 0000644 0001750 0000144 00000010777 03560116604 021654 0 ustar andreh users [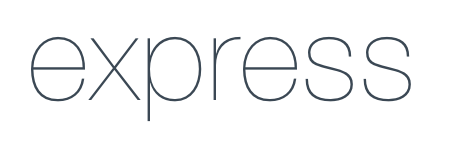](http://expressjs.com/) Fast, unopinionated, minimalist web framework for [node](http://nodejs.org). [![NPM Version][npm-image]][npm-url] [![NPM Downloads][downloads-image]][downloads-url] [![Linux Build][travis-image]][travis-url] [![Windows Build][appveyor-image]][appveyor-url] [![Test Coverage][coveralls-image]][coveralls-url] ```js const express = require('express') const app = express() app.get('/', function (req, res) { res.send('Hello World') }) app.listen(3000) ``` ## Installation This is a [Node.js](https://nodejs.org/en/) module available through the [npm registry](https://www.npmjs.com/). Before installing, [download and install Node.js](https://nodejs.org/en/download/). Node.js 0.10 or higher is required. Installation is done using the [`npm install` command](https://docs.npmjs.com/getting-started/installing-npm-packages-locally): ```bash $ npm install express ``` Follow [our installing guide](http://expressjs.com/en/starter/installing.html) for more information. ## Features * Robust routing * Focus on high performance * Super-high test coverage * HTTP helpers (redirection, caching, etc) * View system supporting 14+ template engines * Content negotiation * Executable for generating applications quickly ## Docs & Community * [Website and Documentation](http://expressjs.com/) - [[website repo](https://github.com/expressjs/expressjs.com)] * [#express](https://webchat.freenode.net/?channels=express) on freenode IRC * [GitHub Organization](https://github.com/expressjs) for Official Middleware & Modules * Visit the [Wiki](https://github.com/expressjs/express/wiki) * [Google Group](https://groups.google.com/group/express-js) for discussion * [Gitter](https://gitter.im/expressjs/express) for support and discussion **PROTIP** Be sure to read [Migrating from 3.x to 4.x](https://github.com/expressjs/express/wiki/Migrating-from-3.x-to-4.x) as well as [New features in 4.x](https://github.com/expressjs/express/wiki/New-features-in-4.x). ### Security Issues If you discover a security vulnerability in Express, please see [Security Policies and Procedures](Security.md). ## Quick Start The quickest way to get started with express is to utilize the executable [`express(1)`](https://github.com/expressjs/generator) to generate an application as shown below: Install the executable. The executable's major version will match Express's: ```bash $ npm install -g express-generator@4 ``` Create the app: ```bash $ express /tmp/foo && cd /tmp/foo ``` Install dependencies: ```bash $ npm install ``` Start the server: ```bash $ npm start ``` View the website at: http://localhost:3000 ## Philosophy The Express philosophy is to provide small, robust tooling for HTTP servers, making it a great solution for single page applications, web sites, hybrids, or public HTTP APIs. Express does not force you to use any specific ORM or template engine. With support for over 14 template engines via [Consolidate.js](https://github.com/tj/consolidate.js), you can quickly craft your perfect framework. ## Examples To view the examples, clone the Express repo and install the dependencies: ```bash $ git clone git://github.com/expressjs/express.git --depth 1 $ cd express $ npm install ``` Then run whichever example you want: ```bash $ node examples/content-negotiation ``` ## Tests To run the test suite, first install the dependencies, then run `npm test`: ```bash $ npm install $ npm test ``` ## Contributing [Contributing Guide](Contributing.md) ## People The original author of Express is [TJ Holowaychuk](https://github.com/tj) The current lead maintainer is [Douglas Christopher Wilson](https://github.com/dougwilson) [List of all contributors](https://github.com/expressjs/express/graphs/contributors) ## License [MIT](LICENSE) [npm-image]: https://img.shields.io/npm/v/express.svg [npm-url]: https://npmjs.org/package/express [downloads-image]: https://img.shields.io/npm/dm/express.svg [downloads-url]: https://npmjs.org/package/express [travis-image]: https://img.shields.io/travis/expressjs/express/master.svg?label=linux [travis-url]: https://travis-ci.org/expressjs/express [appveyor-image]: https://img.shields.io/appveyor/ci/dougwilson/express/master.svg?label=windows [appveyor-url]: https://ci.appveyor.com/project/dougwilson/express [coveralls-image]: https://img.shields.io/coveralls/expressjs/express/master.svg [coveralls-url]: https://coveralls.io/r/expressjs/express?branch=master apollo-server-demo/node_modules/express/History.md 0000644 0001750 0000144 00000326025 03560116604 022114 0 ustar andreh users 4.17.1 / 2019-05-25 =================== * Revert "Improve error message for `null`/`undefined` to `res.status`" 4.17.0 / 2019-05-16 =================== * Add `express.raw` to parse bodies into `Buffer` * Add `express.text` to parse bodies into string * Improve error message for non-strings to `res.sendFile` * Improve error message for `null`/`undefined` to `res.status` * Support multiple hosts in `X-Forwarded-Host` * deps: accepts@~1.3.7 * deps: body-parser@1.19.0 - Add encoding MIK - Add petabyte (`pb`) support - Fix parsing array brackets after index - deps: bytes@3.1.0 - deps: http-errors@1.7.2 - deps: iconv-lite@0.4.24 - deps: qs@6.7.0 - deps: raw-body@2.4.0 - deps: type-is@~1.6.17 * deps: content-disposition@0.5.3 * deps: cookie@0.4.0 - Add `SameSite=None` support * deps: finalhandler@~1.1.2 - Set stricter `Content-Security-Policy` header - deps: parseurl@~1.3.3 - deps: statuses@~1.5.0 * deps: parseurl@~1.3.3 * deps: proxy-addr@~2.0.5 - deps: ipaddr.js@1.9.0 * deps: qs@6.7.0 - Fix parsing array brackets after index * deps: range-parser@~1.2.1 * deps: send@0.17.1 - Set stricter CSP header in redirect & error responses - deps: http-errors@~1.7.2 - deps: mime@1.6.0 - deps: ms@2.1.1 - deps: range-parser@~1.2.1 - deps: statuses@~1.5.0 - perf: remove redundant `path.normalize` call * deps: serve-static@1.14.1 - Set stricter CSP header in redirect response - deps: parseurl@~1.3.3 - deps: send@0.17.1 * deps: setprototypeof@1.1.1 * deps: statuses@~1.5.0 - Add `103 Early Hints` * deps: type-is@~1.6.18 - deps: mime-types@~2.1.24 - perf: prevent internal `throw` on invalid type 4.16.4 / 2018-10-10 =================== * Fix issue where `"Request aborted"` may be logged in `res.sendfile` * Fix JSDoc for `Router` constructor * deps: body-parser@1.18.3 - Fix deprecation warnings on Node.js 10+ - Fix stack trace for strict json parse error - deps: depd@~1.1.2 - deps: http-errors@~1.6.3 - deps: iconv-lite@0.4.23 - deps: qs@6.5.2 - deps: raw-body@2.3.3 - deps: type-is@~1.6.16 * deps: proxy-addr@~2.0.4 - deps: ipaddr.js@1.8.0 * deps: qs@6.5.2 * deps: safe-buffer@5.1.2 4.16.3 / 2018-03-12 =================== * deps: accepts@~1.3.5 - deps: mime-types@~2.1.18 * deps: depd@~1.1.2 - perf: remove argument reassignment * deps: encodeurl@~1.0.2 - Fix encoding `%` as last character * deps: finalhandler@1.1.1 - Fix 404 output for bad / missing pathnames - deps: encodeurl@~1.0.2 - deps: statuses@~1.4.0 * deps: proxy-addr@~2.0.3 - deps: ipaddr.js@1.6.0 * deps: send@0.16.2 - Fix incorrect end tag in default error & redirects - deps: depd@~1.1.2 - deps: encodeurl@~1.0.2 - deps: statuses@~1.4.0 * deps: serve-static@1.13.2 - Fix incorrect end tag in redirects - deps: encodeurl@~1.0.2 - deps: send@0.16.2 * deps: statuses@~1.4.0 * deps: type-is@~1.6.16 - deps: mime-types@~2.1.18 4.16.2 / 2017-10-09 =================== * Fix `TypeError` in `res.send` when given `Buffer` and `ETag` header set * perf: skip parsing of entire `X-Forwarded-Proto` header 4.16.1 / 2017-09-29 =================== * deps: send@0.16.1 * deps: serve-static@1.13.1 - Fix regression when `root` is incorrectly set to a file - deps: send@0.16.1 4.16.0 / 2017-09-28 =================== * Add `"json escape"` setting for `res.json` and `res.jsonp` * Add `express.json` and `express.urlencoded` to parse bodies * Add `options` argument to `res.download` * Improve error message when autoloading invalid view engine * Improve error messages when non-function provided as middleware * Skip `Buffer` encoding when not generating ETag for small response * Use `safe-buffer` for improved Buffer API * deps: accepts@~1.3.4 - deps: mime-types@~2.1.16 * deps: content-type@~1.0.4 - perf: remove argument reassignment - perf: skip parameter parsing when no parameters * deps: etag@~1.8.1 - perf: replace regular expression with substring * deps: finalhandler@1.1.0 - Use `res.headersSent` when available * deps: parseurl@~1.3.2 - perf: reduce overhead for full URLs - perf: unroll the "fast-path" `RegExp` * deps: proxy-addr@~2.0.2 - Fix trimming leading / trailing OWS in `X-Forwarded-For` - deps: forwarded@~0.1.2 - deps: ipaddr.js@1.5.2 - perf: reduce overhead when no `X-Forwarded-For` header * deps: qs@6.5.1 - Fix parsing & compacting very deep objects * deps: send@0.16.0 - Add 70 new types for file extensions - Add `immutable` option - Fix missing `</html>` in default error & redirects - Set charset as "UTF-8" for .js and .json - Use instance methods on steam to check for listeners - deps: mime@1.4.1 - perf: improve path validation speed * deps: serve-static@1.13.0 - Add 70 new types for file extensions - Add `immutable` option - Set charset as "UTF-8" for .js and .json - deps: send@0.16.0 * deps: setprototypeof@1.1.0 * deps: utils-merge@1.0.1 * deps: vary@~1.1.2 - perf: improve header token parsing speed * perf: re-use options object when generating ETags * perf: remove dead `.charset` set in `res.jsonp` 4.15.5 / 2017-09-24 =================== * deps: debug@2.6.9 * deps: finalhandler@~1.0.6 - deps: debug@2.6.9 - deps: parseurl@~1.3.2 * deps: fresh@0.5.2 - Fix handling of modified headers with invalid dates - perf: improve ETag match loop - perf: improve `If-None-Match` token parsing * deps: send@0.15.6 - Fix handling of modified headers with invalid dates - deps: debug@2.6.9 - deps: etag@~1.8.1 - deps: fresh@0.5.2 - perf: improve `If-Match` token parsing * deps: serve-static@1.12.6 - deps: parseurl@~1.3.2 - deps: send@0.15.6 - perf: improve slash collapsing 4.15.4 / 2017-08-06 =================== * deps: debug@2.6.8 * deps: depd@~1.1.1 - Remove unnecessary `Buffer` loading * deps: finalhandler@~1.0.4 - deps: debug@2.6.8 * deps: proxy-addr@~1.1.5 - Fix array argument being altered - deps: ipaddr.js@1.4.0 * deps: qs@6.5.0 * deps: send@0.15.4 - deps: debug@2.6.8 - deps: depd@~1.1.1 - deps: http-errors@~1.6.2 * deps: serve-static@1.12.4 - deps: send@0.15.4 4.15.3 / 2017-05-16 =================== * Fix error when `res.set` cannot add charset to `Content-Type` * deps: debug@2.6.7 - Fix `DEBUG_MAX_ARRAY_LENGTH` - deps: ms@2.0.0 * deps: finalhandler@~1.0.3 - Fix missing `</html>` in HTML document - deps: debug@2.6.7 * deps: proxy-addr@~1.1.4 - deps: ipaddr.js@1.3.0 * deps: send@0.15.3 - deps: debug@2.6.7 - deps: ms@2.0.0 * deps: serve-static@1.12.3 - deps: send@0.15.3 * deps: type-is@~1.6.15 - deps: mime-types@~2.1.15 * deps: vary@~1.1.1 - perf: hoist regular expression 4.15.2 / 2017-03-06 =================== * deps: qs@6.4.0 - Fix regression parsing keys starting with `[` 4.15.1 / 2017-03-05 =================== * deps: send@0.15.1 - Fix issue when `Date.parse` does not return `NaN` on invalid date - Fix strict violation in broken environments * deps: serve-static@1.12.1 - Fix issue when `Date.parse` does not return `NaN` on invalid date - deps: send@0.15.1 4.15.0 / 2017-03-01 =================== * Add debug message when loading view engine * Add `next("router")` to exit from router * Fix case where `router.use` skipped requests routes did not * Remove usage of `res._headers` private field - Improves compatibility with Node.js 8 nightly * Skip routing when `req.url` is not set * Use `%o` in path debug to tell types apart * Use `Object.create` to setup request & response prototypes * Use `setprototypeof` module to replace `__proto__` setting * Use `statuses` instead of `http` module for status messages * deps: debug@2.6.1 - Allow colors in workers - Deprecated `DEBUG_FD` environment variable set to `3` or higher - Fix error when running under React Native - Use same color for same namespace - deps: ms@0.7.2 * deps: etag@~1.8.0 - Use SHA1 instead of MD5 for ETag hashing - Works with FIPS 140-2 OpenSSL configuration * deps: finalhandler@~1.0.0 - Fix exception when `err` cannot be converted to a string - Fully URL-encode the pathname in the 404 - Only include the pathname in the 404 message - Send complete HTML document - Set `Content-Security-Policy: default-src 'self'` header - deps: debug@2.6.1 * deps: fresh@0.5.0 - Fix false detection of `no-cache` request directive - Fix incorrect result when `If-None-Match` has both `*` and ETags - Fix weak `ETag` matching to match spec - perf: delay reading header values until needed - perf: enable strict mode - perf: hoist regular expressions - perf: remove duplicate conditional - perf: remove unnecessary boolean coercions - perf: skip checking modified time if ETag check failed - perf: skip parsing `If-None-Match` when no `ETag` header - perf: use `Date.parse` instead of `new Date` * deps: qs@6.3.1 - Fix array parsing from skipping empty values - Fix compacting nested arrays * deps: send@0.15.0 - Fix false detection of `no-cache` request directive - Fix incorrect result when `If-None-Match` has both `*` and ETags - Fix weak `ETag` matching to match spec - Remove usage of `res._headers` private field - Support `If-Match` and `If-Unmodified-Since` headers - Use `res.getHeaderNames()` when available - Use `res.headersSent` when available - deps: debug@2.6.1 - deps: etag@~1.8.0 - deps: fresh@0.5.0 - deps: http-errors@~1.6.1 * deps: serve-static@1.12.0 - Fix false detection of `no-cache` request directive - Fix incorrect result when `If-None-Match` has both `*` and ETags - Fix weak `ETag` matching to match spec - Remove usage of `res._headers` private field - Send complete HTML document in redirect response - Set default CSP header in redirect response - Support `If-Match` and `If-Unmodified-Since` headers - Use `res.getHeaderNames()` when available - Use `res.headersSent` when available - deps: send@0.15.0 * perf: add fast match path for `*` route * perf: improve `req.ips` performance 4.14.1 / 2017-01-28 =================== * deps: content-disposition@0.5.2 * deps: finalhandler@0.5.1 - Fix exception when `err.headers` is not an object - deps: statuses@~1.3.1 - perf: hoist regular expressions - perf: remove duplicate validation path * deps: proxy-addr@~1.1.3 - deps: ipaddr.js@1.2.0 * deps: send@0.14.2 - deps: http-errors@~1.5.1 - deps: ms@0.7.2 - deps: statuses@~1.3.1 * deps: serve-static@~1.11.2 - deps: send@0.14.2 * deps: type-is@~1.6.14 - deps: mime-types@~2.1.13 4.14.0 / 2016-06-16 =================== * Add `acceptRanges` option to `res.sendFile`/`res.sendfile` * Add `cacheControl` option to `res.sendFile`/`res.sendfile` * Add `options` argument to `req.range` - Includes the `combine` option * Encode URL in `res.location`/`res.redirect` if not already encoded * Fix some redirect handling in `res.sendFile`/`res.sendfile` * Fix Windows absolute path check using forward slashes * Improve error with invalid arguments to `req.get()` * Improve performance for `res.json`/`res.jsonp` in most cases * Improve `Range` header handling in `res.sendFile`/`res.sendfile` * deps: accepts@~1.3.3 - Fix including type extensions in parameters in `Accept` parsing - Fix parsing `Accept` parameters with quoted equals - Fix parsing `Accept` parameters with quoted semicolons - Many performance improvements - deps: mime-types@~2.1.11 - deps: negotiator@0.6.1 * deps: content-type@~1.0.2 - perf: enable strict mode * deps: cookie@0.3.1 - Add `sameSite` option - Fix cookie `Max-Age` to never be a floating point number - Improve error message when `encode` is not a function - Improve error message when `expires` is not a `Date` - Throw better error for invalid argument to parse - Throw on invalid values provided to `serialize` - perf: enable strict mode - perf: hoist regular expression - perf: use for loop in parse - perf: use string concatenation for serialization * deps: finalhandler@0.5.0 - Change invalid or non-numeric status code to 500 - Overwrite status message to match set status code - Prefer `err.statusCode` if `err.status` is invalid - Set response headers from `err.headers` object - Use `statuses` instead of `http` module for status messages * deps: proxy-addr@~1.1.2 - Fix accepting various invalid netmasks - Fix IPv6-mapped IPv4 validation edge cases - IPv4 netmasks must be contiguous - IPv6 addresses cannot be used as a netmask - deps: ipaddr.js@1.1.1 * deps: qs@6.2.0 - Add `decoder` option in `parse` function * deps: range-parser@~1.2.0 - Add `combine` option to combine overlapping ranges - Fix incorrectly returning -1 when there is at least one valid range - perf: remove internal function * deps: send@0.14.1 - Add `acceptRanges` option - Add `cacheControl` option - Attempt to combine multiple ranges into single range - Correctly inherit from `Stream` class - Fix `Content-Range` header in 416 responses when using `start`/`end` options - Fix `Content-Range` header missing from default 416 responses - Fix redirect error when `path` contains raw non-URL characters - Fix redirect when `path` starts with multiple forward slashes - Ignore non-byte `Range` headers - deps: http-errors@~1.5.0 - deps: range-parser@~1.2.0 - deps: statuses@~1.3.0 - perf: remove argument reassignment * deps: serve-static@~1.11.1 - Add `acceptRanges` option - Add `cacheControl` option - Attempt to combine multiple ranges into single range - Fix redirect error when `req.url` contains raw non-URL characters - Ignore non-byte `Range` headers - Use status code 301 for redirects - deps: send@0.14.1 * deps: type-is@~1.6.13 - Fix type error when given invalid type to match against - deps: mime-types@~2.1.11 * deps: vary@~1.1.0 - Only accept valid field names in the `field` argument * perf: use strict equality when possible 4.13.4 / 2016-01-21 =================== * deps: content-disposition@0.5.1 - perf: enable strict mode * deps: cookie@0.1.5 - Throw on invalid values provided to `serialize` * deps: depd@~1.1.0 - Support web browser loading - perf: enable strict mode * deps: escape-html@~1.0.3 - perf: enable strict mode - perf: optimize string replacement - perf: use faster string coercion * deps: finalhandler@0.4.1 - deps: escape-html@~1.0.3 * deps: merge-descriptors@1.0.1 - perf: enable strict mode * deps: methods@~1.1.2 - perf: enable strict mode * deps: parseurl@~1.3.1 - perf: enable strict mode * deps: proxy-addr@~1.0.10 - deps: ipaddr.js@1.0.5 - perf: enable strict mode * deps: range-parser@~1.0.3 - perf: enable strict mode * deps: send@0.13.1 - deps: depd@~1.1.0 - deps: destroy@~1.0.4 - deps: escape-html@~1.0.3 - deps: range-parser@~1.0.3 * deps: serve-static@~1.10.2 - deps: escape-html@~1.0.3 - deps: parseurl@~1.3.0 - deps: send@0.13.1 4.13.3 / 2015-08-02 =================== * Fix infinite loop condition using `mergeParams: true` * Fix inner numeric indices incorrectly altering parent `req.params` 4.13.2 / 2015-07-31 =================== * deps: accepts@~1.2.12 - deps: mime-types@~2.1.4 * deps: array-flatten@1.1.1 - perf: enable strict mode * deps: path-to-regexp@0.1.7 - Fix regression with escaped round brackets and matching groups * deps: type-is@~1.6.6 - deps: mime-types@~2.1.4 4.13.1 / 2015-07-05 =================== * deps: accepts@~1.2.10 - deps: mime-types@~2.1.2 * deps: qs@4.0.0 - Fix dropping parameters like `hasOwnProperty` - Fix various parsing edge cases * deps: type-is@~1.6.4 - deps: mime-types@~2.1.2 - perf: enable strict mode - perf: remove argument reassignment 4.13.0 / 2015-06-20 =================== * Add settings to debug output * Fix `res.format` error when only `default` provided * Fix issue where `next('route')` in `app.param` would incorrectly skip values * Fix hiding platform issues with `decodeURIComponent` - Only `URIError`s are a 400 * Fix using `*` before params in routes * Fix using capture groups before params in routes * Simplify `res.cookie` to call `res.append` * Use `array-flatten` module for flattening arrays * deps: accepts@~1.2.9 - deps: mime-types@~2.1.1 - perf: avoid argument reassignment & argument slice - perf: avoid negotiator recursive construction - perf: enable strict mode - perf: remove unnecessary bitwise operator * deps: cookie@0.1.3 - perf: deduce the scope of try-catch deopt - perf: remove argument reassignments * deps: escape-html@1.0.2 * deps: etag@~1.7.0 - Always include entity length in ETags for hash length extensions - Generate non-Stats ETags using MD5 only (no longer CRC32) - Improve stat performance by removing hashing - Improve support for JXcore - Remove base64 padding in ETags to shorten - Support "fake" stats objects in environments without fs - Use MD5 instead of MD4 in weak ETags over 1KB * deps: finalhandler@0.4.0 - Fix a false-positive when unpiping in Node.js 0.8 - Support `statusCode` property on `Error` objects - Use `unpipe` module for unpiping requests - deps: escape-html@1.0.2 - deps: on-finished@~2.3.0 - perf: enable strict mode - perf: remove argument reassignment * deps: fresh@0.3.0 - Add weak `ETag` matching support * deps: on-finished@~2.3.0 - Add defined behavior for HTTP `CONNECT` requests - Add defined behavior for HTTP `Upgrade` requests - deps: ee-first@1.1.1 * deps: path-to-regexp@0.1.6 * deps: send@0.13.0 - Allow Node.js HTTP server to set `Date` response header - Fix incorrectly removing `Content-Location` on 304 response - Improve the default redirect response headers - Send appropriate headers on default error response - Use `http-errors` for standard emitted errors - Use `statuses` instead of `http` module for status messages - deps: escape-html@1.0.2 - deps: etag@~1.7.0 - deps: fresh@0.3.0 - deps: on-finished@~2.3.0 - perf: enable strict mode - perf: remove unnecessary array allocations * deps: serve-static@~1.10.0 - Add `fallthrough` option - Fix reading options from options prototype - Improve the default redirect response headers - Malformed URLs now `next()` instead of 400 - deps: escape-html@1.0.2 - deps: send@0.13.0 - perf: enable strict mode - perf: remove argument reassignment * deps: type-is@~1.6.3 - deps: mime-types@~2.1.1 - perf: reduce try block size - perf: remove bitwise operations * perf: enable strict mode * perf: isolate `app.render` try block * perf: remove argument reassignments in application * perf: remove argument reassignments in request prototype * perf: remove argument reassignments in response prototype * perf: remove argument reassignments in routing * perf: remove argument reassignments in `View` * perf: skip attempting to decode zero length string * perf: use saved reference to `http.STATUS_CODES` 4.12.4 / 2015-05-17 =================== * deps: accepts@~1.2.7 - deps: mime-types@~2.0.11 - deps: negotiator@0.5.3 * deps: debug@~2.2.0 - deps: ms@0.7.1 * deps: depd@~1.0.1 * deps: etag@~1.6.0 - Improve support for JXcore - Support "fake" stats objects in environments without `fs` * deps: finalhandler@0.3.6 - deps: debug@~2.2.0 - deps: on-finished@~2.2.1 * deps: on-finished@~2.2.1 - Fix `isFinished(req)` when data buffered * deps: proxy-addr@~1.0.8 - deps: ipaddr.js@1.0.1 * deps: qs@2.4.2 - Fix allowing parameters like `constructor` * deps: send@0.12.3 - deps: debug@~2.2.0 - deps: depd@~1.0.1 - deps: etag@~1.6.0 - deps: ms@0.7.1 - deps: on-finished@~2.2.1 * deps: serve-static@~1.9.3 - deps: send@0.12.3 * deps: type-is@~1.6.2 - deps: mime-types@~2.0.11 4.12.3 / 2015-03-17 =================== * deps: accepts@~1.2.5 - deps: mime-types@~2.0.10 * deps: debug@~2.1.3 - Fix high intensity foreground color for bold - deps: ms@0.7.0 * deps: finalhandler@0.3.4 - deps: debug@~2.1.3 * deps: proxy-addr@~1.0.7 - deps: ipaddr.js@0.1.9 * deps: qs@2.4.1 - Fix error when parameter `hasOwnProperty` is present * deps: send@0.12.2 - Throw errors early for invalid `extensions` or `index` options - deps: debug@~2.1.3 * deps: serve-static@~1.9.2 - deps: send@0.12.2 * deps: type-is@~1.6.1 - deps: mime-types@~2.0.10 4.12.2 / 2015-03-02 =================== * Fix regression where `"Request aborted"` is logged using `res.sendFile` 4.12.1 / 2015-03-01 =================== * Fix constructing application with non-configurable prototype properties * Fix `ECONNRESET` errors from `res.sendFile` usage * Fix `req.host` when using "trust proxy" hops count * Fix `req.protocol`/`req.secure` when using "trust proxy" hops count * Fix wrong `code` on aborted connections from `res.sendFile` * deps: merge-descriptors@1.0.0 4.12.0 / 2015-02-23 =================== * Fix `"trust proxy"` setting to inherit when app is mounted * Generate `ETag`s for all request responses - No longer restricted to only responses for `GET` and `HEAD` requests * Use `content-type` to parse `Content-Type` headers * deps: accepts@~1.2.4 - Fix preference sorting to be stable for long acceptable lists - deps: mime-types@~2.0.9 - deps: negotiator@0.5.1 * deps: cookie-signature@1.0.6 * deps: send@0.12.1 - Always read the stat size from the file - Fix mutating passed-in `options` - deps: mime@1.3.4 * deps: serve-static@~1.9.1 - deps: send@0.12.1 * deps: type-is@~1.6.0 - fix argument reassignment - fix false-positives in `hasBody` `Transfer-Encoding` check - support wildcard for both type and subtype (`*/*`) - deps: mime-types@~2.0.9 4.11.2 / 2015-02-01 =================== * Fix `res.redirect` double-calling `res.end` for `HEAD` requests * deps: accepts@~1.2.3 - deps: mime-types@~2.0.8 * deps: proxy-addr@~1.0.6 - deps: ipaddr.js@0.1.8 * deps: type-is@~1.5.6 - deps: mime-types@~2.0.8 4.11.1 / 2015-01-20 =================== * deps: send@0.11.1 - Fix root path disclosure * deps: serve-static@~1.8.1 - Fix redirect loop in Node.js 0.11.14 - Fix root path disclosure - deps: send@0.11.1 4.11.0 / 2015-01-13 =================== * Add `res.append(field, val)` to append headers * Deprecate leading `:` in `name` for `app.param(name, fn)` * Deprecate `req.param()` -- use `req.params`, `req.body`, or `req.query` instead * Deprecate `app.param(fn)` * Fix `OPTIONS` responses to include the `HEAD` method properly * Fix `res.sendFile` not always detecting aborted connection * Match routes iteratively to prevent stack overflows * deps: accepts@~1.2.2 - deps: mime-types@~2.0.7 - deps: negotiator@0.5.0 * deps: send@0.11.0 - deps: debug@~2.1.1 - deps: etag@~1.5.1 - deps: ms@0.7.0 - deps: on-finished@~2.2.0 * deps: serve-static@~1.8.0 - deps: send@0.11.0 4.10.8 / 2015-01-13 =================== * Fix crash from error within `OPTIONS` response handler * deps: proxy-addr@~1.0.5 - deps: ipaddr.js@0.1.6 4.10.7 / 2015-01-04 =================== * Fix `Allow` header for `OPTIONS` to not contain duplicate methods * Fix incorrect "Request aborted" for `res.sendFile` when `HEAD` or 304 * deps: debug@~2.1.1 * deps: finalhandler@0.3.3 - deps: debug@~2.1.1 - deps: on-finished@~2.2.0 * deps: methods@~1.1.1 * deps: on-finished@~2.2.0 * deps: serve-static@~1.7.2 - Fix potential open redirect when mounted at root * deps: type-is@~1.5.5 - deps: mime-types@~2.0.7 4.10.6 / 2014-12-12 =================== * Fix exception in `req.fresh`/`req.stale` without response headers 4.10.5 / 2014-12-10 =================== * Fix `res.send` double-calling `res.end` for `HEAD` requests * deps: accepts@~1.1.4 - deps: mime-types@~2.0.4 * deps: type-is@~1.5.4 - deps: mime-types@~2.0.4 4.10.4 / 2014-11-24 =================== * Fix `res.sendfile` logging standard write errors 4.10.3 / 2014-11-23 =================== * Fix `res.sendFile` logging standard write errors * deps: etag@~1.5.1 * deps: proxy-addr@~1.0.4 - deps: ipaddr.js@0.1.5 * deps: qs@2.3.3 - Fix `arrayLimit` behavior 4.10.2 / 2014-11-09 =================== * Correctly invoke async router callback asynchronously * deps: accepts@~1.1.3 - deps: mime-types@~2.0.3 * deps: type-is@~1.5.3 - deps: mime-types@~2.0.3 4.10.1 / 2014-10-28 =================== * Fix handling of URLs containing `://` in the path * deps: qs@2.3.2 - Fix parsing of mixed objects and values 4.10.0 / 2014-10-23 =================== * Add support for `app.set('views', array)` - Views are looked up in sequence in array of directories * Fix `res.send(status)` to mention `res.sendStatus(status)` * Fix handling of invalid empty URLs * Use `content-disposition` module for `res.attachment`/`res.download` - Sends standards-compliant `Content-Disposition` header - Full Unicode support * Use `path.resolve` in view lookup * deps: debug@~2.1.0 - Implement `DEBUG_FD` env variable support * deps: depd@~1.0.0 * deps: etag@~1.5.0 - Improve string performance - Slightly improve speed for weak ETags over 1KB * deps: finalhandler@0.3.2 - Terminate in progress response only on error - Use `on-finished` to determine request status - deps: debug@~2.1.0 - deps: on-finished@~2.1.1 * deps: on-finished@~2.1.1 - Fix handling of pipelined requests * deps: qs@2.3.0 - Fix parsing of mixed implicit and explicit arrays * deps: send@0.10.1 - deps: debug@~2.1.0 - deps: depd@~1.0.0 - deps: etag@~1.5.0 - deps: on-finished@~2.1.1 * deps: serve-static@~1.7.1 - deps: send@0.10.1 4.9.8 / 2014-10-17 ================== * Fix `res.redirect` body when redirect status specified * deps: accepts@~1.1.2 - Fix error when media type has invalid parameter - deps: negotiator@0.4.9 4.9.7 / 2014-10-10 ================== * Fix using same param name in array of paths 4.9.6 / 2014-10-08 ================== * deps: accepts@~1.1.1 - deps: mime-types@~2.0.2 - deps: negotiator@0.4.8 * deps: serve-static@~1.6.4 - Fix redirect loop when index file serving disabled * deps: type-is@~1.5.2 - deps: mime-types@~2.0.2 4.9.5 / 2014-09-24 ================== * deps: etag@~1.4.0 * deps: proxy-addr@~1.0.3 - Use `forwarded` npm module * deps: send@0.9.3 - deps: etag@~1.4.0 * deps: serve-static@~1.6.3 - deps: send@0.9.3 4.9.4 / 2014-09-19 ================== * deps: qs@2.2.4 - Fix issue with object keys starting with numbers truncated 4.9.3 / 2014-09-18 ================== * deps: proxy-addr@~1.0.2 - Fix a global leak when multiple subnets are trusted - deps: ipaddr.js@0.1.3 4.9.2 / 2014-09-17 ================== * Fix regression for empty string `path` in `app.use` * Fix `router.use` to accept array of middleware without path * Improve error message for bad `app.use` arguments 4.9.1 / 2014-09-16 ================== * Fix `app.use` to accept array of middleware without path * deps: depd@0.4.5 * deps: etag@~1.3.1 * deps: send@0.9.2 - deps: depd@0.4.5 - deps: etag@~1.3.1 - deps: range-parser@~1.0.2 * deps: serve-static@~1.6.2 - deps: send@0.9.2 4.9.0 / 2014-09-08 ================== * Add `res.sendStatus` * Invoke callback for sendfile when client aborts - Applies to `res.sendFile`, `res.sendfile`, and `res.download` - `err` will be populated with request aborted error * Support IP address host in `req.subdomains` * Use `etag` to generate `ETag` headers * deps: accepts@~1.1.0 - update `mime-types` * deps: cookie-signature@1.0.5 * deps: debug@~2.0.0 * deps: finalhandler@0.2.0 - Set `X-Content-Type-Options: nosniff` header - deps: debug@~2.0.0 * deps: fresh@0.2.4 * deps: media-typer@0.3.0 - Throw error when parameter format invalid on parse * deps: qs@2.2.3 - Fix issue where first empty value in array is discarded * deps: range-parser@~1.0.2 * deps: send@0.9.1 - Add `lastModified` option - Use `etag` to generate `ETag` header - deps: debug@~2.0.0 - deps: fresh@0.2.4 * deps: serve-static@~1.6.1 - Add `lastModified` option - deps: send@0.9.1 * deps: type-is@~1.5.1 - fix `hasbody` to be true for `content-length: 0` - deps: media-typer@0.3.0 - deps: mime-types@~2.0.1 * deps: vary@~1.0.0 - Accept valid `Vary` header string as `field` 4.8.8 / 2014-09-04 ================== * deps: send@0.8.5 - Fix a path traversal issue when using `root` - Fix malicious path detection for empty string path * deps: serve-static@~1.5.4 - deps: send@0.8.5 4.8.7 / 2014-08-29 ================== * deps: qs@2.2.2 - Remove unnecessary cloning 4.8.6 / 2014-08-27 ================== * deps: qs@2.2.0 - Array parsing fix - Performance improvements 4.8.5 / 2014-08-18 ================== * deps: send@0.8.3 - deps: destroy@1.0.3 - deps: on-finished@2.1.0 * deps: serve-static@~1.5.3 - deps: send@0.8.3 4.8.4 / 2014-08-14 ================== * deps: qs@1.2.2 * deps: send@0.8.2 - Work around `fd` leak in Node.js 0.10 for `fs.ReadStream` * deps: serve-static@~1.5.2 - deps: send@0.8.2 4.8.3 / 2014-08-10 ================== * deps: parseurl@~1.3.0 * deps: qs@1.2.1 * deps: serve-static@~1.5.1 - Fix parsing of weird `req.originalUrl` values - deps: parseurl@~1.3.0 - deps: utils-merge@1.0.0 4.8.2 / 2014-08-07 ================== * deps: qs@1.2.0 - Fix parsing array of objects 4.8.1 / 2014-08-06 ================== * fix incorrect deprecation warnings on `res.download` * deps: qs@1.1.0 - Accept urlencoded square brackets - Accept empty values in implicit array notation 4.8.0 / 2014-08-05 ================== * add `res.sendFile` - accepts a file system path instead of a URL - requires an absolute path or `root` option specified * deprecate `res.sendfile` -- use `res.sendFile` instead * support mounted app as any argument to `app.use()` * deps: qs@1.0.2 - Complete rewrite - Limits array length to 20 - Limits object depth to 5 - Limits parameters to 1,000 * deps: send@0.8.1 - Add `extensions` option * deps: serve-static@~1.5.0 - Add `extensions` option - deps: send@0.8.1 4.7.4 / 2014-08-04 ================== * fix `res.sendfile` regression for serving directory index files * deps: send@0.7.4 - Fix incorrect 403 on Windows and Node.js 0.11 - Fix serving index files without root dir * deps: serve-static@~1.4.4 - deps: send@0.7.4 4.7.3 / 2014-08-04 ================== * deps: send@0.7.3 - Fix incorrect 403 on Windows and Node.js 0.11 * deps: serve-static@~1.4.3 - Fix incorrect 403 on Windows and Node.js 0.11 - deps: send@0.7.3 4.7.2 / 2014-07-27 ================== * deps: depd@0.4.4 - Work-around v8 generating empty stack traces * deps: send@0.7.2 - deps: depd@0.4.4 * deps: serve-static@~1.4.2 4.7.1 / 2014-07-26 ================== * deps: depd@0.4.3 - Fix exception when global `Error.stackTraceLimit` is too low * deps: send@0.7.1 - deps: depd@0.4.3 * deps: serve-static@~1.4.1 4.7.0 / 2014-07-25 ================== * fix `req.protocol` for proxy-direct connections * configurable query parser with `app.set('query parser', parser)` - `app.set('query parser', 'extended')` parse with "qs" module - `app.set('query parser', 'simple')` parse with "querystring" core module - `app.set('query parser', false)` disable query string parsing - `app.set('query parser', true)` enable simple parsing * deprecate `res.json(status, obj)` -- use `res.status(status).json(obj)` instead * deprecate `res.jsonp(status, obj)` -- use `res.status(status).jsonp(obj)` instead * deprecate `res.send(status, body)` -- use `res.status(status).send(body)` instead * deps: debug@1.0.4 * deps: depd@0.4.2 - Add `TRACE_DEPRECATION` environment variable - Remove non-standard grey color from color output - Support `--no-deprecation` argument - Support `--trace-deprecation` argument * deps: finalhandler@0.1.0 - Respond after request fully read - deps: debug@1.0.4 * deps: parseurl@~1.2.0 - Cache URLs based on original value - Remove no-longer-needed URL mis-parse work-around - Simplify the "fast-path" `RegExp` * deps: send@0.7.0 - Add `dotfiles` option - Cap `maxAge` value to 1 year - deps: debug@1.0.4 - deps: depd@0.4.2 * deps: serve-static@~1.4.0 - deps: parseurl@~1.2.0 - deps: send@0.7.0 * perf: prevent multiple `Buffer` creation in `res.send` 4.6.1 / 2014-07-12 ================== * fix `subapp.mountpath` regression for `app.use(subapp)` 4.6.0 / 2014-07-11 ================== * accept multiple callbacks to `app.use()` * add explicit "Rosetta Flash JSONP abuse" protection - previous versions are not vulnerable; this is just explicit protection * catch errors in multiple `req.param(name, fn)` handlers * deprecate `res.redirect(url, status)` -- use `res.redirect(status, url)` instead * fix `res.send(status, num)` to send `num` as json (not error) * remove unnecessary escaping when `res.jsonp` returns JSON response * support non-string `path` in `app.use(path, fn)` - supports array of paths - supports `RegExp` * router: fix optimization on router exit * router: refactor location of `try` blocks * router: speed up standard `app.use(fn)` * deps: debug@1.0.3 - Add support for multiple wildcards in namespaces * deps: finalhandler@0.0.3 - deps: debug@1.0.3 * deps: methods@1.1.0 - add `CONNECT` * deps: parseurl@~1.1.3 - faster parsing of href-only URLs * deps: path-to-regexp@0.1.3 * deps: send@0.6.0 - deps: debug@1.0.3 * deps: serve-static@~1.3.2 - deps: parseurl@~1.1.3 - deps: send@0.6.0 * perf: fix arguments reassign deopt in some `res` methods 4.5.1 / 2014-07-06 ================== * fix routing regression when altering `req.method` 4.5.0 / 2014-07-04 ================== * add deprecation message to non-plural `req.accepts*` * add deprecation message to `res.send(body, status)` * add deprecation message to `res.vary()` * add `headers` option to `res.sendfile` - use to set headers on successful file transfer * add `mergeParams` option to `Router` - merges `req.params` from parent routes * add `req.hostname` -- correct name for what `req.host` returns * deprecate things with `depd` module * deprecate `req.host` -- use `req.hostname` instead * fix behavior when handling request without routes * fix handling when `route.all` is only route * invoke `router.param()` only when route matches * restore `req.params` after invoking router * use `finalhandler` for final response handling * use `media-typer` to alter content-type charset * deps: accepts@~1.0.7 * deps: send@0.5.0 - Accept string for `maxage` (converted by `ms`) - Include link in default redirect response * deps: serve-static@~1.3.0 - Accept string for `maxAge` (converted by `ms`) - Add `setHeaders` option - Include HTML link in redirect response - deps: send@0.5.0 * deps: type-is@~1.3.2 4.4.5 / 2014-06-26 ================== * deps: cookie-signature@1.0.4 - fix for timing attacks 4.4.4 / 2014-06-20 ================== * fix `res.attachment` Unicode filenames in Safari * fix "trim prefix" debug message in `express:router` * deps: accepts@~1.0.5 * deps: buffer-crc32@0.2.3 4.4.3 / 2014-06-11 ================== * fix persistence of modified `req.params[name]` from `app.param()` * deps: accepts@1.0.3 - deps: negotiator@0.4.6 * deps: debug@1.0.2 * deps: send@0.4.3 - Do not throw uncatchable error on file open race condition - Use `escape-html` for HTML escaping - deps: debug@1.0.2 - deps: finished@1.2.2 - deps: fresh@0.2.2 * deps: serve-static@1.2.3 - Do not throw uncatchable error on file open race condition - deps: send@0.4.3 4.4.2 / 2014-06-09 ================== * fix catching errors from top-level handlers * use `vary` module for `res.vary` * deps: debug@1.0.1 * deps: proxy-addr@1.0.1 * deps: send@0.4.2 - fix "event emitter leak" warnings - deps: debug@1.0.1 - deps: finished@1.2.1 * deps: serve-static@1.2.2 - fix "event emitter leak" warnings - deps: send@0.4.2 * deps: type-is@1.2.1 4.4.1 / 2014-06-02 ================== * deps: methods@1.0.1 * deps: send@0.4.1 - Send `max-age` in `Cache-Control` in correct format * deps: serve-static@1.2.1 - use `escape-html` for escaping - deps: send@0.4.1 4.4.0 / 2014-05-30 ================== * custom etag control with `app.set('etag', val)` - `app.set('etag', function(body, encoding){ return '"etag"' })` custom etag generation - `app.set('etag', 'weak')` weak tag - `app.set('etag', 'strong')` strong etag - `app.set('etag', false)` turn off - `app.set('etag', true)` standard etag * mark `res.send` ETag as weak and reduce collisions * update accepts to 1.0.2 - Fix interpretation when header not in request * update send to 0.4.0 - Calculate ETag with md5 for reduced collisions - Ignore stream errors after request ends - deps: debug@0.8.1 * update serve-static to 1.2.0 - Calculate ETag with md5 for reduced collisions - Ignore stream errors after request ends - deps: send@0.4.0 4.3.2 / 2014-05-28 ================== * fix handling of errors from `router.param()` callbacks 4.3.1 / 2014-05-23 ================== * revert "fix behavior of multiple `app.VERB` for the same path" - this caused a regression in the order of route execution 4.3.0 / 2014-05-21 ================== * add `req.baseUrl` to access the path stripped from `req.url` in routes * fix behavior of multiple `app.VERB` for the same path * fix issue routing requests among sub routers * invoke `router.param()` only when necessary instead of every match * proper proxy trust with `app.set('trust proxy', trust)` - `app.set('trust proxy', 1)` trust first hop - `app.set('trust proxy', 'loopback')` trust loopback addresses - `app.set('trust proxy', '10.0.0.1')` trust single IP - `app.set('trust proxy', '10.0.0.1/16')` trust subnet - `app.set('trust proxy', '10.0.0.1, 10.0.0.2')` trust list - `app.set('trust proxy', false)` turn off - `app.set('trust proxy', true)` trust everything * set proper `charset` in `Content-Type` for `res.send` * update type-is to 1.2.0 - support suffix matching 4.2.0 / 2014-05-11 ================== * deprecate `app.del()` -- use `app.delete()` instead * deprecate `res.json(obj, status)` -- use `res.json(status, obj)` instead - the edge-case `res.json(status, num)` requires `res.status(status).json(num)` * deprecate `res.jsonp(obj, status)` -- use `res.jsonp(status, obj)` instead - the edge-case `res.jsonp(status, num)` requires `res.status(status).jsonp(num)` * fix `req.next` when inside router instance * include `ETag` header in `HEAD` requests * keep previous `Content-Type` for `res.jsonp` * support PURGE method - add `app.purge` - add `router.purge` - include PURGE in `app.all` * update debug to 0.8.0 - add `enable()` method - change from stderr to stdout * update methods to 1.0.0 - add PURGE 4.1.2 / 2014-05-08 ================== * fix `req.host` for IPv6 literals * fix `res.jsonp` error if callback param is object 4.1.1 / 2014-04-27 ================== * fix package.json to reflect supported node version 4.1.0 / 2014-04-24 ================== * pass options from `res.sendfile` to `send` * preserve casing of headers in `res.header` and `res.set` * support unicode file names in `res.attachment` and `res.download` * update accepts to 1.0.1 - deps: negotiator@0.4.0 * update cookie to 0.1.2 - Fix for maxAge == 0 - made compat with expires field * update send to 0.3.0 - Accept API options in options object - Coerce option types - Control whether to generate etags - Default directory access to 403 when index disabled - Fix sending files with dots without root set - Include file path in etag - Make "Can't set headers after they are sent." catchable - Send full entity-body for multi range requests - Set etags to "weak" - Support "If-Range" header - Support multiple index paths - deps: mime@1.2.11 * update serve-static to 1.1.0 - Accept options directly to `send` module - Resolve relative paths at middleware setup - Use parseurl to parse the URL from request - deps: send@0.3.0 * update type-is to 1.1.0 - add non-array values support - add `multipart` as a shorthand 4.0.0 / 2014-04-09 ================== * remove: - node 0.8 support - connect and connect's patches except for charset handling - express(1) - moved to [express-generator](https://github.com/expressjs/generator) - `express.createServer()` - it has been deprecated for a long time. Use `express()` - `app.configure` - use logic in your own app code - `app.router` - is removed - `req.auth` - use `basic-auth` instead - `req.accepted*` - use `req.accepts*()` instead - `res.location` - relative URL resolution is removed - `res.charset` - include the charset in the content type when using `res.set()` - all bundled middleware except `static` * change: - `app.route` -> `app.mountpath` when mounting an express app in another express app - `json spaces` no longer enabled by default in development - `req.accepts*` -> `req.accepts*s` - i.e. `req.acceptsEncoding` -> `req.acceptsEncodings` - `req.params` is now an object instead of an array - `res.locals` is no longer a function. It is a plain js object. Treat it as such. - `res.headerSent` -> `res.headersSent` to match node.js ServerResponse object * refactor: - `req.accepts*` with [accepts](https://github.com/expressjs/accepts) - `req.is` with [type-is](https://github.com/expressjs/type-is) - [path-to-regexp](https://github.com/component/path-to-regexp) * add: - `app.router()` - returns the app Router instance - `app.route()` - Proxy to the app's `Router#route()` method to create a new route - Router & Route - public API 3.21.2 / 2015-07-31 =================== * deps: connect@2.30.2 - deps: body-parser@~1.13.3 - deps: compression@~1.5.2 - deps: errorhandler@~1.4.2 - deps: method-override@~2.3.5 - deps: serve-index@~1.7.2 - deps: type-is@~1.6.6 - deps: vhost@~3.0.1 * deps: vary@~1.0.1 - Fix setting empty header from empty `field` - perf: enable strict mode - perf: remove argument reassignments 3.21.1 / 2015-07-05 =================== * deps: basic-auth@~1.0.3 * deps: connect@2.30.1 - deps: body-parser@~1.13.2 - deps: compression@~1.5.1 - deps: errorhandler@~1.4.1 - deps: morgan@~1.6.1 - deps: pause@0.1.0 - deps: qs@4.0.0 - deps: serve-index@~1.7.1 - deps: type-is@~1.6.4 3.21.0 / 2015-06-18 =================== * deps: basic-auth@1.0.2 - perf: enable strict mode - perf: hoist regular expression - perf: parse with regular expressions - perf: remove argument reassignment * deps: connect@2.30.0 - deps: body-parser@~1.13.1 - deps: bytes@2.1.0 - deps: compression@~1.5.0 - deps: cookie@0.1.3 - deps: cookie-parser@~1.3.5 - deps: csurf@~1.8.3 - deps: errorhandler@~1.4.0 - deps: express-session@~1.11.3 - deps: finalhandler@0.4.0 - deps: fresh@0.3.0 - deps: morgan@~1.6.0 - deps: serve-favicon@~2.3.0 - deps: serve-index@~1.7.0 - deps: serve-static@~1.10.0 - deps: type-is@~1.6.3 * deps: cookie@0.1.3 - perf: deduce the scope of try-catch deopt - perf: remove argument reassignments * deps: escape-html@1.0.2 * deps: etag@~1.7.0 - Always include entity length in ETags for hash length extensions - Generate non-Stats ETags using MD5 only (no longer CRC32) - Improve stat performance by removing hashing - Improve support for JXcore - Remove base64 padding in ETags to shorten - Support "fake" stats objects in environments without fs - Use MD5 instead of MD4 in weak ETags over 1KB * deps: fresh@0.3.0 - Add weak `ETag` matching support * deps: mkdirp@0.5.1 - Work in global strict mode * deps: send@0.13.0 - Allow Node.js HTTP server to set `Date` response header - Fix incorrectly removing `Content-Location` on 304 response - Improve the default redirect response headers - Send appropriate headers on default error response - Use `http-errors` for standard emitted errors - Use `statuses` instead of `http` module for status messages - deps: escape-html@1.0.2 - deps: etag@~1.7.0 - deps: fresh@0.3.0 - deps: on-finished@~2.3.0 - perf: enable strict mode - perf: remove unnecessary array allocations 3.20.3 / 2015-05-17 =================== * deps: connect@2.29.2 - deps: body-parser@~1.12.4 - deps: compression@~1.4.4 - deps: connect-timeout@~1.6.2 - deps: debug@~2.2.0 - deps: depd@~1.0.1 - deps: errorhandler@~1.3.6 - deps: finalhandler@0.3.6 - deps: method-override@~2.3.3 - deps: morgan@~1.5.3 - deps: qs@2.4.2 - deps: response-time@~2.3.1 - deps: serve-favicon@~2.2.1 - deps: serve-index@~1.6.4 - deps: serve-static@~1.9.3 - deps: type-is@~1.6.2 * deps: debug@~2.2.0 - deps: ms@0.7.1 * deps: depd@~1.0.1 * deps: proxy-addr@~1.0.8 - deps: ipaddr.js@1.0.1 * deps: send@0.12.3 - deps: debug@~2.2.0 - deps: depd@~1.0.1 - deps: etag@~1.6.0 - deps: ms@0.7.1 - deps: on-finished@~2.2.1 3.20.2 / 2015-03-16 =================== * deps: connect@2.29.1 - deps: body-parser@~1.12.2 - deps: compression@~1.4.3 - deps: connect-timeout@~1.6.1 - deps: debug@~2.1.3 - deps: errorhandler@~1.3.5 - deps: express-session@~1.10.4 - deps: finalhandler@0.3.4 - deps: method-override@~2.3.2 - deps: morgan@~1.5.2 - deps: qs@2.4.1 - deps: serve-index@~1.6.3 - deps: serve-static@~1.9.2 - deps: type-is@~1.6.1 * deps: debug@~2.1.3 - Fix high intensity foreground color for bold - deps: ms@0.7.0 * deps: merge-descriptors@1.0.0 * deps: proxy-addr@~1.0.7 - deps: ipaddr.js@0.1.9 * deps: send@0.12.2 - Throw errors early for invalid `extensions` or `index` options - deps: debug@~2.1.3 3.20.1 / 2015-02-28 =================== * Fix `req.host` when using "trust proxy" hops count * Fix `req.protocol`/`req.secure` when using "trust proxy" hops count 3.20.0 / 2015-02-18 =================== * Fix `"trust proxy"` setting to inherit when app is mounted * Generate `ETag`s for all request responses - No longer restricted to only responses for `GET` and `HEAD` requests * Use `content-type` to parse `Content-Type` headers * deps: connect@2.29.0 - Use `content-type` to parse `Content-Type` headers - deps: body-parser@~1.12.0 - deps: compression@~1.4.1 - deps: connect-timeout@~1.6.0 - deps: cookie-parser@~1.3.4 - deps: cookie-signature@1.0.6 - deps: csurf@~1.7.0 - deps: errorhandler@~1.3.4 - deps: express-session@~1.10.3 - deps: http-errors@~1.3.1 - deps: response-time@~2.3.0 - deps: serve-index@~1.6.2 - deps: serve-static@~1.9.1 - deps: type-is@~1.6.0 * deps: cookie-signature@1.0.6 * deps: send@0.12.1 - Always read the stat size from the file - Fix mutating passed-in `options` - deps: mime@1.3.4 3.19.2 / 2015-02-01 =================== * deps: connect@2.28.3 - deps: compression@~1.3.1 - deps: csurf@~1.6.6 - deps: errorhandler@~1.3.3 - deps: express-session@~1.10.2 - deps: serve-index@~1.6.1 - deps: type-is@~1.5.6 * deps: proxy-addr@~1.0.6 - deps: ipaddr.js@0.1.8 3.19.1 / 2015-01-20 =================== * deps: connect@2.28.2 - deps: body-parser@~1.10.2 - deps: serve-static@~1.8.1 * deps: send@0.11.1 - Fix root path disclosure 3.19.0 / 2015-01-09 =================== * Fix `OPTIONS` responses to include the `HEAD` method property * Use `readline` for prompt in `express(1)` * deps: commander@2.6.0 * deps: connect@2.28.1 - deps: body-parser@~1.10.1 - deps: compression@~1.3.0 - deps: connect-timeout@~1.5.0 - deps: csurf@~1.6.4 - deps: debug@~2.1.1 - deps: errorhandler@~1.3.2 - deps: express-session@~1.10.1 - deps: finalhandler@0.3.3 - deps: method-override@~2.3.1 - deps: morgan@~1.5.1 - deps: serve-favicon@~2.2.0 - deps: serve-index@~1.6.0 - deps: serve-static@~1.8.0 - deps: type-is@~1.5.5 * deps: debug@~2.1.1 * deps: methods@~1.1.1 * deps: proxy-addr@~1.0.5 - deps: ipaddr.js@0.1.6 * deps: send@0.11.0 - deps: debug@~2.1.1 - deps: etag@~1.5.1 - deps: ms@0.7.0 - deps: on-finished@~2.2.0 3.18.6 / 2014-12-12 =================== * Fix exception in `req.fresh`/`req.stale` without response headers 3.18.5 / 2014-12-11 =================== * deps: connect@2.27.6 - deps: compression@~1.2.2 - deps: express-session@~1.9.3 - deps: http-errors@~1.2.8 - deps: serve-index@~1.5.3 - deps: type-is@~1.5.4 3.18.4 / 2014-11-23 =================== * deps: connect@2.27.4 - deps: body-parser@~1.9.3 - deps: compression@~1.2.1 - deps: errorhandler@~1.2.3 - deps: express-session@~1.9.2 - deps: qs@2.3.3 - deps: serve-favicon@~2.1.7 - deps: serve-static@~1.5.1 - deps: type-is@~1.5.3 * deps: etag@~1.5.1 * deps: proxy-addr@~1.0.4 - deps: ipaddr.js@0.1.5 3.18.3 / 2014-11-09 =================== * deps: connect@2.27.3 - Correctly invoke async callback asynchronously - deps: csurf@~1.6.3 3.18.2 / 2014-10-28 =================== * deps: connect@2.27.2 - Fix handling of URLs containing `://` in the path - deps: body-parser@~1.9.2 - deps: qs@2.3.2 3.18.1 / 2014-10-22 =================== * Fix internal `utils.merge` deprecation warnings * deps: connect@2.27.1 - deps: body-parser@~1.9.1 - deps: express-session@~1.9.1 - deps: finalhandler@0.3.2 - deps: morgan@~1.4.1 - deps: qs@2.3.0 - deps: serve-static@~1.7.1 * deps: send@0.10.1 - deps: on-finished@~2.1.1 3.18.0 / 2014-10-17 =================== * Use `content-disposition` module for `res.attachment`/`res.download` - Sends standards-compliant `Content-Disposition` header - Full Unicode support * Use `etag` module to generate `ETag` headers * deps: connect@2.27.0 - Use `http-errors` module for creating errors - Use `utils-merge` module for merging objects - deps: body-parser@~1.9.0 - deps: compression@~1.2.0 - deps: connect-timeout@~1.4.0 - deps: debug@~2.1.0 - deps: depd@~1.0.0 - deps: express-session@~1.9.0 - deps: finalhandler@0.3.1 - deps: method-override@~2.3.0 - deps: morgan@~1.4.0 - deps: response-time@~2.2.0 - deps: serve-favicon@~2.1.6 - deps: serve-index@~1.5.0 - deps: serve-static@~1.7.0 * deps: debug@~2.1.0 - Implement `DEBUG_FD` env variable support * deps: depd@~1.0.0 * deps: send@0.10.0 - deps: debug@~2.1.0 - deps: depd@~1.0.0 - deps: etag@~1.5.0 3.17.8 / 2014-10-15 =================== * deps: connect@2.26.6 - deps: compression@~1.1.2 - deps: csurf@~1.6.2 - deps: errorhandler@~1.2.2 3.17.7 / 2014-10-08 =================== * deps: connect@2.26.5 - Fix accepting non-object arguments to `logger` - deps: serve-static@~1.6.4 3.17.6 / 2014-10-02 =================== * deps: connect@2.26.4 - deps: morgan@~1.3.2 - deps: type-is@~1.5.2 3.17.5 / 2014-09-24 =================== * deps: connect@2.26.3 - deps: body-parser@~1.8.4 - deps: serve-favicon@~2.1.5 - deps: serve-static@~1.6.3 * deps: proxy-addr@~1.0.3 - Use `forwarded` npm module * deps: send@0.9.3 - deps: etag@~1.4.0 3.17.4 / 2014-09-19 =================== * deps: connect@2.26.2 - deps: body-parser@~1.8.3 - deps: qs@2.2.4 3.17.3 / 2014-09-18 =================== * deps: proxy-addr@~1.0.2 - Fix a global leak when multiple subnets are trusted - deps: ipaddr.js@0.1.3 3.17.2 / 2014-09-15 =================== * Use `crc` instead of `buffer-crc32` for speed * deps: connect@2.26.1 - deps: body-parser@~1.8.2 - deps: depd@0.4.5 - deps: express-session@~1.8.2 - deps: morgan@~1.3.1 - deps: serve-favicon@~2.1.3 - deps: serve-static@~1.6.2 * deps: depd@0.4.5 * deps: send@0.9.2 - deps: depd@0.4.5 - deps: etag@~1.3.1 - deps: range-parser@~1.0.2 3.17.1 / 2014-09-08 =================== * Fix error in `req.subdomains` on empty host 3.17.0 / 2014-09-08 =================== * Support `X-Forwarded-Host` in `req.subdomains` * Support IP address host in `req.subdomains` * deps: connect@2.26.0 - deps: body-parser@~1.8.1 - deps: compression@~1.1.0 - deps: connect-timeout@~1.3.0 - deps: cookie-parser@~1.3.3 - deps: cookie-signature@1.0.5 - deps: csurf@~1.6.1 - deps: debug@~2.0.0 - deps: errorhandler@~1.2.0 - deps: express-session@~1.8.1 - deps: finalhandler@0.2.0 - deps: fresh@0.2.4 - deps: media-typer@0.3.0 - deps: method-override@~2.2.0 - deps: morgan@~1.3.0 - deps: qs@2.2.3 - deps: serve-favicon@~2.1.3 - deps: serve-index@~1.2.1 - deps: serve-static@~1.6.1 - deps: type-is@~1.5.1 - deps: vhost@~3.0.0 * deps: cookie-signature@1.0.5 * deps: debug@~2.0.0 * deps: fresh@0.2.4 * deps: media-typer@0.3.0 - Throw error when parameter format invalid on parse * deps: range-parser@~1.0.2 * deps: send@0.9.1 - Add `lastModified` option - Use `etag` to generate `ETag` header - deps: debug@~2.0.0 - deps: fresh@0.2.4 * deps: vary@~1.0.0 - Accept valid `Vary` header string as `field` 3.16.10 / 2014-09-04 ==================== * deps: connect@2.25.10 - deps: serve-static@~1.5.4 * deps: send@0.8.5 - Fix a path traversal issue when using `root` - Fix malicious path detection for empty string path 3.16.9 / 2014-08-29 =================== * deps: connect@2.25.9 - deps: body-parser@~1.6.7 - deps: qs@2.2.2 3.16.8 / 2014-08-27 =================== * deps: connect@2.25.8 - deps: body-parser@~1.6.6 - deps: csurf@~1.4.1 - deps: qs@2.2.0 3.16.7 / 2014-08-18 =================== * deps: connect@2.25.7 - deps: body-parser@~1.6.5 - deps: express-session@~1.7.6 - deps: morgan@~1.2.3 - deps: serve-static@~1.5.3 * deps: send@0.8.3 - deps: destroy@1.0.3 - deps: on-finished@2.1.0 3.16.6 / 2014-08-14 =================== * deps: connect@2.25.6 - deps: body-parser@~1.6.4 - deps: qs@1.2.2 - deps: serve-static@~1.5.2 * deps: send@0.8.2 - Work around `fd` leak in Node.js 0.10 for `fs.ReadStream` 3.16.5 / 2014-08-11 =================== * deps: connect@2.25.5 - Fix backwards compatibility in `logger` 3.16.4 / 2014-08-10 =================== * Fix original URL parsing in `res.location` * deps: connect@2.25.4 - Fix `query` middleware breaking with argument - deps: body-parser@~1.6.3 - deps: compression@~1.0.11 - deps: connect-timeout@~1.2.2 - deps: express-session@~1.7.5 - deps: method-override@~2.1.3 - deps: on-headers@~1.0.0 - deps: parseurl@~1.3.0 - deps: qs@1.2.1 - deps: response-time@~2.0.1 - deps: serve-index@~1.1.6 - deps: serve-static@~1.5.1 * deps: parseurl@~1.3.0 3.16.3 / 2014-08-07 =================== * deps: connect@2.25.3 - deps: multiparty@3.3.2 3.16.2 / 2014-08-07 =================== * deps: connect@2.25.2 - deps: body-parser@~1.6.2 - deps: qs@1.2.0 3.16.1 / 2014-08-06 =================== * deps: connect@2.25.1 - deps: body-parser@~1.6.1 - deps: qs@1.1.0 3.16.0 / 2014-08-05 =================== * deps: connect@2.25.0 - deps: body-parser@~1.6.0 - deps: compression@~1.0.10 - deps: csurf@~1.4.0 - deps: express-session@~1.7.4 - deps: qs@1.0.2 - deps: serve-static@~1.5.0 * deps: send@0.8.1 - Add `extensions` option 3.15.3 / 2014-08-04 =================== * fix `res.sendfile` regression for serving directory index files * deps: connect@2.24.3 - deps: serve-index@~1.1.5 - deps: serve-static@~1.4.4 * deps: send@0.7.4 - Fix incorrect 403 on Windows and Node.js 0.11 - Fix serving index files without root dir 3.15.2 / 2014-07-27 =================== * deps: connect@2.24.2 - deps: body-parser@~1.5.2 - deps: depd@0.4.4 - deps: express-session@~1.7.2 - deps: morgan@~1.2.2 - deps: serve-static@~1.4.2 * deps: depd@0.4.4 - Work-around v8 generating empty stack traces * deps: send@0.7.2 - deps: depd@0.4.4 3.15.1 / 2014-07-26 =================== * deps: connect@2.24.1 - deps: body-parser@~1.5.1 - deps: depd@0.4.3 - deps: express-session@~1.7.1 - deps: morgan@~1.2.1 - deps: serve-index@~1.1.4 - deps: serve-static@~1.4.1 * deps: depd@0.4.3 - Fix exception when global `Error.stackTraceLimit` is too low * deps: send@0.7.1 - deps: depd@0.4.3 3.15.0 / 2014-07-22 =================== * Fix `req.protocol` for proxy-direct connections * Pass options from `res.sendfile` to `send` * deps: connect@2.24.0 - deps: body-parser@~1.5.0 - deps: compression@~1.0.9 - deps: connect-timeout@~1.2.1 - deps: debug@1.0.4 - deps: depd@0.4.2 - deps: express-session@~1.7.0 - deps: finalhandler@0.1.0 - deps: method-override@~2.1.2 - deps: morgan@~1.2.0 - deps: multiparty@3.3.1 - deps: parseurl@~1.2.0 - deps: serve-static@~1.4.0 * deps: debug@1.0.4 * deps: depd@0.4.2 - Add `TRACE_DEPRECATION` environment variable - Remove non-standard grey color from color output - Support `--no-deprecation` argument - Support `--trace-deprecation` argument * deps: parseurl@~1.2.0 - Cache URLs based on original value - Remove no-longer-needed URL mis-parse work-around - Simplify the "fast-path" `RegExp` * deps: send@0.7.0 - Add `dotfiles` option - Cap `maxAge` value to 1 year - deps: debug@1.0.4 - deps: depd@0.4.2 3.14.0 / 2014-07-11 =================== * add explicit "Rosetta Flash JSONP abuse" protection - previous versions are not vulnerable; this is just explicit protection * deprecate `res.redirect(url, status)` -- use `res.redirect(status, url)` instead * fix `res.send(status, num)` to send `num` as json (not error) * remove unnecessary escaping when `res.jsonp` returns JSON response * deps: basic-auth@1.0.0 - support empty password - support empty username * deps: connect@2.23.0 - deps: debug@1.0.3 - deps: express-session@~1.6.4 - deps: method-override@~2.1.0 - deps: parseurl@~1.1.3 - deps: serve-static@~1.3.1 * deps: debug@1.0.3 - Add support for multiple wildcards in namespaces * deps: methods@1.1.0 - add `CONNECT` * deps: parseurl@~1.1.3 - faster parsing of href-only URLs 3.13.0 / 2014-07-03 =================== * add deprecation message to `app.configure` * add deprecation message to `req.auth` * use `basic-auth` to parse `Authorization` header * deps: connect@2.22.0 - deps: csurf@~1.3.0 - deps: express-session@~1.6.1 - deps: multiparty@3.3.0 - deps: serve-static@~1.3.0 * deps: send@0.5.0 - Accept string for `maxage` (converted by `ms`) - Include link in default redirect response 3.12.1 / 2014-06-26 =================== * deps: connect@2.21.1 - deps: cookie-parser@1.3.2 - deps: cookie-signature@1.0.4 - deps: express-session@~1.5.2 - deps: type-is@~1.3.2 * deps: cookie-signature@1.0.4 - fix for timing attacks 3.12.0 / 2014-06-21 =================== * use `media-typer` to alter content-type charset * deps: connect@2.21.0 - deprecate `connect(middleware)` -- use `app.use(middleware)` instead - deprecate `connect.createServer()` -- use `connect()` instead - fix `res.setHeader()` patch to work with with get -> append -> set pattern - deps: compression@~1.0.8 - deps: errorhandler@~1.1.1 - deps: express-session@~1.5.0 - deps: serve-index@~1.1.3 3.11.0 / 2014-06-19 =================== * deprecate things with `depd` module * deps: buffer-crc32@0.2.3 * deps: connect@2.20.2 - deprecate `verify` option to `json` -- use `body-parser` npm module instead - deprecate `verify` option to `urlencoded` -- use `body-parser` npm module instead - deprecate things with `depd` module - use `finalhandler` for final response handling - use `media-typer` to parse `content-type` for charset - deps: body-parser@1.4.3 - deps: connect-timeout@1.1.1 - deps: cookie-parser@1.3.1 - deps: csurf@1.2.2 - deps: errorhandler@1.1.0 - deps: express-session@1.4.0 - deps: multiparty@3.2.9 - deps: serve-index@1.1.2 - deps: type-is@1.3.1 - deps: vhost@2.0.0 3.10.5 / 2014-06-11 =================== * deps: connect@2.19.6 - deps: body-parser@1.3.1 - deps: compression@1.0.7 - deps: debug@1.0.2 - deps: serve-index@1.1.1 - deps: serve-static@1.2.3 * deps: debug@1.0.2 * deps: send@0.4.3 - Do not throw uncatchable error on file open race condition - Use `escape-html` for HTML escaping - deps: debug@1.0.2 - deps: finished@1.2.2 - deps: fresh@0.2.2 3.10.4 / 2014-06-09 =================== * deps: connect@2.19.5 - fix "event emitter leak" warnings - deps: csurf@1.2.1 - deps: debug@1.0.1 - deps: serve-static@1.2.2 - deps: type-is@1.2.1 * deps: debug@1.0.1 * deps: send@0.4.2 - fix "event emitter leak" warnings - deps: finished@1.2.1 - deps: debug@1.0.1 3.10.3 / 2014-06-05 =================== * use `vary` module for `res.vary` * deps: connect@2.19.4 - deps: errorhandler@1.0.2 - deps: method-override@2.0.2 - deps: serve-favicon@2.0.1 * deps: debug@1.0.0 3.10.2 / 2014-06-03 =================== * deps: connect@2.19.3 - deps: compression@1.0.6 3.10.1 / 2014-06-03 =================== * deps: connect@2.19.2 - deps: compression@1.0.4 * deps: proxy-addr@1.0.1 3.10.0 / 2014-06-02 =================== * deps: connect@2.19.1 - deprecate `methodOverride()` -- use `method-override` npm module instead - deps: body-parser@1.3.0 - deps: method-override@2.0.1 - deps: multiparty@3.2.8 - deps: response-time@2.0.0 - deps: serve-static@1.2.1 * deps: methods@1.0.1 * deps: send@0.4.1 - Send `max-age` in `Cache-Control` in correct format 3.9.0 / 2014-05-30 ================== * custom etag control with `app.set('etag', val)` - `app.set('etag', function(body, encoding){ return '"etag"' })` custom etag generation - `app.set('etag', 'weak')` weak tag - `app.set('etag', 'strong')` strong etag - `app.set('etag', false)` turn off - `app.set('etag', true)` standard etag * Include ETag in HEAD requests * mark `res.send` ETag as weak and reduce collisions * update connect to 2.18.0 - deps: compression@1.0.3 - deps: serve-index@1.1.0 - deps: serve-static@1.2.0 * update send to 0.4.0 - Calculate ETag with md5 for reduced collisions - Ignore stream errors after request ends - deps: debug@0.8.1 3.8.1 / 2014-05-27 ================== * update connect to 2.17.3 - deps: body-parser@1.2.2 - deps: express-session@1.2.1 - deps: method-override@1.0.2 3.8.0 / 2014-05-21 ================== * keep previous `Content-Type` for `res.jsonp` * set proper `charset` in `Content-Type` for `res.send` * update connect to 2.17.1 - fix `res.charset` appending charset when `content-type` has one - deps: express-session@1.2.0 - deps: morgan@1.1.1 - deps: serve-index@1.0.3 3.7.0 / 2014-05-18 ================== * proper proxy trust with `app.set('trust proxy', trust)` - `app.set('trust proxy', 1)` trust first hop - `app.set('trust proxy', 'loopback')` trust loopback addresses - `app.set('trust proxy', '10.0.0.1')` trust single IP - `app.set('trust proxy', '10.0.0.1/16')` trust subnet - `app.set('trust proxy', '10.0.0.1, 10.0.0.2')` trust list - `app.set('trust proxy', false)` turn off - `app.set('trust proxy', true)` trust everything * update connect to 2.16.2 - deprecate `res.headerSent` -- use `res.headersSent` - deprecate `res.on("header")` -- use on-headers module instead - fix edge-case in `res.appendHeader` that would append in wrong order - json: use body-parser - urlencoded: use body-parser - dep: bytes@1.0.0 - dep: cookie-parser@1.1.0 - dep: csurf@1.2.0 - dep: express-session@1.1.0 - dep: method-override@1.0.1 3.6.0 / 2014-05-09 ================== * deprecate `app.del()` -- use `app.delete()` instead * deprecate `res.json(obj, status)` -- use `res.json(status, obj)` instead - the edge-case `res.json(status, num)` requires `res.status(status).json(num)` * deprecate `res.jsonp(obj, status)` -- use `res.jsonp(status, obj)` instead - the edge-case `res.jsonp(status, num)` requires `res.status(status).jsonp(num)` * support PURGE method - add `app.purge` - add `router.purge` - include PURGE in `app.all` * update connect to 2.15.0 * Add `res.appendHeader` * Call error stack even when response has been sent * Patch `res.headerSent` to return Boolean * Patch `res.headersSent` for node.js 0.8 * Prevent default 404 handler after response sent * dep: compression@1.0.2 * dep: connect-timeout@1.1.0 * dep: debug@^0.8.0 * dep: errorhandler@1.0.1 * dep: express-session@1.0.4 * dep: morgan@1.0.1 * dep: serve-favicon@2.0.0 * dep: serve-index@1.0.2 * update debug to 0.8.0 * add `enable()` method * change from stderr to stdout * update methods to 1.0.0 - add PURGE * update mkdirp to 0.5.0 3.5.3 / 2014-05-08 ================== * fix `req.host` for IPv6 literals * fix `res.jsonp` error if callback param is object 3.5.2 / 2014-04-24 ================== * update connect to 2.14.5 * update cookie to 0.1.2 * update mkdirp to 0.4.0 * update send to 0.3.0 3.5.1 / 2014-03-25 ================== * pin less-middleware in generated app 3.5.0 / 2014-03-06 ================== * bump deps 3.4.8 / 2014-01-13 ================== * prevent incorrect automatic OPTIONS responses #1868 @dpatti * update binary and examples for jade 1.0 #1876 @yossi, #1877 @reqshark, #1892 @matheusazzi * throw 400 in case of malformed paths @rlidwka 3.4.7 / 2013-12-10 ================== * update connect 3.4.6 / 2013-12-01 ================== * update connect (raw-body) 3.4.5 / 2013-11-27 ================== * update connect * res.location: remove leading ./ #1802 @kapouer * res.redirect: fix `res.redirect('toString') #1829 @michaelficarra * res.send: always send ETag when content-length > 0 * router: add Router.all() method 3.4.4 / 2013-10-29 ================== * update connect * update supertest * update methods * express(1): replace bodyParser() with urlencoded() and json() #1795 @chirag04 3.4.3 / 2013-10-23 ================== * update connect 3.4.2 / 2013-10-18 ================== * update connect * downgrade commander 3.4.1 / 2013-10-15 ================== * update connect * update commander * jsonp: check if callback is a function * router: wrap encodeURIComponent in a try/catch #1735 (@lxe) * res.format: now includes charset @1747 (@sorribas) * res.links: allow multiple calls @1746 (@sorribas) 3.4.0 / 2013-09-07 ================== * add res.vary(). Closes #1682 * update connect 3.3.8 / 2013-09-02 ================== * update connect 3.3.7 / 2013-08-28 ================== * update connect 3.3.6 / 2013-08-27 ================== * Revert "remove charset from json responses. Closes #1631" (causes issues in some clients) * add: req.accepts take an argument list 3.3.4 / 2013-07-08 ================== * update send and connect 3.3.3 / 2013-07-04 ================== * update connect 3.3.2 / 2013-07-03 ================== * update connect * update send * remove .version export 3.3.1 / 2013-06-27 ================== * update connect 3.3.0 / 2013-06-26 ================== * update connect * add support for multiple X-Forwarded-Proto values. Closes #1646 * change: remove charset from json responses. Closes #1631 * change: return actual booleans from req.accept* functions * fix jsonp callback array throw 3.2.6 / 2013-06-02 ================== * update connect 3.2.5 / 2013-05-21 ================== * update connect * update node-cookie * add: throw a meaningful error when there is no default engine * change generation of ETags with res.send() to GET requests only. Closes #1619 3.2.4 / 2013-05-09 ================== * fix `req.subdomains` when no Host is present * fix `req.host` when no Host is present, return undefined 3.2.3 / 2013-05-07 ================== * update connect / qs 3.2.2 / 2013-05-03 ================== * update qs 3.2.1 / 2013-04-29 ================== * add app.VERB() paths array deprecation warning * update connect * update qs and remove all ~ semver crap * fix: accept number as value of Signed Cookie 3.2.0 / 2013-04-15 ================== * add "view" constructor setting to override view behaviour * add req.acceptsEncoding(name) * add req.acceptedEncodings * revert cookie signature change causing session race conditions * fix sorting of Accept values of the same quality 3.1.2 / 2013-04-12 ================== * add support for custom Accept parameters * update cookie-signature 3.1.1 / 2013-04-01 ================== * add X-Forwarded-Host support to `req.host` * fix relative redirects * update mkdirp * update buffer-crc32 * remove legacy app.configure() method from app template. 3.1.0 / 2013-01-25 ================== * add support for leading "." in "view engine" setting * add array support to `res.set()` * add node 0.8.x to travis.yml * add "subdomain offset" setting for tweaking `req.subdomains` * add `res.location(url)` implementing `res.redirect()`-like setting of Location * use app.get() for x-powered-by setting for inheritance * fix colons in passwords for `req.auth` 3.0.6 / 2013-01-04 ================== * add http verb methods to Router * update connect * fix mangling of the `res.cookie()` options object * fix jsonp whitespace escape. Closes #1132 3.0.5 / 2012-12-19 ================== * add throwing when a non-function is passed to a route * fix: explicitly remove Transfer-Encoding header from 204 and 304 responses * revert "add 'etag' option" 3.0.4 / 2012-12-05 ================== * add 'etag' option to disable `res.send()` Etags * add escaping of urls in text/plain in `res.redirect()` for old browsers interpreting as html * change crc32 module for a more liberal license * update connect 3.0.3 / 2012-11-13 ================== * update connect * update cookie module * fix cookie max-age 3.0.2 / 2012-11-08 ================== * add OPTIONS to cors example. Closes #1398 * fix route chaining regression. Closes #1397 3.0.1 / 2012-11-01 ================== * update connect 3.0.0 / 2012-10-23 ================== * add `make clean` * add "Basic" check to req.auth * add `req.auth` test coverage * add cb && cb(payload) to `res.jsonp()`. Closes #1374 * add backwards compat for `res.redirect()` status. Closes #1336 * add support for `res.json()` to retain previously defined Content-Types. Closes #1349 * update connect * change `res.redirect()` to utilize a pathname-relative Location again. Closes #1382 * remove non-primitive string support for `res.send()` * fix view-locals example. Closes #1370 * fix route-separation example 3.0.0rc5 / 2012-09-18 ================== * update connect * add redis search example * add static-files example * add "x-powered-by" setting (`app.disable('x-powered-by')`) * add "application/octet-stream" redirect Accept test case. Closes #1317 3.0.0rc4 / 2012-08-30 ================== * add `res.jsonp()`. Closes #1307 * add "verbose errors" option to error-pages example * add another route example to express(1) so people are not so confused * add redis online user activity tracking example * update connect dep * fix etag quoting. Closes #1310 * fix error-pages 404 status * fix jsonp callback char restrictions * remove old OPTIONS default response 3.0.0rc3 / 2012-08-13 ================== * update connect dep * fix signed cookies to work with `connect.cookieParser()` ("s:" prefix was missing) [tnydwrds] * fix `res.render()` clobbering of "locals" 3.0.0rc2 / 2012-08-03 ================== * add CORS example * update connect dep * deprecate `.createServer()` & remove old stale examples * fix: escape `res.redirect()` link * fix vhost example 3.0.0rc1 / 2012-07-24 ================== * add more examples to view-locals * add scheme-relative redirects (`res.redirect("//foo.com")`) support * update cookie dep * update connect dep * update send dep * fix `express(1)` -h flag, use -H for hogan. Closes #1245 * fix `res.sendfile()` socket error handling regression 3.0.0beta7 / 2012-07-16 ================== * update connect dep for `send()` root normalization regression 3.0.0beta6 / 2012-07-13 ================== * add `err.view` property for view errors. Closes #1226 * add "jsonp callback name" setting * add support for "/foo/:bar*" non-greedy matches * change `res.sendfile()` to use `send()` module * change `res.send` to use "response-send" module * remove `app.locals.use` and `res.locals.use`, use regular middleware 3.0.0beta5 / 2012-07-03 ================== * add "make check" support * add route-map example * add `res.json(obj, status)` support back for BC * add "methods" dep, remove internal methods module * update connect dep * update auth example to utilize cores pbkdf2 * updated tests to use "supertest" 3.0.0beta4 / 2012-06-25 ================== * Added `req.auth` * Added `req.range(size)` * Added `res.links(obj)` * Added `res.send(body, status)` support back for backwards compat * Added `.default()` support to `res.format()` * Added 2xx / 304 check to `req.fresh` * Revert "Added + support to the router" * Fixed `res.send()` freshness check, respect res.statusCode 3.0.0beta3 / 2012-06-15 ================== * Added hogan `--hjs` to express(1) [nullfirm] * Added another example to content-negotiation * Added `fresh` dep * Changed: `res.send()` always checks freshness * Fixed: expose connects mime module. Closes #1165 3.0.0beta2 / 2012-06-06 ================== * Added `+` support to the router * Added `req.host` * Changed `req.param()` to check route first * Update connect dep 3.0.0beta1 / 2012-06-01 ================== * Added `res.format()` callback to override default 406 behaviour * Fixed `res.redirect()` 406. Closes #1154 3.0.0alpha5 / 2012-05-30 ================== * Added `req.ip` * Added `{ signed: true }` option to `res.cookie()` * Removed `res.signedCookie()` * Changed: dont reverse `req.ips` * Fixed "trust proxy" setting check for `req.ips` 3.0.0alpha4 / 2012-05-09 ================== * Added: allow `[]` in jsonp callback. Closes #1128 * Added `PORT` env var support in generated template. Closes #1118 [benatkin] * Updated: connect 2.2.2 3.0.0alpha3 / 2012-05-04 ================== * Added public `app.routes`. Closes #887 * Added _view-locals_ example * Added _mvc_ example * Added `res.locals.use()`. Closes #1120 * Added conditional-GET support to `res.send()` * Added: coerce `res.set()` values to strings * Changed: moved `static()` in generated apps below router * Changed: `res.send()` only set ETag when not previously set * Changed connect 2.2.1 dep * Changed: `make test` now runs unit / acceptance tests * Fixed req/res proto inheritance 3.0.0alpha2 / 2012-04-26 ================== * Added `make benchmark` back * Added `res.send()` support for `String` objects * Added client-side data exposing example * Added `res.header()` and `req.header()` aliases for BC * Added `express.createServer()` for BC * Perf: memoize parsed urls * Perf: connect 2.2.0 dep * Changed: make `expressInit()` middleware self-aware * Fixed: use app.get() for all core settings * Fixed redis session example * Fixed session example. Closes #1105 * Fixed generated express dep. Closes #1078 3.0.0alpha1 / 2012-04-15 ================== * Added `app.locals.use(callback)` * Added `app.locals` object * Added `app.locals(obj)` * Added `res.locals` object * Added `res.locals(obj)` * Added `res.format()` for content-negotiation * Added `app.engine()` * Added `res.cookie()` JSON cookie support * Added "trust proxy" setting * Added `req.subdomains` * Added `req.protocol` * Added `req.secure` * Added `req.path` * Added `req.ips` * Added `req.fresh` * Added `req.stale` * Added comma-delimited / array support for `req.accepts()` * Added debug instrumentation * Added `res.set(obj)` * Added `res.set(field, value)` * Added `res.get(field)` * Added `app.get(setting)`. Closes #842 * Added `req.acceptsLanguage()` * Added `req.acceptsCharset()` * Added `req.accepted` * Added `req.acceptedLanguages` * Added `req.acceptedCharsets` * Added "json replacer" setting * Added "json spaces" setting * Added X-Forwarded-Proto support to `res.redirect()`. Closes #92 * Added `--less` support to express(1) * Added `express.response` prototype * Added `express.request` prototype * Added `express.application` prototype * Added `app.path()` * Added `app.render()` * Added `res.type()` to replace `res.contentType()` * Changed: `res.redirect()` to add relative support * Changed: enable "jsonp callback" by default * Changed: renamed "case sensitive routes" to "case sensitive routing" * Rewrite of all tests with mocha * Removed "root" setting * Removed `res.redirect('home')` support * Removed `req.notify()` * Removed `app.register()` * Removed `app.redirect()` * Removed `app.is()` * Removed `app.helpers()` * Removed `app.dynamicHelpers()` * Fixed `res.sendfile()` with non-GET. Closes #723 * Fixed express(1) public dir for windows. Closes #866 2.5.9/ 2012-04-02 ================== * Added support for PURGE request method [pbuyle] * Fixed `express(1)` generated app `app.address()` before `listening` [mmalecki] 2.5.8 / 2012-02-08 ================== * Update mkdirp dep. Closes #991 2.5.7 / 2012-02-06 ================== * Fixed `app.all` duplicate DELETE requests [mscdex] 2.5.6 / 2012-01-13 ================== * Updated hamljs dev dep. Closes #953 2.5.5 / 2012-01-08 ================== * Fixed: set `filename` on cached templates [matthewleon] 2.5.4 / 2012-01-02 ================== * Fixed `express(1)` eol on 0.4.x. Closes #947 2.5.3 / 2011-12-30 ================== * Fixed `req.is()` when a charset is present 2.5.2 / 2011-12-10 ================== * Fixed: express(1) LF -> CRLF for windows 2.5.1 / 2011-11-17 ================== * Changed: updated connect to 1.8.x * Removed sass.js support from express(1) 2.5.0 / 2011-10-24 ================== * Added ./routes dir for generated app by default * Added npm install reminder to express(1) app gen * Added 0.5.x support * Removed `make test-cov` since it wont work with node 0.5.x * Fixed express(1) public dir for windows. Closes #866 2.4.7 / 2011-10-05 ================== * Added mkdirp to express(1). Closes #795 * Added simple _json-config_ example * Added shorthand for the parsed request's pathname via `req.path` * Changed connect dep to 1.7.x to fix npm issue... * Fixed `res.redirect()` __HEAD__ support. [reported by xerox] * Fixed `req.flash()`, only escape args * Fixed absolute path checking on windows. Closes #829 [reported by andrewpmckenzie] 2.4.6 / 2011-08-22 ================== * Fixed multiple param callback regression. Closes #824 [reported by TroyGoode] 2.4.5 / 2011-08-19 ================== * Added support for routes to handle errors. Closes #809 * Added `app.routes.all()`. Closes #803 * Added "basepath" setting to work in conjunction with reverse proxies etc. * Refactored `Route` to use a single array of callbacks * Added support for multiple callbacks for `app.param()`. Closes #801 Closes #805 * Changed: removed .call(self) for route callbacks * Dependency: `qs >= 0.3.1` * Fixed `res.redirect()` on windows due to `join()` usage. Closes #808 2.4.4 / 2011-08-05 ================== * Fixed `res.header()` intention of a set, even when `undefined` * Fixed `*`, value no longer required * Fixed `res.send(204)` support. Closes #771 2.4.3 / 2011-07-14 ================== * Added docs for `status` option special-case. Closes #739 * Fixed `options.filename`, exposing the view path to template engines 2.4.2. / 2011-07-06 ================== * Revert "removed jsonp stripping" for XSS 2.4.1 / 2011-07-06 ================== * Added `res.json()` JSONP support. Closes #737 * Added _extending-templates_ example. Closes #730 * Added "strict routing" setting for trailing slashes * Added support for multiple envs in `app.configure()` calls. Closes #735 * Changed: `res.send()` using `res.json()` * Changed: when cookie `path === null` don't default it * Changed; default cookie path to "home" setting. Closes #731 * Removed _pids/logs_ creation from express(1) 2.4.0 / 2011-06-28 ================== * Added chainable `res.status(code)` * Added `res.json()`, an explicit version of `res.send(obj)` * Added simple web-service example 2.3.12 / 2011-06-22 ================== * \#express is now on freenode! come join! * Added `req.get(field, param)` * Added links to Japanese documentation, thanks @hideyukisaito! * Added; the `express(1)` generated app outputs the env * Added `content-negotiation` example * Dependency: connect >= 1.5.1 < 2.0.0 * Fixed view layout bug. Closes #720 * Fixed; ignore body on 304. Closes #701 2.3.11 / 2011-06-04 ================== * Added `npm test` * Removed generation of dummy test file from `express(1)` * Fixed; `express(1)` adds express as a dep * Fixed; prune on `prepublish` 2.3.10 / 2011-05-27 ================== * Added `req.route`, exposing the current route * Added _package.json_ generation support to `express(1)` * Fixed call to `app.param()` function for optional params. Closes #682 2.3.9 / 2011-05-25 ================== * Fixed bug-ish with `../' in `res.partial()` calls 2.3.8 / 2011-05-24 ================== * Fixed `app.options()` 2.3.7 / 2011-05-23 ================== * Added route `Collection`, ex: `app.get('/user/:id').remove();` * Added support for `app.param(fn)` to define param logic * Removed `app.param()` support for callback with return value * Removed module.parent check from express(1) generated app. Closes #670 * Refactored router. Closes #639 2.3.6 / 2011-05-20 ================== * Changed; using devDependencies instead of git submodules * Fixed redis session example * Fixed markdown example * Fixed view caching, should not be enabled in development 2.3.5 / 2011-05-20 ================== * Added export `.view` as alias for `.View` 2.3.4 / 2011-05-08 ================== * Added `./examples/say` * Fixed `res.sendfile()` bug preventing the transfer of files with spaces 2.3.3 / 2011-05-03 ================== * Added "case sensitive routes" option. * Changed; split methods supported per rfc [slaskis] * Fixed route-specific middleware when using the same callback function several times 2.3.2 / 2011-04-27 ================== * Fixed view hints 2.3.1 / 2011-04-26 ================== * Added `app.match()` as `app.match.all()` * Added `app.lookup()` as `app.lookup.all()` * Added `app.remove()` for `app.remove.all()` * Added `app.remove.VERB()` * Fixed template caching collision issue. Closes #644 * Moved router over from connect and started refactor 2.3.0 / 2011-04-25 ================== * Added options support to `res.clearCookie()` * Added `res.helpers()` as alias of `res.locals()` * Added; json defaults to UTF-8 with `res.send()`. Closes #632. [Daniel * Dependency `connect >= 1.4.0` * Changed; auto set Content-Type in res.attachement [Aaron Heckmann] * Renamed "cache views" to "view cache". Closes #628 * Fixed caching of views when using several apps. Closes #637 * Fixed gotcha invoking `app.param()` callbacks once per route middleware. Closes #638 * Fixed partial lookup precedence. Closes #631 Shaw] 2.2.2 / 2011-04-12 ================== * Added second callback support for `res.download()` connection errors * Fixed `filename` option passing to template engine 2.2.1 / 2011-04-04 ================== * Added `layout(path)` helper to change the layout within a view. Closes #610 * Fixed `partial()` collection object support. Previously only anything with `.length` would work. When `.length` is present one must still be aware of holes, however now `{ collection: {foo: 'bar'}}` is valid, exposes `keyInCollection` and `keysInCollection`. * Performance improved with better view caching * Removed `request` and `response` locals * Changed; errorHandler page title is now `Express` instead of `Connect` 2.2.0 / 2011-03-30 ================== * Added `app.lookup.VERB()`, ex `app.lookup.put('/user/:id')`. Closes #606 * Added `app.match.VERB()`, ex `app.match.put('/user/12')`. Closes #606 * Added `app.VERB(path)` as alias of `app.lookup.VERB()`. * Dependency `connect >= 1.2.0` 2.1.1 / 2011-03-29 ================== * Added; expose `err.view` object when failing to locate a view * Fixed `res.partial()` call `next(err)` when no callback is given [reported by aheckmann] * Fixed; `res.send(undefined)` responds with 204 [aheckmann] 2.1.0 / 2011-03-24 ================== * Added `<root>/_?<name>` partial lookup support. Closes #447 * Added `request`, `response`, and `app` local variables * Added `settings` local variable, containing the app's settings * Added `req.flash()` exception if `req.session` is not available * Added `res.send(bool)` support (json response) * Fixed stylus example for latest version * Fixed; wrap try/catch around `res.render()` 2.0.0 / 2011-03-17 ================== * Fixed up index view path alternative. * Changed; `res.locals()` without object returns the locals 2.0.0rc3 / 2011-03-17 ================== * Added `res.locals(obj)` to compliment `res.local(key, val)` * Added `res.partial()` callback support * Fixed recursive error reporting issue in `res.render()` 2.0.0rc2 / 2011-03-17 ================== * Changed; `partial()` "locals" are now optional * Fixed `SlowBuffer` support. Closes #584 [reported by tyrda01] * Fixed .filename view engine option [reported by drudge] * Fixed blog example * Fixed `{req,res}.app` reference when mounting [Ben Weaver] 2.0.0rc / 2011-03-14 ================== * Fixed; expose `HTTPSServer` constructor * Fixed express(1) default test charset. Closes #579 [reported by secoif] * Fixed; default charset to utf-8 instead of utf8 for lame IE [reported by NickP] 2.0.0beta3 / 2011-03-09 ================== * Added support for `res.contentType()` literal The original `res.contentType('.json')`, `res.contentType('application/json')`, and `res.contentType('json')` will work now. * Added `res.render()` status option support back * Added charset option for `res.render()` * Added `.charset` support (via connect 1.0.4) * Added view resolution hints when in development and a lookup fails * Added layout lookup support relative to the page view. For example while rendering `./views/user/index.jade` if you create `./views/user/layout.jade` it will be used in favour of the root layout. * Fixed `res.redirect()`. RFC states absolute url [reported by unlink] * Fixed; default `res.send()` string charset to utf8 * Removed `Partial` constructor (not currently used) 2.0.0beta2 / 2011-03-07 ================== * Added res.render() `.locals` support back to aid in migration process * Fixed flash example 2.0.0beta / 2011-03-03 ================== * Added HTTPS support * Added `res.cookie()` maxAge support * Added `req.header()` _Referrer_ / _Referer_ special-case, either works * Added mount support for `res.redirect()`, now respects the mount-point * Added `union()` util, taking place of `merge(clone())` combo * Added stylus support to express(1) generated app * Added secret to session middleware used in examples and generated app * Added `res.local(name, val)` for progressive view locals * Added default param support to `req.param(name, default)` * Added `app.disabled()` and `app.enabled()` * Added `app.register()` support for omitting leading ".", either works * Added `res.partial()`, using the same interface as `partial()` within a view. Closes #539 * Added `app.param()` to map route params to async/sync logic * Added; aliased `app.helpers()` as `app.locals()`. Closes #481 * Added extname with no leading "." support to `res.contentType()` * Added `cache views` setting, defaulting to enabled in "production" env * Added index file partial resolution, eg: partial('user') may try _views/user/index.jade_. * Added `req.accepts()` support for extensions * Changed; `res.download()` and `res.sendfile()` now utilize Connect's static file server `connect.static.send()`. * Changed; replaced `connect.utils.mime()` with npm _mime_ module * Changed; allow `req.query` to be pre-defined (via middleware or other parent * Changed view partial resolution, now relative to parent view * Changed view engine signature. no longer `engine.render(str, options, callback)`, now `engine.compile(str, options) -> Function`, the returned function accepts `fn(locals)`. * Fixed `req.param()` bug returning Array.prototype methods. Closes #552 * Fixed; using `Stream#pipe()` instead of `sys.pump()` in `res.sendfile()` * Fixed; using _qs_ module instead of _querystring_ * Fixed; strip unsafe chars from jsonp callbacks * Removed "stream threshold" setting 1.0.8 / 2011-03-01 ================== * Allow `req.query` to be pre-defined (via middleware or other parent app) * "connect": ">= 0.5.0 < 1.0.0". Closes #547 * Removed the long deprecated __EXPRESS_ENV__ support 1.0.7 / 2011-02-07 ================== * Fixed `render()` setting inheritance. Mounted apps would not inherit "view engine" 1.0.6 / 2011-02-07 ================== * Fixed `view engine` setting bug when period is in dirname 1.0.5 / 2011-02-05 ================== * Added secret to generated app `session()` call 1.0.4 / 2011-02-05 ================== * Added `qs` dependency to _package.json_ * Fixed namespaced `require()`s for latest connect support 1.0.3 / 2011-01-13 ================== * Remove unsafe characters from JSONP callback names [Ryan Grove] 1.0.2 / 2011-01-10 ================== * Removed nested require, using `connect.router` 1.0.1 / 2010-12-29 ================== * Fixed for middleware stacked via `createServer()` previously the `foo` middleware passed to `createServer(foo)` would not have access to Express methods such as `res.send()` or props like `req.query` etc. 1.0.0 / 2010-11-16 ================== * Added; deduce partial object names from the last segment. For example by default `partial('forum/post', postObject)` will give you the _post_ object, providing a meaningful default. * Added http status code string representation to `res.redirect()` body * Added; `res.redirect()` supporting _text/plain_ and _text/html_ via __Accept__. * Added `req.is()` to aid in content negotiation * Added partial local inheritance [suggested by masylum]. Closes #102 providing access to parent template locals. * Added _-s, --session[s]_ flag to express(1) to add session related middleware * Added _--template_ flag to express(1) to specify the template engine to use. * Added _--css_ flag to express(1) to specify the stylesheet engine to use (or just plain css by default). * Added `app.all()` support [thanks aheckmann] * Added partial direct object support. You may now `partial('user', user)` providing the "user" local, vs previously `partial('user', { object: user })`. * Added _route-separation_ example since many people question ways to do this with CommonJS modules. Also view the _blog_ example for an alternative. * Performance; caching view path derived partial object names * Fixed partial local inheritance precedence. [reported by Nick Poulden] Closes #454 * Fixed jsonp support; _text/javascript_ as per mailinglist discussion 1.0.0rc4 / 2010-10-14 ================== * Added _NODE_ENV_ support, _EXPRESS_ENV_ is deprecated and will be removed in 1.0.0 * Added route-middleware support (very helpful, see the [docs](http://expressjs.com/guide.html#Route-Middleware)) * Added _jsonp callback_ setting to enable/disable jsonp autowrapping [Dav Glass] * Added callback query check on response.send to autowrap JSON objects for simple webservice implementations [Dav Glass] * Added `partial()` support for array-like collections. Closes #434 * Added support for swappable querystring parsers * Added session usage docs. Closes #443 * Added dynamic helper caching. Closes #439 [suggested by maritz] * Added authentication example * Added basic Range support to `res.sendfile()` (and `res.download()` etc) * Changed; `express(1)` generated app using 2 spaces instead of 4 * Default env to "development" again [aheckmann] * Removed _context_ option is no more, use "scope" * Fixed; exposing _./support_ libs to examples so they can run without installs * Fixed mvc example 1.0.0rc3 / 2010-09-20 ================== * Added confirmation for `express(1)` app generation. Closes #391 * Added extending of flash formatters via `app.flashFormatters` * Added flash formatter support. Closes #411 * Added streaming support to `res.sendfile()` using `sys.pump()` when >= "stream threshold" * Added _stream threshold_ setting for `res.sendfile()` * Added `res.send()` __HEAD__ support * Added `res.clearCookie()` * Added `res.cookie()` * Added `res.render()` headers option * Added `res.redirect()` response bodies * Added `res.render()` status option support. Closes #425 [thanks aheckmann] * Fixed `res.sendfile()` responding with 403 on malicious path * Fixed `res.download()` bug; when an error occurs remove _Content-Disposition_ * Fixed; mounted apps settings now inherit from parent app [aheckmann] * Fixed; stripping Content-Length / Content-Type when 204 * Fixed `res.send()` 204. Closes #419 * Fixed multiple _Set-Cookie_ headers via `res.header()`. Closes #402 * Fixed bug messing with error handlers when `listenFD()` is called instead of `listen()`. [thanks guillermo] 1.0.0rc2 / 2010-08-17 ================== * Added `app.register()` for template engine mapping. Closes #390 * Added `res.render()` callback support as second argument (no options) * Added callback support to `res.download()` * Added callback support for `res.sendfile()` * Added support for middleware access via `express.middlewareName()` vs `connect.middlewareName()` * Added "partials" setting to docs * Added default expresso tests to `express(1)` generated app. Closes #384 * Fixed `res.sendfile()` error handling, defer via `next()` * Fixed `res.render()` callback when a layout is used [thanks guillermo] * Fixed; `make install` creating ~/.node_libraries when not present * Fixed issue preventing error handlers from being defined anywhere. Closes #387 1.0.0rc / 2010-07-28 ================== * Added mounted hook. Closes #369 * Added connect dependency to _package.json_ * Removed "reload views" setting and support code development env never caches, production always caches. * Removed _param_ in route callbacks, signature is now simply (req, res, next), previously (req, res, params, next). Use _req.params_ for path captures, _req.query_ for GET params. * Fixed "home" setting * Fixed middleware/router precedence issue. Closes #366 * Fixed; _configure()_ callbacks called immediately. Closes #368 1.0.0beta2 / 2010-07-23 ================== * Added more examples * Added; exporting `Server` constructor * Added `Server#helpers()` for view locals * Added `Server#dynamicHelpers()` for dynamic view locals. Closes #349 * Added support for absolute view paths * Added; _home_ setting defaults to `Server#route` for mounted apps. Closes #363 * Added Guillermo Rauch to the contributor list * Added support for "as" for non-collection partials. Closes #341 * Fixed _install.sh_, ensuring _~/.node_libraries_ exists. Closes #362 [thanks jf] * Fixed `res.render()` exceptions, now passed to `next()` when no callback is given [thanks guillermo] * Fixed instanceof `Array` checks, now `Array.isArray()` * Fixed express(1) expansion of public dirs. Closes #348 * Fixed middleware precedence. Closes #345 * Fixed view watcher, now async [thanks aheckmann] 1.0.0beta / 2010-07-15 ================== * Re-write - much faster - much lighter - Check [ExpressJS.com](http://expressjs.com) for migration guide and updated docs 0.14.0 / 2010-06-15 ================== * Utilize relative requires * Added Static bufferSize option [aheckmann] * Fixed caching of view and partial subdirectories [aheckmann] * Fixed mime.type() comments now that ".ext" is not supported * Updated haml submodule * Updated class submodule * Removed bin/express 0.13.0 / 2010-06-01 ================== * Added node v0.1.97 compatibility * Added support for deleting cookies via Request#cookie('key', null) * Updated haml submodule * Fixed not-found page, now using using charset utf-8 * Fixed show-exceptions page, now using using charset utf-8 * Fixed view support due to fs.readFile Buffers * Changed; mime.type() no longer accepts ".type" due to node extname() changes 0.12.0 / 2010-05-22 ================== * Added node v0.1.96 compatibility * Added view `helpers` export which act as additional local variables * Updated haml submodule * Changed ETag; removed inode, modified time only * Fixed LF to CRLF for setting multiple cookies * Fixed cookie compilation; values are now urlencoded * Fixed cookies parsing; accepts quoted values and url escaped cookies 0.11.0 / 2010-05-06 ================== * Added support for layouts using different engines - this.render('page.html.haml', { layout: 'super-cool-layout.html.ejs' }) - this.render('page.html.haml', { layout: 'foo' }) // assumes 'foo.html.haml' - this.render('page.html.haml', { layout: false }) // no layout * Updated ext submodule * Updated haml submodule * Fixed EJS partial support by passing along the context. Issue #307 0.10.1 / 2010-05-03 ================== * Fixed binary uploads. 0.10.0 / 2010-04-30 ================== * Added charset support via Request#charset (automatically assigned to 'UTF-8' when respond()'s encoding is set to 'utf8' or 'utf-8'. * Added "encoding" option to Request#render(). Closes #299 * Added "dump exceptions" setting, which is enabled by default. * Added simple ejs template engine support * Added error response support for text/plain, application/json. Closes #297 * Added callback function param to Request#error() * Added Request#sendHead() * Added Request#stream() * Added support for Request#respond(304, null) for empty response bodies * Added ETag support to Request#sendfile() * Added options to Request#sendfile(), passed to fs.createReadStream() * Added filename arg to Request#download() * Performance enhanced due to pre-reversing plugins so that plugins.reverse() is not called on each request * Performance enhanced by preventing several calls to toLowerCase() in Router#match() * Changed; Request#sendfile() now streams * Changed; Renamed Request#halt() to Request#respond(). Closes #289 * Changed; Using sys.inspect() instead of JSON.encode() for error output * Changed; run() returns the http.Server instance. Closes #298 * Changed; Defaulting Server#host to null (INADDR_ANY) * Changed; Logger "common" format scale of 0.4f * Removed Logger "request" format * Fixed; Catching ENOENT in view caching, preventing error when "views/partials" is not found * Fixed several issues with http client * Fixed Logger Content-Length output * Fixed bug preventing Opera from retaining the generated session id. Closes #292 0.9.0 / 2010-04-14 ================== * Added DSL level error() route support * Added DSL level notFound() route support * Added Request#error() * Added Request#notFound() * Added Request#render() callback function. Closes #258 * Added "max upload size" setting * Added "magic" variables to collection partials (\_\_index\_\_, \_\_length\_\_, \_\_isFirst\_\_, \_\_isLast\_\_). Closes #254 * Added [haml.js](http://github.com/visionmedia/haml.js) submodule; removed haml-js * Added callback function support to Request#halt() as 3rd/4th arg * Added preprocessing of route param wildcards using param(). Closes #251 * Added view partial support (with collections etc) * Fixed bug preventing falsey params (such as ?page=0). Closes #286 * Fixed setting of multiple cookies. Closes #199 * Changed; view naming convention is now NAME.TYPE.ENGINE (for example page.html.haml) * Changed; session cookie is now httpOnly * Changed; Request is no longer global * Changed; Event is no longer global * Changed; "sys" module is no longer global * Changed; moved Request#download to Static plugin where it belongs * Changed; Request instance created before body parsing. Closes #262 * Changed; Pre-caching views in memory when "cache view contents" is enabled. Closes #253 * Changed; Pre-caching view partials in memory when "cache view partials" is enabled * Updated support to node --version 0.1.90 * Updated dependencies * Removed set("session cookie") in favour of use(Session, { cookie: { ... }}) * Removed utils.mixin(); use Object#mergeDeep() 0.8.0 / 2010-03-19 ================== * Added coffeescript example app. Closes #242 * Changed; cache api now async friendly. Closes #240 * Removed deprecated 'express/static' support. Use 'express/plugins/static' 0.7.6 / 2010-03-19 ================== * Added Request#isXHR. Closes #229 * Added `make install` (for the executable) * Added `express` executable for setting up simple app templates * Added "GET /public/*" to Static plugin, defaulting to <root>/public * Added Static plugin * Fixed; Request#render() only calls cache.get() once * Fixed; Namespacing View caches with "view:" * Fixed; Namespacing Static caches with "static:" * Fixed; Both example apps now use the Static plugin * Fixed set("views"). Closes #239 * Fixed missing space for combined log format * Deprecated Request#sendfile() and 'express/static' * Removed Server#running 0.7.5 / 2010-03-16 ================== * Added Request#flash() support without args, now returns all flashes * Updated ext submodule 0.7.4 / 2010-03-16 ================== * Fixed session reaper * Changed; class.js replacing js-oo Class implementation (quite a bit faster, no browser cruft) 0.7.3 / 2010-03-16 ================== * Added package.json * Fixed requiring of haml / sass due to kiwi removal 0.7.2 / 2010-03-16 ================== * Fixed GIT submodules (HAH!) 0.7.1 / 2010-03-16 ================== * Changed; Express now using submodules again until a PM is adopted * Changed; chat example using millisecond conversions from ext 0.7.0 / 2010-03-15 ================== * Added Request#pass() support (finds the next matching route, or the given path) * Added Logger plugin (default "common" format replaces CommonLogger) * Removed Profiler plugin * Removed CommonLogger plugin 0.6.0 / 2010-03-11 ================== * Added seed.yml for kiwi package management support * Added HTTP client query string support when method is GET. Closes #205 * Added support for arbitrary view engines. For example "foo.engine.html" will now require('engine'), the exports from this module are cached after the first require(). * Added async plugin support * Removed usage of RESTful route funcs as http client get() etc, use http.get() and friends * Removed custom exceptions 0.5.0 / 2010-03-10 ================== * Added ext dependency (library of js extensions) * Removed extname() / basename() utils. Use path module * Removed toArray() util. Use arguments.values * Removed escapeRegexp() util. Use RegExp.escape() * Removed process.mixin() dependency. Use utils.mixin() * Removed Collection * Removed ElementCollection * Shameless self promotion of ebook "Advanced JavaScript" (http://dev-mag.com) ;) 0.4.0 / 2010-02-11 ================== * Added flash() example to sample upload app * Added high level restful http client module (express/http) * Changed; RESTful route functions double as HTTP clients. Closes #69 * Changed; throwing error when routes are added at runtime * Changed; defaulting render() context to the current Request. Closes #197 * Updated haml submodule 0.3.0 / 2010-02-11 ================== * Updated haml / sass submodules. Closes #200 * Added flash message support. Closes #64 * Added accepts() now allows multiple args. fixes #117 * Added support for plugins to halt. Closes #189 * Added alternate layout support. Closes #119 * Removed Route#run(). Closes #188 * Fixed broken specs due to use(Cookie) missing 0.2.1 / 2010-02-05 ================== * Added "plot" format option for Profiler (for gnuplot processing) * Added request number to Profiler plugin * Fixed binary encoding for multipart file uploads, was previously defaulting to UTF8 * Fixed issue with routes not firing when not files are present. Closes #184 * Fixed process.Promise -> events.Promise 0.2.0 / 2010-02-03 ================== * Added parseParam() support for name[] etc. (allows for file inputs with "multiple" attr) Closes #180 * Added Both Cache and Session option "reapInterval" may be "reapEvery". Closes #174 * Added expiration support to cache api with reaper. Closes #133 * Added cache Store.Memory#reap() * Added Cache; cache api now uses first class Cache instances * Added abstract session Store. Closes #172 * Changed; cache Memory.Store#get() utilizing Collection * Renamed MemoryStore -> Store.Memory * Fixed use() of the same plugin several time will always use latest options. Closes #176 0.1.0 / 2010-02-03 ================== * Changed; Hooks (before / after) pass request as arg as well as evaluated in their context * Updated node support to 0.1.27 Closes #169 * Updated dirname(__filename) -> __dirname * Updated libxmljs support to v0.2.0 * Added session support with memory store / reaping * Added quick uid() helper * Added multi-part upload support * Added Sass.js support / submodule * Added production env caching view contents and static files * Added static file caching. Closes #136 * Added cache plugin with memory stores * Added support to StaticFile so that it works with non-textual files. * Removed dirname() helper * Removed several globals (now their modules must be required) 0.0.2 / 2010-01-10 ================== * Added view benchmarks; currently haml vs ejs * Added Request#attachment() specs. Closes #116 * Added use of node's parseQuery() util. Closes #123 * Added `make init` for submodules * Updated Haml * Updated sample chat app to show messages on load * Updated libxmljs parseString -> parseHtmlString * Fixed `make init` to work with older versions of git * Fixed specs can now run independent specs for those who can't build deps. Closes #127 * Fixed issues introduced by the node url module changes. Closes 126. * Fixed two assertions failing due to Collection#keys() returning strings * Fixed faulty Collection#toArray() spec due to keys() returning strings * Fixed `make test` now builds libxmljs.node before testing 0.0.1 / 2010-01-03 ================== * Initial release apollo-server-demo/node_modules/express/package.json 0000644 0001750 0000144 00000005675 03560116604 022424 0 ustar andreh users { "name": "express", "description": "Fast, unopinionated, minimalist web framework", "version": "4.17.1", "author": "TJ Holowaychuk <tj@vision-media.ca>", "contributors": [ "Aaron Heckmann <aaron.heckmann+github@gmail.com>", "Ciaran Jessup <ciaranj@gmail.com>", "Douglas Christopher Wilson <doug@somethingdoug.com>", "Guillermo Rauch <rauchg@gmail.com>", "Jonathan Ong <me@jongleberry.com>", "Roman Shtylman <shtylman+expressjs@gmail.com>", "Young Jae Sim <hanul@hanul.me>" ], "license": "MIT", "repository": "expressjs/express", "homepage": "http://expressjs.com/", "keywords": [ "express", "framework", "sinatra", "web", "rest", "restful", "router", "app", "api" ], "dependencies": { "accepts": "~1.3.7", "array-flatten": "1.1.1", "body-parser": "1.19.0", "content-disposition": "0.5.3", "content-type": "~1.0.4", "cookie": "0.4.0", "cookie-signature": "1.0.6", "debug": "2.6.9", "depd": "~1.1.2", "encodeurl": "~1.0.2", "escape-html": "~1.0.3", "etag": "~1.8.1", "finalhandler": "~1.1.2", "fresh": "0.5.2", "merge-descriptors": "1.0.1", "methods": "~1.1.2", "on-finished": "~2.3.0", "parseurl": "~1.3.3", "path-to-regexp": "0.1.7", "proxy-addr": "~2.0.5", "qs": "6.7.0", "range-parser": "~1.2.1", "safe-buffer": "5.1.2", "send": "0.17.1", "serve-static": "1.14.1", "setprototypeof": "1.1.1", "statuses": "~1.5.0", "type-is": "~1.6.18", "utils-merge": "1.0.1", "vary": "~1.1.2" }, "devDependencies": { "after": "0.8.2", "connect-redis": "3.4.1", "cookie-parser": "~1.4.4", "cookie-session": "1.3.3", "ejs": "2.6.1", "eslint": "2.13.1", "express-session": "1.16.1", "hbs": "4.0.4", "istanbul": "0.4.5", "marked": "0.6.2", "method-override": "3.0.0", "mocha": "5.2.0", "morgan": "1.9.1", "multiparty": "4.2.1", "pbkdf2-password": "1.2.1", "should": "13.2.3", "supertest": "3.3.0", "vhost": "~3.0.2" }, "engines": { "node": ">= 0.10.0" }, "files": [ "LICENSE", "History.md", "Readme.md", "index.js", "lib/" ], "scripts": { "lint": "eslint .", "test": "mocha --require test/support/env --reporter spec --bail --check-leaks test/ test/acceptance/", "test-ci": "istanbul cover node_modules/mocha/bin/_mocha --report lcovonly -- --require test/support/env --reporter spec --check-leaks test/ test/acceptance/", "test-cov": "istanbul cover node_modules/mocha/bin/_mocha -- --require test/support/env --reporter dot --check-leaks test/ test/acceptance/", "test-tap": "mocha --require test/support/env --reporter tap --check-leaks test/ test/acceptance/" } ,"_resolved": "https://registry.npmjs.org/express/-/express-4.17.1.tgz" ,"_integrity": "sha512-mHJ9O79RqluphRrcw2X/GTh3k9tVv8YcoyY4Kkh4WDMUYKRZUq0h1o0w2rrrxBqM7VoeUVqgb27xlEMXTnYt4g==" ,"_from": "express@4.17.1" } apollo-server-demo/node_modules/express/lib/ 0000755 0001750 0000144 00000000000 14067647700 020701 5 ustar andreh users apollo-server-demo/node_modules/express/lib/router/ 0000755 0001750 0000144 00000000000 14067647700 022221 5 ustar andreh users apollo-server-demo/node_modules/express/lib/router/index.js 0000644 0001750 0000144 00000035043 03560116604 023661 0 ustar andreh users /*! * express * Copyright(c) 2009-2013 TJ Holowaychuk * Copyright(c) 2013 Roman Shtylman * Copyright(c) 2014-2015 Douglas Christopher Wilson * MIT Licensed */ 'use strict'; /** * Module dependencies. * @private */ var Route = require('./route'); var Layer = require('./layer'); var methods = require('methods'); var mixin = require('utils-merge'); var debug = require('debug')('express:router'); var deprecate = require('depd')('express'); var flatten = require('array-flatten'); var parseUrl = require('parseurl'); var setPrototypeOf = require('setprototypeof') /** * Module variables. * @private */ var objectRegExp = /^\[object (\S+)\]$/; var slice = Array.prototype.slice; var toString = Object.prototype.toString; /** * Initialize a new `Router` with the given `options`. * * @param {Object} [options] * @return {Router} which is an callable function * @public */ var proto = module.exports = function(options) { var opts = options || {}; function router(req, res, next) { router.handle(req, res, next); } // mixin Router class functions setPrototypeOf(router, proto) router.params = {}; router._params = []; router.caseSensitive = opts.caseSensitive; router.mergeParams = opts.mergeParams; router.strict = opts.strict; router.stack = []; return router; }; /** * Map the given param placeholder `name`(s) to the given callback. * * Parameter mapping is used to provide pre-conditions to routes * which use normalized placeholders. For example a _:user_id_ parameter * could automatically load a user's information from the database without * any additional code, * * The callback uses the same signature as middleware, the only difference * being that the value of the placeholder is passed, in this case the _id_ * of the user. Once the `next()` function is invoked, just like middleware * it will continue on to execute the route, or subsequent parameter functions. * * Just like in middleware, you must either respond to the request or call next * to avoid stalling the request. * * app.param('user_id', function(req, res, next, id){ * User.find(id, function(err, user){ * if (err) { * return next(err); * } else if (!user) { * return next(new Error('failed to load user')); * } * req.user = user; * next(); * }); * }); * * @param {String} name * @param {Function} fn * @return {app} for chaining * @public */ proto.param = function param(name, fn) { // param logic if (typeof name === 'function') { deprecate('router.param(fn): Refactor to use path params'); this._params.push(name); return; } // apply param functions var params = this._params; var len = params.length; var ret; if (name[0] === ':') { deprecate('router.param(' + JSON.stringify(name) + ', fn): Use router.param(' + JSON.stringify(name.substr(1)) + ', fn) instead'); name = name.substr(1); } for (var i = 0; i < len; ++i) { if (ret = params[i](name, fn)) { fn = ret; } } // ensure we end up with a // middleware function if ('function' !== typeof fn) { throw new Error('invalid param() call for ' + name + ', got ' + fn); } (this.params[name] = this.params[name] || []).push(fn); return this; }; /** * Dispatch a req, res into the router. * @private */ proto.handle = function handle(req, res, out) { var self = this; debug('dispatching %s %s', req.method, req.url); var idx = 0; var protohost = getProtohost(req.url) || '' var removed = ''; var slashAdded = false; var paramcalled = {}; // store options for OPTIONS request // only used if OPTIONS request var options = []; // middleware and routes var stack = self.stack; // manage inter-router variables var parentParams = req.params; var parentUrl = req.baseUrl || ''; var done = restore(out, req, 'baseUrl', 'next', 'params'); // setup next layer req.next = next; // for options requests, respond with a default if nothing else responds if (req.method === 'OPTIONS') { done = wrap(done, function(old, err) { if (err || options.length === 0) return old(err); sendOptionsResponse(res, options, old); }); } // setup basic req values req.baseUrl = parentUrl; req.originalUrl = req.originalUrl || req.url; next(); function next(err) { var layerError = err === 'route' ? null : err; // remove added slash if (slashAdded) { req.url = req.url.substr(1); slashAdded = false; } // restore altered req.url if (removed.length !== 0) { req.baseUrl = parentUrl; req.url = protohost + removed + req.url.substr(protohost.length); removed = ''; } // signal to exit router if (layerError === 'router') { setImmediate(done, null) return } // no more matching layers if (idx >= stack.length) { setImmediate(done, layerError); return; } // get pathname of request var path = getPathname(req); if (path == null) { return done(layerError); } // find next matching layer var layer; var match; var route; while (match !== true && idx < stack.length) { layer = stack[idx++]; match = matchLayer(layer, path); route = layer.route; if (typeof match !== 'boolean') { // hold on to layerError layerError = layerError || match; } if (match !== true) { continue; } if (!route) { // process non-route handlers normally continue; } if (layerError) { // routes do not match with a pending error match = false; continue; } var method = req.method; var has_method = route._handles_method(method); // build up automatic options response if (!has_method && method === 'OPTIONS') { appendMethods(options, route._options()); } // don't even bother matching route if (!has_method && method !== 'HEAD') { match = false; continue; } } // no match if (match !== true) { return done(layerError); } // store route for dispatch on change if (route) { req.route = route; } // Capture one-time layer values req.params = self.mergeParams ? mergeParams(layer.params, parentParams) : layer.params; var layerPath = layer.path; // this should be done for the layer self.process_params(layer, paramcalled, req, res, function (err) { if (err) { return next(layerError || err); } if (route) { return layer.handle_request(req, res, next); } trim_prefix(layer, layerError, layerPath, path); }); } function trim_prefix(layer, layerError, layerPath, path) { if (layerPath.length !== 0) { // Validate path breaks on a path separator var c = path[layerPath.length] if (c && c !== '/' && c !== '.') return next(layerError) // Trim off the part of the url that matches the route // middleware (.use stuff) needs to have the path stripped debug('trim prefix (%s) from url %s', layerPath, req.url); removed = layerPath; req.url = protohost + req.url.substr(protohost.length + removed.length); // Ensure leading slash if (!protohost && req.url[0] !== '/') { req.url = '/' + req.url; slashAdded = true; } // Setup base URL (no trailing slash) req.baseUrl = parentUrl + (removed[removed.length - 1] === '/' ? removed.substring(0, removed.length - 1) : removed); } debug('%s %s : %s', layer.name, layerPath, req.originalUrl); if (layerError) { layer.handle_error(layerError, req, res, next); } else { layer.handle_request(req, res, next); } } }; /** * Process any parameters for the layer. * @private */ proto.process_params = function process_params(layer, called, req, res, done) { var params = this.params; // captured parameters from the layer, keys and values var keys = layer.keys; // fast track if (!keys || keys.length === 0) { return done(); } var i = 0; var name; var paramIndex = 0; var key; var paramVal; var paramCallbacks; var paramCalled; // process params in order // param callbacks can be async function param(err) { if (err) { return done(err); } if (i >= keys.length ) { return done(); } paramIndex = 0; key = keys[i++]; name = key.name; paramVal = req.params[name]; paramCallbacks = params[name]; paramCalled = called[name]; if (paramVal === undefined || !paramCallbacks) { return param(); } // param previously called with same value or error occurred if (paramCalled && (paramCalled.match === paramVal || (paramCalled.error && paramCalled.error !== 'route'))) { // restore value req.params[name] = paramCalled.value; // next param return param(paramCalled.error); } called[name] = paramCalled = { error: null, match: paramVal, value: paramVal }; paramCallback(); } // single param callbacks function paramCallback(err) { var fn = paramCallbacks[paramIndex++]; // store updated value paramCalled.value = req.params[key.name]; if (err) { // store error paramCalled.error = err; param(err); return; } if (!fn) return param(); try { fn(req, res, paramCallback, paramVal, key.name); } catch (e) { paramCallback(e); } } param(); }; /** * Use the given middleware function, with optional path, defaulting to "/". * * Use (like `.all`) will run for any http METHOD, but it will not add * handlers for those methods so OPTIONS requests will not consider `.use` * functions even if they could respond. * * The other difference is that _route_ path is stripped and not visible * to the handler function. The main effect of this feature is that mounted * handlers can operate without any code changes regardless of the "prefix" * pathname. * * @public */ proto.use = function use(fn) { var offset = 0; var path = '/'; // default path to '/' // disambiguate router.use([fn]) if (typeof fn !== 'function') { var arg = fn; while (Array.isArray(arg) && arg.length !== 0) { arg = arg[0]; } // first arg is the path if (typeof arg !== 'function') { offset = 1; path = fn; } } var callbacks = flatten(slice.call(arguments, offset)); if (callbacks.length === 0) { throw new TypeError('Router.use() requires a middleware function') } for (var i = 0; i < callbacks.length; i++) { var fn = callbacks[i]; if (typeof fn !== 'function') { throw new TypeError('Router.use() requires a middleware function but got a ' + gettype(fn)) } // add the middleware debug('use %o %s', path, fn.name || '<anonymous>') var layer = new Layer(path, { sensitive: this.caseSensitive, strict: false, end: false }, fn); layer.route = undefined; this.stack.push(layer); } return this; }; /** * Create a new Route for the given path. * * Each route contains a separate middleware stack and VERB handlers. * * See the Route api documentation for details on adding handlers * and middleware to routes. * * @param {String} path * @return {Route} * @public */ proto.route = function route(path) { var route = new Route(path); var layer = new Layer(path, { sensitive: this.caseSensitive, strict: this.strict, end: true }, route.dispatch.bind(route)); layer.route = route; this.stack.push(layer); return route; }; // create Router#VERB functions methods.concat('all').forEach(function(method){ proto[method] = function(path){ var route = this.route(path) route[method].apply(route, slice.call(arguments, 1)); return this; }; }); // append methods to a list of methods function appendMethods(list, addition) { for (var i = 0; i < addition.length; i++) { var method = addition[i]; if (list.indexOf(method) === -1) { list.push(method); } } } // get pathname of request function getPathname(req) { try { return parseUrl(req).pathname; } catch (err) { return undefined; } } // Get get protocol + host for a URL function getProtohost(url) { if (typeof url !== 'string' || url.length === 0 || url[0] === '/') { return undefined } var searchIndex = url.indexOf('?') var pathLength = searchIndex !== -1 ? searchIndex : url.length var fqdnIndex = url.substr(0, pathLength).indexOf('://') return fqdnIndex !== -1 ? url.substr(0, url.indexOf('/', 3 + fqdnIndex)) : undefined } // get type for error message function gettype(obj) { var type = typeof obj; if (type !== 'object') { return type; } // inspect [[Class]] for objects return toString.call(obj) .replace(objectRegExp, '$1'); } /** * Match path to a layer. * * @param {Layer} layer * @param {string} path * @private */ function matchLayer(layer, path) { try { return layer.match(path); } catch (err) { return err; } } // merge params with parent params function mergeParams(params, parent) { if (typeof parent !== 'object' || !parent) { return params; } // make copy of parent for base var obj = mixin({}, parent); // simple non-numeric merging if (!(0 in params) || !(0 in parent)) { return mixin(obj, params); } var i = 0; var o = 0; // determine numeric gaps while (i in params) { i++; } while (o in parent) { o++; } // offset numeric indices in params before merge for (i--; i >= 0; i--) { params[i + o] = params[i]; // create holes for the merge when necessary if (i < o) { delete params[i]; } } return mixin(obj, params); } // restore obj props after function function restore(fn, obj) { var props = new Array(arguments.length - 2); var vals = new Array(arguments.length - 2); for (var i = 0; i < props.length; i++) { props[i] = arguments[i + 2]; vals[i] = obj[props[i]]; } return function () { // restore vals for (var i = 0; i < props.length; i++) { obj[props[i]] = vals[i]; } return fn.apply(this, arguments); }; } // send an OPTIONS response function sendOptionsResponse(res, options, next) { try { var body = options.join(','); res.set('Allow', body); res.send(body); } catch (err) { next(err); } } // wrap a function function wrap(old, fn) { return function proxy() { var args = new Array(arguments.length + 1); args[0] = old; for (var i = 0, len = arguments.length; i < len; i++) { args[i + 1] = arguments[i]; } fn.apply(this, args); }; } apollo-server-demo/node_modules/express/lib/router/layer.js 0000644 0001750 0000144 00000006340 03560116604 023664 0 ustar andreh users /*! * express * Copyright(c) 2009-2013 TJ Holowaychuk * Copyright(c) 2013 Roman Shtylman * Copyright(c) 2014-2015 Douglas Christopher Wilson * MIT Licensed */ 'use strict'; /** * Module dependencies. * @private */ var pathRegexp = require('path-to-regexp'); var debug = require('debug')('express:router:layer'); /** * Module variables. * @private */ var hasOwnProperty = Object.prototype.hasOwnProperty; /** * Module exports. * @public */ module.exports = Layer; function Layer(path, options, fn) { if (!(this instanceof Layer)) { return new Layer(path, options, fn); } debug('new %o', path) var opts = options || {}; this.handle = fn; this.name = fn.name || '<anonymous>'; this.params = undefined; this.path = undefined; this.regexp = pathRegexp(path, this.keys = [], opts); // set fast path flags this.regexp.fast_star = path === '*' this.regexp.fast_slash = path === '/' && opts.end === false } /** * Handle the error for the layer. * * @param {Error} error * @param {Request} req * @param {Response} res * @param {function} next * @api private */ Layer.prototype.handle_error = function handle_error(error, req, res, next) { var fn = this.handle; if (fn.length !== 4) { // not a standard error handler return next(error); } try { fn(error, req, res, next); } catch (err) { next(err); } }; /** * Handle the request for the layer. * * @param {Request} req * @param {Response} res * @param {function} next * @api private */ Layer.prototype.handle_request = function handle(req, res, next) { var fn = this.handle; if (fn.length > 3) { // not a standard request handler return next(); } try { fn(req, res, next); } catch (err) { next(err); } }; /** * Check if this route matches `path`, if so * populate `.params`. * * @param {String} path * @return {Boolean} * @api private */ Layer.prototype.match = function match(path) { var match if (path != null) { // fast path non-ending match for / (any path matches) if (this.regexp.fast_slash) { this.params = {} this.path = '' return true } // fast path for * (everything matched in a param) if (this.regexp.fast_star) { this.params = {'0': decode_param(path)} this.path = path return true } // match the path match = this.regexp.exec(path) } if (!match) { this.params = undefined; this.path = undefined; return false; } // store values this.params = {}; this.path = match[0] var keys = this.keys; var params = this.params; for (var i = 1; i < match.length; i++) { var key = keys[i - 1]; var prop = key.name; var val = decode_param(match[i]) if (val !== undefined || !(hasOwnProperty.call(params, prop))) { params[prop] = val; } } return true; }; /** * Decode param value. * * @param {string} val * @return {string} * @private */ function decode_param(val) { if (typeof val !== 'string' || val.length === 0) { return val; } try { return decodeURIComponent(val); } catch (err) { if (err instanceof URIError) { err.message = 'Failed to decode param \'' + val + '\''; err.status = err.statusCode = 400; } throw err; } } apollo-server-demo/node_modules/express/lib/router/route.js 0000644 0001750 0000144 00000010065 03560116604 023705 0 ustar andreh users /*! * express * Copyright(c) 2009-2013 TJ Holowaychuk * Copyright(c) 2013 Roman Shtylman * Copyright(c) 2014-2015 Douglas Christopher Wilson * MIT Licensed */ 'use strict'; /** * Module dependencies. * @private */ var debug = require('debug')('express:router:route'); var flatten = require('array-flatten'); var Layer = require('./layer'); var methods = require('methods'); /** * Module variables. * @private */ var slice = Array.prototype.slice; var toString = Object.prototype.toString; /** * Module exports. * @public */ module.exports = Route; /** * Initialize `Route` with the given `path`, * * @param {String} path * @public */ function Route(path) { this.path = path; this.stack = []; debug('new %o', path) // route handlers for various http methods this.methods = {}; } /** * Determine if the route handles a given method. * @private */ Route.prototype._handles_method = function _handles_method(method) { if (this.methods._all) { return true; } var name = method.toLowerCase(); if (name === 'head' && !this.methods['head']) { name = 'get'; } return Boolean(this.methods[name]); }; /** * @return {Array} supported HTTP methods * @private */ Route.prototype._options = function _options() { var methods = Object.keys(this.methods); // append automatic head if (this.methods.get && !this.methods.head) { methods.push('head'); } for (var i = 0; i < methods.length; i++) { // make upper case methods[i] = methods[i].toUpperCase(); } return methods; }; /** * dispatch req, res into this route * @private */ Route.prototype.dispatch = function dispatch(req, res, done) { var idx = 0; var stack = this.stack; if (stack.length === 0) { return done(); } var method = req.method.toLowerCase(); if (method === 'head' && !this.methods['head']) { method = 'get'; } req.route = this; next(); function next(err) { // signal to exit route if (err && err === 'route') { return done(); } // signal to exit router if (err && err === 'router') { return done(err) } var layer = stack[idx++]; if (!layer) { return done(err); } if (layer.method && layer.method !== method) { return next(err); } if (err) { layer.handle_error(err, req, res, next); } else { layer.handle_request(req, res, next); } } }; /** * Add a handler for all HTTP verbs to this route. * * Behaves just like middleware and can respond or call `next` * to continue processing. * * You can use multiple `.all` call to add multiple handlers. * * function check_something(req, res, next){ * next(); * }; * * function validate_user(req, res, next){ * next(); * }; * * route * .all(validate_user) * .all(check_something) * .get(function(req, res, next){ * res.send('hello world'); * }); * * @param {function} handler * @return {Route} for chaining * @api public */ Route.prototype.all = function all() { var handles = flatten(slice.call(arguments)); for (var i = 0; i < handles.length; i++) { var handle = handles[i]; if (typeof handle !== 'function') { var type = toString.call(handle); var msg = 'Route.all() requires a callback function but got a ' + type throw new TypeError(msg); } var layer = Layer('/', {}, handle); layer.method = undefined; this.methods._all = true; this.stack.push(layer); } return this; }; methods.forEach(function(method){ Route.prototype[method] = function(){ var handles = flatten(slice.call(arguments)); for (var i = 0; i < handles.length; i++) { var handle = handles[i]; if (typeof handle !== 'function') { var type = toString.call(handle); var msg = 'Route.' + method + '() requires a callback function but got a ' + type throw new Error(msg); } debug('%s %o', method, this.path) var layer = Layer('/', {}, handle); layer.method = method; this.methods[method] = true; this.stack.push(layer); } return this; }; }); apollo-server-demo/node_modules/express/lib/middleware/ 0000755 0001750 0000144 00000000000 14067647700 023016 5 ustar andreh users apollo-server-demo/node_modules/express/lib/middleware/query.js 0000644 0001750 0000144 00000001565 03560116604 024516 0 ustar andreh users /*! * express * Copyright(c) 2009-2013 TJ Holowaychuk * Copyright(c) 2013 Roman Shtylman * Copyright(c) 2014-2015 Douglas Christopher Wilson * MIT Licensed */ 'use strict'; /** * Module dependencies. */ var merge = require('utils-merge') var parseUrl = require('parseurl'); var qs = require('qs'); /** * @param {Object} options * @return {Function} * @api public */ module.exports = function query(options) { var opts = merge({}, options) var queryparse = qs.parse; if (typeof options === 'function') { queryparse = options; opts = undefined; } if (opts !== undefined && opts.allowPrototypes === undefined) { // back-compat for qs module opts.allowPrototypes = true; } return function query(req, res, next){ if (!req.query) { var val = parseUrl(req).query; req.query = queryparse(val, opts); } next(); }; }; apollo-server-demo/node_modules/express/lib/middleware/init.js 0000644 0001750 0000144 00000001525 03560116604 024310 0 ustar andreh users /*! * express * Copyright(c) 2009-2013 TJ Holowaychuk * Copyright(c) 2013 Roman Shtylman * Copyright(c) 2014-2015 Douglas Christopher Wilson * MIT Licensed */ 'use strict'; /** * Module dependencies. * @private */ var setPrototypeOf = require('setprototypeof') /** * Initialization middleware, exposing the * request and response to each other, as well * as defaulting the X-Powered-By header field. * * @param {Function} app * @return {Function} * @api private */ exports.init = function(app){ return function expressInit(req, res, next){ if (app.enabled('x-powered-by')) res.setHeader('X-Powered-By', 'Express'); req.res = res; res.req = req; req.next = next; setPrototypeOf(req, app.request) setPrototypeOf(res, app.response) res.locals = res.locals || Object.create(null); next(); }; }; apollo-server-demo/node_modules/express/lib/application.js 0000644 0001750 0000144 00000033676 03560116604 023547 0 ustar andreh users /*! * express * Copyright(c) 2009-2013 TJ Holowaychuk * Copyright(c) 2013 Roman Shtylman * Copyright(c) 2014-2015 Douglas Christopher Wilson * MIT Licensed */ 'use strict'; /** * Module dependencies. * @private */ var finalhandler = require('finalhandler'); var Router = require('./router'); var methods = require('methods'); var middleware = require('./middleware/init'); var query = require('./middleware/query'); var debug = require('debug')('express:application'); var View = require('./view'); var http = require('http'); var compileETag = require('./utils').compileETag; var compileQueryParser = require('./utils').compileQueryParser; var compileTrust = require('./utils').compileTrust; var deprecate = require('depd')('express'); var flatten = require('array-flatten'); var merge = require('utils-merge'); var resolve = require('path').resolve; var setPrototypeOf = require('setprototypeof') var slice = Array.prototype.slice; /** * Application prototype. */ var app = exports = module.exports = {}; /** * Variable for trust proxy inheritance back-compat * @private */ var trustProxyDefaultSymbol = '@@symbol:trust_proxy_default'; /** * Initialize the server. * * - setup default configuration * - setup default middleware * - setup route reflection methods * * @private */ app.init = function init() { this.cache = {}; this.engines = {}; this.settings = {}; this.defaultConfiguration(); }; /** * Initialize application configuration. * @private */ app.defaultConfiguration = function defaultConfiguration() { var env = process.env.NODE_ENV || 'development'; // default settings this.enable('x-powered-by'); this.set('etag', 'weak'); this.set('env', env); this.set('query parser', 'extended'); this.set('subdomain offset', 2); this.set('trust proxy', false); // trust proxy inherit back-compat Object.defineProperty(this.settings, trustProxyDefaultSymbol, { configurable: true, value: true }); debug('booting in %s mode', env); this.on('mount', function onmount(parent) { // inherit trust proxy if (this.settings[trustProxyDefaultSymbol] === true && typeof parent.settings['trust proxy fn'] === 'function') { delete this.settings['trust proxy']; delete this.settings['trust proxy fn']; } // inherit protos setPrototypeOf(this.request, parent.request) setPrototypeOf(this.response, parent.response) setPrototypeOf(this.engines, parent.engines) setPrototypeOf(this.settings, parent.settings) }); // setup locals this.locals = Object.create(null); // top-most app is mounted at / this.mountpath = '/'; // default locals this.locals.settings = this.settings; // default configuration this.set('view', View); this.set('views', resolve('views')); this.set('jsonp callback name', 'callback'); if (env === 'production') { this.enable('view cache'); } Object.defineProperty(this, 'router', { get: function() { throw new Error('\'app.router\' is deprecated!\nPlease see the 3.x to 4.x migration guide for details on how to update your app.'); } }); }; /** * lazily adds the base router if it has not yet been added. * * We cannot add the base router in the defaultConfiguration because * it reads app settings which might be set after that has run. * * @private */ app.lazyrouter = function lazyrouter() { if (!this._router) { this._router = new Router({ caseSensitive: this.enabled('case sensitive routing'), strict: this.enabled('strict routing') }); this._router.use(query(this.get('query parser fn'))); this._router.use(middleware.init(this)); } }; /** * Dispatch a req, res pair into the application. Starts pipeline processing. * * If no callback is provided, then default error handlers will respond * in the event of an error bubbling through the stack. * * @private */ app.handle = function handle(req, res, callback) { var router = this._router; // final handler var done = callback || finalhandler(req, res, { env: this.get('env'), onerror: logerror.bind(this) }); // no routes if (!router) { debug('no routes defined on app'); done(); return; } router.handle(req, res, done); }; /** * Proxy `Router#use()` to add middleware to the app router. * See Router#use() documentation for details. * * If the _fn_ parameter is an express app, then it will be * mounted at the _route_ specified. * * @public */ app.use = function use(fn) { var offset = 0; var path = '/'; // default path to '/' // disambiguate app.use([fn]) if (typeof fn !== 'function') { var arg = fn; while (Array.isArray(arg) && arg.length !== 0) { arg = arg[0]; } // first arg is the path if (typeof arg !== 'function') { offset = 1; path = fn; } } var fns = flatten(slice.call(arguments, offset)); if (fns.length === 0) { throw new TypeError('app.use() requires a middleware function') } // setup router this.lazyrouter(); var router = this._router; fns.forEach(function (fn) { // non-express app if (!fn || !fn.handle || !fn.set) { return router.use(path, fn); } debug('.use app under %s', path); fn.mountpath = path; fn.parent = this; // restore .app property on req and res router.use(path, function mounted_app(req, res, next) { var orig = req.app; fn.handle(req, res, function (err) { setPrototypeOf(req, orig.request) setPrototypeOf(res, orig.response) next(err); }); }); // mounted an app fn.emit('mount', this); }, this); return this; }; /** * Proxy to the app `Router#route()` * Returns a new `Route` instance for the _path_. * * Routes are isolated middleware stacks for specific paths. * See the Route api docs for details. * * @public */ app.route = function route(path) { this.lazyrouter(); return this._router.route(path); }; /** * Register the given template engine callback `fn` * as `ext`. * * By default will `require()` the engine based on the * file extension. For example if you try to render * a "foo.ejs" file Express will invoke the following internally: * * app.engine('ejs', require('ejs').__express); * * For engines that do not provide `.__express` out of the box, * or if you wish to "map" a different extension to the template engine * you may use this method. For example mapping the EJS template engine to * ".html" files: * * app.engine('html', require('ejs').renderFile); * * In this case EJS provides a `.renderFile()` method with * the same signature that Express expects: `(path, options, callback)`, * though note that it aliases this method as `ejs.__express` internally * so if you're using ".ejs" extensions you dont need to do anything. * * Some template engines do not follow this convention, the * [Consolidate.js](https://github.com/tj/consolidate.js) * library was created to map all of node's popular template * engines to follow this convention, thus allowing them to * work seamlessly within Express. * * @param {String} ext * @param {Function} fn * @return {app} for chaining * @public */ app.engine = function engine(ext, fn) { if (typeof fn !== 'function') { throw new Error('callback function required'); } // get file extension var extension = ext[0] !== '.' ? '.' + ext : ext; // store engine this.engines[extension] = fn; return this; }; /** * Proxy to `Router#param()` with one added api feature. The _name_ parameter * can be an array of names. * * See the Router#param() docs for more details. * * @param {String|Array} name * @param {Function} fn * @return {app} for chaining * @public */ app.param = function param(name, fn) { this.lazyrouter(); if (Array.isArray(name)) { for (var i = 0; i < name.length; i++) { this.param(name[i], fn); } return this; } this._router.param(name, fn); return this; }; /** * Assign `setting` to `val`, or return `setting`'s value. * * app.set('foo', 'bar'); * app.set('foo'); * // => "bar" * * Mounted servers inherit their parent server's settings. * * @param {String} setting * @param {*} [val] * @return {Server} for chaining * @public */ app.set = function set(setting, val) { if (arguments.length === 1) { // app.get(setting) return this.settings[setting]; } debug('set "%s" to %o', setting, val); // set value this.settings[setting] = val; // trigger matched settings switch (setting) { case 'etag': this.set('etag fn', compileETag(val)); break; case 'query parser': this.set('query parser fn', compileQueryParser(val)); break; case 'trust proxy': this.set('trust proxy fn', compileTrust(val)); // trust proxy inherit back-compat Object.defineProperty(this.settings, trustProxyDefaultSymbol, { configurable: true, value: false }); break; } return this; }; /** * Return the app's absolute pathname * based on the parent(s) that have * mounted it. * * For example if the application was * mounted as "/admin", which itself * was mounted as "/blog" then the * return value would be "/blog/admin". * * @return {String} * @private */ app.path = function path() { return this.parent ? this.parent.path() + this.mountpath : ''; }; /** * Check if `setting` is enabled (truthy). * * app.enabled('foo') * // => false * * app.enable('foo') * app.enabled('foo') * // => true * * @param {String} setting * @return {Boolean} * @public */ app.enabled = function enabled(setting) { return Boolean(this.set(setting)); }; /** * Check if `setting` is disabled. * * app.disabled('foo') * // => true * * app.enable('foo') * app.disabled('foo') * // => false * * @param {String} setting * @return {Boolean} * @public */ app.disabled = function disabled(setting) { return !this.set(setting); }; /** * Enable `setting`. * * @param {String} setting * @return {app} for chaining * @public */ app.enable = function enable(setting) { return this.set(setting, true); }; /** * Disable `setting`. * * @param {String} setting * @return {app} for chaining * @public */ app.disable = function disable(setting) { return this.set(setting, false); }; /** * Delegate `.VERB(...)` calls to `router.VERB(...)`. */ methods.forEach(function(method){ app[method] = function(path){ if (method === 'get' && arguments.length === 1) { // app.get(setting) return this.set(path); } this.lazyrouter(); var route = this._router.route(path); route[method].apply(route, slice.call(arguments, 1)); return this; }; }); /** * Special-cased "all" method, applying the given route `path`, * middleware, and callback to _every_ HTTP method. * * @param {String} path * @param {Function} ... * @return {app} for chaining * @public */ app.all = function all(path) { this.lazyrouter(); var route = this._router.route(path); var args = slice.call(arguments, 1); for (var i = 0; i < methods.length; i++) { route[methods[i]].apply(route, args); } return this; }; // del -> delete alias app.del = deprecate.function(app.delete, 'app.del: Use app.delete instead'); /** * Render the given view `name` name with `options` * and a callback accepting an error and the * rendered template string. * * Example: * * app.render('email', { name: 'Tobi' }, function(err, html){ * // ... * }) * * @param {String} name * @param {Object|Function} options or fn * @param {Function} callback * @public */ app.render = function render(name, options, callback) { var cache = this.cache; var done = callback; var engines = this.engines; var opts = options; var renderOptions = {}; var view; // support callback function as second arg if (typeof options === 'function') { done = options; opts = {}; } // merge app.locals merge(renderOptions, this.locals); // merge options._locals if (opts._locals) { merge(renderOptions, opts._locals); } // merge options merge(renderOptions, opts); // set .cache unless explicitly provided if (renderOptions.cache == null) { renderOptions.cache = this.enabled('view cache'); } // primed cache if (renderOptions.cache) { view = cache[name]; } // view if (!view) { var View = this.get('view'); view = new View(name, { defaultEngine: this.get('view engine'), root: this.get('views'), engines: engines }); if (!view.path) { var dirs = Array.isArray(view.root) && view.root.length > 1 ? 'directories "' + view.root.slice(0, -1).join('", "') + '" or "' + view.root[view.root.length - 1] + '"' : 'directory "' + view.root + '"' var err = new Error('Failed to lookup view "' + name + '" in views ' + dirs); err.view = view; return done(err); } // prime the cache if (renderOptions.cache) { cache[name] = view; } } // render tryRender(view, renderOptions, done); }; /** * Listen for connections. * * A node `http.Server` is returned, with this * application (which is a `Function`) as its * callback. If you wish to create both an HTTP * and HTTPS server you may do so with the "http" * and "https" modules as shown here: * * var http = require('http') * , https = require('https') * , express = require('express') * , app = express(); * * http.createServer(app).listen(80); * https.createServer({ ... }, app).listen(443); * * @return {http.Server} * @public */ app.listen = function listen() { var server = http.createServer(this); return server.listen.apply(server, arguments); }; /** * Log error using console.error. * * @param {Error} err * @private */ function logerror(err) { /* istanbul ignore next */ if (this.get('env') !== 'test') console.error(err.stack || err.toString()); } /** * Try rendering a view. * @private */ function tryRender(view, options, callback) { try { view.render(options, callback); } catch (err) { callback(err); } } apollo-server-demo/node_modules/express/lib/request.js 0000644 0001750 0000144 00000030330 03560116604 022714 0 ustar andreh users /*! * express * Copyright(c) 2009-2013 TJ Holowaychuk * Copyright(c) 2013 Roman Shtylman * Copyright(c) 2014-2015 Douglas Christopher Wilson * MIT Licensed */ 'use strict'; /** * Module dependencies. * @private */ var accepts = require('accepts'); var deprecate = require('depd')('express'); var isIP = require('net').isIP; var typeis = require('type-is'); var http = require('http'); var fresh = require('fresh'); var parseRange = require('range-parser'); var parse = require('parseurl'); var proxyaddr = require('proxy-addr'); /** * Request prototype. * @public */ var req = Object.create(http.IncomingMessage.prototype) /** * Module exports. * @public */ module.exports = req /** * Return request header. * * The `Referrer` header field is special-cased, * both `Referrer` and `Referer` are interchangeable. * * Examples: * * req.get('Content-Type'); * // => "text/plain" * * req.get('content-type'); * // => "text/plain" * * req.get('Something'); * // => undefined * * Aliased as `req.header()`. * * @param {String} name * @return {String} * @public */ req.get = req.header = function header(name) { if (!name) { throw new TypeError('name argument is required to req.get'); } if (typeof name !== 'string') { throw new TypeError('name must be a string to req.get'); } var lc = name.toLowerCase(); switch (lc) { case 'referer': case 'referrer': return this.headers.referrer || this.headers.referer; default: return this.headers[lc]; } }; /** * To do: update docs. * * Check if the given `type(s)` is acceptable, returning * the best match when true, otherwise `undefined`, in which * case you should respond with 406 "Not Acceptable". * * The `type` value may be a single MIME type string * such as "application/json", an extension name * such as "json", a comma-delimited list such as "json, html, text/plain", * an argument list such as `"json", "html", "text/plain"`, * or an array `["json", "html", "text/plain"]`. When a list * or array is given, the _best_ match, if any is returned. * * Examples: * * // Accept: text/html * req.accepts('html'); * // => "html" * * // Accept: text/*, application/json * req.accepts('html'); * // => "html" * req.accepts('text/html'); * // => "text/html" * req.accepts('json, text'); * // => "json" * req.accepts('application/json'); * // => "application/json" * * // Accept: text/*, application/json * req.accepts('image/png'); * req.accepts('png'); * // => undefined * * // Accept: text/*;q=.5, application/json * req.accepts(['html', 'json']); * req.accepts('html', 'json'); * req.accepts('html, json'); * // => "json" * * @param {String|Array} type(s) * @return {String|Array|Boolean} * @public */ req.accepts = function(){ var accept = accepts(this); return accept.types.apply(accept, arguments); }; /** * Check if the given `encoding`s are accepted. * * @param {String} ...encoding * @return {String|Array} * @public */ req.acceptsEncodings = function(){ var accept = accepts(this); return accept.encodings.apply(accept, arguments); }; req.acceptsEncoding = deprecate.function(req.acceptsEncodings, 'req.acceptsEncoding: Use acceptsEncodings instead'); /** * Check if the given `charset`s are acceptable, * otherwise you should respond with 406 "Not Acceptable". * * @param {String} ...charset * @return {String|Array} * @public */ req.acceptsCharsets = function(){ var accept = accepts(this); return accept.charsets.apply(accept, arguments); }; req.acceptsCharset = deprecate.function(req.acceptsCharsets, 'req.acceptsCharset: Use acceptsCharsets instead'); /** * Check if the given `lang`s are acceptable, * otherwise you should respond with 406 "Not Acceptable". * * @param {String} ...lang * @return {String|Array} * @public */ req.acceptsLanguages = function(){ var accept = accepts(this); return accept.languages.apply(accept, arguments); }; req.acceptsLanguage = deprecate.function(req.acceptsLanguages, 'req.acceptsLanguage: Use acceptsLanguages instead'); /** * Parse Range header field, capping to the given `size`. * * Unspecified ranges such as "0-" require knowledge of your resource length. In * the case of a byte range this is of course the total number of bytes. If the * Range header field is not given `undefined` is returned, `-1` when unsatisfiable, * and `-2` when syntactically invalid. * * When ranges are returned, the array has a "type" property which is the type of * range that is required (most commonly, "bytes"). Each array element is an object * with a "start" and "end" property for the portion of the range. * * The "combine" option can be set to `true` and overlapping & adjacent ranges * will be combined into a single range. * * NOTE: remember that ranges are inclusive, so for example "Range: users=0-3" * should respond with 4 users when available, not 3. * * @param {number} size * @param {object} [options] * @param {boolean} [options.combine=false] * @return {number|array} * @public */ req.range = function range(size, options) { var range = this.get('Range'); if (!range) return; return parseRange(size, range, options); }; /** * Return the value of param `name` when present or `defaultValue`. * * - Checks route placeholders, ex: _/user/:id_ * - Checks body params, ex: id=12, {"id":12} * - Checks query string params, ex: ?id=12 * * To utilize request bodies, `req.body` * should be an object. This can be done by using * the `bodyParser()` middleware. * * @param {String} name * @param {Mixed} [defaultValue] * @return {String} * @public */ req.param = function param(name, defaultValue) { var params = this.params || {}; var body = this.body || {}; var query = this.query || {}; var args = arguments.length === 1 ? 'name' : 'name, default'; deprecate('req.param(' + args + '): Use req.params, req.body, or req.query instead'); if (null != params[name] && params.hasOwnProperty(name)) return params[name]; if (null != body[name]) return body[name]; if (null != query[name]) return query[name]; return defaultValue; }; /** * Check if the incoming request contains the "Content-Type" * header field, and it contains the give mime `type`. * * Examples: * * // With Content-Type: text/html; charset=utf-8 * req.is('html'); * req.is('text/html'); * req.is('text/*'); * // => true * * // When Content-Type is application/json * req.is('json'); * req.is('application/json'); * req.is('application/*'); * // => true * * req.is('html'); * // => false * * @param {String|Array} types... * @return {String|false|null} * @public */ req.is = function is(types) { var arr = types; // support flattened arguments if (!Array.isArray(types)) { arr = new Array(arguments.length); for (var i = 0; i < arr.length; i++) { arr[i] = arguments[i]; } } return typeis(this, arr); }; /** * Return the protocol string "http" or "https" * when requested with TLS. When the "trust proxy" * setting trusts the socket address, the * "X-Forwarded-Proto" header field will be trusted * and used if present. * * If you're running behind a reverse proxy that * supplies https for you this may be enabled. * * @return {String} * @public */ defineGetter(req, 'protocol', function protocol(){ var proto = this.connection.encrypted ? 'https' : 'http'; var trust = this.app.get('trust proxy fn'); if (!trust(this.connection.remoteAddress, 0)) { return proto; } // Note: X-Forwarded-Proto is normally only ever a // single value, but this is to be safe. var header = this.get('X-Forwarded-Proto') || proto var index = header.indexOf(',') return index !== -1 ? header.substring(0, index).trim() : header.trim() }); /** * Short-hand for: * * req.protocol === 'https' * * @return {Boolean} * @public */ defineGetter(req, 'secure', function secure(){ return this.protocol === 'https'; }); /** * Return the remote address from the trusted proxy. * * The is the remote address on the socket unless * "trust proxy" is set. * * @return {String} * @public */ defineGetter(req, 'ip', function ip(){ var trust = this.app.get('trust proxy fn'); return proxyaddr(this, trust); }); /** * When "trust proxy" is set, trusted proxy addresses + client. * * For example if the value were "client, proxy1, proxy2" * you would receive the array `["client", "proxy1", "proxy2"]` * where "proxy2" is the furthest down-stream and "proxy1" and * "proxy2" were trusted. * * @return {Array} * @public */ defineGetter(req, 'ips', function ips() { var trust = this.app.get('trust proxy fn'); var addrs = proxyaddr.all(this, trust); // reverse the order (to farthest -> closest) // and remove socket address addrs.reverse().pop() return addrs }); /** * Return subdomains as an array. * * Subdomains are the dot-separated parts of the host before the main domain of * the app. By default, the domain of the app is assumed to be the last two * parts of the host. This can be changed by setting "subdomain offset". * * For example, if the domain is "tobi.ferrets.example.com": * If "subdomain offset" is not set, req.subdomains is `["ferrets", "tobi"]`. * If "subdomain offset" is 3, req.subdomains is `["tobi"]`. * * @return {Array} * @public */ defineGetter(req, 'subdomains', function subdomains() { var hostname = this.hostname; if (!hostname) return []; var offset = this.app.get('subdomain offset'); var subdomains = !isIP(hostname) ? hostname.split('.').reverse() : [hostname]; return subdomains.slice(offset); }); /** * Short-hand for `url.parse(req.url).pathname`. * * @return {String} * @public */ defineGetter(req, 'path', function path() { return parse(this).pathname; }); /** * Parse the "Host" header field to a hostname. * * When the "trust proxy" setting trusts the socket * address, the "X-Forwarded-Host" header field will * be trusted. * * @return {String} * @public */ defineGetter(req, 'hostname', function hostname(){ var trust = this.app.get('trust proxy fn'); var host = this.get('X-Forwarded-Host'); if (!host || !trust(this.connection.remoteAddress, 0)) { host = this.get('Host'); } else if (host.indexOf(',') !== -1) { // Note: X-Forwarded-Host is normally only ever a // single value, but this is to be safe. host = host.substring(0, host.indexOf(',')).trimRight() } if (!host) return; // IPv6 literal support var offset = host[0] === '[' ? host.indexOf(']') + 1 : 0; var index = host.indexOf(':', offset); return index !== -1 ? host.substring(0, index) : host; }); // TODO: change req.host to return host in next major defineGetter(req, 'host', deprecate.function(function host(){ return this.hostname; }, 'req.host: Use req.hostname instead')); /** * Check if the request is fresh, aka * Last-Modified and/or the ETag * still match. * * @return {Boolean} * @public */ defineGetter(req, 'fresh', function(){ var method = this.method; var res = this.res var status = res.statusCode // GET or HEAD for weak freshness validation only if ('GET' !== method && 'HEAD' !== method) return false; // 2xx or 304 as per rfc2616 14.26 if ((status >= 200 && status < 300) || 304 === status) { return fresh(this.headers, { 'etag': res.get('ETag'), 'last-modified': res.get('Last-Modified') }) } return false; }); /** * Check if the request is stale, aka * "Last-Modified" and / or the "ETag" for the * resource has changed. * * @return {Boolean} * @public */ defineGetter(req, 'stale', function stale(){ return !this.fresh; }); /** * Check if the request was an _XMLHttpRequest_. * * @return {Boolean} * @public */ defineGetter(req, 'xhr', function xhr(){ var val = this.get('X-Requested-With') || ''; return val.toLowerCase() === 'xmlhttprequest'; }); /** * Helper function for creating a getter on an object. * * @param {Object} obj * @param {String} name * @param {Function} getter * @private */ function defineGetter(obj, name, getter) { Object.defineProperty(obj, name, { configurable: true, enumerable: true, get: getter }); } apollo-server-demo/node_modules/express/lib/utils.js 0000644 0001750 0000144 00000013524 03560116604 022372 0 ustar andreh users /*! * express * Copyright(c) 2009-2013 TJ Holowaychuk * Copyright(c) 2014-2015 Douglas Christopher Wilson * MIT Licensed */ 'use strict'; /** * Module dependencies. * @api private */ var Buffer = require('safe-buffer').Buffer var contentDisposition = require('content-disposition'); var contentType = require('content-type'); var deprecate = require('depd')('express'); var flatten = require('array-flatten'); var mime = require('send').mime; var etag = require('etag'); var proxyaddr = require('proxy-addr'); var qs = require('qs'); var querystring = require('querystring'); /** * Return strong ETag for `body`. * * @param {String|Buffer} body * @param {String} [encoding] * @return {String} * @api private */ exports.etag = createETagGenerator({ weak: false }) /** * Return weak ETag for `body`. * * @param {String|Buffer} body * @param {String} [encoding] * @return {String} * @api private */ exports.wetag = createETagGenerator({ weak: true }) /** * Check if `path` looks absolute. * * @param {String} path * @return {Boolean} * @api private */ exports.isAbsolute = function(path){ if ('/' === path[0]) return true; if (':' === path[1] && ('\\' === path[2] || '/' === path[2])) return true; // Windows device path if ('\\\\' === path.substring(0, 2)) return true; // Microsoft Azure absolute path }; /** * Flatten the given `arr`. * * @param {Array} arr * @return {Array} * @api private */ exports.flatten = deprecate.function(flatten, 'utils.flatten: use array-flatten npm module instead'); /** * Normalize the given `type`, for example "html" becomes "text/html". * * @param {String} type * @return {Object} * @api private */ exports.normalizeType = function(type){ return ~type.indexOf('/') ? acceptParams(type) : { value: mime.lookup(type), params: {} }; }; /** * Normalize `types`, for example "html" becomes "text/html". * * @param {Array} types * @return {Array} * @api private */ exports.normalizeTypes = function(types){ var ret = []; for (var i = 0; i < types.length; ++i) { ret.push(exports.normalizeType(types[i])); } return ret; }; /** * Generate Content-Disposition header appropriate for the filename. * non-ascii filenames are urlencoded and a filename* parameter is added * * @param {String} filename * @return {String} * @api private */ exports.contentDisposition = deprecate.function(contentDisposition, 'utils.contentDisposition: use content-disposition npm module instead'); /** * Parse accept params `str` returning an * object with `.value`, `.quality` and `.params`. * also includes `.originalIndex` for stable sorting * * @param {String} str * @return {Object} * @api private */ function acceptParams(str, index) { var parts = str.split(/ *; */); var ret = { value: parts[0], quality: 1, params: {}, originalIndex: index }; for (var i = 1; i < parts.length; ++i) { var pms = parts[i].split(/ *= */); if ('q' === pms[0]) { ret.quality = parseFloat(pms[1]); } else { ret.params[pms[0]] = pms[1]; } } return ret; } /** * Compile "etag" value to function. * * @param {Boolean|String|Function} val * @return {Function} * @api private */ exports.compileETag = function(val) { var fn; if (typeof val === 'function') { return val; } switch (val) { case true: fn = exports.wetag; break; case false: break; case 'strong': fn = exports.etag; break; case 'weak': fn = exports.wetag; break; default: throw new TypeError('unknown value for etag function: ' + val); } return fn; } /** * Compile "query parser" value to function. * * @param {String|Function} val * @return {Function} * @api private */ exports.compileQueryParser = function compileQueryParser(val) { var fn; if (typeof val === 'function') { return val; } switch (val) { case true: fn = querystring.parse; break; case false: fn = newObject; break; case 'extended': fn = parseExtendedQueryString; break; case 'simple': fn = querystring.parse; break; default: throw new TypeError('unknown value for query parser function: ' + val); } return fn; } /** * Compile "proxy trust" value to function. * * @param {Boolean|String|Number|Array|Function} val * @return {Function} * @api private */ exports.compileTrust = function(val) { if (typeof val === 'function') return val; if (val === true) { // Support plain true/false return function(){ return true }; } if (typeof val === 'number') { // Support trusting hop count return function(a, i){ return i < val }; } if (typeof val === 'string') { // Support comma-separated values val = val.split(/ *, */); } return proxyaddr.compile(val || []); } /** * Set the charset in a given Content-Type string. * * @param {String} type * @param {String} charset * @return {String} * @api private */ exports.setCharset = function setCharset(type, charset) { if (!type || !charset) { return type; } // parse type var parsed = contentType.parse(type); // set charset parsed.parameters.charset = charset; // format type return contentType.format(parsed); }; /** * Create an ETag generator function, generating ETags with * the given options. * * @param {object} options * @return {function} * @private */ function createETagGenerator (options) { return function generateETag (body, encoding) { var buf = !Buffer.isBuffer(body) ? Buffer.from(body, encoding) : body return etag(buf, options) } } /** * Parse an extended query string with qs. * * @return {Object} * @private */ function parseExtendedQueryString(str) { return qs.parse(str, { allowPrototypes: true }); } /** * Return new empty object. * * @return {Object} * @api private */ function newObject() { return {}; } apollo-server-demo/node_modules/express/lib/express.js 0000644 0001750 0000144 00000004551 03560116604 022723 0 ustar andreh users /*! * express * Copyright(c) 2009-2013 TJ Holowaychuk * Copyright(c) 2013 Roman Shtylman * Copyright(c) 2014-2015 Douglas Christopher Wilson * MIT Licensed */ 'use strict'; /** * Module dependencies. */ var bodyParser = require('body-parser') var EventEmitter = require('events').EventEmitter; var mixin = require('merge-descriptors'); var proto = require('./application'); var Route = require('./router/route'); var Router = require('./router'); var req = require('./request'); var res = require('./response'); /** * Expose `createApplication()`. */ exports = module.exports = createApplication; /** * Create an express application. * * @return {Function} * @api public */ function createApplication() { var app = function(req, res, next) { app.handle(req, res, next); }; mixin(app, EventEmitter.prototype, false); mixin(app, proto, false); // expose the prototype that will get set on requests app.request = Object.create(req, { app: { configurable: true, enumerable: true, writable: true, value: app } }) // expose the prototype that will get set on responses app.response = Object.create(res, { app: { configurable: true, enumerable: true, writable: true, value: app } }) app.init(); return app; } /** * Expose the prototypes. */ exports.application = proto; exports.request = req; exports.response = res; /** * Expose constructors. */ exports.Route = Route; exports.Router = Router; /** * Expose middleware */ exports.json = bodyParser.json exports.query = require('./middleware/query'); exports.raw = bodyParser.raw exports.static = require('serve-static'); exports.text = bodyParser.text exports.urlencoded = bodyParser.urlencoded /** * Replace removed middleware with an appropriate error message. */ var removedMiddlewares = [ 'bodyParser', 'compress', 'cookieSession', 'session', 'logger', 'cookieParser', 'favicon', 'responseTime', 'errorHandler', 'timeout', 'methodOverride', 'vhost', 'csrf', 'directory', 'limit', 'multipart', 'staticCache' ] removedMiddlewares.forEach(function (name) { Object.defineProperty(exports, name, { get: function () { throw new Error('Most middleware (like ' + name + ') is no longer bundled with Express and must be installed separately. Please see https://github.com/senchalabs/connect#middleware.'); }, configurable: true }); }); apollo-server-demo/node_modules/express/lib/view.js 0000644 0001750 0000144 00000006376 03560116604 022213 0 ustar andreh users /*! * express * Copyright(c) 2009-2013 TJ Holowaychuk * Copyright(c) 2013 Roman Shtylman * Copyright(c) 2014-2015 Douglas Christopher Wilson * MIT Licensed */ 'use strict'; /** * Module dependencies. * @private */ var debug = require('debug')('express:view'); var path = require('path'); var fs = require('fs'); /** * Module variables. * @private */ var dirname = path.dirname; var basename = path.basename; var extname = path.extname; var join = path.join; var resolve = path.resolve; /** * Module exports. * @public */ module.exports = View; /** * Initialize a new `View` with the given `name`. * * Options: * * - `defaultEngine` the default template engine name * - `engines` template engine require() cache * - `root` root path for view lookup * * @param {string} name * @param {object} options * @public */ function View(name, options) { var opts = options || {}; this.defaultEngine = opts.defaultEngine; this.ext = extname(name); this.name = name; this.root = opts.root; if (!this.ext && !this.defaultEngine) { throw new Error('No default engine was specified and no extension was provided.'); } var fileName = name; if (!this.ext) { // get extension from default engine name this.ext = this.defaultEngine[0] !== '.' ? '.' + this.defaultEngine : this.defaultEngine; fileName += this.ext; } if (!opts.engines[this.ext]) { // load engine var mod = this.ext.substr(1) debug('require "%s"', mod) // default engine export var fn = require(mod).__express if (typeof fn !== 'function') { throw new Error('Module "' + mod + '" does not provide a view engine.') } opts.engines[this.ext] = fn } // store loaded engine this.engine = opts.engines[this.ext]; // lookup path this.path = this.lookup(fileName); } /** * Lookup view by the given `name` * * @param {string} name * @private */ View.prototype.lookup = function lookup(name) { var path; var roots = [].concat(this.root); debug('lookup "%s"', name); for (var i = 0; i < roots.length && !path; i++) { var root = roots[i]; // resolve the path var loc = resolve(root, name); var dir = dirname(loc); var file = basename(loc); // resolve the file path = this.resolve(dir, file); } return path; }; /** * Render with the given options. * * @param {object} options * @param {function} callback * @private */ View.prototype.render = function render(options, callback) { debug('render "%s"', this.path); this.engine(this.path, options, callback); }; /** * Resolve the file within the given directory. * * @param {string} dir * @param {string} file * @private */ View.prototype.resolve = function resolve(dir, file) { var ext = this.ext; // <path>.<ext> var path = join(dir, file); var stat = tryStat(path); if (stat && stat.isFile()) { return path; } // <path>/index.<ext> path = join(dir, basename(file, ext), 'index' + ext); stat = tryStat(path); if (stat && stat.isFile()) { return path; } }; /** * Return a stat, maybe. * * @param {string} path * @return {fs.Stats} * @private */ function tryStat(path) { debug('stat "%s"', path); try { return fs.statSync(path); } catch (e) { return undefined; } } apollo-server-demo/node_modules/express/lib/response.js 0000644 0001750 0000144 00000064764 03560116604 023104 0 ustar andreh users /*! * express * Copyright(c) 2009-2013 TJ Holowaychuk * Copyright(c) 2014-2015 Douglas Christopher Wilson * MIT Licensed */ 'use strict'; /** * Module dependencies. * @private */ var Buffer = require('safe-buffer').Buffer var contentDisposition = require('content-disposition'); var deprecate = require('depd')('express'); var encodeUrl = require('encodeurl'); var escapeHtml = require('escape-html'); var http = require('http'); var isAbsolute = require('./utils').isAbsolute; var onFinished = require('on-finished'); var path = require('path'); var statuses = require('statuses') var merge = require('utils-merge'); var sign = require('cookie-signature').sign; var normalizeType = require('./utils').normalizeType; var normalizeTypes = require('./utils').normalizeTypes; var setCharset = require('./utils').setCharset; var cookie = require('cookie'); var send = require('send'); var extname = path.extname; var mime = send.mime; var resolve = path.resolve; var vary = require('vary'); /** * Response prototype. * @public */ var res = Object.create(http.ServerResponse.prototype) /** * Module exports. * @public */ module.exports = res /** * Module variables. * @private */ var charsetRegExp = /;\s*charset\s*=/; /** * Set status `code`. * * @param {Number} code * @return {ServerResponse} * @public */ res.status = function status(code) { this.statusCode = code; return this; }; /** * Set Link header field with the given `links`. * * Examples: * * res.links({ * next: 'http://api.example.com/users?page=2', * last: 'http://api.example.com/users?page=5' * }); * * @param {Object} links * @return {ServerResponse} * @public */ res.links = function(links){ var link = this.get('Link') || ''; if (link) link += ', '; return this.set('Link', link + Object.keys(links).map(function(rel){ return '<' + links[rel] + '>; rel="' + rel + '"'; }).join(', ')); }; /** * Send a response. * * Examples: * * res.send(Buffer.from('wahoo')); * res.send({ some: 'json' }); * res.send('<p>some html</p>'); * * @param {string|number|boolean|object|Buffer} body * @public */ res.send = function send(body) { var chunk = body; var encoding; var req = this.req; var type; // settings var app = this.app; // allow status / body if (arguments.length === 2) { // res.send(body, status) backwards compat if (typeof arguments[0] !== 'number' && typeof arguments[1] === 'number') { deprecate('res.send(body, status): Use res.status(status).send(body) instead'); this.statusCode = arguments[1]; } else { deprecate('res.send(status, body): Use res.status(status).send(body) instead'); this.statusCode = arguments[0]; chunk = arguments[1]; } } // disambiguate res.send(status) and res.send(status, num) if (typeof chunk === 'number' && arguments.length === 1) { // res.send(status) will set status message as text string if (!this.get('Content-Type')) { this.type('txt'); } deprecate('res.send(status): Use res.sendStatus(status) instead'); this.statusCode = chunk; chunk = statuses[chunk] } switch (typeof chunk) { // string defaulting to html case 'string': if (!this.get('Content-Type')) { this.type('html'); } break; case 'boolean': case 'number': case 'object': if (chunk === null) { chunk = ''; } else if (Buffer.isBuffer(chunk)) { if (!this.get('Content-Type')) { this.type('bin'); } } else { return this.json(chunk); } break; } // write strings in utf-8 if (typeof chunk === 'string') { encoding = 'utf8'; type = this.get('Content-Type'); // reflect this in content-type if (typeof type === 'string') { this.set('Content-Type', setCharset(type, 'utf-8')); } } // determine if ETag should be generated var etagFn = app.get('etag fn') var generateETag = !this.get('ETag') && typeof etagFn === 'function' // populate Content-Length var len if (chunk !== undefined) { if (Buffer.isBuffer(chunk)) { // get length of Buffer len = chunk.length } else if (!generateETag && chunk.length < 1000) { // just calculate length when no ETag + small chunk len = Buffer.byteLength(chunk, encoding) } else { // convert chunk to Buffer and calculate chunk = Buffer.from(chunk, encoding) encoding = undefined; len = chunk.length } this.set('Content-Length', len); } // populate ETag var etag; if (generateETag && len !== undefined) { if ((etag = etagFn(chunk, encoding))) { this.set('ETag', etag); } } // freshness if (req.fresh) this.statusCode = 304; // strip irrelevant headers if (204 === this.statusCode || 304 === this.statusCode) { this.removeHeader('Content-Type'); this.removeHeader('Content-Length'); this.removeHeader('Transfer-Encoding'); chunk = ''; } if (req.method === 'HEAD') { // skip body for HEAD this.end(); } else { // respond this.end(chunk, encoding); } return this; }; /** * Send JSON response. * * Examples: * * res.json(null); * res.json({ user: 'tj' }); * * @param {string|number|boolean|object} obj * @public */ res.json = function json(obj) { var val = obj; // allow status / body if (arguments.length === 2) { // res.json(body, status) backwards compat if (typeof arguments[1] === 'number') { deprecate('res.json(obj, status): Use res.status(status).json(obj) instead'); this.statusCode = arguments[1]; } else { deprecate('res.json(status, obj): Use res.status(status).json(obj) instead'); this.statusCode = arguments[0]; val = arguments[1]; } } // settings var app = this.app; var escape = app.get('json escape') var replacer = app.get('json replacer'); var spaces = app.get('json spaces'); var body = stringify(val, replacer, spaces, escape) // content-type if (!this.get('Content-Type')) { this.set('Content-Type', 'application/json'); } return this.send(body); }; /** * Send JSON response with JSONP callback support. * * Examples: * * res.jsonp(null); * res.jsonp({ user: 'tj' }); * * @param {string|number|boolean|object} obj * @public */ res.jsonp = function jsonp(obj) { var val = obj; // allow status / body if (arguments.length === 2) { // res.json(body, status) backwards compat if (typeof arguments[1] === 'number') { deprecate('res.jsonp(obj, status): Use res.status(status).json(obj) instead'); this.statusCode = arguments[1]; } else { deprecate('res.jsonp(status, obj): Use res.status(status).jsonp(obj) instead'); this.statusCode = arguments[0]; val = arguments[1]; } } // settings var app = this.app; var escape = app.get('json escape') var replacer = app.get('json replacer'); var spaces = app.get('json spaces'); var body = stringify(val, replacer, spaces, escape) var callback = this.req.query[app.get('jsonp callback name')]; // content-type if (!this.get('Content-Type')) { this.set('X-Content-Type-Options', 'nosniff'); this.set('Content-Type', 'application/json'); } // fixup callback if (Array.isArray(callback)) { callback = callback[0]; } // jsonp if (typeof callback === 'string' && callback.length !== 0) { this.set('X-Content-Type-Options', 'nosniff'); this.set('Content-Type', 'text/javascript'); // restrict callback charset callback = callback.replace(/[^\[\]\w$.]/g, ''); // replace chars not allowed in JavaScript that are in JSON body = body .replace(/\u2028/g, '\\u2028') .replace(/\u2029/g, '\\u2029'); // the /**/ is a specific security mitigation for "Rosetta Flash JSONP abuse" // the typeof check is just to reduce client error noise body = '/**/ typeof ' + callback + ' === \'function\' && ' + callback + '(' + body + ');'; } return this.send(body); }; /** * Send given HTTP status code. * * Sets the response status to `statusCode` and the body of the * response to the standard description from node's http.STATUS_CODES * or the statusCode number if no description. * * Examples: * * res.sendStatus(200); * * @param {number} statusCode * @public */ res.sendStatus = function sendStatus(statusCode) { var body = statuses[statusCode] || String(statusCode) this.statusCode = statusCode; this.type('txt'); return this.send(body); }; /** * Transfer the file at the given `path`. * * Automatically sets the _Content-Type_ response header field. * The callback `callback(err)` is invoked when the transfer is complete * or when an error occurs. Be sure to check `res.sentHeader` * if you wish to attempt responding, as the header and some data * may have already been transferred. * * Options: * * - `maxAge` defaulting to 0 (can be string converted by `ms`) * - `root` root directory for relative filenames * - `headers` object of headers to serve with file * - `dotfiles` serve dotfiles, defaulting to false; can be `"allow"` to send them * * Other options are passed along to `send`. * * Examples: * * The following example illustrates how `res.sendFile()` may * be used as an alternative for the `static()` middleware for * dynamic situations. The code backing `res.sendFile()` is actually * the same code, so HTTP cache support etc is identical. * * app.get('/user/:uid/photos/:file', function(req, res){ * var uid = req.params.uid * , file = req.params.file; * * req.user.mayViewFilesFrom(uid, function(yes){ * if (yes) { * res.sendFile('/uploads/' + uid + '/' + file); * } else { * res.send(403, 'Sorry! you cant see that.'); * } * }); * }); * * @public */ res.sendFile = function sendFile(path, options, callback) { var done = callback; var req = this.req; var res = this; var next = req.next; var opts = options || {}; if (!path) { throw new TypeError('path argument is required to res.sendFile'); } if (typeof path !== 'string') { throw new TypeError('path must be a string to res.sendFile') } // support function as second arg if (typeof options === 'function') { done = options; opts = {}; } if (!opts.root && !isAbsolute(path)) { throw new TypeError('path must be absolute or specify root to res.sendFile'); } // create file stream var pathname = encodeURI(path); var file = send(req, pathname, opts); // transfer sendfile(res, file, opts, function (err) { if (done) return done(err); if (err && err.code === 'EISDIR') return next(); // next() all but write errors if (err && err.code !== 'ECONNABORTED' && err.syscall !== 'write') { next(err); } }); }; /** * Transfer the file at the given `path`. * * Automatically sets the _Content-Type_ response header field. * The callback `callback(err)` is invoked when the transfer is complete * or when an error occurs. Be sure to check `res.sentHeader` * if you wish to attempt responding, as the header and some data * may have already been transferred. * * Options: * * - `maxAge` defaulting to 0 (can be string converted by `ms`) * - `root` root directory for relative filenames * - `headers` object of headers to serve with file * - `dotfiles` serve dotfiles, defaulting to false; can be `"allow"` to send them * * Other options are passed along to `send`. * * Examples: * * The following example illustrates how `res.sendfile()` may * be used as an alternative for the `static()` middleware for * dynamic situations. The code backing `res.sendfile()` is actually * the same code, so HTTP cache support etc is identical. * * app.get('/user/:uid/photos/:file', function(req, res){ * var uid = req.params.uid * , file = req.params.file; * * req.user.mayViewFilesFrom(uid, function(yes){ * if (yes) { * res.sendfile('/uploads/' + uid + '/' + file); * } else { * res.send(403, 'Sorry! you cant see that.'); * } * }); * }); * * @public */ res.sendfile = function (path, options, callback) { var done = callback; var req = this.req; var res = this; var next = req.next; var opts = options || {}; // support function as second arg if (typeof options === 'function') { done = options; opts = {}; } // create file stream var file = send(req, path, opts); // transfer sendfile(res, file, opts, function (err) { if (done) return done(err); if (err && err.code === 'EISDIR') return next(); // next() all but write errors if (err && err.code !== 'ECONNABORTED' && err.syscall !== 'write') { next(err); } }); }; res.sendfile = deprecate.function(res.sendfile, 'res.sendfile: Use res.sendFile instead'); /** * Transfer the file at the given `path` as an attachment. * * Optionally providing an alternate attachment `filename`, * and optional callback `callback(err)`. The callback is invoked * when the data transfer is complete, or when an error has * ocurred. Be sure to check `res.headersSent` if you plan to respond. * * Optionally providing an `options` object to use with `res.sendFile()`. * This function will set the `Content-Disposition` header, overriding * any `Content-Disposition` header passed as header options in order * to set the attachment and filename. * * This method uses `res.sendFile()`. * * @public */ res.download = function download (path, filename, options, callback) { var done = callback; var name = filename; var opts = options || null // support function as second or third arg if (typeof filename === 'function') { done = filename; name = null; opts = null } else if (typeof options === 'function') { done = options opts = null } // set Content-Disposition when file is sent var headers = { 'Content-Disposition': contentDisposition(name || path) }; // merge user-provided headers if (opts && opts.headers) { var keys = Object.keys(opts.headers) for (var i = 0; i < keys.length; i++) { var key = keys[i] if (key.toLowerCase() !== 'content-disposition') { headers[key] = opts.headers[key] } } } // merge user-provided options opts = Object.create(opts) opts.headers = headers // Resolve the full path for sendFile var fullPath = resolve(path); // send file return this.sendFile(fullPath, opts, done) }; /** * Set _Content-Type_ response header with `type` through `mime.lookup()` * when it does not contain "/", or set the Content-Type to `type` otherwise. * * Examples: * * res.type('.html'); * res.type('html'); * res.type('json'); * res.type('application/json'); * res.type('png'); * * @param {String} type * @return {ServerResponse} for chaining * @public */ res.contentType = res.type = function contentType(type) { var ct = type.indexOf('/') === -1 ? mime.lookup(type) : type; return this.set('Content-Type', ct); }; /** * Respond to the Acceptable formats using an `obj` * of mime-type callbacks. * * This method uses `req.accepted`, an array of * acceptable types ordered by their quality values. * When "Accept" is not present the _first_ callback * is invoked, otherwise the first match is used. When * no match is performed the server responds with * 406 "Not Acceptable". * * Content-Type is set for you, however if you choose * you may alter this within the callback using `res.type()` * or `res.set('Content-Type', ...)`. * * res.format({ * 'text/plain': function(){ * res.send('hey'); * }, * * 'text/html': function(){ * res.send('<p>hey</p>'); * }, * * 'appliation/json': function(){ * res.send({ message: 'hey' }); * } * }); * * In addition to canonicalized MIME types you may * also use extnames mapped to these types: * * res.format({ * text: function(){ * res.send('hey'); * }, * * html: function(){ * res.send('<p>hey</p>'); * }, * * json: function(){ * res.send({ message: 'hey' }); * } * }); * * By default Express passes an `Error` * with a `.status` of 406 to `next(err)` * if a match is not made. If you provide * a `.default` callback it will be invoked * instead. * * @param {Object} obj * @return {ServerResponse} for chaining * @public */ res.format = function(obj){ var req = this.req; var next = req.next; var fn = obj.default; if (fn) delete obj.default; var keys = Object.keys(obj); var key = keys.length > 0 ? req.accepts(keys) : false; this.vary("Accept"); if (key) { this.set('Content-Type', normalizeType(key).value); obj[key](req, this, next); } else if (fn) { fn(); } else { var err = new Error('Not Acceptable'); err.status = err.statusCode = 406; err.types = normalizeTypes(keys).map(function(o){ return o.value }); next(err); } return this; }; /** * Set _Content-Disposition_ header to _attachment_ with optional `filename`. * * @param {String} filename * @return {ServerResponse} * @public */ res.attachment = function attachment(filename) { if (filename) { this.type(extname(filename)); } this.set('Content-Disposition', contentDisposition(filename)); return this; }; /** * Append additional header `field` with value `val`. * * Example: * * res.append('Link', ['<http://localhost/>', '<http://localhost:3000/>']); * res.append('Set-Cookie', 'foo=bar; Path=/; HttpOnly'); * res.append('Warning', '199 Miscellaneous warning'); * * @param {String} field * @param {String|Array} val * @return {ServerResponse} for chaining * @public */ res.append = function append(field, val) { var prev = this.get(field); var value = val; if (prev) { // concat the new and prev vals value = Array.isArray(prev) ? prev.concat(val) : Array.isArray(val) ? [prev].concat(val) : [prev, val]; } return this.set(field, value); }; /** * Set header `field` to `val`, or pass * an object of header fields. * * Examples: * * res.set('Foo', ['bar', 'baz']); * res.set('Accept', 'application/json'); * res.set({ Accept: 'text/plain', 'X-API-Key': 'tobi' }); * * Aliased as `res.header()`. * * @param {String|Object} field * @param {String|Array} val * @return {ServerResponse} for chaining * @public */ res.set = res.header = function header(field, val) { if (arguments.length === 2) { var value = Array.isArray(val) ? val.map(String) : String(val); // add charset to content-type if (field.toLowerCase() === 'content-type') { if (Array.isArray(value)) { throw new TypeError('Content-Type cannot be set to an Array'); } if (!charsetRegExp.test(value)) { var charset = mime.charsets.lookup(value.split(';')[0]); if (charset) value += '; charset=' + charset.toLowerCase(); } } this.setHeader(field, value); } else { for (var key in field) { this.set(key, field[key]); } } return this; }; /** * Get value for header `field`. * * @param {String} field * @return {String} * @public */ res.get = function(field){ return this.getHeader(field); }; /** * Clear cookie `name`. * * @param {String} name * @param {Object} [options] * @return {ServerResponse} for chaining * @public */ res.clearCookie = function clearCookie(name, options) { var opts = merge({ expires: new Date(1), path: '/' }, options); return this.cookie(name, '', opts); }; /** * Set cookie `name` to `value`, with the given `options`. * * Options: * * - `maxAge` max-age in milliseconds, converted to `expires` * - `signed` sign the cookie * - `path` defaults to "/" * * Examples: * * // "Remember Me" for 15 minutes * res.cookie('rememberme', '1', { expires: new Date(Date.now() + 900000), httpOnly: true }); * * // same as above * res.cookie('rememberme', '1', { maxAge: 900000, httpOnly: true }) * * @param {String} name * @param {String|Object} value * @param {Object} [options] * @return {ServerResponse} for chaining * @public */ res.cookie = function (name, value, options) { var opts = merge({}, options); var secret = this.req.secret; var signed = opts.signed; if (signed && !secret) { throw new Error('cookieParser("secret") required for signed cookies'); } var val = typeof value === 'object' ? 'j:' + JSON.stringify(value) : String(value); if (signed) { val = 's:' + sign(val, secret); } if ('maxAge' in opts) { opts.expires = new Date(Date.now() + opts.maxAge); opts.maxAge /= 1000; } if (opts.path == null) { opts.path = '/'; } this.append('Set-Cookie', cookie.serialize(name, String(val), opts)); return this; }; /** * Set the location header to `url`. * * The given `url` can also be "back", which redirects * to the _Referrer_ or _Referer_ headers or "/". * * Examples: * * res.location('/foo/bar').; * res.location('http://example.com'); * res.location('../login'); * * @param {String} url * @return {ServerResponse} for chaining * @public */ res.location = function location(url) { var loc = url; // "back" is an alias for the referrer if (url === 'back') { loc = this.req.get('Referrer') || '/'; } // set location return this.set('Location', encodeUrl(loc)); }; /** * Redirect to the given `url` with optional response `status` * defaulting to 302. * * The resulting `url` is determined by `res.location()`, so * it will play nicely with mounted apps, relative paths, * `"back"` etc. * * Examples: * * res.redirect('/foo/bar'); * res.redirect('http://example.com'); * res.redirect(301, 'http://example.com'); * res.redirect('../login'); // /blog/post/1 -> /blog/login * * @public */ res.redirect = function redirect(url) { var address = url; var body; var status = 302; // allow status / url if (arguments.length === 2) { if (typeof arguments[0] === 'number') { status = arguments[0]; address = arguments[1]; } else { deprecate('res.redirect(url, status): Use res.redirect(status, url) instead'); status = arguments[1]; } } // Set location header address = this.location(address).get('Location'); // Support text/{plain,html} by default this.format({ text: function(){ body = statuses[status] + '. Redirecting to ' + address }, html: function(){ var u = escapeHtml(address); body = '<p>' + statuses[status] + '. Redirecting to <a href="' + u + '">' + u + '</a></p>' }, default: function(){ body = ''; } }); // Respond this.statusCode = status; this.set('Content-Length', Buffer.byteLength(body)); if (this.req.method === 'HEAD') { this.end(); } else { this.end(body); } }; /** * Add `field` to Vary. If already present in the Vary set, then * this call is simply ignored. * * @param {Array|String} field * @return {ServerResponse} for chaining * @public */ res.vary = function(field){ // checks for back-compat if (!field || (Array.isArray(field) && !field.length)) { deprecate('res.vary(): Provide a field name'); return this; } vary(this, field); return this; }; /** * Render `view` with the given `options` and optional callback `fn`. * When a callback function is given a response will _not_ be made * automatically, otherwise a response of _200_ and _text/html_ is given. * * Options: * * - `cache` boolean hinting to the engine it should cache * - `filename` filename of the view being rendered * * @public */ res.render = function render(view, options, callback) { var app = this.req.app; var done = callback; var opts = options || {}; var req = this.req; var self = this; // support callback function as second arg if (typeof options === 'function') { done = options; opts = {}; } // merge res.locals opts._locals = self.locals; // default callback to respond done = done || function (err, str) { if (err) return req.next(err); self.send(str); }; // render app.render(view, opts, done); }; // pipe the send file stream function sendfile(res, file, options, callback) { var done = false; var streaming; // request aborted function onaborted() { if (done) return; done = true; var err = new Error('Request aborted'); err.code = 'ECONNABORTED'; callback(err); } // directory function ondirectory() { if (done) return; done = true; var err = new Error('EISDIR, read'); err.code = 'EISDIR'; callback(err); } // errors function onerror(err) { if (done) return; done = true; callback(err); } // ended function onend() { if (done) return; done = true; callback(); } // file function onfile() { streaming = false; } // finished function onfinish(err) { if (err && err.code === 'ECONNRESET') return onaborted(); if (err) return onerror(err); if (done) return; setImmediate(function () { if (streaming !== false && !done) { onaborted(); return; } if (done) return; done = true; callback(); }); } // streaming function onstream() { streaming = true; } file.on('directory', ondirectory); file.on('end', onend); file.on('error', onerror); file.on('file', onfile); file.on('stream', onstream); onFinished(res, onfinish); if (options.headers) { // set headers on successful transfer file.on('headers', function headers(res) { var obj = options.headers; var keys = Object.keys(obj); for (var i = 0; i < keys.length; i++) { var k = keys[i]; res.setHeader(k, obj[k]); } }); } // pipe file.pipe(res); } /** * Stringify JSON, like JSON.stringify, but v8 optimized, with the * ability to escape characters that can trigger HTML sniffing. * * @param {*} value * @param {function} replaces * @param {number} spaces * @param {boolean} escape * @returns {string} * @private */ function stringify (value, replacer, spaces, escape) { // v8 checks arguments.length for optimizing simple call // https://bugs.chromium.org/p/v8/issues/detail?id=4730 var json = replacer || spaces ? JSON.stringify(value, replacer, spaces) : JSON.stringify(value); if (escape) { json = json.replace(/[<>&]/g, function (c) { switch (c.charCodeAt(0)) { case 0x3c: return '\\u003c' case 0x3e: return '\\u003e' case 0x26: return '\\u0026' /* istanbul ignore next: unreachable default */ default: return c } }) } return json } apollo-server-demo/node_modules/cors/ 0000755 0001750 0000144 00000000000 14067647700 017410 5 ustar andreh users apollo-server-demo/node_modules/cors/LICENSE 0000644 0001750 0000144 00000002107 03560116604 020403 0 ustar andreh users (The MIT License) Copyright (c) 2013 Troy Goode <troygoode@gmail.com> Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the 'Software'), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/cors/CONTRIBUTING.md 0000644 0001750 0000144 00000002004 03560116604 021623 0 ustar andreh users # contributing to `cors` CORS is a node.js package for providing a [connect](http://www.senchalabs.org/connect/)/[express](http://expressjs.com/) middleware that can be used to enable [CORS](http://en.wikipedia.org/wiki/Cross-origin_resource_sharing) with various options. Learn more about the project in [the README](README.md). ## The CORS Spec [http://www.w3.org/TR/cors/](http://www.w3.org/TR/cors/) ## Pull Requests Welcome * Include `'use strict';` in every javascript file. * 2 space indentation. * Please run the testing steps below before submitting. ## Testing ```bash $ npm install $ npm test ``` ## Interactive Testing Harness [http://node-cors-client.herokuapp.com](http://node-cors-client.herokuapp.com) Related git repositories: * [https://github.com/TroyGoode/node-cors-server](https://github.com/TroyGoode/node-cors-server) * [https://github.com/TroyGoode/node-cors-client](https://github.com/TroyGoode/node-cors-client) ## License [MIT License](http://www.opensource.org/licenses/mit-license.php) apollo-server-demo/node_modules/cors/HISTORY.md 0000644 0001750 0000144 00000002221 03560116604 021056 0 ustar andreh users 2.8.5 / 2018-11-04 ================== * Fix setting `maxAge` option to `0` 2.8.4 / 2017-07-12 ================== * Work-around Safari bug in default pre-flight response 2.8.3 / 2017-03-29 ================== * Fix error when options delegate missing `methods` option 2.8.2 / 2017-03-28 ================== * Fix error when frozen options are passed * Send "Vary: Origin" when using regular expressions * Send "Vary: Access-Control-Request-Headers" when dynamic `allowedHeaders` 2.8.1 / 2016-09-08 ================== This release only changed documentation. 2.8.0 / 2016-08-23 ================== * Add `optionsSuccessStatus` option 2.7.2 / 2016-08-23 ================== * Fix error when Node.js running in strict mode 2.7.1 / 2015-05-28 ================== * Move module into expressjs organization 2.7.0 / 2015-05-28 ================== * Allow array of matching condition as `origin` option * Allow regular expression as `origin` option 2.6.1 / 2015-05-28 ================== * Update `license` in package.json 2.6.0 / 2015-04-27 ================== * Add `preflightContinue` option * Fix "Vary: Origin" header added for "*" apollo-server-demo/node_modules/cors/README.md 0000644 0001750 0000144 00000021766 03560116604 020671 0 ustar andreh users # cors [![NPM Version][npm-image]][npm-url] [![NPM Downloads][downloads-image]][downloads-url] [![Build Status][travis-image]][travis-url] [![Test Coverage][coveralls-image]][coveralls-url] CORS is a node.js package for providing a [Connect](http://www.senchalabs.org/connect/)/[Express](http://expressjs.com/) middleware that can be used to enable [CORS](http://en.wikipedia.org/wiki/Cross-origin_resource_sharing) with various options. **[Follow me (@troygoode) on Twitter!](https://twitter.com/intent/user?screen_name=troygoode)** * [Installation](#installation) * [Usage](#usage) * [Simple Usage](#simple-usage-enable-all-cors-requests) * [Enable CORS for a Single Route](#enable-cors-for-a-single-route) * [Configuring CORS](#configuring-cors) * [Configuring CORS Asynchronously](#configuring-cors-asynchronously) * [Enabling CORS Pre-Flight](#enabling-cors-pre-flight) * [Configuration Options](#configuration-options) * [Demo](#demo) * [License](#license) * [Author](#author) ## Installation This is a [Node.js](https://nodejs.org/en/) module available through the [npm registry](https://www.npmjs.com/). Installation is done using the [`npm install` command](https://docs.npmjs.com/getting-started/installing-npm-packages-locally): ```sh $ npm install cors ``` ## Usage ### Simple Usage (Enable *All* CORS Requests) ```javascript var express = require('express') var cors = require('cors') var app = express() app.use(cors()) app.get('/products/:id', function (req, res, next) { res.json({msg: 'This is CORS-enabled for all origins!'}) }) app.listen(80, function () { console.log('CORS-enabled web server listening on port 80') }) ``` ### Enable CORS for a Single Route ```javascript var express = require('express') var cors = require('cors') var app = express() app.get('/products/:id', cors(), function (req, res, next) { res.json({msg: 'This is CORS-enabled for a Single Route'}) }) app.listen(80, function () { console.log('CORS-enabled web server listening on port 80') }) ``` ### Configuring CORS ```javascript var express = require('express') var cors = require('cors') var app = express() var corsOptions = { origin: 'http://example.com', optionsSuccessStatus: 200 // some legacy browsers (IE11, various SmartTVs) choke on 204 } app.get('/products/:id', cors(corsOptions), function (req, res, next) { res.json({msg: 'This is CORS-enabled for only example.com.'}) }) app.listen(80, function () { console.log('CORS-enabled web server listening on port 80') }) ``` ### Configuring CORS w/ Dynamic Origin ```javascript var express = require('express') var cors = require('cors') var app = express() var whitelist = ['http://example1.com', 'http://example2.com'] var corsOptions = { origin: function (origin, callback) { if (whitelist.indexOf(origin) !== -1) { callback(null, true) } else { callback(new Error('Not allowed by CORS')) } } } app.get('/products/:id', cors(corsOptions), function (req, res, next) { res.json({msg: 'This is CORS-enabled for a whitelisted domain.'}) }) app.listen(80, function () { console.log('CORS-enabled web server listening on port 80') }) ``` If you do not want to block REST tools or server-to-server requests, add a `!origin` check in the origin function like so: ```javascript var corsOptions = { origin: function (origin, callback) { if (whitelist.indexOf(origin) !== -1 || !origin) { callback(null, true) } else { callback(new Error('Not allowed by CORS')) } } } ``` ### Enabling CORS Pre-Flight Certain CORS requests are considered 'complex' and require an initial `OPTIONS` request (called the "pre-flight request"). An example of a 'complex' CORS request is one that uses an HTTP verb other than GET/HEAD/POST (such as DELETE) or that uses custom headers. To enable pre-flighting, you must add a new OPTIONS handler for the route you want to support: ```javascript var express = require('express') var cors = require('cors') var app = express() app.options('/products/:id', cors()) // enable pre-flight request for DELETE request app.del('/products/:id', cors(), function (req, res, next) { res.json({msg: 'This is CORS-enabled for all origins!'}) }) app.listen(80, function () { console.log('CORS-enabled web server listening on port 80') }) ``` You can also enable pre-flight across-the-board like so: ```javascript app.options('*', cors()) // include before other routes ``` ### Configuring CORS Asynchronously ```javascript var express = require('express') var cors = require('cors') var app = express() var whitelist = ['http://example1.com', 'http://example2.com'] var corsOptionsDelegate = function (req, callback) { var corsOptions; if (whitelist.indexOf(req.header('Origin')) !== -1) { corsOptions = { origin: true } // reflect (enable) the requested origin in the CORS response } else { corsOptions = { origin: false } // disable CORS for this request } callback(null, corsOptions) // callback expects two parameters: error and options } app.get('/products/:id', cors(corsOptionsDelegate), function (req, res, next) { res.json({msg: 'This is CORS-enabled for a whitelisted domain.'}) }) app.listen(80, function () { console.log('CORS-enabled web server listening on port 80') }) ``` ## Configuration Options * `origin`: Configures the **Access-Control-Allow-Origin** CORS header. Possible values: - `Boolean` - set `origin` to `true` to reflect the [request origin](http://tools.ietf.org/html/draft-abarth-origin-09), as defined by `req.header('Origin')`, or set it to `false` to disable CORS. - `String` - set `origin` to a specific origin. For example if you set it to `"http://example.com"` only requests from "http://example.com" will be allowed. - `RegExp` - set `origin` to a regular expression pattern which will be used to test the request origin. If it's a match, the request origin will be reflected. For example the pattern `/example\.com$/` will reflect any request that is coming from an origin ending with "example.com". - `Array` - set `origin` to an array of valid origins. Each origin can be a `String` or a `RegExp`. For example `["http://example1.com", /\.example2\.com$/]` will accept any request from "http://example1.com" or from a subdomain of "example2.com". - `Function` - set `origin` to a function implementing some custom logic. The function takes the request origin as the first parameter and a callback (which expects the signature `err [object], allow [bool]`) as the second. * `methods`: Configures the **Access-Control-Allow-Methods** CORS header. Expects a comma-delimited string (ex: 'GET,PUT,POST') or an array (ex: `['GET', 'PUT', 'POST']`). * `allowedHeaders`: Configures the **Access-Control-Allow-Headers** CORS header. Expects a comma-delimited string (ex: 'Content-Type,Authorization') or an array (ex: `['Content-Type', 'Authorization']`). If not specified, defaults to reflecting the headers specified in the request's **Access-Control-Request-Headers** header. * `exposedHeaders`: Configures the **Access-Control-Expose-Headers** CORS header. Expects a comma-delimited string (ex: 'Content-Range,X-Content-Range') or an array (ex: `['Content-Range', 'X-Content-Range']`). If not specified, no custom headers are exposed. * `credentials`: Configures the **Access-Control-Allow-Credentials** CORS header. Set to `true` to pass the header, otherwise it is omitted. * `maxAge`: Configures the **Access-Control-Max-Age** CORS header. Set to an integer to pass the header, otherwise it is omitted. * `preflightContinue`: Pass the CORS preflight response to the next handler. * `optionsSuccessStatus`: Provides a status code to use for successful `OPTIONS` requests, since some legacy browsers (IE11, various SmartTVs) choke on `204`. The default configuration is the equivalent of: ```json { "origin": "*", "methods": "GET,HEAD,PUT,PATCH,POST,DELETE", "preflightContinue": false, "optionsSuccessStatus": 204 } ``` For details on the effect of each CORS header, read [this](http://www.html5rocks.com/en/tutorials/cors/) article on HTML5 Rocks. ## Demo A demo that illustrates CORS working (and not working) using jQuery is available here: [http://node-cors-client.herokuapp.com/](http://node-cors-client.herokuapp.com/) Code for that demo can be found here: * Client: [https://github.com/TroyGoode/node-cors-client](https://github.com/TroyGoode/node-cors-client) * Server: [https://github.com/TroyGoode/node-cors-server](https://github.com/TroyGoode/node-cors-server) ## License [MIT License](http://www.opensource.org/licenses/mit-license.php) ## Author [Troy Goode](https://github.com/TroyGoode) ([troygoode@gmail.com](mailto:troygoode@gmail.com)) [coveralls-image]: https://img.shields.io/coveralls/expressjs/cors/master.svg [coveralls-url]: https://coveralls.io/r/expressjs/cors?branch=master [downloads-image]: https://img.shields.io/npm/dm/cors.svg [downloads-url]: https://npmjs.org/package/cors [npm-image]: https://img.shields.io/npm/v/cors.svg [npm-url]: https://npmjs.org/package/cors [travis-image]: https://img.shields.io/travis/expressjs/cors/master.svg [travis-url]: https://travis-ci.org/expressjs/cors apollo-server-demo/node_modules/cors/package.json 0000644 0001750 0000144 00000002054 03560116604 021665 0 ustar andreh users { "name": "cors", "description": "Node.js CORS middleware", "version": "2.8.5", "author": "Troy Goode <troygoode@gmail.com> (https://github.com/troygoode/)", "license": "MIT", "keywords": [ "cors", "express", "connect", "middleware" ], "repository": "expressjs/cors", "main": "./lib/index.js", "dependencies": { "object-assign": "^4", "vary": "^1" }, "devDependencies": { "after": "0.8.2", "eslint": "2.13.1", "express": "4.16.3", "mocha": "5.2.0", "nyc": "13.1.0", "supertest": "3.3.0" }, "files": [ "lib/index.js", "CONTRIBUTING.md", "HISTORY.md", "LICENSE", "README.md" ], "engines": { "node": ">= 0.10" }, "scripts": { "test": "npm run lint && nyc --reporter=html --reporter=text mocha --require test/support/env", "lint": "eslint lib test" } ,"_resolved": "https://registry.npmjs.org/cors/-/cors-2.8.5.tgz" ,"_integrity": "sha512-KIHbLJqu73RGr/hnbrO9uBeixNGuvSQjul/jdFvS/KFSIH1hWVd1ng7zOHx+YrEfInLG7q4n6GHQ9cDtxv/P6g==" ,"_from": "cors@2.8.5" } apollo-server-demo/node_modules/cors/lib/ 0000755 0001750 0000144 00000000000 14067647700 020156 5 ustar andreh users apollo-server-demo/node_modules/cors/lib/index.js 0000644 0001750 0000144 00000014737 03560116604 021625 0 ustar andreh users (function () { 'use strict'; var assign = require('object-assign'); var vary = require('vary'); var defaults = { origin: '*', methods: 'GET,HEAD,PUT,PATCH,POST,DELETE', preflightContinue: false, optionsSuccessStatus: 204 }; function isString(s) { return typeof s === 'string' || s instanceof String; } function isOriginAllowed(origin, allowedOrigin) { if (Array.isArray(allowedOrigin)) { for (var i = 0; i < allowedOrigin.length; ++i) { if (isOriginAllowed(origin, allowedOrigin[i])) { return true; } } return false; } else if (isString(allowedOrigin)) { return origin === allowedOrigin; } else if (allowedOrigin instanceof RegExp) { return allowedOrigin.test(origin); } else { return !!allowedOrigin; } } function configureOrigin(options, req) { var requestOrigin = req.headers.origin, headers = [], isAllowed; if (!options.origin || options.origin === '*') { // allow any origin headers.push([{ key: 'Access-Control-Allow-Origin', value: '*' }]); } else if (isString(options.origin)) { // fixed origin headers.push([{ key: 'Access-Control-Allow-Origin', value: options.origin }]); headers.push([{ key: 'Vary', value: 'Origin' }]); } else { isAllowed = isOriginAllowed(requestOrigin, options.origin); // reflect origin headers.push([{ key: 'Access-Control-Allow-Origin', value: isAllowed ? requestOrigin : false }]); headers.push([{ key: 'Vary', value: 'Origin' }]); } return headers; } function configureMethods(options) { var methods = options.methods; if (methods.join) { methods = options.methods.join(','); // .methods is an array, so turn it into a string } return { key: 'Access-Control-Allow-Methods', value: methods }; } function configureCredentials(options) { if (options.credentials === true) { return { key: 'Access-Control-Allow-Credentials', value: 'true' }; } return null; } function configureAllowedHeaders(options, req) { var allowedHeaders = options.allowedHeaders || options.headers; var headers = []; if (!allowedHeaders) { allowedHeaders = req.headers['access-control-request-headers']; // .headers wasn't specified, so reflect the request headers headers.push([{ key: 'Vary', value: 'Access-Control-Request-Headers' }]); } else if (allowedHeaders.join) { allowedHeaders = allowedHeaders.join(','); // .headers is an array, so turn it into a string } if (allowedHeaders && allowedHeaders.length) { headers.push([{ key: 'Access-Control-Allow-Headers', value: allowedHeaders }]); } return headers; } function configureExposedHeaders(options) { var headers = options.exposedHeaders; if (!headers) { return null; } else if (headers.join) { headers = headers.join(','); // .headers is an array, so turn it into a string } if (headers && headers.length) { return { key: 'Access-Control-Expose-Headers', value: headers }; } return null; } function configureMaxAge(options) { var maxAge = (typeof options.maxAge === 'number' || options.maxAge) && options.maxAge.toString() if (maxAge && maxAge.length) { return { key: 'Access-Control-Max-Age', value: maxAge }; } return null; } function applyHeaders(headers, res) { for (var i = 0, n = headers.length; i < n; i++) { var header = headers[i]; if (header) { if (Array.isArray(header)) { applyHeaders(header, res); } else if (header.key === 'Vary' && header.value) { vary(res, header.value); } else if (header.value) { res.setHeader(header.key, header.value); } } } } function cors(options, req, res, next) { var headers = [], method = req.method && req.method.toUpperCase && req.method.toUpperCase(); if (method === 'OPTIONS') { // preflight headers.push(configureOrigin(options, req)); headers.push(configureCredentials(options, req)); headers.push(configureMethods(options, req)); headers.push(configureAllowedHeaders(options, req)); headers.push(configureMaxAge(options, req)); headers.push(configureExposedHeaders(options, req)); applyHeaders(headers, res); if (options.preflightContinue) { next(); } else { // Safari (and potentially other browsers) need content-length 0, // for 204 or they just hang waiting for a body res.statusCode = options.optionsSuccessStatus; res.setHeader('Content-Length', '0'); res.end(); } } else { // actual response headers.push(configureOrigin(options, req)); headers.push(configureCredentials(options, req)); headers.push(configureExposedHeaders(options, req)); applyHeaders(headers, res); next(); } } function middlewareWrapper(o) { // if options are static (either via defaults or custom options passed in), wrap in a function var optionsCallback = null; if (typeof o === 'function') { optionsCallback = o; } else { optionsCallback = function (req, cb) { cb(null, o); }; } return function corsMiddleware(req, res, next) { optionsCallback(req, function (err, options) { if (err) { next(err); } else { var corsOptions = assign({}, defaults, options); var originCallback = null; if (corsOptions.origin && typeof corsOptions.origin === 'function') { originCallback = corsOptions.origin; } else if (corsOptions.origin) { originCallback = function (origin, cb) { cb(null, corsOptions.origin); }; } if (originCallback) { originCallback(req.headers.origin, function (err2, origin) { if (err2 || !origin) { next(err2); } else { corsOptions.origin = origin; cors(corsOptions, req, res, next); } }); } else { next(); } } }); }; } // can pass either an options hash, an options delegate, or nothing module.exports = middlewareWrapper; }()); apollo-server-demo/node_modules/destroy/ 0000755 0001750 0000144 00000000000 14067647700 020133 5 ustar andreh users apollo-server-demo/node_modules/destroy/index.js 0000644 0001750 0000144 00000002023 12645473630 021574 0 ustar andreh users /*! * destroy * Copyright(c) 2014 Jonathan Ong * MIT Licensed */ 'use strict' /** * Module dependencies. * @private */ var ReadStream = require('fs').ReadStream var Stream = require('stream') /** * Module exports. * @public */ module.exports = destroy /** * Destroy a stream. * * @param {object} stream * @public */ function destroy(stream) { if (stream instanceof ReadStream) { return destroyReadStream(stream) } if (!(stream instanceof Stream)) { return stream } if (typeof stream.destroy === 'function') { stream.destroy() } return stream } /** * Destroy a ReadStream. * * @param {object} stream * @private */ function destroyReadStream(stream) { stream.destroy() if (typeof stream.close === 'function') { // node.js core bug work-around stream.on('open', onOpenClose) } return stream } /** * On open handler to close stream. * @private */ function onOpenClose() { if (typeof this.fd === 'number') { // actually close down the fd this.close() } } apollo-server-demo/node_modules/destroy/LICENSE 0000644 0001750 0000144 00000002113 12373217043 021124 0 ustar andreh users The MIT License (MIT) Copyright (c) 2014 Jonathan Ong me@jongleberry.com Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/destroy/README.md 0000644 0001750 0000144 00000004211 12645475561 021414 0 ustar andreh users # Destroy [![NPM version][npm-image]][npm-url] [![Build status][travis-image]][travis-url] [![Test coverage][coveralls-image]][coveralls-url] [![License][license-image]][license-url] [![Downloads][downloads-image]][downloads-url] [![Gittip][gittip-image]][gittip-url] Destroy a stream. This module is meant to ensure a stream gets destroyed, handling different APIs and Node.js bugs. ## API ```js var destroy = require('destroy') ``` ### destroy(stream) Destroy the given stream. In most cases, this is identical to a simple `stream.destroy()` call. The rules are as follows for a given stream: 1. If the `stream` is an instance of `ReadStream`, then call `stream.destroy()` and add a listener to the `open` event to call `stream.close()` if it is fired. This is for a Node.js bug that will leak a file descriptor if `.destroy()` is called before `open`. 2. If the `stream` is not an instance of `Stream`, then nothing happens. 3. If the `stream` has a `.destroy()` method, then call it. The function returns the `stream` passed in as the argument. ## Example ```js var destroy = require('destroy') var fs = require('fs') var stream = fs.createReadStream('package.json') // ... and later destroy(stream) ``` [npm-image]: https://img.shields.io/npm/v/destroy.svg?style=flat-square [npm-url]: https://npmjs.org/package/destroy [github-tag]: http://img.shields.io/github/tag/stream-utils/destroy.svg?style=flat-square [github-url]: https://github.com/stream-utils/destroy/tags [travis-image]: https://img.shields.io/travis/stream-utils/destroy.svg?style=flat-square [travis-url]: https://travis-ci.org/stream-utils/destroy [coveralls-image]: https://img.shields.io/coveralls/stream-utils/destroy.svg?style=flat-square [coveralls-url]: https://coveralls.io/r/stream-utils/destroy?branch=master [license-image]: http://img.shields.io/npm/l/destroy.svg?style=flat-square [license-url]: LICENSE.md [downloads-image]: http://img.shields.io/npm/dm/destroy.svg?style=flat-square [downloads-url]: https://npmjs.org/package/destroy [gittip-image]: https://img.shields.io/gittip/jonathanong.svg?style=flat-square [gittip-url]: https://www.gittip.com/jonathanong/ apollo-server-demo/node_modules/destroy/package.json 0000644 0001750 0000144 00000001772 12646332263 022424 0 ustar andreh users { "name": "destroy", "description": "destroy a stream if possible", "version": "1.0.4", "author": { "name": "Jonathan Ong", "email": "me@jongleberry.com", "url": "http://jongleberry.com", "twitter": "https://twitter.com/jongleberry" }, "contributors": [ "Douglas Christopher Wilson <doug@somethingdoug.com>" ], "license": "MIT", "repository": "stream-utils/destroy", "devDependencies": { "istanbul": "0.4.2", "mocha": "2.3.4" }, "scripts": { "test": "mocha --reporter spec", "test-cov": "istanbul cover node_modules/mocha/bin/_mocha -- --reporter dot", "test-travis": "istanbul cover node_modules/mocha/bin/_mocha --report lcovonly -- --reporter dot" }, "files": [ "index.js", "LICENSE" ], "keywords": [ "stream", "streams", "destroy", "cleanup", "leak", "fd" ] ,"_resolved": "https://registry.npmjs.org/destroy/-/destroy-1.0.4.tgz" ,"_integrity": "sha1-l4hXRCxEdJ5CBmE+N5RiBYJqvYA=" ,"_from": "destroy@1.0.4" } apollo-server-demo/node_modules/apollo-graphql/ 0000755 0001750 0000144 00000000000 14067647700 021364 5 ustar andreh users apollo-server-demo/node_modules/apollo-graphql/LICENSE 0000644 0001750 0000144 00000002111 03560116604 022352 0 ustar andreh users The MIT License (MIT) Copyright (c) 2016 Meteor Development Group, Inc. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/apollo-graphql/src/ 0000755 0001750 0000144 00000000000 14067647701 022154 5 ustar andreh users apollo-server-demo/node_modules/apollo-graphql/src/__tests__/ 0000755 0001750 0000144 00000000000 14067647701 024112 5 ustar andreh users apollo-server-demo/node_modules/apollo-graphql/src/__tests__/operationId.test.ts 0000644 0001750 0000144 00000013231 03560116604 027702 0 ustar andreh users import { default as gql, disableFragmentWarnings } from "graphql-tag"; import { defaultUsageReportingSignature, operationRegistrySignature } from "../operationId"; // The gql duplicate fragment warning feature really is just warnings; nothing // breaks if you turn it off in tests. disableFragmentWarnings(); describe("defaultUsageReportingSignature", () => { const cases = [ // Test cases borrowed from optics-agent-js. { name: "basic test", operationName: "", input: gql` { user { name } } ` }, { name: "basic test with query", operationName: "", input: gql` query { user { name } } ` }, { name: "basic with operation name", operationName: "OpName", input: gql` query OpName { user { name } } ` }, { name: "with various inline types", operationName: "OpName", input: gql` query OpName { user { name(apple: [[10]], cat: ENUM_VALUE, bag: { input: "value" }) } } ` }, { name: "with various argument types", operationName: "OpName", input: gql` query OpName($c: Int!, $a: [[Boolean!]!], $b: EnumType) { user { name(apple: $a, cat: $c, bag: $b) } } ` }, { name: "fragment", operationName: "", input: gql` { user { name ...Bar } } fragment Bar on User { asd } fragment Baz on User { jkl } ` }, { name: "fragments in various order", operationName: "", input: gql` fragment Bar on User { asd } { user { name ...Bar } } fragment Baz on User { jkl } ` }, { name: "full test", operationName: "Foo", input: gql` query Foo($b: Int, $a: Boolean) { user(name: "hello", age: 5) { ...Bar ... on User { hello bee } tz aliased: name } } fragment Baz on User { asd } fragment Bar on User { age @skip(if: $a) ...Nested } fragment Nested on User { blah } ` } ]; cases.forEach(({ name, operationName, input }) => { test(name, () => { expect( defaultUsageReportingSignature(input, operationName) ).toMatchSnapshot(); }); }); }); describe("operationRegistrySignature", () => { const cases = [ // Test cases borrowed from optics-agent-js. { name: "basic test", operationName: "", input: gql` { user { name } } ` }, { name: "basic test with query", operationName: "", input: gql` query { user { name } } ` }, { name: "basic with operation name", operationName: "OpName", input: gql` query OpName { user { name } } ` }, { name: "with various inline types", operationName: "OpName", input: gql` query OpName { user { name(apple: [[10]], cat: ENUM_VALUE, bag: { input: "value" }) } } ` }, { name: "with various argument types", operationName: "OpName", input: gql` query OpName($c: Int!, $a: [[Boolean!]!], $b: EnumType) { user { name(apple: $a, cat: $c, bag: $b) } } ` }, { name: "fragment", operationName: "", input: gql` { user { name ...Bar } } fragment Bar on User { asd } fragment Baz on User { jkl } ` }, { name: "fragments in various order", operationName: "", input: gql` fragment Bar on User { asd } { user { name ...Bar } } fragment Baz on User { jkl } ` }, { name: "full test", operationName: "Foo", input: gql` query Foo($b: Int, $a: Boolean) { user(name: "hello", age: 5) { ...Bar ... on User { hello bee } tz aliased: name } } fragment Baz on User { asd } fragment Bar on User { age @skip(if: $a) ...Nested } fragment Nested on User { blah } ` }, { name: "test with preserveStringAndNumericLiterals=true", operationName: "Foo", input: gql` query Foo($b: Int) { user(name: "hello", age: 5) { ...Bar a @skip(if: true) b @include(if: false) c(value: 4) { d } ... on User { hello @directive(arg: "Value!") } } } `, options: { preserveStringAndNumericLiterals: true } } ]; cases.forEach(({ name, operationName, input, options }) => { test(name, () => { expect( operationRegistrySignature(input, operationName, options) ).toMatchSnapshot(); }); }); }); apollo-server-demo/node_modules/apollo-graphql/src/__tests__/__snapshots__/ 0000755 0001750 0000144 00000000000 14067647700 026727 5 ustar andreh users apollo-server-demo/node_modules/apollo-graphql/src/__tests__/__snapshots__/operationId.test.ts.snap 0000644 0001750 0000144 00000004424 03560116604 033464 0 ustar andreh users // Jest Snapshot v1, https://goo.gl/fbAQLP exports[`defaultUsageReportingSignature basic test 1`] = `"{user{name}}"`; exports[`defaultUsageReportingSignature basic test with query 1`] = `"{user{name}}"`; exports[`defaultUsageReportingSignature basic with operation name 1`] = `"query OpName{user{name}}"`; exports[`defaultUsageReportingSignature fragment 1`] = `"fragment Bar on User{asd}{user{name...Bar}}"`; exports[`defaultUsageReportingSignature fragments in various order 1`] = `"fragment Bar on User{asd}{user{name...Bar}}"`; exports[`defaultUsageReportingSignature full test 1`] = `"fragment Bar on User{age@skip(if:$a)...Nested}fragment Nested on User{blah}query Foo($a:Boolean,$b:Int){user(age:0,name:\\"\\"){name tz...Bar...on User{bee hello}}}"`; exports[`defaultUsageReportingSignature with various argument types 1`] = `"query OpName($a:[[Boolean!]!],$b:EnumType,$c:Int!){user{name(apple:$a,bag:$b,cat:$c)}}"`; exports[`defaultUsageReportingSignature with various inline types 1`] = `"query OpName{user{name(apple:[],bag:{},cat:ENUM_VALUE)}}"`; exports[`operationRegistrySignature basic test 1`] = `"{user{name}}"`; exports[`operationRegistrySignature basic test with query 1`] = `"{user{name}}"`; exports[`operationRegistrySignature basic with operation name 1`] = `"query OpName{user{name}}"`; exports[`operationRegistrySignature fragment 1`] = `"fragment Bar on User{asd}{user{name...Bar}}"`; exports[`operationRegistrySignature fragments in various order 1`] = `"fragment Bar on User{asd}{user{name...Bar}}"`; exports[`operationRegistrySignature full test 1`] = `"fragment Bar on User{age@skip(if:$a)...Nested}fragment Nested on User{blah}query Foo($a:Boolean,$b:Int){user(age:0,name:\\"\\"){aliased:name tz...Bar...on User{bee hello}}}"`; exports[`operationRegistrySignature test with preserveStringAndNumericLiterals=true 1`] = `"query Foo($b:Int){user(age:5,name:\\"hello\\"){a@skip(if:true)b@include(if:false)c(value:4){d}...Bar...on User{hello@directive(arg:\\"Value!\\")}}}"`; exports[`operationRegistrySignature with various argument types 1`] = `"query OpName($a:[[Boolean!]!],$b:EnumType,$c:Int!){user{name(apple:$a,bag:$b,cat:$c)}}"`; exports[`operationRegistrySignature with various inline types 1`] = `"query OpName{user{name(apple:[[0]],bag:{input:\\"\\"},cat:ENUM_VALUE)}}"`; apollo-server-demo/node_modules/apollo-graphql/src/__tests__/tsconfig.json 0000644 0001750 0000144 00000000156 03560116604 026610 0 ustar andreh users { "extends": "../../tsconfig.test", "include": ["**/*"], "references": [ { "path": "../../" } ] } apollo-server-demo/node_modules/apollo-graphql/src/__tests__/transforms.test.ts 0000644 0001750 0000144 00000003677 03560116604 027640 0 ustar andreh users import { default as gql, disableFragmentWarnings } from "graphql-tag"; import { printWithReducedWhitespace, hideLiterals } from "../transforms"; // The gql duplicate fragment warning feature really is just warnings; nothing // breaks if you turn it off in tests. disableFragmentWarnings(); describe("printWithReducedWhitespace", () => { const cases = [ { name: "lots of whitespace", // Note: there's a tab after "tab->", which prettier wants to keep as a // literal tab rather than \t. In the output, there should be a literal // backslash-t. input: gql` query Foo($a: Int) { user( name: " tab-> yay" other: """ apple bag cat """ ) { name } } `, output: 'query Foo($a:Int){user(name:" tab->\\tyay",other:"apple\\n bag\\ncat"){name}}' } ]; cases.forEach(({ name, input, output }) => { test(name, () => { expect(printWithReducedWhitespace(input)).toEqual(output); }); }); }); describe("hideLiterals", () => { const cases = [ { name: "full test", input: gql` query Foo($b: Int, $a: Boolean) { user(name: "hello", age: 5) { ...Bar ... on User { hello bee } tz aliased: name } } fragment Bar on User { age @skip(if: $a) ...Nested } fragment Nested on User { blah } `, output: 'query Foo($b:Int,$a:Boolean){user(name:"",age:0){...Bar...on User{hello bee}tz aliased:name}}' + "fragment Bar on User{age@skip(if:$a)...Nested}fragment Nested on User{blah}" } ]; cases.forEach(({ name, input, output }) => { test(name, () => { expect(printWithReducedWhitespace(hideLiterals(input))).toEqual(output); }); }); }); apollo-server-demo/node_modules/apollo-graphql/src/schema/ 0000755 0001750 0000144 00000000000 14067647701 023414 5 ustar andreh users apollo-server-demo/node_modules/apollo-graphql/src/schema/__tests__/ 0000755 0001750 0000144 00000000000 14067647700 025351 5 ustar andreh users apollo-server-demo/node_modules/apollo-graphql/src/schema/__tests__/snapshotSerializers/ 0000755 0001750 0000144 00000000000 14067647701 031426 5 ustar andreh users ././@LongLink 0000644 0000000 0000000 00000000162 00000000000 011602 L ustar root root apollo-server-demo/node_modules/apollo-graphql/src/schema/__tests__/snapshotSerializers/selectionSetSerializer.ts apollo-server-demo/node_modules/apollo-graphql/src/schema/__tests__/snapshotSerializers/selectionSet0000644 0001750 0000144 00000001033 03560116604 033774 0 ustar andreh users import { print, SelectionNode, isSelectionNode } from "graphql"; import { Plugin, Config, Refs, Printer } from "pretty-format"; export = (({ test(value: any) { return ( Array.isArray(value) && value.length > 0 && value.every(isSelectionNode) ); }, serialize( value: SelectionNode[], config: Config, indentation: string, depth: number, refs: Refs, printer: Printer ): string { return String(print(value)).replace(",", "\n"); } } as Plugin) as unknown) as jest.SnapshotSerializerPlugin; ././@LongLink 0000644 0000000 0000000 00000000151 00000000000 011600 L ustar root root apollo-server-demo/node_modules/apollo-graphql/src/schema/__tests__/snapshotSerializers/astSerializer.ts apollo-server-demo/node_modules/apollo-graphql/src/schema/__tests__/snapshotSerializers/astSerialize0000644 0001750 0000144 00000001020 03560116604 033766 0 ustar andreh users import { ASTNode, print } from "graphql"; import { Plugin, Config, Refs, Printer } from "pretty-format"; export = (({ test(value: any) { return value && typeof value.kind === "string"; }, serialize( value: ASTNode, config: Config, indentation: string, depth: number, refs: Refs, printer: Printer ): string { return ( indentation + print(value) .trim() .replace(/\n/g, "\n" + indentation) ); } } as Plugin) as unknown) as jest.SnapshotSerializerPlugin; ././@LongLink 0000644 0000000 0000000 00000000161 00000000000 011601 L ustar root root apollo-server-demo/node_modules/apollo-graphql/src/schema/__tests__/snapshotSerializers/graphQLTypeSerializer.ts apollo-server-demo/node_modules/apollo-graphql/src/schema/__tests__/snapshotSerializers/graphQLTypeS0000644 0001750 0000144 00000000715 03560116604 033664 0 ustar andreh users import { isNamedType, GraphQLNamedType, printType } from "graphql"; import { Plugin, Config, Refs, Printer } from "pretty-format"; export = (({ test(value: any) { return value && isNamedType(value); }, serialize( value: GraphQLNamedType, config: Config, indentation: string, depth: number, refs: Refs, printer: Printer ): string { return printType(value); } } as Plugin) as unknown) as jest.SnapshotSerializerPlugin; apollo-server-demo/node_modules/apollo-graphql/src/schema/__tests__/buildSchemaFromSDL.test.ts 0000644 0001750 0000144 00000032422 03560116604 032277 0 ustar andreh users import gql from "graphql-tag"; import { buildSchemaFromSDL } from "../buildSchemaFromSDL"; import { GraphQLSchema, GraphQLDirective, DirectiveLocation, GraphQLObjectType, GraphQLAbstractType, GraphQLScalarType, GraphQLScalarTypeConfig, GraphQLEnumType, Kind, execute, ExecutionResult } from "graphql"; import astSerializer from "./snapshotSerializers/astSerializer"; import graphQLTypeSerializer from "./snapshotSerializers/graphQLTypeSerializer"; import selectionSetSerializer from "./snapshotSerializers/selectionSetSerializer"; expect.addSnapshotSerializer(astSerializer); expect.addSnapshotSerializer(graphQLTypeSerializer); expect.addSnapshotSerializer(selectionSetSerializer); describe("buildSchemaFromSDL", () => { describe(`type definitions`, () => { it(`should construct types from definitions`, () => { const schema = buildSchemaFromSDL( gql` type User { name: String } type Post { title: String } ` ); expect(schema.getType("User")).toMatchInlineSnapshot(` type User { name: String } `); expect(schema.getType("Post")).toMatchInlineSnapshot(` type Post { title: String } `); }); it(`should not allow multiple type definitions with the same name`, () => { expect(() => buildSchemaFromSDL( gql` type User { name: String } type User { title: String } ` ) ).toThrowErrorMatchingInlineSnapshot( `"There can be only one type named \\"User\\"."` ); }); }); describe(`type extension`, () => { it(`should allow extending a type defined in the same document`, () => { const schema = buildSchemaFromSDL( gql` type User { name: String } extend type User { email: String } ` ); expect(schema.getType("User")).toMatchInlineSnapshot(` type User { name: String email: String } `); }); it(`should allow extending a non-existent type`, () => { const schema = buildSchemaFromSDL( gql` extend type User { email: String } ` ); expect(schema.getType("User")).toMatchInlineSnapshot(` type User { email: String } `); }); it.skip(`should report an error when extending a non-existent type`, () => { expect(() => buildSchemaFromSDL( gql` extend type User { email: String } ` ) ).toThrowErrorMatchingInlineSnapshot( `"Cannot extend type \\"User\\" because it is not defined."` ); }); }); describe(`root operation types`, () => { it(`should include a root type with a default type name`, () => { const schema = buildSchemaFromSDL( gql` type Query { rootField: String } ` ); expect(schema.getType("Query")).toMatchInlineSnapshot(` type Query { rootField: String } `); expect(schema.getQueryType()).toEqual(schema.getType("Query")); }); it(`should include a root type with a non-default type name`, () => { const schema = buildSchemaFromSDL( gql` schema { query: Query } type Query { rootField: String } ` ); expect(schema.getType("Query")).toMatchInlineSnapshot(` type Query { rootField: String } `); expect(schema.getQueryType()).toEqual(schema.getType("Query")); }); it(`should include a root type with a non-default type name specified in a schema extension`, () => { const schema = buildSchemaFromSDL( gql` extend schema { query: Query } type Query { rootField: String } ` ); expect(schema.getType("Query")).toMatchInlineSnapshot(` type Query { rootField: String } `); expect(schema.getQueryType()).toEqual(schema.getType("Query")); }); describe(`extending root operation types that aren't defined elsewhere`, () => { it(`should be allowed`, () => { const schema = buildSchemaFromSDL( gql` extend type Query { rootField: String } ` ); expect(schema.getType("Query")).toMatchInlineSnapshot(` type Query { rootField: String } `); expect(schema.getQueryType()).toEqual(schema.getType("Query")); }); it(`should be allowed with a non-default type name`, () => { const schema = buildSchemaFromSDL( gql` schema { query: QueryRoot } extend type QueryRoot { rootField: String } ` ); expect(schema.getType("QueryRoot")).toMatchInlineSnapshot(` type QueryRoot { rootField: String } `); expect(schema.getQueryType()).toEqual(schema.getType("QueryRoot")); }); it(`should be allowed with a non-default name specified in a schema extension`, () => { const schema = buildSchemaFromSDL( gql` schema { query: QueryRoot } type QueryRoot { rootField: String } extend schema { mutation: MutationRoot } extend type MutationRoot { rootField: String } ` ); expect(schema.getType("MutationRoot")).toMatchInlineSnapshot(` type MutationRoot { rootField: String } `); expect(schema.getQueryType()).toEqual(schema.getType("QueryRoot")); expect(schema.getMutationType()).toEqual( schema.getType("MutationRoot") ); }); }); }); describe(`directives`, () => { it(`should construct directives from definitions`, () => { const schema = buildSchemaFromSDL( gql` directive @something on FIELD_DEFINITION directive @another on FIELD_DEFINITION ` ); expect(schema.getDirective("something")).toMatchInlineSnapshot( `"@something"` ); expect(schema.getDirective("another")).toMatchInlineSnapshot( `"@another"` ); }); it(`should not allow multiple directive definitions with the same name`, () => { expect(() => buildSchemaFromSDL( gql` directive @something on FIELD_DEFINITION directive @something on FIELD_DEFINITION ` ) ).toThrowErrorMatchingInlineSnapshot( `"There can be only one directive named \\"@something\\"."` ); }); it(`should not allow a directive definition with the same name as a predefined schema directive`, () => { expect(() => buildSchemaFromSDL( gql` directive @something on FIELD_DEFINITION `, new GraphQLSchema({ query: undefined, directives: [ new GraphQLDirective({ name: "something", locations: [DirectiveLocation.FIELD_DEFINITION] }) ] }) ) ).toThrowErrorMatchingInlineSnapshot( `"Directive \\"@something\\" already exists in the schema. It cannot be redefined."` ); }); it(`should allow predefined schema directives to be used`, () => { const schema = buildSchemaFromSDL( gql` type User { name: String @something } `, new GraphQLSchema({ query: undefined, directives: [ new GraphQLDirective({ name: "something", locations: [DirectiveLocation.FIELD_DEFINITION] }) ] }) ); }); it(`should allow schema directives to be used in the same document they are defined in`, () => { const schema = buildSchemaFromSDL( gql` directive @something on FIELD_DEFINITION type User { name: String @something } ` ); }); it(`should report an error for unknown schema directives`, () => { expect(() => buildSchemaFromSDL( gql` type User { name: String @something } ` ) ).toThrowErrorMatchingInlineSnapshot( `"Unknown directive \\"@something\\"."` ); }); }); describe(`resolvers`, () => { it(`should add a resolver for a field`, () => { const name = () => {}; const schema = buildSchemaFromSDL([ { typeDefs: gql` type User { name: String } `, resolvers: { User: { name } } } ]); const userType = schema.getType("User"); expect(userType).toBeDefined(); const nameField = (userType! as GraphQLObjectType).getFields()["name"]; expect(nameField).toBeDefined(); expect(nameField.resolve).toEqual(name); }); it(`should add meta fields to abstract types`, () => { const typeDefs = gql` union Animal = Dog interface Creature { name: String! legs: Int! } type Dog { id: ID! } type Cat implements Creature { meow: Boolean! } `; const resolveTypeUnion = (obj: any) => { return "Dog"; }; const resolveTypeInterface = (obj: any) => { if (obj.meow) { return "Cat"; } throw Error("Couldn't resolve interface"); }; const resolvers = { Animal: { __resolveType: resolveTypeUnion }, Creature: { __resolveType: resolveTypeInterface } }; const schema = buildSchemaFromSDL([{ typeDefs, resolvers }]); const animalUnion = schema.getType("Animal") as GraphQLAbstractType; const creatureInterface = schema.getType( "Creature" ) as GraphQLAbstractType; expect(animalUnion.resolveType).toBe(resolveTypeUnion); expect(creatureInterface.resolveType).toBe(resolveTypeInterface); }); it(`should add resolvers for scalar types`, () => { const typeDefs = gql` scalar Custom `; const customTypeConfig: GraphQLScalarTypeConfig<string, string> = { name: "Custom", serialize: value => value, parseValue: value => value, parseLiteral: input => { if (input.kind !== Kind.STRING) { throw new Error("Expected value to be string"); } return input.value; } }; const CustomType = new GraphQLScalarType(customTypeConfig); const resolvers = { Custom: CustomType }; const schema = buildSchemaFromSDL([{ typeDefs, resolvers }]); const custom = schema.getType("Custom") as GraphQLScalarType; expect(custom.parseLiteral).toBe(CustomType.parseLiteral); expect(custom.parseValue).toBe(CustomType.parseValue); expect(custom.serialize).toBe(CustomType.serialize); }); it(`should add resolvers to enum types`, () => { const typeDefs = gql` enum AllowedColor { RED GREEN BLUE } type Query { favoriteColor: AllowedColor avatar(borderColor: AllowedColor): String } `; const mockResolver = jest.fn(); const resolvers = { AllowedColor: { RED: "#f00", GREEN: "#0f0", BLUE: "#00f" }, Query: { favoriteColor: () => "#f00", avatar: (_: any, params: any) => mockResolver(_, params) } }; const schema = buildSchemaFromSDL([{ typeDefs, resolvers }]); const colorEnum = schema.getType("AllowedColor") as GraphQLEnumType; let result = execute( schema, gql` query { favoriteColor avatar(borderColor: RED) } ` ); expect((result as ExecutionResult).data!.favoriteColor).toBe("RED"); expect(colorEnum.getValue("RED")!.value).toBe("#f00"); expect(mockResolver).toBeCalledWith(undefined, { borderColor: "#f00" }); }); it(`should add resolvers to enum types with 0 value`, () => { const typeDefs = gql` enum CustomerType { EXISTING NEW } type Query { existingCustomer: CustomerType newCustomer: CustomerType } `; const resolvers = { CustomerType: { EXISTING: 0, NEW: 1 }, Query: { existingCustomer: () => 0, newCustomer: () => 1 } }; const schema = buildSchemaFromSDL([{ typeDefs, resolvers }]); const customerTypeEnum = schema.getType( "CustomerType" ) as GraphQLEnumType; let result = execute( schema, gql` query { existingCustomer newCustomer } ` ); expect((result as ExecutionResult).data!.existingCustomer).toBe( "EXISTING" ); expect(customerTypeEnum.getValue("EXISTING")!.value).toBe(0); expect((result as ExecutionResult).data!.newCustomer).toBe("NEW"); expect(customerTypeEnum.getValue("NEW")!.value).toBe(1); }); }); }); apollo-server-demo/node_modules/apollo-graphql/src/schema/__tests__/tsconfig.json 0000644 0001750 0000144 00000000165 03560116604 030050 0 ustar andreh users { "extends": "../../../tsconfig.test", "include": ["**/*"], "references": [ { "path": "../../../" }, ] } apollo-server-demo/node_modules/apollo-graphql/src/schema/buildSchemaFromSDL.ts 0000644 0001750 0000144 00000020373 03560116604 027365 0 ustar andreh users import { concatAST, DocumentNode, extendSchema, GraphQLSchema, isObjectType, isTypeDefinitionNode, isTypeExtensionNode, Kind, TypeDefinitionNode, TypeExtensionNode, DirectiveDefinitionNode, SchemaDefinitionNode, SchemaExtensionNode, OperationTypeNode, GraphQLObjectType, GraphQLEnumType, isAbstractType, isScalarType, isEnumType, GraphQLEnumValueConfig } from "graphql"; import { validateSDL } from "graphql/validation/validate"; import { isDocumentNode, isNode } from "../utilities/graphql"; import { GraphQLResolverMap } from "./resolverMap"; import { GraphQLSchemaValidationError } from "./GraphQLSchemaValidationError"; import { specifiedSDLRules } from "graphql/validation/specifiedRules"; import { KnownTypeNamesRule, UniqueDirectivesPerLocationRule, ValidationRule } from "graphql/validation"; import { mapValues, isNotNullOrUndefined } from "apollo-env"; export interface GraphQLSchemaModule { typeDefs: DocumentNode; resolvers?: GraphQLResolverMap<any>; } const skippedSDLRules: ValidationRule[] = [ KnownTypeNamesRule, UniqueDirectivesPerLocationRule ]; // BREAKING VERSION: Remove this when graphql-js 15 is minimum version. // Currently, this PossibleTypeExtensions rule is experimental and thus not // exposed directly from the rules module above. This may change in the future! // Additionally, it does not exist in prior graphql versions. Thus this try/catch. try { const PossibleTypeExtensions: typeof import("graphql/validation/rules/PossibleTypeExtensions").PossibleTypeExtensions = require("graphql/validation/rules/PossibleTypeExtensions") .PossibleTypeExtensions; if (PossibleTypeExtensions) { skippedSDLRules.push(PossibleTypeExtensions); } } catch (e) { // No need to fail in this case. Instead, if this validation rule is missing, we will assume its not used // by the version of `graphql` that is available to us. } const sdlRules = specifiedSDLRules.filter( rule => !skippedSDLRules.includes(rule) ); export function modulesFromSDL( modulesOrSDL: (GraphQLSchemaModule | DocumentNode)[] | DocumentNode ): GraphQLSchemaModule[] { if (Array.isArray(modulesOrSDL)) { return modulesOrSDL.map(moduleOrSDL => { if (isNode(moduleOrSDL) && isDocumentNode(moduleOrSDL)) { return { typeDefs: moduleOrSDL }; } else { return moduleOrSDL; } }); } else { return [{ typeDefs: modulesOrSDL }]; } } export function buildSchemaFromSDL( modulesOrSDL: (GraphQLSchemaModule | DocumentNode)[] | DocumentNode, schemaToExtend?: GraphQLSchema ): GraphQLSchema { const modules = modulesFromSDL(modulesOrSDL); const documentAST = concatAST(modules.map(module => module.typeDefs)); const errors = validateSDL(documentAST, schemaToExtend, sdlRules); if (errors.length > 0) { throw new GraphQLSchemaValidationError(errors); } const definitionsMap: { [name: string]: TypeDefinitionNode[]; } = Object.create(null); const extensionsMap: { [name: string]: TypeExtensionNode[]; } = Object.create(null); const directiveDefinitions: DirectiveDefinitionNode[] = []; const schemaDefinitions: SchemaDefinitionNode[] = []; const schemaExtensions: SchemaExtensionNode[] = []; for (const definition of documentAST.definitions) { if (isTypeDefinitionNode(definition)) { const typeName = definition.name.value; if (definitionsMap[typeName]) { definitionsMap[typeName].push(definition); } else { definitionsMap[typeName] = [definition]; } } else if (isTypeExtensionNode(definition)) { const typeName = definition.name.value; if (extensionsMap[typeName]) { extensionsMap[typeName].push(definition); } else { extensionsMap[typeName] = [definition]; } } else if (definition.kind === Kind.DIRECTIVE_DEFINITION) { directiveDefinitions.push(definition); } else if (definition.kind === Kind.SCHEMA_DEFINITION) { schemaDefinitions.push(definition); } else if (definition.kind === Kind.SCHEMA_EXTENSION) { schemaExtensions.push(definition); } } let schema = schemaToExtend ? schemaToExtend : new GraphQLSchema({ query: undefined }); const missingTypeDefinitions: TypeDefinitionNode[] = []; for (const [extendedTypeName, extensions] of Object.entries(extensionsMap)) { if (!definitionsMap[extendedTypeName]) { const extension = extensions[0]; const kind = extension.kind; const definition = { kind: extKindToDefKind[kind], name: extension.name } as TypeDefinitionNode; missingTypeDefinitions.push(definition); } } schema = extendSchema( schema, { kind: Kind.DOCUMENT, definitions: [ ...Object.values(definitionsMap).flat(), ...missingTypeDefinitions, ...directiveDefinitions ] }, { assumeValidSDL: true } ); schema = extendSchema( schema, { kind: Kind.DOCUMENT, definitions: Object.values(extensionsMap).flat() }, { assumeValidSDL: true } ); let operationTypeMap: { [operation in OperationTypeNode]?: string }; if (schemaDefinitions.length > 0 || schemaExtensions.length > 0) { operationTypeMap = {}; const operationTypes = [...schemaDefinitions, ...schemaExtensions] .map(node => node.operationTypes) .filter(isNotNullOrUndefined) .flat(); for (const { operation, type } of operationTypes) { operationTypeMap[operation] = type.name.value; } } else { operationTypeMap = { query: "Query", mutation: "Mutation", subscription: "Subscription" }; } schema = new GraphQLSchema({ ...schema.toConfig(), ...mapValues(operationTypeMap, typeName => typeName ? (schema.getType(typeName) as GraphQLObjectType<any, any>) : undefined ) }); for (const module of modules) { if (!module.resolvers) continue; addResolversToSchema(schema, module.resolvers); } return schema; } const extKindToDefKind = { [Kind.SCALAR_TYPE_EXTENSION]: Kind.SCALAR_TYPE_DEFINITION, [Kind.OBJECT_TYPE_EXTENSION]: Kind.OBJECT_TYPE_DEFINITION, [Kind.INTERFACE_TYPE_EXTENSION]: Kind.INTERFACE_TYPE_DEFINITION, [Kind.UNION_TYPE_EXTENSION]: Kind.UNION_TYPE_DEFINITION, [Kind.ENUM_TYPE_EXTENSION]: Kind.ENUM_TYPE_DEFINITION, [Kind.INPUT_OBJECT_TYPE_EXTENSION]: Kind.INPUT_OBJECT_TYPE_DEFINITION }; export function addResolversToSchema( schema: GraphQLSchema, resolvers: GraphQLResolverMap<any> ) { for (const [typeName, fieldConfigs] of Object.entries(resolvers)) { const type = schema.getType(typeName); if (isAbstractType(type)) { for (const [fieldName, fieldConfig] of Object.entries(fieldConfigs)) { if (fieldName.startsWith("__")) { (type as any)[fieldName.substring(2)] = fieldConfig; } } } if (isScalarType(type)) { for (const fn in fieldConfigs) { (type as any)[fn] = (fieldConfigs as any)[fn]; } } if (isEnumType(type)) { const values = type.getValues(); const newValues: { [key: string]: GraphQLEnumValueConfig } = {}; values.forEach(value => { let newValue = (fieldConfigs as any)[value.name]; if (newValue === undefined) { newValue = value.name; } newValues[value.name] = { value: newValue, deprecationReason: value.deprecationReason, description: value.description, astNode: value.astNode, extensions: undefined }; }); // In place updating hack to get around pulling in the full // schema walking and immutable updating machinery from graphql-tools Object.assign( type, new GraphQLEnumType({ ...type.toConfig(), values: newValues }) ); } if (!isObjectType(type)) continue; const fieldMap = type.getFields(); for (const [fieldName, fieldConfig] of Object.entries(fieldConfigs)) { if (fieldName.startsWith("__")) { (type as any)[fieldName.substring(2)] = fieldConfig; continue; } const field = fieldMap[fieldName]; if (!field) continue; if (typeof fieldConfig === "function") { field.resolve = fieldConfig; } else { field.resolve = fieldConfig.resolve; } } } } apollo-server-demo/node_modules/apollo-graphql/src/schema/transformSchema.ts 0000644 0001750 0000144 00000010034 03560116604 027103 0 ustar andreh users import { GraphQLSchema, GraphQLNamedType, isIntrospectionType, isObjectType, GraphQLObjectType, GraphQLType, isListType, GraphQLList, isNonNullType, GraphQLNonNull, GraphQLFieldConfigMap, GraphQLFieldConfigArgumentMap, GraphQLOutputType, GraphQLInputType, isInterfaceType, GraphQLInterfaceType, isUnionType, GraphQLUnionType, isInputObjectType, GraphQLInputObjectType, GraphQLInputFieldConfigMap } from "graphql"; import { mapValues } from "apollo-env"; type TypeTransformer = ( type: GraphQLNamedType ) => GraphQLNamedType | null | undefined; export function transformSchema( schema: GraphQLSchema, transformType: TypeTransformer ): GraphQLSchema { const typeMap: { [typeName: string]: GraphQLNamedType } = Object.create(null); for (const oldType of Object.values(schema.getTypeMap())) { if (isIntrospectionType(oldType)) continue; const result = transformType(oldType); // Returning `null` removes the type. if (result === null) continue; // Returning `undefined` keeps the old type. const newType = result || oldType; typeMap[newType.name] = recreateNamedType(newType); } const schemaConfig = schema.toConfig(); return new GraphQLSchema({ ...schemaConfig, types: Object.values(typeMap), query: replaceMaybeType(schemaConfig.query), mutation: replaceMaybeType(schemaConfig.mutation), subscription: replaceMaybeType(schemaConfig.subscription) }); function recreateNamedType(type: GraphQLNamedType): GraphQLNamedType { if (isObjectType(type)) { const config = type.toConfig(); return new GraphQLObjectType({ ...config, interfaces: () => config.interfaces.map(replaceNamedType), fields: () => replaceFields(config.fields) }); } else if (isInterfaceType(type)) { const config = type.toConfig(); return new GraphQLInterfaceType({ ...config, fields: () => replaceFields(config.fields) }); } else if (isUnionType(type)) { const config = type.toConfig(); return new GraphQLUnionType({ ...config, types: () => config.types.map(replaceNamedType) }); } else if (isInputObjectType(type)) { const config = type.toConfig(); return new GraphQLInputObjectType({ ...config, fields: () => replaceInputFields(config.fields) }); } return type; } function replaceType<T extends GraphQLType>( type: GraphQLList<T> ): GraphQLList<T>; function replaceType<T extends GraphQLType>( type: GraphQLNonNull<T> ): GraphQLNonNull<T>; function replaceType(type: GraphQLNamedType): GraphQLNamedType; function replaceType(type: GraphQLOutputType): GraphQLOutputType; function replaceType(type: GraphQLInputType): GraphQLInputType; function replaceType(type: GraphQLType): GraphQLType { if (isListType(type)) { return new GraphQLList(replaceType(type.ofType)); } else if (isNonNullType(type)) { return new GraphQLNonNull(replaceType(type.ofType)); } return replaceNamedType(type); } function replaceNamedType<T extends GraphQLNamedType>(type: T): T { const newType = typeMap[type.name] as T; return newType ? newType : type; } function replaceMaybeType<T extends GraphQLNamedType>( type: T | null | undefined ): T | undefined { return type ? replaceNamedType(type) : undefined; } function replaceFields<TSource, TContext>( fieldsMap: GraphQLFieldConfigMap<TSource, TContext> ): GraphQLFieldConfigMap<TSource, TContext> { return mapValues(fieldsMap, field => ({ ...field, type: replaceType(field.type), args: field.args ? replaceArgs(field.args) : undefined })); } function replaceInputFields( fieldsMap: GraphQLInputFieldConfigMap ): GraphQLInputFieldConfigMap { return mapValues(fieldsMap, field => ({ ...field, type: replaceType(field.type) })); } function replaceArgs(args: GraphQLFieldConfigArgumentMap) { return mapValues(args, arg => ({ ...arg, type: replaceType(arg.type) })); } } apollo-server-demo/node_modules/apollo-graphql/src/schema/resolveObject.ts 0000644 0001750 0000144 00000000767 03560116604 026571 0 ustar andreh users import { GraphQLResolveInfo, FieldNode } from "graphql"; export type GraphQLObjectResolver<TSource, TContext> = ( source: TSource, fields: Record<string, ReadonlyArray<FieldNode>>, context: TContext, info: GraphQLResolveInfo ) => any; declare module "graphql/type/definition" { interface GraphQLObjectType { resolveObject?: GraphQLObjectResolver<any, any>; } interface GraphQLObjectTypeConfig<TSource, TContext> { resolveObject?: GraphQLObjectResolver<TSource, TContext>; } } apollo-server-demo/node_modules/apollo-graphql/src/schema/index.ts 0000644 0001750 0000144 00000000271 03560116604 025060 0 ustar andreh users export * from "./buildSchemaFromSDL"; export * from "./GraphQLSchemaValidationError"; export * from "./transformSchema"; export * from "./resolverMap"; export * from "./resolveObject"; apollo-server-demo/node_modules/apollo-graphql/src/schema/resolverMap.ts 0000644 0001750 0000144 00000000677 03560116604 026262 0 ustar andreh users import { GraphQLFieldResolver, GraphQLScalarType } from "graphql"; export interface GraphQLResolverMap<TContext = {}> { [typeName: string]: | { [fieldName: string]: | GraphQLFieldResolver<any, TContext> | { requires?: string; resolve: GraphQLFieldResolver<any, TContext>; }; } | GraphQLScalarType | { [enumValue: string]: string | number; }; } apollo-server-demo/node_modules/apollo-graphql/src/schema/GraphQLSchemaValidationError.ts 0000644 0001750 0000144 00000000523 03560116604 031415 0 ustar andreh users import { GraphQLError } from "graphql"; export class GraphQLSchemaValidationError extends Error { constructor(public errors: ReadonlyArray<GraphQLError>) { super(); this.name = this.constructor.name; Error.captureStackTrace(this, this.constructor); this.message = errors.map(error => error.message).join("\n\n"); } } apollo-server-demo/node_modules/apollo-graphql/src/utilities/ 0000755 0001750 0000144 00000000000 14067647700 024166 5 ustar andreh users apollo-server-demo/node_modules/apollo-graphql/src/utilities/graphql.ts 0000644 0001750 0000144 00000000462 03560116604 026164 0 ustar andreh users import { ASTNode, DocumentNode, Kind } from "graphql"; export function isNode(maybeNode: any): maybeNode is ASTNode { return maybeNode && typeof maybeNode.kind === "string"; } export function isDocumentNode(node: ASTNode): node is DocumentNode { return isNode(node) && node.kind === Kind.DOCUMENT; } apollo-server-demo/node_modules/apollo-graphql/src/operationId.ts 0000644 0001750 0000144 00000011733 03560116604 024773 0 ustar andreh users // In Apollo Studio, we want to group requests making the same query together, // and treat different queries distinctly. But what does it mean for two queries // to be "the same"? And what if you don't want to send the full text of the // query to Apollo's servers, either because it contains sensitive data // or because it contains extraneous operations or fragments? // // To solve these problems, ApolloServerPluginUsageReporting has the concept of // "signatures". We don't (by default) send the full query string of queries to // Apollo's servers. Instead, each trace has its query string's "signature". // // You can technically specify any function mapping a GraphQL query AST // (DocumentNode) to string as your signature algorithm by providing it as the // 'calculateSignature' option to ApolloServerPluginUsageReporting. (This option // is not recommended, because Apollo's servers make some assumptions about the // semantics of your operation based on the signature.) This file defines the // default function used for this purpose: defaultUsageReportingSignature // (formerly known as defaultEngineReportingSignature). // // This module utilizes several AST transformations from the adjacent // 'transforms' module (which are also for writing your own signature method). // - dropUnusedDefinitions, which removes operations and fragments that // aren't going to be used in execution // - hideLiterals, which replaces all numeric and string literals as well // as list and object input values with "empty" values // - removeAliases, which removes field aliasing from the query // - sortAST, which sorts the children of most multi-child nodes // consistently // - printWithReducedWhitespace, a variant on graphql-js's 'print' // which gets rid of unneeded whitespace // // defaultUsageReportingSignature consists of applying all of these building // blocks. // // Historical note: the default signature algorithm of the Go engineproxy // performed all of the above operations, and Apollo's servers then re-ran a // mostly identical signature implementation on received traces. This was // primarily to deal with edge cases where some users used literal interpolation // instead of GraphQL variables, included randomized alias names, etc. In // addition, the servers relied on the fact that dropUnusedDefinitions had been // called in order (and that the signature could be parsed as GraphQL) to // extract the name of the operation for display. This caused confusion, as the // query document shown in the Studio UI wasn't the same as the one actually // sent. ApolloServerPluginUsageReporting (previously apollo-engine-reporting) // uses a reporting API which requires it to explicitly include the operation // name with each signature; this means that the server no longer needs to parse // the signature or run its own signature algorithm on it, and the details of // the signature algorithm are now up to the reporting agent. That said, not all // Studio features will work properly if your signature function changes the // signature in unexpected ways. // // This file also exports operationRegistrySignature and // defaultOperationRegistrySignature, which are slightly different normalization // functions used in other contextes. import { DocumentNode } from "graphql"; import { createHash } from "apollo-env"; import { printWithReducedWhitespace, dropUnusedDefinitions, sortAST, hideStringAndNumericLiterals, removeAliases, hideLiterals } from "./transforms"; // The usage reporting signature function consists of removing extra whitespace, // sorting the AST in a deterministic manner, hiding literals, and removing // unused definitions. export function defaultUsageReportingSignature( ast: DocumentNode, operationName: string ): string { return printWithReducedWhitespace( sortAST( removeAliases(hideLiterals(dropUnusedDefinitions(ast, operationName))) ) ); } // The operation registry signature function consists of removing extra whitespace, // sorting the AST in a deterministic manner, potentially hiding string and numeric // literals, and removing unused definitions. This is a less aggressive transform // than its usage reporting signature counterpart. export function operationRegistrySignature( ast: DocumentNode, operationName: string, options: { preserveStringAndNumericLiterals: boolean } = { preserveStringAndNumericLiterals: false } ): string { const withoutUnusedDefs = dropUnusedDefinitions(ast, operationName); const maybeWithLiterals = options.preserveStringAndNumericLiterals ? withoutUnusedDefs : hideStringAndNumericLiterals(withoutUnusedDefs); return printWithReducedWhitespace(sortAST(maybeWithLiterals)); } export function defaultOperationRegistrySignature( ast: DocumentNode, operationName: string ): string { return operationRegistrySignature(ast, operationName, { preserveStringAndNumericLiterals: false }); } export function operationHash(operation: string): string { return createHash("sha256") .update(operation) .digest("hex"); } apollo-server-demo/node_modules/apollo-graphql/src/index.ts 0000644 0001750 0000144 00000000435 03560116604 023622 0 ustar andreh users export { defaultOperationRegistrySignature, defaultUsageReportingSignature, operationRegistrySignature, operationHash, // deprecated name for this function: defaultUsageReportingSignature as defaultEngineReportingSignature } from "./operationId"; export * from "./schema"; apollo-server-demo/node_modules/apollo-graphql/src/transforms.ts 0000644 0001750 0000144 00000016651 03560116604 024720 0 ustar andreh users import { visit } from "graphql/language/visitor"; import { DocumentNode, FloatValueNode, IntValueNode, StringValueNode, OperationDefinitionNode, SelectionSetNode, FragmentSpreadNode, InlineFragmentNode, DirectiveNode, FieldNode, FragmentDefinitionNode, ObjectValueNode, ListValueNode } from "graphql/language/ast"; import { print } from "graphql/language/printer"; import { separateOperations } from "graphql/utilities"; // We'll only fetch the `ListIteratee` type from the `@types/lodash`, but get // `sortBy` from the modularized version of the package to avoid bringing in // all of `lodash`. import { ListIteratee } from "lodash"; import sortBy from "lodash.sortby"; // Replace numeric, string, list, and object literals with "empty" // values. Leaves enums alone (since there's no consistent "zero" enum). This // can help combine similar queries if you substitute values directly into // queries rather than use GraphQL variables, and can hide sensitive data in // your query (say, a hardcoded API key) from Apollo's servers, but in general // avoiding those situations is better than working around them. export function hideLiterals(ast: DocumentNode): DocumentNode { return visit(ast, { IntValue(node: IntValueNode): IntValueNode { return { ...node, value: "0" }; }, FloatValue(node: FloatValueNode): FloatValueNode { return { ...node, value: "0" }; }, StringValue(node: StringValueNode): StringValueNode { return { ...node, value: "", block: false }; }, ListValue(node: ListValueNode): ListValueNode { return { ...node, values: [] }; }, ObjectValue(node: ObjectValueNode): ObjectValueNode { return { ...node, fields: [] }; } }); } // In the same spirit as the similarly named `hideLiterals` function, only // hide string and numeric literals. export function hideStringAndNumericLiterals(ast: DocumentNode): DocumentNode { return visit(ast, { IntValue(node: IntValueNode): IntValueNode { return { ...node, value: "0" }; }, FloatValue(node: FloatValueNode): FloatValueNode { return { ...node, value: "0" }; }, StringValue(node: StringValueNode): StringValueNode { return { ...node, value: "", block: false }; } }); } // A GraphQL query may contain multiple named operations, with the operation to // use specified separately by the client. This transformation drops unused // operations from the query, as well as any fragment definitions that are not // referenced. (In general we recommend that unused definitions are dropped on // the client before sending to the server to save bandwidth and parsing time.) export function dropUnusedDefinitions( ast: DocumentNode, operationName: string ): DocumentNode { const separated = separateOperations(ast)[operationName]; if (!separated) { // If the given operationName isn't found, just make this whole transform a // no-op instead of crashing. return ast; } return separated; } // Like lodash's sortBy, but sorted(undefined) === undefined rather than []. It // is a stable non-in-place sort. function sorted<T>( items: ReadonlyArray<T> | undefined, ...iteratees: Array<ListIteratee<T>> ): Array<T> | undefined { if (items) { return sortBy(items, ...iteratees); } return undefined; } // sortAST sorts most multi-child nodes alphabetically. Using this as part of // your signature calculation function may make it easier to tell the difference // between queries that are similar to each other, and if for some reason your // GraphQL client generates query strings with elements in nondeterministic // order, it can make sure the queries are treated as identical. export function sortAST(ast: DocumentNode): DocumentNode { return visit(ast, { Document(node: DocumentNode) { return { ...node, // Use sortBy because 'definitions' is not optional. // The sort on "kind" places fragments before operations within the document definitions: sortBy(node.definitions, "kind", "name.value") }; }, OperationDefinition( node: OperationDefinitionNode ): OperationDefinitionNode { return { ...node, variableDefinitions: sorted( node.variableDefinitions, "variable.name.value" ) }; }, SelectionSet(node: SelectionSetNode): SelectionSetNode { return { ...node, // Define an ordering for field names in a SelectionSet. Field first, // then FragmentSpread, then InlineFragment. By a lovely coincidence, // the order we want them to appear in is alphabetical by node.kind. // Use sortBy instead of sorted because 'selections' is not optional. selections: sortBy(node.selections, "kind", "name.value") }; }, Field(node: FieldNode): FieldNode { return { ...node, arguments: sorted(node.arguments, "name.value") }; }, FragmentSpread(node: FragmentSpreadNode): FragmentSpreadNode { return { ...node, directives: sorted(node.directives, "name.value") }; }, InlineFragment(node: InlineFragmentNode): InlineFragmentNode { return { ...node, directives: sorted(node.directives, "name.value") }; }, FragmentDefinition(node: FragmentDefinitionNode): FragmentDefinitionNode { return { ...node, directives: sorted(node.directives, "name.value"), variableDefinitions: sorted( node.variableDefinitions, "variable.name.value" ) }; }, Directive(node: DirectiveNode): DirectiveNode { return { ...node, arguments: sorted(node.arguments, "name.value") }; } }); } // removeAliases gets rid of GraphQL aliases, a feature by which you can tell a // server to return a field's data under a different name from the field // name. Maybe this is useful if somebody somewhere inserts random aliases into // their queries. export function removeAliases(ast: DocumentNode): DocumentNode { return visit(ast, { Field(node: FieldNode): FieldNode { return { ...node, alias: undefined }; } }); } // Like the graphql-js print function, but deleting whitespace wherever // feasible. Specifically, all whitespace (outside of string literals) is // reduced to at most one space, and even that space is removed anywhere except // for between two alphanumerics. export function printWithReducedWhitespace(ast: DocumentNode): string { // In a GraphQL AST (which notably does not contain comments), the only place // where meaningful whitespace (or double quotes) can exist is in // StringNodes. So to print with reduced whitespace, we: // - temporarily sanitize strings by replacing their contents with hex // - use the default GraphQL printer // - minimize the whitespace with a simple regexp replacement // - convert strings back to their actual value // We normalize all strings to non-block strings for simplicity. const sanitizedAST = visit(ast, { StringValue(node: StringValueNode): StringValueNode { return { ...node, value: Buffer.from(node.value, "utf8").toString("hex"), block: false }; } }); const withWhitespace = print(sanitizedAST); const minimizedButStillHex = withWhitespace .replace(/\s+/g, " ") .replace(/([^_a-zA-Z0-9]) /g, (_, c) => c) .replace(/ ([^_a-zA-Z0-9])/g, (_, c) => c); return minimizedButStillHex.replace(/"([a-f0-9]+)"/g, (_, hex) => JSON.stringify(Buffer.from(hex, "hex").toString("utf8")) ); } apollo-server-demo/node_modules/apollo-graphql/README.md 0000644 0001750 0000144 00000000023 03560116604 022624 0 ustar andreh users # `apollo-graphql` apollo-server-demo/node_modules/apollo-graphql/package.json 0000644 0001750 0000144 00000002370 03560116604 023642 0 ustar andreh users { "name": "apollo-graphql", "version": "0.6.0", "description": "Apollo GraphQL utility library", "main": "lib/index.js", "types": "lib/index.d.ts", "keywords": [], "author": "Apollo <opensource@apollographql.com>", "license": "MIT", "engines": { "node": ">=6" }, "dependencies": { "apollo-env": "^0.6.5", "lodash.sortby": "^4.7.0" }, "peerDependencies": { "graphql": "^14.2.1 || ^15.0.0" }, "jest": { "preset": "ts-jest", "transformIgnorePatterns": [ "/node_modules/" ], "testEnvironment": "node", "testMatch": [ "**/__tests__/*.(js|ts)" ], "testPathIgnorePatterns": [ "<rootDir>/node_modules/", "<rootDir>/lib/", "<rootDir>/test/fixtures/", "<rootDir>/test/test-utils" ], "moduleFileExtensions": [ "ts", "js" ], "globals": { "ts-jest": { "tsConfig": "<rootDir>/tsconfig.test.json", "diagnostics": false } } }, "gitHead": "05b6a0d776cf91df3cc402d09e2007220c5b4a25" ,"_resolved": "https://registry.npmjs.org/apollo-graphql/-/apollo-graphql-0.6.0.tgz" ,"_integrity": "sha512-BxTf5LOQe649e9BNTPdyCGItVv4Ll8wZ2BKnmiYpRAocYEXAVrQPWuSr3dO4iipqAU8X0gvle/Xu9mSqg5b7Qg==" ,"_from": "apollo-graphql@0.6.0" } apollo-server-demo/node_modules/apollo-graphql/CHANGELOG.md 0000644 0001750 0000144 00000000031 03560116604 023155 0 ustar andreh users # Change Log ### vNEXT apollo-server-demo/node_modules/apollo-graphql/lib/ 0000755 0001750 0000144 00000000000 14067647701 022133 5 ustar andreh users apollo-server-demo/node_modules/apollo-graphql/lib/operationId.js 0000644 0001750 0000144 00000002731 03560116604 024736 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); const apollo_env_1 = require("apollo-env"); const transforms_1 = require("./transforms"); function defaultUsageReportingSignature(ast, operationName) { return transforms_1.printWithReducedWhitespace(transforms_1.sortAST(transforms_1.removeAliases(transforms_1.hideLiterals(transforms_1.dropUnusedDefinitions(ast, operationName))))); } exports.defaultUsageReportingSignature = defaultUsageReportingSignature; function operationRegistrySignature(ast, operationName, options = { preserveStringAndNumericLiterals: false }) { const withoutUnusedDefs = transforms_1.dropUnusedDefinitions(ast, operationName); const maybeWithLiterals = options.preserveStringAndNumericLiterals ? withoutUnusedDefs : transforms_1.hideStringAndNumericLiterals(withoutUnusedDefs); return transforms_1.printWithReducedWhitespace(transforms_1.sortAST(maybeWithLiterals)); } exports.operationRegistrySignature = operationRegistrySignature; function defaultOperationRegistrySignature(ast, operationName) { return operationRegistrySignature(ast, operationName, { preserveStringAndNumericLiterals: false }); } exports.defaultOperationRegistrySignature = defaultOperationRegistrySignature; function operationHash(operation) { return apollo_env_1.createHash("sha256") .update(operation) .digest("hex"); } exports.operationHash = operationHash; //# sourceMappingURL=operationId.js.map apollo-server-demo/node_modules/apollo-graphql/lib/index.js 0000644 0001750 0000144 00000001254 03560116604 023567 0 ustar andreh users "use strict"; function __export(m) { for (var p in m) if (!exports.hasOwnProperty(p)) exports[p] = m[p]; } Object.defineProperty(exports, "__esModule", { value: true }); var operationId_1 = require("./operationId"); exports.defaultOperationRegistrySignature = operationId_1.defaultOperationRegistrySignature; exports.defaultUsageReportingSignature = operationId_1.defaultUsageReportingSignature; exports.operationRegistrySignature = operationId_1.operationRegistrySignature; exports.operationHash = operationId_1.operationHash; exports.defaultEngineReportingSignature = operationId_1.defaultUsageReportingSignature; __export(require("./schema")); //# sourceMappingURL=index.js.map apollo-server-demo/node_modules/apollo-graphql/lib/transforms.js.map 0000644 0001750 0000144 00000006337 03560116604 025441 0 ustar andreh users {"version":3,"file":"transforms.js","sourceRoot":"","sources":["../src/transforms.ts"],"names":[],"mappings":";;;;;AAAA,sDAAiD;AAgBjD,sDAAiD;AACjD,iDAAuD;AAKvD,kEAAmC;AAQnC,SAAgB,YAAY,CAAC,GAAiB;IAC5C,OAAO,eAAK,CAAC,GAAG,EAAE;QAChB,QAAQ,CAAC,IAAkB;YACzB,uCAAY,IAAI,KAAE,KAAK,EAAE,GAAG,IAAG;QACjC,CAAC;QACD,UAAU,CAAC,IAAoB;YAC7B,uCAAY,IAAI,KAAE,KAAK,EAAE,GAAG,IAAG;QACjC,CAAC;QACD,WAAW,CAAC,IAAqB;YAC/B,uCAAY,IAAI,KAAE,KAAK,EAAE,EAAE,EAAE,KAAK,EAAE,KAAK,IAAG;QAC9C,CAAC;QACD,SAAS,CAAC,IAAmB;YAC3B,uCAAY,IAAI,KAAE,MAAM,EAAE,EAAE,IAAG;QACjC,CAAC;QACD,WAAW,CAAC,IAAqB;YAC/B,uCAAY,IAAI,KAAE,MAAM,EAAE,EAAE,IAAG;QACjC,CAAC;KACF,CAAC,CAAC;AACL,CAAC;AAlBD,oCAkBC;AAID,SAAgB,4BAA4B,CAAC,GAAiB;IAC5D,OAAO,eAAK,CAAC,GAAG,EAAE;QAChB,QAAQ,CAAC,IAAkB;YACzB,uCAAY,IAAI,KAAE,KAAK,EAAE,GAAG,IAAG;QACjC,CAAC;QACD,UAAU,CAAC,IAAoB;YAC7B,uCAAY,IAAI,KAAE,KAAK,EAAE,GAAG,IAAG;QACjC,CAAC;QACD,WAAW,CAAC,IAAqB;YAC/B,uCAAY,IAAI,KAAE,KAAK,EAAE,EAAE,EAAE,KAAK,EAAE,KAAK,IAAG;QAC9C,CAAC;KACF,CAAC,CAAC;AACL,CAAC;AAZD,oEAYC;AAOD,SAAgB,qBAAqB,CACnC,GAAiB,EACjB,aAAqB;IAErB,MAAM,SAAS,GAAG,8BAAkB,CAAC,GAAG,CAAC,CAAC,aAAa,CAAC,CAAC;IACzD,IAAI,CAAC,SAAS,EAAE;QAGd,OAAO,GAAG,CAAC;KACZ;IACD,OAAO,SAAS,CAAC;AACnB,CAAC;AAXD,sDAWC;AAID,SAAS,MAAM,CACb,KAAmC,EACnC,GAAG,SAAiC;IAEpC,IAAI,KAAK,EAAE;QACT,OAAO,uBAAM,CAAC,KAAK,EAAE,GAAG,SAAS,CAAC,CAAC;KACpC;IACD,OAAO,SAAS,CAAC;AACnB,CAAC;AAOD,SAAgB,OAAO,CAAC,GAAiB;IACvC,OAAO,eAAK,CAAC,GAAG,EAAE;QAChB,QAAQ,CAAC,IAAkB;YACzB,uCACK,IAAI,KAGP,WAAW,EAAE,uBAAM,CAAC,IAAI,CAAC,WAAW,EAAE,MAAM,EAAE,YAAY,CAAC,IAC3D;QACJ,CAAC;QACD,mBAAmB,CACjB,IAA6B;YAE7B,uCACK,IAAI,KACP,mBAAmB,EAAE,MAAM,CACzB,IAAI,CAAC,mBAAmB,EACxB,qBAAqB,CACtB,IACD;QACJ,CAAC;QACD,YAAY,CAAC,IAAsB;YACjC,uCACK,IAAI,KAKP,UAAU,EAAE,uBAAM,CAAC,IAAI,CAAC,UAAU,EAAE,MAAM,EAAE,YAAY,CAAC,IACzD;QACJ,CAAC;QACD,KAAK,CAAC,IAAe;YACnB,uCACK,IAAI,KACP,SAAS,EAAE,MAAM,CAAC,IAAI,CAAC,SAAS,EAAE,YAAY,CAAC,IAC/C;QACJ,CAAC;QACD,cAAc,CAAC,IAAwB;YACrC,uCAAY,IAAI,KAAE,UAAU,EAAE,MAAM,CAAC,IAAI,CAAC,UAAU,EAAE,YAAY,CAAC,IAAG;QACxE,CAAC;QACD,cAAc,CAAC,IAAwB;YACrC,uCAAY,IAAI,KAAE,UAAU,EAAE,MAAM,CAAC,IAAI,CAAC,UAAU,EAAE,YAAY,CAAC,IAAG;QACxE,CAAC;QACD,kBAAkB,CAAC,IAA4B;YAC7C,uCACK,IAAI,KACP,UAAU,EAAE,MAAM,CAAC,IAAI,CAAC,UAAU,EAAE,YAAY,CAAC,EACjD,mBAAmB,EAAE,MAAM,CACzB,IAAI,CAAC,mBAAmB,EACxB,qBAAqB,CACtB,IACD;QACJ,CAAC;QACD,SAAS,CAAC,IAAmB;YAC3B,uCAAY,IAAI,KAAE,SAAS,EAAE,MAAM,CAAC,IAAI,CAAC,SAAS,EAAE,YAAY,CAAC,IAAG;QACtE,CAAC;KACF,CAAC,CAAC;AACL,CAAC;AAzDD,0BAyDC;AAMD,SAAgB,aAAa,CAAC,GAAiB;IAC7C,OAAO,eAAK,CAAC,GAAG,EAAE;QAChB,KAAK,CAAC,IAAe;YACnB,uCACK,IAAI,KACP,KAAK,EAAE,SAAS,IAChB;QACJ,CAAC;KACF,CAAC,CAAC;AACL,CAAC;AATD,sCASC;AAMD,SAAgB,0BAA0B,CAAC,GAAiB;IAU1D,MAAM,YAAY,GAAG,eAAK,CAAC,GAAG,EAAE;QAC9B,WAAW,CAAC,IAAqB;YAC/B,uCACK,IAAI,KACP,KAAK,EAAE,MAAM,CAAC,IAAI,CAAC,IAAI,CAAC,KAAK,EAAE,MAAM,CAAC,CAAC,QAAQ,CAAC,KAAK,CAAC,EACtD,KAAK,EAAE,KAAK,IACZ;QACJ,CAAC;KACF,CAAC,CAAC;IACH,MAAM,cAAc,GAAG,eAAK,CAAC,YAAY,CAAC,CAAC;IAC3C,MAAM,oBAAoB,GAAG,cAAc;SACxC,OAAO,CAAC,MAAM,EAAE,GAAG,CAAC;SACpB,OAAO,CAAC,mBAAmB,EAAE,CAAC,CAAC,EAAE,CAAC,EAAE,EAAE,CAAC,CAAC,CAAC;SACzC,OAAO,CAAC,mBAAmB,EAAE,CAAC,CAAC,EAAE,CAAC,EAAE,EAAE,CAAC,CAAC,CAAC,CAAC;IAC7C,OAAO,oBAAoB,CAAC,OAAO,CAAC,gBAAgB,EAAE,CAAC,CAAC,EAAE,GAAG,EAAE,EAAE,CAC/D,IAAI,CAAC,SAAS,CAAC,MAAM,CAAC,IAAI,CAAC,GAAG,EAAE,KAAK,CAAC,CAAC,QAAQ,CAAC,MAAM,CAAC,CAAC,CACzD,CAAC;AACJ,CAAC;AA3BD,gEA2BC"} apollo-server-demo/node_modules/apollo-graphql/lib/index.d.ts 0000644 0001750 0000144 00000000416 03560116604 024022 0 ustar andreh users export { defaultOperationRegistrySignature, defaultUsageReportingSignature, operationRegistrySignature, operationHash, defaultUsageReportingSignature as defaultEngineReportingSignature } from "./operationId"; export * from "./schema"; //# sourceMappingURL=index.d.ts.map apollo-server-demo/node_modules/apollo-graphql/lib/schema/ 0000755 0001750 0000144 00000000000 14067647701 023373 5 ustar andreh users apollo-server-demo/node_modules/apollo-graphql/lib/schema/index.js 0000644 0001750 0000144 00000000527 03560116604 025031 0 ustar andreh users "use strict"; function __export(m) { for (var p in m) if (!exports.hasOwnProperty(p)) exports[p] = m[p]; } Object.defineProperty(exports, "__esModule", { value: true }); __export(require("./buildSchemaFromSDL")); __export(require("./GraphQLSchemaValidationError")); __export(require("./transformSchema")); //# sourceMappingURL=index.js.map apollo-server-demo/node_modules/apollo-graphql/lib/schema/resolveObject.d.ts.map 0000644 0001750 0000144 00000001105 03560116604 027531 0 ustar andreh users {"version":3,"file":"resolveObject.d.ts","sourceRoot":"","sources":["../../src/schema/resolveObject.ts"],"names":[],"mappings":"AAAA,OAAO,EAAE,kBAAkB,EAAE,SAAS,EAAE,MAAM,SAAS,CAAC;AAExD,oBAAY,qBAAqB,CAAC,OAAO,EAAE,QAAQ,IAAI,CACrD,MAAM,EAAE,OAAO,EACf,MAAM,EAAE,MAAM,CAAC,MAAM,EAAE,aAAa,CAAC,SAAS,CAAC,CAAC,EAChD,OAAO,EAAE,QAAQ,EACjB,IAAI,EAAE,kBAAkB,KACrB,GAAG,CAAC;AAET,OAAO,QAAQ,yBAAyB,CAAC;IACvC,UAAU,iBAAiB;QACzB,aAAa,CAAC,EAAE,qBAAqB,CAAC,GAAG,EAAE,GAAG,CAAC,CAAC;KACjD;IAED,UAAU,uBAAuB,CAAC,OAAO,EAAE,QAAQ;QACjD,aAAa,CAAC,EAAE,qBAAqB,CAAC,OAAO,EAAE,QAAQ,CAAC,CAAC;KAC1D;CACF"} apollo-server-demo/node_modules/apollo-graphql/lib/schema/index.d.ts 0000644 0001750 0000144 00000000334 03560116604 025261 0 ustar andreh users export * from "./buildSchemaFromSDL"; export * from "./GraphQLSchemaValidationError"; export * from "./transformSchema"; export * from "./resolverMap"; export * from "./resolveObject"; //# sourceMappingURL=index.d.ts.map apollo-server-demo/node_modules/apollo-graphql/lib/schema/GraphQLSchemaValidationError.js 0000644 0001750 0000144 00000000772 03560116604 031370 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); class GraphQLSchemaValidationError extends Error { constructor(errors) { super(); this.errors = errors; this.name = this.constructor.name; Error.captureStackTrace(this, this.constructor); this.message = errors.map(error => error.message).join("\n\n"); } } exports.GraphQLSchemaValidationError = GraphQLSchemaValidationError; //# sourceMappingURL=GraphQLSchemaValidationError.js.map apollo-server-demo/node_modules/apollo-graphql/lib/schema/GraphQLSchemaValidationError.d.ts.map 0000644 0001750 0000144 00000000463 03560116604 032375 0 ustar andreh users {"version":3,"file":"GraphQLSchemaValidationError.d.ts","sourceRoot":"","sources":["../../src/schema/GraphQLSchemaValidationError.ts"],"names":[],"mappings":"AAAA,OAAO,EAAE,YAAY,EAAE,MAAM,SAAS,CAAC;AAEvC,qBAAa,4BAA6B,SAAQ,KAAK;IAClC,MAAM,EAAE,aAAa,CAAC,YAAY,CAAC;gBAAnC,MAAM,EAAE,aAAa,CAAC,YAAY,CAAC;CAOvD"} apollo-server-demo/node_modules/apollo-graphql/lib/schema/resolverMap.js 0000644 0001750 0000144 00000000164 03560116604 026216 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); //# sourceMappingURL=resolverMap.js.map apollo-server-demo/node_modules/apollo-graphql/lib/schema/buildSchemaFromSDL.js.map 0000644 0001750 0000144 00000014205 03560116604 030103 0 ustar andreh users {"version":3,"file":"buildSchemaFromSDL.js","sourceRoot":"","sources":["../../src/schema/buildSchemaFromSDL.ts"],"names":[],"mappings":";;AAAA,qCAqBiB;AACjB,0DAA0D;AAC1D,kDAA8D;AAE9D,iFAA8E;AAC9E,sEAAsE;AACtE,mDAI4B;AAC5B,2CAA6D;AAO7D,MAAM,eAAe,GAAqB;IACxC,+BAAkB;IAClB,4CAA+B;CAChC,CAAC;AAMF,IAAI;IACF,MAAM,sBAAsB,GAA4F,OAAO,CAAC,iDAAiD,CAAC;SAC/K,sBAAsB,CAAC;IAC1B,IAAI,sBAAsB,EAAE;QAC1B,eAAe,CAAC,IAAI,CAAC,sBAAsB,CAAC,CAAC;KAC9C;CACF;AAAC,OAAO,CAAC,EAAE;CAGX;AAED,MAAM,QAAQ,GAAG,kCAAiB,CAAC,MAAM,CACvC,IAAI,CAAC,EAAE,CAAC,CAAC,eAAe,CAAC,QAAQ,CAAC,IAAI,CAAC,CACxC,CAAC;AAEF,SAAgB,cAAc,CAC5B,YAAmE;IAEnE,IAAI,KAAK,CAAC,OAAO,CAAC,YAAY,CAAC,EAAE;QAC/B,OAAO,YAAY,CAAC,GAAG,CAAC,WAAW,CAAC,EAAE;YACpC,IAAI,gBAAM,CAAC,WAAW,CAAC,IAAI,wBAAc,CAAC,WAAW,CAAC,EAAE;gBACtD,OAAO,EAAE,QAAQ,EAAE,WAAW,EAAE,CAAC;aAClC;iBAAM;gBACL,OAAO,WAAW,CAAC;aACpB;QACH,CAAC,CAAC,CAAC;KACJ;SAAM;QACL,OAAO,CAAC,EAAE,QAAQ,EAAE,YAAY,EAAE,CAAC,CAAC;KACrC;AACH,CAAC;AAdD,wCAcC;AAED,SAAgB,kBAAkB,CAChC,YAAmE,EACnE,cAA8B;IAE9B,MAAM,OAAO,GAAG,cAAc,CAAC,YAAY,CAAC,CAAC;IAE7C,MAAM,WAAW,GAAG,mBAAS,CAAC,OAAO,CAAC,GAAG,CAAC,MAAM,CAAC,EAAE,CAAC,MAAM,CAAC,QAAQ,CAAC,CAAC,CAAC;IAEtE,MAAM,MAAM,GAAG,sBAAW,CAAC,WAAW,EAAE,cAAc,EAAE,QAAQ,CAAC,CAAC;IAClE,IAAI,MAAM,CAAC,MAAM,GAAG,CAAC,EAAE;QACrB,MAAM,IAAI,2DAA4B,CAAC,MAAM,CAAC,CAAC;KAChD;IAED,MAAM,cAAc,GAEhB,MAAM,CAAC,MAAM,CAAC,IAAI,CAAC,CAAC;IAExB,MAAM,aAAa,GAEf,MAAM,CAAC,MAAM,CAAC,IAAI,CAAC,CAAC;IAExB,MAAM,oBAAoB,GAA8B,EAAE,CAAC;IAE3D,MAAM,iBAAiB,GAA2B,EAAE,CAAC;IACrD,MAAM,gBAAgB,GAA0B,EAAE,CAAC;IAEnD,KAAK,MAAM,UAAU,IAAI,WAAW,CAAC,WAAW,EAAE;QAChD,IAAI,8BAAoB,CAAC,UAAU,CAAC,EAAE;YACpC,MAAM,QAAQ,GAAG,UAAU,CAAC,IAAI,CAAC,KAAK,CAAC;YAEvC,IAAI,cAAc,CAAC,QAAQ,CAAC,EAAE;gBAC5B,cAAc,CAAC,QAAQ,CAAC,CAAC,IAAI,CAAC,UAAU,CAAC,CAAC;aAC3C;iBAAM;gBACL,cAAc,CAAC,QAAQ,CAAC,GAAG,CAAC,UAAU,CAAC,CAAC;aACzC;SACF;aAAM,IAAI,6BAAmB,CAAC,UAAU,CAAC,EAAE;YAC1C,MAAM,QAAQ,GAAG,UAAU,CAAC,IAAI,CAAC,KAAK,CAAC;YAEvC,IAAI,aAAa,CAAC,QAAQ,CAAC,EAAE;gBAC3B,aAAa,CAAC,QAAQ,CAAC,CAAC,IAAI,CAAC,UAAU,CAAC,CAAC;aAC1C;iBAAM;gBACL,aAAa,CAAC,QAAQ,CAAC,GAAG,CAAC,UAAU,CAAC,CAAC;aACxC;SACF;aAAM,IAAI,UAAU,CAAC,IAAI,KAAK,cAAI,CAAC,oBAAoB,EAAE;YACxD,oBAAoB,CAAC,IAAI,CAAC,UAAU,CAAC,CAAC;SACvC;aAAM,IAAI,UAAU,CAAC,IAAI,KAAK,cAAI,CAAC,iBAAiB,EAAE;YACrD,iBAAiB,CAAC,IAAI,CAAC,UAAU,CAAC,CAAC;SACpC;aAAM,IAAI,UAAU,CAAC,IAAI,KAAK,cAAI,CAAC,gBAAgB,EAAE;YACpD,gBAAgB,CAAC,IAAI,CAAC,UAAU,CAAC,CAAC;SACnC;KACF;IAED,IAAI,MAAM,GAAG,cAAc;QACzB,CAAC,CAAC,cAAc;QAChB,CAAC,CAAC,IAAI,uBAAa,CAAC;YAChB,KAAK,EAAE,SAAS;SACjB,CAAC,CAAC;IAEP,MAAM,sBAAsB,GAAyB,EAAE,CAAC;IAExD,KAAK,MAAM,CAAC,gBAAgB,EAAE,UAAU,CAAC,IAAI,MAAM,CAAC,OAAO,CAAC,aAAa,CAAC,EAAE;QAC1E,IAAI,CAAC,cAAc,CAAC,gBAAgB,CAAC,EAAE;YACrC,MAAM,SAAS,GAAG,UAAU,CAAC,CAAC,CAAC,CAAC;YAEhC,MAAM,IAAI,GAAG,SAAS,CAAC,IAAI,CAAC;YAC5B,MAAM,UAAU,GAAG;gBACjB,IAAI,EAAE,gBAAgB,CAAC,IAAI,CAAC;gBAC5B,IAAI,EAAE,SAAS,CAAC,IAAI;aACC,CAAC;YAExB,sBAAsB,CAAC,IAAI,CAAC,UAAU,CAAC,CAAC;SACzC;KACF;IAED,MAAM,GAAG,sBAAY,CACnB,MAAM,EACN;QACE,IAAI,EAAE,cAAI,CAAC,QAAQ;QACnB,WAAW,EAAE;YACX,GAAG,MAAM,CAAC,MAAM,CAAC,cAAc,CAAC,CAAC,IAAI,EAAE;YACvC,GAAG,sBAAsB;YACzB,GAAG,oBAAoB;SACxB;KACF,EACD;QACE,cAAc,EAAE,IAAI;KACrB,CACF,CAAC;IAEF,MAAM,GAAG,sBAAY,CACnB,MAAM,EACN;QACE,IAAI,EAAE,cAAI,CAAC,QAAQ;QACnB,WAAW,EAAE,MAAM,CAAC,MAAM,CAAC,aAAa,CAAC,CAAC,IAAI,EAAE;KACjD,EACD;QACE,cAAc,EAAE,IAAI;KACrB,CACF,CAAC;IAEF,IAAI,gBAA+D,CAAC;IAEpE,IAAI,iBAAiB,CAAC,MAAM,GAAG,CAAC,IAAI,gBAAgB,CAAC,MAAM,GAAG,CAAC,EAAE;QAC/D,gBAAgB,GAAG,EAAE,CAAC;QAEtB,MAAM,cAAc,GAAG,CAAC,GAAG,iBAAiB,EAAE,GAAG,gBAAgB,CAAC;aAC/D,GAAG,CAAC,IAAI,CAAC,EAAE,CAAC,IAAI,CAAC,cAAc,CAAC;aAChC,MAAM,CAAC,iCAAoB,CAAC;aAC5B,IAAI,EAAE,CAAC;QAEV,KAAK,MAAM,EAAE,SAAS,EAAE,IAAI,EAAE,IAAI,cAAc,EAAE;YAChD,gBAAgB,CAAC,SAAS,CAAC,GAAG,IAAI,CAAC,IAAI,CAAC,KAAK,CAAC;SAC/C;KACF;SAAM;QACL,gBAAgB,GAAG;YACjB,KAAK,EAAE,OAAO;YACd,QAAQ,EAAE,UAAU;YACpB,YAAY,EAAE,cAAc;SAC7B,CAAC;KACH;IAED,MAAM,GAAG,IAAI,uBAAa,iCACrB,MAAM,CAAC,QAAQ,EAAE,GACjB,sBAAS,CAAC,gBAAgB,EAAE,QAAQ,CAAC,EAAE,CACxC,QAAQ;QACN,CAAC,CAAE,MAAM,CAAC,OAAO,CAAC,QAAQ,CAAiC;QAC3D,CAAC,CAAC,SAAS,CACd,EACD,CAAC;IAEH,KAAK,MAAM,MAAM,IAAI,OAAO,EAAE;QAC5B,IAAI,CAAC,MAAM,CAAC,SAAS;YAAE,SAAS;QAChC,oBAAoB,CAAC,MAAM,EAAE,MAAM,CAAC,SAAS,CAAC,CAAC;KAChD;IAED,OAAO,MAAM,CAAC;AAChB,CAAC;AAxID,gDAwIC;AAED,MAAM,gBAAgB,GAAG;IACvB,CAAC,cAAI,CAAC,qBAAqB,CAAC,EAAE,cAAI,CAAC,sBAAsB;IACzD,CAAC,cAAI,CAAC,qBAAqB,CAAC,EAAE,cAAI,CAAC,sBAAsB;IACzD,CAAC,cAAI,CAAC,wBAAwB,CAAC,EAAE,cAAI,CAAC,yBAAyB;IAC/D,CAAC,cAAI,CAAC,oBAAoB,CAAC,EAAE,cAAI,CAAC,qBAAqB;IACvD,CAAC,cAAI,CAAC,mBAAmB,CAAC,EAAE,cAAI,CAAC,oBAAoB;IACrD,CAAC,cAAI,CAAC,2BAA2B,CAAC,EAAE,cAAI,CAAC,4BAA4B;CACtE,CAAC;AAEF,SAAgB,oBAAoB,CAClC,MAAqB,EACrB,SAAkC;IAElC,KAAK,MAAM,CAAC,QAAQ,EAAE,YAAY,CAAC,IAAI,MAAM,CAAC,OAAO,CAAC,SAAS,CAAC,EAAE;QAChE,MAAM,IAAI,GAAG,MAAM,CAAC,OAAO,CAAC,QAAQ,CAAC,CAAC;QAEtC,IAAI,wBAAc,CAAC,IAAI,CAAC,EAAE;YACxB,KAAK,MAAM,CAAC,SAAS,EAAE,WAAW,CAAC,IAAI,MAAM,CAAC,OAAO,CAAC,YAAY,CAAC,EAAE;gBACnE,IAAI,SAAS,CAAC,UAAU,CAAC,IAAI,CAAC,EAAE;oBAC7B,IAAY,CAAC,SAAS,CAAC,SAAS,CAAC,CAAC,CAAC,CAAC,GAAG,WAAW,CAAC;iBACrD;aACF;SACF;QAED,IAAI,sBAAY,CAAC,IAAI,CAAC,EAAE;YACtB,KAAK,MAAM,EAAE,IAAI,YAAY,EAAE;gBAC5B,IAAY,CAAC,EAAE,CAAC,GAAI,YAAoB,CAAC,EAAE,CAAC,CAAC;aAC/C;SACF;QAED,IAAI,oBAAU,CAAC,IAAI,CAAC,EAAE;YACpB,MAAM,MAAM,GAAG,IAAI,CAAC,SAAS,EAAE,CAAC;YAChC,MAAM,SAAS,GAA8C,EAAE,CAAC;YAChE,MAAM,CAAC,OAAO,CAAC,KAAK,CAAC,EAAE;gBACrB,IAAI,QAAQ,GAAI,YAAoB,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC;gBACjD,IAAI,QAAQ,KAAK,SAAS,EAAE;oBAC1B,QAAQ,GAAG,KAAK,CAAC,IAAI,CAAC;iBACvB;gBAED,SAAS,CAAC,KAAK,CAAC,IAAI,CAAC,GAAG;oBACtB,KAAK,EAAE,QAAQ;oBACf,iBAAiB,EAAE,KAAK,CAAC,iBAAiB;oBAC1C,WAAW,EAAE,KAAK,CAAC,WAAW;oBAC9B,OAAO,EAAE,KAAK,CAAC,OAAO;oBACtB,UAAU,EAAE,SAAS;iBACtB,CAAC;YACJ,CAAC,CAAC,CAAC;YAIH,MAAM,CAAC,MAAM,CACX,IAAI,EACJ,IAAI,yBAAe,iCACd,IAAI,CAAC,QAAQ,EAAE,KAClB,MAAM,EAAE,SAAS,IACjB,CACH,CAAC;SACH;QAED,IAAI,CAAC,sBAAY,CAAC,IAAI,CAAC;YAAE,SAAS;QAElC,MAAM,QAAQ,GAAG,IAAI,CAAC,SAAS,EAAE,CAAC;QAElC,KAAK,MAAM,CAAC,SAAS,EAAE,WAAW,CAAC,IAAI,MAAM,CAAC,OAAO,CAAC,YAAY,CAAC,EAAE;YACnE,IAAI,SAAS,CAAC,UAAU,CAAC,IAAI,CAAC,EAAE;gBAC7B,IAAY,CAAC,SAAS,CAAC,SAAS,CAAC,CAAC,CAAC,CAAC,GAAG,WAAW,CAAC;gBACpD,SAAS;aACV;YAED,MAAM,KAAK,GAAG,QAAQ,CAAC,SAAS,CAAC,CAAC;YAClC,IAAI,CAAC,KAAK;gBAAE,SAAS;YAErB,IAAI,OAAO,WAAW,KAAK,UAAU,EAAE;gBACrC,KAAK,CAAC,OAAO,GAAG,WAAW,CAAC;aAC7B;iBAAM;gBACL,KAAK,CAAC,OAAO,GAAG,WAAW,CAAC,OAAO,CAAC;aACrC;SACF;KACF;AACH,CAAC;AAtED,oDAsEC"} apollo-server-demo/node_modules/apollo-graphql/lib/schema/index.d.ts.map 0000644 0001750 0000144 00000000341 03560116604 026033 0 ustar andreh users {"version":3,"file":"index.d.ts","sourceRoot":"","sources":["../../src/schema/index.ts"],"names":[],"mappings":"AAAA,cAAc,sBAAsB,CAAC;AACrC,cAAc,gCAAgC,CAAC;AAC/C,cAAc,mBAAmB,CAAC;AAClC,cAAc,eAAe,CAAC;AAC9B,cAAc,iBAAiB,CAAC"} apollo-server-demo/node_modules/apollo-graphql/lib/schema/transformSchema.d.ts.map 0000644 0001750 0000144 00000000532 03560116604 030062 0 ustar andreh users {"version":3,"file":"transformSchema.d.ts","sourceRoot":"","sources":["../../src/schema/transformSchema.ts"],"names":[],"mappings":"AAAA,OAAO,EACL,aAAa,EACb,gBAAgB,EAoBjB,MAAM,SAAS,CAAC;AAGjB,aAAK,eAAe,GAAG,CACrB,IAAI,EAAE,gBAAgB,KACnB,gBAAgB,GAAG,IAAI,GAAG,SAAS,CAAC;AAEzC,wBAAgB,eAAe,CAC7B,MAAM,EAAE,aAAa,EACrB,aAAa,EAAE,eAAe,GAC7B,aAAa,CAmHf"} apollo-server-demo/node_modules/apollo-graphql/lib/schema/buildSchemaFromSDL.d.ts 0000644 0001750 0000144 00000001234 03560116604 027561 0 ustar andreh users import { DocumentNode, GraphQLSchema } from "graphql"; import { GraphQLResolverMap } from "./resolverMap"; export interface GraphQLSchemaModule { typeDefs: DocumentNode; resolvers?: GraphQLResolverMap<any>; } export declare function modulesFromSDL(modulesOrSDL: (GraphQLSchemaModule | DocumentNode)[] | DocumentNode): GraphQLSchemaModule[]; export declare function buildSchemaFromSDL(modulesOrSDL: (GraphQLSchemaModule | DocumentNode)[] | DocumentNode, schemaToExtend?: GraphQLSchema): GraphQLSchema; export declare function addResolversToSchema(schema: GraphQLSchema, resolvers: GraphQLResolverMap<any>): void; //# sourceMappingURL=buildSchemaFromSDL.d.ts.map apollo-server-demo/node_modules/apollo-graphql/lib/schema/GraphQLSchemaValidationError.js.map 0000644 0001750 0000144 00000001001 03560116604 032126 0 ustar andreh users {"version":3,"file":"GraphQLSchemaValidationError.js","sourceRoot":"","sources":["../../src/schema/GraphQLSchemaValidationError.ts"],"names":[],"mappings":";;AAEA,MAAa,4BAA6B,SAAQ,KAAK;IACrD,YAAmB,MAAmC;QACpD,KAAK,EAAE,CAAC;QADS,WAAM,GAAN,MAAM,CAA6B;QAGpD,IAAI,CAAC,IAAI,GAAG,IAAI,CAAC,WAAW,CAAC,IAAI,CAAC;QAClC,KAAK,CAAC,iBAAiB,CAAC,IAAI,EAAE,IAAI,CAAC,WAAW,CAAC,CAAC;QAChD,IAAI,CAAC,OAAO,GAAG,MAAM,CAAC,GAAG,CAAC,KAAK,CAAC,EAAE,CAAC,KAAK,CAAC,OAAO,CAAC,CAAC,IAAI,CAAC,MAAM,CAAC,CAAC;IACjE,CAAC;CACF;AARD,oEAQC"} apollo-server-demo/node_modules/apollo-graphql/lib/schema/resolveObject.js 0000644 0001750 0000144 00000000166 03560116604 026527 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); //# sourceMappingURL=resolveObject.js.map apollo-server-demo/node_modules/apollo-graphql/lib/schema/resolveObject.js.map 0000644 0001750 0000144 00000000200 03560116604 027270 0 ustar andreh users {"version":3,"file":"resolveObject.js","sourceRoot":"","sources":["../../src/schema/resolveObject.ts"],"names":[],"mappings":""} apollo-server-demo/node_modules/apollo-graphql/lib/schema/transformSchema.d.ts 0000644 0001750 0000144 00000000502 03560116604 027303 0 ustar andreh users import { GraphQLSchema, GraphQLNamedType } from "graphql"; declare type TypeTransformer = (type: GraphQLNamedType) => GraphQLNamedType | null | undefined; export declare function transformSchema(schema: GraphQLSchema, transformType: TypeTransformer): GraphQLSchema; export {}; //# sourceMappingURL=transformSchema.d.ts.map apollo-server-demo/node_modules/apollo-graphql/lib/schema/resolverMap.d.ts 0000644 0001750 0000144 00000000671 03560116604 026455 0 ustar andreh users import { GraphQLFieldResolver, GraphQLScalarType } from "graphql"; export interface GraphQLResolverMap<TContext = {}> { [typeName: string]: { [fieldName: string]: GraphQLFieldResolver<any, TContext> | { requires?: string; resolve: GraphQLFieldResolver<any, TContext>; }; } | GraphQLScalarType | { [enumValue: string]: string | number; }; } //# sourceMappingURL=resolverMap.d.ts.map apollo-server-demo/node_modules/apollo-graphql/lib/schema/GraphQLSchemaValidationError.d.ts 0000644 0001750 0000144 00000000405 03560116604 031615 0 ustar andreh users import { GraphQLError } from "graphql"; export declare class GraphQLSchemaValidationError extends Error { errors: ReadonlyArray<GraphQLError>; constructor(errors: ReadonlyArray<GraphQLError>); } //# sourceMappingURL=GraphQLSchemaValidationError.d.ts.map apollo-server-demo/node_modules/apollo-graphql/lib/schema/index.js.map 0000644 0001750 0000144 00000000232 03560116604 025576 0 ustar andreh users {"version":3,"file":"index.js","sourceRoot":"","sources":["../../src/schema/index.ts"],"names":[],"mappings":";;;;;AAAA,0CAAqC;AACrC,oDAA+C;AAC/C,uCAAkC"} apollo-server-demo/node_modules/apollo-graphql/lib/schema/buildSchemaFromSDL.d.ts.map 0000644 0001750 0000144 00000001201 03560116604 030327 0 ustar andreh users {"version":3,"file":"buildSchemaFromSDL.d.ts","sourceRoot":"","sources":["../../src/schema/buildSchemaFromSDL.ts"],"names":[],"mappings":"AAAA,OAAO,EAEL,YAAY,EAEZ,aAAa,EAiBd,MAAM,SAAS,CAAC;AAGjB,OAAO,EAAE,kBAAkB,EAAE,MAAM,eAAe,CAAC;AAUnD,MAAM,WAAW,mBAAmB;IAClC,QAAQ,EAAE,YAAY,CAAC;IACvB,SAAS,CAAC,EAAE,kBAAkB,CAAC,GAAG,CAAC,CAAC;CACrC;AA0BD,wBAAgB,cAAc,CAC5B,YAAY,EAAE,CAAC,mBAAmB,GAAG,YAAY,CAAC,EAAE,GAAG,YAAY,GAClE,mBAAmB,EAAE,CAYvB;AAED,wBAAgB,kBAAkB,CAChC,YAAY,EAAE,CAAC,mBAAmB,GAAG,YAAY,CAAC,EAAE,GAAG,YAAY,EACnE,cAAc,CAAC,EAAE,aAAa,GAC7B,aAAa,CAqIf;AAWD,wBAAgB,oBAAoB,CAClC,MAAM,EAAE,aAAa,EACrB,SAAS,EAAE,kBAAkB,CAAC,GAAG,CAAC,QAoEnC"} apollo-server-demo/node_modules/apollo-graphql/lib/schema/transformSchema.js.map 0000644 0001750 0000144 00000005600 03560116604 027627 0 ustar andreh users {"version":3,"file":"transformSchema.js","sourceRoot":"","sources":["../../src/schema/transformSchema.ts"],"names":[],"mappings":";;AAAA,qCAsBiB;AACjB,2CAAuC;AAMvC,SAAgB,eAAe,CAC7B,MAAqB,EACrB,aAA8B;IAE9B,MAAM,OAAO,GAA6C,MAAM,CAAC,MAAM,CAAC,IAAI,CAAC,CAAC;IAE9E,KAAK,MAAM,OAAO,IAAI,MAAM,CAAC,MAAM,CAAC,MAAM,CAAC,UAAU,EAAE,CAAC,EAAE;QACxD,IAAI,6BAAmB,CAAC,OAAO,CAAC;YAAE,SAAS;QAE3C,MAAM,MAAM,GAAG,aAAa,CAAC,OAAO,CAAC,CAAC;QAGtC,IAAI,MAAM,KAAK,IAAI;YAAE,SAAS;QAG9B,MAAM,OAAO,GAAG,MAAM,IAAI,OAAO,CAAC;QAClC,OAAO,CAAC,OAAO,CAAC,IAAI,CAAC,GAAG,iBAAiB,CAAC,OAAO,CAAC,CAAC;KACpD;IAED,MAAM,YAAY,GAAG,MAAM,CAAC,QAAQ,EAAE,CAAC;IAEvC,OAAO,IAAI,uBAAa,iCACnB,YAAY,KACf,KAAK,EAAE,MAAM,CAAC,MAAM,CAAC,OAAO,CAAC,EAC7B,KAAK,EAAE,gBAAgB,CAAC,YAAY,CAAC,KAAK,CAAC,EAC3C,QAAQ,EAAE,gBAAgB,CAAC,YAAY,CAAC,QAAQ,CAAC,EACjD,YAAY,EAAE,gBAAgB,CAAC,YAAY,CAAC,YAAY,CAAC,IACzD,CAAC;IAEH,SAAS,iBAAiB,CAAC,IAAsB;QAC/C,IAAI,sBAAY,CAAC,IAAI,CAAC,EAAE;YACtB,MAAM,MAAM,GAAG,IAAI,CAAC,QAAQ,EAAE,CAAC;YAE/B,OAAO,IAAI,2BAAiB,iCACvB,MAAM,KACT,UAAU,EAAE,GAAG,EAAE,CAAC,MAAM,CAAC,UAAU,CAAC,GAAG,CAAC,gBAAgB,CAAC,EACzD,MAAM,EAAE,GAAG,EAAE,CAAC,aAAa,CAAC,MAAM,CAAC,MAAM,CAAC,IAC1C,CAAC;SACJ;aAAM,IAAI,yBAAe,CAAC,IAAI,CAAC,EAAE;YAChC,MAAM,MAAM,GAAG,IAAI,CAAC,QAAQ,EAAE,CAAC;YAE/B,OAAO,IAAI,8BAAoB,iCAC1B,MAAM,KACT,MAAM,EAAE,GAAG,EAAE,CAAC,aAAa,CAAC,MAAM,CAAC,MAAM,CAAC,IAC1C,CAAC;SACJ;aAAM,IAAI,qBAAW,CAAC,IAAI,CAAC,EAAE;YAC5B,MAAM,MAAM,GAAG,IAAI,CAAC,QAAQ,EAAE,CAAC;YAE/B,OAAO,IAAI,0BAAgB,iCACtB,MAAM,KACT,KAAK,EAAE,GAAG,EAAE,CAAC,MAAM,CAAC,KAAK,CAAC,GAAG,CAAC,gBAAgB,CAAC,IAC/C,CAAC;SACJ;aAAM,IAAI,2BAAiB,CAAC,IAAI,CAAC,EAAE;YAClC,MAAM,MAAM,GAAG,IAAI,CAAC,QAAQ,EAAE,CAAC;YAE/B,OAAO,IAAI,gCAAsB,iCAC5B,MAAM,KACT,MAAM,EAAE,GAAG,EAAE,CAAC,kBAAkB,CAAC,MAAM,CAAC,MAAM,CAAC,IAC/C,CAAC;SACJ;QAED,OAAO,IAAI,CAAC;IACd,CAAC;IAWD,SAAS,WAAW,CAAC,IAAiB;QACpC,IAAI,oBAAU,CAAC,IAAI,CAAC,EAAE;YACpB,OAAO,IAAI,qBAAW,CAAC,WAAW,CAAC,IAAI,CAAC,MAAM,CAAC,CAAC,CAAC;SAClD;aAAM,IAAI,uBAAa,CAAC,IAAI,CAAC,EAAE;YAC9B,OAAO,IAAI,wBAAc,CAAC,WAAW,CAAC,IAAI,CAAC,MAAM,CAAC,CAAC,CAAC;SACrD;QACD,OAAO,gBAAgB,CAAC,IAAI,CAAC,CAAC;IAChC,CAAC;IAED,SAAS,gBAAgB,CAA6B,IAAO;QAC3D,MAAM,OAAO,GAAG,OAAO,CAAC,IAAI,CAAC,IAAI,CAAM,CAAC;QACxC,OAAO,OAAO,CAAC,CAAC,CAAC,OAAO,CAAC,CAAC,CAAC,IAAI,CAAC;IAClC,CAAC;IAED,SAAS,gBAAgB,CACvB,IAA0B;QAE1B,OAAO,IAAI,CAAC,CAAC,CAAC,gBAAgB,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC,SAAS,CAAC;IACnD,CAAC;IAED,SAAS,aAAa,CACpB,SAAmD;QAEnD,OAAO,sBAAS,CAAC,SAAS,EAAE,KAAK,CAAC,EAAE,CAAC,iCAChC,KAAK,KACR,IAAI,EAAE,WAAW,CAAC,KAAK,CAAC,IAAI,CAAC,EAC7B,IAAI,EAAE,KAAK,CAAC,IAAI,CAAC,CAAC,CAAC,WAAW,CAAC,KAAK,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC,SAAS,IACtD,CAAC,CAAC;IACN,CAAC;IAED,SAAS,kBAAkB,CACzB,SAAqC;QAErC,OAAO,sBAAS,CAAC,SAAS,EAAE,KAAK,CAAC,EAAE,CAAC,iCAChC,KAAK,KACR,IAAI,EAAE,WAAW,CAAC,KAAK,CAAC,IAAI,CAAC,IAC7B,CAAC,CAAC;IACN,CAAC;IAED,SAAS,WAAW,CAAC,IAAmC;QACtD,OAAO,sBAAS,CAAC,IAAI,EAAE,GAAG,CAAC,EAAE,CAAC,iCACzB,GAAG,KACN,IAAI,EAAE,WAAW,CAAC,GAAG,CAAC,IAAI,CAAC,IAC3B,CAAC,CAAC;IACN,CAAC;AACH,CAAC;AAtHD,0CAsHC"} apollo-server-demo/node_modules/apollo-graphql/lib/schema/resolveObject.d.ts 0000644 0001750 0000144 00000001055 03560116604 026761 0 ustar andreh users import { GraphQLResolveInfo, FieldNode } from "graphql"; export declare type GraphQLObjectResolver<TSource, TContext> = (source: TSource, fields: Record<string, ReadonlyArray<FieldNode>>, context: TContext, info: GraphQLResolveInfo) => any; declare module "graphql/type/definition" { interface GraphQLObjectType { resolveObject?: GraphQLObjectResolver<any, any>; } interface GraphQLObjectTypeConfig<TSource, TContext> { resolveObject?: GraphQLObjectResolver<TSource, TContext>; } } //# sourceMappingURL=resolveObject.d.ts.map apollo-server-demo/node_modules/apollo-graphql/lib/schema/buildSchemaFromSDL.js 0000644 0001750 0000144 00000017374 03560116604 027341 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); const graphql_1 = require("graphql"); const validate_1 = require("graphql/validation/validate"); const graphql_2 = require("../utilities/graphql"); const GraphQLSchemaValidationError_1 = require("./GraphQLSchemaValidationError"); const specifiedRules_1 = require("graphql/validation/specifiedRules"); const validation_1 = require("graphql/validation"); const apollo_env_1 = require("apollo-env"); const skippedSDLRules = [ validation_1.KnownTypeNamesRule, validation_1.UniqueDirectivesPerLocationRule ]; try { const PossibleTypeExtensions = require("graphql/validation/rules/PossibleTypeExtensions") .PossibleTypeExtensions; if (PossibleTypeExtensions) { skippedSDLRules.push(PossibleTypeExtensions); } } catch (e) { } const sdlRules = specifiedRules_1.specifiedSDLRules.filter(rule => !skippedSDLRules.includes(rule)); function modulesFromSDL(modulesOrSDL) { if (Array.isArray(modulesOrSDL)) { return modulesOrSDL.map(moduleOrSDL => { if (graphql_2.isNode(moduleOrSDL) && graphql_2.isDocumentNode(moduleOrSDL)) { return { typeDefs: moduleOrSDL }; } else { return moduleOrSDL; } }); } else { return [{ typeDefs: modulesOrSDL }]; } } exports.modulesFromSDL = modulesFromSDL; function buildSchemaFromSDL(modulesOrSDL, schemaToExtend) { const modules = modulesFromSDL(modulesOrSDL); const documentAST = graphql_1.concatAST(modules.map(module => module.typeDefs)); const errors = validate_1.validateSDL(documentAST, schemaToExtend, sdlRules); if (errors.length > 0) { throw new GraphQLSchemaValidationError_1.GraphQLSchemaValidationError(errors); } const definitionsMap = Object.create(null); const extensionsMap = Object.create(null); const directiveDefinitions = []; const schemaDefinitions = []; const schemaExtensions = []; for (const definition of documentAST.definitions) { if (graphql_1.isTypeDefinitionNode(definition)) { const typeName = definition.name.value; if (definitionsMap[typeName]) { definitionsMap[typeName].push(definition); } else { definitionsMap[typeName] = [definition]; } } else if (graphql_1.isTypeExtensionNode(definition)) { const typeName = definition.name.value; if (extensionsMap[typeName]) { extensionsMap[typeName].push(definition); } else { extensionsMap[typeName] = [definition]; } } else if (definition.kind === graphql_1.Kind.DIRECTIVE_DEFINITION) { directiveDefinitions.push(definition); } else if (definition.kind === graphql_1.Kind.SCHEMA_DEFINITION) { schemaDefinitions.push(definition); } else if (definition.kind === graphql_1.Kind.SCHEMA_EXTENSION) { schemaExtensions.push(definition); } } let schema = schemaToExtend ? schemaToExtend : new graphql_1.GraphQLSchema({ query: undefined }); const missingTypeDefinitions = []; for (const [extendedTypeName, extensions] of Object.entries(extensionsMap)) { if (!definitionsMap[extendedTypeName]) { const extension = extensions[0]; const kind = extension.kind; const definition = { kind: extKindToDefKind[kind], name: extension.name }; missingTypeDefinitions.push(definition); } } schema = graphql_1.extendSchema(schema, { kind: graphql_1.Kind.DOCUMENT, definitions: [ ...Object.values(definitionsMap).flat(), ...missingTypeDefinitions, ...directiveDefinitions ] }, { assumeValidSDL: true }); schema = graphql_1.extendSchema(schema, { kind: graphql_1.Kind.DOCUMENT, definitions: Object.values(extensionsMap).flat() }, { assumeValidSDL: true }); let operationTypeMap; if (schemaDefinitions.length > 0 || schemaExtensions.length > 0) { operationTypeMap = {}; const operationTypes = [...schemaDefinitions, ...schemaExtensions] .map(node => node.operationTypes) .filter(apollo_env_1.isNotNullOrUndefined) .flat(); for (const { operation, type } of operationTypes) { operationTypeMap[operation] = type.name.value; } } else { operationTypeMap = { query: "Query", mutation: "Mutation", subscription: "Subscription" }; } schema = new graphql_1.GraphQLSchema(Object.assign(Object.assign({}, schema.toConfig()), apollo_env_1.mapValues(operationTypeMap, typeName => typeName ? schema.getType(typeName) : undefined))); for (const module of modules) { if (!module.resolvers) continue; addResolversToSchema(schema, module.resolvers); } return schema; } exports.buildSchemaFromSDL = buildSchemaFromSDL; const extKindToDefKind = { [graphql_1.Kind.SCALAR_TYPE_EXTENSION]: graphql_1.Kind.SCALAR_TYPE_DEFINITION, [graphql_1.Kind.OBJECT_TYPE_EXTENSION]: graphql_1.Kind.OBJECT_TYPE_DEFINITION, [graphql_1.Kind.INTERFACE_TYPE_EXTENSION]: graphql_1.Kind.INTERFACE_TYPE_DEFINITION, [graphql_1.Kind.UNION_TYPE_EXTENSION]: graphql_1.Kind.UNION_TYPE_DEFINITION, [graphql_1.Kind.ENUM_TYPE_EXTENSION]: graphql_1.Kind.ENUM_TYPE_DEFINITION, [graphql_1.Kind.INPUT_OBJECT_TYPE_EXTENSION]: graphql_1.Kind.INPUT_OBJECT_TYPE_DEFINITION }; function addResolversToSchema(schema, resolvers) { for (const [typeName, fieldConfigs] of Object.entries(resolvers)) { const type = schema.getType(typeName); if (graphql_1.isAbstractType(type)) { for (const [fieldName, fieldConfig] of Object.entries(fieldConfigs)) { if (fieldName.startsWith("__")) { type[fieldName.substring(2)] = fieldConfig; } } } if (graphql_1.isScalarType(type)) { for (const fn in fieldConfigs) { type[fn] = fieldConfigs[fn]; } } if (graphql_1.isEnumType(type)) { const values = type.getValues(); const newValues = {}; values.forEach(value => { let newValue = fieldConfigs[value.name]; if (newValue === undefined) { newValue = value.name; } newValues[value.name] = { value: newValue, deprecationReason: value.deprecationReason, description: value.description, astNode: value.astNode, extensions: undefined }; }); Object.assign(type, new graphql_1.GraphQLEnumType(Object.assign(Object.assign({}, type.toConfig()), { values: newValues }))); } if (!graphql_1.isObjectType(type)) continue; const fieldMap = type.getFields(); for (const [fieldName, fieldConfig] of Object.entries(fieldConfigs)) { if (fieldName.startsWith("__")) { type[fieldName.substring(2)] = fieldConfig; continue; } const field = fieldMap[fieldName]; if (!field) continue; if (typeof fieldConfig === "function") { field.resolve = fieldConfig; } else { field.resolve = fieldConfig.resolve; } } } } exports.addResolversToSchema = addResolversToSchema; //# sourceMappingURL=buildSchemaFromSDL.js.map apollo-server-demo/node_modules/apollo-graphql/lib/schema/resolverMap.d.ts.map 0000644 0001750 0000144 00000000770 03560116604 027231 0 ustar andreh users {"version":3,"file":"resolverMap.d.ts","sourceRoot":"","sources":["../../src/schema/resolverMap.ts"],"names":[],"mappings":"AAAA,OAAO,EAAE,oBAAoB,EAAE,iBAAiB,EAAE,MAAM,SAAS,CAAC;AAElE,MAAM,WAAW,kBAAkB,CAAC,QAAQ,GAAG,EAAE;IAC/C,CAAC,QAAQ,EAAE,MAAM,GACb;QACE,CAAC,SAAS,EAAE,MAAM,GACd,oBAAoB,CAAC,GAAG,EAAE,QAAQ,CAAC,GACnC;YACE,QAAQ,CAAC,EAAE,MAAM,CAAC;YAClB,OAAO,EAAE,oBAAoB,CAAC,GAAG,EAAE,QAAQ,CAAC,CAAC;SAC9C,CAAC;KACP,GACD,iBAAiB,GACjB;QACE,CAAC,SAAS,EAAE,MAAM,GAAG,MAAM,GAAG,MAAM,CAAC;KACtC,CAAC;CACP"} apollo-server-demo/node_modules/apollo-graphql/lib/schema/resolverMap.js.map 0000644 0001750 0000144 00000000174 03560116604 026773 0 ustar andreh users {"version":3,"file":"resolverMap.js","sourceRoot":"","sources":["../../src/schema/resolverMap.ts"],"names":[],"mappings":""} apollo-server-demo/node_modules/apollo-graphql/lib/schema/transformSchema.js 0000644 0001750 0000144 00000006331 03560116604 027055 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); const graphql_1 = require("graphql"); const apollo_env_1 = require("apollo-env"); function transformSchema(schema, transformType) { const typeMap = Object.create(null); for (const oldType of Object.values(schema.getTypeMap())) { if (graphql_1.isIntrospectionType(oldType)) continue; const result = transformType(oldType); if (result === null) continue; const newType = result || oldType; typeMap[newType.name] = recreateNamedType(newType); } const schemaConfig = schema.toConfig(); return new graphql_1.GraphQLSchema(Object.assign(Object.assign({}, schemaConfig), { types: Object.values(typeMap), query: replaceMaybeType(schemaConfig.query), mutation: replaceMaybeType(schemaConfig.mutation), subscription: replaceMaybeType(schemaConfig.subscription) })); function recreateNamedType(type) { if (graphql_1.isObjectType(type)) { const config = type.toConfig(); return new graphql_1.GraphQLObjectType(Object.assign(Object.assign({}, config), { interfaces: () => config.interfaces.map(replaceNamedType), fields: () => replaceFields(config.fields) })); } else if (graphql_1.isInterfaceType(type)) { const config = type.toConfig(); return new graphql_1.GraphQLInterfaceType(Object.assign(Object.assign({}, config), { fields: () => replaceFields(config.fields) })); } else if (graphql_1.isUnionType(type)) { const config = type.toConfig(); return new graphql_1.GraphQLUnionType(Object.assign(Object.assign({}, config), { types: () => config.types.map(replaceNamedType) })); } else if (graphql_1.isInputObjectType(type)) { const config = type.toConfig(); return new graphql_1.GraphQLInputObjectType(Object.assign(Object.assign({}, config), { fields: () => replaceInputFields(config.fields) })); } return type; } function replaceType(type) { if (graphql_1.isListType(type)) { return new graphql_1.GraphQLList(replaceType(type.ofType)); } else if (graphql_1.isNonNullType(type)) { return new graphql_1.GraphQLNonNull(replaceType(type.ofType)); } return replaceNamedType(type); } function replaceNamedType(type) { const newType = typeMap[type.name]; return newType ? newType : type; } function replaceMaybeType(type) { return type ? replaceNamedType(type) : undefined; } function replaceFields(fieldsMap) { return apollo_env_1.mapValues(fieldsMap, field => (Object.assign(Object.assign({}, field), { type: replaceType(field.type), args: field.args ? replaceArgs(field.args) : undefined }))); } function replaceInputFields(fieldsMap) { return apollo_env_1.mapValues(fieldsMap, field => (Object.assign(Object.assign({}, field), { type: replaceType(field.type) }))); } function replaceArgs(args) { return apollo_env_1.mapValues(args, arg => (Object.assign(Object.assign({}, arg), { type: replaceType(arg.type) }))); } } exports.transformSchema = transformSchema; //# sourceMappingURL=transformSchema.js.map apollo-server-demo/node_modules/apollo-graphql/lib/index.d.ts.map 0000644 0001750 0000144 00000000344 03560116604 024576 0 ustar andreh users {"version":3,"file":"index.d.ts","sourceRoot":"","sources":["../src/index.ts"],"names":[],"mappings":"AAAA,OAAO,EACL,iCAAiC,EACjC,8BAA8B,EAC9B,0BAA0B,EAC1B,aAAa,EAEb,8BAA8B,IAAI,+BAA+B,EAClE,MAAM,eAAe,CAAC;AACvB,cAAc,UAAU,CAAC"} apollo-server-demo/node_modules/apollo-graphql/lib/utilities/ 0000755 0001750 0000144 00000000000 14067647700 024145 5 ustar andreh users apollo-server-demo/node_modules/apollo-graphql/lib/utilities/graphql.d.ts.map 0000644 0001750 0000144 00000000451 03560116604 027137 0 ustar andreh users {"version":3,"file":"graphql.d.ts","sourceRoot":"","sources":["../../src/utilities/graphql.ts"],"names":[],"mappings":"AAAA,OAAO,EAAE,OAAO,EAAE,YAAY,EAAQ,MAAM,SAAS,CAAC;AAEtD,wBAAgB,MAAM,CAAC,SAAS,EAAE,GAAG,GAAG,SAAS,IAAI,OAAO,CAE3D;AAED,wBAAgB,cAAc,CAAC,IAAI,EAAE,OAAO,GAAG,IAAI,IAAI,YAAY,CAElE"} apollo-server-demo/node_modules/apollo-graphql/lib/utilities/graphql.js.map 0000644 0001750 0000144 00000000551 03560116604 026704 0 ustar andreh users {"version":3,"file":"graphql.js","sourceRoot":"","sources":["../../src/utilities/graphql.ts"],"names":[],"mappings":";;AAAA,qCAAsD;AAEtD,SAAgB,MAAM,CAAC,SAAc;IACnC,OAAO,SAAS,IAAI,OAAO,SAAS,CAAC,IAAI,KAAK,QAAQ,CAAC;AACzD,CAAC;AAFD,wBAEC;AAED,SAAgB,cAAc,CAAC,IAAa;IAC1C,OAAO,MAAM,CAAC,IAAI,CAAC,IAAI,IAAI,CAAC,IAAI,KAAK,cAAI,CAAC,QAAQ,CAAC;AACrD,CAAC;AAFD,wCAEC"} apollo-server-demo/node_modules/apollo-graphql/lib/utilities/graphql.d.ts 0000644 0001750 0000144 00000000351 03560116604 026362 0 ustar andreh users import { ASTNode, DocumentNode } from "graphql"; export declare function isNode(maybeNode: any): maybeNode is ASTNode; export declare function isDocumentNode(node: ASTNode): node is DocumentNode; //# sourceMappingURL=graphql.d.ts.map apollo-server-demo/node_modules/apollo-graphql/lib/utilities/graphql.js 0000644 0001750 0000144 00000000627 03560116604 026134 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); const graphql_1 = require("graphql"); function isNode(maybeNode) { return maybeNode && typeof maybeNode.kind === "string"; } exports.isNode = isNode; function isDocumentNode(node) { return isNode(node) && node.kind === graphql_1.Kind.DOCUMENT; } exports.isDocumentNode = isDocumentNode; //# sourceMappingURL=graphql.js.map apollo-server-demo/node_modules/apollo-graphql/lib/transforms.js 0000644 0001750 0000144 00000010611 03560116604 024653 0 ustar andreh users "use strict"; var __importDefault = (this && this.__importDefault) || function (mod) { return (mod && mod.__esModule) ? mod : { "default": mod }; }; Object.defineProperty(exports, "__esModule", { value: true }); const visitor_1 = require("graphql/language/visitor"); const printer_1 = require("graphql/language/printer"); const utilities_1 = require("graphql/utilities"); const lodash_sortby_1 = __importDefault(require("lodash.sortby")); function hideLiterals(ast) { return visitor_1.visit(ast, { IntValue(node) { return Object.assign(Object.assign({}, node), { value: "0" }); }, FloatValue(node) { return Object.assign(Object.assign({}, node), { value: "0" }); }, StringValue(node) { return Object.assign(Object.assign({}, node), { value: "", block: false }); }, ListValue(node) { return Object.assign(Object.assign({}, node), { values: [] }); }, ObjectValue(node) { return Object.assign(Object.assign({}, node), { fields: [] }); } }); } exports.hideLiterals = hideLiterals; function hideStringAndNumericLiterals(ast) { return visitor_1.visit(ast, { IntValue(node) { return Object.assign(Object.assign({}, node), { value: "0" }); }, FloatValue(node) { return Object.assign(Object.assign({}, node), { value: "0" }); }, StringValue(node) { return Object.assign(Object.assign({}, node), { value: "", block: false }); } }); } exports.hideStringAndNumericLiterals = hideStringAndNumericLiterals; function dropUnusedDefinitions(ast, operationName) { const separated = utilities_1.separateOperations(ast)[operationName]; if (!separated) { return ast; } return separated; } exports.dropUnusedDefinitions = dropUnusedDefinitions; function sorted(items, ...iteratees) { if (items) { return lodash_sortby_1.default(items, ...iteratees); } return undefined; } function sortAST(ast) { return visitor_1.visit(ast, { Document(node) { return Object.assign(Object.assign({}, node), { definitions: lodash_sortby_1.default(node.definitions, "kind", "name.value") }); }, OperationDefinition(node) { return Object.assign(Object.assign({}, node), { variableDefinitions: sorted(node.variableDefinitions, "variable.name.value") }); }, SelectionSet(node) { return Object.assign(Object.assign({}, node), { selections: lodash_sortby_1.default(node.selections, "kind", "name.value") }); }, Field(node) { return Object.assign(Object.assign({}, node), { arguments: sorted(node.arguments, "name.value") }); }, FragmentSpread(node) { return Object.assign(Object.assign({}, node), { directives: sorted(node.directives, "name.value") }); }, InlineFragment(node) { return Object.assign(Object.assign({}, node), { directives: sorted(node.directives, "name.value") }); }, FragmentDefinition(node) { return Object.assign(Object.assign({}, node), { directives: sorted(node.directives, "name.value"), variableDefinitions: sorted(node.variableDefinitions, "variable.name.value") }); }, Directive(node) { return Object.assign(Object.assign({}, node), { arguments: sorted(node.arguments, "name.value") }); } }); } exports.sortAST = sortAST; function removeAliases(ast) { return visitor_1.visit(ast, { Field(node) { return Object.assign(Object.assign({}, node), { alias: undefined }); } }); } exports.removeAliases = removeAliases; function printWithReducedWhitespace(ast) { const sanitizedAST = visitor_1.visit(ast, { StringValue(node) { return Object.assign(Object.assign({}, node), { value: Buffer.from(node.value, "utf8").toString("hex"), block: false }); } }); const withWhitespace = printer_1.print(sanitizedAST); const minimizedButStillHex = withWhitespace .replace(/\s+/g, " ") .replace(/([^_a-zA-Z0-9]) /g, (_, c) => c) .replace(/ ([^_a-zA-Z0-9])/g, (_, c) => c); return minimizedButStillHex.replace(/"([a-f0-9]+)"/g, (_, hex) => JSON.stringify(Buffer.from(hex, "hex").toString("utf8"))); } exports.printWithReducedWhitespace = printWithReducedWhitespace; //# sourceMappingURL=transforms.js.map apollo-server-demo/node_modules/apollo-graphql/lib/transforms.d.ts.map 0000644 0001750 0000144 00000000772 03560116604 025672 0 ustar andreh users {"version":3,"file":"transforms.d.ts","sourceRoot":"","sources":["../src/transforms.ts"],"names":[],"mappings":"AACA,OAAO,EACL,YAAY,EAab,MAAM,sBAAsB,CAAC;AAe9B,wBAAgB,YAAY,CAAC,GAAG,EAAE,YAAY,GAAG,YAAY,CAkB5D;AAID,wBAAgB,4BAA4B,CAAC,GAAG,EAAE,YAAY,GAAG,YAAY,CAY5E;AAOD,wBAAgB,qBAAqB,CACnC,GAAG,EAAE,YAAY,EACjB,aAAa,EAAE,MAAM,GACpB,YAAY,CAQd;AAmBD,wBAAgB,OAAO,CAAC,GAAG,EAAE,YAAY,GAAG,YAAY,CAyDvD;AAMD,wBAAgB,aAAa,CAAC,GAAG,EAAE,YAAY,GAAG,YAAY,CAS7D;AAMD,wBAAgB,0BAA0B,CAAC,GAAG,EAAE,YAAY,GAAG,MAAM,CA2BpE"} apollo-server-demo/node_modules/apollo-graphql/lib/operationId.d.ts 0000644 0001750 0000144 00000001016 03560116604 025165 0 ustar andreh users import { DocumentNode } from "graphql"; export declare function defaultUsageReportingSignature(ast: DocumentNode, operationName: string): string; export declare function operationRegistrySignature(ast: DocumentNode, operationName: string, options?: { preserveStringAndNumericLiterals: boolean; }): string; export declare function defaultOperationRegistrySignature(ast: DocumentNode, operationName: string): string; export declare function operationHash(operation: string): string; //# sourceMappingURL=operationId.d.ts.map apollo-server-demo/node_modules/apollo-graphql/lib/index.js.map 0000644 0001750 0000144 00000000371 03560116604 024342 0 ustar andreh users {"version":3,"file":"index.js","sourceRoot":"","sources":["../src/index.ts"],"names":[],"mappings":";;;;;AAAA,6CAOuB;AANrB,0DAAA,iCAAiC,CAAA;AACjC,uDAAA,8BAA8B,CAAA;AAC9B,mDAAA,0BAA0B,CAAA;AAC1B,sCAAA,aAAa,CAAA;AAEb,wDAAA,8BAA8B,CAAmC;AAEnE,8BAAyB"} apollo-server-demo/node_modules/apollo-graphql/lib/operationId.js.map 0000644 0001750 0000144 00000001643 03560116604 025513 0 ustar andreh users {"version":3,"file":"operationId.js","sourceRoot":"","sources":["../src/operationId.ts"],"names":[],"mappings":";;AAuDA,2CAAwC;AACxC,6CAOsB;AAKtB,SAAgB,8BAA8B,CAC5C,GAAiB,EACjB,aAAqB;IAErB,OAAO,uCAA0B,CAC/B,oBAAO,CACL,0BAAa,CAAC,yBAAY,CAAC,kCAAqB,CAAC,GAAG,EAAE,aAAa,CAAC,CAAC,CAAC,CACvE,CACF,CAAC;AACJ,CAAC;AATD,wEASC;AAMD,SAAgB,0BAA0B,CACxC,GAAiB,EACjB,aAAqB,EACrB,UAAyD;IACvD,gCAAgC,EAAE,KAAK;CACxC;IAED,MAAM,iBAAiB,GAAG,kCAAqB,CAAC,GAAG,EAAE,aAAa,CAAC,CAAC;IACpE,MAAM,iBAAiB,GAAG,OAAO,CAAC,gCAAgC;QAChE,CAAC,CAAC,iBAAiB;QACnB,CAAC,CAAC,yCAA4B,CAAC,iBAAiB,CAAC,CAAC;IACpD,OAAO,uCAA0B,CAAC,oBAAO,CAAC,iBAAiB,CAAC,CAAC,CAAC;AAChE,CAAC;AAZD,gEAYC;AAED,SAAgB,iCAAiC,CAC/C,GAAiB,EACjB,aAAqB;IAErB,OAAO,0BAA0B,CAAC,GAAG,EAAE,aAAa,EAAE;QACpD,gCAAgC,EAAE,KAAK;KACxC,CAAC,CAAC;AACL,CAAC;AAPD,8EAOC;AAED,SAAgB,aAAa,CAAC,SAAiB;IAC7C,OAAO,uBAAU,CAAC,QAAQ,CAAC;SACxB,MAAM,CAAC,SAAS,CAAC;SACjB,MAAM,CAAC,KAAK,CAAC,CAAC;AACnB,CAAC;AAJD,sCAIC"} apollo-server-demo/node_modules/apollo-graphql/lib/transforms.d.ts 0000644 0001750 0000144 00000001073 03560116604 025111 0 ustar andreh users import { DocumentNode } from "graphql/language/ast"; export declare function hideLiterals(ast: DocumentNode): DocumentNode; export declare function hideStringAndNumericLiterals(ast: DocumentNode): DocumentNode; export declare function dropUnusedDefinitions(ast: DocumentNode, operationName: string): DocumentNode; export declare function sortAST(ast: DocumentNode): DocumentNode; export declare function removeAliases(ast: DocumentNode): DocumentNode; export declare function printWithReducedWhitespace(ast: DocumentNode): string; //# sourceMappingURL=transforms.d.ts.map apollo-server-demo/node_modules/apollo-graphql/lib/operationId.d.ts.map 0000644 0001750 0000144 00000000751 03560116604 025746 0 ustar andreh users {"version":3,"file":"operationId.d.ts","sourceRoot":"","sources":["../src/operationId.ts"],"names":[],"mappings":"AAsDA,OAAO,EAAE,YAAY,EAAE,MAAM,SAAS,CAAC;AAcvC,wBAAgB,8BAA8B,CAC5C,GAAG,EAAE,YAAY,EACjB,aAAa,EAAE,MAAM,GACpB,MAAM,CAMR;AAMD,wBAAgB,0BAA0B,CACxC,GAAG,EAAE,YAAY,EACjB,aAAa,EAAE,MAAM,EACrB,OAAO,GAAE;IAAE,gCAAgC,EAAE,OAAO,CAAA;CAEnD,GACA,MAAM,CAMR;AAED,wBAAgB,iCAAiC,CAC/C,GAAG,EAAE,YAAY,EACjB,aAAa,EAAE,MAAM,GACpB,MAAM,CAIR;AAED,wBAAgB,aAAa,CAAC,SAAS,EAAE,MAAM,GAAG,MAAM,CAIvD"} apollo-server-demo/node_modules/zen-observable/ 0000755 0001750 0000144 00000000000 14067647701 021361 5 ustar andreh users apollo-server-demo/node_modules/zen-observable/extras.js 0000644 0001750 0000144 00000000055 03560116604 023212 0 ustar andreh users module.exports = require('./lib/extras.js'); apollo-server-demo/node_modules/zen-observable/index.js 0000644 0001750 0000144 00000000074 03560116604 023014 0 ustar andreh users module.exports = require('./lib/Observable.js').Observable; apollo-server-demo/node_modules/zen-observable/LICENSE 0000644 0001750 0000144 00000002054 03560116604 022354 0 ustar andreh users Copyright (c) 2018 zenparsing (Kevin Smith) Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/zen-observable/src/ 0000755 0001750 0000144 00000000000 14067647701 022150 5 ustar andreh users apollo-server-demo/node_modules/zen-observable/src/extras.js 0000644 0001750 0000144 00000004573 03560116604 024012 0 ustar andreh users import { Observable } from './Observable.js'; // Emits all values from all inputs in parallel export function merge(...sources) { return new Observable(observer => { if (sources.length === 0) return Observable.from([]); let count = sources.length; let subscriptions = sources.map(source => Observable.from(source).subscribe({ next(v) { observer.next(v); }, error(e) { observer.error(e); }, complete() { if (--count === 0) observer.complete(); }, })); return () => subscriptions.forEach(s => s.unsubscribe()); }); } // Emits arrays containing the most current values from each input export function combineLatest(...sources) { return new Observable(observer => { if (sources.length === 0) return Observable.from([]); let count = sources.length; let seen = new Set(); let seenAll = false; let values = sources.map(() => undefined); let subscriptions = sources.map((source, index) => Observable.from(source).subscribe({ next(v) { values[index] = v; if (!seenAll) { seen.add(index); if (seen.size !== sources.length) return; seen = null; seenAll = true; } observer.next(Array.from(values)); }, error(e) { observer.error(e); }, complete() { if (--count === 0) observer.complete(); }, })); return () => subscriptions.forEach(s => s.unsubscribe()); }); } // Emits arrays containing the matching index values from each input export function zip(...sources) { return new Observable(observer => { if (sources.length === 0) return Observable.from([]); let queues = sources.map(() => []); function done() { return queues.some((q, i) => q.length === 0 && subscriptions[i].closed); } let subscriptions = sources.map((source, index) => Observable.from(source).subscribe({ next(v) { queues[index].push(v); if (queues.every(q => q.length > 0)) { observer.next(queues.map(q => q.shift())); if (done()) observer.complete(); } }, error(e) { observer.error(e); }, complete() { if (done()) observer.complete(); }, })); return () => subscriptions.forEach(s => s.unsubscribe()); }); } apollo-server-demo/node_modules/zen-observable/src/Observable.js 0000644 0001750 0000144 00000025540 03560116604 024565 0 ustar andreh users // === Symbol Support === const hasSymbols = () => typeof Symbol === 'function'; const hasSymbol = name => hasSymbols() && Boolean(Symbol[name]); const getSymbol = name => hasSymbol(name) ? Symbol[name] : '@@' + name; if (hasSymbols() && !hasSymbol('observable')) { Symbol.observable = Symbol('observable'); } const SymbolIterator = getSymbol('iterator'); const SymbolObservable = getSymbol('observable'); const SymbolSpecies = getSymbol('species'); // === Abstract Operations === function getMethod(obj, key) { let value = obj[key]; if (value == null) return undefined; if (typeof value !== 'function') throw new TypeError(value + ' is not a function'); return value; } function getSpecies(obj) { let ctor = obj.constructor; if (ctor !== undefined) { ctor = ctor[SymbolSpecies]; if (ctor === null) { ctor = undefined; } } return ctor !== undefined ? ctor : Observable; } function isObservable(x) { return x instanceof Observable; // SPEC: Brand check } function hostReportError(e) { if (hostReportError.log) { hostReportError.log(e); } else { setTimeout(() => { throw e }); } } function enqueue(fn) { Promise.resolve().then(() => { try { fn() } catch (e) { hostReportError(e) } }); } function cleanupSubscription(subscription) { let cleanup = subscription._cleanup; if (cleanup === undefined) return; subscription._cleanup = undefined; if (!cleanup) { return; } try { if (typeof cleanup === 'function') { cleanup(); } else { let unsubscribe = getMethod(cleanup, 'unsubscribe'); if (unsubscribe) { unsubscribe.call(cleanup); } } } catch (e) { hostReportError(e); } } function closeSubscription(subscription) { subscription._observer = undefined; subscription._queue = undefined; subscription._state = 'closed'; } function flushSubscription(subscription) { let queue = subscription._queue; if (!queue) { return; } subscription._queue = undefined; subscription._state = 'ready'; for (let i = 0; i < queue.length; ++i) { notifySubscription(subscription, queue[i].type, queue[i].value); if (subscription._state === 'closed') break; } } function notifySubscription(subscription, type, value) { subscription._state = 'running'; let observer = subscription._observer; try { let m = getMethod(observer, type); switch (type) { case 'next': if (m) m.call(observer, value); break; case 'error': closeSubscription(subscription); if (m) m.call(observer, value); else throw value; break; case 'complete': closeSubscription(subscription); if (m) m.call(observer); break; } } catch (e) { hostReportError(e); } if (subscription._state === 'closed') cleanupSubscription(subscription); else if (subscription._state === 'running') subscription._state = 'ready'; } function onNotify(subscription, type, value) { if (subscription._state === 'closed') return; if (subscription._state === 'buffering') { subscription._queue.push({ type, value }); return; } if (subscription._state !== 'ready') { subscription._state = 'buffering'; subscription._queue = [{ type, value }]; enqueue(() => flushSubscription(subscription)); return; } notifySubscription(subscription, type, value); } class Subscription { constructor(observer, subscriber) { // ASSERT: observer is an object // ASSERT: subscriber is callable this._cleanup = undefined; this._observer = observer; this._queue = undefined; this._state = 'initializing'; let subscriptionObserver = new SubscriptionObserver(this); try { this._cleanup = subscriber.call(undefined, subscriptionObserver); } catch (e) { subscriptionObserver.error(e); } if (this._state === 'initializing') this._state = 'ready'; } get closed() { return this._state === 'closed'; } unsubscribe() { if (this._state !== 'closed') { closeSubscription(this); cleanupSubscription(this); } } } class SubscriptionObserver { constructor(subscription) { this._subscription = subscription } get closed() { return this._subscription._state === 'closed' } next(value) { onNotify(this._subscription, 'next', value) } error(value) { onNotify(this._subscription, 'error', value) } complete() { onNotify(this._subscription, 'complete') } } export class Observable { constructor(subscriber) { if (!(this instanceof Observable)) throw new TypeError('Observable cannot be called as a function'); if (typeof subscriber !== 'function') throw new TypeError('Observable initializer must be a function'); this._subscriber = subscriber; } subscribe(observer) { if (typeof observer !== 'object' || observer === null) { observer = { next: observer, error: arguments[1], complete: arguments[2], }; } return new Subscription(observer, this._subscriber); } forEach(fn) { return new Promise((resolve, reject) => { if (typeof fn !== 'function') { reject(new TypeError(fn + ' is not a function')); return; } function done() { subscription.unsubscribe(); resolve(); } let subscription = this.subscribe({ next(value) { try { fn(value, done); } catch (e) { reject(e); subscription.unsubscribe(); } }, error: reject, complete: resolve, }); }); } map(fn) { if (typeof fn !== 'function') throw new TypeError(fn + ' is not a function'); let C = getSpecies(this); return new C(observer => this.subscribe({ next(value) { try { value = fn(value) } catch (e) { return observer.error(e) } observer.next(value); }, error(e) { observer.error(e) }, complete() { observer.complete() }, })); } filter(fn) { if (typeof fn !== 'function') throw new TypeError(fn + ' is not a function'); let C = getSpecies(this); return new C(observer => this.subscribe({ next(value) { try { if (!fn(value)) return; } catch (e) { return observer.error(e) } observer.next(value); }, error(e) { observer.error(e) }, complete() { observer.complete() }, })); } reduce(fn) { if (typeof fn !== 'function') throw new TypeError(fn + ' is not a function'); let C = getSpecies(this); let hasSeed = arguments.length > 1; let hasValue = false; let seed = arguments[1]; let acc = seed; return new C(observer => this.subscribe({ next(value) { let first = !hasValue; hasValue = true; if (!first || hasSeed) { try { acc = fn(acc, value) } catch (e) { return observer.error(e) } } else { acc = value; } }, error(e) { observer.error(e) }, complete() { if (!hasValue && !hasSeed) return observer.error(new TypeError('Cannot reduce an empty sequence')); observer.next(acc); observer.complete(); }, })); } concat(...sources) { let C = getSpecies(this); return new C(observer => { let subscription; let index = 0; function startNext(next) { subscription = next.subscribe({ next(v) { observer.next(v) }, error(e) { observer.error(e) }, complete() { if (index === sources.length) { subscription = undefined; observer.complete(); } else { startNext(C.from(sources[index++])); } }, }); } startNext(this); return () => { if (subscription) { subscription.unsubscribe(); subscription = undefined; } }; }); } flatMap(fn) { if (typeof fn !== 'function') throw new TypeError(fn + ' is not a function'); let C = getSpecies(this); return new C(observer => { let subscriptions = []; let outer = this.subscribe({ next(value) { if (fn) { try { value = fn(value) } catch (e) { return observer.error(e) } } let inner = C.from(value).subscribe({ next(value) { observer.next(value) }, error(e) { observer.error(e) }, complete() { let i = subscriptions.indexOf(inner); if (i >= 0) subscriptions.splice(i, 1); completeIfDone(); }, }); subscriptions.push(inner); }, error(e) { observer.error(e) }, complete() { completeIfDone() }, }); function completeIfDone() { if (outer.closed && subscriptions.length === 0) observer.complete(); } return () => { subscriptions.forEach(s => s.unsubscribe()); outer.unsubscribe(); }; }); } [SymbolObservable]() { return this } static from(x) { let C = typeof this === 'function' ? this : Observable; if (x == null) throw new TypeError(x + ' is not an object'); let method = getMethod(x, SymbolObservable); if (method) { let observable = method.call(x); if (Object(observable) !== observable) throw new TypeError(observable + ' is not an object'); if (isObservable(observable) && observable.constructor === C) return observable; return new C(observer => observable.subscribe(observer)); } if (hasSymbol('iterator')) { method = getMethod(x, SymbolIterator); if (method) { return new C(observer => { enqueue(() => { if (observer.closed) return; for (let item of method.call(x)) { observer.next(item); if (observer.closed) return; } observer.complete(); }); }); } } if (Array.isArray(x)) { return new C(observer => { enqueue(() => { if (observer.closed) return; for (let i = 0; i < x.length; ++i) { observer.next(x[i]); if (observer.closed) return; } observer.complete(); }); }); } throw new TypeError(x + ' is not observable'); } static of(...items) { let C = typeof this === 'function' ? this : Observable; return new C(observer => { enqueue(() => { if (observer.closed) return; for (let i = 0; i < items.length; ++i) { observer.next(items[i]); if (observer.closed) return; } observer.complete(); }); }); } static get [SymbolSpecies]() { return this } } if (hasSymbols()) { Object.defineProperty(Observable, Symbol('extensions'), { value: { symbol: SymbolObservable, hostReportError, }, configurable: true, }); } apollo-server-demo/node_modules/zen-observable/README.md 0000644 0001750 0000144 00000011134 03560116604 022625 0 ustar andreh users # zen-observable An implementation of Observables for JavaScript. Requires Promises or a Promise polyfill. ## Install ```sh npm install zen-observable ``` ## Usage ```js import Observable from 'zen-observable'; Observable.of(1, 2, 3).subscribe(x => console.log(x)); ``` ## API ### new Observable(subscribe) ```js let observable = new Observable(observer => { // Emit a single value after 1 second let timer = setTimeout(() => { observer.next('hello'); observer.complete(); }, 1000); // On unsubscription, cancel the timer return () => clearTimeout(timer); }); ``` Creates a new Observable object using the specified subscriber function. The subscriber function is called whenever the `subscribe` method of the observable object is invoked. The subscriber function is passed an *observer* object which has the following methods: - `next(value)` Sends the next value in the sequence. - `error(exception)` Terminates the sequence with an exception. - `complete()` Terminates the sequence successfully. - `closed` A boolean property whose value is `true` if the observer's subscription is closed. The subscriber function can optionally return either a cleanup function or a subscription object. If it returns a cleanup function, that function will be called when the subscription has closed. If it returns a subscription object, then the subscription's `unsubscribe` method will be invoked when the subscription has closed. ### Observable.of(...items) ```js // Logs 1, 2, 3 Observable.of(1, 2, 3).subscribe(x => { console.log(x); }); ``` Returns an observable which will emit each supplied argument. ### Observable.from(value) ```js let list = [1, 2, 3]; // Iterate over an object Observable.from(list).subscribe(x => { console.log(x); }); ``` ```js // Convert something 'observable' to an Observable instance Observable.from(otherObservable).subscribe(x => { console.log(x); }); ``` Converts `value` to an Observable. - If `value` is an implementation of Observable, then it is converted to an instance of Observable as defined by this library. - Otherwise, it is converted to an Observable which synchronously iterates over `value`. ### observable.subscribe([observer]) ```js let subscription = observable.subscribe({ next(x) { console.log(x) }, error(err) { console.log(`Finished with error: ${ err }`) }, complete() { console.log('Finished') } }); ``` Subscribes to the observable. Observer objects may have any of the following methods: - `next(value)` Receives the next value of the sequence. - `error(exception)` Receives the terminating error of the sequence. - `complete()` Called when the stream has completed successfully. Returns a subscription object that can be used to cancel the stream. ### observable.subscribe(nextCallback[, errorCallback, completeCallback]) ```js let subscription = observable.subscribe( x => console.log(x), err => console.log(`Finished with error: ${ err }`), () => console.log('Finished') ); ``` Subscribes to the observable with callback functions. Returns a subscription object that can be used to cancel the stream. ### observable.forEach(callback) ```js observable.forEach(x => { console.log(`Received value: ${ x }`); }).then(() => { console.log('Finished successfully') }).catch(err => { console.log(`Finished with error: ${ err }`); }) ``` Subscribes to the observable and returns a Promise for the completion value of the stream. The `callback` argument is called once for each value in the stream. ### observable.filter(callback) ```js Observable.of(1, 2, 3).filter(value => { return value > 2; }).subscribe(value => { console.log(value); }); // 3 ``` Returns a new Observable that emits all values which pass the test implemented by the `callback` argument. ### observable.map(callback) Returns a new Observable that emits the results of calling the `callback` argument for every value in the stream. ```js Observable.of(1, 2, 3).map(value => { return value * 2; }).subscribe(value => { console.log(value); }); // 2 // 4 // 6 ``` ### observable.reduce(callback [,initialValue]) ```js Observable.of(0, 1, 2, 3, 4).reduce((previousValue, currentValue) => { return previousValue + currentValue; }).subscribe(result => { console.log(result); }); // 10 ``` Returns a new Observable that applies a function against an accumulator and each value of the stream to reduce it to a single value. ### observable.concat(...sources) ```js Observable.of(1, 2, 3).concat( Observable.of(4, 5, 6), Observable.of(7, 8, 9) ).subscribe(result => { console.log(result); }); // 1, 2, 3, 4, 5, 6, 7, 8, 9 ``` Merges the current observable with additional observables. apollo-server-demo/node_modules/zen-observable/.editorconfig 0000644 0001750 0000144 00000000144 03560116604 024022 0 ustar andreh users root = true [*] end_of_line = lf insert_final_newline = false indent_style = space indent_size = 2 apollo-server-demo/node_modules/zen-observable/esm.js 0000644 0001750 0000144 00000000206 03560116604 022466 0 ustar andreh users import { Observable } from './src/Observable.js'; export default Observable; export { Observable }; export * from './src/extras.js'; apollo-server-demo/node_modules/zen-observable/package.json 0000644 0001750 0000144 00000001645 03560116604 023642 0 ustar andreh users { "name": "zen-observable", "version": "0.8.15", "repository": "zenparsing/zen-observable", "description": "An Implementation of ES Observables", "homepage": "https://github.com/zenparsing/zen-observable", "license": "MIT", "devDependencies": { "@babel/cli": "^7.6.0", "@babel/core": "^7.6.0", "@babel/preset-env": "^7.6.0", "@babel/register": "^7.6.0", "eslint": "^6.5.0", "mocha": "^6.2.0" }, "dependencies": {}, "scripts": { "test": "mocha --recursive --require ./scripts/mocha-require", "lint": "eslint src/*", "build": "git clean -dfX ./lib && node ./scripts/build", "prepublishOnly": "npm run lint && npm test && npm run build" } ,"_resolved": "https://registry.npmjs.org/zen-observable/-/zen-observable-0.8.15.tgz" ,"_integrity": "sha512-PQ2PC7R9rslx84ndNBZB/Dkv8V8fZEpk83RLgXtYd0fwUgEjseMn1Dgajh2x6S8QbZAFa9p2qVCEuYZNgve0dQ==" ,"_from": "zen-observable@0.8.15" } apollo-server-demo/node_modules/zen-observable/.eslintrc.js 0000644 0001750 0000144 00000002703 03560116604 023607 0 ustar andreh users module.exports = { "extends": ["eslint:recommended"], "env": { "es6": true, "node": true }, "globals": { "setTimeout": true }, "parserOptions": { "sourceType": "module" }, "rules": { "no-console": ["error", { "allow": ["warn", "error"] }], "no-unsafe-finally": ["off"], "camelcase": ["error", { "properties": "always" }], "brace-style": ["off"], "eqeqeq": ["error", "smart"], "indent": ["error", 2, { "SwitchCase": 1 }], "no-throw-literal": ["error"], "comma-spacing": ["error", { "before": false, "after": true }], "comma-style": ["error", "last"], "comma-dangle": ["error", "always-multiline"], "keyword-spacing": ["error"], "no-trailing-spaces": ["error"], "no-multi-spaces": ["error"], "no-spaced-func": ["error"], "no-whitespace-before-property": ["error"], "space-before-blocks": ["error"], "space-before-function-paren": ["error", "never"], "space-in-parens": ["error", "never"], "eol-last": ["error"], "quotes": ["error", "single", { "avoidEscape": true }], "no-implicit-globals": ["error"], "no-useless-concat": ["error"], "space-infix-ops": ["error", { "int32Hint": true }], "semi-spacing": ["error", { "before": false, "after": true }], "semi": ["error", "always", { "omitLastInOneLineBlock": true }], "object-curly-spacing": ["error", "always"], "array-bracket-spacing": ["error"], "max-len": ["error", 100] } }; apollo-server-demo/node_modules/zen-observable/scripts/ 0000755 0001750 0000144 00000000000 14067647701 023050 5 ustar andreh users apollo-server-demo/node_modules/zen-observable/scripts/mocha-require.js 0000644 0001750 0000144 00000000110 03560116604 026124 0 ustar andreh users require('@babel/register')({ plugins: require('./babel-plugins'), }); apollo-server-demo/node_modules/zen-observable/scripts/build.js 0000644 0001750 0000144 00000000315 03560116604 024471 0 ustar andreh users const { execSync } = require('child_process'); const plugins = require('./babel-plugins'); execSync('babel src --out-dir lib --plugins=' + plugins.join(','), { env: process.env, stdio: 'inherit', }); apollo-server-demo/node_modules/zen-observable/scripts/babel-plugins.js 0000644 0001750 0000144 00000001164 03560116604 026121 0 ustar andreh users module.exports = [ '@babel/plugin-transform-arrow-functions', '@babel/plugin-transform-block-scoped-functions', '@babel/plugin-transform-block-scoping', '@babel/plugin-transform-classes', '@babel/plugin-transform-computed-properties', '@babel/plugin-transform-destructuring', '@babel/plugin-transform-duplicate-keys', '@babel/plugin-transform-for-of', '@babel/plugin-transform-literals', '@babel/plugin-transform-modules-commonjs', '@babel/plugin-transform-parameters', '@babel/plugin-transform-shorthand-properties', '@babel/plugin-transform-spread', '@babel/plugin-transform-template-literals', ]; apollo-server-demo/node_modules/zen-observable/test/ 0000755 0001750 0000144 00000000000 14067647701 022340 5 ustar andreh users apollo-server-demo/node_modules/zen-observable/test/reduce.js 0000644 0001750 0000144 00000001563 03560116604 024137 0 ustar andreh users import assert from 'assert'; describe('reduce', () => { it('reduces without a seed', async () => { await Observable.from([1, 2, 3, 4, 5, 6]).reduce((a, b) => { return a + b; }).forEach(x => { assert.equal(x, 21); }); }); it('errors if empty and no seed', async () => { try { await Observable.from([]).reduce((a, b) => { return a + b; }).forEach(() => null); assert.ok(false); } catch (err) { assert.ok(true); } }); it('reduces with a seed', async () => { Observable.from([1, 2, 3, 4, 5, 6]).reduce((a, b) => { return a + b; }, 100).forEach(x => { assert.equal(x, 121); }); }); it('reduces an empty list with a seed', async () => { await Observable.from([]).reduce((a, b) => { return a + b; }, 100).forEach(x => { assert.equal(x, 100); }); }); }); apollo-server-demo/node_modules/zen-observable/test/setup.js 0000644 0001750 0000144 00000000463 03560116604 024026 0 ustar andreh users import { Observable } from '../src/Observable.js'; beforeEach(() => { global.Observable = Observable; global.hostError = null; let $extensions = Object.getOwnPropertySymbols(Observable)[1]; let { hostReportError } = Observable[$extensions]; hostReportError.log = (e => global.hostError = e); }); apollo-server-demo/node_modules/zen-observable/test/map.js 0000644 0001750 0000144 00000000436 03560116604 023443 0 ustar andreh users import assert from 'assert'; describe('map', () => { it('maps the results using the supplied callback', async () => { let list = []; await Observable.from([1, 2, 3]) .map(x => x * 2) .forEach(x => list.push(x)); assert.deepEqual(list, [2, 4, 6]); }); }); apollo-server-demo/node_modules/zen-observable/test/observer-next.js 0000644 0001750 0000144 00000007106 03560116604 025472 0 ustar andreh users import assert from 'assert'; import { testMethodProperty } from './properties.js'; describe('observer.next', () => { function getObserver(inner) { let observer; new Observable(x => { observer = x }).subscribe(inner); return observer; } it('is a method of SubscriptionObserver', () => { let observer = getObserver(); testMethodProperty(Object.getPrototypeOf(observer), 'next', { configurable: true, writable: true, length: 1, }); }); it('forwards the first argument', () => { let args; let observer = getObserver({ next(...a) { args = a } }); observer.next(1, 2); assert.deepEqual(args, [1]); }); it('does not return a value', () => { let observer = getObserver({ next() { return 1 } }); assert.equal(observer.next(), undefined); }); it('does not forward when the subscription is complete', () => { let count = 0; let observer = getObserver({ next() { count++ } }); observer.complete(); observer.next(); assert.equal(count, 0); }); it('does not forward when the subscription is cancelled', () => { let count = 0; let observer; let subscription = new Observable(x => { observer = x }).subscribe({ next() { count++ }, }); subscription.unsubscribe(); observer.next(); assert.equal(count, 0); }); it('remains closed if the subscription is cancelled from "next"', () => { let observer; let subscription = new Observable(x => { observer = x }).subscribe({ next() { subscription.unsubscribe() }, }); observer.next(); assert.equal(observer.closed, true); }); it('queues if the subscription is not initialized', async () => { let values = []; let observer; new Observable(x => { observer = x, x.next(1) }).subscribe({ next(val) { values.push(val); if (val === 1) { observer.next(3); } }, }); observer.next(2); assert.deepEqual(values, []); await null; assert.deepEqual(values, [1, 2]); await null; assert.deepEqual(values, [1, 2, 3]); }); it('drops queue if subscription is closed', async () => { let values = []; let subscription = new Observable(x => { x.next(1) }).subscribe({ next(val) { values.push(val) }, }); assert.deepEqual(values, []); subscription.unsubscribe(); await null; assert.deepEqual(values, []); }); it('queues if the observer is running', async () => { let observer; let values = []; new Observable(x => { observer = x }).subscribe({ next(val) { values.push(val); if (val === 1) observer.next(2); }, }); observer.next(1); assert.deepEqual(values, [1]); await null; assert.deepEqual(values, [1, 2]); }); it('reports error if "next" is not a method', () => { let observer = getObserver({ next: 1 }); observer.next(); assert.ok(hostError); }); it('does not report error if "next" is undefined', () => { let observer = getObserver({ next: undefined }); observer.next(); assert.ok(!hostError); }); it('does not report error if "next" is null', () => { let observer = getObserver({ next: null }); observer.next(); assert.ok(!hostError); }); it('reports error if "next" throws', () => { let error = {}; let observer = getObserver({ next() { throw error } }); observer.next(); assert.equal(hostError, error); }); it('does not close the subscription on error', () => { let observer = getObserver({ next() { throw {} } }); observer.next(); assert.equal(observer.closed, false); }); }); apollo-server-demo/node_modules/zen-observable/test/extras/ 0000755 0001750 0000144 00000000000 14067647701 023646 5 ustar andreh users apollo-server-demo/node_modules/zen-observable/test/extras/merge.js 0000644 0001750 0000144 00000000622 03560116604 025270 0 ustar andreh users import assert from 'assert'; import { parse } from './parse.js'; import { merge } from '../../src/extras.js'; describe('extras/merge', () => { it('should emit all data from each input in parallel', async () => { let output = ''; await merge( parse('a-b-c-d'), parse('-A-B-C-D') ).forEach( value => output += value ); assert.equal(output, 'aAbBcCdD'); }); }); apollo-server-demo/node_modules/zen-observable/test/extras/zip.js 0000644 0001750 0000144 00000000710 03560116604 024771 0 ustar andreh users import assert from 'assert'; import { parse } from './parse.js'; import { zip } from '../../src/extras.js'; describe('extras/zip', () => { it('should emit pairs of corresponding index values', async () => { let output = []; await zip( parse('a-b-c-d'), parse('-A-B-C-D') ).forEach( value => output.push(value.join('')) ); assert.deepEqual(output, [ 'aA', 'bB', 'cC', 'dD', ]); }); }); apollo-server-demo/node_modules/zen-observable/test/extras/combine-latest.js 0000644 0001750 0000144 00000001556 03560116604 027106 0 ustar andreh users import assert from 'assert'; import { parse } from './parse.js'; import { combineLatest } from '../../src/extras.js'; describe('extras/combineLatest', () => { it('should emit arrays containing the most recent values', async () => { let output = []; await combineLatest( parse('a-b-c-d'), parse('-A-B-C-D') ).forEach( value => output.push(value.join('')) ); assert.deepEqual(output, [ 'aA', 'bA', 'bB', 'cB', 'cC', 'dC', 'dD', ]); }); it('should emit values in the correct order', async () => { let output = []; await combineLatest( parse('-a-b-c-d'), parse('A-B-C-D') ).forEach( value => output.push(value.join('')) ); assert.deepEqual(output, [ 'aA', 'aB', 'bB', 'bC', 'cC', 'cD', 'dD', ]); }); }); apollo-server-demo/node_modules/zen-observable/test/extras/parse.js 0000644 0001750 0000144 00000000411 03560116604 025277 0 ustar andreh users export function parse(string) { return new Observable(async observer => { await null; for (let char of string) { if (observer.closed) return; else if (char !== '-') observer.next(char); await null; } observer.complete(); }); } apollo-server-demo/node_modules/zen-observable/test/flat-map.js 0000644 0001750 0000144 00000000742 03560116604 024367 0 ustar andreh users import assert from 'assert'; describe('flatMap', () => { it('maps and flattens the results using the supplied callback', async () => { let list = []; await Observable.of('a', 'b', 'c').flatMap(x => Observable.of(1, 2, 3).map(y => [x, y]) ).forEach(x => list.push(x)); assert.deepEqual(list, [ ['a', 1], ['a', 2], ['a', 3], ['b', 1], ['b', 2], ['b', 3], ['c', 1], ['c', 2], ['c', 3], ]); }); }); apollo-server-demo/node_modules/zen-observable/test/subscribe.js 0000644 0001750 0000144 00000006644 03560116604 024656 0 ustar andreh users import assert from 'assert'; import { testMethodProperty } from './properties.js'; describe('subscribe', () => { it('is a method of Observable.prototype', () => { testMethodProperty(Observable.prototype, 'subscribe', { configurable: true, writable: true, length: 1, }); }); it('accepts an observer argument', () => { let observer; let nextValue; new Observable(x => observer = x).subscribe({ next(v) { nextValue = v }, }); observer.next(1); assert.equal(nextValue, 1); }); it('accepts a next function argument', () => { let observer; let nextValue; new Observable(x => observer = x).subscribe( v => nextValue = v ); observer.next(1); assert.equal(nextValue, 1); }); it('accepts an error function argument', () => { let observer; let errorValue; let error = {}; new Observable(x => observer = x).subscribe( null, e => errorValue = e ); observer.error(error); assert.equal(errorValue, error); }); it('accepts a complete function argument', () => { let observer; let completed = false; new Observable(x => observer = x).subscribe( null, null, () => completed = true ); observer.complete(); assert.equal(completed, true); }); it('uses function overload if first argument is null', () => { let observer; let completed = false; new Observable(x => observer = x).subscribe( null, null, () => completed = true ); observer.complete(); assert.equal(completed, true); }); it('uses function overload if first argument is undefined', () => { let observer; let completed = false; new Observable(x => observer = x).subscribe( undefined, null, () => completed = true ); observer.complete(); assert.equal(completed, true); }); it('uses function overload if first argument is a primative', () => { let observer; let completed = false; new Observable(x => observer = x).subscribe( 'abc', null, () => completed = true ); observer.complete(); assert.equal(completed, true); }); it('enqueues a job to send error if subscriber throws', async () => { let error = {}; let errorValue = undefined; new Observable(() => { throw error }).subscribe({ error(e) { errorValue = e }, }); assert.equal(errorValue, undefined); await null; assert.equal(errorValue, error); }); it('does not send error if unsubscribed', async () => { let error = {}; let errorValue = undefined; let subscription = new Observable(() => { throw error }).subscribe({ error(e) { errorValue = e }, }); subscription.unsubscribe(); assert.equal(errorValue, undefined); await null; assert.equal(errorValue, undefined); }); it('accepts a cleanup function from the subscriber function', () => { let cleanupCalled = false; let subscription = new Observable(() => { return () => cleanupCalled = true; }).subscribe(); subscription.unsubscribe(); assert.equal(cleanupCalled, true); }); it('accepts a subscription object from the subscriber function', () => { let cleanupCalled = false; let subscription = new Observable(() => { return { unsubscribe() { cleanupCalled = true }, }; }).subscribe(); subscription.unsubscribe(); assert.equal(cleanupCalled, true); }); }); apollo-server-demo/node_modules/zen-observable/test/observer-complete.js 0000644 0001750 0000144 00000007510 03560116604 026323 0 ustar andreh users import assert from 'assert'; import { testMethodProperty } from './properties.js'; describe('observer.complete', () => { function getObserver(inner) { let observer; new Observable(x => { observer = x }).subscribe(inner); return observer; } it('is a method of SubscriptionObserver', () => { let observer = getObserver(); testMethodProperty(Object.getPrototypeOf(observer), 'complete', { configurable: true, writable: true, length: 0, }); }); it('does not forward arguments', () => { let args; let observer = getObserver({ complete(...a) { args = a } }); observer.complete(1); assert.deepEqual(args, []); }); it('does not return a value', () => { let observer = getObserver({ complete() { return 1 } }); assert.equal(observer.complete(), undefined); }); it('does not forward when the subscription is complete', () => { let count = 0; let observer = getObserver({ complete() { count++ } }); observer.complete(); observer.complete(); assert.equal(count, 1); }); it('does not forward when the subscription is cancelled', () => { let count = 0; let observer; let subscription = new Observable(x => { observer = x }).subscribe({ complete() { count++ }, }); subscription.unsubscribe(); observer.complete(); assert.equal(count, 0); }); it('queues if the subscription is not initialized', async () => { let completed = false; new Observable(x => { x.complete() }).subscribe({ complete() { completed = true }, }); assert.equal(completed, false); await null; assert.equal(completed, true); }); it('queues if the observer is running', async () => { let observer; let completed = false new Observable(x => { observer = x }).subscribe({ next() { observer.complete() }, complete() { completed = true }, }); observer.next(); assert.equal(completed, false); await null; assert.equal(completed, true); }); it('closes the subscription before invoking inner observer', () => { let closed; let observer = getObserver({ complete() { closed = observer.closed }, }); observer.complete(); assert.equal(closed, true); }); it('reports error if "complete" is not a method', () => { let observer = getObserver({ complete: 1 }); observer.complete(); assert.ok(hostError instanceof Error); }); it('does not report error if "complete" is undefined', () => { let observer = getObserver({ complete: undefined }); observer.complete(); assert.ok(!hostError); }); it('does not report error if "complete" is null', () => { let observer = getObserver({ complete: null }); observer.complete(); assert.ok(!hostError); }); it('reports error if "complete" throws', () => { let error = {}; let observer = getObserver({ complete() { throw error } }); observer.complete(); assert.equal(hostError, error); }); it('calls the cleanup method after "complete"', () => { let calls = []; let observer; new Observable(x => { observer = x; return () => { calls.push('cleanup') }; }).subscribe({ complete() { calls.push('complete') }, }); observer.complete(); assert.deepEqual(calls, ['complete', 'cleanup']); }); it('calls the cleanup method if there is no "complete"', () => { let calls = []; let observer; new Observable(x => { observer = x; return () => { calls.push('cleanup') }; }).subscribe({}); observer.complete(); assert.deepEqual(calls, ['cleanup']); }); it('reports error if the cleanup function throws', () => { let error = {}; let observer; new Observable(x => { observer = x; return () => { throw error }; }).subscribe(); observer.complete(); assert.equal(hostError, error); }); }); apollo-server-demo/node_modules/zen-observable/test/constructor.js 0000644 0001750 0000144 00000001772 03560116604 025257 0 ustar andreh users import assert from 'assert'; import { testMethodProperty } from './properties.js'; describe('constructor', () => { it('throws if called as a function', () => { assert.throws(() => Observable(() => {})); assert.throws(() => Observable.call({}, () => {})); }); it('throws if the argument is not callable', () => { assert.throws(() => new Observable({})); assert.throws(() => new Observable()); assert.throws(() => new Observable(1)); assert.throws(() => new Observable('string')); }); it('accepts a function argument', () => { let result = new Observable(() => {}); assert.ok(result instanceof Observable); }); it('is the value of Observable.prototype.constructor', () => { testMethodProperty(Observable.prototype, 'constructor', { configurable: true, writable: true, length: 1, }); }); it('does not call the subscriber function', () => { let called = 0; new Observable(() => { called++ }); assert.equal(called, 0); }); }); apollo-server-demo/node_modules/zen-observable/test/observer-error.js 0000644 0001750 0000144 00000007227 03560116604 025651 0 ustar andreh users import assert from 'assert'; import { testMethodProperty } from './properties.js'; describe('observer.error', () => { function getObserver(inner) { let observer; new Observable(x => { observer = x }).subscribe(inner); return observer; } it('is a method of SubscriptionObserver', () => { let observer = getObserver(); testMethodProperty(Object.getPrototypeOf(observer), 'error', { configurable: true, writable: true, length: 1, }); }); it('forwards the argument', () => { let args; let observer = getObserver({ error(...a) { args = a } }); observer.error(1); assert.deepEqual(args, [1]); }); it('does not return a value', () => { let observer = getObserver({ error() { return 1 } }); assert.equal(observer.error(), undefined); }); it('does not throw when the subscription is complete', () => { let observer = getObserver({ error() {} }); observer.complete(); observer.error('error'); }); it('does not throw when the subscription is cancelled', () => { let observer; let subscription = new Observable(x => { observer = x }).subscribe({ error() {}, }); subscription.unsubscribe(); observer.error(1); assert.ok(!hostError); }); it('queues if the subscription is not initialized', async () => { let error; new Observable(x => { x.error({}) }).subscribe({ error(err) { error = err }, }); assert.equal(error, undefined); await null; assert.ok(error); }); it('queues if the observer is running', async () => { let observer; let error; new Observable(x => { observer = x }).subscribe({ next() { observer.error({}) }, error(e) { error = e }, }); observer.next(); assert.ok(!error); await null; assert.ok(error); }); it('closes the subscription before invoking inner observer', () => { let closed; let observer = getObserver({ error() { closed = observer.closed }, }); observer.error(1); assert.equal(closed, true); }); it('reports an error if "error" is not a method', () => { let observer = getObserver({ error: 1 }); observer.error(1); assert.ok(hostError); }); it('reports an error if "error" is undefined', () => { let error = {}; let observer = getObserver({ error: undefined }); observer.error(error); assert.equal(hostError, error); }); it('reports an error if "error" is null', () => { let error = {}; let observer = getObserver({ error: null }); observer.error(error); assert.equal(hostError, error); }); it('reports error if "error" throws', () => { let error = {}; let observer = getObserver({ error() { throw error } }); observer.error(1); assert.equal(hostError, error); }); it('calls the cleanup method after "error"', () => { let calls = []; let observer; new Observable(x => { observer = x; return () => { calls.push('cleanup') }; }).subscribe({ error() { calls.push('error') }, }); observer.error(); assert.deepEqual(calls, ['error', 'cleanup']); }); it('calls the cleanup method if there is no "error"', () => { let calls = []; let observer; new Observable(x => { observer = x; return () => { calls.push('cleanup') }; }).subscribe({}); try { observer.error(); } catch (err) {} assert.deepEqual(calls, ['cleanup']); }); it('reports error if the cleanup function throws', () => { let error = {}; let observer; new Observable(x => { observer = x; return () => { throw error }; }).subscribe(); observer.error(1); assert.equal(hostError, error); }); }); apollo-server-demo/node_modules/zen-observable/test/from.js 0000644 0001750 0000144 00000005001 03560116604 023622 0 ustar andreh users import assert from 'assert'; import { testMethodProperty } from './properties.js'; describe('from', () => { const iterable = { *[Symbol.iterator]() { yield 1; yield 2; yield 3; }, }; it('is a method on Observable', () => { testMethodProperty(Observable, 'from', { configurable: true, writable: true, length: 1, }); }); it('throws if the argument is null', () => { assert.throws(() => Observable.from(null)); }); it('throws if the argument is undefined', () => { assert.throws(() => Observable.from(undefined)); }); it('throws if the argument is not observable or iterable', () => { assert.throws(() => Observable.from({})); }); describe('observables', () => { it('returns the input if the constructor matches "this"', () => { let ctor = function() {}; let observable = new Observable(() => {}); observable.constructor = ctor; assert.equal(Observable.from.call(ctor, observable), observable); }); it('wraps the input if it is not an instance of Observable', () => { let obj = { 'constructor': Observable, [Symbol.observable]() { return this }, }; assert.ok(Observable.from(obj) !== obj); }); it('throws if @@observable property is not a method', () => { assert.throws(() => Observable.from({ [Symbol.observable]: 1 })); }); it('returns an observable wrapping @@observable result', () => { let inner = { subscribe(x) { observer = x; return () => { cleanupCalled = true }; }, }; let observer; let cleanupCalled = true; let observable = Observable.from({ [Symbol.observable]() { return inner }, }); observable.subscribe(); assert.equal(typeof observer.next, 'function'); observer.complete(); assert.equal(cleanupCalled, true); }); }); describe('iterables', () => { it('throws if @@iterator is not a method', () => { assert.throws(() => Observable.from({ [Symbol.iterator]: 1 })); }); it('returns an observable wrapping iterables', async () => { let calls = []; let subscription = Observable.from(iterable).subscribe({ next(v) { calls.push(['next', v]) }, complete() { calls.push(['complete']) }, }); assert.deepEqual(calls, []); await null; assert.deepEqual(calls, [ ['next', 1], ['next', 2], ['next', 3], ['complete'], ]); }); }); }); apollo-server-demo/node_modules/zen-observable/test/properties.js 0000644 0001750 0000144 00000002347 03560116604 025065 0 ustar andreh users import assert from 'assert'; export function testMethodProperty(object, key, options) { let desc = Object.getOwnPropertyDescriptor(object, key); let { enumerable = false, configurable = false, writable = false, length } = options; assert.ok(desc, `Property ${ key.toString() } exists`); if (options.get || options.set) { if (options.get) { assert.equal(typeof desc.get, 'function', 'Getter is a function'); assert.equal(desc.get.length, 0, 'Getter length is 0'); } else { assert.equal(desc.get, undefined, 'Getter is undefined'); } if (options.set) { assert.equal(typeof desc.set, 'function', 'Setter is a function'); assert.equal(desc.set.length, 1, 'Setter length is 1'); } else { assert.equal(desc.set, undefined, 'Setter is undefined'); } } else { assert.equal(typeof desc.value, 'function', 'Value is a function'); assert.equal(desc.value.length, length, `Function length is ${ length }`); assert.equal(desc.writable, writable, `Writable property is correct ${ writable }`); } assert.equal(desc.enumerable, enumerable, `Enumerable property is ${ enumerable }`); assert.equal(desc.configurable, configurable, `Configurable property is ${ configurable }`); } apollo-server-demo/node_modules/zen-observable/test/observer-closed.js 0000644 0001750 0000144 00000001771 03560116604 025767 0 ustar andreh users import assert from 'assert'; import { testMethodProperty } from './properties.js'; describe('observer.closed', () => { it('is a getter on SubscriptionObserver.prototype', () => { let observer; new Observable(x => { observer = x }).subscribe(); testMethodProperty(Object.getPrototypeOf(observer), 'closed', { get: true, configurable: true, writable: true, length: 1 }); }); it('returns false when the subscription is open', () => { new Observable(observer => { assert.equal(observer.closed, false); }).subscribe(); }); it('returns true when the subscription is completed', () => { let observer; new Observable(x => { observer = x; }).subscribe(); observer.complete(); assert.equal(observer.closed, true); }); it('returns true when the subscription is errored', () => { let observer; new Observable(x => { observer = x; }).subscribe(null, () => {}); observer.error(); assert.equal(observer.closed, true); }); }); apollo-server-demo/node_modules/zen-observable/test/species.js 0000644 0001750 0000144 00000001660 03560116604 024321 0 ustar andreh users import assert from 'assert'; describe('species', () => { it('uses Observable when constructor is undefined', () => { let instance = new Observable(() => {}); instance.constructor = undefined; assert.ok(instance.map(x => x) instanceof Observable); }); it('uses Observable if species is null', () => { let instance = new Observable(() => {}); instance.constructor = { [Symbol.species]: null }; assert.ok(instance.map(x => x) instanceof Observable); }); it('uses Observable if species is undefined', () => { let instance = new Observable(() => {}); instance.constructor = { [Symbol.species]: undefined }; assert.ok(instance.map(x => x) instanceof Observable); }); it('uses value of Symbol.species', () => { function ctor() {} let instance = new Observable(() => {}); instance.constructor = { [Symbol.species]: ctor }; assert.ok(instance.map(x => x) instanceof ctor); }); }); apollo-server-demo/node_modules/zen-observable/test/filter.js 0000644 0001750 0000144 00000000456 03560116604 024155 0 ustar andreh users import assert from 'assert'; describe('filter', () => { it('filters the results using the supplied callback', async () => { let list = []; await Observable .from([1, 2, 3, 4]) .filter(x => x > 2) .forEach(x => list.push(x)); assert.deepEqual(list, [3, 4]); }); }); apollo-server-demo/node_modules/zen-observable/test/concat.js 0000644 0001750 0000144 00000001407 03560116604 024134 0 ustar andreh users import assert from 'assert'; describe('concat', () => { it('concatenates the supplied Observable arguments', async () => { let list = []; await Observable .from([1, 2, 3, 4]) .concat(Observable.of(5, 6, 7)) .forEach(x => list.push(x)); assert.deepEqual(list, [1, 2, 3, 4, 5, 6, 7]); }); it('can be used multiple times to produce the same results', async () => { const list1 = []; const list2 = []; const concatenated = Observable.from([1, 2, 3, 4]) .concat(Observable.of(5, 6, 7)); await concatenated .forEach(x => list1.push(x)); await concatenated .forEach(x => list2.push(x)); assert.deepEqual(list1, [1, 2, 3, 4, 5, 6, 7]); assert.deepEqual(list2, [1, 2, 3, 4, 5, 6, 7]); }); }); apollo-server-demo/node_modules/zen-observable/test/for-each.js 0000644 0001750 0000144 00000003301 03560116604 024344 0 ustar andreh users import assert from 'assert'; describe('forEach', () => { it('rejects if the argument is not a function', async () => { let promise = Observable.of(1, 2, 3).forEach(); try { await promise; assert.ok(false); } catch (err) { assert.equal(err.name, 'TypeError'); } }); it('rejects if the callback throws', async () => { let error = {}; try { await Observable.of(1, 2, 3).forEach(x => { throw error }); assert.ok(false); } catch (err) { assert.equal(err, error); } }); it('does not execute callback after callback throws', async () => { let calls = []; try { await Observable.of(1, 2, 3).forEach(x => { calls.push(x); throw {}; }); assert.ok(false); } catch (err) { assert.deepEqual(calls, [1]); } }); it('rejects if the producer calls error', async () => { let error = {}; try { let observer; let promise = new Observable(x => { observer = x }).forEach(() => {}); observer.error(error); await promise; assert.ok(false); } catch (err) { assert.equal(err, error); } }); it('resolves with undefined if the producer calls complete', async () => { let observer; let promise = new Observable(x => { observer = x }).forEach(() => {}); observer.complete(); assert.equal(await promise, undefined); }); it('provides a cancellation function as the second argument', async () => { let observer; let results = []; await Observable.of(1, 2, 3).forEach((value, cancel) => { results.push(value); if (value > 1) { return cancel(); } }); assert.deepEqual(results, [1, 2]); }); }); apollo-server-demo/node_modules/zen-observable/test/subscription.js 0000644 0001750 0000144 00000002120 03560116604 025402 0 ustar andreh users import assert from 'assert'; import { testMethodProperty } from './properties.js'; describe('subscription', () => { function getSubscription(subscriber = () => {}) { return new Observable(subscriber).subscribe(); } describe('unsubscribe', () => { it('is a method on Subscription.prototype', () => { let subscription = getSubscription(); testMethodProperty(Object.getPrototypeOf(subscription), 'unsubscribe', { configurable: true, writable: true, length: 0, }); }); it('reports an error if the cleanup function throws', () => { let error = {}; let subscription = getSubscription(() => { return () => { throw error }; }); subscription.unsubscribe(); assert.equal(hostError, error); }); }); describe('closed', () => { it('is a getter on Subscription.prototype', () => { let subscription = getSubscription(); testMethodProperty(Object.getPrototypeOf(subscription), 'closed', { configurable: true, writable: true, get: true, }); }); }); }); apollo-server-demo/node_modules/zen-observable/test/of.js 0000644 0001750 0000144 00000001562 03560116604 023273 0 ustar andreh users import assert from 'assert'; import { testMethodProperty } from './properties.js'; describe('of', () => { it('is a method on Observable', () => { testMethodProperty(Observable, 'of', { configurable: true, writable: true, length: 0, }); }); it('uses the this value if it is a function', () => { let usesThis = false; Observable.of.call(function() { usesThis = true; }); assert.ok(usesThis); }); it('uses Observable if the this value is not a function', () => { let result = Observable.of.call({}, 1, 2, 3, 4); assert.ok(result instanceof Observable); }); it('delivers arguments to next in a job', async () => { let values = []; let turns = 0; Observable.of(1, 2, 3, 4).subscribe(v => values.push(v)); assert.equal(values.length, 0); await null; assert.deepEqual(values, [1, 2, 3, 4]); }); }); apollo-server-demo/node_modules/zen-observable/lib/ 0000755 0001750 0000144 00000000000 14067647701 022127 5 ustar andreh users apollo-server-demo/node_modules/zen-observable/lib/extras.js 0000644 0001750 0000144 00000007077 03560116604 023773 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); exports.merge = merge; exports.combineLatest = combineLatest; exports.zip = zip; var _Observable = require("./Observable.js"); // Emits all values from all inputs in parallel function merge() { for (var _len = arguments.length, sources = new Array(_len), _key = 0; _key < _len; _key++) { sources[_key] = arguments[_key]; } return new _Observable.Observable(function (observer) { if (sources.length === 0) return _Observable.Observable.from([]); var count = sources.length; var subscriptions = sources.map(function (source) { return _Observable.Observable.from(source).subscribe({ next: function (v) { observer.next(v); }, error: function (e) { observer.error(e); }, complete: function () { if (--count === 0) observer.complete(); } }); }); return function () { return subscriptions.forEach(function (s) { return s.unsubscribe(); }); }; }); } // Emits arrays containing the most current values from each input function combineLatest() { for (var _len2 = arguments.length, sources = new Array(_len2), _key2 = 0; _key2 < _len2; _key2++) { sources[_key2] = arguments[_key2]; } return new _Observable.Observable(function (observer) { if (sources.length === 0) return _Observable.Observable.from([]); var count = sources.length; var seen = new Set(); var seenAll = false; var values = sources.map(function () { return undefined; }); var subscriptions = sources.map(function (source, index) { return _Observable.Observable.from(source).subscribe({ next: function (v) { values[index] = v; if (!seenAll) { seen.add(index); if (seen.size !== sources.length) return; seen = null; seenAll = true; } observer.next(Array.from(values)); }, error: function (e) { observer.error(e); }, complete: function () { if (--count === 0) observer.complete(); } }); }); return function () { return subscriptions.forEach(function (s) { return s.unsubscribe(); }); }; }); } // Emits arrays containing the matching index values from each input function zip() { for (var _len3 = arguments.length, sources = new Array(_len3), _key3 = 0; _key3 < _len3; _key3++) { sources[_key3] = arguments[_key3]; } return new _Observable.Observable(function (observer) { if (sources.length === 0) return _Observable.Observable.from([]); var queues = sources.map(function () { return []; }); function done() { return queues.some(function (q, i) { return q.length === 0 && subscriptions[i].closed; }); } var subscriptions = sources.map(function (source, index) { return _Observable.Observable.from(source).subscribe({ next: function (v) { queues[index].push(v); if (queues.every(function (q) { return q.length > 0; })) { observer.next(queues.map(function (q) { return q.shift(); })); if (done()) observer.complete(); } }, error: function (e) { observer.error(e); }, complete: function () { if (done()) observer.complete(); } }); }); return function () { return subscriptions.forEach(function (s) { return s.unsubscribe(); }); }; }); } apollo-server-demo/node_modules/zen-observable/lib/Observable.js 0000644 0001750 0000144 00000036670 03560116604 024552 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); exports.Observable = void 0; function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } } function _defineProperties(target, props) { for (var i = 0; i < props.length; i++) { var descriptor = props[i]; descriptor.enumerable = descriptor.enumerable || false; descriptor.configurable = true; if ("value" in descriptor) descriptor.writable = true; Object.defineProperty(target, descriptor.key, descriptor); } } function _createClass(Constructor, protoProps, staticProps) { if (protoProps) _defineProperties(Constructor.prototype, protoProps); if (staticProps) _defineProperties(Constructor, staticProps); return Constructor; } // === Symbol Support === var hasSymbols = function () { return typeof Symbol === 'function'; }; var hasSymbol = function (name) { return hasSymbols() && Boolean(Symbol[name]); }; var getSymbol = function (name) { return hasSymbol(name) ? Symbol[name] : '@@' + name; }; if (hasSymbols() && !hasSymbol('observable')) { Symbol.observable = Symbol('observable'); } var SymbolIterator = getSymbol('iterator'); var SymbolObservable = getSymbol('observable'); var SymbolSpecies = getSymbol('species'); // === Abstract Operations === function getMethod(obj, key) { var value = obj[key]; if (value == null) return undefined; if (typeof value !== 'function') throw new TypeError(value + ' is not a function'); return value; } function getSpecies(obj) { var ctor = obj.constructor; if (ctor !== undefined) { ctor = ctor[SymbolSpecies]; if (ctor === null) { ctor = undefined; } } return ctor !== undefined ? ctor : Observable; } function isObservable(x) { return x instanceof Observable; // SPEC: Brand check } function hostReportError(e) { if (hostReportError.log) { hostReportError.log(e); } else { setTimeout(function () { throw e; }); } } function enqueue(fn) { Promise.resolve().then(function () { try { fn(); } catch (e) { hostReportError(e); } }); } function cleanupSubscription(subscription) { var cleanup = subscription._cleanup; if (cleanup === undefined) return; subscription._cleanup = undefined; if (!cleanup) { return; } try { if (typeof cleanup === 'function') { cleanup(); } else { var unsubscribe = getMethod(cleanup, 'unsubscribe'); if (unsubscribe) { unsubscribe.call(cleanup); } } } catch (e) { hostReportError(e); } } function closeSubscription(subscription) { subscription._observer = undefined; subscription._queue = undefined; subscription._state = 'closed'; } function flushSubscription(subscription) { var queue = subscription._queue; if (!queue) { return; } subscription._queue = undefined; subscription._state = 'ready'; for (var i = 0; i < queue.length; ++i) { notifySubscription(subscription, queue[i].type, queue[i].value); if (subscription._state === 'closed') break; } } function notifySubscription(subscription, type, value) { subscription._state = 'running'; var observer = subscription._observer; try { var m = getMethod(observer, type); switch (type) { case 'next': if (m) m.call(observer, value); break; case 'error': closeSubscription(subscription); if (m) m.call(observer, value);else throw value; break; case 'complete': closeSubscription(subscription); if (m) m.call(observer); break; } } catch (e) { hostReportError(e); } if (subscription._state === 'closed') cleanupSubscription(subscription);else if (subscription._state === 'running') subscription._state = 'ready'; } function onNotify(subscription, type, value) { if (subscription._state === 'closed') return; if (subscription._state === 'buffering') { subscription._queue.push({ type: type, value: value }); return; } if (subscription._state !== 'ready') { subscription._state = 'buffering'; subscription._queue = [{ type: type, value: value }]; enqueue(function () { return flushSubscription(subscription); }); return; } notifySubscription(subscription, type, value); } var Subscription = /*#__PURE__*/ function () { function Subscription(observer, subscriber) { _classCallCheck(this, Subscription); // ASSERT: observer is an object // ASSERT: subscriber is callable this._cleanup = undefined; this._observer = observer; this._queue = undefined; this._state = 'initializing'; var subscriptionObserver = new SubscriptionObserver(this); try { this._cleanup = subscriber.call(undefined, subscriptionObserver); } catch (e) { subscriptionObserver.error(e); } if (this._state === 'initializing') this._state = 'ready'; } _createClass(Subscription, [{ key: "unsubscribe", value: function unsubscribe() { if (this._state !== 'closed') { closeSubscription(this); cleanupSubscription(this); } } }, { key: "closed", get: function () { return this._state === 'closed'; } }]); return Subscription; }(); var SubscriptionObserver = /*#__PURE__*/ function () { function SubscriptionObserver(subscription) { _classCallCheck(this, SubscriptionObserver); this._subscription = subscription; } _createClass(SubscriptionObserver, [{ key: "next", value: function next(value) { onNotify(this._subscription, 'next', value); } }, { key: "error", value: function error(value) { onNotify(this._subscription, 'error', value); } }, { key: "complete", value: function complete() { onNotify(this._subscription, 'complete'); } }, { key: "closed", get: function () { return this._subscription._state === 'closed'; } }]); return SubscriptionObserver; }(); var Observable = /*#__PURE__*/ function () { function Observable(subscriber) { _classCallCheck(this, Observable); if (!(this instanceof Observable)) throw new TypeError('Observable cannot be called as a function'); if (typeof subscriber !== 'function') throw new TypeError('Observable initializer must be a function'); this._subscriber = subscriber; } _createClass(Observable, [{ key: "subscribe", value: function subscribe(observer) { if (typeof observer !== 'object' || observer === null) { observer = { next: observer, error: arguments[1], complete: arguments[2] }; } return new Subscription(observer, this._subscriber); } }, { key: "forEach", value: function forEach(fn) { var _this = this; return new Promise(function (resolve, reject) { if (typeof fn !== 'function') { reject(new TypeError(fn + ' is not a function')); return; } function done() { subscription.unsubscribe(); resolve(); } var subscription = _this.subscribe({ next: function (value) { try { fn(value, done); } catch (e) { reject(e); subscription.unsubscribe(); } }, error: reject, complete: resolve }); }); } }, { key: "map", value: function map(fn) { var _this2 = this; if (typeof fn !== 'function') throw new TypeError(fn + ' is not a function'); var C = getSpecies(this); return new C(function (observer) { return _this2.subscribe({ next: function (value) { try { value = fn(value); } catch (e) { return observer.error(e); } observer.next(value); }, error: function (e) { observer.error(e); }, complete: function () { observer.complete(); } }); }); } }, { key: "filter", value: function filter(fn) { var _this3 = this; if (typeof fn !== 'function') throw new TypeError(fn + ' is not a function'); var C = getSpecies(this); return new C(function (observer) { return _this3.subscribe({ next: function (value) { try { if (!fn(value)) return; } catch (e) { return observer.error(e); } observer.next(value); }, error: function (e) { observer.error(e); }, complete: function () { observer.complete(); } }); }); } }, { key: "reduce", value: function reduce(fn) { var _this4 = this; if (typeof fn !== 'function') throw new TypeError(fn + ' is not a function'); var C = getSpecies(this); var hasSeed = arguments.length > 1; var hasValue = false; var seed = arguments[1]; var acc = seed; return new C(function (observer) { return _this4.subscribe({ next: function (value) { var first = !hasValue; hasValue = true; if (!first || hasSeed) { try { acc = fn(acc, value); } catch (e) { return observer.error(e); } } else { acc = value; } }, error: function (e) { observer.error(e); }, complete: function () { if (!hasValue && !hasSeed) return observer.error(new TypeError('Cannot reduce an empty sequence')); observer.next(acc); observer.complete(); } }); }); } }, { key: "concat", value: function concat() { var _this5 = this; for (var _len = arguments.length, sources = new Array(_len), _key = 0; _key < _len; _key++) { sources[_key] = arguments[_key]; } var C = getSpecies(this); return new C(function (observer) { var subscription; var index = 0; function startNext(next) { subscription = next.subscribe({ next: function (v) { observer.next(v); }, error: function (e) { observer.error(e); }, complete: function () { if (index === sources.length) { subscription = undefined; observer.complete(); } else { startNext(C.from(sources[index++])); } } }); } startNext(_this5); return function () { if (subscription) { subscription.unsubscribe(); subscription = undefined; } }; }); } }, { key: "flatMap", value: function flatMap(fn) { var _this6 = this; if (typeof fn !== 'function') throw new TypeError(fn + ' is not a function'); var C = getSpecies(this); return new C(function (observer) { var subscriptions = []; var outer = _this6.subscribe({ next: function (value) { if (fn) { try { value = fn(value); } catch (e) { return observer.error(e); } } var inner = C.from(value).subscribe({ next: function (value) { observer.next(value); }, error: function (e) { observer.error(e); }, complete: function () { var i = subscriptions.indexOf(inner); if (i >= 0) subscriptions.splice(i, 1); completeIfDone(); } }); subscriptions.push(inner); }, error: function (e) { observer.error(e); }, complete: function () { completeIfDone(); } }); function completeIfDone() { if (outer.closed && subscriptions.length === 0) observer.complete(); } return function () { subscriptions.forEach(function (s) { return s.unsubscribe(); }); outer.unsubscribe(); }; }); } }, { key: SymbolObservable, value: function () { return this; } }], [{ key: "from", value: function from(x) { var C = typeof this === 'function' ? this : Observable; if (x == null) throw new TypeError(x + ' is not an object'); var method = getMethod(x, SymbolObservable); if (method) { var observable = method.call(x); if (Object(observable) !== observable) throw new TypeError(observable + ' is not an object'); if (isObservable(observable) && observable.constructor === C) return observable; return new C(function (observer) { return observable.subscribe(observer); }); } if (hasSymbol('iterator')) { method = getMethod(x, SymbolIterator); if (method) { return new C(function (observer) { enqueue(function () { if (observer.closed) return; var _iteratorNormalCompletion = true; var _didIteratorError = false; var _iteratorError = undefined; try { for (var _iterator = method.call(x)[Symbol.iterator](), _step; !(_iteratorNormalCompletion = (_step = _iterator.next()).done); _iteratorNormalCompletion = true) { var _item = _step.value; observer.next(_item); if (observer.closed) return; } } catch (err) { _didIteratorError = true; _iteratorError = err; } finally { try { if (!_iteratorNormalCompletion && _iterator.return != null) { _iterator.return(); } } finally { if (_didIteratorError) { throw _iteratorError; } } } observer.complete(); }); }); } } if (Array.isArray(x)) { return new C(function (observer) { enqueue(function () { if (observer.closed) return; for (var i = 0; i < x.length; ++i) { observer.next(x[i]); if (observer.closed) return; } observer.complete(); }); }); } throw new TypeError(x + ' is not observable'); } }, { key: "of", value: function of() { for (var _len2 = arguments.length, items = new Array(_len2), _key2 = 0; _key2 < _len2; _key2++) { items[_key2] = arguments[_key2]; } var C = typeof this === 'function' ? this : Observable; return new C(function (observer) { enqueue(function () { if (observer.closed) return; for (var i = 0; i < items.length; ++i) { observer.next(items[i]); if (observer.closed) return; } observer.complete(); }); }); } }, { key: SymbolSpecies, get: function () { return this; } }]); return Observable; }(); exports.Observable = Observable; if (hasSymbols()) { Object.defineProperty(Observable, Symbol('extensions'), { value: { symbol: SymbolObservable, hostReportError: hostReportError }, configurable: true }); } apollo-server-demo/node_modules/ts-invariant/ 0000755 0001750 0000144 00000000000 14067647701 021062 5 ustar andreh users apollo-server-demo/node_modules/ts-invariant/LICENSE 0000644 0001750 0000144 00000002057 03560116604 022060 0 ustar andreh users MIT License Copyright (c) 2019 Apollo GraphQL Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/ts-invariant/README.md 0000644 0001750 0000144 00000000233 03560116604 022324 0 ustar andreh users # ts-invariant [TypeScript](https://www.typescriptlang.org) implementation of [`invariant(condition, message)`](https://www.npmjs.com/package/invariant). apollo-server-demo/node_modules/ts-invariant/tsconfig.json 0000644 0001750 0000144 00000000161 03560116604 023554 0 ustar andreh users { "extends": "../../tsconfig.json", "compilerOptions": { "rootDir": "./src", "outDir": "./lib" } } apollo-server-demo/node_modules/ts-invariant/package.json 0000644 0001750 0000144 00000002532 03560116604 023337 0 ustar andreh users { "name": "ts-invariant", "version": "0.4.4", "author": "Ben Newman <ben@apollographql.com>", "description": "TypeScript implementation of invariant(condition, message)", "license": "MIT", "main": "lib/invariant.js", "module": "lib/invariant.esm.js", "types": "lib/invariant.d.ts", "keywords": [ "invariant", "assertion", "precondition", "TypeScript" ], "homepage": "https://github.com/apollographql/invariant-packages", "repository": { "type": "git", "url": "git+https://github.com/apollographql/invariant-packages.git" }, "bugs": { "url": "https://github.com/apollographql/invariant-packages/issues" }, "scripts": { "build": "tsc && rollup -c", "mocha": "mocha --reporter spec --full-trace lib/tests.js", "prepublish": "npm run build", "test": "npm run build && npm run mocha" }, "dependencies": { "tslib": "^1.9.3" }, "devDependencies": { "@types/invariant": "^2.2.29", "invariant": "^2.2.4", "mocha": "^5.2.0", "rollup": "^1.1.2", "rollup-plugin-typescript2": "^0.19.2" }, "gitHead": "c83c2aeb7917b93751d17706d800a7ea16d8fe3e" ,"_resolved": "https://registry.npmjs.org/ts-invariant/-/ts-invariant-0.4.4.tgz" ,"_integrity": "sha512-uEtWkFM/sdZvRNNDL3Ehu4WVpwaulhwQszV8mrtcdeE8nN00BV9mAmQ88RkrBhFgl9gMgvjJLAQcZbnPXI9mlA==" ,"_from": "ts-invariant@0.4.4" } apollo-server-demo/node_modules/ts-invariant/lib/ 0000755 0001750 0000144 00000000000 14067647701 021630 5 ustar andreh users apollo-server-demo/node_modules/ts-invariant/lib/invariant.esm.js 0000644 0001750 0000144 00000005002 03560116604 024726 0 ustar andreh users import { __extends } from 'tslib'; var genericMessage = "Invariant Violation"; var _a = Object.setPrototypeOf, setPrototypeOf = _a === void 0 ? function (obj, proto) { obj.__proto__ = proto; return obj; } : _a; var InvariantError = /** @class */ (function (_super) { __extends(InvariantError, _super); function InvariantError(message) { if (message === void 0) { message = genericMessage; } var _this = _super.call(this, typeof message === "number" ? genericMessage + ": " + message + " (see https://github.com/apollographql/invariant-packages)" : message) || this; _this.framesToPop = 1; _this.name = genericMessage; setPrototypeOf(_this, InvariantError.prototype); return _this; } return InvariantError; }(Error)); function invariant(condition, message) { if (!condition) { throw new InvariantError(message); } } function wrapConsoleMethod(method) { return function () { return console[method].apply(console, arguments); }; } (function (invariant) { invariant.warn = wrapConsoleMethod("warn"); invariant.error = wrapConsoleMethod("error"); })(invariant || (invariant = {})); // Code that uses ts-invariant with rollup-plugin-invariant may want to // import this process stub to avoid errors evaluating process.env.NODE_ENV. // However, because most ESM-to-CJS compilers will rewrite the process import // as tsInvariant.process, which prevents proper replacement by minifiers, we // also attempt to define the stub globally when it is not already defined. var processStub = { env: {} }; if (typeof process === "object") { processStub = process; } else try { // Using Function to evaluate this assignment in global scope also escapes // the strict mode of the current module, thereby allowing the assignment. // Inspired by https://github.com/facebook/regenerator/pull/369. Function("stub", "process = stub")(processStub); } catch (atLeastWeTried) { // The assignment can fail if a Content Security Policy heavy-handedly // forbids Function usage. In those environments, developers should take // extra care to replace process.env.NODE_ENV in their production builds, // or define an appropriate global.process polyfill. } var invariant$1 = invariant; export default invariant$1; export { InvariantError, invariant, processStub as process }; //# sourceMappingURL=invariant.esm.js.map apollo-server-demo/node_modules/ts-invariant/lib/invariant.js 0000644 0001750 0000144 00000005143 03560116604 024151 0 ustar andreh users 'use strict'; Object.defineProperty(exports, '__esModule', { value: true }); var tslib = require('tslib'); var genericMessage = "Invariant Violation"; var _a = Object.setPrototypeOf, setPrototypeOf = _a === void 0 ? function (obj, proto) { obj.__proto__ = proto; return obj; } : _a; var InvariantError = /** @class */ (function (_super) { tslib.__extends(InvariantError, _super); function InvariantError(message) { if (message === void 0) { message = genericMessage; } var _this = _super.call(this, typeof message === "number" ? genericMessage + ": " + message + " (see https://github.com/apollographql/invariant-packages)" : message) || this; _this.framesToPop = 1; _this.name = genericMessage; setPrototypeOf(_this, InvariantError.prototype); return _this; } return InvariantError; }(Error)); function invariant(condition, message) { if (!condition) { throw new InvariantError(message); } } function wrapConsoleMethod(method) { return function () { return console[method].apply(console, arguments); }; } (function (invariant) { invariant.warn = wrapConsoleMethod("warn"); invariant.error = wrapConsoleMethod("error"); })(invariant || (invariant = {})); // Code that uses ts-invariant with rollup-plugin-invariant may want to // import this process stub to avoid errors evaluating process.env.NODE_ENV. // However, because most ESM-to-CJS compilers will rewrite the process import // as tsInvariant.process, which prevents proper replacement by minifiers, we // also attempt to define the stub globally when it is not already defined. exports.process = { env: {} }; if (typeof process === "object") { exports.process = process; } else try { // Using Function to evaluate this assignment in global scope also escapes // the strict mode of the current module, thereby allowing the assignment. // Inspired by https://github.com/facebook/regenerator/pull/369. Function("stub", "process = stub")(exports.process); } catch (atLeastWeTried) { // The assignment can fail if a Content Security Policy heavy-handedly // forbids Function usage. In those environments, developers should take // extra care to replace process.env.NODE_ENV in their production builds, // or define an appropriate global.process polyfill. } var invariant$1 = invariant; exports.default = invariant$1; exports.InvariantError = InvariantError; exports.invariant = invariant; //# sourceMappingURL=invariant.js.map apollo-server-demo/node_modules/ts-invariant/lib/invariant.esm.js.map 0000644 0001750 0000144 00000006426 03560116604 025515 0 ustar andreh users {"version":3,"file":"invariant.esm.js","sources":["../src/invariant.ts"],"sourcesContent":["const genericMessage = \"Invariant Violation\";\nconst {\n setPrototypeOf = function (obj: any, proto: any) {\n obj.__proto__ = proto;\n return obj;\n },\n} = Object as any;\n\nexport class InvariantError extends Error {\n framesToPop = 1;\n name = genericMessage;\n constructor(message: string | number = genericMessage) {\n super(\n typeof message === \"number\"\n ? `${genericMessage}: ${message} (see https://github.com/apollographql/invariant-packages)`\n : message\n );\n setPrototypeOf(this, InvariantError.prototype);\n }\n}\n\nexport function invariant(condition: any, message?: string | number) {\n if (!condition) {\n throw new InvariantError(message);\n }\n}\n\nfunction wrapConsoleMethod(method: \"warn\" | \"error\") {\n return function () {\n return console[method].apply(console, arguments as any);\n } as (...args: any[]) => void;\n}\n\nexport namespace invariant {\n export const warn = wrapConsoleMethod(\"warn\");\n export const error = wrapConsoleMethod(\"error\");\n}\n\n// Code that uses ts-invariant with rollup-plugin-invariant may want to\n// import this process stub to avoid errors evaluating process.env.NODE_ENV.\n// However, because most ESM-to-CJS compilers will rewrite the process import\n// as tsInvariant.process, which prevents proper replacement by minifiers, we\n// also attempt to define the stub globally when it is not already defined.\nlet processStub: NodeJS.Process = { env: {} } as any;\nexport { processStub as process };\nif (typeof process === \"object\") {\n processStub = process;\n} else try {\n // Using Function to evaluate this assignment in global scope also escapes\n // the strict mode of the current module, thereby allowing the assignment.\n // Inspired by https://github.com/facebook/regenerator/pull/369.\n Function(\"stub\", \"process = stub\")(processStub);\n} catch (atLeastWeTried) {\n // The assignment can fail if a Content Security Policy heavy-handedly\n // forbids Function usage. In those environments, developers should take\n // extra care to replace process.env.NODE_ENV in their production builds,\n // or define an appropriate global.process polyfill.\n}\n\nexport default invariant;\n"],"names":["tslib_1.__extends"],"mappings":";;AAAA,IAAM,cAAc,GAAG,qBAAqB,CAAC;AAE3C,IAAA,0BAGC,EAHD;;;MAGC,CACe;AAElB;IAAoCA,kCAAK;IAGvC,wBAAY,OAAyC;QAAzC,wBAAA,EAAA,wBAAyC;QAArD,YACE,kBACE,OAAO,OAAO,KAAK,QAAQ;cACpB,cAAc,UAAK,OAAO,+DAA4D;cACzF,OAAO,CACZ,SAEF;QATD,iBAAW,GAAG,CAAC,CAAC;QAChB,UAAI,GAAG,cAAc,CAAC;QAOpB,cAAc,CAAC,KAAI,EAAE,cAAc,CAAC,SAAS,CAAC,CAAC;;KAChD;IACH,qBAAC;CAXD,CAAoC,KAAK,GAWxC;SAEe,SAAS,CAAC,SAAc,EAAE,OAAyB;IACjE,IAAI,CAAC,SAAS,EAAE;QACd,MAAM,IAAI,cAAc,CAAC,OAAO,CAAC,CAAC;KACnC;CACF;AAED,SAAS,iBAAiB,CAAC,MAAwB;IACjD,OAAO;QACL,OAAO,OAAO,CAAC,MAAM,CAAC,CAAC,KAAK,CAAC,OAAO,EAAE,SAAgB,CAAC,CAAC;KAC7B,CAAC;CAC/B;AAED,WAAiB,SAAS;IACX,cAAI,GAAG,iBAAiB,CAAC,MAAM,CAAC,CAAC;IACjC,eAAK,GAAG,iBAAiB,CAAC,OAAO,CAAC,CAAC;CACjD,EAHgB,SAAS,KAAT,SAAS,QAGzB;;;;;;AAOD,IAAI,WAAW,GAAmB,EAAE,GAAG,EAAE,EAAE,EAAS,CAAC;AACrD,AACA,IAAI,OAAO,OAAO,KAAK,QAAQ,EAAE;IAC/B,WAAW,GAAG,OAAO,CAAC;CACvB;;IAAM,IAAI;;;;QAIT,QAAQ,CAAC,MAAM,EAAE,gBAAgB,CAAC,CAAC,WAAW,CAAC,CAAC;KACjD;IAAC,OAAO,cAAc,EAAE;;;;;KAKxB;AAED,kBAAe,SAAS,CAAC;;;;;"} apollo-server-demo/node_modules/ts-invariant/lib/invariant.d.ts 0000644 0001750 0000144 00000000760 03560116604 024405 0 ustar andreh users /// <reference types="node" /> export declare class InvariantError extends Error { framesToPop: number; name: string; constructor(message?: string | number); } export declare function invariant(condition: any, message?: string | number): void; export declare namespace invariant { const warn: (...args: any[]) => void; const error: (...args: any[]) => void; } declare let processStub: NodeJS.Process; export { processStub as process }; export default invariant; apollo-server-demo/node_modules/ts-invariant/lib/invariant.js.map 0000644 0001750 0000144 00000010270 03560116604 024722 0 ustar andreh users {"version":3,"file":"invariant.js","sources":["invariant.esm.js"],"sourcesContent":["import { __extends } from 'tslib';\n\nvar genericMessage = \"Invariant Violation\";\r\nvar _a = Object.setPrototypeOf, setPrototypeOf = _a === void 0 ? function (obj, proto) {\r\n obj.__proto__ = proto;\r\n return obj;\r\n} : _a;\r\nvar InvariantError = /** @class */ (function (_super) {\r\n __extends(InvariantError, _super);\r\n function InvariantError(message) {\r\n if (message === void 0) { message = genericMessage; }\r\n var _this = _super.call(this, typeof message === \"number\"\r\n ? genericMessage + \": \" + message + \" (see https://github.com/apollographql/invariant-packages)\"\r\n : message) || this;\r\n _this.framesToPop = 1;\r\n _this.name = genericMessage;\r\n setPrototypeOf(_this, InvariantError.prototype);\r\n return _this;\r\n }\r\n return InvariantError;\r\n}(Error));\r\nfunction invariant(condition, message) {\r\n if (!condition) {\r\n throw new InvariantError(message);\r\n }\r\n}\r\nfunction wrapConsoleMethod(method) {\r\n return function () {\r\n return console[method].apply(console, arguments);\r\n };\r\n}\r\n(function (invariant) {\r\n invariant.warn = wrapConsoleMethod(\"warn\");\r\n invariant.error = wrapConsoleMethod(\"error\");\r\n})(invariant || (invariant = {}));\r\n// Code that uses ts-invariant with rollup-plugin-invariant may want to\r\n// import this process stub to avoid errors evaluating process.env.NODE_ENV.\r\n// However, because most ESM-to-CJS compilers will rewrite the process import\r\n// as tsInvariant.process, which prevents proper replacement by minifiers, we\r\n// also attempt to define the stub globally when it is not already defined.\r\nvar processStub = { env: {} };\r\nif (typeof process === \"object\") {\r\n processStub = process;\r\n}\r\nelse\r\n try {\r\n // Using Function to evaluate this assignment in global scope also escapes\r\n // the strict mode of the current module, thereby allowing the assignment.\r\n // Inspired by https://github.com/facebook/regenerator/pull/369.\r\n Function(\"stub\", \"process = stub\")(processStub);\r\n }\r\n catch (atLeastWeTried) {\r\n // The assignment can fail if a Content Security Policy heavy-handedly\r\n // forbids Function usage. In those environments, developers should take\r\n // extra care to replace process.env.NODE_ENV in their production builds,\r\n // or define an appropriate global.process polyfill.\r\n }\r\nvar invariant$1 = invariant;\n\nexport default invariant$1;\nexport { InvariantError, invariant, processStub as process };\n//# sourceMappingURL=invariant.esm.js.map\n"],"names":["__extends","processStub"],"mappings":";;;;;;AAEA,IAAI,cAAc,GAAG,qBAAqB,CAAC;AAC3C,IAAI,EAAE,GAAG,MAAM,CAAC,cAAc,EAAE,cAAc,GAAG,EAAE,KAAK,KAAK,CAAC,GAAG,UAAU,GAAG,EAAE,KAAK,EAAE;IACnF,GAAG,CAAC,SAAS,GAAG,KAAK,CAAC;IACtB,OAAO,GAAG,CAAC;CACd,GAAG,EAAE,CAAC;AACP,AAAG,IAAC,cAAc,kBAAkB,UAAU,MAAM,EAAE;IAClDA,eAAS,CAAC,cAAc,EAAE,MAAM,CAAC,CAAC;IAClC,SAAS,cAAc,CAAC,OAAO,EAAE;QAC7B,IAAI,OAAO,KAAK,KAAK,CAAC,EAAE,EAAE,OAAO,GAAG,cAAc,CAAC,EAAE;QACrD,IAAI,KAAK,GAAG,MAAM,CAAC,IAAI,CAAC,IAAI,EAAE,OAAO,OAAO,KAAK,QAAQ;cACnD,cAAc,GAAG,IAAI,GAAG,OAAO,GAAG,4DAA4D;cAC9F,OAAO,CAAC,IAAI,IAAI,CAAC;QACvB,KAAK,CAAC,WAAW,GAAG,CAAC,CAAC;QACtB,KAAK,CAAC,IAAI,GAAG,cAAc,CAAC;QAC5B,cAAc,CAAC,KAAK,EAAE,cAAc,CAAC,SAAS,CAAC,CAAC;QAChD,OAAO,KAAK,CAAC;KAChB;IACD,OAAO,cAAc,CAAC;CACzB,CAAC,KAAK,CAAC,CAAC,CAAC;AACV,SAAS,SAAS,CAAC,SAAS,EAAE,OAAO,EAAE;IACnC,IAAI,CAAC,SAAS,EAAE;QACZ,MAAM,IAAI,cAAc,CAAC,OAAO,CAAC,CAAC;KACrC;CACJ;AACD,SAAS,iBAAiB,CAAC,MAAM,EAAE;IAC/B,OAAO,YAAY;QACf,OAAO,OAAO,CAAC,MAAM,CAAC,CAAC,KAAK,CAAC,OAAO,EAAE,SAAS,CAAC,CAAC;KACpD,CAAC;CACL;AACD,CAAC,UAAU,SAAS,EAAE;IAClB,SAAS,CAAC,IAAI,GAAG,iBAAiB,CAAC,MAAM,CAAC,CAAC;IAC3C,SAAS,CAAC,KAAK,GAAG,iBAAiB,CAAC,OAAO,CAAC,CAAC;CAChD,EAAE,SAAS,KAAK,SAAS,GAAG,EAAE,CAAC,CAAC,CAAC;;;;;;AAMlC,AAAIC,eAAW,GAAG,EAAE,GAAG,EAAE,EAAE,EAAE,CAAC;AAC9B,IAAI,OAAO,OAAO,KAAK,QAAQ,EAAE;IAC7BA,eAAW,GAAG,OAAO,CAAC;CACzB;;IAEG,IAAI;;;;QAIA,QAAQ,CAAC,MAAM,EAAE,gBAAgB,CAAC,CAACA,eAAW,CAAC,CAAC;KACnD;IACD,OAAO,cAAc,EAAE;;;;;KAKtB;AACL,IAAI,WAAW,GAAG,SAAS,CAAC;;;;;;"} apollo-server-demo/node_modules/ts-invariant/rollup.config.js 0000644 0001750 0000144 00000001355 03560116604 024172 0 ustar andreh users import typescriptPlugin from 'rollup-plugin-typescript2'; import typescript from 'typescript'; const globals = { __proto__: null, tslib: "tslib", }; function external(id) { return id in globals; } export default [{ input: "src/invariant.ts", external, output: { file: "lib/invariant.esm.js", format: "esm", sourcemap: true, globals, }, plugins: [ typescriptPlugin({ typescript, tsconfig: "./tsconfig.rollup.json", }), ], }, { input: "lib/invariant.esm.js", external, output: { // Intentionally overwrite the invariant.js file written by tsc: file: "lib/invariant.js", format: "cjs", exports: "named", sourcemap: true, name: "ts-invariant", globals, }, }]; apollo-server-demo/node_modules/ts-invariant/tsconfig.rollup.json 0000644 0001750 0000144 00000000130 03560116604 025064 0 ustar andreh users { "extends": "./tsconfig.json", "compilerOptions": { "module": "es2015", }, } apollo-server-demo/node_modules/string.prototype.trimstart/ 0000755 0001750 0000144 00000000000 14067647701 024045 5 ustar andreh users apollo-server-demo/node_modules/string.prototype.trimstart/index.js 0000644 0001750 0000144 00000000565 03560116604 025505 0 ustar andreh users 'use strict'; var callBind = require('call-bind'); var define = require('define-properties'); var implementation = require('./implementation'); var getPolyfill = require('./polyfill'); var shim = require('./shim'); var bound = callBind(getPolyfill()); define(bound, { getPolyfill: getPolyfill, implementation: implementation, shim: shim }); module.exports = bound; apollo-server-demo/node_modules/string.prototype.trimstart/LICENSE 0000644 0001750 0000144 00000002061 03560116604 025036 0 ustar andreh users MIT License Copyright (c) 2017 Khaled Al-Ansari Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/string.prototype.trimstart/.eslintrc 0000644 0001750 0000144 00000000242 03560116604 025654 0 ustar andreh users { "root": true, "extends": "@ljharb", "overrides": [ { "files": "test/**", "rules": { "id-length": 0, "no-invalid-this": 1, }, }, ], } apollo-server-demo/node_modules/string.prototype.trimstart/.github/ 0000755 0001750 0000144 00000000000 14067647701 025405 5 ustar andreh users apollo-server-demo/node_modules/string.prototype.trimstart/.github/workflows/ 0000755 0001750 0000144 00000000000 14067647701 027442 5 ustar andreh users apollo-server-demo/node_modules/string.prototype.trimstart/.github/workflows/node-zero.yml 0000644 0001750 0000144 00000003040 03560116604 032051 0 ustar andreh users name: 'Tests: node.js (0.x)' on: [pull_request, push] jobs: matrix: runs-on: ubuntu-latest outputs: stable: ${{ steps.set-matrix.outputs.requireds }} unstable: ${{ steps.set-matrix.outputs.optionals }} steps: - uses: ljharb/actions/node/matrix@main id: set-matrix with: preset: '0.x' stable: needs: [matrix] name: 'stable minors' runs-on: ubuntu-latest strategy: matrix: ${{ fromJson(needs.matrix.outputs.stable) }} steps: - uses: actions/checkout@v2 - uses: ljharb/actions/node/run@main with: node-version: ${{ matrix.node-version }} command: 'tests-only' cache-node-modules-key: node_modules-${{ github.workflow }}-${{ github.action }}-${{ github.run_id }} skip-ls-check: true unstable: needs: [matrix, stable] name: 'unstable minors' continue-on-error: true if: ${{ !github.head_ref || !startsWith(github.head_ref, 'renovate') }} runs-on: ubuntu-latest strategy: matrix: ${{ fromJson(needs.matrix.outputs.unstable) }} steps: - uses: actions/checkout@v2 - uses: ljharb/actions/node/run@main with: node-version: ${{ matrix.node-version }} command: 'tests-only' cache-node-modules-key: node_modules-${{ github.workflow }}-${{ github.action }}-${{ github.run_id }} skip-ls-check: true node: name: 'node 0.x' needs: [stable, unstable] runs-on: ubuntu-latest steps: - run: 'echo tests completed' apollo-server-demo/node_modules/string.prototype.trimstart/.github/workflows/require-allow-edits.yml0000644 0001750 0000144 00000000376 03560116604 034056 0 ustar andreh users name: Require “Allow Edits†on: [pull_request_target] jobs: _: name: "Require “Allow Editsâ€" runs-on: ubuntu-latest steps: - uses: ljharb/require-allow-edits@main env: GITHUB_TOKEN: ${{ secrets.GITHUB_TOKEN }} apollo-server-demo/node_modules/string.prototype.trimstart/.github/workflows/rebase.yml 0000644 0001750 0000144 00000000401 03560116604 031406 0 ustar andreh users name: Automatic Rebase on: [pull_request_target] jobs: _: name: "Automatic Rebase" runs-on: ubuntu-latest steps: - uses: actions/checkout@v1 - uses: ljharb/rebase@master env: GITHUB_TOKEN: ${{ secrets.GITHUB_TOKEN }} apollo-server-demo/node_modules/string.prototype.trimstart/.github/workflows/node-4+.yml 0000644 0001750 0000144 00000002450 03560116604 031314 0 ustar andreh users name: 'Tests: node.js' on: [pull_request, push] jobs: matrix: runs-on: ubuntu-latest outputs: latest: ${{ steps.set-matrix.outputs.requireds }} minors: ${{ steps.set-matrix.outputs.optionals }} steps: - uses: ljharb/actions/node/matrix@main id: set-matrix with: preset: '>=4' latest: needs: [matrix] name: 'latest minors' runs-on: ubuntu-latest strategy: matrix: ${{ fromJson(needs.matrix.outputs.latest) }} steps: - uses: actions/checkout@v2 - uses: ljharb/actions/node/run@main name: 'npm install && npm run tests-only' with: node-version: ${{ matrix.node-version }} command: 'tests-only' minors: needs: [matrix, latest] name: 'non-latest minors' continue-on-error: true if: ${{ !github.head_ref || !startsWith(github.head_ref, 'renovate') }} runs-on: ubuntu-latest strategy: matrix: ${{ fromJson(needs.matrix.outputs.minors) }} steps: - uses: actions/checkout@v2 - uses: ljharb/actions/node/run@main with: node-version: ${{ matrix.node-version }} command: 'tests-only' node: name: 'node 4+' needs: [latest, minors] runs-on: ubuntu-latest steps: - run: 'echo tests completed' apollo-server-demo/node_modules/string.prototype.trimstart/.github/workflows/node-iojs.yml 0000644 0001750 0000144 00000002636 03560116604 032050 0 ustar andreh users name: 'Tests: node.js (io.js)' on: [pull_request, push] jobs: matrix: runs-on: ubuntu-latest outputs: latest: ${{ steps.set-matrix.outputs.requireds }} minors: ${{ steps.set-matrix.outputs.optionals }} steps: - uses: ljharb/actions/node/matrix@main id: set-matrix with: preset: 'iojs' latest: needs: [matrix] name: 'latest minors' runs-on: ubuntu-latest strategy: matrix: ${{ fromJson(needs.matrix.outputs.latest) }} steps: - uses: actions/checkout@v2 - uses: ljharb/actions/node/run@main name: 'npm install && npm run tests-only' with: node-version: ${{ matrix.node-version }} command: 'tests-only' skip-ls-check: true minors: needs: [matrix, latest] name: 'non-latest minors' continue-on-error: true if: ${{ !github.head_ref || !startsWith(github.head_ref, 'renovate') }} runs-on: ubuntu-latest strategy: matrix: ${{ fromJson(needs.matrix.outputs.minors) }} steps: - uses: actions/checkout@v2 - uses: ljharb/actions/node/run@main name: 'npm install && npm run tests-only' with: node-version: ${{ matrix.node-version }} command: 'tests-only' skip-ls-check: true node: name: 'io.js' needs: [latest, minors] runs-on: ubuntu-latest steps: - run: 'echo tests completed' apollo-server-demo/node_modules/string.prototype.trimstart/.github/workflows/node-pretest.yml 0000644 0001750 0000144 00000001067 03560116604 032567 0 ustar andreh users name: 'Tests: pretest/posttest' on: [pull_request, push] jobs: pretest: runs-on: ubuntu-latest steps: - uses: actions/checkout@v2 - uses: ljharb/actions/node/run@main name: 'npm install && npm run pretest' with: node-version: 'lts/*' command: 'pretest' posttest: runs-on: ubuntu-latest steps: - uses: actions/checkout@v2 - uses: ljharb/actions/node/run@main name: 'npm install && npm run posttest' with: node-version: 'lts/*' command: 'posttest' apollo-server-demo/node_modules/string.prototype.trimstart/README.md 0000644 0001750 0000144 00000004376 03560116604 025323 0 ustar andreh users String.prototype.trimStart <sup>[![Version Badge][npm-version-svg]][package-url]</sup> [![Build Status][travis-svg]][travis-url] [![dependency status][deps-svg]][deps-url] [![dev dependency status][dev-deps-svg]][dev-deps-url] [![License][license-image]][license-url] [![Downloads][downloads-image]][downloads-url] [![npm badge][npm-badge-png]][package-url] [![browser support][testling-svg]][testling-url] An ES2019-spec-compliant `String.prototype.trimStart` shim. Invoke its "shim" method to shim `String.prototype.trimStart` if it is unavailable. This package implements the [es-shim API](https://github.com/es-shims/api) interface. It works in an ES3-supported environment and complies with the [spec](http://www.ecma-international.org/ecma-262/6.0/#sec-object.assign). In an ES6 environment, it will also work properly with `Symbol`s. Most common usage: ```js var trimStart = require('string.prototype.trimstart'); assert(trimStart(' \t\na \t\n') === 'a \t\n'); if (!String.prototype.trimStart) { trimStart.shim(); } assert(trimStart(' \t\na \t\n') === ' \t\na \t\n'.trimStart()); ``` ## Tests Simply clone the repo, `npm install`, and run `npm test` [package-url]: https://npmjs.com/package/string.prototype.trimstart [npm-version-svg]: http://vb.teelaun.ch/es-shims/String.prototype.trimStart.svg [travis-svg]: https://travis-ci.org/es-shims/String.prototype.trimStart.svg [travis-url]: https://travis-ci.org/es-shims/String.prototype.trimStart [deps-svg]: https://david-dm.org/es-shims/String.prototype.trimStart.svg [deps-url]: https://david-dm.org/es-shims/String.prototype.trimStart [dev-deps-svg]: https://david-dm.org/es-shims/String.prototype.trimStart/dev-status.svg [dev-deps-url]: https://david-dm.org/es-shims/String.prototype.trimStart#info=devDependencies [testling-svg]: https://ci.testling.com/es-shims/String.prototype.trimStart.png [testling-url]: https://ci.testling.com/es-shims/String.prototype.trimStart [npm-badge-png]: https://nodei.co/npm/string.prototype.trimstart.png?downloads=true&stars=true [license-image]: http://img.shields.io/npm/l/string.prototype.trimstart.svg [license-url]: LICENSE [downloads-image]: http://img.shields.io/npm/dm/string.prototype.trimstart.svg [downloads-url]: http://npm-stat.com/charts.html?package=string.prototype.trimstart apollo-server-demo/node_modules/string.prototype.trimstart/.editorconfig 0000644 0001750 0000144 00000000436 03560116604 026512 0 ustar andreh users root = true [*] indent_style = tab indent_size = 4 end_of_line = lf charset = utf-8 trim_trailing_whitespace = true insert_final_newline = true max_line_length = 150 [CHANGELOG.md] indent_style = space indent_size = 2 [*.json] max_line_length = off [Makefile] max_line_length = off apollo-server-demo/node_modules/string.prototype.trimstart/package.json 0000644 0001750 0000144 00000003715 03560116604 026326 0 ustar andreh users { "name": "string.prototype.trimstart", "version": "1.0.3", "author": "Jordan Harband <ljharb@gmail.com>", "contributors": [ "Jordan Harband <ljharb@gmail.com>", "Khaled Al-Ansari <khaledelansari@gmail.com>" ], "funding": { "url": "https://github.com/sponsors/ljharb" }, "description": "ES2019 spec-compliant String.prototype.trimStart shim.", "license": "MIT", "main": "index.js", "scripts": { "prepublish": "safe-publish-latest", "lint": "eslint .", "postlint": "es-shim-api --bound", "pretest": "npm run lint", "test": "npm run tests-only", "posttest": "aud --production", "tests-only": "nyc tape 'test/**/*.js'", "version": "auto-changelog && git add CHANGELOG.md", "postversion": "auto-changelog && git add CHANGELOG.md && git commit --no-edit --amend && git tag -f \"v$(node -e \"console.log(require('./package.json').version)\")\"" }, "repository": { "type": "git", "url": "git://github.com/es-shims/String.prototype.trimStart.git" }, "keywords": [ "es6", "es7", "es8", "javascript", "prototype", "polyfill", "utility", "trim", "trimLeft", "trimRight", "trimStart", "trimEnd", "tc39" ], "devDependencies": { "@es-shims/api": "^2.1.2", "@ljharb/eslint-config": "^17.2.0", "aud": "^1.1.3", "auto-changelog": "^2.2.1", "eslint": "^7.14.0", "functions-have-names": "^1.2.1", "has-strict-mode": "^1.0.0", "nyc": "^10.3.2", "safe-publish-latest": "^1.1.4", "tape": "^5.0.1" }, "auto-changelog": { "output": "CHANGELOG.md", "template": "keepachangelog", "unreleased": false, "commitLimit": false, "backfillLimit": false, "hideCredit": true }, "dependencies": { "call-bind": "^1.0.0", "define-properties": "^1.1.3" } ,"_resolved": "https://registry.npmjs.org/string.prototype.trimstart/-/string.prototype.trimstart-1.0.3.tgz" ,"_integrity": "sha512-oBIBUy5lea5tt0ovtOFiEQaBkoBBkyJhZXzJYrSmDo5IUUqbOPvVezuRs/agBIdZ2p2Eo1FD6bD9USyBLfl3xg==" ,"_from": "string.prototype.trimstart@1.0.3" } apollo-server-demo/node_modules/string.prototype.trimstart/auto.js 0000644 0001750 0000144 00000000044 03560116604 025336 0 ustar andreh users 'use strict'; require('./shim')(); apollo-server-demo/node_modules/string.prototype.trimstart/shim.js 0000644 0001750 0000144 00000000521 03560116604 025326 0 ustar andreh users 'use strict'; var define = require('define-properties'); var getPolyfill = require('./polyfill'); module.exports = function shimTrimStart() { var polyfill = getPolyfill(); define( String.prototype, { trimStart: polyfill }, { trimStart: function () { return String.prototype.trimStart !== polyfill; } } ); return polyfill; }; apollo-server-demo/node_modules/string.prototype.trimstart/implementation.js 0000644 0001750 0000144 00000000706 03560116604 027420 0 ustar andreh users 'use strict'; var callBound = require('call-bind/callBound'); var $replace = callBound('String.prototype.replace'); /* eslint-disable no-control-regex */ var startWhitespace = /^[\x09\x0A\x0B\x0C\x0D\x20\xA0\u1680\u180E\u2000\u2001\u2002\u2003\u2004\u2005\u2006\u2007\u2008\u2009\u200A\u202F\u205F\u3000\u2028\u2029\uFEFF]*/; /* eslint-enable no-control-regex */ module.exports = function trimStart() { return $replace(this, startWhitespace, ''); }; apollo-server-demo/node_modules/string.prototype.trimstart/.nycrc 0000644 0001750 0000144 00000000330 03560116604 025145 0 ustar andreh users { "all": true, "check-coverage": false, "reporter": ["text-summary", "text", "html", "json"], "lines": 86, "statements": 85.93, "functions": 82.43, "branches": 76.06, "exclude": [ "coverage", "test" ] } apollo-server-demo/node_modules/string.prototype.trimstart/test/ 0000755 0001750 0000144 00000000000 14067647701 025024 5 ustar andreh users apollo-server-demo/node_modules/string.prototype.trimstart/test/index.js 0000644 0001750 0000144 00000000677 03560116604 026470 0 ustar andreh users 'use strict'; var trimStart = require('../'); var test = require('tape'); var runTests = require('./tests'); test('as a function', function (t) { t.test('bad array/this value', function (st) { st['throws'](function () { trimStart(undefined, 'a'); }, TypeError, 'undefined is not an object'); st['throws'](function () { trimStart(null, 'a'); }, TypeError, 'null is not an object'); st.end(); }); runTests(trimStart, t); t.end(); }); apollo-server-demo/node_modules/string.prototype.trimstart/test/tests.js 0000644 0001750 0000144 00000002243 03560116604 026512 0 ustar andreh users 'use strict'; module.exports = function (trimStart, t) { t.test('normal cases', function (st) { st.equal(trimStart(' \t\na \t\n'), 'a \t\n', 'strips whitespace off the left side'); st.equal(trimStart('a'), 'a', 'noop when no whitespace'); var allWhitespaceChars = '\x09\x0A\x0B\x0C\x0D\x20\xA0\u1680\u2000\u2001\u2002\u2003\u2004\u2005\u2006\u2007\u2008\u2009\u200A\u202F\u205F\u3000\u2028\u2029\uFEFF'; st.equal(trimStart(allWhitespaceChars + 'a' + allWhitespaceChars), 'a' + allWhitespaceChars, 'all expected whitespace chars are trimmed'); st.end(); }); // see https://codeblog.jonskeet.uk/2014/12/01/when-is-an-identifier-not-an-identifier-attack-of-the-mongolian-vowel-separator/ var mongolianVowelSeparator = '\u180E'; t.test('unicode >= 4 && < 6.3', { skip: !(/^\s$/).test(mongolianVowelSeparator) }, function (st) { st.equal(trimStart(mongolianVowelSeparator + 'a' + mongolianVowelSeparator), 'a' + mongolianVowelSeparator, 'mongolian vowel separator is whitespace'); st.end(); }); t.test('zero-width spaces', function (st) { var zeroWidth = '\u200b'; st.equal(trimStart(zeroWidth), zeroWidth, 'zero width space does not trim'); st.end(); }); }; apollo-server-demo/node_modules/string.prototype.trimstart/test/shimmed.js 0000644 0001750 0000144 00000002515 03560116604 027000 0 ustar andreh users 'use strict'; var trimStart = require('../'); trimStart.shim(); var runTests = require('./tests'); var test = require('tape'); var defineProperties = require('define-properties'); var callBind = require('call-bind'); var isEnumerable = Object.prototype.propertyIsEnumerable; var functionsHaveNames = require('functions-have-names')(); test('shimmed', function (t) { t.equal(String.prototype.trimStart.length, 0, 'String#trimStart has a length of 0'); t.test('Function name', { skip: !functionsHaveNames }, function (st) { st.equal((/^(?:trimLeft|trimStart)$/).test(String.prototype.trimStart.name), true, 'String#trimStart has name "trimLeft" or "trimStart"'); st.end(); }); t.test('enumerability', { skip: !defineProperties.supportsDescriptors }, function (et) { et.equal(false, isEnumerable.call(String.prototype, 'trimStart'), 'String#trimStart is not enumerable'); et.end(); }); var supportsStrictMode = (function () { return typeof this === 'undefined'; }()); t.test('bad string/this value', { skip: !supportsStrictMode }, function (st) { st['throws'](function () { return trimStart(undefined, 'a'); }, TypeError, 'undefined is not an object'); st['throws'](function () { return trimStart(null, 'a'); }, TypeError, 'null is not an object'); st.end(); }); runTests(callBind(String.prototype.trimStart), t); t.end(); }); apollo-server-demo/node_modules/string.prototype.trimstart/test/implementation.js 0000644 0001750 0000144 00000001175 03560116604 030400 0 ustar andreh users 'use strict'; var implementation = require('../implementation'); var callBind = require('call-bind'); var test = require('tape'); var hasStrictMode = require('has-strict-mode')(); var runTests = require('./tests'); test('as a function', function (t) { t.test('bad array/this value', { skip: !hasStrictMode }, function (st) { /* eslint no-useless-call: 0 */ st['throws'](function () { implementation.call(undefined); }, TypeError, 'undefined is not an object'); st['throws'](function () { implementation.call(null); }, TypeError, 'null is not an object'); st.end(); }); runTests(callBind(implementation), t); t.end(); }); apollo-server-demo/node_modules/string.prototype.trimstart/.eslintignore 0000644 0001750 0000144 00000000012 03560116604 026526 0 ustar andreh users coverage/ apollo-server-demo/node_modules/string.prototype.trimstart/CHANGELOG.md 0000644 0001750 0000144 00000011257 03560116604 025651 0 ustar andreh users # Changelog All notable changes to this project will be documented in this file. The format is based on [Keep a Changelog](https://keepachangelog.com/en/1.0.0/) and this project adheres to [Semantic Versioning](https://semver.org/spec/v2.0.0.html). ## [v1.0.3](https://github.com/es-shims/String.prototype.trimStart/compare/v1.0.2...v1.0.3) - 2020-11-21 ### Commits - [Tests] migrate tests to Github Actions [`fbc7519`](https://github.com/es-shims/String.prototype.trimStart/commit/fbc7519cce2b5bfff9fe28dea96fb5f6f82e19fd) - [Tests] add `implementation` test; run `es-shim-api` in postlint; use `tape` runner [`3c9330b`](https://github.com/es-shims/String.prototype.trimStart/commit/3c9330be9ad02497f78ff0fd94b7c918c3a4bc21) - [Tests] run `nyc` on all tests [`52229ca`](https://github.com/es-shims/String.prototype.trimStart/commit/52229ca28426be516c3826743e417be85144673e) - [Deps] replace `es-abstract` with `call-bind` [`5e5068d`](https://github.com/es-shims/String.prototype.trimStart/commit/5e5068d2cc85d0a6f2a441ea984521ee70470537) - [Dev Deps] update `eslint`, `aud`; add `safe-publish-latest` [`42a853e`](https://github.com/es-shims/String.prototype.trimStart/commit/42a853e2cb419378085098cb66e421ee94eed3ab) ## [v1.0.2](https://github.com/es-shims/String.prototype.trimStart/compare/v1.0.1...v1.0.2) - 2020-10-20 ### Commits - [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `aud`, `auto-changelog`, `tape` [`d032b38`](https://github.com/es-shims/String.prototype.trimStart/commit/d032b38aac7e9ebae7bf5c4195492c508af2815a) - [actions] add "Allow Edits" workflow [`83e30ba`](https://github.com/es-shims/String.prototype.trimStart/commit/83e30bac01572b6dba6358fec6e339c55dc431c9) - [Deps] update `es-abstract` [`707d85d`](https://github.com/es-shims/String.prototype.trimStart/commit/707d85d827d9c537a144f199fdecc47edaade1cd) - [actions] switch Automatic Rebase workflow to `pull_request_target` event [`096c6d9`](https://github.com/es-shims/String.prototype.trimStart/commit/096c6d9dc142286c750da7024e7a88ed698a4953) ## [v1.0.1](https://github.com/es-shims/String.prototype.trimStart/compare/v1.0.0...v1.0.1) - 2020-04-09 ### Commits - [meta] add some missing repo metadata [`3385da3`](https://github.com/es-shims/String.prototype.trimStart/commit/3385da3bbb87819de11a869981ca954887a6a092) - [Dev Deps] update `auto-changelog` [`879377d`](https://github.com/es-shims/String.prototype.trimStart/commit/879377df9c1ff97d8f0b3eac800683f1d68a304c) ## [v1.0.0](https://github.com/es-shims/String.prototype.trimStart/compare/v0.1.0...v1.0.0) - 2020-03-30 ### Commits - [Breaking] convert to es-shim API [`970922c`](https://github.com/es-shims/String.prototype.trimStart/commit/970922c494c78b033c351c77f61a8aefd49c30d9) - [meta] add `auto-changelog` [`ff30c09`](https://github.com/es-shims/String.prototype.trimStart/commit/ff30c0996289113d2c3dbbfca7e280ff151bf36d) - [meta] update readme [`816291d`](https://github.com/es-shims/String.prototype.trimStart/commit/816291d01e0eaf85da9b732c179cfb2454bd282e) - [Tests] add `npm run lint` [`3341104`](https://github.com/es-shims/String.prototype.trimStart/commit/3341104450bc6ac84f3b70a6d6c0fbeb4df5131e) - Only apps should have lockfiles [`f008df7`](https://github.com/es-shims/String.prototype.trimStart/commit/f008df73fbf3dcf8dfad6d5cad86de7050d0ae09) - [actions] add automatic rebasing / merge commit blocking [`e5ba35c`](https://github.com/es-shims/String.prototype.trimStart/commit/e5ba35c1a14fcf652336cc9c4be49d232981161e) - [Tests] use shared travis-ci configs [`46516b1`](https://github.com/es-shims/String.prototype.trimStart/commit/46516b137a8c07ed5807d751bd61199688ef9baa) - [meta] add `funding` field [`34ae856`](https://github.com/es-shims/String.prototype.trimStart/commit/34ae8563f115bd4a5e5f5d2d786c0fa0a420fa2a) - [meta] fix non-updated version number [`3b0e262`](https://github.com/es-shims/String.prototype.trimStart/commit/3b0e262e2f4eeee2e1b99fe890f8ca17bed8f2fd) ## [v0.1.0](https://github.com/es-shims/String.prototype.trimStart/compare/v0.0.1...v0.1.0) - 2017-12-19 ### Commits - updated README [`ab2f6ac`](https://github.com/es-shims/String.prototype.trimStart/commit/ab2f6ac8813ed336a0f2dc3aa8cdb52f4d52814b) ## v0.0.1 - 2017-12-19 ### Commits - finished polyfill [`1c7ca20`](https://github.com/es-shims/String.prototype.trimStart/commit/1c7ca2043e3383b6e743870bc622ad4a38477147) - created README file: [`192ecad`](https://github.com/es-shims/String.prototype.trimStart/commit/192ecaded4e0d5baaa65cd41e590b8d837520d44) - Initial commit [`14044f8`](https://github.com/es-shims/String.prototype.trimStart/commit/14044f8a0fe1d155fe7403a8327bdbaf135da2d6) - updated README [`d4fb6be`](https://github.com/es-shims/String.prototype.trimStart/commit/d4fb6be15455dd68fc4b306bee1d30dd4afc96e7) apollo-server-demo/node_modules/string.prototype.trimstart/polyfill.js 0000644 0001750 0000144 00000000717 03560116604 026227 0 ustar andreh users 'use strict'; var implementation = require('./implementation'); module.exports = function getPolyfill() { if (!String.prototype.trimStart && !String.prototype.trimLeft) { return implementation; } var zeroWidthSpace = '\u200b'; var trimmed = zeroWidthSpace.trimStart ? zeroWidthSpace.trimStart() : zeroWidthSpace.trimLeft(); if (trimmed !== zeroWidthSpace) { return implementation; } return String.prototype.trimStart || String.prototype.trimLeft; }; apollo-server-demo/node_modules/ws/ 0000755 0001750 0000144 00000000000 14067647700 017073 5 ustar andreh users apollo-server-demo/node_modules/ws/browser.js 0000644 0001750 0000144 00000000257 03560116604 021106 0 ustar andreh users 'use strict'; module.exports = function() { throw new Error( 'ws does not work in the browser. Browser clients must use the native ' + 'WebSocket object' ); }; apollo-server-demo/node_modules/ws/index.js 0000644 0001750 0000144 00000000355 03560116604 020531 0 ustar andreh users 'use strict'; const WebSocket = require('./lib/websocket'); WebSocket.Server = require('./lib/websocket-server'); WebSocket.Receiver = require('./lib/receiver'); WebSocket.Sender = require('./lib/sender'); module.exports = WebSocket; apollo-server-demo/node_modules/ws/LICENSE 0000644 0001750 0000144 00000002122 03560116604 020063 0 ustar andreh users The MIT License (MIT) Copyright (c) 2011 Einar Otto Stangvik <einaros@gmail.com> Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/ws/README.md 0000644 0001750 0000144 00000031142 03560116604 020341 0 ustar andreh users # ws: a Node.js WebSocket library [](https://www.npmjs.com/package/ws) [](https://travis-ci.org/websockets/ws) [](https://ci.appveyor.com/project/lpinca/ws) [](https://coveralls.io/github/websockets/ws) ws is a simple to use, blazing fast, and thoroughly tested WebSocket client and server implementation. Passes the quite extensive Autobahn test suite: [server][server-report], [client][client-report]. **Note**: This module does not work in the browser. The client in the docs is a reference to a back end with the role of a client in the WebSocket communication. Browser clients must use the native [`WebSocket`](https://developer.mozilla.org/en-US/docs/Web/API/WebSocket) object. To make the same code work seamlessly on Node.js and the browser, you can use one of the many wrappers available on npm, like [isomorphic-ws](https://github.com/heineiuo/isomorphic-ws). ## Table of Contents - [Protocol support](#protocol-support) - [Installing](#installing) - [Opt-in for performance and spec compliance](#opt-in-for-performance-and-spec-compliance) - [API docs](#api-docs) - [WebSocket compression](#websocket-compression) - [Usage examples](#usage-examples) - [Sending and receiving text data](#sending-and-receiving-text-data) - [Sending binary data](#sending-binary-data) - [Simple server](#simple-server) - [External HTTP/S server](#external-https-server) - [Multiple servers sharing a single HTTP/S server](#multiple-servers-sharing-a-single-https-server) - [Server broadcast](#server-broadcast) - [echo.websocket.org demo](#echowebsocketorg-demo) - [Other examples](#other-examples) - [Error handling best practices](#error-handling-best-practices) - [FAQ](#faq) - [How to get the IP address of the client?](#how-to-get-the-ip-address-of-the-client) - [How to detect and close broken connections?](#how-to-detect-and-close-broken-connections) - [How to connect via a proxy?](#how-to-connect-via-a-proxy) - [Changelog](#changelog) - [License](#license) ## Protocol support - **HyBi drafts 07-12** (Use the option `protocolVersion: 8`) - **HyBi drafts 13-17** (Current default, alternatively option `protocolVersion: 13`) ## Installing ``` npm install ws ``` ### Opt-in for performance and spec compliance There are 2 optional modules that can be installed along side with the ws module. These modules are binary addons which improve certain operations. Prebuilt binaries are available for the most popular platforms so you don't necessarily need to have a C++ compiler installed on your machine. - `npm install --save-optional bufferutil`: Allows to efficiently perform operations such as masking and unmasking the data payload of the WebSocket frames. - `npm install --save-optional utf-8-validate`: Allows to efficiently check if a message contains valid UTF-8 as required by the spec. ## API docs See [`/doc/ws.md`](./doc/ws.md) for Node.js-like docs for the ws classes. ## WebSocket compression ws supports the [permessage-deflate extension][permessage-deflate] which enables the client and server to negotiate a compression algorithm and its parameters, and then selectively apply it to the data payloads of each WebSocket message. The extension is disabled by default on the server and enabled by default on the client. It adds a significant overhead in terms of performance and memory consumption so we suggest to enable it only if it is really needed. Note that Node.js has a variety of issues with high-performance compression, where increased concurrency, especially on Linux, can lead to [catastrophic memory fragmentation][node-zlib-bug] and slow performance. If you intend to use permessage-deflate in production, it is worthwhile to set up a test representative of your workload and ensure Node.js/zlib will handle it with acceptable performance and memory usage. Tuning of permessage-deflate can be done via the options defined below. You can also use `zlibDeflateOptions` and `zlibInflateOptions`, which is passed directly into the creation of [raw deflate/inflate streams][node-zlib-deflaterawdocs]. See [the docs][ws-server-options] for more options. ```js const WebSocket = require('ws'); const wss = new WebSocket.Server({ port: 8080, perMessageDeflate: { zlibDeflateOptions: { // See zlib defaults. chunkSize: 1024, memLevel: 7, level: 3 }, zlibInflateOptions: { chunkSize: 10 * 1024 }, // Other options settable: clientNoContextTakeover: true, // Defaults to negotiated value. serverNoContextTakeover: true, // Defaults to negotiated value. serverMaxWindowBits: 10, // Defaults to negotiated value. // Below options specified as default values. concurrencyLimit: 10, // Limits zlib concurrency for perf. threshold: 1024 // Size (in bytes) below which messages // should not be compressed. } }); ``` The client will only use the extension if it is supported and enabled on the server. To always disable the extension on the client set the `perMessageDeflate` option to `false`. ```js const WebSocket = require('ws'); const ws = new WebSocket('ws://www.host.com/path', { perMessageDeflate: false }); ``` ## Usage examples ### Sending and receiving text data ```js const WebSocket = require('ws'); const ws = new WebSocket('ws://www.host.com/path'); ws.on('open', function open() { ws.send('something'); }); ws.on('message', function incoming(data) { console.log(data); }); ``` ### Sending binary data ```js const WebSocket = require('ws'); const ws = new WebSocket('ws://www.host.com/path'); ws.on('open', function open() { const array = new Float32Array(5); for (var i = 0; i < array.length; ++i) { array[i] = i / 2; } ws.send(array); }); ``` ### Simple server ```js const WebSocket = require('ws'); const wss = new WebSocket.Server({ port: 8080 }); wss.on('connection', function connection(ws) { ws.on('message', function incoming(message) { console.log('received: %s', message); }); ws.send('something'); }); ``` ### External HTTP/S server ```js const fs = require('fs'); const https = require('https'); const WebSocket = require('ws'); const server = new https.createServer({ cert: fs.readFileSync('/path/to/cert.pem'), key: fs.readFileSync('/path/to/key.pem') }); const wss = new WebSocket.Server({ server }); wss.on('connection', function connection(ws) { ws.on('message', function incoming(message) { console.log('received: %s', message); }); ws.send('something'); }); server.listen(8080); ``` ### Multiple servers sharing a single HTTP/S server ```js const http = require('http'); const WebSocket = require('ws'); const server = http.createServer(); const wss1 = new WebSocket.Server({ noServer: true }); const wss2 = new WebSocket.Server({ noServer: true }); wss1.on('connection', function connection(ws) { // ... }); wss2.on('connection', function connection(ws) { // ... }); server.on('upgrade', function upgrade(request, socket, head) { const pathname = url.parse(request.url).pathname; if (pathname === '/foo') { wss1.handleUpgrade(request, socket, head, function done(ws) { wss1.emit('connection', ws, request); }); } else if (pathname === '/bar') { wss2.handleUpgrade(request, socket, head, function done(ws) { wss2.emit('connection', ws, request); }); } else { socket.destroy(); } }); server.listen(8080); ``` ### Server broadcast ```js const WebSocket = require('ws'); const wss = new WebSocket.Server({ port: 8080 }); // Broadcast to all. wss.broadcast = function broadcast(data) { wss.clients.forEach(function each(client) { if (client.readyState === WebSocket.OPEN) { client.send(data); } }); }; wss.on('connection', function connection(ws) { ws.on('message', function incoming(data) { // Broadcast to everyone else. wss.clients.forEach(function each(client) { if (client !== ws && client.readyState === WebSocket.OPEN) { client.send(data); } }); }); }); ``` ### echo.websocket.org demo ```js const WebSocket = require('ws'); const ws = new WebSocket('wss://echo.websocket.org/', { origin: 'https://websocket.org' }); ws.on('open', function open() { console.log('connected'); ws.send(Date.now()); }); ws.on('close', function close() { console.log('disconnected'); }); ws.on('message', function incoming(data) { console.log(`Roundtrip time: ${Date.now() - data} ms`); setTimeout(function timeout() { ws.send(Date.now()); }, 500); }); ``` ### Other examples For a full example with a browser client communicating with a ws server, see the examples folder. Otherwise, see the test cases. ## Error handling best practices ```js // If the WebSocket is closed before the following send is attempted ws.send('something'); // Errors (both immediate and async write errors) can be detected in an optional // callback. The callback is also the only way of being notified that data has // actually been sent. ws.send('something', function ack(error) { // If error is not defined, the send has been completed, otherwise the error // object will indicate what failed. }); // Immediate errors can also be handled with `try...catch`, but **note** that // since sends are inherently asynchronous, socket write failures will *not* be // captured when this technique is used. try { ws.send('something'); } catch (e) { /* handle error */ } ``` ## FAQ ### How to get the IP address of the client? The remote IP address can be obtained from the raw socket. ```js const WebSocket = require('ws'); const wss = new WebSocket.Server({ port: 8080 }); wss.on('connection', function connection(ws, req) { const ip = req.connection.remoteAddress; }); ``` When the server runs behind a proxy like NGINX, the de-facto standard is to use the `X-Forwarded-For` header. ```js wss.on('connection', function connection(ws, req) { const ip = req.headers['x-forwarded-for'].split(/\s*,\s*/)[0]; }); ``` ### How to detect and close broken connections? Sometimes the link between the server and the client can be interrupted in a way that keeps both the server and the client unaware of the broken state of the connection (e.g. when pulling the cord). In these cases ping messages can be used as a means to verify that the remote endpoint is still responsive. ```js const WebSocket = require('ws'); function noop() {} function heartbeat() { this.isAlive = true; } const wss = new WebSocket.Server({ port: 8080 }); wss.on('connection', function connection(ws) { ws.isAlive = true; ws.on('pong', heartbeat); }); const interval = setInterval(function ping() { wss.clients.forEach(function each(ws) { if (ws.isAlive === false) return ws.terminate(); ws.isAlive = false; ws.ping(noop); }); }, 30000); ``` Pong messages are automatically sent in response to ping messages as required by the spec. Just like the server example above your clients might as well lose connection without knowing it. You might want to add a ping listener on your clients to prevent that. A simple implementation would be: ```js const WebSocket = require('ws'); function heartbeat() { clearTimeout(this.pingTimeout); // Use `WebSocket#terminate()` and not `WebSocket#close()`. Delay should be // equal to the interval at which your server sends out pings plus a // conservative assumption of the latency. this.pingTimeout = setTimeout(() => { this.terminate(); }, 30000 + 1000); } const client = new WebSocket('wss://echo.websocket.org/'); client.on('open', heartbeat); client.on('ping', heartbeat); client.on('close', function clear() { clearTimeout(this.pingTimeout); }); ``` ### How to connect via a proxy? Use a custom `http.Agent` implementation like [https-proxy-agent][] or [socks-proxy-agent][]. ## Changelog We're using the GitHub [releases][changelog] for changelog entries. ## License [MIT](LICENSE) [https-proxy-agent]: https://github.com/TooTallNate/node-https-proxy-agent [socks-proxy-agent]: https://github.com/TooTallNate/node-socks-proxy-agent [client-report]: http://websockets.github.io/ws/autobahn/clients/ [server-report]: http://websockets.github.io/ws/autobahn/servers/ [permessage-deflate]: https://tools.ietf.org/html/rfc7692 [changelog]: https://github.com/websockets/ws/releases [node-zlib-bug]: https://github.com/nodejs/node/issues/8871 [node-zlib-deflaterawdocs]: https://nodejs.org/api/zlib.html#zlib_zlib_createdeflateraw_options [ws-server-options]: https://github.com/websockets/ws/blob/master/doc/ws.md#new-websocketserveroptions-callback apollo-server-demo/node_modules/ws/package.json 0000644 0001750 0000144 00000002667 03560116604 021362 0 ustar andreh users { "name": "ws", "version": "6.2.1", "description": "Simple to use, blazing fast and thoroughly tested websocket client and server for Node.js", "keywords": [ "HyBi", "Push", "RFC-6455", "WebSocket", "WebSockets", "real-time" ], "homepage": "https://github.com/websockets/ws", "bugs": "https://github.com/websockets/ws/issues", "repository": "websockets/ws", "author": "Einar Otto Stangvik <einaros@gmail.com> (http://2x.io)", "license": "MIT", "main": "index.js", "browser": "browser.js", "files": [ "browser.js", "index.js", "lib/*.js" ], "scripts": { "test": "npm run lint && nyc --reporter=html --reporter=text mocha test/*.test.js", "integration": "npm run lint && mocha test/*.integration.js", "lint": "eslint --ignore-path .gitignore . && prettier --check --ignore-path .gitignore \"**/*.{json,md,yml}\"" }, "dependencies": { "async-limiter": "~1.0.0" }, "devDependencies": { "benchmark": "~2.1.4", "bufferutil": "~4.0.0", "coveralls": "~3.0.3", "eslint": "~5.15.0", "eslint-config-prettier": "~4.1.0", "eslint-plugin-prettier": "~3.0.0", "mocha": "~6.0.0", "nyc": "~13.3.0", "prettier": "~1.16.1", "utf-8-validate": "~5.0.0" } ,"_resolved": "https://registry.npmjs.org/ws/-/ws-6.2.1.tgz" ,"_integrity": "sha512-GIyAXC2cB7LjvpgMt9EKS2ldqr0MTrORaleiOno6TweZ6r3TKtoFQWay/2PceJ3RuBasOHzXNn5Lrw1X0bEjqA==" ,"_from": "ws@6.2.1" } apollo-server-demo/node_modules/ws/lib/ 0000755 0001750 0000144 00000000000 14067647700 017641 5 ustar andreh users apollo-server-demo/node_modules/ws/lib/websocket-server.js 0000644 0001750 0000144 00000025715 03560116604 023471 0 ustar andreh users 'use strict'; const EventEmitter = require('events'); const crypto = require('crypto'); const http = require('http'); const PerMessageDeflate = require('./permessage-deflate'); const extension = require('./extension'); const WebSocket = require('./websocket'); const { GUID } = require('./constants'); const keyRegex = /^[+/0-9A-Za-z]{22}==$/; /** * Class representing a WebSocket server. * * @extends EventEmitter */ class WebSocketServer extends EventEmitter { /** * Create a `WebSocketServer` instance. * * @param {Object} options Configuration options * @param {Number} options.backlog The maximum length of the queue of pending * connections * @param {Boolean} options.clientTracking Specifies whether or not to track * clients * @param {Function} options.handleProtocols An hook to handle protocols * @param {String} options.host The hostname where to bind the server * @param {Number} options.maxPayload The maximum allowed message size * @param {Boolean} options.noServer Enable no server mode * @param {String} options.path Accept only connections matching this path * @param {(Boolean|Object)} options.perMessageDeflate Enable/disable * permessage-deflate * @param {Number} options.port The port where to bind the server * @param {http.Server} options.server A pre-created HTTP/S server to use * @param {Function} options.verifyClient An hook to reject connections * @param {Function} callback A listener for the `listening` event */ constructor(options, callback) { super(); options = Object.assign( { maxPayload: 100 * 1024 * 1024, perMessageDeflate: false, handleProtocols: null, clientTracking: true, verifyClient: null, noServer: false, backlog: null, // use default (511 as implemented in net.js) server: null, host: null, path: null, port: null }, options ); if (options.port == null && !options.server && !options.noServer) { throw new TypeError( 'One of the "port", "server", or "noServer" options must be specified' ); } if (options.port != null) { this._server = http.createServer((req, res) => { const body = http.STATUS_CODES[426]; res.writeHead(426, { 'Content-Length': body.length, 'Content-Type': 'text/plain' }); res.end(body); }); this._server.listen( options.port, options.host, options.backlog, callback ); } else if (options.server) { this._server = options.server; } if (this._server) { this._removeListeners = addListeners(this._server, { listening: this.emit.bind(this, 'listening'), error: this.emit.bind(this, 'error'), upgrade: (req, socket, head) => { this.handleUpgrade(req, socket, head, (ws) => { this.emit('connection', ws, req); }); } }); } if (options.perMessageDeflate === true) options.perMessageDeflate = {}; if (options.clientTracking) this.clients = new Set(); this.options = options; } /** * Returns the bound address, the address family name, and port of the server * as reported by the operating system if listening on an IP socket. * If the server is listening on a pipe or UNIX domain socket, the name is * returned as a string. * * @return {(Object|String|null)} The address of the server * @public */ address() { if (this.options.noServer) { throw new Error('The server is operating in "noServer" mode'); } if (!this._server) return null; return this._server.address(); } /** * Close the server. * * @param {Function} cb Callback * @public */ close(cb) { if (cb) this.once('close', cb); // // Terminate all associated clients. // if (this.clients) { for (const client of this.clients) client.terminate(); } const server = this._server; if (server) { this._removeListeners(); this._removeListeners = this._server = null; // // Close the http server if it was internally created. // if (this.options.port != null) { server.close(() => this.emit('close')); return; } } process.nextTick(emitClose, this); } /** * See if a given request should be handled by this server instance. * * @param {http.IncomingMessage} req Request object to inspect * @return {Boolean} `true` if the request is valid, else `false` * @public */ shouldHandle(req) { if (this.options.path) { const index = req.url.indexOf('?'); const pathname = index !== -1 ? req.url.slice(0, index) : req.url; if (pathname !== this.options.path) return false; } return true; } /** * Handle a HTTP Upgrade request. * * @param {http.IncomingMessage} req The request object * @param {net.Socket} socket The network socket between the server and client * @param {Buffer} head The first packet of the upgraded stream * @param {Function} cb Callback * @public */ handleUpgrade(req, socket, head, cb) { socket.on('error', socketOnError); const key = req.headers['sec-websocket-key'] !== undefined ? req.headers['sec-websocket-key'].trim() : false; const version = +req.headers['sec-websocket-version']; const extensions = {}; if ( req.method !== 'GET' || req.headers.upgrade.toLowerCase() !== 'websocket' || !key || !keyRegex.test(key) || (version !== 8 && version !== 13) || !this.shouldHandle(req) ) { return abortHandshake(socket, 400); } if (this.options.perMessageDeflate) { const perMessageDeflate = new PerMessageDeflate( this.options.perMessageDeflate, true, this.options.maxPayload ); try { const offers = extension.parse(req.headers['sec-websocket-extensions']); if (offers[PerMessageDeflate.extensionName]) { perMessageDeflate.accept(offers[PerMessageDeflate.extensionName]); extensions[PerMessageDeflate.extensionName] = perMessageDeflate; } } catch (err) { return abortHandshake(socket, 400); } } // // Optionally call external client verification handler. // if (this.options.verifyClient) { const info = { origin: req.headers[`${version === 8 ? 'sec-websocket-origin' : 'origin'}`], secure: !!(req.connection.authorized || req.connection.encrypted), req }; if (this.options.verifyClient.length === 2) { this.options.verifyClient(info, (verified, code, message, headers) => { if (!verified) { return abortHandshake(socket, code || 401, message, headers); } this.completeUpgrade(key, extensions, req, socket, head, cb); }); return; } if (!this.options.verifyClient(info)) return abortHandshake(socket, 401); } this.completeUpgrade(key, extensions, req, socket, head, cb); } /** * Upgrade the connection to WebSocket. * * @param {String} key The value of the `Sec-WebSocket-Key` header * @param {Object} extensions The accepted extensions * @param {http.IncomingMessage} req The request object * @param {net.Socket} socket The network socket between the server and client * @param {Buffer} head The first packet of the upgraded stream * @param {Function} cb Callback * @private */ completeUpgrade(key, extensions, req, socket, head, cb) { // // Destroy the socket if the client has already sent a FIN packet. // if (!socket.readable || !socket.writable) return socket.destroy(); const digest = crypto .createHash('sha1') .update(key + GUID) .digest('base64'); const headers = [ 'HTTP/1.1 101 Switching Protocols', 'Upgrade: websocket', 'Connection: Upgrade', `Sec-WebSocket-Accept: ${digest}` ]; const ws = new WebSocket(null); var protocol = req.headers['sec-websocket-protocol']; if (protocol) { protocol = protocol.trim().split(/ *, */); // // Optionally call external protocol selection handler. // if (this.options.handleProtocols) { protocol = this.options.handleProtocols(protocol, req); } else { protocol = protocol[0]; } if (protocol) { headers.push(`Sec-WebSocket-Protocol: ${protocol}`); ws.protocol = protocol; } } if (extensions[PerMessageDeflate.extensionName]) { const params = extensions[PerMessageDeflate.extensionName].params; const value = extension.format({ [PerMessageDeflate.extensionName]: [params] }); headers.push(`Sec-WebSocket-Extensions: ${value}`); ws._extensions = extensions; } // // Allow external modification/inspection of handshake headers. // this.emit('headers', headers, req); socket.write(headers.concat('\r\n').join('\r\n')); socket.removeListener('error', socketOnError); ws.setSocket(socket, head, this.options.maxPayload); if (this.clients) { this.clients.add(ws); ws.on('close', () => this.clients.delete(ws)); } cb(ws); } } module.exports = WebSocketServer; /** * Add event listeners on an `EventEmitter` using a map of <event, listener> * pairs. * * @param {EventEmitter} server The event emitter * @param {Object.<String, Function>} map The listeners to add * @return {Function} A function that will remove the added listeners when called * @private */ function addListeners(server, map) { for (const event of Object.keys(map)) server.on(event, map[event]); return function removeListeners() { for (const event of Object.keys(map)) { server.removeListener(event, map[event]); } }; } /** * Emit a `'close'` event on an `EventEmitter`. * * @param {EventEmitter} server The event emitter * @private */ function emitClose(server) { server.emit('close'); } /** * Handle premature socket errors. * * @private */ function socketOnError() { this.destroy(); } /** * Close the connection when preconditions are not fulfilled. * * @param {net.Socket} socket The socket of the upgrade request * @param {Number} code The HTTP response status code * @param {String} [message] The HTTP response body * @param {Object} [headers] Additional HTTP response headers * @private */ function abortHandshake(socket, code, message, headers) { if (socket.writable) { message = message || http.STATUS_CODES[code]; headers = Object.assign( { Connection: 'close', 'Content-type': 'text/html', 'Content-Length': Buffer.byteLength(message) }, headers ); socket.write( `HTTP/1.1 ${code} ${http.STATUS_CODES[code]}\r\n` + Object.keys(headers) .map((h) => `${h}: ${headers[h]}`) .join('\r\n') + '\r\n\r\n' + message ); } socket.removeListener('error', socketOnError); socket.destroy(); } apollo-server-demo/node_modules/ws/lib/extension.js 0000644 0001750 0000144 00000015236 03560116604 022210 0 ustar andreh users 'use strict'; // // Allowed token characters: // // '!', '#', '$', '%', '&', ''', '*', '+', '-', // '.', 0-9, A-Z, '^', '_', '`', a-z, '|', '~' // // tokenChars[32] === 0 // ' ' // tokenChars[33] === 1 // '!' // tokenChars[34] === 0 // '"' // ... // // prettier-ignore const tokenChars = [ 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, // 0 - 15 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, // 16 - 31 0, 1, 0, 1, 1, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 0, // 32 - 47 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0, 0, // 48 - 63 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, // 64 - 79 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, // 80 - 95 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, // 96 - 111 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 0 // 112 - 127 ]; /** * Adds an offer to the map of extension offers or a parameter to the map of * parameters. * * @param {Object} dest The map of extension offers or parameters * @param {String} name The extension or parameter name * @param {(Object|Boolean|String)} elem The extension parameters or the * parameter value * @private */ function push(dest, name, elem) { if (Object.prototype.hasOwnProperty.call(dest, name)) dest[name].push(elem); else dest[name] = [elem]; } /** * Parses the `Sec-WebSocket-Extensions` header into an object. * * @param {String} header The field value of the header * @return {Object} The parsed object * @public */ function parse(header) { const offers = {}; if (header === undefined || header === '') return offers; var params = {}; var mustUnescape = false; var isEscaping = false; var inQuotes = false; var extensionName; var paramName; var start = -1; var end = -1; for (var i = 0; i < header.length; i++) { const code = header.charCodeAt(i); if (extensionName === undefined) { if (end === -1 && tokenChars[code] === 1) { if (start === -1) start = i; } else if (code === 0x20 /* ' ' */ || code === 0x09 /* '\t' */) { if (end === -1 && start !== -1) end = i; } else if (code === 0x3b /* ';' */ || code === 0x2c /* ',' */) { if (start === -1) { throw new SyntaxError(`Unexpected character at index ${i}`); } if (end === -1) end = i; const name = header.slice(start, end); if (code === 0x2c) { push(offers, name, params); params = {}; } else { extensionName = name; } start = end = -1; } else { throw new SyntaxError(`Unexpected character at index ${i}`); } } else if (paramName === undefined) { if (end === -1 && tokenChars[code] === 1) { if (start === -1) start = i; } else if (code === 0x20 || code === 0x09) { if (end === -1 && start !== -1) end = i; } else if (code === 0x3b || code === 0x2c) { if (start === -1) { throw new SyntaxError(`Unexpected character at index ${i}`); } if (end === -1) end = i; push(params, header.slice(start, end), true); if (code === 0x2c) { push(offers, extensionName, params); params = {}; extensionName = undefined; } start = end = -1; } else if (code === 0x3d /* '=' */ && start !== -1 && end === -1) { paramName = header.slice(start, i); start = end = -1; } else { throw new SyntaxError(`Unexpected character at index ${i}`); } } else { // // The value of a quoted-string after unescaping must conform to the // token ABNF, so only token characters are valid. // Ref: https://tools.ietf.org/html/rfc6455#section-9.1 // if (isEscaping) { if (tokenChars[code] !== 1) { throw new SyntaxError(`Unexpected character at index ${i}`); } if (start === -1) start = i; else if (!mustUnescape) mustUnescape = true; isEscaping = false; } else if (inQuotes) { if (tokenChars[code] === 1) { if (start === -1) start = i; } else if (code === 0x22 /* '"' */ && start !== -1) { inQuotes = false; end = i; } else if (code === 0x5c /* '\' */) { isEscaping = true; } else { throw new SyntaxError(`Unexpected character at index ${i}`); } } else if (code === 0x22 && header.charCodeAt(i - 1) === 0x3d) { inQuotes = true; } else if (end === -1 && tokenChars[code] === 1) { if (start === -1) start = i; } else if (start !== -1 && (code === 0x20 || code === 0x09)) { if (end === -1) end = i; } else if (code === 0x3b || code === 0x2c) { if (start === -1) { throw new SyntaxError(`Unexpected character at index ${i}`); } if (end === -1) end = i; var value = header.slice(start, end); if (mustUnescape) { value = value.replace(/\\/g, ''); mustUnescape = false; } push(params, paramName, value); if (code === 0x2c) { push(offers, extensionName, params); params = {}; extensionName = undefined; } paramName = undefined; start = end = -1; } else { throw new SyntaxError(`Unexpected character at index ${i}`); } } } if (start === -1 || inQuotes) { throw new SyntaxError('Unexpected end of input'); } if (end === -1) end = i; const token = header.slice(start, end); if (extensionName === undefined) { push(offers, token, {}); } else { if (paramName === undefined) { push(params, token, true); } else if (mustUnescape) { push(params, paramName, token.replace(/\\/g, '')); } else { push(params, paramName, token); } push(offers, extensionName, params); } return offers; } /** * Builds the `Sec-WebSocket-Extensions` header field value. * * @param {Object} extensions The map of extensions and parameters to format * @return {String} A string representing the given object * @public */ function format(extensions) { return Object.keys(extensions) .map((extension) => { var configurations = extensions[extension]; if (!Array.isArray(configurations)) configurations = [configurations]; return configurations .map((params) => { return [extension] .concat( Object.keys(params).map((k) => { var values = params[k]; if (!Array.isArray(values)) values = [values]; return values .map((v) => (v === true ? k : `${k}=${v}`)) .join('; '); }) ) .join('; '); }) .join(', '); }) .join(', '); } module.exports = { format, parse }; apollo-server-demo/node_modules/ws/lib/event-target.js 0000644 0001750 0000144 00000007541 03560116604 022601 0 ustar andreh users 'use strict'; /** * Class representing an event. * * @private */ class Event { /** * Create a new `Event`. * * @param {String} type The name of the event * @param {Object} target A reference to the target to which the event was dispatched */ constructor(type, target) { this.target = target; this.type = type; } } /** * Class representing a message event. * * @extends Event * @private */ class MessageEvent extends Event { /** * Create a new `MessageEvent`. * * @param {(String|Buffer|ArrayBuffer|Buffer[])} data The received data * @param {WebSocket} target A reference to the target to which the event was dispatched */ constructor(data, target) { super('message', target); this.data = data; } } /** * Class representing a close event. * * @extends Event * @private */ class CloseEvent extends Event { /** * Create a new `CloseEvent`. * * @param {Number} code The status code explaining why the connection is being closed * @param {String} reason A human-readable string explaining why the connection is closing * @param {WebSocket} target A reference to the target to which the event was dispatched */ constructor(code, reason, target) { super('close', target); this.wasClean = target._closeFrameReceived && target._closeFrameSent; this.reason = reason; this.code = code; } } /** * Class representing an open event. * * @extends Event * @private */ class OpenEvent extends Event { /** * Create a new `OpenEvent`. * * @param {WebSocket} target A reference to the target to which the event was dispatched */ constructor(target) { super('open', target); } } /** * Class representing an error event. * * @extends Event * @private */ class ErrorEvent extends Event { /** * Create a new `ErrorEvent`. * * @param {Object} error The error that generated this event * @param {WebSocket} target A reference to the target to which the event was dispatched */ constructor(error, target) { super('error', target); this.message = error.message; this.error = error; } } /** * This provides methods for emulating the `EventTarget` interface. It's not * meant to be used directly. * * @mixin */ const EventTarget = { /** * Register an event listener. * * @param {String} method A string representing the event type to listen for * @param {Function} listener The listener to add * @public */ addEventListener(method, listener) { if (typeof listener !== 'function') return; function onMessage(data) { listener.call(this, new MessageEvent(data, this)); } function onClose(code, message) { listener.call(this, new CloseEvent(code, message, this)); } function onError(error) { listener.call(this, new ErrorEvent(error, this)); } function onOpen() { listener.call(this, new OpenEvent(this)); } if (method === 'message') { onMessage._listener = listener; this.on(method, onMessage); } else if (method === 'close') { onClose._listener = listener; this.on(method, onClose); } else if (method === 'error') { onError._listener = listener; this.on(method, onError); } else if (method === 'open') { onOpen._listener = listener; this.on(method, onOpen); } else { this.on(method, listener); } }, /** * Remove an event listener. * * @param {String} method A string representing the event type to remove * @param {Function} listener The listener to remove * @public */ removeEventListener(method, listener) { const listeners = this.listeners(method); for (var i = 0; i < listeners.length; i++) { if (listeners[i] === listener || listeners[i]._listener === listener) { this.removeListener(method, listeners[i]); } } } }; module.exports = EventTarget; apollo-server-demo/node_modules/ws/lib/permessage-deflate.js 0000644 0001750 0000144 00000033463 03560116604 023733 0 ustar andreh users 'use strict'; const Limiter = require('async-limiter'); const zlib = require('zlib'); const bufferUtil = require('./buffer-util'); const { kStatusCode, NOOP } = require('./constants'); const TRAILER = Buffer.from([0x00, 0x00, 0xff, 0xff]); const EMPTY_BLOCK = Buffer.from([0x00]); const kPerMessageDeflate = Symbol('permessage-deflate'); const kTotalLength = Symbol('total-length'); const kCallback = Symbol('callback'); const kBuffers = Symbol('buffers'); const kError = Symbol('error'); // // We limit zlib concurrency, which prevents severe memory fragmentation // as documented in https://github.com/nodejs/node/issues/8871#issuecomment-250915913 // and https://github.com/websockets/ws/issues/1202 // // Intentionally global; it's the global thread pool that's an issue. // let zlibLimiter; /** * permessage-deflate implementation. */ class PerMessageDeflate { /** * Creates a PerMessageDeflate instance. * * @param {Object} options Configuration options * @param {Boolean} options.serverNoContextTakeover Request/accept disabling * of server context takeover * @param {Boolean} options.clientNoContextTakeover Advertise/acknowledge * disabling of client context takeover * @param {(Boolean|Number)} options.serverMaxWindowBits Request/confirm the * use of a custom server window size * @param {(Boolean|Number)} options.clientMaxWindowBits Advertise support * for, or request, a custom client window size * @param {Object} options.zlibDeflateOptions Options to pass to zlib on deflate * @param {Object} options.zlibInflateOptions Options to pass to zlib on inflate * @param {Number} options.threshold Size (in bytes) below which messages * should not be compressed * @param {Number} options.concurrencyLimit The number of concurrent calls to * zlib * @param {Boolean} isServer Create the instance in either server or client * mode * @param {Number} maxPayload The maximum allowed message length */ constructor(options, isServer, maxPayload) { this._maxPayload = maxPayload | 0; this._options = options || {}; this._threshold = this._options.threshold !== undefined ? this._options.threshold : 1024; this._isServer = !!isServer; this._deflate = null; this._inflate = null; this.params = null; if (!zlibLimiter) { const concurrency = this._options.concurrencyLimit !== undefined ? this._options.concurrencyLimit : 10; zlibLimiter = new Limiter({ concurrency }); } } /** * @type {String} */ static get extensionName() { return 'permessage-deflate'; } /** * Create an extension negotiation offer. * * @return {Object} Extension parameters * @public */ offer() { const params = {}; if (this._options.serverNoContextTakeover) { params.server_no_context_takeover = true; } if (this._options.clientNoContextTakeover) { params.client_no_context_takeover = true; } if (this._options.serverMaxWindowBits) { params.server_max_window_bits = this._options.serverMaxWindowBits; } if (this._options.clientMaxWindowBits) { params.client_max_window_bits = this._options.clientMaxWindowBits; } else if (this._options.clientMaxWindowBits == null) { params.client_max_window_bits = true; } return params; } /** * Accept an extension negotiation offer/response. * * @param {Array} configurations The extension negotiation offers/reponse * @return {Object} Accepted configuration * @public */ accept(configurations) { configurations = this.normalizeParams(configurations); this.params = this._isServer ? this.acceptAsServer(configurations) : this.acceptAsClient(configurations); return this.params; } /** * Releases all resources used by the extension. * * @public */ cleanup() { if (this._inflate) { this._inflate.close(); this._inflate = null; } if (this._deflate) { this._deflate.close(); this._deflate = null; } } /** * Accept an extension negotiation offer. * * @param {Array} offers The extension negotiation offers * @return {Object} Accepted configuration * @private */ acceptAsServer(offers) { const opts = this._options; const accepted = offers.find((params) => { if ( (opts.serverNoContextTakeover === false && params.server_no_context_takeover) || (params.server_max_window_bits && (opts.serverMaxWindowBits === false || (typeof opts.serverMaxWindowBits === 'number' && opts.serverMaxWindowBits > params.server_max_window_bits))) || (typeof opts.clientMaxWindowBits === 'number' && !params.client_max_window_bits) ) { return false; } return true; }); if (!accepted) { throw new Error('None of the extension offers can be accepted'); } if (opts.serverNoContextTakeover) { accepted.server_no_context_takeover = true; } if (opts.clientNoContextTakeover) { accepted.client_no_context_takeover = true; } if (typeof opts.serverMaxWindowBits === 'number') { accepted.server_max_window_bits = opts.serverMaxWindowBits; } if (typeof opts.clientMaxWindowBits === 'number') { accepted.client_max_window_bits = opts.clientMaxWindowBits; } else if ( accepted.client_max_window_bits === true || opts.clientMaxWindowBits === false ) { delete accepted.client_max_window_bits; } return accepted; } /** * Accept the extension negotiation response. * * @param {Array} response The extension negotiation response * @return {Object} Accepted configuration * @private */ acceptAsClient(response) { const params = response[0]; if ( this._options.clientNoContextTakeover === false && params.client_no_context_takeover ) { throw new Error('Unexpected parameter "client_no_context_takeover"'); } if (!params.client_max_window_bits) { if (typeof this._options.clientMaxWindowBits === 'number') { params.client_max_window_bits = this._options.clientMaxWindowBits; } } else if ( this._options.clientMaxWindowBits === false || (typeof this._options.clientMaxWindowBits === 'number' && params.client_max_window_bits > this._options.clientMaxWindowBits) ) { throw new Error( 'Unexpected or invalid parameter "client_max_window_bits"' ); } return params; } /** * Normalize parameters. * * @param {Array} configurations The extension negotiation offers/reponse * @return {Array} The offers/response with normalized parameters * @private */ normalizeParams(configurations) { configurations.forEach((params) => { Object.keys(params).forEach((key) => { var value = params[key]; if (value.length > 1) { throw new Error(`Parameter "${key}" must have only a single value`); } value = value[0]; if (key === 'client_max_window_bits') { if (value !== true) { const num = +value; if (!Number.isInteger(num) || num < 8 || num > 15) { throw new TypeError( `Invalid value for parameter "${key}": ${value}` ); } value = num; } else if (!this._isServer) { throw new TypeError( `Invalid value for parameter "${key}": ${value}` ); } } else if (key === 'server_max_window_bits') { const num = +value; if (!Number.isInteger(num) || num < 8 || num > 15) { throw new TypeError( `Invalid value for parameter "${key}": ${value}` ); } value = num; } else if ( key === 'client_no_context_takeover' || key === 'server_no_context_takeover' ) { if (value !== true) { throw new TypeError( `Invalid value for parameter "${key}": ${value}` ); } } else { throw new Error(`Unknown parameter "${key}"`); } params[key] = value; }); }); return configurations; } /** * Decompress data. Concurrency limited by async-limiter. * * @param {Buffer} data Compressed data * @param {Boolean} fin Specifies whether or not this is the last fragment * @param {Function} callback Callback * @public */ decompress(data, fin, callback) { zlibLimiter.push((done) => { this._decompress(data, fin, (err, result) => { done(); callback(err, result); }); }); } /** * Compress data. Concurrency limited by async-limiter. * * @param {Buffer} data Data to compress * @param {Boolean} fin Specifies whether or not this is the last fragment * @param {Function} callback Callback * @public */ compress(data, fin, callback) { zlibLimiter.push((done) => { this._compress(data, fin, (err, result) => { done(); callback(err, result); }); }); } /** * Decompress data. * * @param {Buffer} data Compressed data * @param {Boolean} fin Specifies whether or not this is the last fragment * @param {Function} callback Callback * @private */ _decompress(data, fin, callback) { const endpoint = this._isServer ? 'client' : 'server'; if (!this._inflate) { const key = `${endpoint}_max_window_bits`; const windowBits = typeof this.params[key] !== 'number' ? zlib.Z_DEFAULT_WINDOWBITS : this.params[key]; this._inflate = zlib.createInflateRaw( Object.assign({}, this._options.zlibInflateOptions, { windowBits }) ); this._inflate[kPerMessageDeflate] = this; this._inflate[kTotalLength] = 0; this._inflate[kBuffers] = []; this._inflate.on('error', inflateOnError); this._inflate.on('data', inflateOnData); } this._inflate[kCallback] = callback; this._inflate.write(data); if (fin) this._inflate.write(TRAILER); this._inflate.flush(() => { const err = this._inflate[kError]; if (err) { this._inflate.close(); this._inflate = null; callback(err); return; } const data = bufferUtil.concat( this._inflate[kBuffers], this._inflate[kTotalLength] ); if (fin && this.params[`${endpoint}_no_context_takeover`]) { this._inflate.close(); this._inflate = null; } else { this._inflate[kTotalLength] = 0; this._inflate[kBuffers] = []; } callback(null, data); }); } /** * Compress data. * * @param {Buffer} data Data to compress * @param {Boolean} fin Specifies whether or not this is the last fragment * @param {Function} callback Callback * @private */ _compress(data, fin, callback) { if (!data || data.length === 0) { process.nextTick(callback, null, EMPTY_BLOCK); return; } const endpoint = this._isServer ? 'server' : 'client'; if (!this._deflate) { const key = `${endpoint}_max_window_bits`; const windowBits = typeof this.params[key] !== 'number' ? zlib.Z_DEFAULT_WINDOWBITS : this.params[key]; this._deflate = zlib.createDeflateRaw( Object.assign({}, this._options.zlibDeflateOptions, { windowBits }) ); this._deflate[kTotalLength] = 0; this._deflate[kBuffers] = []; // // An `'error'` event is emitted, only on Node.js < 10.0.0, if the // `zlib.DeflateRaw` instance is closed while data is being processed. // This can happen if `PerMessageDeflate#cleanup()` is called at the wrong // time due to an abnormal WebSocket closure. // this._deflate.on('error', NOOP); this._deflate.on('data', deflateOnData); } this._deflate.write(data); this._deflate.flush(zlib.Z_SYNC_FLUSH, () => { if (!this._deflate) { // // This `if` statement is only needed for Node.js < 10.0.0 because as of // commit https://github.com/nodejs/node/commit/5e3f5164, the flush // callback is no longer called if the deflate stream is closed while // data is being processed. // return; } var data = bufferUtil.concat( this._deflate[kBuffers], this._deflate[kTotalLength] ); if (fin) data = data.slice(0, data.length - 4); if (fin && this.params[`${endpoint}_no_context_takeover`]) { this._deflate.close(); this._deflate = null; } else { this._deflate[kTotalLength] = 0; this._deflate[kBuffers] = []; } callback(null, data); }); } } module.exports = PerMessageDeflate; /** * The listener of the `zlib.DeflateRaw` stream `'data'` event. * * @param {Buffer} chunk A chunk of data * @private */ function deflateOnData(chunk) { this[kBuffers].push(chunk); this[kTotalLength] += chunk.length; } /** * The listener of the `zlib.InflateRaw` stream `'data'` event. * * @param {Buffer} chunk A chunk of data * @private */ function inflateOnData(chunk) { this[kTotalLength] += chunk.length; if ( this[kPerMessageDeflate]._maxPayload < 1 || this[kTotalLength] <= this[kPerMessageDeflate]._maxPayload ) { this[kBuffers].push(chunk); return; } this[kError] = new RangeError('Max payload size exceeded'); this[kError][kStatusCode] = 1009; this.removeListener('data', inflateOnData); this.reset(); } /** * The listener of the `zlib.InflateRaw` stream `'error'` event. * * @param {Error} err The emitted error * @private */ function inflateOnError(err) { // // There is no need to call `Zlib#close()` as the handle is automatically // closed when an error is emitted. // this[kPerMessageDeflate]._inflate = null; err[kStatusCode] = 1007; this[kCallback](err); } apollo-server-demo/node_modules/ws/lib/receiver.js 0000644 0001750 0000144 00000027211 03560116604 021774 0 ustar andreh users 'use strict'; const { Writable } = require('stream'); const PerMessageDeflate = require('./permessage-deflate'); const { BINARY_TYPES, EMPTY_BUFFER, kStatusCode, kWebSocket } = require('./constants'); const { concat, toArrayBuffer, unmask } = require('./buffer-util'); const { isValidStatusCode, isValidUTF8 } = require('./validation'); const GET_INFO = 0; const GET_PAYLOAD_LENGTH_16 = 1; const GET_PAYLOAD_LENGTH_64 = 2; const GET_MASK = 3; const GET_DATA = 4; const INFLATING = 5; /** * HyBi Receiver implementation. * * @extends stream.Writable */ class Receiver extends Writable { /** * Creates a Receiver instance. * * @param {String} binaryType The type for binary data * @param {Object} extensions An object containing the negotiated extensions * @param {Number} maxPayload The maximum allowed message length */ constructor(binaryType, extensions, maxPayload) { super(); this._binaryType = binaryType || BINARY_TYPES[0]; this[kWebSocket] = undefined; this._extensions = extensions || {}; this._maxPayload = maxPayload | 0; this._bufferedBytes = 0; this._buffers = []; this._compressed = false; this._payloadLength = 0; this._mask = undefined; this._fragmented = 0; this._masked = false; this._fin = false; this._opcode = 0; this._totalPayloadLength = 0; this._messageLength = 0; this._fragments = []; this._state = GET_INFO; this._loop = false; } /** * Implements `Writable.prototype._write()`. * * @param {Buffer} chunk The chunk of data to write * @param {String} encoding The character encoding of `chunk` * @param {Function} cb Callback */ _write(chunk, encoding, cb) { if (this._opcode === 0x08 && this._state == GET_INFO) return cb(); this._bufferedBytes += chunk.length; this._buffers.push(chunk); this.startLoop(cb); } /** * Consumes `n` bytes from the buffered data. * * @param {Number} n The number of bytes to consume * @return {Buffer} The consumed bytes * @private */ consume(n) { this._bufferedBytes -= n; if (n === this._buffers[0].length) return this._buffers.shift(); if (n < this._buffers[0].length) { const buf = this._buffers[0]; this._buffers[0] = buf.slice(n); return buf.slice(0, n); } const dst = Buffer.allocUnsafe(n); do { const buf = this._buffers[0]; if (n >= buf.length) { this._buffers.shift().copy(dst, dst.length - n); } else { buf.copy(dst, dst.length - n, 0, n); this._buffers[0] = buf.slice(n); } n -= buf.length; } while (n > 0); return dst; } /** * Starts the parsing loop. * * @param {Function} cb Callback * @private */ startLoop(cb) { var err; this._loop = true; do { switch (this._state) { case GET_INFO: err = this.getInfo(); break; case GET_PAYLOAD_LENGTH_16: err = this.getPayloadLength16(); break; case GET_PAYLOAD_LENGTH_64: err = this.getPayloadLength64(); break; case GET_MASK: this.getMask(); break; case GET_DATA: err = this.getData(cb); break; default: // `INFLATING` this._loop = false; return; } } while (this._loop); cb(err); } /** * Reads the first two bytes of a frame. * * @return {(RangeError|undefined)} A possible error * @private */ getInfo() { if (this._bufferedBytes < 2) { this._loop = false; return; } const buf = this.consume(2); if ((buf[0] & 0x30) !== 0x00) { this._loop = false; return error(RangeError, 'RSV2 and RSV3 must be clear', true, 1002); } const compressed = (buf[0] & 0x40) === 0x40; if (compressed && !this._extensions[PerMessageDeflate.extensionName]) { this._loop = false; return error(RangeError, 'RSV1 must be clear', true, 1002); } this._fin = (buf[0] & 0x80) === 0x80; this._opcode = buf[0] & 0x0f; this._payloadLength = buf[1] & 0x7f; if (this._opcode === 0x00) { if (compressed) { this._loop = false; return error(RangeError, 'RSV1 must be clear', true, 1002); } if (!this._fragmented) { this._loop = false; return error(RangeError, 'invalid opcode 0', true, 1002); } this._opcode = this._fragmented; } else if (this._opcode === 0x01 || this._opcode === 0x02) { if (this._fragmented) { this._loop = false; return error(RangeError, `invalid opcode ${this._opcode}`, true, 1002); } this._compressed = compressed; } else if (this._opcode > 0x07 && this._opcode < 0x0b) { if (!this._fin) { this._loop = false; return error(RangeError, 'FIN must be set', true, 1002); } if (compressed) { this._loop = false; return error(RangeError, 'RSV1 must be clear', true, 1002); } if (this._payloadLength > 0x7d) { this._loop = false; return error( RangeError, `invalid payload length ${this._payloadLength}`, true, 1002 ); } } else { this._loop = false; return error(RangeError, `invalid opcode ${this._opcode}`, true, 1002); } if (!this._fin && !this._fragmented) this._fragmented = this._opcode; this._masked = (buf[1] & 0x80) === 0x80; if (this._payloadLength === 126) this._state = GET_PAYLOAD_LENGTH_16; else if (this._payloadLength === 127) this._state = GET_PAYLOAD_LENGTH_64; else return this.haveLength(); } /** * Gets extended payload length (7+16). * * @return {(RangeError|undefined)} A possible error * @private */ getPayloadLength16() { if (this._bufferedBytes < 2) { this._loop = false; return; } this._payloadLength = this.consume(2).readUInt16BE(0); return this.haveLength(); } /** * Gets extended payload length (7+64). * * @return {(RangeError|undefined)} A possible error * @private */ getPayloadLength64() { if (this._bufferedBytes < 8) { this._loop = false; return; } const buf = this.consume(8); const num = buf.readUInt32BE(0); // // The maximum safe integer in JavaScript is 2^53 - 1. An error is returned // if payload length is greater than this number. // if (num > Math.pow(2, 53 - 32) - 1) { this._loop = false; return error( RangeError, 'Unsupported WebSocket frame: payload length > 2^53 - 1', false, 1009 ); } this._payloadLength = num * Math.pow(2, 32) + buf.readUInt32BE(4); return this.haveLength(); } /** * Payload length has been read. * * @return {(RangeError|undefined)} A possible error * @private */ haveLength() { if (this._payloadLength && this._opcode < 0x08) { this._totalPayloadLength += this._payloadLength; if (this._totalPayloadLength > this._maxPayload && this._maxPayload > 0) { this._loop = false; return error(RangeError, 'Max payload size exceeded', false, 1009); } } if (this._masked) this._state = GET_MASK; else this._state = GET_DATA; } /** * Reads mask bytes. * * @private */ getMask() { if (this._bufferedBytes < 4) { this._loop = false; return; } this._mask = this.consume(4); this._state = GET_DATA; } /** * Reads data bytes. * * @param {Function} cb Callback * @return {(Error|RangeError|undefined)} A possible error * @private */ getData(cb) { var data = EMPTY_BUFFER; if (this._payloadLength) { if (this._bufferedBytes < this._payloadLength) { this._loop = false; return; } data = this.consume(this._payloadLength); if (this._masked) unmask(data, this._mask); } if (this._opcode > 0x07) return this.controlMessage(data); if (this._compressed) { this._state = INFLATING; this.decompress(data, cb); return; } if (data.length) { // // This message is not compressed so its lenght is the sum of the payload // length of all fragments. // this._messageLength = this._totalPayloadLength; this._fragments.push(data); } return this.dataMessage(); } /** * Decompresses data. * * @param {Buffer} data Compressed data * @param {Function} cb Callback * @private */ decompress(data, cb) { const perMessageDeflate = this._extensions[PerMessageDeflate.extensionName]; perMessageDeflate.decompress(data, this._fin, (err, buf) => { if (err) return cb(err); if (buf.length) { this._messageLength += buf.length; if (this._messageLength > this._maxPayload && this._maxPayload > 0) { return cb( error(RangeError, 'Max payload size exceeded', false, 1009) ); } this._fragments.push(buf); } const er = this.dataMessage(); if (er) return cb(er); this.startLoop(cb); }); } /** * Handles a data message. * * @return {(Error|undefined)} A possible error * @private */ dataMessage() { if (this._fin) { const messageLength = this._messageLength; const fragments = this._fragments; this._totalPayloadLength = 0; this._messageLength = 0; this._fragmented = 0; this._fragments = []; if (this._opcode === 2) { var data; if (this._binaryType === 'nodebuffer') { data = concat(fragments, messageLength); } else if (this._binaryType === 'arraybuffer') { data = toArrayBuffer(concat(fragments, messageLength)); } else { data = fragments; } this.emit('message', data); } else { const buf = concat(fragments, messageLength); if (!isValidUTF8(buf)) { this._loop = false; return error(Error, 'invalid UTF-8 sequence', true, 1007); } this.emit('message', buf.toString()); } } this._state = GET_INFO; } /** * Handles a control message. * * @param {Buffer} data Data to handle * @return {(Error|RangeError|undefined)} A possible error * @private */ controlMessage(data) { if (this._opcode === 0x08) { this._loop = false; if (data.length === 0) { this.emit('conclude', 1005, ''); this.end(); } else if (data.length === 1) { return error(RangeError, 'invalid payload length 1', true, 1002); } else { const code = data.readUInt16BE(0); if (!isValidStatusCode(code)) { return error(RangeError, `invalid status code ${code}`, true, 1002); } const buf = data.slice(2); if (!isValidUTF8(buf)) { return error(Error, 'invalid UTF-8 sequence', true, 1007); } this.emit('conclude', code, buf.toString()); this.end(); } } else if (this._opcode === 0x09) { this.emit('ping', data); } else { this.emit('pong', data); } this._state = GET_INFO; } } module.exports = Receiver; /** * Builds an error object. * * @param {(Error|RangeError)} ErrorCtor The error constructor * @param {String} message The error message * @param {Boolean} prefix Specifies whether or not to add a default prefix to * `message` * @param {Number} statusCode The status code * @return {(Error|RangeError)} The error * @private */ function error(ErrorCtor, message, prefix, statusCode) { const err = new ErrorCtor( prefix ? `Invalid WebSocket frame: ${message}` : message ); Error.captureStackTrace(err, error); err[kStatusCode] = statusCode; return err; } apollo-server-demo/node_modules/ws/lib/validation.js 0000644 0001750 0000144 00000001267 03560116604 022325 0 ustar andreh users 'use strict'; try { const isValidUTF8 = require('utf-8-validate'); exports.isValidUTF8 = typeof isValidUTF8 === 'object' ? isValidUTF8.Validation.isValidUTF8 // utf-8-validate@<3.0.0 : isValidUTF8; } catch (e) /* istanbul ignore next */ { exports.isValidUTF8 = () => true; } /** * Checks if a status code is allowed in a close frame. * * @param {Number} code The status code * @return {Boolean} `true` if the status code is valid, else `false` * @public */ exports.isValidStatusCode = (code) => { return ( (code >= 1000 && code <= 1013 && code !== 1004 && code !== 1005 && code !== 1006) || (code >= 3000 && code <= 4999) ); }; apollo-server-demo/node_modules/ws/lib/buffer-util.js 0000644 0001750 0000144 00000006401 03560116604 022412 0 ustar andreh users 'use strict'; const { EMPTY_BUFFER } = require('./constants'); /** * Merges an array of buffers into a new buffer. * * @param {Buffer[]} list The array of buffers to concat * @param {Number} totalLength The total length of buffers in the list * @return {Buffer} The resulting buffer * @public */ function concat(list, totalLength) { if (list.length === 0) return EMPTY_BUFFER; if (list.length === 1) return list[0]; const target = Buffer.allocUnsafe(totalLength); var offset = 0; for (var i = 0; i < list.length; i++) { const buf = list[i]; buf.copy(target, offset); offset += buf.length; } return target; } /** * Masks a buffer using the given mask. * * @param {Buffer} source The buffer to mask * @param {Buffer} mask The mask to use * @param {Buffer} output The buffer where to store the result * @param {Number} offset The offset at which to start writing * @param {Number} length The number of bytes to mask. * @public */ function _mask(source, mask, output, offset, length) { for (var i = 0; i < length; i++) { output[offset + i] = source[i] ^ mask[i & 3]; } } /** * Unmasks a buffer using the given mask. * * @param {Buffer} buffer The buffer to unmask * @param {Buffer} mask The mask to use * @public */ function _unmask(buffer, mask) { // Required until https://github.com/nodejs/node/issues/9006 is resolved. const length = buffer.length; for (var i = 0; i < length; i++) { buffer[i] ^= mask[i & 3]; } } /** * Converts a buffer to an `ArrayBuffer`. * * @param {Buffer} buf The buffer to convert * @return {ArrayBuffer} Converted buffer * @public */ function toArrayBuffer(buf) { if (buf.byteLength === buf.buffer.byteLength) { return buf.buffer; } return buf.buffer.slice(buf.byteOffset, buf.byteOffset + buf.byteLength); } /** * Converts `data` to a `Buffer`. * * @param {*} data The data to convert * @return {Buffer} The buffer * @throws {TypeError} * @public */ function toBuffer(data) { toBuffer.readOnly = true; if (Buffer.isBuffer(data)) return data; var buf; if (data instanceof ArrayBuffer) { buf = Buffer.from(data); } else if (ArrayBuffer.isView(data)) { buf = viewToBuffer(data); } else { buf = Buffer.from(data); toBuffer.readOnly = false; } return buf; } /** * Converts an `ArrayBuffer` view into a buffer. * * @param {(DataView|TypedArray)} view The view to convert * @return {Buffer} Converted view * @private */ function viewToBuffer(view) { const buf = Buffer.from(view.buffer); if (view.byteLength !== view.buffer.byteLength) { return buf.slice(view.byteOffset, view.byteOffset + view.byteLength); } return buf; } try { const bufferUtil = require('bufferutil'); const bu = bufferUtil.BufferUtil || bufferUtil; module.exports = { concat, mask(source, mask, output, offset, length) { if (length < 48) _mask(source, mask, output, offset, length); else bu.mask(source, mask, output, offset, length); }, toArrayBuffer, toBuffer, unmask(buffer, mask) { if (buffer.length < 32) _unmask(buffer, mask); else bu.unmask(buffer, mask); } }; } catch (e) /* istanbul ignore next */ { module.exports = { concat, mask: _mask, toArrayBuffer, toBuffer, unmask: _unmask }; } apollo-server-demo/node_modules/ws/lib/websocket.js 0000644 0001750 0000144 00000056660 03560116604 022170 0 ustar andreh users 'use strict'; const EventEmitter = require('events'); const crypto = require('crypto'); const https = require('https'); const http = require('http'); const net = require('net'); const tls = require('tls'); const url = require('url'); const PerMessageDeflate = require('./permessage-deflate'); const EventTarget = require('./event-target'); const extension = require('./extension'); const Receiver = require('./receiver'); const Sender = require('./sender'); const { BINARY_TYPES, EMPTY_BUFFER, GUID, kStatusCode, kWebSocket, NOOP } = require('./constants'); const readyStates = ['CONNECTING', 'OPEN', 'CLOSING', 'CLOSED']; const protocolVersions = [8, 13]; const closeTimeout = 30 * 1000; /** * Class representing a WebSocket. * * @extends EventEmitter */ class WebSocket extends EventEmitter { /** * Create a new `WebSocket`. * * @param {(String|url.Url|url.URL)} address The URL to which to connect * @param {(String|String[])} protocols The subprotocols * @param {Object} options Connection options */ constructor(address, protocols, options) { super(); this.readyState = WebSocket.CONNECTING; this.protocol = ''; this._binaryType = BINARY_TYPES[0]; this._closeFrameReceived = false; this._closeFrameSent = false; this._closeMessage = ''; this._closeTimer = null; this._closeCode = 1006; this._extensions = {}; this._receiver = null; this._sender = null; this._socket = null; if (address !== null) { this._isServer = false; this._redirects = 0; if (Array.isArray(protocols)) { protocols = protocols.join(', '); } else if (typeof protocols === 'object' && protocols !== null) { options = protocols; protocols = undefined; } initAsClient(this, address, protocols, options); } else { this._isServer = true; } } get CONNECTING() { return WebSocket.CONNECTING; } get CLOSING() { return WebSocket.CLOSING; } get CLOSED() { return WebSocket.CLOSED; } get OPEN() { return WebSocket.OPEN; } /** * This deviates from the WHATWG interface since ws doesn't support the * required default "blob" type (instead we define a custom "nodebuffer" * type). * * @type {String} */ get binaryType() { return this._binaryType; } set binaryType(type) { if (!BINARY_TYPES.includes(type)) return; this._binaryType = type; // // Allow to change `binaryType` on the fly. // if (this._receiver) this._receiver._binaryType = type; } /** * @type {Number} */ get bufferedAmount() { if (!this._socket) return 0; // // `socket.bufferSize` is `undefined` if the socket is closed. // return (this._socket.bufferSize || 0) + this._sender._bufferedBytes; } /** * @type {String} */ get extensions() { return Object.keys(this._extensions).join(); } /** * Set up the socket and the internal resources. * * @param {net.Socket} socket The network socket between the server and client * @param {Buffer} head The first packet of the upgraded stream * @param {Number} maxPayload The maximum allowed message size * @private */ setSocket(socket, head, maxPayload) { const receiver = new Receiver( this._binaryType, this._extensions, maxPayload ); this._sender = new Sender(socket, this._extensions); this._receiver = receiver; this._socket = socket; receiver[kWebSocket] = this; socket[kWebSocket] = this; receiver.on('conclude', receiverOnConclude); receiver.on('drain', receiverOnDrain); receiver.on('error', receiverOnError); receiver.on('message', receiverOnMessage); receiver.on('ping', receiverOnPing); receiver.on('pong', receiverOnPong); socket.setTimeout(0); socket.setNoDelay(); if (head.length > 0) socket.unshift(head); socket.on('close', socketOnClose); socket.on('data', socketOnData); socket.on('end', socketOnEnd); socket.on('error', socketOnError); this.readyState = WebSocket.OPEN; this.emit('open'); } /** * Emit the `'close'` event. * * @private */ emitClose() { this.readyState = WebSocket.CLOSED; if (!this._socket) { this.emit('close', this._closeCode, this._closeMessage); return; } if (this._extensions[PerMessageDeflate.extensionName]) { this._extensions[PerMessageDeflate.extensionName].cleanup(); } this._receiver.removeAllListeners(); this.emit('close', this._closeCode, this._closeMessage); } /** * Start a closing handshake. * * +----------+ +-----------+ +----------+ * - - -|ws.close()|-->|close frame|-->|ws.close()|- - - * | +----------+ +-----------+ +----------+ | * +----------+ +-----------+ | * CLOSING |ws.close()|<--|close frame|<--+-----+ CLOSING * +----------+ +-----------+ | * | | | +---+ | * +------------------------+-->|fin| - - - - * | +---+ | +---+ * - - - - -|fin|<---------------------+ * +---+ * * @param {Number} code Status code explaining why the connection is closing * @param {String} data A string explaining why the connection is closing * @public */ close(code, data) { if (this.readyState === WebSocket.CLOSED) return; if (this.readyState === WebSocket.CONNECTING) { const msg = 'WebSocket was closed before the connection was established'; return abortHandshake(this, this._req, msg); } if (this.readyState === WebSocket.CLOSING) { if (this._closeFrameSent && this._closeFrameReceived) this._socket.end(); return; } this.readyState = WebSocket.CLOSING; this._sender.close(code, data, !this._isServer, (err) => { // // This error is handled by the `'error'` listener on the socket. We only // want to know if the close frame has been sent here. // if (err) return; this._closeFrameSent = true; if (this._closeFrameReceived) this._socket.end(); }); // // Specify a timeout for the closing handshake to complete. // this._closeTimer = setTimeout( this._socket.destroy.bind(this._socket), closeTimeout ); } /** * Send a ping. * * @param {*} data The data to send * @param {Boolean} mask Indicates whether or not to mask `data` * @param {Function} cb Callback which is executed when the ping is sent * @public */ ping(data, mask, cb) { if (typeof data === 'function') { cb = data; data = mask = undefined; } else if (typeof mask === 'function') { cb = mask; mask = undefined; } if (this.readyState !== WebSocket.OPEN) { const err = new Error( `WebSocket is not open: readyState ${this.readyState} ` + `(${readyStates[this.readyState]})` ); if (cb) return cb(err); throw err; } if (typeof data === 'number') data = data.toString(); if (mask === undefined) mask = !this._isServer; this._sender.ping(data || EMPTY_BUFFER, mask, cb); } /** * Send a pong. * * @param {*} data The data to send * @param {Boolean} mask Indicates whether or not to mask `data` * @param {Function} cb Callback which is executed when the pong is sent * @public */ pong(data, mask, cb) { if (typeof data === 'function') { cb = data; data = mask = undefined; } else if (typeof mask === 'function') { cb = mask; mask = undefined; } if (this.readyState !== WebSocket.OPEN) { const err = new Error( `WebSocket is not open: readyState ${this.readyState} ` + `(${readyStates[this.readyState]})` ); if (cb) return cb(err); throw err; } if (typeof data === 'number') data = data.toString(); if (mask === undefined) mask = !this._isServer; this._sender.pong(data || EMPTY_BUFFER, mask, cb); } /** * Send a data message. * * @param {*} data The message to send * @param {Object} options Options object * @param {Boolean} options.compress Specifies whether or not to compress `data` * @param {Boolean} options.binary Specifies whether `data` is binary or text * @param {Boolean} options.fin Specifies whether the fragment is the last one * @param {Boolean} options.mask Specifies whether or not to mask `data` * @param {Function} cb Callback which is executed when data is written out * @public */ send(data, options, cb) { if (typeof options === 'function') { cb = options; options = {}; } if (this.readyState !== WebSocket.OPEN) { const err = new Error( `WebSocket is not open: readyState ${this.readyState} ` + `(${readyStates[this.readyState]})` ); if (cb) return cb(err); throw err; } if (typeof data === 'number') data = data.toString(); const opts = Object.assign( { binary: typeof data !== 'string', mask: !this._isServer, compress: true, fin: true }, options ); if (!this._extensions[PerMessageDeflate.extensionName]) { opts.compress = false; } this._sender.send(data || EMPTY_BUFFER, opts, cb); } /** * Forcibly close the connection. * * @public */ terminate() { if (this.readyState === WebSocket.CLOSED) return; if (this.readyState === WebSocket.CONNECTING) { const msg = 'WebSocket was closed before the connection was established'; return abortHandshake(this, this._req, msg); } if (this._socket) { this.readyState = WebSocket.CLOSING; this._socket.destroy(); } } } readyStates.forEach((readyState, i) => { WebSocket[readyState] = i; }); // // Add the `onopen`, `onerror`, `onclose`, and `onmessage` attributes. // See https://html.spec.whatwg.org/multipage/comms.html#the-websocket-interface // ['open', 'error', 'close', 'message'].forEach((method) => { Object.defineProperty(WebSocket.prototype, `on${method}`, { /** * Return the listener of the event. * * @return {(Function|undefined)} The event listener or `undefined` * @public */ get() { const listeners = this.listeners(method); for (var i = 0; i < listeners.length; i++) { if (listeners[i]._listener) return listeners[i]._listener; } return undefined; }, /** * Add a listener for the event. * * @param {Function} listener The listener to add * @public */ set(listener) { const listeners = this.listeners(method); for (var i = 0; i < listeners.length; i++) { // // Remove only the listeners added via `addEventListener`. // if (listeners[i]._listener) this.removeListener(method, listeners[i]); } this.addEventListener(method, listener); } }); }); WebSocket.prototype.addEventListener = EventTarget.addEventListener; WebSocket.prototype.removeEventListener = EventTarget.removeEventListener; module.exports = WebSocket; /** * Initialize a WebSocket client. * * @param {WebSocket} websocket The client to initialize * @param {(String|url.Url|url.URL)} address The URL to which to connect * @param {String} protocols The subprotocols * @param {Object} options Connection options * @param {(Boolean|Object)} options.perMessageDeflate Enable/disable * permessage-deflate * @param {Number} options.handshakeTimeout Timeout in milliseconds for the * handshake request * @param {Number} options.protocolVersion Value of the `Sec-WebSocket-Version` * header * @param {String} options.origin Value of the `Origin` or * `Sec-WebSocket-Origin` header * @param {Number} options.maxPayload The maximum allowed message size * @param {Boolean} options.followRedirects Whether or not to follow redirects * @param {Number} options.maxRedirects The maximum number of redirects allowed * @private */ function initAsClient(websocket, address, protocols, options) { const opts = Object.assign( { protocolVersion: protocolVersions[1], maxPayload: 100 * 1024 * 1024, perMessageDeflate: true, followRedirects: false, maxRedirects: 10 }, options, { createConnection: undefined, socketPath: undefined, hostname: undefined, protocol: undefined, timeout: undefined, method: undefined, auth: undefined, host: undefined, path: undefined, port: undefined } ); if (!protocolVersions.includes(opts.protocolVersion)) { throw new RangeError( `Unsupported protocol version: ${opts.protocolVersion} ` + `(supported versions: ${protocolVersions.join(', ')})` ); } var parsedUrl; if (typeof address === 'object' && address.href !== undefined) { parsedUrl = address; websocket.url = address.href; } else { // // The WHATWG URL constructor is not available on Node.js < 6.13.0 // parsedUrl = url.URL ? new url.URL(address) : url.parse(address); websocket.url = address; } const isUnixSocket = parsedUrl.protocol === 'ws+unix:'; if (!parsedUrl.host && (!isUnixSocket || !parsedUrl.pathname)) { throw new Error(`Invalid URL: ${websocket.url}`); } const isSecure = parsedUrl.protocol === 'wss:' || parsedUrl.protocol === 'https:'; const defaultPort = isSecure ? 443 : 80; const key = crypto.randomBytes(16).toString('base64'); const get = isSecure ? https.get : http.get; const path = parsedUrl.search ? `${parsedUrl.pathname || '/'}${parsedUrl.search}` : parsedUrl.pathname || '/'; var perMessageDeflate; opts.createConnection = isSecure ? tlsConnect : netConnect; opts.defaultPort = opts.defaultPort || defaultPort; opts.port = parsedUrl.port || defaultPort; opts.host = parsedUrl.hostname.startsWith('[') ? parsedUrl.hostname.slice(1, -1) : parsedUrl.hostname; opts.headers = Object.assign( { 'Sec-WebSocket-Version': opts.protocolVersion, 'Sec-WebSocket-Key': key, Connection: 'Upgrade', Upgrade: 'websocket' }, opts.headers ); opts.path = path; opts.timeout = opts.handshakeTimeout; if (opts.perMessageDeflate) { perMessageDeflate = new PerMessageDeflate( opts.perMessageDeflate !== true ? opts.perMessageDeflate : {}, false, opts.maxPayload ); opts.headers['Sec-WebSocket-Extensions'] = extension.format({ [PerMessageDeflate.extensionName]: perMessageDeflate.offer() }); } if (protocols) { opts.headers['Sec-WebSocket-Protocol'] = protocols; } if (opts.origin) { if (opts.protocolVersion < 13) { opts.headers['Sec-WebSocket-Origin'] = opts.origin; } else { opts.headers.Origin = opts.origin; } } if (parsedUrl.auth) { opts.auth = parsedUrl.auth; } else if (parsedUrl.username || parsedUrl.password) { opts.auth = `${parsedUrl.username}:${parsedUrl.password}`; } if (isUnixSocket) { const parts = path.split(':'); opts.socketPath = parts[0]; opts.path = parts[1]; } var req = (websocket._req = get(opts)); if (opts.timeout) { req.on('timeout', () => { abortHandshake(websocket, req, 'Opening handshake has timed out'); }); } req.on('error', (err) => { if (websocket._req.aborted) return; req = websocket._req = null; websocket.readyState = WebSocket.CLOSING; websocket.emit('error', err); websocket.emitClose(); }); req.on('response', (res) => { const location = res.headers.location; const statusCode = res.statusCode; if ( location && opts.followRedirects && statusCode >= 300 && statusCode < 400 ) { if (++websocket._redirects > opts.maxRedirects) { abortHandshake(websocket, req, 'Maximum redirects exceeded'); return; } req.abort(); const addr = url.URL ? new url.URL(location, address) : url.resolve(address, location); initAsClient(websocket, addr, protocols, options); } else if (!websocket.emit('unexpected-response', req, res)) { abortHandshake( websocket, req, `Unexpected server response: ${res.statusCode}` ); } }); req.on('upgrade', (res, socket, head) => { websocket.emit('upgrade', res); // // The user may have closed the connection from a listener of the `upgrade` // event. // if (websocket.readyState !== WebSocket.CONNECTING) return; req = websocket._req = null; const digest = crypto .createHash('sha1') .update(key + GUID) .digest('base64'); if (res.headers['sec-websocket-accept'] !== digest) { abortHandshake(websocket, socket, 'Invalid Sec-WebSocket-Accept header'); return; } const serverProt = res.headers['sec-websocket-protocol']; const protList = (protocols || '').split(/, */); var protError; if (!protocols && serverProt) { protError = 'Server sent a subprotocol but none was requested'; } else if (protocols && !serverProt) { protError = 'Server sent no subprotocol'; } else if (serverProt && !protList.includes(serverProt)) { protError = 'Server sent an invalid subprotocol'; } if (protError) { abortHandshake(websocket, socket, protError); return; } if (serverProt) websocket.protocol = serverProt; if (perMessageDeflate) { try { const extensions = extension.parse( res.headers['sec-websocket-extensions'] ); if (extensions[PerMessageDeflate.extensionName]) { perMessageDeflate.accept(extensions[PerMessageDeflate.extensionName]); websocket._extensions[ PerMessageDeflate.extensionName ] = perMessageDeflate; } } catch (err) { abortHandshake( websocket, socket, 'Invalid Sec-WebSocket-Extensions header' ); return; } } websocket.setSocket(socket, head, opts.maxPayload); }); } /** * Create a `net.Socket` and initiate a connection. * * @param {Object} options Connection options * @return {net.Socket} The newly created socket used to start the connection * @private */ function netConnect(options) { // // Override `options.path` only if `options` is a copy of the original options // object. This is always true on Node.js >= 8 but not on Node.js 6 where // `options.socketPath` might be `undefined` even if the `socketPath` option // was originally set. // if (options.protocolVersion) options.path = options.socketPath; return net.connect(options); } /** * Create a `tls.TLSSocket` and initiate a connection. * * @param {Object} options Connection options * @return {tls.TLSSocket} The newly created socket used to start the connection * @private */ function tlsConnect(options) { options.path = undefined; options.servername = options.servername || options.host; return tls.connect(options); } /** * Abort the handshake and emit an error. * * @param {WebSocket} websocket The WebSocket instance * @param {(http.ClientRequest|net.Socket)} stream The request to abort or the * socket to destroy * @param {String} message The error message * @private */ function abortHandshake(websocket, stream, message) { websocket.readyState = WebSocket.CLOSING; const err = new Error(message); Error.captureStackTrace(err, abortHandshake); if (stream.setHeader) { stream.abort(); stream.once('abort', websocket.emitClose.bind(websocket)); websocket.emit('error', err); } else { stream.destroy(err); stream.once('error', websocket.emit.bind(websocket, 'error')); stream.once('close', websocket.emitClose.bind(websocket)); } } /** * The listener of the `Receiver` `'conclude'` event. * * @param {Number} code The status code * @param {String} reason The reason for closing * @private */ function receiverOnConclude(code, reason) { const websocket = this[kWebSocket]; websocket._socket.removeListener('data', socketOnData); websocket._socket.resume(); websocket._closeFrameReceived = true; websocket._closeMessage = reason; websocket._closeCode = code; if (code === 1005) websocket.close(); else websocket.close(code, reason); } /** * The listener of the `Receiver` `'drain'` event. * * @private */ function receiverOnDrain() { this[kWebSocket]._socket.resume(); } /** * The listener of the `Receiver` `'error'` event. * * @param {(RangeError|Error)} err The emitted error * @private */ function receiverOnError(err) { const websocket = this[kWebSocket]; websocket._socket.removeListener('data', socketOnData); websocket.readyState = WebSocket.CLOSING; websocket._closeCode = err[kStatusCode]; websocket.emit('error', err); websocket._socket.destroy(); } /** * The listener of the `Receiver` `'finish'` event. * * @private */ function receiverOnFinish() { this[kWebSocket].emitClose(); } /** * The listener of the `Receiver` `'message'` event. * * @param {(String|Buffer|ArrayBuffer|Buffer[])} data The message * @private */ function receiverOnMessage(data) { this[kWebSocket].emit('message', data); } /** * The listener of the `Receiver` `'ping'` event. * * @param {Buffer} data The data included in the ping frame * @private */ function receiverOnPing(data) { const websocket = this[kWebSocket]; websocket.pong(data, !websocket._isServer, NOOP); websocket.emit('ping', data); } /** * The listener of the `Receiver` `'pong'` event. * * @param {Buffer} data The data included in the pong frame * @private */ function receiverOnPong(data) { this[kWebSocket].emit('pong', data); } /** * The listener of the `net.Socket` `'close'` event. * * @private */ function socketOnClose() { const websocket = this[kWebSocket]; this.removeListener('close', socketOnClose); this.removeListener('end', socketOnEnd); websocket.readyState = WebSocket.CLOSING; // // The close frame might not have been received or the `'end'` event emitted, // for example, if the socket was destroyed due to an error. Ensure that the // `receiver` stream is closed after writing any remaining buffered data to // it. If the readable side of the socket is in flowing mode then there is no // buffered data as everything has been already written and `readable.read()` // will return `null`. If instead, the socket is paused, any possible buffered // data will be read as a single chunk and emitted synchronously in a single // `'data'` event. // websocket._socket.read(); websocket._receiver.end(); this.removeListener('data', socketOnData); this[kWebSocket] = undefined; clearTimeout(websocket._closeTimer); if ( websocket._receiver._writableState.finished || websocket._receiver._writableState.errorEmitted ) { websocket.emitClose(); } else { websocket._receiver.on('error', receiverOnFinish); websocket._receiver.on('finish', receiverOnFinish); } } /** * The listener of the `net.Socket` `'data'` event. * * @param {Buffer} chunk A chunk of data * @private */ function socketOnData(chunk) { if (!this[kWebSocket]._receiver.write(chunk)) { this.pause(); } } /** * The listener of the `net.Socket` `'end'` event. * * @private */ function socketOnEnd() { const websocket = this[kWebSocket]; websocket.readyState = WebSocket.CLOSING; websocket._receiver.end(); this.end(); } /** * The listener of the `net.Socket` `'error'` event. * * @private */ function socketOnError() { const websocket = this[kWebSocket]; this.removeListener('error', socketOnError); this.on('error', NOOP); websocket.readyState = WebSocket.CLOSING; this.destroy(); } apollo-server-demo/node_modules/ws/lib/constants.js 0000644 0001750 0000144 00000000414 03560116604 022200 0 ustar andreh users 'use strict'; module.exports = { BINARY_TYPES: ['nodebuffer', 'arraybuffer', 'fragments'], GUID: '258EAFA5-E914-47DA-95CA-C5AB0DC85B11', kStatusCode: Symbol('status-code'), kWebSocket: Symbol('websocket'), EMPTY_BUFFER: Buffer.alloc(0), NOOP: () => {} }; apollo-server-demo/node_modules/ws/lib/sender.js 0000644 0001750 0000144 00000022411 03560116604 021445 0 ustar andreh users 'use strict'; const { randomBytes } = require('crypto'); const PerMessageDeflate = require('./permessage-deflate'); const { EMPTY_BUFFER } = require('./constants'); const { isValidStatusCode } = require('./validation'); const { mask: applyMask, toBuffer } = require('./buffer-util'); /** * HyBi Sender implementation. */ class Sender { /** * Creates a Sender instance. * * @param {net.Socket} socket The connection socket * @param {Object} extensions An object containing the negotiated extensions */ constructor(socket, extensions) { this._extensions = extensions || {}; this._socket = socket; this._firstFragment = true; this._compress = false; this._bufferedBytes = 0; this._deflating = false; this._queue = []; } /** * Frames a piece of data according to the HyBi WebSocket protocol. * * @param {Buffer} data The data to frame * @param {Object} options Options object * @param {Number} options.opcode The opcode * @param {Boolean} options.readOnly Specifies whether `data` can be modified * @param {Boolean} options.fin Specifies whether or not to set the FIN bit * @param {Boolean} options.mask Specifies whether or not to mask `data` * @param {Boolean} options.rsv1 Specifies whether or not to set the RSV1 bit * @return {Buffer[]} The framed data as a list of `Buffer` instances * @public */ static frame(data, options) { const merge = options.mask && options.readOnly; var offset = options.mask ? 6 : 2; var payloadLength = data.length; if (data.length >= 65536) { offset += 8; payloadLength = 127; } else if (data.length > 125) { offset += 2; payloadLength = 126; } const target = Buffer.allocUnsafe(merge ? data.length + offset : offset); target[0] = options.fin ? options.opcode | 0x80 : options.opcode; if (options.rsv1) target[0] |= 0x40; target[1] = payloadLength; if (payloadLength === 126) { target.writeUInt16BE(data.length, 2); } else if (payloadLength === 127) { target.writeUInt32BE(0, 2); target.writeUInt32BE(data.length, 6); } if (!options.mask) return [target, data]; const mask = randomBytes(4); target[1] |= 0x80; target[offset - 4] = mask[0]; target[offset - 3] = mask[1]; target[offset - 2] = mask[2]; target[offset - 1] = mask[3]; if (merge) { applyMask(data, mask, target, offset, data.length); return [target]; } applyMask(data, mask, data, 0, data.length); return [target, data]; } /** * Sends a close message to the other peer. * * @param {(Number|undefined)} code The status code component of the body * @param {String} data The message component of the body * @param {Boolean} mask Specifies whether or not to mask the message * @param {Function} cb Callback * @public */ close(code, data, mask, cb) { var buf; if (code === undefined) { buf = EMPTY_BUFFER; } else if (typeof code !== 'number' || !isValidStatusCode(code)) { throw new TypeError('First argument must be a valid error code number'); } else if (data === undefined || data === '') { buf = Buffer.allocUnsafe(2); buf.writeUInt16BE(code, 0); } else { buf = Buffer.allocUnsafe(2 + Buffer.byteLength(data)); buf.writeUInt16BE(code, 0); buf.write(data, 2); } if (this._deflating) { this.enqueue([this.doClose, buf, mask, cb]); } else { this.doClose(buf, mask, cb); } } /** * Frames and sends a close message. * * @param {Buffer} data The message to send * @param {Boolean} mask Specifies whether or not to mask `data` * @param {Function} cb Callback * @private */ doClose(data, mask, cb) { this.sendFrame( Sender.frame(data, { fin: true, rsv1: false, opcode: 0x08, mask, readOnly: false }), cb ); } /** * Sends a ping message to the other peer. * * @param {*} data The message to send * @param {Boolean} mask Specifies whether or not to mask `data` * @param {Function} cb Callback * @public */ ping(data, mask, cb) { const buf = toBuffer(data); if (this._deflating) { this.enqueue([this.doPing, buf, mask, toBuffer.readOnly, cb]); } else { this.doPing(buf, mask, toBuffer.readOnly, cb); } } /** * Frames and sends a ping message. * * @param {*} data The message to send * @param {Boolean} mask Specifies whether or not to mask `data` * @param {Boolean} readOnly Specifies whether `data` can be modified * @param {Function} cb Callback * @private */ doPing(data, mask, readOnly, cb) { this.sendFrame( Sender.frame(data, { fin: true, rsv1: false, opcode: 0x09, mask, readOnly }), cb ); } /** * Sends a pong message to the other peer. * * @param {*} data The message to send * @param {Boolean} mask Specifies whether or not to mask `data` * @param {Function} cb Callback * @public */ pong(data, mask, cb) { const buf = toBuffer(data); if (this._deflating) { this.enqueue([this.doPong, buf, mask, toBuffer.readOnly, cb]); } else { this.doPong(buf, mask, toBuffer.readOnly, cb); } } /** * Frames and sends a pong message. * * @param {*} data The message to send * @param {Boolean} mask Specifies whether or not to mask `data` * @param {Boolean} readOnly Specifies whether `data` can be modified * @param {Function} cb Callback * @private */ doPong(data, mask, readOnly, cb) { this.sendFrame( Sender.frame(data, { fin: true, rsv1: false, opcode: 0x0a, mask, readOnly }), cb ); } /** * Sends a data message to the other peer. * * @param {*} data The message to send * @param {Object} options Options object * @param {Boolean} options.compress Specifies whether or not to compress `data` * @param {Boolean} options.binary Specifies whether `data` is binary or text * @param {Boolean} options.fin Specifies whether the fragment is the last one * @param {Boolean} options.mask Specifies whether or not to mask `data` * @param {Function} cb Callback * @public */ send(data, options, cb) { const buf = toBuffer(data); const perMessageDeflate = this._extensions[PerMessageDeflate.extensionName]; var opcode = options.binary ? 2 : 1; var rsv1 = options.compress; if (this._firstFragment) { this._firstFragment = false; if (rsv1 && perMessageDeflate) { rsv1 = buf.length >= perMessageDeflate._threshold; } this._compress = rsv1; } else { rsv1 = false; opcode = 0; } if (options.fin) this._firstFragment = true; if (perMessageDeflate) { const opts = { fin: options.fin, rsv1, opcode, mask: options.mask, readOnly: toBuffer.readOnly }; if (this._deflating) { this.enqueue([this.dispatch, buf, this._compress, opts, cb]); } else { this.dispatch(buf, this._compress, opts, cb); } } else { this.sendFrame( Sender.frame(buf, { fin: options.fin, rsv1: false, opcode, mask: options.mask, readOnly: toBuffer.readOnly }), cb ); } } /** * Dispatches a data message. * * @param {Buffer} data The message to send * @param {Boolean} compress Specifies whether or not to compress `data` * @param {Object} options Options object * @param {Number} options.opcode The opcode * @param {Boolean} options.readOnly Specifies whether `data` can be modified * @param {Boolean} options.fin Specifies whether or not to set the FIN bit * @param {Boolean} options.mask Specifies whether or not to mask `data` * @param {Boolean} options.rsv1 Specifies whether or not to set the RSV1 bit * @param {Function} cb Callback * @private */ dispatch(data, compress, options, cb) { if (!compress) { this.sendFrame(Sender.frame(data, options), cb); return; } const perMessageDeflate = this._extensions[PerMessageDeflate.extensionName]; this._deflating = true; perMessageDeflate.compress(data, options.fin, (_, buf) => { this._deflating = false; options.readOnly = false; this.sendFrame(Sender.frame(buf, options), cb); this.dequeue(); }); } /** * Executes queued send operations. * * @private */ dequeue() { while (!this._deflating && this._queue.length) { const params = this._queue.shift(); this._bufferedBytes -= params[1].length; params[0].apply(this, params.slice(1)); } } /** * Enqueues a send operation. * * @param {Array} params Send operation parameters. * @private */ enqueue(params) { this._bufferedBytes += params[1].length; this._queue.push(params); } /** * Sends a frame. * * @param {Buffer[]} list The frame to send * @param {Function} cb Callback * @private */ sendFrame(list, cb) { if (list.length === 2) { this._socket.cork(); this._socket.write(list[0]); this._socket.write(list[1], cb); this._socket.uncork(); } else { this._socket.write(list[0], cb); } } } module.exports = Sender; apollo-server-demo/node_modules/fs-capacitor/ 0000755 0001750 0000144 00000000000 14067647701 021016 5 ustar andreh users apollo-server-demo/node_modules/fs-capacitor/readme.md 0000644 0001750 0000144 00000006021 13433541316 022563 0 ustar andreh users [](https://travis-ci.org/mike-marcacci/fs-capacitor) [](https://npm.im/fs-capacitor)  # FS Capacitor FS Capacitor is a filesystem buffer for finite node streams. It supports simultaneous read/write, and can be used to create multiple independent readable streams, each starting at the beginning of the buffer. This is useful for file uploads and other situations where you want to avoid delays to the source stream, but have slow downstream transformations to apply: ```js import fs from "fs"; import http from "http"; import WriteStream from "fs-capacitor"; http.createServer((req, res) => { const capacitor = new WriteStream(); const destination = fs.createReadStream("destination.txt"); // pipe data to the capacitor req.pipe(capacitor); // read data from the capacitor capacitor .createReadStream() .pipe(/* some slow Transform streams here */) .pipe(destination); // read data from the very beginning setTimeout(() => { capacitor.createReadStream().pipe(/* elsewhere */); // you can destroy a capacitor as soon as no more read streams are needed // without worrying if existing streams are fully consumed capacitor.destroy(); }, 100); }); ``` It is especially important to use cases like [`graphql-upload`](https://github.com/jaydenseric/graphql-upload) where server code may need to stash earler parts of a stream until later parts have been processed, and needs to attach multiple consumers at different times. FS Capacitor creates its temporary files in the directory ideneified by `os.tmpdir()` and attempts to remove them: - after `readStream.destroy()` has been called and all read streams are fully consumed or destroyed - before the process exits Please do note that FS Capacitor does NOT release disk space _as data is consumed_, and therefore is not suitable for use with infinite streams or those larger than the filesystem. ## API ### WriteStream `WriteStream` inherets all the methods of [`fs.WriteStream`](https://nodejs.org/api/fs.html#fs_class_fs_writestream) #### `new WriteStream()` Create a new `WriteStream` instance. #### `.createReadStream(): ReadStream` Create a new `ReadStream` instance attached to the `WriteStream` instance. Once a `WriteStream` is fully destroyed, calling `.createReadStream()` will throw a `ReadAfterDestroyedError` error. As soon as a `ReadStream` ends or is closed (such as by calling `readStream.destroy()`), it is detached from its `WriteStream`. #### `.destroy(error?: ?Error): void` - If `error` is present, `WriteStream`s still attached are destroyed with the same error. - If `error` is null or undefined, destruction of underlying resources is delayed until no `ReadStream`s are attached the `WriteStream` instance. ### ReadStream `ReadStream` inherets all the methods of [`fs.ReadStream`](https://nodejs.org/api/fs.html#fs_class_fs_readstream). apollo-server-demo/node_modules/fs-capacitor/src/ 0000755 0001750 0000144 00000000000 14067647701 021605 5 ustar andreh users apollo-server-demo/node_modules/fs-capacitor/src/index.mjs 0000644 0001750 0000144 00000013715 13463633543 023434 0 ustar andreh users import crypto from "crypto"; import fs from "fs"; import os from "os"; import path from "path"; export class ReadAfterDestroyedError extends Error {} export class ReadStream extends fs.ReadStream { constructor(writeStream, name) { super("", {}); this.name = name; this._writeStream = writeStream; // Persist terminating events. this.error = this._writeStream.error; this.addListener("error", error => { this.error = error; }); this.open(); } get ended() { return this._readableState.ended; } _read(n) { if (typeof this.fd !== "number") return this.once("open", function() { this._read(n); }); // The writer has finished, so the reader can continue uninterupted. if (this._writeStream.finished || this._writeStream.closed) return super._read(n); // Make sure there's something to read. const unread = this._writeStream.bytesWritten - this.bytesRead; if (unread === 0) { const retry = () => { this._writeStream.removeListener("finish", retry); this._writeStream.removeListener("write", retry); this._read(n); }; this._writeStream.addListener("finish", retry); this._writeStream.addListener("write", retry); return; } // Make sure we don't get ahead of our writer. return super._read(Math.min(n, unread)); } _destroy(error, callback) { if (typeof this.fd !== "number") { this.once("open", this._destroy.bind(this, error, callback)); return; } fs.close(this.fd, closeError => { callback(closeError || error); this.fd = null; this.closed = true; this.emit("close"); }); } open() { if (!this._writeStream) return; if (typeof this._writeStream.fd !== "number") { this._writeStream.once("open", () => this.open()); return; } this.path = this._writeStream.path; super.open(); } } export class WriteStream extends fs.WriteStream { constructor() { super("", { autoClose: false }); this._readStreams = new Set(); this.error = null; this._cleanupSync = () => { process.removeListener("exit", this._cleanupSync); process.removeListener("SIGINT", this._cleanupSync); if (typeof this.fd === "number") try { fs.closeSync(this.fd); } catch (error) { // An error here probably means the fd was already closed, but we can // still try to unlink the file. } try { fs.unlinkSync(this.path); } catch (error) { // If we are unable to unlink the file, the operating system will clean up // on next restart, since we use store thes in `os.tmpdir()` } }; } get finished() { return this._writableState.finished; } open() { // generage a random tmp path crypto.randomBytes(16, (error, buffer) => { if (error) { this.destroy(error); return; } this.path = path.join( os.tmpdir(), `capacitor-${buffer.toString("hex")}.tmp` ); // create the file fs.open(this.path, "wx", this.mode, (error, fd) => { if (error) { this.destroy(error); return; } // cleanup when our stream closes or when the process exits process.addListener("exit", this._cleanupSync); process.addListener("SIGINT", this._cleanupSync); this.fd = fd; this.emit("open", fd); this.emit("ready"); }); }); } _write(chunk, encoding, callback) { super._write(chunk, encoding, error => { if (!error) this.emit("write"); callback(error); }); } _destroy(error, callback) { if (typeof this.fd !== "number") { this.once("open", this._destroy.bind(this, error, callback)); return; } process.removeListener("exit", this._cleanupSync); process.removeListener("SIGINT", this._cleanupSync); const unlink = error => { fs.unlink(this.path, unlinkError => { // If we are unable to unlink the file, the operating system will // clean up on next restart, since we use store thes in `os.tmpdir()` callback(unlinkError || error); this.fd = null; this.closed = true; this.emit("close"); }); }; if (typeof this.fd === "number") { fs.close(this.fd, closeError => { // An error here probably means the fd was already closed, but we can // still try to unlink the file. unlink(closeError || error); }); return; } unlink(error); } destroy(error, callback) { if (error) this.error = error; // This is already destroyed. if (this.destroyed) return super.destroy(error, callback); // Call the callback once destroyed. if (typeof callback === "function") this.once("close", callback.bind(this, error)); // All read streams have terminated, so we can destroy this. if (this._readStreams.size === 0) { super.destroy(error, callback); return; } // Wait until all read streams have terminated before destroying this. this._destroyPending = true; // If there is an error, destroy all read streams with the error. if (error) for (let readStream of this._readStreams) readStream.destroy(error); } createReadStream(name) { if (this.destroyed) throw new ReadAfterDestroyedError( "A ReadStream cannot be created from a destroyed WriteStream." ); const readStream = new ReadStream(this, name); this._readStreams.add(readStream); const remove = () => { this._deleteReadStream(readStream); readStream.removeListener("end", remove); readStream.removeListener("close", remove); }; readStream.addListener("end", remove); readStream.addListener("close", remove); return readStream; } _deleteReadStream(readStream) { if (this._readStreams.delete(readStream) && this._destroyPending) this.destroy(); } } export default WriteStream; apollo-server-demo/node_modules/fs-capacitor/src/test.mjs 0000644 0001750 0000144 00000027723 13463756503 023312 0 ustar andreh users import "leaked-handles"; import fs from "fs"; import stream from "stream"; import t from "tap"; import WriteStream, { ReadAfterDestroyedError } from "."; const streamToString = stream => new Promise((resolve, reject) => { let ended = false; let data = ""; stream .on("error", reject) .on("data", chunk => { if (ended) throw new Error("`data` emitted after `end`"); data += chunk; }) .on("end", () => { ended = true; resolve(data); }); }); const waitForBytesWritten = (stream, bytes, resolve) => { if (stream.bytesWritten >= bytes) { setImmediate(resolve); return; } setImmediate(() => waitForBytesWritten(stream, bytes, resolve)); }; t.test("Data from a complete stream.", async t => { let data = ""; const source = new stream.Readable({ read() {} }); // Add the first chunk of data (without any consumer) const chunk1 = "1".repeat(10); source.push(chunk1); source.push(null); data += chunk1; // Create a new capacitor let capacitor1 = new WriteStream(); t.strictSame( capacitor1._readStreams.size, 0, "should start with 0 read streams" ); // Pipe data to the capacitor source.pipe(capacitor1); // Attach a read stream const capacitor1Stream1 = capacitor1.createReadStream("capacitor1Stream1"); t.strictSame( capacitor1._readStreams.size, 1, "should attach a new read stream before receiving data" ); // Wait until capacitor is finished writing all data const result = await streamToString(capacitor1Stream1); t.sameStrict(result, data, "should stream all data"); t.sameStrict( capacitor1._readStreams.size, 0, "should no longer have any attacheds read streams" ); }); t.test("Data from an open stream, 1 chunk, no read streams.", async t => { let data = ""; const source = new stream.Readable({ read() {} }); // Create a new capacitor let capacitor1 = new WriteStream(); t.strictSame( capacitor1._readStreams.size, 0, "should start with 0 read streams" ); // Pipe data to the capacitor source.pipe(capacitor1); // Add the first chunk of data (without any read streams) const chunk1 = "1".repeat(10); source.push(chunk1); source.push(null); data += chunk1; // Attach a read stream const capacitor1Stream1 = capacitor1.createReadStream("capacitor1Stream1"); t.strictSame( capacitor1._readStreams.size, 1, "should attach a new read stream before receiving data" ); // Wait until capacitor is finished writing all data const result = await streamToString(capacitor1Stream1); t.sameStrict(result, data, "should stream all data"); t.sameStrict( capacitor1._readStreams.size, 0, "should no longer have any attacheds read streams" ); }); t.test("Data from an open stream, 1 chunk, 1 read stream.", async t => { let data = ""; const source = new stream.Readable({ read() {} }); // Create a new capacitor let capacitor1 = new WriteStream(); t.strictSame( capacitor1._readStreams.size, 0, "should start with 0 read streams" ); // Pipe data to the capacitor source.pipe(capacitor1); // Attach a read stream const capacitor1Stream1 = capacitor1.createReadStream("capacitor1Stream1"); t.strictSame( capacitor1._readStreams.size, 1, "should attach a new read stream before receiving data" ); // Add the first chunk of data (with 1 read stream) const chunk1 = "1".repeat(10); source.push(chunk1); source.push(null); data += chunk1; // Wait until capacitor is finished writing all data const result = await streamToString(capacitor1Stream1); t.sameStrict(result, data, "should stream all data"); t.sameStrict( capacitor1._readStreams.size, 0, "should no longer have any attacheds read streams" ); }); const withChunkSize = size => t.test(`--- with chunk size: ${size}`, async t => { let data = ""; const source = new stream.Readable({ read() {} }); // Create a new capacitor and read stream before any data has been written let capacitor1; let capacitor1Stream1; await t.test( "can add a read stream before any data has been written", async t => { capacitor1 = new WriteStream(); t.strictSame( capacitor1._readStreams.size, 0, "should start with 0 read streams" ); capacitor1Stream1 = capacitor1.createReadStream("capacitor1Stream1"); t.strictSame( capacitor1._readStreams.size, 1, "should attach a new read stream before receiving data" ); await t.test("creates a temporary file", async t => { t.plan(3); await new Promise(resolve => capacitor1.on("open", resolve)); t.type( capacitor1.path, "string", "capacitor1.path should be a string" ); t.type(capacitor1.fd, "number", "capacitor1.fd should be a number"); t.ok(fs.existsSync(capacitor1.path), "creates a temp file"); }); } ); // Pipe data to the capacitor source.pipe(capacitor1); // Add the first chunk of data (without any read streams) const chunk1 = "1".repeat(size); source.push(chunk1); data += chunk1; // Wait until this chunk has been written to the buffer await new Promise(resolve => waitForBytesWritten(capacitor1, size, resolve) ); // Create a new stream after some data has been written let capacitor1Stream2; t.test("can add a read stream after data has been written", t => { capacitor1Stream2 = capacitor1.createReadStream("capacitor1Stream2"); t.strictSame( capacitor1._readStreams.size, 2, "should attach a new read stream after first write" ); t.end(); }); const writeEventBytesWritten = new Promise(resolve => { capacitor1.once("write", () => { resolve(capacitor1.bytesWritten); }); }); // Add a second chunk of data const chunk2 = "2".repeat(size); source.push(chunk2); data += chunk2; // Wait until this chunk has been written to the buffer await new Promise(resolve => waitForBytesWritten(capacitor1, 2 * size, resolve) ); // Make sure write event is called after bytes are written to the filesystem await t.test("write event emitted after bytes are written", async t => { t.strictSame( await writeEventBytesWritten, 2 * size, "bytesWritten should include new chunk" ); }); // End the source & wait until capacitor is finished const finished = new Promise(resolve => capacitor1.once("finish", resolve)); source.push(null); await finished; // Create a new stream after the source has ended let capacitor1Stream3; let capacitor1Stream4; t.test("can create a read stream after the source has ended", t => { capacitor1Stream3 = capacitor1.createReadStream("capacitor1Stream3"); capacitor1Stream4 = capacitor1.createReadStream("capacitor1Stream4"); t.strictSame( capacitor1._readStreams.size, 4, "should attach new read streams after end" ); t.end(); }); // Consume capacitor1Stream2, capacitor1Stream4 await t.test("streams complete data to a read stream", async t => { const result2 = await streamToString(capacitor1Stream2); t.strictSame( capacitor1Stream2.ended, true, "should mark read stream as ended" ); t.strictSame(result2, data, "should stream complete data"); const result4 = await streamToString(capacitor1Stream4); t.strictSame( capacitor1Stream4.ended, true, "should mark read stream as ended" ); t.strictSame(result4, data, "should stream complete data"); t.strictSame( capacitor1._readStreams.size, 2, "should detach an ended read stream" ); }); // Destroy capacitor1Stream1 await t.test("can destroy a read stream", async t => { await new Promise(resolve => { capacitor1Stream1.once("error", resolve); capacitor1Stream1.destroy(new Error("test")); }); t.strictSame( capacitor1Stream1.destroyed, true, "should mark read stream as destroyed" ); t.type( capacitor1Stream1.error, Error, "should store an error on read stream" ); t.strictSame( capacitor1._readStreams.size, 1, "should detach a destroyed read stream" ); }); // Destroy the capacitor (without an error) t.test("can delay destruction of a capacitor", t => { capacitor1.destroy(null); t.strictSame( capacitor1.destroyed, false, "should not destroy while read streams exist" ); t.strictSame( capacitor1._destroyPending, true, "should mark for future destruction" ); t.end(); }); // Destroy capacitor1Stream2 await t.test("destroys capacitor once no read streams exist", async t => { const readStreamDestroyed = new Promise(resolve => capacitor1Stream3.on("close", resolve) ); const capacitorDestroyed = new Promise(resolve => capacitor1.on("close", resolve) ); capacitor1Stream3.destroy(null); await readStreamDestroyed; t.strictSame( capacitor1Stream3.destroyed, true, "should mark read stream as destroyed" ); t.strictSame( capacitor1Stream3.error, null, "should not store an error on read stream" ); t.strictSame( capacitor1._readStreams.size, 0, "should detach a destroyed read stream" ); await capacitorDestroyed; t.strictSame(capacitor1.closed, true, "should mark capacitor as closed"); t.strictSame(capacitor1.fd, null, "should set fd to null"); t.strictSame( capacitor1.destroyed, true, "should mark capacitor as destroyed" ); t.notOk(fs.existsSync(capacitor1.path), "removes its temp file"); }); // Try to create a new read stream t.test("cannot create a read stream after destruction", t => { try { capacitor1.createReadStream(); } catch (error) { t.ok( error instanceof ReadAfterDestroyedError, "should not create a read stream once destroyed" ); t.end(); } }); const capacitor2 = new WriteStream(); const capacitor2Stream1 = capacitor2.createReadStream("capacitor2Stream1"); const capacitor2Stream2 = capacitor2.createReadStream("capacitor2Stream2"); const capacitor2ReadStream1Destroyed = new Promise(resolve => capacitor2Stream1.on("close", resolve) ); const capacitor2Destroyed = new Promise(resolve => capacitor2.on("close", resolve) ); capacitor2Stream1.destroy(); await capacitor2ReadStream1Destroyed; await t.test("propagates errors to attached read streams", async t => { capacitor2.destroy(); await new Promise(resolve => setImmediate(resolve)); t.strictSame( capacitor2Stream2.destroyed, false, "should not immediately mark attached read streams as destroyed" ); capacitor2.destroy(new Error("test")); await capacitor2Destroyed; t.type(capacitor2.error, Error, "should store an error on capacitor"); t.strictSame( capacitor2.destroyed, true, "should mark capacitor as destroyed" ); t.type( capacitor2Stream2.error, Error, "should store an error on attached read streams" ); t.strictSame( capacitor2Stream2.destroyed, true, "should mark attached read streams as destroyed" ); t.strictSame( capacitor2Stream1.error, null, "should not store an error on detached read streams" ); }); }); // Test with small (sub-highWaterMark, 16384) chunks withChunkSize(10); // Test with large (above-highWaterMark, 16384) chunks withChunkSize(100000); apollo-server-demo/node_modules/fs-capacitor/babel.config.js 0000644 0001750 0000144 00000000333 13463756503 023664 0 ustar andreh users module.exports = { comments: false, presets: [ [ "@babel/env", { modules: process.env.BABEL_ESM ? false : "commonjs", shippedProposals: true, loose: true } ] ] }; apollo-server-demo/node_modules/fs-capacitor/.prettierignore 0000644 0001750 0000144 00000000037 13463756503 024061 0 ustar andreh users package.json package-lock.json apollo-server-demo/node_modules/fs-capacitor/yarn-error.log 0000644 0001750 0000144 00000630165 13463667023 023632 0 ustar andreh users Arguments: /usr/local/Cellar/node/11.12.0/bin/node /usr/local/Cellar/yarn/1.15.2/libexec/bin/yarn.js add --dev @babel/plugin-transform-modules-commonjs, PATH: /Users/mike/Applications/google-cloud-sdk/bin:/Applications/Sublime Text.app/Contents/SharedSupport/bin:/usr/local/bin:/usr/local/sbin:/Users/mike/.cargo/bin:/usr/local/bin:/usr/local/sbin:/usr/bin:/bin:/usr/sbin:/sbin:/Users/mike/Code/go/bin:/usr/local/opt/go/libexec/bin Yarn version: 1.15.2 Node version: 11.12.0 Platform: darwin x64 Trace: Error: https://registry.yarnpkg.com/@babel%2fplugin-transform-modules-commonjs,: Not found at Request.params.callback [as _callback] (/usr/local/Cellar/yarn/1.15.2/libexec/lib/cli.js:66058:18) at Request.self.callback (/usr/local/Cellar/yarn/1.15.2/libexec/lib/cli.js:129541:22) at Request.emit (events.js:197:13) at Request.<anonymous> (/usr/local/Cellar/yarn/1.15.2/libexec/lib/cli.js:130513:10) at Request.emit (events.js:197:13) at IncomingMessage.<anonymous> (/usr/local/Cellar/yarn/1.15.2/libexec/lib/cli.js:130435:12) at Object.onceWrapper (events.js:285:20) at IncomingMessage.emit (events.js:202:15) at endReadableNT (_stream_readable.js:1132:12) at processTicksAndRejections (internal/process/next_tick.js:76:17) npm manifest: { "name": "fs-capacitor", "version": "2.0.3", "description": "Filesystem-buffered, passthrough stream that buffers indefinitely rather than propagate backpressure from downstream consumers.", "license": "MIT", "author": { "name": "Mike Marcacci", "email": "mike.marcacci@gmail.com" }, "repository": "github:mike-marcacci/fs-capacitor", "homepage": "https://github.com/mike-marcacci/fs-capacitor#readme", "bugs": "https://github.com/mike-marcacci/fs-capacitor/issues", "keywords": [ "stream", "buffer", "file", "split", "clone" ], "files": [ "lib", "!lib/test.*" ], "main": "lib/index.js", "module": "src/index.mjs", "engines": { "node": ">=8.5" }, "devDependencies": { "@babel/cli": "^7.1.2", "@babel/core": "^7.3.3", "@babel/plugin-transform-modules-commonjs": "^7.4.4", "babel-eslint": "^10.0.1", "eslint": "^5.14.1", "eslint-config-env": "^5.0.0", "eslint-config-prettier": "^4.0.0", "eslint-plugin-import": "^2.16.0", "eslint-plugin-import-order-alphabetical": "^0.0.2", "eslint-plugin-node": "^9.0.1", "eslint-plugin-prettier": "^3.0.0", "husky": "^2.2.0", "leaked-handles": "^5.2.0", "lint-staged": "^8.1.4", "prettier": "^1.16.4", "tap": "^13.1.2" }, "scripts": { "prepare": "rm -rf lib && babel src/index.mjs -d lib && prettier 'lib/**/*.js' --write", "test": "npm run test:eslint && npm run test:prettier && npm run test:tap", "test:eslint": "eslint . --ext mjs,js", "test:prettier": "prettier '**/*.{json,yml,md}' -l", "test:tap": "node --experimental-modules --no-warnings src/test | tap-mocha-reporter spec", "prepublishOnly": "npm run prepare && npm test" } } yarn manifest: No manifest Lockfile: # THIS IS AN AUTOGENERATED FILE. DO NOT EDIT THIS FILE DIRECTLY. # yarn lockfile v1 "@babel/cli@^7.1.2": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/cli/-/cli-7.4.4.tgz#5454bb7112f29026a4069d8e6f0e1794e651966c" integrity sha512-XGr5YjQSjgTa6OzQZY57FAJsdeVSAKR/u/KA5exWIz66IKtv/zXtHy+fIZcMry/EgYegwuHE7vzGnrFhjdIAsQ== dependencies: commander "^2.8.1" convert-source-map "^1.1.0" fs-readdir-recursive "^1.1.0" glob "^7.0.0" lodash "^4.17.11" mkdirp "^0.5.1" output-file-sync "^2.0.0" slash "^2.0.0" source-map "^0.5.0" optionalDependencies: chokidar "^2.0.4" "@babel/code-frame@^7.0.0": version "7.0.0" resolved "https://registry.yarnpkg.com/@babel/code-frame/-/code-frame-7.0.0.tgz#06e2ab19bdb535385559aabb5ba59729482800f8" integrity sha512-OfC2uemaknXr87bdLUkWog7nYuliM9Ij5HUcajsVcMCpQrcLmtxRbVFTIqmcSkSeYRBFBRxs2FiUqFJDLdiebA== dependencies: "@babel/highlight" "^7.0.0" "@babel/core@^7.3.3": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/core/-/core-7.4.4.tgz#84055750b05fcd50f9915a826b44fa347a825250" integrity sha512-lQgGX3FPRgbz2SKmhMtYgJvVzGZrmjaF4apZ2bLwofAKiSjxU0drPh4S/VasyYXwaTs+A1gvQ45BN8SQJzHsQQ== dependencies: "@babel/code-frame" "^7.0.0" "@babel/generator" "^7.4.4" "@babel/helpers" "^7.4.4" "@babel/parser" "^7.4.4" "@babel/template" "^7.4.4" "@babel/traverse" "^7.4.4" "@babel/types" "^7.4.4" convert-source-map "^1.1.0" debug "^4.1.0" json5 "^2.1.0" lodash "^4.17.11" resolve "^1.3.2" semver "^5.4.1" source-map "^0.5.0" "@babel/generator@^7.2.2": version "7.2.2" resolved "https://registry.yarnpkg.com/@babel/generator/-/generator-7.2.2.tgz#18c816c70962640eab42fe8cae5f3947a5c65ccc" integrity sha512-I4o675J/iS8k+P38dvJ3IBGqObLXyQLTxtrR4u9cSUJOURvafeEWb/pFMOTwtNrmq73mJzyF6ueTbO1BtN0Zeg== dependencies: "@babel/types" "^7.2.2" jsesc "^2.5.1" lodash "^4.17.10" source-map "^0.5.0" trim-right "^1.0.1" "@babel/generator@^7.4.0", "@babel/generator@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/generator/-/generator-7.4.4.tgz#174a215eb843fc392c7edcaabeaa873de6e8f041" integrity sha512-53UOLK6TVNqKxf7RUh8NE851EHRxOOeVXKbK2bivdb+iziMyk03Sr4eaE9OELCbyZAAafAKPDwF2TPUES5QbxQ== dependencies: "@babel/types" "^7.4.4" jsesc "^2.5.1" lodash "^4.17.11" source-map "^0.5.0" trim-right "^1.0.1" "@babel/helper-function-name@^7.1.0": version "7.1.0" resolved "https://registry.yarnpkg.com/@babel/helper-function-name/-/helper-function-name-7.1.0.tgz#a0ceb01685f73355d4360c1247f582bfafc8ff53" integrity sha512-A95XEoCpb3TO+KZzJ4S/5uW5fNe26DjBGqf1o9ucyLyCmi1dXq/B3c8iaWTfBk3VvetUxl16e8tIrd5teOCfGw== dependencies: "@babel/helper-get-function-arity" "^7.0.0" "@babel/template" "^7.1.0" "@babel/types" "^7.0.0" "@babel/helper-get-function-arity@^7.0.0": version "7.0.0" resolved "https://registry.yarnpkg.com/@babel/helper-get-function-arity/-/helper-get-function-arity-7.0.0.tgz#83572d4320e2a4657263734113c42868b64e49c3" integrity sha512-r2DbJeg4svYvt3HOS74U4eWKsUAMRH01Z1ds1zx8KNTPtpTL5JAsdFv8BNyOpVqdFhHkkRDIg5B4AsxmkjAlmQ== dependencies: "@babel/types" "^7.0.0" "@babel/helper-module-imports@^7.0.0": version "7.0.0" resolved "https://registry.yarnpkg.com/@babel/helper-module-imports/-/helper-module-imports-7.0.0.tgz#96081b7111e486da4d2cd971ad1a4fe216cc2e3d" integrity sha512-aP/hlLq01DWNEiDg4Jn23i+CXxW/owM4WpDLFUbpjxe4NS3BhLVZQ5i7E0ZrxuQ/vwekIeciyamgB1UIYxxM6A== dependencies: "@babel/types" "^7.0.0" "@babel/helper-module-transforms@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/helper-module-transforms/-/helper-module-transforms-7.4.4.tgz#96115ea42a2f139e619e98ed46df6019b94414b8" integrity sha512-3Z1yp8TVQf+B4ynN7WoHPKS8EkdTbgAEy0nU0rs/1Kw4pDgmvYH3rz3aI11KgxKCba2cn7N+tqzV1mY2HMN96w== dependencies: "@babel/helper-module-imports" "^7.0.0" "@babel/helper-simple-access" "^7.1.0" "@babel/helper-split-export-declaration" "^7.4.4" "@babel/template" "^7.4.4" "@babel/types" "^7.4.4" lodash "^4.17.11" "@babel/helper-plugin-utils@^7.0.0": version "7.0.0" resolved "https://registry.yarnpkg.com/@babel/helper-plugin-utils/-/helper-plugin-utils-7.0.0.tgz#bbb3fbee98661c569034237cc03967ba99b4f250" integrity sha512-CYAOUCARwExnEixLdB6sDm2dIJ/YgEAKDM1MOeMeZu9Ld/bDgVo8aiWrXwcY7OBh+1Ea2uUcVRcxKk0GJvW7QA== "@babel/helper-simple-access@^7.1.0": version "7.1.0" resolved "https://registry.yarnpkg.com/@babel/helper-simple-access/-/helper-simple-access-7.1.0.tgz#65eeb954c8c245beaa4e859da6188f39d71e585c" integrity sha512-Vk+78hNjRbsiu49zAPALxTb+JUQCz1aolpd8osOF16BGnLtseD21nbHgLPGUwrXEurZgiCOUmvs3ExTu4F5x6w== dependencies: "@babel/template" "^7.1.0" "@babel/types" "^7.0.0" "@babel/helper-split-export-declaration@^7.0.0": version "7.0.0" resolved "https://registry.yarnpkg.com/@babel/helper-split-export-declaration/-/helper-split-export-declaration-7.0.0.tgz#3aae285c0311c2ab095d997b8c9a94cad547d813" integrity sha512-MXkOJqva62dfC0w85mEf/LucPPS/1+04nmmRMPEBUB++hiiThQ2zPtX/mEWQ3mtzCEjIJvPY8nuwxXtQeQwUag== dependencies: "@babel/types" "^7.0.0" "@babel/helper-split-export-declaration@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/helper-split-export-declaration/-/helper-split-export-declaration-7.4.4.tgz#ff94894a340be78f53f06af038b205c49d993677" integrity sha512-Ro/XkzLf3JFITkW6b+hNxzZ1n5OQ80NvIUdmHspih1XAhtN3vPTuUFT4eQnela+2MaZ5ulH+iyP513KJrxbN7Q== dependencies: "@babel/types" "^7.4.4" "@babel/helpers@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/helpers/-/helpers-7.4.4.tgz#868b0ef59c1dd4e78744562d5ce1b59c89f2f2a5" integrity sha512-igczbR/0SeuPR8RFfC7tGrbdTbFL3QTvH6D+Z6zNxnTe//GyqmtHmDkzrqDmyZ3eSwPqB/LhyKoU5DXsp+Vp2A== dependencies: "@babel/template" "^7.4.4" "@babel/traverse" "^7.4.4" "@babel/types" "^7.4.4" "@babel/highlight@^7.0.0": version "7.0.0" resolved "https://registry.yarnpkg.com/@babel/highlight/-/highlight-7.0.0.tgz#f710c38c8d458e6dd9a201afb637fcb781ce99e4" integrity sha512-UFMC4ZeFC48Tpvj7C8UgLvtkaUuovQX+5xNWrsIoMG8o2z+XFKjKaN9iVmS84dPwVN00W4wPmqvYoZF3EGAsfw== dependencies: chalk "^2.0.0" esutils "^2.0.2" js-tokens "^4.0.0" "@babel/parser@^7.0.0", "@babel/parser@^7.2.2", "@babel/parser@^7.2.3": version "7.2.3" resolved "https://registry.yarnpkg.com/@babel/parser/-/parser-7.2.3.tgz#32f5df65744b70888d17872ec106b02434ba1489" integrity sha512-0LyEcVlfCoFmci8mXx8A5oIkpkOgyo8dRHtxBnK9RRBwxO2+JZPNsqtVEZQ7mJFPxnXF9lfmU24mHOPI0qnlkA== "@babel/parser@^7.4.3", "@babel/parser@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/parser/-/parser-7.4.4.tgz#5977129431b8fe33471730d255ce8654ae1250b6" integrity sha512-5pCS4mOsL+ANsFZGdvNLybx4wtqAZJ0MJjMHxvzI3bvIsz6sQvzW8XX92EYIkiPtIvcfG3Aj+Ir5VNyjnZhP7w== "@babel/plugin-transform-modules-commonjs@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-modules-commonjs/-/plugin-transform-modules-commonjs-7.4.4.tgz#0bef4713d30f1d78c2e59b3d6db40e60192cac1e" integrity sha512-4sfBOJt58sEo9a2BQXnZq+Q3ZTSAUXyK3E30o36BOGnJ+tvJ6YSxF0PG6kERvbeISgProodWuI9UVG3/FMY6iw== dependencies: "@babel/helper-module-transforms" "^7.4.4" "@babel/helper-plugin-utils" "^7.0.0" "@babel/helper-simple-access" "^7.1.0" "@babel/runtime@^7.0.0", "@babel/runtime@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/runtime/-/runtime-7.4.4.tgz#dc2e34982eb236803aa27a07fea6857af1b9171d" integrity sha512-w0+uT71b6Yi7i5SE0co4NioIpSYS6lLiXvCzWzGSKvpK5vdQtCbICHMj+gbAKAOtxiV6HsVh/MBdaF9EQ6faSg== dependencies: regenerator-runtime "^0.13.2" "@babel/template@^7.1.0": version "7.2.2" resolved "https://registry.yarnpkg.com/@babel/template/-/template-7.2.2.tgz#005b3fdf0ed96e88041330379e0da9a708eb2907" integrity sha512-zRL0IMM02AUDwghf5LMSSDEz7sBCO2YnNmpg3uWTZj/v1rcG2BmQUvaGU8GhU8BvfMh1k2KIAYZ7Ji9KXPUg7g== dependencies: "@babel/code-frame" "^7.0.0" "@babel/parser" "^7.2.2" "@babel/types" "^7.2.2" "@babel/template@^7.4.0", "@babel/template@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/template/-/template-7.4.4.tgz#f4b88d1225689a08f5bc3a17483545be9e4ed237" integrity sha512-CiGzLN9KgAvgZsnivND7rkA+AeJ9JB0ciPOD4U59GKbQP2iQl+olF1l76kJOupqidozfZ32ghwBEJDhnk9MEcw== dependencies: "@babel/code-frame" "^7.0.0" "@babel/parser" "^7.4.4" "@babel/types" "^7.4.4" "@babel/traverse@^7.0.0": version "7.2.3" resolved "https://registry.yarnpkg.com/@babel/traverse/-/traverse-7.2.3.tgz#7ff50cefa9c7c0bd2d81231fdac122f3957748d8" integrity sha512-Z31oUD/fJvEWVR0lNZtfgvVt512ForCTNKYcJBGbPb1QZfve4WGH8Wsy7+Mev33/45fhP/hwQtvgusNdcCMgSw== dependencies: "@babel/code-frame" "^7.0.0" "@babel/generator" "^7.2.2" "@babel/helper-function-name" "^7.1.0" "@babel/helper-split-export-declaration" "^7.0.0" "@babel/parser" "^7.2.3" "@babel/types" "^7.2.2" debug "^4.1.0" globals "^11.1.0" lodash "^4.17.10" "@babel/traverse@^7.4.3", "@babel/traverse@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/traverse/-/traverse-7.4.4.tgz#0776f038f6d78361860b6823887d4f3937133fe8" integrity sha512-Gw6qqkw/e6AGzlyj9KnkabJX7VcubqPtkUQVAwkc0wUMldr3A/hezNB3Rc5eIvId95iSGkGIOe5hh1kMKf951A== dependencies: "@babel/code-frame" "^7.0.0" "@babel/generator" "^7.4.4" "@babel/helper-function-name" "^7.1.0" "@babel/helper-split-export-declaration" "^7.4.4" "@babel/parser" "^7.4.4" "@babel/types" "^7.4.4" debug "^4.1.0" globals "^11.1.0" lodash "^4.17.11" "@babel/types@^7.0.0", "@babel/types@^7.2.2": version "7.2.2" resolved "https://registry.yarnpkg.com/@babel/types/-/types-7.2.2.tgz#44e10fc24e33af524488b716cdaee5360ea8ed1e" integrity sha512-fKCuD6UFUMkR541eDWL+2ih/xFZBXPOg/7EQFeTluMDebfqR4jrpaCjLhkWlQS4hT6nRa2PMEgXKbRB5/H2fpg== dependencies: esutils "^2.0.2" lodash "^4.17.10" to-fast-properties "^2.0.0" "@babel/types@^7.4.0", "@babel/types@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/types/-/types-7.4.4.tgz#8db9e9a629bb7c29370009b4b779ed93fe57d5f0" integrity sha512-dOllgYdnEFOebhkKCjzSVFqw/PmmB8pH6RGOWkY4GsboQNd47b1fBThBSwlHAq9alF9vc1M3+6oqR47R50L0tQ== dependencies: esutils "^2.0.2" lodash "^4.17.11" to-fast-properties "^2.0.0" "@samverschueren/stream-to-observable@^0.3.0": version "0.3.0" resolved "https://registry.yarnpkg.com/@samverschueren/stream-to-observable/-/stream-to-observable-0.3.0.tgz#ecdf48d532c58ea477acfcab80348424f8d0662f" integrity sha512-MI4Xx6LHs4Webyvi6EbspgyAb4D2Q2VtnCQ1blOJcoLS6mVa8lNN2rkIy1CVxfTUpoyIbCTkXES1rLXztFD1lg== dependencies: any-observable "^0.3.0" "@types/normalize-package-data@^2.4.0": version "2.4.0" resolved "https://registry.yarnpkg.com/@types/normalize-package-data/-/normalize-package-data-2.4.0.tgz#e486d0d97396d79beedd0a6e33f4534ff6b4973e" integrity sha512-f5j5b/Gf71L+dbqxIpQ4Z2WlmI/mPJ0fOkGGmFgtb6sAu97EPczzbS3/tJKxmcYDj55OX6ssqwDAWOHIYDRDGA== "@types/prop-types@*": version "15.7.1" resolved "https://registry.yarnpkg.com/@types/prop-types/-/prop-types-15.7.1.tgz#f1a11e7babb0c3cad68100be381d1e064c68f1f6" integrity sha512-CFzn9idOEpHrgdw8JsoTkaDDyRWk1jrzIV8djzcgpq0y9tG4B4lFT+Nxh52DVpDXV+n4+NPNv7M1Dj5uMp6XFg== "@types/react@^16.8.12", "@types/react@^16.8.6": version "16.8.16" resolved "https://registry.yarnpkg.com/@types/react/-/react-16.8.16.tgz#2bf980b4fb29cceeb01b2c139b3e185e57d3e08e" integrity sha512-A0+6kS6zwPtvubOLiCJmZ8li5bm3wKIkoKV0h3RdMDOnCj9cYkUnj3bWbE03/lcICdQmwBmUfoFiHeNhbFiyHQ== dependencies: "@types/prop-types" "*" csstype "^2.2.0" abbrev@1: version "1.1.1" resolved "https://registry.yarnpkg.com/abbrev/-/abbrev-1.1.1.tgz#f8f2c887ad10bf67f634f005b6987fed3179aac8" integrity sha512-nne9/IiQ/hzIhY6pdDnbBtz7DjPTKrY00P/zvPSm5pOFkl6xuGrGnXn/VtTNNfNtAfZ9/1RtehkszU9qcTii0Q== acorn-jsx@^5.0.0: version "5.0.1" resolved "https://registry.yarnpkg.com/acorn-jsx/-/acorn-jsx-5.0.1.tgz#32a064fd925429216a09b141102bfdd185fae40e" integrity sha512-HJ7CfNHrfJLlNTzIEUTj43LNWGkqpRLxm3YjAlcD0ACydk9XynzYsCBHxut+iqt+1aBXkx9UP/w/ZqMr13XIzg== acorn@^6.0.7: version "6.1.0" resolved "https://registry.yarnpkg.com/acorn/-/acorn-6.1.0.tgz#b0a3be31752c97a0f7013c5f4903b71a05db6818" integrity sha512-MW/FjM+IvU9CgBzjO3UIPCE2pyEwUsoFl+VGdczOPEdxfGFjuKny/gN54mOuX7Qxmb9Rg9MCn2oKiSUeW+pjrw== ajv@^6.5.5: version "6.10.0" resolved "https://registry.yarnpkg.com/ajv/-/ajv-6.10.0.tgz#90d0d54439da587cd7e843bfb7045f50bd22bdf1" integrity sha512-nffhOpkymDECQyR0mnsUtoCE8RlX38G0rYP+wgLWFyZuUyuuojSSvi/+euOiQBIn63whYwYVIIH1TvE3tu4OEg== dependencies: fast-deep-equal "^2.0.1" fast-json-stable-stringify "^2.0.0" json-schema-traverse "^0.4.1" uri-js "^4.2.2" ajv@^6.9.1: version "6.9.1" resolved "https://registry.yarnpkg.com/ajv/-/ajv-6.9.1.tgz#a4d3683d74abc5670e75f0b16520f70a20ea8dc1" integrity sha512-XDN92U311aINL77ieWHmqCcNlwjoP5cHXDxIxbf2MaPYuCXOHS7gHH8jktxeK5omgd52XbSTX6a4Piwd1pQmzA== dependencies: fast-deep-equal "^2.0.1" fast-json-stable-stringify "^2.0.0" json-schema-traverse "^0.4.1" uri-js "^4.2.2" ansi-escapes@^3.0.0: version "3.1.0" resolved "https://registry.yarnpkg.com/ansi-escapes/-/ansi-escapes-3.1.0.tgz#f73207bb81207d75fd6c83f125af26eea378ca30" integrity sha512-UgAb8H9D41AQnu/PbWlCofQVcnV4Gs2bBJi9eZPxfU/hgglFh3SMDMENRIqdr7H6XFnXdoknctFByVsCOotTVw== ansi-escapes@^3.2.0: version "3.2.0" resolved "https://registry.yarnpkg.com/ansi-escapes/-/ansi-escapes-3.2.0.tgz#8780b98ff9dbf5638152d1f1fe5c1d7b4442976b" integrity sha512-cBhpre4ma+U0T1oM5fXg7Dy1Jw7zzwv7lt/GoCpr+hDQJoYnKVPLL4dCvSEFMmQurOQvSrwT7SL/DAlhBI97RQ== ansi-regex@^2.0.0: version "2.1.1" resolved "https://registry.yarnpkg.com/ansi-regex/-/ansi-regex-2.1.1.tgz#c3b33ab5ee360d86e0e628f0468ae7ef27d654df" integrity sha1-w7M6te42DYbg5ijwRorn7yfWVN8= ansi-regex@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/ansi-regex/-/ansi-regex-3.0.0.tgz#ed0317c322064f79466c02966bddb605ab37d998" integrity sha1-7QMXwyIGT3lGbAKWa922Bas32Zg= ansi-regex@^4.0.0: version "4.0.0" resolved "https://registry.yarnpkg.com/ansi-regex/-/ansi-regex-4.0.0.tgz#70de791edf021404c3fd615aa89118ae0432e5a9" integrity sha512-iB5Dda8t/UqpPI/IjsejXu5jOGDrzn41wJyljwPH65VCIbk6+1BzFIMJGFwTNrYXT1CrD+B4l19U7awiQ8rk7w== ansi-styles@^2.2.1: version "2.2.1" resolved "https://registry.yarnpkg.com/ansi-styles/-/ansi-styles-2.2.1.tgz#b432dd3358b634cf75e1e4664368240533c1ddbe" integrity sha1-tDLdM1i2NM914eRmQ2gkBTPB3b4= ansi-styles@^3.2.0, ansi-styles@^3.2.1: version "3.2.1" resolved "https://registry.yarnpkg.com/ansi-styles/-/ansi-styles-3.2.1.tgz#41fbb20243e50b12be0f04b8dedbf07520ce841d" integrity sha512-VT0ZI6kZRdTh8YyJw3SMbYm/u+NqfsAxEpWO0Pf9sq8/e94WxxOpPKx9FR1FlyCtOVDNOQ+8ntlqFxiRc+r5qA== dependencies: color-convert "^1.9.0" ansicolors@~0.3.2: version "0.3.2" resolved "https://registry.yarnpkg.com/ansicolors/-/ansicolors-0.3.2.tgz#665597de86a9ffe3aa9bfbe6cae5c6ea426b4979" integrity sha1-ZlWX3oap/+Oqm/vmyuXG6kJrSXk= any-observable@^0.3.0: version "0.3.0" resolved "https://registry.yarnpkg.com/any-observable/-/any-observable-0.3.0.tgz#af933475e5806a67d0d7df090dd5e8bef65d119b" integrity sha512-/FQM1EDkTsf63Ub2C6O7GuYFDsSXUwsaZDurV0np41ocwq0jthUAYCmhBX9f+KwlaCgIuWyr/4WlUQUBfKfZog== anymatch@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/anymatch/-/anymatch-2.0.0.tgz#bcb24b4f37934d9aa7ac17b4adaf89e7c76ef2eb" integrity sha512-5teOsQWABXHHBFP9y3skS5P3d/WfWXpv3FUpy+LorMrNYaT9pI4oLMQX7jzQ2KklNpGpWHzdCXTDT2Y3XGlZBw== dependencies: micromatch "^3.1.4" normalize-path "^2.1.1" app-root-path@^2.2.1: version "2.2.1" resolved "https://registry.yarnpkg.com/app-root-path/-/app-root-path-2.2.1.tgz#d0df4a682ee408273583d43f6f79e9892624bc9a" integrity sha512-91IFKeKk7FjfmezPKkwtaRvSpnUc4gDwPAjA1YZ9Gn0q0PPeW+vbeUsZuyDwjI7+QTHhcLen2v25fi/AmhvbJA== append-transform@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/append-transform/-/append-transform-1.0.0.tgz#046a52ae582a228bd72f58acfbe2967c678759ab" integrity sha512-P009oYkeHyU742iSZJzZZywj4QRJdnTWffaKuJQLablCZ1uz6/cW4yaRgcDaoQ+uwOxxnt0gRUcwfsNP2ri0gw== dependencies: default-require-extensions "^2.0.0" aproba@^1.0.3: version "1.2.0" resolved "https://registry.yarnpkg.com/aproba/-/aproba-1.2.0.tgz#6802e6264efd18c790a1b0d517f0f2627bf2c94a" integrity sha512-Y9J6ZjXtoYh8RnXVCMOU/ttDmk1aBjunq9vO0ta5x85WDQiQfUF9sIPBITdbiiIVcBo03Hi3jMxigBtsddlXRw== archy@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/archy/-/archy-1.0.0.tgz#f9c8c13757cc1dd7bc379ac77b2c62a5c2868c40" integrity sha1-+cjBN1fMHde8N5rHeyxipcKGjEA= are-we-there-yet@~1.1.2: version "1.1.5" resolved "https://registry.yarnpkg.com/are-we-there-yet/-/are-we-there-yet-1.1.5.tgz#4b35c2944f062a8bfcda66410760350fe9ddfc21" integrity sha512-5hYdAkZlcG8tOLujVDTgCT+uPX0VnpAH28gWsLfzpXYm7wP6mp5Q/gYyR7YQ0cKVJcXJnl3j2kpBan13PtQf6w== dependencies: delegates "^1.0.0" readable-stream "^2.0.6" arg@^4.1.0: version "4.1.0" resolved "https://registry.yarnpkg.com/arg/-/arg-4.1.0.tgz#583c518199419e0037abb74062c37f8519e575f0" integrity sha512-ZWc51jO3qegGkVh8Hwpv636EkbesNV5ZNQPCtRa+0qytRYPEs9IYT9qITY9buezqUH5uqyzlWLcufrzU2rffdg== argparse@^1.0.7: version "1.0.10" resolved "https://registry.yarnpkg.com/argparse/-/argparse-1.0.10.tgz#bcd6791ea5ae09725e17e5ad988134cd40b3d911" integrity sha512-o5Roy6tNG4SL/FOkCAN6RzjiakZS25RLYFrcMttJqbdd8BWrnA+fGz57iN5Pb06pvBGvl5gQ0B48dJlslXvoTg== dependencies: sprintf-js "~1.0.2" arr-diff@^4.0.0: version "4.0.0" resolved "https://registry.yarnpkg.com/arr-diff/-/arr-diff-4.0.0.tgz#d6461074febfec71e7e15235761a329a5dc7c520" integrity sha1-1kYQdP6/7HHn4VI1dhoyml3HxSA= arr-flatten@^1.1.0: version "1.1.0" resolved "https://registry.yarnpkg.com/arr-flatten/-/arr-flatten-1.1.0.tgz#36048bbff4e7b47e136644316c99669ea5ae91f1" integrity sha512-L3hKV5R/p5o81R7O02IGnwpDmkp6E982XhtbuwSe3O4qOtMMMtodicASA1Cny2U+aCXcNpml+m4dPsvsJ3jatg== arr-union@^3.1.0: version "3.1.0" resolved "https://registry.yarnpkg.com/arr-union/-/arr-union-3.1.0.tgz#e39b09aea9def866a8f206e288af63919bae39c4" integrity sha1-45sJrqne+Gao8gbiiK9jkZuuOcQ= array-includes@^3.0.3: version "3.0.3" resolved "https://registry.yarnpkg.com/array-includes/-/array-includes-3.0.3.tgz#184b48f62d92d7452bb31b323165c7f8bd02266d" integrity sha1-GEtI9i2S10UrsxsyMWXH+L0CJm0= dependencies: define-properties "^1.1.2" es-abstract "^1.7.0" array-union@^1.0.1: version "1.0.2" resolved "https://registry.yarnpkg.com/array-union/-/array-union-1.0.2.tgz#9a34410e4f4e3da23dea375be5be70f24778ec39" integrity sha1-mjRBDk9OPaI96jdb5b5w8kd47Dk= dependencies: array-uniq "^1.0.1" array-uniq@^1.0.1: version "1.0.3" resolved "https://registry.yarnpkg.com/array-uniq/-/array-uniq-1.0.3.tgz#af6ac877a25cc7f74e058894753858dfdb24fdb6" integrity sha1-r2rId6Jcx/dOBYiUdThY39sk/bY= array-unique@^0.3.2: version "0.3.2" resolved "https://registry.yarnpkg.com/array-unique/-/array-unique-0.3.2.tgz#a894b75d4bc4f6cd679ef3244a9fd8f46ae2d428" integrity sha1-qJS3XUvE9s1nnvMkSp/Y9Gri1Cg= arrify@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/arrify/-/arrify-1.0.1.tgz#898508da2226f380df904728456849c1501a4b0d" integrity sha1-iYUI2iIm84DfkEcoRWhJwVAaSw0= asn1@~0.2.3: version "0.2.4" resolved "https://registry.yarnpkg.com/asn1/-/asn1-0.2.4.tgz#8d2475dfab553bb33e77b54e59e880bb8ce23136" integrity sha512-jxwzQpLQjSmWXgwaCZE9Nz+glAG01yF1QnWgbhGwHI5A6FRIEY6IVqtHhIepHqI7/kyEyQEagBC5mBEFlIYvdg== dependencies: safer-buffer "~2.1.0" assert-plus@1.0.0, assert-plus@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/assert-plus/-/assert-plus-1.0.0.tgz#f12e0f3c5d77b0b1cdd9146942e4e96c1e4dd525" integrity sha1-8S4PPF13sLHN2RRpQuTpbB5N1SU= assign-symbols@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/assign-symbols/-/assign-symbols-1.0.0.tgz#59667f41fadd4f20ccbc2bb96b8d4f7f78ec0367" integrity sha1-WWZ/QfrdTyDMvCu5a41Pf3jsA2c= astral-regex@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/astral-regex/-/astral-regex-1.0.0.tgz#6c8c3fb827dd43ee3918f27b82782ab7658a6fd9" integrity sha512-+Ryf6g3BKoRc7jfp7ad8tM4TtMiaWvbF/1/sQcZPkkS7ag3D5nMBCe2UfOTONtAkaG0tO0ij3C5Lwmf1EiyjHg== async-each@^1.0.1: version "1.0.3" resolved "https://registry.yarnpkg.com/async-each/-/async-each-1.0.3.tgz#b727dbf87d7651602f06f4d4ac387f47d91b0cbf" integrity sha512-z/WhQ5FPySLdvREByI2vZiTWwCnF0moMJ1hK9YQwDTHKh6I7/uSckMetoRGb5UBZPC1z0jlw+n/XCgjeH7y1AQ== async-hook-domain@^1.1.0: version "1.1.0" resolved "https://registry.yarnpkg.com/async-hook-domain/-/async-hook-domain-1.1.0.tgz#76855e572425c2bd1f7171f989bd40d70f12f9d6" integrity sha512-NH7V97d1yCbIanu2oDLyPT2GFNct0esPeJyRfkk8J5hTztHVSQp4UiNfL2O42sCA9XZPU8OgHvzOmt9ewBhVqA== dependencies: source-map-support "^0.5.11" asynckit@^0.4.0: version "0.4.0" resolved "https://registry.yarnpkg.com/asynckit/-/asynckit-0.4.0.tgz#c79ed97f7f34cb8f2ba1bc9790bcc366474b4b79" integrity sha1-x57Zf380y48robyXkLzDZkdLS3k= atob@^2.1.1: version "2.1.2" resolved "https://registry.yarnpkg.com/atob/-/atob-2.1.2.tgz#6d9517eb9e030d2436666651e86bd9f6f13533c9" integrity sha512-Wm6ukoaOGJi/73p/cl2GvLjTI5JM1k/O14isD73YML8StrH/7/lRFgmg8nICZgD3bZZvjwCGxtMOD3wWNAu8cg== auto-bind@^2.0.0: version "2.1.0" resolved "https://registry.yarnpkg.com/auto-bind/-/auto-bind-2.1.0.tgz#254e12d53063d7cab90446ce021accfb3faa1464" integrity sha512-qZuFvkes1eh9lB2mg8/HG18C+5GIO51r+RrCSst/lh+i5B1CtVlkhTE488M805Nr3dKl0sM/pIFKSKUIlg3zUg== dependencies: "@types/react" "^16.8.12" aws-sign2@~0.7.0: version "0.7.0" resolved "https://registry.yarnpkg.com/aws-sign2/-/aws-sign2-0.7.0.tgz#b46e890934a9591f2d2f6f86d7e6a9f1b3fe76a8" integrity sha1-tG6JCTSpWR8tL2+G1+ap8bP+dqg= aws4@^1.8.0: version "1.8.0" resolved "https://registry.yarnpkg.com/aws4/-/aws4-1.8.0.tgz#f0e003d9ca9e7f59c7a508945d7b2ef9a04a542f" integrity sha512-ReZxvNHIOv88FlT7rxcXIIC0fPt4KZqZbOlivyWtXLt8ESx84zd3kMC6iK5jVeS2qt+g7ftS7ye4fi06X5rtRQ== babel-code-frame@^6.26.0: version "6.26.0" resolved "https://registry.yarnpkg.com/babel-code-frame/-/babel-code-frame-6.26.0.tgz#63fd43f7dc1e3bb7ce35947db8fe369a3f58c74b" integrity sha1-Y/1D99weO7fONZR9uP42mj9Yx0s= dependencies: chalk "^1.1.3" esutils "^2.0.2" js-tokens "^3.0.2" babel-core@^6.25.0, babel-core@^6.26.0: version "6.26.3" resolved "https://registry.yarnpkg.com/babel-core/-/babel-core-6.26.3.tgz#b2e2f09e342d0f0c88e2f02e067794125e75c207" integrity sha512-6jyFLuDmeidKmUEb3NM+/yawG0M2bDZ9Z1qbZP59cyHLz8kYGKYwpJP0UwUKKUiTRNvxfLesJnTedqczP7cTDA== dependencies: babel-code-frame "^6.26.0" babel-generator "^6.26.0" babel-helpers "^6.24.1" babel-messages "^6.23.0" babel-register "^6.26.0" babel-runtime "^6.26.0" babel-template "^6.26.0" babel-traverse "^6.26.0" babel-types "^6.26.0" babylon "^6.18.0" convert-source-map "^1.5.1" debug "^2.6.9" json5 "^0.5.1" lodash "^4.17.4" minimatch "^3.0.4" path-is-absolute "^1.0.1" private "^0.1.8" slash "^1.0.0" source-map "^0.5.7" babel-eslint@^10.0.1: version "10.0.1" resolved "https://registry.yarnpkg.com/babel-eslint/-/babel-eslint-10.0.1.tgz#919681dc099614cd7d31d45c8908695092a1faed" integrity sha512-z7OT1iNV+TjOwHNLLyJk+HN+YVWX+CLE6fPD2SymJZOZQBs+QIexFjhm4keGTm8MW9xr4EC9Q0PbaLB24V5GoQ== dependencies: "@babel/code-frame" "^7.0.0" "@babel/parser" "^7.0.0" "@babel/traverse" "^7.0.0" "@babel/types" "^7.0.0" eslint-scope "3.7.1" eslint-visitor-keys "^1.0.0" babel-generator@^6.26.0: version "6.26.1" resolved "https://registry.yarnpkg.com/babel-generator/-/babel-generator-6.26.1.tgz#1844408d3b8f0d35a404ea7ac180f087a601bd90" integrity sha512-HyfwY6ApZj7BYTcJURpM5tznulaBvyio7/0d4zFOeMPUmfxkCjHocCuoLa2SAGzBI8AREcH3eP3758F672DppA== dependencies: babel-messages "^6.23.0" babel-runtime "^6.26.0" babel-types "^6.26.0" detect-indent "^4.0.0" jsesc "^1.3.0" lodash "^4.17.4" source-map "^0.5.7" trim-right "^1.0.1" babel-helper-builder-react-jsx@^6.24.1: version "6.26.0" resolved "https://registry.yarnpkg.com/babel-helper-builder-react-jsx/-/babel-helper-builder-react-jsx-6.26.0.tgz#39ff8313b75c8b65dceff1f31d383e0ff2a408a0" integrity sha1-Of+DE7dci2Xc7/HzHTg+D/KkCKA= dependencies: babel-runtime "^6.26.0" babel-types "^6.26.0" esutils "^2.0.2" babel-helpers@^6.24.1: version "6.24.1" resolved "https://registry.yarnpkg.com/babel-helpers/-/babel-helpers-6.24.1.tgz#3471de9caec388e5c850e597e58a26ddf37602b2" integrity sha1-NHHenK7DiOXIUOWX5Yom3fN2ArI= dependencies: babel-runtime "^6.22.0" babel-template "^6.24.1" babel-messages@^6.23.0: version "6.23.0" resolved "https://registry.yarnpkg.com/babel-messages/-/babel-messages-6.23.0.tgz#f3cdf4703858035b2a2951c6ec5edf6c62f2630e" integrity sha1-8830cDhYA1sqKVHG7F7fbGLyYw4= dependencies: babel-runtime "^6.22.0" babel-plugin-syntax-jsx@^6.8.0: version "6.18.0" resolved "https://registry.yarnpkg.com/babel-plugin-syntax-jsx/-/babel-plugin-syntax-jsx-6.18.0.tgz#0af32a9a6e13ca7a3fd5069e62d7b0f58d0d8946" integrity sha1-CvMqmm4Tyno/1QaeYtew9Y0NiUY= babel-plugin-syntax-object-rest-spread@^6.8.0: version "6.13.0" resolved "https://registry.yarnpkg.com/babel-plugin-syntax-object-rest-spread/-/babel-plugin-syntax-object-rest-spread-6.13.0.tgz#fd6536f2bce13836ffa3a5458c4903a597bb3bf5" integrity sha1-/WU28rzhODb/o6VFjEkDpZe7O/U= babel-plugin-transform-es2015-destructuring@^6.23.0: version "6.23.0" resolved "https://registry.yarnpkg.com/babel-plugin-transform-es2015-destructuring/-/babel-plugin-transform-es2015-destructuring-6.23.0.tgz#997bb1f1ab967f682d2b0876fe358d60e765c56d" integrity sha1-mXux8auWf2gtKwh2/jWNYOdlxW0= dependencies: babel-runtime "^6.22.0" babel-plugin-transform-object-rest-spread@^6.23.0: version "6.26.0" resolved "https://registry.yarnpkg.com/babel-plugin-transform-object-rest-spread/-/babel-plugin-transform-object-rest-spread-6.26.0.tgz#0f36692d50fef6b7e2d4b3ac1478137a963b7b06" integrity sha1-DzZpLVD+9rfi1LOsFHgTepY7ewY= dependencies: babel-plugin-syntax-object-rest-spread "^6.8.0" babel-runtime "^6.26.0" babel-plugin-transform-react-jsx@^6.24.1: version "6.24.1" resolved "https://registry.yarnpkg.com/babel-plugin-transform-react-jsx/-/babel-plugin-transform-react-jsx-6.24.1.tgz#840a028e7df460dfc3a2d29f0c0d91f6376e66a3" integrity sha1-hAoCjn30YN/DotKfDA2R9jduZqM= dependencies: babel-helper-builder-react-jsx "^6.24.1" babel-plugin-syntax-jsx "^6.8.0" babel-runtime "^6.22.0" babel-register@^6.26.0: version "6.26.0" resolved "https://registry.yarnpkg.com/babel-register/-/babel-register-6.26.0.tgz#6ed021173e2fcb486d7acb45c6009a856f647071" integrity sha1-btAhFz4vy0htestFxgCahW9kcHE= dependencies: babel-core "^6.26.0" babel-runtime "^6.26.0" core-js "^2.5.0" home-or-tmp "^2.0.0" lodash "^4.17.4" mkdirp "^0.5.1" source-map-support "^0.4.15" babel-runtime@^6.22.0, babel-runtime@^6.26.0: version "6.26.0" resolved "https://registry.yarnpkg.com/babel-runtime/-/babel-runtime-6.26.0.tgz#965c7058668e82b55d7bfe04ff2337bc8b5647fe" integrity sha1-llxwWGaOgrVde/4E/yM3vItWR/4= dependencies: core-js "^2.4.0" regenerator-runtime "^0.11.0" babel-template@^6.24.1, babel-template@^6.26.0: version "6.26.0" resolved "https://registry.yarnpkg.com/babel-template/-/babel-template-6.26.0.tgz#de03e2d16396b069f46dd9fff8521fb1a0e35e02" integrity sha1-3gPi0WOWsGn0bdn/+FIfsaDjXgI= dependencies: babel-runtime "^6.26.0" babel-traverse "^6.26.0" babel-types "^6.26.0" babylon "^6.18.0" lodash "^4.17.4" babel-traverse@^6.26.0: version "6.26.0" resolved "https://registry.yarnpkg.com/babel-traverse/-/babel-traverse-6.26.0.tgz#46a9cbd7edcc62c8e5c064e2d2d8d0f4035766ee" integrity sha1-RqnL1+3MYsjlwGTi0tjQ9ANXZu4= dependencies: babel-code-frame "^6.26.0" babel-messages "^6.23.0" babel-runtime "^6.26.0" babel-types "^6.26.0" babylon "^6.18.0" debug "^2.6.8" globals "^9.18.0" invariant "^2.2.2" lodash "^4.17.4" babel-types@^6.26.0: version "6.26.0" resolved "https://registry.yarnpkg.com/babel-types/-/babel-types-6.26.0.tgz#a3b073f94ab49eb6fa55cd65227a334380632497" integrity sha1-o7Bz+Uq0nrb6Vc1lInozQ4BjJJc= dependencies: babel-runtime "^6.26.0" esutils "^2.0.2" lodash "^4.17.4" to-fast-properties "^1.0.3" babylon@^6.18.0: version "6.18.0" resolved "https://registry.yarnpkg.com/babylon/-/babylon-6.18.0.tgz#af2f3b88fa6f5c1e4c634d1a0f8eac4f55b395e3" integrity sha512-q/UEjfGJ2Cm3oKV71DJz9d25TPnq5rhBVL2Q4fA5wcC3jcrdn7+SssEybFIxwAvvP+YCsCYNKughoF33GxgycQ== balanced-match@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/balanced-match/-/balanced-match-1.0.0.tgz#89b4d199ab2bee49de164ea02b89ce462d71b767" integrity sha1-ibTRmasr7kneFk6gK4nORi1xt2c= base@^0.11.1: version "0.11.2" resolved "https://registry.yarnpkg.com/base/-/base-0.11.2.tgz#7bde5ced145b6d551a90db87f83c558b4eb48a8f" integrity sha512-5T6P4xPgpp0YDFvSWwEZ4NoE3aM4QBQXDzmVbraCkFj8zHM+mba8SyqB5DbZWyR7mYHo6Y7BdQo3MoA4m0TeQg== dependencies: cache-base "^1.0.1" class-utils "^0.3.5" component-emitter "^1.2.1" define-property "^1.0.0" isobject "^3.0.1" mixin-deep "^1.2.0" pascalcase "^0.1.1" bcrypt-pbkdf@^1.0.0: version "1.0.2" resolved "https://registry.yarnpkg.com/bcrypt-pbkdf/-/bcrypt-pbkdf-1.0.2.tgz#a4301d389b6a43f9b67ff3ca11a3f6637e360e9e" integrity sha1-pDAdOJtqQ/m2f/PKEaP2Y342Dp4= dependencies: tweetnacl "^0.14.3" binary-extensions@^1.0.0: version "1.12.0" resolved "https://registry.yarnpkg.com/binary-extensions/-/binary-extensions-1.12.0.tgz#c2d780f53d45bba8317a8902d4ceeaf3a6385b14" integrity sha512-DYWGk01lDcxeS/K9IHPGWfT8PsJmbXRtRd2Sx72Tnb8pcYZQFF1oSDb8hJtS1vhp212q1Rzi5dUf9+nq0o9UIg== bind-obj-methods@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/bind-obj-methods/-/bind-obj-methods-2.0.0.tgz#0178140dbe7b7bb67dc74892ace59bc0247f06f0" integrity sha512-3/qRXczDi2Cdbz6jE+W3IflJOutRVica8frpBn14de1mBOkzDo+6tY33kNhvkw54Kn3PzRRD2VnGbGPcTAk4sw== brace-expansion@^1.1.7: version "1.1.11" resolved "https://registry.yarnpkg.com/brace-expansion/-/brace-expansion-1.1.11.tgz#3c7fcbf529d87226f3d2f52b966ff5271eb441dd" integrity sha512-iCuPHDFgrHX7H2vEI/5xpz07zSHB00TpugqhmYtVmMO6518mCuRMoOYFldEBl0g187ufozdaHgWKcYFb61qGiA== dependencies: balanced-match "^1.0.0" concat-map "0.0.1" braces@^2.3.1, braces@^2.3.2: version "2.3.2" resolved "https://registry.yarnpkg.com/braces/-/braces-2.3.2.tgz#5979fd3f14cd531565e5fa2df1abfff1dfaee729" integrity sha512-aNdbnj9P8PjdXU4ybaWLK2IF3jc/EoDYbC7AazW6to3TRsfXxscC9UXOB5iDiEQrkyIbWp2SLQda4+QAa7nc3w== dependencies: arr-flatten "^1.1.0" array-unique "^0.3.2" extend-shallow "^2.0.1" fill-range "^4.0.0" isobject "^3.0.1" repeat-element "^1.1.2" snapdragon "^0.8.1" snapdragon-node "^2.0.1" split-string "^3.0.2" to-regex "^3.0.1" browser-process-hrtime@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/browser-process-hrtime/-/browser-process-hrtime-1.0.0.tgz#3c9b4b7d782c8121e56f10106d84c0d0ffc94626" integrity sha512-9o5UecI3GhkpM6DrXr69PblIuWxPKk9Y0jHBRhdocZ2y7YECBFCsHm79Pr3OyR2AvjhDkabFJaDJMYRazHgsow== buffer-from@^1.0.0: version "1.1.1" resolved "https://registry.yarnpkg.com/buffer-from/-/buffer-from-1.1.1.tgz#32713bc028f75c02fdb710d7c7bcec1f2c6070ef" integrity sha512-MQcXEUbCKtEo7bhqEs6560Hyd4XaovZlO/k9V3hjVUF/zwW7KBVdSK4gIt/bzwS9MbR5qob+F5jusZsb0YQK2A== builtin-modules@^1.0.0: version "1.1.1" resolved "https://registry.yarnpkg.com/builtin-modules/-/builtin-modules-1.1.1.tgz#270f076c5a72c02f5b65a47df94c5fe3a278892f" integrity sha1-Jw8HbFpywC9bZaR9+Uxf46J4iS8= cache-base@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/cache-base/-/cache-base-1.0.1.tgz#0a7f46416831c8b662ee36fe4e7c59d76f666ab2" integrity sha512-AKcdTnFSWATd5/GCPRxr2ChwIJ85CeyrEyjRHlKxQ56d4XJMGym0uAiKn0xbLOGOl3+yRpOTi484dVCEc5AUzQ== dependencies: collection-visit "^1.0.0" component-emitter "^1.2.1" get-value "^2.0.6" has-value "^1.0.0" isobject "^3.0.1" set-value "^2.0.0" to-object-path "^0.3.0" union-value "^1.0.0" unset-value "^1.0.0" caching-transform@^3.0.2: version "3.0.2" resolved "https://registry.yarnpkg.com/caching-transform/-/caching-transform-3.0.2.tgz#601d46b91eca87687a281e71cef99791b0efca70" integrity sha512-Mtgcv3lh3U0zRii/6qVgQODdPA4G3zhG+jtbCWj39RXuUFTMzH0vcdMtaJS1jPowd+It2Pqr6y3NJMQqOqCE2w== dependencies: hasha "^3.0.0" make-dir "^2.0.0" package-hash "^3.0.0" write-file-atomic "^2.4.2" caller-callsite@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/caller-callsite/-/caller-callsite-2.0.0.tgz#847e0fce0a223750a9a027c54b33731ad3154134" integrity sha1-hH4PzgoiN1CpoCfFSzNzGtMVQTQ= dependencies: callsites "^2.0.0" caller-path@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/caller-path/-/caller-path-2.0.0.tgz#468f83044e369ab2010fac5f06ceee15bb2cb1f4" integrity sha1-Ro+DBE42mrIBD6xfBs7uFbsssfQ= dependencies: caller-callsite "^2.0.0" callsites@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/callsites/-/callsites-2.0.0.tgz#06eb84f00eea413da86affefacbffb36093b3c50" integrity sha1-BuuE8A7qQT2oav/vrL/7Ngk7PFA= callsites@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/callsites/-/callsites-3.0.0.tgz#fb7eb569b72ad7a45812f93fd9430a3e410b3dd3" integrity sha512-tWnkwu9YEq2uzlBDI4RcLn8jrFvF9AOi8PxDNU3hZZjJcjkcRAq3vCI+vZcg1SuxISDYe86k9VZFwAxDiJGoAw== camelcase@^5.0.0: version "5.3.1" resolved "https://registry.yarnpkg.com/camelcase/-/camelcase-5.3.1.tgz#e3c9b31569e106811df242f715725a1f4c494320" integrity sha512-L28STB170nwWS63UjtlEOE3dldQApaJXZkOI1uMFfzf3rRuPegHaHesyee+YxQ+W6SvRDQV6UrdOdRiR153wJg== capture-stack-trace@^1.0.0: version "1.0.1" resolved "https://registry.yarnpkg.com/capture-stack-trace/-/capture-stack-trace-1.0.1.tgz#a6c0bbe1f38f3aa0b92238ecb6ff42c344d4135d" integrity sha512-mYQLZnx5Qt1JgB1WEiMCf2647plpGeQ2NMR/5L0HNZzGQo4fuSPnK+wjfPnKZV0aiJDgzmWqqkV/g7JD+DW0qw== cardinal@^2.1.1: version "2.1.1" resolved "https://registry.yarnpkg.com/cardinal/-/cardinal-2.1.1.tgz#7cc1055d822d212954d07b085dea251cc7bc5505" integrity sha1-fMEFXYItISlU0HsIXeolHMe8VQU= dependencies: ansicolors "~0.3.2" redeyed "~2.1.0" caseless@~0.12.0: version "0.12.0" resolved "https://registry.yarnpkg.com/caseless/-/caseless-0.12.0.tgz#1b681c21ff84033c826543090689420d187151dc" integrity sha1-G2gcIf+EAzyCZUMJBolCDRhxUdw= chalk@^1.0.0, chalk@^1.1.3: version "1.1.3" resolved "https://registry.yarnpkg.com/chalk/-/chalk-1.1.3.tgz#a8115c55e4a702fe4d150abd3872822a7e09fc98" integrity sha1-qBFcVeSnAv5NFQq9OHKCKn4J/Jg= dependencies: ansi-styles "^2.2.1" escape-string-regexp "^1.0.2" has-ansi "^2.0.0" strip-ansi "^3.0.0" supports-color "^2.0.0" chalk@^2.0.0, chalk@^2.0.1, chalk@^2.1.0, chalk@^2.3.1, chalk@^2.4.1, chalk@^2.4.2: version "2.4.2" resolved "https://registry.yarnpkg.com/chalk/-/chalk-2.4.2.tgz#cd42541677a54333cf541a49108c1432b44c9424" integrity sha512-Mti+f9lpJNcwF4tWV8/OrTTtF1gZi+f8FqlyAdouralcFWFQWF2+NgCHShjkCb+IFBLq9buZwE1xckQU4peSuQ== dependencies: ansi-styles "^3.2.1" escape-string-regexp "^1.0.5" supports-color "^5.3.0" chardet@^0.7.0: version "0.7.0" resolved "https://registry.yarnpkg.com/chardet/-/chardet-0.7.0.tgz#90094849f0937f2eedc2425d0d28a9e5f0cbad9e" integrity sha512-mT8iDcrh03qDGRRmoA2hmBJnxpllMR+0/0qlzjqZES6NdiWDcZkCNAk4rPFZ9Q85r27unkiNNg8ZOiwZXBHwcA== chokidar@^2.0.4, chokidar@^2.1.5: version "2.1.5" resolved "https://registry.yarnpkg.com/chokidar/-/chokidar-2.1.5.tgz#0ae8434d962281a5f56c72869e79cb6d9d86ad4d" integrity sha512-i0TprVWp+Kj4WRPtInjexJ8Q+BqTE909VpH8xVhXrJkoc5QC8VO9TryGOqTr+2hljzc1sC62t22h5tZePodM/A== dependencies: anymatch "^2.0.0" async-each "^1.0.1" braces "^2.3.2" glob-parent "^3.1.0" inherits "^2.0.3" is-binary-path "^1.0.0" is-glob "^4.0.0" normalize-path "^3.0.0" path-is-absolute "^1.0.0" readdirp "^2.2.1" upath "^1.1.1" optionalDependencies: fsevents "^1.2.7" chownr@^1.1.1: version "1.1.1" resolved "https://registry.yarnpkg.com/chownr/-/chownr-1.1.1.tgz#54726b8b8fff4df053c42187e801fb4412df1494" integrity sha512-j38EvO5+LHX84jlo6h4UzmOwi0UgW61WRyPtJz4qaadK5eY3BTS5TY/S1Stc3Uk2lIM6TPevAlULiEJwie860g== ci-info@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/ci-info/-/ci-info-2.0.0.tgz#67a9e964be31a51e15e5010d58e6f12834002f46" integrity sha512-5tK7EtrZ0N+OLFMthtqOj4fI2Jeb88C4CAZPu25LDVUgXJ0A3Js4PMGqrn0JU1W0Mh1/Z8wZzYPxqUrXeBboCQ== class-utils@^0.3.5: version "0.3.6" resolved "https://registry.yarnpkg.com/class-utils/-/class-utils-0.3.6.tgz#f93369ae8b9a7ce02fd41faad0ca83033190c463" integrity sha512-qOhPa/Fj7s6TY8H8esGu5QNpMMQxz79h+urzrNYN6mn+9BnxlDGf5QZ+XeCDsxSjPqsSR56XOZOJmpeurnLMeg== dependencies: arr-union "^3.1.0" define-property "^0.2.5" isobject "^3.0.0" static-extend "^0.1.1" cli-cursor@^2.0.0, cli-cursor@^2.1.0: version "2.1.0" resolved "https://registry.yarnpkg.com/cli-cursor/-/cli-cursor-2.1.0.tgz#b35dac376479facc3e94747d41d0d0f5238ffcb5" integrity sha1-s12sN2R5+sw+lHR9QdDQ9SOP/LU= dependencies: restore-cursor "^2.0.0" cli-truncate@^0.2.1: version "0.2.1" resolved "https://registry.yarnpkg.com/cli-truncate/-/cli-truncate-0.2.1.tgz#9f15cfbb0705005369216c626ac7d05ab90dd574" integrity sha1-nxXPuwcFAFNpIWxiasfQWrkN1XQ= dependencies: slice-ansi "0.0.4" string-width "^1.0.1" cli-truncate@^1.1.0: version "1.1.0" resolved "https://registry.yarnpkg.com/cli-truncate/-/cli-truncate-1.1.0.tgz#2b2dfd83c53cfd3572b87fc4d430a808afb04086" integrity sha512-bAtZo0u82gCfaAGfSNxUdTI9mNyza7D8w4CVCcaOsy7sgwDzvx6ekr6cuWJqY3UGzgnQ1+4wgENup5eIhgxEYA== dependencies: slice-ansi "^1.0.0" string-width "^2.0.0" cli-width@^2.0.0: version "2.2.0" resolved "https://registry.yarnpkg.com/cli-width/-/cli-width-2.2.0.tgz#ff19ede8a9a5e579324147b0c11f0fbcbabed639" integrity sha1-/xnt6Kml5XkyQUewwR8PvLq+1jk= cliui@^4.0.0, cliui@^4.1.0: version "4.1.0" resolved "https://registry.yarnpkg.com/cliui/-/cliui-4.1.0.tgz#348422dbe82d800b3022eef4f6ac10bf2e4d1b49" integrity sha512-4FG+RSG9DL7uEwRUZXZn3SS34DiDPfzP0VOiEwtUWlE+AR2EIg+hSyvrIgUUfhdgR/UkAeW2QHgeP+hWrXs7jQ== dependencies: string-width "^2.1.1" strip-ansi "^4.0.0" wrap-ansi "^2.0.0" code-point-at@^1.0.0: version "1.1.0" resolved "https://registry.yarnpkg.com/code-point-at/-/code-point-at-1.1.0.tgz#0d070b4d043a5bea33a2f1a40e2edb3d9a4ccf77" integrity sha1-DQcLTQQ6W+ozovGkDi7bPZpMz3c= collection-visit@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/collection-visit/-/collection-visit-1.0.0.tgz#4bc0373c164bc3291b4d368c829cf1a80a59dca0" integrity sha1-S8A3PBZLwykbTTaMgpzxqApZ3KA= dependencies: map-visit "^1.0.0" object-visit "^1.0.0" color-convert@^1.9.0: version "1.9.3" resolved "https://registry.yarnpkg.com/color-convert/-/color-convert-1.9.3.tgz#bb71850690e1f136567de629d2d5471deda4c1e8" integrity sha512-QfAUtd+vFdAtFQcC8CCyYt1fYWxSqAiK2cSD6zDB8N3cpsEBAvRxp9zOGg6G/SHHJYAT88/az/IuDGALsNVbGg== dependencies: color-name "1.1.3" color-name@1.1.3: version "1.1.3" resolved "https://registry.yarnpkg.com/color-name/-/color-name-1.1.3.tgz#a7d0558bd89c42f795dd42328f740831ca53bc25" integrity sha1-p9BVi9icQveV3UIyj3QIMcpTvCU= color-support@^1.1.0: version "1.1.3" resolved "https://registry.yarnpkg.com/color-support/-/color-support-1.1.3.tgz#93834379a1cc9a0c61f82f52f0d04322251bd5a2" integrity sha512-qiBjkpbMLO/HL68y+lh4q0/O1MZFj2RX6X/KmMa3+gJD3z+WwI1ZzDHysvqHGS3mP6mznPckpXmw1nI9cJjyRg== combined-stream@^1.0.6, combined-stream@~1.0.6: version "1.0.7" resolved "https://registry.yarnpkg.com/combined-stream/-/combined-stream-1.0.7.tgz#2d1d24317afb8abe95d6d2c0b07b57813539d828" integrity sha512-brWl9y6vOB1xYPZcpZde3N9zDByXTosAeMDo4p1wzo6UMOX4vumB+TP1RZ76sfE6Md68Q0NJSrE/gbezd4Ul+w== dependencies: delayed-stream "~1.0.0" commander@^2.14.1, commander@^2.8.1, commander@^2.9.0: version "2.19.0" resolved "https://registry.yarnpkg.com/commander/-/commander-2.19.0.tgz#f6198aa84e5b83c46054b94ddedbfed5ee9ff12a" integrity sha512-6tvAOO+D6OENvRAh524Dh9jcfKTYDQAqvqezbCW82xj5X0pSrcpxtvRKHLG0yBY6SD7PSDrJaj+0AiOcKVd1Xg== commander@~2.20.0: version "2.20.0" resolved "https://registry.yarnpkg.com/commander/-/commander-2.20.0.tgz#d58bb2b5c1ee8f87b0d340027e9e94e222c5a422" integrity sha512-7j2y+40w61zy6YC2iRNpUe/NwhNyoXrYpHMrSunaMG64nRnaf96zO/KMQR4OyN/UnE5KLyEBnKHd4aG3rskjpQ== commondir@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/commondir/-/commondir-1.0.1.tgz#ddd800da0c66127393cca5950ea968a3aaf1253b" integrity sha1-3dgA2gxmEnOTzKWVDqloo6rxJTs= component-emitter@^1.2.1: version "1.2.1" resolved "https://registry.yarnpkg.com/component-emitter/-/component-emitter-1.2.1.tgz#137918d6d78283f7df7a6b7c5a63e140e69425e6" integrity sha1-E3kY1teCg/ffemt8WmPhQOaUJeY= concat-map@0.0.1: version "0.0.1" resolved "https://registry.yarnpkg.com/concat-map/-/concat-map-0.0.1.tgz#d8a96bd77fd68df7793a73036a3ba0d5405d477b" integrity sha1-2Klr13/Wjfd5OnMDajug1UBdR3s= console-control-strings@^1.0.0, console-control-strings@~1.1.0: version "1.1.0" resolved "https://registry.yarnpkg.com/console-control-strings/-/console-control-strings-1.1.0.tgz#3d7cf4464db6446ea644bf4b39507f9851008e8e" integrity sha1-PXz0Rk22RG6mRL9LOVB/mFEAjo4= contains-path@^0.1.0: version "0.1.0" resolved "https://registry.yarnpkg.com/contains-path/-/contains-path-0.1.0.tgz#fe8cf184ff6670b6baef01a9d4861a5cbec4120a" integrity sha1-/ozxhP9mcLa67wGp1IYaXL7EEgo= convert-source-map@^1.1.0, convert-source-map@^1.5.1, convert-source-map@^1.6.0: version "1.6.0" resolved "https://registry.yarnpkg.com/convert-source-map/-/convert-source-map-1.6.0.tgz#51b537a8c43e0f04dec1993bffcdd504e758ac20" integrity sha512-eFu7XigvxdZ1ETfbgPBohgyQ/Z++C0eEhTor0qRwBw9unw+L0/6V8wkSuGgzdThkiS5lSpdptOQPD8Ak40a+7A== dependencies: safe-buffer "~5.1.1" copy-descriptor@^0.1.0: version "0.1.1" resolved "https://registry.yarnpkg.com/copy-descriptor/-/copy-descriptor-0.1.1.tgz#676f6eb3c39997c2ee1ac3a924fd6124748f578d" integrity sha1-Z29us8OZl8LuGsOpJP1hJHSPV40= core-js@^2.4.0, core-js@^2.5.0: version "2.6.5" resolved "https://registry.yarnpkg.com/core-js/-/core-js-2.6.5.tgz#44bc8d249e7fb2ff5d00e0341a7ffb94fbf67895" integrity sha512-klh/kDpwX8hryYL14M9w/xei6vrv6sE8gTHDG7/T/+SEovB/G4ejwcfE/CBzO6Edsu+OETZMZ3wcX/EjUkrl5A== core-util-is@1.0.2, core-util-is@~1.0.0: version "1.0.2" resolved "https://registry.yarnpkg.com/core-util-is/-/core-util-is-1.0.2.tgz#b5fd54220aa2bc5ab57aab7140c940754503c1a7" integrity sha1-tf1UIgqivFq1eqtxQMlAdUUDwac= cosmiconfig@^5.0.2: version "5.1.0" resolved "https://registry.yarnpkg.com/cosmiconfig/-/cosmiconfig-5.1.0.tgz#6c5c35e97f37f985061cdf653f114784231185cf" integrity sha512-kCNPvthka8gvLtzAxQXvWo4FxqRB+ftRZyPZNuab5ngvM9Y7yw7hbEysglptLgpkGX9nAOKTBVkHUAe8xtYR6Q== dependencies: import-fresh "^2.0.0" is-directory "^0.3.1" js-yaml "^3.9.0" lodash.get "^4.4.2" parse-json "^4.0.0" cosmiconfig@^5.2.0: version "5.2.0" resolved "https://registry.yarnpkg.com/cosmiconfig/-/cosmiconfig-5.2.0.tgz#45038e4d28a7fe787203aede9c25bca4a08b12c8" integrity sha512-nxt+Nfc3JAqf4WIWd0jXLjTJZmsPLrA9DDc4nRw2KFJQJK7DNooqSXrNI7tzLG50CF8axczly5UV929tBmh/7g== dependencies: import-fresh "^2.0.0" is-directory "^0.3.1" js-yaml "^3.13.0" parse-json "^4.0.0" coveralls@^3.0.3: version "3.0.3" resolved "https://registry.yarnpkg.com/coveralls/-/coveralls-3.0.3.tgz#83b1c64aea1c6afa69beaf50b55ac1bc4d13e2b8" integrity sha512-viNfeGlda2zJr8Gj1zqXpDMRjw9uM54p7wzZdvLRyOgnAfCe974Dq4veZkjJdxQXbmdppu6flEajFYseHYaUhg== dependencies: growl "~> 1.10.0" js-yaml "^3.11.0" lcov-parse "^0.0.10" log-driver "^1.2.7" minimist "^1.2.0" request "^2.86.0" cp-file@^6.2.0: version "6.2.0" resolved "https://registry.yarnpkg.com/cp-file/-/cp-file-6.2.0.tgz#40d5ea4a1def2a9acdd07ba5c0b0246ef73dc10d" integrity sha512-fmvV4caBnofhPe8kOcitBwSn2f39QLjnAnGq3gO9dfd75mUytzKNZB1hde6QHunW2Rt+OwuBOMc3i1tNElbszA== dependencies: graceful-fs "^4.1.2" make-dir "^2.0.0" nested-error-stacks "^2.0.0" pify "^4.0.1" safe-buffer "^5.0.1" cross-spawn@^4: version "4.0.2" resolved "https://registry.yarnpkg.com/cross-spawn/-/cross-spawn-4.0.2.tgz#7b9247621c23adfdd3856004a823cbe397424d41" integrity sha1-e5JHYhwjrf3ThWAEqCPL45dCTUE= dependencies: lru-cache "^4.0.1" which "^1.2.9" cross-spawn@^6.0.0, cross-spawn@^6.0.5: version "6.0.5" resolved "https://registry.yarnpkg.com/cross-spawn/-/cross-spawn-6.0.5.tgz#4a5ec7c64dfae22c3a14124dbacdee846d80cbc4" integrity sha512-eTVLrBSt7fjbDygz805pMnstIs2VTBNkRm0qxZd+M7A5XDdxVRWO5MxGBXZhjY4cqLYLdtrGqRf8mBPmzwSpWQ== dependencies: nice-try "^1.0.4" path-key "^2.0.1" semver "^5.5.0" shebang-command "^1.2.0" which "^1.2.9" csstype@^2.2.0: version "2.6.4" resolved "https://registry.yarnpkg.com/csstype/-/csstype-2.6.4.tgz#d585a6062096e324e7187f80e04f92bd0f00e37f" integrity sha512-lAJUJP3M6HxFXbqtGRc0iZrdyeN+WzOWeY0q/VnFzI+kqVrYIzC7bWlKqCW7oCIdzoPkvfp82EVvrTlQ8zsWQg== dashdash@^1.12.0: version "1.14.1" resolved "https://registry.yarnpkg.com/dashdash/-/dashdash-1.14.1.tgz#853cfa0f7cbe2fed5de20326b8dd581035f6e2f0" integrity sha1-hTz6D3y+L+1d4gMmuN1YEDX24vA= dependencies: assert-plus "^1.0.0" date-fns@^1.27.2: version "1.30.1" resolved "https://registry.yarnpkg.com/date-fns/-/date-fns-1.30.1.tgz#2e71bf0b119153dbb4cc4e88d9ea5acfb50dc05c" integrity sha512-hBSVCvSmWC+QypYObzwGOd9wqdDpOt+0wl0KbU+R+uuZBS1jN8VsD1ss3irQDknRj5NvxiTF6oj/nDRnN/UQNw== debug@^2.1.2, debug@^2.1.3, debug@^2.2.0, debug@^2.3.3, debug@^2.6.8, debug@^2.6.9: version "2.6.9" resolved "https://registry.yarnpkg.com/debug/-/debug-2.6.9.tgz#5d128515df134ff327e90a4c93f4e077a536341f" integrity sha512-bC7ElrdJaJnPbAP+1EotYvqZsb3ecl5wi6Bfi6BJTUcNowp6cvspg0jXznRTKDjm/E7AdgFBVeAPVMNcKGsHMA== dependencies: ms "2.0.0" debug@^3.1.0: version "3.2.6" resolved "https://registry.yarnpkg.com/debug/-/debug-3.2.6.tgz#e83d17de16d8a7efb7717edbe5fb10135eee629b" integrity sha512-mel+jf7nrtEl5Pn1Qx46zARXKDpBbvzezse7p7LqINmdoIk8PYP5SySaxEmYv6TZ0JyEKA1hsCId6DIhgITtWQ== dependencies: ms "^2.1.1" debug@^4.0.1, debug@^4.1.0, debug@^4.1.1: version "4.1.1" resolved "https://registry.yarnpkg.com/debug/-/debug-4.1.1.tgz#3b72260255109c6b589cee050f1d516139664791" integrity sha512-pYAIzeRo8J6KPEaJ0VWOh5Pzkbw/RetuzehGM7QRRX5he4fPHx2rdKMB256ehJCkX+XRQm16eZLqLNS8RSZXZw== dependencies: ms "^2.1.1" decamelize@^1.2.0: version "1.2.0" resolved "https://registry.yarnpkg.com/decamelize/-/decamelize-1.2.0.tgz#f6534d15148269b20352e7bee26f501f9a191290" integrity sha1-9lNNFRSCabIDUue+4m9QH5oZEpA= decode-uri-component@^0.2.0: version "0.2.0" resolved "https://registry.yarnpkg.com/decode-uri-component/-/decode-uri-component-0.2.0.tgz#eb3913333458775cb84cd1a1fae062106bb87545" integrity sha1-6zkTMzRYd1y4TNGh+uBiEGu4dUU= dedent@^0.7.0: version "0.7.0" resolved "https://registry.yarnpkg.com/dedent/-/dedent-0.7.0.tgz#2495ddbaf6eb874abb0e1be9df22d2e5a544326c" integrity sha1-JJXduvbrh0q7Dhvp3yLS5aVEMmw= deep-extend@^0.6.0: version "0.6.0" resolved "https://registry.yarnpkg.com/deep-extend/-/deep-extend-0.6.0.tgz#c4fa7c95404a17a9c3e8ca7e1537312b736330ac" integrity sha512-LOHxIOaPYdHlJRtCQfDIVZtfw/ufM8+rVj649RIHzcm/vGwQRXFt6OPqIFWsm2XEMrNIEtWR64sY1LEKD2vAOA== deep-is@~0.1.3: version "0.1.3" resolved "https://registry.yarnpkg.com/deep-is/-/deep-is-0.1.3.tgz#b369d6fb5dbc13eecf524f91b070feedc357cf34" integrity sha1-s2nW+128E+7PUk+RsHD+7cNXzzQ= default-require-extensions@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/default-require-extensions/-/default-require-extensions-2.0.0.tgz#f5f8fbb18a7d6d50b21f641f649ebb522cfe24f7" integrity sha1-9fj7sYp9bVCyH2QfZJ67Uiz+JPc= dependencies: strip-bom "^3.0.0" define-properties@^1.1.2: version "1.1.3" resolved "https://registry.yarnpkg.com/define-properties/-/define-properties-1.1.3.tgz#cf88da6cbee26fe6db7094f61d870cbd84cee9f1" integrity sha512-3MqfYKj2lLzdMSf8ZIZE/V+Zuy+BgD6f164e8K2w7dgnpKArBDerGYpM46IYYcjnkdPNMjPk9A6VFB8+3SKlXQ== dependencies: object-keys "^1.0.12" define-property@^0.2.5: version "0.2.5" resolved "https://registry.yarnpkg.com/define-property/-/define-property-0.2.5.tgz#c35b1ef918ec3c990f9a5bc57be04aacec5c8116" integrity sha1-w1se+RjsPJkPmlvFe+BKrOxcgRY= dependencies: is-descriptor "^0.1.0" define-property@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/define-property/-/define-property-1.0.0.tgz#769ebaaf3f4a63aad3af9e8d304c9bbe79bfb0e6" integrity sha1-dp66rz9KY6rTr56NMEybvnm/sOY= dependencies: is-descriptor "^1.0.0" define-property@^2.0.2: version "2.0.2" resolved "https://registry.yarnpkg.com/define-property/-/define-property-2.0.2.tgz#d459689e8d654ba77e02a817f8710d702cb16e9d" integrity sha512-jwK2UV4cnPpbcG7+VRARKTZPUWowwXA8bzH5NP6ud0oeAxyYPuGZUAC7hMugpCdz4BeSZl2Dl9k66CHJ/46ZYQ== dependencies: is-descriptor "^1.0.2" isobject "^3.0.1" del@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/del/-/del-3.0.0.tgz#53ecf699ffcbcb39637691ab13baf160819766e5" integrity sha1-U+z2mf/LyzljdpGrE7rxYIGXZuU= dependencies: globby "^6.1.0" is-path-cwd "^1.0.0" is-path-in-cwd "^1.0.0" p-map "^1.1.1" pify "^3.0.0" rimraf "^2.2.8" delayed-stream@~1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/delayed-stream/-/delayed-stream-1.0.0.tgz#df3ae199acadfb7d440aaae0b29e2272b24ec619" integrity sha1-3zrhmayt+31ECqrgsp4icrJOxhk= delegates@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/delegates/-/delegates-1.0.0.tgz#84c6e159b81904fdca59a0ef44cd870d31250f9a" integrity sha1-hMbhWbgZBP3KWaDvRM2HDTElD5o= detect-indent@^4.0.0: version "4.0.0" resolved "https://registry.yarnpkg.com/detect-indent/-/detect-indent-4.0.0.tgz#f76d064352cdf43a1cb6ce619c4ee3a9475de208" integrity sha1-920GQ1LN9Docts5hnE7jqUdd4gg= dependencies: repeating "^2.0.0" detect-libc@^1.0.2: version "1.0.3" resolved "https://registry.yarnpkg.com/detect-libc/-/detect-libc-1.0.3.tgz#fa137c4bd698edf55cd5cd02ac559f91a4c4ba9b" integrity sha1-+hN8S9aY7fVc1c0CrFWfkaTEups= diff@^1.3.2: version "1.4.0" resolved "https://registry.yarnpkg.com/diff/-/diff-1.4.0.tgz#7f28d2eb9ee7b15a97efd89ce63dcfdaa3ccbabf" integrity sha1-fyjS657nsVqX79ic5j3P2qPMur8= diff@^3.1.0: version "3.5.0" resolved "https://registry.yarnpkg.com/diff/-/diff-3.5.0.tgz#800c0dd1e0a8bfbc95835c202ad220fe317e5a12" integrity sha512-A46qtFgd+g7pDZinpnwiRJtxbC1hpgf0uzP3iG89scHk0AUC7A1TGxf5OiiOUv/JMZR8GOt8hL900hV0bOy5xA== diff@^4.0.1: version "4.0.1" resolved "https://registry.yarnpkg.com/diff/-/diff-4.0.1.tgz#0c667cb467ebbb5cea7f14f135cc2dba7780a8ff" integrity sha512-s2+XdvhPCOF01LRQBC8hf4vhbVmI2CGS5aZnxLJlT5FtdhPCDFq80q++zK2KlrVorVDdL5BOGZ/VfLrVtYNF+Q== doctrine@1.5.0: version "1.5.0" resolved "https://registry.yarnpkg.com/doctrine/-/doctrine-1.5.0.tgz#379dce730f6166f76cefa4e6707a159b02c5a6fa" integrity sha1-N53Ocw9hZvds76TmcHoVmwLFpvo= dependencies: esutils "^2.0.2" isarray "^1.0.0" doctrine@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/doctrine/-/doctrine-3.0.0.tgz#addebead72a6574db783639dc87a121773973961" integrity sha512-yS+Q5i3hBf7GBkd4KG8a7eBNNWNGLTaEwwYWUijIYM7zrlYDM0BFXHjjPWlWZ1Rg7UaddZeIDmi9jF3HmqiQ2w== dependencies: esutils "^2.0.2" domain-browser@^1.2.0: version "1.2.0" resolved "https://registry.yarnpkg.com/domain-browser/-/domain-browser-1.2.0.tgz#3d31f50191a6749dd1375a7f522e823d42e54eda" integrity sha512-jnjyiM6eRyZl2H+W8Q/zLMA481hzi0eszAaBUzIVnmYVDBbnLxVNnfu1HgEBvCbL+71FrxMl3E6lpKH7Ge3OXA== ecc-jsbn@~0.1.1: version "0.1.2" resolved "https://registry.yarnpkg.com/ecc-jsbn/-/ecc-jsbn-0.1.2.tgz#3a83a904e54353287874c564b7549386849a98c9" integrity sha1-OoOpBOVDUyh4dMVkt1SThoSamMk= dependencies: jsbn "~0.1.0" safer-buffer "^2.1.0" elegant-spinner@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/elegant-spinner/-/elegant-spinner-1.0.1.tgz#db043521c95d7e303fd8f345bedc3349cfb0729e" integrity sha1-2wQ1IcldfjA/2PNFvtwzSc+wcp4= emoji-regex@^7.0.1: version "7.0.3" resolved "https://registry.yarnpkg.com/emoji-regex/-/emoji-regex-7.0.3.tgz#933a04052860c85e83c122479c4748a8e4c72156" integrity sha512-CwBLREIQ7LvYFB0WyRvwhq5N5qPhc6PMjD6bYggFlI5YyDgl+0vxq5VHbMOFqLg7hfWzmu8T5Z1QofhmTIhItA== end-of-stream@^1.1.0: version "1.4.1" resolved "https://registry.yarnpkg.com/end-of-stream/-/end-of-stream-1.4.1.tgz#ed29634d19baba463b6ce6b80a37213eab71ec43" integrity sha512-1MkrZNvWTKCaigbn+W15elq2BB/L22nqrSY5DKlo3X6+vclJm8Bb5djXJBmEX6fS3+zCh/F4VBK5Z2KxJt4s2Q== dependencies: once "^1.4.0" error-ex@^1.2.0, error-ex@^1.3.1: version "1.3.2" resolved "https://registry.yarnpkg.com/error-ex/-/error-ex-1.3.2.tgz#b4ac40648107fdcdcfae242f428bea8a14d4f1bf" integrity sha512-7dFHNmqeFSEt2ZBsCriorKnn3Z2pj+fd9kmI6QoWw4//DL+icEBfc0U7qJCisqrTsKTjw4fNFy2pW9OqStD84g== dependencies: is-arrayish "^0.2.1" es-abstract@^1.7.0: version "1.13.0" resolved "https://registry.yarnpkg.com/es-abstract/-/es-abstract-1.13.0.tgz#ac86145fdd5099d8dd49558ccba2eaf9b88e24e9" integrity sha512-vDZfg/ykNxQVwup/8E1BZhVzFfBxs9NqMzGcvIJrqg5k2/5Za2bWo40dK2J1pgLngZ7c+Shh8lwYtLGyrwPutg== dependencies: es-to-primitive "^1.2.0" function-bind "^1.1.1" has "^1.0.3" is-callable "^1.1.4" is-regex "^1.0.4" object-keys "^1.0.12" es-to-primitive@^1.2.0: version "1.2.0" resolved "https://registry.yarnpkg.com/es-to-primitive/-/es-to-primitive-1.2.0.tgz#edf72478033456e8dda8ef09e00ad9650707f377" integrity sha512-qZryBOJjV//LaxLTV6UC//WewneB3LcXOL9NP++ozKVXsIIIpm/2c13UDiD9Jp2eThsecw9m3jPqDwTyobcdbg== dependencies: is-callable "^1.1.4" is-date-object "^1.0.1" is-symbol "^1.0.2" es6-error@^4.0.1: version "4.1.1" resolved "https://registry.yarnpkg.com/es6-error/-/es6-error-4.1.1.tgz#9e3af407459deed47e9a91f9b885a84eb05c561d" integrity sha512-Um/+FxMr9CISWh0bi5Zv0iOD+4cFh5qLeks1qhAopKVAJw3drgKbKySikp7wGhDL0HPeaja0P5ULZrxLkniUVg== escape-string-regexp@^1.0.2, escape-string-regexp@^1.0.3, escape-string-regexp@^1.0.4, escape-string-regexp@^1.0.5: version "1.0.5" resolved "https://registry.yarnpkg.com/escape-string-regexp/-/escape-string-regexp-1.0.5.tgz#1b61c0562190a8dff6ae3bb2cf0200ca130b86d4" integrity sha1-G2HAViGQqN/2rjuyzwIAyhMLhtQ= eslint-config-env@^5.0.0: version "5.0.0" resolved "https://registry.yarnpkg.com/eslint-config-env/-/eslint-config-env-5.0.0.tgz#ee3c557e3cdb627c0dbcc7f898e473ff05182143" integrity sha512-oCFGqTFY77B/xAYulluZUiI5PbN/Mgeoyqb/jRdab0YM2g2UvOdHMHQYDZgq8hXH1kvSyZRwj+/7lO9Z7M0wKg== dependencies: app-root-path "^2.2.1" read-pkg-up "^5.0.0" semver "^6.0.0" eslint-config-prettier@^4.0.0: version "4.2.0" resolved "https://registry.yarnpkg.com/eslint-config-prettier/-/eslint-config-prettier-4.2.0.tgz#70b946b629cd0e3e98233fd9ecde4cb9778de96c" integrity sha512-y0uWc/FRfrHhpPZCYflWC8aE0KRJRY04rdZVfl8cL3sEZmOYyaBdhdlQPjKZBnuRMyLVK+JUZr7HaZFClQiH4w== dependencies: get-stdin "^6.0.0" eslint-import-resolver-node@^0.3.2: version "0.3.2" resolved "https://registry.yarnpkg.com/eslint-import-resolver-node/-/eslint-import-resolver-node-0.3.2.tgz#58f15fb839b8d0576ca980413476aab2472db66a" integrity sha512-sfmTqJfPSizWu4aymbPr4Iidp5yKm8yDkHp+Ir3YiTHiiDfxh69mOUsmiqW6RZ9zRXFaF64GtYmN7e+8GHBv6Q== dependencies: debug "^2.6.9" resolve "^1.5.0" eslint-module-utils@^2.2.0: version "2.2.0" resolved "https://registry.yarnpkg.com/eslint-module-utils/-/eslint-module-utils-2.2.0.tgz#b270362cd88b1a48ad308976ce7fa54e98411746" integrity sha1-snA2LNiLGkitMIl2zn+lTphBF0Y= dependencies: debug "^2.6.8" pkg-dir "^1.0.0" eslint-module-utils@^2.4.0: version "2.4.0" resolved "https://registry.yarnpkg.com/eslint-module-utils/-/eslint-module-utils-2.4.0.tgz#8b93499e9b00eab80ccb6614e69f03678e84e09a" integrity sha512-14tltLm38Eu3zS+mt0KvILC3q8jyIAH518MlG+HO0p+yK885Lb1UHTY/UgR91eOyGdmxAPb+OLoW4znqIT6Ndw== dependencies: debug "^2.6.8" pkg-dir "^2.0.0" eslint-plugin-es@^1.4.0: version "1.4.0" resolved "https://registry.yarnpkg.com/eslint-plugin-es/-/eslint-plugin-es-1.4.0.tgz#475f65bb20c993fc10e8c8fe77d1d60068072da6" integrity sha512-XfFmgFdIUDgvaRAlaXUkxrRg5JSADoRC8IkKLc/cISeR3yHVMefFHQZpcyXXEUUPHfy5DwviBcrfqlyqEwlQVw== dependencies: eslint-utils "^1.3.0" regexpp "^2.0.1" eslint-plugin-import-order-alphabetical@^0.0.2: version "0.0.2" resolved "https://registry.yarnpkg.com/eslint-plugin-import-order-alphabetical/-/eslint-plugin-import-order-alphabetical-0.0.2.tgz#301e1a7d7cef3790f417d4dceb4b62291ac5ddff" integrity sha512-9WBxC3RzQrJTAJ/epl64k4pvug/Le1OvhydICoQydK/2M4+36lnAlsn/3dsvXR+1WnRuc2mziNTkFUs+tx+22w== dependencies: eslint-module-utils "^2.2.0" lodash "^4.17.4" resolve "^1.6.0" eslint-plugin-import@^2.16.0: version "2.17.2" resolved "https://registry.yarnpkg.com/eslint-plugin-import/-/eslint-plugin-import-2.17.2.tgz#d227d5c6dc67eca71eb590d2bb62fb38d86e9fcb" integrity sha512-m+cSVxM7oLsIpmwNn2WXTJoReOF9f/CtLMo7qOVmKd1KntBy0hEcuNZ3erTmWjx+DxRO0Zcrm5KwAvI9wHcV5g== dependencies: array-includes "^3.0.3" contains-path "^0.1.0" debug "^2.6.9" doctrine "1.5.0" eslint-import-resolver-node "^0.3.2" eslint-module-utils "^2.4.0" has "^1.0.3" lodash "^4.17.11" minimatch "^3.0.4" read-pkg-up "^2.0.0" resolve "^1.10.0" eslint-plugin-node@^9.0.1: version "9.0.1" resolved "https://registry.yarnpkg.com/eslint-plugin-node/-/eslint-plugin-node-9.0.1.tgz#93e44626fa62bcb6efea528cee9687663dc03b62" integrity sha512-fljT5Uyy3lkJzuqhxrYanLSsvaILs9I7CmQ31atTtZ0DoIzRbbvInBh4cQ1CrthFHInHYBQxfPmPt6KLHXNXdw== dependencies: eslint-plugin-es "^1.4.0" eslint-utils "^1.3.1" ignore "^5.1.1" minimatch "^3.0.4" resolve "^1.10.1" semver "^6.0.0" eslint-plugin-prettier@^3.0.0: version "3.0.1" resolved "https://registry.yarnpkg.com/eslint-plugin-prettier/-/eslint-plugin-prettier-3.0.1.tgz#19d521e3981f69dd6d14f64aec8c6a6ac6eb0b0d" integrity sha512-/PMttrarPAY78PLvV3xfWibMOdMDl57hmlQ2XqFeA37wd+CJ7WSxV7txqjVPHi/AAFKd2lX0ZqfsOc/i5yFCSQ== dependencies: prettier-linter-helpers "^1.0.0" eslint-scope@3.7.1: version "3.7.1" resolved "https://registry.yarnpkg.com/eslint-scope/-/eslint-scope-3.7.1.tgz#3d63c3edfda02e06e01a452ad88caacc7cdcb6e8" integrity sha1-PWPD7f2gLgbgGkUq2IyqzHzctug= dependencies: esrecurse "^4.1.0" estraverse "^4.1.1" eslint-scope@^4.0.3: version "4.0.3" resolved "https://registry.yarnpkg.com/eslint-scope/-/eslint-scope-4.0.3.tgz#ca03833310f6889a3264781aa82e63eb9cfe7848" integrity sha512-p7VutNr1O/QrxysMo3E45FjYDTeXBy0iTltPFNSqKAIfjDSXC+4dj+qfyuD8bfAXrW/y6lW3O76VaYNPKfpKrg== dependencies: esrecurse "^4.1.0" estraverse "^4.1.1" eslint-utils@^1.3.0, eslint-utils@^1.3.1: version "1.3.1" resolved "https://registry.yarnpkg.com/eslint-utils/-/eslint-utils-1.3.1.tgz#9a851ba89ee7c460346f97cf8939c7298827e512" integrity sha512-Z7YjnIldX+2XMcjr7ZkgEsOj/bREONV60qYeB/bjMAqqqZ4zxKyWX+BOUkdmRmA9riiIPVvo5x86m5elviOk0Q== eslint-visitor-keys@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/eslint-visitor-keys/-/eslint-visitor-keys-1.0.0.tgz#3f3180fb2e291017716acb4c9d6d5b5c34a6a81d" integrity sha512-qzm/XxIbxm/FHyH341ZrbnMUpe+5Bocte9xkmFMzPMjRaZMcXww+MpBptFvtU+79L362nqiLhekCxCxDPaUMBQ== eslint@^5.14.1: version "5.16.0" resolved "https://registry.yarnpkg.com/eslint/-/eslint-5.16.0.tgz#a1e3ac1aae4a3fbd8296fcf8f7ab7314cbb6abea" integrity sha512-S3Rz11i7c8AA5JPv7xAH+dOyq/Cu/VXHiHXBPOU1k/JAM5dXqQPt3qcrhpHSorXmrpu2g0gkIBVXAqCpzfoZIg== dependencies: "@babel/code-frame" "^7.0.0" ajv "^6.9.1" chalk "^2.1.0" cross-spawn "^6.0.5" debug "^4.0.1" doctrine "^3.0.0" eslint-scope "^4.0.3" eslint-utils "^1.3.1" eslint-visitor-keys "^1.0.0" espree "^5.0.1" esquery "^1.0.1" esutils "^2.0.2" file-entry-cache "^5.0.1" functional-red-black-tree "^1.0.1" glob "^7.1.2" globals "^11.7.0" ignore "^4.0.6" import-fresh "^3.0.0" imurmurhash "^0.1.4" inquirer "^6.2.2" js-yaml "^3.13.0" json-stable-stringify-without-jsonify "^1.0.1" levn "^0.3.0" lodash "^4.17.11" minimatch "^3.0.4" mkdirp "^0.5.1" natural-compare "^1.4.0" optionator "^0.8.2" path-is-inside "^1.0.2" progress "^2.0.0" regexpp "^2.0.1" semver "^5.5.1" strip-ansi "^4.0.0" strip-json-comments "^2.0.1" table "^5.2.3" text-table "^0.2.0" esm@^3.2.22: version "3.2.22" resolved "https://registry.yarnpkg.com/esm/-/esm-3.2.22.tgz#5062c2e22fee3ccfee4e8f20da768330da90d6e3" integrity sha512-z8YG7U44L82j1XrdEJcqZOLUnjxco8pO453gKOlaMD1/md1n/5QrscAmYG+oKUspsmDLuBFZrpbxI6aQ67yRxA== espree@^5.0.1: version "5.0.1" resolved "https://registry.yarnpkg.com/espree/-/espree-5.0.1.tgz#5d6526fa4fc7f0788a5cf75b15f30323e2f81f7a" integrity sha512-qWAZcWh4XE/RwzLJejfcofscgMc9CamR6Tn1+XRXNzrvUSSbiAjGOI/fggztjIi7y9VLPqnICMIPiGyr8JaZ0A== dependencies: acorn "^6.0.7" acorn-jsx "^5.0.0" eslint-visitor-keys "^1.0.0" esprima@^4.0.0, esprima@~4.0.0: version "4.0.1" resolved "https://registry.yarnpkg.com/esprima/-/esprima-4.0.1.tgz#13b04cdb3e6c5d19df91ab6987a8695619b0aa71" integrity sha512-eGuFFw7Upda+g4p+QHvnW0RyTX/SVeJBDM/gCtMARO0cLuT2HcEKnTPvhjV6aGeqrCB/sbNop0Kszm0jsaWU4A== esquery@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/esquery/-/esquery-1.0.1.tgz#406c51658b1f5991a5f9b62b1dc25b00e3e5c708" integrity sha512-SmiyZ5zIWH9VM+SRUReLS5Q8a7GxtRdxEBVZpm98rJM7Sb+A9DVCndXfkeFUd3byderg+EbDkfnevfCwynWaNA== dependencies: estraverse "^4.0.0" esrecurse@^4.1.0: version "4.2.1" resolved "https://registry.yarnpkg.com/esrecurse/-/esrecurse-4.2.1.tgz#007a3b9fdbc2b3bb87e4879ea19c92fdbd3942cf" integrity sha512-64RBB++fIOAXPw3P9cy89qfMlvZEXZkqqJkjqqXIvzP5ezRZjW+lPWjw35UX/3EhUPFYbg5ER4JYgDw4007/DQ== dependencies: estraverse "^4.1.0" estraverse@^4.0.0, estraverse@^4.1.0, estraverse@^4.1.1: version "4.2.0" resolved "https://registry.yarnpkg.com/estraverse/-/estraverse-4.2.0.tgz#0dee3fed31fcd469618ce7342099fc1afa0bdb13" integrity sha1-De4/7TH81GlhjOc0IJn8GvoL2xM= esutils@^2.0.2: version "2.0.2" resolved "https://registry.yarnpkg.com/esutils/-/esutils-2.0.2.tgz#0abf4f1caa5bcb1f7a9d8acc6dea4faaa04bac9b" integrity sha1-Cr9PHKpbyx96nYrMbepPqqBLrJs= events-to-array@^1.0.1: version "1.1.2" resolved "https://registry.yarnpkg.com/events-to-array/-/events-to-array-1.1.2.tgz#2d41f563e1fe400ed4962fe1a4d5c6a7539df7f6" integrity sha1-LUH1Y+H+QA7Uli/hpNXGp1Od9/Y= execa@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/execa/-/execa-1.0.0.tgz#c6236a5bb4df6d6f15e88e7f017798216749ddd8" integrity sha512-adbxcyWV46qiHyvSp50TKt05tB4tK3HcmF7/nxfAdhnox83seTDbwnaqKO4sXRy7roHAIFqJP/Rw/AuEbX61LA== dependencies: cross-spawn "^6.0.0" get-stream "^4.0.0" is-stream "^1.1.0" npm-run-path "^2.0.0" p-finally "^1.0.0" signal-exit "^3.0.0" strip-eof "^1.0.0" expand-brackets@^2.1.4: version "2.1.4" resolved "https://registry.yarnpkg.com/expand-brackets/-/expand-brackets-2.1.4.tgz#b77735e315ce30f6b6eff0f83b04151a22449622" integrity sha1-t3c14xXOMPa27/D4OwQVGiJEliI= dependencies: debug "^2.3.3" define-property "^0.2.5" extend-shallow "^2.0.1" posix-character-classes "^0.1.0" regex-not "^1.0.0" snapdragon "^0.8.1" to-regex "^3.0.1" extend-shallow@^2.0.1: version "2.0.1" resolved "https://registry.yarnpkg.com/extend-shallow/-/extend-shallow-2.0.1.tgz#51af7d614ad9a9f610ea1bafbb989d6b1c56890f" integrity sha1-Ua99YUrZqfYQ6huvu5idaxxWiQ8= dependencies: is-extendable "^0.1.0" extend-shallow@^3.0.0, extend-shallow@^3.0.2: version "3.0.2" resolved "https://registry.yarnpkg.com/extend-shallow/-/extend-shallow-3.0.2.tgz#26a71aaf073b39fb2127172746131c2704028db8" integrity sha1-Jqcarwc7OfshJxcnRhMcJwQCjbg= dependencies: assign-symbols "^1.0.0" is-extendable "^1.0.1" extend@~3.0.2: version "3.0.2" resolved "https://registry.yarnpkg.com/extend/-/extend-3.0.2.tgz#f8b1136b4071fbd8eb140aff858b1019ec2915fa" integrity sha512-fjquC59cD7CyW6urNXK0FBufkZcoiGG80wTuPujX590cB5Ttln20E2UB4S/WARVqhXffZl2LNgS+gQdPIIim/g== external-editor@^3.0.3: version "3.0.3" resolved "https://registry.yarnpkg.com/external-editor/-/external-editor-3.0.3.tgz#5866db29a97826dbe4bf3afd24070ead9ea43a27" integrity sha512-bn71H9+qWoOQKyZDo25mOMVpSmXROAsTJVVVYzrrtol3d4y+AsKjf4Iwl2Q+IuT0kFSQ1qo166UuIwqYq7mGnA== dependencies: chardet "^0.7.0" iconv-lite "^0.4.24" tmp "^0.0.33" extglob@^2.0.4: version "2.0.4" resolved "https://registry.yarnpkg.com/extglob/-/extglob-2.0.4.tgz#ad00fe4dc612a9232e8718711dc5cb5ab0285543" integrity sha512-Nmb6QXkELsuBr24CJSkilo6UHHgbekK5UiZgfE6UHD3Eb27YC6oD+bhcT+tJ6cl8dmsgdQxnWlcry8ksBIBLpw== dependencies: array-unique "^0.3.2" define-property "^1.0.0" expand-brackets "^2.1.4" extend-shallow "^2.0.1" fragment-cache "^0.2.1" regex-not "^1.0.0" snapdragon "^0.8.1" to-regex "^3.0.1" extsprintf@1.3.0: version "1.3.0" resolved "https://registry.yarnpkg.com/extsprintf/-/extsprintf-1.3.0.tgz#96918440e3041a7a414f8c52e3c574eb3c3e1e05" integrity sha1-lpGEQOMEGnpBT4xS48V06zw+HgU= extsprintf@^1.2.0: version "1.4.0" resolved "https://registry.yarnpkg.com/extsprintf/-/extsprintf-1.4.0.tgz#e2689f8f356fad62cca65a3a91c5df5f9551692f" integrity sha1-4mifjzVvrWLMplo6kcXfX5VRaS8= fast-deep-equal@^2.0.1: version "2.0.1" resolved "https://registry.yarnpkg.com/fast-deep-equal/-/fast-deep-equal-2.0.1.tgz#7b05218ddf9667bf7f370bf7fdb2cb15fdd0aa49" integrity sha1-ewUhjd+WZ79/Nwv3/bLLFf3Qqkk= fast-diff@^1.1.2: version "1.2.0" resolved "https://registry.yarnpkg.com/fast-diff/-/fast-diff-1.2.0.tgz#73ee11982d86caaf7959828d519cfe927fac5f03" integrity sha512-xJuoT5+L99XlZ8twedaRf6Ax2TgQVxvgZOYoPKqZufmJib0tL2tegPBOZb1pVNgIhlqDlA0eO0c3wBvQcmzx4w== fast-json-stable-stringify@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/fast-json-stable-stringify/-/fast-json-stable-stringify-2.0.0.tgz#d5142c0caee6b1189f87d3a76111064f86c8bbf2" integrity sha1-1RQsDK7msRifh9OnYREGT4bIu/I= fast-levenshtein@~2.0.4: version "2.0.6" resolved "https://registry.yarnpkg.com/fast-levenshtein/-/fast-levenshtein-2.0.6.tgz#3d8a5c66883a16a30ca8643e851f19baa7797917" integrity sha1-PYpcZog6FqMMqGQ+hR8Zuqd5eRc= figures@^1.7.0: version "1.7.0" resolved "https://registry.yarnpkg.com/figures/-/figures-1.7.0.tgz#cbe1e3affcf1cd44b80cadfed28dc793a9701d2e" integrity sha1-y+Hjr/zxzUS4DK3+0o3Hk6lwHS4= dependencies: escape-string-regexp "^1.0.5" object-assign "^4.1.0" figures@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/figures/-/figures-2.0.0.tgz#3ab1a2d2a62c8bfb431a0c94cb797a2fce27c962" integrity sha1-OrGi0qYsi/tDGgyUy3l6L84nyWI= dependencies: escape-string-regexp "^1.0.5" file-entry-cache@^5.0.1: version "5.0.1" resolved "https://registry.yarnpkg.com/file-entry-cache/-/file-entry-cache-5.0.1.tgz#ca0f6efa6dd3d561333fb14515065c2fafdf439c" integrity sha512-bCg29ictuBaKUwwArK4ouCaqDgLZcysCFLmM/Yn/FDoqndh/9vNuQfXRDvTuXKLxfD/JtZQGKFT8MGcJBK644g== dependencies: flat-cache "^2.0.1" fill-range@^4.0.0: version "4.0.0" resolved "https://registry.yarnpkg.com/fill-range/-/fill-range-4.0.0.tgz#d544811d428f98eb06a63dc402d2403c328c38f7" integrity sha1-1USBHUKPmOsGpj3EAtJAPDKMOPc= dependencies: extend-shallow "^2.0.1" is-number "^3.0.0" repeat-string "^1.6.1" to-regex-range "^2.1.0" find-cache-dir@^2.1.0: version "2.1.0" resolved "https://registry.yarnpkg.com/find-cache-dir/-/find-cache-dir-2.1.0.tgz#8d0f94cd13fe43c6c7c261a0d86115ca918c05f7" integrity sha512-Tq6PixE0w/VMFfCgbONnkiQIVol/JJL7nRMi20fqzA4NRs9AfeqMGeRdPi3wIhYkxjeBaWh2rxwapn5Tu3IqOQ== dependencies: commondir "^1.0.1" make-dir "^2.0.0" pkg-dir "^3.0.0" find-parent-dir@^0.3.0: version "0.3.0" resolved "https://registry.yarnpkg.com/find-parent-dir/-/find-parent-dir-0.3.0.tgz#33c44b429ab2b2f0646299c5f9f718f376ff8d54" integrity sha1-M8RLQpqysvBkYpnF+fcY83b/jVQ= find-up@^1.0.0: version "1.1.2" resolved "https://registry.yarnpkg.com/find-up/-/find-up-1.1.2.tgz#6b2e9822b1a2ce0a60ab64d610eccad53cb24d0f" integrity sha1-ay6YIrGizgpgq2TWEOzK1TyyTQ8= dependencies: path-exists "^2.0.0" pinkie-promise "^2.0.0" find-up@^2.0.0, find-up@^2.1.0: version "2.1.0" resolved "https://registry.yarnpkg.com/find-up/-/find-up-2.1.0.tgz#45d1b7e506c717ddd482775a2b77920a3c0c57a7" integrity sha1-RdG35QbHF93UgndaK3eSCjwMV6c= dependencies: locate-path "^2.0.0" find-up@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/find-up/-/find-up-3.0.0.tgz#49169f1d7993430646da61ecc5ae355c21c97b73" integrity sha512-1yD6RmLI1XBfxugvORwlck6f75tYL+iR0jqwsOrOxMZyGYqUuDhJ0l4AXdO1iX/FTs9cBAMEk1gWSEx1kSbylg== dependencies: locate-path "^3.0.0" findit@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/findit/-/findit-2.0.0.tgz#6509f0126af4c178551cfa99394e032e13a4d56e" integrity sha1-ZQnwEmr0wXhVHPqZOU4DLhOk1W4= flat-cache@^2.0.1: version "2.0.1" resolved "https://registry.yarnpkg.com/flat-cache/-/flat-cache-2.0.1.tgz#5d296d6f04bda44a4630a301413bdbc2ec085ec0" integrity sha512-LoQe6yDuUMDzQAEH8sgmh4Md6oZnc/7PjtwjNFSzveXqSHt6ka9fPBuso7IGf9Rz4uqnSnWiFH2B/zj24a5ReA== dependencies: flatted "^2.0.0" rimraf "2.6.3" write "1.0.3" flatted@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/flatted/-/flatted-2.0.0.tgz#55122b6536ea496b4b44893ee2608141d10d9916" integrity sha512-R+H8IZclI8AAkSBRQJLVOsxwAoHd6WC40b4QTNWIjzAa6BXOBfQcM587MXDTVPeYaopFNWHUFLx7eNmHDSxMWg== fn-name@~2.0.1: version "2.0.1" resolved "https://registry.yarnpkg.com/fn-name/-/fn-name-2.0.1.tgz#5214d7537a4d06a4a301c0cc262feb84188002e7" integrity sha1-UhTXU3pNBqSjAcDMJi/rhBiAAuc= for-in@^1.0.2: version "1.0.2" resolved "https://registry.yarnpkg.com/for-in/-/for-in-1.0.2.tgz#81068d295a8142ec0ac726c6e2200c30fb6d5e80" integrity sha1-gQaNKVqBQuwKxybG4iAMMPttXoA= foreground-child@^1.3.3, foreground-child@^1.5.6: version "1.5.6" resolved "https://registry.yarnpkg.com/foreground-child/-/foreground-child-1.5.6.tgz#4fd71ad2dfde96789b980a5c0a295937cb2f5ce9" integrity sha1-T9ca0t/elnibmApcCilZN8svXOk= dependencies: cross-spawn "^4" signal-exit "^3.0.0" forever-agent@~0.6.1: version "0.6.1" resolved "https://registry.yarnpkg.com/forever-agent/-/forever-agent-0.6.1.tgz#fbc71f0c41adeb37f96c577ad1ed42d8fdacca91" integrity sha1-+8cfDEGt6zf5bFd60e1C2P2sypE= form-data@~2.3.2: version "2.3.3" resolved "https://registry.yarnpkg.com/form-data/-/form-data-2.3.3.tgz#dcce52c05f644f298c6a7ab936bd724ceffbf3a6" integrity sha512-1lLKB2Mu3aGP1Q/2eCOx0fNbRMe7XdwktwOruhfqqd0rIJWwN4Dh+E3hrPSlDCXnSR7UtZ1N38rVXm+6+MEhJQ== dependencies: asynckit "^0.4.0" combined-stream "^1.0.6" mime-types "^2.1.12" fragment-cache@^0.2.1: version "0.2.1" resolved "https://registry.yarnpkg.com/fragment-cache/-/fragment-cache-0.2.1.tgz#4290fad27f13e89be7f33799c6bc5a0abfff0d19" integrity sha1-QpD60n8T6Jvn8zeZxrxaCr//DRk= dependencies: map-cache "^0.2.2" fs-exists-cached@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/fs-exists-cached/-/fs-exists-cached-1.0.0.tgz#cf25554ca050dc49ae6656b41de42258989dcbce" integrity sha1-zyVVTKBQ3EmuZla0HeQiWJidy84= fs-minipass@^1.2.5: version "1.2.5" resolved "https://registry.yarnpkg.com/fs-minipass/-/fs-minipass-1.2.5.tgz#06c277218454ec288df77ada54a03b8702aacb9d" integrity sha512-JhBl0skXjUPCFH7x6x61gQxrKyXsxB5gcgePLZCwfyCGGsTISMoIeObbrvVeP6Xmyaudw4TT43qV2Gz+iyd2oQ== dependencies: minipass "^2.2.1" fs-readdir-recursive@^1.1.0: version "1.1.0" resolved "https://registry.yarnpkg.com/fs-readdir-recursive/-/fs-readdir-recursive-1.1.0.tgz#e32fc030a2ccee44a6b5371308da54be0b397d27" integrity sha512-GNanXlVr2pf02+sPN40XN8HG+ePaNcvM0q5mZBd668Obwb0yD5GiUbZOFgwn8kGMY6I3mdyDJzieUy3PTYyTRA== fs.realpath@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/fs.realpath/-/fs.realpath-1.0.0.tgz#1504ad2523158caa40db4a2787cb01411994ea4f" integrity sha1-FQStJSMVjKpA20onh8sBQRmU6k8= fsevents@^1.2.7: version "1.2.9" resolved "https://registry.yarnpkg.com/fsevents/-/fsevents-1.2.9.tgz#3f5ed66583ccd6f400b5a00db6f7e861363e388f" integrity sha512-oeyj2H3EjjonWcFjD5NvZNE9Rqe4UW+nQBU2HNeKw0koVLEFIhtyETyAakeAM3de7Z/SW5kcA+fZUait9EApnw== dependencies: nan "^2.12.1" node-pre-gyp "^0.12.0" function-bind@^1.1.1: version "1.1.1" resolved "https://registry.yarnpkg.com/function-bind/-/function-bind-1.1.1.tgz#a56899d3ea3c9bab874bb9773b7c5ede92f4895d" integrity sha512-yIovAzMX49sF8Yl58fSCWJ5svSLuaibPxXQJFLmBObTuCr0Mf1KiPopGM9NiFjiYBCbfaa2Fh6breQ6ANVTI0A== function-loop@^1.0.2: version "1.0.2" resolved "https://registry.yarnpkg.com/function-loop/-/function-loop-1.0.2.tgz#16b93dd757845eacfeca1a8061a6a65c106e0cb2" integrity sha512-Iw4MzMfS3udk/rqxTiDDCllhGwlOrsr50zViTOO/W6lS/9y6B1J0BD2VZzrnWUYBJsl3aeqjgR5v7bWWhZSYbA== functional-red-black-tree@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/functional-red-black-tree/-/functional-red-black-tree-1.0.1.tgz#1b0ab3bd553b2a0d6399d29c0e3ea0b252078327" integrity sha1-GwqzvVU7Kg1jmdKcDj6gslIHgyc= g-status@^2.0.2: version "2.0.2" resolved "https://registry.yarnpkg.com/g-status/-/g-status-2.0.2.tgz#270fd32119e8fc9496f066fe5fe88e0a6bc78b97" integrity sha512-kQoE9qH+T1AHKgSSD0Hkv98bobE90ILQcXAF4wvGgsr7uFqNvwmh8j+Lq3l0RVt3E3HjSbv2B9biEGcEtpHLCA== dependencies: arrify "^1.0.1" matcher "^1.0.0" simple-git "^1.85.0" gauge@~2.7.3: version "2.7.4" resolved "https://registry.yarnpkg.com/gauge/-/gauge-2.7.4.tgz#2c03405c7538c39d7eb37b317022e325fb018bf7" integrity sha1-LANAXHU4w51+s3sxcCLjJfsBi/c= dependencies: aproba "^1.0.3" console-control-strings "^1.0.0" has-unicode "^2.0.0" object-assign "^4.1.0" signal-exit "^3.0.0" string-width "^1.0.1" strip-ansi "^3.0.1" wide-align "^1.1.0" get-caller-file@^2.0.1: version "2.0.5" resolved "https://registry.yarnpkg.com/get-caller-file/-/get-caller-file-2.0.5.tgz#4f94412a82db32f36e3b0b9741f8a97feb031f7e" integrity sha512-DyFP3BM/3YHTQOCUL/w0OZHR0lpKeGrxotcHWcqNEdnltqFwXVfhEBQ94eIo34AfQpo0rGki4cyIiftY06h2Fg== get-own-enumerable-property-symbols@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/get-own-enumerable-property-symbols/-/get-own-enumerable-property-symbols-3.0.0.tgz#b877b49a5c16aefac3655f2ed2ea5b684df8d203" integrity sha512-CIJYJC4GGF06TakLg8z4GQKvDsx9EMspVxOYih7LerEL/WosUnFIww45CGfxfeKHqlg3twgUrYRT1O3WQqjGCg== get-stdin@^6.0.0: version "6.0.0" resolved "https://registry.yarnpkg.com/get-stdin/-/get-stdin-6.0.0.tgz#9e09bf712b360ab9225e812048f71fde9c89657b" integrity sha512-jp4tHawyV7+fkkSKyvjuLZswblUtz+SQKzSWnBbii16BuZksJlU1wuBYXY75r+duh/llF1ur6oNwi+2ZzjKZ7g== get-stdin@^7.0.0: version "7.0.0" resolved "https://registry.yarnpkg.com/get-stdin/-/get-stdin-7.0.0.tgz#8d5de98f15171a125c5e516643c7a6d0ea8a96f6" integrity sha512-zRKcywvrXlXsA0v0i9Io4KDRaAw7+a1ZpjRwl9Wox8PFlVCCHra7E9c4kqXCoCM9nR5tBkaTTZRBoCm60bFqTQ== get-stream@^4.0.0: version "4.1.0" resolved "https://registry.yarnpkg.com/get-stream/-/get-stream-4.1.0.tgz#c1b255575f3dc21d59bfc79cd3d2b46b1c3a54b5" integrity sha512-GMat4EJ5161kIy2HevLlr4luNjBgvmj413KaQA7jt4V8B4RDsfpHk7WQ9GVqfYyyx8OS/L66Kox+rJRNklLK7w== dependencies: pump "^3.0.0" get-value@^2.0.3, get-value@^2.0.6: version "2.0.6" resolved "https://registry.yarnpkg.com/get-value/-/get-value-2.0.6.tgz#dc15ca1c672387ca76bd37ac0a395ba2042a2c28" integrity sha1-3BXKHGcjh8p2vTesCjlbogQqLCg= getpass@^0.1.1: version "0.1.7" resolved "https://registry.yarnpkg.com/getpass/-/getpass-0.1.7.tgz#5eff8e3e684d569ae4cb2b1282604e8ba62149fa" integrity sha1-Xv+OPmhNVprkyysSgmBOi6YhSfo= dependencies: assert-plus "^1.0.0" glob-parent@^3.1.0: version "3.1.0" resolved "https://registry.yarnpkg.com/glob-parent/-/glob-parent-3.1.0.tgz#9e6af6299d8d3bd2bd40430832bd113df906c5ae" integrity sha1-nmr2KZ2NO9K9QEMIMr0RPfkGxa4= dependencies: is-glob "^3.1.0" path-dirname "^1.0.0" glob@^7.0.0, glob@^7.0.3, glob@^7.0.5, glob@^7.1.2, glob@^7.1.3: version "7.1.3" resolved "https://registry.yarnpkg.com/glob/-/glob-7.1.3.tgz#3960832d3f1574108342dafd3a67b332c0969df1" integrity sha512-vcfuiIxogLV4DlGBHIUOwI0IbrJ8HWPc4MU7HzviGeNho/UJDfi6B5p3sHeWIQ0KGIU0Jpxi5ZHxemQfLkkAwQ== dependencies: fs.realpath "^1.0.0" inflight "^1.0.4" inherits "2" minimatch "^3.0.4" once "^1.3.0" path-is-absolute "^1.0.0" globals@^11.1.0, globals@^11.7.0: version "11.10.0" resolved "https://registry.yarnpkg.com/globals/-/globals-11.10.0.tgz#1e09776dffda5e01816b3bb4077c8b59c24eaa50" integrity sha512-0GZF1RiPKU97IHUO5TORo9w1PwrH/NBPl+fS7oMLdaTRiYmYbwK4NWoZWrAdd0/abG9R2BU+OiwyQpTpE6pdfQ== globals@^9.18.0: version "9.18.0" resolved "https://registry.yarnpkg.com/globals/-/globals-9.18.0.tgz#aa3896b3e69b487f17e31ed2143d69a8e30c2d8a" integrity sha512-S0nG3CLEQiY/ILxqtztTWH/3iRRdyBLw6KMDxnKMchrtbj2OFmehVh0WUCfW3DUrIgx/qFrJPICrq4Z4sTR9UQ== globby@^6.1.0: version "6.1.0" resolved "https://registry.yarnpkg.com/globby/-/globby-6.1.0.tgz#f5a6d70e8395e21c858fb0489d64df02424d506c" integrity sha1-9abXDoOV4hyFj7BInWTfAkJNUGw= dependencies: array-union "^1.0.1" glob "^7.0.3" object-assign "^4.0.1" pify "^2.0.0" pinkie-promise "^2.0.0" graceful-fs@^4.1.11, graceful-fs@^4.1.15, graceful-fs@^4.1.2: version "4.1.15" resolved "https://registry.yarnpkg.com/graceful-fs/-/graceful-fs-4.1.15.tgz#ffb703e1066e8a0eeaa4c8b80ba9253eeefbfb00" integrity sha512-6uHUhOPEBgQ24HM+r6b/QwWfZq+yiFcipKFrOFiBEnWdy5sdzYoi+pJeQaPI5qOLRFqWmAXUPQNsielzdLoecA== "growl@~> 1.10.0": version "1.10.5" resolved "https://registry.yarnpkg.com/growl/-/growl-1.10.5.tgz#f2735dc2283674fa67478b10181059355c369e5e" integrity sha512-qBr4OuELkhPenW6goKVXiv47US3clb3/IbuWF9KNKEijAy9oeHxU9IgzjvJhHkUzhaj7rOUD7+YGWqUjLp5oSA== handlebars@^4.1.2: version "4.1.2" resolved "https://registry.yarnpkg.com/handlebars/-/handlebars-4.1.2.tgz#b6b37c1ced0306b221e094fc7aca3ec23b131b67" integrity sha512-nvfrjqvt9xQ8Z/w0ijewdD/vvWDTOweBUm96NTr66Wfvo1mJenBLwcYmPs3TIBP5ruzYGD7Hx/DaM9RmhroGPw== dependencies: neo-async "^2.6.0" optimist "^0.6.1" source-map "^0.6.1" optionalDependencies: uglify-js "^3.1.4" har-schema@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/har-schema/-/har-schema-2.0.0.tgz#a94c2224ebcac04782a0d9035521f24735b7ec92" integrity sha1-qUwiJOvKwEeCoNkDVSHyRzW37JI= har-validator@~5.1.0: version "5.1.3" resolved "https://registry.yarnpkg.com/har-validator/-/har-validator-5.1.3.tgz#1ef89ebd3e4996557675eed9893110dc350fa080" integrity sha512-sNvOCzEQNr/qrvJgc3UG/kD4QtlHycrzwS+6mfTrrSq97BvaYcPZZI1ZSqGSPR73Cxn4LKTD4PttRwfU7jWq5g== dependencies: ajv "^6.5.5" har-schema "^2.0.0" has-ansi@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/has-ansi/-/has-ansi-2.0.0.tgz#34f5049ce1ecdf2b0649af3ef24e45ed35416d91" integrity sha1-NPUEnOHs3ysGSa8+8k5F7TVBbZE= dependencies: ansi-regex "^2.0.0" has-flag@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/has-flag/-/has-flag-3.0.0.tgz#b5d454dc2199ae225699f3467e5a07f3b955bafd" integrity sha1-tdRU3CGZriJWmfNGfloH87lVuv0= has-symbols@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/has-symbols/-/has-symbols-1.0.0.tgz#ba1a8f1af2a0fc39650f5c850367704122063b44" integrity sha1-uhqPGvKg/DllD1yFA2dwQSIGO0Q= has-unicode@^2.0.0: version "2.0.1" resolved "https://registry.yarnpkg.com/has-unicode/-/has-unicode-2.0.1.tgz#e0e6fe6a28cf51138855e086d1691e771de2a8b9" integrity sha1-4Ob+aijPUROIVeCG0Wkedx3iqLk= has-value@^0.3.1: version "0.3.1" resolved "https://registry.yarnpkg.com/has-value/-/has-value-0.3.1.tgz#7b1f58bada62ca827ec0a2078025654845995e1f" integrity sha1-ex9YutpiyoJ+wKIHgCVlSEWZXh8= dependencies: get-value "^2.0.3" has-values "^0.1.4" isobject "^2.0.0" has-value@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/has-value/-/has-value-1.0.0.tgz#18b281da585b1c5c51def24c930ed29a0be6b177" integrity sha1-GLKB2lhbHFxR3vJMkw7SmgvmsXc= dependencies: get-value "^2.0.6" has-values "^1.0.0" isobject "^3.0.0" has-values@^0.1.4: version "0.1.4" resolved "https://registry.yarnpkg.com/has-values/-/has-values-0.1.4.tgz#6d61de95d91dfca9b9a02089ad384bff8f62b771" integrity sha1-bWHeldkd/Km5oCCJrThL/49it3E= has-values@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/has-values/-/has-values-1.0.0.tgz#95b0b63fec2146619a6fe57fe75628d5a39efe4f" integrity sha1-lbC2P+whRmGab+V/51Yo1aOe/k8= dependencies: is-number "^3.0.0" kind-of "^4.0.0" has@^1.0.1, has@^1.0.3: version "1.0.3" resolved "https://registry.yarnpkg.com/has/-/has-1.0.3.tgz#722d7cbfc1f6aa8241f16dd814e011e1f41e8796" integrity sha512-f2dvO0VU6Oej7RkWJGrehjbzMAjFp5/VKPp5tTpWIV4JHHZK1/BxbFRtf/siA2SWTe09caDmVtYYzWEIbBS4zw== dependencies: function-bind "^1.1.1" hasha@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/hasha/-/hasha-3.0.0.tgz#52a32fab8569d41ca69a61ff1a214f8eb7c8bd39" integrity sha1-UqMvq4Vp1BymmmH/GiFPjrfIvTk= dependencies: is-stream "^1.0.1" home-or-tmp@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/home-or-tmp/-/home-or-tmp-2.0.0.tgz#e36c3f2d2cae7d746a857e38d18d5f32a7882db8" integrity sha1-42w/LSyufXRqhX440Y1fMqeILbg= dependencies: os-homedir "^1.0.0" os-tmpdir "^1.0.1" hosted-git-info@^2.1.4: version "2.7.1" resolved "https://registry.yarnpkg.com/hosted-git-info/-/hosted-git-info-2.7.1.tgz#97f236977bd6e125408930ff6de3eec6281ec047" integrity sha512-7T/BxH19zbcCTa8XkMlbK5lTo1WtgkFi3GvdWEyNuc4Vex7/9Dqbnpsf4JMydcfj9HCg4zUWFTL3Za6lapg5/w== http-signature@~1.2.0: version "1.2.0" resolved "https://registry.yarnpkg.com/http-signature/-/http-signature-1.2.0.tgz#9aecd925114772f3d95b65a60abb8f7c18fbace1" integrity sha1-muzZJRFHcvPZW2WmCruPfBj7rOE= dependencies: assert-plus "^1.0.0" jsprim "^1.2.2" sshpk "^1.7.0" husky@^2.2.0: version "2.2.0" resolved "https://registry.yarnpkg.com/husky/-/husky-2.2.0.tgz#4dda4370ba0f145b6594be4a4e4e4d4c82a6f2d5" integrity sha512-lG33E7zq6v//H/DQIojPEi1ZL9ebPFt3MxUMD8MR0lrS2ljEPiuUUxlziKIs/o9EafF0chL7bAtLQkcPvXmdnA== dependencies: cosmiconfig "^5.2.0" execa "^1.0.0" find-up "^3.0.0" get-stdin "^7.0.0" is-ci "^2.0.0" pkg-dir "^4.1.0" please-upgrade-node "^3.1.1" read-pkg "^5.0.0" run-node "^1.0.0" slash "^2.0.0" iconv-lite@^0.4.24, iconv-lite@^0.4.4: version "0.4.24" resolved "https://registry.yarnpkg.com/iconv-lite/-/iconv-lite-0.4.24.tgz#2022b4b25fbddc21d2f524974a474aafe733908b" integrity sha512-v3MXnZAcvnywkTUEZomIActle7RXXeedOR31wwl7VlyoXO4Qi9arvSenNQWne1TcRwhCL1HwLI21bEqdpj8/rA== dependencies: safer-buffer ">= 2.1.2 < 3" ignore-walk@^3.0.1: version "3.0.1" resolved "https://registry.yarnpkg.com/ignore-walk/-/ignore-walk-3.0.1.tgz#a83e62e7d272ac0e3b551aaa82831a19b69f82f8" integrity sha512-DTVlMx3IYPe0/JJcYP7Gxg7ttZZu3IInhuEhbchuqneY9wWe5Ojy2mXLBaQFUQmo0AW2r3qG7m1mg86js+gnlQ== dependencies: minimatch "^3.0.4" ignore@^4.0.6: version "4.0.6" resolved "https://registry.yarnpkg.com/ignore/-/ignore-4.0.6.tgz#750e3db5862087b4737ebac8207ffd1ef27b25fc" integrity sha512-cyFDKrqc/YdcWFniJhzI42+AzS+gNwmUzOSFcRCQYwySuBBBy/KjuxWLZ/FHEH6Moq1NizMOBWyTcv8O4OZIMg== ignore@^5.1.1: version "5.1.1" resolved "https://registry.yarnpkg.com/ignore/-/ignore-5.1.1.tgz#2fc6b8f518aff48fef65a7f348ed85632448e4a5" integrity sha512-DWjnQIFLenVrwyRCKZT+7a7/U4Cqgar4WG8V++K3hw+lrW1hc/SIwdiGmtxKCVACmHULTuGeBbHJmbwW7/sAvA== import-fresh@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/import-fresh/-/import-fresh-2.0.0.tgz#d81355c15612d386c61f9ddd3922d4304822a546" integrity sha1-2BNVwVYS04bGH53dOSLUMEgipUY= dependencies: caller-path "^2.0.0" resolve-from "^3.0.0" import-fresh@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/import-fresh/-/import-fresh-3.0.0.tgz#a3d897f420cab0e671236897f75bc14b4885c390" integrity sha512-pOnA9tfM3Uwics+SaBLCNyZZZbK+4PTu0OPZtLlMIrv17EdBoC15S9Kn8ckJ9TZTyKb3ywNE5y1yeDxxGA7nTQ== dependencies: parent-module "^1.0.0" resolve-from "^4.0.0" import-jsx@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/import-jsx/-/import-jsx-2.0.0.tgz#5ec3283f75a38c154714586c2aae72403eb216de" integrity sha512-xmrgtiRnAdjIaRzKwsHut54FA8nx59WqN4MpQvPFr/8yD6BamavkmKHrA5dotAlnIiF4uqMzg/lA5yhPdpIXsA== dependencies: babel-core "^6.25.0" babel-plugin-transform-es2015-destructuring "^6.23.0" babel-plugin-transform-object-rest-spread "^6.23.0" babel-plugin-transform-react-jsx "^6.24.1" caller-path "^2.0.0" resolve-from "^3.0.0" imurmurhash@^0.1.4: version "0.1.4" resolved "https://registry.yarnpkg.com/imurmurhash/-/imurmurhash-0.1.4.tgz#9218b9b2b928a238b13dc4fb6b6d576f231453ea" integrity sha1-khi5srkoojixPcT7a21XbyMUU+o= indent-string@^3.0.0: version "3.2.0" resolved "https://registry.yarnpkg.com/indent-string/-/indent-string-3.2.0.tgz#4a5fd6d27cc332f37e5419a504dbb837105c9289" integrity sha1-Sl/W0nzDMvN+VBmlBNu4NxBckok= inflight@^1.0.4: version "1.0.6" resolved "https://registry.yarnpkg.com/inflight/-/inflight-1.0.6.tgz#49bd6331d7d02d0c09bc910a1075ba8165b56df9" integrity sha1-Sb1jMdfQLQwJvJEKEHW6gWW1bfk= dependencies: once "^1.3.0" wrappy "1" inherits@2, inherits@^2.0.3, inherits@~2.0.3: version "2.0.3" resolved "https://registry.yarnpkg.com/inherits/-/inherits-2.0.3.tgz#633c2c83e3da42a502f52466022480f4208261de" integrity sha1-Yzwsg+PaQqUC9SRmAiSA9CCCYd4= ini@~1.3.0: version "1.3.5" resolved "https://registry.yarnpkg.com/ini/-/ini-1.3.5.tgz#eee25f56db1c9ec6085e0c22778083f596abf927" integrity sha512-RZY5huIKCMRWDUqZlEi72f/lmXKMvuszcMBduliQ3nnWbx9X/ZBQO7DijMEYS9EhHBb2qacRUMtC7svLwe0lcw== ink@^2.1.1: version "2.1.1" resolved "https://registry.yarnpkg.com/ink/-/ink-2.1.1.tgz#efb2adbd30be79b4f640d7c67b524bfb030205ba" integrity sha512-vP1yE/uJoiY6uB9yHalczUA02I9fg7xDUbTEZitPK5y6dvnPo9a/6UWqIB2uCYkHOhEZMN+D/TsVr4v2sz8qYA== dependencies: "@types/react" "^16.8.6" arrify "^1.0.1" auto-bind "^2.0.0" chalk "^2.4.1" cli-cursor "^2.1.0" cli-truncate "^1.1.0" is-ci "^2.0.0" lodash.throttle "^4.1.1" log-update "^3.0.0" prop-types "^15.6.2" react-reconciler "^0.20.0" scheduler "^0.13.2" signal-exit "^3.0.2" slice-ansi "^1.0.0" string-length "^2.0.0" widest-line "^2.0.0" wrap-ansi "^5.0.0" yoga-layout-prebuilt "^1.9.3" inquirer@^6.2.2: version "6.2.2" resolved "https://registry.yarnpkg.com/inquirer/-/inquirer-6.2.2.tgz#46941176f65c9eb20804627149b743a218f25406" integrity sha512-Z2rREiXA6cHRR9KBOarR3WuLlFzlIfAEIiB45ll5SSadMg7WqOh1MKEjjndfuH5ewXdixWCxqnVfGOQzPeiztA== dependencies: ansi-escapes "^3.2.0" chalk "^2.4.2" cli-cursor "^2.1.0" cli-width "^2.0.0" external-editor "^3.0.3" figures "^2.0.0" lodash "^4.17.11" mute-stream "0.0.7" run-async "^2.2.0" rxjs "^6.4.0" string-width "^2.1.0" strip-ansi "^5.0.0" through "^2.3.6" invariant@^2.2.2: version "2.2.4" resolved "https://registry.yarnpkg.com/invariant/-/invariant-2.2.4.tgz#610f3c92c9359ce1db616e538008d23ff35158e6" integrity sha512-phJfQVBuaJM5raOpJjSfkiD6BpbCE4Ns//LaXl6wGYtUBY83nWS6Rf9tXm2e8VaK60JEjYldbPif/A2B1C2gNA== dependencies: loose-envify "^1.0.0" invert-kv@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/invert-kv/-/invert-kv-2.0.0.tgz#7393f5afa59ec9ff5f67a27620d11c226e3eec02" integrity sha512-wPVv/y/QQ/Uiirj/vh3oP+1Ww+AWehmi1g5fFWGPF6IpCBCDVrhgHRMvrLfdYcwDh3QJbGXDW4JAuzxElLSqKA== is-accessor-descriptor@^0.1.6: version "0.1.6" resolved "https://registry.yarnpkg.com/is-accessor-descriptor/-/is-accessor-descriptor-0.1.6.tgz#a9e12cb3ae8d876727eeef3843f8a0897b5c98d6" integrity sha1-qeEss66Nh2cn7u84Q/igiXtcmNY= dependencies: kind-of "^3.0.2" is-accessor-descriptor@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/is-accessor-descriptor/-/is-accessor-descriptor-1.0.0.tgz#169c2f6d3df1f992618072365c9b0ea1f6878656" integrity sha512-m5hnHTkcVsPfqx3AKlyttIPb7J+XykHvJP2B9bZDjlhLIoEq4XoK64Vg7boZlVWYK6LUY94dYPEE7Lh0ZkZKcQ== dependencies: kind-of "^6.0.0" is-arrayish@^0.2.1: version "0.2.1" resolved "https://registry.yarnpkg.com/is-arrayish/-/is-arrayish-0.2.1.tgz#77c99840527aa8ecb1a8ba697b80645a7a926a9d" integrity sha1-d8mYQFJ6qOyxqLppe4BkWnqSap0= is-binary-path@^1.0.0: version "1.0.1" resolved "https://registry.yarnpkg.com/is-binary-path/-/is-binary-path-1.0.1.tgz#75f16642b480f187a711c814161fd3a4a7655898" integrity sha1-dfFmQrSA8YenEcgUFh/TpKdlWJg= dependencies: binary-extensions "^1.0.0" is-buffer@^1.1.5: version "1.1.6" resolved "https://registry.yarnpkg.com/is-buffer/-/is-buffer-1.1.6.tgz#efaa2ea9daa0d7ab2ea13a97b2b8ad51fefbe8be" integrity sha512-NcdALwpXkTm5Zvvbk7owOUSvVvBKDgKP5/ewfXEznmQFfs4ZRmanOeKBTjRVjka3QFoN6XJ+9F3USqfHqTaU5w== is-builtin-module@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/is-builtin-module/-/is-builtin-module-1.0.0.tgz#540572d34f7ac3119f8f76c30cbc1b1e037affbe" integrity sha1-VAVy0096wxGfj3bDDLwbHgN6/74= dependencies: builtin-modules "^1.0.0" is-callable@^1.1.4: version "1.1.4" resolved "https://registry.yarnpkg.com/is-callable/-/is-callable-1.1.4.tgz#1e1adf219e1eeb684d691f9d6a05ff0d30a24d75" integrity sha512-r5p9sxJjYnArLjObpjA4xu5EKI3CuKHkJXMhT7kwbpUyIFD1n5PMAsoPvWnvtZiNz7LjkYDRZhd7FlI0eMijEA== is-ci@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/is-ci/-/is-ci-2.0.0.tgz#6bc6334181810e04b5c22b3d589fdca55026404c" integrity sha512-YfJT7rkpQB0updsdHLGWrvhBJfcfzNNawYDNIyQXJz0IViGf75O8EBPKSdvw2rF+LGCsX4FZ8tcr3b19LcZq4w== dependencies: ci-info "^2.0.0" is-data-descriptor@^0.1.4: version "0.1.4" resolved "https://registry.yarnpkg.com/is-data-descriptor/-/is-data-descriptor-0.1.4.tgz#0b5ee648388e2c860282e793f1856fec3f301b56" integrity sha1-C17mSDiOLIYCgueT8YVv7D8wG1Y= dependencies: kind-of "^3.0.2" is-data-descriptor@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/is-data-descriptor/-/is-data-descriptor-1.0.0.tgz#d84876321d0e7add03990406abbbbd36ba9268c7" integrity sha512-jbRXy1FmtAoCjQkVmIVYwuuqDFUbaOeDjmed1tOGPrsMhtJA4rD9tkgA0F1qJ3gRFRXcHYVkdeaP50Q5rE/jLQ== dependencies: kind-of "^6.0.0" is-date-object@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/is-date-object/-/is-date-object-1.0.1.tgz#9aa20eb6aeebbff77fbd33e74ca01b33581d3a16" integrity sha1-mqIOtq7rv/d/vTPnTKAbM1gdOhY= is-descriptor@^0.1.0: version "0.1.6" resolved "https://registry.yarnpkg.com/is-descriptor/-/is-descriptor-0.1.6.tgz#366d8240dde487ca51823b1ab9f07a10a78251ca" integrity sha512-avDYr0SB3DwO9zsMov0gKCESFYqCnE4hq/4z3TdUlukEy5t9C0YRq7HLrsN52NAcqXKaepeCD0n+B0arnVG3Hg== dependencies: is-accessor-descriptor "^0.1.6" is-data-descriptor "^0.1.4" kind-of "^5.0.0" is-descriptor@^1.0.0, is-descriptor@^1.0.2: version "1.0.2" resolved "https://registry.yarnpkg.com/is-descriptor/-/is-descriptor-1.0.2.tgz#3b159746a66604b04f8c81524ba365c5f14d86ec" integrity sha512-2eis5WqQGV7peooDyLmNEPUrps9+SXX5c9pL3xEB+4e9HnGuDa7mB7kHxHw4CbqS9k1T2hOH3miL8n8WtiYVtg== dependencies: is-accessor-descriptor "^1.0.0" is-data-descriptor "^1.0.0" kind-of "^6.0.2" is-directory@^0.3.1: version "0.3.1" resolved "https://registry.yarnpkg.com/is-directory/-/is-directory-0.3.1.tgz#61339b6f2475fc772fd9c9d83f5c8575dc154ae1" integrity sha1-YTObbyR1/Hcv2cnYP1yFddwVSuE= is-extendable@^0.1.0, is-extendable@^0.1.1: version "0.1.1" resolved "https://registry.yarnpkg.com/is-extendable/-/is-extendable-0.1.1.tgz#62b110e289a471418e3ec36a617d472e301dfc89" integrity sha1-YrEQ4omkcUGOPsNqYX1HLjAd/Ik= is-extendable@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/is-extendable/-/is-extendable-1.0.1.tgz#a7470f9e426733d81bd81e1155264e3a3507cab4" integrity sha512-arnXMxT1hhoKo9k1LZdmlNyJdDDfy2v0fXjFlmok4+i8ul/6WlbVge9bhM74OpNPQPMGUToDtz+KXa1PneJxOA== dependencies: is-plain-object "^2.0.4" is-extglob@^2.1.0, is-extglob@^2.1.1: version "2.1.1" resolved "https://registry.yarnpkg.com/is-extglob/-/is-extglob-2.1.1.tgz#a88c02535791f02ed37c76a1b9ea9773c833f8c2" integrity sha1-qIwCU1eR8C7TfHahueqXc8gz+MI= is-finite@^1.0.0: version "1.0.2" resolved "https://registry.yarnpkg.com/is-finite/-/is-finite-1.0.2.tgz#cc6677695602be550ef11e8b4aa6305342b6d0aa" integrity sha1-zGZ3aVYCvlUO8R6LSqYwU0K20Ko= dependencies: number-is-nan "^1.0.0" is-fullwidth-code-point@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/is-fullwidth-code-point/-/is-fullwidth-code-point-1.0.0.tgz#ef9e31386f031a7f0d643af82fde50c457ef00cb" integrity sha1-754xOG8DGn8NZDr4L95QxFfvAMs= dependencies: number-is-nan "^1.0.0" is-fullwidth-code-point@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/is-fullwidth-code-point/-/is-fullwidth-code-point-2.0.0.tgz#a3b30a5c4f199183167aaab93beefae3ddfb654f" integrity sha1-o7MKXE8ZkYMWeqq5O+764937ZU8= is-glob@^3.1.0: version "3.1.0" resolved "https://registry.yarnpkg.com/is-glob/-/is-glob-3.1.0.tgz#7ba5ae24217804ac70707b96922567486cc3e84a" integrity sha1-e6WuJCF4BKxwcHuWkiVnSGzD6Eo= dependencies: is-extglob "^2.1.0" is-glob@^4.0.0: version "4.0.0" resolved "https://registry.yarnpkg.com/is-glob/-/is-glob-4.0.0.tgz#9521c76845cc2610a85203ddf080a958c2ffabc0" integrity sha1-lSHHaEXMJhCoUgPd8ICpWML/q8A= dependencies: is-extglob "^2.1.1" is-number@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/is-number/-/is-number-3.0.0.tgz#24fd6201a4782cf50561c810276afc7d12d71195" integrity sha1-JP1iAaR4LPUFYcgQJ2r8fRLXEZU= dependencies: kind-of "^3.0.2" is-obj@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/is-obj/-/is-obj-1.0.1.tgz#3e4729ac1f5fde025cd7d83a896dab9f4f67db0f" integrity sha1-PkcprB9f3gJc19g6iW2rn09n2w8= is-observable@^1.1.0: version "1.1.0" resolved "https://registry.yarnpkg.com/is-observable/-/is-observable-1.1.0.tgz#b3e986c8f44de950867cab5403f5a3465005975e" integrity sha512-NqCa4Sa2d+u7BWc6CukaObG3Fh+CU9bvixbpcXYhy2VvYS7vVGIdAgnIS5Ks3A/cqk4rebLJ9s8zBstT2aKnIA== dependencies: symbol-observable "^1.1.0" is-path-cwd@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/is-path-cwd/-/is-path-cwd-1.0.0.tgz#d225ec23132e89edd38fda767472e62e65f1106d" integrity sha1-0iXsIxMuie3Tj9p2dHLmLmXxEG0= is-path-in-cwd@^1.0.0: version "1.0.1" resolved "https://registry.yarnpkg.com/is-path-in-cwd/-/is-path-in-cwd-1.0.1.tgz#5ac48b345ef675339bd6c7a48a912110b241cf52" integrity sha512-FjV1RTW48E7CWM7eE/J2NJvAEEVektecDBVBE5Hh3nM1Jd0kvhHtX68Pr3xsDf857xt3Y4AkwVULK1Vku62aaQ== dependencies: is-path-inside "^1.0.0" is-path-inside@^1.0.0: version "1.0.1" resolved "https://registry.yarnpkg.com/is-path-inside/-/is-path-inside-1.0.1.tgz#8ef5b7de50437a3fdca6b4e865ef7aa55cb48036" integrity sha1-jvW33lBDej/cprToZe96pVy0gDY= dependencies: path-is-inside "^1.0.1" is-plain-obj@^1.1.0: version "1.1.0" resolved "https://registry.yarnpkg.com/is-plain-obj/-/is-plain-obj-1.1.0.tgz#71a50c8429dfca773c92a390a4a03b39fcd51d3e" integrity sha1-caUMhCnfync8kqOQpKA7OfzVHT4= is-plain-object@^2.0.1, is-plain-object@^2.0.3, is-plain-object@^2.0.4: version "2.0.4" resolved "https://registry.yarnpkg.com/is-plain-object/-/is-plain-object-2.0.4.tgz#2c163b3fafb1b606d9d17928f05c2a1c38e07677" integrity sha512-h5PpgXkWitc38BBMYawTYMWJHFZJVnBquFE57xFpjB8pJFiF6gZ+bU+WyI/yqXiFR5mdLsgYNaPe8uao6Uv9Og== dependencies: isobject "^3.0.1" is-promise@^2.1.0: version "2.1.0" resolved "https://registry.yarnpkg.com/is-promise/-/is-promise-2.1.0.tgz#79a2a9ece7f096e80f36d2b2f3bc16c1ff4bf3fa" integrity sha1-eaKp7OfwlugPNtKy87wWwf9L8/o= is-regex@^1.0.4: version "1.0.4" resolved "https://registry.yarnpkg.com/is-regex/-/is-regex-1.0.4.tgz#5517489b547091b0930e095654ced25ee97e9491" integrity sha1-VRdIm1RwkbCTDglWVM7SXul+lJE= dependencies: has "^1.0.1" is-regexp@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/is-regexp/-/is-regexp-1.0.0.tgz#fd2d883545c46bac5a633e7b9a09e87fa2cb5069" integrity sha1-/S2INUXEa6xaYz57mgnof6LLUGk= is-stream@^1.0.1, is-stream@^1.1.0: version "1.1.0" resolved "https://registry.yarnpkg.com/is-stream/-/is-stream-1.1.0.tgz#12d4a3dd4e68e0b79ceb8dbc84173ae80d91ca44" integrity sha1-EtSj3U5o4Lec6428hBc66A2RykQ= is-symbol@^1.0.2: version "1.0.2" resolved "https://registry.yarnpkg.com/is-symbol/-/is-symbol-1.0.2.tgz#a055f6ae57192caee329e7a860118b497a950f38" integrity sha512-HS8bZ9ox60yCJLH9snBpIwv9pYUAkcuLhSA1oero1UB5y9aiQpRA8y2ex945AOtCZL1lJDeIk3G5LthswI46Lw== dependencies: has-symbols "^1.0.0" is-typedarray@~1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/is-typedarray/-/is-typedarray-1.0.0.tgz#e479c80858df0c1b11ddda6940f96011fcda4a9a" integrity sha1-5HnICFjfDBsR3dppQPlgEfzaSpo= is-windows@^1.0.2: version "1.0.2" resolved "https://registry.yarnpkg.com/is-windows/-/is-windows-1.0.2.tgz#d1850eb9791ecd18e6182ce12a30f396634bb19d" integrity sha512-eXK1UInq2bPmjyX6e3VHIzMLobc4J94i4AWn+Hpq3OU5KkrRC96OAcR3PRJ/pGu6m8TRnBHP9dkXQVsT/COVIA== isarray@1.0.0, isarray@^1.0.0, isarray@~1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/isarray/-/isarray-1.0.0.tgz#bb935d48582cba168c06834957a54a3e07124f11" integrity sha1-u5NdSFgsuhaMBoNJV6VKPgcSTxE= isexe@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/isexe/-/isexe-2.0.0.tgz#e8fbf374dc556ff8947a10dcb0572d633f2cfa10" integrity sha1-6PvzdNxVb/iUehDcsFctYz8s+hA= isobject@^2.0.0: version "2.1.0" resolved "https://registry.yarnpkg.com/isobject/-/isobject-2.1.0.tgz#f065561096a3f1da2ef46272f815c840d87e0c89" integrity sha1-8GVWEJaj8dou9GJy+BXIQNh+DIk= dependencies: isarray "1.0.0" isobject@^3.0.0, isobject@^3.0.1: version "3.0.1" resolved "https://registry.yarnpkg.com/isobject/-/isobject-3.0.1.tgz#4e431e92b11a9731636aa1f9c8d1ccbcfdab78df" integrity sha1-TkMekrEalzFjaqH5yNHMvP2reN8= isstream@~0.1.2: version "0.1.2" resolved "https://registry.yarnpkg.com/isstream/-/isstream-0.1.2.tgz#47e63f7af55afa6f92e1500e690eb8b8529c099a" integrity sha1-R+Y/evVa+m+S4VAOaQ64uFKcCZo= istanbul-lib-coverage@^2.0.3, istanbul-lib-coverage@^2.0.5: version "2.0.5" resolved "https://registry.yarnpkg.com/istanbul-lib-coverage/-/istanbul-lib-coverage-2.0.5.tgz#675f0ab69503fad4b1d849f736baaca803344f49" integrity sha512-8aXznuEPCJvGnMSRft4udDRDtb1V3pkQkMMI5LI+6HuQz5oQ4J2UFn1H82raA3qJtyOLkkwVqICBQkjnGtn5mA== istanbul-lib-hook@^2.0.7: version "2.0.7" resolved "https://registry.yarnpkg.com/istanbul-lib-hook/-/istanbul-lib-hook-2.0.7.tgz#c95695f383d4f8f60df1f04252a9550e15b5b133" integrity sha512-vrRztU9VRRFDyC+aklfLoeXyNdTfga2EI3udDGn4cZ6fpSXpHLV9X6CHvfoMCPtggg8zvDDmC4b9xfu0z6/llA== dependencies: append-transform "^1.0.0" istanbul-lib-instrument@^3.3.0: version "3.3.0" resolved "https://registry.yarnpkg.com/istanbul-lib-instrument/-/istanbul-lib-instrument-3.3.0.tgz#a5f63d91f0bbc0c3e479ef4c5de027335ec6d630" integrity sha512-5nnIN4vo5xQZHdXno/YDXJ0G+I3dAm4XgzfSVTPLQpj/zAV2dV6Juy0yaf10/zrJOJeHoN3fraFe+XRq2bFVZA== dependencies: "@babel/generator" "^7.4.0" "@babel/parser" "^7.4.3" "@babel/template" "^7.4.0" "@babel/traverse" "^7.4.3" "@babel/types" "^7.4.0" istanbul-lib-coverage "^2.0.5" semver "^6.0.0" istanbul-lib-processinfo@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/istanbul-lib-processinfo/-/istanbul-lib-processinfo-1.0.0.tgz#6c0b59411d2897313ea09165fd95464a32be5610" integrity sha512-FY0cPmWa4WoQNlvB8VOcafiRoB5nB+l2Pz2xGuXHRSy1KM8QFOYfz/rN+bGMCAeejrY3mrpF5oJHcN0s/garCg== dependencies: archy "^1.0.0" cross-spawn "^6.0.5" istanbul-lib-coverage "^2.0.3" rimraf "^2.6.3" uuid "^3.3.2" istanbul-lib-report@^2.0.8: version "2.0.8" resolved "https://registry.yarnpkg.com/istanbul-lib-report/-/istanbul-lib-report-2.0.8.tgz#5a8113cd746d43c4889eba36ab10e7d50c9b4f33" integrity sha512-fHBeG573EIihhAblwgxrSenp0Dby6tJMFR/HvlerBsrCTD5bkUuoNtn3gVh29ZCS824cGGBPn7Sg7cNk+2xUsQ== dependencies: istanbul-lib-coverage "^2.0.5" make-dir "^2.1.0" supports-color "^6.1.0" istanbul-lib-source-maps@^3.0.6: version "3.0.6" resolved "https://registry.yarnpkg.com/istanbul-lib-source-maps/-/istanbul-lib-source-maps-3.0.6.tgz#284997c48211752ec486253da97e3879defba8c8" integrity sha512-R47KzMtDJH6X4/YW9XTx+jrLnZnscW4VpNN+1PViSYTejLVPWv7oov+Duf8YQSPyVRUvueQqz1TcsC6mooZTXw== dependencies: debug "^4.1.1" istanbul-lib-coverage "^2.0.5" make-dir "^2.1.0" rimraf "^2.6.3" source-map "^0.6.1" istanbul-reports@^2.2.4: version "2.2.4" resolved "https://registry.yarnpkg.com/istanbul-reports/-/istanbul-reports-2.2.4.tgz#4e0d0ddf0f0ad5b49a314069d31b4f06afe49ad3" integrity sha512-QCHGyZEK0bfi9GR215QSm+NJwFKEShbtc7tfbUdLAEzn3kKhLDDZqvljn8rPZM9v8CEOhzL1nlYoO4r1ryl67w== dependencies: handlebars "^4.1.2" jackspeak@^1.3.7: version "1.3.7" resolved "https://registry.yarnpkg.com/jackspeak/-/jackspeak-1.3.7.tgz#840d50a33ddba0f122bc6c4efdf6dc966f5218a0" integrity sha512-Z4iSFpaCV7Cocpcl5t9/UyPkisxenbmaqminyTgK6lDDMXcm9EvIZ9Bwr/uFbGOjfWlz1UZwKwFY5AvtgNlHuw== dependencies: cliui "^4.1.0" "js-tokens@^3.0.0 || ^4.0.0", js-tokens@^4.0.0: version "4.0.0" resolved "https://registry.yarnpkg.com/js-tokens/-/js-tokens-4.0.0.tgz#19203fb59991df98e3a287050d4647cdeaf32499" integrity sha512-RdJUflcE3cUzKiMqQgsCu06FPu9UdIJO0beYbPhHN4k6apgJtifcoCtT9bcxOpYBtpD2kCM6Sbzg4CausW/PKQ== js-tokens@^3.0.2: version "3.0.2" resolved "https://registry.yarnpkg.com/js-tokens/-/js-tokens-3.0.2.tgz#9866df395102130e38f7f996bceb65443209c25b" integrity sha1-mGbfOVECEw449/mWvOtlRDIJwls= js-yaml@^3.11.0, js-yaml@^3.13.0, js-yaml@^3.13.1: version "3.13.1" resolved "https://registry.yarnpkg.com/js-yaml/-/js-yaml-3.13.1.tgz#aff151b30bfdfa8e49e05da22e7415e9dfa37847" integrity sha512-YfbcO7jXDdyj0DGxYVSlSeQNHbD7XPWvrVWeVUujrQEoZzWJIRrCPoyk6kL6IAjAG2IolMK4T0hNUe0HOUs5Jw== dependencies: argparse "^1.0.7" esprima "^4.0.0" js-yaml@^3.9.0: version "3.12.1" resolved "https://registry.yarnpkg.com/js-yaml/-/js-yaml-3.12.1.tgz#295c8632a18a23e054cf5c9d3cecafe678167600" integrity sha512-um46hB9wNOKlwkHgiuyEVAybXBjwFUV0Z/RaHJblRd9DXltue9FTYvzCr9ErQrK9Adz5MU4gHWVaNUfdmrC8qA== dependencies: argparse "^1.0.7" esprima "^4.0.0" jsbn@~0.1.0: version "0.1.1" resolved "https://registry.yarnpkg.com/jsbn/-/jsbn-0.1.1.tgz#a5e654c2e5a2deb5f201d96cefbca80c0ef2f513" integrity sha1-peZUwuWi3rXyAdls77yoDA7y9RM= jsesc@^1.3.0: version "1.3.0" resolved "https://registry.yarnpkg.com/jsesc/-/jsesc-1.3.0.tgz#46c3fec8c1892b12b0833db9bc7622176dbab34b" integrity sha1-RsP+yMGJKxKwgz25vHYiF226s0s= jsesc@^2.5.1: version "2.5.2" resolved "https://registry.yarnpkg.com/jsesc/-/jsesc-2.5.2.tgz#80564d2e483dacf6e8ef209650a67df3f0c283a4" integrity sha512-OYu7XEzjkCQ3C5Ps3QIZsQfNpqoJyZZA99wd9aWd05NCtC5pWOkShK2mkL6HXQR6/Cy2lbNdPlZBpuQHXE63gA== json-parse-better-errors@^1.0.1: version "1.0.2" resolved "https://registry.yarnpkg.com/json-parse-better-errors/-/json-parse-better-errors-1.0.2.tgz#bb867cfb3450e69107c131d1c514bab3dc8bcaa9" integrity sha512-mrqyZKfX5EhL7hvqcV6WG1yYjnjeuYDzDhhcAAUrq8Po85NBQBJP+ZDUT75qZQ98IkUoBqdkExkukOU7Ts2wrw== json-schema-traverse@^0.4.1: version "0.4.1" resolved "https://registry.yarnpkg.com/json-schema-traverse/-/json-schema-traverse-0.4.1.tgz#69f6a87d9513ab8bb8fe63bdb0979c448e684660" integrity sha512-xbbCH5dCYU5T8LcEhhuh7HJ88HXuW3qsI3Y0zOZFKfZEHcpWiHU/Jxzk629Brsab/mMiHQti9wMP+845RPe3Vg== json-schema@0.2.3: version "0.2.3" resolved "https://registry.yarnpkg.com/json-schema/-/json-schema-0.2.3.tgz#b480c892e59a2f05954ce727bd3f2a4e882f9e13" integrity sha1-tIDIkuWaLwWVTOcnvT8qTogvnhM= json-stable-stringify-without-jsonify@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/json-stable-stringify-without-jsonify/-/json-stable-stringify-without-jsonify-1.0.1.tgz#9db7b59496ad3f3cfef30a75142d2d930ad72651" integrity sha1-nbe1lJatPzz+8wp1FC0tkwrXJlE= json-stringify-safe@~5.0.1: version "5.0.1" resolved "https://registry.yarnpkg.com/json-stringify-safe/-/json-stringify-safe-5.0.1.tgz#1296a2d58fd45f19a0f6ce01d65701e2c735b6eb" integrity sha1-Epai1Y/UXxmg9s4B1lcB4sc1tus= json5@^0.5.1: version "0.5.1" resolved "https://registry.yarnpkg.com/json5/-/json5-0.5.1.tgz#1eade7acc012034ad84e2396767ead9fa5495821" integrity sha1-Hq3nrMASA0rYTiOWdn6tn6VJWCE= json5@^2.1.0: version "2.1.0" resolved "https://registry.yarnpkg.com/json5/-/json5-2.1.0.tgz#e7a0c62c48285c628d20a10b85c89bb807c32850" integrity sha512-8Mh9h6xViijj36g7Dxi+Y4S6hNGV96vcJZr/SrlHh1LR/pEn/8j/+qIBbs44YKl69Lrfctp4QD+AdWLTMqEZAQ== dependencies: minimist "^1.2.0" jsprim@^1.2.2: version "1.4.1" resolved "https://registry.yarnpkg.com/jsprim/-/jsprim-1.4.1.tgz#313e66bc1e5cc06e438bc1b7499c2e5c56acb6a2" integrity sha1-MT5mvB5cwG5Di8G3SZwuXFastqI= dependencies: assert-plus "1.0.0" extsprintf "1.3.0" json-schema "0.2.3" verror "1.10.0" kind-of@^3.0.2, kind-of@^3.0.3, kind-of@^3.2.0: version "3.2.2" resolved "https://registry.yarnpkg.com/kind-of/-/kind-of-3.2.2.tgz#31ea21a734bab9bbb0f32466d893aea51e4a3c64" integrity sha1-MeohpzS6ubuw8yRm2JOupR5KPGQ= dependencies: is-buffer "^1.1.5" kind-of@^4.0.0: version "4.0.0" resolved "https://registry.yarnpkg.com/kind-of/-/kind-of-4.0.0.tgz#20813df3d712928b207378691a45066fae72dd57" integrity sha1-IIE989cSkosgc3hpGkUGb65y3Vc= dependencies: is-buffer "^1.1.5" kind-of@^5.0.0: version "5.1.0" resolved "https://registry.yarnpkg.com/kind-of/-/kind-of-5.1.0.tgz#729c91e2d857b7a419a1f9aa65685c4c33f5845d" integrity sha512-NGEErnH6F2vUuXDh+OlbcKW7/wOcfdRHaZ7VWtqCztfHri/++YKmP51OdWeGPuqCOba6kk2OTe5d02VmTB80Pw== kind-of@^6.0.0, kind-of@^6.0.2: version "6.0.2" resolved "https://registry.yarnpkg.com/kind-of/-/kind-of-6.0.2.tgz#01146b36a6218e64e58f3a8d66de5d7fc6f6d051" integrity sha512-s5kLOcnH0XqDO+FvuaLX8DDjZ18CGFk7VygH40QoKPUQhW4e2rvM0rwUq0t8IQDOwYSeLK01U90OjzBTme2QqA== lcid@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/lcid/-/lcid-2.0.0.tgz#6ef5d2df60e52f82eb228a4c373e8d1f397253cf" integrity sha512-avPEb8P8EGnwXKClwsNUgryVjllcRqtMYa49NTsbQagYuT1DcXnl1915oxWjoyGrXR6zH/Y0Zc96xWsPcoDKeA== dependencies: invert-kv "^2.0.0" lcov-parse@^0.0.10: version "0.0.10" resolved "https://registry.yarnpkg.com/lcov-parse/-/lcov-parse-0.0.10.tgz#1b0b8ff9ac9c7889250582b70b71315d9da6d9a3" integrity sha1-GwuP+ayceIklBYK3C3ExXZ2m2aM= leaked-handles@^5.2.0: version "5.2.0" resolved "https://registry.yarnpkg.com/leaked-handles/-/leaked-handles-5.2.0.tgz#67228e90293b7e0ee36c4190e1f541cddece627f" integrity sha1-ZyKOkCk7fg7jbEGQ4fVBzd7OYn8= dependencies: process "^0.10.0" weakmap-shim "^1.1.0" xtend "^4.0.0" levn@^0.3.0, levn@~0.3.0: version "0.3.0" resolved "https://registry.yarnpkg.com/levn/-/levn-0.3.0.tgz#3b09924edf9f083c0490fdd4c0bc4421e04764ee" integrity sha1-OwmSTt+fCDwEkP3UwLxEIeBHZO4= dependencies: prelude-ls "~1.1.2" type-check "~0.3.2" lint-staged@^8.1.4: version "8.1.6" resolved "https://registry.yarnpkg.com/lint-staged/-/lint-staged-8.1.6.tgz#128a9bc5effbf69a359fb8f7eeb2da71a998daf6" integrity sha512-QT13AniHN6swAtTjsrzxOfE4TVCiQ39xESwLmjGVNCMMZ/PK5aopwvbxLrzw+Zf9OxM3cQG6WCx9lceLzETOnQ== dependencies: chalk "^2.3.1" commander "^2.14.1" cosmiconfig "^5.0.2" debug "^3.1.0" dedent "^0.7.0" del "^3.0.0" execa "^1.0.0" find-parent-dir "^0.3.0" g-status "^2.0.2" is-glob "^4.0.0" is-windows "^1.0.2" listr "^0.14.2" listr-update-renderer "^0.5.0" lodash "^4.17.11" log-symbols "^2.2.0" micromatch "^3.1.8" npm-which "^3.0.1" p-map "^1.1.1" path-is-inside "^1.0.2" pify "^3.0.0" please-upgrade-node "^3.0.2" staged-git-files "1.1.2" string-argv "^0.0.2" stringify-object "^3.2.2" yup "^0.27.0" listr-silent-renderer@^1.1.1: version "1.1.1" resolved "https://registry.yarnpkg.com/listr-silent-renderer/-/listr-silent-renderer-1.1.1.tgz#924b5a3757153770bf1a8e3fbf74b8bbf3f9242e" integrity sha1-kktaN1cVN3C/Go4/v3S4u/P5JC4= listr-update-renderer@^0.5.0: version "0.5.0" resolved "https://registry.yarnpkg.com/listr-update-renderer/-/listr-update-renderer-0.5.0.tgz#4ea8368548a7b8aecb7e06d8c95cb45ae2ede6a2" integrity sha512-tKRsZpKz8GSGqoI/+caPmfrypiaq+OQCbd+CovEC24uk1h952lVj5sC7SqyFUm+OaJ5HN/a1YLt5cit2FMNsFA== dependencies: chalk "^1.1.3" cli-truncate "^0.2.1" elegant-spinner "^1.0.1" figures "^1.7.0" indent-string "^3.0.0" log-symbols "^1.0.2" log-update "^2.3.0" strip-ansi "^3.0.1" listr-verbose-renderer@^0.5.0: version "0.5.0" resolved "https://registry.yarnpkg.com/listr-verbose-renderer/-/listr-verbose-renderer-0.5.0.tgz#f1132167535ea4c1261102b9f28dac7cba1e03db" integrity sha512-04PDPqSlsqIOaaaGZ+41vq5FejI9auqTInicFRndCBgE3bXG8D6W1I+mWhk+1nqbHmyhla/6BUrd5OSiHwKRXw== dependencies: chalk "^2.4.1" cli-cursor "^2.1.0" date-fns "^1.27.2" figures "^2.0.0" listr@^0.14.2: version "0.14.3" resolved "https://registry.yarnpkg.com/listr/-/listr-0.14.3.tgz#2fea909604e434be464c50bddba0d496928fa586" integrity sha512-RmAl7su35BFd/xoMamRjpIE4j3v+L28o8CT5YhAXQJm1fD+1l9ngXY8JAQRJ+tFK2i5njvi0iRUKV09vPwA0iA== dependencies: "@samverschueren/stream-to-observable" "^0.3.0" is-observable "^1.1.0" is-promise "^2.1.0" is-stream "^1.1.0" listr-silent-renderer "^1.1.1" listr-update-renderer "^0.5.0" listr-verbose-renderer "^0.5.0" p-map "^2.0.0" rxjs "^6.3.3" load-json-file@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/load-json-file/-/load-json-file-2.0.0.tgz#7947e42149af80d696cbf797bcaabcfe1fe29ca8" integrity sha1-eUfkIUmvgNaWy/eXvKq8/h/inKg= dependencies: graceful-fs "^4.1.2" parse-json "^2.2.0" pify "^2.0.0" strip-bom "^3.0.0" load-json-file@^4.0.0: version "4.0.0" resolved "https://registry.yarnpkg.com/load-json-file/-/load-json-file-4.0.0.tgz#2f5f45ab91e33216234fd53adab668eb4ec0993b" integrity sha1-L19Fq5HjMhYjT9U62rZo607AmTs= dependencies: graceful-fs "^4.1.2" parse-json "^4.0.0" pify "^3.0.0" strip-bom "^3.0.0" locate-path@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/locate-path/-/locate-path-2.0.0.tgz#2b568b265eec944c6d9c0de9c3dbbbca0354cd8e" integrity sha1-K1aLJl7slExtnA3pw9u7ygNUzY4= dependencies: p-locate "^2.0.0" path-exists "^3.0.0" locate-path@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/locate-path/-/locate-path-3.0.0.tgz#dbec3b3ab759758071b58fe59fc41871af21400e" integrity sha512-7AO748wWnIhNqAuaty2ZWHkQHRSNfPVIsPIfwEOWO22AmaoVrWavlOcMR5nzTLNYvp36X220/maaRsrec1G65A== dependencies: p-locate "^3.0.0" path-exists "^3.0.0" lodash.flattendeep@^4.4.0: version "4.4.0" resolved "https://registry.yarnpkg.com/lodash.flattendeep/-/lodash.flattendeep-4.4.0.tgz#fb030917f86a3134e5bc9bec0d69e0013ddfedb2" integrity sha1-+wMJF/hqMTTlvJvsDWngAT3f7bI= lodash.get@^4.4.2: version "4.4.2" resolved "https://registry.yarnpkg.com/lodash.get/-/lodash.get-4.4.2.tgz#2d177f652fa31e939b4438d5341499dfa3825e99" integrity sha1-LRd/ZS+jHpObRDjVNBSZ36OCXpk= lodash.throttle@^4.1.1: version "4.1.1" resolved "https://registry.yarnpkg.com/lodash.throttle/-/lodash.throttle-4.1.1.tgz#c23e91b710242ac70c37f1e1cda9274cc39bf2f4" integrity sha1-wj6RtxAkKscMN/HhzaknTMOb8vQ= lodash@^4.17.10, lodash@^4.17.11, lodash@^4.17.4: version "4.17.11" resolved "https://registry.yarnpkg.com/lodash/-/lodash-4.17.11.tgz#b39ea6229ef607ecd89e2c8df12536891cac9b8d" integrity sha512-cQKh8igo5QUhZ7lg38DYWAxMvjSAKG0A8wGSVimP07SIUEK2UO+arSRKbRZWtelMtN5V0Hkwh5ryOto/SshYIg== log-driver@^1.2.7: version "1.2.7" resolved "https://registry.yarnpkg.com/log-driver/-/log-driver-1.2.7.tgz#63b95021f0702fedfa2c9bb0a24e7797d71871d8" integrity sha512-U7KCmLdqsGHBLeWqYlFA0V0Sl6P08EE1ZrmA9cxjUE0WVqT9qnyVDPz1kzpFEP0jdJuFnasWIfSd7fsaNXkpbg== log-symbols@^1.0.2: version "1.0.2" resolved "https://registry.yarnpkg.com/log-symbols/-/log-symbols-1.0.2.tgz#376ff7b58ea3086a0f09facc74617eca501e1a18" integrity sha1-N2/3tY6jCGoPCfrMdGF+ylAeGhg= dependencies: chalk "^1.0.0" log-symbols@^2.2.0: version "2.2.0" resolved "https://registry.yarnpkg.com/log-symbols/-/log-symbols-2.2.0.tgz#5740e1c5d6f0dfda4ad9323b5332107ef6b4c40a" integrity sha512-VeIAFslyIerEJLXHziedo2basKbMKtTw3vfn5IzG0XTjhAVEJyNHnL2p7vc+wBDSdQuUpNw3M2u6xb9QsAY5Eg== dependencies: chalk "^2.0.1" log-update@^2.3.0: version "2.3.0" resolved "https://registry.yarnpkg.com/log-update/-/log-update-2.3.0.tgz#88328fd7d1ce7938b29283746f0b1bc126b24708" integrity sha1-iDKP19HOeTiykoN0bwsbwSayRwg= dependencies: ansi-escapes "^3.0.0" cli-cursor "^2.0.0" wrap-ansi "^3.0.1" log-update@^3.0.0: version "3.2.0" resolved "https://registry.yarnpkg.com/log-update/-/log-update-3.2.0.tgz#719f24293250d65d0165f4e2ec2ed805ff062eec" integrity sha512-KJ6zAPIHWo7Xg1jYror6IUDFJBq1bQ4Bi4wAEp2y/0ScjBBVi/g0thr0sUVhuvuXauWzczt7T2QHghPDNnKBuw== dependencies: ansi-escapes "^3.2.0" cli-cursor "^2.1.0" wrap-ansi "^5.0.0" loose-envify@^1.0.0, loose-envify@^1.1.0, loose-envify@^1.4.0: version "1.4.0" resolved "https://registry.yarnpkg.com/loose-envify/-/loose-envify-1.4.0.tgz#71ee51fa7be4caec1a63839f7e682d8132d30caf" integrity sha512-lyuxPGr/Wfhrlem2CL/UcnUc1zcqKAImBDzukY7Y5F/yQiNdko6+fRLevlw1HgMySw7f611UIY408EtxRSoK3Q== dependencies: js-tokens "^3.0.0 || ^4.0.0" lru-cache@^4.0.1: version "4.1.5" resolved "https://registry.yarnpkg.com/lru-cache/-/lru-cache-4.1.5.tgz#8bbe50ea85bed59bc9e33dcab8235ee9bcf443cd" integrity sha512-sWZlbEP2OsHNkXrMl5GYk/jKk70MBng6UU4YI/qGDYbgf6YbP4EvmqISbXCoJiRKs+1bSpFHVgQxvJ17F2li5g== dependencies: pseudomap "^1.0.2" yallist "^2.1.2" make-dir@^2.0.0, make-dir@^2.1.0: version "2.1.0" resolved "https://registry.yarnpkg.com/make-dir/-/make-dir-2.1.0.tgz#5f0310e18b8be898cc07009295a30ae41e91e6f5" integrity sha512-LS9X+dc8KLxXCb8dni79fLIIUA5VyZoyjSMCwTluaXA0o27cCK0bhXkpgw+sTXVpPy/lSO57ilRixqk0vDmtRA== dependencies: pify "^4.0.1" semver "^5.6.0" make-error@^1.1.1: version "1.3.5" resolved "https://registry.yarnpkg.com/make-error/-/make-error-1.3.5.tgz#efe4e81f6db28cadd605c70f29c831b58ef776c8" integrity sha512-c3sIjNUow0+8swNwVpqoH4YCShKNFkMaw6oH1mNS2haDZQqkeZFlHS3dhoeEbKKmJB4vXpJucU6oH75aDYeE9g== map-age-cleaner@^0.1.1: version "0.1.3" resolved "https://registry.yarnpkg.com/map-age-cleaner/-/map-age-cleaner-0.1.3.tgz#7d583a7306434c055fe474b0f45078e6e1b4b92a" integrity sha512-bJzx6nMoP6PDLPBFmg7+xRKeFZvFboMrGlxmNj9ClvX53KrmvM5bXFXEWjbz4cz1AFn+jWJ9z/DJSz7hrs0w3w== dependencies: p-defer "^1.0.0" map-cache@^0.2.2: version "0.2.2" resolved "https://registry.yarnpkg.com/map-cache/-/map-cache-0.2.2.tgz#c32abd0bd6525d9b051645bb4f26ac5dc98a0dbf" integrity sha1-wyq9C9ZSXZsFFkW7TyasXcmKDb8= map-visit@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/map-visit/-/map-visit-1.0.0.tgz#ecdca8f13144e660f1b5bd41f12f3479d98dfb8f" integrity sha1-7Nyo8TFE5mDxtb1B8S80edmN+48= dependencies: object-visit "^1.0.0" matcher@^1.0.0: version "1.1.1" resolved "https://registry.yarnpkg.com/matcher/-/matcher-1.1.1.tgz#51d8301e138f840982b338b116bb0c09af62c1c2" integrity sha512-+BmqxWIubKTRKNWx/ahnCkk3mG8m7OturVlqq6HiojGJTd5hVYbgZm6WzcYPCoB+KBT4Vd6R7WSRG2OADNaCjg== dependencies: escape-string-regexp "^1.0.4" mem@^4.0.0: version "4.3.0" resolved "https://registry.yarnpkg.com/mem/-/mem-4.3.0.tgz#461af497bc4ae09608cdb2e60eefb69bff744178" integrity sha512-qX2bG48pTqYRVmDB37rn/6PT7LcR8T7oAX3bf99u1Tt1nzxYfxkgqDwUwolPlXweM0XzBOBFzSx4kfp7KP1s/w== dependencies: map-age-cleaner "^0.1.1" mimic-fn "^2.0.0" p-is-promise "^2.0.0" merge-source-map@^1.1.0: version "1.1.0" resolved "https://registry.yarnpkg.com/merge-source-map/-/merge-source-map-1.1.0.tgz#2fdde7e6020939f70906a68f2d7ae685e4c8c646" integrity sha512-Qkcp7P2ygktpMPh2mCQZaf3jhN6D3Z/qVZHSdWvQ+2Ef5HgRAPBO57A77+ENm0CPx2+1Ce/MYKi3ymqdfuqibw== dependencies: source-map "^0.6.1" micromatch@^3.1.10, micromatch@^3.1.4, micromatch@^3.1.8: version "3.1.10" resolved "https://registry.yarnpkg.com/micromatch/-/micromatch-3.1.10.tgz#70859bc95c9840952f359a068a3fc49f9ecfac23" integrity sha512-MWikgl9n9M3w+bpsY3He8L+w9eF9338xRl8IAO5viDizwSzziFEyUzo2xrrloB64ADbTf8uA8vRqqttDTOmccg== dependencies: arr-diff "^4.0.0" array-unique "^0.3.2" braces "^2.3.1" define-property "^2.0.2" extend-shallow "^3.0.2" extglob "^2.0.4" fragment-cache "^0.2.1" kind-of "^6.0.2" nanomatch "^1.2.9" object.pick "^1.3.0" regex-not "^1.0.0" snapdragon "^0.8.1" to-regex "^3.0.2" mime-db@1.40.0: version "1.40.0" resolved "https://registry.yarnpkg.com/mime-db/-/mime-db-1.40.0.tgz#a65057e998db090f732a68f6c276d387d4126c32" integrity sha512-jYdeOMPy9vnxEqFRRo6ZvTZ8d9oPb+k18PKoYNYUe2stVEBPPwsln/qWzdbmaIvnhZ9v2P+CuecK+fpUfsV2mA== mime-types@^2.1.12, mime-types@~2.1.19: version "2.1.24" resolved "https://registry.yarnpkg.com/mime-types/-/mime-types-2.1.24.tgz#b6f8d0b3e951efb77dedeca194cff6d16f676f81" integrity sha512-WaFHS3MCl5fapm3oLxU4eYDw77IQM2ACcxQ9RIxfaC3ooc6PFuBMGZZsYpvoXS5D5QTWPieo1jjLdAm3TBP3cQ== dependencies: mime-db "1.40.0" mimic-fn@^1.0.0: version "1.2.0" resolved "https://registry.yarnpkg.com/mimic-fn/-/mimic-fn-1.2.0.tgz#820c86a39334640e99516928bd03fca88057d022" integrity sha512-jf84uxzwiuiIVKiOLpfYk7N46TSy8ubTonmneY9vrpHNAnp0QBt2BxWV9dO3/j+BoVAb+a5G6YDPW3M5HOdMWQ== mimic-fn@^2.0.0: version "2.1.0" resolved "https://registry.yarnpkg.com/mimic-fn/-/mimic-fn-2.1.0.tgz#7ed2c2ccccaf84d3ffcb7a69b57711fc2083401b" integrity sha512-OqbOk5oEQeAZ8WXWydlu9HJjz9WVdEIvamMCcXmuqUYjTknH/sqsWvhQ3vgwKFRR1HpjvNBKQ37nbJgYzGqGcg== minimatch@^3.0.4: version "3.0.4" resolved "https://registry.yarnpkg.com/minimatch/-/minimatch-3.0.4.tgz#5166e286457f03306064be5497e8dbb0c3d32083" integrity sha512-yJHVQEhyqPLUTgt9B83PXu6W3rx4MvvHvSUvToogpwoGDOUQ+yDrR0HRot+yOCdCO7u4hX3pWft6kWBBcqh0UA== dependencies: brace-expansion "^1.1.7" minimist@0.0.8: version "0.0.8" resolved "https://registry.yarnpkg.com/minimist/-/minimist-0.0.8.tgz#857fcabfc3397d2625b8228262e86aa7a011b05d" integrity sha1-hX/Kv8M5fSYluCKCYuhqp6ARsF0= minimist@^1.2.0: version "1.2.0" resolved "https://registry.yarnpkg.com/minimist/-/minimist-1.2.0.tgz#a35008b20f41383eec1fb914f4cd5df79a264284" integrity sha1-o1AIsg9BOD7sH7kU9M1d95omQoQ= minimist@~0.0.1: version "0.0.10" resolved "https://registry.yarnpkg.com/minimist/-/minimist-0.0.10.tgz#de3f98543dbf96082be48ad1a0c7cda836301dcf" integrity sha1-3j+YVD2/lggr5IrRoMfNqDYwHc8= minipass@^2.2.0, minipass@^2.2.1, minipass@^2.3.4, minipass@^2.3.5: version "2.3.5" resolved "https://registry.yarnpkg.com/minipass/-/minipass-2.3.5.tgz#cacebe492022497f656b0f0f51e2682a9ed2d848" integrity sha512-Gi1W4k059gyRbyVUZQ4mEqLm0YIUiGYfvxhF6SIlk3ui1WVxMTGfGdQ2SInh3PDrRTVvPKgULkpJtT4RH10+VA== dependencies: safe-buffer "^5.1.2" yallist "^3.0.0" minizlib@^1.1.1: version "1.2.1" resolved "https://registry.yarnpkg.com/minizlib/-/minizlib-1.2.1.tgz#dd27ea6136243c7c880684e8672bb3a45fd9b614" integrity sha512-7+4oTUOWKg7AuL3vloEWekXY2/D20cevzsrNT2kGWm+39J9hGTCBv8VI5Pm5lXZ/o3/mdR4f8rflAPhnQb8mPA== dependencies: minipass "^2.2.1" mixin-deep@^1.2.0: version "1.3.1" resolved "https://registry.yarnpkg.com/mixin-deep/-/mixin-deep-1.3.1.tgz#a49e7268dce1a0d9698e45326c5626df3543d0fe" integrity sha512-8ZItLHeEgaqEvd5lYBXfm4EZSFCX29Jb9K+lAHhDKzReKBQKj3R+7NOF6tjqYi9t4oI8VUfaWITJQm86wnXGNQ== dependencies: for-in "^1.0.2" is-extendable "^1.0.1" mkdirp@^0.5.0, mkdirp@^0.5.1: version "0.5.1" resolved "https://registry.yarnpkg.com/mkdirp/-/mkdirp-0.5.1.tgz#30057438eac6cf7f8c4767f38648d6697d75c903" integrity sha1-MAV0OOrGz3+MR2fzhkjWaX11yQM= dependencies: minimist "0.0.8" ms@2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/ms/-/ms-2.0.0.tgz#5608aeadfc00be6c2901df5f9861788de0d597c8" integrity sha1-VgiurfwAvmwpAd9fmGF4jeDVl8g= ms@^2.1.1: version "2.1.1" resolved "https://registry.yarnpkg.com/ms/-/ms-2.1.1.tgz#30a5864eb3ebb0a66f2ebe6d727af06a09d86e0a" integrity sha512-tgp+dl5cGk28utYktBsrFqA7HKgrhgPsg6Z/EfhWI4gl1Hwq8B/GmY/0oXZ6nF8hDVesS/FpnYaD/kOWhYQvyg== mute-stream@0.0.7: version "0.0.7" resolved "https://registry.yarnpkg.com/mute-stream/-/mute-stream-0.0.7.tgz#3075ce93bc21b8fab43e1bc4da7e8115ed1e7bab" integrity sha1-MHXOk7whuPq0PhvE2n6BFe0ee6s= nan@^2.12.1: version "2.13.2" resolved "https://registry.yarnpkg.com/nan/-/nan-2.13.2.tgz#f51dc7ae66ba7d5d55e1e6d4d8092e802c9aefe7" integrity sha512-TghvYc72wlMGMVMluVo9WRJc0mB8KxxF/gZ4YYFy7V2ZQX9l7rgbPg7vjS9mt6U5HXODVFVI2bOduCzwOMv/lw== nanomatch@^1.2.9: version "1.2.13" resolved "https://registry.yarnpkg.com/nanomatch/-/nanomatch-1.2.13.tgz#b87a8aa4fc0de8fe6be88895b38983ff265bd119" integrity sha512-fpoe2T0RbHwBTBUOftAfBPaDEi06ufaUai0mE6Yn1kacc3SnTErfb/h+X94VXzI64rKFHYImXSvdwGGCmwOqCA== dependencies: arr-diff "^4.0.0" array-unique "^0.3.2" define-property "^2.0.2" extend-shallow "^3.0.2" fragment-cache "^0.2.1" is-windows "^1.0.2" kind-of "^6.0.2" object.pick "^1.3.0" regex-not "^1.0.0" snapdragon "^0.8.1" to-regex "^3.0.1" natural-compare@^1.4.0: version "1.4.0" resolved "https://registry.yarnpkg.com/natural-compare/-/natural-compare-1.4.0.tgz#4abebfeed7541f2c27acfb29bdbbd15c8d5ba4f7" integrity sha1-Sr6/7tdUHywnrPspvbvRXI1bpPc= needle@^2.2.1: version "2.2.4" resolved "https://registry.yarnpkg.com/needle/-/needle-2.2.4.tgz#51931bff82533b1928b7d1d69e01f1b00ffd2a4e" integrity sha512-HyoqEb4wr/rsoaIDfTH2aVL9nWtQqba2/HvMv+++m8u0dz808MaagKILxtfeSN7QU7nvbQ79zk3vYOJp9zsNEA== dependencies: debug "^2.1.2" iconv-lite "^0.4.4" sax "^1.2.4" neo-async@^2.6.0: version "2.6.0" resolved "https://registry.yarnpkg.com/neo-async/-/neo-async-2.6.0.tgz#b9d15e4d71c6762908654b5183ed38b753340835" integrity sha512-MFh0d/Wa7vkKO3Y3LlacqAEeHK0mckVqzDieUKTT+KGxi+zIpeVsFxymkIiRpbpDziHc290Xr9A1O4Om7otoRA== nested-error-stacks@^2.0.0: version "2.1.0" resolved "https://registry.yarnpkg.com/nested-error-stacks/-/nested-error-stacks-2.1.0.tgz#0fbdcf3e13fe4994781280524f8b96b0cdff9c61" integrity sha512-AO81vsIO1k1sM4Zrd6Hu7regmJN1NSiAja10gc4bX3F0wd+9rQmcuHQaHVQCYIEC8iFXnE+mavh23GOt7wBgug== nice-try@^1.0.4: version "1.0.5" resolved "https://registry.yarnpkg.com/nice-try/-/nice-try-1.0.5.tgz#a3378a7696ce7d223e88fc9b764bd7ef1089e366" integrity sha512-1nh45deeb5olNY7eX82BkPO7SSxR5SSYJiPTrTdFUVYwAl8CKMA5N9PjTYkHiRjisVcxcQ1HXdLhx2qxxJzLNQ== node-pre-gyp@^0.12.0: version "0.12.0" resolved "https://registry.yarnpkg.com/node-pre-gyp/-/node-pre-gyp-0.12.0.tgz#39ba4bb1439da030295f899e3b520b7785766149" integrity sha512-4KghwV8vH5k+g2ylT+sLTjy5wmUOb9vPhnM8NHvRf9dHmnW/CndrFXy2aRPaPST6dugXSdHXfeaHQm77PIz/1A== dependencies: detect-libc "^1.0.2" mkdirp "^0.5.1" needle "^2.2.1" nopt "^4.0.1" npm-packlist "^1.1.6" npmlog "^4.0.2" rc "^1.2.7" rimraf "^2.6.1" semver "^5.3.0" tar "^4" nopt@^4.0.1: version "4.0.1" resolved "https://registry.yarnpkg.com/nopt/-/nopt-4.0.1.tgz#d0d4685afd5415193c8c7505602d0d17cd64474d" integrity sha1-0NRoWv1UFRk8jHUFYC0NF81kR00= dependencies: abbrev "1" osenv "^0.1.4" normalize-package-data@^2.3.2: version "2.4.0" resolved "https://registry.yarnpkg.com/normalize-package-data/-/normalize-package-data-2.4.0.tgz#12f95a307d58352075a04907b84ac8be98ac012f" integrity sha512-9jjUFbTPfEy3R/ad/2oNbKtW9Hgovl5O1FvFWKkKblNXoN/Oou6+9+KKohPK13Yc3/TyunyWhJp6gvRNR/PPAw== dependencies: hosted-git-info "^2.1.4" is-builtin-module "^1.0.0" semver "2 || 3 || 4 || 5" validate-npm-package-license "^3.0.1" normalize-package-data@^2.5.0: version "2.5.0" resolved "https://registry.yarnpkg.com/normalize-package-data/-/normalize-package-data-2.5.0.tgz#e66db1838b200c1dfc233225d12cb36520e234a8" integrity sha512-/5CMN3T0R4XTj4DcGaexo+roZSdSFW/0AOOTROrjxzCG1wrWXEsGbRKevjlIL+ZDE4sZlJr5ED4YW0yqmkK+eA== dependencies: hosted-git-info "^2.1.4" resolve "^1.10.0" semver "2 || 3 || 4 || 5" validate-npm-package-license "^3.0.1" normalize-path@^2.1.1: version "2.1.1" resolved "https://registry.yarnpkg.com/normalize-path/-/normalize-path-2.1.1.tgz#1ab28b556e198363a8c1a6f7e6fa20137fe6aed9" integrity sha1-GrKLVW4Zg2Oowab35vogE3/mrtk= dependencies: remove-trailing-separator "^1.0.1" normalize-path@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/normalize-path/-/normalize-path-3.0.0.tgz#0dcd69ff23a1c9b11fd0978316644a0388216a65" integrity sha512-6eZs5Ls3WtCisHWp9S2GUy8dqkpGi4BVSz3GaqiE6ezub0512ESztXUwUB6C6IKbQkY2Pnb/mD4WYojCRwcwLA== npm-bundled@^1.0.1: version "1.0.5" resolved "https://registry.yarnpkg.com/npm-bundled/-/npm-bundled-1.0.5.tgz#3c1732b7ba936b3a10325aef616467c0ccbcc979" integrity sha512-m/e6jgWu8/v5niCUKQi9qQl8QdeEduFA96xHDDzFGqly0OOjI7c+60KM/2sppfnUU9JJagf+zs+yGhqSOFj71g== npm-packlist@^1.1.6: version "1.2.0" resolved "https://registry.yarnpkg.com/npm-packlist/-/npm-packlist-1.2.0.tgz#55a60e793e272f00862c7089274439a4cc31fc7f" integrity sha512-7Mni4Z8Xkx0/oegoqlcao/JpPCPEMtUvsmB0q7mgvlMinykJLSRTYuFqoQLYgGY8biuxIeiHO+QNJKbCfljewQ== dependencies: ignore-walk "^3.0.1" npm-bundled "^1.0.1" npm-path@^2.0.2: version "2.0.4" resolved "https://registry.yarnpkg.com/npm-path/-/npm-path-2.0.4.tgz#c641347a5ff9d6a09e4d9bce5580c4f505278e64" integrity sha512-IFsj0R9C7ZdR5cP+ET342q77uSRdtWOlWpih5eC+lu29tIDbNEgDbzgVJ5UFvYHWhxDZ5TFkJafFioO0pPQjCw== dependencies: which "^1.2.10" npm-run-path@^2.0.0: version "2.0.2" resolved "https://registry.yarnpkg.com/npm-run-path/-/npm-run-path-2.0.2.tgz#35a9232dfa35d7067b4cb2ddf2357b1871536c5f" integrity sha1-NakjLfo11wZ7TLLd8jV7GHFTbF8= dependencies: path-key "^2.0.0" npm-which@^3.0.1: version "3.0.1" resolved "https://registry.yarnpkg.com/npm-which/-/npm-which-3.0.1.tgz#9225f26ec3a285c209cae67c3b11a6b4ab7140aa" integrity sha1-kiXybsOihcIJyuZ8OxGmtKtxQKo= dependencies: commander "^2.9.0" npm-path "^2.0.2" which "^1.2.10" npmlog@^4.0.2: version "4.1.2" resolved "https://registry.yarnpkg.com/npmlog/-/npmlog-4.1.2.tgz#08a7f2a8bf734604779a9efa4ad5cc717abb954b" integrity sha512-2uUqazuKlTaSI/dC8AzicUck7+IrEaOnN/e0jd3Xtt1KcGpwx30v50mL7oPyr/h9bL3E4aZccVwpwP+5W9Vjkg== dependencies: are-we-there-yet "~1.1.2" console-control-strings "~1.1.0" gauge "~2.7.3" set-blocking "~2.0.0" number-is-nan@^1.0.0: version "1.0.1" resolved "https://registry.yarnpkg.com/number-is-nan/-/number-is-nan-1.0.1.tgz#097b602b53422a522c1afb8790318336941a011d" integrity sha1-CXtgK1NCKlIsGvuHkDGDNpQaAR0= nyc@^14.1.0: version "14.1.0" resolved "https://registry.yarnpkg.com/nyc/-/nyc-14.1.0.tgz#ae864913a4c5a947bfaebeb66a488bdb1868c9a3" integrity sha512-iy9fEV8Emevz3z/AanIZsoGa8F4U2p0JKevZ/F0sk+/B2r9E6Qn+EPs0bpxEhnAt6UPlTL8mQZIaSJy8sK0ZFw== dependencies: archy "^1.0.0" caching-transform "^3.0.2" convert-source-map "^1.6.0" cp-file "^6.2.0" find-cache-dir "^2.1.0" find-up "^3.0.0" foreground-child "^1.5.6" glob "^7.1.3" istanbul-lib-coverage "^2.0.5" istanbul-lib-hook "^2.0.7" istanbul-lib-instrument "^3.3.0" istanbul-lib-report "^2.0.8" istanbul-lib-source-maps "^3.0.6" istanbul-reports "^2.2.4" js-yaml "^3.13.1" make-dir "^2.1.0" merge-source-map "^1.1.0" resolve-from "^4.0.0" rimraf "^2.6.3" signal-exit "^3.0.2" spawn-wrap "^1.4.2" test-exclude "^5.2.3" uuid "^3.3.2" yargs "^13.2.2" yargs-parser "^13.0.0" oauth-sign@~0.9.0: version "0.9.0" resolved "https://registry.yarnpkg.com/oauth-sign/-/oauth-sign-0.9.0.tgz#47a7b016baa68b5fa0ecf3dee08a85c679ac6455" integrity sha512-fexhUFFPTGV8ybAtSIGbV6gOkSv8UtRbDBnAyLQw4QPKkgNlsH2ByPGtMUqdWkos6YCRmAqViwgZrJc/mRDzZQ== object-assign@^4.0.1, object-assign@^4.1.0, object-assign@^4.1.1: version "4.1.1" resolved "https://registry.yarnpkg.com/object-assign/-/object-assign-4.1.1.tgz#2109adc7965887cfc05cbbd442cac8bfbb360863" integrity sha1-IQmtx5ZYh8/AXLvUQsrIv7s2CGM= object-copy@^0.1.0: version "0.1.0" resolved "https://registry.yarnpkg.com/object-copy/-/object-copy-0.1.0.tgz#7e7d858b781bd7c991a41ba975ed3812754e998c" integrity sha1-fn2Fi3gb18mRpBupde04EnVOmYw= dependencies: copy-descriptor "^0.1.0" define-property "^0.2.5" kind-of "^3.0.3" object-keys@^1.0.12: version "1.1.1" resolved "https://registry.yarnpkg.com/object-keys/-/object-keys-1.1.1.tgz#1c47f272df277f3b1daf061677d9c82e2322c60e" integrity sha512-NuAESUOUMrlIXOfHKzD6bpPu3tYt3xvjNdRIQ+FeT0lNb4K8WR70CaDxhuNguS2XG+GjkyMwOzsN5ZktImfhLA== object-visit@^1.0.0: version "1.0.1" resolved "https://registry.yarnpkg.com/object-visit/-/object-visit-1.0.1.tgz#f79c4493af0c5377b59fe39d395e41042dd045bb" integrity sha1-95xEk68MU3e1n+OdOV5BBC3QRbs= dependencies: isobject "^3.0.0" object.pick@^1.3.0: version "1.3.0" resolved "https://registry.yarnpkg.com/object.pick/-/object.pick-1.3.0.tgz#87a10ac4c1694bd2e1cbf53591a66141fb5dd747" integrity sha1-h6EKxMFpS9Lhy/U1kaZhQftd10c= dependencies: isobject "^3.0.1" once@^1.3.0, once@^1.3.1, once@^1.4.0: version "1.4.0" resolved "https://registry.yarnpkg.com/once/-/once-1.4.0.tgz#583b1aa775961d4b113ac17d9c50baef9dd76bd1" integrity sha1-WDsap3WWHUsROsF9nFC6753Xa9E= dependencies: wrappy "1" onetime@^2.0.0: version "2.0.1" resolved "https://registry.yarnpkg.com/onetime/-/onetime-2.0.1.tgz#067428230fd67443b2794b22bba528b6867962d4" integrity sha1-BnQoIw/WdEOyeUsiu6UotoZ5YtQ= dependencies: mimic-fn "^1.0.0" opener@^1.5.1: version "1.5.1" resolved "https://registry.yarnpkg.com/opener/-/opener-1.5.1.tgz#6d2f0e77f1a0af0032aca716c2c1fbb8e7e8abed" integrity sha512-goYSy5c2UXE4Ra1xixabeVh1guIX/ZV/YokJksb6q2lubWu6UbvPQ20p542/sFIll1nl8JnCyK9oBaOcCWXwvA== optimist@^0.6.1: version "0.6.1" resolved "https://registry.yarnpkg.com/optimist/-/optimist-0.6.1.tgz#da3ea74686fa21a19a111c326e90eb15a0196686" integrity sha1-2j6nRob6IaGaERwybpDrFaAZZoY= dependencies: minimist "~0.0.1" wordwrap "~0.0.2" optionator@^0.8.2: version "0.8.2" resolved "https://registry.yarnpkg.com/optionator/-/optionator-0.8.2.tgz#364c5e409d3f4d6301d6c0b4c05bba50180aeb64" integrity sha1-NkxeQJ0/TWMB1sC0wFu6UBgK62Q= dependencies: deep-is "~0.1.3" fast-levenshtein "~2.0.4" levn "~0.3.0" prelude-ls "~1.1.2" type-check "~0.3.2" wordwrap "~1.0.0" os-homedir@^1.0.0, os-homedir@^1.0.1: version "1.0.2" resolved "https://registry.yarnpkg.com/os-homedir/-/os-homedir-1.0.2.tgz#ffbc4988336e0e833de0c168c7ef152121aa7fb3" integrity sha1-/7xJiDNuDoM94MFox+8VISGqf7M= os-locale@^3.1.0: version "3.1.0" resolved "https://registry.yarnpkg.com/os-locale/-/os-locale-3.1.0.tgz#a802a6ee17f24c10483ab9935719cef4ed16bf1a" integrity sha512-Z8l3R4wYWM40/52Z+S265okfFj8Kt2cC2MKY+xNi3kFs+XGI7WXu/I309QQQYbRW4ijiZ+yxs9pqEhJh0DqW3Q== dependencies: execa "^1.0.0" lcid "^2.0.0" mem "^4.0.0" os-tmpdir@^1.0.0, os-tmpdir@^1.0.1, os-tmpdir@~1.0.2: version "1.0.2" resolved "https://registry.yarnpkg.com/os-tmpdir/-/os-tmpdir-1.0.2.tgz#bbe67406c79aa85c5cfec766fe5734555dfa1274" integrity sha1-u+Z0BseaqFxc/sdm/lc0VV36EnQ= osenv@^0.1.4: version "0.1.5" resolved "https://registry.yarnpkg.com/osenv/-/osenv-0.1.5.tgz#85cdfafaeb28e8677f416e287592b5f3f49ea410" integrity sha512-0CWcCECdMVc2Rw3U5w9ZjqX6ga6ubk1xDVKxtBQPK7wis/0F2r9T6k4ydGYhecl7YUBxBVxhL5oisPsNxAPe2g== dependencies: os-homedir "^1.0.0" os-tmpdir "^1.0.0" output-file-sync@^2.0.0: version "2.0.1" resolved "https://registry.yarnpkg.com/output-file-sync/-/output-file-sync-2.0.1.tgz#f53118282f5f553c2799541792b723a4c71430c0" integrity sha512-mDho4qm7WgIXIGf4eYU1RHN2UU5tPfVYVSRwDJw0uTmj35DQUt/eNp19N7v6T3SrR0ESTEf2up2CGO73qI35zQ== dependencies: graceful-fs "^4.1.11" is-plain-obj "^1.1.0" mkdirp "^0.5.1" own-or-env@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/own-or-env/-/own-or-env-1.0.1.tgz#54ce601d3bf78236c5c65633aa1c8ec03f8007e4" integrity sha512-y8qULRbRAlL6x2+M0vIe7jJbJx/kmUTzYonRAa2ayesR2qWLswninkVyeJe4x3IEXhdgoNodzjQRKAoEs6Fmrw== dependencies: own-or "^1.0.0" own-or@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/own-or/-/own-or-1.0.0.tgz#4e877fbeda9a2ec8000fbc0bcae39645ee8bf8dc" integrity sha1-Tod/vtqaLsgAD7wLyuOWRe6L+Nw= p-defer@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/p-defer/-/p-defer-1.0.0.tgz#9f6eb182f6c9aa8cd743004a7d4f96b196b0fb0c" integrity sha1-n26xgvbJqozXQwBKfU+WsZaw+ww= p-finally@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/p-finally/-/p-finally-1.0.0.tgz#3fbcfb15b899a44123b34b6dcc18b724336a2cae" integrity sha1-P7z7FbiZpEEjs0ttzBi3JDNqLK4= p-is-promise@^2.0.0: version "2.1.0" resolved "https://registry.yarnpkg.com/p-is-promise/-/p-is-promise-2.1.0.tgz#918cebaea248a62cf7ffab8e3bca8c5f882fc42e" integrity sha512-Y3W0wlRPK8ZMRbNq97l4M5otioeA5lm1z7bkNkxCka8HSPjR0xRWmpCmc9utiaLP9Jb1eD8BgeIxTW4AIF45Pg== p-limit@^1.1.0: version "1.3.0" resolved "https://registry.yarnpkg.com/p-limit/-/p-limit-1.3.0.tgz#b86bd5f0c25690911c7590fcbfc2010d54b3ccb8" integrity sha512-vvcXsLAJ9Dr5rQOPk7toZQZJApBl2K4J6dANSsEuh6QI41JYcsS/qhTGa9ErIUUgK3WNQoJYvylxvjqmiqEA9Q== dependencies: p-try "^1.0.0" p-limit@^2.0.0: version "2.1.0" resolved "https://registry.yarnpkg.com/p-limit/-/p-limit-2.1.0.tgz#1d5a0d20fb12707c758a655f6bbc4386b5930d68" integrity sha512-NhURkNcrVB+8hNfLuysU8enY5xn2KXphsHBaC2YmRNTZRc7RWusw6apSpdEj3jo4CMb6W9nrF6tTnsJsJeyu6g== dependencies: p-try "^2.0.0" p-locate@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/p-locate/-/p-locate-2.0.0.tgz#20a0103b222a70c8fd39cc2e580680f3dde5ec43" integrity sha1-IKAQOyIqcMj9OcwuWAaA893l7EM= dependencies: p-limit "^1.1.0" p-locate@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/p-locate/-/p-locate-3.0.0.tgz#322d69a05c0264b25997d9f40cd8a891ab0064a4" integrity sha512-x+12w/To+4GFfgJhBEpiDcLozRJGegY+Ei7/z0tSLkMmxGZNybVMSfWj9aJn8Z5Fc7dBUNJOOVgPv2H7IwulSQ== dependencies: p-limit "^2.0.0" p-map@^1.1.1: version "1.2.0" resolved "https://registry.yarnpkg.com/p-map/-/p-map-1.2.0.tgz#e4e94f311eabbc8633a1e79908165fca26241b6b" integrity sha512-r6zKACMNhjPJMTl8KcFH4li//gkrXWfbD6feV8l6doRHlzljFWGJ2AP6iKaCJXyZmAUMOPtvbW7EXkbWO/pLEA== p-map@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/p-map/-/p-map-2.0.0.tgz#be18c5a5adeb8e156460651421aceca56c213a50" integrity sha512-GO107XdrSUmtHxVoi60qc9tUl/KkNKm+X2CF4P9amalpGxv5YqVPJNfSb0wcA+syCopkZvYYIzW8OVTQW59x/w== p-try@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/p-try/-/p-try-1.0.0.tgz#cbc79cdbaf8fd4228e13f621f2b1a237c1b207b3" integrity sha1-y8ec26+P1CKOE/Yh8rGiN8GyB7M= p-try@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/p-try/-/p-try-2.0.0.tgz#85080bb87c64688fa47996fe8f7dfbe8211760b1" integrity sha512-hMp0onDKIajHfIkdRk3P4CdCmErkYAxxDtP3Wx/4nZ3aGlau2VKh3mZpcuFkH27WQkL/3WBCPOktzA9ZOAnMQQ== package-hash@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/package-hash/-/package-hash-3.0.0.tgz#50183f2d36c9e3e528ea0a8605dff57ce976f88e" integrity sha512-lOtmukMDVvtkL84rJHI7dpTYq+0rli8N2wlnqUcBuDWCfVhRUfOmnR9SsoHFMLpACvEV60dX7rd0rFaYDZI+FA== dependencies: graceful-fs "^4.1.15" hasha "^3.0.0" lodash.flattendeep "^4.4.0" release-zalgo "^1.0.0" parent-module@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/parent-module/-/parent-module-1.0.0.tgz#df250bdc5391f4a085fb589dad761f5ad6b865b5" integrity sha512-8Mf5juOMmiE4FcmzYc4IaiS9L3+9paz2KOiXzkRviCP6aDmN49Hz6EMWz0lGNp9pX80GvvAuLADtyGfW/Em3TA== dependencies: callsites "^3.0.0" parse-json@^2.2.0: version "2.2.0" resolved "https://registry.yarnpkg.com/parse-json/-/parse-json-2.2.0.tgz#f480f40434ef80741f8469099f8dea18f55a4dc9" integrity sha1-9ID0BDTvgHQfhGkJn43qGPVaTck= dependencies: error-ex "^1.2.0" parse-json@^4.0.0: version "4.0.0" resolved "https://registry.yarnpkg.com/parse-json/-/parse-json-4.0.0.tgz#be35f5425be1f7f6c747184f98a788cb99477ee0" integrity sha1-vjX1Qlvh9/bHRxhPmKeIy5lHfuA= dependencies: error-ex "^1.3.1" json-parse-better-errors "^1.0.1" pascalcase@^0.1.1: version "0.1.1" resolved "https://registry.yarnpkg.com/pascalcase/-/pascalcase-0.1.1.tgz#b363e55e8006ca6fe21784d2db22bd15d7917f14" integrity sha1-s2PlXoAGym/iF4TS2yK9FdeRfxQ= path-dirname@^1.0.0: version "1.0.2" resolved "https://registry.yarnpkg.com/path-dirname/-/path-dirname-1.0.2.tgz#cc33d24d525e099a5388c0336c6e32b9160609e0" integrity sha1-zDPSTVJeCZpTiMAzbG4yuRYGCeA= path-exists@^2.0.0: version "2.1.0" resolved "https://registry.yarnpkg.com/path-exists/-/path-exists-2.1.0.tgz#0feb6c64f0fc518d9a754dd5efb62c7022761f4b" integrity sha1-D+tsZPD8UY2adU3V77YscCJ2H0s= dependencies: pinkie-promise "^2.0.0" path-exists@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/path-exists/-/path-exists-3.0.0.tgz#ce0ebeaa5f78cb18925ea7d810d7b59b010fd515" integrity sha1-zg6+ql94yxiSXqfYENe1mwEP1RU= path-is-absolute@^1.0.0, path-is-absolute@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/path-is-absolute/-/path-is-absolute-1.0.1.tgz#174b9268735534ffbc7ace6bf53a5a9e1b5c5f5f" integrity sha1-F0uSaHNVNP+8es5r9TpanhtcX18= path-is-inside@^1.0.1, path-is-inside@^1.0.2: version "1.0.2" resolved "https://registry.yarnpkg.com/path-is-inside/-/path-is-inside-1.0.2.tgz#365417dede44430d1c11af61027facf074bdfc53" integrity sha1-NlQX3t5EQw0cEa9hAn+s8HS9/FM= path-key@^2.0.0, path-key@^2.0.1: version "2.0.1" resolved "https://registry.yarnpkg.com/path-key/-/path-key-2.0.1.tgz#411cadb574c5a140d3a4b1910d40d80cc9f40b40" integrity sha1-QRyttXTFoUDTpLGRDUDYDMn0C0A= path-parse@^1.0.6: version "1.0.6" resolved "https://registry.yarnpkg.com/path-parse/-/path-parse-1.0.6.tgz#d62dbb5679405d72c4737ec58600e9ddcf06d24c" integrity sha512-GSmOT2EbHrINBf9SR7CDELwlJ8AENk3Qn7OikK4nFYAu3Ote2+JYNVvkpAEQm3/TLNEJFD/xZJjzyxg3KBWOzw== path-type@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/path-type/-/path-type-2.0.0.tgz#f012ccb8415b7096fc2daa1054c3d72389594c73" integrity sha1-8BLMuEFbcJb8LaoQVMPXI4lZTHM= dependencies: pify "^2.0.0" path-type@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/path-type/-/path-type-3.0.0.tgz#cef31dc8e0a1a3bb0d105c0cd97cf3bf47f4e36f" integrity sha512-T2ZUsdZFHgA3u4e5PfPbjd7HDDpxPnQb5jN0SrDsjNSuVXHJqtwTnWqG0B1jZrgmJ/7lj1EmVIByWt1gxGkWvg== dependencies: pify "^3.0.0" performance-now@^2.1.0: version "2.1.0" resolved "https://registry.yarnpkg.com/performance-now/-/performance-now-2.1.0.tgz#6309f4e0e5fa913ec1c69307ae364b4b377c9e7b" integrity sha1-Ywn04OX6kT7BxpMHrjZLSzd8nns= pify@^2.0.0: version "2.3.0" resolved "https://registry.yarnpkg.com/pify/-/pify-2.3.0.tgz#ed141a6ac043a849ea588498e7dca8b15330e90c" integrity sha1-7RQaasBDqEnqWISY59yosVMw6Qw= pify@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/pify/-/pify-3.0.0.tgz#e5a4acd2c101fdf3d9a4d07f0dbc4db49dd28176" integrity sha1-5aSs0sEB/fPZpNB/DbxNtJ3SgXY= pify@^4.0.1: version "4.0.1" resolved "https://registry.yarnpkg.com/pify/-/pify-4.0.1.tgz#4b2cd25c50d598735c50292224fd8c6df41e3231" integrity sha512-uB80kBFb/tfd68bVleG9T5GGsGPjJrLAUpR5PZIrhBnIaRTQRjqdJSsIKkOP6OAIFbj7GOrcudc5pNjZ+geV2g== pinkie-promise@^2.0.0: version "2.0.1" resolved "https://registry.yarnpkg.com/pinkie-promise/-/pinkie-promise-2.0.1.tgz#2135d6dfa7a358c069ac9b178776288228450ffa" integrity sha1-ITXW36ejWMBprJsXh3YogihFD/o= dependencies: pinkie "^2.0.0" pinkie@^2.0.0: version "2.0.4" resolved "https://registry.yarnpkg.com/pinkie/-/pinkie-2.0.4.tgz#72556b80cfa0d48a974e80e77248e80ed4f7f870" integrity sha1-clVrgM+g1IqXToDnckjoDtT3+HA= pkg-dir@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/pkg-dir/-/pkg-dir-1.0.0.tgz#7a4b508a8d5bb2d629d447056ff4e9c9314cf3d4" integrity sha1-ektQio1bstYp1EcFb/TpyTFM89Q= dependencies: find-up "^1.0.0" pkg-dir@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/pkg-dir/-/pkg-dir-2.0.0.tgz#f6d5d1109e19d63edf428e0bd57e12777615334b" integrity sha1-9tXREJ4Z1j7fQo4L1X4Sd3YVM0s= dependencies: find-up "^2.1.0" pkg-dir@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/pkg-dir/-/pkg-dir-3.0.0.tgz#2749020f239ed990881b1f71210d51eb6523bea3" integrity sha512-/E57AYkoeQ25qkxMj5PBOVgF8Kiu/h7cYS30Z5+R7WaiCCBfLq58ZI/dSeaEKb9WVJV5n/03QwrN3IeWIFllvw== dependencies: find-up "^3.0.0" pkg-dir@^4.1.0: version "4.1.0" resolved "https://registry.yarnpkg.com/pkg-dir/-/pkg-dir-4.1.0.tgz#aaeb91c0d3b9c4f74a44ad849f4de34781ae01de" integrity sha512-55k9QN4saZ8q518lE6EFgYiu95u3BWkSajCifhdQjvLvmr8IpnRbhI+UGpWJQfa0KzDguHeeWT1ccO1PmkOi3A== dependencies: find-up "^3.0.0" please-upgrade-node@^3.0.2, please-upgrade-node@^3.1.1: version "3.1.1" resolved "https://registry.yarnpkg.com/please-upgrade-node/-/please-upgrade-node-3.1.1.tgz#ed320051dfcc5024fae696712c8288993595e8ac" integrity sha512-KY1uHnQ2NlQHqIJQpnh/i54rKkuxCEBx+voJIS/Mvb+L2iYd2NMotwduhKTMjfC1uKoX3VXOxLjIYG66dfJTVQ== dependencies: semver-compare "^1.0.0" posix-character-classes@^0.1.0: version "0.1.1" resolved "https://registry.yarnpkg.com/posix-character-classes/-/posix-character-classes-0.1.1.tgz#01eac0fe3b5af71a2a6c02feabb8c1fef7e00eab" integrity sha1-AerA/jta9xoqbAL+q7jB/vfgDqs= prelude-ls@~1.1.2: version "1.1.2" resolved "https://registry.yarnpkg.com/prelude-ls/-/prelude-ls-1.1.2.tgz#21932a549f5e52ffd9a827f570e04be62a97da54" integrity sha1-IZMqVJ9eUv/ZqCf1cOBL5iqX2lQ= prettier-linter-helpers@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/prettier-linter-helpers/-/prettier-linter-helpers-1.0.0.tgz#d23d41fe1375646de2d0104d3454a3008802cf7b" integrity sha512-GbK2cP9nraSSUF9N2XwUwqfzlAFlMNYYl+ShE/V+H8a9uNl/oUqB1w2EL54Jh0OlyRSd8RfWYJ3coVS4TROP2w== dependencies: fast-diff "^1.1.2" prettier@^1.16.4: version "1.17.0" resolved "https://registry.yarnpkg.com/prettier/-/prettier-1.17.0.tgz#53b303676eed22cc14a9f0cec09b477b3026c008" integrity sha512-sXe5lSt2WQlCbydGETgfm1YBShgOX4HxQkFPvbxkcwgDvGDeqVau8h+12+lmSVlP3rHPz0oavfddSZg/q+Szjw== private@^0.1.8: version "0.1.8" resolved "https://registry.yarnpkg.com/private/-/private-0.1.8.tgz#2381edb3689f7a53d653190060fcf822d2f368ff" integrity sha512-VvivMrbvd2nKkiG38qjULzlc+4Vx4wm/whI9pQD35YrARNnhxeiRktSOhSukRLFNlzg6Br/cJPet5J/u19r/mg== process-nextick-args@~2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/process-nextick-args/-/process-nextick-args-2.0.0.tgz#a37d732f4271b4ab1ad070d35508e8290788ffaa" integrity sha512-MtEC1TqN0EU5nephaJ4rAtThHtC86dNN9qCuEhtshvpVBkAW5ZO7BASN9REnF9eoXGcRub+pFuKEpOHE+HbEMw== process@^0.10.0: version "0.10.1" resolved "https://registry.yarnpkg.com/process/-/process-0.10.1.tgz#842457cc51cfed72dc775afeeafb8c6034372725" integrity sha1-hCRXzFHP7XLcd1r+6vuMYDQ3JyU= progress@^2.0.0: version "2.0.3" resolved "https://registry.yarnpkg.com/progress/-/progress-2.0.3.tgz#7e8cf8d8f5b8f239c1bc68beb4eb78567d572ef8" integrity sha512-7PiHtLll5LdnKIMw100I+8xJXR5gW2QwWYkT6iJva0bXitZKa/XMrSbdmg3r2Xnaidz9Qumd0VPaMrZlF9V9sA== prop-types@^15.6.2: version "15.7.2" resolved "https://registry.yarnpkg.com/prop-types/-/prop-types-15.7.2.tgz#52c41e75b8c87e72b9d9360e0206b99dcbffa6c5" integrity sha512-8QQikdH7//R2vurIJSutZ1smHYTcLpRWEOlHnzcWHmBYrOGUysKwSsrC89BCiFj3CbrfJ/nXFdJepOVrY1GCHQ== dependencies: loose-envify "^1.4.0" object-assign "^4.1.1" react-is "^16.8.1" property-expr@^1.5.0: version "1.5.1" resolved "https://registry.yarnpkg.com/property-expr/-/property-expr-1.5.1.tgz#22e8706894a0c8e28d58735804f6ba3a3673314f" integrity sha512-CGuc0VUTGthpJXL36ydB6jnbyOf/rAHFvmVrJlH+Rg0DqqLFQGAP6hIaxD/G0OAmBJPhXDHuEJigrp0e0wFV6g== pseudomap@^1.0.2: version "1.0.2" resolved "https://registry.yarnpkg.com/pseudomap/-/pseudomap-1.0.2.tgz#f052a28da70e618917ef0a8ac34c1ae5a68286b3" integrity sha1-8FKijacOYYkX7wqKw0wa5aaChrM= psl@^1.1.24: version "1.1.31" resolved "https://registry.yarnpkg.com/psl/-/psl-1.1.31.tgz#e9aa86d0101b5b105cbe93ac6b784cd547276184" integrity sha512-/6pt4+C+T+wZUieKR620OpzN/LlnNKuWjy1iFLQ/UG35JqHlR/89MP1d96dUfkf6Dne3TuLQzOYEYshJ+Hx8mw== pump@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/pump/-/pump-3.0.0.tgz#b4a2116815bde2f4e1ea602354e8c75565107a64" integrity sha512-LwZy+p3SFs1Pytd/jYct4wpv49HiYCqd9Rlc5ZVdk0V+8Yzv6jR5Blk3TRmPL1ft69TxP0IMZGJ+WPFU2BFhww== dependencies: end-of-stream "^1.1.0" once "^1.3.1" punycode@^1.3.2, punycode@^1.4.1: version "1.4.1" resolved "https://registry.yarnpkg.com/punycode/-/punycode-1.4.1.tgz#c0d5a63b2718800ad8e1eb0fa5269c84dd41845e" integrity sha1-wNWmOycYgArY4esPpSachN1BhF4= punycode@^2.0.0, punycode@^2.1.0: version "2.1.1" resolved "https://registry.yarnpkg.com/punycode/-/punycode-2.1.1.tgz#b58b010ac40c22c5657616c8d2c2c02c7bf479ec" integrity sha512-XRsRjdf+j5ml+y/6GKHPZbrF/8p2Yga0JPtdqTIY2Xe5ohJPD9saDJJLPvp9+NSBprVvevdXZybnj2cv8OEd0A== qs@~6.5.2: version "6.5.2" resolved "https://registry.yarnpkg.com/qs/-/qs-6.5.2.tgz#cb3ae806e8740444584ef154ce8ee98d403f3e36" integrity sha512-N5ZAX4/LxJmF+7wN74pUD6qAh9/wnvdQcjq9TZjevvXzSUo7bfmw91saqMjzGS2xq91/odN2dW/WOl7qQHNDGA== rc@^1.2.7: version "1.2.8" resolved "https://registry.yarnpkg.com/rc/-/rc-1.2.8.tgz#cd924bf5200a075b83c188cd6b9e211b7fc0d3ed" integrity sha512-y3bGgqKj3QBdxLbLkomlohkvsA8gdAiUQlSBJnBhfn+BPxg4bc62d8TcBW15wavDfgexCgccckhcZvywyQYPOw== dependencies: deep-extend "^0.6.0" ini "~1.3.0" minimist "^1.2.0" strip-json-comments "~2.0.1" react-is@^16.8.1: version "16.8.6" resolved "https://registry.yarnpkg.com/react-is/-/react-is-16.8.6.tgz#5bbc1e2d29141c9fbdfed456343fe2bc430a6a16" integrity sha512-aUk3bHfZ2bRSVFFbbeVS4i+lNPZr3/WM5jT2J5omUVV1zzcs1nAaf3l51ctA5FFvCRbhrH0bdAsRRQddFJZPtA== react-reconciler@^0.20.0: version "0.20.4" resolved "https://registry.yarnpkg.com/react-reconciler/-/react-reconciler-0.20.4.tgz#3da6a95841592f849cb4edd3d38676c86fd920b2" integrity sha512-kxERc4H32zV2lXMg/iMiwQHOtyqf15qojvkcZ5Ja2CPkjVohHw9k70pdDBwrnQhLVetUJBSYyqU3yqrlVTOajA== dependencies: loose-envify "^1.1.0" object-assign "^4.1.1" prop-types "^15.6.2" scheduler "^0.13.6" react@^16.8.6: version "16.8.6" resolved "https://registry.yarnpkg.com/react/-/react-16.8.6.tgz#ad6c3a9614fd3a4e9ef51117f54d888da01f2bbe" integrity sha512-pC0uMkhLaHm11ZSJULfOBqV4tIZkx87ZLvbbQYunNixAAvjnC+snJCg0XQXn9VIsttVsbZP/H/ewzgsd5fxKXw== dependencies: loose-envify "^1.1.0" object-assign "^4.1.1" prop-types "^15.6.2" scheduler "^0.13.6" read-pkg-up@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/read-pkg-up/-/read-pkg-up-2.0.0.tgz#6b72a8048984e0c41e79510fd5e9fa99b3b549be" integrity sha1-a3KoBImE4MQeeVEP1en6mbO1Sb4= dependencies: find-up "^2.0.0" read-pkg "^2.0.0" read-pkg-up@^4.0.0: version "4.0.0" resolved "https://registry.yarnpkg.com/read-pkg-up/-/read-pkg-up-4.0.0.tgz#1b221c6088ba7799601c808f91161c66e58f8978" integrity sha512-6etQSH7nJGsK0RbG/2TeDzZFa8shjQ1um+SwQQ5cwKy0dhSXdOncEhb1CPpvQG4h7FyOV6EB6YlV0yJvZQNAkA== dependencies: find-up "^3.0.0" read-pkg "^3.0.0" read-pkg-up@^5.0.0: version "5.0.0" resolved "https://registry.yarnpkg.com/read-pkg-up/-/read-pkg-up-5.0.0.tgz#b6a6741cb144ed3610554f40162aa07a6db621b8" integrity sha512-XBQjqOBtTzyol2CpsQOw8LHV0XbDZVG7xMMjmXAJomlVY03WOBRmYgDJETlvcg0H63AJvPRwT7GFi5rvOzUOKg== dependencies: find-up "^3.0.0" read-pkg "^5.0.0" read-pkg@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/read-pkg/-/read-pkg-2.0.0.tgz#8ef1c0623c6a6db0dc6713c4bfac46332b2368f8" integrity sha1-jvHAYjxqbbDcZxPEv6xGMysjaPg= dependencies: load-json-file "^2.0.0" normalize-package-data "^2.3.2" path-type "^2.0.0" read-pkg@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/read-pkg/-/read-pkg-3.0.0.tgz#9cbc686978fee65d16c00e2b19c237fcf6e38389" integrity sha1-nLxoaXj+5l0WwA4rGcI3/Pbjg4k= dependencies: load-json-file "^4.0.0" normalize-package-data "^2.3.2" path-type "^3.0.0" read-pkg@^5.0.0: version "5.1.1" resolved "https://registry.yarnpkg.com/read-pkg/-/read-pkg-5.1.1.tgz#5cf234dde7a405c90c88a519ab73c467e9cb83f5" integrity sha512-dFcTLQi6BZ+aFUaICg7er+/usEoqFdQxiEBsEMNGoipenihtxxtdrQuBXvyANCEI8VuUIVYFgeHGx9sLLvim4w== dependencies: "@types/normalize-package-data" "^2.4.0" normalize-package-data "^2.5.0" parse-json "^4.0.0" type-fest "^0.4.1" readable-stream@^2.0.2, readable-stream@^2.0.6, readable-stream@^2.1.5: version "2.3.6" resolved "https://registry.yarnpkg.com/readable-stream/-/readable-stream-2.3.6.tgz#b11c27d88b8ff1fbe070643cf94b0c79ae1b0aaf" integrity sha512-tQtKA9WIAhBF3+VLAseyMqZeBjW0AHJoxOtYqSUZNJxauErmLbVm2FW1y+J/YA9dUrAC39ITejlZWhVIwawkKw== dependencies: core-util-is "~1.0.0" inherits "~2.0.3" isarray "~1.0.0" process-nextick-args "~2.0.0" safe-buffer "~5.1.1" string_decoder "~1.1.1" util-deprecate "~1.0.1" readdirp@^2.2.1: version "2.2.1" resolved "https://registry.yarnpkg.com/readdirp/-/readdirp-2.2.1.tgz#0e87622a3325aa33e892285caf8b4e846529a525" integrity sha512-1JU/8q+VgFZyxwrJ+SVIOsh+KywWGpds3NTqikiKpDMZWScmAYyKIgqkO+ARvNWJfXeXR1zxz7aHF4u4CyH6vQ== dependencies: graceful-fs "^4.1.11" micromatch "^3.1.10" readable-stream "^2.0.2" redeyed@~2.1.0: version "2.1.1" resolved "https://registry.yarnpkg.com/redeyed/-/redeyed-2.1.1.tgz#8984b5815d99cb220469c99eeeffe38913e6cc0b" integrity sha1-iYS1gV2ZyyIEacme7v/jiRPmzAs= dependencies: esprima "~4.0.0" regenerator-runtime@^0.11.0: version "0.11.1" resolved "https://registry.yarnpkg.com/regenerator-runtime/-/regenerator-runtime-0.11.1.tgz#be05ad7f9bf7d22e056f9726cee5017fbf19e2e9" integrity sha512-MguG95oij0fC3QV3URf4V2SDYGJhJnJGqvIIgdECeODCT98wSWDAJ94SSuVpYQUoTcGUIL6L4yNB7j1DFFHSBg== regenerator-runtime@^0.13.2: version "0.13.2" resolved "https://registry.yarnpkg.com/regenerator-runtime/-/regenerator-runtime-0.13.2.tgz#32e59c9a6fb9b1a4aff09b4930ca2d4477343447" integrity sha512-S/TQAZJO+D3m9xeN1WTI8dLKBBiRgXBlTJvbWjCThHWZj9EvHK70Ff50/tYj2J/fvBY6JtFVwRuazHN2E7M9BA== regex-not@^1.0.0, regex-not@^1.0.2: version "1.0.2" resolved "https://registry.yarnpkg.com/regex-not/-/regex-not-1.0.2.tgz#1f4ece27e00b0b65e0247a6810e6a85d83a5752c" integrity sha512-J6SDjUgDxQj5NusnOtdFxDwN/+HWykR8GELwctJ7mdqhcyy1xEc4SRFHUXvxTp661YaVKAjfRLZ9cCqS6tn32A== dependencies: extend-shallow "^3.0.2" safe-regex "^1.1.0" regexpp@^2.0.1: version "2.0.1" resolved "https://registry.yarnpkg.com/regexpp/-/regexpp-2.0.1.tgz#8d19d31cf632482b589049f8281f93dbcba4d07f" integrity sha512-lv0M6+TkDVniA3aD1Eg0DVpfU/booSu7Eev3TDO/mZKHBfVjgCGTV4t4buppESEYDtkArYFOxTJWv6S5C+iaNw== release-zalgo@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/release-zalgo/-/release-zalgo-1.0.0.tgz#09700b7e5074329739330e535c5a90fb67851730" integrity sha1-CXALflB0Mpc5Mw5TXFqQ+2eFFzA= dependencies: es6-error "^4.0.1" remove-trailing-separator@^1.0.1: version "1.1.0" resolved "https://registry.yarnpkg.com/remove-trailing-separator/-/remove-trailing-separator-1.1.0.tgz#c24bce2a283adad5bc3f58e0d48249b92379d8ef" integrity sha1-wkvOKig62tW8P1jg1IJJuSN52O8= repeat-element@^1.1.2: version "1.1.3" resolved "https://registry.yarnpkg.com/repeat-element/-/repeat-element-1.1.3.tgz#782e0d825c0c5a3bb39731f84efee6b742e6b1ce" integrity sha512-ahGq0ZnV5m5XtZLMb+vP76kcAM5nkLqk0lpqAuojSKGgQtn4eRi4ZZGm2olo2zKFH+sMsWaqOCW1dqAnOru72g== repeat-string@^1.6.1: version "1.6.1" resolved "https://registry.yarnpkg.com/repeat-string/-/repeat-string-1.6.1.tgz#8dcae470e1c88abc2d600fff4a776286da75e637" integrity sha1-jcrkcOHIirwtYA//Sndihtp15jc= repeating@^2.0.0: version "2.0.1" resolved "https://registry.yarnpkg.com/repeating/-/repeating-2.0.1.tgz#5214c53a926d3552707527fbab415dbc08d06dda" integrity sha1-UhTFOpJtNVJwdSf7q0FdvAjQbdo= dependencies: is-finite "^1.0.0" request@^2.86.0: version "2.88.0" resolved "https://registry.yarnpkg.com/request/-/request-2.88.0.tgz#9c2fca4f7d35b592efe57c7f0a55e81052124fef" integrity sha512-NAqBSrijGLZdM0WZNsInLJpkJokL72XYjUpnB0iwsRgxh7dB6COrHnTBNwN0E+lHDAJzu7kLAkDeY08z2/A0hg== dependencies: aws-sign2 "~0.7.0" aws4 "^1.8.0" caseless "~0.12.0" combined-stream "~1.0.6" extend "~3.0.2" forever-agent "~0.6.1" form-data "~2.3.2" har-validator "~5.1.0" http-signature "~1.2.0" is-typedarray "~1.0.0" isstream "~0.1.2" json-stringify-safe "~5.0.1" mime-types "~2.1.19" oauth-sign "~0.9.0" performance-now "^2.1.0" qs "~6.5.2" safe-buffer "^5.1.2" tough-cookie "~2.4.3" tunnel-agent "^0.6.0" uuid "^3.3.2" require-directory@^2.1.1: version "2.1.1" resolved "https://registry.yarnpkg.com/require-directory/-/require-directory-2.1.1.tgz#8c64ad5fd30dab1c976e2344ffe7f792a6a6df42" integrity sha1-jGStX9MNqxyXbiNE/+f3kqam30I= require-main-filename@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/require-main-filename/-/require-main-filename-2.0.0.tgz#d0b329ecc7cc0f61649f62215be69af54aa8989b" integrity sha512-NKN5kMDylKuldxYLSUfrbo5Tuzh4hd+2E8NPPX02mZtn1VuREQToYe/ZdlJy+J3uCpfaiGF05e7B8W0iXbQHmg== resolve-from@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/resolve-from/-/resolve-from-3.0.0.tgz#b22c7af7d9d6881bc8b6e653335eebcb0a188748" integrity sha1-six699nWiBvItuZTM17rywoYh0g= resolve-from@^4.0.0: version "4.0.0" resolved "https://registry.yarnpkg.com/resolve-from/-/resolve-from-4.0.0.tgz#4abcd852ad32dd7baabfe9b40e00a36db5f392e6" integrity sha512-pb/MYmXstAkysRFx8piNI1tGFNQIFA3vkE3Gq4EuA1dF6gHp/+vgZqsCGJapvy8N3Q+4o7FwvquPJcnZ7RYy4g== resolve-url@^0.2.1: version "0.2.1" resolved "https://registry.yarnpkg.com/resolve-url/-/resolve-url-0.2.1.tgz#2c637fe77c893afd2a663fe21aa9080068e2052a" integrity sha1-LGN/53yJOv0qZj/iGqkIAGjiBSo= resolve@^1.10.0, resolve@^1.10.1: version "1.10.1" resolved "https://registry.yarnpkg.com/resolve/-/resolve-1.10.1.tgz#664842ac960795bbe758221cdccda61fb64b5f18" integrity sha512-KuIe4mf++td/eFb6wkaPbMDnP6kObCaEtIDuHOUED6MNUo4K670KZUHuuvYPZDxNF0WVLw49n06M2m2dXphEzA== dependencies: path-parse "^1.0.6" resolve@^1.3.2, resolve@^1.5.0, resolve@^1.6.0: version "1.9.0" resolved "https://registry.yarnpkg.com/resolve/-/resolve-1.9.0.tgz#a14c6fdfa8f92a7df1d996cb7105fa744658ea06" integrity sha512-TZNye00tI67lwYvzxCxHGjwTNlUV70io54/Ed4j6PscB8xVfuBJpRenI/o6dVk0cY0PYTY27AgCoGGxRnYuItQ== dependencies: path-parse "^1.0.6" restore-cursor@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/restore-cursor/-/restore-cursor-2.0.0.tgz#9f7ee287f82fd326d4fd162923d62129eee0dfaf" integrity sha1-n37ih/gv0ybU/RYpI9YhKe7g368= dependencies: onetime "^2.0.0" signal-exit "^3.0.2" ret@~0.1.10: version "0.1.15" resolved "https://registry.yarnpkg.com/ret/-/ret-0.1.15.tgz#b8a4825d5bdb1fc3f6f53c2bc33f81388681c7bc" integrity sha512-TTlYpa+OL+vMMNG24xSlQGEJ3B/RzEfUlLct7b5G/ytav+wPrplCpVMFuwzXbkecJrb6IYo1iFb0S9v37754mg== rimraf@2.6.3, rimraf@^2.2.8, rimraf@^2.6.1, rimraf@^2.6.2, rimraf@^2.6.3: version "2.6.3" resolved "https://registry.yarnpkg.com/rimraf/-/rimraf-2.6.3.tgz#b2d104fe0d8fb27cf9e0a1cda8262dd3833c6cab" integrity sha512-mwqeW5XsA2qAejG46gYdENaxXjx9onRNCfn7L0duuP4hCuTIi/QO7PDK07KJfp1d+izWPrzEJDcSqBa0OZQriA== dependencies: glob "^7.1.3" run-async@^2.2.0: version "2.3.0" resolved "https://registry.yarnpkg.com/run-async/-/run-async-2.3.0.tgz#0371ab4ae0bdd720d4166d7dfda64ff7a445a6c0" integrity sha1-A3GrSuC91yDUFm19/aZP96RFpsA= dependencies: is-promise "^2.1.0" run-node@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/run-node/-/run-node-1.0.0.tgz#46b50b946a2aa2d4947ae1d886e9856fd9cabe5e" integrity sha512-kc120TBlQ3mih1LSzdAJXo4xn/GWS2ec0l3S+syHDXP9uRr0JAT8Qd3mdMuyjqCzeZktgP3try92cEgf9Nks8A== rxjs@^6.3.3: version "6.3.3" resolved "https://registry.yarnpkg.com/rxjs/-/rxjs-6.3.3.tgz#3c6a7fa420e844a81390fb1158a9ec614f4bad55" integrity sha512-JTWmoY9tWCs7zvIk/CvRjhjGaOd+OVBM987mxFo+OW66cGpdKjZcpmc74ES1sB//7Kl/PAe8+wEakuhG4pcgOw== dependencies: tslib "^1.9.0" rxjs@^6.4.0: version "6.4.0" resolved "https://registry.yarnpkg.com/rxjs/-/rxjs-6.4.0.tgz#f3bb0fe7bda7fb69deac0c16f17b50b0b8790504" integrity sha512-Z9Yfa11F6B9Sg/BK9MnqnQ+aQYicPLtilXBp2yUtDt2JRCE0h26d33EnfO3ZxoNxG0T92OUucP3Ct7cpfkdFfw== dependencies: tslib "^1.9.0" safe-buffer@^5.0.1, safe-buffer@^5.1.2, safe-buffer@~5.1.0, safe-buffer@~5.1.1: version "5.1.2" resolved "https://registry.yarnpkg.com/safe-buffer/-/safe-buffer-5.1.2.tgz#991ec69d296e0313747d59bdfd2b745c35f8828d" integrity sha512-Gd2UZBJDkXlY7GbJxfsE8/nvKkUEU1G38c1siN6QP6a9PT9MmHB8GnpscSmMJSoF8LOIrt8ud/wPtojys4G6+g== safe-regex@^1.1.0: version "1.1.0" resolved "https://registry.yarnpkg.com/safe-regex/-/safe-regex-1.1.0.tgz#40a3669f3b077d1e943d44629e157dd48023bf2e" integrity sha1-QKNmnzsHfR6UPURinhV91IAjvy4= dependencies: ret "~0.1.10" "safer-buffer@>= 2.1.2 < 3", safer-buffer@^2.0.2, safer-buffer@^2.1.0, safer-buffer@~2.1.0: version "2.1.2" resolved "https://registry.yarnpkg.com/safer-buffer/-/safer-buffer-2.1.2.tgz#44fa161b0187b9549dd84bb91802f9bd8385cd6a" integrity sha512-YZo3K82SD7Riyi0E1EQPojLz7kpepnSQI9IyPbHHg1XXXevb5dJI7tpyN2ADxGcQbHG7vcyRHk0cbwqcQriUtg== sax@^1.2.4: version "1.2.4" resolved "https://registry.yarnpkg.com/sax/-/sax-1.2.4.tgz#2816234e2378bddc4e5354fab5caa895df7100d9" integrity sha512-NqVDv9TpANUjFm0N8uM5GxL36UgKi9/atZw+x7YFnQ8ckwFGKrl4xX4yWtrey3UJm5nP1kUbnYgLopqWNSRhWw== scheduler@^0.13.2, scheduler@^0.13.6: version "0.13.6" resolved "https://registry.yarnpkg.com/scheduler/-/scheduler-0.13.6.tgz#466a4ec332467b31a91b9bf74e5347072e4cd889" integrity sha512-IWnObHt413ucAYKsD9J1QShUKkbKLQQHdxRyw73sw4FN26iWr3DY/H34xGPe4nmL1DwXyWmSWmMrA9TfQbE/XQ== dependencies: loose-envify "^1.1.0" object-assign "^4.1.1" semver-compare@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/semver-compare/-/semver-compare-1.0.0.tgz#0dee216a1c941ab37e9efb1788f6afc5ff5537fc" integrity sha1-De4hahyUGrN+nvsXiPavxf9VN/w= "semver@2 || 3 || 4 || 5", semver@^5.3.0, semver@^5.4.1, semver@^5.5.0, semver@^5.5.1: version "5.6.0" resolved "https://registry.yarnpkg.com/semver/-/semver-5.6.0.tgz#7e74256fbaa49c75aa7c7a205cc22799cac80004" integrity sha512-RS9R6R35NYgQn++fkDWaOmqGoj4Ek9gGs+DPxNUZKuwE183xjJroKvyo1IzVFeXvUrvmALy6FWD5xrdJT25gMg== semver@^5.6.0: version "5.7.0" resolved "https://registry.yarnpkg.com/semver/-/semver-5.7.0.tgz#790a7cf6fea5459bac96110b29b60412dc8ff96b" integrity sha512-Ya52jSX2u7QKghxeoFGpLwCtGlt7j0oY9DYb5apt9nPlJ42ID+ulTXESnt/qAQcoSERyZ5sl3LDIOw0nAn/5DA== semver@^6.0.0: version "6.0.0" resolved "https://registry.yarnpkg.com/semver/-/semver-6.0.0.tgz#05e359ee571e5ad7ed641a6eec1e547ba52dea65" integrity sha512-0UewU+9rFapKFnlbirLi3byoOuhrSsli/z/ihNnvM24vgF+8sNBiI1LZPBSH9wJKUwaUbw+s3hToDLCXkrghrQ== set-blocking@^2.0.0, set-blocking@~2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/set-blocking/-/set-blocking-2.0.0.tgz#045f9782d011ae9a6803ddd382b24392b3d890f7" integrity sha1-BF+XgtARrppoA93TgrJDkrPYkPc= set-value@^0.4.3: version "0.4.3" resolved "https://registry.yarnpkg.com/set-value/-/set-value-0.4.3.tgz#7db08f9d3d22dc7f78e53af3c3bf4666ecdfccf1" integrity sha1-fbCPnT0i3H945Trzw79GZuzfzPE= dependencies: extend-shallow "^2.0.1" is-extendable "^0.1.1" is-plain-object "^2.0.1" to-object-path "^0.3.0" set-value@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/set-value/-/set-value-2.0.0.tgz#71ae4a88f0feefbbf52d1ea604f3fb315ebb6274" integrity sha512-hw0yxk9GT/Hr5yJEYnHNKYXkIA8mVJgd9ditYZCe16ZczcaELYYcfvaXesNACk2O8O0nTiPQcQhGUQj8JLzeeg== dependencies: extend-shallow "^2.0.1" is-extendable "^0.1.1" is-plain-object "^2.0.3" split-string "^3.0.1" shebang-command@^1.2.0: version "1.2.0" resolved "https://registry.yarnpkg.com/shebang-command/-/shebang-command-1.2.0.tgz#44aac65b695b03398968c39f363fee5deafdf1ea" integrity sha1-RKrGW2lbAzmJaMOfNj/uXer98eo= dependencies: shebang-regex "^1.0.0" shebang-regex@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/shebang-regex/-/shebang-regex-1.0.0.tgz#da42f49740c0b42db2ca9728571cb190c98efea3" integrity sha1-2kL0l0DAtC2yypcoVxyxkMmO/qM= signal-exit@^3.0.0, signal-exit@^3.0.2: version "3.0.2" resolved "https://registry.yarnpkg.com/signal-exit/-/signal-exit-3.0.2.tgz#b5fdc08f1287ea1178628e415e25132b73646c6d" integrity sha1-tf3AjxKH6hF4Yo5BXiUTK3NkbG0= simple-git@^1.85.0: version "1.107.0" resolved "https://registry.yarnpkg.com/simple-git/-/simple-git-1.107.0.tgz#12cffaf261c14d6f450f7fdb86c21ccee968b383" integrity sha512-t4OK1JRlp4ayKRfcW6owrWcRVLyHRUlhGd0uN6ZZTqfDq8a5XpcUdOKiGRNobHEuMtNqzp0vcJNvhYWwh5PsQA== dependencies: debug "^4.0.1" slash@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/slash/-/slash-1.0.0.tgz#c41f2f6c39fc16d1cd17ad4b5d896114ae470d55" integrity sha1-xB8vbDn8FtHNF61LXYlhFK5HDVU= slash@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/slash/-/slash-2.0.0.tgz#de552851a1759df3a8f206535442f5ec4ddeab44" integrity sha512-ZYKh3Wh2z1PpEXWr0MpSBZ0V6mZHAQfYevttO11c51CaWjGTaadiKZ+wVt1PbMlDV5qhMFslpZCemhwOK7C89A== slice-ansi@0.0.4: version "0.0.4" resolved "https://registry.yarnpkg.com/slice-ansi/-/slice-ansi-0.0.4.tgz#edbf8903f66f7ce2f8eafd6ceed65e264c831b35" integrity sha1-7b+JA/ZvfOL46v1s7tZeJkyDGzU= slice-ansi@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/slice-ansi/-/slice-ansi-1.0.0.tgz#044f1a49d8842ff307aad6b505ed178bd950134d" integrity sha512-POqxBK6Lb3q6s047D/XsDVNPnF9Dl8JSaqe9h9lURl0OdNqy/ujDrOiIHtsqXMGbWWTIomRzAMaTyawAU//Reg== dependencies: is-fullwidth-code-point "^2.0.0" slice-ansi@^2.1.0: version "2.1.0" resolved "https://registry.yarnpkg.com/slice-ansi/-/slice-ansi-2.1.0.tgz#cacd7693461a637a5788d92a7dd4fba068e81636" integrity sha512-Qu+VC3EwYLldKa1fCxuuvULvSJOKEgk9pi8dZeCVK7TqBfUNTH4sFkk4joj8afVSfAYgJoSOetjx9QWOJ5mYoQ== dependencies: ansi-styles "^3.2.0" astral-regex "^1.0.0" is-fullwidth-code-point "^2.0.0" snapdragon-node@^2.0.1: version "2.1.1" resolved "https://registry.yarnpkg.com/snapdragon-node/-/snapdragon-node-2.1.1.tgz#6c175f86ff14bdb0724563e8f3c1b021a286853b" integrity sha512-O27l4xaMYt/RSQ5TR3vpWCAB5Kb/czIcqUFOM/C4fYcLnbZUc1PkjTAMjof2pBWaSTwOUd6qUHcFGVGj7aIwnw== dependencies: define-property "^1.0.0" isobject "^3.0.0" snapdragon-util "^3.0.1" snapdragon-util@^3.0.1: version "3.0.1" resolved "https://registry.yarnpkg.com/snapdragon-util/-/snapdragon-util-3.0.1.tgz#f956479486f2acd79700693f6f7b805e45ab56e2" integrity sha512-mbKkMdQKsjX4BAL4bRYTj21edOf8cN7XHdYUJEe+Zn99hVEYcMvKPct1IqNe7+AZPirn8BCDOQBHQZknqmKlZQ== dependencies: kind-of "^3.2.0" snapdragon@^0.8.1: version "0.8.2" resolved "https://registry.yarnpkg.com/snapdragon/-/snapdragon-0.8.2.tgz#64922e7c565b0e14204ba1aa7d6964278d25182d" integrity sha512-FtyOnWN/wCHTVXOMwvSv26d+ko5vWlIDD6zoUJ7LW8vh+ZBC8QdljveRP+crNrtBwioEUWy/4dMtbBjA4ioNlg== dependencies: base "^0.11.1" debug "^2.2.0" define-property "^0.2.5" extend-shallow "^2.0.1" map-cache "^0.2.2" source-map "^0.5.6" source-map-resolve "^0.5.0" use "^3.1.0" source-map-resolve@^0.5.0: version "0.5.2" resolved "https://registry.yarnpkg.com/source-map-resolve/-/source-map-resolve-0.5.2.tgz#72e2cc34095543e43b2c62b2c4c10d4a9054f259" integrity sha512-MjqsvNwyz1s0k81Goz/9vRBe9SZdB09Bdw+/zYyO+3CuPk6fouTaxscHkgtE8jKvf01kVfl8riHzERQ/kefaSA== dependencies: atob "^2.1.1" decode-uri-component "^0.2.0" resolve-url "^0.2.1" source-map-url "^0.4.0" urix "^0.1.0" source-map-support@^0.4.15: version "0.4.18" resolved "https://registry.yarnpkg.com/source-map-support/-/source-map-support-0.4.18.tgz#0286a6de8be42641338594e97ccea75f0a2c585f" integrity sha512-try0/JqxPLF9nOjvSta7tVondkP5dwgyLDjVoyMDlmjugT2lRZ1OfsrYTkCd2hkDnJTKRbO/Rl3orm8vlsUzbA== dependencies: source-map "^0.5.6" source-map-support@^0.5.11, source-map-support@^0.5.12, source-map-support@^0.5.6: version "0.5.12" resolved "https://registry.yarnpkg.com/source-map-support/-/source-map-support-0.5.12.tgz#b4f3b10d51857a5af0138d3ce8003b201613d599" integrity sha512-4h2Pbvyy15EE02G+JOZpUCmqWJuqrs+sEkzewTm++BPi7Hvn/HwcqLAcNxYAyI0x13CpPPn+kMjl+hplXMHITQ== dependencies: buffer-from "^1.0.0" source-map "^0.6.0" source-map-url@^0.4.0: version "0.4.0" resolved "https://registry.yarnpkg.com/source-map-url/-/source-map-url-0.4.0.tgz#3e935d7ddd73631b97659956d55128e87b5084a3" integrity sha1-PpNdfd1zYxuXZZlW1VEo6HtQhKM= source-map@^0.5.0, source-map@^0.5.6, source-map@^0.5.7: version "0.5.7" resolved "https://registry.yarnpkg.com/source-map/-/source-map-0.5.7.tgz#8a039d2d1021d22d1ea14c80d8ea468ba2ef3fcc" integrity sha1-igOdLRAh0i0eoUyA2OpGi6LvP8w= source-map@^0.6.0, source-map@^0.6.1, source-map@~0.6.1: version "0.6.1" resolved "https://registry.yarnpkg.com/source-map/-/source-map-0.6.1.tgz#74722af32e9614e9c287a8d0bbde48b5e2f1a263" integrity sha512-UjgapumWlbMhkBgzT7Ykc5YXUT46F0iKu8SGXq0bcwP5dz/h0Plj6enJqjz1Zbq2l5WaqYnrVbwWOWMyF3F47g== spawn-wrap@^1.4.2: version "1.4.2" resolved "https://registry.yarnpkg.com/spawn-wrap/-/spawn-wrap-1.4.2.tgz#cff58e73a8224617b6561abdc32586ea0c82248c" integrity sha512-vMwR3OmmDhnxCVxM8M+xO/FtIp6Ju/mNaDfCMMW7FDcLRTPFWUswec4LXJHTJE2hwTI9O0YBfygu4DalFl7Ylg== dependencies: foreground-child "^1.5.6" mkdirp "^0.5.0" os-homedir "^1.0.1" rimraf "^2.6.2" signal-exit "^3.0.2" which "^1.3.0" spdx-correct@^3.0.0: version "3.1.0" resolved "https://registry.yarnpkg.com/spdx-correct/-/spdx-correct-3.1.0.tgz#fb83e504445268f154b074e218c87c003cd31df4" integrity sha512-lr2EZCctC2BNR7j7WzJ2FpDznxky1sjfxvvYEyzxNyb6lZXHODmEoJeFu4JupYlkfha1KZpJyoqiJ7pgA1qq8Q== dependencies: spdx-expression-parse "^3.0.0" spdx-license-ids "^3.0.0" spdx-exceptions@^2.1.0: version "2.2.0" resolved "https://registry.yarnpkg.com/spdx-exceptions/-/spdx-exceptions-2.2.0.tgz#2ea450aee74f2a89bfb94519c07fcd6f41322977" integrity sha512-2XQACfElKi9SlVb1CYadKDXvoajPgBVPn/gOQLrTvHdElaVhr7ZEbqJaRnJLVNeaI4cMEAgVCeBMKF6MWRDCRA== spdx-expression-parse@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/spdx-expression-parse/-/spdx-expression-parse-3.0.0.tgz#99e119b7a5da00e05491c9fa338b7904823b41d0" integrity sha512-Yg6D3XpRD4kkOmTpdgbUiEJFKghJH03fiC1OPll5h/0sO6neh2jqRDVHOQ4o/LMea0tgCkbMgea5ip/e+MkWyg== dependencies: spdx-exceptions "^2.1.0" spdx-license-ids "^3.0.0" spdx-license-ids@^3.0.0: version "3.0.3" resolved "https://registry.yarnpkg.com/spdx-license-ids/-/spdx-license-ids-3.0.3.tgz#81c0ce8f21474756148bbb5f3bfc0f36bf15d76e" integrity sha512-uBIcIl3Ih6Phe3XHK1NqboJLdGfwr1UN3k6wSD1dZpmPsIkb8AGNbZYJ1fOBk834+Gxy8rpfDxrS6XLEMZMY2g== split-string@^3.0.1, split-string@^3.0.2: version "3.1.0" resolved "https://registry.yarnpkg.com/split-string/-/split-string-3.1.0.tgz#7cb09dda3a86585705c64b39a6466038682e8fe2" integrity sha512-NzNVhJDYpwceVVii8/Hu6DKfD2G+NrQHlS/V/qgv763EYudVwEcMQNxd2lh+0VrUByXN/oJkl5grOhYWvQUYiw== dependencies: extend-shallow "^3.0.0" sprintf-js@~1.0.2: version "1.0.3" resolved "https://registry.yarnpkg.com/sprintf-js/-/sprintf-js-1.0.3.tgz#04e6926f662895354f3dd015203633b857297e2c" integrity sha1-BOaSb2YolTVPPdAVIDYzuFcpfiw= sshpk@^1.7.0: version "1.16.1" resolved "https://registry.yarnpkg.com/sshpk/-/sshpk-1.16.1.tgz#fb661c0bef29b39db40769ee39fa70093d6f6877" integrity sha512-HXXqVUq7+pcKeLqqZj6mHFUMvXtOJt1uoUx09pFW6011inTMxqI8BA8PM95myrIyyKwdnzjdFjLiE6KBPVtJIg== dependencies: asn1 "~0.2.3" assert-plus "^1.0.0" bcrypt-pbkdf "^1.0.0" dashdash "^1.12.0" ecc-jsbn "~0.1.1" getpass "^0.1.1" jsbn "~0.1.0" safer-buffer "^2.0.2" tweetnacl "~0.14.0" stack-utils@^1.0.2: version "1.0.2" resolved "https://registry.yarnpkg.com/stack-utils/-/stack-utils-1.0.2.tgz#33eba3897788558bebfc2db059dc158ec36cebb8" integrity sha512-MTX+MeG5U994cazkjd/9KNAapsHnibjMLnfXodlkXw76JEea0UiNzrqidzo1emMwk7w5Qhc9jd4Bn9TBb1MFwA== staged-git-files@1.1.2: version "1.1.2" resolved "https://registry.yarnpkg.com/staged-git-files/-/staged-git-files-1.1.2.tgz#4326d33886dc9ecfa29a6193bf511ba90a46454b" integrity sha512-0Eyrk6uXW6tg9PYkhi/V/J4zHp33aNyi2hOCmhFLqLTIhbgqWn5jlSzI+IU0VqrZq6+DbHcabQl/WP6P3BG0QA== static-extend@^0.1.1: version "0.1.2" resolved "https://registry.yarnpkg.com/static-extend/-/static-extend-0.1.2.tgz#60809c39cbff55337226fd5e0b520f341f1fb5c6" integrity sha1-YICcOcv/VTNyJv1eC1IPNB8ftcY= dependencies: define-property "^0.2.5" object-copy "^0.1.0" string-argv@^0.0.2: version "0.0.2" resolved "https://registry.yarnpkg.com/string-argv/-/string-argv-0.0.2.tgz#dac30408690c21f3c3630a3ff3a05877bdcbd736" integrity sha1-2sMECGkMIfPDYwo/86BYd73L1zY= string-length@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/string-length/-/string-length-2.0.0.tgz#d40dbb686a3ace960c1cffca562bf2c45f8363ed" integrity sha1-1A27aGo6zpYMHP/KVivyxF+DY+0= dependencies: astral-regex "^1.0.0" strip-ansi "^4.0.0" string-width@^1.0.1: version "1.0.2" resolved "https://registry.yarnpkg.com/string-width/-/string-width-1.0.2.tgz#118bdf5b8cdc51a2a7e70d211e07e2b0b9b107d3" integrity sha1-EYvfW4zcUaKn5w0hHgfisLmxB9M= dependencies: code-point-at "^1.0.0" is-fullwidth-code-point "^1.0.0" strip-ansi "^3.0.0" "string-width@^1.0.2 || 2", string-width@^2.0.0, string-width@^2.1.0, string-width@^2.1.1: version "2.1.1" resolved "https://registry.yarnpkg.com/string-width/-/string-width-2.1.1.tgz#ab93f27a8dc13d28cac815c462143a6d9012ae9e" integrity sha512-nOqH59deCq9SRHlxq1Aw85Jnt4w6KvLKqWVik6oA9ZklXLNIOlqg4F2yrT1MVaTjAqvVwdfeZ7w7aCvJD7ugkw== dependencies: is-fullwidth-code-point "^2.0.0" strip-ansi "^4.0.0" string-width@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/string-width/-/string-width-3.0.0.tgz#5a1690a57cc78211fffd9bf24bbe24d090604eb1" integrity sha512-rr8CUxBbvOZDUvc5lNIJ+OC1nPVpz+Siw9VBtUjB9b6jZehZLFt0JMCZzShFHIsI8cbhm0EsNIfWJMFV3cu3Ew== dependencies: emoji-regex "^7.0.1" is-fullwidth-code-point "^2.0.0" strip-ansi "^5.0.0" string_decoder@~1.1.1: version "1.1.1" resolved "https://registry.yarnpkg.com/string_decoder/-/string_decoder-1.1.1.tgz#9cf1611ba62685d7030ae9e4ba34149c3af03fc8" integrity sha512-n/ShnvDi6FHbbVfviro+WojiFzv+s8MPMHBczVePfUpDJLwoLT0ht1l4YwBCbi8pJAveEEdnkHyPyTP/mzRfwg== dependencies: safe-buffer "~5.1.0" stringify-object@^3.2.2: version "3.3.0" resolved "https://registry.yarnpkg.com/stringify-object/-/stringify-object-3.3.0.tgz#703065aefca19300d3ce88af4f5b3956d7556629" integrity sha512-rHqiFh1elqCQ9WPLIC8I0Q/g/wj5J1eMkyoiD6eoQApWHP0FtlK7rqnhmabL5VUY9JQCcqwwvlOaSuutekgyrw== dependencies: get-own-enumerable-property-symbols "^3.0.0" is-obj "^1.0.1" is-regexp "^1.0.0" strip-ansi@^3.0.0, strip-ansi@^3.0.1: version "3.0.1" resolved "https://registry.yarnpkg.com/strip-ansi/-/strip-ansi-3.0.1.tgz#6a385fb8853d952d5ff05d0e8aaf94278dc63dcf" integrity sha1-ajhfuIU9lS1f8F0Oiq+UJ43GPc8= dependencies: ansi-regex "^2.0.0" strip-ansi@^4.0.0: version "4.0.0" resolved "https://registry.yarnpkg.com/strip-ansi/-/strip-ansi-4.0.0.tgz#a8479022eb1ac368a871389b635262c505ee368f" integrity sha1-qEeQIusaw2iocTibY1JixQXuNo8= dependencies: ansi-regex "^3.0.0" strip-ansi@^5.0.0: version "5.0.0" resolved "https://registry.yarnpkg.com/strip-ansi/-/strip-ansi-5.0.0.tgz#f78f68b5d0866c20b2c9b8c61b5298508dc8756f" integrity sha512-Uu7gQyZI7J7gn5qLn1Np3G9vcYGTVqB+lFTytnDJv83dd8T22aGH451P3jueT2/QemInJDfxHB5Tde5OzgG1Ow== dependencies: ansi-regex "^4.0.0" strip-bom@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/strip-bom/-/strip-bom-3.0.0.tgz#2334c18e9c759f7bdd56fdef7e9ae3d588e68ed3" integrity sha1-IzTBjpx1n3vdVv3vfprj1YjmjtM= strip-eof@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/strip-eof/-/strip-eof-1.0.0.tgz#bb43ff5598a6eb05d89b59fcd129c983313606bf" integrity sha1-u0P/VZim6wXYm1n80SnJgzE2Br8= strip-json-comments@^2.0.1, strip-json-comments@~2.0.1: version "2.0.1" resolved "https://registry.yarnpkg.com/strip-json-comments/-/strip-json-comments-2.0.1.tgz#3c531942e908c2697c0ec344858c286c7ca0a60a" integrity sha1-PFMZQukIwml8DsNEhYwobHygpgo= supports-color@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/supports-color/-/supports-color-2.0.0.tgz#535d045ce6b6363fa40117084629995e9df324c7" integrity sha1-U10EXOa2Nj+kARcIRimZXp3zJMc= supports-color@^5.3.0: version "5.5.0" resolved "https://registry.yarnpkg.com/supports-color/-/supports-color-5.5.0.tgz#e2e69a44ac8772f78a1ec0b35b689df6530efc8f" integrity sha512-QjVjwdXIt408MIiAqCX4oUKsgU2EqAGzs2Ppkm4aQYbjm+ZEWEcW4SfFNTr4uMNZma0ey4f5lgLrkB0aX0QMow== dependencies: has-flag "^3.0.0" supports-color@^6.1.0: version "6.1.0" resolved "https://registry.yarnpkg.com/supports-color/-/supports-color-6.1.0.tgz#0764abc69c63d5ac842dd4867e8d025e880df8f3" integrity sha512-qe1jfm1Mg7Nq/NSh6XE24gPXROEVsWHxC1LIx//XNlD9iw7YZQGjZNjYN7xGaEG6iKdA8EtNFW6R0gjnVXp+wQ== dependencies: has-flag "^3.0.0" symbol-observable@^1.1.0: version "1.2.0" resolved "https://registry.yarnpkg.com/symbol-observable/-/symbol-observable-1.2.0.tgz#c22688aed4eab3cdc2dfeacbb561660560a00804" integrity sha512-e900nM8RRtGhlV36KGEU9k65K3mPb1WV70OdjfxlG2EAuM1noi/E/BaW/uMhL7bPEssK8QV57vN3esixjUvcXQ== synchronous-promise@^2.0.6: version "2.0.7" resolved "https://registry.yarnpkg.com/synchronous-promise/-/synchronous-promise-2.0.7.tgz#3574b3d2fae86b145356a4b89103e1577f646fe3" integrity sha512-16GbgwTmFMYFyQMLvtQjvNWh30dsFe1cAW5Fg1wm5+dg84L9Pe36mftsIRU95/W2YsISxsz/xq4VB23sqpgb/A== table@^5.2.3: version "5.2.3" resolved "https://registry.yarnpkg.com/table/-/table-5.2.3.tgz#cde0cc6eb06751c009efab27e8c820ca5b67b7f2" integrity sha512-N2RsDAMvDLvYwFcwbPyF3VmVSSkuF+G1e+8inhBLtHpvwXGw4QRPEZhihQNeEN0i1up6/f6ObCJXNdlRG3YVyQ== dependencies: ajv "^6.9.1" lodash "^4.17.11" slice-ansi "^2.1.0" string-width "^3.0.0" tap-mocha-reporter@^4.0.1: version "4.0.1" resolved "https://registry.yarnpkg.com/tap-mocha-reporter/-/tap-mocha-reporter-4.0.1.tgz#f613229c42c2e5b5f096f2f022e88724c9380b10" integrity sha512-/KfXaaYeSPn8qBi5Be8WSIP3iKV83s2uj2vzImJAXmjNu22kzqZ+1Dv1riYWa53sPCiyo1R1w1jbJrftF8SpcQ== dependencies: color-support "^1.1.0" debug "^2.1.3" diff "^1.3.2" escape-string-regexp "^1.0.3" glob "^7.0.5" tap-parser "^8.0.0" tap-yaml "0 || 1" unicode-length "^1.0.0" optionalDependencies: readable-stream "^2.1.5" tap-parser@^8.0.0: version "8.1.0" resolved "https://registry.yarnpkg.com/tap-parser/-/tap-parser-8.1.0.tgz#6aff5221c0fe9b39757d60eafc4c436c3a9188fc" integrity sha512-GgOzgDwThYLxhVR83RbS1JUR1TxcT+jfZsrETgPAvFdr12lUOnuvrHOBaUQgpkAp6ZyeW6r2Nwd91t88M0ru3w== dependencies: events-to-array "^1.0.1" minipass "^2.2.0" tap-yaml "0 || 1" tap-parser@^9.3.2: version "9.3.2" resolved "https://registry.yarnpkg.com/tap-parser/-/tap-parser-9.3.2.tgz#a4fcd566dfc15584b721e6e16f3152ebe4937cc8" integrity sha512-bQ76sD6ZP9loxdk/KiqXgWDEfjJYiZUj0a7ElnLMSny7Q8G72UMcOQee85j5ddKHA9fzGAoL6cfJbg3rur7S5g== dependencies: events-to-array "^1.0.1" minipass "^2.2.0" tap-yaml "^1.0.0" "tap-yaml@0 || 1", tap-yaml@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/tap-yaml/-/tap-yaml-1.0.0.tgz#4e31443a5489e05ca8bbb3e36cef71b5dec69635" integrity sha512-Rxbx4EnrWkYk0/ztcm5u3/VznbyFJpyXO12dDBHKWiDVxy7O2Qw6MRrwO5H6Ww0U5YhRY/4C/VzWmFPhBQc4qQ== dependencies: yaml "^1.5.0" tap@^13.1.2: version "13.1.2" resolved "https://registry.yarnpkg.com/tap/-/tap-13.1.2.tgz#ce273a596b0ff822ba24fe96f9f037cfce3e2f98" integrity sha512-ALs3MbwHvq1BfFwE74Tfi4ypCPvg4+C6Ix4BnUDXh8N3wGGzVqBER5j4zY7NloPUUWlS8Td0OdgZU5Am5DJH8A== dependencies: async-hook-domain "^1.1.0" bind-obj-methods "^2.0.0" browser-process-hrtime "^1.0.0" capture-stack-trace "^1.0.0" chokidar "^2.1.5" color-support "^1.1.0" coveralls "^3.0.3" diff "^4.0.1" domain-browser "^1.2.0" esm "^3.2.22" findit "^2.0.0" foreground-child "^1.3.3" fs-exists-cached "^1.0.0" function-loop "^1.0.2" glob "^7.1.3" import-jsx "^2.0.0" isexe "^2.0.0" istanbul-lib-processinfo "^1.0.0" jackspeak "^1.3.7" minipass "^2.3.5" mkdirp "^0.5.1" nyc "^14.1.0" opener "^1.5.1" own-or "^1.0.0" own-or-env "^1.0.1" rimraf "^2.6.3" signal-exit "^3.0.0" source-map-support "^0.5.12" stack-utils "^1.0.2" tap-mocha-reporter "^4.0.1" tap-parser "^9.3.2" tap-yaml "^1.0.0" tcompare "^2.2.0" treport "^0.3.0" trivial-deferred "^1.0.1" ts-node "^8.1.0" typescript "^3.4.3" which "^1.3.1" write-file-atomic "^2.4.2" yaml "^1.5.0" yapool "^1.0.0" tar@^4: version "4.4.8" resolved "https://registry.yarnpkg.com/tar/-/tar-4.4.8.tgz#b19eec3fde2a96e64666df9fdb40c5ca1bc3747d" integrity sha512-LzHF64s5chPQQS0IYBn9IN5h3i98c12bo4NCO7e0sGM2llXQ3p2FGC5sdENN4cTW48O915Sh+x+EXx7XW96xYQ== dependencies: chownr "^1.1.1" fs-minipass "^1.2.5" minipass "^2.3.4" minizlib "^1.1.1" mkdirp "^0.5.0" safe-buffer "^5.1.2" yallist "^3.0.2" tcompare@^2.2.0: version "2.2.0" resolved "https://registry.yarnpkg.com/tcompare/-/tcompare-2.2.0.tgz#873b20a54917f4e91789e2bc20e2c85c4476f754" integrity sha512-+Mr0UBIE3ncNn0wJvKsw8ph61QoaDvR6Q8WkxWIHxWLcKf8SHGyTTkGLMX//4NKQ/Pe1Uu64oXYJsxLyAXXWdA== test-exclude@^5.2.3: version "5.2.3" resolved "https://registry.yarnpkg.com/test-exclude/-/test-exclude-5.2.3.tgz#c3d3e1e311eb7ee405e092dac10aefd09091eac0" integrity sha512-M+oxtseCFO3EDtAaGH7iiej3CBkzXqFMbzqYAACdzKui4eZA+pq3tZEwChvOdNfa7xxy8BfbmgJSIr43cC/+2g== dependencies: glob "^7.1.3" minimatch "^3.0.4" read-pkg-up "^4.0.0" require-main-filename "^2.0.0" text-table@^0.2.0: version "0.2.0" resolved "https://registry.yarnpkg.com/text-table/-/text-table-0.2.0.tgz#7f5ee823ae805207c00af2df4a84ec3fcfa570b4" integrity sha1-f17oI66AUgfACvLfSoTsP8+lcLQ= through@^2.3.6: version "2.3.8" resolved "https://registry.yarnpkg.com/through/-/through-2.3.8.tgz#0dd4c9ffaabc357960b1b724115d7e0e86a2e1f5" integrity sha1-DdTJ/6q8NXlgsbckEV1+Doai4fU= tmp@^0.0.33: version "0.0.33" resolved "https://registry.yarnpkg.com/tmp/-/tmp-0.0.33.tgz#6d34335889768d21b2bcda0aa277ced3b1bfadf9" integrity sha512-jRCJlojKnZ3addtTOjdIqoRuPEKBvNXcGYqzO6zWZX8KfKEpnGY5jfggJQ3EjKuu8D4bJRr0y+cYJFmYbImXGw== dependencies: os-tmpdir "~1.0.2" to-fast-properties@^1.0.3: version "1.0.3" resolved "https://registry.yarnpkg.com/to-fast-properties/-/to-fast-properties-1.0.3.tgz#b83571fa4d8c25b82e231b06e3a3055de4ca1a47" integrity sha1-uDVx+k2MJbguIxsG46MFXeTKGkc= to-fast-properties@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/to-fast-properties/-/to-fast-properties-2.0.0.tgz#dc5e698cbd079265bc73e0377681a4e4e83f616e" integrity sha1-3F5pjL0HkmW8c+A3doGk5Og/YW4= to-object-path@^0.3.0: version "0.3.0" resolved "https://registry.yarnpkg.com/to-object-path/-/to-object-path-0.3.0.tgz#297588b7b0e7e0ac08e04e672f85c1f4999e17af" integrity sha1-KXWIt7Dn4KwI4E5nL4XB9JmeF68= dependencies: kind-of "^3.0.2" to-regex-range@^2.1.0: version "2.1.1" resolved "https://registry.yarnpkg.com/to-regex-range/-/to-regex-range-2.1.1.tgz#7c80c17b9dfebe599e27367e0d4dd5590141db38" integrity sha1-fIDBe53+vlmeJzZ+DU3VWQFB2zg= dependencies: is-number "^3.0.0" repeat-string "^1.6.1" to-regex@^3.0.1, to-regex@^3.0.2: version "3.0.2" resolved "https://registry.yarnpkg.com/to-regex/-/to-regex-3.0.2.tgz#13cfdd9b336552f30b51f33a8ae1b42a7a7599ce" integrity sha512-FWtleNAtZ/Ki2qtqej2CXTOayOH9bHDQF+Q48VpWyDXjbYxA4Yz8iDB31zXOBUlOHHKidDbqGVrTUvQMPmBGBw== dependencies: define-property "^2.0.2" extend-shallow "^3.0.2" regex-not "^1.0.2" safe-regex "^1.1.0" toposort@^2.0.2: version "2.0.2" resolved "https://registry.yarnpkg.com/toposort/-/toposort-2.0.2.tgz#ae21768175d1559d48bef35420b2f4962f09c330" integrity sha1-riF2gXXRVZ1IvvNUILL0li8JwzA= tough-cookie@~2.4.3: version "2.4.3" resolved "https://registry.yarnpkg.com/tough-cookie/-/tough-cookie-2.4.3.tgz#53f36da3f47783b0925afa06ff9f3b165280f781" integrity sha512-Q5srk/4vDM54WJsJio3XNn6K2sCG+CQ8G5Wz6bZhRZoAe/+TxjWB/GlFAnYEbkYVlON9FMk/fE3h2RLpPXo4lQ== dependencies: psl "^1.1.24" punycode "^1.4.1" treport@^0.3.0: version "0.3.0" resolved "https://registry.yarnpkg.com/treport/-/treport-0.3.0.tgz#023d7409ba236f0428d8b0acf3bb7678355d146f" integrity sha512-THr7NS5iLJewcAiaBkxAuCVoakw84hAVY9n2kFNZqlFKHrZeGw8sNR4VhmT23JB1/JnCmPcIOmooTdYYVTI5hA== dependencies: cardinal "^2.1.1" chalk "^2.4.2" import-jsx "^2.0.0" ink "^2.1.1" ms "^2.1.1" react "^16.8.6" string-length "^2.0.0" tap-parser "^9.3.2" unicode-length "^2.0.1" trim-right@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/trim-right/-/trim-right-1.0.1.tgz#cb2e1203067e0c8de1f614094b9fe45704ea6003" integrity sha1-yy4SAwZ+DI3h9hQJS5/kVwTqYAM= trivial-deferred@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/trivial-deferred/-/trivial-deferred-1.0.1.tgz#376d4d29d951d6368a6f7a0ae85c2f4d5e0658f3" integrity sha1-N21NKdlR1jaKb3oK6FwvTV4GWPM= ts-node@^8.1.0: version "8.1.0" resolved "https://registry.yarnpkg.com/ts-node/-/ts-node-8.1.0.tgz#8c4b37036abd448577db22a061fd7a67d47e658e" integrity sha512-34jpuOrxDuf+O6iW1JpgTRDFynUZ1iEqtYruBqh35gICNjN8x+LpVcPAcwzLPi9VU6mdA3ym+x233nZmZp445A== dependencies: arg "^4.1.0" diff "^3.1.0" make-error "^1.1.1" source-map-support "^0.5.6" yn "^3.0.0" tslib@^1.9.0: version "1.9.3" resolved "https://registry.yarnpkg.com/tslib/-/tslib-1.9.3.tgz#d7e4dd79245d85428c4d7e4822a79917954ca286" integrity sha512-4krF8scpejhaOgqzBEcGM7yDIEfi0/8+8zDRZhNZZ2kjmHJ4hv3zCbQWxoJGz1iw5U0Jl0nma13xzHXcncMavQ== tunnel-agent@^0.6.0: version "0.6.0" resolved "https://registry.yarnpkg.com/tunnel-agent/-/tunnel-agent-0.6.0.tgz#27a5dea06b36b04a0a9966774b290868f0fc40fd" integrity sha1-J6XeoGs2sEoKmWZ3SykIaPD8QP0= dependencies: safe-buffer "^5.0.1" tweetnacl@^0.14.3, tweetnacl@~0.14.0: version "0.14.5" resolved "https://registry.yarnpkg.com/tweetnacl/-/tweetnacl-0.14.5.tgz#5ae68177f192d4456269d108afa93ff8743f4f64" integrity sha1-WuaBd/GS1EViadEIr6k/+HQ/T2Q= type-check@~0.3.2: version "0.3.2" resolved "https://registry.yarnpkg.com/type-check/-/type-check-0.3.2.tgz#5884cab512cf1d355e3fb784f30804b2b520db72" integrity sha1-WITKtRLPHTVeP7eE8wgEsrUg23I= dependencies: prelude-ls "~1.1.2" type-fest@^0.4.1: version "0.4.1" resolved "https://registry.yarnpkg.com/type-fest/-/type-fest-0.4.1.tgz#8bdf77743385d8a4f13ba95f610f5ccd68c728f8" integrity sha512-IwzA/LSfD2vC1/YDYMv/zHP4rDF1usCwllsDpbolT3D4fUepIO7f9K70jjmUewU/LmGUKJcwcVtDCpnKk4BPMw== typescript@^3.4.3: version "3.4.5" resolved "https://registry.yarnpkg.com/typescript/-/typescript-3.4.5.tgz#2d2618d10bb566572b8d7aad5180d84257d70a99" integrity sha512-YycBxUb49UUhdNMU5aJ7z5Ej2XGmaIBL0x34vZ82fn3hGvD+bgrMrVDpatgz2f7YxUMJxMkbWxJZeAvDxVe7Vw== uglify-js@^3.1.4: version "3.5.10" resolved "https://registry.yarnpkg.com/uglify-js/-/uglify-js-3.5.10.tgz#652bef39f86d9dbfd6674407ee05a5e2d372cf2d" integrity sha512-/GTF0nosyPLbdJBd+AwYiZ+Hu5z8KXWnO0WCGt1BQ/u9Iamhejykqmz5o1OHJ53+VAk6xVxychonnApDjuqGsw== dependencies: commander "~2.20.0" source-map "~0.6.1" unicode-length@^1.0.0: version "1.0.3" resolved "https://registry.yarnpkg.com/unicode-length/-/unicode-length-1.0.3.tgz#5ada7a7fed51841a418a328cf149478ac8358abb" integrity sha1-Wtp6f+1RhBpBijKM8UlHisg1irs= dependencies: punycode "^1.3.2" strip-ansi "^3.0.1" unicode-length@^2.0.1: version "2.0.1" resolved "https://registry.yarnpkg.com/unicode-length/-/unicode-length-2.0.1.tgz#84ddc309c2323635f642e86d448e9eaec084c04a" integrity sha1-hN3DCcIyNjX2QuhtRI6ersCEwEo= dependencies: punycode "^2.0.0" strip-ansi "^3.0.1" union-value@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/union-value/-/union-value-1.0.0.tgz#5c71c34cb5bad5dcebe3ea0cd08207ba5aa1aea4" integrity sha1-XHHDTLW61dzr4+oM0IIHulqhrqQ= dependencies: arr-union "^3.1.0" get-value "^2.0.6" is-extendable "^0.1.1" set-value "^0.4.3" unset-value@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/unset-value/-/unset-value-1.0.0.tgz#8376873f7d2335179ffb1e6fc3a8ed0dfc8ab559" integrity sha1-g3aHP30jNRef+x5vw6jtDfyKtVk= dependencies: has-value "^0.3.1" isobject "^3.0.0" upath@^1.1.1: version "1.1.2" resolved "https://registry.yarnpkg.com/upath/-/upath-1.1.2.tgz#3db658600edaeeccbe6db5e684d67ee8c2acd068" integrity sha512-kXpym8nmDmlCBr7nKdIx8P2jNBa+pBpIUFRnKJ4dr8htyYGJFokkr2ZvERRtUN+9SY+JqXouNgUPtv6JQva/2Q== uri-js@^4.2.2: version "4.2.2" resolved "https://registry.yarnpkg.com/uri-js/-/uri-js-4.2.2.tgz#94c540e1ff772956e2299507c010aea6c8838eb0" integrity sha512-KY9Frmirql91X2Qgjry0Wd4Y+YTdrdZheS8TFwvkbLWf/G5KNJDCh6pKL5OZctEW4+0Baa5idK2ZQuELRwPznQ== dependencies: punycode "^2.1.0" urix@^0.1.0: version "0.1.0" resolved "https://registry.yarnpkg.com/urix/-/urix-0.1.0.tgz#da937f7a62e21fec1fd18d49b35c2935067a6c72" integrity sha1-2pN/emLiH+wf0Y1Js1wpNQZ6bHI= use@^3.1.0: version "3.1.1" resolved "https://registry.yarnpkg.com/use/-/use-3.1.1.tgz#d50c8cac79a19fbc20f2911f56eb973f4e10070f" integrity sha512-cwESVXlO3url9YWlFW/TA9cshCEhtu7IKJ/p5soJ/gGpj7vbvFrAY/eIioQ6Dw23KjZhYgiIo8HOs1nQ2vr/oQ== util-deprecate@~1.0.1: version "1.0.2" resolved "https://registry.yarnpkg.com/util-deprecate/-/util-deprecate-1.0.2.tgz#450d4dc9fa70de732762fbd2d4a28981419a0ccf" integrity sha1-RQ1Nyfpw3nMnYvvS1KKJgUGaDM8= uuid@^3.3.2: version "3.3.2" resolved "https://registry.yarnpkg.com/uuid/-/uuid-3.3.2.tgz#1b4af4955eb3077c501c23872fc6513811587131" integrity sha512-yXJmeNaw3DnnKAOKJE51sL/ZaYfWJRl1pK9dr19YFCu0ObS231AB1/LbqTKRAQ5kw8A90rA6fr4riOUpTZvQZA== validate-npm-package-license@^3.0.1: version "3.0.4" resolved "https://registry.yarnpkg.com/validate-npm-package-license/-/validate-npm-package-license-3.0.4.tgz#fc91f6b9c7ba15c857f4cb2c5defeec39d4f410a" integrity sha512-DpKm2Ui/xN7/HQKCtpZxoRWBhZ9Z0kqtygG8XCgNQ8ZlDnxuQmWhj566j8fN4Cu3/JmbhsDo7fcAJq4s9h27Ew== dependencies: spdx-correct "^3.0.0" spdx-expression-parse "^3.0.0" verror@1.10.0: version "1.10.0" resolved "https://registry.yarnpkg.com/verror/-/verror-1.10.0.tgz#3a105ca17053af55d6e270c1f8288682e18da400" integrity sha1-OhBcoXBTr1XW4nDB+CiGguGNpAA= dependencies: assert-plus "^1.0.0" core-util-is "1.0.2" extsprintf "^1.2.0" weakmap-shim@^1.1.0: version "1.1.1" resolved "https://registry.yarnpkg.com/weakmap-shim/-/weakmap-shim-1.1.1.tgz#d65afd784109b2166e00ff571c33150ec2a40b49" integrity sha1-1lr9eEEJshZuAP9XHDMVDsKkC0k= which-module@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/which-module/-/which-module-2.0.0.tgz#d9ef07dce77b9902b8a3a8fa4b31c3e3f7e6e87a" integrity sha1-2e8H3Od7mQK4o6j6SzHD4/fm6Ho= which@^1.2.10, which@^1.2.9, which@^1.3.0, which@^1.3.1: version "1.3.1" resolved "https://registry.yarnpkg.com/which/-/which-1.3.1.tgz#a45043d54f5805316da8d62f9f50918d3da70b0a" integrity sha512-HxJdYWq1MTIQbJ3nw0cqssHoTNU267KlrDuGZ1WYlxDStUtKUhOaJmh112/TZmHxxUfuJqPXSOm7tDyas0OSIQ== dependencies: isexe "^2.0.0" wide-align@^1.1.0: version "1.1.3" resolved "https://registry.yarnpkg.com/wide-align/-/wide-align-1.1.3.tgz#ae074e6bdc0c14a431e804e624549c633b000457" integrity sha512-QGkOQc8XL6Bt5PwnsExKBPuMKBxnGxWWW3fU55Xt4feHozMUhdUMaBCk290qpm/wG5u/RSKzwdAC4i51YigihA== dependencies: string-width "^1.0.2 || 2" widest-line@^2.0.0: version "2.0.1" resolved "https://registry.yarnpkg.com/widest-line/-/widest-line-2.0.1.tgz#7438764730ec7ef4381ce4df82fb98a53142a3fc" integrity sha512-Ba5m9/Fa4Xt9eb2ELXt77JxVDV8w7qQrH0zS/TWSJdLyAwQjWoOzpzj5lwVftDz6n/EOu3tNACS84v509qwnJA== dependencies: string-width "^2.1.1" wordwrap@~0.0.2: version "0.0.3" resolved "https://registry.yarnpkg.com/wordwrap/-/wordwrap-0.0.3.tgz#a3d5da6cd5c0bc0008d37234bbaf1bed63059107" integrity sha1-o9XabNXAvAAI03I0u68b7WMFkQc= wordwrap@~1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/wordwrap/-/wordwrap-1.0.0.tgz#27584810891456a4171c8d0226441ade90cbcaeb" integrity sha1-J1hIEIkUVqQXHI0CJkQa3pDLyus= wrap-ansi@^2.0.0: version "2.1.0" resolved "https://registry.yarnpkg.com/wrap-ansi/-/wrap-ansi-2.1.0.tgz#d8fc3d284dd05794fe84973caecdd1cf824fdd85" integrity sha1-2Pw9KE3QV5T+hJc8rs3Rz4JP3YU= dependencies: string-width "^1.0.1" strip-ansi "^3.0.1" wrap-ansi@^3.0.1: version "3.0.1" resolved "https://registry.yarnpkg.com/wrap-ansi/-/wrap-ansi-3.0.1.tgz#288a04d87eda5c286e060dfe8f135ce8d007f8ba" integrity sha1-KIoE2H7aXChuBg3+jxNc6NAH+Lo= dependencies: string-width "^2.1.1" strip-ansi "^4.0.0" wrap-ansi@^5.0.0: version "5.1.0" resolved "https://registry.yarnpkg.com/wrap-ansi/-/wrap-ansi-5.1.0.tgz#1fd1f67235d5b6d0fee781056001bfb694c03b09" integrity sha512-QC1/iN/2/RPVJ5jYK8BGttj5z83LmSKmvbvrXPNCLZSEb32KKVDJDl/MOt2N01qU2H/FkzEa9PKto1BqDjtd7Q== dependencies: ansi-styles "^3.2.0" string-width "^3.0.0" strip-ansi "^5.0.0" wrappy@1: version "1.0.2" resolved "https://registry.yarnpkg.com/wrappy/-/wrappy-1.0.2.tgz#b5243d8f3ec1aa35f1364605bc0d1036e30ab69f" integrity sha1-tSQ9jz7BqjXxNkYFvA0QNuMKtp8= write-file-atomic@^2.4.2: version "2.4.2" resolved "https://registry.yarnpkg.com/write-file-atomic/-/write-file-atomic-2.4.2.tgz#a7181706dfba17855d221140a9c06e15fcdd87b9" integrity sha512-s0b6vB3xIVRLWywa6X9TOMA7k9zio0TMOsl9ZnDkliA/cfJlpHXAscj0gbHVJiTdIuAYpIyqS5GW91fqm6gG5g== dependencies: graceful-fs "^4.1.11" imurmurhash "^0.1.4" signal-exit "^3.0.2" write@1.0.3: version "1.0.3" resolved "https://registry.yarnpkg.com/write/-/write-1.0.3.tgz#0800e14523b923a387e415123c865616aae0f5c3" integrity sha512-/lg70HAjtkUgWPVZhZcm+T4hkL8Zbtp1nFNOn3lRrxnlv50SRBv7cR7RqR+GMsd3hUXy9hWBo4CHTbFTcOYwig== dependencies: mkdirp "^0.5.1" xtend@^4.0.0: version "4.0.1" resolved "https://registry.yarnpkg.com/xtend/-/xtend-4.0.1.tgz#a5c6d532be656e23db820efb943a1f04998d63af" integrity sha1-pcbVMr5lbiPbgg77lDofBJmNY68= y18n@^4.0.0: version "4.0.0" resolved "https://registry.yarnpkg.com/y18n/-/y18n-4.0.0.tgz#95ef94f85ecc81d007c264e190a120f0a3c8566b" integrity sha512-r9S/ZyXu/Xu9q1tYlpsLIsa3EeLXXk0VwlxqTcFRfg9EhMW+17kbt9G0NrgCmhGb5vT2hyhJZLfDGx+7+5Uj/w== yallist@^2.1.2: version "2.1.2" resolved "https://registry.yarnpkg.com/yallist/-/yallist-2.1.2.tgz#1c11f9218f076089a47dd512f93c6699a6a81d52" integrity sha1-HBH5IY8HYImkfdUS+TxmmaaoHVI= yallist@^3.0.0, yallist@^3.0.2: version "3.0.3" resolved "https://registry.yarnpkg.com/yallist/-/yallist-3.0.3.tgz#b4b049e314be545e3ce802236d6cd22cd91c3de9" integrity sha512-S+Zk8DEWE6oKpV+vI3qWkaK+jSbIK86pCwe2IF/xwIpQ8jEuxpw9NyaGjmp9+BoJv5FV2piqCDcoCtStppiq2A== yaml@^1.5.0: version "1.5.1" resolved "https://registry.yarnpkg.com/yaml/-/yaml-1.5.1.tgz#e8201678064fbcfef6afe4122ef802573b6cade8" integrity sha512-btfJvMOgVthGZSgHBMrDkLuQu4YxOycw6kwuC67cUEOKJmmNozjIa02eKvuSq7usqqqpwwCvflGTF6JcDvSudw== dependencies: "@babel/runtime" "^7.4.4" yapool@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/yapool/-/yapool-1.0.0.tgz#f693f29a315b50d9a9da2646a7a6645c96985b6a" integrity sha1-9pPymjFbUNmp2iZGp6ZkXJaYW2o= yargs-parser@^13.0.0: version "13.0.0" resolved "https://registry.yarnpkg.com/yargs-parser/-/yargs-parser-13.0.0.tgz#3fc44f3e76a8bdb1cc3602e860108602e5ccde8b" integrity sha512-w2LXjoL8oRdRQN+hOyppuXs+V/fVAYtpcrRxZuF7Kt/Oc+Jr2uAcVntaUTNT6w5ihoWfFDpNY8CPx1QskxZ/pw== dependencies: camelcase "^5.0.0" decamelize "^1.2.0" yargs@^13.2.2: version "13.2.2" resolved "https://registry.yarnpkg.com/yargs/-/yargs-13.2.2.tgz#0c101f580ae95cea7f39d927e7770e3fdc97f993" integrity sha512-WyEoxgyTD3w5XRpAQNYUB9ycVH/PQrToaTXdYXRdOXvEy1l19br+VJsc0vcO8PTGg5ro/l/GY7F/JMEBmI0BxA== dependencies: cliui "^4.0.0" find-up "^3.0.0" get-caller-file "^2.0.1" os-locale "^3.1.0" require-directory "^2.1.1" require-main-filename "^2.0.0" set-blocking "^2.0.0" string-width "^3.0.0" which-module "^2.0.0" y18n "^4.0.0" yargs-parser "^13.0.0" yn@^3.0.0: version "3.1.0" resolved "https://registry.yarnpkg.com/yn/-/yn-3.1.0.tgz#fcbe2db63610361afcc5eb9e0ac91e976d046114" integrity sha512-kKfnnYkbTfrAdd0xICNFw7Atm8nKpLcLv9AZGEt+kczL/WQVai4e2V6ZN8U/O+iI6WrNuJjNNOyu4zfhl9D3Hg== yoga-layout-prebuilt@^1.9.3: version "1.9.3" resolved "https://registry.yarnpkg.com/yoga-layout-prebuilt/-/yoga-layout-prebuilt-1.9.3.tgz#11e3be29096afe3c284e5d963cc2d628148c1372" integrity sha512-9SNQpwuEh2NucU83i2KMZnONVudZ86YNcFk9tq74YaqrQfgJWO3yB9uzH1tAg8iqh5c9F5j0wuyJ2z72wcum2w== yup@^0.27.0: version "0.27.0" resolved "https://registry.yarnpkg.com/yup/-/yup-0.27.0.tgz#f8cb198c8e7dd2124beddc2457571329096b06e7" integrity sha512-v1yFnE4+u9za42gG/b/081E7uNW9mUj3qtkmelLbW5YPROZzSH/KUUyJu9Wt8vxFJcT9otL/eZopS0YK1L5yPQ== dependencies: "@babel/runtime" "^7.0.0" fn-name "~2.0.1" lodash "^4.17.11" property-expr "^1.5.0" synchronous-promise "^2.0.6" toposort "^2.0.2" apollo-server-demo/node_modules/fs-capacitor/package.json 0000644 0001750 0000144 00000004353 13463757003 023305 0 ustar andreh users { "name": "fs-capacitor", "version": "2.0.4", "description": "Filesystem-buffered, passthrough stream that buffers indefinitely rather than propagate backpressure from downstream consumers.", "license": "MIT", "author": { "name": "Mike Marcacci", "email": "mike.marcacci@gmail.com" }, "repository": "github:mike-marcacci/fs-capacitor", "homepage": "https://github.com/mike-marcacci/fs-capacitor#readme", "bugs": "https://github.com/mike-marcacci/fs-capacitor/issues", "keywords": [ "stream", "buffer", "file", "split", "clone" ], "files": [ "lib", "!lib/test.*" ], "main": "lib", "engines": { "node": ">=8.5" }, "browserslist": "node >= 8.5", "devDependencies": { "@babel/cli": "^7.1.2", "@babel/core": "^7.3.3", "@babel/preset-env": "^7.4.4", "babel-eslint": "^10.0.1", "eslint": "^5.14.1", "eslint-config-env": "^5.0.0", "eslint-config-prettier": "^4.0.0", "eslint-plugin-import": "^2.16.0", "eslint-plugin-import-order-alphabetical": "^0.0.2", "eslint-plugin-node": "^9.0.1", "eslint-plugin-prettier": "^3.0.0", "husky": "^2.2.0", "if-ver": "^1.1.0", "leaked-handles": "^5.2.0", "lint-staged": "^8.1.4", "prettier": "^1.16.4", "tap": "^13.1.2" }, "scripts": { "prepare": "npm run prepare:clean && npm run prepare:mjs && npm run prepare:js && npm run prepare:prettier", "prepare:clean": "rm -rf lib", "prepare:mjs": "BABEL_ESM=1 babel src -d lib --keep-file-extension", "prepare:js": "babel src -d lib", "prepare:prettier": "prettier 'lib/**/*.{mjs,js}' --write", "test": "npm run test:eslint && npm run test:prettier && npm run test:mjs && npm run test:js", "test:eslint": "eslint . --ext mjs,js", "test:prettier": "prettier '**/*.{json,yml,md}' -l", "test:mjs": "if-ver -lt 12 || exit 0; node --experimental-modules --no-warnings lib/test | tap-mocha-reporter classic", "test:js": "node lib/test | tap-mocha-reporter classic", "prepublishOnly": "npm test" } ,"_resolved": "https://registry.npmjs.org/fs-capacitor/-/fs-capacitor-2.0.4.tgz" ,"_integrity": "sha512-8S4f4WsCryNw2mJJchi46YgB6CR5Ze+4L1h8ewl9tEpL4SJ3ZO+c/bS4BWhB8bK+O3TMqhuZarTitd0S0eh2pA==" ,"_from": "fs-capacitor@2.0.4" } apollo-server-demo/node_modules/fs-capacitor/changelog.md 0000644 0001750 0000144 00000003551 13463756765 023305 0 ustar andreh users # fs-capacitor changelog ## 1.0.0 - Initial release. ### 1.0.1 - Use default fs flags and mode ## 2.0.0 - Updated dependencies. - Add tests for special stream scenarios. - BREAKING: Remove special handling of terminating events, see [jaydenseric/graphql-upload#131](https://github.com/jaydenseric/graphql-upload/issues/131) ### 2.0.1 - Updated dependencies. - Move configs out of package.json - Use `wx` file flag instead of default `w` (thanks to @mattbretl via #8) ### 2.0.2 - Updated dev dependencies. - Fix mjs structure to work with node v12. - Fix a bug that would pause consumption of read streams until completion. (thanks to @Nikosmonaut's investigation in #9). ### 2.0.3 - Emit write event _after_ bytes have been written to the filesystem. ### 2.0.4 - Revert support for Node.js v12 `--experimental-modules` mode that was published in [v2.0.2](https://github.com/mike-marcacci/fs-capacitor/releases/tag/v2.0.2) that broke compatibility with earlier Node.js versions and test both ESM and CJS builds (skipping `--experimental-modules` tests for Node.js v12), via [#11](https://github.com/mike-marcacci/fs-capacitor/pull/11). - Use package `browserslist` field instead of configuring `@babel/preset-env` directly. - Configure `@babel/preset-env` to use shipped proposals and loose mode. - Give dev tool config files `.json` extensions so they can be Prettier linted. - Don't Prettier ignore the `lib` directory; it's meant to be pretty. - Prettier ignore `package.json` and `package-lock.json` so npm can own the formatting. - Configure [`eslint-plugin-node`](https://npm.im/eslint-plugin-node) to resolve `.mjs` before `.js` and other extensions, for compatibility with the pre Node.js v12 `--experimental-modules` behavior. - Don't ESLint ignore `node_modules`, as it's already ignored by default. - Use the `classic` TAP reporter for tests as it has more compact output. apollo-server-demo/node_modules/fs-capacitor/.npmrc 0000644 0001750 0000144 00000000023 13417167754 022134 0 ustar andreh users package-lock=false apollo-server-demo/node_modules/fs-capacitor/.huskyrc.json 0000644 0001750 0000144 00000000062 13463756503 023455 0 ustar andreh users { "hooks": { "pre-commit": "npm test" } } apollo-server-demo/node_modules/fs-capacitor/.eslintrc.json 0000644 0001750 0000144 00000000250 13463756503 023607 0 ustar andreh users { "extends": ["env"], "rules": { "require-jsdoc": "off" }, "settings": { "node": { "tryExtensions": [".mjs", ".js", ".json", ".node"] } } } apollo-server-demo/node_modules/fs-capacitor/.npmignore 0000644 0001750 0000144 00000000124 13417167754 023015 0 ustar andreh users .DS_Store node_modules package-lock.json npm-debug.log yarn.lock yarn-error.log lib apollo-server-demo/node_modules/fs-capacitor/.eslintignore 0000644 0001750 0000144 00000000004 13463756503 023513 0 ustar andreh users lib apollo-server-demo/node_modules/fs-capacitor/.prettierrc.json 0000644 0001750 0000144 00000000033 13463756503 024146 0 ustar andreh users { "proseWrap": "never" } apollo-server-demo/node_modules/fs-capacitor/.travis.yml 0000644 0001750 0000144 00000000136 13463631605 023122 0 ustar andreh users language: node_js node_js: - "8" - "10" - "12" - "node" notifications: email: false apollo-server-demo/node_modules/fs-capacitor/lib/ 0000755 0001750 0000144 00000000000 14067647701 021564 5 ustar andreh users apollo-server-demo/node_modules/fs-capacitor/lib/index.mjs 0000644 0001750 0000144 00000011450 13463757005 023405 0 ustar andreh users import crypto from "crypto"; import fs from "fs"; import os from "os"; import path from "path"; export class ReadAfterDestroyedError extends Error {} export class ReadStream extends fs.ReadStream { constructor(writeStream, name) { super("", {}); this.name = name; this._writeStream = writeStream; this.error = this._writeStream.error; this.addListener("error", error => { this.error = error; }); this.open(); } get ended() { return this._readableState.ended; } _read(n) { if (typeof this.fd !== "number") return this.once("open", function() { this._read(n); }); if (this._writeStream.finished || this._writeStream.closed) return super._read(n); const unread = this._writeStream.bytesWritten - this.bytesRead; if (unread === 0) { const retry = () => { this._writeStream.removeListener("finish", retry); this._writeStream.removeListener("write", retry); this._read(n); }; this._writeStream.addListener("finish", retry); this._writeStream.addListener("write", retry); return; } return super._read(Math.min(n, unread)); } _destroy(error, callback) { if (typeof this.fd !== "number") { this.once("open", this._destroy.bind(this, error, callback)); return; } fs.close(this.fd, closeError => { callback(closeError || error); this.fd = null; this.closed = true; this.emit("close"); }); } open() { if (!this._writeStream) return; if (typeof this._writeStream.fd !== "number") { this._writeStream.once("open", () => this.open()); return; } this.path = this._writeStream.path; super.open(); } } export class WriteStream extends fs.WriteStream { constructor() { super("", { autoClose: false }); this._readStreams = new Set(); this.error = null; this._cleanupSync = () => { process.removeListener("exit", this._cleanupSync); process.removeListener("SIGINT", this._cleanupSync); if (typeof this.fd === "number") try { fs.closeSync(this.fd); } catch (error) {} try { fs.unlinkSync(this.path); } catch (error) {} }; } get finished() { return this._writableState.finished; } open() { crypto.randomBytes(16, (error, buffer) => { if (error) { this.destroy(error); return; } this.path = path.join( os.tmpdir(), `capacitor-${buffer.toString("hex")}.tmp` ); fs.open(this.path, "wx", this.mode, (error, fd) => { if (error) { this.destroy(error); return; } process.addListener("exit", this._cleanupSync); process.addListener("SIGINT", this._cleanupSync); this.fd = fd; this.emit("open", fd); this.emit("ready"); }); }); } _write(chunk, encoding, callback) { super._write(chunk, encoding, error => { if (!error) this.emit("write"); callback(error); }); } _destroy(error, callback) { if (typeof this.fd !== "number") { this.once("open", this._destroy.bind(this, error, callback)); return; } process.removeListener("exit", this._cleanupSync); process.removeListener("SIGINT", this._cleanupSync); const unlink = error => { fs.unlink(this.path, unlinkError => { callback(unlinkError || error); this.fd = null; this.closed = true; this.emit("close"); }); }; if (typeof this.fd === "number") { fs.close(this.fd, closeError => { unlink(closeError || error); }); return; } unlink(error); } destroy(error, callback) { if (error) this.error = error; if (this.destroyed) return super.destroy(error, callback); if (typeof callback === "function") this.once("close", callback.bind(this, error)); if (this._readStreams.size === 0) { super.destroy(error, callback); return; } this._destroyPending = true; if (error) for (let readStream of this._readStreams) readStream.destroy(error); } createReadStream(name) { if (this.destroyed) throw new ReadAfterDestroyedError( "A ReadStream cannot be created from a destroyed WriteStream." ); const readStream = new ReadStream(this, name); this._readStreams.add(readStream); const remove = () => { this._deleteReadStream(readStream); readStream.removeListener("end", remove); readStream.removeListener("close", remove); }; readStream.addListener("end", remove); readStream.addListener("close", remove); return readStream; } _deleteReadStream(readStream) { if (this._readStreams.delete(readStream) && this._destroyPending) this.destroy(); } } export default WriteStream; apollo-server-demo/node_modules/fs-capacitor/lib/index.js 0000644 0001750 0000144 00000012601 13463757005 023227 0 ustar andreh users "use strict"; exports.__esModule = true; exports.default = exports.WriteStream = exports.ReadStream = exports.ReadAfterDestroyedError = void 0; var _crypto = _interopRequireDefault(require("crypto")); var _fs = _interopRequireDefault(require("fs")); var _os = _interopRequireDefault(require("os")); var _path = _interopRequireDefault(require("path")); function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; } class ReadAfterDestroyedError extends Error {} exports.ReadAfterDestroyedError = ReadAfterDestroyedError; class ReadStream extends _fs.default.ReadStream { constructor(writeStream, name) { super("", {}); this.name = name; this._writeStream = writeStream; this.error = this._writeStream.error; this.addListener("error", error => { this.error = error; }); this.open(); } get ended() { return this._readableState.ended; } _read(n) { if (typeof this.fd !== "number") return this.once("open", function() { this._read(n); }); if (this._writeStream.finished || this._writeStream.closed) return super._read(n); const unread = this._writeStream.bytesWritten - this.bytesRead; if (unread === 0) { const retry = () => { this._writeStream.removeListener("finish", retry); this._writeStream.removeListener("write", retry); this._read(n); }; this._writeStream.addListener("finish", retry); this._writeStream.addListener("write", retry); return; } return super._read(Math.min(n, unread)); } _destroy(error, callback) { if (typeof this.fd !== "number") { this.once("open", this._destroy.bind(this, error, callback)); return; } _fs.default.close(this.fd, closeError => { callback(closeError || error); this.fd = null; this.closed = true; this.emit("close"); }); } open() { if (!this._writeStream) return; if (typeof this._writeStream.fd !== "number") { this._writeStream.once("open", () => this.open()); return; } this.path = this._writeStream.path; super.open(); } } exports.ReadStream = ReadStream; class WriteStream extends _fs.default.WriteStream { constructor() { super("", { autoClose: false }); this._readStreams = new Set(); this.error = null; this._cleanupSync = () => { process.removeListener("exit", this._cleanupSync); process.removeListener("SIGINT", this._cleanupSync); if (typeof this.fd === "number") try { _fs.default.closeSync(this.fd); } catch (error) {} try { _fs.default.unlinkSync(this.path); } catch (error) {} }; } get finished() { return this._writableState.finished; } open() { _crypto.default.randomBytes(16, (error, buffer) => { if (error) { this.destroy(error); return; } this.path = _path.default.join( _os.default.tmpdir(), `capacitor-${buffer.toString("hex")}.tmp` ); _fs.default.open(this.path, "wx", this.mode, (error, fd) => { if (error) { this.destroy(error); return; } process.addListener("exit", this._cleanupSync); process.addListener("SIGINT", this._cleanupSync); this.fd = fd; this.emit("open", fd); this.emit("ready"); }); }); } _write(chunk, encoding, callback) { super._write(chunk, encoding, error => { if (!error) this.emit("write"); callback(error); }); } _destroy(error, callback) { if (typeof this.fd !== "number") { this.once("open", this._destroy.bind(this, error, callback)); return; } process.removeListener("exit", this._cleanupSync); process.removeListener("SIGINT", this._cleanupSync); const unlink = error => { _fs.default.unlink(this.path, unlinkError => { callback(unlinkError || error); this.fd = null; this.closed = true; this.emit("close"); }); }; if (typeof this.fd === "number") { _fs.default.close(this.fd, closeError => { unlink(closeError || error); }); return; } unlink(error); } destroy(error, callback) { if (error) this.error = error; if (this.destroyed) return super.destroy(error, callback); if (typeof callback === "function") this.once("close", callback.bind(this, error)); if (this._readStreams.size === 0) { super.destroy(error, callback); return; } this._destroyPending = true; if (error) for (let readStream of this._readStreams) readStream.destroy(error); } createReadStream(name) { if (this.destroyed) throw new ReadAfterDestroyedError( "A ReadStream cannot be created from a destroyed WriteStream." ); const readStream = new ReadStream(this, name); this._readStreams.add(readStream); const remove = () => { this._deleteReadStream(readStream); readStream.removeListener("end", remove); readStream.removeListener("close", remove); }; readStream.addListener("end", remove); readStream.addListener("close", remove); return readStream; } _deleteReadStream(readStream) { if (this._readStreams.delete(readStream) && this._destroyPending) this.destroy(); } } exports.WriteStream = WriteStream; var _default = WriteStream; exports.default = _default; apollo-server-demo/node_modules/fs-capacitor/lib/test.mjs 0000644 0001750 0000144 00000024726 13463757005 023267 0 ustar andreh users import "leaked-handles"; import fs from "fs"; import stream from "stream"; import t from "tap"; import WriteStream, { ReadAfterDestroyedError } from "."; const streamToString = stream => new Promise((resolve, reject) => { let ended = false; let data = ""; stream .on("error", reject) .on("data", chunk => { if (ended) throw new Error("`data` emitted after `end`"); data += chunk; }) .on("end", () => { ended = true; resolve(data); }); }); const waitForBytesWritten = (stream, bytes, resolve) => { if (stream.bytesWritten >= bytes) { setImmediate(resolve); return; } setImmediate(() => waitForBytesWritten(stream, bytes, resolve)); }; t.test("Data from a complete stream.", async t => { let data = ""; const source = new stream.Readable({ read() {} }); const chunk1 = "1".repeat(10); source.push(chunk1); source.push(null); data += chunk1; let capacitor1 = new WriteStream(); t.strictSame( capacitor1._readStreams.size, 0, "should start with 0 read streams" ); source.pipe(capacitor1); const capacitor1Stream1 = capacitor1.createReadStream("capacitor1Stream1"); t.strictSame( capacitor1._readStreams.size, 1, "should attach a new read stream before receiving data" ); const result = await streamToString(capacitor1Stream1); t.sameStrict(result, data, "should stream all data"); t.sameStrict( capacitor1._readStreams.size, 0, "should no longer have any attacheds read streams" ); }); t.test("Data from an open stream, 1 chunk, no read streams.", async t => { let data = ""; const source = new stream.Readable({ read() {} }); let capacitor1 = new WriteStream(); t.strictSame( capacitor1._readStreams.size, 0, "should start with 0 read streams" ); source.pipe(capacitor1); const chunk1 = "1".repeat(10); source.push(chunk1); source.push(null); data += chunk1; const capacitor1Stream1 = capacitor1.createReadStream("capacitor1Stream1"); t.strictSame( capacitor1._readStreams.size, 1, "should attach a new read stream before receiving data" ); const result = await streamToString(capacitor1Stream1); t.sameStrict(result, data, "should stream all data"); t.sameStrict( capacitor1._readStreams.size, 0, "should no longer have any attacheds read streams" ); }); t.test("Data from an open stream, 1 chunk, 1 read stream.", async t => { let data = ""; const source = new stream.Readable({ read() {} }); let capacitor1 = new WriteStream(); t.strictSame( capacitor1._readStreams.size, 0, "should start with 0 read streams" ); source.pipe(capacitor1); const capacitor1Stream1 = capacitor1.createReadStream("capacitor1Stream1"); t.strictSame( capacitor1._readStreams.size, 1, "should attach a new read stream before receiving data" ); const chunk1 = "1".repeat(10); source.push(chunk1); source.push(null); data += chunk1; const result = await streamToString(capacitor1Stream1); t.sameStrict(result, data, "should stream all data"); t.sameStrict( capacitor1._readStreams.size, 0, "should no longer have any attacheds read streams" ); }); const withChunkSize = size => t.test(`--- with chunk size: ${size}`, async t => { let data = ""; const source = new stream.Readable({ read() {} }); let capacitor1; let capacitor1Stream1; await t.test( "can add a read stream before any data has been written", async t => { capacitor1 = new WriteStream(); t.strictSame( capacitor1._readStreams.size, 0, "should start with 0 read streams" ); capacitor1Stream1 = capacitor1.createReadStream("capacitor1Stream1"); t.strictSame( capacitor1._readStreams.size, 1, "should attach a new read stream before receiving data" ); await t.test("creates a temporary file", async t => { t.plan(3); await new Promise(resolve => capacitor1.on("open", resolve)); t.type( capacitor1.path, "string", "capacitor1.path should be a string" ); t.type(capacitor1.fd, "number", "capacitor1.fd should be a number"); t.ok(fs.existsSync(capacitor1.path), "creates a temp file"); }); } ); source.pipe(capacitor1); const chunk1 = "1".repeat(size); source.push(chunk1); data += chunk1; await new Promise(resolve => waitForBytesWritten(capacitor1, size, resolve) ); let capacitor1Stream2; t.test("can add a read stream after data has been written", t => { capacitor1Stream2 = capacitor1.createReadStream("capacitor1Stream2"); t.strictSame( capacitor1._readStreams.size, 2, "should attach a new read stream after first write" ); t.end(); }); const writeEventBytesWritten = new Promise(resolve => { capacitor1.once("write", () => { resolve(capacitor1.bytesWritten); }); }); const chunk2 = "2".repeat(size); source.push(chunk2); data += chunk2; await new Promise(resolve => waitForBytesWritten(capacitor1, 2 * size, resolve) ); await t.test("write event emitted after bytes are written", async t => { t.strictSame( await writeEventBytesWritten, 2 * size, "bytesWritten should include new chunk" ); }); const finished = new Promise(resolve => capacitor1.once("finish", resolve)); source.push(null); await finished; let capacitor1Stream3; let capacitor1Stream4; t.test("can create a read stream after the source has ended", t => { capacitor1Stream3 = capacitor1.createReadStream("capacitor1Stream3"); capacitor1Stream4 = capacitor1.createReadStream("capacitor1Stream4"); t.strictSame( capacitor1._readStreams.size, 4, "should attach new read streams after end" ); t.end(); }); await t.test("streams complete data to a read stream", async t => { const result2 = await streamToString(capacitor1Stream2); t.strictSame( capacitor1Stream2.ended, true, "should mark read stream as ended" ); t.strictSame(result2, data, "should stream complete data"); const result4 = await streamToString(capacitor1Stream4); t.strictSame( capacitor1Stream4.ended, true, "should mark read stream as ended" ); t.strictSame(result4, data, "should stream complete data"); t.strictSame( capacitor1._readStreams.size, 2, "should detach an ended read stream" ); }); await t.test("can destroy a read stream", async t => { await new Promise(resolve => { capacitor1Stream1.once("error", resolve); capacitor1Stream1.destroy(new Error("test")); }); t.strictSame( capacitor1Stream1.destroyed, true, "should mark read stream as destroyed" ); t.type( capacitor1Stream1.error, Error, "should store an error on read stream" ); t.strictSame( capacitor1._readStreams.size, 1, "should detach a destroyed read stream" ); }); t.test("can delay destruction of a capacitor", t => { capacitor1.destroy(null); t.strictSame( capacitor1.destroyed, false, "should not destroy while read streams exist" ); t.strictSame( capacitor1._destroyPending, true, "should mark for future destruction" ); t.end(); }); await t.test("destroys capacitor once no read streams exist", async t => { const readStreamDestroyed = new Promise(resolve => capacitor1Stream3.on("close", resolve) ); const capacitorDestroyed = new Promise(resolve => capacitor1.on("close", resolve) ); capacitor1Stream3.destroy(null); await readStreamDestroyed; t.strictSame( capacitor1Stream3.destroyed, true, "should mark read stream as destroyed" ); t.strictSame( capacitor1Stream3.error, null, "should not store an error on read stream" ); t.strictSame( capacitor1._readStreams.size, 0, "should detach a destroyed read stream" ); await capacitorDestroyed; t.strictSame(capacitor1.closed, true, "should mark capacitor as closed"); t.strictSame(capacitor1.fd, null, "should set fd to null"); t.strictSame( capacitor1.destroyed, true, "should mark capacitor as destroyed" ); t.notOk(fs.existsSync(capacitor1.path), "removes its temp file"); }); t.test("cannot create a read stream after destruction", t => { try { capacitor1.createReadStream(); } catch (error) { t.ok( error instanceof ReadAfterDestroyedError, "should not create a read stream once destroyed" ); t.end(); } }); const capacitor2 = new WriteStream(); const capacitor2Stream1 = capacitor2.createReadStream("capacitor2Stream1"); const capacitor2Stream2 = capacitor2.createReadStream("capacitor2Stream2"); const capacitor2ReadStream1Destroyed = new Promise(resolve => capacitor2Stream1.on("close", resolve) ); const capacitor2Destroyed = new Promise(resolve => capacitor2.on("close", resolve) ); capacitor2Stream1.destroy(); await capacitor2ReadStream1Destroyed; await t.test("propagates errors to attached read streams", async t => { capacitor2.destroy(); await new Promise(resolve => setImmediate(resolve)); t.strictSame( capacitor2Stream2.destroyed, false, "should not immediately mark attached read streams as destroyed" ); capacitor2.destroy(new Error("test")); await capacitor2Destroyed; t.type(capacitor2.error, Error, "should store an error on capacitor"); t.strictSame( capacitor2.destroyed, true, "should mark capacitor as destroyed" ); t.type( capacitor2Stream2.error, Error, "should store an error on attached read streams" ); t.strictSame( capacitor2Stream2.destroyed, true, "should mark attached read streams as destroyed" ); t.strictSame( capacitor2Stream1.error, null, "should not store an error on detached read streams" ); }); }); withChunkSize(10); withChunkSize(100000); apollo-server-demo/node_modules/fs-capacitor/lib/test.js 0000644 0001750 0000144 00000025564 13463757005 023113 0 ustar andreh users "use strict"; require("leaked-handles"); var _fs = _interopRequireDefault(require("fs")); var _stream = _interopRequireDefault(require("stream")); var _tap = _interopRequireDefault(require("tap")); var _ = _interopRequireDefault(require(".")); function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; } const streamToString = stream => new Promise((resolve, reject) => { let ended = false; let data = ""; stream .on("error", reject) .on("data", chunk => { if (ended) throw new Error("`data` emitted after `end`"); data += chunk; }) .on("end", () => { ended = true; resolve(data); }); }); const waitForBytesWritten = (stream, bytes, resolve) => { if (stream.bytesWritten >= bytes) { setImmediate(resolve); return; } setImmediate(() => waitForBytesWritten(stream, bytes, resolve)); }; _tap.default.test("Data from a complete stream.", async t => { let data = ""; const source = new _stream.default.Readable({ read() {} }); const chunk1 = "1".repeat(10); source.push(chunk1); source.push(null); data += chunk1; let capacitor1 = new _.default(); t.strictSame( capacitor1._readStreams.size, 0, "should start with 0 read streams" ); source.pipe(capacitor1); const capacitor1Stream1 = capacitor1.createReadStream("capacitor1Stream1"); t.strictSame( capacitor1._readStreams.size, 1, "should attach a new read stream before receiving data" ); const result = await streamToString(capacitor1Stream1); t.sameStrict(result, data, "should stream all data"); t.sameStrict( capacitor1._readStreams.size, 0, "should no longer have any attacheds read streams" ); }); _tap.default.test( "Data from an open stream, 1 chunk, no read streams.", async t => { let data = ""; const source = new _stream.default.Readable({ read() {} }); let capacitor1 = new _.default(); t.strictSame( capacitor1._readStreams.size, 0, "should start with 0 read streams" ); source.pipe(capacitor1); const chunk1 = "1".repeat(10); source.push(chunk1); source.push(null); data += chunk1; const capacitor1Stream1 = capacitor1.createReadStream("capacitor1Stream1"); t.strictSame( capacitor1._readStreams.size, 1, "should attach a new read stream before receiving data" ); const result = await streamToString(capacitor1Stream1); t.sameStrict(result, data, "should stream all data"); t.sameStrict( capacitor1._readStreams.size, 0, "should no longer have any attacheds read streams" ); } ); _tap.default.test( "Data from an open stream, 1 chunk, 1 read stream.", async t => { let data = ""; const source = new _stream.default.Readable({ read() {} }); let capacitor1 = new _.default(); t.strictSame( capacitor1._readStreams.size, 0, "should start with 0 read streams" ); source.pipe(capacitor1); const capacitor1Stream1 = capacitor1.createReadStream("capacitor1Stream1"); t.strictSame( capacitor1._readStreams.size, 1, "should attach a new read stream before receiving data" ); const chunk1 = "1".repeat(10); source.push(chunk1); source.push(null); data += chunk1; const result = await streamToString(capacitor1Stream1); t.sameStrict(result, data, "should stream all data"); t.sameStrict( capacitor1._readStreams.size, 0, "should no longer have any attacheds read streams" ); } ); const withChunkSize = size => _tap.default.test(`--- with chunk size: ${size}`, async t => { let data = ""; const source = new _stream.default.Readable({ read() {} }); let capacitor1; let capacitor1Stream1; await t.test( "can add a read stream before any data has been written", async t => { capacitor1 = new _.default(); t.strictSame( capacitor1._readStreams.size, 0, "should start with 0 read streams" ); capacitor1Stream1 = capacitor1.createReadStream("capacitor1Stream1"); t.strictSame( capacitor1._readStreams.size, 1, "should attach a new read stream before receiving data" ); await t.test("creates a temporary file", async t => { t.plan(3); await new Promise(resolve => capacitor1.on("open", resolve)); t.type( capacitor1.path, "string", "capacitor1.path should be a string" ); t.type(capacitor1.fd, "number", "capacitor1.fd should be a number"); t.ok(_fs.default.existsSync(capacitor1.path), "creates a temp file"); }); } ); source.pipe(capacitor1); const chunk1 = "1".repeat(size); source.push(chunk1); data += chunk1; await new Promise(resolve => waitForBytesWritten(capacitor1, size, resolve) ); let capacitor1Stream2; t.test("can add a read stream after data has been written", t => { capacitor1Stream2 = capacitor1.createReadStream("capacitor1Stream2"); t.strictSame( capacitor1._readStreams.size, 2, "should attach a new read stream after first write" ); t.end(); }); const writeEventBytesWritten = new Promise(resolve => { capacitor1.once("write", () => { resolve(capacitor1.bytesWritten); }); }); const chunk2 = "2".repeat(size); source.push(chunk2); data += chunk2; await new Promise(resolve => waitForBytesWritten(capacitor1, 2 * size, resolve) ); await t.test("write event emitted after bytes are written", async t => { t.strictSame( await writeEventBytesWritten, 2 * size, "bytesWritten should include new chunk" ); }); const finished = new Promise(resolve => capacitor1.once("finish", resolve)); source.push(null); await finished; let capacitor1Stream3; let capacitor1Stream4; t.test("can create a read stream after the source has ended", t => { capacitor1Stream3 = capacitor1.createReadStream("capacitor1Stream3"); capacitor1Stream4 = capacitor1.createReadStream("capacitor1Stream4"); t.strictSame( capacitor1._readStreams.size, 4, "should attach new read streams after end" ); t.end(); }); await t.test("streams complete data to a read stream", async t => { const result2 = await streamToString(capacitor1Stream2); t.strictSame( capacitor1Stream2.ended, true, "should mark read stream as ended" ); t.strictSame(result2, data, "should stream complete data"); const result4 = await streamToString(capacitor1Stream4); t.strictSame( capacitor1Stream4.ended, true, "should mark read stream as ended" ); t.strictSame(result4, data, "should stream complete data"); t.strictSame( capacitor1._readStreams.size, 2, "should detach an ended read stream" ); }); await t.test("can destroy a read stream", async t => { await new Promise(resolve => { capacitor1Stream1.once("error", resolve); capacitor1Stream1.destroy(new Error("test")); }); t.strictSame( capacitor1Stream1.destroyed, true, "should mark read stream as destroyed" ); t.type( capacitor1Stream1.error, Error, "should store an error on read stream" ); t.strictSame( capacitor1._readStreams.size, 1, "should detach a destroyed read stream" ); }); t.test("can delay destruction of a capacitor", t => { capacitor1.destroy(null); t.strictSame( capacitor1.destroyed, false, "should not destroy while read streams exist" ); t.strictSame( capacitor1._destroyPending, true, "should mark for future destruction" ); t.end(); }); await t.test("destroys capacitor once no read streams exist", async t => { const readStreamDestroyed = new Promise(resolve => capacitor1Stream3.on("close", resolve) ); const capacitorDestroyed = new Promise(resolve => capacitor1.on("close", resolve) ); capacitor1Stream3.destroy(null); await readStreamDestroyed; t.strictSame( capacitor1Stream3.destroyed, true, "should mark read stream as destroyed" ); t.strictSame( capacitor1Stream3.error, null, "should not store an error on read stream" ); t.strictSame( capacitor1._readStreams.size, 0, "should detach a destroyed read stream" ); await capacitorDestroyed; t.strictSame(capacitor1.closed, true, "should mark capacitor as closed"); t.strictSame(capacitor1.fd, null, "should set fd to null"); t.strictSame( capacitor1.destroyed, true, "should mark capacitor as destroyed" ); t.notOk(_fs.default.existsSync(capacitor1.path), "removes its temp file"); }); t.test("cannot create a read stream after destruction", t => { try { capacitor1.createReadStream(); } catch (error) { t.ok( error instanceof _.ReadAfterDestroyedError, "should not create a read stream once destroyed" ); t.end(); } }); const capacitor2 = new _.default(); const capacitor2Stream1 = capacitor2.createReadStream("capacitor2Stream1"); const capacitor2Stream2 = capacitor2.createReadStream("capacitor2Stream2"); const capacitor2ReadStream1Destroyed = new Promise(resolve => capacitor2Stream1.on("close", resolve) ); const capacitor2Destroyed = new Promise(resolve => capacitor2.on("close", resolve) ); capacitor2Stream1.destroy(); await capacitor2ReadStream1Destroyed; await t.test("propagates errors to attached read streams", async t => { capacitor2.destroy(); await new Promise(resolve => setImmediate(resolve)); t.strictSame( capacitor2Stream2.destroyed, false, "should not immediately mark attached read streams as destroyed" ); capacitor2.destroy(new Error("test")); await capacitor2Destroyed; t.type(capacitor2.error, Error, "should store an error on capacitor"); t.strictSame( capacitor2.destroyed, true, "should mark capacitor as destroyed" ); t.type( capacitor2Stream2.error, Error, "should store an error on attached read streams" ); t.strictSame( capacitor2Stream2.destroyed, true, "should mark attached read streams as destroyed" ); t.strictSame( capacitor2Stream1.error, null, "should not store an error on detached read streams" ); }); }); withChunkSize(10); withChunkSize(100000); apollo-server-demo/node_modules/fs-capacitor/yarn.lock 0000644 0001750 0000144 00000675076 13463756512 022666 0 ustar andreh users # THIS IS AN AUTOGENERATED FILE. DO NOT EDIT THIS FILE DIRECTLY. # yarn lockfile v1 "@babel/cli@^7.1.2": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/cli/-/cli-7.4.4.tgz#5454bb7112f29026a4069d8e6f0e1794e651966c" integrity sha512-XGr5YjQSjgTa6OzQZY57FAJsdeVSAKR/u/KA5exWIz66IKtv/zXtHy+fIZcMry/EgYegwuHE7vzGnrFhjdIAsQ== dependencies: commander "^2.8.1" convert-source-map "^1.1.0" fs-readdir-recursive "^1.1.0" glob "^7.0.0" lodash "^4.17.11" mkdirp "^0.5.1" output-file-sync "^2.0.0" slash "^2.0.0" source-map "^0.5.0" optionalDependencies: chokidar "^2.0.4" "@babel/code-frame@^7.0.0": version "7.0.0" resolved "https://registry.yarnpkg.com/@babel/code-frame/-/code-frame-7.0.0.tgz#06e2ab19bdb535385559aabb5ba59729482800f8" integrity sha512-OfC2uemaknXr87bdLUkWog7nYuliM9Ij5HUcajsVcMCpQrcLmtxRbVFTIqmcSkSeYRBFBRxs2FiUqFJDLdiebA== dependencies: "@babel/highlight" "^7.0.0" "@babel/core@^7.3.3": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/core/-/core-7.4.4.tgz#84055750b05fcd50f9915a826b44fa347a825250" integrity sha512-lQgGX3FPRgbz2SKmhMtYgJvVzGZrmjaF4apZ2bLwofAKiSjxU0drPh4S/VasyYXwaTs+A1gvQ45BN8SQJzHsQQ== dependencies: "@babel/code-frame" "^7.0.0" "@babel/generator" "^7.4.4" "@babel/helpers" "^7.4.4" "@babel/parser" "^7.4.4" "@babel/template" "^7.4.4" "@babel/traverse" "^7.4.4" "@babel/types" "^7.4.4" convert-source-map "^1.1.0" debug "^4.1.0" json5 "^2.1.0" lodash "^4.17.11" resolve "^1.3.2" semver "^5.4.1" source-map "^0.5.0" "@babel/generator@^7.2.2": version "7.2.2" resolved "https://registry.yarnpkg.com/@babel/generator/-/generator-7.2.2.tgz#18c816c70962640eab42fe8cae5f3947a5c65ccc" integrity sha512-I4o675J/iS8k+P38dvJ3IBGqObLXyQLTxtrR4u9cSUJOURvafeEWb/pFMOTwtNrmq73mJzyF6ueTbO1BtN0Zeg== dependencies: "@babel/types" "^7.2.2" jsesc "^2.5.1" lodash "^4.17.10" source-map "^0.5.0" trim-right "^1.0.1" "@babel/generator@^7.4.0", "@babel/generator@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/generator/-/generator-7.4.4.tgz#174a215eb843fc392c7edcaabeaa873de6e8f041" integrity sha512-53UOLK6TVNqKxf7RUh8NE851EHRxOOeVXKbK2bivdb+iziMyk03Sr4eaE9OELCbyZAAafAKPDwF2TPUES5QbxQ== dependencies: "@babel/types" "^7.4.4" jsesc "^2.5.1" lodash "^4.17.11" source-map "^0.5.0" trim-right "^1.0.1" "@babel/helper-annotate-as-pure@^7.0.0": version "7.0.0" resolved "https://registry.yarnpkg.com/@babel/helper-annotate-as-pure/-/helper-annotate-as-pure-7.0.0.tgz#323d39dd0b50e10c7c06ca7d7638e6864d8c5c32" integrity sha512-3UYcJUj9kvSLbLbUIfQTqzcy5VX7GRZ/CCDrnOaZorFFM01aXp1+GJwuFGV4NDDoAS+mOUyHcO6UD/RfqOks3Q== dependencies: "@babel/types" "^7.0.0" "@babel/helper-builder-binary-assignment-operator-visitor@^7.1.0": version "7.1.0" resolved "https://registry.yarnpkg.com/@babel/helper-builder-binary-assignment-operator-visitor/-/helper-builder-binary-assignment-operator-visitor-7.1.0.tgz#6b69628dfe4087798e0c4ed98e3d4a6b2fbd2f5f" integrity sha512-qNSR4jrmJ8M1VMM9tibvyRAHXQs2PmaksQF7c1CGJNipfe3D8p+wgNwgso/P2A2r2mdgBWAXljNWR0QRZAMW8w== dependencies: "@babel/helper-explode-assignable-expression" "^7.1.0" "@babel/types" "^7.0.0" "@babel/helper-call-delegate@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/helper-call-delegate/-/helper-call-delegate-7.4.4.tgz#87c1f8ca19ad552a736a7a27b1c1fcf8b1ff1f43" integrity sha512-l79boDFJ8S1c5hvQvG+rc+wHw6IuH7YldmRKsYtpbawsxURu/paVy57FZMomGK22/JckepaikOkY0MoAmdyOlQ== dependencies: "@babel/helper-hoist-variables" "^7.4.4" "@babel/traverse" "^7.4.4" "@babel/types" "^7.4.4" "@babel/helper-define-map@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/helper-define-map/-/helper-define-map-7.4.4.tgz#6969d1f570b46bdc900d1eba8e5d59c48ba2c12a" integrity sha512-IX3Ln8gLhZpSuqHJSnTNBWGDE9kdkTEWl21A/K7PQ00tseBwbqCHTvNLHSBd9M0R5rER4h5Rsvj9vw0R5SieBg== dependencies: "@babel/helper-function-name" "^7.1.0" "@babel/types" "^7.4.4" lodash "^4.17.11" "@babel/helper-explode-assignable-expression@^7.1.0": version "7.1.0" resolved "https://registry.yarnpkg.com/@babel/helper-explode-assignable-expression/-/helper-explode-assignable-expression-7.1.0.tgz#537fa13f6f1674df745b0c00ec8fe4e99681c8f6" integrity sha512-NRQpfHrJ1msCHtKjbzs9YcMmJZOg6mQMmGRB+hbamEdG5PNpaSm95275VD92DvJKuyl0s2sFiDmMZ+EnnvufqA== dependencies: "@babel/traverse" "^7.1.0" "@babel/types" "^7.0.0" "@babel/helper-function-name@^7.1.0": version "7.1.0" resolved "https://registry.yarnpkg.com/@babel/helper-function-name/-/helper-function-name-7.1.0.tgz#a0ceb01685f73355d4360c1247f582bfafc8ff53" integrity sha512-A95XEoCpb3TO+KZzJ4S/5uW5fNe26DjBGqf1o9ucyLyCmi1dXq/B3c8iaWTfBk3VvetUxl16e8tIrd5teOCfGw== dependencies: "@babel/helper-get-function-arity" "^7.0.0" "@babel/template" "^7.1.0" "@babel/types" "^7.0.0" "@babel/helper-get-function-arity@^7.0.0": version "7.0.0" resolved "https://registry.yarnpkg.com/@babel/helper-get-function-arity/-/helper-get-function-arity-7.0.0.tgz#83572d4320e2a4657263734113c42868b64e49c3" integrity sha512-r2DbJeg4svYvt3HOS74U4eWKsUAMRH01Z1ds1zx8KNTPtpTL5JAsdFv8BNyOpVqdFhHkkRDIg5B4AsxmkjAlmQ== dependencies: "@babel/types" "^7.0.0" "@babel/helper-hoist-variables@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/helper-hoist-variables/-/helper-hoist-variables-7.4.4.tgz#0298b5f25c8c09c53102d52ac4a98f773eb2850a" integrity sha512-VYk2/H/BnYbZDDg39hr3t2kKyifAm1W6zHRfhx8jGjIHpQEBv9dry7oQ2f3+J703TLu69nYdxsovl0XYfcnK4w== dependencies: "@babel/types" "^7.4.4" "@babel/helper-member-expression-to-functions@^7.0.0": version "7.0.0" resolved "https://registry.yarnpkg.com/@babel/helper-member-expression-to-functions/-/helper-member-expression-to-functions-7.0.0.tgz#8cd14b0a0df7ff00f009e7d7a436945f47c7a16f" integrity sha512-avo+lm/QmZlv27Zsi0xEor2fKcqWG56D5ae9dzklpIaY7cQMK5N8VSpaNVPPagiqmy7LrEjK1IWdGMOqPu5csg== dependencies: "@babel/types" "^7.0.0" "@babel/helper-module-imports@^7.0.0": version "7.0.0" resolved "https://registry.yarnpkg.com/@babel/helper-module-imports/-/helper-module-imports-7.0.0.tgz#96081b7111e486da4d2cd971ad1a4fe216cc2e3d" integrity sha512-aP/hlLq01DWNEiDg4Jn23i+CXxW/owM4WpDLFUbpjxe4NS3BhLVZQ5i7E0ZrxuQ/vwekIeciyamgB1UIYxxM6A== dependencies: "@babel/types" "^7.0.0" "@babel/helper-module-transforms@^7.1.0", "@babel/helper-module-transforms@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/helper-module-transforms/-/helper-module-transforms-7.4.4.tgz#96115ea42a2f139e619e98ed46df6019b94414b8" integrity sha512-3Z1yp8TVQf+B4ynN7WoHPKS8EkdTbgAEy0nU0rs/1Kw4pDgmvYH3rz3aI11KgxKCba2cn7N+tqzV1mY2HMN96w== dependencies: "@babel/helper-module-imports" "^7.0.0" "@babel/helper-simple-access" "^7.1.0" "@babel/helper-split-export-declaration" "^7.4.4" "@babel/template" "^7.4.4" "@babel/types" "^7.4.4" lodash "^4.17.11" "@babel/helper-optimise-call-expression@^7.0.0": version "7.0.0" resolved "https://registry.yarnpkg.com/@babel/helper-optimise-call-expression/-/helper-optimise-call-expression-7.0.0.tgz#a2920c5702b073c15de51106200aa8cad20497d5" integrity sha512-u8nd9NQePYNQV8iPWu/pLLYBqZBa4ZaY1YWRFMuxrid94wKI1QNt67NEZ7GAe5Kc/0LLScbim05xZFWkAdrj9g== dependencies: "@babel/types" "^7.0.0" "@babel/helper-plugin-utils@^7.0.0": version "7.0.0" resolved "https://registry.yarnpkg.com/@babel/helper-plugin-utils/-/helper-plugin-utils-7.0.0.tgz#bbb3fbee98661c569034237cc03967ba99b4f250" integrity sha512-CYAOUCARwExnEixLdB6sDm2dIJ/YgEAKDM1MOeMeZu9Ld/bDgVo8aiWrXwcY7OBh+1Ea2uUcVRcxKk0GJvW7QA== "@babel/helper-regex@^7.0.0", "@babel/helper-regex@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/helper-regex/-/helper-regex-7.4.4.tgz#a47e02bc91fb259d2e6727c2a30013e3ac13c4a2" integrity sha512-Y5nuB/kESmR3tKjU8Nkn1wMGEx1tjJX076HBMeL3XLQCu6vA/YRzuTW0bbb+qRnXvQGn+d6Rx953yffl8vEy7Q== dependencies: lodash "^4.17.11" "@babel/helper-remap-async-to-generator@^7.1.0": version "7.1.0" resolved "https://registry.yarnpkg.com/@babel/helper-remap-async-to-generator/-/helper-remap-async-to-generator-7.1.0.tgz#361d80821b6f38da75bd3f0785ece20a88c5fe7f" integrity sha512-3fOK0L+Fdlg8S5al8u/hWE6vhufGSn0bN09xm2LXMy//REAF8kDCrYoOBKYmA8m5Nom+sV9LyLCwrFynA8/slg== dependencies: "@babel/helper-annotate-as-pure" "^7.0.0" "@babel/helper-wrap-function" "^7.1.0" "@babel/template" "^7.1.0" "@babel/traverse" "^7.1.0" "@babel/types" "^7.0.0" "@babel/helper-replace-supers@^7.1.0", "@babel/helper-replace-supers@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/helper-replace-supers/-/helper-replace-supers-7.4.4.tgz#aee41783ebe4f2d3ab3ae775e1cc6f1a90cefa27" integrity sha512-04xGEnd+s01nY1l15EuMS1rfKktNF+1CkKmHoErDppjAAZL+IUBZpzT748x262HF7fibaQPhbvWUl5HeSt1EXg== dependencies: "@babel/helper-member-expression-to-functions" "^7.0.0" "@babel/helper-optimise-call-expression" "^7.0.0" "@babel/traverse" "^7.4.4" "@babel/types" "^7.4.4" "@babel/helper-simple-access@^7.1.0": version "7.1.0" resolved "https://registry.yarnpkg.com/@babel/helper-simple-access/-/helper-simple-access-7.1.0.tgz#65eeb954c8c245beaa4e859da6188f39d71e585c" integrity sha512-Vk+78hNjRbsiu49zAPALxTb+JUQCz1aolpd8osOF16BGnLtseD21nbHgLPGUwrXEurZgiCOUmvs3ExTu4F5x6w== dependencies: "@babel/template" "^7.1.0" "@babel/types" "^7.0.0" "@babel/helper-split-export-declaration@^7.0.0": version "7.0.0" resolved "https://registry.yarnpkg.com/@babel/helper-split-export-declaration/-/helper-split-export-declaration-7.0.0.tgz#3aae285c0311c2ab095d997b8c9a94cad547d813" integrity sha512-MXkOJqva62dfC0w85mEf/LucPPS/1+04nmmRMPEBUB++hiiThQ2zPtX/mEWQ3mtzCEjIJvPY8nuwxXtQeQwUag== dependencies: "@babel/types" "^7.0.0" "@babel/helper-split-export-declaration@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/helper-split-export-declaration/-/helper-split-export-declaration-7.4.4.tgz#ff94894a340be78f53f06af038b205c49d993677" integrity sha512-Ro/XkzLf3JFITkW6b+hNxzZ1n5OQ80NvIUdmHspih1XAhtN3vPTuUFT4eQnela+2MaZ5ulH+iyP513KJrxbN7Q== dependencies: "@babel/types" "^7.4.4" "@babel/helper-wrap-function@^7.1.0": version "7.2.0" resolved "https://registry.yarnpkg.com/@babel/helper-wrap-function/-/helper-wrap-function-7.2.0.tgz#c4e0012445769e2815b55296ead43a958549f6fa" integrity sha512-o9fP1BZLLSrYlxYEYyl2aS+Flun5gtjTIG8iln+XuEzQTs0PLagAGSXUcqruJwD5fM48jzIEggCKpIfWTcR7pQ== dependencies: "@babel/helper-function-name" "^7.1.0" "@babel/template" "^7.1.0" "@babel/traverse" "^7.1.0" "@babel/types" "^7.2.0" "@babel/helpers@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/helpers/-/helpers-7.4.4.tgz#868b0ef59c1dd4e78744562d5ce1b59c89f2f2a5" integrity sha512-igczbR/0SeuPR8RFfC7tGrbdTbFL3QTvH6D+Z6zNxnTe//GyqmtHmDkzrqDmyZ3eSwPqB/LhyKoU5DXsp+Vp2A== dependencies: "@babel/template" "^7.4.4" "@babel/traverse" "^7.4.4" "@babel/types" "^7.4.4" "@babel/highlight@^7.0.0": version "7.0.0" resolved "https://registry.yarnpkg.com/@babel/highlight/-/highlight-7.0.0.tgz#f710c38c8d458e6dd9a201afb637fcb781ce99e4" integrity sha512-UFMC4ZeFC48Tpvj7C8UgLvtkaUuovQX+5xNWrsIoMG8o2z+XFKjKaN9iVmS84dPwVN00W4wPmqvYoZF3EGAsfw== dependencies: chalk "^2.0.0" esutils "^2.0.2" js-tokens "^4.0.0" "@babel/parser@^7.0.0", "@babel/parser@^7.2.2", "@babel/parser@^7.2.3": version "7.2.3" resolved "https://registry.yarnpkg.com/@babel/parser/-/parser-7.2.3.tgz#32f5df65744b70888d17872ec106b02434ba1489" integrity sha512-0LyEcVlfCoFmci8mXx8A5oIkpkOgyo8dRHtxBnK9RRBwxO2+JZPNsqtVEZQ7mJFPxnXF9lfmU24mHOPI0qnlkA== "@babel/parser@^7.4.3", "@babel/parser@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/parser/-/parser-7.4.4.tgz#5977129431b8fe33471730d255ce8654ae1250b6" integrity sha512-5pCS4mOsL+ANsFZGdvNLybx4wtqAZJ0MJjMHxvzI3bvIsz6sQvzW8XX92EYIkiPtIvcfG3Aj+Ir5VNyjnZhP7w== "@babel/plugin-proposal-async-generator-functions@^7.2.0": version "7.2.0" resolved "https://registry.yarnpkg.com/@babel/plugin-proposal-async-generator-functions/-/plugin-proposal-async-generator-functions-7.2.0.tgz#b289b306669dce4ad20b0252889a15768c9d417e" integrity sha512-+Dfo/SCQqrwx48ptLVGLdE39YtWRuKc/Y9I5Fy0P1DDBB9lsAHpjcEJQt+4IifuSOSTLBKJObJqMvaO1pIE8LQ== dependencies: "@babel/helper-plugin-utils" "^7.0.0" "@babel/helper-remap-async-to-generator" "^7.1.0" "@babel/plugin-syntax-async-generators" "^7.2.0" "@babel/plugin-proposal-json-strings@^7.2.0": version "7.2.0" resolved "https://registry.yarnpkg.com/@babel/plugin-proposal-json-strings/-/plugin-proposal-json-strings-7.2.0.tgz#568ecc446c6148ae6b267f02551130891e29f317" integrity sha512-MAFV1CA/YVmYwZG0fBQyXhmj0BHCB5egZHCKWIFVv/XCxAeVGIHfos3SwDck4LvCllENIAg7xMKOG5kH0dzyUg== dependencies: "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-syntax-json-strings" "^7.2.0" "@babel/plugin-proposal-object-rest-spread@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/plugin-proposal-object-rest-spread/-/plugin-proposal-object-rest-spread-7.4.4.tgz#1ef173fcf24b3e2df92a678f027673b55e7e3005" integrity sha512-dMBG6cSPBbHeEBdFXeQ2QLc5gUpg4Vkaz8octD4aoW/ISO+jBOcsuxYL7bsb5WSu8RLP6boxrBIALEHgoHtO9g== dependencies: "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-syntax-object-rest-spread" "^7.2.0" "@babel/plugin-proposal-optional-catch-binding@^7.2.0": version "7.2.0" resolved "https://registry.yarnpkg.com/@babel/plugin-proposal-optional-catch-binding/-/plugin-proposal-optional-catch-binding-7.2.0.tgz#135d81edb68a081e55e56ec48541ece8065c38f5" integrity sha512-mgYj3jCcxug6KUcX4OBoOJz3CMrwRfQELPQ5560F70YQUBZB7uac9fqaWamKR1iWUzGiK2t0ygzjTScZnVz75g== dependencies: "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-syntax-optional-catch-binding" "^7.2.0" "@babel/plugin-proposal-unicode-property-regex@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/plugin-proposal-unicode-property-regex/-/plugin-proposal-unicode-property-regex-7.4.4.tgz#501ffd9826c0b91da22690720722ac7cb1ca9c78" integrity sha512-j1NwnOqMG9mFUOH58JTFsA/+ZYzQLUZ/drqWUqxCYLGeu2JFZL8YrNC9hBxKmWtAuOCHPcRpgv7fhap09Fb4kA== dependencies: "@babel/helper-plugin-utils" "^7.0.0" "@babel/helper-regex" "^7.4.4" regexpu-core "^4.5.4" "@babel/plugin-syntax-async-generators@^7.2.0": version "7.2.0" resolved "https://registry.yarnpkg.com/@babel/plugin-syntax-async-generators/-/plugin-syntax-async-generators-7.2.0.tgz#69e1f0db34c6f5a0cf7e2b3323bf159a76c8cb7f" integrity sha512-1ZrIRBv2t0GSlcwVoQ6VgSLpLgiN/FVQUzt9znxo7v2Ov4jJrs8RY8tv0wvDmFN3qIdMKWrmMMW6yZ0G19MfGg== dependencies: "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-syntax-json-strings@^7.2.0": version "7.2.0" resolved "https://registry.yarnpkg.com/@babel/plugin-syntax-json-strings/-/plugin-syntax-json-strings-7.2.0.tgz#72bd13f6ffe1d25938129d2a186b11fd62951470" integrity sha512-5UGYnMSLRE1dqqZwug+1LISpA403HzlSfsg6P9VXU6TBjcSHeNlw4DxDx7LgpF+iKZoOG/+uzqoRHTdcUpiZNg== dependencies: "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-syntax-object-rest-spread@^7.2.0": version "7.2.0" resolved "https://registry.yarnpkg.com/@babel/plugin-syntax-object-rest-spread/-/plugin-syntax-object-rest-spread-7.2.0.tgz#3b7a3e733510c57e820b9142a6579ac8b0dfad2e" integrity sha512-t0JKGgqk2We+9may3t0xDdmneaXmyxq0xieYcKHxIsrJO64n1OiMWNUtc5gQK1PA0NpdCRrtZp4z+IUaKugrSA== dependencies: "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-syntax-optional-catch-binding@^7.2.0": version "7.2.0" resolved "https://registry.yarnpkg.com/@babel/plugin-syntax-optional-catch-binding/-/plugin-syntax-optional-catch-binding-7.2.0.tgz#a94013d6eda8908dfe6a477e7f9eda85656ecf5c" integrity sha512-bDe4xKNhb0LI7IvZHiA13kff0KEfaGX/Hv4lMA9+7TEc63hMNvfKo6ZFpXhKuEp+II/q35Gc4NoMeDZyaUbj9w== dependencies: "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-transform-arrow-functions@^7.2.0": version "7.2.0" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-arrow-functions/-/plugin-transform-arrow-functions-7.2.0.tgz#9aeafbe4d6ffc6563bf8f8372091628f00779550" integrity sha512-ER77Cax1+8/8jCB9fo4Ud161OZzWN5qawi4GusDuRLcDbDG+bIGYY20zb2dfAFdTRGzrfq2xZPvF0R64EHnimg== dependencies: "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-transform-async-to-generator@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-async-to-generator/-/plugin-transform-async-to-generator-7.4.4.tgz#a3f1d01f2f21cadab20b33a82133116f14fb5894" integrity sha512-YiqW2Li8TXmzgbXw+STsSqPBPFnGviiaSp6CYOq55X8GQ2SGVLrXB6pNid8HkqkZAzOH6knbai3snhP7v0fNwA== dependencies: "@babel/helper-module-imports" "^7.0.0" "@babel/helper-plugin-utils" "^7.0.0" "@babel/helper-remap-async-to-generator" "^7.1.0" "@babel/plugin-transform-block-scoped-functions@^7.2.0": version "7.2.0" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-block-scoped-functions/-/plugin-transform-block-scoped-functions-7.2.0.tgz#5d3cc11e8d5ddd752aa64c9148d0db6cb79fd190" integrity sha512-ntQPR6q1/NKuphly49+QiQiTN0O63uOwjdD6dhIjSWBI5xlrbUFh720TIpzBhpnrLfv2tNH/BXvLIab1+BAI0w== dependencies: "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-transform-block-scoping@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-block-scoping/-/plugin-transform-block-scoping-7.4.4.tgz#c13279fabf6b916661531841a23c4b7dae29646d" integrity sha512-jkTUyWZcTrwxu5DD4rWz6rDB5Cjdmgz6z7M7RLXOJyCUkFBawssDGcGh8M/0FTSB87avyJI1HsTwUXp9nKA1PA== dependencies: "@babel/helper-plugin-utils" "^7.0.0" lodash "^4.17.11" "@babel/plugin-transform-classes@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-classes/-/plugin-transform-classes-7.4.4.tgz#0ce4094cdafd709721076d3b9c38ad31ca715eb6" integrity sha512-/e44eFLImEGIpL9qPxSRat13I5QNRgBLu2hOQJCF7VLy/otSM/sypV1+XaIw5+502RX/+6YaSAPmldk+nhHDPw== dependencies: "@babel/helper-annotate-as-pure" "^7.0.0" "@babel/helper-define-map" "^7.4.4" "@babel/helper-function-name" "^7.1.0" "@babel/helper-optimise-call-expression" "^7.0.0" "@babel/helper-plugin-utils" "^7.0.0" "@babel/helper-replace-supers" "^7.4.4" "@babel/helper-split-export-declaration" "^7.4.4" globals "^11.1.0" "@babel/plugin-transform-computed-properties@^7.2.0": version "7.2.0" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-computed-properties/-/plugin-transform-computed-properties-7.2.0.tgz#83a7df6a658865b1c8f641d510c6f3af220216da" integrity sha512-kP/drqTxY6Xt3NNpKiMomfgkNn4o7+vKxK2DDKcBG9sHj51vHqMBGy8wbDS/J4lMxnqs153/T3+DmCEAkC5cpA== dependencies: "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-transform-destructuring@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-destructuring/-/plugin-transform-destructuring-7.4.4.tgz#9d964717829cc9e4b601fc82a26a71a4d8faf20f" integrity sha512-/aOx+nW0w8eHiEHm+BTERB2oJn5D127iye/SUQl7NjHy0lf+j7h4MKMMSOwdazGq9OxgiNADncE+SRJkCxjZpQ== dependencies: "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-transform-dotall-regex@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-dotall-regex/-/plugin-transform-dotall-regex-7.4.4.tgz#361a148bc951444312c69446d76ed1ea8e4450c3" integrity sha512-P05YEhRc2h53lZDjRPk/OektxCVevFzZs2Gfjd545Wde3k+yFDbXORgl2e0xpbq8mLcKJ7Idss4fAg0zORN/zg== dependencies: "@babel/helper-plugin-utils" "^7.0.0" "@babel/helper-regex" "^7.4.4" regexpu-core "^4.5.4" "@babel/plugin-transform-duplicate-keys@^7.2.0": version "7.2.0" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-duplicate-keys/-/plugin-transform-duplicate-keys-7.2.0.tgz#d952c4930f312a4dbfff18f0b2914e60c35530b3" integrity sha512-q+yuxW4DsTjNceUiTzK0L+AfQ0zD9rWaTLiUqHA8p0gxx7lu1EylenfzjeIWNkPy6e/0VG/Wjw9uf9LueQwLOw== dependencies: "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-transform-exponentiation-operator@^7.2.0": version "7.2.0" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-exponentiation-operator/-/plugin-transform-exponentiation-operator-7.2.0.tgz#a63868289e5b4007f7054d46491af51435766008" integrity sha512-umh4hR6N7mu4Elq9GG8TOu9M0bakvlsREEC+ialrQN6ABS4oDQ69qJv1VtR3uxlKMCQMCvzk7vr17RHKcjx68A== dependencies: "@babel/helper-builder-binary-assignment-operator-visitor" "^7.1.0" "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-transform-for-of@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-for-of/-/plugin-transform-for-of-7.4.4.tgz#0267fc735e24c808ba173866c6c4d1440fc3c556" integrity sha512-9T/5Dlr14Z9TIEXLXkt8T1DU7F24cbhwhMNUziN3hB1AXoZcdzPcTiKGRn/6iOymDqtTKWnr/BtRKN9JwbKtdQ== dependencies: "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-transform-function-name@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-function-name/-/plugin-transform-function-name-7.4.4.tgz#e1436116abb0610c2259094848754ac5230922ad" integrity sha512-iU9pv7U+2jC9ANQkKeNF6DrPy4GBa4NWQtl6dHB4Pb3izX2JOEvDTFarlNsBj/63ZEzNNIAMs3Qw4fNCcSOXJA== dependencies: "@babel/helper-function-name" "^7.1.0" "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-transform-literals@^7.2.0": version "7.2.0" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-literals/-/plugin-transform-literals-7.2.0.tgz#690353e81f9267dad4fd8cfd77eafa86aba53ea1" integrity sha512-2ThDhm4lI4oV7fVQ6pNNK+sx+c/GM5/SaML0w/r4ZB7sAneD/piDJtwdKlNckXeyGK7wlwg2E2w33C/Hh+VFCg== dependencies: "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-transform-member-expression-literals@^7.2.0": version "7.2.0" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-member-expression-literals/-/plugin-transform-member-expression-literals-7.2.0.tgz#fa10aa5c58a2cb6afcf2c9ffa8cb4d8b3d489a2d" integrity sha512-HiU3zKkSU6scTidmnFJ0bMX8hz5ixC93b4MHMiYebmk2lUVNGOboPsqQvx5LzooihijUoLR/v7Nc1rbBtnc7FA== dependencies: "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-transform-modules-amd@^7.2.0": version "7.2.0" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-modules-amd/-/plugin-transform-modules-amd-7.2.0.tgz#82a9bce45b95441f617a24011dc89d12da7f4ee6" integrity sha512-mK2A8ucqz1qhrdqjS9VMIDfIvvT2thrEsIQzbaTdc5QFzhDjQv2CkJJ5f6BXIkgbmaoax3zBr2RyvV/8zeoUZw== dependencies: "@babel/helper-module-transforms" "^7.1.0" "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-transform-modules-commonjs@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-modules-commonjs/-/plugin-transform-modules-commonjs-7.4.4.tgz#0bef4713d30f1d78c2e59b3d6db40e60192cac1e" integrity sha512-4sfBOJt58sEo9a2BQXnZq+Q3ZTSAUXyK3E30o36BOGnJ+tvJ6YSxF0PG6kERvbeISgProodWuI9UVG3/FMY6iw== dependencies: "@babel/helper-module-transforms" "^7.4.4" "@babel/helper-plugin-utils" "^7.0.0" "@babel/helper-simple-access" "^7.1.0" "@babel/plugin-transform-modules-systemjs@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-modules-systemjs/-/plugin-transform-modules-systemjs-7.4.4.tgz#dc83c5665b07d6c2a7b224c00ac63659ea36a405" integrity sha512-MSiModfILQc3/oqnG7NrP1jHaSPryO6tA2kOMmAQApz5dayPxWiHqmq4sWH2xF5LcQK56LlbKByCd8Aah/OIkQ== dependencies: "@babel/helper-hoist-variables" "^7.4.4" "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-transform-modules-umd@^7.2.0": version "7.2.0" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-modules-umd/-/plugin-transform-modules-umd-7.2.0.tgz#7678ce75169f0877b8eb2235538c074268dd01ae" integrity sha512-BV3bw6MyUH1iIsGhXlOK6sXhmSarZjtJ/vMiD9dNmpY8QXFFQTj+6v92pcfy1iqa8DeAfJFwoxcrS/TUZda6sw== dependencies: "@babel/helper-module-transforms" "^7.1.0" "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-transform-named-capturing-groups-regex@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-named-capturing-groups-regex/-/plugin-transform-named-capturing-groups-regex-7.4.4.tgz#5611d96d987dfc4a3a81c4383bb173361037d68d" integrity sha512-Ki+Y9nXBlKfhD+LXaRS7v95TtTGYRAf9Y1rTDiE75zf8YQz4GDaWRXosMfJBXxnk88mGFjWdCRIeqDbon7spYA== dependencies: regexp-tree "^0.1.0" "@babel/plugin-transform-new-target@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-new-target/-/plugin-transform-new-target-7.4.4.tgz#18d120438b0cc9ee95a47f2c72bc9768fbed60a5" integrity sha512-r1z3T2DNGQwwe2vPGZMBNjioT2scgWzK9BCnDEh+46z8EEwXBq24uRzd65I7pjtugzPSj921aM15RpESgzsSuA== dependencies: "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-transform-object-super@^7.2.0": version "7.2.0" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-object-super/-/plugin-transform-object-super-7.2.0.tgz#b35d4c10f56bab5d650047dad0f1d8e8814b6598" integrity sha512-VMyhPYZISFZAqAPVkiYb7dUe2AsVi2/wCT5+wZdsNO31FojQJa9ns40hzZ6U9f50Jlq4w6qwzdBB2uwqZ00ebg== dependencies: "@babel/helper-plugin-utils" "^7.0.0" "@babel/helper-replace-supers" "^7.1.0" "@babel/plugin-transform-parameters@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-parameters/-/plugin-transform-parameters-7.4.4.tgz#7556cf03f318bd2719fe4c922d2d808be5571e16" integrity sha512-oMh5DUO1V63nZcu/ZVLQFqiihBGo4OpxJxR1otF50GMeCLiRx5nUdtokd+u9SuVJrvvuIh9OosRFPP4pIPnwmw== dependencies: "@babel/helper-call-delegate" "^7.4.4" "@babel/helper-get-function-arity" "^7.0.0" "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-transform-property-literals@^7.2.0": version "7.2.0" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-property-literals/-/plugin-transform-property-literals-7.2.0.tgz#03e33f653f5b25c4eb572c98b9485055b389e905" integrity sha512-9q7Dbk4RhgcLp8ebduOpCbtjh7C0itoLYHXd9ueASKAG/is5PQtMR5VJGka9NKqGhYEGn5ITahd4h9QeBMylWQ== dependencies: "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-transform-regenerator@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-regenerator/-/plugin-transform-regenerator-7.4.4.tgz#5b4da4df79391895fca9e28f99e87e22cfc02072" integrity sha512-Zz3w+pX1SI0KMIiqshFZkwnVGUhDZzpX2vtPzfJBKQQq8WsP/Xy9DNdELWivxcKOCX/Pywge4SiEaPaLtoDT4g== dependencies: regenerator-transform "^0.13.4" "@babel/plugin-transform-reserved-words@^7.2.0": version "7.2.0" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-reserved-words/-/plugin-transform-reserved-words-7.2.0.tgz#4792af87c998a49367597d07fedf02636d2e1634" integrity sha512-fz43fqW8E1tAB3DKF19/vxbpib1fuyCwSPE418ge5ZxILnBhWyhtPgz8eh1RCGGJlwvksHkyxMxh0eenFi+kFw== dependencies: "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-transform-shorthand-properties@^7.2.0": version "7.2.0" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-shorthand-properties/-/plugin-transform-shorthand-properties-7.2.0.tgz#6333aee2f8d6ee7e28615457298934a3b46198f0" integrity sha512-QP4eUM83ha9zmYtpbnyjTLAGKQritA5XW/iG9cjtuOI8s1RuL/3V6a3DeSHfKutJQ+ayUfeZJPcnCYEQzaPQqg== dependencies: "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-transform-spread@^7.2.0": version "7.2.2" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-spread/-/plugin-transform-spread-7.2.2.tgz#3103a9abe22f742b6d406ecd3cd49b774919b406" integrity sha512-KWfky/58vubwtS0hLqEnrWJjsMGaOeSBn90Ezn5Jeg9Z8KKHmELbP1yGylMlm5N6TPKeY9A2+UaSYLdxahg01w== dependencies: "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-transform-sticky-regex@^7.2.0": version "7.2.0" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-sticky-regex/-/plugin-transform-sticky-regex-7.2.0.tgz#a1e454b5995560a9c1e0d537dfc15061fd2687e1" integrity sha512-KKYCoGaRAf+ckH8gEL3JHUaFVyNHKe3ASNsZ+AlktgHevvxGigoIttrEJb8iKN03Q7Eazlv1s6cx2B2cQ3Jabw== dependencies: "@babel/helper-plugin-utils" "^7.0.0" "@babel/helper-regex" "^7.0.0" "@babel/plugin-transform-template-literals@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-template-literals/-/plugin-transform-template-literals-7.4.4.tgz#9d28fea7bbce637fb7612a0750989d8321d4bcb0" integrity sha512-mQrEC4TWkhLN0z8ygIvEL9ZEToPhG5K7KDW3pzGqOfIGZ28Jb0POUkeWcoz8HnHvhFy6dwAT1j8OzqN8s804+g== dependencies: "@babel/helper-annotate-as-pure" "^7.0.0" "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-transform-typeof-symbol@^7.2.0": version "7.2.0" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-typeof-symbol/-/plugin-transform-typeof-symbol-7.2.0.tgz#117d2bcec2fbf64b4b59d1f9819894682d29f2b2" integrity sha512-2LNhETWYxiYysBtrBTqL8+La0jIoQQnIScUJc74OYvUGRmkskNY4EzLCnjHBzdmb38wqtTaixpo1NctEcvMDZw== dependencies: "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-transform-unicode-regex@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/plugin-transform-unicode-regex/-/plugin-transform-unicode-regex-7.4.4.tgz#ab4634bb4f14d36728bf5978322b35587787970f" integrity sha512-il+/XdNw01i93+M9J9u4T7/e/Ue/vWfNZE4IRUQjplu2Mqb/AFTDimkw2tdEdSH50wuQXZAbXSql0UphQke+vA== dependencies: "@babel/helper-plugin-utils" "^7.0.0" "@babel/helper-regex" "^7.4.4" regexpu-core "^4.5.4" "@babel/preset-env@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/preset-env/-/preset-env-7.4.4.tgz#b6f6825bfb27b3e1394ca3de4f926482722c1d6f" integrity sha512-FU1H+ACWqZZqfw1x2G1tgtSSYSfxJLkpaUQL37CenULFARDo+h4xJoVHzRoHbK+85ViLciuI7ME4WTIhFRBBlw== dependencies: "@babel/helper-module-imports" "^7.0.0" "@babel/helper-plugin-utils" "^7.0.0" "@babel/plugin-proposal-async-generator-functions" "^7.2.0" "@babel/plugin-proposal-json-strings" "^7.2.0" "@babel/plugin-proposal-object-rest-spread" "^7.4.4" "@babel/plugin-proposal-optional-catch-binding" "^7.2.0" "@babel/plugin-proposal-unicode-property-regex" "^7.4.4" "@babel/plugin-syntax-async-generators" "^7.2.0" "@babel/plugin-syntax-json-strings" "^7.2.0" "@babel/plugin-syntax-object-rest-spread" "^7.2.0" "@babel/plugin-syntax-optional-catch-binding" "^7.2.0" "@babel/plugin-transform-arrow-functions" "^7.2.0" "@babel/plugin-transform-async-to-generator" "^7.4.4" "@babel/plugin-transform-block-scoped-functions" "^7.2.0" "@babel/plugin-transform-block-scoping" "^7.4.4" "@babel/plugin-transform-classes" "^7.4.4" "@babel/plugin-transform-computed-properties" "^7.2.0" "@babel/plugin-transform-destructuring" "^7.4.4" "@babel/plugin-transform-dotall-regex" "^7.4.4" "@babel/plugin-transform-duplicate-keys" "^7.2.0" "@babel/plugin-transform-exponentiation-operator" "^7.2.0" "@babel/plugin-transform-for-of" "^7.4.4" "@babel/plugin-transform-function-name" "^7.4.4" "@babel/plugin-transform-literals" "^7.2.0" "@babel/plugin-transform-member-expression-literals" "^7.2.0" "@babel/plugin-transform-modules-amd" "^7.2.0" "@babel/plugin-transform-modules-commonjs" "^7.4.4" "@babel/plugin-transform-modules-systemjs" "^7.4.4" "@babel/plugin-transform-modules-umd" "^7.2.0" "@babel/plugin-transform-named-capturing-groups-regex" "^7.4.4" "@babel/plugin-transform-new-target" "^7.4.4" "@babel/plugin-transform-object-super" "^7.2.0" "@babel/plugin-transform-parameters" "^7.4.4" "@babel/plugin-transform-property-literals" "^7.2.0" "@babel/plugin-transform-regenerator" "^7.4.4" "@babel/plugin-transform-reserved-words" "^7.2.0" "@babel/plugin-transform-shorthand-properties" "^7.2.0" "@babel/plugin-transform-spread" "^7.2.0" "@babel/plugin-transform-sticky-regex" "^7.2.0" "@babel/plugin-transform-template-literals" "^7.4.4" "@babel/plugin-transform-typeof-symbol" "^7.2.0" "@babel/plugin-transform-unicode-regex" "^7.4.4" "@babel/types" "^7.4.4" browserslist "^4.5.2" core-js-compat "^3.0.0" invariant "^2.2.2" js-levenshtein "^1.1.3" semver "^5.5.0" "@babel/runtime@^7.0.0", "@babel/runtime@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/runtime/-/runtime-7.4.4.tgz#dc2e34982eb236803aa27a07fea6857af1b9171d" integrity sha512-w0+uT71b6Yi7i5SE0co4NioIpSYS6lLiXvCzWzGSKvpK5vdQtCbICHMj+gbAKAOtxiV6HsVh/MBdaF9EQ6faSg== dependencies: regenerator-runtime "^0.13.2" "@babel/template@^7.1.0": version "7.2.2" resolved "https://registry.yarnpkg.com/@babel/template/-/template-7.2.2.tgz#005b3fdf0ed96e88041330379e0da9a708eb2907" integrity sha512-zRL0IMM02AUDwghf5LMSSDEz7sBCO2YnNmpg3uWTZj/v1rcG2BmQUvaGU8GhU8BvfMh1k2KIAYZ7Ji9KXPUg7g== dependencies: "@babel/code-frame" "^7.0.0" "@babel/parser" "^7.2.2" "@babel/types" "^7.2.2" "@babel/template@^7.4.0", "@babel/template@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/template/-/template-7.4.4.tgz#f4b88d1225689a08f5bc3a17483545be9e4ed237" integrity sha512-CiGzLN9KgAvgZsnivND7rkA+AeJ9JB0ciPOD4U59GKbQP2iQl+olF1l76kJOupqidozfZ32ghwBEJDhnk9MEcw== dependencies: "@babel/code-frame" "^7.0.0" "@babel/parser" "^7.4.4" "@babel/types" "^7.4.4" "@babel/traverse@^7.0.0": version "7.2.3" resolved "https://registry.yarnpkg.com/@babel/traverse/-/traverse-7.2.3.tgz#7ff50cefa9c7c0bd2d81231fdac122f3957748d8" integrity sha512-Z31oUD/fJvEWVR0lNZtfgvVt512ForCTNKYcJBGbPb1QZfve4WGH8Wsy7+Mev33/45fhP/hwQtvgusNdcCMgSw== dependencies: "@babel/code-frame" "^7.0.0" "@babel/generator" "^7.2.2" "@babel/helper-function-name" "^7.1.0" "@babel/helper-split-export-declaration" "^7.0.0" "@babel/parser" "^7.2.3" "@babel/types" "^7.2.2" debug "^4.1.0" globals "^11.1.0" lodash "^4.17.10" "@babel/traverse@^7.1.0", "@babel/traverse@^7.4.3", "@babel/traverse@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/traverse/-/traverse-7.4.4.tgz#0776f038f6d78361860b6823887d4f3937133fe8" integrity sha512-Gw6qqkw/e6AGzlyj9KnkabJX7VcubqPtkUQVAwkc0wUMldr3A/hezNB3Rc5eIvId95iSGkGIOe5hh1kMKf951A== dependencies: "@babel/code-frame" "^7.0.0" "@babel/generator" "^7.4.4" "@babel/helper-function-name" "^7.1.0" "@babel/helper-split-export-declaration" "^7.4.4" "@babel/parser" "^7.4.4" "@babel/types" "^7.4.4" debug "^4.1.0" globals "^11.1.0" lodash "^4.17.11" "@babel/types@^7.0.0", "@babel/types@^7.2.2": version "7.2.2" resolved "https://registry.yarnpkg.com/@babel/types/-/types-7.2.2.tgz#44e10fc24e33af524488b716cdaee5360ea8ed1e" integrity sha512-fKCuD6UFUMkR541eDWL+2ih/xFZBXPOg/7EQFeTluMDebfqR4jrpaCjLhkWlQS4hT6nRa2PMEgXKbRB5/H2fpg== dependencies: esutils "^2.0.2" lodash "^4.17.10" to-fast-properties "^2.0.0" "@babel/types@^7.2.0", "@babel/types@^7.4.0", "@babel/types@^7.4.4": version "7.4.4" resolved "https://registry.yarnpkg.com/@babel/types/-/types-7.4.4.tgz#8db9e9a629bb7c29370009b4b779ed93fe57d5f0" integrity sha512-dOllgYdnEFOebhkKCjzSVFqw/PmmB8pH6RGOWkY4GsboQNd47b1fBThBSwlHAq9alF9vc1M3+6oqR47R50L0tQ== dependencies: esutils "^2.0.2" lodash "^4.17.11" to-fast-properties "^2.0.0" "@samverschueren/stream-to-observable@^0.3.0": version "0.3.0" resolved "https://registry.yarnpkg.com/@samverschueren/stream-to-observable/-/stream-to-observable-0.3.0.tgz#ecdf48d532c58ea477acfcab80348424f8d0662f" integrity sha512-MI4Xx6LHs4Webyvi6EbspgyAb4D2Q2VtnCQ1blOJcoLS6mVa8lNN2rkIy1CVxfTUpoyIbCTkXES1rLXztFD1lg== dependencies: any-observable "^0.3.0" "@types/normalize-package-data@^2.4.0": version "2.4.0" resolved "https://registry.yarnpkg.com/@types/normalize-package-data/-/normalize-package-data-2.4.0.tgz#e486d0d97396d79beedd0a6e33f4534ff6b4973e" integrity sha512-f5j5b/Gf71L+dbqxIpQ4Z2WlmI/mPJ0fOkGGmFgtb6sAu97EPczzbS3/tJKxmcYDj55OX6ssqwDAWOHIYDRDGA== "@types/prop-types@*": version "15.7.1" resolved "https://registry.yarnpkg.com/@types/prop-types/-/prop-types-15.7.1.tgz#f1a11e7babb0c3cad68100be381d1e064c68f1f6" integrity sha512-CFzn9idOEpHrgdw8JsoTkaDDyRWk1jrzIV8djzcgpq0y9tG4B4lFT+Nxh52DVpDXV+n4+NPNv7M1Dj5uMp6XFg== "@types/react@^16.8.12", "@types/react@^16.8.6": version "16.8.16" resolved "https://registry.yarnpkg.com/@types/react/-/react-16.8.16.tgz#2bf980b4fb29cceeb01b2c139b3e185e57d3e08e" integrity sha512-A0+6kS6zwPtvubOLiCJmZ8li5bm3wKIkoKV0h3RdMDOnCj9cYkUnj3bWbE03/lcICdQmwBmUfoFiHeNhbFiyHQ== dependencies: "@types/prop-types" "*" csstype "^2.2.0" abbrev@1: version "1.1.1" resolved "https://registry.yarnpkg.com/abbrev/-/abbrev-1.1.1.tgz#f8f2c887ad10bf67f634f005b6987fed3179aac8" integrity sha512-nne9/IiQ/hzIhY6pdDnbBtz7DjPTKrY00P/zvPSm5pOFkl6xuGrGnXn/VtTNNfNtAfZ9/1RtehkszU9qcTii0Q== acorn-jsx@^5.0.0: version "5.0.1" resolved "https://registry.yarnpkg.com/acorn-jsx/-/acorn-jsx-5.0.1.tgz#32a064fd925429216a09b141102bfdd185fae40e" integrity sha512-HJ7CfNHrfJLlNTzIEUTj43LNWGkqpRLxm3YjAlcD0ACydk9XynzYsCBHxut+iqt+1aBXkx9UP/w/ZqMr13XIzg== acorn@^6.0.7: version "6.1.0" resolved "https://registry.yarnpkg.com/acorn/-/acorn-6.1.0.tgz#b0a3be31752c97a0f7013c5f4903b71a05db6818" integrity sha512-MW/FjM+IvU9CgBzjO3UIPCE2pyEwUsoFl+VGdczOPEdxfGFjuKny/gN54mOuX7Qxmb9Rg9MCn2oKiSUeW+pjrw== ajv@^6.5.5: version "6.10.0" resolved "https://registry.yarnpkg.com/ajv/-/ajv-6.10.0.tgz#90d0d54439da587cd7e843bfb7045f50bd22bdf1" integrity sha512-nffhOpkymDECQyR0mnsUtoCE8RlX38G0rYP+wgLWFyZuUyuuojSSvi/+euOiQBIn63whYwYVIIH1TvE3tu4OEg== dependencies: fast-deep-equal "^2.0.1" fast-json-stable-stringify "^2.0.0" json-schema-traverse "^0.4.1" uri-js "^4.2.2" ajv@^6.9.1: version "6.9.1" resolved "https://registry.yarnpkg.com/ajv/-/ajv-6.9.1.tgz#a4d3683d74abc5670e75f0b16520f70a20ea8dc1" integrity sha512-XDN92U311aINL77ieWHmqCcNlwjoP5cHXDxIxbf2MaPYuCXOHS7gHH8jktxeK5omgd52XbSTX6a4Piwd1pQmzA== dependencies: fast-deep-equal "^2.0.1" fast-json-stable-stringify "^2.0.0" json-schema-traverse "^0.4.1" uri-js "^4.2.2" ansi-escapes@^3.0.0: version "3.1.0" resolved "https://registry.yarnpkg.com/ansi-escapes/-/ansi-escapes-3.1.0.tgz#f73207bb81207d75fd6c83f125af26eea378ca30" integrity sha512-UgAb8H9D41AQnu/PbWlCofQVcnV4Gs2bBJi9eZPxfU/hgglFh3SMDMENRIqdr7H6XFnXdoknctFByVsCOotTVw== ansi-escapes@^3.2.0: version "3.2.0" resolved "https://registry.yarnpkg.com/ansi-escapes/-/ansi-escapes-3.2.0.tgz#8780b98ff9dbf5638152d1f1fe5c1d7b4442976b" integrity sha512-cBhpre4ma+U0T1oM5fXg7Dy1Jw7zzwv7lt/GoCpr+hDQJoYnKVPLL4dCvSEFMmQurOQvSrwT7SL/DAlhBI97RQ== ansi-regex@^2.0.0: version "2.1.1" resolved "https://registry.yarnpkg.com/ansi-regex/-/ansi-regex-2.1.1.tgz#c3b33ab5ee360d86e0e628f0468ae7ef27d654df" integrity sha1-w7M6te42DYbg5ijwRorn7yfWVN8= ansi-regex@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/ansi-regex/-/ansi-regex-3.0.0.tgz#ed0317c322064f79466c02966bddb605ab37d998" integrity sha1-7QMXwyIGT3lGbAKWa922Bas32Zg= ansi-regex@^4.0.0: version "4.0.0" resolved "https://registry.yarnpkg.com/ansi-regex/-/ansi-regex-4.0.0.tgz#70de791edf021404c3fd615aa89118ae0432e5a9" integrity sha512-iB5Dda8t/UqpPI/IjsejXu5jOGDrzn41wJyljwPH65VCIbk6+1BzFIMJGFwTNrYXT1CrD+B4l19U7awiQ8rk7w== ansi-styles@^2.2.1: version "2.2.1" resolved "https://registry.yarnpkg.com/ansi-styles/-/ansi-styles-2.2.1.tgz#b432dd3358b634cf75e1e4664368240533c1ddbe" integrity sha1-tDLdM1i2NM914eRmQ2gkBTPB3b4= ansi-styles@^3.2.0, ansi-styles@^3.2.1: version "3.2.1" resolved "https://registry.yarnpkg.com/ansi-styles/-/ansi-styles-3.2.1.tgz#41fbb20243e50b12be0f04b8dedbf07520ce841d" integrity sha512-VT0ZI6kZRdTh8YyJw3SMbYm/u+NqfsAxEpWO0Pf9sq8/e94WxxOpPKx9FR1FlyCtOVDNOQ+8ntlqFxiRc+r5qA== dependencies: color-convert "^1.9.0" ansicolors@~0.3.2: version "0.3.2" resolved "https://registry.yarnpkg.com/ansicolors/-/ansicolors-0.3.2.tgz#665597de86a9ffe3aa9bfbe6cae5c6ea426b4979" integrity sha1-ZlWX3oap/+Oqm/vmyuXG6kJrSXk= any-observable@^0.3.0: version "0.3.0" resolved "https://registry.yarnpkg.com/any-observable/-/any-observable-0.3.0.tgz#af933475e5806a67d0d7df090dd5e8bef65d119b" integrity sha512-/FQM1EDkTsf63Ub2C6O7GuYFDsSXUwsaZDurV0np41ocwq0jthUAYCmhBX9f+KwlaCgIuWyr/4WlUQUBfKfZog== anymatch@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/anymatch/-/anymatch-2.0.0.tgz#bcb24b4f37934d9aa7ac17b4adaf89e7c76ef2eb" integrity sha512-5teOsQWABXHHBFP9y3skS5P3d/WfWXpv3FUpy+LorMrNYaT9pI4oLMQX7jzQ2KklNpGpWHzdCXTDT2Y3XGlZBw== dependencies: micromatch "^3.1.4" normalize-path "^2.1.1" app-root-path@^2.2.1: version "2.2.1" resolved "https://registry.yarnpkg.com/app-root-path/-/app-root-path-2.2.1.tgz#d0df4a682ee408273583d43f6f79e9892624bc9a" integrity sha512-91IFKeKk7FjfmezPKkwtaRvSpnUc4gDwPAjA1YZ9Gn0q0PPeW+vbeUsZuyDwjI7+QTHhcLen2v25fi/AmhvbJA== append-transform@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/append-transform/-/append-transform-1.0.0.tgz#046a52ae582a228bd72f58acfbe2967c678759ab" integrity sha512-P009oYkeHyU742iSZJzZZywj4QRJdnTWffaKuJQLablCZ1uz6/cW4yaRgcDaoQ+uwOxxnt0gRUcwfsNP2ri0gw== dependencies: default-require-extensions "^2.0.0" aproba@^1.0.3: version "1.2.0" resolved "https://registry.yarnpkg.com/aproba/-/aproba-1.2.0.tgz#6802e6264efd18c790a1b0d517f0f2627bf2c94a" integrity sha512-Y9J6ZjXtoYh8RnXVCMOU/ttDmk1aBjunq9vO0ta5x85WDQiQfUF9sIPBITdbiiIVcBo03Hi3jMxigBtsddlXRw== archy@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/archy/-/archy-1.0.0.tgz#f9c8c13757cc1dd7bc379ac77b2c62a5c2868c40" integrity sha1-+cjBN1fMHde8N5rHeyxipcKGjEA= are-we-there-yet@~1.1.2: version "1.1.5" resolved "https://registry.yarnpkg.com/are-we-there-yet/-/are-we-there-yet-1.1.5.tgz#4b35c2944f062a8bfcda66410760350fe9ddfc21" integrity sha512-5hYdAkZlcG8tOLujVDTgCT+uPX0VnpAH28gWsLfzpXYm7wP6mp5Q/gYyR7YQ0cKVJcXJnl3j2kpBan13PtQf6w== dependencies: delegates "^1.0.0" readable-stream "^2.0.6" arg@^4.1.0: version "4.1.0" resolved "https://registry.yarnpkg.com/arg/-/arg-4.1.0.tgz#583c518199419e0037abb74062c37f8519e575f0" integrity sha512-ZWc51jO3qegGkVh8Hwpv636EkbesNV5ZNQPCtRa+0qytRYPEs9IYT9qITY9buezqUH5uqyzlWLcufrzU2rffdg== argparse@^1.0.7: version "1.0.10" resolved "https://registry.yarnpkg.com/argparse/-/argparse-1.0.10.tgz#bcd6791ea5ae09725e17e5ad988134cd40b3d911" integrity sha512-o5Roy6tNG4SL/FOkCAN6RzjiakZS25RLYFrcMttJqbdd8BWrnA+fGz57iN5Pb06pvBGvl5gQ0B48dJlslXvoTg== dependencies: sprintf-js "~1.0.2" arr-diff@^4.0.0: version "4.0.0" resolved "https://registry.yarnpkg.com/arr-diff/-/arr-diff-4.0.0.tgz#d6461074febfec71e7e15235761a329a5dc7c520" integrity sha1-1kYQdP6/7HHn4VI1dhoyml3HxSA= arr-flatten@^1.1.0: version "1.1.0" resolved "https://registry.yarnpkg.com/arr-flatten/-/arr-flatten-1.1.0.tgz#36048bbff4e7b47e136644316c99669ea5ae91f1" integrity sha512-L3hKV5R/p5o81R7O02IGnwpDmkp6E982XhtbuwSe3O4qOtMMMtodicASA1Cny2U+aCXcNpml+m4dPsvsJ3jatg== arr-union@^3.1.0: version "3.1.0" resolved "https://registry.yarnpkg.com/arr-union/-/arr-union-3.1.0.tgz#e39b09aea9def866a8f206e288af63919bae39c4" integrity sha1-45sJrqne+Gao8gbiiK9jkZuuOcQ= array-includes@^3.0.3: version "3.0.3" resolved "https://registry.yarnpkg.com/array-includes/-/array-includes-3.0.3.tgz#184b48f62d92d7452bb31b323165c7f8bd02266d" integrity sha1-GEtI9i2S10UrsxsyMWXH+L0CJm0= dependencies: define-properties "^1.1.2" es-abstract "^1.7.0" array-union@^1.0.1: version "1.0.2" resolved "https://registry.yarnpkg.com/array-union/-/array-union-1.0.2.tgz#9a34410e4f4e3da23dea375be5be70f24778ec39" integrity sha1-mjRBDk9OPaI96jdb5b5w8kd47Dk= dependencies: array-uniq "^1.0.1" array-uniq@^1.0.1: version "1.0.3" resolved "https://registry.yarnpkg.com/array-uniq/-/array-uniq-1.0.3.tgz#af6ac877a25cc7f74e058894753858dfdb24fdb6" integrity sha1-r2rId6Jcx/dOBYiUdThY39sk/bY= array-unique@^0.3.2: version "0.3.2" resolved "https://registry.yarnpkg.com/array-unique/-/array-unique-0.3.2.tgz#a894b75d4bc4f6cd679ef3244a9fd8f46ae2d428" integrity sha1-qJS3XUvE9s1nnvMkSp/Y9Gri1Cg= arrify@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/arrify/-/arrify-1.0.1.tgz#898508da2226f380df904728456849c1501a4b0d" integrity sha1-iYUI2iIm84DfkEcoRWhJwVAaSw0= asn1@~0.2.3: version "0.2.4" resolved "https://registry.yarnpkg.com/asn1/-/asn1-0.2.4.tgz#8d2475dfab553bb33e77b54e59e880bb8ce23136" integrity sha512-jxwzQpLQjSmWXgwaCZE9Nz+glAG01yF1QnWgbhGwHI5A6FRIEY6IVqtHhIepHqI7/kyEyQEagBC5mBEFlIYvdg== dependencies: safer-buffer "~2.1.0" assert-plus@1.0.0, assert-plus@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/assert-plus/-/assert-plus-1.0.0.tgz#f12e0f3c5d77b0b1cdd9146942e4e96c1e4dd525" integrity sha1-8S4PPF13sLHN2RRpQuTpbB5N1SU= assign-symbols@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/assign-symbols/-/assign-symbols-1.0.0.tgz#59667f41fadd4f20ccbc2bb96b8d4f7f78ec0367" integrity sha1-WWZ/QfrdTyDMvCu5a41Pf3jsA2c= astral-regex@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/astral-regex/-/astral-regex-1.0.0.tgz#6c8c3fb827dd43ee3918f27b82782ab7658a6fd9" integrity sha512-+Ryf6g3BKoRc7jfp7ad8tM4TtMiaWvbF/1/sQcZPkkS7ag3D5nMBCe2UfOTONtAkaG0tO0ij3C5Lwmf1EiyjHg== async-each@^1.0.1: version "1.0.3" resolved "https://registry.yarnpkg.com/async-each/-/async-each-1.0.3.tgz#b727dbf87d7651602f06f4d4ac387f47d91b0cbf" integrity sha512-z/WhQ5FPySLdvREByI2vZiTWwCnF0moMJ1hK9YQwDTHKh6I7/uSckMetoRGb5UBZPC1z0jlw+n/XCgjeH7y1AQ== async-hook-domain@^1.1.0: version "1.1.0" resolved "https://registry.yarnpkg.com/async-hook-domain/-/async-hook-domain-1.1.0.tgz#76855e572425c2bd1f7171f989bd40d70f12f9d6" integrity sha512-NH7V97d1yCbIanu2oDLyPT2GFNct0esPeJyRfkk8J5hTztHVSQp4UiNfL2O42sCA9XZPU8OgHvzOmt9ewBhVqA== dependencies: source-map-support "^0.5.11" asynckit@^0.4.0: version "0.4.0" resolved "https://registry.yarnpkg.com/asynckit/-/asynckit-0.4.0.tgz#c79ed97f7f34cb8f2ba1bc9790bcc366474b4b79" integrity sha1-x57Zf380y48robyXkLzDZkdLS3k= atob@^2.1.1: version "2.1.2" resolved "https://registry.yarnpkg.com/atob/-/atob-2.1.2.tgz#6d9517eb9e030d2436666651e86bd9f6f13533c9" integrity sha512-Wm6ukoaOGJi/73p/cl2GvLjTI5JM1k/O14isD73YML8StrH/7/lRFgmg8nICZgD3bZZvjwCGxtMOD3wWNAu8cg== auto-bind@^2.0.0: version "2.1.0" resolved "https://registry.yarnpkg.com/auto-bind/-/auto-bind-2.1.0.tgz#254e12d53063d7cab90446ce021accfb3faa1464" integrity sha512-qZuFvkes1eh9lB2mg8/HG18C+5GIO51r+RrCSst/lh+i5B1CtVlkhTE488M805Nr3dKl0sM/pIFKSKUIlg3zUg== dependencies: "@types/react" "^16.8.12" aws-sign2@~0.7.0: version "0.7.0" resolved "https://registry.yarnpkg.com/aws-sign2/-/aws-sign2-0.7.0.tgz#b46e890934a9591f2d2f6f86d7e6a9f1b3fe76a8" integrity sha1-tG6JCTSpWR8tL2+G1+ap8bP+dqg= aws4@^1.8.0: version "1.8.0" resolved "https://registry.yarnpkg.com/aws4/-/aws4-1.8.0.tgz#f0e003d9ca9e7f59c7a508945d7b2ef9a04a542f" integrity sha512-ReZxvNHIOv88FlT7rxcXIIC0fPt4KZqZbOlivyWtXLt8ESx84zd3kMC6iK5jVeS2qt+g7ftS7ye4fi06X5rtRQ== babel-code-frame@^6.26.0: version "6.26.0" resolved "https://registry.yarnpkg.com/babel-code-frame/-/babel-code-frame-6.26.0.tgz#63fd43f7dc1e3bb7ce35947db8fe369a3f58c74b" integrity sha1-Y/1D99weO7fONZR9uP42mj9Yx0s= dependencies: chalk "^1.1.3" esutils "^2.0.2" js-tokens "^3.0.2" babel-core@^6.25.0, babel-core@^6.26.0: version "6.26.3" resolved "https://registry.yarnpkg.com/babel-core/-/babel-core-6.26.3.tgz#b2e2f09e342d0f0c88e2f02e067794125e75c207" integrity sha512-6jyFLuDmeidKmUEb3NM+/yawG0M2bDZ9Z1qbZP59cyHLz8kYGKYwpJP0UwUKKUiTRNvxfLesJnTedqczP7cTDA== dependencies: babel-code-frame "^6.26.0" babel-generator "^6.26.0" babel-helpers "^6.24.1" babel-messages "^6.23.0" babel-register "^6.26.0" babel-runtime "^6.26.0" babel-template "^6.26.0" babel-traverse "^6.26.0" babel-types "^6.26.0" babylon "^6.18.0" convert-source-map "^1.5.1" debug "^2.6.9" json5 "^0.5.1" lodash "^4.17.4" minimatch "^3.0.4" path-is-absolute "^1.0.1" private "^0.1.8" slash "^1.0.0" source-map "^0.5.7" babel-eslint@^10.0.1: version "10.0.1" resolved "https://registry.yarnpkg.com/babel-eslint/-/babel-eslint-10.0.1.tgz#919681dc099614cd7d31d45c8908695092a1faed" integrity sha512-z7OT1iNV+TjOwHNLLyJk+HN+YVWX+CLE6fPD2SymJZOZQBs+QIexFjhm4keGTm8MW9xr4EC9Q0PbaLB24V5GoQ== dependencies: "@babel/code-frame" "^7.0.0" "@babel/parser" "^7.0.0" "@babel/traverse" "^7.0.0" "@babel/types" "^7.0.0" eslint-scope "3.7.1" eslint-visitor-keys "^1.0.0" babel-generator@^6.26.0: version "6.26.1" resolved "https://registry.yarnpkg.com/babel-generator/-/babel-generator-6.26.1.tgz#1844408d3b8f0d35a404ea7ac180f087a601bd90" integrity sha512-HyfwY6ApZj7BYTcJURpM5tznulaBvyio7/0d4zFOeMPUmfxkCjHocCuoLa2SAGzBI8AREcH3eP3758F672DppA== dependencies: babel-messages "^6.23.0" babel-runtime "^6.26.0" babel-types "^6.26.0" detect-indent "^4.0.0" jsesc "^1.3.0" lodash "^4.17.4" source-map "^0.5.7" trim-right "^1.0.1" babel-helper-builder-react-jsx@^6.24.1: version "6.26.0" resolved "https://registry.yarnpkg.com/babel-helper-builder-react-jsx/-/babel-helper-builder-react-jsx-6.26.0.tgz#39ff8313b75c8b65dceff1f31d383e0ff2a408a0" integrity sha1-Of+DE7dci2Xc7/HzHTg+D/KkCKA= dependencies: babel-runtime "^6.26.0" babel-types "^6.26.0" esutils "^2.0.2" babel-helpers@^6.24.1: version "6.24.1" resolved "https://registry.yarnpkg.com/babel-helpers/-/babel-helpers-6.24.1.tgz#3471de9caec388e5c850e597e58a26ddf37602b2" integrity sha1-NHHenK7DiOXIUOWX5Yom3fN2ArI= dependencies: babel-runtime "^6.22.0" babel-template "^6.24.1" babel-messages@^6.23.0: version "6.23.0" resolved "https://registry.yarnpkg.com/babel-messages/-/babel-messages-6.23.0.tgz#f3cdf4703858035b2a2951c6ec5edf6c62f2630e" integrity sha1-8830cDhYA1sqKVHG7F7fbGLyYw4= dependencies: babel-runtime "^6.22.0" babel-plugin-syntax-jsx@^6.8.0: version "6.18.0" resolved "https://registry.yarnpkg.com/babel-plugin-syntax-jsx/-/babel-plugin-syntax-jsx-6.18.0.tgz#0af32a9a6e13ca7a3fd5069e62d7b0f58d0d8946" integrity sha1-CvMqmm4Tyno/1QaeYtew9Y0NiUY= babel-plugin-syntax-object-rest-spread@^6.8.0: version "6.13.0" resolved "https://registry.yarnpkg.com/babel-plugin-syntax-object-rest-spread/-/babel-plugin-syntax-object-rest-spread-6.13.0.tgz#fd6536f2bce13836ffa3a5458c4903a597bb3bf5" integrity sha1-/WU28rzhODb/o6VFjEkDpZe7O/U= babel-plugin-transform-es2015-destructuring@^6.23.0: version "6.23.0" resolved "https://registry.yarnpkg.com/babel-plugin-transform-es2015-destructuring/-/babel-plugin-transform-es2015-destructuring-6.23.0.tgz#997bb1f1ab967f682d2b0876fe358d60e765c56d" integrity sha1-mXux8auWf2gtKwh2/jWNYOdlxW0= dependencies: babel-runtime "^6.22.0" babel-plugin-transform-object-rest-spread@^6.23.0: version "6.26.0" resolved "https://registry.yarnpkg.com/babel-plugin-transform-object-rest-spread/-/babel-plugin-transform-object-rest-spread-6.26.0.tgz#0f36692d50fef6b7e2d4b3ac1478137a963b7b06" integrity sha1-DzZpLVD+9rfi1LOsFHgTepY7ewY= dependencies: babel-plugin-syntax-object-rest-spread "^6.8.0" babel-runtime "^6.26.0" babel-plugin-transform-react-jsx@^6.24.1: version "6.24.1" resolved "https://registry.yarnpkg.com/babel-plugin-transform-react-jsx/-/babel-plugin-transform-react-jsx-6.24.1.tgz#840a028e7df460dfc3a2d29f0c0d91f6376e66a3" integrity sha1-hAoCjn30YN/DotKfDA2R9jduZqM= dependencies: babel-helper-builder-react-jsx "^6.24.1" babel-plugin-syntax-jsx "^6.8.0" babel-runtime "^6.22.0" babel-register@^6.26.0: version "6.26.0" resolved "https://registry.yarnpkg.com/babel-register/-/babel-register-6.26.0.tgz#6ed021173e2fcb486d7acb45c6009a856f647071" integrity sha1-btAhFz4vy0htestFxgCahW9kcHE= dependencies: babel-core "^6.26.0" babel-runtime "^6.26.0" core-js "^2.5.0" home-or-tmp "^2.0.0" lodash "^4.17.4" mkdirp "^0.5.1" source-map-support "^0.4.15" babel-runtime@^6.22.0, babel-runtime@^6.26.0: version "6.26.0" resolved "https://registry.yarnpkg.com/babel-runtime/-/babel-runtime-6.26.0.tgz#965c7058668e82b55d7bfe04ff2337bc8b5647fe" integrity sha1-llxwWGaOgrVde/4E/yM3vItWR/4= dependencies: core-js "^2.4.0" regenerator-runtime "^0.11.0" babel-template@^6.24.1, babel-template@^6.26.0: version "6.26.0" resolved "https://registry.yarnpkg.com/babel-template/-/babel-template-6.26.0.tgz#de03e2d16396b069f46dd9fff8521fb1a0e35e02" integrity sha1-3gPi0WOWsGn0bdn/+FIfsaDjXgI= dependencies: babel-runtime "^6.26.0" babel-traverse "^6.26.0" babel-types "^6.26.0" babylon "^6.18.0" lodash "^4.17.4" babel-traverse@^6.26.0: version "6.26.0" resolved "https://registry.yarnpkg.com/babel-traverse/-/babel-traverse-6.26.0.tgz#46a9cbd7edcc62c8e5c064e2d2d8d0f4035766ee" integrity sha1-RqnL1+3MYsjlwGTi0tjQ9ANXZu4= dependencies: babel-code-frame "^6.26.0" babel-messages "^6.23.0" babel-runtime "^6.26.0" babel-types "^6.26.0" babylon "^6.18.0" debug "^2.6.8" globals "^9.18.0" invariant "^2.2.2" lodash "^4.17.4" babel-types@^6.26.0: version "6.26.0" resolved "https://registry.yarnpkg.com/babel-types/-/babel-types-6.26.0.tgz#a3b073f94ab49eb6fa55cd65227a334380632497" integrity sha1-o7Bz+Uq0nrb6Vc1lInozQ4BjJJc= dependencies: babel-runtime "^6.26.0" esutils "^2.0.2" lodash "^4.17.4" to-fast-properties "^1.0.3" babylon@^6.18.0: version "6.18.0" resolved "https://registry.yarnpkg.com/babylon/-/babylon-6.18.0.tgz#af2f3b88fa6f5c1e4c634d1a0f8eac4f55b395e3" integrity sha512-q/UEjfGJ2Cm3oKV71DJz9d25TPnq5rhBVL2Q4fA5wcC3jcrdn7+SssEybFIxwAvvP+YCsCYNKughoF33GxgycQ== balanced-match@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/balanced-match/-/balanced-match-1.0.0.tgz#89b4d199ab2bee49de164ea02b89ce462d71b767" integrity sha1-ibTRmasr7kneFk6gK4nORi1xt2c= base@^0.11.1: version "0.11.2" resolved "https://registry.yarnpkg.com/base/-/base-0.11.2.tgz#7bde5ced145b6d551a90db87f83c558b4eb48a8f" integrity sha512-5T6P4xPgpp0YDFvSWwEZ4NoE3aM4QBQXDzmVbraCkFj8zHM+mba8SyqB5DbZWyR7mYHo6Y7BdQo3MoA4m0TeQg== dependencies: cache-base "^1.0.1" class-utils "^0.3.5" component-emitter "^1.2.1" define-property "^1.0.0" isobject "^3.0.1" mixin-deep "^1.2.0" pascalcase "^0.1.1" bcrypt-pbkdf@^1.0.0: version "1.0.2" resolved "https://registry.yarnpkg.com/bcrypt-pbkdf/-/bcrypt-pbkdf-1.0.2.tgz#a4301d389b6a43f9b67ff3ca11a3f6637e360e9e" integrity sha1-pDAdOJtqQ/m2f/PKEaP2Y342Dp4= dependencies: tweetnacl "^0.14.3" binary-extensions@^1.0.0: version "1.12.0" resolved "https://registry.yarnpkg.com/binary-extensions/-/binary-extensions-1.12.0.tgz#c2d780f53d45bba8317a8902d4ceeaf3a6385b14" integrity sha512-DYWGk01lDcxeS/K9IHPGWfT8PsJmbXRtRd2Sx72Tnb8pcYZQFF1oSDb8hJtS1vhp212q1Rzi5dUf9+nq0o9UIg== bind-obj-methods@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/bind-obj-methods/-/bind-obj-methods-2.0.0.tgz#0178140dbe7b7bb67dc74892ace59bc0247f06f0" integrity sha512-3/qRXczDi2Cdbz6jE+W3IflJOutRVica8frpBn14de1mBOkzDo+6tY33kNhvkw54Kn3PzRRD2VnGbGPcTAk4sw== brace-expansion@^1.1.7: version "1.1.11" resolved "https://registry.yarnpkg.com/brace-expansion/-/brace-expansion-1.1.11.tgz#3c7fcbf529d87226f3d2f52b966ff5271eb441dd" integrity sha512-iCuPHDFgrHX7H2vEI/5xpz07zSHB00TpugqhmYtVmMO6518mCuRMoOYFldEBl0g187ufozdaHgWKcYFb61qGiA== dependencies: balanced-match "^1.0.0" concat-map "0.0.1" braces@^2.3.1, braces@^2.3.2: version "2.3.2" resolved "https://registry.yarnpkg.com/braces/-/braces-2.3.2.tgz#5979fd3f14cd531565e5fa2df1abfff1dfaee729" integrity sha512-aNdbnj9P8PjdXU4ybaWLK2IF3jc/EoDYbC7AazW6to3TRsfXxscC9UXOB5iDiEQrkyIbWp2SLQda4+QAa7nc3w== dependencies: arr-flatten "^1.1.0" array-unique "^0.3.2" extend-shallow "^2.0.1" fill-range "^4.0.0" isobject "^3.0.1" repeat-element "^1.1.2" snapdragon "^0.8.1" snapdragon-node "^2.0.1" split-string "^3.0.2" to-regex "^3.0.1" browser-process-hrtime@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/browser-process-hrtime/-/browser-process-hrtime-1.0.0.tgz#3c9b4b7d782c8121e56f10106d84c0d0ffc94626" integrity sha512-9o5UecI3GhkpM6DrXr69PblIuWxPKk9Y0jHBRhdocZ2y7YECBFCsHm79Pr3OyR2AvjhDkabFJaDJMYRazHgsow== browserslist@^4.5.2, browserslist@^4.5.4: version "4.5.6" resolved "https://registry.yarnpkg.com/browserslist/-/browserslist-4.5.6.tgz#ea42e8581ca2513fa7f371d4dd66da763938163d" integrity sha512-o/hPOtbU9oX507lIqon+UvPYqpx3mHc8cV3QemSBTXwkG8gSQSK6UKvXcE/DcleU3+A59XTUHyCvZ5qGy8xVAg== dependencies: caniuse-lite "^1.0.30000963" electron-to-chromium "^1.3.127" node-releases "^1.1.17" buffer-from@^1.0.0: version "1.1.1" resolved "https://registry.yarnpkg.com/buffer-from/-/buffer-from-1.1.1.tgz#32713bc028f75c02fdb710d7c7bcec1f2c6070ef" integrity sha512-MQcXEUbCKtEo7bhqEs6560Hyd4XaovZlO/k9V3hjVUF/zwW7KBVdSK4gIt/bzwS9MbR5qob+F5jusZsb0YQK2A== builtin-modules@^1.0.0: version "1.1.1" resolved "https://registry.yarnpkg.com/builtin-modules/-/builtin-modules-1.1.1.tgz#270f076c5a72c02f5b65a47df94c5fe3a278892f" integrity sha1-Jw8HbFpywC9bZaR9+Uxf46J4iS8= cache-base@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/cache-base/-/cache-base-1.0.1.tgz#0a7f46416831c8b662ee36fe4e7c59d76f666ab2" integrity sha512-AKcdTnFSWATd5/GCPRxr2ChwIJ85CeyrEyjRHlKxQ56d4XJMGym0uAiKn0xbLOGOl3+yRpOTi484dVCEc5AUzQ== dependencies: collection-visit "^1.0.0" component-emitter "^1.2.1" get-value "^2.0.6" has-value "^1.0.0" isobject "^3.0.1" set-value "^2.0.0" to-object-path "^0.3.0" union-value "^1.0.0" unset-value "^1.0.0" caching-transform@^3.0.2: version "3.0.2" resolved "https://registry.yarnpkg.com/caching-transform/-/caching-transform-3.0.2.tgz#601d46b91eca87687a281e71cef99791b0efca70" integrity sha512-Mtgcv3lh3U0zRii/6qVgQODdPA4G3zhG+jtbCWj39RXuUFTMzH0vcdMtaJS1jPowd+It2Pqr6y3NJMQqOqCE2w== dependencies: hasha "^3.0.0" make-dir "^2.0.0" package-hash "^3.0.0" write-file-atomic "^2.4.2" caller-callsite@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/caller-callsite/-/caller-callsite-2.0.0.tgz#847e0fce0a223750a9a027c54b33731ad3154134" integrity sha1-hH4PzgoiN1CpoCfFSzNzGtMVQTQ= dependencies: callsites "^2.0.0" caller-path@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/caller-path/-/caller-path-2.0.0.tgz#468f83044e369ab2010fac5f06ceee15bb2cb1f4" integrity sha1-Ro+DBE42mrIBD6xfBs7uFbsssfQ= dependencies: caller-callsite "^2.0.0" callsites@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/callsites/-/callsites-2.0.0.tgz#06eb84f00eea413da86affefacbffb36093b3c50" integrity sha1-BuuE8A7qQT2oav/vrL/7Ngk7PFA= callsites@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/callsites/-/callsites-3.0.0.tgz#fb7eb569b72ad7a45812f93fd9430a3e410b3dd3" integrity sha512-tWnkwu9YEq2uzlBDI4RcLn8jrFvF9AOi8PxDNU3hZZjJcjkcRAq3vCI+vZcg1SuxISDYe86k9VZFwAxDiJGoAw== camelcase@^5.0.0: version "5.3.1" resolved "https://registry.yarnpkg.com/camelcase/-/camelcase-5.3.1.tgz#e3c9b31569e106811df242f715725a1f4c494320" integrity sha512-L28STB170nwWS63UjtlEOE3dldQApaJXZkOI1uMFfzf3rRuPegHaHesyee+YxQ+W6SvRDQV6UrdOdRiR153wJg== caniuse-lite@^1.0.30000963: version "1.0.30000966" resolved "https://registry.yarnpkg.com/caniuse-lite/-/caniuse-lite-1.0.30000966.tgz#f3c6fefacfbfbfb981df6dfa68f2aae7bff41b64" integrity sha512-qqLQ/uYrpZmFhPY96VuBkMEo8NhVFBZ9y/Bh+KnvGzGJ5I8hvpIaWlF2pw5gqe4PLAL+ZjsPgMOvoXSpX21Keg== capture-stack-trace@^1.0.0: version "1.0.1" resolved "https://registry.yarnpkg.com/capture-stack-trace/-/capture-stack-trace-1.0.1.tgz#a6c0bbe1f38f3aa0b92238ecb6ff42c344d4135d" integrity sha512-mYQLZnx5Qt1JgB1WEiMCf2647plpGeQ2NMR/5L0HNZzGQo4fuSPnK+wjfPnKZV0aiJDgzmWqqkV/g7JD+DW0qw== cardinal@^2.1.1: version "2.1.1" resolved "https://registry.yarnpkg.com/cardinal/-/cardinal-2.1.1.tgz#7cc1055d822d212954d07b085dea251cc7bc5505" integrity sha1-fMEFXYItISlU0HsIXeolHMe8VQU= dependencies: ansicolors "~0.3.2" redeyed "~2.1.0" caseless@~0.12.0: version "0.12.0" resolved "https://registry.yarnpkg.com/caseless/-/caseless-0.12.0.tgz#1b681c21ff84033c826543090689420d187151dc" integrity sha1-G2gcIf+EAzyCZUMJBolCDRhxUdw= chalk@^1.0.0, chalk@^1.1.3: version "1.1.3" resolved "https://registry.yarnpkg.com/chalk/-/chalk-1.1.3.tgz#a8115c55e4a702fe4d150abd3872822a7e09fc98" integrity sha1-qBFcVeSnAv5NFQq9OHKCKn4J/Jg= dependencies: ansi-styles "^2.2.1" escape-string-regexp "^1.0.2" has-ansi "^2.0.0" strip-ansi "^3.0.0" supports-color "^2.0.0" chalk@^2.0.0, chalk@^2.0.1, chalk@^2.1.0, chalk@^2.3.1, chalk@^2.4.1, chalk@^2.4.2: version "2.4.2" resolved "https://registry.yarnpkg.com/chalk/-/chalk-2.4.2.tgz#cd42541677a54333cf541a49108c1432b44c9424" integrity sha512-Mti+f9lpJNcwF4tWV8/OrTTtF1gZi+f8FqlyAdouralcFWFQWF2+NgCHShjkCb+IFBLq9buZwE1xckQU4peSuQ== dependencies: ansi-styles "^3.2.1" escape-string-regexp "^1.0.5" supports-color "^5.3.0" chardet@^0.7.0: version "0.7.0" resolved "https://registry.yarnpkg.com/chardet/-/chardet-0.7.0.tgz#90094849f0937f2eedc2425d0d28a9e5f0cbad9e" integrity sha512-mT8iDcrh03qDGRRmoA2hmBJnxpllMR+0/0qlzjqZES6NdiWDcZkCNAk4rPFZ9Q85r27unkiNNg8ZOiwZXBHwcA== chokidar@^2.0.4, chokidar@^2.1.5: version "2.1.5" resolved "https://registry.yarnpkg.com/chokidar/-/chokidar-2.1.5.tgz#0ae8434d962281a5f56c72869e79cb6d9d86ad4d" integrity sha512-i0TprVWp+Kj4WRPtInjexJ8Q+BqTE909VpH8xVhXrJkoc5QC8VO9TryGOqTr+2hljzc1sC62t22h5tZePodM/A== dependencies: anymatch "^2.0.0" async-each "^1.0.1" braces "^2.3.2" glob-parent "^3.1.0" inherits "^2.0.3" is-binary-path "^1.0.0" is-glob "^4.0.0" normalize-path "^3.0.0" path-is-absolute "^1.0.0" readdirp "^2.2.1" upath "^1.1.1" optionalDependencies: fsevents "^1.2.7" chownr@^1.1.1: version "1.1.1" resolved "https://registry.yarnpkg.com/chownr/-/chownr-1.1.1.tgz#54726b8b8fff4df053c42187e801fb4412df1494" integrity sha512-j38EvO5+LHX84jlo6h4UzmOwi0UgW61WRyPtJz4qaadK5eY3BTS5TY/S1Stc3Uk2lIM6TPevAlULiEJwie860g== ci-info@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/ci-info/-/ci-info-2.0.0.tgz#67a9e964be31a51e15e5010d58e6f12834002f46" integrity sha512-5tK7EtrZ0N+OLFMthtqOj4fI2Jeb88C4CAZPu25LDVUgXJ0A3Js4PMGqrn0JU1W0Mh1/Z8wZzYPxqUrXeBboCQ== class-utils@^0.3.5: version "0.3.6" resolved "https://registry.yarnpkg.com/class-utils/-/class-utils-0.3.6.tgz#f93369ae8b9a7ce02fd41faad0ca83033190c463" integrity sha512-qOhPa/Fj7s6TY8H8esGu5QNpMMQxz79h+urzrNYN6mn+9BnxlDGf5QZ+XeCDsxSjPqsSR56XOZOJmpeurnLMeg== dependencies: arr-union "^3.1.0" define-property "^0.2.5" isobject "^3.0.0" static-extend "^0.1.1" cli-cursor@^2.0.0, cli-cursor@^2.1.0: version "2.1.0" resolved "https://registry.yarnpkg.com/cli-cursor/-/cli-cursor-2.1.0.tgz#b35dac376479facc3e94747d41d0d0f5238ffcb5" integrity sha1-s12sN2R5+sw+lHR9QdDQ9SOP/LU= dependencies: restore-cursor "^2.0.0" cli-truncate@^0.2.1: version "0.2.1" resolved "https://registry.yarnpkg.com/cli-truncate/-/cli-truncate-0.2.1.tgz#9f15cfbb0705005369216c626ac7d05ab90dd574" integrity sha1-nxXPuwcFAFNpIWxiasfQWrkN1XQ= dependencies: slice-ansi "0.0.4" string-width "^1.0.1" cli-truncate@^1.1.0: version "1.1.0" resolved "https://registry.yarnpkg.com/cli-truncate/-/cli-truncate-1.1.0.tgz#2b2dfd83c53cfd3572b87fc4d430a808afb04086" integrity sha512-bAtZo0u82gCfaAGfSNxUdTI9mNyza7D8w4CVCcaOsy7sgwDzvx6ekr6cuWJqY3UGzgnQ1+4wgENup5eIhgxEYA== dependencies: slice-ansi "^1.0.0" string-width "^2.0.0" cli-width@^2.0.0: version "2.2.0" resolved "https://registry.yarnpkg.com/cli-width/-/cli-width-2.2.0.tgz#ff19ede8a9a5e579324147b0c11f0fbcbabed639" integrity sha1-/xnt6Kml5XkyQUewwR8PvLq+1jk= cliui@^4.0.0, cliui@^4.1.0: version "4.1.0" resolved "https://registry.yarnpkg.com/cliui/-/cliui-4.1.0.tgz#348422dbe82d800b3022eef4f6ac10bf2e4d1b49" integrity sha512-4FG+RSG9DL7uEwRUZXZn3SS34DiDPfzP0VOiEwtUWlE+AR2EIg+hSyvrIgUUfhdgR/UkAeW2QHgeP+hWrXs7jQ== dependencies: string-width "^2.1.1" strip-ansi "^4.0.0" wrap-ansi "^2.0.0" code-point-at@^1.0.0: version "1.1.0" resolved "https://registry.yarnpkg.com/code-point-at/-/code-point-at-1.1.0.tgz#0d070b4d043a5bea33a2f1a40e2edb3d9a4ccf77" integrity sha1-DQcLTQQ6W+ozovGkDi7bPZpMz3c= collection-visit@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/collection-visit/-/collection-visit-1.0.0.tgz#4bc0373c164bc3291b4d368c829cf1a80a59dca0" integrity sha1-S8A3PBZLwykbTTaMgpzxqApZ3KA= dependencies: map-visit "^1.0.0" object-visit "^1.0.0" color-convert@^1.9.0: version "1.9.3" resolved "https://registry.yarnpkg.com/color-convert/-/color-convert-1.9.3.tgz#bb71850690e1f136567de629d2d5471deda4c1e8" integrity sha512-QfAUtd+vFdAtFQcC8CCyYt1fYWxSqAiK2cSD6zDB8N3cpsEBAvRxp9zOGg6G/SHHJYAT88/az/IuDGALsNVbGg== dependencies: color-name "1.1.3" color-name@1.1.3: version "1.1.3" resolved "https://registry.yarnpkg.com/color-name/-/color-name-1.1.3.tgz#a7d0558bd89c42f795dd42328f740831ca53bc25" integrity sha1-p9BVi9icQveV3UIyj3QIMcpTvCU= color-support@^1.1.0: version "1.1.3" resolved "https://registry.yarnpkg.com/color-support/-/color-support-1.1.3.tgz#93834379a1cc9a0c61f82f52f0d04322251bd5a2" integrity sha512-qiBjkpbMLO/HL68y+lh4q0/O1MZFj2RX6X/KmMa3+gJD3z+WwI1ZzDHysvqHGS3mP6mznPckpXmw1nI9cJjyRg== combined-stream@^1.0.6, combined-stream@~1.0.6: version "1.0.7" resolved "https://registry.yarnpkg.com/combined-stream/-/combined-stream-1.0.7.tgz#2d1d24317afb8abe95d6d2c0b07b57813539d828" integrity sha512-brWl9y6vOB1xYPZcpZde3N9zDByXTosAeMDo4p1wzo6UMOX4vumB+TP1RZ76sfE6Md68Q0NJSrE/gbezd4Ul+w== dependencies: delayed-stream "~1.0.0" commander@^2.14.1, commander@^2.8.1, commander@^2.9.0: version "2.19.0" resolved "https://registry.yarnpkg.com/commander/-/commander-2.19.0.tgz#f6198aa84e5b83c46054b94ddedbfed5ee9ff12a" integrity sha512-6tvAOO+D6OENvRAh524Dh9jcfKTYDQAqvqezbCW82xj5X0pSrcpxtvRKHLG0yBY6SD7PSDrJaj+0AiOcKVd1Xg== commander@~2.20.0: version "2.20.0" resolved "https://registry.yarnpkg.com/commander/-/commander-2.20.0.tgz#d58bb2b5c1ee8f87b0d340027e9e94e222c5a422" integrity sha512-7j2y+40w61zy6YC2iRNpUe/NwhNyoXrYpHMrSunaMG64nRnaf96zO/KMQR4OyN/UnE5KLyEBnKHd4aG3rskjpQ== commondir@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/commondir/-/commondir-1.0.1.tgz#ddd800da0c66127393cca5950ea968a3aaf1253b" integrity sha1-3dgA2gxmEnOTzKWVDqloo6rxJTs= component-emitter@^1.2.1: version "1.2.1" resolved "https://registry.yarnpkg.com/component-emitter/-/component-emitter-1.2.1.tgz#137918d6d78283f7df7a6b7c5a63e140e69425e6" integrity sha1-E3kY1teCg/ffemt8WmPhQOaUJeY= concat-map@0.0.1: version "0.0.1" resolved "https://registry.yarnpkg.com/concat-map/-/concat-map-0.0.1.tgz#d8a96bd77fd68df7793a73036a3ba0d5405d477b" integrity sha1-2Klr13/Wjfd5OnMDajug1UBdR3s= console-control-strings@^1.0.0, console-control-strings@~1.1.0: version "1.1.0" resolved "https://registry.yarnpkg.com/console-control-strings/-/console-control-strings-1.1.0.tgz#3d7cf4464db6446ea644bf4b39507f9851008e8e" integrity sha1-PXz0Rk22RG6mRL9LOVB/mFEAjo4= contains-path@^0.1.0: version "0.1.0" resolved "https://registry.yarnpkg.com/contains-path/-/contains-path-0.1.0.tgz#fe8cf184ff6670b6baef01a9d4861a5cbec4120a" integrity sha1-/ozxhP9mcLa67wGp1IYaXL7EEgo= convert-source-map@^1.1.0, convert-source-map@^1.5.1, convert-source-map@^1.6.0: version "1.6.0" resolved "https://registry.yarnpkg.com/convert-source-map/-/convert-source-map-1.6.0.tgz#51b537a8c43e0f04dec1993bffcdd504e758ac20" integrity sha512-eFu7XigvxdZ1ETfbgPBohgyQ/Z++C0eEhTor0qRwBw9unw+L0/6V8wkSuGgzdThkiS5lSpdptOQPD8Ak40a+7A== dependencies: safe-buffer "~5.1.1" copy-descriptor@^0.1.0: version "0.1.1" resolved "https://registry.yarnpkg.com/copy-descriptor/-/copy-descriptor-0.1.1.tgz#676f6eb3c39997c2ee1ac3a924fd6124748f578d" integrity sha1-Z29us8OZl8LuGsOpJP1hJHSPV40= core-js-compat@^3.0.0: version "3.0.1" resolved "https://registry.yarnpkg.com/core-js-compat/-/core-js-compat-3.0.1.tgz#bff73ba31ca8687431b9c88f78d3362646fb76f0" integrity sha512-2pC3e+Ht/1/gD7Sim/sqzvRplMiRnFQVlPpDVaHtY9l7zZP7knamr3VRD6NyGfHd84MrDC0tAM9ulNxYMW0T3g== dependencies: browserslist "^4.5.4" core-js "3.0.1" core-js-pure "3.0.1" semver "^6.0.0" core-js-pure@3.0.1: version "3.0.1" resolved "https://registry.yarnpkg.com/core-js-pure/-/core-js-pure-3.0.1.tgz#37358fb0d024e6b86d443d794f4e37e949098cbe" integrity sha512-mSxeQ6IghKW3MoyF4cz19GJ1cMm7761ON+WObSyLfTu/Jn3x7w4NwNFnrZxgl4MTSvYYepVLNuRtlB4loMwJ5g== core-js@3.0.1: version "3.0.1" resolved "https://registry.yarnpkg.com/core-js/-/core-js-3.0.1.tgz#1343182634298f7f38622f95e73f54e48ddf4738" integrity sha512-sco40rF+2KlE0ROMvydjkrVMMG1vYilP2ALoRXcYR4obqbYIuV3Bg+51GEDW+HF8n7NRA+iaA4qD0nD9lo9mew== core-js@^2.4.0, core-js@^2.5.0: version "2.6.5" resolved "https://registry.yarnpkg.com/core-js/-/core-js-2.6.5.tgz#44bc8d249e7fb2ff5d00e0341a7ffb94fbf67895" integrity sha512-klh/kDpwX8hryYL14M9w/xei6vrv6sE8gTHDG7/T/+SEovB/G4ejwcfE/CBzO6Edsu+OETZMZ3wcX/EjUkrl5A== core-util-is@1.0.2, core-util-is@~1.0.0: version "1.0.2" resolved "https://registry.yarnpkg.com/core-util-is/-/core-util-is-1.0.2.tgz#b5fd54220aa2bc5ab57aab7140c940754503c1a7" integrity sha1-tf1UIgqivFq1eqtxQMlAdUUDwac= cosmiconfig@^5.0.2: version "5.1.0" resolved "https://registry.yarnpkg.com/cosmiconfig/-/cosmiconfig-5.1.0.tgz#6c5c35e97f37f985061cdf653f114784231185cf" integrity sha512-kCNPvthka8gvLtzAxQXvWo4FxqRB+ftRZyPZNuab5ngvM9Y7yw7hbEysglptLgpkGX9nAOKTBVkHUAe8xtYR6Q== dependencies: import-fresh "^2.0.0" is-directory "^0.3.1" js-yaml "^3.9.0" lodash.get "^4.4.2" parse-json "^4.0.0" cosmiconfig@^5.2.0: version "5.2.0" resolved "https://registry.yarnpkg.com/cosmiconfig/-/cosmiconfig-5.2.0.tgz#45038e4d28a7fe787203aede9c25bca4a08b12c8" integrity sha512-nxt+Nfc3JAqf4WIWd0jXLjTJZmsPLrA9DDc4nRw2KFJQJK7DNooqSXrNI7tzLG50CF8axczly5UV929tBmh/7g== dependencies: import-fresh "^2.0.0" is-directory "^0.3.1" js-yaml "^3.13.0" parse-json "^4.0.0" coveralls@^3.0.3: version "3.0.3" resolved "https://registry.yarnpkg.com/coveralls/-/coveralls-3.0.3.tgz#83b1c64aea1c6afa69beaf50b55ac1bc4d13e2b8" integrity sha512-viNfeGlda2zJr8Gj1zqXpDMRjw9uM54p7wzZdvLRyOgnAfCe974Dq4veZkjJdxQXbmdppu6flEajFYseHYaUhg== dependencies: growl "~> 1.10.0" js-yaml "^3.11.0" lcov-parse "^0.0.10" log-driver "^1.2.7" minimist "^1.2.0" request "^2.86.0" cp-file@^6.2.0: version "6.2.0" resolved "https://registry.yarnpkg.com/cp-file/-/cp-file-6.2.0.tgz#40d5ea4a1def2a9acdd07ba5c0b0246ef73dc10d" integrity sha512-fmvV4caBnofhPe8kOcitBwSn2f39QLjnAnGq3gO9dfd75mUytzKNZB1hde6QHunW2Rt+OwuBOMc3i1tNElbszA== dependencies: graceful-fs "^4.1.2" make-dir "^2.0.0" nested-error-stacks "^2.0.0" pify "^4.0.1" safe-buffer "^5.0.1" cross-spawn@^4: version "4.0.2" resolved "https://registry.yarnpkg.com/cross-spawn/-/cross-spawn-4.0.2.tgz#7b9247621c23adfdd3856004a823cbe397424d41" integrity sha1-e5JHYhwjrf3ThWAEqCPL45dCTUE= dependencies: lru-cache "^4.0.1" which "^1.2.9" cross-spawn@^6.0.0, cross-spawn@^6.0.5: version "6.0.5" resolved "https://registry.yarnpkg.com/cross-spawn/-/cross-spawn-6.0.5.tgz#4a5ec7c64dfae22c3a14124dbacdee846d80cbc4" integrity sha512-eTVLrBSt7fjbDygz805pMnstIs2VTBNkRm0qxZd+M7A5XDdxVRWO5MxGBXZhjY4cqLYLdtrGqRf8mBPmzwSpWQ== dependencies: nice-try "^1.0.4" path-key "^2.0.1" semver "^5.5.0" shebang-command "^1.2.0" which "^1.2.9" csstype@^2.2.0: version "2.6.4" resolved "https://registry.yarnpkg.com/csstype/-/csstype-2.6.4.tgz#d585a6062096e324e7187f80e04f92bd0f00e37f" integrity sha512-lAJUJP3M6HxFXbqtGRc0iZrdyeN+WzOWeY0q/VnFzI+kqVrYIzC7bWlKqCW7oCIdzoPkvfp82EVvrTlQ8zsWQg== dashdash@^1.12.0: version "1.14.1" resolved "https://registry.yarnpkg.com/dashdash/-/dashdash-1.14.1.tgz#853cfa0f7cbe2fed5de20326b8dd581035f6e2f0" integrity sha1-hTz6D3y+L+1d4gMmuN1YEDX24vA= dependencies: assert-plus "^1.0.0" date-fns@^1.27.2: version "1.30.1" resolved "https://registry.yarnpkg.com/date-fns/-/date-fns-1.30.1.tgz#2e71bf0b119153dbb4cc4e88d9ea5acfb50dc05c" integrity sha512-hBSVCvSmWC+QypYObzwGOd9wqdDpOt+0wl0KbU+R+uuZBS1jN8VsD1ss3irQDknRj5NvxiTF6oj/nDRnN/UQNw== debug@^2.1.2, debug@^2.1.3, debug@^2.2.0, debug@^2.3.3, debug@^2.6.8, debug@^2.6.9: version "2.6.9" resolved "https://registry.yarnpkg.com/debug/-/debug-2.6.9.tgz#5d128515df134ff327e90a4c93f4e077a536341f" integrity sha512-bC7ElrdJaJnPbAP+1EotYvqZsb3ecl5wi6Bfi6BJTUcNowp6cvspg0jXznRTKDjm/E7AdgFBVeAPVMNcKGsHMA== dependencies: ms "2.0.0" debug@^3.1.0: version "3.2.6" resolved "https://registry.yarnpkg.com/debug/-/debug-3.2.6.tgz#e83d17de16d8a7efb7717edbe5fb10135eee629b" integrity sha512-mel+jf7nrtEl5Pn1Qx46zARXKDpBbvzezse7p7LqINmdoIk8PYP5SySaxEmYv6TZ0JyEKA1hsCId6DIhgITtWQ== dependencies: ms "^2.1.1" debug@^4.0.1, debug@^4.1.0, debug@^4.1.1: version "4.1.1" resolved "https://registry.yarnpkg.com/debug/-/debug-4.1.1.tgz#3b72260255109c6b589cee050f1d516139664791" integrity sha512-pYAIzeRo8J6KPEaJ0VWOh5Pzkbw/RetuzehGM7QRRX5he4fPHx2rdKMB256ehJCkX+XRQm16eZLqLNS8RSZXZw== dependencies: ms "^2.1.1" decamelize@^1.2.0: version "1.2.0" resolved "https://registry.yarnpkg.com/decamelize/-/decamelize-1.2.0.tgz#f6534d15148269b20352e7bee26f501f9a191290" integrity sha1-9lNNFRSCabIDUue+4m9QH5oZEpA= decode-uri-component@^0.2.0: version "0.2.0" resolved "https://registry.yarnpkg.com/decode-uri-component/-/decode-uri-component-0.2.0.tgz#eb3913333458775cb84cd1a1fae062106bb87545" integrity sha1-6zkTMzRYd1y4TNGh+uBiEGu4dUU= dedent@^0.7.0: version "0.7.0" resolved "https://registry.yarnpkg.com/dedent/-/dedent-0.7.0.tgz#2495ddbaf6eb874abb0e1be9df22d2e5a544326c" integrity sha1-JJXduvbrh0q7Dhvp3yLS5aVEMmw= deep-extend@^0.6.0: version "0.6.0" resolved "https://registry.yarnpkg.com/deep-extend/-/deep-extend-0.6.0.tgz#c4fa7c95404a17a9c3e8ca7e1537312b736330ac" integrity sha512-LOHxIOaPYdHlJRtCQfDIVZtfw/ufM8+rVj649RIHzcm/vGwQRXFt6OPqIFWsm2XEMrNIEtWR64sY1LEKD2vAOA== deep-is@~0.1.3: version "0.1.3" resolved "https://registry.yarnpkg.com/deep-is/-/deep-is-0.1.3.tgz#b369d6fb5dbc13eecf524f91b070feedc357cf34" integrity sha1-s2nW+128E+7PUk+RsHD+7cNXzzQ= default-require-extensions@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/default-require-extensions/-/default-require-extensions-2.0.0.tgz#f5f8fbb18a7d6d50b21f641f649ebb522cfe24f7" integrity sha1-9fj7sYp9bVCyH2QfZJ67Uiz+JPc= dependencies: strip-bom "^3.0.0" define-properties@^1.1.2: version "1.1.3" resolved "https://registry.yarnpkg.com/define-properties/-/define-properties-1.1.3.tgz#cf88da6cbee26fe6db7094f61d870cbd84cee9f1" integrity sha512-3MqfYKj2lLzdMSf8ZIZE/V+Zuy+BgD6f164e8K2w7dgnpKArBDerGYpM46IYYcjnkdPNMjPk9A6VFB8+3SKlXQ== dependencies: object-keys "^1.0.12" define-property@^0.2.5: version "0.2.5" resolved "https://registry.yarnpkg.com/define-property/-/define-property-0.2.5.tgz#c35b1ef918ec3c990f9a5bc57be04aacec5c8116" integrity sha1-w1se+RjsPJkPmlvFe+BKrOxcgRY= dependencies: is-descriptor "^0.1.0" define-property@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/define-property/-/define-property-1.0.0.tgz#769ebaaf3f4a63aad3af9e8d304c9bbe79bfb0e6" integrity sha1-dp66rz9KY6rTr56NMEybvnm/sOY= dependencies: is-descriptor "^1.0.0" define-property@^2.0.2: version "2.0.2" resolved "https://registry.yarnpkg.com/define-property/-/define-property-2.0.2.tgz#d459689e8d654ba77e02a817f8710d702cb16e9d" integrity sha512-jwK2UV4cnPpbcG7+VRARKTZPUWowwXA8bzH5NP6ud0oeAxyYPuGZUAC7hMugpCdz4BeSZl2Dl9k66CHJ/46ZYQ== dependencies: is-descriptor "^1.0.2" isobject "^3.0.1" del@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/del/-/del-3.0.0.tgz#53ecf699ffcbcb39637691ab13baf160819766e5" integrity sha1-U+z2mf/LyzljdpGrE7rxYIGXZuU= dependencies: globby "^6.1.0" is-path-cwd "^1.0.0" is-path-in-cwd "^1.0.0" p-map "^1.1.1" pify "^3.0.0" rimraf "^2.2.8" delayed-stream@~1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/delayed-stream/-/delayed-stream-1.0.0.tgz#df3ae199acadfb7d440aaae0b29e2272b24ec619" integrity sha1-3zrhmayt+31ECqrgsp4icrJOxhk= delegates@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/delegates/-/delegates-1.0.0.tgz#84c6e159b81904fdca59a0ef44cd870d31250f9a" integrity sha1-hMbhWbgZBP3KWaDvRM2HDTElD5o= detect-indent@^4.0.0: version "4.0.0" resolved "https://registry.yarnpkg.com/detect-indent/-/detect-indent-4.0.0.tgz#f76d064352cdf43a1cb6ce619c4ee3a9475de208" integrity sha1-920GQ1LN9Docts5hnE7jqUdd4gg= dependencies: repeating "^2.0.0" detect-libc@^1.0.2: version "1.0.3" resolved "https://registry.yarnpkg.com/detect-libc/-/detect-libc-1.0.3.tgz#fa137c4bd698edf55cd5cd02ac559f91a4c4ba9b" integrity sha1-+hN8S9aY7fVc1c0CrFWfkaTEups= diff@^1.3.2: version "1.4.0" resolved "https://registry.yarnpkg.com/diff/-/diff-1.4.0.tgz#7f28d2eb9ee7b15a97efd89ce63dcfdaa3ccbabf" integrity sha1-fyjS657nsVqX79ic5j3P2qPMur8= diff@^3.1.0: version "3.5.0" resolved "https://registry.yarnpkg.com/diff/-/diff-3.5.0.tgz#800c0dd1e0a8bfbc95835c202ad220fe317e5a12" integrity sha512-A46qtFgd+g7pDZinpnwiRJtxbC1hpgf0uzP3iG89scHk0AUC7A1TGxf5OiiOUv/JMZR8GOt8hL900hV0bOy5xA== diff@^4.0.1: version "4.0.1" resolved "https://registry.yarnpkg.com/diff/-/diff-4.0.1.tgz#0c667cb467ebbb5cea7f14f135cc2dba7780a8ff" integrity sha512-s2+XdvhPCOF01LRQBC8hf4vhbVmI2CGS5aZnxLJlT5FtdhPCDFq80q++zK2KlrVorVDdL5BOGZ/VfLrVtYNF+Q== doctrine@1.5.0: version "1.5.0" resolved "https://registry.yarnpkg.com/doctrine/-/doctrine-1.5.0.tgz#379dce730f6166f76cefa4e6707a159b02c5a6fa" integrity sha1-N53Ocw9hZvds76TmcHoVmwLFpvo= dependencies: esutils "^2.0.2" isarray "^1.0.0" doctrine@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/doctrine/-/doctrine-3.0.0.tgz#addebead72a6574db783639dc87a121773973961" integrity sha512-yS+Q5i3hBf7GBkd4KG8a7eBNNWNGLTaEwwYWUijIYM7zrlYDM0BFXHjjPWlWZ1Rg7UaddZeIDmi9jF3HmqiQ2w== dependencies: esutils "^2.0.2" domain-browser@^1.2.0: version "1.2.0" resolved "https://registry.yarnpkg.com/domain-browser/-/domain-browser-1.2.0.tgz#3d31f50191a6749dd1375a7f522e823d42e54eda" integrity sha512-jnjyiM6eRyZl2H+W8Q/zLMA481hzi0eszAaBUzIVnmYVDBbnLxVNnfu1HgEBvCbL+71FrxMl3E6lpKH7Ge3OXA== ecc-jsbn@~0.1.1: version "0.1.2" resolved "https://registry.yarnpkg.com/ecc-jsbn/-/ecc-jsbn-0.1.2.tgz#3a83a904e54353287874c564b7549386849a98c9" integrity sha1-OoOpBOVDUyh4dMVkt1SThoSamMk= dependencies: jsbn "~0.1.0" safer-buffer "^2.1.0" electron-to-chromium@^1.3.127: version "1.3.131" resolved "https://registry.yarnpkg.com/electron-to-chromium/-/electron-to-chromium-1.3.131.tgz#205a0b7a276b3f56bc056f19178909243054252a" integrity sha512-NSO4jLeyGLWrT4mzzfYX8vt1MYCoMI5LxSYAjt0H9+LF/14JyiKJSyyjA6AJTxflZlEM5v3QU33F0ohbPMCAPg== elegant-spinner@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/elegant-spinner/-/elegant-spinner-1.0.1.tgz#db043521c95d7e303fd8f345bedc3349cfb0729e" integrity sha1-2wQ1IcldfjA/2PNFvtwzSc+wcp4= emoji-regex@^7.0.1: version "7.0.3" resolved "https://registry.yarnpkg.com/emoji-regex/-/emoji-regex-7.0.3.tgz#933a04052860c85e83c122479c4748a8e4c72156" integrity sha512-CwBLREIQ7LvYFB0WyRvwhq5N5qPhc6PMjD6bYggFlI5YyDgl+0vxq5VHbMOFqLg7hfWzmu8T5Z1QofhmTIhItA== end-of-stream@^1.1.0: version "1.4.1" resolved "https://registry.yarnpkg.com/end-of-stream/-/end-of-stream-1.4.1.tgz#ed29634d19baba463b6ce6b80a37213eab71ec43" integrity sha512-1MkrZNvWTKCaigbn+W15elq2BB/L22nqrSY5DKlo3X6+vclJm8Bb5djXJBmEX6fS3+zCh/F4VBK5Z2KxJt4s2Q== dependencies: once "^1.4.0" error-ex@^1.2.0, error-ex@^1.3.1: version "1.3.2" resolved "https://registry.yarnpkg.com/error-ex/-/error-ex-1.3.2.tgz#b4ac40648107fdcdcfae242f428bea8a14d4f1bf" integrity sha512-7dFHNmqeFSEt2ZBsCriorKnn3Z2pj+fd9kmI6QoWw4//DL+icEBfc0U7qJCisqrTsKTjw4fNFy2pW9OqStD84g== dependencies: is-arrayish "^0.2.1" es-abstract@^1.7.0: version "1.13.0" resolved "https://registry.yarnpkg.com/es-abstract/-/es-abstract-1.13.0.tgz#ac86145fdd5099d8dd49558ccba2eaf9b88e24e9" integrity sha512-vDZfg/ykNxQVwup/8E1BZhVzFfBxs9NqMzGcvIJrqg5k2/5Za2bWo40dK2J1pgLngZ7c+Shh8lwYtLGyrwPutg== dependencies: es-to-primitive "^1.2.0" function-bind "^1.1.1" has "^1.0.3" is-callable "^1.1.4" is-regex "^1.0.4" object-keys "^1.0.12" es-to-primitive@^1.2.0: version "1.2.0" resolved "https://registry.yarnpkg.com/es-to-primitive/-/es-to-primitive-1.2.0.tgz#edf72478033456e8dda8ef09e00ad9650707f377" integrity sha512-qZryBOJjV//LaxLTV6UC//WewneB3LcXOL9NP++ozKVXsIIIpm/2c13UDiD9Jp2eThsecw9m3jPqDwTyobcdbg== dependencies: is-callable "^1.1.4" is-date-object "^1.0.1" is-symbol "^1.0.2" es6-error@^4.0.1: version "4.1.1" resolved "https://registry.yarnpkg.com/es6-error/-/es6-error-4.1.1.tgz#9e3af407459deed47e9a91f9b885a84eb05c561d" integrity sha512-Um/+FxMr9CISWh0bi5Zv0iOD+4cFh5qLeks1qhAopKVAJw3drgKbKySikp7wGhDL0HPeaja0P5ULZrxLkniUVg== escape-string-regexp@^1.0.2, escape-string-regexp@^1.0.3, escape-string-regexp@^1.0.4, escape-string-regexp@^1.0.5: version "1.0.5" resolved "https://registry.yarnpkg.com/escape-string-regexp/-/escape-string-regexp-1.0.5.tgz#1b61c0562190a8dff6ae3bb2cf0200ca130b86d4" integrity sha1-G2HAViGQqN/2rjuyzwIAyhMLhtQ= eslint-config-env@^5.0.0: version "5.0.0" resolved "https://registry.yarnpkg.com/eslint-config-env/-/eslint-config-env-5.0.0.tgz#ee3c557e3cdb627c0dbcc7f898e473ff05182143" integrity sha512-oCFGqTFY77B/xAYulluZUiI5PbN/Mgeoyqb/jRdab0YM2g2UvOdHMHQYDZgq8hXH1kvSyZRwj+/7lO9Z7M0wKg== dependencies: app-root-path "^2.2.1" read-pkg-up "^5.0.0" semver "^6.0.0" eslint-config-prettier@^4.0.0: version "4.2.0" resolved "https://registry.yarnpkg.com/eslint-config-prettier/-/eslint-config-prettier-4.2.0.tgz#70b946b629cd0e3e98233fd9ecde4cb9778de96c" integrity sha512-y0uWc/FRfrHhpPZCYflWC8aE0KRJRY04rdZVfl8cL3sEZmOYyaBdhdlQPjKZBnuRMyLVK+JUZr7HaZFClQiH4w== dependencies: get-stdin "^6.0.0" eslint-import-resolver-node@^0.3.2: version "0.3.2" resolved "https://registry.yarnpkg.com/eslint-import-resolver-node/-/eslint-import-resolver-node-0.3.2.tgz#58f15fb839b8d0576ca980413476aab2472db66a" integrity sha512-sfmTqJfPSizWu4aymbPr4Iidp5yKm8yDkHp+Ir3YiTHiiDfxh69mOUsmiqW6RZ9zRXFaF64GtYmN7e+8GHBv6Q== dependencies: debug "^2.6.9" resolve "^1.5.0" eslint-module-utils@^2.2.0: version "2.2.0" resolved "https://registry.yarnpkg.com/eslint-module-utils/-/eslint-module-utils-2.2.0.tgz#b270362cd88b1a48ad308976ce7fa54e98411746" integrity sha1-snA2LNiLGkitMIl2zn+lTphBF0Y= dependencies: debug "^2.6.8" pkg-dir "^1.0.0" eslint-module-utils@^2.4.0: version "2.4.0" resolved "https://registry.yarnpkg.com/eslint-module-utils/-/eslint-module-utils-2.4.0.tgz#8b93499e9b00eab80ccb6614e69f03678e84e09a" integrity sha512-14tltLm38Eu3zS+mt0KvILC3q8jyIAH518MlG+HO0p+yK885Lb1UHTY/UgR91eOyGdmxAPb+OLoW4znqIT6Ndw== dependencies: debug "^2.6.8" pkg-dir "^2.0.0" eslint-plugin-es@^1.4.0: version "1.4.0" resolved "https://registry.yarnpkg.com/eslint-plugin-es/-/eslint-plugin-es-1.4.0.tgz#475f65bb20c993fc10e8c8fe77d1d60068072da6" integrity sha512-XfFmgFdIUDgvaRAlaXUkxrRg5JSADoRC8IkKLc/cISeR3yHVMefFHQZpcyXXEUUPHfy5DwviBcrfqlyqEwlQVw== dependencies: eslint-utils "^1.3.0" regexpp "^2.0.1" eslint-plugin-import-order-alphabetical@^0.0.2: version "0.0.2" resolved "https://registry.yarnpkg.com/eslint-plugin-import-order-alphabetical/-/eslint-plugin-import-order-alphabetical-0.0.2.tgz#301e1a7d7cef3790f417d4dceb4b62291ac5ddff" integrity sha512-9WBxC3RzQrJTAJ/epl64k4pvug/Le1OvhydICoQydK/2M4+36lnAlsn/3dsvXR+1WnRuc2mziNTkFUs+tx+22w== dependencies: eslint-module-utils "^2.2.0" lodash "^4.17.4" resolve "^1.6.0" eslint-plugin-import@^2.16.0: version "2.17.2" resolved "https://registry.yarnpkg.com/eslint-plugin-import/-/eslint-plugin-import-2.17.2.tgz#d227d5c6dc67eca71eb590d2bb62fb38d86e9fcb" integrity sha512-m+cSVxM7oLsIpmwNn2WXTJoReOF9f/CtLMo7qOVmKd1KntBy0hEcuNZ3erTmWjx+DxRO0Zcrm5KwAvI9wHcV5g== dependencies: array-includes "^3.0.3" contains-path "^0.1.0" debug "^2.6.9" doctrine "1.5.0" eslint-import-resolver-node "^0.3.2" eslint-module-utils "^2.4.0" has "^1.0.3" lodash "^4.17.11" minimatch "^3.0.4" read-pkg-up "^2.0.0" resolve "^1.10.0" eslint-plugin-node@^9.0.1: version "9.0.1" resolved "https://registry.yarnpkg.com/eslint-plugin-node/-/eslint-plugin-node-9.0.1.tgz#93e44626fa62bcb6efea528cee9687663dc03b62" integrity sha512-fljT5Uyy3lkJzuqhxrYanLSsvaILs9I7CmQ31atTtZ0DoIzRbbvInBh4cQ1CrthFHInHYBQxfPmPt6KLHXNXdw== dependencies: eslint-plugin-es "^1.4.0" eslint-utils "^1.3.1" ignore "^5.1.1" minimatch "^3.0.4" resolve "^1.10.1" semver "^6.0.0" eslint-plugin-prettier@^3.0.0: version "3.0.1" resolved "https://registry.yarnpkg.com/eslint-plugin-prettier/-/eslint-plugin-prettier-3.0.1.tgz#19d521e3981f69dd6d14f64aec8c6a6ac6eb0b0d" integrity sha512-/PMttrarPAY78PLvV3xfWibMOdMDl57hmlQ2XqFeA37wd+CJ7WSxV7txqjVPHi/AAFKd2lX0ZqfsOc/i5yFCSQ== dependencies: prettier-linter-helpers "^1.0.0" eslint-scope@3.7.1: version "3.7.1" resolved "https://registry.yarnpkg.com/eslint-scope/-/eslint-scope-3.7.1.tgz#3d63c3edfda02e06e01a452ad88caacc7cdcb6e8" integrity sha1-PWPD7f2gLgbgGkUq2IyqzHzctug= dependencies: esrecurse "^4.1.0" estraverse "^4.1.1" eslint-scope@^4.0.3: version "4.0.3" resolved "https://registry.yarnpkg.com/eslint-scope/-/eslint-scope-4.0.3.tgz#ca03833310f6889a3264781aa82e63eb9cfe7848" integrity sha512-p7VutNr1O/QrxysMo3E45FjYDTeXBy0iTltPFNSqKAIfjDSXC+4dj+qfyuD8bfAXrW/y6lW3O76VaYNPKfpKrg== dependencies: esrecurse "^4.1.0" estraverse "^4.1.1" eslint-utils@^1.3.0, eslint-utils@^1.3.1: version "1.3.1" resolved "https://registry.yarnpkg.com/eslint-utils/-/eslint-utils-1.3.1.tgz#9a851ba89ee7c460346f97cf8939c7298827e512" integrity sha512-Z7YjnIldX+2XMcjr7ZkgEsOj/bREONV60qYeB/bjMAqqqZ4zxKyWX+BOUkdmRmA9riiIPVvo5x86m5elviOk0Q== eslint-visitor-keys@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/eslint-visitor-keys/-/eslint-visitor-keys-1.0.0.tgz#3f3180fb2e291017716acb4c9d6d5b5c34a6a81d" integrity sha512-qzm/XxIbxm/FHyH341ZrbnMUpe+5Bocte9xkmFMzPMjRaZMcXww+MpBptFvtU+79L362nqiLhekCxCxDPaUMBQ== eslint@^5.14.1: version "5.16.0" resolved "https://registry.yarnpkg.com/eslint/-/eslint-5.16.0.tgz#a1e3ac1aae4a3fbd8296fcf8f7ab7314cbb6abea" integrity sha512-S3Rz11i7c8AA5JPv7xAH+dOyq/Cu/VXHiHXBPOU1k/JAM5dXqQPt3qcrhpHSorXmrpu2g0gkIBVXAqCpzfoZIg== dependencies: "@babel/code-frame" "^7.0.0" ajv "^6.9.1" chalk "^2.1.0" cross-spawn "^6.0.5" debug "^4.0.1" doctrine "^3.0.0" eslint-scope "^4.0.3" eslint-utils "^1.3.1" eslint-visitor-keys "^1.0.0" espree "^5.0.1" esquery "^1.0.1" esutils "^2.0.2" file-entry-cache "^5.0.1" functional-red-black-tree "^1.0.1" glob "^7.1.2" globals "^11.7.0" ignore "^4.0.6" import-fresh "^3.0.0" imurmurhash "^0.1.4" inquirer "^6.2.2" js-yaml "^3.13.0" json-stable-stringify-without-jsonify "^1.0.1" levn "^0.3.0" lodash "^4.17.11" minimatch "^3.0.4" mkdirp "^0.5.1" natural-compare "^1.4.0" optionator "^0.8.2" path-is-inside "^1.0.2" progress "^2.0.0" regexpp "^2.0.1" semver "^5.5.1" strip-ansi "^4.0.0" strip-json-comments "^2.0.1" table "^5.2.3" text-table "^0.2.0" esm@^3.2.22: version "3.2.22" resolved "https://registry.yarnpkg.com/esm/-/esm-3.2.22.tgz#5062c2e22fee3ccfee4e8f20da768330da90d6e3" integrity sha512-z8YG7U44L82j1XrdEJcqZOLUnjxco8pO453gKOlaMD1/md1n/5QrscAmYG+oKUspsmDLuBFZrpbxI6aQ67yRxA== espree@^5.0.1: version "5.0.1" resolved "https://registry.yarnpkg.com/espree/-/espree-5.0.1.tgz#5d6526fa4fc7f0788a5cf75b15f30323e2f81f7a" integrity sha512-qWAZcWh4XE/RwzLJejfcofscgMc9CamR6Tn1+XRXNzrvUSSbiAjGOI/fggztjIi7y9VLPqnICMIPiGyr8JaZ0A== dependencies: acorn "^6.0.7" acorn-jsx "^5.0.0" eslint-visitor-keys "^1.0.0" esprima@^4.0.0, esprima@~4.0.0: version "4.0.1" resolved "https://registry.yarnpkg.com/esprima/-/esprima-4.0.1.tgz#13b04cdb3e6c5d19df91ab6987a8695619b0aa71" integrity sha512-eGuFFw7Upda+g4p+QHvnW0RyTX/SVeJBDM/gCtMARO0cLuT2HcEKnTPvhjV6aGeqrCB/sbNop0Kszm0jsaWU4A== esquery@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/esquery/-/esquery-1.0.1.tgz#406c51658b1f5991a5f9b62b1dc25b00e3e5c708" integrity sha512-SmiyZ5zIWH9VM+SRUReLS5Q8a7GxtRdxEBVZpm98rJM7Sb+A9DVCndXfkeFUd3byderg+EbDkfnevfCwynWaNA== dependencies: estraverse "^4.0.0" esrecurse@^4.1.0: version "4.2.1" resolved "https://registry.yarnpkg.com/esrecurse/-/esrecurse-4.2.1.tgz#007a3b9fdbc2b3bb87e4879ea19c92fdbd3942cf" integrity sha512-64RBB++fIOAXPw3P9cy89qfMlvZEXZkqqJkjqqXIvzP5ezRZjW+lPWjw35UX/3EhUPFYbg5ER4JYgDw4007/DQ== dependencies: estraverse "^4.1.0" estraverse@^4.0.0, estraverse@^4.1.0, estraverse@^4.1.1: version "4.2.0" resolved "https://registry.yarnpkg.com/estraverse/-/estraverse-4.2.0.tgz#0dee3fed31fcd469618ce7342099fc1afa0bdb13" integrity sha1-De4/7TH81GlhjOc0IJn8GvoL2xM= esutils@^2.0.2: version "2.0.2" resolved "https://registry.yarnpkg.com/esutils/-/esutils-2.0.2.tgz#0abf4f1caa5bcb1f7a9d8acc6dea4faaa04bac9b" integrity sha1-Cr9PHKpbyx96nYrMbepPqqBLrJs= events-to-array@^1.0.1: version "1.1.2" resolved "https://registry.yarnpkg.com/events-to-array/-/events-to-array-1.1.2.tgz#2d41f563e1fe400ed4962fe1a4d5c6a7539df7f6" integrity sha1-LUH1Y+H+QA7Uli/hpNXGp1Od9/Y= execa@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/execa/-/execa-1.0.0.tgz#c6236a5bb4df6d6f15e88e7f017798216749ddd8" integrity sha512-adbxcyWV46qiHyvSp50TKt05tB4tK3HcmF7/nxfAdhnox83seTDbwnaqKO4sXRy7roHAIFqJP/Rw/AuEbX61LA== dependencies: cross-spawn "^6.0.0" get-stream "^4.0.0" is-stream "^1.1.0" npm-run-path "^2.0.0" p-finally "^1.0.0" signal-exit "^3.0.0" strip-eof "^1.0.0" expand-brackets@^2.1.4: version "2.1.4" resolved "https://registry.yarnpkg.com/expand-brackets/-/expand-brackets-2.1.4.tgz#b77735e315ce30f6b6eff0f83b04151a22449622" integrity sha1-t3c14xXOMPa27/D4OwQVGiJEliI= dependencies: debug "^2.3.3" define-property "^0.2.5" extend-shallow "^2.0.1" posix-character-classes "^0.1.0" regex-not "^1.0.0" snapdragon "^0.8.1" to-regex "^3.0.1" extend-shallow@^2.0.1: version "2.0.1" resolved "https://registry.yarnpkg.com/extend-shallow/-/extend-shallow-2.0.1.tgz#51af7d614ad9a9f610ea1bafbb989d6b1c56890f" integrity sha1-Ua99YUrZqfYQ6huvu5idaxxWiQ8= dependencies: is-extendable "^0.1.0" extend-shallow@^3.0.0, extend-shallow@^3.0.2: version "3.0.2" resolved "https://registry.yarnpkg.com/extend-shallow/-/extend-shallow-3.0.2.tgz#26a71aaf073b39fb2127172746131c2704028db8" integrity sha1-Jqcarwc7OfshJxcnRhMcJwQCjbg= dependencies: assign-symbols "^1.0.0" is-extendable "^1.0.1" extend@~3.0.2: version "3.0.2" resolved "https://registry.yarnpkg.com/extend/-/extend-3.0.2.tgz#f8b1136b4071fbd8eb140aff858b1019ec2915fa" integrity sha512-fjquC59cD7CyW6urNXK0FBufkZcoiGG80wTuPujX590cB5Ttln20E2UB4S/WARVqhXffZl2LNgS+gQdPIIim/g== external-editor@^3.0.3: version "3.0.3" resolved "https://registry.yarnpkg.com/external-editor/-/external-editor-3.0.3.tgz#5866db29a97826dbe4bf3afd24070ead9ea43a27" integrity sha512-bn71H9+qWoOQKyZDo25mOMVpSmXROAsTJVVVYzrrtol3d4y+AsKjf4Iwl2Q+IuT0kFSQ1qo166UuIwqYq7mGnA== dependencies: chardet "^0.7.0" iconv-lite "^0.4.24" tmp "^0.0.33" extglob@^2.0.4: version "2.0.4" resolved "https://registry.yarnpkg.com/extglob/-/extglob-2.0.4.tgz#ad00fe4dc612a9232e8718711dc5cb5ab0285543" integrity sha512-Nmb6QXkELsuBr24CJSkilo6UHHgbekK5UiZgfE6UHD3Eb27YC6oD+bhcT+tJ6cl8dmsgdQxnWlcry8ksBIBLpw== dependencies: array-unique "^0.3.2" define-property "^1.0.0" expand-brackets "^2.1.4" extend-shallow "^2.0.1" fragment-cache "^0.2.1" regex-not "^1.0.0" snapdragon "^0.8.1" to-regex "^3.0.1" extsprintf@1.3.0: version "1.3.0" resolved "https://registry.yarnpkg.com/extsprintf/-/extsprintf-1.3.0.tgz#96918440e3041a7a414f8c52e3c574eb3c3e1e05" integrity sha1-lpGEQOMEGnpBT4xS48V06zw+HgU= extsprintf@^1.2.0: version "1.4.0" resolved "https://registry.yarnpkg.com/extsprintf/-/extsprintf-1.4.0.tgz#e2689f8f356fad62cca65a3a91c5df5f9551692f" integrity sha1-4mifjzVvrWLMplo6kcXfX5VRaS8= fast-deep-equal@^2.0.1: version "2.0.1" resolved "https://registry.yarnpkg.com/fast-deep-equal/-/fast-deep-equal-2.0.1.tgz#7b05218ddf9667bf7f370bf7fdb2cb15fdd0aa49" integrity sha1-ewUhjd+WZ79/Nwv3/bLLFf3Qqkk= fast-diff@^1.1.2: version "1.2.0" resolved "https://registry.yarnpkg.com/fast-diff/-/fast-diff-1.2.0.tgz#73ee11982d86caaf7959828d519cfe927fac5f03" integrity sha512-xJuoT5+L99XlZ8twedaRf6Ax2TgQVxvgZOYoPKqZufmJib0tL2tegPBOZb1pVNgIhlqDlA0eO0c3wBvQcmzx4w== fast-json-stable-stringify@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/fast-json-stable-stringify/-/fast-json-stable-stringify-2.0.0.tgz#d5142c0caee6b1189f87d3a76111064f86c8bbf2" integrity sha1-1RQsDK7msRifh9OnYREGT4bIu/I= fast-levenshtein@~2.0.4: version "2.0.6" resolved "https://registry.yarnpkg.com/fast-levenshtein/-/fast-levenshtein-2.0.6.tgz#3d8a5c66883a16a30ca8643e851f19baa7797917" integrity sha1-PYpcZog6FqMMqGQ+hR8Zuqd5eRc= figures@^1.7.0: version "1.7.0" resolved "https://registry.yarnpkg.com/figures/-/figures-1.7.0.tgz#cbe1e3affcf1cd44b80cadfed28dc793a9701d2e" integrity sha1-y+Hjr/zxzUS4DK3+0o3Hk6lwHS4= dependencies: escape-string-regexp "^1.0.5" object-assign "^4.1.0" figures@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/figures/-/figures-2.0.0.tgz#3ab1a2d2a62c8bfb431a0c94cb797a2fce27c962" integrity sha1-OrGi0qYsi/tDGgyUy3l6L84nyWI= dependencies: escape-string-regexp "^1.0.5" file-entry-cache@^5.0.1: version "5.0.1" resolved "https://registry.yarnpkg.com/file-entry-cache/-/file-entry-cache-5.0.1.tgz#ca0f6efa6dd3d561333fb14515065c2fafdf439c" integrity sha512-bCg29ictuBaKUwwArK4ouCaqDgLZcysCFLmM/Yn/FDoqndh/9vNuQfXRDvTuXKLxfD/JtZQGKFT8MGcJBK644g== dependencies: flat-cache "^2.0.1" fill-range@^4.0.0: version "4.0.0" resolved "https://registry.yarnpkg.com/fill-range/-/fill-range-4.0.0.tgz#d544811d428f98eb06a63dc402d2403c328c38f7" integrity sha1-1USBHUKPmOsGpj3EAtJAPDKMOPc= dependencies: extend-shallow "^2.0.1" is-number "^3.0.0" repeat-string "^1.6.1" to-regex-range "^2.1.0" find-cache-dir@^2.1.0: version "2.1.0" resolved "https://registry.yarnpkg.com/find-cache-dir/-/find-cache-dir-2.1.0.tgz#8d0f94cd13fe43c6c7c261a0d86115ca918c05f7" integrity sha512-Tq6PixE0w/VMFfCgbONnkiQIVol/JJL7nRMi20fqzA4NRs9AfeqMGeRdPi3wIhYkxjeBaWh2rxwapn5Tu3IqOQ== dependencies: commondir "^1.0.1" make-dir "^2.0.0" pkg-dir "^3.0.0" find-parent-dir@^0.3.0: version "0.3.0" resolved "https://registry.yarnpkg.com/find-parent-dir/-/find-parent-dir-0.3.0.tgz#33c44b429ab2b2f0646299c5f9f718f376ff8d54" integrity sha1-M8RLQpqysvBkYpnF+fcY83b/jVQ= find-up@^1.0.0: version "1.1.2" resolved "https://registry.yarnpkg.com/find-up/-/find-up-1.1.2.tgz#6b2e9822b1a2ce0a60ab64d610eccad53cb24d0f" integrity sha1-ay6YIrGizgpgq2TWEOzK1TyyTQ8= dependencies: path-exists "^2.0.0" pinkie-promise "^2.0.0" find-up@^2.0.0, find-up@^2.1.0: version "2.1.0" resolved "https://registry.yarnpkg.com/find-up/-/find-up-2.1.0.tgz#45d1b7e506c717ddd482775a2b77920a3c0c57a7" integrity sha1-RdG35QbHF93UgndaK3eSCjwMV6c= dependencies: locate-path "^2.0.0" find-up@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/find-up/-/find-up-3.0.0.tgz#49169f1d7993430646da61ecc5ae355c21c97b73" integrity sha512-1yD6RmLI1XBfxugvORwlck6f75tYL+iR0jqwsOrOxMZyGYqUuDhJ0l4AXdO1iX/FTs9cBAMEk1gWSEx1kSbylg== dependencies: locate-path "^3.0.0" findit@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/findit/-/findit-2.0.0.tgz#6509f0126af4c178551cfa99394e032e13a4d56e" integrity sha1-ZQnwEmr0wXhVHPqZOU4DLhOk1W4= flat-cache@^2.0.1: version "2.0.1" resolved "https://registry.yarnpkg.com/flat-cache/-/flat-cache-2.0.1.tgz#5d296d6f04bda44a4630a301413bdbc2ec085ec0" integrity sha512-LoQe6yDuUMDzQAEH8sgmh4Md6oZnc/7PjtwjNFSzveXqSHt6ka9fPBuso7IGf9Rz4uqnSnWiFH2B/zj24a5ReA== dependencies: flatted "^2.0.0" rimraf "2.6.3" write "1.0.3" flatted@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/flatted/-/flatted-2.0.0.tgz#55122b6536ea496b4b44893ee2608141d10d9916" integrity sha512-R+H8IZclI8AAkSBRQJLVOsxwAoHd6WC40b4QTNWIjzAa6BXOBfQcM587MXDTVPeYaopFNWHUFLx7eNmHDSxMWg== fn-name@~2.0.1: version "2.0.1" resolved "https://registry.yarnpkg.com/fn-name/-/fn-name-2.0.1.tgz#5214d7537a4d06a4a301c0cc262feb84188002e7" integrity sha1-UhTXU3pNBqSjAcDMJi/rhBiAAuc= for-in@^1.0.2: version "1.0.2" resolved "https://registry.yarnpkg.com/for-in/-/for-in-1.0.2.tgz#81068d295a8142ec0ac726c6e2200c30fb6d5e80" integrity sha1-gQaNKVqBQuwKxybG4iAMMPttXoA= foreground-child@^1.3.3, foreground-child@^1.5.6: version "1.5.6" resolved "https://registry.yarnpkg.com/foreground-child/-/foreground-child-1.5.6.tgz#4fd71ad2dfde96789b980a5c0a295937cb2f5ce9" integrity sha1-T9ca0t/elnibmApcCilZN8svXOk= dependencies: cross-spawn "^4" signal-exit "^3.0.0" forever-agent@~0.6.1: version "0.6.1" resolved "https://registry.yarnpkg.com/forever-agent/-/forever-agent-0.6.1.tgz#fbc71f0c41adeb37f96c577ad1ed42d8fdacca91" integrity sha1-+8cfDEGt6zf5bFd60e1C2P2sypE= form-data@~2.3.2: version "2.3.3" resolved "https://registry.yarnpkg.com/form-data/-/form-data-2.3.3.tgz#dcce52c05f644f298c6a7ab936bd724ceffbf3a6" integrity sha512-1lLKB2Mu3aGP1Q/2eCOx0fNbRMe7XdwktwOruhfqqd0rIJWwN4Dh+E3hrPSlDCXnSR7UtZ1N38rVXm+6+MEhJQ== dependencies: asynckit "^0.4.0" combined-stream "^1.0.6" mime-types "^2.1.12" fragment-cache@^0.2.1: version "0.2.1" resolved "https://registry.yarnpkg.com/fragment-cache/-/fragment-cache-0.2.1.tgz#4290fad27f13e89be7f33799c6bc5a0abfff0d19" integrity sha1-QpD60n8T6Jvn8zeZxrxaCr//DRk= dependencies: map-cache "^0.2.2" fs-exists-cached@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/fs-exists-cached/-/fs-exists-cached-1.0.0.tgz#cf25554ca050dc49ae6656b41de42258989dcbce" integrity sha1-zyVVTKBQ3EmuZla0HeQiWJidy84= fs-minipass@^1.2.5: version "1.2.5" resolved "https://registry.yarnpkg.com/fs-minipass/-/fs-minipass-1.2.5.tgz#06c277218454ec288df77ada54a03b8702aacb9d" integrity sha512-JhBl0skXjUPCFH7x6x61gQxrKyXsxB5gcgePLZCwfyCGGsTISMoIeObbrvVeP6Xmyaudw4TT43qV2Gz+iyd2oQ== dependencies: minipass "^2.2.1" fs-readdir-recursive@^1.1.0: version "1.1.0" resolved "https://registry.yarnpkg.com/fs-readdir-recursive/-/fs-readdir-recursive-1.1.0.tgz#e32fc030a2ccee44a6b5371308da54be0b397d27" integrity sha512-GNanXlVr2pf02+sPN40XN8HG+ePaNcvM0q5mZBd668Obwb0yD5GiUbZOFgwn8kGMY6I3mdyDJzieUy3PTYyTRA== fs.realpath@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/fs.realpath/-/fs.realpath-1.0.0.tgz#1504ad2523158caa40db4a2787cb01411994ea4f" integrity sha1-FQStJSMVjKpA20onh8sBQRmU6k8= fsevents@^1.2.7: version "1.2.9" resolved "https://registry.yarnpkg.com/fsevents/-/fsevents-1.2.9.tgz#3f5ed66583ccd6f400b5a00db6f7e861363e388f" integrity sha512-oeyj2H3EjjonWcFjD5NvZNE9Rqe4UW+nQBU2HNeKw0koVLEFIhtyETyAakeAM3de7Z/SW5kcA+fZUait9EApnw== dependencies: nan "^2.12.1" node-pre-gyp "^0.12.0" function-bind@^1.1.1: version "1.1.1" resolved "https://registry.yarnpkg.com/function-bind/-/function-bind-1.1.1.tgz#a56899d3ea3c9bab874bb9773b7c5ede92f4895d" integrity sha512-yIovAzMX49sF8Yl58fSCWJ5svSLuaibPxXQJFLmBObTuCr0Mf1KiPopGM9NiFjiYBCbfaa2Fh6breQ6ANVTI0A== function-loop@^1.0.2: version "1.0.2" resolved "https://registry.yarnpkg.com/function-loop/-/function-loop-1.0.2.tgz#16b93dd757845eacfeca1a8061a6a65c106e0cb2" integrity sha512-Iw4MzMfS3udk/rqxTiDDCllhGwlOrsr50zViTOO/W6lS/9y6B1J0BD2VZzrnWUYBJsl3aeqjgR5v7bWWhZSYbA== functional-red-black-tree@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/functional-red-black-tree/-/functional-red-black-tree-1.0.1.tgz#1b0ab3bd553b2a0d6399d29c0e3ea0b252078327" integrity sha1-GwqzvVU7Kg1jmdKcDj6gslIHgyc= g-status@^2.0.2: version "2.0.2" resolved "https://registry.yarnpkg.com/g-status/-/g-status-2.0.2.tgz#270fd32119e8fc9496f066fe5fe88e0a6bc78b97" integrity sha512-kQoE9qH+T1AHKgSSD0Hkv98bobE90ILQcXAF4wvGgsr7uFqNvwmh8j+Lq3l0RVt3E3HjSbv2B9biEGcEtpHLCA== dependencies: arrify "^1.0.1" matcher "^1.0.0" simple-git "^1.85.0" gauge@~2.7.3: version "2.7.4" resolved "https://registry.yarnpkg.com/gauge/-/gauge-2.7.4.tgz#2c03405c7538c39d7eb37b317022e325fb018bf7" integrity sha1-LANAXHU4w51+s3sxcCLjJfsBi/c= dependencies: aproba "^1.0.3" console-control-strings "^1.0.0" has-unicode "^2.0.0" object-assign "^4.1.0" signal-exit "^3.0.0" string-width "^1.0.1" strip-ansi "^3.0.1" wide-align "^1.1.0" get-caller-file@^2.0.1: version "2.0.5" resolved "https://registry.yarnpkg.com/get-caller-file/-/get-caller-file-2.0.5.tgz#4f94412a82db32f36e3b0b9741f8a97feb031f7e" integrity sha512-DyFP3BM/3YHTQOCUL/w0OZHR0lpKeGrxotcHWcqNEdnltqFwXVfhEBQ94eIo34AfQpo0rGki4cyIiftY06h2Fg== get-own-enumerable-property-symbols@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/get-own-enumerable-property-symbols/-/get-own-enumerable-property-symbols-3.0.0.tgz#b877b49a5c16aefac3655f2ed2ea5b684df8d203" integrity sha512-CIJYJC4GGF06TakLg8z4GQKvDsx9EMspVxOYih7LerEL/WosUnFIww45CGfxfeKHqlg3twgUrYRT1O3WQqjGCg== get-stdin@^6.0.0: version "6.0.0" resolved "https://registry.yarnpkg.com/get-stdin/-/get-stdin-6.0.0.tgz#9e09bf712b360ab9225e812048f71fde9c89657b" integrity sha512-jp4tHawyV7+fkkSKyvjuLZswblUtz+SQKzSWnBbii16BuZksJlU1wuBYXY75r+duh/llF1ur6oNwi+2ZzjKZ7g== get-stdin@^7.0.0: version "7.0.0" resolved "https://registry.yarnpkg.com/get-stdin/-/get-stdin-7.0.0.tgz#8d5de98f15171a125c5e516643c7a6d0ea8a96f6" integrity sha512-zRKcywvrXlXsA0v0i9Io4KDRaAw7+a1ZpjRwl9Wox8PFlVCCHra7E9c4kqXCoCM9nR5tBkaTTZRBoCm60bFqTQ== get-stream@^4.0.0: version "4.1.0" resolved "https://registry.yarnpkg.com/get-stream/-/get-stream-4.1.0.tgz#c1b255575f3dc21d59bfc79cd3d2b46b1c3a54b5" integrity sha512-GMat4EJ5161kIy2HevLlr4luNjBgvmj413KaQA7jt4V8B4RDsfpHk7WQ9GVqfYyyx8OS/L66Kox+rJRNklLK7w== dependencies: pump "^3.0.0" get-value@^2.0.3, get-value@^2.0.6: version "2.0.6" resolved "https://registry.yarnpkg.com/get-value/-/get-value-2.0.6.tgz#dc15ca1c672387ca76bd37ac0a395ba2042a2c28" integrity sha1-3BXKHGcjh8p2vTesCjlbogQqLCg= getpass@^0.1.1: version "0.1.7" resolved "https://registry.yarnpkg.com/getpass/-/getpass-0.1.7.tgz#5eff8e3e684d569ae4cb2b1282604e8ba62149fa" integrity sha1-Xv+OPmhNVprkyysSgmBOi6YhSfo= dependencies: assert-plus "^1.0.0" glob-parent@^3.1.0: version "3.1.0" resolved "https://registry.yarnpkg.com/glob-parent/-/glob-parent-3.1.0.tgz#9e6af6299d8d3bd2bd40430832bd113df906c5ae" integrity sha1-nmr2KZ2NO9K9QEMIMr0RPfkGxa4= dependencies: is-glob "^3.1.0" path-dirname "^1.0.0" glob@^7.0.0, glob@^7.0.3, glob@^7.0.5, glob@^7.1.2, glob@^7.1.3: version "7.1.3" resolved "https://registry.yarnpkg.com/glob/-/glob-7.1.3.tgz#3960832d3f1574108342dafd3a67b332c0969df1" integrity sha512-vcfuiIxogLV4DlGBHIUOwI0IbrJ8HWPc4MU7HzviGeNho/UJDfi6B5p3sHeWIQ0KGIU0Jpxi5ZHxemQfLkkAwQ== dependencies: fs.realpath "^1.0.0" inflight "^1.0.4" inherits "2" minimatch "^3.0.4" once "^1.3.0" path-is-absolute "^1.0.0" globals@^11.1.0, globals@^11.7.0: version "11.10.0" resolved "https://registry.yarnpkg.com/globals/-/globals-11.10.0.tgz#1e09776dffda5e01816b3bb4077c8b59c24eaa50" integrity sha512-0GZF1RiPKU97IHUO5TORo9w1PwrH/NBPl+fS7oMLdaTRiYmYbwK4NWoZWrAdd0/abG9R2BU+OiwyQpTpE6pdfQ== globals@^9.18.0: version "9.18.0" resolved "https://registry.yarnpkg.com/globals/-/globals-9.18.0.tgz#aa3896b3e69b487f17e31ed2143d69a8e30c2d8a" integrity sha512-S0nG3CLEQiY/ILxqtztTWH/3iRRdyBLw6KMDxnKMchrtbj2OFmehVh0WUCfW3DUrIgx/qFrJPICrq4Z4sTR9UQ== globby@^6.1.0: version "6.1.0" resolved "https://registry.yarnpkg.com/globby/-/globby-6.1.0.tgz#f5a6d70e8395e21c858fb0489d64df02424d506c" integrity sha1-9abXDoOV4hyFj7BInWTfAkJNUGw= dependencies: array-union "^1.0.1" glob "^7.0.3" object-assign "^4.0.1" pify "^2.0.0" pinkie-promise "^2.0.0" graceful-fs@^4.1.11, graceful-fs@^4.1.15, graceful-fs@^4.1.2: version "4.1.15" resolved "https://registry.yarnpkg.com/graceful-fs/-/graceful-fs-4.1.15.tgz#ffb703e1066e8a0eeaa4c8b80ba9253eeefbfb00" integrity sha512-6uHUhOPEBgQ24HM+r6b/QwWfZq+yiFcipKFrOFiBEnWdy5sdzYoi+pJeQaPI5qOLRFqWmAXUPQNsielzdLoecA== "growl@~> 1.10.0": version "1.10.5" resolved "https://registry.yarnpkg.com/growl/-/growl-1.10.5.tgz#f2735dc2283674fa67478b10181059355c369e5e" integrity sha512-qBr4OuELkhPenW6goKVXiv47US3clb3/IbuWF9KNKEijAy9oeHxU9IgzjvJhHkUzhaj7rOUD7+YGWqUjLp5oSA== handlebars@^4.1.2: version "4.1.2" resolved "https://registry.yarnpkg.com/handlebars/-/handlebars-4.1.2.tgz#b6b37c1ced0306b221e094fc7aca3ec23b131b67" integrity sha512-nvfrjqvt9xQ8Z/w0ijewdD/vvWDTOweBUm96NTr66Wfvo1mJenBLwcYmPs3TIBP5ruzYGD7Hx/DaM9RmhroGPw== dependencies: neo-async "^2.6.0" optimist "^0.6.1" source-map "^0.6.1" optionalDependencies: uglify-js "^3.1.4" har-schema@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/har-schema/-/har-schema-2.0.0.tgz#a94c2224ebcac04782a0d9035521f24735b7ec92" integrity sha1-qUwiJOvKwEeCoNkDVSHyRzW37JI= har-validator@~5.1.0: version "5.1.3" resolved "https://registry.yarnpkg.com/har-validator/-/har-validator-5.1.3.tgz#1ef89ebd3e4996557675eed9893110dc350fa080" integrity sha512-sNvOCzEQNr/qrvJgc3UG/kD4QtlHycrzwS+6mfTrrSq97BvaYcPZZI1ZSqGSPR73Cxn4LKTD4PttRwfU7jWq5g== dependencies: ajv "^6.5.5" har-schema "^2.0.0" has-ansi@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/has-ansi/-/has-ansi-2.0.0.tgz#34f5049ce1ecdf2b0649af3ef24e45ed35416d91" integrity sha1-NPUEnOHs3ysGSa8+8k5F7TVBbZE= dependencies: ansi-regex "^2.0.0" has-flag@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/has-flag/-/has-flag-3.0.0.tgz#b5d454dc2199ae225699f3467e5a07f3b955bafd" integrity sha1-tdRU3CGZriJWmfNGfloH87lVuv0= has-symbols@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/has-symbols/-/has-symbols-1.0.0.tgz#ba1a8f1af2a0fc39650f5c850367704122063b44" integrity sha1-uhqPGvKg/DllD1yFA2dwQSIGO0Q= has-unicode@^2.0.0: version "2.0.1" resolved "https://registry.yarnpkg.com/has-unicode/-/has-unicode-2.0.1.tgz#e0e6fe6a28cf51138855e086d1691e771de2a8b9" integrity sha1-4Ob+aijPUROIVeCG0Wkedx3iqLk= has-value@^0.3.1: version "0.3.1" resolved "https://registry.yarnpkg.com/has-value/-/has-value-0.3.1.tgz#7b1f58bada62ca827ec0a2078025654845995e1f" integrity sha1-ex9YutpiyoJ+wKIHgCVlSEWZXh8= dependencies: get-value "^2.0.3" has-values "^0.1.4" isobject "^2.0.0" has-value@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/has-value/-/has-value-1.0.0.tgz#18b281da585b1c5c51def24c930ed29a0be6b177" integrity sha1-GLKB2lhbHFxR3vJMkw7SmgvmsXc= dependencies: get-value "^2.0.6" has-values "^1.0.0" isobject "^3.0.0" has-values@^0.1.4: version "0.1.4" resolved "https://registry.yarnpkg.com/has-values/-/has-values-0.1.4.tgz#6d61de95d91dfca9b9a02089ad384bff8f62b771" integrity sha1-bWHeldkd/Km5oCCJrThL/49it3E= has-values@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/has-values/-/has-values-1.0.0.tgz#95b0b63fec2146619a6fe57fe75628d5a39efe4f" integrity sha1-lbC2P+whRmGab+V/51Yo1aOe/k8= dependencies: is-number "^3.0.0" kind-of "^4.0.0" has@^1.0.1, has@^1.0.3: version "1.0.3" resolved "https://registry.yarnpkg.com/has/-/has-1.0.3.tgz#722d7cbfc1f6aa8241f16dd814e011e1f41e8796" integrity sha512-f2dvO0VU6Oej7RkWJGrehjbzMAjFp5/VKPp5tTpWIV4JHHZK1/BxbFRtf/siA2SWTe09caDmVtYYzWEIbBS4zw== dependencies: function-bind "^1.1.1" hasha@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/hasha/-/hasha-3.0.0.tgz#52a32fab8569d41ca69a61ff1a214f8eb7c8bd39" integrity sha1-UqMvq4Vp1BymmmH/GiFPjrfIvTk= dependencies: is-stream "^1.0.1" home-or-tmp@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/home-or-tmp/-/home-or-tmp-2.0.0.tgz#e36c3f2d2cae7d746a857e38d18d5f32a7882db8" integrity sha1-42w/LSyufXRqhX440Y1fMqeILbg= dependencies: os-homedir "^1.0.0" os-tmpdir "^1.0.1" hosted-git-info@^2.1.4: version "2.7.1" resolved "https://registry.yarnpkg.com/hosted-git-info/-/hosted-git-info-2.7.1.tgz#97f236977bd6e125408930ff6de3eec6281ec047" integrity sha512-7T/BxH19zbcCTa8XkMlbK5lTo1WtgkFi3GvdWEyNuc4Vex7/9Dqbnpsf4JMydcfj9HCg4zUWFTL3Za6lapg5/w== http-signature@~1.2.0: version "1.2.0" resolved "https://registry.yarnpkg.com/http-signature/-/http-signature-1.2.0.tgz#9aecd925114772f3d95b65a60abb8f7c18fbace1" integrity sha1-muzZJRFHcvPZW2WmCruPfBj7rOE= dependencies: assert-plus "^1.0.0" jsprim "^1.2.2" sshpk "^1.7.0" husky@^2.2.0: version "2.2.0" resolved "https://registry.yarnpkg.com/husky/-/husky-2.2.0.tgz#4dda4370ba0f145b6594be4a4e4e4d4c82a6f2d5" integrity sha512-lG33E7zq6v//H/DQIojPEi1ZL9ebPFt3MxUMD8MR0lrS2ljEPiuUUxlziKIs/o9EafF0chL7bAtLQkcPvXmdnA== dependencies: cosmiconfig "^5.2.0" execa "^1.0.0" find-up "^3.0.0" get-stdin "^7.0.0" is-ci "^2.0.0" pkg-dir "^4.1.0" please-upgrade-node "^3.1.1" read-pkg "^5.0.0" run-node "^1.0.0" slash "^2.0.0" iconv-lite@^0.4.24, iconv-lite@^0.4.4: version "0.4.24" resolved "https://registry.yarnpkg.com/iconv-lite/-/iconv-lite-0.4.24.tgz#2022b4b25fbddc21d2f524974a474aafe733908b" integrity sha512-v3MXnZAcvnywkTUEZomIActle7RXXeedOR31wwl7VlyoXO4Qi9arvSenNQWne1TcRwhCL1HwLI21bEqdpj8/rA== dependencies: safer-buffer ">= 2.1.2 < 3" if-ver@^1.1.0: version "1.1.0" resolved "https://registry.yarnpkg.com/if-ver/-/if-ver-1.1.0.tgz#41814b32354042807415e9476203f99d7083b2ff" integrity sha512-hzYfMiy0CA/vNTv2wmpJDLrhZa4r5LOIHQXsPKQloguXsTd1obWf2iSOuD/xDyTa0QQGTFQ/hAWOtJGML/uECA== ignore-walk@^3.0.1: version "3.0.1" resolved "https://registry.yarnpkg.com/ignore-walk/-/ignore-walk-3.0.1.tgz#a83e62e7d272ac0e3b551aaa82831a19b69f82f8" integrity sha512-DTVlMx3IYPe0/JJcYP7Gxg7ttZZu3IInhuEhbchuqneY9wWe5Ojy2mXLBaQFUQmo0AW2r3qG7m1mg86js+gnlQ== dependencies: minimatch "^3.0.4" ignore@^4.0.6: version "4.0.6" resolved "https://registry.yarnpkg.com/ignore/-/ignore-4.0.6.tgz#750e3db5862087b4737ebac8207ffd1ef27b25fc" integrity sha512-cyFDKrqc/YdcWFniJhzI42+AzS+gNwmUzOSFcRCQYwySuBBBy/KjuxWLZ/FHEH6Moq1NizMOBWyTcv8O4OZIMg== ignore@^5.1.1: version "5.1.1" resolved "https://registry.yarnpkg.com/ignore/-/ignore-5.1.1.tgz#2fc6b8f518aff48fef65a7f348ed85632448e4a5" integrity sha512-DWjnQIFLenVrwyRCKZT+7a7/U4Cqgar4WG8V++K3hw+lrW1hc/SIwdiGmtxKCVACmHULTuGeBbHJmbwW7/sAvA== import-fresh@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/import-fresh/-/import-fresh-2.0.0.tgz#d81355c15612d386c61f9ddd3922d4304822a546" integrity sha1-2BNVwVYS04bGH53dOSLUMEgipUY= dependencies: caller-path "^2.0.0" resolve-from "^3.0.0" import-fresh@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/import-fresh/-/import-fresh-3.0.0.tgz#a3d897f420cab0e671236897f75bc14b4885c390" integrity sha512-pOnA9tfM3Uwics+SaBLCNyZZZbK+4PTu0OPZtLlMIrv17EdBoC15S9Kn8ckJ9TZTyKb3ywNE5y1yeDxxGA7nTQ== dependencies: parent-module "^1.0.0" resolve-from "^4.0.0" import-jsx@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/import-jsx/-/import-jsx-2.0.0.tgz#5ec3283f75a38c154714586c2aae72403eb216de" integrity sha512-xmrgtiRnAdjIaRzKwsHut54FA8nx59WqN4MpQvPFr/8yD6BamavkmKHrA5dotAlnIiF4uqMzg/lA5yhPdpIXsA== dependencies: babel-core "^6.25.0" babel-plugin-transform-es2015-destructuring "^6.23.0" babel-plugin-transform-object-rest-spread "^6.23.0" babel-plugin-transform-react-jsx "^6.24.1" caller-path "^2.0.0" resolve-from "^3.0.0" imurmurhash@^0.1.4: version "0.1.4" resolved "https://registry.yarnpkg.com/imurmurhash/-/imurmurhash-0.1.4.tgz#9218b9b2b928a238b13dc4fb6b6d576f231453ea" integrity sha1-khi5srkoojixPcT7a21XbyMUU+o= indent-string@^3.0.0: version "3.2.0" resolved "https://registry.yarnpkg.com/indent-string/-/indent-string-3.2.0.tgz#4a5fd6d27cc332f37e5419a504dbb837105c9289" integrity sha1-Sl/W0nzDMvN+VBmlBNu4NxBckok= inflight@^1.0.4: version "1.0.6" resolved "https://registry.yarnpkg.com/inflight/-/inflight-1.0.6.tgz#49bd6331d7d02d0c09bc910a1075ba8165b56df9" integrity sha1-Sb1jMdfQLQwJvJEKEHW6gWW1bfk= dependencies: once "^1.3.0" wrappy "1" inherits@2, inherits@^2.0.3, inherits@~2.0.3: version "2.0.3" resolved "https://registry.yarnpkg.com/inherits/-/inherits-2.0.3.tgz#633c2c83e3da42a502f52466022480f4208261de" integrity sha1-Yzwsg+PaQqUC9SRmAiSA9CCCYd4= ini@~1.3.0: version "1.3.5" resolved "https://registry.yarnpkg.com/ini/-/ini-1.3.5.tgz#eee25f56db1c9ec6085e0c22778083f596abf927" integrity sha512-RZY5huIKCMRWDUqZlEi72f/lmXKMvuszcMBduliQ3nnWbx9X/ZBQO7DijMEYS9EhHBb2qacRUMtC7svLwe0lcw== ink@^2.1.1: version "2.1.1" resolved "https://registry.yarnpkg.com/ink/-/ink-2.1.1.tgz#efb2adbd30be79b4f640d7c67b524bfb030205ba" integrity sha512-vP1yE/uJoiY6uB9yHalczUA02I9fg7xDUbTEZitPK5y6dvnPo9a/6UWqIB2uCYkHOhEZMN+D/TsVr4v2sz8qYA== dependencies: "@types/react" "^16.8.6" arrify "^1.0.1" auto-bind "^2.0.0" chalk "^2.4.1" cli-cursor "^2.1.0" cli-truncate "^1.1.0" is-ci "^2.0.0" lodash.throttle "^4.1.1" log-update "^3.0.0" prop-types "^15.6.2" react-reconciler "^0.20.0" scheduler "^0.13.2" signal-exit "^3.0.2" slice-ansi "^1.0.0" string-length "^2.0.0" widest-line "^2.0.0" wrap-ansi "^5.0.0" yoga-layout-prebuilt "^1.9.3" inquirer@^6.2.2: version "6.2.2" resolved "https://registry.yarnpkg.com/inquirer/-/inquirer-6.2.2.tgz#46941176f65c9eb20804627149b743a218f25406" integrity sha512-Z2rREiXA6cHRR9KBOarR3WuLlFzlIfAEIiB45ll5SSadMg7WqOh1MKEjjndfuH5ewXdixWCxqnVfGOQzPeiztA== dependencies: ansi-escapes "^3.2.0" chalk "^2.4.2" cli-cursor "^2.1.0" cli-width "^2.0.0" external-editor "^3.0.3" figures "^2.0.0" lodash "^4.17.11" mute-stream "0.0.7" run-async "^2.2.0" rxjs "^6.4.0" string-width "^2.1.0" strip-ansi "^5.0.0" through "^2.3.6" invariant@^2.2.2: version "2.2.4" resolved "https://registry.yarnpkg.com/invariant/-/invariant-2.2.4.tgz#610f3c92c9359ce1db616e538008d23ff35158e6" integrity sha512-phJfQVBuaJM5raOpJjSfkiD6BpbCE4Ns//LaXl6wGYtUBY83nWS6Rf9tXm2e8VaK60JEjYldbPif/A2B1C2gNA== dependencies: loose-envify "^1.0.0" invert-kv@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/invert-kv/-/invert-kv-2.0.0.tgz#7393f5afa59ec9ff5f67a27620d11c226e3eec02" integrity sha512-wPVv/y/QQ/Uiirj/vh3oP+1Ww+AWehmi1g5fFWGPF6IpCBCDVrhgHRMvrLfdYcwDh3QJbGXDW4JAuzxElLSqKA== is-accessor-descriptor@^0.1.6: version "0.1.6" resolved "https://registry.yarnpkg.com/is-accessor-descriptor/-/is-accessor-descriptor-0.1.6.tgz#a9e12cb3ae8d876727eeef3843f8a0897b5c98d6" integrity sha1-qeEss66Nh2cn7u84Q/igiXtcmNY= dependencies: kind-of "^3.0.2" is-accessor-descriptor@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/is-accessor-descriptor/-/is-accessor-descriptor-1.0.0.tgz#169c2f6d3df1f992618072365c9b0ea1f6878656" integrity sha512-m5hnHTkcVsPfqx3AKlyttIPb7J+XykHvJP2B9bZDjlhLIoEq4XoK64Vg7boZlVWYK6LUY94dYPEE7Lh0ZkZKcQ== dependencies: kind-of "^6.0.0" is-arrayish@^0.2.1: version "0.2.1" resolved "https://registry.yarnpkg.com/is-arrayish/-/is-arrayish-0.2.1.tgz#77c99840527aa8ecb1a8ba697b80645a7a926a9d" integrity sha1-d8mYQFJ6qOyxqLppe4BkWnqSap0= is-binary-path@^1.0.0: version "1.0.1" resolved "https://registry.yarnpkg.com/is-binary-path/-/is-binary-path-1.0.1.tgz#75f16642b480f187a711c814161fd3a4a7655898" integrity sha1-dfFmQrSA8YenEcgUFh/TpKdlWJg= dependencies: binary-extensions "^1.0.0" is-buffer@^1.1.5: version "1.1.6" resolved "https://registry.yarnpkg.com/is-buffer/-/is-buffer-1.1.6.tgz#efaa2ea9daa0d7ab2ea13a97b2b8ad51fefbe8be" integrity sha512-NcdALwpXkTm5Zvvbk7owOUSvVvBKDgKP5/ewfXEznmQFfs4ZRmanOeKBTjRVjka3QFoN6XJ+9F3USqfHqTaU5w== is-builtin-module@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/is-builtin-module/-/is-builtin-module-1.0.0.tgz#540572d34f7ac3119f8f76c30cbc1b1e037affbe" integrity sha1-VAVy0096wxGfj3bDDLwbHgN6/74= dependencies: builtin-modules "^1.0.0" is-callable@^1.1.4: version "1.1.4" resolved "https://registry.yarnpkg.com/is-callable/-/is-callable-1.1.4.tgz#1e1adf219e1eeb684d691f9d6a05ff0d30a24d75" integrity sha512-r5p9sxJjYnArLjObpjA4xu5EKI3CuKHkJXMhT7kwbpUyIFD1n5PMAsoPvWnvtZiNz7LjkYDRZhd7FlI0eMijEA== is-ci@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/is-ci/-/is-ci-2.0.0.tgz#6bc6334181810e04b5c22b3d589fdca55026404c" integrity sha512-YfJT7rkpQB0updsdHLGWrvhBJfcfzNNawYDNIyQXJz0IViGf75O8EBPKSdvw2rF+LGCsX4FZ8tcr3b19LcZq4w== dependencies: ci-info "^2.0.0" is-data-descriptor@^0.1.4: version "0.1.4" resolved "https://registry.yarnpkg.com/is-data-descriptor/-/is-data-descriptor-0.1.4.tgz#0b5ee648388e2c860282e793f1856fec3f301b56" integrity sha1-C17mSDiOLIYCgueT8YVv7D8wG1Y= dependencies: kind-of "^3.0.2" is-data-descriptor@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/is-data-descriptor/-/is-data-descriptor-1.0.0.tgz#d84876321d0e7add03990406abbbbd36ba9268c7" integrity sha512-jbRXy1FmtAoCjQkVmIVYwuuqDFUbaOeDjmed1tOGPrsMhtJA4rD9tkgA0F1qJ3gRFRXcHYVkdeaP50Q5rE/jLQ== dependencies: kind-of "^6.0.0" is-date-object@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/is-date-object/-/is-date-object-1.0.1.tgz#9aa20eb6aeebbff77fbd33e74ca01b33581d3a16" integrity sha1-mqIOtq7rv/d/vTPnTKAbM1gdOhY= is-descriptor@^0.1.0: version "0.1.6" resolved "https://registry.yarnpkg.com/is-descriptor/-/is-descriptor-0.1.6.tgz#366d8240dde487ca51823b1ab9f07a10a78251ca" integrity sha512-avDYr0SB3DwO9zsMov0gKCESFYqCnE4hq/4z3TdUlukEy5t9C0YRq7HLrsN52NAcqXKaepeCD0n+B0arnVG3Hg== dependencies: is-accessor-descriptor "^0.1.6" is-data-descriptor "^0.1.4" kind-of "^5.0.0" is-descriptor@^1.0.0, is-descriptor@^1.0.2: version "1.0.2" resolved "https://registry.yarnpkg.com/is-descriptor/-/is-descriptor-1.0.2.tgz#3b159746a66604b04f8c81524ba365c5f14d86ec" integrity sha512-2eis5WqQGV7peooDyLmNEPUrps9+SXX5c9pL3xEB+4e9HnGuDa7mB7kHxHw4CbqS9k1T2hOH3miL8n8WtiYVtg== dependencies: is-accessor-descriptor "^1.0.0" is-data-descriptor "^1.0.0" kind-of "^6.0.2" is-directory@^0.3.1: version "0.3.1" resolved "https://registry.yarnpkg.com/is-directory/-/is-directory-0.3.1.tgz#61339b6f2475fc772fd9c9d83f5c8575dc154ae1" integrity sha1-YTObbyR1/Hcv2cnYP1yFddwVSuE= is-extendable@^0.1.0, is-extendable@^0.1.1: version "0.1.1" resolved "https://registry.yarnpkg.com/is-extendable/-/is-extendable-0.1.1.tgz#62b110e289a471418e3ec36a617d472e301dfc89" integrity sha1-YrEQ4omkcUGOPsNqYX1HLjAd/Ik= is-extendable@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/is-extendable/-/is-extendable-1.0.1.tgz#a7470f9e426733d81bd81e1155264e3a3507cab4" integrity sha512-arnXMxT1hhoKo9k1LZdmlNyJdDDfy2v0fXjFlmok4+i8ul/6WlbVge9bhM74OpNPQPMGUToDtz+KXa1PneJxOA== dependencies: is-plain-object "^2.0.4" is-extglob@^2.1.0, is-extglob@^2.1.1: version "2.1.1" resolved "https://registry.yarnpkg.com/is-extglob/-/is-extglob-2.1.1.tgz#a88c02535791f02ed37c76a1b9ea9773c833f8c2" integrity sha1-qIwCU1eR8C7TfHahueqXc8gz+MI= is-finite@^1.0.0: version "1.0.2" resolved "https://registry.yarnpkg.com/is-finite/-/is-finite-1.0.2.tgz#cc6677695602be550ef11e8b4aa6305342b6d0aa" integrity sha1-zGZ3aVYCvlUO8R6LSqYwU0K20Ko= dependencies: number-is-nan "^1.0.0" is-fullwidth-code-point@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/is-fullwidth-code-point/-/is-fullwidth-code-point-1.0.0.tgz#ef9e31386f031a7f0d643af82fde50c457ef00cb" integrity sha1-754xOG8DGn8NZDr4L95QxFfvAMs= dependencies: number-is-nan "^1.0.0" is-fullwidth-code-point@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/is-fullwidth-code-point/-/is-fullwidth-code-point-2.0.0.tgz#a3b30a5c4f199183167aaab93beefae3ddfb654f" integrity sha1-o7MKXE8ZkYMWeqq5O+764937ZU8= is-glob@^3.1.0: version "3.1.0" resolved "https://registry.yarnpkg.com/is-glob/-/is-glob-3.1.0.tgz#7ba5ae24217804ac70707b96922567486cc3e84a" integrity sha1-e6WuJCF4BKxwcHuWkiVnSGzD6Eo= dependencies: is-extglob "^2.1.0" is-glob@^4.0.0: version "4.0.0" resolved "https://registry.yarnpkg.com/is-glob/-/is-glob-4.0.0.tgz#9521c76845cc2610a85203ddf080a958c2ffabc0" integrity sha1-lSHHaEXMJhCoUgPd8ICpWML/q8A= dependencies: is-extglob "^2.1.1" is-number@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/is-number/-/is-number-3.0.0.tgz#24fd6201a4782cf50561c810276afc7d12d71195" integrity sha1-JP1iAaR4LPUFYcgQJ2r8fRLXEZU= dependencies: kind-of "^3.0.2" is-obj@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/is-obj/-/is-obj-1.0.1.tgz#3e4729ac1f5fde025cd7d83a896dab9f4f67db0f" integrity sha1-PkcprB9f3gJc19g6iW2rn09n2w8= is-observable@^1.1.0: version "1.1.0" resolved "https://registry.yarnpkg.com/is-observable/-/is-observable-1.1.0.tgz#b3e986c8f44de950867cab5403f5a3465005975e" integrity sha512-NqCa4Sa2d+u7BWc6CukaObG3Fh+CU9bvixbpcXYhy2VvYS7vVGIdAgnIS5Ks3A/cqk4rebLJ9s8zBstT2aKnIA== dependencies: symbol-observable "^1.1.0" is-path-cwd@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/is-path-cwd/-/is-path-cwd-1.0.0.tgz#d225ec23132e89edd38fda767472e62e65f1106d" integrity sha1-0iXsIxMuie3Tj9p2dHLmLmXxEG0= is-path-in-cwd@^1.0.0: version "1.0.1" resolved "https://registry.yarnpkg.com/is-path-in-cwd/-/is-path-in-cwd-1.0.1.tgz#5ac48b345ef675339bd6c7a48a912110b241cf52" integrity sha512-FjV1RTW48E7CWM7eE/J2NJvAEEVektecDBVBE5Hh3nM1Jd0kvhHtX68Pr3xsDf857xt3Y4AkwVULK1Vku62aaQ== dependencies: is-path-inside "^1.0.0" is-path-inside@^1.0.0: version "1.0.1" resolved "https://registry.yarnpkg.com/is-path-inside/-/is-path-inside-1.0.1.tgz#8ef5b7de50437a3fdca6b4e865ef7aa55cb48036" integrity sha1-jvW33lBDej/cprToZe96pVy0gDY= dependencies: path-is-inside "^1.0.1" is-plain-obj@^1.1.0: version "1.1.0" resolved "https://registry.yarnpkg.com/is-plain-obj/-/is-plain-obj-1.1.0.tgz#71a50c8429dfca773c92a390a4a03b39fcd51d3e" integrity sha1-caUMhCnfync8kqOQpKA7OfzVHT4= is-plain-object@^2.0.1, is-plain-object@^2.0.3, is-plain-object@^2.0.4: version "2.0.4" resolved "https://registry.yarnpkg.com/is-plain-object/-/is-plain-object-2.0.4.tgz#2c163b3fafb1b606d9d17928f05c2a1c38e07677" integrity sha512-h5PpgXkWitc38BBMYawTYMWJHFZJVnBquFE57xFpjB8pJFiF6gZ+bU+WyI/yqXiFR5mdLsgYNaPe8uao6Uv9Og== dependencies: isobject "^3.0.1" is-promise@^2.1.0: version "2.1.0" resolved "https://registry.yarnpkg.com/is-promise/-/is-promise-2.1.0.tgz#79a2a9ece7f096e80f36d2b2f3bc16c1ff4bf3fa" integrity sha1-eaKp7OfwlugPNtKy87wWwf9L8/o= is-regex@^1.0.4: version "1.0.4" resolved "https://registry.yarnpkg.com/is-regex/-/is-regex-1.0.4.tgz#5517489b547091b0930e095654ced25ee97e9491" integrity sha1-VRdIm1RwkbCTDglWVM7SXul+lJE= dependencies: has "^1.0.1" is-regexp@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/is-regexp/-/is-regexp-1.0.0.tgz#fd2d883545c46bac5a633e7b9a09e87fa2cb5069" integrity sha1-/S2INUXEa6xaYz57mgnof6LLUGk= is-stream@^1.0.1, is-stream@^1.1.0: version "1.1.0" resolved "https://registry.yarnpkg.com/is-stream/-/is-stream-1.1.0.tgz#12d4a3dd4e68e0b79ceb8dbc84173ae80d91ca44" integrity sha1-EtSj3U5o4Lec6428hBc66A2RykQ= is-symbol@^1.0.2: version "1.0.2" resolved "https://registry.yarnpkg.com/is-symbol/-/is-symbol-1.0.2.tgz#a055f6ae57192caee329e7a860118b497a950f38" integrity sha512-HS8bZ9ox60yCJLH9snBpIwv9pYUAkcuLhSA1oero1UB5y9aiQpRA8y2ex945AOtCZL1lJDeIk3G5LthswI46Lw== dependencies: has-symbols "^1.0.0" is-typedarray@~1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/is-typedarray/-/is-typedarray-1.0.0.tgz#e479c80858df0c1b11ddda6940f96011fcda4a9a" integrity sha1-5HnICFjfDBsR3dppQPlgEfzaSpo= is-windows@^1.0.2: version "1.0.2" resolved "https://registry.yarnpkg.com/is-windows/-/is-windows-1.0.2.tgz#d1850eb9791ecd18e6182ce12a30f396634bb19d" integrity sha512-eXK1UInq2bPmjyX6e3VHIzMLobc4J94i4AWn+Hpq3OU5KkrRC96OAcR3PRJ/pGu6m8TRnBHP9dkXQVsT/COVIA== isarray@1.0.0, isarray@^1.0.0, isarray@~1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/isarray/-/isarray-1.0.0.tgz#bb935d48582cba168c06834957a54a3e07124f11" integrity sha1-u5NdSFgsuhaMBoNJV6VKPgcSTxE= isexe@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/isexe/-/isexe-2.0.0.tgz#e8fbf374dc556ff8947a10dcb0572d633f2cfa10" integrity sha1-6PvzdNxVb/iUehDcsFctYz8s+hA= isobject@^2.0.0: version "2.1.0" resolved "https://registry.yarnpkg.com/isobject/-/isobject-2.1.0.tgz#f065561096a3f1da2ef46272f815c840d87e0c89" integrity sha1-8GVWEJaj8dou9GJy+BXIQNh+DIk= dependencies: isarray "1.0.0" isobject@^3.0.0, isobject@^3.0.1: version "3.0.1" resolved "https://registry.yarnpkg.com/isobject/-/isobject-3.0.1.tgz#4e431e92b11a9731636aa1f9c8d1ccbcfdab78df" integrity sha1-TkMekrEalzFjaqH5yNHMvP2reN8= isstream@~0.1.2: version "0.1.2" resolved "https://registry.yarnpkg.com/isstream/-/isstream-0.1.2.tgz#47e63f7af55afa6f92e1500e690eb8b8529c099a" integrity sha1-R+Y/evVa+m+S4VAOaQ64uFKcCZo= istanbul-lib-coverage@^2.0.3, istanbul-lib-coverage@^2.0.5: version "2.0.5" resolved "https://registry.yarnpkg.com/istanbul-lib-coverage/-/istanbul-lib-coverage-2.0.5.tgz#675f0ab69503fad4b1d849f736baaca803344f49" integrity sha512-8aXznuEPCJvGnMSRft4udDRDtb1V3pkQkMMI5LI+6HuQz5oQ4J2UFn1H82raA3qJtyOLkkwVqICBQkjnGtn5mA== istanbul-lib-hook@^2.0.7: version "2.0.7" resolved "https://registry.yarnpkg.com/istanbul-lib-hook/-/istanbul-lib-hook-2.0.7.tgz#c95695f383d4f8f60df1f04252a9550e15b5b133" integrity sha512-vrRztU9VRRFDyC+aklfLoeXyNdTfga2EI3udDGn4cZ6fpSXpHLV9X6CHvfoMCPtggg8zvDDmC4b9xfu0z6/llA== dependencies: append-transform "^1.0.0" istanbul-lib-instrument@^3.3.0: version "3.3.0" resolved "https://registry.yarnpkg.com/istanbul-lib-instrument/-/istanbul-lib-instrument-3.3.0.tgz#a5f63d91f0bbc0c3e479ef4c5de027335ec6d630" integrity sha512-5nnIN4vo5xQZHdXno/YDXJ0G+I3dAm4XgzfSVTPLQpj/zAV2dV6Juy0yaf10/zrJOJeHoN3fraFe+XRq2bFVZA== dependencies: "@babel/generator" "^7.4.0" "@babel/parser" "^7.4.3" "@babel/template" "^7.4.0" "@babel/traverse" "^7.4.3" "@babel/types" "^7.4.0" istanbul-lib-coverage "^2.0.5" semver "^6.0.0" istanbul-lib-processinfo@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/istanbul-lib-processinfo/-/istanbul-lib-processinfo-1.0.0.tgz#6c0b59411d2897313ea09165fd95464a32be5610" integrity sha512-FY0cPmWa4WoQNlvB8VOcafiRoB5nB+l2Pz2xGuXHRSy1KM8QFOYfz/rN+bGMCAeejrY3mrpF5oJHcN0s/garCg== dependencies: archy "^1.0.0" cross-spawn "^6.0.5" istanbul-lib-coverage "^2.0.3" rimraf "^2.6.3" uuid "^3.3.2" istanbul-lib-report@^2.0.8: version "2.0.8" resolved "https://registry.yarnpkg.com/istanbul-lib-report/-/istanbul-lib-report-2.0.8.tgz#5a8113cd746d43c4889eba36ab10e7d50c9b4f33" integrity sha512-fHBeG573EIihhAblwgxrSenp0Dby6tJMFR/HvlerBsrCTD5bkUuoNtn3gVh29ZCS824cGGBPn7Sg7cNk+2xUsQ== dependencies: istanbul-lib-coverage "^2.0.5" make-dir "^2.1.0" supports-color "^6.1.0" istanbul-lib-source-maps@^3.0.6: version "3.0.6" resolved "https://registry.yarnpkg.com/istanbul-lib-source-maps/-/istanbul-lib-source-maps-3.0.6.tgz#284997c48211752ec486253da97e3879defba8c8" integrity sha512-R47KzMtDJH6X4/YW9XTx+jrLnZnscW4VpNN+1PViSYTejLVPWv7oov+Duf8YQSPyVRUvueQqz1TcsC6mooZTXw== dependencies: debug "^4.1.1" istanbul-lib-coverage "^2.0.5" make-dir "^2.1.0" rimraf "^2.6.3" source-map "^0.6.1" istanbul-reports@^2.2.4: version "2.2.4" resolved "https://registry.yarnpkg.com/istanbul-reports/-/istanbul-reports-2.2.4.tgz#4e0d0ddf0f0ad5b49a314069d31b4f06afe49ad3" integrity sha512-QCHGyZEK0bfi9GR215QSm+NJwFKEShbtc7tfbUdLAEzn3kKhLDDZqvljn8rPZM9v8CEOhzL1nlYoO4r1ryl67w== dependencies: handlebars "^4.1.2" jackspeak@^1.3.7: version "1.3.7" resolved "https://registry.yarnpkg.com/jackspeak/-/jackspeak-1.3.7.tgz#840d50a33ddba0f122bc6c4efdf6dc966f5218a0" integrity sha512-Z4iSFpaCV7Cocpcl5t9/UyPkisxenbmaqminyTgK6lDDMXcm9EvIZ9Bwr/uFbGOjfWlz1UZwKwFY5AvtgNlHuw== dependencies: cliui "^4.1.0" js-levenshtein@^1.1.3: version "1.1.6" resolved "https://registry.yarnpkg.com/js-levenshtein/-/js-levenshtein-1.1.6.tgz#c6cee58eb3550372df8deb85fad5ce66ce01d59d" integrity sha512-X2BB11YZtrRqY4EnQcLX5Rh373zbK4alC1FW7D7MBhL2gtcC17cTnr6DmfHZeS0s2rTHjUTMMHfG7gO8SSdw+g== "js-tokens@^3.0.0 || ^4.0.0", js-tokens@^4.0.0: version "4.0.0" resolved "https://registry.yarnpkg.com/js-tokens/-/js-tokens-4.0.0.tgz#19203fb59991df98e3a287050d4647cdeaf32499" integrity sha512-RdJUflcE3cUzKiMqQgsCu06FPu9UdIJO0beYbPhHN4k6apgJtifcoCtT9bcxOpYBtpD2kCM6Sbzg4CausW/PKQ== js-tokens@^3.0.2: version "3.0.2" resolved "https://registry.yarnpkg.com/js-tokens/-/js-tokens-3.0.2.tgz#9866df395102130e38f7f996bceb65443209c25b" integrity sha1-mGbfOVECEw449/mWvOtlRDIJwls= js-yaml@^3.11.0, js-yaml@^3.13.0, js-yaml@^3.13.1: version "3.13.1" resolved "https://registry.yarnpkg.com/js-yaml/-/js-yaml-3.13.1.tgz#aff151b30bfdfa8e49e05da22e7415e9dfa37847" integrity sha512-YfbcO7jXDdyj0DGxYVSlSeQNHbD7XPWvrVWeVUujrQEoZzWJIRrCPoyk6kL6IAjAG2IolMK4T0hNUe0HOUs5Jw== dependencies: argparse "^1.0.7" esprima "^4.0.0" js-yaml@^3.9.0: version "3.12.1" resolved "https://registry.yarnpkg.com/js-yaml/-/js-yaml-3.12.1.tgz#295c8632a18a23e054cf5c9d3cecafe678167600" integrity sha512-um46hB9wNOKlwkHgiuyEVAybXBjwFUV0Z/RaHJblRd9DXltue9FTYvzCr9ErQrK9Adz5MU4gHWVaNUfdmrC8qA== dependencies: argparse "^1.0.7" esprima "^4.0.0" jsbn@~0.1.0: version "0.1.1" resolved "https://registry.yarnpkg.com/jsbn/-/jsbn-0.1.1.tgz#a5e654c2e5a2deb5f201d96cefbca80c0ef2f513" integrity sha1-peZUwuWi3rXyAdls77yoDA7y9RM= jsesc@^1.3.0: version "1.3.0" resolved "https://registry.yarnpkg.com/jsesc/-/jsesc-1.3.0.tgz#46c3fec8c1892b12b0833db9bc7622176dbab34b" integrity sha1-RsP+yMGJKxKwgz25vHYiF226s0s= jsesc@^2.5.1: version "2.5.2" resolved "https://registry.yarnpkg.com/jsesc/-/jsesc-2.5.2.tgz#80564d2e483dacf6e8ef209650a67df3f0c283a4" integrity sha512-OYu7XEzjkCQ3C5Ps3QIZsQfNpqoJyZZA99wd9aWd05NCtC5pWOkShK2mkL6HXQR6/Cy2lbNdPlZBpuQHXE63gA== jsesc@~0.5.0: version "0.5.0" resolved "https://registry.yarnpkg.com/jsesc/-/jsesc-0.5.0.tgz#e7dee66e35d6fc16f710fe91d5cf69f70f08911d" integrity sha1-597mbjXW/Bb3EP6R1c9p9w8IkR0= json-parse-better-errors@^1.0.1: version "1.0.2" resolved "https://registry.yarnpkg.com/json-parse-better-errors/-/json-parse-better-errors-1.0.2.tgz#bb867cfb3450e69107c131d1c514bab3dc8bcaa9" integrity sha512-mrqyZKfX5EhL7hvqcV6WG1yYjnjeuYDzDhhcAAUrq8Po85NBQBJP+ZDUT75qZQ98IkUoBqdkExkukOU7Ts2wrw== json-schema-traverse@^0.4.1: version "0.4.1" resolved "https://registry.yarnpkg.com/json-schema-traverse/-/json-schema-traverse-0.4.1.tgz#69f6a87d9513ab8bb8fe63bdb0979c448e684660" integrity sha512-xbbCH5dCYU5T8LcEhhuh7HJ88HXuW3qsI3Y0zOZFKfZEHcpWiHU/Jxzk629Brsab/mMiHQti9wMP+845RPe3Vg== json-schema@0.2.3: version "0.2.3" resolved "https://registry.yarnpkg.com/json-schema/-/json-schema-0.2.3.tgz#b480c892e59a2f05954ce727bd3f2a4e882f9e13" integrity sha1-tIDIkuWaLwWVTOcnvT8qTogvnhM= json-stable-stringify-without-jsonify@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/json-stable-stringify-without-jsonify/-/json-stable-stringify-without-jsonify-1.0.1.tgz#9db7b59496ad3f3cfef30a75142d2d930ad72651" integrity sha1-nbe1lJatPzz+8wp1FC0tkwrXJlE= json-stringify-safe@~5.0.1: version "5.0.1" resolved "https://registry.yarnpkg.com/json-stringify-safe/-/json-stringify-safe-5.0.1.tgz#1296a2d58fd45f19a0f6ce01d65701e2c735b6eb" integrity sha1-Epai1Y/UXxmg9s4B1lcB4sc1tus= json5@^0.5.1: version "0.5.1" resolved "https://registry.yarnpkg.com/json5/-/json5-0.5.1.tgz#1eade7acc012034ad84e2396767ead9fa5495821" integrity sha1-Hq3nrMASA0rYTiOWdn6tn6VJWCE= json5@^2.1.0: version "2.1.0" resolved "https://registry.yarnpkg.com/json5/-/json5-2.1.0.tgz#e7a0c62c48285c628d20a10b85c89bb807c32850" integrity sha512-8Mh9h6xViijj36g7Dxi+Y4S6hNGV96vcJZr/SrlHh1LR/pEn/8j/+qIBbs44YKl69Lrfctp4QD+AdWLTMqEZAQ== dependencies: minimist "^1.2.0" jsprim@^1.2.2: version "1.4.1" resolved "https://registry.yarnpkg.com/jsprim/-/jsprim-1.4.1.tgz#313e66bc1e5cc06e438bc1b7499c2e5c56acb6a2" integrity sha1-MT5mvB5cwG5Di8G3SZwuXFastqI= dependencies: assert-plus "1.0.0" extsprintf "1.3.0" json-schema "0.2.3" verror "1.10.0" kind-of@^3.0.2, kind-of@^3.0.3, kind-of@^3.2.0: version "3.2.2" resolved "https://registry.yarnpkg.com/kind-of/-/kind-of-3.2.2.tgz#31ea21a734bab9bbb0f32466d893aea51e4a3c64" integrity sha1-MeohpzS6ubuw8yRm2JOupR5KPGQ= dependencies: is-buffer "^1.1.5" kind-of@^4.0.0: version "4.0.0" resolved "https://registry.yarnpkg.com/kind-of/-/kind-of-4.0.0.tgz#20813df3d712928b207378691a45066fae72dd57" integrity sha1-IIE989cSkosgc3hpGkUGb65y3Vc= dependencies: is-buffer "^1.1.5" kind-of@^5.0.0: version "5.1.0" resolved "https://registry.yarnpkg.com/kind-of/-/kind-of-5.1.0.tgz#729c91e2d857b7a419a1f9aa65685c4c33f5845d" integrity sha512-NGEErnH6F2vUuXDh+OlbcKW7/wOcfdRHaZ7VWtqCztfHri/++YKmP51OdWeGPuqCOba6kk2OTe5d02VmTB80Pw== kind-of@^6.0.0, kind-of@^6.0.2: version "6.0.2" resolved "https://registry.yarnpkg.com/kind-of/-/kind-of-6.0.2.tgz#01146b36a6218e64e58f3a8d66de5d7fc6f6d051" integrity sha512-s5kLOcnH0XqDO+FvuaLX8DDjZ18CGFk7VygH40QoKPUQhW4e2rvM0rwUq0t8IQDOwYSeLK01U90OjzBTme2QqA== lcid@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/lcid/-/lcid-2.0.0.tgz#6ef5d2df60e52f82eb228a4c373e8d1f397253cf" integrity sha512-avPEb8P8EGnwXKClwsNUgryVjllcRqtMYa49NTsbQagYuT1DcXnl1915oxWjoyGrXR6zH/Y0Zc96xWsPcoDKeA== dependencies: invert-kv "^2.0.0" lcov-parse@^0.0.10: version "0.0.10" resolved "https://registry.yarnpkg.com/lcov-parse/-/lcov-parse-0.0.10.tgz#1b0b8ff9ac9c7889250582b70b71315d9da6d9a3" integrity sha1-GwuP+ayceIklBYK3C3ExXZ2m2aM= leaked-handles@^5.2.0: version "5.2.0" resolved "https://registry.yarnpkg.com/leaked-handles/-/leaked-handles-5.2.0.tgz#67228e90293b7e0ee36c4190e1f541cddece627f" integrity sha1-ZyKOkCk7fg7jbEGQ4fVBzd7OYn8= dependencies: process "^0.10.0" weakmap-shim "^1.1.0" xtend "^4.0.0" levn@^0.3.0, levn@~0.3.0: version "0.3.0" resolved "https://registry.yarnpkg.com/levn/-/levn-0.3.0.tgz#3b09924edf9f083c0490fdd4c0bc4421e04764ee" integrity sha1-OwmSTt+fCDwEkP3UwLxEIeBHZO4= dependencies: prelude-ls "~1.1.2" type-check "~0.3.2" lint-staged@^8.1.4: version "8.1.6" resolved "https://registry.yarnpkg.com/lint-staged/-/lint-staged-8.1.6.tgz#128a9bc5effbf69a359fb8f7eeb2da71a998daf6" integrity sha512-QT13AniHN6swAtTjsrzxOfE4TVCiQ39xESwLmjGVNCMMZ/PK5aopwvbxLrzw+Zf9OxM3cQG6WCx9lceLzETOnQ== dependencies: chalk "^2.3.1" commander "^2.14.1" cosmiconfig "^5.0.2" debug "^3.1.0" dedent "^0.7.0" del "^3.0.0" execa "^1.0.0" find-parent-dir "^0.3.0" g-status "^2.0.2" is-glob "^4.0.0" is-windows "^1.0.2" listr "^0.14.2" listr-update-renderer "^0.5.0" lodash "^4.17.11" log-symbols "^2.2.0" micromatch "^3.1.8" npm-which "^3.0.1" p-map "^1.1.1" path-is-inside "^1.0.2" pify "^3.0.0" please-upgrade-node "^3.0.2" staged-git-files "1.1.2" string-argv "^0.0.2" stringify-object "^3.2.2" yup "^0.27.0" listr-silent-renderer@^1.1.1: version "1.1.1" resolved "https://registry.yarnpkg.com/listr-silent-renderer/-/listr-silent-renderer-1.1.1.tgz#924b5a3757153770bf1a8e3fbf74b8bbf3f9242e" integrity sha1-kktaN1cVN3C/Go4/v3S4u/P5JC4= listr-update-renderer@^0.5.0: version "0.5.0" resolved "https://registry.yarnpkg.com/listr-update-renderer/-/listr-update-renderer-0.5.0.tgz#4ea8368548a7b8aecb7e06d8c95cb45ae2ede6a2" integrity sha512-tKRsZpKz8GSGqoI/+caPmfrypiaq+OQCbd+CovEC24uk1h952lVj5sC7SqyFUm+OaJ5HN/a1YLt5cit2FMNsFA== dependencies: chalk "^1.1.3" cli-truncate "^0.2.1" elegant-spinner "^1.0.1" figures "^1.7.0" indent-string "^3.0.0" log-symbols "^1.0.2" log-update "^2.3.0" strip-ansi "^3.0.1" listr-verbose-renderer@^0.5.0: version "0.5.0" resolved "https://registry.yarnpkg.com/listr-verbose-renderer/-/listr-verbose-renderer-0.5.0.tgz#f1132167535ea4c1261102b9f28dac7cba1e03db" integrity sha512-04PDPqSlsqIOaaaGZ+41vq5FejI9auqTInicFRndCBgE3bXG8D6W1I+mWhk+1nqbHmyhla/6BUrd5OSiHwKRXw== dependencies: chalk "^2.4.1" cli-cursor "^2.1.0" date-fns "^1.27.2" figures "^2.0.0" listr@^0.14.2: version "0.14.3" resolved "https://registry.yarnpkg.com/listr/-/listr-0.14.3.tgz#2fea909604e434be464c50bddba0d496928fa586" integrity sha512-RmAl7su35BFd/xoMamRjpIE4j3v+L28o8CT5YhAXQJm1fD+1l9ngXY8JAQRJ+tFK2i5njvi0iRUKV09vPwA0iA== dependencies: "@samverschueren/stream-to-observable" "^0.3.0" is-observable "^1.1.0" is-promise "^2.1.0" is-stream "^1.1.0" listr-silent-renderer "^1.1.1" listr-update-renderer "^0.5.0" listr-verbose-renderer "^0.5.0" p-map "^2.0.0" rxjs "^6.3.3" load-json-file@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/load-json-file/-/load-json-file-2.0.0.tgz#7947e42149af80d696cbf797bcaabcfe1fe29ca8" integrity sha1-eUfkIUmvgNaWy/eXvKq8/h/inKg= dependencies: graceful-fs "^4.1.2" parse-json "^2.2.0" pify "^2.0.0" strip-bom "^3.0.0" load-json-file@^4.0.0: version "4.0.0" resolved "https://registry.yarnpkg.com/load-json-file/-/load-json-file-4.0.0.tgz#2f5f45ab91e33216234fd53adab668eb4ec0993b" integrity sha1-L19Fq5HjMhYjT9U62rZo607AmTs= dependencies: graceful-fs "^4.1.2" parse-json "^4.0.0" pify "^3.0.0" strip-bom "^3.0.0" locate-path@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/locate-path/-/locate-path-2.0.0.tgz#2b568b265eec944c6d9c0de9c3dbbbca0354cd8e" integrity sha1-K1aLJl7slExtnA3pw9u7ygNUzY4= dependencies: p-locate "^2.0.0" path-exists "^3.0.0" locate-path@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/locate-path/-/locate-path-3.0.0.tgz#dbec3b3ab759758071b58fe59fc41871af21400e" integrity sha512-7AO748wWnIhNqAuaty2ZWHkQHRSNfPVIsPIfwEOWO22AmaoVrWavlOcMR5nzTLNYvp36X220/maaRsrec1G65A== dependencies: p-locate "^3.0.0" path-exists "^3.0.0" lodash.flattendeep@^4.4.0: version "4.4.0" resolved "https://registry.yarnpkg.com/lodash.flattendeep/-/lodash.flattendeep-4.4.0.tgz#fb030917f86a3134e5bc9bec0d69e0013ddfedb2" integrity sha1-+wMJF/hqMTTlvJvsDWngAT3f7bI= lodash.get@^4.4.2: version "4.4.2" resolved "https://registry.yarnpkg.com/lodash.get/-/lodash.get-4.4.2.tgz#2d177f652fa31e939b4438d5341499dfa3825e99" integrity sha1-LRd/ZS+jHpObRDjVNBSZ36OCXpk= lodash.throttle@^4.1.1: version "4.1.1" resolved "https://registry.yarnpkg.com/lodash.throttle/-/lodash.throttle-4.1.1.tgz#c23e91b710242ac70c37f1e1cda9274cc39bf2f4" integrity sha1-wj6RtxAkKscMN/HhzaknTMOb8vQ= lodash@^4.17.10, lodash@^4.17.11, lodash@^4.17.4: version "4.17.11" resolved "https://registry.yarnpkg.com/lodash/-/lodash-4.17.11.tgz#b39ea6229ef607ecd89e2c8df12536891cac9b8d" integrity sha512-cQKh8igo5QUhZ7lg38DYWAxMvjSAKG0A8wGSVimP07SIUEK2UO+arSRKbRZWtelMtN5V0Hkwh5ryOto/SshYIg== log-driver@^1.2.7: version "1.2.7" resolved "https://registry.yarnpkg.com/log-driver/-/log-driver-1.2.7.tgz#63b95021f0702fedfa2c9bb0a24e7797d71871d8" integrity sha512-U7KCmLdqsGHBLeWqYlFA0V0Sl6P08EE1ZrmA9cxjUE0WVqT9qnyVDPz1kzpFEP0jdJuFnasWIfSd7fsaNXkpbg== log-symbols@^1.0.2: version "1.0.2" resolved "https://registry.yarnpkg.com/log-symbols/-/log-symbols-1.0.2.tgz#376ff7b58ea3086a0f09facc74617eca501e1a18" integrity sha1-N2/3tY6jCGoPCfrMdGF+ylAeGhg= dependencies: chalk "^1.0.0" log-symbols@^2.2.0: version "2.2.0" resolved "https://registry.yarnpkg.com/log-symbols/-/log-symbols-2.2.0.tgz#5740e1c5d6f0dfda4ad9323b5332107ef6b4c40a" integrity sha512-VeIAFslyIerEJLXHziedo2basKbMKtTw3vfn5IzG0XTjhAVEJyNHnL2p7vc+wBDSdQuUpNw3M2u6xb9QsAY5Eg== dependencies: chalk "^2.0.1" log-update@^2.3.0: version "2.3.0" resolved "https://registry.yarnpkg.com/log-update/-/log-update-2.3.0.tgz#88328fd7d1ce7938b29283746f0b1bc126b24708" integrity sha1-iDKP19HOeTiykoN0bwsbwSayRwg= dependencies: ansi-escapes "^3.0.0" cli-cursor "^2.0.0" wrap-ansi "^3.0.1" log-update@^3.0.0: version "3.2.0" resolved "https://registry.yarnpkg.com/log-update/-/log-update-3.2.0.tgz#719f24293250d65d0165f4e2ec2ed805ff062eec" integrity sha512-KJ6zAPIHWo7Xg1jYror6IUDFJBq1bQ4Bi4wAEp2y/0ScjBBVi/g0thr0sUVhuvuXauWzczt7T2QHghPDNnKBuw== dependencies: ansi-escapes "^3.2.0" cli-cursor "^2.1.0" wrap-ansi "^5.0.0" loose-envify@^1.0.0, loose-envify@^1.1.0, loose-envify@^1.4.0: version "1.4.0" resolved "https://registry.yarnpkg.com/loose-envify/-/loose-envify-1.4.0.tgz#71ee51fa7be4caec1a63839f7e682d8132d30caf" integrity sha512-lyuxPGr/Wfhrlem2CL/UcnUc1zcqKAImBDzukY7Y5F/yQiNdko6+fRLevlw1HgMySw7f611UIY408EtxRSoK3Q== dependencies: js-tokens "^3.0.0 || ^4.0.0" lru-cache@^4.0.1: version "4.1.5" resolved "https://registry.yarnpkg.com/lru-cache/-/lru-cache-4.1.5.tgz#8bbe50ea85bed59bc9e33dcab8235ee9bcf443cd" integrity sha512-sWZlbEP2OsHNkXrMl5GYk/jKk70MBng6UU4YI/qGDYbgf6YbP4EvmqISbXCoJiRKs+1bSpFHVgQxvJ17F2li5g== dependencies: pseudomap "^1.0.2" yallist "^2.1.2" make-dir@^2.0.0, make-dir@^2.1.0: version "2.1.0" resolved "https://registry.yarnpkg.com/make-dir/-/make-dir-2.1.0.tgz#5f0310e18b8be898cc07009295a30ae41e91e6f5" integrity sha512-LS9X+dc8KLxXCb8dni79fLIIUA5VyZoyjSMCwTluaXA0o27cCK0bhXkpgw+sTXVpPy/lSO57ilRixqk0vDmtRA== dependencies: pify "^4.0.1" semver "^5.6.0" make-error@^1.1.1: version "1.3.5" resolved "https://registry.yarnpkg.com/make-error/-/make-error-1.3.5.tgz#efe4e81f6db28cadd605c70f29c831b58ef776c8" integrity sha512-c3sIjNUow0+8swNwVpqoH4YCShKNFkMaw6oH1mNS2haDZQqkeZFlHS3dhoeEbKKmJB4vXpJucU6oH75aDYeE9g== map-age-cleaner@^0.1.1: version "0.1.3" resolved "https://registry.yarnpkg.com/map-age-cleaner/-/map-age-cleaner-0.1.3.tgz#7d583a7306434c055fe474b0f45078e6e1b4b92a" integrity sha512-bJzx6nMoP6PDLPBFmg7+xRKeFZvFboMrGlxmNj9ClvX53KrmvM5bXFXEWjbz4cz1AFn+jWJ9z/DJSz7hrs0w3w== dependencies: p-defer "^1.0.0" map-cache@^0.2.2: version "0.2.2" resolved "https://registry.yarnpkg.com/map-cache/-/map-cache-0.2.2.tgz#c32abd0bd6525d9b051645bb4f26ac5dc98a0dbf" integrity sha1-wyq9C9ZSXZsFFkW7TyasXcmKDb8= map-visit@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/map-visit/-/map-visit-1.0.0.tgz#ecdca8f13144e660f1b5bd41f12f3479d98dfb8f" integrity sha1-7Nyo8TFE5mDxtb1B8S80edmN+48= dependencies: object-visit "^1.0.0" matcher@^1.0.0: version "1.1.1" resolved "https://registry.yarnpkg.com/matcher/-/matcher-1.1.1.tgz#51d8301e138f840982b338b116bb0c09af62c1c2" integrity sha512-+BmqxWIubKTRKNWx/ahnCkk3mG8m7OturVlqq6HiojGJTd5hVYbgZm6WzcYPCoB+KBT4Vd6R7WSRG2OADNaCjg== dependencies: escape-string-regexp "^1.0.4" mem@^4.0.0: version "4.3.0" resolved "https://registry.yarnpkg.com/mem/-/mem-4.3.0.tgz#461af497bc4ae09608cdb2e60eefb69bff744178" integrity sha512-qX2bG48pTqYRVmDB37rn/6PT7LcR8T7oAX3bf99u1Tt1nzxYfxkgqDwUwolPlXweM0XzBOBFzSx4kfp7KP1s/w== dependencies: map-age-cleaner "^0.1.1" mimic-fn "^2.0.0" p-is-promise "^2.0.0" merge-source-map@^1.1.0: version "1.1.0" resolved "https://registry.yarnpkg.com/merge-source-map/-/merge-source-map-1.1.0.tgz#2fdde7e6020939f70906a68f2d7ae685e4c8c646" integrity sha512-Qkcp7P2ygktpMPh2mCQZaf3jhN6D3Z/qVZHSdWvQ+2Ef5HgRAPBO57A77+ENm0CPx2+1Ce/MYKi3ymqdfuqibw== dependencies: source-map "^0.6.1" micromatch@^3.1.10, micromatch@^3.1.4, micromatch@^3.1.8: version "3.1.10" resolved "https://registry.yarnpkg.com/micromatch/-/micromatch-3.1.10.tgz#70859bc95c9840952f359a068a3fc49f9ecfac23" integrity sha512-MWikgl9n9M3w+bpsY3He8L+w9eF9338xRl8IAO5viDizwSzziFEyUzo2xrrloB64ADbTf8uA8vRqqttDTOmccg== dependencies: arr-diff "^4.0.0" array-unique "^0.3.2" braces "^2.3.1" define-property "^2.0.2" extend-shallow "^3.0.2" extglob "^2.0.4" fragment-cache "^0.2.1" kind-of "^6.0.2" nanomatch "^1.2.9" object.pick "^1.3.0" regex-not "^1.0.0" snapdragon "^0.8.1" to-regex "^3.0.2" mime-db@1.40.0: version "1.40.0" resolved "https://registry.yarnpkg.com/mime-db/-/mime-db-1.40.0.tgz#a65057e998db090f732a68f6c276d387d4126c32" integrity sha512-jYdeOMPy9vnxEqFRRo6ZvTZ8d9oPb+k18PKoYNYUe2stVEBPPwsln/qWzdbmaIvnhZ9v2P+CuecK+fpUfsV2mA== mime-types@^2.1.12, mime-types@~2.1.19: version "2.1.24" resolved "https://registry.yarnpkg.com/mime-types/-/mime-types-2.1.24.tgz#b6f8d0b3e951efb77dedeca194cff6d16f676f81" integrity sha512-WaFHS3MCl5fapm3oLxU4eYDw77IQM2ACcxQ9RIxfaC3ooc6PFuBMGZZsYpvoXS5D5QTWPieo1jjLdAm3TBP3cQ== dependencies: mime-db "1.40.0" mimic-fn@^1.0.0: version "1.2.0" resolved "https://registry.yarnpkg.com/mimic-fn/-/mimic-fn-1.2.0.tgz#820c86a39334640e99516928bd03fca88057d022" integrity sha512-jf84uxzwiuiIVKiOLpfYk7N46TSy8ubTonmneY9vrpHNAnp0QBt2BxWV9dO3/j+BoVAb+a5G6YDPW3M5HOdMWQ== mimic-fn@^2.0.0: version "2.1.0" resolved "https://registry.yarnpkg.com/mimic-fn/-/mimic-fn-2.1.0.tgz#7ed2c2ccccaf84d3ffcb7a69b57711fc2083401b" integrity sha512-OqbOk5oEQeAZ8WXWydlu9HJjz9WVdEIvamMCcXmuqUYjTknH/sqsWvhQ3vgwKFRR1HpjvNBKQ37nbJgYzGqGcg== minimatch@^3.0.4: version "3.0.4" resolved "https://registry.yarnpkg.com/minimatch/-/minimatch-3.0.4.tgz#5166e286457f03306064be5497e8dbb0c3d32083" integrity sha512-yJHVQEhyqPLUTgt9B83PXu6W3rx4MvvHvSUvToogpwoGDOUQ+yDrR0HRot+yOCdCO7u4hX3pWft6kWBBcqh0UA== dependencies: brace-expansion "^1.1.7" minimist@0.0.8: version "0.0.8" resolved "https://registry.yarnpkg.com/minimist/-/minimist-0.0.8.tgz#857fcabfc3397d2625b8228262e86aa7a011b05d" integrity sha1-hX/Kv8M5fSYluCKCYuhqp6ARsF0= minimist@^1.2.0: version "1.2.0" resolved "https://registry.yarnpkg.com/minimist/-/minimist-1.2.0.tgz#a35008b20f41383eec1fb914f4cd5df79a264284" integrity sha1-o1AIsg9BOD7sH7kU9M1d95omQoQ= minimist@~0.0.1: version "0.0.10" resolved "https://registry.yarnpkg.com/minimist/-/minimist-0.0.10.tgz#de3f98543dbf96082be48ad1a0c7cda836301dcf" integrity sha1-3j+YVD2/lggr5IrRoMfNqDYwHc8= minipass@^2.2.0, minipass@^2.2.1, minipass@^2.3.4, minipass@^2.3.5: version "2.3.5" resolved "https://registry.yarnpkg.com/minipass/-/minipass-2.3.5.tgz#cacebe492022497f656b0f0f51e2682a9ed2d848" integrity sha512-Gi1W4k059gyRbyVUZQ4mEqLm0YIUiGYfvxhF6SIlk3ui1WVxMTGfGdQ2SInh3PDrRTVvPKgULkpJtT4RH10+VA== dependencies: safe-buffer "^5.1.2" yallist "^3.0.0" minizlib@^1.1.1: version "1.2.1" resolved "https://registry.yarnpkg.com/minizlib/-/minizlib-1.2.1.tgz#dd27ea6136243c7c880684e8672bb3a45fd9b614" integrity sha512-7+4oTUOWKg7AuL3vloEWekXY2/D20cevzsrNT2kGWm+39J9hGTCBv8VI5Pm5lXZ/o3/mdR4f8rflAPhnQb8mPA== dependencies: minipass "^2.2.1" mixin-deep@^1.2.0: version "1.3.1" resolved "https://registry.yarnpkg.com/mixin-deep/-/mixin-deep-1.3.1.tgz#a49e7268dce1a0d9698e45326c5626df3543d0fe" integrity sha512-8ZItLHeEgaqEvd5lYBXfm4EZSFCX29Jb9K+lAHhDKzReKBQKj3R+7NOF6tjqYi9t4oI8VUfaWITJQm86wnXGNQ== dependencies: for-in "^1.0.2" is-extendable "^1.0.1" mkdirp@^0.5.0, mkdirp@^0.5.1: version "0.5.1" resolved "https://registry.yarnpkg.com/mkdirp/-/mkdirp-0.5.1.tgz#30057438eac6cf7f8c4767f38648d6697d75c903" integrity sha1-MAV0OOrGz3+MR2fzhkjWaX11yQM= dependencies: minimist "0.0.8" ms@2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/ms/-/ms-2.0.0.tgz#5608aeadfc00be6c2901df5f9861788de0d597c8" integrity sha1-VgiurfwAvmwpAd9fmGF4jeDVl8g= ms@^2.1.1: version "2.1.1" resolved "https://registry.yarnpkg.com/ms/-/ms-2.1.1.tgz#30a5864eb3ebb0a66f2ebe6d727af06a09d86e0a" integrity sha512-tgp+dl5cGk28utYktBsrFqA7HKgrhgPsg6Z/EfhWI4gl1Hwq8B/GmY/0oXZ6nF8hDVesS/FpnYaD/kOWhYQvyg== mute-stream@0.0.7: version "0.0.7" resolved "https://registry.yarnpkg.com/mute-stream/-/mute-stream-0.0.7.tgz#3075ce93bc21b8fab43e1bc4da7e8115ed1e7bab" integrity sha1-MHXOk7whuPq0PhvE2n6BFe0ee6s= nan@^2.12.1: version "2.13.2" resolved "https://registry.yarnpkg.com/nan/-/nan-2.13.2.tgz#f51dc7ae66ba7d5d55e1e6d4d8092e802c9aefe7" integrity sha512-TghvYc72wlMGMVMluVo9WRJc0mB8KxxF/gZ4YYFy7V2ZQX9l7rgbPg7vjS9mt6U5HXODVFVI2bOduCzwOMv/lw== nanomatch@^1.2.9: version "1.2.13" resolved "https://registry.yarnpkg.com/nanomatch/-/nanomatch-1.2.13.tgz#b87a8aa4fc0de8fe6be88895b38983ff265bd119" integrity sha512-fpoe2T0RbHwBTBUOftAfBPaDEi06ufaUai0mE6Yn1kacc3SnTErfb/h+X94VXzI64rKFHYImXSvdwGGCmwOqCA== dependencies: arr-diff "^4.0.0" array-unique "^0.3.2" define-property "^2.0.2" extend-shallow "^3.0.2" fragment-cache "^0.2.1" is-windows "^1.0.2" kind-of "^6.0.2" object.pick "^1.3.0" regex-not "^1.0.0" snapdragon "^0.8.1" to-regex "^3.0.1" natural-compare@^1.4.0: version "1.4.0" resolved "https://registry.yarnpkg.com/natural-compare/-/natural-compare-1.4.0.tgz#4abebfeed7541f2c27acfb29bdbbd15c8d5ba4f7" integrity sha1-Sr6/7tdUHywnrPspvbvRXI1bpPc= needle@^2.2.1: version "2.2.4" resolved "https://registry.yarnpkg.com/needle/-/needle-2.2.4.tgz#51931bff82533b1928b7d1d69e01f1b00ffd2a4e" integrity sha512-HyoqEb4wr/rsoaIDfTH2aVL9nWtQqba2/HvMv+++m8u0dz808MaagKILxtfeSN7QU7nvbQ79zk3vYOJp9zsNEA== dependencies: debug "^2.1.2" iconv-lite "^0.4.4" sax "^1.2.4" neo-async@^2.6.0: version "2.6.0" resolved "https://registry.yarnpkg.com/neo-async/-/neo-async-2.6.0.tgz#b9d15e4d71c6762908654b5183ed38b753340835" integrity sha512-MFh0d/Wa7vkKO3Y3LlacqAEeHK0mckVqzDieUKTT+KGxi+zIpeVsFxymkIiRpbpDziHc290Xr9A1O4Om7otoRA== nested-error-stacks@^2.0.0: version "2.1.0" resolved "https://registry.yarnpkg.com/nested-error-stacks/-/nested-error-stacks-2.1.0.tgz#0fbdcf3e13fe4994781280524f8b96b0cdff9c61" integrity sha512-AO81vsIO1k1sM4Zrd6Hu7regmJN1NSiAja10gc4bX3F0wd+9rQmcuHQaHVQCYIEC8iFXnE+mavh23GOt7wBgug== nice-try@^1.0.4: version "1.0.5" resolved "https://registry.yarnpkg.com/nice-try/-/nice-try-1.0.5.tgz#a3378a7696ce7d223e88fc9b764bd7ef1089e366" integrity sha512-1nh45deeb5olNY7eX82BkPO7SSxR5SSYJiPTrTdFUVYwAl8CKMA5N9PjTYkHiRjisVcxcQ1HXdLhx2qxxJzLNQ== node-pre-gyp@^0.12.0: version "0.12.0" resolved "https://registry.yarnpkg.com/node-pre-gyp/-/node-pre-gyp-0.12.0.tgz#39ba4bb1439da030295f899e3b520b7785766149" integrity sha512-4KghwV8vH5k+g2ylT+sLTjy5wmUOb9vPhnM8NHvRf9dHmnW/CndrFXy2aRPaPST6dugXSdHXfeaHQm77PIz/1A== dependencies: detect-libc "^1.0.2" mkdirp "^0.5.1" needle "^2.2.1" nopt "^4.0.1" npm-packlist "^1.1.6" npmlog "^4.0.2" rc "^1.2.7" rimraf "^2.6.1" semver "^5.3.0" tar "^4" node-releases@^1.1.17: version "1.1.17" resolved "https://registry.yarnpkg.com/node-releases/-/node-releases-1.1.17.tgz#71ea4631f0a97d5cd4f65f7d04ecf9072eac711a" integrity sha512-/SCjetyta1m7YXLgtACZGDYJdCSIBAWorDWkGCGZlydP2Ll7J48l7j/JxNYZ+xsgSPbWfdulVS/aY+GdjUsQ7Q== dependencies: semver "^5.3.0" nopt@^4.0.1: version "4.0.1" resolved "https://registry.yarnpkg.com/nopt/-/nopt-4.0.1.tgz#d0d4685afd5415193c8c7505602d0d17cd64474d" integrity sha1-0NRoWv1UFRk8jHUFYC0NF81kR00= dependencies: abbrev "1" osenv "^0.1.4" normalize-package-data@^2.3.2: version "2.4.0" resolved "https://registry.yarnpkg.com/normalize-package-data/-/normalize-package-data-2.4.0.tgz#12f95a307d58352075a04907b84ac8be98ac012f" integrity sha512-9jjUFbTPfEy3R/ad/2oNbKtW9Hgovl5O1FvFWKkKblNXoN/Oou6+9+KKohPK13Yc3/TyunyWhJp6gvRNR/PPAw== dependencies: hosted-git-info "^2.1.4" is-builtin-module "^1.0.0" semver "2 || 3 || 4 || 5" validate-npm-package-license "^3.0.1" normalize-package-data@^2.5.0: version "2.5.0" resolved "https://registry.yarnpkg.com/normalize-package-data/-/normalize-package-data-2.5.0.tgz#e66db1838b200c1dfc233225d12cb36520e234a8" integrity sha512-/5CMN3T0R4XTj4DcGaexo+roZSdSFW/0AOOTROrjxzCG1wrWXEsGbRKevjlIL+ZDE4sZlJr5ED4YW0yqmkK+eA== dependencies: hosted-git-info "^2.1.4" resolve "^1.10.0" semver "2 || 3 || 4 || 5" validate-npm-package-license "^3.0.1" normalize-path@^2.1.1: version "2.1.1" resolved "https://registry.yarnpkg.com/normalize-path/-/normalize-path-2.1.1.tgz#1ab28b556e198363a8c1a6f7e6fa20137fe6aed9" integrity sha1-GrKLVW4Zg2Oowab35vogE3/mrtk= dependencies: remove-trailing-separator "^1.0.1" normalize-path@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/normalize-path/-/normalize-path-3.0.0.tgz#0dcd69ff23a1c9b11fd0978316644a0388216a65" integrity sha512-6eZs5Ls3WtCisHWp9S2GUy8dqkpGi4BVSz3GaqiE6ezub0512ESztXUwUB6C6IKbQkY2Pnb/mD4WYojCRwcwLA== npm-bundled@^1.0.1: version "1.0.5" resolved "https://registry.yarnpkg.com/npm-bundled/-/npm-bundled-1.0.5.tgz#3c1732b7ba936b3a10325aef616467c0ccbcc979" integrity sha512-m/e6jgWu8/v5niCUKQi9qQl8QdeEduFA96xHDDzFGqly0OOjI7c+60KM/2sppfnUU9JJagf+zs+yGhqSOFj71g== npm-packlist@^1.1.6: version "1.2.0" resolved "https://registry.yarnpkg.com/npm-packlist/-/npm-packlist-1.2.0.tgz#55a60e793e272f00862c7089274439a4cc31fc7f" integrity sha512-7Mni4Z8Xkx0/oegoqlcao/JpPCPEMtUvsmB0q7mgvlMinykJLSRTYuFqoQLYgGY8biuxIeiHO+QNJKbCfljewQ== dependencies: ignore-walk "^3.0.1" npm-bundled "^1.0.1" npm-path@^2.0.2: version "2.0.4" resolved "https://registry.yarnpkg.com/npm-path/-/npm-path-2.0.4.tgz#c641347a5ff9d6a09e4d9bce5580c4f505278e64" integrity sha512-IFsj0R9C7ZdR5cP+ET342q77uSRdtWOlWpih5eC+lu29tIDbNEgDbzgVJ5UFvYHWhxDZ5TFkJafFioO0pPQjCw== dependencies: which "^1.2.10" npm-run-path@^2.0.0: version "2.0.2" resolved "https://registry.yarnpkg.com/npm-run-path/-/npm-run-path-2.0.2.tgz#35a9232dfa35d7067b4cb2ddf2357b1871536c5f" integrity sha1-NakjLfo11wZ7TLLd8jV7GHFTbF8= dependencies: path-key "^2.0.0" npm-which@^3.0.1: version "3.0.1" resolved "https://registry.yarnpkg.com/npm-which/-/npm-which-3.0.1.tgz#9225f26ec3a285c209cae67c3b11a6b4ab7140aa" integrity sha1-kiXybsOihcIJyuZ8OxGmtKtxQKo= dependencies: commander "^2.9.0" npm-path "^2.0.2" which "^1.2.10" npmlog@^4.0.2: version "4.1.2" resolved "https://registry.yarnpkg.com/npmlog/-/npmlog-4.1.2.tgz#08a7f2a8bf734604779a9efa4ad5cc717abb954b" integrity sha512-2uUqazuKlTaSI/dC8AzicUck7+IrEaOnN/e0jd3Xtt1KcGpwx30v50mL7oPyr/h9bL3E4aZccVwpwP+5W9Vjkg== dependencies: are-we-there-yet "~1.1.2" console-control-strings "~1.1.0" gauge "~2.7.3" set-blocking "~2.0.0" number-is-nan@^1.0.0: version "1.0.1" resolved "https://registry.yarnpkg.com/number-is-nan/-/number-is-nan-1.0.1.tgz#097b602b53422a522c1afb8790318336941a011d" integrity sha1-CXtgK1NCKlIsGvuHkDGDNpQaAR0= nyc@^14.1.0: version "14.1.0" resolved "https://registry.yarnpkg.com/nyc/-/nyc-14.1.0.tgz#ae864913a4c5a947bfaebeb66a488bdb1868c9a3" integrity sha512-iy9fEV8Emevz3z/AanIZsoGa8F4U2p0JKevZ/F0sk+/B2r9E6Qn+EPs0bpxEhnAt6UPlTL8mQZIaSJy8sK0ZFw== dependencies: archy "^1.0.0" caching-transform "^3.0.2" convert-source-map "^1.6.0" cp-file "^6.2.0" find-cache-dir "^2.1.0" find-up "^3.0.0" foreground-child "^1.5.6" glob "^7.1.3" istanbul-lib-coverage "^2.0.5" istanbul-lib-hook "^2.0.7" istanbul-lib-instrument "^3.3.0" istanbul-lib-report "^2.0.8" istanbul-lib-source-maps "^3.0.6" istanbul-reports "^2.2.4" js-yaml "^3.13.1" make-dir "^2.1.0" merge-source-map "^1.1.0" resolve-from "^4.0.0" rimraf "^2.6.3" signal-exit "^3.0.2" spawn-wrap "^1.4.2" test-exclude "^5.2.3" uuid "^3.3.2" yargs "^13.2.2" yargs-parser "^13.0.0" oauth-sign@~0.9.0: version "0.9.0" resolved "https://registry.yarnpkg.com/oauth-sign/-/oauth-sign-0.9.0.tgz#47a7b016baa68b5fa0ecf3dee08a85c679ac6455" integrity sha512-fexhUFFPTGV8ybAtSIGbV6gOkSv8UtRbDBnAyLQw4QPKkgNlsH2ByPGtMUqdWkos6YCRmAqViwgZrJc/mRDzZQ== object-assign@^4.0.1, object-assign@^4.1.0, object-assign@^4.1.1: version "4.1.1" resolved "https://registry.yarnpkg.com/object-assign/-/object-assign-4.1.1.tgz#2109adc7965887cfc05cbbd442cac8bfbb360863" integrity sha1-IQmtx5ZYh8/AXLvUQsrIv7s2CGM= object-copy@^0.1.0: version "0.1.0" resolved "https://registry.yarnpkg.com/object-copy/-/object-copy-0.1.0.tgz#7e7d858b781bd7c991a41ba975ed3812754e998c" integrity sha1-fn2Fi3gb18mRpBupde04EnVOmYw= dependencies: copy-descriptor "^0.1.0" define-property "^0.2.5" kind-of "^3.0.3" object-keys@^1.0.12: version "1.1.1" resolved "https://registry.yarnpkg.com/object-keys/-/object-keys-1.1.1.tgz#1c47f272df277f3b1daf061677d9c82e2322c60e" integrity sha512-NuAESUOUMrlIXOfHKzD6bpPu3tYt3xvjNdRIQ+FeT0lNb4K8WR70CaDxhuNguS2XG+GjkyMwOzsN5ZktImfhLA== object-visit@^1.0.0: version "1.0.1" resolved "https://registry.yarnpkg.com/object-visit/-/object-visit-1.0.1.tgz#f79c4493af0c5377b59fe39d395e41042dd045bb" integrity sha1-95xEk68MU3e1n+OdOV5BBC3QRbs= dependencies: isobject "^3.0.0" object.pick@^1.3.0: version "1.3.0" resolved "https://registry.yarnpkg.com/object.pick/-/object.pick-1.3.0.tgz#87a10ac4c1694bd2e1cbf53591a66141fb5dd747" integrity sha1-h6EKxMFpS9Lhy/U1kaZhQftd10c= dependencies: isobject "^3.0.1" once@^1.3.0, once@^1.3.1, once@^1.4.0: version "1.4.0" resolved "https://registry.yarnpkg.com/once/-/once-1.4.0.tgz#583b1aa775961d4b113ac17d9c50baef9dd76bd1" integrity sha1-WDsap3WWHUsROsF9nFC6753Xa9E= dependencies: wrappy "1" onetime@^2.0.0: version "2.0.1" resolved "https://registry.yarnpkg.com/onetime/-/onetime-2.0.1.tgz#067428230fd67443b2794b22bba528b6867962d4" integrity sha1-BnQoIw/WdEOyeUsiu6UotoZ5YtQ= dependencies: mimic-fn "^1.0.0" opener@^1.5.1: version "1.5.1" resolved "https://registry.yarnpkg.com/opener/-/opener-1.5.1.tgz#6d2f0e77f1a0af0032aca716c2c1fbb8e7e8abed" integrity sha512-goYSy5c2UXE4Ra1xixabeVh1guIX/ZV/YokJksb6q2lubWu6UbvPQ20p542/sFIll1nl8JnCyK9oBaOcCWXwvA== optimist@^0.6.1: version "0.6.1" resolved "https://registry.yarnpkg.com/optimist/-/optimist-0.6.1.tgz#da3ea74686fa21a19a111c326e90eb15a0196686" integrity sha1-2j6nRob6IaGaERwybpDrFaAZZoY= dependencies: minimist "~0.0.1" wordwrap "~0.0.2" optionator@^0.8.2: version "0.8.2" resolved "https://registry.yarnpkg.com/optionator/-/optionator-0.8.2.tgz#364c5e409d3f4d6301d6c0b4c05bba50180aeb64" integrity sha1-NkxeQJ0/TWMB1sC0wFu6UBgK62Q= dependencies: deep-is "~0.1.3" fast-levenshtein "~2.0.4" levn "~0.3.0" prelude-ls "~1.1.2" type-check "~0.3.2" wordwrap "~1.0.0" os-homedir@^1.0.0, os-homedir@^1.0.1: version "1.0.2" resolved "https://registry.yarnpkg.com/os-homedir/-/os-homedir-1.0.2.tgz#ffbc4988336e0e833de0c168c7ef152121aa7fb3" integrity sha1-/7xJiDNuDoM94MFox+8VISGqf7M= os-locale@^3.1.0: version "3.1.0" resolved "https://registry.yarnpkg.com/os-locale/-/os-locale-3.1.0.tgz#a802a6ee17f24c10483ab9935719cef4ed16bf1a" integrity sha512-Z8l3R4wYWM40/52Z+S265okfFj8Kt2cC2MKY+xNi3kFs+XGI7WXu/I309QQQYbRW4ijiZ+yxs9pqEhJh0DqW3Q== dependencies: execa "^1.0.0" lcid "^2.0.0" mem "^4.0.0" os-tmpdir@^1.0.0, os-tmpdir@^1.0.1, os-tmpdir@~1.0.2: version "1.0.2" resolved "https://registry.yarnpkg.com/os-tmpdir/-/os-tmpdir-1.0.2.tgz#bbe67406c79aa85c5cfec766fe5734555dfa1274" integrity sha1-u+Z0BseaqFxc/sdm/lc0VV36EnQ= osenv@^0.1.4: version "0.1.5" resolved "https://registry.yarnpkg.com/osenv/-/osenv-0.1.5.tgz#85cdfafaeb28e8677f416e287592b5f3f49ea410" integrity sha512-0CWcCECdMVc2Rw3U5w9ZjqX6ga6ubk1xDVKxtBQPK7wis/0F2r9T6k4ydGYhecl7YUBxBVxhL5oisPsNxAPe2g== dependencies: os-homedir "^1.0.0" os-tmpdir "^1.0.0" output-file-sync@^2.0.0: version "2.0.1" resolved "https://registry.yarnpkg.com/output-file-sync/-/output-file-sync-2.0.1.tgz#f53118282f5f553c2799541792b723a4c71430c0" integrity sha512-mDho4qm7WgIXIGf4eYU1RHN2UU5tPfVYVSRwDJw0uTmj35DQUt/eNp19N7v6T3SrR0ESTEf2up2CGO73qI35zQ== dependencies: graceful-fs "^4.1.11" is-plain-obj "^1.1.0" mkdirp "^0.5.1" own-or-env@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/own-or-env/-/own-or-env-1.0.1.tgz#54ce601d3bf78236c5c65633aa1c8ec03f8007e4" integrity sha512-y8qULRbRAlL6x2+M0vIe7jJbJx/kmUTzYonRAa2ayesR2qWLswninkVyeJe4x3IEXhdgoNodzjQRKAoEs6Fmrw== dependencies: own-or "^1.0.0" own-or@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/own-or/-/own-or-1.0.0.tgz#4e877fbeda9a2ec8000fbc0bcae39645ee8bf8dc" integrity sha1-Tod/vtqaLsgAD7wLyuOWRe6L+Nw= p-defer@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/p-defer/-/p-defer-1.0.0.tgz#9f6eb182f6c9aa8cd743004a7d4f96b196b0fb0c" integrity sha1-n26xgvbJqozXQwBKfU+WsZaw+ww= p-finally@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/p-finally/-/p-finally-1.0.0.tgz#3fbcfb15b899a44123b34b6dcc18b724336a2cae" integrity sha1-P7z7FbiZpEEjs0ttzBi3JDNqLK4= p-is-promise@^2.0.0: version "2.1.0" resolved "https://registry.yarnpkg.com/p-is-promise/-/p-is-promise-2.1.0.tgz#918cebaea248a62cf7ffab8e3bca8c5f882fc42e" integrity sha512-Y3W0wlRPK8ZMRbNq97l4M5otioeA5lm1z7bkNkxCka8HSPjR0xRWmpCmc9utiaLP9Jb1eD8BgeIxTW4AIF45Pg== p-limit@^1.1.0: version "1.3.0" resolved "https://registry.yarnpkg.com/p-limit/-/p-limit-1.3.0.tgz#b86bd5f0c25690911c7590fcbfc2010d54b3ccb8" integrity sha512-vvcXsLAJ9Dr5rQOPk7toZQZJApBl2K4J6dANSsEuh6QI41JYcsS/qhTGa9ErIUUgK3WNQoJYvylxvjqmiqEA9Q== dependencies: p-try "^1.0.0" p-limit@^2.0.0: version "2.1.0" resolved "https://registry.yarnpkg.com/p-limit/-/p-limit-2.1.0.tgz#1d5a0d20fb12707c758a655f6bbc4386b5930d68" integrity sha512-NhURkNcrVB+8hNfLuysU8enY5xn2KXphsHBaC2YmRNTZRc7RWusw6apSpdEj3jo4CMb6W9nrF6tTnsJsJeyu6g== dependencies: p-try "^2.0.0" p-locate@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/p-locate/-/p-locate-2.0.0.tgz#20a0103b222a70c8fd39cc2e580680f3dde5ec43" integrity sha1-IKAQOyIqcMj9OcwuWAaA893l7EM= dependencies: p-limit "^1.1.0" p-locate@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/p-locate/-/p-locate-3.0.0.tgz#322d69a05c0264b25997d9f40cd8a891ab0064a4" integrity sha512-x+12w/To+4GFfgJhBEpiDcLozRJGegY+Ei7/z0tSLkMmxGZNybVMSfWj9aJn8Z5Fc7dBUNJOOVgPv2H7IwulSQ== dependencies: p-limit "^2.0.0" p-map@^1.1.1: version "1.2.0" resolved "https://registry.yarnpkg.com/p-map/-/p-map-1.2.0.tgz#e4e94f311eabbc8633a1e79908165fca26241b6b" integrity sha512-r6zKACMNhjPJMTl8KcFH4li//gkrXWfbD6feV8l6doRHlzljFWGJ2AP6iKaCJXyZmAUMOPtvbW7EXkbWO/pLEA== p-map@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/p-map/-/p-map-2.0.0.tgz#be18c5a5adeb8e156460651421aceca56c213a50" integrity sha512-GO107XdrSUmtHxVoi60qc9tUl/KkNKm+X2CF4P9amalpGxv5YqVPJNfSb0wcA+syCopkZvYYIzW8OVTQW59x/w== p-try@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/p-try/-/p-try-1.0.0.tgz#cbc79cdbaf8fd4228e13f621f2b1a237c1b207b3" integrity sha1-y8ec26+P1CKOE/Yh8rGiN8GyB7M= p-try@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/p-try/-/p-try-2.0.0.tgz#85080bb87c64688fa47996fe8f7dfbe8211760b1" integrity sha512-hMp0onDKIajHfIkdRk3P4CdCmErkYAxxDtP3Wx/4nZ3aGlau2VKh3mZpcuFkH27WQkL/3WBCPOktzA9ZOAnMQQ== package-hash@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/package-hash/-/package-hash-3.0.0.tgz#50183f2d36c9e3e528ea0a8605dff57ce976f88e" integrity sha512-lOtmukMDVvtkL84rJHI7dpTYq+0rli8N2wlnqUcBuDWCfVhRUfOmnR9SsoHFMLpACvEV60dX7rd0rFaYDZI+FA== dependencies: graceful-fs "^4.1.15" hasha "^3.0.0" lodash.flattendeep "^4.4.0" release-zalgo "^1.0.0" parent-module@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/parent-module/-/parent-module-1.0.0.tgz#df250bdc5391f4a085fb589dad761f5ad6b865b5" integrity sha512-8Mf5juOMmiE4FcmzYc4IaiS9L3+9paz2KOiXzkRviCP6aDmN49Hz6EMWz0lGNp9pX80GvvAuLADtyGfW/Em3TA== dependencies: callsites "^3.0.0" parse-json@^2.2.0: version "2.2.0" resolved "https://registry.yarnpkg.com/parse-json/-/parse-json-2.2.0.tgz#f480f40434ef80741f8469099f8dea18f55a4dc9" integrity sha1-9ID0BDTvgHQfhGkJn43qGPVaTck= dependencies: error-ex "^1.2.0" parse-json@^4.0.0: version "4.0.0" resolved "https://registry.yarnpkg.com/parse-json/-/parse-json-4.0.0.tgz#be35f5425be1f7f6c747184f98a788cb99477ee0" integrity sha1-vjX1Qlvh9/bHRxhPmKeIy5lHfuA= dependencies: error-ex "^1.3.1" json-parse-better-errors "^1.0.1" pascalcase@^0.1.1: version "0.1.1" resolved "https://registry.yarnpkg.com/pascalcase/-/pascalcase-0.1.1.tgz#b363e55e8006ca6fe21784d2db22bd15d7917f14" integrity sha1-s2PlXoAGym/iF4TS2yK9FdeRfxQ= path-dirname@^1.0.0: version "1.0.2" resolved "https://registry.yarnpkg.com/path-dirname/-/path-dirname-1.0.2.tgz#cc33d24d525e099a5388c0336c6e32b9160609e0" integrity sha1-zDPSTVJeCZpTiMAzbG4yuRYGCeA= path-exists@^2.0.0: version "2.1.0" resolved "https://registry.yarnpkg.com/path-exists/-/path-exists-2.1.0.tgz#0feb6c64f0fc518d9a754dd5efb62c7022761f4b" integrity sha1-D+tsZPD8UY2adU3V77YscCJ2H0s= dependencies: pinkie-promise "^2.0.0" path-exists@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/path-exists/-/path-exists-3.0.0.tgz#ce0ebeaa5f78cb18925ea7d810d7b59b010fd515" integrity sha1-zg6+ql94yxiSXqfYENe1mwEP1RU= path-is-absolute@^1.0.0, path-is-absolute@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/path-is-absolute/-/path-is-absolute-1.0.1.tgz#174b9268735534ffbc7ace6bf53a5a9e1b5c5f5f" integrity sha1-F0uSaHNVNP+8es5r9TpanhtcX18= path-is-inside@^1.0.1, path-is-inside@^1.0.2: version "1.0.2" resolved "https://registry.yarnpkg.com/path-is-inside/-/path-is-inside-1.0.2.tgz#365417dede44430d1c11af61027facf074bdfc53" integrity sha1-NlQX3t5EQw0cEa9hAn+s8HS9/FM= path-key@^2.0.0, path-key@^2.0.1: version "2.0.1" resolved "https://registry.yarnpkg.com/path-key/-/path-key-2.0.1.tgz#411cadb574c5a140d3a4b1910d40d80cc9f40b40" integrity sha1-QRyttXTFoUDTpLGRDUDYDMn0C0A= path-parse@^1.0.6: version "1.0.6" resolved "https://registry.yarnpkg.com/path-parse/-/path-parse-1.0.6.tgz#d62dbb5679405d72c4737ec58600e9ddcf06d24c" integrity sha512-GSmOT2EbHrINBf9SR7CDELwlJ8AENk3Qn7OikK4nFYAu3Ote2+JYNVvkpAEQm3/TLNEJFD/xZJjzyxg3KBWOzw== path-type@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/path-type/-/path-type-2.0.0.tgz#f012ccb8415b7096fc2daa1054c3d72389594c73" integrity sha1-8BLMuEFbcJb8LaoQVMPXI4lZTHM= dependencies: pify "^2.0.0" path-type@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/path-type/-/path-type-3.0.0.tgz#cef31dc8e0a1a3bb0d105c0cd97cf3bf47f4e36f" integrity sha512-T2ZUsdZFHgA3u4e5PfPbjd7HDDpxPnQb5jN0SrDsjNSuVXHJqtwTnWqG0B1jZrgmJ/7lj1EmVIByWt1gxGkWvg== dependencies: pify "^3.0.0" performance-now@^2.1.0: version "2.1.0" resolved "https://registry.yarnpkg.com/performance-now/-/performance-now-2.1.0.tgz#6309f4e0e5fa913ec1c69307ae364b4b377c9e7b" integrity sha1-Ywn04OX6kT7BxpMHrjZLSzd8nns= pify@^2.0.0: version "2.3.0" resolved "https://registry.yarnpkg.com/pify/-/pify-2.3.0.tgz#ed141a6ac043a849ea588498e7dca8b15330e90c" integrity sha1-7RQaasBDqEnqWISY59yosVMw6Qw= pify@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/pify/-/pify-3.0.0.tgz#e5a4acd2c101fdf3d9a4d07f0dbc4db49dd28176" integrity sha1-5aSs0sEB/fPZpNB/DbxNtJ3SgXY= pify@^4.0.1: version "4.0.1" resolved "https://registry.yarnpkg.com/pify/-/pify-4.0.1.tgz#4b2cd25c50d598735c50292224fd8c6df41e3231" integrity sha512-uB80kBFb/tfd68bVleG9T5GGsGPjJrLAUpR5PZIrhBnIaRTQRjqdJSsIKkOP6OAIFbj7GOrcudc5pNjZ+geV2g== pinkie-promise@^2.0.0: version "2.0.1" resolved "https://registry.yarnpkg.com/pinkie-promise/-/pinkie-promise-2.0.1.tgz#2135d6dfa7a358c069ac9b178776288228450ffa" integrity sha1-ITXW36ejWMBprJsXh3YogihFD/o= dependencies: pinkie "^2.0.0" pinkie@^2.0.0: version "2.0.4" resolved "https://registry.yarnpkg.com/pinkie/-/pinkie-2.0.4.tgz#72556b80cfa0d48a974e80e77248e80ed4f7f870" integrity sha1-clVrgM+g1IqXToDnckjoDtT3+HA= pkg-dir@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/pkg-dir/-/pkg-dir-1.0.0.tgz#7a4b508a8d5bb2d629d447056ff4e9c9314cf3d4" integrity sha1-ektQio1bstYp1EcFb/TpyTFM89Q= dependencies: find-up "^1.0.0" pkg-dir@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/pkg-dir/-/pkg-dir-2.0.0.tgz#f6d5d1109e19d63edf428e0bd57e12777615334b" integrity sha1-9tXREJ4Z1j7fQo4L1X4Sd3YVM0s= dependencies: find-up "^2.1.0" pkg-dir@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/pkg-dir/-/pkg-dir-3.0.0.tgz#2749020f239ed990881b1f71210d51eb6523bea3" integrity sha512-/E57AYkoeQ25qkxMj5PBOVgF8Kiu/h7cYS30Z5+R7WaiCCBfLq58ZI/dSeaEKb9WVJV5n/03QwrN3IeWIFllvw== dependencies: find-up "^3.0.0" pkg-dir@^4.1.0: version "4.1.0" resolved "https://registry.yarnpkg.com/pkg-dir/-/pkg-dir-4.1.0.tgz#aaeb91c0d3b9c4f74a44ad849f4de34781ae01de" integrity sha512-55k9QN4saZ8q518lE6EFgYiu95u3BWkSajCifhdQjvLvmr8IpnRbhI+UGpWJQfa0KzDguHeeWT1ccO1PmkOi3A== dependencies: find-up "^3.0.0" please-upgrade-node@^3.0.2, please-upgrade-node@^3.1.1: version "3.1.1" resolved "https://registry.yarnpkg.com/please-upgrade-node/-/please-upgrade-node-3.1.1.tgz#ed320051dfcc5024fae696712c8288993595e8ac" integrity sha512-KY1uHnQ2NlQHqIJQpnh/i54rKkuxCEBx+voJIS/Mvb+L2iYd2NMotwduhKTMjfC1uKoX3VXOxLjIYG66dfJTVQ== dependencies: semver-compare "^1.0.0" posix-character-classes@^0.1.0: version "0.1.1" resolved "https://registry.yarnpkg.com/posix-character-classes/-/posix-character-classes-0.1.1.tgz#01eac0fe3b5af71a2a6c02feabb8c1fef7e00eab" integrity sha1-AerA/jta9xoqbAL+q7jB/vfgDqs= prelude-ls@~1.1.2: version "1.1.2" resolved "https://registry.yarnpkg.com/prelude-ls/-/prelude-ls-1.1.2.tgz#21932a549f5e52ffd9a827f570e04be62a97da54" integrity sha1-IZMqVJ9eUv/ZqCf1cOBL5iqX2lQ= prettier-linter-helpers@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/prettier-linter-helpers/-/prettier-linter-helpers-1.0.0.tgz#d23d41fe1375646de2d0104d3454a3008802cf7b" integrity sha512-GbK2cP9nraSSUF9N2XwUwqfzlAFlMNYYl+ShE/V+H8a9uNl/oUqB1w2EL54Jh0OlyRSd8RfWYJ3coVS4TROP2w== dependencies: fast-diff "^1.1.2" prettier@^1.16.4: version "1.17.0" resolved "https://registry.yarnpkg.com/prettier/-/prettier-1.17.0.tgz#53b303676eed22cc14a9f0cec09b477b3026c008" integrity sha512-sXe5lSt2WQlCbydGETgfm1YBShgOX4HxQkFPvbxkcwgDvGDeqVau8h+12+lmSVlP3rHPz0oavfddSZg/q+Szjw== private@^0.1.6, private@^0.1.8: version "0.1.8" resolved "https://registry.yarnpkg.com/private/-/private-0.1.8.tgz#2381edb3689f7a53d653190060fcf822d2f368ff" integrity sha512-VvivMrbvd2nKkiG38qjULzlc+4Vx4wm/whI9pQD35YrARNnhxeiRktSOhSukRLFNlzg6Br/cJPet5J/u19r/mg== process-nextick-args@~2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/process-nextick-args/-/process-nextick-args-2.0.0.tgz#a37d732f4271b4ab1ad070d35508e8290788ffaa" integrity sha512-MtEC1TqN0EU5nephaJ4rAtThHtC86dNN9qCuEhtshvpVBkAW5ZO7BASN9REnF9eoXGcRub+pFuKEpOHE+HbEMw== process@^0.10.0: version "0.10.1" resolved "https://registry.yarnpkg.com/process/-/process-0.10.1.tgz#842457cc51cfed72dc775afeeafb8c6034372725" integrity sha1-hCRXzFHP7XLcd1r+6vuMYDQ3JyU= progress@^2.0.0: version "2.0.3" resolved "https://registry.yarnpkg.com/progress/-/progress-2.0.3.tgz#7e8cf8d8f5b8f239c1bc68beb4eb78567d572ef8" integrity sha512-7PiHtLll5LdnKIMw100I+8xJXR5gW2QwWYkT6iJva0bXitZKa/XMrSbdmg3r2Xnaidz9Qumd0VPaMrZlF9V9sA== prop-types@^15.6.2: version "15.7.2" resolved "https://registry.yarnpkg.com/prop-types/-/prop-types-15.7.2.tgz#52c41e75b8c87e72b9d9360e0206b99dcbffa6c5" integrity sha512-8QQikdH7//R2vurIJSutZ1smHYTcLpRWEOlHnzcWHmBYrOGUysKwSsrC89BCiFj3CbrfJ/nXFdJepOVrY1GCHQ== dependencies: loose-envify "^1.4.0" object-assign "^4.1.1" react-is "^16.8.1" property-expr@^1.5.0: version "1.5.1" resolved "https://registry.yarnpkg.com/property-expr/-/property-expr-1.5.1.tgz#22e8706894a0c8e28d58735804f6ba3a3673314f" integrity sha512-CGuc0VUTGthpJXL36ydB6jnbyOf/rAHFvmVrJlH+Rg0DqqLFQGAP6hIaxD/G0OAmBJPhXDHuEJigrp0e0wFV6g== pseudomap@^1.0.2: version "1.0.2" resolved "https://registry.yarnpkg.com/pseudomap/-/pseudomap-1.0.2.tgz#f052a28da70e618917ef0a8ac34c1ae5a68286b3" integrity sha1-8FKijacOYYkX7wqKw0wa5aaChrM= psl@^1.1.24: version "1.1.31" resolved "https://registry.yarnpkg.com/psl/-/psl-1.1.31.tgz#e9aa86d0101b5b105cbe93ac6b784cd547276184" integrity sha512-/6pt4+C+T+wZUieKR620OpzN/LlnNKuWjy1iFLQ/UG35JqHlR/89MP1d96dUfkf6Dne3TuLQzOYEYshJ+Hx8mw== pump@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/pump/-/pump-3.0.0.tgz#b4a2116815bde2f4e1ea602354e8c75565107a64" integrity sha512-LwZy+p3SFs1Pytd/jYct4wpv49HiYCqd9Rlc5ZVdk0V+8Yzv6jR5Blk3TRmPL1ft69TxP0IMZGJ+WPFU2BFhww== dependencies: end-of-stream "^1.1.0" once "^1.3.1" punycode@^1.3.2, punycode@^1.4.1: version "1.4.1" resolved "https://registry.yarnpkg.com/punycode/-/punycode-1.4.1.tgz#c0d5a63b2718800ad8e1eb0fa5269c84dd41845e" integrity sha1-wNWmOycYgArY4esPpSachN1BhF4= punycode@^2.0.0, punycode@^2.1.0: version "2.1.1" resolved "https://registry.yarnpkg.com/punycode/-/punycode-2.1.1.tgz#b58b010ac40c22c5657616c8d2c2c02c7bf479ec" integrity sha512-XRsRjdf+j5ml+y/6GKHPZbrF/8p2Yga0JPtdqTIY2Xe5ohJPD9saDJJLPvp9+NSBprVvevdXZybnj2cv8OEd0A== qs@~6.5.2: version "6.5.2" resolved "https://registry.yarnpkg.com/qs/-/qs-6.5.2.tgz#cb3ae806e8740444584ef154ce8ee98d403f3e36" integrity sha512-N5ZAX4/LxJmF+7wN74pUD6qAh9/wnvdQcjq9TZjevvXzSUo7bfmw91saqMjzGS2xq91/odN2dW/WOl7qQHNDGA== rc@^1.2.7: version "1.2.8" resolved "https://registry.yarnpkg.com/rc/-/rc-1.2.8.tgz#cd924bf5200a075b83c188cd6b9e211b7fc0d3ed" integrity sha512-y3bGgqKj3QBdxLbLkomlohkvsA8gdAiUQlSBJnBhfn+BPxg4bc62d8TcBW15wavDfgexCgccckhcZvywyQYPOw== dependencies: deep-extend "^0.6.0" ini "~1.3.0" minimist "^1.2.0" strip-json-comments "~2.0.1" react-is@^16.8.1: version "16.8.6" resolved "https://registry.yarnpkg.com/react-is/-/react-is-16.8.6.tgz#5bbc1e2d29141c9fbdfed456343fe2bc430a6a16" integrity sha512-aUk3bHfZ2bRSVFFbbeVS4i+lNPZr3/WM5jT2J5omUVV1zzcs1nAaf3l51ctA5FFvCRbhrH0bdAsRRQddFJZPtA== react-reconciler@^0.20.0: version "0.20.4" resolved "https://registry.yarnpkg.com/react-reconciler/-/react-reconciler-0.20.4.tgz#3da6a95841592f849cb4edd3d38676c86fd920b2" integrity sha512-kxERc4H32zV2lXMg/iMiwQHOtyqf15qojvkcZ5Ja2CPkjVohHw9k70pdDBwrnQhLVetUJBSYyqU3yqrlVTOajA== dependencies: loose-envify "^1.1.0" object-assign "^4.1.1" prop-types "^15.6.2" scheduler "^0.13.6" react@^16.8.6: version "16.8.6" resolved "https://registry.yarnpkg.com/react/-/react-16.8.6.tgz#ad6c3a9614fd3a4e9ef51117f54d888da01f2bbe" integrity sha512-pC0uMkhLaHm11ZSJULfOBqV4tIZkx87ZLvbbQYunNixAAvjnC+snJCg0XQXn9VIsttVsbZP/H/ewzgsd5fxKXw== dependencies: loose-envify "^1.1.0" object-assign "^4.1.1" prop-types "^15.6.2" scheduler "^0.13.6" read-pkg-up@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/read-pkg-up/-/read-pkg-up-2.0.0.tgz#6b72a8048984e0c41e79510fd5e9fa99b3b549be" integrity sha1-a3KoBImE4MQeeVEP1en6mbO1Sb4= dependencies: find-up "^2.0.0" read-pkg "^2.0.0" read-pkg-up@^4.0.0: version "4.0.0" resolved "https://registry.yarnpkg.com/read-pkg-up/-/read-pkg-up-4.0.0.tgz#1b221c6088ba7799601c808f91161c66e58f8978" integrity sha512-6etQSH7nJGsK0RbG/2TeDzZFa8shjQ1um+SwQQ5cwKy0dhSXdOncEhb1CPpvQG4h7FyOV6EB6YlV0yJvZQNAkA== dependencies: find-up "^3.0.0" read-pkg "^3.0.0" read-pkg-up@^5.0.0: version "5.0.0" resolved "https://registry.yarnpkg.com/read-pkg-up/-/read-pkg-up-5.0.0.tgz#b6a6741cb144ed3610554f40162aa07a6db621b8" integrity sha512-XBQjqOBtTzyol2CpsQOw8LHV0XbDZVG7xMMjmXAJomlVY03WOBRmYgDJETlvcg0H63AJvPRwT7GFi5rvOzUOKg== dependencies: find-up "^3.0.0" read-pkg "^5.0.0" read-pkg@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/read-pkg/-/read-pkg-2.0.0.tgz#8ef1c0623c6a6db0dc6713c4bfac46332b2368f8" integrity sha1-jvHAYjxqbbDcZxPEv6xGMysjaPg= dependencies: load-json-file "^2.0.0" normalize-package-data "^2.3.2" path-type "^2.0.0" read-pkg@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/read-pkg/-/read-pkg-3.0.0.tgz#9cbc686978fee65d16c00e2b19c237fcf6e38389" integrity sha1-nLxoaXj+5l0WwA4rGcI3/Pbjg4k= dependencies: load-json-file "^4.0.0" normalize-package-data "^2.3.2" path-type "^3.0.0" read-pkg@^5.0.0: version "5.1.1" resolved "https://registry.yarnpkg.com/read-pkg/-/read-pkg-5.1.1.tgz#5cf234dde7a405c90c88a519ab73c467e9cb83f5" integrity sha512-dFcTLQi6BZ+aFUaICg7er+/usEoqFdQxiEBsEMNGoipenihtxxtdrQuBXvyANCEI8VuUIVYFgeHGx9sLLvim4w== dependencies: "@types/normalize-package-data" "^2.4.0" normalize-package-data "^2.5.0" parse-json "^4.0.0" type-fest "^0.4.1" readable-stream@^2.0.2, readable-stream@^2.0.6, readable-stream@^2.1.5: version "2.3.6" resolved "https://registry.yarnpkg.com/readable-stream/-/readable-stream-2.3.6.tgz#b11c27d88b8ff1fbe070643cf94b0c79ae1b0aaf" integrity sha512-tQtKA9WIAhBF3+VLAseyMqZeBjW0AHJoxOtYqSUZNJxauErmLbVm2FW1y+J/YA9dUrAC39ITejlZWhVIwawkKw== dependencies: core-util-is "~1.0.0" inherits "~2.0.3" isarray "~1.0.0" process-nextick-args "~2.0.0" safe-buffer "~5.1.1" string_decoder "~1.1.1" util-deprecate "~1.0.1" readdirp@^2.2.1: version "2.2.1" resolved "https://registry.yarnpkg.com/readdirp/-/readdirp-2.2.1.tgz#0e87622a3325aa33e892285caf8b4e846529a525" integrity sha512-1JU/8q+VgFZyxwrJ+SVIOsh+KywWGpds3NTqikiKpDMZWScmAYyKIgqkO+ARvNWJfXeXR1zxz7aHF4u4CyH6vQ== dependencies: graceful-fs "^4.1.11" micromatch "^3.1.10" readable-stream "^2.0.2" redeyed@~2.1.0: version "2.1.1" resolved "https://registry.yarnpkg.com/redeyed/-/redeyed-2.1.1.tgz#8984b5815d99cb220469c99eeeffe38913e6cc0b" integrity sha1-iYS1gV2ZyyIEacme7v/jiRPmzAs= dependencies: esprima "~4.0.0" regenerate-unicode-properties@^8.0.2: version "8.0.2" resolved "https://registry.yarnpkg.com/regenerate-unicode-properties/-/regenerate-unicode-properties-8.0.2.tgz#7b38faa296252376d363558cfbda90c9ce709662" integrity sha512-SbA/iNrBUf6Pv2zU8Ekv1Qbhv92yxL4hiDa2siuxs4KKn4oOoMDHXjAf7+Nz9qinUQ46B1LcWEi/PhJfPWpZWQ== dependencies: regenerate "^1.4.0" regenerate@^1.4.0: version "1.4.0" resolved "https://registry.yarnpkg.com/regenerate/-/regenerate-1.4.0.tgz#4a856ec4b56e4077c557589cae85e7a4c8869a11" integrity sha512-1G6jJVDWrt0rK99kBjvEtziZNCICAuvIPkSiUFIQxVP06RCVpq3dmDo2oi6ABpYaDYaTRr67BEhL8r1wgEZZKg== regenerator-runtime@^0.11.0: version "0.11.1" resolved "https://registry.yarnpkg.com/regenerator-runtime/-/regenerator-runtime-0.11.1.tgz#be05ad7f9bf7d22e056f9726cee5017fbf19e2e9" integrity sha512-MguG95oij0fC3QV3URf4V2SDYGJhJnJGqvIIgdECeODCT98wSWDAJ94SSuVpYQUoTcGUIL6L4yNB7j1DFFHSBg== regenerator-runtime@^0.13.2: version "0.13.2" resolved "https://registry.yarnpkg.com/regenerator-runtime/-/regenerator-runtime-0.13.2.tgz#32e59c9a6fb9b1a4aff09b4930ca2d4477343447" integrity sha512-S/TQAZJO+D3m9xeN1WTI8dLKBBiRgXBlTJvbWjCThHWZj9EvHK70Ff50/tYj2J/fvBY6JtFVwRuazHN2E7M9BA== regenerator-transform@^0.13.4: version "0.13.4" resolved "https://registry.yarnpkg.com/regenerator-transform/-/regenerator-transform-0.13.4.tgz#18f6763cf1382c69c36df76c6ce122cc694284fb" integrity sha512-T0QMBjK3J0MtxjPmdIMXm72Wvj2Abb0Bd4HADdfijwMdoIsyQZ6fWC7kDFhk2YinBBEMZDL7Y7wh0J1sGx3S4A== dependencies: private "^0.1.6" regex-not@^1.0.0, regex-not@^1.0.2: version "1.0.2" resolved "https://registry.yarnpkg.com/regex-not/-/regex-not-1.0.2.tgz#1f4ece27e00b0b65e0247a6810e6a85d83a5752c" integrity sha512-J6SDjUgDxQj5NusnOtdFxDwN/+HWykR8GELwctJ7mdqhcyy1xEc4SRFHUXvxTp661YaVKAjfRLZ9cCqS6tn32A== dependencies: extend-shallow "^3.0.2" safe-regex "^1.1.0" regexp-tree@^0.1.0: version "0.1.6" resolved "https://registry.yarnpkg.com/regexp-tree/-/regexp-tree-0.1.6.tgz#84900fa12fdf428a2ac25f04300382a7c0148479" integrity sha512-LFrA98Dw/heXqDojz7qKFdygZmFoiVlvE1Zp7Cq2cvF+ZA+03Gmhy0k0PQlsC1jvHPiTUSs+pDHEuSWv6+6D7w== regexpp@^2.0.1: version "2.0.1" resolved "https://registry.yarnpkg.com/regexpp/-/regexpp-2.0.1.tgz#8d19d31cf632482b589049f8281f93dbcba4d07f" integrity sha512-lv0M6+TkDVniA3aD1Eg0DVpfU/booSu7Eev3TDO/mZKHBfVjgCGTV4t4buppESEYDtkArYFOxTJWv6S5C+iaNw== regexpu-core@^4.5.4: version "4.5.4" resolved "https://registry.yarnpkg.com/regexpu-core/-/regexpu-core-4.5.4.tgz#080d9d02289aa87fe1667a4f5136bc98a6aebaae" integrity sha512-BtizvGtFQKGPUcTy56o3nk1bGRp4SZOTYrDtGNlqCQufptV5IkkLN6Emw+yunAJjzf+C9FQFtvq7IoA3+oMYHQ== dependencies: regenerate "^1.4.0" regenerate-unicode-properties "^8.0.2" regjsgen "^0.5.0" regjsparser "^0.6.0" unicode-match-property-ecmascript "^1.0.4" unicode-match-property-value-ecmascript "^1.1.0" regjsgen@^0.5.0: version "0.5.0" resolved "https://registry.yarnpkg.com/regjsgen/-/regjsgen-0.5.0.tgz#a7634dc08f89209c2049adda3525711fb97265dd" integrity sha512-RnIrLhrXCX5ow/E5/Mh2O4e/oa1/jW0eaBKTSy3LaCj+M3Bqvm97GWDp2yUtzIs4LEn65zR2yiYGFqb2ApnzDA== regjsparser@^0.6.0: version "0.6.0" resolved "https://registry.yarnpkg.com/regjsparser/-/regjsparser-0.6.0.tgz#f1e6ae8b7da2bae96c99399b868cd6c933a2ba9c" integrity sha512-RQ7YyokLiQBomUJuUG8iGVvkgOLxwyZM8k6d3q5SAXpg4r5TZJZigKFvC6PpD+qQ98bCDC5YelPeA3EucDoNeQ== dependencies: jsesc "~0.5.0" release-zalgo@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/release-zalgo/-/release-zalgo-1.0.0.tgz#09700b7e5074329739330e535c5a90fb67851730" integrity sha1-CXALflB0Mpc5Mw5TXFqQ+2eFFzA= dependencies: es6-error "^4.0.1" remove-trailing-separator@^1.0.1: version "1.1.0" resolved "https://registry.yarnpkg.com/remove-trailing-separator/-/remove-trailing-separator-1.1.0.tgz#c24bce2a283adad5bc3f58e0d48249b92379d8ef" integrity sha1-wkvOKig62tW8P1jg1IJJuSN52O8= repeat-element@^1.1.2: version "1.1.3" resolved "https://registry.yarnpkg.com/repeat-element/-/repeat-element-1.1.3.tgz#782e0d825c0c5a3bb39731f84efee6b742e6b1ce" integrity sha512-ahGq0ZnV5m5XtZLMb+vP76kcAM5nkLqk0lpqAuojSKGgQtn4eRi4ZZGm2olo2zKFH+sMsWaqOCW1dqAnOru72g== repeat-string@^1.6.1: version "1.6.1" resolved "https://registry.yarnpkg.com/repeat-string/-/repeat-string-1.6.1.tgz#8dcae470e1c88abc2d600fff4a776286da75e637" integrity sha1-jcrkcOHIirwtYA//Sndihtp15jc= repeating@^2.0.0: version "2.0.1" resolved "https://registry.yarnpkg.com/repeating/-/repeating-2.0.1.tgz#5214c53a926d3552707527fbab415dbc08d06dda" integrity sha1-UhTFOpJtNVJwdSf7q0FdvAjQbdo= dependencies: is-finite "^1.0.0" request@^2.86.0: version "2.88.0" resolved "https://registry.yarnpkg.com/request/-/request-2.88.0.tgz#9c2fca4f7d35b592efe57c7f0a55e81052124fef" integrity sha512-NAqBSrijGLZdM0WZNsInLJpkJokL72XYjUpnB0iwsRgxh7dB6COrHnTBNwN0E+lHDAJzu7kLAkDeY08z2/A0hg== dependencies: aws-sign2 "~0.7.0" aws4 "^1.8.0" caseless "~0.12.0" combined-stream "~1.0.6" extend "~3.0.2" forever-agent "~0.6.1" form-data "~2.3.2" har-validator "~5.1.0" http-signature "~1.2.0" is-typedarray "~1.0.0" isstream "~0.1.2" json-stringify-safe "~5.0.1" mime-types "~2.1.19" oauth-sign "~0.9.0" performance-now "^2.1.0" qs "~6.5.2" safe-buffer "^5.1.2" tough-cookie "~2.4.3" tunnel-agent "^0.6.0" uuid "^3.3.2" require-directory@^2.1.1: version "2.1.1" resolved "https://registry.yarnpkg.com/require-directory/-/require-directory-2.1.1.tgz#8c64ad5fd30dab1c976e2344ffe7f792a6a6df42" integrity sha1-jGStX9MNqxyXbiNE/+f3kqam30I= require-main-filename@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/require-main-filename/-/require-main-filename-2.0.0.tgz#d0b329ecc7cc0f61649f62215be69af54aa8989b" integrity sha512-NKN5kMDylKuldxYLSUfrbo5Tuzh4hd+2E8NPPX02mZtn1VuREQToYe/ZdlJy+J3uCpfaiGF05e7B8W0iXbQHmg== resolve-from@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/resolve-from/-/resolve-from-3.0.0.tgz#b22c7af7d9d6881bc8b6e653335eebcb0a188748" integrity sha1-six699nWiBvItuZTM17rywoYh0g= resolve-from@^4.0.0: version "4.0.0" resolved "https://registry.yarnpkg.com/resolve-from/-/resolve-from-4.0.0.tgz#4abcd852ad32dd7baabfe9b40e00a36db5f392e6" integrity sha512-pb/MYmXstAkysRFx8piNI1tGFNQIFA3vkE3Gq4EuA1dF6gHp/+vgZqsCGJapvy8N3Q+4o7FwvquPJcnZ7RYy4g== resolve-url@^0.2.1: version "0.2.1" resolved "https://registry.yarnpkg.com/resolve-url/-/resolve-url-0.2.1.tgz#2c637fe77c893afd2a663fe21aa9080068e2052a" integrity sha1-LGN/53yJOv0qZj/iGqkIAGjiBSo= resolve@^1.10.0, resolve@^1.10.1: version "1.10.1" resolved "https://registry.yarnpkg.com/resolve/-/resolve-1.10.1.tgz#664842ac960795bbe758221cdccda61fb64b5f18" integrity sha512-KuIe4mf++td/eFb6wkaPbMDnP6kObCaEtIDuHOUED6MNUo4K670KZUHuuvYPZDxNF0WVLw49n06M2m2dXphEzA== dependencies: path-parse "^1.0.6" resolve@^1.3.2, resolve@^1.5.0, resolve@^1.6.0: version "1.9.0" resolved "https://registry.yarnpkg.com/resolve/-/resolve-1.9.0.tgz#a14c6fdfa8f92a7df1d996cb7105fa744658ea06" integrity sha512-TZNye00tI67lwYvzxCxHGjwTNlUV70io54/Ed4j6PscB8xVfuBJpRenI/o6dVk0cY0PYTY27AgCoGGxRnYuItQ== dependencies: path-parse "^1.0.6" restore-cursor@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/restore-cursor/-/restore-cursor-2.0.0.tgz#9f7ee287f82fd326d4fd162923d62129eee0dfaf" integrity sha1-n37ih/gv0ybU/RYpI9YhKe7g368= dependencies: onetime "^2.0.0" signal-exit "^3.0.2" ret@~0.1.10: version "0.1.15" resolved "https://registry.yarnpkg.com/ret/-/ret-0.1.15.tgz#b8a4825d5bdb1fc3f6f53c2bc33f81388681c7bc" integrity sha512-TTlYpa+OL+vMMNG24xSlQGEJ3B/RzEfUlLct7b5G/ytav+wPrplCpVMFuwzXbkecJrb6IYo1iFb0S9v37754mg== rimraf@2.6.3, rimraf@^2.2.8, rimraf@^2.6.1, rimraf@^2.6.2, rimraf@^2.6.3: version "2.6.3" resolved "https://registry.yarnpkg.com/rimraf/-/rimraf-2.6.3.tgz#b2d104fe0d8fb27cf9e0a1cda8262dd3833c6cab" integrity sha512-mwqeW5XsA2qAejG46gYdENaxXjx9onRNCfn7L0duuP4hCuTIi/QO7PDK07KJfp1d+izWPrzEJDcSqBa0OZQriA== dependencies: glob "^7.1.3" run-async@^2.2.0: version "2.3.0" resolved "https://registry.yarnpkg.com/run-async/-/run-async-2.3.0.tgz#0371ab4ae0bdd720d4166d7dfda64ff7a445a6c0" integrity sha1-A3GrSuC91yDUFm19/aZP96RFpsA= dependencies: is-promise "^2.1.0" run-node@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/run-node/-/run-node-1.0.0.tgz#46b50b946a2aa2d4947ae1d886e9856fd9cabe5e" integrity sha512-kc120TBlQ3mih1LSzdAJXo4xn/GWS2ec0l3S+syHDXP9uRr0JAT8Qd3mdMuyjqCzeZktgP3try92cEgf9Nks8A== rxjs@^6.3.3: version "6.3.3" resolved "https://registry.yarnpkg.com/rxjs/-/rxjs-6.3.3.tgz#3c6a7fa420e844a81390fb1158a9ec614f4bad55" integrity sha512-JTWmoY9tWCs7zvIk/CvRjhjGaOd+OVBM987mxFo+OW66cGpdKjZcpmc74ES1sB//7Kl/PAe8+wEakuhG4pcgOw== dependencies: tslib "^1.9.0" rxjs@^6.4.0: version "6.4.0" resolved "https://registry.yarnpkg.com/rxjs/-/rxjs-6.4.0.tgz#f3bb0fe7bda7fb69deac0c16f17b50b0b8790504" integrity sha512-Z9Yfa11F6B9Sg/BK9MnqnQ+aQYicPLtilXBp2yUtDt2JRCE0h26d33EnfO3ZxoNxG0T92OUucP3Ct7cpfkdFfw== dependencies: tslib "^1.9.0" safe-buffer@^5.0.1, safe-buffer@^5.1.2, safe-buffer@~5.1.0, safe-buffer@~5.1.1: version "5.1.2" resolved "https://registry.yarnpkg.com/safe-buffer/-/safe-buffer-5.1.2.tgz#991ec69d296e0313747d59bdfd2b745c35f8828d" integrity sha512-Gd2UZBJDkXlY7GbJxfsE8/nvKkUEU1G38c1siN6QP6a9PT9MmHB8GnpscSmMJSoF8LOIrt8ud/wPtojys4G6+g== safe-regex@^1.1.0: version "1.1.0" resolved "https://registry.yarnpkg.com/safe-regex/-/safe-regex-1.1.0.tgz#40a3669f3b077d1e943d44629e157dd48023bf2e" integrity sha1-QKNmnzsHfR6UPURinhV91IAjvy4= dependencies: ret "~0.1.10" "safer-buffer@>= 2.1.2 < 3", safer-buffer@^2.0.2, safer-buffer@^2.1.0, safer-buffer@~2.1.0: version "2.1.2" resolved "https://registry.yarnpkg.com/safer-buffer/-/safer-buffer-2.1.2.tgz#44fa161b0187b9549dd84bb91802f9bd8385cd6a" integrity sha512-YZo3K82SD7Riyi0E1EQPojLz7kpepnSQI9IyPbHHg1XXXevb5dJI7tpyN2ADxGcQbHG7vcyRHk0cbwqcQriUtg== sax@^1.2.4: version "1.2.4" resolved "https://registry.yarnpkg.com/sax/-/sax-1.2.4.tgz#2816234e2378bddc4e5354fab5caa895df7100d9" integrity sha512-NqVDv9TpANUjFm0N8uM5GxL36UgKi9/atZw+x7YFnQ8ckwFGKrl4xX4yWtrey3UJm5nP1kUbnYgLopqWNSRhWw== scheduler@^0.13.2, scheduler@^0.13.6: version "0.13.6" resolved "https://registry.yarnpkg.com/scheduler/-/scheduler-0.13.6.tgz#466a4ec332467b31a91b9bf74e5347072e4cd889" integrity sha512-IWnObHt413ucAYKsD9J1QShUKkbKLQQHdxRyw73sw4FN26iWr3DY/H34xGPe4nmL1DwXyWmSWmMrA9TfQbE/XQ== dependencies: loose-envify "^1.1.0" object-assign "^4.1.1" semver-compare@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/semver-compare/-/semver-compare-1.0.0.tgz#0dee216a1c941ab37e9efb1788f6afc5ff5537fc" integrity sha1-De4hahyUGrN+nvsXiPavxf9VN/w= "semver@2 || 3 || 4 || 5", semver@^5.3.0, semver@^5.4.1, semver@^5.5.0, semver@^5.5.1: version "5.6.0" resolved "https://registry.yarnpkg.com/semver/-/semver-5.6.0.tgz#7e74256fbaa49c75aa7c7a205cc22799cac80004" integrity sha512-RS9R6R35NYgQn++fkDWaOmqGoj4Ek9gGs+DPxNUZKuwE183xjJroKvyo1IzVFeXvUrvmALy6FWD5xrdJT25gMg== semver@^5.6.0: version "5.7.0" resolved "https://registry.yarnpkg.com/semver/-/semver-5.7.0.tgz#790a7cf6fea5459bac96110b29b60412dc8ff96b" integrity sha512-Ya52jSX2u7QKghxeoFGpLwCtGlt7j0oY9DYb5apt9nPlJ42ID+ulTXESnt/qAQcoSERyZ5sl3LDIOw0nAn/5DA== semver@^6.0.0: version "6.0.0" resolved "https://registry.yarnpkg.com/semver/-/semver-6.0.0.tgz#05e359ee571e5ad7ed641a6eec1e547ba52dea65" integrity sha512-0UewU+9rFapKFnlbirLi3byoOuhrSsli/z/ihNnvM24vgF+8sNBiI1LZPBSH9wJKUwaUbw+s3hToDLCXkrghrQ== set-blocking@^2.0.0, set-blocking@~2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/set-blocking/-/set-blocking-2.0.0.tgz#045f9782d011ae9a6803ddd382b24392b3d890f7" integrity sha1-BF+XgtARrppoA93TgrJDkrPYkPc= set-value@^0.4.3: version "0.4.3" resolved "https://registry.yarnpkg.com/set-value/-/set-value-0.4.3.tgz#7db08f9d3d22dc7f78e53af3c3bf4666ecdfccf1" integrity sha1-fbCPnT0i3H945Trzw79GZuzfzPE= dependencies: extend-shallow "^2.0.1" is-extendable "^0.1.1" is-plain-object "^2.0.1" to-object-path "^0.3.0" set-value@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/set-value/-/set-value-2.0.0.tgz#71ae4a88f0feefbbf52d1ea604f3fb315ebb6274" integrity sha512-hw0yxk9GT/Hr5yJEYnHNKYXkIA8mVJgd9ditYZCe16ZczcaELYYcfvaXesNACk2O8O0nTiPQcQhGUQj8JLzeeg== dependencies: extend-shallow "^2.0.1" is-extendable "^0.1.1" is-plain-object "^2.0.3" split-string "^3.0.1" shebang-command@^1.2.0: version "1.2.0" resolved "https://registry.yarnpkg.com/shebang-command/-/shebang-command-1.2.0.tgz#44aac65b695b03398968c39f363fee5deafdf1ea" integrity sha1-RKrGW2lbAzmJaMOfNj/uXer98eo= dependencies: shebang-regex "^1.0.0" shebang-regex@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/shebang-regex/-/shebang-regex-1.0.0.tgz#da42f49740c0b42db2ca9728571cb190c98efea3" integrity sha1-2kL0l0DAtC2yypcoVxyxkMmO/qM= signal-exit@^3.0.0, signal-exit@^3.0.2: version "3.0.2" resolved "https://registry.yarnpkg.com/signal-exit/-/signal-exit-3.0.2.tgz#b5fdc08f1287ea1178628e415e25132b73646c6d" integrity sha1-tf3AjxKH6hF4Yo5BXiUTK3NkbG0= simple-git@^1.85.0: version "1.107.0" resolved "https://registry.yarnpkg.com/simple-git/-/simple-git-1.107.0.tgz#12cffaf261c14d6f450f7fdb86c21ccee968b383" integrity sha512-t4OK1JRlp4ayKRfcW6owrWcRVLyHRUlhGd0uN6ZZTqfDq8a5XpcUdOKiGRNobHEuMtNqzp0vcJNvhYWwh5PsQA== dependencies: debug "^4.0.1" slash@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/slash/-/slash-1.0.0.tgz#c41f2f6c39fc16d1cd17ad4b5d896114ae470d55" integrity sha1-xB8vbDn8FtHNF61LXYlhFK5HDVU= slash@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/slash/-/slash-2.0.0.tgz#de552851a1759df3a8f206535442f5ec4ddeab44" integrity sha512-ZYKh3Wh2z1PpEXWr0MpSBZ0V6mZHAQfYevttO11c51CaWjGTaadiKZ+wVt1PbMlDV5qhMFslpZCemhwOK7C89A== slice-ansi@0.0.4: version "0.0.4" resolved "https://registry.yarnpkg.com/slice-ansi/-/slice-ansi-0.0.4.tgz#edbf8903f66f7ce2f8eafd6ceed65e264c831b35" integrity sha1-7b+JA/ZvfOL46v1s7tZeJkyDGzU= slice-ansi@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/slice-ansi/-/slice-ansi-1.0.0.tgz#044f1a49d8842ff307aad6b505ed178bd950134d" integrity sha512-POqxBK6Lb3q6s047D/XsDVNPnF9Dl8JSaqe9h9lURl0OdNqy/ujDrOiIHtsqXMGbWWTIomRzAMaTyawAU//Reg== dependencies: is-fullwidth-code-point "^2.0.0" slice-ansi@^2.1.0: version "2.1.0" resolved "https://registry.yarnpkg.com/slice-ansi/-/slice-ansi-2.1.0.tgz#cacd7693461a637a5788d92a7dd4fba068e81636" integrity sha512-Qu+VC3EwYLldKa1fCxuuvULvSJOKEgk9pi8dZeCVK7TqBfUNTH4sFkk4joj8afVSfAYgJoSOetjx9QWOJ5mYoQ== dependencies: ansi-styles "^3.2.0" astral-regex "^1.0.0" is-fullwidth-code-point "^2.0.0" snapdragon-node@^2.0.1: version "2.1.1" resolved "https://registry.yarnpkg.com/snapdragon-node/-/snapdragon-node-2.1.1.tgz#6c175f86ff14bdb0724563e8f3c1b021a286853b" integrity sha512-O27l4xaMYt/RSQ5TR3vpWCAB5Kb/czIcqUFOM/C4fYcLnbZUc1PkjTAMjof2pBWaSTwOUd6qUHcFGVGj7aIwnw== dependencies: define-property "^1.0.0" isobject "^3.0.0" snapdragon-util "^3.0.1" snapdragon-util@^3.0.1: version "3.0.1" resolved "https://registry.yarnpkg.com/snapdragon-util/-/snapdragon-util-3.0.1.tgz#f956479486f2acd79700693f6f7b805e45ab56e2" integrity sha512-mbKkMdQKsjX4BAL4bRYTj21edOf8cN7XHdYUJEe+Zn99hVEYcMvKPct1IqNe7+AZPirn8BCDOQBHQZknqmKlZQ== dependencies: kind-of "^3.2.0" snapdragon@^0.8.1: version "0.8.2" resolved "https://registry.yarnpkg.com/snapdragon/-/snapdragon-0.8.2.tgz#64922e7c565b0e14204ba1aa7d6964278d25182d" integrity sha512-FtyOnWN/wCHTVXOMwvSv26d+ko5vWlIDD6zoUJ7LW8vh+ZBC8QdljveRP+crNrtBwioEUWy/4dMtbBjA4ioNlg== dependencies: base "^0.11.1" debug "^2.2.0" define-property "^0.2.5" extend-shallow "^2.0.1" map-cache "^0.2.2" source-map "^0.5.6" source-map-resolve "^0.5.0" use "^3.1.0" source-map-resolve@^0.5.0: version "0.5.2" resolved "https://registry.yarnpkg.com/source-map-resolve/-/source-map-resolve-0.5.2.tgz#72e2cc34095543e43b2c62b2c4c10d4a9054f259" integrity sha512-MjqsvNwyz1s0k81Goz/9vRBe9SZdB09Bdw+/zYyO+3CuPk6fouTaxscHkgtE8jKvf01kVfl8riHzERQ/kefaSA== dependencies: atob "^2.1.1" decode-uri-component "^0.2.0" resolve-url "^0.2.1" source-map-url "^0.4.0" urix "^0.1.0" source-map-support@^0.4.15: version "0.4.18" resolved "https://registry.yarnpkg.com/source-map-support/-/source-map-support-0.4.18.tgz#0286a6de8be42641338594e97ccea75f0a2c585f" integrity sha512-try0/JqxPLF9nOjvSta7tVondkP5dwgyLDjVoyMDlmjugT2lRZ1OfsrYTkCd2hkDnJTKRbO/Rl3orm8vlsUzbA== dependencies: source-map "^0.5.6" source-map-support@^0.5.11, source-map-support@^0.5.12, source-map-support@^0.5.6: version "0.5.12" resolved "https://registry.yarnpkg.com/source-map-support/-/source-map-support-0.5.12.tgz#b4f3b10d51857a5af0138d3ce8003b201613d599" integrity sha512-4h2Pbvyy15EE02G+JOZpUCmqWJuqrs+sEkzewTm++BPi7Hvn/HwcqLAcNxYAyI0x13CpPPn+kMjl+hplXMHITQ== dependencies: buffer-from "^1.0.0" source-map "^0.6.0" source-map-url@^0.4.0: version "0.4.0" resolved "https://registry.yarnpkg.com/source-map-url/-/source-map-url-0.4.0.tgz#3e935d7ddd73631b97659956d55128e87b5084a3" integrity sha1-PpNdfd1zYxuXZZlW1VEo6HtQhKM= source-map@^0.5.0, source-map@^0.5.6, source-map@^0.5.7: version "0.5.7" resolved "https://registry.yarnpkg.com/source-map/-/source-map-0.5.7.tgz#8a039d2d1021d22d1ea14c80d8ea468ba2ef3fcc" integrity sha1-igOdLRAh0i0eoUyA2OpGi6LvP8w= source-map@^0.6.0, source-map@^0.6.1, source-map@~0.6.1: version "0.6.1" resolved "https://registry.yarnpkg.com/source-map/-/source-map-0.6.1.tgz#74722af32e9614e9c287a8d0bbde48b5e2f1a263" integrity sha512-UjgapumWlbMhkBgzT7Ykc5YXUT46F0iKu8SGXq0bcwP5dz/h0Plj6enJqjz1Zbq2l5WaqYnrVbwWOWMyF3F47g== spawn-wrap@^1.4.2: version "1.4.2" resolved "https://registry.yarnpkg.com/spawn-wrap/-/spawn-wrap-1.4.2.tgz#cff58e73a8224617b6561abdc32586ea0c82248c" integrity sha512-vMwR3OmmDhnxCVxM8M+xO/FtIp6Ju/mNaDfCMMW7FDcLRTPFWUswec4LXJHTJE2hwTI9O0YBfygu4DalFl7Ylg== dependencies: foreground-child "^1.5.6" mkdirp "^0.5.0" os-homedir "^1.0.1" rimraf "^2.6.2" signal-exit "^3.0.2" which "^1.3.0" spdx-correct@^3.0.0: version "3.1.0" resolved "https://registry.yarnpkg.com/spdx-correct/-/spdx-correct-3.1.0.tgz#fb83e504445268f154b074e218c87c003cd31df4" integrity sha512-lr2EZCctC2BNR7j7WzJ2FpDznxky1sjfxvvYEyzxNyb6lZXHODmEoJeFu4JupYlkfha1KZpJyoqiJ7pgA1qq8Q== dependencies: spdx-expression-parse "^3.0.0" spdx-license-ids "^3.0.0" spdx-exceptions@^2.1.0: version "2.2.0" resolved "https://registry.yarnpkg.com/spdx-exceptions/-/spdx-exceptions-2.2.0.tgz#2ea450aee74f2a89bfb94519c07fcd6f41322977" integrity sha512-2XQACfElKi9SlVb1CYadKDXvoajPgBVPn/gOQLrTvHdElaVhr7ZEbqJaRnJLVNeaI4cMEAgVCeBMKF6MWRDCRA== spdx-expression-parse@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/spdx-expression-parse/-/spdx-expression-parse-3.0.0.tgz#99e119b7a5da00e05491c9fa338b7904823b41d0" integrity sha512-Yg6D3XpRD4kkOmTpdgbUiEJFKghJH03fiC1OPll5h/0sO6neh2jqRDVHOQ4o/LMea0tgCkbMgea5ip/e+MkWyg== dependencies: spdx-exceptions "^2.1.0" spdx-license-ids "^3.0.0" spdx-license-ids@^3.0.0: version "3.0.3" resolved "https://registry.yarnpkg.com/spdx-license-ids/-/spdx-license-ids-3.0.3.tgz#81c0ce8f21474756148bbb5f3bfc0f36bf15d76e" integrity sha512-uBIcIl3Ih6Phe3XHK1NqboJLdGfwr1UN3k6wSD1dZpmPsIkb8AGNbZYJ1fOBk834+Gxy8rpfDxrS6XLEMZMY2g== split-string@^3.0.1, split-string@^3.0.2: version "3.1.0" resolved "https://registry.yarnpkg.com/split-string/-/split-string-3.1.0.tgz#7cb09dda3a86585705c64b39a6466038682e8fe2" integrity sha512-NzNVhJDYpwceVVii8/Hu6DKfD2G+NrQHlS/V/qgv763EYudVwEcMQNxd2lh+0VrUByXN/oJkl5grOhYWvQUYiw== dependencies: extend-shallow "^3.0.0" sprintf-js@~1.0.2: version "1.0.3" resolved "https://registry.yarnpkg.com/sprintf-js/-/sprintf-js-1.0.3.tgz#04e6926f662895354f3dd015203633b857297e2c" integrity sha1-BOaSb2YolTVPPdAVIDYzuFcpfiw= sshpk@^1.7.0: version "1.16.1" resolved "https://registry.yarnpkg.com/sshpk/-/sshpk-1.16.1.tgz#fb661c0bef29b39db40769ee39fa70093d6f6877" integrity sha512-HXXqVUq7+pcKeLqqZj6mHFUMvXtOJt1uoUx09pFW6011inTMxqI8BA8PM95myrIyyKwdnzjdFjLiE6KBPVtJIg== dependencies: asn1 "~0.2.3" assert-plus "^1.0.0" bcrypt-pbkdf "^1.0.0" dashdash "^1.12.0" ecc-jsbn "~0.1.1" getpass "^0.1.1" jsbn "~0.1.0" safer-buffer "^2.0.2" tweetnacl "~0.14.0" stack-utils@^1.0.2: version "1.0.2" resolved "https://registry.yarnpkg.com/stack-utils/-/stack-utils-1.0.2.tgz#33eba3897788558bebfc2db059dc158ec36cebb8" integrity sha512-MTX+MeG5U994cazkjd/9KNAapsHnibjMLnfXodlkXw76JEea0UiNzrqidzo1emMwk7w5Qhc9jd4Bn9TBb1MFwA== staged-git-files@1.1.2: version "1.1.2" resolved "https://registry.yarnpkg.com/staged-git-files/-/staged-git-files-1.1.2.tgz#4326d33886dc9ecfa29a6193bf511ba90a46454b" integrity sha512-0Eyrk6uXW6tg9PYkhi/V/J4zHp33aNyi2hOCmhFLqLTIhbgqWn5jlSzI+IU0VqrZq6+DbHcabQl/WP6P3BG0QA== static-extend@^0.1.1: version "0.1.2" resolved "https://registry.yarnpkg.com/static-extend/-/static-extend-0.1.2.tgz#60809c39cbff55337226fd5e0b520f341f1fb5c6" integrity sha1-YICcOcv/VTNyJv1eC1IPNB8ftcY= dependencies: define-property "^0.2.5" object-copy "^0.1.0" string-argv@^0.0.2: version "0.0.2" resolved "https://registry.yarnpkg.com/string-argv/-/string-argv-0.0.2.tgz#dac30408690c21f3c3630a3ff3a05877bdcbd736" integrity sha1-2sMECGkMIfPDYwo/86BYd73L1zY= string-length@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/string-length/-/string-length-2.0.0.tgz#d40dbb686a3ace960c1cffca562bf2c45f8363ed" integrity sha1-1A27aGo6zpYMHP/KVivyxF+DY+0= dependencies: astral-regex "^1.0.0" strip-ansi "^4.0.0" string-width@^1.0.1: version "1.0.2" resolved "https://registry.yarnpkg.com/string-width/-/string-width-1.0.2.tgz#118bdf5b8cdc51a2a7e70d211e07e2b0b9b107d3" integrity sha1-EYvfW4zcUaKn5w0hHgfisLmxB9M= dependencies: code-point-at "^1.0.0" is-fullwidth-code-point "^1.0.0" strip-ansi "^3.0.0" "string-width@^1.0.2 || 2", string-width@^2.0.0, string-width@^2.1.0, string-width@^2.1.1: version "2.1.1" resolved "https://registry.yarnpkg.com/string-width/-/string-width-2.1.1.tgz#ab93f27a8dc13d28cac815c462143a6d9012ae9e" integrity sha512-nOqH59deCq9SRHlxq1Aw85Jnt4w6KvLKqWVik6oA9ZklXLNIOlqg4F2yrT1MVaTjAqvVwdfeZ7w7aCvJD7ugkw== dependencies: is-fullwidth-code-point "^2.0.0" strip-ansi "^4.0.0" string-width@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/string-width/-/string-width-3.0.0.tgz#5a1690a57cc78211fffd9bf24bbe24d090604eb1" integrity sha512-rr8CUxBbvOZDUvc5lNIJ+OC1nPVpz+Siw9VBtUjB9b6jZehZLFt0JMCZzShFHIsI8cbhm0EsNIfWJMFV3cu3Ew== dependencies: emoji-regex "^7.0.1" is-fullwidth-code-point "^2.0.0" strip-ansi "^5.0.0" string_decoder@~1.1.1: version "1.1.1" resolved "https://registry.yarnpkg.com/string_decoder/-/string_decoder-1.1.1.tgz#9cf1611ba62685d7030ae9e4ba34149c3af03fc8" integrity sha512-n/ShnvDi6FHbbVfviro+WojiFzv+s8MPMHBczVePfUpDJLwoLT0ht1l4YwBCbi8pJAveEEdnkHyPyTP/mzRfwg== dependencies: safe-buffer "~5.1.0" stringify-object@^3.2.2: version "3.3.0" resolved "https://registry.yarnpkg.com/stringify-object/-/stringify-object-3.3.0.tgz#703065aefca19300d3ce88af4f5b3956d7556629" integrity sha512-rHqiFh1elqCQ9WPLIC8I0Q/g/wj5J1eMkyoiD6eoQApWHP0FtlK7rqnhmabL5VUY9JQCcqwwvlOaSuutekgyrw== dependencies: get-own-enumerable-property-symbols "^3.0.0" is-obj "^1.0.1" is-regexp "^1.0.0" strip-ansi@^3.0.0, strip-ansi@^3.0.1: version "3.0.1" resolved "https://registry.yarnpkg.com/strip-ansi/-/strip-ansi-3.0.1.tgz#6a385fb8853d952d5ff05d0e8aaf94278dc63dcf" integrity sha1-ajhfuIU9lS1f8F0Oiq+UJ43GPc8= dependencies: ansi-regex "^2.0.0" strip-ansi@^4.0.0: version "4.0.0" resolved "https://registry.yarnpkg.com/strip-ansi/-/strip-ansi-4.0.0.tgz#a8479022eb1ac368a871389b635262c505ee368f" integrity sha1-qEeQIusaw2iocTibY1JixQXuNo8= dependencies: ansi-regex "^3.0.0" strip-ansi@^5.0.0: version "5.0.0" resolved "https://registry.yarnpkg.com/strip-ansi/-/strip-ansi-5.0.0.tgz#f78f68b5d0866c20b2c9b8c61b5298508dc8756f" integrity sha512-Uu7gQyZI7J7gn5qLn1Np3G9vcYGTVqB+lFTytnDJv83dd8T22aGH451P3jueT2/QemInJDfxHB5Tde5OzgG1Ow== dependencies: ansi-regex "^4.0.0" strip-bom@^3.0.0: version "3.0.0" resolved "https://registry.yarnpkg.com/strip-bom/-/strip-bom-3.0.0.tgz#2334c18e9c759f7bdd56fdef7e9ae3d588e68ed3" integrity sha1-IzTBjpx1n3vdVv3vfprj1YjmjtM= strip-eof@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/strip-eof/-/strip-eof-1.0.0.tgz#bb43ff5598a6eb05d89b59fcd129c983313606bf" integrity sha1-u0P/VZim6wXYm1n80SnJgzE2Br8= strip-json-comments@^2.0.1, strip-json-comments@~2.0.1: version "2.0.1" resolved "https://registry.yarnpkg.com/strip-json-comments/-/strip-json-comments-2.0.1.tgz#3c531942e908c2697c0ec344858c286c7ca0a60a" integrity sha1-PFMZQukIwml8DsNEhYwobHygpgo= supports-color@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/supports-color/-/supports-color-2.0.0.tgz#535d045ce6b6363fa40117084629995e9df324c7" integrity sha1-U10EXOa2Nj+kARcIRimZXp3zJMc= supports-color@^5.3.0: version "5.5.0" resolved "https://registry.yarnpkg.com/supports-color/-/supports-color-5.5.0.tgz#e2e69a44ac8772f78a1ec0b35b689df6530efc8f" integrity sha512-QjVjwdXIt408MIiAqCX4oUKsgU2EqAGzs2Ppkm4aQYbjm+ZEWEcW4SfFNTr4uMNZma0ey4f5lgLrkB0aX0QMow== dependencies: has-flag "^3.0.0" supports-color@^6.1.0: version "6.1.0" resolved "https://registry.yarnpkg.com/supports-color/-/supports-color-6.1.0.tgz#0764abc69c63d5ac842dd4867e8d025e880df8f3" integrity sha512-qe1jfm1Mg7Nq/NSh6XE24gPXROEVsWHxC1LIx//XNlD9iw7YZQGjZNjYN7xGaEG6iKdA8EtNFW6R0gjnVXp+wQ== dependencies: has-flag "^3.0.0" symbol-observable@^1.1.0: version "1.2.0" resolved "https://registry.yarnpkg.com/symbol-observable/-/symbol-observable-1.2.0.tgz#c22688aed4eab3cdc2dfeacbb561660560a00804" integrity sha512-e900nM8RRtGhlV36KGEU9k65K3mPb1WV70OdjfxlG2EAuM1noi/E/BaW/uMhL7bPEssK8QV57vN3esixjUvcXQ== synchronous-promise@^2.0.6: version "2.0.7" resolved "https://registry.yarnpkg.com/synchronous-promise/-/synchronous-promise-2.0.7.tgz#3574b3d2fae86b145356a4b89103e1577f646fe3" integrity sha512-16GbgwTmFMYFyQMLvtQjvNWh30dsFe1cAW5Fg1wm5+dg84L9Pe36mftsIRU95/W2YsISxsz/xq4VB23sqpgb/A== table@^5.2.3: version "5.2.3" resolved "https://registry.yarnpkg.com/table/-/table-5.2.3.tgz#cde0cc6eb06751c009efab27e8c820ca5b67b7f2" integrity sha512-N2RsDAMvDLvYwFcwbPyF3VmVSSkuF+G1e+8inhBLtHpvwXGw4QRPEZhihQNeEN0i1up6/f6ObCJXNdlRG3YVyQ== dependencies: ajv "^6.9.1" lodash "^4.17.11" slice-ansi "^2.1.0" string-width "^3.0.0" tap-mocha-reporter@^4.0.1: version "4.0.1" resolved "https://registry.yarnpkg.com/tap-mocha-reporter/-/tap-mocha-reporter-4.0.1.tgz#f613229c42c2e5b5f096f2f022e88724c9380b10" integrity sha512-/KfXaaYeSPn8qBi5Be8WSIP3iKV83s2uj2vzImJAXmjNu22kzqZ+1Dv1riYWa53sPCiyo1R1w1jbJrftF8SpcQ== dependencies: color-support "^1.1.0" debug "^2.1.3" diff "^1.3.2" escape-string-regexp "^1.0.3" glob "^7.0.5" tap-parser "^8.0.0" tap-yaml "0 || 1" unicode-length "^1.0.0" optionalDependencies: readable-stream "^2.1.5" tap-parser@^8.0.0: version "8.1.0" resolved "https://registry.yarnpkg.com/tap-parser/-/tap-parser-8.1.0.tgz#6aff5221c0fe9b39757d60eafc4c436c3a9188fc" integrity sha512-GgOzgDwThYLxhVR83RbS1JUR1TxcT+jfZsrETgPAvFdr12lUOnuvrHOBaUQgpkAp6ZyeW6r2Nwd91t88M0ru3w== dependencies: events-to-array "^1.0.1" minipass "^2.2.0" tap-yaml "0 || 1" tap-parser@^9.3.2: version "9.3.2" resolved "https://registry.yarnpkg.com/tap-parser/-/tap-parser-9.3.2.tgz#a4fcd566dfc15584b721e6e16f3152ebe4937cc8" integrity sha512-bQ76sD6ZP9loxdk/KiqXgWDEfjJYiZUj0a7ElnLMSny7Q8G72UMcOQee85j5ddKHA9fzGAoL6cfJbg3rur7S5g== dependencies: events-to-array "^1.0.1" minipass "^2.2.0" tap-yaml "^1.0.0" "tap-yaml@0 || 1", tap-yaml@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/tap-yaml/-/tap-yaml-1.0.0.tgz#4e31443a5489e05ca8bbb3e36cef71b5dec69635" integrity sha512-Rxbx4EnrWkYk0/ztcm5u3/VznbyFJpyXO12dDBHKWiDVxy7O2Qw6MRrwO5H6Ww0U5YhRY/4C/VzWmFPhBQc4qQ== dependencies: yaml "^1.5.0" tap@^13.1.2: version "13.1.2" resolved "https://registry.yarnpkg.com/tap/-/tap-13.1.2.tgz#ce273a596b0ff822ba24fe96f9f037cfce3e2f98" integrity sha512-ALs3MbwHvq1BfFwE74Tfi4ypCPvg4+C6Ix4BnUDXh8N3wGGzVqBER5j4zY7NloPUUWlS8Td0OdgZU5Am5DJH8A== dependencies: async-hook-domain "^1.1.0" bind-obj-methods "^2.0.0" browser-process-hrtime "^1.0.0" capture-stack-trace "^1.0.0" chokidar "^2.1.5" color-support "^1.1.0" coveralls "^3.0.3" diff "^4.0.1" domain-browser "^1.2.0" esm "^3.2.22" findit "^2.0.0" foreground-child "^1.3.3" fs-exists-cached "^1.0.0" function-loop "^1.0.2" glob "^7.1.3" import-jsx "^2.0.0" isexe "^2.0.0" istanbul-lib-processinfo "^1.0.0" jackspeak "^1.3.7" minipass "^2.3.5" mkdirp "^0.5.1" nyc "^14.1.0" opener "^1.5.1" own-or "^1.0.0" own-or-env "^1.0.1" rimraf "^2.6.3" signal-exit "^3.0.0" source-map-support "^0.5.12" stack-utils "^1.0.2" tap-mocha-reporter "^4.0.1" tap-parser "^9.3.2" tap-yaml "^1.0.0" tcompare "^2.2.0" treport "^0.3.0" trivial-deferred "^1.0.1" ts-node "^8.1.0" typescript "^3.4.3" which "^1.3.1" write-file-atomic "^2.4.2" yaml "^1.5.0" yapool "^1.0.0" tar@^4: version "4.4.8" resolved "https://registry.yarnpkg.com/tar/-/tar-4.4.8.tgz#b19eec3fde2a96e64666df9fdb40c5ca1bc3747d" integrity sha512-LzHF64s5chPQQS0IYBn9IN5h3i98c12bo4NCO7e0sGM2llXQ3p2FGC5sdENN4cTW48O915Sh+x+EXx7XW96xYQ== dependencies: chownr "^1.1.1" fs-minipass "^1.2.5" minipass "^2.3.4" minizlib "^1.1.1" mkdirp "^0.5.0" safe-buffer "^5.1.2" yallist "^3.0.2" tcompare@^2.2.0: version "2.2.0" resolved "https://registry.yarnpkg.com/tcompare/-/tcompare-2.2.0.tgz#873b20a54917f4e91789e2bc20e2c85c4476f754" integrity sha512-+Mr0UBIE3ncNn0wJvKsw8ph61QoaDvR6Q8WkxWIHxWLcKf8SHGyTTkGLMX//4NKQ/Pe1Uu64oXYJsxLyAXXWdA== test-exclude@^5.2.3: version "5.2.3" resolved "https://registry.yarnpkg.com/test-exclude/-/test-exclude-5.2.3.tgz#c3d3e1e311eb7ee405e092dac10aefd09091eac0" integrity sha512-M+oxtseCFO3EDtAaGH7iiej3CBkzXqFMbzqYAACdzKui4eZA+pq3tZEwChvOdNfa7xxy8BfbmgJSIr43cC/+2g== dependencies: glob "^7.1.3" minimatch "^3.0.4" read-pkg-up "^4.0.0" require-main-filename "^2.0.0" text-table@^0.2.0: version "0.2.0" resolved "https://registry.yarnpkg.com/text-table/-/text-table-0.2.0.tgz#7f5ee823ae805207c00af2df4a84ec3fcfa570b4" integrity sha1-f17oI66AUgfACvLfSoTsP8+lcLQ= through@^2.3.6: version "2.3.8" resolved "https://registry.yarnpkg.com/through/-/through-2.3.8.tgz#0dd4c9ffaabc357960b1b724115d7e0e86a2e1f5" integrity sha1-DdTJ/6q8NXlgsbckEV1+Doai4fU= tmp@^0.0.33: version "0.0.33" resolved "https://registry.yarnpkg.com/tmp/-/tmp-0.0.33.tgz#6d34335889768d21b2bcda0aa277ced3b1bfadf9" integrity sha512-jRCJlojKnZ3addtTOjdIqoRuPEKBvNXcGYqzO6zWZX8KfKEpnGY5jfggJQ3EjKuu8D4bJRr0y+cYJFmYbImXGw== dependencies: os-tmpdir "~1.0.2" to-fast-properties@^1.0.3: version "1.0.3" resolved "https://registry.yarnpkg.com/to-fast-properties/-/to-fast-properties-1.0.3.tgz#b83571fa4d8c25b82e231b06e3a3055de4ca1a47" integrity sha1-uDVx+k2MJbguIxsG46MFXeTKGkc= to-fast-properties@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/to-fast-properties/-/to-fast-properties-2.0.0.tgz#dc5e698cbd079265bc73e0377681a4e4e83f616e" integrity sha1-3F5pjL0HkmW8c+A3doGk5Og/YW4= to-object-path@^0.3.0: version "0.3.0" resolved "https://registry.yarnpkg.com/to-object-path/-/to-object-path-0.3.0.tgz#297588b7b0e7e0ac08e04e672f85c1f4999e17af" integrity sha1-KXWIt7Dn4KwI4E5nL4XB9JmeF68= dependencies: kind-of "^3.0.2" to-regex-range@^2.1.0: version "2.1.1" resolved "https://registry.yarnpkg.com/to-regex-range/-/to-regex-range-2.1.1.tgz#7c80c17b9dfebe599e27367e0d4dd5590141db38" integrity sha1-fIDBe53+vlmeJzZ+DU3VWQFB2zg= dependencies: is-number "^3.0.0" repeat-string "^1.6.1" to-regex@^3.0.1, to-regex@^3.0.2: version "3.0.2" resolved "https://registry.yarnpkg.com/to-regex/-/to-regex-3.0.2.tgz#13cfdd9b336552f30b51f33a8ae1b42a7a7599ce" integrity sha512-FWtleNAtZ/Ki2qtqej2CXTOayOH9bHDQF+Q48VpWyDXjbYxA4Yz8iDB31zXOBUlOHHKidDbqGVrTUvQMPmBGBw== dependencies: define-property "^2.0.2" extend-shallow "^3.0.2" regex-not "^1.0.2" safe-regex "^1.1.0" toposort@^2.0.2: version "2.0.2" resolved "https://registry.yarnpkg.com/toposort/-/toposort-2.0.2.tgz#ae21768175d1559d48bef35420b2f4962f09c330" integrity sha1-riF2gXXRVZ1IvvNUILL0li8JwzA= tough-cookie@~2.4.3: version "2.4.3" resolved "https://registry.yarnpkg.com/tough-cookie/-/tough-cookie-2.4.3.tgz#53f36da3f47783b0925afa06ff9f3b165280f781" integrity sha512-Q5srk/4vDM54WJsJio3XNn6K2sCG+CQ8G5Wz6bZhRZoAe/+TxjWB/GlFAnYEbkYVlON9FMk/fE3h2RLpPXo4lQ== dependencies: psl "^1.1.24" punycode "^1.4.1" treport@^0.3.0: version "0.3.0" resolved "https://registry.yarnpkg.com/treport/-/treport-0.3.0.tgz#023d7409ba236f0428d8b0acf3bb7678355d146f" integrity sha512-THr7NS5iLJewcAiaBkxAuCVoakw84hAVY9n2kFNZqlFKHrZeGw8sNR4VhmT23JB1/JnCmPcIOmooTdYYVTI5hA== dependencies: cardinal "^2.1.1" chalk "^2.4.2" import-jsx "^2.0.0" ink "^2.1.1" ms "^2.1.1" react "^16.8.6" string-length "^2.0.0" tap-parser "^9.3.2" unicode-length "^2.0.1" trim-right@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/trim-right/-/trim-right-1.0.1.tgz#cb2e1203067e0c8de1f614094b9fe45704ea6003" integrity sha1-yy4SAwZ+DI3h9hQJS5/kVwTqYAM= trivial-deferred@^1.0.1: version "1.0.1" resolved "https://registry.yarnpkg.com/trivial-deferred/-/trivial-deferred-1.0.1.tgz#376d4d29d951d6368a6f7a0ae85c2f4d5e0658f3" integrity sha1-N21NKdlR1jaKb3oK6FwvTV4GWPM= ts-node@^8.1.0: version "8.1.0" resolved "https://registry.yarnpkg.com/ts-node/-/ts-node-8.1.0.tgz#8c4b37036abd448577db22a061fd7a67d47e658e" integrity sha512-34jpuOrxDuf+O6iW1JpgTRDFynUZ1iEqtYruBqh35gICNjN8x+LpVcPAcwzLPi9VU6mdA3ym+x233nZmZp445A== dependencies: arg "^4.1.0" diff "^3.1.0" make-error "^1.1.1" source-map-support "^0.5.6" yn "^3.0.0" tslib@^1.9.0: version "1.9.3" resolved "https://registry.yarnpkg.com/tslib/-/tslib-1.9.3.tgz#d7e4dd79245d85428c4d7e4822a79917954ca286" integrity sha512-4krF8scpejhaOgqzBEcGM7yDIEfi0/8+8zDRZhNZZ2kjmHJ4hv3zCbQWxoJGz1iw5U0Jl0nma13xzHXcncMavQ== tunnel-agent@^0.6.0: version "0.6.0" resolved "https://registry.yarnpkg.com/tunnel-agent/-/tunnel-agent-0.6.0.tgz#27a5dea06b36b04a0a9966774b290868f0fc40fd" integrity sha1-J6XeoGs2sEoKmWZ3SykIaPD8QP0= dependencies: safe-buffer "^5.0.1" tweetnacl@^0.14.3, tweetnacl@~0.14.0: version "0.14.5" resolved "https://registry.yarnpkg.com/tweetnacl/-/tweetnacl-0.14.5.tgz#5ae68177f192d4456269d108afa93ff8743f4f64" integrity sha1-WuaBd/GS1EViadEIr6k/+HQ/T2Q= type-check@~0.3.2: version "0.3.2" resolved "https://registry.yarnpkg.com/type-check/-/type-check-0.3.2.tgz#5884cab512cf1d355e3fb784f30804b2b520db72" integrity sha1-WITKtRLPHTVeP7eE8wgEsrUg23I= dependencies: prelude-ls "~1.1.2" type-fest@^0.4.1: version "0.4.1" resolved "https://registry.yarnpkg.com/type-fest/-/type-fest-0.4.1.tgz#8bdf77743385d8a4f13ba95f610f5ccd68c728f8" integrity sha512-IwzA/LSfD2vC1/YDYMv/zHP4rDF1usCwllsDpbolT3D4fUepIO7f9K70jjmUewU/LmGUKJcwcVtDCpnKk4BPMw== typescript@^3.4.3: version "3.4.5" resolved "https://registry.yarnpkg.com/typescript/-/typescript-3.4.5.tgz#2d2618d10bb566572b8d7aad5180d84257d70a99" integrity sha512-YycBxUb49UUhdNMU5aJ7z5Ej2XGmaIBL0x34vZ82fn3hGvD+bgrMrVDpatgz2f7YxUMJxMkbWxJZeAvDxVe7Vw== uglify-js@^3.1.4: version "3.5.10" resolved "https://registry.yarnpkg.com/uglify-js/-/uglify-js-3.5.10.tgz#652bef39f86d9dbfd6674407ee05a5e2d372cf2d" integrity sha512-/GTF0nosyPLbdJBd+AwYiZ+Hu5z8KXWnO0WCGt1BQ/u9Iamhejykqmz5o1OHJ53+VAk6xVxychonnApDjuqGsw== dependencies: commander "~2.20.0" source-map "~0.6.1" unicode-canonical-property-names-ecmascript@^1.0.4: version "1.0.4" resolved "https://registry.yarnpkg.com/unicode-canonical-property-names-ecmascript/-/unicode-canonical-property-names-ecmascript-1.0.4.tgz#2619800c4c825800efdd8343af7dd9933cbe2818" integrity sha512-jDrNnXWHd4oHiTZnx/ZG7gtUTVp+gCcTTKr8L0HjlwphROEW3+Him+IpvC+xcJEFegapiMZyZe02CyuOnRmbnQ== unicode-length@^1.0.0: version "1.0.3" resolved "https://registry.yarnpkg.com/unicode-length/-/unicode-length-1.0.3.tgz#5ada7a7fed51841a418a328cf149478ac8358abb" integrity sha1-Wtp6f+1RhBpBijKM8UlHisg1irs= dependencies: punycode "^1.3.2" strip-ansi "^3.0.1" unicode-length@^2.0.1: version "2.0.1" resolved "https://registry.yarnpkg.com/unicode-length/-/unicode-length-2.0.1.tgz#84ddc309c2323635f642e86d448e9eaec084c04a" integrity sha1-hN3DCcIyNjX2QuhtRI6ersCEwEo= dependencies: punycode "^2.0.0" strip-ansi "^3.0.1" unicode-match-property-ecmascript@^1.0.4: version "1.0.4" resolved "https://registry.yarnpkg.com/unicode-match-property-ecmascript/-/unicode-match-property-ecmascript-1.0.4.tgz#8ed2a32569961bce9227d09cd3ffbb8fed5f020c" integrity sha512-L4Qoh15vTfntsn4P1zqnHulG0LdXgjSO035fEpdtp6YxXhMT51Q6vgM5lYdG/5X3MjS+k/Y9Xw4SFCY9IkR0rg== dependencies: unicode-canonical-property-names-ecmascript "^1.0.4" unicode-property-aliases-ecmascript "^1.0.4" unicode-match-property-value-ecmascript@^1.1.0: version "1.1.0" resolved "https://registry.yarnpkg.com/unicode-match-property-value-ecmascript/-/unicode-match-property-value-ecmascript-1.1.0.tgz#5b4b426e08d13a80365e0d657ac7a6c1ec46a277" integrity sha512-hDTHvaBk3RmFzvSl0UVrUmC3PuW9wKVnpoUDYH0JDkSIovzw+J5viQmeYHxVSBptubnr7PbH2e0fnpDRQnQl5g== unicode-property-aliases-ecmascript@^1.0.4: version "1.0.5" resolved "https://registry.yarnpkg.com/unicode-property-aliases-ecmascript/-/unicode-property-aliases-ecmascript-1.0.5.tgz#a9cc6cc7ce63a0a3023fc99e341b94431d405a57" integrity sha512-L5RAqCfXqAwR3RriF8pM0lU0w4Ryf/GgzONwi6KnL1taJQa7x1TCxdJnILX59WIGOwR57IVxn7Nej0fz1Ny6fw== union-value@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/union-value/-/union-value-1.0.0.tgz#5c71c34cb5bad5dcebe3ea0cd08207ba5aa1aea4" integrity sha1-XHHDTLW61dzr4+oM0IIHulqhrqQ= dependencies: arr-union "^3.1.0" get-value "^2.0.6" is-extendable "^0.1.1" set-value "^0.4.3" unset-value@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/unset-value/-/unset-value-1.0.0.tgz#8376873f7d2335179ffb1e6fc3a8ed0dfc8ab559" integrity sha1-g3aHP30jNRef+x5vw6jtDfyKtVk= dependencies: has-value "^0.3.1" isobject "^3.0.0" upath@^1.1.1: version "1.1.2" resolved "https://registry.yarnpkg.com/upath/-/upath-1.1.2.tgz#3db658600edaeeccbe6db5e684d67ee8c2acd068" integrity sha512-kXpym8nmDmlCBr7nKdIx8P2jNBa+pBpIUFRnKJ4dr8htyYGJFokkr2ZvERRtUN+9SY+JqXouNgUPtv6JQva/2Q== uri-js@^4.2.2: version "4.2.2" resolved "https://registry.yarnpkg.com/uri-js/-/uri-js-4.2.2.tgz#94c540e1ff772956e2299507c010aea6c8838eb0" integrity sha512-KY9Frmirql91X2Qgjry0Wd4Y+YTdrdZheS8TFwvkbLWf/G5KNJDCh6pKL5OZctEW4+0Baa5idK2ZQuELRwPznQ== dependencies: punycode "^2.1.0" urix@^0.1.0: version "0.1.0" resolved "https://registry.yarnpkg.com/urix/-/urix-0.1.0.tgz#da937f7a62e21fec1fd18d49b35c2935067a6c72" integrity sha1-2pN/emLiH+wf0Y1Js1wpNQZ6bHI= use@^3.1.0: version "3.1.1" resolved "https://registry.yarnpkg.com/use/-/use-3.1.1.tgz#d50c8cac79a19fbc20f2911f56eb973f4e10070f" integrity sha512-cwESVXlO3url9YWlFW/TA9cshCEhtu7IKJ/p5soJ/gGpj7vbvFrAY/eIioQ6Dw23KjZhYgiIo8HOs1nQ2vr/oQ== util-deprecate@~1.0.1: version "1.0.2" resolved "https://registry.yarnpkg.com/util-deprecate/-/util-deprecate-1.0.2.tgz#450d4dc9fa70de732762fbd2d4a28981419a0ccf" integrity sha1-RQ1Nyfpw3nMnYvvS1KKJgUGaDM8= uuid@^3.3.2: version "3.3.2" resolved "https://registry.yarnpkg.com/uuid/-/uuid-3.3.2.tgz#1b4af4955eb3077c501c23872fc6513811587131" integrity sha512-yXJmeNaw3DnnKAOKJE51sL/ZaYfWJRl1pK9dr19YFCu0ObS231AB1/LbqTKRAQ5kw8A90rA6fr4riOUpTZvQZA== validate-npm-package-license@^3.0.1: version "3.0.4" resolved "https://registry.yarnpkg.com/validate-npm-package-license/-/validate-npm-package-license-3.0.4.tgz#fc91f6b9c7ba15c857f4cb2c5defeec39d4f410a" integrity sha512-DpKm2Ui/xN7/HQKCtpZxoRWBhZ9Z0kqtygG8XCgNQ8ZlDnxuQmWhj566j8fN4Cu3/JmbhsDo7fcAJq4s9h27Ew== dependencies: spdx-correct "^3.0.0" spdx-expression-parse "^3.0.0" verror@1.10.0: version "1.10.0" resolved "https://registry.yarnpkg.com/verror/-/verror-1.10.0.tgz#3a105ca17053af55d6e270c1f8288682e18da400" integrity sha1-OhBcoXBTr1XW4nDB+CiGguGNpAA= dependencies: assert-plus "^1.0.0" core-util-is "1.0.2" extsprintf "^1.2.0" weakmap-shim@^1.1.0: version "1.1.1" resolved "https://registry.yarnpkg.com/weakmap-shim/-/weakmap-shim-1.1.1.tgz#d65afd784109b2166e00ff571c33150ec2a40b49" integrity sha1-1lr9eEEJshZuAP9XHDMVDsKkC0k= which-module@^2.0.0: version "2.0.0" resolved "https://registry.yarnpkg.com/which-module/-/which-module-2.0.0.tgz#d9ef07dce77b9902b8a3a8fa4b31c3e3f7e6e87a" integrity sha1-2e8H3Od7mQK4o6j6SzHD4/fm6Ho= which@^1.2.10, which@^1.2.9, which@^1.3.0, which@^1.3.1: version "1.3.1" resolved "https://registry.yarnpkg.com/which/-/which-1.3.1.tgz#a45043d54f5805316da8d62f9f50918d3da70b0a" integrity sha512-HxJdYWq1MTIQbJ3nw0cqssHoTNU267KlrDuGZ1WYlxDStUtKUhOaJmh112/TZmHxxUfuJqPXSOm7tDyas0OSIQ== dependencies: isexe "^2.0.0" wide-align@^1.1.0: version "1.1.3" resolved "https://registry.yarnpkg.com/wide-align/-/wide-align-1.1.3.tgz#ae074e6bdc0c14a431e804e624549c633b000457" integrity sha512-QGkOQc8XL6Bt5PwnsExKBPuMKBxnGxWWW3fU55Xt4feHozMUhdUMaBCk290qpm/wG5u/RSKzwdAC4i51YigihA== dependencies: string-width "^1.0.2 || 2" widest-line@^2.0.0: version "2.0.1" resolved "https://registry.yarnpkg.com/widest-line/-/widest-line-2.0.1.tgz#7438764730ec7ef4381ce4df82fb98a53142a3fc" integrity sha512-Ba5m9/Fa4Xt9eb2ELXt77JxVDV8w7qQrH0zS/TWSJdLyAwQjWoOzpzj5lwVftDz6n/EOu3tNACS84v509qwnJA== dependencies: string-width "^2.1.1" wordwrap@~0.0.2: version "0.0.3" resolved "https://registry.yarnpkg.com/wordwrap/-/wordwrap-0.0.3.tgz#a3d5da6cd5c0bc0008d37234bbaf1bed63059107" integrity sha1-o9XabNXAvAAI03I0u68b7WMFkQc= wordwrap@~1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/wordwrap/-/wordwrap-1.0.0.tgz#27584810891456a4171c8d0226441ade90cbcaeb" integrity sha1-J1hIEIkUVqQXHI0CJkQa3pDLyus= wrap-ansi@^2.0.0: version "2.1.0" resolved "https://registry.yarnpkg.com/wrap-ansi/-/wrap-ansi-2.1.0.tgz#d8fc3d284dd05794fe84973caecdd1cf824fdd85" integrity sha1-2Pw9KE3QV5T+hJc8rs3Rz4JP3YU= dependencies: string-width "^1.0.1" strip-ansi "^3.0.1" wrap-ansi@^3.0.1: version "3.0.1" resolved "https://registry.yarnpkg.com/wrap-ansi/-/wrap-ansi-3.0.1.tgz#288a04d87eda5c286e060dfe8f135ce8d007f8ba" integrity sha1-KIoE2H7aXChuBg3+jxNc6NAH+Lo= dependencies: string-width "^2.1.1" strip-ansi "^4.0.0" wrap-ansi@^5.0.0: version "5.1.0" resolved "https://registry.yarnpkg.com/wrap-ansi/-/wrap-ansi-5.1.0.tgz#1fd1f67235d5b6d0fee781056001bfb694c03b09" integrity sha512-QC1/iN/2/RPVJ5jYK8BGttj5z83LmSKmvbvrXPNCLZSEb32KKVDJDl/MOt2N01qU2H/FkzEa9PKto1BqDjtd7Q== dependencies: ansi-styles "^3.2.0" string-width "^3.0.0" strip-ansi "^5.0.0" wrappy@1: version "1.0.2" resolved "https://registry.yarnpkg.com/wrappy/-/wrappy-1.0.2.tgz#b5243d8f3ec1aa35f1364605bc0d1036e30ab69f" integrity sha1-tSQ9jz7BqjXxNkYFvA0QNuMKtp8= write-file-atomic@^2.4.2: version "2.4.2" resolved "https://registry.yarnpkg.com/write-file-atomic/-/write-file-atomic-2.4.2.tgz#a7181706dfba17855d221140a9c06e15fcdd87b9" integrity sha512-s0b6vB3xIVRLWywa6X9TOMA7k9zio0TMOsl9ZnDkliA/cfJlpHXAscj0gbHVJiTdIuAYpIyqS5GW91fqm6gG5g== dependencies: graceful-fs "^4.1.11" imurmurhash "^0.1.4" signal-exit "^3.0.2" write@1.0.3: version "1.0.3" resolved "https://registry.yarnpkg.com/write/-/write-1.0.3.tgz#0800e14523b923a387e415123c865616aae0f5c3" integrity sha512-/lg70HAjtkUgWPVZhZcm+T4hkL8Zbtp1nFNOn3lRrxnlv50SRBv7cR7RqR+GMsd3hUXy9hWBo4CHTbFTcOYwig== dependencies: mkdirp "^0.5.1" xtend@^4.0.0: version "4.0.1" resolved "https://registry.yarnpkg.com/xtend/-/xtend-4.0.1.tgz#a5c6d532be656e23db820efb943a1f04998d63af" integrity sha1-pcbVMr5lbiPbgg77lDofBJmNY68= y18n@^4.0.0: version "4.0.0" resolved "https://registry.yarnpkg.com/y18n/-/y18n-4.0.0.tgz#95ef94f85ecc81d007c264e190a120f0a3c8566b" integrity sha512-r9S/ZyXu/Xu9q1tYlpsLIsa3EeLXXk0VwlxqTcFRfg9EhMW+17kbt9G0NrgCmhGb5vT2hyhJZLfDGx+7+5Uj/w== yallist@^2.1.2: version "2.1.2" resolved "https://registry.yarnpkg.com/yallist/-/yallist-2.1.2.tgz#1c11f9218f076089a47dd512f93c6699a6a81d52" integrity sha1-HBH5IY8HYImkfdUS+TxmmaaoHVI= yallist@^3.0.0, yallist@^3.0.2: version "3.0.3" resolved "https://registry.yarnpkg.com/yallist/-/yallist-3.0.3.tgz#b4b049e314be545e3ce802236d6cd22cd91c3de9" integrity sha512-S+Zk8DEWE6oKpV+vI3qWkaK+jSbIK86pCwe2IF/xwIpQ8jEuxpw9NyaGjmp9+BoJv5FV2piqCDcoCtStppiq2A== yaml@^1.5.0: version "1.5.1" resolved "https://registry.yarnpkg.com/yaml/-/yaml-1.5.1.tgz#e8201678064fbcfef6afe4122ef802573b6cade8" integrity sha512-btfJvMOgVthGZSgHBMrDkLuQu4YxOycw6kwuC67cUEOKJmmNozjIa02eKvuSq7usqqqpwwCvflGTF6JcDvSudw== dependencies: "@babel/runtime" "^7.4.4" yapool@^1.0.0: version "1.0.0" resolved "https://registry.yarnpkg.com/yapool/-/yapool-1.0.0.tgz#f693f29a315b50d9a9da2646a7a6645c96985b6a" integrity sha1-9pPymjFbUNmp2iZGp6ZkXJaYW2o= yargs-parser@^13.0.0: version "13.0.0" resolved "https://registry.yarnpkg.com/yargs-parser/-/yargs-parser-13.0.0.tgz#3fc44f3e76a8bdb1cc3602e860108602e5ccde8b" integrity sha512-w2LXjoL8oRdRQN+hOyppuXs+V/fVAYtpcrRxZuF7Kt/Oc+Jr2uAcVntaUTNT6w5ihoWfFDpNY8CPx1QskxZ/pw== dependencies: camelcase "^5.0.0" decamelize "^1.2.0" yargs@^13.2.2: version "13.2.2" resolved "https://registry.yarnpkg.com/yargs/-/yargs-13.2.2.tgz#0c101f580ae95cea7f39d927e7770e3fdc97f993" integrity sha512-WyEoxgyTD3w5XRpAQNYUB9ycVH/PQrToaTXdYXRdOXvEy1l19br+VJsc0vcO8PTGg5ro/l/GY7F/JMEBmI0BxA== dependencies: cliui "^4.0.0" find-up "^3.0.0" get-caller-file "^2.0.1" os-locale "^3.1.0" require-directory "^2.1.1" require-main-filename "^2.0.0" set-blocking "^2.0.0" string-width "^3.0.0" which-module "^2.0.0" y18n "^4.0.0" yargs-parser "^13.0.0" yn@^3.0.0: version "3.1.0" resolved "https://registry.yarnpkg.com/yn/-/yn-3.1.0.tgz#fcbe2db63610361afcc5eb9e0ac91e976d046114" integrity sha512-kKfnnYkbTfrAdd0xICNFw7Atm8nKpLcLv9AZGEt+kczL/WQVai4e2V6ZN8U/O+iI6WrNuJjNNOyu4zfhl9D3Hg== yoga-layout-prebuilt@^1.9.3: version "1.9.3" resolved "https://registry.yarnpkg.com/yoga-layout-prebuilt/-/yoga-layout-prebuilt-1.9.3.tgz#11e3be29096afe3c284e5d963cc2d628148c1372" integrity sha512-9SNQpwuEh2NucU83i2KMZnONVudZ86YNcFk9tq74YaqrQfgJWO3yB9uzH1tAg8iqh5c9F5j0wuyJ2z72wcum2w== yup@^0.27.0: version "0.27.0" resolved "https://registry.yarnpkg.com/yup/-/yup-0.27.0.tgz#f8cb198c8e7dd2124beddc2457571329096b06e7" integrity sha512-v1yFnE4+u9za42gG/b/081E7uNW9mUj3qtkmelLbW5YPROZzSH/KUUyJu9Wt8vxFJcT9otL/eZopS0YK1L5yPQ== dependencies: "@babel/runtime" "^7.0.0" fn-name "~2.0.1" lodash "^4.17.11" property-expr "^1.5.0" synchronous-promise "^2.0.6" toposort "^2.0.2" apollo-server-demo/node_modules/fs-capacitor/.lintstagedrc.json 0000644 0001750 0000144 00000000101 13463756503 024442 0 ustar andreh users { "*.{mjs,js}": "eslint", "*.{json,yml,md}": "prettier -l" } apollo-server-demo/node_modules/send/ 0000755 0001750 0000144 00000000000 14067647700 017373 5 ustar andreh users apollo-server-demo/node_modules/send/index.js 0000644 0001750 0000144 00000055367 03560116604 021046 0 ustar andreh users /*! * send * Copyright(c) 2012 TJ Holowaychuk * Copyright(c) 2014-2016 Douglas Christopher Wilson * MIT Licensed */ 'use strict' /** * Module dependencies. * @private */ var createError = require('http-errors') var debug = require('debug')('send') var deprecate = require('depd')('send') var destroy = require('destroy') var encodeUrl = require('encodeurl') var escapeHtml = require('escape-html') var etag = require('etag') var fresh = require('fresh') var fs = require('fs') var mime = require('mime') var ms = require('ms') var onFinished = require('on-finished') var parseRange = require('range-parser') var path = require('path') var statuses = require('statuses') var Stream = require('stream') var util = require('util') /** * Path function references. * @private */ var extname = path.extname var join = path.join var normalize = path.normalize var resolve = path.resolve var sep = path.sep /** * Regular expression for identifying a bytes Range header. * @private */ var BYTES_RANGE_REGEXP = /^ *bytes=/ /** * Maximum value allowed for the max age. * @private */ var MAX_MAXAGE = 60 * 60 * 24 * 365 * 1000 // 1 year /** * Regular expression to match a path with a directory up component. * @private */ var UP_PATH_REGEXP = /(?:^|[\\/])\.\.(?:[\\/]|$)/ /** * Module exports. * @public */ module.exports = send module.exports.mime = mime /** * Return a `SendStream` for `req` and `path`. * * @param {object} req * @param {string} path * @param {object} [options] * @return {SendStream} * @public */ function send (req, path, options) { return new SendStream(req, path, options) } /** * Initialize a `SendStream` with the given `path`. * * @param {Request} req * @param {String} path * @param {object} [options] * @private */ function SendStream (req, path, options) { Stream.call(this) var opts = options || {} this.options = opts this.path = path this.req = req this._acceptRanges = opts.acceptRanges !== undefined ? Boolean(opts.acceptRanges) : true this._cacheControl = opts.cacheControl !== undefined ? Boolean(opts.cacheControl) : true this._etag = opts.etag !== undefined ? Boolean(opts.etag) : true this._dotfiles = opts.dotfiles !== undefined ? opts.dotfiles : 'ignore' if (this._dotfiles !== 'ignore' && this._dotfiles !== 'allow' && this._dotfiles !== 'deny') { throw new TypeError('dotfiles option must be "allow", "deny", or "ignore"') } this._hidden = Boolean(opts.hidden) if (opts.hidden !== undefined) { deprecate('hidden: use dotfiles: \'' + (this._hidden ? 'allow' : 'ignore') + '\' instead') } // legacy support if (opts.dotfiles === undefined) { this._dotfiles = undefined } this._extensions = opts.extensions !== undefined ? normalizeList(opts.extensions, 'extensions option') : [] this._immutable = opts.immutable !== undefined ? Boolean(opts.immutable) : false this._index = opts.index !== undefined ? normalizeList(opts.index, 'index option') : ['index.html'] this._lastModified = opts.lastModified !== undefined ? Boolean(opts.lastModified) : true this._maxage = opts.maxAge || opts.maxage this._maxage = typeof this._maxage === 'string' ? ms(this._maxage) : Number(this._maxage) this._maxage = !isNaN(this._maxage) ? Math.min(Math.max(0, this._maxage), MAX_MAXAGE) : 0 this._root = opts.root ? resolve(opts.root) : null if (!this._root && opts.from) { this.from(opts.from) } } /** * Inherits from `Stream`. */ util.inherits(SendStream, Stream) /** * Enable or disable etag generation. * * @param {Boolean} val * @return {SendStream} * @api public */ SendStream.prototype.etag = deprecate.function(function etag (val) { this._etag = Boolean(val) debug('etag %s', this._etag) return this }, 'send.etag: pass etag as option') /** * Enable or disable "hidden" (dot) files. * * @param {Boolean} path * @return {SendStream} * @api public */ SendStream.prototype.hidden = deprecate.function(function hidden (val) { this._hidden = Boolean(val) this._dotfiles = undefined debug('hidden %s', this._hidden) return this }, 'send.hidden: use dotfiles option') /** * Set index `paths`, set to a falsy * value to disable index support. * * @param {String|Boolean|Array} paths * @return {SendStream} * @api public */ SendStream.prototype.index = deprecate.function(function index (paths) { var index = !paths ? [] : normalizeList(paths, 'paths argument') debug('index %o', paths) this._index = index return this }, 'send.index: pass index as option') /** * Set root `path`. * * @param {String} path * @return {SendStream} * @api public */ SendStream.prototype.root = function root (path) { this._root = resolve(String(path)) debug('root %s', this._root) return this } SendStream.prototype.from = deprecate.function(SendStream.prototype.root, 'send.from: pass root as option') SendStream.prototype.root = deprecate.function(SendStream.prototype.root, 'send.root: pass root as option') /** * Set max-age to `maxAge`. * * @param {Number} maxAge * @return {SendStream} * @api public */ SendStream.prototype.maxage = deprecate.function(function maxage (maxAge) { this._maxage = typeof maxAge === 'string' ? ms(maxAge) : Number(maxAge) this._maxage = !isNaN(this._maxage) ? Math.min(Math.max(0, this._maxage), MAX_MAXAGE) : 0 debug('max-age %d', this._maxage) return this }, 'send.maxage: pass maxAge as option') /** * Emit error with `status`. * * @param {number} status * @param {Error} [err] * @private */ SendStream.prototype.error = function error (status, err) { // emit if listeners instead of responding if (hasListeners(this, 'error')) { return this.emit('error', createError(status, err, { expose: false })) } var res = this.res var msg = statuses[status] || String(status) var doc = createHtmlDocument('Error', escapeHtml(msg)) // clear existing headers clearHeaders(res) // add error headers if (err && err.headers) { setHeaders(res, err.headers) } // send basic response res.statusCode = status res.setHeader('Content-Type', 'text/html; charset=UTF-8') res.setHeader('Content-Length', Buffer.byteLength(doc)) res.setHeader('Content-Security-Policy', "default-src 'none'") res.setHeader('X-Content-Type-Options', 'nosniff') res.end(doc) } /** * Check if the pathname ends with "/". * * @return {boolean} * @private */ SendStream.prototype.hasTrailingSlash = function hasTrailingSlash () { return this.path[this.path.length - 1] === '/' } /** * Check if this is a conditional GET request. * * @return {Boolean} * @api private */ SendStream.prototype.isConditionalGET = function isConditionalGET () { return this.req.headers['if-match'] || this.req.headers['if-unmodified-since'] || this.req.headers['if-none-match'] || this.req.headers['if-modified-since'] } /** * Check if the request preconditions failed. * * @return {boolean} * @private */ SendStream.prototype.isPreconditionFailure = function isPreconditionFailure () { var req = this.req var res = this.res // if-match var match = req.headers['if-match'] if (match) { var etag = res.getHeader('ETag') return !etag || (match !== '*' && parseTokenList(match).every(function (match) { return match !== etag && match !== 'W/' + etag && 'W/' + match !== etag })) } // if-unmodified-since var unmodifiedSince = parseHttpDate(req.headers['if-unmodified-since']) if (!isNaN(unmodifiedSince)) { var lastModified = parseHttpDate(res.getHeader('Last-Modified')) return isNaN(lastModified) || lastModified > unmodifiedSince } return false } /** * Strip content-* header fields. * * @private */ SendStream.prototype.removeContentHeaderFields = function removeContentHeaderFields () { var res = this.res var headers = getHeaderNames(res) for (var i = 0; i < headers.length; i++) { var header = headers[i] if (header.substr(0, 8) === 'content-' && header !== 'content-location') { res.removeHeader(header) } } } /** * Respond with 304 not modified. * * @api private */ SendStream.prototype.notModified = function notModified () { var res = this.res debug('not modified') this.removeContentHeaderFields() res.statusCode = 304 res.end() } /** * Raise error that headers already sent. * * @api private */ SendStream.prototype.headersAlreadySent = function headersAlreadySent () { var err = new Error('Can\'t set headers after they are sent.') debug('headers already sent') this.error(500, err) } /** * Check if the request is cacheable, aka * responded with 2xx or 304 (see RFC 2616 section 14.2{5,6}). * * @return {Boolean} * @api private */ SendStream.prototype.isCachable = function isCachable () { var statusCode = this.res.statusCode return (statusCode >= 200 && statusCode < 300) || statusCode === 304 } /** * Handle stat() error. * * @param {Error} error * @private */ SendStream.prototype.onStatError = function onStatError (error) { switch (error.code) { case 'ENAMETOOLONG': case 'ENOENT': case 'ENOTDIR': this.error(404, error) break default: this.error(500, error) break } } /** * Check if the cache is fresh. * * @return {Boolean} * @api private */ SendStream.prototype.isFresh = function isFresh () { return fresh(this.req.headers, { 'etag': this.res.getHeader('ETag'), 'last-modified': this.res.getHeader('Last-Modified') }) } /** * Check if the range is fresh. * * @return {Boolean} * @api private */ SendStream.prototype.isRangeFresh = function isRangeFresh () { var ifRange = this.req.headers['if-range'] if (!ifRange) { return true } // if-range as etag if (ifRange.indexOf('"') !== -1) { var etag = this.res.getHeader('ETag') return Boolean(etag && ifRange.indexOf(etag) !== -1) } // if-range as modified date var lastModified = this.res.getHeader('Last-Modified') return parseHttpDate(lastModified) <= parseHttpDate(ifRange) } /** * Redirect to path. * * @param {string} path * @private */ SendStream.prototype.redirect = function redirect (path) { var res = this.res if (hasListeners(this, 'directory')) { this.emit('directory', res, path) return } if (this.hasTrailingSlash()) { this.error(403) return } var loc = encodeUrl(collapseLeadingSlashes(this.path + '/')) var doc = createHtmlDocument('Redirecting', 'Redirecting to <a href="' + escapeHtml(loc) + '">' + escapeHtml(loc) + '</a>') // redirect res.statusCode = 301 res.setHeader('Content-Type', 'text/html; charset=UTF-8') res.setHeader('Content-Length', Buffer.byteLength(doc)) res.setHeader('Content-Security-Policy', "default-src 'none'") res.setHeader('X-Content-Type-Options', 'nosniff') res.setHeader('Location', loc) res.end(doc) } /** * Pipe to `res. * * @param {Stream} res * @return {Stream} res * @api public */ SendStream.prototype.pipe = function pipe (res) { // root path var root = this._root // references this.res = res // decode the path var path = decode(this.path) if (path === -1) { this.error(400) return res } // null byte(s) if (~path.indexOf('\0')) { this.error(400) return res } var parts if (root !== null) { // normalize if (path) { path = normalize('.' + sep + path) } // malicious path if (UP_PATH_REGEXP.test(path)) { debug('malicious path "%s"', path) this.error(403) return res } // explode path parts parts = path.split(sep) // join / normalize from optional root dir path = normalize(join(root, path)) } else { // ".." is malicious without "root" if (UP_PATH_REGEXP.test(path)) { debug('malicious path "%s"', path) this.error(403) return res } // explode path parts parts = normalize(path).split(sep) // resolve the path path = resolve(path) } // dotfile handling if (containsDotFile(parts)) { var access = this._dotfiles // legacy support if (access === undefined) { access = parts[parts.length - 1][0] === '.' ? (this._hidden ? 'allow' : 'ignore') : 'allow' } debug('%s dotfile "%s"', access, path) switch (access) { case 'allow': break case 'deny': this.error(403) return res case 'ignore': default: this.error(404) return res } } // index file support if (this._index.length && this.hasTrailingSlash()) { this.sendIndex(path) return res } this.sendFile(path) return res } /** * Transfer `path`. * * @param {String} path * @api public */ SendStream.prototype.send = function send (path, stat) { var len = stat.size var options = this.options var opts = {} var res = this.res var req = this.req var ranges = req.headers.range var offset = options.start || 0 if (headersSent(res)) { // impossible to send now this.headersAlreadySent() return } debug('pipe "%s"', path) // set header fields this.setHeader(path, stat) // set content-type this.type(path) // conditional GET support if (this.isConditionalGET()) { if (this.isPreconditionFailure()) { this.error(412) return } if (this.isCachable() && this.isFresh()) { this.notModified() return } } // adjust len to start/end options len = Math.max(0, len - offset) if (options.end !== undefined) { var bytes = options.end - offset + 1 if (len > bytes) len = bytes } // Range support if (this._acceptRanges && BYTES_RANGE_REGEXP.test(ranges)) { // parse ranges = parseRange(len, ranges, { combine: true }) // If-Range support if (!this.isRangeFresh()) { debug('range stale') ranges = -2 } // unsatisfiable if (ranges === -1) { debug('range unsatisfiable') // Content-Range res.setHeader('Content-Range', contentRange('bytes', len)) // 416 Requested Range Not Satisfiable return this.error(416, { headers: { 'Content-Range': res.getHeader('Content-Range') } }) } // valid (syntactically invalid/multiple ranges are treated as a regular response) if (ranges !== -2 && ranges.length === 1) { debug('range %j', ranges) // Content-Range res.statusCode = 206 res.setHeader('Content-Range', contentRange('bytes', len, ranges[0])) // adjust for requested range offset += ranges[0].start len = ranges[0].end - ranges[0].start + 1 } } // clone options for (var prop in options) { opts[prop] = options[prop] } // set read options opts.start = offset opts.end = Math.max(offset, offset + len - 1) // content-length res.setHeader('Content-Length', len) // HEAD support if (req.method === 'HEAD') { res.end() return } this.stream(path, opts) } /** * Transfer file for `path`. * * @param {String} path * @api private */ SendStream.prototype.sendFile = function sendFile (path) { var i = 0 var self = this debug('stat "%s"', path) fs.stat(path, function onstat (err, stat) { if (err && err.code === 'ENOENT' && !extname(path) && path[path.length - 1] !== sep) { // not found, check extensions return next(err) } if (err) return self.onStatError(err) if (stat.isDirectory()) return self.redirect(path) self.emit('file', path, stat) self.send(path, stat) }) function next (err) { if (self._extensions.length <= i) { return err ? self.onStatError(err) : self.error(404) } var p = path + '.' + self._extensions[i++] debug('stat "%s"', p) fs.stat(p, function (err, stat) { if (err) return next(err) if (stat.isDirectory()) return next() self.emit('file', p, stat) self.send(p, stat) }) } } /** * Transfer index for `path`. * * @param {String} path * @api private */ SendStream.prototype.sendIndex = function sendIndex (path) { var i = -1 var self = this function next (err) { if (++i >= self._index.length) { if (err) return self.onStatError(err) return self.error(404) } var p = join(path, self._index[i]) debug('stat "%s"', p) fs.stat(p, function (err, stat) { if (err) return next(err) if (stat.isDirectory()) return next() self.emit('file', p, stat) self.send(p, stat) }) } next() } /** * Stream `path` to the response. * * @param {String} path * @param {Object} options * @api private */ SendStream.prototype.stream = function stream (path, options) { // TODO: this is all lame, refactor meeee var finished = false var self = this var res = this.res // pipe var stream = fs.createReadStream(path, options) this.emit('stream', stream) stream.pipe(res) // response finished, done with the fd onFinished(res, function onfinished () { finished = true destroy(stream) }) // error handling code-smell stream.on('error', function onerror (err) { // request already finished if (finished) return // clean up stream finished = true destroy(stream) // error self.onStatError(err) }) // end stream.on('end', function onend () { self.emit('end') }) } /** * Set content-type based on `path` * if it hasn't been explicitly set. * * @param {String} path * @api private */ SendStream.prototype.type = function type (path) { var res = this.res if (res.getHeader('Content-Type')) return var type = mime.lookup(path) if (!type) { debug('no content-type') return } var charset = mime.charsets.lookup(type) debug('content-type %s', type) res.setHeader('Content-Type', type + (charset ? '; charset=' + charset : '')) } /** * Set response header fields, most * fields may be pre-defined. * * @param {String} path * @param {Object} stat * @api private */ SendStream.prototype.setHeader = function setHeader (path, stat) { var res = this.res this.emit('headers', res, path, stat) if (this._acceptRanges && !res.getHeader('Accept-Ranges')) { debug('accept ranges') res.setHeader('Accept-Ranges', 'bytes') } if (this._cacheControl && !res.getHeader('Cache-Control')) { var cacheControl = 'public, max-age=' + Math.floor(this._maxage / 1000) if (this._immutable) { cacheControl += ', immutable' } debug('cache-control %s', cacheControl) res.setHeader('Cache-Control', cacheControl) } if (this._lastModified && !res.getHeader('Last-Modified')) { var modified = stat.mtime.toUTCString() debug('modified %s', modified) res.setHeader('Last-Modified', modified) } if (this._etag && !res.getHeader('ETag')) { var val = etag(stat) debug('etag %s', val) res.setHeader('ETag', val) } } /** * Clear all headers from a response. * * @param {object} res * @private */ function clearHeaders (res) { var headers = getHeaderNames(res) for (var i = 0; i < headers.length; i++) { res.removeHeader(headers[i]) } } /** * Collapse all leading slashes into a single slash * * @param {string} str * @private */ function collapseLeadingSlashes (str) { for (var i = 0; i < str.length; i++) { if (str[i] !== '/') { break } } return i > 1 ? '/' + str.substr(i) : str } /** * Determine if path parts contain a dotfile. * * @api private */ function containsDotFile (parts) { for (var i = 0; i < parts.length; i++) { var part = parts[i] if (part.length > 1 && part[0] === '.') { return true } } return false } /** * Create a Content-Range header. * * @param {string} type * @param {number} size * @param {array} [range] */ function contentRange (type, size, range) { return type + ' ' + (range ? range.start + '-' + range.end : '*') + '/' + size } /** * Create a minimal HTML document. * * @param {string} title * @param {string} body * @private */ function createHtmlDocument (title, body) { return '<!DOCTYPE html>\n' + '<html lang="en">\n' + '<head>\n' + '<meta charset="utf-8">\n' + '<title>' + title + '</title>\n' + '</head>\n' + '<body>\n' + '<pre>' + body + '</pre>\n' + '</body>\n' + '</html>\n' } /** * decodeURIComponent. * * Allows V8 to only deoptimize this fn instead of all * of send(). * * @param {String} path * @api private */ function decode (path) { try { return decodeURIComponent(path) } catch (err) { return -1 } } /** * Get the header names on a respnse. * * @param {object} res * @returns {array[string]} * @private */ function getHeaderNames (res) { return typeof res.getHeaderNames !== 'function' ? Object.keys(res._headers || {}) : res.getHeaderNames() } /** * Determine if emitter has listeners of a given type. * * The way to do this check is done three different ways in Node.js >= 0.8 * so this consolidates them into a minimal set using instance methods. * * @param {EventEmitter} emitter * @param {string} type * @returns {boolean} * @private */ function hasListeners (emitter, type) { var count = typeof emitter.listenerCount !== 'function' ? emitter.listeners(type).length : emitter.listenerCount(type) return count > 0 } /** * Determine if the response headers have been sent. * * @param {object} res * @returns {boolean} * @private */ function headersSent (res) { return typeof res.headersSent !== 'boolean' ? Boolean(res._header) : res.headersSent } /** * Normalize the index option into an array. * * @param {boolean|string|array} val * @param {string} name * @private */ function normalizeList (val, name) { var list = [].concat(val || []) for (var i = 0; i < list.length; i++) { if (typeof list[i] !== 'string') { throw new TypeError(name + ' must be array of strings or false') } } return list } /** * Parse an HTTP Date into a number. * * @param {string} date * @private */ function parseHttpDate (date) { var timestamp = date && Date.parse(date) return typeof timestamp === 'number' ? timestamp : NaN } /** * Parse a HTTP token list. * * @param {string} str * @private */ function parseTokenList (str) { var end = 0 var list = [] var start = 0 // gather tokens for (var i = 0, len = str.length; i < len; i++) { switch (str.charCodeAt(i)) { case 0x20: /* */ if (start === end) { start = end = i + 1 } break case 0x2c: /* , */ list.push(str.substring(start, end)) start = end = i + 1 break default: end = i + 1 break } } // final token list.push(str.substring(start, end)) return list } /** * Set an object of headers on a response. * * @param {object} res * @param {object} headers * @private */ function setHeaders (res, headers) { var keys = Object.keys(headers) for (var i = 0; i < keys.length; i++) { var key = keys[i] res.setHeader(key, headers[key]) } } apollo-server-demo/node_modules/send/LICENSE 0000644 0001750 0000144 00000002150 03560116604 020364 0 ustar andreh users (The MIT License) Copyright (c) 2012 TJ Holowaychuk Copyright (c) 2014-2016 Douglas Christopher Wilson Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the 'Software'), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/send/HISTORY.md 0000644 0001750 0000144 00000030543 03560116604 021051 0 ustar andreh users 0.17.1 / 2019-05-10 =================== * Set stricter CSP header in redirect & error responses * deps: range-parser@~1.2.1 0.17.0 / 2019-05-03 =================== * deps: http-errors@~1.7.2 - Set constructor name when possible - Use `toidentifier` module to make class names - deps: depd@~1.1.2 - deps: setprototypeof@1.1.1 - deps: statuses@'>= 1.5.0 < 2' * deps: mime@1.6.0 - Add extensions for JPEG-2000 images - Add new `font/*` types from IANA - Add WASM mapping - Update `.bdoc` to `application/bdoc` - Update `.bmp` to `image/bmp` - Update `.m4a` to `audio/mp4` - Update `.rtf` to `application/rtf` - Update `.wav` to `audio/wav` - Update `.xml` to `application/xml` - Update generic extensions to `application/octet-stream`: `.deb`, `.dll`, `.dmg`, `.exe`, `.iso`, `.msi` - Use mime-score module to resolve extension conflicts * deps: ms@2.1.1 - Add `week`/`w` support - Fix negative number handling * deps: statuses@~1.5.0 * perf: remove redundant `path.normalize` call 0.16.2 / 2018-02-07 =================== * Fix incorrect end tag in default error & redirects * deps: depd@~1.1.2 - perf: remove argument reassignment * deps: encodeurl@~1.0.2 - Fix encoding `%` as last character * deps: statuses@~1.4.0 0.16.1 / 2017-09-29 =================== * Fix regression in edge-case behavior for empty `path` 0.16.0 / 2017-09-27 =================== * Add `immutable` option * Fix missing `</html>` in default error & redirects * Use instance methods on steam to check for listeners * deps: mime@1.4.1 - Add 70 new types for file extensions - Set charset as "UTF-8" for .js and .json * perf: improve path validation speed 0.15.6 / 2017-09-22 =================== * deps: debug@2.6.9 * perf: improve `If-Match` token parsing 0.15.5 / 2017-09-20 =================== * deps: etag@~1.8.1 - perf: replace regular expression with substring * deps: fresh@0.5.2 - Fix handling of modified headers with invalid dates - perf: improve ETag match loop - perf: improve `If-None-Match` token parsing 0.15.4 / 2017-08-05 =================== * deps: debug@2.6.8 * deps: depd@~1.1.1 - Remove unnecessary `Buffer` loading * deps: http-errors@~1.6.2 - deps: depd@1.1.1 0.15.3 / 2017-05-16 =================== * deps: debug@2.6.7 - deps: ms@2.0.0 * deps: ms@2.0.0 0.15.2 / 2017-04-26 =================== * deps: debug@2.6.4 - Fix `DEBUG_MAX_ARRAY_LENGTH` - deps: ms@0.7.3 * deps: ms@1.0.0 0.15.1 / 2017-03-04 =================== * Fix issue when `Date.parse` does not return `NaN` on invalid date * Fix strict violation in broken environments 0.15.0 / 2017-02-25 =================== * Support `If-Match` and `If-Unmodified-Since` headers * Add `res` and `path` arguments to `directory` event * Remove usage of `res._headers` private field - Improves compatibility with Node.js 8 nightly * Send complete HTML document in redirect & error responses * Set default CSP header in redirect & error responses * Use `res.getHeaderNames()` when available * Use `res.headersSent` when available * deps: debug@2.6.1 - Allow colors in workers - Deprecated `DEBUG_FD` environment variable set to `3` or higher - Fix error when running under React Native - Use same color for same namespace - deps: ms@0.7.2 * deps: etag@~1.8.0 * deps: fresh@0.5.0 - Fix false detection of `no-cache` request directive - Fix incorrect result when `If-None-Match` has both `*` and ETags - Fix weak `ETag` matching to match spec - perf: delay reading header values until needed - perf: enable strict mode - perf: hoist regular expressions - perf: remove duplicate conditional - perf: remove unnecessary boolean coercions - perf: skip checking modified time if ETag check failed - perf: skip parsing `If-None-Match` when no `ETag` header - perf: use `Date.parse` instead of `new Date` * deps: http-errors@~1.6.1 - Make `message` property enumerable for `HttpError`s - deps: setprototypeof@1.0.3 0.14.2 / 2017-01-23 =================== * deps: http-errors@~1.5.1 - deps: inherits@2.0.3 - deps: setprototypeof@1.0.2 - deps: statuses@'>= 1.3.1 < 2' * deps: ms@0.7.2 * deps: statuses@~1.3.1 0.14.1 / 2016-06-09 =================== * Fix redirect error when `path` contains raw non-URL characters * Fix redirect when `path` starts with multiple forward slashes 0.14.0 / 2016-06-06 =================== * Add `acceptRanges` option * Add `cacheControl` option * Attempt to combine multiple ranges into single range * Correctly inherit from `Stream` class * Fix `Content-Range` header in 416 responses when using `start`/`end` options * Fix `Content-Range` header missing from default 416 responses * Ignore non-byte `Range` headers * deps: http-errors@~1.5.0 - Add `HttpError` export, for `err instanceof createError.HttpError` - Support new code `421 Misdirected Request` - Use `setprototypeof` module to replace `__proto__` setting - deps: inherits@2.0.1 - deps: statuses@'>= 1.3.0 < 2' - perf: enable strict mode * deps: range-parser@~1.2.0 - Fix incorrectly returning -1 when there is at least one valid range - perf: remove internal function * deps: statuses@~1.3.0 - Add `421 Misdirected Request` - perf: enable strict mode * perf: remove argument reassignment 0.13.2 / 2016-03-05 =================== * Fix invalid `Content-Type` header when `send.mime.default_type` unset 0.13.1 / 2016-01-16 =================== * deps: depd@~1.1.0 - Support web browser loading - perf: enable strict mode * deps: destroy@~1.0.4 - perf: enable strict mode * deps: escape-html@~1.0.3 - perf: enable strict mode - perf: optimize string replacement - perf: use faster string coercion * deps: range-parser@~1.0.3 - perf: enable strict mode 0.13.0 / 2015-06-16 =================== * Allow Node.js HTTP server to set `Date` response header * Fix incorrectly removing `Content-Location` on 304 response * Improve the default redirect response headers * Send appropriate headers on default error response * Use `http-errors` for standard emitted errors * Use `statuses` instead of `http` module for status messages * deps: escape-html@1.0.2 * deps: etag@~1.7.0 - Improve stat performance by removing hashing * deps: fresh@0.3.0 - Add weak `ETag` matching support * deps: on-finished@~2.3.0 - Add defined behavior for HTTP `CONNECT` requests - Add defined behavior for HTTP `Upgrade` requests - deps: ee-first@1.1.1 * perf: enable strict mode * perf: remove unnecessary array allocations 0.12.3 / 2015-05-13 =================== * deps: debug@~2.2.0 - deps: ms@0.7.1 * deps: depd@~1.0.1 * deps: etag@~1.6.0 - Improve support for JXcore - Support "fake" stats objects in environments without `fs` * deps: ms@0.7.1 - Prevent extraordinarily long inputs * deps: on-finished@~2.2.1 0.12.2 / 2015-03-13 =================== * Throw errors early for invalid `extensions` or `index` options * deps: debug@~2.1.3 - Fix high intensity foreground color for bold - deps: ms@0.7.0 0.12.1 / 2015-02-17 =================== * Fix regression sending zero-length files 0.12.0 / 2015-02-16 =================== * Always read the stat size from the file * Fix mutating passed-in `options` * deps: mime@1.3.4 0.11.1 / 2015-01-20 =================== * Fix `root` path disclosure 0.11.0 / 2015-01-05 =================== * deps: debug@~2.1.1 * deps: etag@~1.5.1 - deps: crc@3.2.1 * deps: ms@0.7.0 - Add `milliseconds` - Add `msecs` - Add `secs` - Add `mins` - Add `hrs` - Add `yrs` * deps: on-finished@~2.2.0 0.10.1 / 2014-10-22 =================== * deps: on-finished@~2.1.1 - Fix handling of pipelined requests 0.10.0 / 2014-10-15 =================== * deps: debug@~2.1.0 - Implement `DEBUG_FD` env variable support * deps: depd@~1.0.0 * deps: etag@~1.5.0 - Improve string performance - Slightly improve speed for weak ETags over 1KB 0.9.3 / 2014-09-24 ================== * deps: etag@~1.4.0 - Support "fake" stats objects 0.9.2 / 2014-09-15 ================== * deps: depd@0.4.5 * deps: etag@~1.3.1 * deps: range-parser@~1.0.2 0.9.1 / 2014-09-07 ================== * deps: fresh@0.2.4 0.9.0 / 2014-09-07 ================== * Add `lastModified` option * Use `etag` to generate `ETag` header * deps: debug@~2.0.0 0.8.5 / 2014-09-04 ================== * Fix malicious path detection for empty string path 0.8.4 / 2014-09-04 ================== * Fix a path traversal issue when using `root` 0.8.3 / 2014-08-16 ================== * deps: destroy@1.0.3 - renamed from dethroy * deps: on-finished@2.1.0 0.8.2 / 2014-08-14 ================== * Work around `fd` leak in Node.js 0.10 for `fs.ReadStream` * deps: dethroy@1.0.2 0.8.1 / 2014-08-05 ================== * Fix `extensions` behavior when file already has extension 0.8.0 / 2014-08-05 ================== * Add `extensions` option 0.7.4 / 2014-08-04 ================== * Fix serving index files without root dir 0.7.3 / 2014-07-29 ================== * Fix incorrect 403 on Windows and Node.js 0.11 0.7.2 / 2014-07-27 ================== * deps: depd@0.4.4 - Work-around v8 generating empty stack traces 0.7.1 / 2014-07-26 ================== * deps: depd@0.4.3 - Fix exception when global `Error.stackTraceLimit` is too low 0.7.0 / 2014-07-20 ================== * Deprecate `hidden` option; use `dotfiles` option * Add `dotfiles` option * deps: debug@1.0.4 * deps: depd@0.4.2 - Add `TRACE_DEPRECATION` environment variable - Remove non-standard grey color from color output - Support `--no-deprecation` argument - Support `--trace-deprecation` argument 0.6.0 / 2014-07-11 ================== * Deprecate `from` option; use `root` option * Deprecate `send.etag()` -- use `etag` in `options` * Deprecate `send.hidden()` -- use `hidden` in `options` * Deprecate `send.index()` -- use `index` in `options` * Deprecate `send.maxage()` -- use `maxAge` in `options` * Deprecate `send.root()` -- use `root` in `options` * Cap `maxAge` value to 1 year * deps: debug@1.0.3 - Add support for multiple wildcards in namespaces 0.5.0 / 2014-06-28 ================== * Accept string for `maxAge` (converted by `ms`) * Add `headers` event * Include link in default redirect response * Use `EventEmitter.listenerCount` to count listeners 0.4.3 / 2014-06-11 ================== * Do not throw un-catchable error on file open race condition * Use `escape-html` for HTML escaping * deps: debug@1.0.2 - fix some debugging output colors on node.js 0.8 * deps: finished@1.2.2 * deps: fresh@0.2.2 0.4.2 / 2014-06-09 ================== * fix "event emitter leak" warnings * deps: debug@1.0.1 * deps: finished@1.2.1 0.4.1 / 2014-06-02 ================== * Send `max-age` in `Cache-Control` in correct format 0.4.0 / 2014-05-27 ================== * Calculate ETag with md5 for reduced collisions * Fix wrong behavior when index file matches directory * Ignore stream errors after request ends - Goodbye `EBADF, read` * Skip directories in index file search * deps: debug@0.8.1 0.3.0 / 2014-04-24 ================== * Fix sending files with dots without root set * Coerce option types * Accept API options in options object * Set etags to "weak" * Include file path in etag * Make "Can't set headers after they are sent." catchable * Send full entity-body for multi range requests * Default directory access to 403 when index disabled * Support multiple index paths * Support "If-Range" header * Control whether to generate etags * deps: mime@1.2.11 0.2.0 / 2014-01-29 ================== * update range-parser and fresh 0.1.4 / 2013-08-11 ================== * update fresh 0.1.3 / 2013-07-08 ================== * Revert "Fix fd leak" 0.1.2 / 2013-07-03 ================== * Fix fd leak 0.1.0 / 2012-08-25 ================== * add options parameter to send() that is passed to fs.createReadStream() [kanongil] 0.0.4 / 2012-08-16 ================== * allow custom "Accept-Ranges" definition 0.0.3 / 2012-07-16 ================== * fix normalization of the root directory. Closes #3 0.0.2 / 2012-07-09 ================== * add passing of req explicitly for now (YUCK) 0.0.1 / 2010-01-03 ================== * Initial release apollo-server-demo/node_modules/send/README.md 0000644 0001750 0000144 00000022342 03560116604 020643 0 ustar andreh users # send [![NPM Version][npm-version-image]][npm-url] [![NPM Downloads][npm-downloads-image]][npm-url] [![Linux Build][travis-image]][travis-url] [![Windows Build][appveyor-image]][appveyor-url] [![Test Coverage][coveralls-image]][coveralls-url] Send is a library for streaming files from the file system as a http response supporting partial responses (Ranges), conditional-GET negotiation (If-Match, If-Unmodified-Since, If-None-Match, If-Modified-Since), high test coverage, and granular events which may be leveraged to take appropriate actions in your application or framework. Looking to serve up entire folders mapped to URLs? Try [serve-static](https://www.npmjs.org/package/serve-static). ## Installation This is a [Node.js](https://nodejs.org/en/) module available through the [npm registry](https://www.npmjs.com/). Installation is done using the [`npm install` command](https://docs.npmjs.com/getting-started/installing-npm-packages-locally): ```bash $ npm install send ``` ## API <!-- eslint-disable no-unused-vars --> ```js var send = require('send') ``` ### send(req, path, [options]) Create a new `SendStream` for the given path to send to a `res`. The `req` is the Node.js HTTP request and the `path` is a urlencoded path to send (urlencoded, not the actual file-system path). #### Options ##### acceptRanges Enable or disable accepting ranged requests, defaults to true. Disabling this will not send `Accept-Ranges` and ignore the contents of the `Range` request header. ##### cacheControl Enable or disable setting `Cache-Control` response header, defaults to true. Disabling this will ignore the `immutable` and `maxAge` options. ##### dotfiles Set how "dotfiles" are treated when encountered. A dotfile is a file or directory that begins with a dot ("."). Note this check is done on the path itself without checking if the path actually exists on the disk. If `root` is specified, only the dotfiles above the root are checked (i.e. the root itself can be within a dotfile when when set to "deny"). - `'allow'` No special treatment for dotfiles. - `'deny'` Send a 403 for any request for a dotfile. - `'ignore'` Pretend like the dotfile does not exist and 404. The default value is _similar_ to `'ignore'`, with the exception that this default will not ignore the files within a directory that begins with a dot, for backward-compatibility. ##### end Byte offset at which the stream ends, defaults to the length of the file minus 1. The end is inclusive in the stream, meaning `end: 3` will include the 4th byte in the stream. ##### etag Enable or disable etag generation, defaults to true. ##### extensions If a given file doesn't exist, try appending one of the given extensions, in the given order. By default, this is disabled (set to `false`). An example value that will serve extension-less HTML files: `['html', 'htm']`. This is skipped if the requested file already has an extension. ##### immutable Enable or diable the `immutable` directive in the `Cache-Control` response header, defaults to `false`. If set to `true`, the `maxAge` option should also be specified to enable caching. The `immutable` directive will prevent supported clients from making conditional requests during the life of the `maxAge` option to check if the file has changed. ##### index By default send supports "index.html" files, to disable this set `false` or to supply a new index pass a string or an array in preferred order. ##### lastModified Enable or disable `Last-Modified` header, defaults to true. Uses the file system's last modified value. ##### maxAge Provide a max-age in milliseconds for http caching, defaults to 0. This can also be a string accepted by the [ms](https://www.npmjs.org/package/ms#readme) module. ##### root Serve files relative to `path`. ##### start Byte offset at which the stream starts, defaults to 0. The start is inclusive, meaning `start: 2` will include the 3rd byte in the stream. #### Events The `SendStream` is an event emitter and will emit the following events: - `error` an error occurred `(err)` - `directory` a directory was requested `(res, path)` - `file` a file was requested `(path, stat)` - `headers` the headers are about to be set on a file `(res, path, stat)` - `stream` file streaming has started `(stream)` - `end` streaming has completed #### .pipe The `pipe` method is used to pipe the response into the Node.js HTTP response object, typically `send(req, path, options).pipe(res)`. ### .mime The `mime` export is the global instance of of the [`mime` npm module](https://www.npmjs.com/package/mime). This is used to configure the MIME types that are associated with file extensions as well as other options for how to resolve the MIME type of a file (like the default type to use for an unknown file extension). ## Error-handling By default when no `error` listeners are present an automatic response will be made, otherwise you have full control over the response, aka you may show a 5xx page etc. ## Caching It does _not_ perform internal caching, you should use a reverse proxy cache such as Varnish for this, or those fancy things called CDNs. If your application is small enough that it would benefit from single-node memory caching, it's small enough that it does not need caching at all ;). ## Debugging To enable `debug()` instrumentation output export __DEBUG__: ``` $ DEBUG=send node app ``` ## Running tests ``` $ npm install $ npm test ``` ## Examples ### Serve a specific file This simple example will send a specific file to all requests. ```js var http = require('http') var send = require('send') var server = http.createServer(function onRequest (req, res) { send(req, '/path/to/index.html') .pipe(res) }) server.listen(3000) ``` ### Serve all files from a directory This simple example will just serve up all the files in a given directory as the top-level. For example, a request `GET /foo.txt` will send back `/www/public/foo.txt`. ```js var http = require('http') var parseUrl = require('parseurl') var send = require('send') var server = http.createServer(function onRequest (req, res) { send(req, parseUrl(req).pathname, { root: '/www/public' }) .pipe(res) }) server.listen(3000) ``` ### Custom file types ```js var http = require('http') var parseUrl = require('parseurl') var send = require('send') // Default unknown types to text/plain send.mime.default_type = 'text/plain' // Add a custom type send.mime.define({ 'application/x-my-type': ['x-mt', 'x-mtt'] }) var server = http.createServer(function onRequest (req, res) { send(req, parseUrl(req).pathname, { root: '/www/public' }) .pipe(res) }) server.listen(3000) ``` ### Custom directory index view This is a example of serving up a structure of directories with a custom function to render a listing of a directory. ```js var http = require('http') var fs = require('fs') var parseUrl = require('parseurl') var send = require('send') // Transfer arbitrary files from within /www/example.com/public/* // with a custom handler for directory listing var server = http.createServer(function onRequest (req, res) { send(req, parseUrl(req).pathname, { index: false, root: '/www/public' }) .once('directory', directory) .pipe(res) }) server.listen(3000) // Custom directory handler function directory (res, path) { var stream = this // redirect to trailing slash for consistent url if (!stream.hasTrailingSlash()) { return stream.redirect(path) } // get directory list fs.readdir(path, function onReaddir (err, list) { if (err) return stream.error(err) // render an index for the directory res.setHeader('Content-Type', 'text/plain; charset=UTF-8') res.end(list.join('\n') + '\n') }) } ``` ### Serving from a root directory with custom error-handling ```js var http = require('http') var parseUrl = require('parseurl') var send = require('send') var server = http.createServer(function onRequest (req, res) { // your custom error-handling logic: function error (err) { res.statusCode = err.status || 500 res.end(err.message) } // your custom headers function headers (res, path, stat) { // serve all files for download res.setHeader('Content-Disposition', 'attachment') } // your custom directory handling logic: function redirect () { res.statusCode = 301 res.setHeader('Location', req.url + '/') res.end('Redirecting to ' + req.url + '/') } // transfer arbitrary files from within // /www/example.com/public/* send(req, parseUrl(req).pathname, { root: '/www/public' }) .on('error', error) .on('directory', redirect) .on('headers', headers) .pipe(res) }) server.listen(3000) ``` ## License [MIT](LICENSE) [appveyor-image]: https://badgen.net/appveyor/ci/dougwilson/send/master?label=windows [appveyor-url]: https://ci.appveyor.com/project/dougwilson/send [coveralls-image]: https://badgen.net/coveralls/c/github/pillarjs/send/master [coveralls-url]: https://coveralls.io/r/pillarjs/send?branch=master [node-image]: https://badgen.net/npm/node/send [node-url]: https://nodejs.org/en/download/ [npm-downloads-image]: https://badgen.net/npm/dm/send [npm-url]: https://npmjs.org/package/send [npm-version-image]: https://badgen.net/npm/v/send [travis-image]: https://badgen.net/travis/pillarjs/send/master?label=linux [travis-url]: https://travis-ci.org/pillarjs/send apollo-server-demo/node_modules/send/package.json 0000644 0001750 0000144 00000003524 03560116604 021653 0 ustar andreh users { "name": "send", "description": "Better streaming static file server with Range and conditional-GET support", "version": "0.17.1", "author": "TJ Holowaychuk <tj@vision-media.ca>", "contributors": [ "Douglas Christopher Wilson <doug@somethingdoug.com>", "James Wyatt Cready <jcready@gmail.com>", "Jesús Leganés Combarro <piranna@gmail.com>" ], "license": "MIT", "repository": "pillarjs/send", "keywords": [ "static", "file", "server" ], "dependencies": { "debug": "2.6.9", "depd": "~1.1.2", "destroy": "~1.0.4", "encodeurl": "~1.0.2", "escape-html": "~1.0.3", "etag": "~1.8.1", "fresh": "0.5.2", "http-errors": "~1.7.2", "mime": "1.6.0", "ms": "2.1.1", "on-finished": "~2.3.0", "range-parser": "~1.2.1", "statuses": "~1.5.0" }, "devDependencies": { "after": "0.8.2", "eslint": "5.16.0", "eslint-config-standard": "12.0.0", "eslint-plugin-import": "2.17.2", "eslint-plugin-markdown": "1.0.0", "eslint-plugin-node": "8.0.1", "eslint-plugin-promise": "4.1.1", "eslint-plugin-standard": "4.0.0", "istanbul": "0.4.5", "mocha": "6.1.4", "supertest": "4.0.2" }, "files": [ "HISTORY.md", "LICENSE", "README.md", "index.js" ], "engines": { "node": ">= 0.8.0" }, "scripts": { "lint": "eslint --plugin markdown --ext js,md .", "test": "mocha --check-leaks --reporter spec --bail", "test-ci": "istanbul cover node_modules/mocha/bin/_mocha --report lcovonly -- --check-leaks --reporter spec", "test-cov": "istanbul cover node_modules/mocha/bin/_mocha -- --check-leaks --reporter dot" } ,"_resolved": "https://registry.npmjs.org/send/-/send-0.17.1.tgz" ,"_integrity": "sha512-BsVKsiGcQMFwT8UxypobUKyv7irCNRHk1T0G680vk88yf6LBByGcZJOTJCrTP2xVN6yI+XjPJcNuE3V4fT9sAg==" ,"_from": "send@0.17.1" } apollo-server-demo/node_modules/send/node_modules/ 0000755 0001750 0000144 00000000000 14067647700 022050 5 ustar andreh users apollo-server-demo/node_modules/send/node_modules/ms/ 0000755 0001750 0000144 00000000000 14067647700 022467 5 ustar andreh users apollo-server-demo/node_modules/send/node_modules/ms/index.js 0000644 0001750 0000144 00000005732 13210045461 024124 0 ustar andreh users /** * Helpers. */ var s = 1000; var m = s * 60; var h = m * 60; var d = h * 24; var w = d * 7; var y = d * 365.25; /** * Parse or format the given `val`. * * Options: * * - `long` verbose formatting [false] * * @param {String|Number} val * @param {Object} [options] * @throws {Error} throw an error if val is not a non-empty string or a number * @return {String|Number} * @api public */ module.exports = function(val, options) { options = options || {}; var type = typeof val; if (type === 'string' && val.length > 0) { return parse(val); } else if (type === 'number' && isNaN(val) === false) { return options.long ? fmtLong(val) : fmtShort(val); } throw new Error( 'val is not a non-empty string or a valid number. val=' + JSON.stringify(val) ); }; /** * Parse the given `str` and return milliseconds. * * @param {String} str * @return {Number} * @api private */ function parse(str) { str = String(str); if (str.length > 100) { return; } var match = /^((?:\d+)?\-?\d?\.?\d+) *(milliseconds?|msecs?|ms|seconds?|secs?|s|minutes?|mins?|m|hours?|hrs?|h|days?|d|weeks?|w|years?|yrs?|y)?$/i.exec( str ); if (!match) { return; } var n = parseFloat(match[1]); var type = (match[2] || 'ms').toLowerCase(); switch (type) { case 'years': case 'year': case 'yrs': case 'yr': case 'y': return n * y; case 'weeks': case 'week': case 'w': return n * w; case 'days': case 'day': case 'd': return n * d; case 'hours': case 'hour': case 'hrs': case 'hr': case 'h': return n * h; case 'minutes': case 'minute': case 'mins': case 'min': case 'm': return n * m; case 'seconds': case 'second': case 'secs': case 'sec': case 's': return n * s; case 'milliseconds': case 'millisecond': case 'msecs': case 'msec': case 'ms': return n; default: return undefined; } } /** * Short format for `ms`. * * @param {Number} ms * @return {String} * @api private */ function fmtShort(ms) { var msAbs = Math.abs(ms); if (msAbs >= d) { return Math.round(ms / d) + 'd'; } if (msAbs >= h) { return Math.round(ms / h) + 'h'; } if (msAbs >= m) { return Math.round(ms / m) + 'm'; } if (msAbs >= s) { return Math.round(ms / s) + 's'; } return ms + 'ms'; } /** * Long format for `ms`. * * @param {Number} ms * @return {String} * @api private */ function fmtLong(ms) { var msAbs = Math.abs(ms); if (msAbs >= d) { return plural(ms, msAbs, d, 'day'); } if (msAbs >= h) { return plural(ms, msAbs, h, 'hour'); } if (msAbs >= m) { return plural(ms, msAbs, m, 'minute'); } if (msAbs >= s) { return plural(ms, msAbs, s, 'second'); } return ms + ' ms'; } /** * Pluralization helper. */ function plural(ms, msAbs, n, name) { var isPlural = msAbs >= n * 1.5; return Math.round(ms / n) + ' ' + name + (isPlural ? 's' : ''); } apollo-server-demo/node_modules/send/node_modules/ms/readme.md 0000644 0001750 0000144 00000003722 13210045461 024233 0 ustar andreh users # ms [](https://travis-ci.org/zeit/ms) [](https://zeit.chat/) Use this package to easily convert various time formats to milliseconds. ## Examples ```js ms('2 days') // 172800000 ms('1d') // 86400000 ms('10h') // 36000000 ms('2.5 hrs') // 9000000 ms('2h') // 7200000 ms('1m') // 60000 ms('5s') // 5000 ms('1y') // 31557600000 ms('100') // 100 ms('-3 days') // -259200000 ms('-1h') // -3600000 ms('-200') // -200 ``` ### Convert from Milliseconds ```js ms(60000) // "1m" ms(2 * 60000) // "2m" ms(-3 * 60000) // "-3m" ms(ms('10 hours')) // "10h" ``` ### Time Format Written-Out ```js ms(60000, { long: true }) // "1 minute" ms(2 * 60000, { long: true }) // "2 minutes" ms(-3 * 60000, { long: true }) // "-3 minutes" ms(ms('10 hours'), { long: true }) // "10 hours" ``` ## Features - Works both in [Node.js](https://nodejs.org) and in the browser - If a number is supplied to `ms`, a string with a unit is returned - If a string that contains the number is supplied, it returns it as a number (e.g.: it returns `100` for `'100'`) - If you pass a string with a number and a valid unit, the number of equivalent milliseconds is returned ## Related Packages - [ms.macro](https://github.com/knpwrs/ms.macro) - Run `ms` as a macro at build-time. ## Caught a Bug? 1. [Fork](https://help.github.com/articles/fork-a-repo/) this repository to your own GitHub account and then [clone](https://help.github.com/articles/cloning-a-repository/) it to your local device 2. Link the package to the global module directory: `npm link` 3. Within the module you want to test your local development instance of ms, just link it to the dependencies: `npm link ms`. Instead of the default one from npm, Node.js will now use your clone of ms! As always, you can run the tests using: `npm test` apollo-server-demo/node_modules/send/node_modules/ms/package.json 0000644 0001750 0000144 00000001604 13210045554 024742 0 ustar andreh users { "name": "ms", "version": "2.1.1", "description": "Tiny millisecond conversion utility", "repository": "zeit/ms", "main": "./index", "files": [ "index.js" ], "scripts": { "precommit": "lint-staged", "lint": "eslint lib/* bin/*", "test": "mocha tests.js" }, "eslintConfig": { "extends": "eslint:recommended", "env": { "node": true, "es6": true } }, "lint-staged": { "*.js": [ "npm run lint", "prettier --single-quote --write", "git add" ] }, "license": "MIT", "devDependencies": { "eslint": "4.12.1", "expect.js": "0.3.1", "husky": "0.14.3", "lint-staged": "5.0.0", "mocha": "4.0.1" } ,"_resolved": "https://registry.npmjs.org/ms/-/ms-2.1.1.tgz" ,"_integrity": "sha512-tgp+dl5cGk28utYktBsrFqA7HKgrhgPsg6Z/EfhWI4gl1Hwq8B/GmY/0oXZ6nF8hDVesS/FpnYaD/kOWhYQvyg==" ,"_from": "ms@2.1.1" } apollo-server-demo/node_modules/send/node_modules/ms/license.md 0000644 0001750 0000144 00000002065 13210033361 024413 0 ustar andreh users The MIT License (MIT) Copyright (c) 2016 Zeit, Inc. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/apollo-env/ 0000755 0001750 0000144 00000000000 14067647701 020517 5 ustar andreh users apollo-server-demo/node_modules/apollo-env/LICENSE 0000644 0001750 0000144 00000002111 03560116604 021504 0 ustar andreh users The MIT License (MIT) Copyright (c) 2016 Meteor Development Group, Inc. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/apollo-env/src/ 0000755 0001750 0000144 00000000000 14067647701 021306 5 ustar andreh users apollo-server-demo/node_modules/apollo-env/src/typescript-utility-types.ts 0000644 0001750 0000144 00000000220 03560116604 026706 0 ustar andreh users export type WithRequired<T, K extends keyof T> = T & Required<Pick<T, K>>; export type DeepPartial<T> = { [P in keyof T]?: DeepPartial<T[P]> }; apollo-server-demo/node_modules/apollo-env/src/polyfills/ 0000755 0001750 0000144 00000000000 14067647701 023323 5 ustar andreh users apollo-server-demo/node_modules/apollo-env/src/polyfills/array.ts 0000644 0001750 0000144 00000001121 03560116604 024771 0 ustar andreh users /// <reference lib="esnext.array" /> import "core-js/features/array/flat"; import "core-js/features/array/flat-map"; // The built-in Array.flat typings don't contain an overload for ReadonlyArray<U>[], // which means the return type is inferred to be any[] instead of U[], hence this augmentation. declare global { interface Array<T> { /** * Returns a new array with all sub-array elements concatenated into it recursively up to the * specified depth. * * @param depth The maximum recursion depth */ flat<U>(this: ReadonlyArray<U>[], depth?: 1): U[]; } } apollo-server-demo/node_modules/apollo-env/src/polyfills/object.ts 0000644 0001750 0000144 00000000252 03560116604 025125 0 ustar andreh users import "core-js/features/object/from-entries"; declare global { interface ObjectConstructor { fromEntries<K extends string, V>(map: [K, V][]): Record<K, V>; } } apollo-server-demo/node_modules/apollo-env/src/polyfills/index.ts 0000644 0001750 0000144 00000000045 03560116604 024766 0 ustar andreh users import "./array"; import "./object"; apollo-server-demo/node_modules/apollo-env/src/utils/ 0000755 0001750 0000144 00000000000 14067647701 022446 5 ustar andreh users apollo-server-demo/node_modules/apollo-env/src/utils/createHash.ts 0000644 0001750 0000144 00000000554 03560116604 025056 0 ustar andreh users import { isNodeLike } from "./isNodeLike"; export function createHash(kind: string): import("crypto").Hash { if (isNodeLike) { // Use module.require instead of just require to avoid bundling whatever // crypto polyfills a non-Node bundler might fall back to. return module.require("crypto").createHash(kind); } return require("sha.js")(kind); } apollo-server-demo/node_modules/apollo-env/src/utils/predicates.ts 0000644 0001750 0000144 00000000222 03560116604 025122 0 ustar andreh users export function isNotNullOrUndefined<T>( value: T | null | undefined ): value is T { return value !== null && typeof value !== "undefined"; } apollo-server-demo/node_modules/apollo-env/src/utils/isNodeLike.ts 0000644 0001750 0000144 00000000240 03560116604 025025 0 ustar andreh users export const isNodeLike = typeof process === "object" && process && process.release && process.versions && typeof process.versions.node === "string"; apollo-server-demo/node_modules/apollo-env/src/utils/index.ts 0000644 0001750 0000144 00000000167 03560116604 024116 0 ustar andreh users export * from "./createHash"; export * from "./isNodeLike"; export * from "./mapValues"; export * from "./predicates"; apollo-server-demo/node_modules/apollo-env/src/utils/mapValues.ts 0000644 0001750 0000144 00000000441 03560116604 024737 0 ustar andreh users export function mapValues<T, U = T>( object: Record<string, T>, callback: (value: T) => U ): Record<string, U> { const result: Record<string, U> = Object.create(null); for (const [key, value] of Object.entries(object)) { result[key] = callback(value); } return result; } apollo-server-demo/node_modules/apollo-env/src/index.ts 0000644 0001750 0000144 00000000165 03560116604 022754 0 ustar andreh users import "./polyfills"; export * from "./typescript-utility-types"; export * from "./fetch"; export * from "./utils"; apollo-server-demo/node_modules/apollo-env/src/fetch/ 0000755 0001750 0000144 00000000000 14067647701 022377 5 ustar andreh users apollo-server-demo/node_modules/apollo-env/src/fetch/index.d.ts 0000644 0001750 0000144 00000000060 03560116604 024261 0 ustar andreh users export * from "./fetch"; export * from "./url"; apollo-server-demo/node_modules/apollo-env/src/fetch/global.ts 0000644 0001750 0000144 00000002040 03560116604 024170 0 ustar andreh users declare function fetch( input?: RequestInfo, init?: RequestInit ): Promise<Response>; declare interface GlobalFetch { fetch(input: RequestInfo, init?: RequestInit): Promise<Response>; } type RequestInfo = import("./fetch").RequestInfo; type Headers = import("./fetch").Headers; type HeadersInit = import("./fetch").HeadersInit; type Body = import("./fetch").Body; type Request = import("./fetch").Request; type RequestAgent = import("./fetch").RequestAgent; type RequestInit = import("./fetch").RequestInit; type RequestMode = import("./fetch").RequestMode; type RequestCredentials = import("./fetch").RequestCredentials; type RequestCache = import("./fetch").RequestCache; type RequestRedirect = import("./fetch").RequestRedirect; type ReferrerPolicy = import("./fetch").ReferrerPolicy; type Response = import("./fetch").Response; type ResponseInit = import("./fetch").ResponseInit; type BodyInit = import("./fetch").BodyInit; type URLSearchParams = import("./url").URLSearchParams; type URLSearchParamsInit = import("./url").URLSearchParamsInit; apollo-server-demo/node_modules/apollo-env/src/fetch/tsconfig.json 0000644 0001750 0000144 00000000417 03560116604 025075 0 ustar andreh users { "extends": "../../../../tsconfig.base", "compilerOptions": { "composite": false, "rootDir": ".", "outDir": "../../lib/fetch", "allowJs": true, "declaration": false, "declarationMap": false, "types": ["node"] }, "include": ["**/*"] } apollo-server-demo/node_modules/apollo-env/src/fetch/fetch.ts 0000644 0001750 0000144 00000001133 03560116604 024023 0 ustar andreh users import { Agent as HttpAgent } from "http"; import { Agent as HttpsAgent } from "https"; export type RequestAgent = HttpAgent | HttpsAgent; export type ReferrerPolicy = | "" | "no-referrer" | "no-referrer-when-downgrade" | "same-origin" | "origin" | "strict-origin" | "origin-when-cross-origin" | "strict-origin-when-cross-origin" | "unsafe-url"; export { default as fetch, Request, Response, Headers, ResponseInit, BodyInit, RequestInfo, HeadersInit, Body, RequestInit, RequestMode, RequestCredentials, RequestCache, RequestRedirect } from "node-fetch"; apollo-server-demo/node_modules/apollo-env/src/fetch/url.ts 0000644 0001750 0000144 00000000406 03560116604 023536 0 ustar andreh users import { URLSearchParams } from "url"; export { URL, URLSearchParams } from "url"; export type URLSearchParamsInit = | URLSearchParams | string | { [key: string]: Object | Object[] | undefined } | Iterable<[string, Object]> | Array<[string, Object]>; apollo-server-demo/node_modules/apollo-env/src/fetch/index.ts 0000644 0001750 0000144 00000000060 03560116604 024037 0 ustar andreh users export * from "./fetch"; export * from "./url"; apollo-server-demo/node_modules/apollo-env/tsconfig.json 0000644 0001750 0000144 00000000243 03560116604 023212 0 ustar andreh users { "extends": "../../tsconfig.base", "compilerOptions": { "rootDir": "./src", "outDir": "./lib", "types": ["node"] }, "include": ["src/**/*"] } apollo-server-demo/node_modules/apollo-env/package.json 0000644 0001750 0000144 00000001536 03560116604 022777 0 ustar andreh users { "name": "apollo-env", "version": "0.6.5", "author": "opensource@apollographql.com", "license": "MIT", "repository": { "type": "git", "url": "git+https://github.com/apollographql/apollo-tooling.git" }, "homepage": "https://github.com/apollographql/apollo-tooling", "bugs": "https://github.com/apollographql/apollo-tooling/issues", "main": "lib/index.js", "types": "lib/index.d.ts", "engines": { "node": ">=8" }, "dependencies": { "@types/node-fetch": "2.5.7", "core-js": "^3.0.1", "node-fetch": "^2.2.0", "sha.js": "^2.4.11" }, "gitHead": "7cc66acbbfc681da0491a7868150deccc7ca368f" ,"_resolved": "https://registry.npmjs.org/apollo-env/-/apollo-env-0.6.5.tgz" ,"_integrity": "sha512-jeBUVsGymeTHYWp3me0R2CZRZrFeuSZeICZHCeRflHTfnQtlmbSXdy5E0pOyRM9CU4JfQkKDC98S1YglQj7Bzg==" ,"_from": "apollo-env@0.6.5" } apollo-server-demo/node_modules/apollo-env/lib/ 0000755 0001750 0000144 00000000000 14067647701 021265 5 ustar andreh users apollo-server-demo/node_modules/apollo-env/lib/index.js 0000644 0001750 0000144 00000000443 03560116604 022720 0 ustar andreh users "use strict"; function __export(m) { for (var p in m) if (!exports.hasOwnProperty(p)) exports[p] = m[p]; } Object.defineProperty(exports, "__esModule", { value: true }); require("./polyfills"); __export(require("./fetch")); __export(require("./utils")); //# sourceMappingURL=index.js.map apollo-server-demo/node_modules/apollo-env/lib/typescript-utility-types.js.map 0000644 0001750 0000144 00000000214 03560116604 027432 0 ustar andreh users {"version":3,"file":"typescript-utility-types.js","sourceRoot":"","sources":["../src/typescript-utility-types.ts"],"names":[],"mappings":""} apollo-server-demo/node_modules/apollo-env/lib/polyfills/ 0000755 0001750 0000144 00000000000 14067647701 023302 5 ustar andreh users apollo-server-demo/node_modules/apollo-env/lib/polyfills/object.js.map 0000644 0001750 0000144 00000000202 03560116604 025641 0 ustar andreh users {"version":3,"file":"object.js","sourceRoot":"","sources":["../../src/polyfills/object.ts"],"names":[],"mappings":";;AAAA,gDAA8C"} apollo-server-demo/node_modules/apollo-env/lib/polyfills/index.js 0000644 0001750 0000144 00000000227 03560116604 024735 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); require("./array"); require("./object"); //# sourceMappingURL=index.js.map apollo-server-demo/node_modules/apollo-env/lib/polyfills/index.d.ts 0000644 0001750 0000144 00000000110 03560116604 025160 0 ustar andreh users import "./array"; import "./object"; //# sourceMappingURL=index.d.ts.map apollo-server-demo/node_modules/apollo-env/lib/polyfills/object.js 0000644 0001750 0000144 00000000240 03560116604 025067 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); require("core-js/features/object/from-entries"); //# sourceMappingURL=object.js.map apollo-server-demo/node_modules/apollo-env/lib/polyfills/index.d.ts.map 0000644 0001750 0000144 00000000235 03560116604 025744 0 ustar andreh users {"version":3,"file":"index.d.ts","sourceRoot":"","sources":["../../src/polyfills/index.ts"],"names":[],"mappings":"AAAA,OAAO,SAAS,CAAC;AACjB,OAAO,UAAU,CAAC"} apollo-server-demo/node_modules/apollo-env/lib/polyfills/array.d.ts 0000644 0001750 0000144 00000000405 03560116604 025176 0 ustar andreh users /// <reference lib="esnext.array" /> import "core-js/features/array/flat"; import "core-js/features/array/flat-map"; declare global { interface Array<T> { flat<U>(this: ReadonlyArray<U>[], depth?: 1): U[]; } } //# sourceMappingURL=array.d.ts.map apollo-server-demo/node_modules/apollo-env/lib/polyfills/object.d.ts.map 0000644 0001750 0000144 00000000500 03560116604 026076 0 ustar andreh users {"version":3,"file":"object.d.ts","sourceRoot":"","sources":["../../src/polyfills/object.ts"],"names":[],"mappings":"AAAA,OAAO,sCAAsC,CAAC;AAE9C,OAAO,CAAC,MAAM,CAAC;IACb,UAAU,iBAAiB;QACzB,WAAW,CAAC,CAAC,SAAS,MAAM,EAAE,CAAC,EAAE,GAAG,EAAE,CAAC,CAAC,EAAE,CAAC,CAAC,EAAE,GAAG,MAAM,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC;KAC/D;CACF"} apollo-server-demo/node_modules/apollo-env/lib/polyfills/array.js 0000644 0001750 0000144 00000000302 03560116604 024736 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); require("core-js/features/array/flat"); require("core-js/features/array/flat-map"); //# sourceMappingURL=array.js.map apollo-server-demo/node_modules/apollo-env/lib/polyfills/index.js.map 0000644 0001750 0000144 00000000215 03560116604 025506 0 ustar andreh users {"version":3,"file":"index.js","sourceRoot":"","sources":["../../src/polyfills/index.ts"],"names":[],"mappings":";;AAAA,mBAAiB;AACjB,oBAAkB"} apollo-server-demo/node_modules/apollo-env/lib/polyfills/array.js.map 0000644 0001750 0000144 00000000215 03560116604 025515 0 ustar andreh users {"version":3,"file":"array.js","sourceRoot":"","sources":["../../src/polyfills/array.ts"],"names":[],"mappings":";;AACA,uCAAqC;AACrC,2CAAyC"} apollo-server-demo/node_modules/apollo-env/lib/polyfills/object.d.ts 0000644 0001750 0000144 00000000325 03560116604 025327 0 ustar andreh users import "core-js/features/object/from-entries"; declare global { interface ObjectConstructor { fromEntries<K extends string, V>(map: [K, V][]): Record<K, V>; } } //# sourceMappingURL=object.d.ts.map apollo-server-demo/node_modules/apollo-env/lib/polyfills/array.d.ts.map 0000644 0001750 0000144 00000000511 03560116604 025750 0 ustar andreh users {"version":3,"file":"array.d.ts","sourceRoot":"","sources":["../../src/polyfills/array.ts"],"names":[],"mappings":";AACA,OAAO,6BAA6B,CAAC;AACrC,OAAO,iCAAiC,CAAC;AAIzC,OAAO,CAAC,MAAM,CAAC;IACb,UAAU,KAAK,CAAC,CAAC;QAOf,IAAI,CAAC,CAAC,EAAE,IAAI,EAAE,aAAa,CAAC,CAAC,CAAC,EAAE,EAAE,KAAK,CAAC,EAAE,CAAC,GAAG,CAAC,EAAE,CAAC;KACnD;CACF"} apollo-server-demo/node_modules/apollo-env/lib/index.d.ts 0000644 0001750 0000144 00000000227 03560116604 023154 0 ustar andreh users import "./polyfills"; export * from "./typescript-utility-types"; export * from "./fetch"; export * from "./utils"; //# sourceMappingURL=index.d.ts.map apollo-server-demo/node_modules/apollo-env/lib/utils/ 0000755 0001750 0000144 00000000000 14067647701 022425 5 ustar andreh users apollo-server-demo/node_modules/apollo-env/lib/utils/predicates.d.ts 0000644 0001750 0000144 00000000202 03560116604 025321 0 ustar andreh users export declare function isNotNullOrUndefined<T>(value: T | null | undefined): value is T; //# sourceMappingURL=predicates.d.ts.map apollo-server-demo/node_modules/apollo-env/lib/utils/createHash.js 0000644 0001750 0000144 00000000547 03560116604 025025 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); const isNodeLike_1 = require("./isNodeLike"); function createHash(kind) { if (isNodeLike_1.isNodeLike) { return module.require("crypto").createHash(kind); } return require("sha.js")(kind); } exports.createHash = createHash; //# sourceMappingURL=createHash.js.map apollo-server-demo/node_modules/apollo-env/lib/utils/index.js 0000644 0001750 0000144 00000000532 03560116604 024057 0 ustar andreh users "use strict"; function __export(m) { for (var p in m) if (!exports.hasOwnProperty(p)) exports[p] = m[p]; } Object.defineProperty(exports, "__esModule", { value: true }); __export(require("./createHash")); __export(require("./isNodeLike")); __export(require("./mapValues")); __export(require("./predicates")); //# sourceMappingURL=index.js.map apollo-server-demo/node_modules/apollo-env/lib/utils/createHash.js.map 0000644 0001750 0000144 00000000513 03560116604 025572 0 ustar andreh users {"version":3,"file":"createHash.js","sourceRoot":"","sources":["../../src/utils/createHash.ts"],"names":[],"mappings":";;AAAA,6CAA0C;AAE1C,SAAgB,UAAU,CAAC,IAAY;IACrC,IAAI,uBAAU,EAAE;QAGd,OAAO,MAAM,CAAC,OAAO,CAAC,QAAQ,CAAC,CAAC,UAAU,CAAC,IAAI,CAAC,CAAC;KAClD;IACD,OAAO,OAAO,CAAC,QAAQ,CAAC,CAAC,IAAI,CAAC,CAAC;AACjC,CAAC;AAPD,gCAOC"} apollo-server-demo/node_modules/apollo-env/lib/utils/createHash.d.ts.map 0000644 0001750 0000144 00000000276 03560116604 026034 0 ustar andreh users {"version":3,"file":"createHash.d.ts","sourceRoot":"","sources":["../../src/utils/createHash.ts"],"names":[],"mappings":"AAEA,wBAAgB,UAAU,CAAC,IAAI,EAAE,MAAM,GAAG,OAAO,QAAQ,EAAE,IAAI,CAO9D"} apollo-server-demo/node_modules/apollo-env/lib/utils/isNodeLike.d.ts.map 0000644 0001750 0000144 00000000231 03560116604 026002 0 ustar andreh users {"version":3,"file":"isNodeLike.d.ts","sourceRoot":"","sources":["../../src/utils/isNodeLike.ts"],"names":[],"mappings":"AAAA,eAAO,MAAM,UAAU,SAKoB,CAAC"} apollo-server-demo/node_modules/apollo-env/lib/utils/createHash.d.ts 0000644 0001750 0000144 00000000161 03560116604 025251 0 ustar andreh users export declare function createHash(kind: string): import("crypto").Hash; //# sourceMappingURL=createHash.d.ts.map apollo-server-demo/node_modules/apollo-env/lib/utils/isNodeLike.d.ts 0000644 0001750 0000144 00000000122 03560116604 025225 0 ustar andreh users export declare const isNodeLike: boolean; //# sourceMappingURL=isNodeLike.d.ts.map apollo-server-demo/node_modules/apollo-env/lib/utils/isNodeLike.js 0000644 0001750 0000144 00000000424 03560116604 024776 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); exports.isNodeLike = typeof process === "object" && process && process.release && process.versions && typeof process.versions.node === "string"; //# sourceMappingURL=isNodeLike.js.map apollo-server-demo/node_modules/apollo-env/lib/utils/index.d.ts 0000644 0001750 0000144 00000000232 03560116604 024310 0 ustar andreh users export * from "./createHash"; export * from "./isNodeLike"; export * from "./mapValues"; export * from "./predicates"; //# sourceMappingURL=index.d.ts.map apollo-server-demo/node_modules/apollo-env/lib/utils/mapValues.js.map 0000644 0001750 0000144 00000000622 03560116604 025461 0 ustar andreh users {"version":3,"file":"mapValues.js","sourceRoot":"","sources":["../../src/utils/mapValues.ts"],"names":[],"mappings":";;AAAA,SAAgB,SAAS,CACvB,MAAyB,EACzB,QAAyB;IAEzB,MAAM,MAAM,GAAsB,MAAM,CAAC,MAAM,CAAC,IAAI,CAAC,CAAC;IAEtD,KAAK,MAAM,CAAC,GAAG,EAAE,KAAK,CAAC,IAAI,MAAM,CAAC,OAAO,CAAC,MAAM,CAAC,EAAE;QACjD,MAAM,CAAC,GAAG,CAAC,GAAG,QAAQ,CAAC,KAAK,CAAC,CAAC;KAC/B;IAED,OAAO,MAAM,CAAC;AAChB,CAAC;AAXD,8BAWC"} apollo-server-demo/node_modules/apollo-env/lib/utils/index.d.ts.map 0000644 0001750 0000144 00000000303 03560116604 025063 0 ustar andreh users {"version":3,"file":"index.d.ts","sourceRoot":"","sources":["../../src/utils/index.ts"],"names":[],"mappings":"AAAA,cAAc,cAAc,CAAC;AAC7B,cAAc,cAAc,CAAC;AAC7B,cAAc,aAAa,CAAC;AAC5B,cAAc,cAAc,CAAC"} apollo-server-demo/node_modules/apollo-env/lib/utils/mapValues.d.ts 0000644 0001750 0000144 00000000235 03560116604 025141 0 ustar andreh users export declare function mapValues<T, U = T>(object: Record<string, T>, callback: (value: T) => U): Record<string, U>; //# sourceMappingURL=mapValues.d.ts.map apollo-server-demo/node_modules/apollo-env/lib/utils/predicates.js.map 0000644 0001750 0000144 00000000346 03560116604 025652 0 ustar andreh users {"version":3,"file":"predicates.js","sourceRoot":"","sources":["../../src/utils/predicates.ts"],"names":[],"mappings":";;AAAA,SAAgB,oBAAoB,CAClC,KAA2B;IAE3B,OAAO,KAAK,KAAK,IAAI,IAAI,OAAO,KAAK,KAAK,WAAW,CAAC;AACxD,CAAC;AAJD,oDAIC"} apollo-server-demo/node_modules/apollo-env/lib/utils/predicates.d.ts.map 0000644 0001750 0000144 00000000332 03560116604 026101 0 ustar andreh users {"version":3,"file":"predicates.d.ts","sourceRoot":"","sources":["../../src/utils/predicates.ts"],"names":[],"mappings":"AAAA,wBAAgB,oBAAoB,CAAC,CAAC,EACpC,KAAK,EAAE,CAAC,GAAG,IAAI,GAAG,SAAS,GAC1B,KAAK,IAAI,CAAC,CAEZ"} apollo-server-demo/node_modules/apollo-env/lib/utils/mapValues.d.ts.map 0000644 0001750 0000144 00000000455 03560116604 025721 0 ustar andreh users {"version":3,"file":"mapValues.d.ts","sourceRoot":"","sources":["../../src/utils/mapValues.ts"],"names":[],"mappings":"AAAA,wBAAgB,SAAS,CAAC,CAAC,EAAE,CAAC,GAAG,CAAC,EAChC,MAAM,EAAE,MAAM,CAAC,MAAM,EAAE,CAAC,CAAC,EACzB,QAAQ,EAAE,CAAC,KAAK,EAAE,CAAC,KAAK,CAAC,GACxB,MAAM,CAAC,MAAM,EAAE,CAAC,CAAC,CAQnB"} apollo-server-demo/node_modules/apollo-env/lib/utils/index.js.map 0000644 0001750 0000144 00000000246 03560116604 024635 0 ustar andreh users {"version":3,"file":"index.js","sourceRoot":"","sources":["../../src/utils/index.ts"],"names":[],"mappings":";;;;;AAAA,kCAA6B;AAC7B,kCAA6B;AAC7B,iCAA4B;AAC5B,kCAA6B"} apollo-server-demo/node_modules/apollo-env/lib/utils/isNodeLike.js.map 0000644 0001750 0000144 00000000411 03560116604 025546 0 ustar andreh users {"version":3,"file":"isNodeLike.js","sourceRoot":"","sources":["../../src/utils/isNodeLike.ts"],"names":[],"mappings":";;AAAa,QAAA,UAAU,GACrB,OAAO,OAAO,KAAK,QAAQ;IAC3B,OAAO;IACP,OAAO,CAAC,OAAO;IACf,OAAO,CAAC,QAAQ;IAChB,OAAO,OAAO,CAAC,QAAQ,CAAC,IAAI,KAAK,QAAQ,CAAC"} apollo-server-demo/node_modules/apollo-env/lib/utils/predicates.js 0000644 0001750 0000144 00000000414 03560116604 025072 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); function isNotNullOrUndefined(value) { return value !== null && typeof value !== "undefined"; } exports.isNotNullOrUndefined = isNotNullOrUndefined; //# sourceMappingURL=predicates.js.map apollo-server-demo/node_modules/apollo-env/lib/utils/mapValues.js 0000644 0001750 0000144 00000000533 03560116604 024706 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); function mapValues(object, callback) { const result = Object.create(null); for (const [key, value] of Object.entries(object)) { result[key] = callback(value); } return result; } exports.mapValues = mapValues; //# sourceMappingURL=mapValues.js.map apollo-server-demo/node_modules/apollo-env/lib/index.d.ts.map 0000644 0001750 0000144 00000000274 03560116604 023732 0 ustar andreh users {"version":3,"file":"index.d.ts","sourceRoot":"","sources":["../src/index.ts"],"names":[],"mappings":"AAAA,OAAO,aAAa,CAAC;AAErB,cAAc,4BAA4B,CAAC;AAC3C,cAAc,SAAS,CAAC;AACxB,cAAc,SAAS,CAAC"} apollo-server-demo/node_modules/apollo-env/lib/typescript-utility-types.d.ts.map 0000644 0001750 0000144 00000000566 03560116604 027700 0 ustar andreh users {"version":3,"file":"typescript-utility-types.d.ts","sourceRoot":"","sources":["../src/typescript-utility-types.ts"],"names":[],"mappings":"AAAA,oBAAY,YAAY,CAAC,CAAC,EAAE,CAAC,SAAS,MAAM,CAAC,IAAI,CAAC,GAAG,QAAQ,CAAC,IAAI,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC,CAAC;AAC1E,oBAAY,WAAW,CAAC,CAAC,IAAI;KAAG,CAAC,IAAI,MAAM,CAAC,CAAC,CAAC,EAAE,WAAW,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;CAAE,CAAC"} apollo-server-demo/node_modules/apollo-env/lib/typescript-utility-types.d.ts 0000644 0001750 0000144 00000000333 03560116604 027114 0 ustar andreh users export declare type WithRequired<T, K extends keyof T> = T & Required<Pick<T, K>>; export declare type DeepPartial<T> = { [P in keyof T]?: DeepPartial<T[P]>; }; //# sourceMappingURL=typescript-utility-types.d.ts.map apollo-server-demo/node_modules/apollo-env/lib/typescript-utility-types.js 0000644 0001750 0000144 00000000201 03560116604 026652 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); //# sourceMappingURL=typescript-utility-types.js.map apollo-server-demo/node_modules/apollo-env/lib/index.js.map 0000644 0001750 0000144 00000000220 03560116604 023465 0 ustar andreh users {"version":3,"file":"index.js","sourceRoot":"","sources":["../src/index.ts"],"names":[],"mappings":";;;;;AAAA,uBAAqB;AAGrB,6BAAwB;AACxB,6BAAwB"} apollo-server-demo/node_modules/apollo-env/lib/fetch/ 0000755 0001750 0000144 00000000000 14067647701 022356 5 ustar andreh users apollo-server-demo/node_modules/apollo-env/lib/fetch/fetch.d.ts 0000644 0001750 0000144 00000001172 03560116604 024227 0 ustar andreh users /// <reference types="node" /> import { Agent as HttpAgent } from "http"; import { Agent as HttpsAgent } from "https"; export declare type RequestAgent = HttpAgent | HttpsAgent; export declare type ReferrerPolicy = "" | "no-referrer" | "no-referrer-when-downgrade" | "same-origin" | "origin" | "strict-origin" | "origin-when-cross-origin" | "strict-origin-when-cross-origin" | "unsafe-url"; export { default as fetch, Request, Response, Headers, ResponseInit, BodyInit, RequestInfo, HeadersInit, Body, RequestInit, RequestMode, RequestCredentials, RequestCache, RequestRedirect } from "node-fetch"; //# sourceMappingURL=fetch.d.ts.map apollo-server-demo/node_modules/apollo-env/lib/fetch/index.js 0000644 0001750 0000144 00000000411 03560116604 024004 0 ustar andreh users "use strict"; function __export(m) { for (var p in m) if (!exports.hasOwnProperty(p)) exports[p] = m[p]; } Object.defineProperty(exports, "__esModule", { value: true }); __export(require("./fetch")); __export(require("./url")); //# sourceMappingURL=index.js.map apollo-server-demo/node_modules/apollo-env/lib/fetch/index.d.ts 0000644 0001750 0000144 00000000123 03560116604 024240 0 ustar andreh users export * from "./fetch"; export * from "./url"; //# sourceMappingURL=index.d.ts.map apollo-server-demo/node_modules/apollo-env/lib/fetch/fetch.d.ts.map 0000644 0001750 0000144 00000001064 03560116604 025003 0 ustar andreh users {"version":3,"file":"fetch.d.ts","sourceRoot":"","sources":["../../src/fetch/fetch.ts"],"names":[],"mappings":";AAAA,OAAO,EAAE,KAAK,IAAI,SAAS,EAAE,MAAM,MAAM,CAAC;AAC1C,OAAO,EAAE,KAAK,IAAI,UAAU,EAAE,MAAM,OAAO,CAAC;AAE5C,oBAAY,YAAY,GAAG,SAAS,GAAG,UAAU,CAAC;AAElD,oBAAY,cAAc,GACtB,EAAE,GACF,aAAa,GACb,4BAA4B,GAC5B,aAAa,GACb,QAAQ,GACR,eAAe,GACf,0BAA0B,GAC1B,iCAAiC,GACjC,YAAY,CAAC;AAEjB,OAAO,EACL,OAAO,IAAI,KAAK,EAChB,OAAO,EACP,QAAQ,EACR,OAAO,EACP,YAAY,EACZ,QAAQ,EACR,WAAW,EACX,WAAW,EACX,IAAI,EACJ,WAAW,EACX,WAAW,EACX,kBAAkB,EAClB,YAAY,EACZ,eAAe,EAChB,MAAM,YAAY,CAAC"} apollo-server-demo/node_modules/apollo-env/lib/fetch/index.d.ts.map 0000644 0001750 0000144 00000000231 03560116604 025014 0 ustar andreh users {"version":3,"file":"index.d.ts","sourceRoot":"","sources":["../../src/fetch/index.ts"],"names":[],"mappings":"AAAA,cAAc,SAAS,CAAC;AACxB,cAAc,OAAO,CAAC"} apollo-server-demo/node_modules/apollo-env/lib/fetch/global.js 0000644 0001750 0000144 00000000060 03560116604 024135 0 ustar andreh users "use strict"; //# sourceMappingURL=global.js.map apollo-server-demo/node_modules/apollo-env/lib/fetch/url.d.ts 0000644 0001750 0000144 00000000506 03560116604 023740 0 ustar andreh users /// <reference types="node" /> import { URLSearchParams } from "url"; export { URL, URLSearchParams } from "url"; export declare type URLSearchParamsInit = URLSearchParams | string | { [key: string]: Object | Object[] | undefined; } | Iterable<[string, Object]> | Array<[string, Object]>; //# sourceMappingURL=url.d.ts.map apollo-server-demo/node_modules/apollo-env/lib/fetch/global.d.ts 0000644 0001750 0000144 00000002306 03560116604 024376 0 ustar andreh users declare function fetch(input?: RequestInfo, init?: RequestInit): Promise<Response>; declare interface GlobalFetch { fetch(input: RequestInfo, init?: RequestInit): Promise<Response>; } declare type RequestInfo = import("./fetch").RequestInfo; declare type Headers = import("./fetch").Headers; declare type HeadersInit = import("./fetch").HeadersInit; declare type Body = import("./fetch").Body; declare type Request = import("./fetch").Request; declare type RequestAgent = import("./fetch").RequestAgent; declare type RequestInit = import("./fetch").RequestInit; declare type RequestMode = import("./fetch").RequestMode; declare type RequestCredentials = import("./fetch").RequestCredentials; declare type RequestCache = import("./fetch").RequestCache; declare type RequestRedirect = import("./fetch").RequestRedirect; declare type ReferrerPolicy = import("./fetch").ReferrerPolicy; declare type Response = import("./fetch").Response; declare type ResponseInit = import("./fetch").ResponseInit; declare type BodyInit = import("./fetch").BodyInit; declare type URLSearchParams = import("./url").URLSearchParams; declare type URLSearchParamsInit = import("./url").URLSearchParamsInit; //# sourceMappingURL=global.d.ts.map apollo-server-demo/node_modules/apollo-env/lib/fetch/global.js.map 0000644 0001750 0000144 00000000161 03560116604 024713 0 ustar andreh users {"version":3,"file":"global.js","sourceRoot":"","sources":["../../src/fetch/global.ts"],"names":[],"mappings":""} apollo-server-demo/node_modules/apollo-env/lib/fetch/global.d.ts.map 0000644 0001750 0000144 00000002137 03560116604 025154 0 ustar andreh users {"version":3,"file":"global.d.ts","sourceRoot":"","sources":["../../src/fetch/global.ts"],"names":[],"mappings":"AAAA,OAAO,UAAU,KAAK,CACpB,KAAK,CAAC,EAAE,WAAW,EACnB,IAAI,CAAC,EAAE,WAAW,GACjB,OAAO,CAAC,QAAQ,CAAC,CAAC;AAErB,OAAO,WAAW,WAAW;IAC3B,KAAK,CAAC,KAAK,EAAE,WAAW,EAAE,IAAI,CAAC,EAAE,WAAW,GAAG,OAAO,CAAC,QAAQ,CAAC,CAAC;CAClE;AAED,aAAK,WAAW,GAAG,OAAO,SAAS,EAAE,WAAW,CAAC;AACjD,aAAK,OAAO,GAAG,OAAO,SAAS,EAAE,OAAO,CAAC;AACzC,aAAK,WAAW,GAAG,OAAO,SAAS,EAAE,WAAW,CAAC;AACjD,aAAK,IAAI,GAAG,OAAO,SAAS,EAAE,IAAI,CAAC;AACnC,aAAK,OAAO,GAAG,OAAO,SAAS,EAAE,OAAO,CAAC;AACzC,aAAK,YAAY,GAAG,OAAO,SAAS,EAAE,YAAY,CAAC;AACnD,aAAK,WAAW,GAAG,OAAO,SAAS,EAAE,WAAW,CAAC;AACjD,aAAK,WAAW,GAAG,OAAO,SAAS,EAAE,WAAW,CAAC;AACjD,aAAK,kBAAkB,GAAG,OAAO,SAAS,EAAE,kBAAkB,CAAC;AAC/D,aAAK,YAAY,GAAG,OAAO,SAAS,EAAE,YAAY,CAAC;AACnD,aAAK,eAAe,GAAG,OAAO,SAAS,EAAE,eAAe,CAAC;AACzD,aAAK,cAAc,GAAG,OAAO,SAAS,EAAE,cAAc,CAAC;AACvD,aAAK,QAAQ,GAAG,OAAO,SAAS,EAAE,QAAQ,CAAC;AAC3C,aAAK,YAAY,GAAG,OAAO,SAAS,EAAE,YAAY,CAAC;AACnD,aAAK,QAAQ,GAAG,OAAO,SAAS,EAAE,QAAQ,CAAC;AAC3C,aAAK,eAAe,GAAG,OAAO,OAAO,EAAE,eAAe,CAAC;AACvD,aAAK,mBAAmB,GAAG,OAAO,OAAO,EAAE,mBAAmB,CAAC"} apollo-server-demo/node_modules/apollo-env/lib/fetch/url.js.map 0000644 0001750 0000144 00000000243 03560116604 024256 0 ustar andreh users {"version":3,"file":"url.js","sourceRoot":"","sources":["../../src/fetch/url.ts"],"names":[],"mappings":";;AACA,2BAA2C;AAAlC,oBAAA,GAAG,CAAA;AAAE,gCAAA,eAAe,CAAA"} apollo-server-demo/node_modules/apollo-env/lib/fetch/fetch.js 0000644 0001750 0000144 00000000532 03560116604 023772 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); var node_fetch_1 = require("node-fetch"); exports.fetch = node_fetch_1.default; exports.Request = node_fetch_1.Request; exports.Response = node_fetch_1.Response; exports.Headers = node_fetch_1.Headers; exports.Body = node_fetch_1.Body; //# sourceMappingURL=fetch.js.map apollo-server-demo/node_modules/apollo-env/lib/fetch/index.js.map 0000644 0001750 0000144 00000000214 03560116604 024561 0 ustar andreh users {"version":3,"file":"index.js","sourceRoot":"","sources":["../../src/fetch/index.ts"],"names":[],"mappings":";;;;;AAAA,6BAAwB;AACxB,2BAAsB"} apollo-server-demo/node_modules/apollo-env/lib/fetch/url.d.ts.map 0000644 0001750 0000144 00000000634 03560116604 024516 0 ustar andreh users {"version":3,"file":"url.d.ts","sourceRoot":"","sources":["../../src/fetch/url.ts"],"names":[],"mappings":";AAAA,OAAO,EAAE,eAAe,EAAE,MAAM,KAAK,CAAC;AACtC,OAAO,EAAE,GAAG,EAAE,eAAe,EAAE,MAAM,KAAK,CAAC;AAE3C,oBAAY,mBAAmB,GAC3B,eAAe,GACf,MAAM,GACN;IAAE,CAAC,GAAG,EAAE,MAAM,GAAG,MAAM,GAAG,MAAM,EAAE,GAAG,SAAS,CAAA;CAAE,GAChD,QAAQ,CAAC,CAAC,MAAM,EAAE,MAAM,CAAC,CAAC,GAC1B,KAAK,CAAC,CAAC,MAAM,EAAE,MAAM,CAAC,CAAC,CAAC"} apollo-server-demo/node_modules/apollo-env/lib/fetch/url.js 0000644 0001750 0000144 00000000322 03560116604 023500 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); var url_1 = require("url"); exports.URL = url_1.URL; exports.URLSearchParams = url_1.URLSearchParams; //# sourceMappingURL=url.js.map apollo-server-demo/node_modules/apollo-env/lib/fetch/fetch.js.map 0000644 0001750 0000144 00000000350 03560116604 024544 0 ustar andreh users {"version":3,"file":"fetch.js","sourceRoot":"","sources":["../../src/fetch/fetch.ts"],"names":[],"mappings":";;AAgBA,yCAeoB;AAdlB,6BAAA,OAAO,CAAS;AAChB,+BAAA,OAAO,CAAA;AACP,gCAAA,QAAQ,CAAA;AACR,+BAAA,OAAO,CAAA;AAKP,4BAAA,IAAI,CAAA"} apollo-server-demo/node_modules/apollo-env/tsconfig.tsbuildinfo 0000644 0001750 0000144 00000066203 03560116604 024573 0 ustar andreh users { "program": { "fileInfos": { "../../node_modules/typescript/lib/lib.es5.d.ts": { "version": "fc43680ad3a1a4ec8c7b8d908af1ec9ddff87845346de5f02c735c9171fa98ea", "signature": "fc43680ad3a1a4ec8c7b8d908af1ec9ddff87845346de5f02c735c9171fa98ea" }, "../../node_modules/typescript/lib/lib.es2015.d.ts": { "version": "7994d44005046d1413ea31d046577cdda33b8b2470f30281fd9c8b3c99fe2d96", "signature": "7994d44005046d1413ea31d046577cdda33b8b2470f30281fd9c8b3c99fe2d96" }, "../../node_modules/typescript/lib/lib.es2016.d.ts": { "version": "5f217838d25704474d9ef93774f04164889169ca31475fe423a9de6758f058d1", "signature": "5f217838d25704474d9ef93774f04164889169ca31475fe423a9de6758f058d1" }, "../../node_modules/typescript/lib/lib.es2017.d.ts": { "version": "459097c7bdd88fc5731367e56591e4f465f2c9de81a35427a7bd473165c34743", "signature": "459097c7bdd88fc5731367e56591e4f465f2c9de81a35427a7bd473165c34743" }, "../../node_modules/typescript/lib/lib.es2015.core.d.ts": { "version": "734ddc145e147fbcd55f07d034f50ccff1086f5a880107665ec326fb368876f6", "signature": "734ddc145e147fbcd55f07d034f50ccff1086f5a880107665ec326fb368876f6" }, "../../node_modules/typescript/lib/lib.es2015.collection.d.ts": { "version": "4a0862a21f4700de873db3b916f70e41570e2f558da77d2087c9490f5a0615d8", "signature": "4a0862a21f4700de873db3b916f70e41570e2f558da77d2087c9490f5a0615d8" }, "../../node_modules/typescript/lib/lib.es2015.generator.d.ts": { "version": "765e0e9c9d74cf4d031ca8b0bdb269a853e7d81eda6354c8510218d03db12122", "signature": "765e0e9c9d74cf4d031ca8b0bdb269a853e7d81eda6354c8510218d03db12122" }, "../../node_modules/typescript/lib/lib.es2015.iterable.d.ts": { "version": "285958e7699f1babd76d595830207f18d719662a0c30fac7baca7df7162a9210", "signature": "285958e7699f1babd76d595830207f18d719662a0c30fac7baca7df7162a9210" }, "../../node_modules/typescript/lib/lib.es2015.promise.d.ts": { "version": "d4deaafbb18680e3143e8b471acd650ed6f72a408a33137f0a0dd104fbe7f8ca", "signature": "d4deaafbb18680e3143e8b471acd650ed6f72a408a33137f0a0dd104fbe7f8ca" }, "../../node_modules/typescript/lib/lib.es2015.proxy.d.ts": { "version": "5e72f949a89717db444e3bd9433468890068bb21a5638d8ab15a1359e05e54fe", "signature": "5e72f949a89717db444e3bd9433468890068bb21a5638d8ab15a1359e05e54fe" }, "../../node_modules/typescript/lib/lib.es2015.reflect.d.ts": { "version": "f5b242136ae9bfb1cc99a5971cccc44e99947ae6b5ef6fd8aa54b5ade553b976", "signature": "f5b242136ae9bfb1cc99a5971cccc44e99947ae6b5ef6fd8aa54b5ade553b976" }, "../../node_modules/typescript/lib/lib.es2015.symbol.d.ts": { "version": "9ae2860252d6b5f16e2026d8a2c2069db7b2a3295e98b6031d01337b96437230", "signature": "9ae2860252d6b5f16e2026d8a2c2069db7b2a3295e98b6031d01337b96437230" }, "../../node_modules/typescript/lib/lib.es2015.symbol.wellknown.d.ts": { "version": "3e0a459888f32b42138d5a39f706ff2d55d500ab1031e0988b5568b0f67c2303", "signature": "3e0a459888f32b42138d5a39f706ff2d55d500ab1031e0988b5568b0f67c2303" }, "../../node_modules/typescript/lib/lib.es2016.array.include.d.ts": { "version": "3f96f1e570aedbd97bf818c246727151e873125d0512e4ae904330286c721bc0", "signature": "3f96f1e570aedbd97bf818c246727151e873125d0512e4ae904330286c721bc0" }, "../../node_modules/typescript/lib/lib.es2017.object.d.ts": { "version": "c2d60b2e558d44384e4704b00e6b3d154334721a911f094d3133c35f0917b408", "signature": "c2d60b2e558d44384e4704b00e6b3d154334721a911f094d3133c35f0917b408" }, "../../node_modules/typescript/lib/lib.es2017.sharedmemory.d.ts": { "version": "b8667586a618c5cf64523d4e500ae39e781428abfb28f3de441fc66b56144b6f", "signature": "b8667586a618c5cf64523d4e500ae39e781428abfb28f3de441fc66b56144b6f" }, "../../node_modules/typescript/lib/lib.es2017.string.d.ts": { "version": "21df2e0059f14dcb4c3a0e125859f6b6ff01332ee24b0065a741d121250bc71c", "signature": "21df2e0059f14dcb4c3a0e125859f6b6ff01332ee24b0065a741d121250bc71c" }, "../../node_modules/typescript/lib/lib.es2017.intl.d.ts": { "version": "c1759cb171c7619af0d2234f2f8fb2a871ee88e956e2ed91bb61778e41f272c6", "signature": "c1759cb171c7619af0d2234f2f8fb2a871ee88e956e2ed91bb61778e41f272c6" }, "../../node_modules/typescript/lib/lib.es2017.typedarrays.d.ts": { "version": "28569d59e07d4378cb3d54979c4c60f9f06305c9bb6999ffe6cab758957adc46", "signature": "28569d59e07d4378cb3d54979c4c60f9f06305c9bb6999ffe6cab758957adc46" }, "../../node_modules/typescript/lib/lib.es2018.asynciterable.d.ts": { "version": "85085a0783532dc04b66894748dc4a49983b2fbccb0679b81356947021d7a215", "signature": "85085a0783532dc04b66894748dc4a49983b2fbccb0679b81356947021d7a215" }, "../../node_modules/typescript/lib/lib.es2019.array.d.ts": { "version": "7054111c49ea06f0f2e623eab292a9c1ae9b7d04854bd546b78f2b8b57e13d13", "signature": "7054111c49ea06f0f2e623eab292a9c1ae9b7d04854bd546b78f2b8b57e13d13" }, "./src/polyfills/array.ts": { "version": "5e4f637eab5a63e7916f07886ee9b8aa0da7ae9bfe9e51d78fb8c11736cf1663", "signature": "86abf2ee3ef84a8d40947efc8141596e7b071239c3649bbe05c3b7cbf4ff25aa" }, "./src/polyfills/object.ts": { "version": "7d8d9bb30b4d13d1a5af1e84b3dc2427994fdbed38638f8ff3df22c631edad4b", "signature": "7e834906dceaaa112cc0ee0cbc277d0688cb2401d5d22d1cc8fd50982895507d" }, "./src/polyfills/index.ts": { "version": "7e4738201f97ec91ae6dbd2b5fdbe4ed91aaf9f0e504b99ea2dc24219412f7b3", "signature": "b97a55b37476e5d8a355ff53ce54d39d93e04326f286a28c72b1631bc489c7e4" }, "./src/typescript-utility-types.ts": { "version": "2fa65536f87a0d6c3c4fb173bafd9bdbc075937ae86a0bb85712ff5cbae79eb6", "signature": "644f7a7f1a6918d41a4549f24b73d8423512ef64bf00ede77c6f496429f653ce" }, "../../node_modules/@types/events/index.d.ts": { "version": "400db42c3a46984118bff14260d60cec580057dc1ab4c2d7310beb643e4f5935", "signature": "400db42c3a46984118bff14260d60cec580057dc1ab4c2d7310beb643e4f5935" }, "../../node_modules/@types/node/inspector.d.ts": { "version": "7e49dbf1543b3ee54853ade4c5e9fa460b6a4eca967efe6bf943e0c505d087ed", "signature": "7e49dbf1543b3ee54853ade4c5e9fa460b6a4eca967efe6bf943e0c505d087ed" }, "../../node_modules/@types/node/base.d.ts": { "version": "39daac3cc4e13d9f1031c4b208c4cd10cb206782a381e71dbaa2353d170b41b4", "signature": "39daac3cc4e13d9f1031c4b208c4cd10cb206782a381e71dbaa2353d170b41b4" }, "../../node_modules/@types/node/ts3.2/index.d.ts": { "version": "1de0ff6200b92798a5aef43f57029c79dbf69932037dee1c007fdd2c562db258", "signature": "1de0ff6200b92798a5aef43f57029c79dbf69932037dee1c007fdd2c562db258" }, "../../node_modules/@types/node-fetch/node_modules/form-data/index.d.ts": { "version": "cbb7029e32a6a72178cda8baa9129b1ee6d1d779a35e46c780e38b4909d42a89", "signature": "cbb7029e32a6a72178cda8baa9129b1ee6d1d779a35e46c780e38b4909d42a89" }, "../../node_modules/@types/node-fetch/externals.d.ts": { "version": "972f1e91dab93b182624a17eeed02f683b8cb3fefbda7b689cc84570029d5f73", "signature": "972f1e91dab93b182624a17eeed02f683b8cb3fefbda7b689cc84570029d5f73" }, "../../node_modules/@types/node-fetch/index.d.ts": { "version": "f51382950fa81e3a54e9fd9f343fe583cfbb221f15a51936e12411699347effe", "signature": "f51382950fa81e3a54e9fd9f343fe583cfbb221f15a51936e12411699347effe" }, "./src/fetch/fetch.ts": { "version": "bdef9550ac994393927fd97ce62c122c4c870a43144391cd28a88ccfb6945b35", "signature": "97b59dfb4a4ce0f64e56899f781dfc88c6980da0d6579e90a9419326ef1ea527" }, "./src/fetch/url.ts": { "version": "e976710e1c2d5793796dbadd507f8b6c054938ceeb1bdaba9042b65d2f86da75", "signature": "f2266e9e985339c2bce2521131502b6de12ce658c71f75fb23518ae73060aa23" }, "./src/fetch/index.ts": { "version": "b7129b2d214aab183347c7212528591d7dcb1e3bf02a66205c4d137a6e7a375d", "signature": "0f397719640ef73aaff6344f5b3fc27c69d0850dcd3f20854e322671cc8da5ae" }, "./src/utils/isNodeLike.ts": { "version": "c426e98f38047b223fa8b7df6d312707d87b7c58901665e408ab75d53e009dd6", "signature": "69edcc3a497cf02aba61a4a0e602786a8ae1dc2e650b54e89f8e19a75ef9d62f" }, "./src/utils/createHash.ts": { "version": "f79cbe092445e25090fbbae7aed63c5706313621b5949bd876b26d4a8e0c3275", "signature": "134fce06b423352ff05e15abd8d25f766e0ebcc939a4b80bb5464b7c37795f0e" }, "./src/utils/mapValues.ts": { "version": "af281a979620cd92701733b09c5d6459ae3b50427934a34ed24b9c17436b7af9", "signature": "b5e284217b0f1b760fbecd6e5bd72d8e7292d6d2a91131718a1617a7600895fc" }, "./src/utils/predicates.ts": { "version": "fb1cf51797e17db9546d8d3f8cfba424ac5574cf8aee5b7d5d2a9f782c2d4f7b", "signature": "bbe60ef612ccc9b627648fd0d56e4e04c52bf670c25c4cd2b681cdbedfe16927" }, "./src/utils/index.ts": { "version": "a451f2125572fc1d7ad968cc5d12572a841ece6c66e319bb75bbbc4fc9e2ef28", "signature": "bfb6158d32d15a5f518529d2941030215c6d6d31ba468faf805a427a9056f192" }, "./src/index.ts": { "version": "f3d90ad0c87d3210b99535483c8c12542012daee0d1996045de53f028c0f12bc", "signature": "0115d4b56667395d868039054ea4487f0cd08c7d93364d957bc27d6ddc3803a7" }, "./src/fetch/global.ts": { "version": "c5bac4724d17fe10c11e33616db249786f4e7ccf737c85c6625a62d84b64b40d", "signature": "c04b0cf66ca3ca383bbb435449533e4d999b75edba454c4654b1e9a412e6f694" } }, "options": { "composite": true, "target": 4, "module": 1, "moduleResolution": 2, "esModuleInterop": true, "sourceMap": true, "declaration": true, "declarationMap": true, "removeComments": true, "strict": true, "noImplicitAny": true, "noImplicitReturns": true, "noFallthroughCasesInSwitch": true, "noUnusedParameters": false, "noUnusedLocals": false, "forceConsistentCasingInFileNames": true, "lib": [ "lib.es2017.d.ts", "lib.es2018.asynciterable.d.ts" ], "types": [ "node" ], "baseUrl": "../..", "paths": { "*": [ "types/*" ] }, "rootDir": "./src", "outDir": "./lib", "configFilePath": "./tsconfig.json" }, "referencedMap": { "../../node_modules/typescript/lib/lib.es5.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2015.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2016.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2017.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2015.core.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2015.collection.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2015.generator.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2015.iterable.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2015.promise.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2015.proxy.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2015.reflect.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2015.symbol.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2015.symbol.wellknown.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2016.array.include.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2017.object.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2017.sharedmemory.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2017.string.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2017.intl.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2017.typedarrays.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2018.asynciterable.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2019.array.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "./src/polyfills/array.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "./src/polyfills/object.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "./src/polyfills/index.ts": [ "./src/polyfills/array.ts", "./src/polyfills/object.ts", "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "./src/typescript-utility-types.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/@types/events/index.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/@types/node/inspector.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/@types/node/base.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/inspector.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/@types/node/ts3.2/index.d.ts": [ "../../node_modules/@types/node/base.d.ts" ], "../../node_modules/@types/node-fetch/node_modules/form-data/index.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/@types/node-fetch/externals.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/@types/node-fetch/index.d.ts": [ "../../node_modules/@types/node-fetch/node_modules/form-data/index.d.ts", "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node-fetch/externals.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "./src/fetch/fetch.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node-fetch/index.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "./src/fetch/url.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "./src/fetch/index.ts": [ "./src/fetch/fetch.ts", "./src/fetch/url.ts", "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "./src/utils/isNodeLike.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "./src/utils/createHash.ts": [ "./src/utils/isNodeLike.ts", "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "./src/utils/mapValues.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "./src/utils/predicates.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "./src/utils/index.ts": [ "./src/utils/createHash.ts", "./src/utils/isNodeLike.ts", "./src/utils/mapValues.ts", "./src/utils/predicates.ts", "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "./src/index.ts": [ "./src/polyfills/index.ts", "./src/typescript-utility-types.ts", "./src/fetch/index.ts", "./src/utils/index.ts", "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "./src/fetch/global.ts": [ "./src/fetch/fetch.ts", "./src/fetch/url.ts", "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ] }, "exportedModulesMap": { "../../node_modules/typescript/lib/lib.es5.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2015.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2016.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2017.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2015.core.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2015.collection.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2015.generator.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2015.iterable.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2015.promise.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2015.proxy.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2015.reflect.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2015.symbol.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2015.symbol.wellknown.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2016.array.include.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2017.object.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2017.sharedmemory.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2017.string.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2017.intl.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2017.typedarrays.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2018.asynciterable.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/typescript/lib/lib.es2019.array.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "./src/polyfills/index.ts": [ "./src/polyfills/array.ts", "./src/polyfills/object.ts" ], "./src/index.ts": [ "./src/polyfills/index.ts", "./src/typescript-utility-types.ts", "./src/fetch/index.ts", "./src/utils/index.ts" ], "../../node_modules/@types/events/index.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/@types/node/inspector.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/@types/node/base.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/inspector.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "./src/fetch/global.ts": [ "./src/fetch/fetch.ts", "./src/fetch/url.ts" ], "./src/utils/index.ts": [ "./src/utils/createHash.ts", "./src/utils/isNodeLike.ts", "./src/utils/mapValues.ts", "./src/utils/predicates.ts" ], "./src/utils/createHash.ts": [ "../../node_modules/@types/node/base.d.ts" ], "./src/fetch/index.ts": [ "./src/fetch/fetch.ts", "./src/fetch/url.ts" ], "./src/fetch/url.ts": [ "../../node_modules/@types/node/base.d.ts" ], "./src/fetch/fetch.ts": [ "../../node_modules/@types/node-fetch/index.d.ts", "../../node_modules/@types/node/base.d.ts" ], "../../node_modules/@types/node-fetch/index.d.ts": [ "../../node_modules/@types/node-fetch/node_modules/form-data/index.d.ts", "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node-fetch/externals.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/@types/node-fetch/externals.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/@types/node-fetch/node_modules/form-data/index.d.ts": [ "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts" ], "../../node_modules/@types/node/ts3.2/index.d.ts": [ "../../node_modules/@types/node/base.d.ts" ] }, "semanticDiagnosticsPerFile": [ "./src/polyfills/array.ts", "./src/polyfills/object.ts", "./src/polyfills/index.ts", "./src/typescript-utility-types.ts", "../../node_modules/@types/events/index.d.ts", "../../node_modules/@types/node/inspector.d.ts", "../../node_modules/@types/node/base.d.ts", "../../node_modules/@types/node/ts3.2/index.d.ts", "../../node_modules/@types/node-fetch/node_modules/form-data/index.d.ts", "../../node_modules/@types/node-fetch/externals.d.ts", "../../node_modules/@types/node-fetch/index.d.ts", "./src/fetch/fetch.ts", "./src/fetch/url.ts", "./src/fetch/index.ts", "./src/utils/isNodeLike.ts", "./src/utils/createHash.ts", "./src/utils/mapValues.ts", "./src/utils/predicates.ts", "./src/utils/index.ts", "./src/index.ts", "./src/fetch/global.ts", "../../node_modules/typescript/lib/lib.es2015.d.ts", "../../node_modules/typescript/lib/lib.es2016.d.ts", "../../node_modules/typescript/lib/lib.es2017.d.ts", "../../node_modules/typescript/lib/lib.es2019.array.d.ts", "../../node_modules/typescript/lib/lib.es2018.asynciterable.d.ts", "../../node_modules/typescript/lib/lib.es2017.typedarrays.d.ts", "../../node_modules/typescript/lib/lib.es2017.intl.d.ts", "../../node_modules/typescript/lib/lib.es2017.string.d.ts", "../../node_modules/typescript/lib/lib.es2017.sharedmemory.d.ts", "../../node_modules/typescript/lib/lib.es2017.object.d.ts", "../../node_modules/typescript/lib/lib.es2016.array.include.d.ts", "../../node_modules/typescript/lib/lib.es2015.symbol.wellknown.d.ts", "../../node_modules/typescript/lib/lib.es2015.symbol.d.ts", "../../node_modules/typescript/lib/lib.es2015.reflect.d.ts", "../../node_modules/typescript/lib/lib.es2015.proxy.d.ts", "../../node_modules/typescript/lib/lib.es2015.promise.d.ts", "../../node_modules/typescript/lib/lib.es2015.iterable.d.ts", "../../node_modules/typescript/lib/lib.es2015.generator.d.ts", "../../node_modules/typescript/lib/lib.es2015.collection.d.ts", "../../node_modules/typescript/lib/lib.es2015.core.d.ts", "../../node_modules/typescript/lib/lib.es5.d.ts" ] }, "version": "3.7.5" } apollo-server-demo/node_modules/is-date-object/ 0000755 0001750 0000144 00000000000 14067647701 021235 5 ustar andreh users apollo-server-demo/node_modules/is-date-object/index.js 0000644 0001750 0000144 00000001056 03560116604 022671 0 ustar andreh users 'use strict'; var getDay = Date.prototype.getDay; var tryDateObject = function tryDateGetDayCall(value) { try { getDay.call(value); return true; } catch (e) { return false; } }; var toStr = Object.prototype.toString; var dateClass = '[object Date]'; var hasToStringTag = typeof Symbol === 'function' && typeof Symbol.toStringTag === 'symbol'; module.exports = function isDateObject(value) { if (typeof value !== 'object' || value === null) { return false; } return hasToStringTag ? tryDateObject(value) : toStr.call(value) === dateClass; }; apollo-server-demo/node_modules/is-date-object/LICENSE 0000644 0001750 0000144 00000002072 03560116604 022230 0 ustar andreh users The MIT License (MIT) Copyright (c) 2015 Jordan Harband Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/is-date-object/.eslintrc 0000644 0001750 0000144 00000000127 03560116604 023046 0 ustar andreh users { "root": true, "extends": "@ljharb", "rules": { "max-statements": [2, 12] } } apollo-server-demo/node_modules/is-date-object/.github/ 0000755 0001750 0000144 00000000000 14067647701 022575 5 ustar andreh users apollo-server-demo/node_modules/is-date-object/.github/FUNDING.yml 0000644 0001750 0000144 00000001111 03560116604 024371 0 ustar andreh users # These are supported funding model platforms github: [ljharb] patreon: # Replace with a single Patreon username open_collective: # Replace with a single Open Collective username ko_fi: # Replace with a single Ko-fi username tidelift: npm/is-date-object community_bridge: # Replace with a single Community Bridge project-name e.g., cloud-foundry liberapay: # Replace with a single Liberapay username issuehunt: # Replace with a single IssueHunt username otechie: # Replace with a single Otechie username custom: # Replace with up to 4 custom sponsorship URLs e.g., ['link1', 'link2'] apollo-server-demo/node_modules/is-date-object/.github/workflows/ 0000755 0001750 0000144 00000000000 14067647701 024632 5 ustar andreh users apollo-server-demo/node_modules/is-date-object/.github/workflows/rebase.yml 0000644 0001750 0000144 00000000372 03560116604 026605 0 ustar andreh users name: Automatic Rebase on: [pull_request] jobs: _: name: "Automatic Rebase" runs-on: ubuntu-latest steps: - uses: actions/checkout@v1 - uses: ljharb/rebase@master env: GITHUB_TOKEN: ${{ secrets.GITHUB_TOKEN }} apollo-server-demo/node_modules/is-date-object/README.md 0000644 0001750 0000144 00000003327 03560116604 022506 0 ustar andreh users # is-date-object <sup>[![Version Badge][2]][1]</sup> [![Build Status][3]][4] [![dependency status][5]][6] [![dev dependency status][7]][8] [![License][license-image]][license-url] [![Downloads][downloads-image]][downloads-url] [![npm badge][11]][1] [![browser support][9]][10] Is this value a JS Date object? This module works cross-realm/iframe, and despite ES6 @@toStringTag. ## Example ```js var isDate = require('is-date-object'); var assert = require('assert'); assert.notOk(isDate(undefined)); assert.notOk(isDate(null)); assert.notOk(isDate(false)); assert.notOk(isDate(true)); assert.notOk(isDate(42)); assert.notOk(isDate('foo')); assert.notOk(isDate(function () {})); assert.notOk(isDate([])); assert.notOk(isDate({})); assert.notOk(isDate(/a/g)); assert.notOk(isDate(new RegExp('a', 'g'))); assert.ok(isDate(new Date())); ``` ## Tests Simply clone the repo, `npm install`, and run `npm test` [1]: https://npmjs.org/package/is-date-object [2]: http://versionbadg.es/ljharb/is-date-object.svg [3]: https://travis-ci.org/ljharb/is-date-object.svg [4]: https://travis-ci.org/ljharb/is-date-object [5]: https://david-dm.org/ljharb/is-date-object.svg [6]: https://david-dm.org/ljharb/is-date-object [7]: https://david-dm.org/ljharb/is-date-object/dev-status.svg [8]: https://david-dm.org/ljharb/is-date-object#info=devDependencies [9]: https://ci.testling.com/ljharb/is-date-object.png [10]: https://ci.testling.com/ljharb/is-date-object [11]: https://nodei.co/npm/is-date-object.png?downloads=true&stars=true [license-image]: http://img.shields.io/npm/l/is-date-object.svg [license-url]: LICENSE [downloads-image]: http://img.shields.io/npm/dm/is-date-object.svg [downloads-url]: http://npm-stat.com/charts.html?package=is-date-object apollo-server-demo/node_modules/is-date-object/package.json 0000644 0001750 0000144 00000003776 03560116604 023525 0 ustar andreh users { "name": "is-date-object", "version": "1.0.2", "author": "Jordan Harband", "funding": { "url": "https://github.com/sponsors/ljharb" }, "description": "Is this value a JS Date object? This module works cross-realm/iframe, and despite ES6 @@toStringTag.", "license": "MIT", "main": "index.js", "scripts": { "prepublish": "safe-publish-latest", "pretest": "npm run lint", "test": "npm run tests-only", "tests-only": "node --harmony --es-staging test", "posttest": "npx aud", "coverage": "covert test/index.js", "lint": "eslint .", "version": "auto-changelog && git add CHANGELOG.md", "postversion": "auto-changelog && git add CHANGELOG.md && git commit --no-edit --amend && git tag -f \"v$(node -e \"console.log(require('./package.json').version)\")\"" }, "repository": { "type": "git", "url": "git://github.com/ljharb/is-date-object.git" }, "keywords": [ "Date", "ES6", "toStringTag", "@@toStringTag", "Date object" ], "dependencies": {}, "devDependencies": { "@ljharb/eslint-config": "^15.0.2", "auto-changelog": "^1.16.2", "covert": "^1.1.1", "eslint": "^6.7.2", "foreach": "^2.0.5", "indexof": "^0.0.1", "is": "^3.3.0", "safe-publish-latest": "^1.1.4", "tape": "^4.12.0" }, "testling": { "files": "test/index.js", "browsers": [ "iexplore/6.0..latest", "firefox/3.0..6.0", "firefox/15.0..latest", "firefox/nightly", "chrome/4.0..10.0", "chrome/20.0..latest", "chrome/canary", "opera/10.0..latest", "opera/next", "safari/4.0..latest", "ipad/6.0..latest", "iphone/6.0..latest", "android-browser/4.2" ] }, "engines": { "node": ">= 0.4" }, "auto-changelog": { "output": "CHANGELOG.md", "template": "keepachangelog", "unreleased": false, "commitLimit": false, "backfillLimit": false } ,"_resolved": "https://registry.npmjs.org/is-date-object/-/is-date-object-1.0.2.tgz" ,"_integrity": "sha512-USlDT524woQ08aoZFzh3/Z6ch9Y/EWXEHQ/AaRN0SkKq4t2Jw2R2339tSXmwuVoY7LLlBCbOIlx2myP/L5zk0g==" ,"_from": "is-date-object@1.0.2" } apollo-server-demo/node_modules/is-date-object/test/ 0000755 0001750 0000144 00000000000 14067647701 022214 5 ustar andreh users apollo-server-demo/node_modules/is-date-object/test/index.js 0000644 0001750 0000144 00000002265 03560116604 023653 0 ustar andreh users 'use strict'; var test = require('tape'); var isDate = require('../'); var hasSymbols = typeof Symbol === 'function' && typeof Symbol('') === 'symbol'; test('not Dates', function (t) { t.notOk(isDate(), 'undefined is not Date'); t.notOk(isDate(null), 'null is not Date'); t.notOk(isDate(false), 'false is not Date'); t.notOk(isDate(true), 'true is not Date'); t.notOk(isDate(42), 'number is not Date'); t.notOk(isDate('foo'), 'string is not Date'); t.notOk(isDate([]), 'array is not Date'); t.notOk(isDate({}), 'object is not Date'); t.notOk(isDate(function () {}), 'function is not Date'); t.notOk(isDate(/a/g), 'regex literal is not Date'); t.notOk(isDate(new RegExp('a', 'g')), 'regex object is not Date'); t.end(); }); test('@@toStringTag', { skip: !hasSymbols || !Symbol.toStringTag }, function (t) { var realDate = new Date(); var fakeDate = { toString: function () { return String(realDate); }, valueOf: function () { return realDate.getTime(); } }; fakeDate[Symbol.toStringTag] = 'Date'; t.notOk(isDate(fakeDate), 'fake Date with @@toStringTag "Date" is not Date'); t.end(); }); test('Dates', function (t) { t.ok(isDate(new Date()), 'new Date() is Date'); t.end(); }); apollo-server-demo/node_modules/is-date-object/.jscs.json 0000644 0001750 0000144 00000010040 03560116604 023130 0 ustar andreh users { "es3": true, "additionalRules": [], "requireSemicolons": true, "disallowMultipleSpaces": true, "disallowIdentifierNames": [], "requireCurlyBraces": { "allExcept": [], "keywords": ["if", "else", "for", "while", "do", "try", "catch"] }, "requireSpaceAfterKeywords": ["if", "else", "for", "while", "do", "switch", "return", "try", "catch", "function"], "disallowSpaceAfterKeywords": [], "disallowSpaceBeforeComma": true, "disallowSpaceAfterComma": false, "disallowSpaceBeforeSemicolon": true, "disallowNodeTypes": [ "DebuggerStatement", "ForInStatement", "LabeledStatement", "SwitchCase", "SwitchStatement", "WithStatement" ], "requireObjectKeysOnNewLine": { "allExcept": ["sameLine"] }, "requireSpacesInAnonymousFunctionExpression": { "beforeOpeningRoundBrace": true, "beforeOpeningCurlyBrace": true }, "requireSpacesInNamedFunctionExpression": { "beforeOpeningCurlyBrace": true }, "disallowSpacesInNamedFunctionExpression": { "beforeOpeningRoundBrace": true }, "requireSpacesInFunctionDeclaration": { "beforeOpeningCurlyBrace": true }, "disallowSpacesInFunctionDeclaration": { "beforeOpeningRoundBrace": true }, "requireSpaceBetweenArguments": true, "disallowSpacesInsideParentheses": true, "disallowSpacesInsideArrayBrackets": true, "disallowQuotedKeysInObjects": { "allExcept": ["reserved"] }, "disallowSpaceAfterObjectKeys": true, "requireCommaBeforeLineBreak": true, "disallowSpaceAfterPrefixUnaryOperators": ["++", "--", "+", "-", "~", "!"], "requireSpaceAfterPrefixUnaryOperators": [], "disallowSpaceBeforePostfixUnaryOperators": ["++", "--"], "requireSpaceBeforePostfixUnaryOperators": [], "disallowSpaceBeforeBinaryOperators": [], "requireSpaceBeforeBinaryOperators": ["+", "-", "/", "*", "=", "==", "===", "!=", "!=="], "requireSpaceAfterBinaryOperators": ["+", "-", "/", "*", "=", "==", "===", "!=", "!=="], "disallowSpaceAfterBinaryOperators": [], "disallowImplicitTypeConversion": ["binary", "string"], "disallowKeywords": ["with", "eval"], "requireKeywordsOnNewLine": [], "disallowKeywordsOnNewLine": ["else"], "requireLineFeedAtFileEnd": true, "disallowTrailingWhitespace": true, "disallowTrailingComma": true, "excludeFiles": ["node_modules/**", "vendor/**"], "disallowMultipleLineStrings": true, "requireDotNotation": { "allExcept": ["keywords"] }, "requireParenthesesAroundIIFE": true, "validateLineBreaks": "LF", "validateQuoteMarks": { "escape": true, "mark": "'" }, "disallowOperatorBeforeLineBreak": [], "requireSpaceBeforeKeywords": [ "do", "for", "if", "else", "switch", "case", "try", "catch", "finally", "while", "with", "return" ], "validateAlignedFunctionParameters": { "lineBreakAfterOpeningBraces": true, "lineBreakBeforeClosingBraces": true }, "requirePaddingNewLinesBeforeExport": true, "validateNewlineAfterArrayElements": { "maximum": 1 }, "requirePaddingNewLinesAfterUseStrict": true, "disallowArrowFunctions": true, "disallowMultiLineTernary": true, "validateOrderInObjectKeys": "asc-insensitive", "disallowIdenticalDestructuringNames": true, "disallowNestedTernaries": { "maxLevel": 1 }, "requireSpaceAfterComma": { "allExcept": ["trailing"] }, "requireAlignedMultilineParams": false, "requireSpacesInGenerator": { "afterStar": true }, "disallowSpacesInGenerator": { "beforeStar": true }, "disallowVar": false, "requireArrayDestructuring": false, "requireEnhancedObjectLiterals": false, "requireObjectDestructuring": false, "requireEarlyReturn": false, "requireCapitalizedConstructorsNew": { "allExcept": ["Function", "String", "Object", "Symbol", "Number", "Date", "RegExp", "Error", "Boolean", "Array"] }, "requireImportAlphabetized": false, "requireSpaceBeforeObjectValues": true, "requireSpaceBeforeDestructuredValues": true, "disallowSpacesInsideTemplateStringPlaceholders": true, "disallowArrayDestructuringReturn": false, "requireNewlineBeforeSingleStatementsInIf": false, "disallowUnusedVariables": true, "requireSpacesInsideImportedObjectBraces": true, "requireUseStrict": true } apollo-server-demo/node_modules/is-date-object/CHANGELOG.md 0000644 0001750 0000144 00000021036 03560116604 023035 0 ustar andreh users # Changelog All notable changes to this project will be documented in this file. The format is based on [Keep a Changelog](https://keepachangelog.com/en/1.0.0/) and this project adheres to [Semantic Versioning](https://semver.org/spec/v2.0.0.html). Generated by [`auto-changelog`](https://github.com/CookPete/auto-changelog). ## [v1.0.2](https://github.com/inspect-js/is-date-object/compare/v1.0.1...v1.0.2) - 2019-12-19 ### Commits - [Tests] use shared travis-ci configs [`8a378b8`](https://github.com/inspect-js/is-date-object/commit/8a378b8fd6a4202fffc9ec193aca02efe937bc35) - [Tests] on all node minors; use `nvm install-latest-npm`; fix scripts; improve matrix [`6e97a21`](https://github.com/inspect-js/is-date-object/commit/6e97a21276cf448ce424fb9ea13edd4587f289f1) - [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `is`, `jscs`, `nsp`, `semver`, `tape` [`8472b90`](https://github.com/inspect-js/is-date-object/commit/8472b90f82e5153c22e7a8a7726a5cc6110e93d7) - [Tests] up to `node` `v10.0`, `v9.11`, `v8.11`, `v6.14`, `v4.9` [`ae73e38`](https://github.com/inspect-js/is-date-object/commit/ae73e3890df7da0bc4449088e30340cb4df3294d) - [meta] add `auto-changelog` [`82f8f47`](https://github.com/inspect-js/is-date-object/commit/82f8f473a6ee45e2b66810cb743e0122c18381c5) - [meta] remove unused Makefile and associated utilities [`788a2cd`](https://github.com/inspect-js/is-date-object/commit/788a2cdfd0bc8f1903967219897f6d00c4c6a26b) - [Tests] up to `node` `v11.4`, `v10.14`, `v8.14`, `v6.15` [`b9caf7c`](https://github.com/inspect-js/is-date-object/commit/b9caf7c814e5e2549454cb444f8b739f9ce1a388) - [Tests] up to `node` `v12.4`, `v11.15`, `v10.15`, `v8.15`, `v6.17`; use `nvm install-latest-npm` [`cda0abc`](https://github.com/inspect-js/is-date-object/commit/cda0abc04a21c9b5ec72eabd010155c988032056) - [Tests] up to `node` `v12.10`, `v10.16`, `v8.16` [`49bc482`](https://github.com/inspect-js/is-date-object/commit/49bc482fd9f71436b663c07144083a8423697299) - [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `semver`, `tape`; add `safe-publish-latest` [`f77fec4`](https://github.com/inspect-js/is-date-object/commit/f77fec48057e156b2276b4c14cf303306116b9f6) - [actions] add automatic rebasing / merge commit blocking [`68605fc`](https://github.com/inspect-js/is-date-object/commit/68605fcb6bc0341ff0aae14a94bf5d18e1bc73be) - [meta] create FUNDING.yml [`4f82d88`](https://github.com/inspect-js/is-date-object/commit/4f82d88e1e6ac1b97f0ce96aa0aa057ad758a581) - [Dev Deps] update `tape`, `jscs`, `eslint`, `@ljharb/eslint-config` [`3cbf28a`](https://github.com/inspect-js/is-date-object/commit/3cbf28a185ced940cfce8a09fa8479cc83575876) - [Dev Deps] update `eslint`, `@ljharb/eslint-config@`, `is`, `semver`, `tape` [`abf9fb0`](https://github.com/inspect-js/is-date-object/commit/abf9fb0d55ef0697e64e888d74f2e5fe53d7cdcb) - [Tests] switch from `nsp` to `npm audit` [`6543c7d`](https://github.com/inspect-js/is-date-object/commit/6543c7d559d1fb79215b46c8b79e0e3e2a83f5de) - [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `covert`, `tape` [`ba5d2d7`](https://github.com/inspect-js/is-date-object/commit/ba5d2d7fc0975d7c03b8f2b7f43a09af93e365ba) - [Dev Deps] update `eslint`, `nsp`, `semver`, `tape` [`c1e3525`](https://github.com/inspect-js/is-date-object/commit/c1e3525afa76a696f7cf1b58aab7f55d220b2c20) - [Tests] use `npx aud` instead of `nsp` or `npm audit` with hoops [`14e4824`](https://github.com/inspect-js/is-date-object/commit/14e4824188c85207ed3b86627b09e9f64b135db7) - [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `safe-publish-latest` [`68ead64`](https://github.com/inspect-js/is-date-object/commit/68ead64a07e0de282ea3cd38e12cc8b0e0f6d3cd) - [Dev Deps] update `eslint`, semver`, `tape`, `semver` [`f55453f`](https://github.com/inspect-js/is-date-object/commit/f55453f200903277465d7e9307a9c49120a4f419) - Only apps should have lockfiles [`6c848eb`](https://github.com/inspect-js/is-date-object/commit/6c848eba982cc58053d4cca08c01f12a433f3695) - [Tests] remove `jscs` [`3fd3a62`](https://github.com/inspect-js/is-date-object/commit/3fd3a62121607ad074b7fc977f3fc6575b66f755) - [Dev Deps] update `eslint`, `tape` [`77d3130`](https://github.com/inspect-js/is-date-object/commit/77d3130a0039e5dae24c17de790dd510c265edc6) - [meta] add `funding` field [`9ef6d58`](https://github.com/inspect-js/is-date-object/commit/9ef6d5888bf829a5812b3b091dc99839d48c355e) ## [v1.0.1](https://github.com/inspect-js/is-date-object/compare/v1.0.0...v1.0.1) - 2015-09-27 ### Commits - Update `tape`, `semver`, `eslint`; use my personal shared `eslint` config. [`731aa13`](https://github.com/inspect-js/is-date-object/commit/731aa134b0b8dc84e302d0b2264a415cb456ccab) - Update `is`, `tape`, `covert`, `jscs`, `editorconfig-tools`, `nsp`, `eslint`, `semver` [`53e43a6`](https://github.com/inspect-js/is-date-object/commit/53e43a627dd01757cf3d469599f3dffd9d72b150) - Update `eslint` [`d2fc304`](https://github.com/inspect-js/is-date-object/commit/d2fc3046f087b0026448ffde0cf46b1f741cbd4e) - Update `tape`, `jscs`, `eslint`, `@ljharb/eslint-config` [`c9568df`](https://github.com/inspect-js/is-date-object/commit/c9568df228fa698dc6fcc9553b5d612e7ee427aa) - Test on latest `node` and `io.js` versions. [`a21d537`](https://github.com/inspect-js/is-date-object/commit/a21d537562166ebd18bde3a262fd157dd774ae17) - Update `nsp`, `eslint`, `semver` [`9e1d908`](https://github.com/inspect-js/is-date-object/commit/9e1d9087c0c79c34fcb2abfc701cdfa1efcb327c) - Update `covert`, `jscs`, `eslint`, `semver` [`f198f6b`](https://github.com/inspect-js/is-date-object/commit/f198f6b997912da10a3d821a089e1581edc730a0) - [Dev Deps] update `tape`, `jscs`, `eslint` [`ab9bdbb`](https://github.com/inspect-js/is-date-object/commit/ab9bdbbc189cef033346508db47cd1feb04a69d3) - If `@@toStringTag` is not present, use the old-school `Object#toString` test. [`c03afce`](https://github.com/inspect-js/is-date-object/commit/c03afce001368b29eb929900075749b113a252c8) - [Dev Deps] update `jscs`, `nsp`, `tape`, `eslint`, `@ljharb/eslint-config` [`9d94ccb`](https://github.com/inspect-js/is-date-object/commit/9d94ccbab4160d2fa649123e37951d86b69a8b15) - [Dev Deps] update `is`, `eslint`, `@ljharb/eslint-config`, `semver` [`35cbff7`](https://github.com/inspect-js/is-date-object/commit/35cbff7f7c8216fbb79c799f74b2336eaf0d726a) - Test up to `io.js` `v2.3` [`be5d11e`](https://github.com/inspect-js/is-date-object/commit/be5d11e7ebd9473d7ae554179b3769082485f6f4) - [Tests] on `io.js` `v3.3`, up to `node` `v4.1` [`20221a3`](https://github.com/inspect-js/is-date-object/commit/20221a34858d2b21e23bdc2c08df23f0bc08d11e) - [Tests] up to `io.js` `v3.2 ` [`7009b4a`](https://github.com/inspect-js/is-date-object/commit/7009b4a9999e14eacbdf6068afd82f478473f007) - Test on `io.js` `v2.1` [`68b29b1`](https://github.com/inspect-js/is-date-object/commit/68b29b19a07e6589a7ca37ab764be28f144ac88e) - Remove `editorconfig-tools` [`8d3972c`](https://github.com/inspect-js/is-date-object/commit/8d3972c1795fdcfd337680e11ab610e4885fb079) - [Dev Deps] update `tape` [`204945d`](https://github.com/inspect-js/is-date-object/commit/204945d8658a3513ca6315ddf795e4034adb4545) - Switch from vb.teelaun.ch to versionbadg.es for the npm version badge SVG. [`7bff214`](https://github.com/inspect-js/is-date-object/commit/7bff214dcb2317b96219921476f990814afbb401) - Test on `io.js` `v2.5` [`92f7bd6`](https://github.com/inspect-js/is-date-object/commit/92f7bd6747e3259b0ddc9c287876f46a9cd4c270) - Test on `io.js` `v2.4` [`ebb34bf`](https://github.com/inspect-js/is-date-object/commit/ebb34bf1f58949768063f86ac012f1ca5d7cf6d9) - Fix tests for faked @@toStringTag [`3b9c26c`](https://github.com/inspect-js/is-date-object/commit/3b9c26c15040af6a87f8d77ce6c85a7bef7a4304) - Test on `io.js` `v3.0` [`5eedf4b`](https://github.com/inspect-js/is-date-object/commit/5eedf4bea76380a08813fd0977469c2480302a82) ## v1.0.0 - 2015-01-28 ### Commits - Dotfiles. [`5b6a929`](https://github.com/inspect-js/is-date-object/commit/5b6a9298c6f70882e78e66d64c9c019f85790f52) - `make release` [`e8d40ce`](https://github.com/inspect-js/is-date-object/commit/e8d40ceca85acd0aa4b2753faa6e41c0c54cf6c3) - package.json [`a107259`](https://github.com/inspect-js/is-date-object/commit/a1072591ea510a2998298be6cef827b123f4643f) - Read me [`eb92695`](https://github.com/inspect-js/is-date-object/commit/eb92695664bdee8fc49891cd73aa2f41075f53cb) - Initial commit [`4fc7755`](https://github.com/inspect-js/is-date-object/commit/4fc7755ff12f1d7a55cf841d486bf6b2350fe5a0) - Tests. [`b6f432f`](https://github.com/inspect-js/is-date-object/commit/b6f432fb6801c5ff8d89cfec7601d59478e23dd1) - Implementation. [`dd0fd96`](https://github.com/inspect-js/is-date-object/commit/dd0fd96c4016a66cec7cd59db0fde37c2ef3cdb5) apollo-server-demo/node_modules/is-date-object/.travis.yml 0000644 0001750 0000144 00000000374 03560116604 023337 0 ustar andreh users version: ~> 1.0 language: node_js os: - linux import: - ljharb/travis-ci:node/all.yml - ljharb/travis-ci:node/pretest.yml - ljharb/travis-ci:node/posttest.yml - ljharb/travis-ci:node/coverage.yml matrix: allow_failures: - env: COVERAGE=true apollo-server-demo/node_modules/lru-cache/ 0000755 0001750 0000144 00000000000 14067647700 020305 5 ustar andreh users apollo-server-demo/node_modules/lru-cache/index.js 0000644 0001750 0000144 00000017772 03560116604 021756 0 ustar andreh users 'use strict' // A linked list to keep track of recently-used-ness const Yallist = require('yallist') const MAX = Symbol('max') const LENGTH = Symbol('length') const LENGTH_CALCULATOR = Symbol('lengthCalculator') const ALLOW_STALE = Symbol('allowStale') const MAX_AGE = Symbol('maxAge') const DISPOSE = Symbol('dispose') const NO_DISPOSE_ON_SET = Symbol('noDisposeOnSet') const LRU_LIST = Symbol('lruList') const CACHE = Symbol('cache') const UPDATE_AGE_ON_GET = Symbol('updateAgeOnGet') const naiveLength = () => 1 // lruList is a yallist where the head is the youngest // item, and the tail is the oldest. the list contains the Hit // objects as the entries. // Each Hit object has a reference to its Yallist.Node. This // never changes. // // cache is a Map (or PseudoMap) that matches the keys to // the Yallist.Node object. class LRUCache { constructor (options) { if (typeof options === 'number') options = { max: options } if (!options) options = {} if (options.max && (typeof options.max !== 'number' || options.max < 0)) throw new TypeError('max must be a non-negative number') // Kind of weird to have a default max of Infinity, but oh well. const max = this[MAX] = options.max || Infinity const lc = options.length || naiveLength this[LENGTH_CALCULATOR] = (typeof lc !== 'function') ? naiveLength : lc this[ALLOW_STALE] = options.stale || false if (options.maxAge && typeof options.maxAge !== 'number') throw new TypeError('maxAge must be a number') this[MAX_AGE] = options.maxAge || 0 this[DISPOSE] = options.dispose this[NO_DISPOSE_ON_SET] = options.noDisposeOnSet || false this[UPDATE_AGE_ON_GET] = options.updateAgeOnGet || false this.reset() } // resize the cache when the max changes. set max (mL) { if (typeof mL !== 'number' || mL < 0) throw new TypeError('max must be a non-negative number') this[MAX] = mL || Infinity trim(this) } get max () { return this[MAX] } set allowStale (allowStale) { this[ALLOW_STALE] = !!allowStale } get allowStale () { return this[ALLOW_STALE] } set maxAge (mA) { if (typeof mA !== 'number') throw new TypeError('maxAge must be a non-negative number') this[MAX_AGE] = mA trim(this) } get maxAge () { return this[MAX_AGE] } // resize the cache when the lengthCalculator changes. set lengthCalculator (lC) { if (typeof lC !== 'function') lC = naiveLength if (lC !== this[LENGTH_CALCULATOR]) { this[LENGTH_CALCULATOR] = lC this[LENGTH] = 0 this[LRU_LIST].forEach(hit => { hit.length = this[LENGTH_CALCULATOR](hit.value, hit.key) this[LENGTH] += hit.length }) } trim(this) } get lengthCalculator () { return this[LENGTH_CALCULATOR] } get length () { return this[LENGTH] } get itemCount () { return this[LRU_LIST].length } rforEach (fn, thisp) { thisp = thisp || this for (let walker = this[LRU_LIST].tail; walker !== null;) { const prev = walker.prev forEachStep(this, fn, walker, thisp) walker = prev } } forEach (fn, thisp) { thisp = thisp || this for (let walker = this[LRU_LIST].head; walker !== null;) { const next = walker.next forEachStep(this, fn, walker, thisp) walker = next } } keys () { return this[LRU_LIST].toArray().map(k => k.key) } values () { return this[LRU_LIST].toArray().map(k => k.value) } reset () { if (this[DISPOSE] && this[LRU_LIST] && this[LRU_LIST].length) { this[LRU_LIST].forEach(hit => this[DISPOSE](hit.key, hit.value)) } this[CACHE] = new Map() // hash of items by key this[LRU_LIST] = new Yallist() // list of items in order of use recency this[LENGTH] = 0 // length of items in the list } dump () { return this[LRU_LIST].map(hit => isStale(this, hit) ? false : { k: hit.key, v: hit.value, e: hit.now + (hit.maxAge || 0) }).toArray().filter(h => h) } dumpLru () { return this[LRU_LIST] } set (key, value, maxAge) { maxAge = maxAge || this[MAX_AGE] if (maxAge && typeof maxAge !== 'number') throw new TypeError('maxAge must be a number') const now = maxAge ? Date.now() : 0 const len = this[LENGTH_CALCULATOR](value, key) if (this[CACHE].has(key)) { if (len > this[MAX]) { del(this, this[CACHE].get(key)) return false } const node = this[CACHE].get(key) const item = node.value // dispose of the old one before overwriting // split out into 2 ifs for better coverage tracking if (this[DISPOSE]) { if (!this[NO_DISPOSE_ON_SET]) this[DISPOSE](key, item.value) } item.now = now item.maxAge = maxAge item.value = value this[LENGTH] += len - item.length item.length = len this.get(key) trim(this) return true } const hit = new Entry(key, value, len, now, maxAge) // oversized objects fall out of cache automatically. if (hit.length > this[MAX]) { if (this[DISPOSE]) this[DISPOSE](key, value) return false } this[LENGTH] += hit.length this[LRU_LIST].unshift(hit) this[CACHE].set(key, this[LRU_LIST].head) trim(this) return true } has (key) { if (!this[CACHE].has(key)) return false const hit = this[CACHE].get(key).value return !isStale(this, hit) } get (key) { return get(this, key, true) } peek (key) { return get(this, key, false) } pop () { const node = this[LRU_LIST].tail if (!node) return null del(this, node) return node.value } del (key) { del(this, this[CACHE].get(key)) } load (arr) { // reset the cache this.reset() const now = Date.now() // A previous serialized cache has the most recent items first for (let l = arr.length - 1; l >= 0; l--) { const hit = arr[l] const expiresAt = hit.e || 0 if (expiresAt === 0) // the item was created without expiration in a non aged cache this.set(hit.k, hit.v) else { const maxAge = expiresAt - now // dont add already expired items if (maxAge > 0) { this.set(hit.k, hit.v, maxAge) } } } } prune () { this[CACHE].forEach((value, key) => get(this, key, false)) } } const get = (self, key, doUse) => { const node = self[CACHE].get(key) if (node) { const hit = node.value if (isStale(self, hit)) { del(self, node) if (!self[ALLOW_STALE]) return undefined } else { if (doUse) { if (self[UPDATE_AGE_ON_GET]) node.value.now = Date.now() self[LRU_LIST].unshiftNode(node) } } return hit.value } } const isStale = (self, hit) => { if (!hit || (!hit.maxAge && !self[MAX_AGE])) return false const diff = Date.now() - hit.now return hit.maxAge ? diff > hit.maxAge : self[MAX_AGE] && (diff > self[MAX_AGE]) } const trim = self => { if (self[LENGTH] > self[MAX]) { for (let walker = self[LRU_LIST].tail; self[LENGTH] > self[MAX] && walker !== null;) { // We know that we're about to delete this one, and also // what the next least recently used key will be, so just // go ahead and set it now. const prev = walker.prev del(self, walker) walker = prev } } } const del = (self, node) => { if (node) { const hit = node.value if (self[DISPOSE]) self[DISPOSE](hit.key, hit.value) self[LENGTH] -= hit.length self[CACHE].delete(hit.key) self[LRU_LIST].removeNode(node) } } class Entry { constructor (key, value, length, now, maxAge) { this.key = key this.value = value this.length = length this.now = now this.maxAge = maxAge || 0 } } const forEachStep = (self, fn, node, thisp) => { let hit = node.value if (isStale(self, hit)) { del(self, node) if (!self[ALLOW_STALE]) hit = undefined } if (hit) fn.call(thisp, hit.value, hit.key, self) } module.exports = LRUCache apollo-server-demo/node_modules/lru-cache/LICENSE 0000644 0001750 0000144 00000001375 03560116604 021306 0 ustar andreh users The ISC License Copyright (c) Isaac Z. Schlueter and Contributors Permission to use, copy, modify, and/or distribute this software for any purpose with or without fee is hereby granted, provided that the above copyright notice and this permission notice appear in all copies. THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE. apollo-server-demo/node_modules/lru-cache/README.md 0000644 0001750 0000144 00000013543 03560116604 021560 0 ustar andreh users # lru cache A cache object that deletes the least-recently-used items. [](https://travis-ci.org/isaacs/node-lru-cache) [](https://coveralls.io/github/isaacs/node-lru-cache) ## Installation: ```javascript npm install lru-cache --save ``` ## Usage: ```javascript var LRU = require("lru-cache") , options = { max: 500 , length: function (n, key) { return n * 2 + key.length } , dispose: function (key, n) { n.close() } , maxAge: 1000 * 60 * 60 } , cache = new LRU(options) , otherCache = new LRU(50) // sets just the max size cache.set("key", "value") cache.get("key") // "value" // non-string keys ARE fully supported // but note that it must be THE SAME object, not // just a JSON-equivalent object. var someObject = { a: 1 } cache.set(someObject, 'a value') // Object keys are not toString()-ed cache.set('[object Object]', 'a different value') assert.equal(cache.get(someObject), 'a value') // A similar object with same keys/values won't work, // because it's a different object identity assert.equal(cache.get({ a: 1 }), undefined) cache.reset() // empty the cache ``` If you put more stuff in it, then items will fall out. If you try to put an oversized thing in it, then it'll fall out right away. ## Options * `max` The maximum size of the cache, checked by applying the length function to all values in the cache. Not setting this is kind of silly, since that's the whole purpose of this lib, but it defaults to `Infinity`. Setting it to a non-number or negative number will throw a `TypeError`. Setting it to 0 makes it be `Infinity`. * `maxAge` Maximum age in ms. Items are not pro-actively pruned out as they age, but if you try to get an item that is too old, it'll drop it and return undefined instead of giving it to you. Setting this to a negative value will make everything seem old! Setting it to a non-number will throw a `TypeError`. * `length` Function that is used to calculate the length of stored items. If you're storing strings or buffers, then you probably want to do something like `function(n, key){return n.length}`. The default is `function(){return 1}`, which is fine if you want to store `max` like-sized things. The item is passed as the first argument, and the key is passed as the second argumnet. * `dispose` Function that is called on items when they are dropped from the cache. This can be handy if you want to close file descriptors or do other cleanup tasks when items are no longer accessible. Called with `key, value`. It's called *before* actually removing the item from the internal cache, so if you want to immediately put it back in, you'll have to do that in a `nextTick` or `setTimeout` callback or it won't do anything. * `stale` By default, if you set a `maxAge`, it'll only actually pull stale items out of the cache when you `get(key)`. (That is, it's not pre-emptively doing a `setTimeout` or anything.) If you set `stale:true`, it'll return the stale value before deleting it. If you don't set this, then it'll return `undefined` when you try to get a stale entry, as if it had already been deleted. * `noDisposeOnSet` By default, if you set a `dispose()` method, then it'll be called whenever a `set()` operation overwrites an existing key. If you set this option, `dispose()` will only be called when a key falls out of the cache, not when it is overwritten. * `updateAgeOnGet` When using time-expiring entries with `maxAge`, setting this to `true` will make each item's effective time update to the current time whenever it is retrieved from cache, causing it to not expire. (It can still fall out of cache based on recency of use, of course.) ## API * `set(key, value, maxAge)` * `get(key) => value` Both of these will update the "recently used"-ness of the key. They do what you think. `maxAge` is optional and overrides the cache `maxAge` option if provided. If the key is not found, `get()` will return `undefined`. The key and val can be any value. * `peek(key)` Returns the key value (or `undefined` if not found) without updating the "recently used"-ness of the key. (If you find yourself using this a lot, you *might* be using the wrong sort of data structure, but there are some use cases where it's handy.) * `del(key)` Deletes a key out of the cache. * `reset()` Clear the cache entirely, throwing away all values. * `has(key)` Check if a key is in the cache, without updating the recent-ness or deleting it for being stale. * `forEach(function(value,key,cache), [thisp])` Just like `Array.prototype.forEach`. Iterates over all the keys in the cache, in order of recent-ness. (Ie, more recently used items are iterated over first.) * `rforEach(function(value,key,cache), [thisp])` The same as `cache.forEach(...)` but items are iterated over in reverse order. (ie, less recently used items are iterated over first.) * `keys()` Return an array of the keys in the cache. * `values()` Return an array of the values in the cache. * `length` Return total length of objects in cache taking into account `length` options function. * `itemCount` Return total quantity of objects currently in cache. Note, that `stale` (see options) items are returned as part of this item count. * `dump()` Return an array of the cache entries ready for serialization and usage with 'destinationCache.load(arr)`. * `load(cacheEntriesArray)` Loads another cache entries array, obtained with `sourceCache.dump()`, into the cache. The destination cache is reset before loading new entries * `prune()` Manually iterates over the entire cache proactively pruning old entries apollo-server-demo/node_modules/lru-cache/package.json 0000644 0001750 0000144 00000001631 03560116604 022562 0 ustar andreh users { "name": "lru-cache", "description": "A cache object that deletes the least-recently-used items.", "version": "6.0.0", "author": "Isaac Z. Schlueter <i@izs.me>", "keywords": [ "mru", "lru", "cache" ], "scripts": { "test": "tap", "snap": "tap", "preversion": "npm test", "postversion": "npm publish", "prepublishOnly": "git push origin --follow-tags" }, "main": "index.js", "repository": "git://github.com/isaacs/node-lru-cache.git", "devDependencies": { "benchmark": "^2.1.4", "tap": "^14.10.7" }, "license": "ISC", "dependencies": { "yallist": "^4.0.0" }, "files": [ "index.js" ], "engines": { "node": ">=10" } ,"_resolved": "https://registry.npmjs.org/lru-cache/-/lru-cache-6.0.0.tgz" ,"_integrity": "sha512-Jo6dJ04CmSjuznwJSS3pUeWmd/H0ffTlkXXgwZi+eq1UCmqQwCh+eLsYOYCwY991i2Fah4h1BEMCx4qThGbsiA==" ,"_from": "lru-cache@6.0.0" } apollo-server-demo/node_modules/@protobufjs/ 0000755 0001750 0000144 00000000000 14067647701 020740 5 ustar andreh users apollo-server-demo/node_modules/@protobufjs/inquire/ 0000755 0001750 0000144 00000000000 14067647701 022414 5 ustar andreh users apollo-server-demo/node_modules/@protobufjs/inquire/index.js 0000644 0001750 0000144 00000001040 13032752311 024035 0 ustar andreh users "use strict"; module.exports = inquire; /** * Requires a module only if available. * @memberof util * @param {string} moduleName Module to require * @returns {?Object} Required module if available and not empty, otherwise `null` */ function inquire(moduleName) { try { var mod = eval("quire".replace(/^/,"re"))(moduleName); // eslint-disable-line no-eval if (mod && (mod.length || Object.keys(mod).length)) return mod; } catch (e) {} // eslint-disable-line no-empty return null; } apollo-server-demo/node_modules/@protobufjs/inquire/LICENSE 0000644 0001750 0000144 00000002741 13026320625 023410 0 ustar andreh users Copyright (c) 2016, Daniel Wirtz All rights reserved. Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met: * Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer. * Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution. * Neither the name of its author, nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission. THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. apollo-server-demo/node_modules/@protobufjs/inquire/index.d.ts 0000644 0001750 0000144 00000000427 13031473640 024305 0 ustar andreh users export = inquire; /** * Requires a module only if available. * @memberof util * @param {string} moduleName Module to require * @returns {?Object} Required module if available and not empty, otherwise `null` */ declare function inquire(moduleName: string): Object; apollo-server-demo/node_modules/@protobufjs/inquire/README.md 0000644 0001750 0000144 00000000657 13026321606 023666 0 ustar andreh users @protobufjs/inquire =================== [](https://www.npmjs.com/package/@protobufjs/inquire) Requires a module only if available and hides the require call from bundlers. API --- * **inquire(moduleName: `string`): `?Object`**<br /> Requires a module only if available. **License:** [BSD 3-Clause License](https://opensource.org/licenses/BSD-3-Clause) apollo-server-demo/node_modules/@protobufjs/inquire/package.json 0000644 0001750 0000144 00000001407 13042165021 024662 0 ustar andreh users { "name": "@protobufjs/inquire", "description": "Requires a module only if available and hides the require call from bundlers.", "version": "1.1.0", "author": "Daniel Wirtz <dcode+protobufjs@dcode.io>", "repository": { "type": "git", "url": "https://github.com/dcodeIO/protobuf.js.git" }, "license": "BSD-3-Clause", "main": "index.js", "types": "index.d.ts", "devDependencies": { "istanbul": "^0.4.5", "tape": "^4.6.3" }, "scripts": { "test": "tape tests/*.js", "coverage": "istanbul cover node_modules/tape/bin/tape tests/*.js" } ,"_resolved": "https://registry.npmjs.org/@protobufjs/inquire/-/inquire-1.1.0.tgz" ,"_integrity": "sha1-/yAOPnzyQp4tyvwRQIKOjMY48Ik=" ,"_from": "@protobufjs/inquire@1.1.0" } apollo-server-demo/node_modules/@protobufjs/inquire/.npmignore 0000644 0001750 0000144 00000000047 13042003347 024374 0 ustar andreh users npm-debug.* node_modules/ coverage/ apollo-server-demo/node_modules/@protobufjs/inquire/tests/ 0000755 0001750 0000144 00000000000 14067647701 023556 5 ustar andreh users apollo-server-demo/node_modules/@protobufjs/inquire/tests/index.js 0000644 0001750 0000144 00000001430 13042144561 025205 0 ustar andreh users var tape = require("tape"); var inquire = require(".."); tape.test("inquire", function(test) { test.equal(inquire("buffer").Buffer, Buffer, "should be able to require \"buffer\""); test.equal(inquire("%invalid"), null, "should not be able to require \"%invalid\""); test.equal(inquire("./tests/data/emptyObject"), null, "should return null when requiring a module exporting an empty object"); test.equal(inquire("./tests/data/emptyArray"), null, "should return null when requiring a module exporting an empty array"); test.same(inquire("./tests/data/object"), { a: 1 }, "should return the object if a non-empty object"); test.same(inquire("./tests/data/array"), [ 1 ], "should return the module if a non-empty array"); test.end(); }); apollo-server-demo/node_modules/@protobufjs/inquire/tests/data/ 0000755 0001750 0000144 00000000000 14067647701 024467 5 ustar andreh users apollo-server-demo/node_modules/@protobufjs/inquire/tests/data/emptyArray.js 0000644 0001750 0000144 00000000026 13042004024 027131 0 ustar andreh users module.exports = []; apollo-server-demo/node_modules/@protobufjs/inquire/tests/data/object.js 0000644 0001750 0000144 00000000034 13042004062 026243 0 ustar andreh users module.exports = { a: 1 }; apollo-server-demo/node_modules/@protobufjs/inquire/tests/data/array.js 0000644 0001750 0000144 00000000027 13042004050 026112 0 ustar andreh users module.exports = [1]; apollo-server-demo/node_modules/@protobufjs/inquire/tests/data/emptyObject.js 0000644 0001750 0000144 00000000026 13042004032 027260 0 ustar andreh users module.exports = {}; apollo-server-demo/node_modules/@protobufjs/codegen/ 0000755 0001750 0000144 00000000000 14067647701 022344 5 ustar andreh users apollo-server-demo/node_modules/@protobufjs/codegen/index.js 0000644 0001750 0000144 00000007452 13077224603 024011 0 ustar andreh users "use strict"; module.exports = codegen; /** * Begins generating a function. * @memberof util * @param {string[]} functionParams Function parameter names * @param {string} [functionName] Function name if not anonymous * @returns {Codegen} Appender that appends code to the function's body */ function codegen(functionParams, functionName) { /* istanbul ignore if */ if (typeof functionParams === "string") { functionName = functionParams; functionParams = undefined; } var body = []; /** * Appends code to the function's body or finishes generation. * @typedef Codegen * @type {function} * @param {string|Object.<string,*>} [formatStringOrScope] Format string or, to finish the function, an object of additional scope variables, if any * @param {...*} [formatParams] Format parameters * @returns {Codegen|Function} Itself or the generated function if finished * @throws {Error} If format parameter counts do not match */ function Codegen(formatStringOrScope) { // note that explicit array handling below makes this ~50% faster // finish the function if (typeof formatStringOrScope !== "string") { var source = toString(); if (codegen.verbose) console.log("codegen: " + source); // eslint-disable-line no-console source = "return " + source; if (formatStringOrScope) { var scopeKeys = Object.keys(formatStringOrScope), scopeParams = new Array(scopeKeys.length + 1), scopeValues = new Array(scopeKeys.length), scopeOffset = 0; while (scopeOffset < scopeKeys.length) { scopeParams[scopeOffset] = scopeKeys[scopeOffset]; scopeValues[scopeOffset] = formatStringOrScope[scopeKeys[scopeOffset++]]; } scopeParams[scopeOffset] = source; return Function.apply(null, scopeParams).apply(null, scopeValues); // eslint-disable-line no-new-func } return Function(source)(); // eslint-disable-line no-new-func } // otherwise append to body var formatParams = new Array(arguments.length - 1), formatOffset = 0; while (formatOffset < formatParams.length) formatParams[formatOffset] = arguments[++formatOffset]; formatOffset = 0; formatStringOrScope = formatStringOrScope.replace(/%([%dfijs])/g, function replace($0, $1) { var value = formatParams[formatOffset++]; switch ($1) { case "d": case "f": return String(Number(value)); case "i": return String(Math.floor(value)); case "j": return JSON.stringify(value); case "s": return String(value); } return "%"; }); if (formatOffset !== formatParams.length) throw Error("parameter count mismatch"); body.push(formatStringOrScope); return Codegen; } function toString(functionNameOverride) { return "function " + (functionNameOverride || functionName || "") + "(" + (functionParams && functionParams.join(",") || "") + "){\n " + body.join("\n ") + "\n}"; } Codegen.toString = toString; return Codegen; } /** * Begins generating a function. * @memberof util * @function codegen * @param {string} [functionName] Function name if not anonymous * @returns {Codegen} Appender that appends code to the function's body * @variation 2 */ /** * When set to `true`, codegen will log generated code to console. Useful for debugging. * @name util.codegen.verbose * @type {boolean} */ codegen.verbose = false; apollo-server-demo/node_modules/@protobufjs/codegen/LICENSE 0000644 0001750 0000144 00000002741 13026320507 023337 0 ustar andreh users Copyright (c) 2016, Daniel Wirtz All rights reserved. Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met: * Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer. * Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution. * Neither the name of its author, nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission. THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. apollo-server-demo/node_modules/@protobufjs/codegen/index.d.ts 0000644 0001750 0000144 00000002212 13116605411 024224 0 ustar andreh users export = codegen; /** * Appends code to the function's body. * @param [formatStringOrScope] Format string or, to finish the function, an object of additional scope variables, if any * @param [formatParams] Format parameters * @returns Itself or the generated function if finished * @throws {Error} If format parameter counts do not match */ type Codegen = (formatStringOrScope?: (string|{ [k: string]: any }), ...formatParams: any[]) => (Codegen|Function); /** * Begins generating a function. * @param functionParams Function parameter names * @param [functionName] Function name if not anonymous * @returns Appender that appends code to the function's body */ declare function codegen(functionParams: string[], functionName?: string): Codegen; /** * Begins generating a function. * @param [functionName] Function name if not anonymous * @returns Appender that appends code to the function's body */ declare function codegen(functionName?: string): Codegen; declare namespace codegen { /** When set to `true`, codegen will log generated code to console. Useful for debugging. */ let verbose: boolean; } apollo-server-demo/node_modules/@protobufjs/codegen/README.md 0000644 0001750 0000144 00000003520 13077224410 023607 0 ustar andreh users @protobufjs/codegen =================== [](https://www.npmjs.com/package/@protobufjs/codegen) A minimalistic code generation utility. API --- * **codegen([functionParams: `string[]`], [functionName: string]): `Codegen`**<br /> Begins generating a function. * **codegen.verbose = `false`**<br /> When set to true, codegen will log generated code to console. Useful for debugging. Invoking **codegen** returns an appender function that appends code to the function's body and returns itself: * **Codegen(formatString: `string`, [...formatParams: `any`]): Codegen**<br /> Appends code to the function's body. The format string can contain placeholders specifying the types of inserted format parameters: * `%d`: Number (integer or floating point value) * `%f`: Floating point value * `%i`: Integer value * `%j`: JSON.stringify'ed value * `%s`: String value * `%%`: Percent sign<br /> * **Codegen([scope: `Object.<string,*>`]): `Function`**<br /> Finishes the function and returns it. * **Codegen.toString([functionNameOverride: `string`]): `string`**<br /> Returns the function as a string. Example ------- ```js var codegen = require("@protobufjs/codegen"); var add = codegen(["a", "b"], "add") // A function with parameters "a" and "b" named "add" ("// awesome comment") // adds the line to the function's body ("return a + b - c + %d", 1) // replaces %d with 1 and adds the line to the body ({ c: 1 }); // adds "c" with a value of 1 to the function's scope console.log(add.toString()); // function add(a, b) { return a + b - c + 1 } console.log(add(1, 2)); // calculates 1 + 2 - 1 + 1 = 3 ``` **License:** [BSD 3-Clause License](https://opensource.org/licenses/BSD-3-Clause) apollo-server-demo/node_modules/@protobufjs/codegen/package.json 0000644 0001750 0000144 00000001122 13116605463 024617 0 ustar andreh users { "name": "@protobufjs/codegen", "description": "A minimalistic code generation utility.", "version": "2.0.4", "author": "Daniel Wirtz <dcode+protobufjs@dcode.io>", "repository": { "type": "git", "url": "https://github.com/dcodeIO/protobuf.js.git" }, "license": "BSD-3-Clause", "main": "index.js", "types": "index.d.ts" ,"_resolved": "https://registry.npmjs.org/@protobufjs/codegen/-/codegen-2.0.4.tgz" ,"_integrity": "sha512-YyFaikqM5sH0ziFZCN3xDC7zeGaB/d0IUb9CATugHWbd1FRFwWwt4ld4OYMPWu5a3Xe01mGAULCdqhMlPl29Jg==" ,"_from": "@protobufjs/codegen@2.0.4" } apollo-server-demo/node_modules/@protobufjs/codegen/tests/ 0000755 0001750 0000144 00000000000 14067647701 023506 5 ustar andreh users apollo-server-demo/node_modules/@protobufjs/codegen/tests/index.js 0000644 0001750 0000144 00000000544 13077223676 025157 0 ustar andreh users var codegen = require(".."); // new require("benchmark").Suite().add("add", function() { var add = codegen(["a", "b"], "add") ("// awesome comment") ("return a + b - c + %d", 1) ({ c: 1 }); if (add(1, 2) !== 3) throw Error("failed"); // }).on("cycle", function(event) { process.stdout.write(String(event.target) + "\n"); }).run(); apollo-server-demo/node_modules/@protobufjs/path/ 0000755 0001750 0000144 00000000000 14067647701 021674 5 ustar andreh users apollo-server-demo/node_modules/@protobufjs/path/index.js 0000644 0001750 0000144 00000004001 13053610133 023313 0 ustar andreh users "use strict"; /** * A minimal path module to resolve Unix, Windows and URL paths alike. * @memberof util * @namespace */ var path = exports; var isAbsolute = /** * Tests if the specified path is absolute. * @param {string} path Path to test * @returns {boolean} `true` if path is absolute */ path.isAbsolute = function isAbsolute(path) { return /^(?:\/|\w+:)/.test(path); }; var normalize = /** * Normalizes the specified path. * @param {string} path Path to normalize * @returns {string} Normalized path */ path.normalize = function normalize(path) { path = path.replace(/\\/g, "/") .replace(/\/{2,}/g, "/"); var parts = path.split("/"), absolute = isAbsolute(path), prefix = ""; if (absolute) prefix = parts.shift() + "/"; for (var i = 0; i < parts.length;) { if (parts[i] === "..") { if (i > 0 && parts[i - 1] !== "..") parts.splice(--i, 2); else if (absolute) parts.splice(i, 1); else ++i; } else if (parts[i] === ".") parts.splice(i, 1); else ++i; } return prefix + parts.join("/"); }; /** * Resolves the specified include path against the specified origin path. * @param {string} originPath Path to the origin file * @param {string} includePath Include path relative to origin path * @param {boolean} [alreadyNormalized=false] `true` if both paths are already known to be normalized * @returns {string} Path to the include file */ path.resolve = function resolve(originPath, includePath, alreadyNormalized) { if (!alreadyNormalized) includePath = normalize(includePath); if (isAbsolute(includePath)) return includePath; if (!alreadyNormalized) originPath = normalize(originPath); return (originPath = originPath.replace(/(?:\/|^)[^/]+$/, "")).length ? normalize(originPath + "/" + includePath) : includePath; }; apollo-server-demo/node_modules/@protobufjs/path/LICENSE 0000644 0001750 0000144 00000002741 13026320645 022672 0 ustar andreh users Copyright (c) 2016, Daniel Wirtz All rights reserved. Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met: * Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer. * Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution. * Neither the name of its author, nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission. THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. apollo-server-demo/node_modules/@protobufjs/path/index.d.ts 0000644 0001750 0000144 00000001506 13042165423 023563 0 ustar andreh users /** * Tests if the specified path is absolute. * @param {string} path Path to test * @returns {boolean} `true` if path is absolute */ export function isAbsolute(path: string): boolean; /** * Normalizes the specified path. * @param {string} path Path to normalize * @returns {string} Normalized path */ export function normalize(path: string): string; /** * Resolves the specified include path against the specified origin path. * @param {string} originPath Path to the origin file * @param {string} includePath Include path relative to origin path * @param {boolean} [alreadyNormalized=false] `true` if both paths are already known to be normalized * @returns {string} Path to the include file */ export function resolve(originPath: string, includePath: string, alreadyNormalized?: boolean): string; apollo-server-demo/node_modules/@protobufjs/path/README.md 0000644 0001750 0000144 00000001274 13026321612 023137 0 ustar andreh users @protobufjs/path ================ [](https://www.npmjs.com/package/@protobufjs/path) A minimal path module to resolve Unix, Windows and URL paths alike. API --- * **path.isAbsolute(path: `string`): `boolean`**<br /> Tests if the specified path is absolute. * **path.normalize(path: `string`): `string`**<br /> Normalizes the specified path. * **path.resolve(originPath: `string`, includePath: `string`, [alreadyNormalized=false: `boolean`]): `string`**<br /> Resolves the specified include path against the specified origin path. **License:** [BSD 3-Clause License](https://opensource.org/licenses/BSD-3-Clause) apollo-server-demo/node_modules/@protobufjs/path/package.json 0000644 0001750 0000144 00000001361 13053610304 024142 0 ustar andreh users { "name": "@protobufjs/path", "description": "A minimal path module to resolve Unix, Windows and URL paths alike.", "version": "1.1.2", "author": "Daniel Wirtz <dcode+protobufjs@dcode.io>", "repository": { "type": "git", "url": "https://github.com/dcodeIO/protobuf.js.git" }, "license": "BSD-3-Clause", "main": "index.js", "types": "index.d.ts", "devDependencies": { "istanbul": "^0.4.5", "tape": "^4.6.3" }, "scripts": { "test": "tape tests/*.js", "coverage": "istanbul cover node_modules/tape/bin/tape tests/*.js" } ,"_resolved": "https://registry.npmjs.org/@protobufjs/path/-/path-1.1.2.tgz" ,"_integrity": "sha1-bMKyDFya1q0NzP0hynZz2Nf79o0=" ,"_from": "@protobufjs/path@1.1.2" } apollo-server-demo/node_modules/@protobufjs/path/tests/ 0000755 0001750 0000144 00000000000 14067647701 023036 5 ustar andreh users apollo-server-demo/node_modules/@protobufjs/path/tests/index.js 0000644 0001750 0000144 00000004051 13042141515 024463 0 ustar andreh users var tape = require("tape"); var path = require(".."); tape.test("path", function(test) { test.ok(path.isAbsolute("X:\\some\\path\\file.js"), "should identify absolute windows paths"); test.ok(path.isAbsolute("/some/path/file.js"), "should identify absolute unix paths"); test.notOk(path.isAbsolute("some\\path\\file.js"), "should identify relative windows paths"); test.notOk(path.isAbsolute("some/path/file.js"), "should identify relative unix paths"); var paths = [ { actual: "X:\\some\\..\\.\\path\\\\file.js", normal: "X:/path/file.js", resolve: { origin: "X:/path/origin.js", expected: "X:/path/file.js" } }, { actual: "some\\..\\.\\path\\\\file.js", normal: "path/file.js", resolve: { origin: "X:/path/origin.js", expected: "X:/path/path/file.js" } }, { actual: "/some/.././path//file.js", normal: "/path/file.js", resolve: { origin: "/path/origin.js", expected: "/path/file.js" } }, { actual: "some/.././path//file.js", normal: "path/file.js", resolve: { origin: "", expected: "path/file.js" } }, { actual: ".././path//file.js", normal: "../path/file.js" }, { actual: "/.././path//file.js", normal: "/path/file.js" } ]; paths.forEach(function(p) { test.equal(path.normalize(p.actual), p.normal, "should normalize " + p.actual); if (p.resolve) { test.equal(path.resolve(p.resolve.origin, p.actual), p.resolve.expected, "should resolve " + p.actual); test.equal(path.resolve(p.resolve.origin, p.normal, true), p.resolve.expected, "should resolve " + p.normal + " (already normalized)"); } }); test.end(); }); apollo-server-demo/node_modules/@protobufjs/utf8/ 0000755 0001750 0000144 00000000000 14067647701 021626 5 ustar andreh users apollo-server-demo/node_modules/@protobufjs/utf8/index.js 0000644 0001750 0000144 00000006455 13041673370 023275 0 ustar andreh users "use strict"; /** * A minimal UTF8 implementation for number arrays. * @memberof util * @namespace */ var utf8 = exports; /** * Calculates the UTF8 byte length of a string. * @param {string} string String * @returns {number} Byte length */ utf8.length = function utf8_length(string) { var len = 0, c = 0; for (var i = 0; i < string.length; ++i) { c = string.charCodeAt(i); if (c < 128) len += 1; else if (c < 2048) len += 2; else if ((c & 0xFC00) === 0xD800 && (string.charCodeAt(i + 1) & 0xFC00) === 0xDC00) { ++i; len += 4; } else len += 3; } return len; }; /** * Reads UTF8 bytes as a string. * @param {Uint8Array} buffer Source buffer * @param {number} start Source start * @param {number} end Source end * @returns {string} String read */ utf8.read = function utf8_read(buffer, start, end) { var len = end - start; if (len < 1) return ""; var parts = null, chunk = [], i = 0, // char offset t; // temporary while (start < end) { t = buffer[start++]; if (t < 128) chunk[i++] = t; else if (t > 191 && t < 224) chunk[i++] = (t & 31) << 6 | buffer[start++] & 63; else if (t > 239 && t < 365) { t = ((t & 7) << 18 | (buffer[start++] & 63) << 12 | (buffer[start++] & 63) << 6 | buffer[start++] & 63) - 0x10000; chunk[i++] = 0xD800 + (t >> 10); chunk[i++] = 0xDC00 + (t & 1023); } else chunk[i++] = (t & 15) << 12 | (buffer[start++] & 63) << 6 | buffer[start++] & 63; if (i > 8191) { (parts || (parts = [])).push(String.fromCharCode.apply(String, chunk)); i = 0; } } if (parts) { if (i) parts.push(String.fromCharCode.apply(String, chunk.slice(0, i))); return parts.join(""); } return String.fromCharCode.apply(String, chunk.slice(0, i)); }; /** * Writes a string as UTF8 bytes. * @param {string} string Source string * @param {Uint8Array} buffer Destination buffer * @param {number} offset Destination offset * @returns {number} Bytes written */ utf8.write = function utf8_write(string, buffer, offset) { var start = offset, c1, // character 1 c2; // character 2 for (var i = 0; i < string.length; ++i) { c1 = string.charCodeAt(i); if (c1 < 128) { buffer[offset++] = c1; } else if (c1 < 2048) { buffer[offset++] = c1 >> 6 | 192; buffer[offset++] = c1 & 63 | 128; } else if ((c1 & 0xFC00) === 0xD800 && ((c2 = string.charCodeAt(i + 1)) & 0xFC00) === 0xDC00) { c1 = 0x10000 + ((c1 & 0x03FF) << 10) + (c2 & 0x03FF); ++i; buffer[offset++] = c1 >> 18 | 240; buffer[offset++] = c1 >> 12 & 63 | 128; buffer[offset++] = c1 >> 6 & 63 | 128; buffer[offset++] = c1 & 63 | 128; } else { buffer[offset++] = c1 >> 12 | 224; buffer[offset++] = c1 >> 6 & 63 | 128; buffer[offset++] = c1 & 63 | 128; } } return offset - start; }; apollo-server-demo/node_modules/@protobufjs/utf8/LICENSE 0000644 0001750 0000144 00000002741 13026320724 022622 0 ustar andreh users Copyright (c) 2016, Daniel Wirtz All rights reserved. Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met: * Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer. * Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution. * Neither the name of its author, nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission. THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. apollo-server-demo/node_modules/@protobufjs/utf8/index.d.ts 0000644 0001750 0000144 00000001366 13041674601 023523 0 ustar andreh users /** * Calculates the UTF8 byte length of a string. * @param {string} string String * @returns {number} Byte length */ export function length(string: string): number; /** * Reads UTF8 bytes as a string. * @param {Uint8Array} buffer Source buffer * @param {number} start Source start * @param {number} end Source end * @returns {string} String read */ export function read(buffer: Uint8Array, start: number, end: number): string; /** * Writes a string as UTF8 bytes. * @param {string} string Source string * @param {Uint8Array} buffer Destination buffer * @param {number} offset Destination offset * @returns {number} Bytes written */ export function write(string: string, buffer: Uint8Array, offset: number): number; apollo-server-demo/node_modules/@protobufjs/utf8/README.md 0000644 0001750 0000144 00000001211 13026321624 023063 0 ustar andreh users @protobufjs/utf8 ================ [](https://www.npmjs.com/package/@protobufjs/utf8) A minimal UTF8 implementation for number arrays. API --- * **utf8.length(string: `string`): `number`**<br /> Calculates the UTF8 byte length of a string. * **utf8.read(buffer: `Uint8Array`, start: `number`, end: `number`): `string`**<br /> Reads UTF8 bytes as a string. * **utf8.write(string: `string`, buffer: `Uint8Array`, offset: `number`): `number`**<br /> Writes a string as UTF8 bytes. **License:** [BSD 3-Clause License](https://opensource.org/licenses/BSD-3-Clause) apollo-server-demo/node_modules/@protobufjs/utf8/package.json 0000644 0001750 0000144 00000001312 13042165046 024076 0 ustar andreh users { "name": "@protobufjs/utf8", "description": "A minimal UTF8 implementation for number arrays.", "version": "1.1.0", "author": "Daniel Wirtz <dcode+protobufjs@dcode.io>", "repository": { "type": "git", "url": "https://github.com/dcodeIO/protobuf.js.git" }, "license": "BSD-3-Clause", "main": "index.js", "types": "index.d.ts", "devDependencies": { "istanbul": "^0.4.5", "tape": "^4.6.3" }, "scripts": { "test": "tape tests/*.js", "coverage": "istanbul cover node_modules/tape/bin/tape tests/*.js" } ,"_resolved": "https://registry.npmjs.org/@protobufjs/utf8/-/utf8-1.1.0.tgz" ,"_integrity": "sha1-p3c2C1s5oaLlEG+OhY8v0tBgxXA=" ,"_from": "@protobufjs/utf8@1.1.0" } apollo-server-demo/node_modules/@protobufjs/utf8/.npmignore 0000644 0001750 0000144 00000000047 13041674241 023614 0 ustar andreh users npm-debug.* node_modules/ coverage/ apollo-server-demo/node_modules/@protobufjs/utf8/tests/ 0000755 0001750 0000144 00000000000 14067647701 022770 5 ustar andreh users apollo-server-demo/node_modules/@protobufjs/utf8/tests/index.js 0000644 0001750 0000144 00000004304 13041751702 024422 0 ustar andreh users var tape = require("tape"); var utf8 = require(".."); var data = require("fs").readFileSync(require.resolve("./data/utf8.txt")), dataStr = data.toString("utf8"); tape.test("utf8", function(test) { test.test(test.name + " - length", function(test) { test.equal(utf8.length(""), 0, "should return a byte length of zero for an empty string"); test.equal(utf8.length(dataStr), Buffer.byteLength(dataStr), "should return the same byte length as node buffers"); test.end(); }); test.test(test.name + " - read", function(test) { var comp = utf8.read([], 0, 0); test.equal(comp, "", "should decode an empty buffer to an empty string"); comp = utf8.read(data, 0, data.length); test.equal(comp, data.toString("utf8"), "should decode to the same byte data as node buffers"); var longData = Buffer.concat([data, data, data, data]); comp = utf8.read(longData, 0, longData.length); test.equal(comp, longData.toString("utf8"), "should decode to the same byte data as node buffers (long)"); var chunkData = new Buffer(data.toString("utf8").substring(0, 8192)); comp = utf8.read(chunkData, 0, chunkData.length); test.equal(comp, chunkData.toString("utf8"), "should decode to the same byte data as node buffers (chunk size)"); test.end(); }); test.test(test.name + " - write", function(test) { var buf = new Buffer(0); test.equal(utf8.write("", buf, 0), 0, "should encode an empty string to an empty buffer"); var len = utf8.length(dataStr); buf = new Buffer(len); test.equal(utf8.write(dataStr, buf, 0), len, "should encode to exactly " + len + " bytes"); test.equal(buf.length, data.length, "should encode to a buffer length equal to that of node buffers"); for (var i = 0; i < buf.length; ++i) { if (buf[i] !== data[i]) { test.fail("should encode to the same buffer data as node buffers (offset " + i + ")"); return; } } test.pass("should encode to the same buffer data as node buffers"); test.end(); }); }); apollo-server-demo/node_modules/@protobufjs/utf8/tests/data/ 0000755 0001750 0000144 00000000000 14067647701 023701 5 ustar andreh users apollo-server-demo/node_modules/@protobufjs/utf8/tests/data/utf8.txt 0000644 0001750 0000144 00000034055 13041674055 025330 0 ustar andreh users UTF-8 encoded sample plain-text file ‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾ Markus Kuhn [ˈmaʳkÊŠs kuËn] <http://www.cl.cam.ac.uk/~mgk25/> — 2002-07-25 CC BY The ASCII compatible UTF-8 encoding used in this plain-text file is defined in Unicode, ISO 10646-1, and RFC 2279. Using Unicode/UTF-8, you can write in emails and source code things such as Mathematics and sciences: ∮ Eâ‹…da = Q, n → ∞, ∑ f(i) = ∠g(i), ⎧⎡⎛┌─────â”⎞⎤⎫ ⎪⎢⎜│a²+b³ ⎟⎥⎪ ∀x∈â„: ⌈x⌉ = −⌊−x⌋, α ∧ ¬β = ¬(¬α ∨ β), ⎪⎢⎜│───── ⎟⎥⎪ ⎪⎢⎜⎷ c₈ ⎟⎥⎪ â„• ⊆ â„•â‚€ ⊂ ℤ ⊂ ℚ ⊂ ℠⊂ â„‚, ⎨⎢⎜ ⎟⎥⎬ ⎪⎢⎜ ∞ ⎟⎥⎪ ⊥ < a ≠b ≡ c ≤ d ≪ ⊤ ⇒ (⟦A⟧ ⇔ ⟪B⟫), ⎪⎢⎜ ⎲ ⎟⎥⎪ ⎪⎢⎜ ⎳aâ±-bâ±âŽŸâŽ¥âŽª 2Hâ‚‚ + Oâ‚‚ ⇌ 2Hâ‚‚O, R = 4.7 kΩ, ⌀ 200 mm ⎩⎣âŽi=1 ⎠⎦⎠Linguistics and dictionaries: ði ıntəˈnæʃənÉ™l fəˈnÉ›tık É™soÊŠsiˈeıʃn Y [ˈÊpsilÉ”n], Yen [jÉ›n], Yoga [ˈjoËgÉ‘] APL: ((Vâ³V)=â³â´V)/Vâ†,V ⌷â†â³â†’â´âˆ†âˆ‡âŠƒâ€¾âŽâ•⌈ Nicer typography in plain text files: â•”â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•— â•‘ â•‘ â•‘ • ‘single’ and “double†quotes â•‘ â•‘ â•‘ â•‘ • Curly apostrophes: “We’ve been here†║ â•‘ â•‘ â•‘ • Latin-1 apostrophe and accents: '´` â•‘ â•‘ â•‘ â•‘ • ‚deutsche‘ „Anführungszeichen“ â•‘ â•‘ â•‘ â•‘ • †, ‡, ‰, •, 3–4, —, −5/+5, â„¢, … â•‘ â•‘ â•‘ â•‘ • ASCII safety test: 1lI|, 0OD, 8B â•‘ â•‘ â•─────────╮ â•‘ â•‘ • the euro symbol: │ 14.95 € │ â•‘ â•‘ ╰─────────╯ â•‘ ╚â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â•â• Combining characters: STARGΛ̊TE SG-1, a = v̇ = r̈, a⃑ ⊥ b⃑ Greek (in Polytonic): The Greek anthem: Σὲ γνωÏίζω ἀπὸ τὴν κόψη τοῦ σπαθιοῦ τὴν Ï„ÏομεÏá½µ, σὲ γνωÏίζω ἀπὸ τὴν ὄψη ποὺ μὲ βία μετÏάει τὴ γῆ. ᾿Απ᾿ τὰ κόκκαλα βγαλμένη τῶν ῾Ελλήνων τὰ ἱεÏá½± καὶ σὰν Ï€Ïῶτα ἀνδÏειωμένη χαῖÏε, ὦ χαῖÏε, ᾿ΕλευθεÏιά! From a speech of Demosthenes in the 4th century BC: Οá½Ï‡á½¶ ταá½Ï„á½° παÏίσταταί μοι γιγνώσκειν, ὦ ἄνδÏες ᾿Αθηναῖοι, ὅταν τ᾿ εἰς τὰ Ï€Ïάγματα ἀποβλέψω καὶ ὅταν Ï€Ïὸς τοὺς λόγους οὓς ἀκούω· τοὺς μὲν Î³á½°Ï Î»á½¹Î³Î¿Ï…Ï‚ πεÏá½¶ τοῦ τιμωÏήσασθαι Φίλιππον á½Ïá¿¶ γιγνομένους, τὰ δὲ Ï€Ïάγματ᾿ εἰς τοῦτο Ï€Ïοήκοντα, ὥσθ᾿ ὅπως μὴ πεισόμεθ᾿ αá½Ï„οὶ Ï€ÏότεÏον κακῶς σκέψασθαι δέον. οá½Î´á½³Î½ οὖν ἄλλο μοι δοκοῦσιν οἱ τὰ τοιαῦτα λέγοντες á¼¢ τὴν ὑπόθεσιν, πεÏá½¶ á¼§Ï‚ βουλεύεσθαι, οá½Ï‡á½¶ τὴν οὖσαν παÏιστάντες ὑμῖν á¼Î¼Î±Ïτάνειν. á¼Î³á½¼ δέ, ὅτι μέν ποτ᾿ á¼Î¾á¿†Î½ τῇ πόλει καὶ τὰ αὑτῆς ἔχειν ἀσφαλῶς καὶ Φίλιππον τιμωÏήσασθαι, καὶ μάλ᾿ ἀκÏιβῶς οἶδα· á¼Ï€á¾¿ á¼Î¼Î¿á¿¦ γάÏ, οὠπάλαι γέγονεν ταῦτ᾿ ἀμφότεÏα· νῦν μέντοι πέπεισμαι τοῦθ᾿ ἱκανὸν Ï€Ïολαβεῖν ἡμῖν εἶναι τὴν Ï€Ïώτην, ὅπως τοὺς συμμάχους σώσομεν. á¼á½°Î½ Î³á½°Ï Ï„Î¿á¿¦Ï„Î¿ βεβαίως ὑπάÏξῃ, τότε καὶ πεÏá½¶ τοῦ τίνα τιμωÏήσεταί τις καὶ ὃν Ï„Ïόπον á¼Î¾á½³ÏƒÏ„αι σκοπεῖν· Ï€Ïὶν δὲ τὴν á¼€Ïχὴν á½€Ïθῶς ὑποθέσθαι, μάταιον ἡγοῦμαι πεÏá½¶ τῆς τελευτῆς á½Î½Ï„ινοῦν ποιεῖσθαι λόγον. Δημοσθένους, Γ´ ᾿Ολυνθιακὸς Georgian: From a Unicode conference invitation: გთხáƒáƒ•თ áƒáƒ®áƒšáƒáƒ•ე გáƒáƒ˜áƒáƒ áƒáƒ— რეგისტრáƒáƒªáƒ˜áƒ Unicode-ის მეáƒáƒ—ე სáƒáƒ”რთáƒáƒ¨áƒáƒ ისრკáƒáƒœáƒ¤áƒ”რენციáƒáƒ–ე დáƒáƒ¡áƒáƒ¡áƒ¬áƒ ებáƒáƒ“, რáƒáƒ›áƒ”ლიც გáƒáƒ˜áƒ›áƒáƒ თებრ10-12 მáƒáƒ ტს, ქ. მáƒáƒ˜áƒœáƒªáƒ¨áƒ˜, გერმáƒáƒœáƒ˜áƒáƒ¨áƒ˜. კáƒáƒœáƒ¤áƒ”რენცირშეჰკრებს ერთáƒáƒ“ მსáƒáƒ¤áƒšáƒ˜áƒáƒ¡ ექსპერტებს ისეთ დáƒáƒ გებში რáƒáƒ’áƒáƒ იცáƒáƒ ინტერნეტი დრUnicode-ი, ინტერნáƒáƒªáƒ˜áƒáƒœáƒáƒšáƒ˜áƒ–áƒáƒªáƒ˜áƒ დრლáƒáƒ™áƒáƒšáƒ˜áƒ–áƒáƒªáƒ˜áƒ, Unicode-ის გáƒáƒ›áƒáƒ§áƒ”ნებრáƒáƒžáƒ”რáƒáƒªáƒ˜áƒ£áƒš სისტემებსáƒ, დრგáƒáƒ›áƒáƒ§áƒ”ნებით პრáƒáƒ’რáƒáƒ›áƒ”ბში, შრიფტებში, ტექსტების დáƒáƒ›áƒ£áƒ¨áƒáƒ•ებáƒáƒ¡áƒ დრმრáƒáƒ•áƒáƒšáƒ”ნáƒáƒ•áƒáƒœ კáƒáƒ›áƒžáƒ˜áƒ£áƒ¢áƒ”რულ სისტემებში. Russian: From a Unicode conference invitation: ЗарегиÑтрируйтеÑÑŒ ÑÐµÐ¹Ñ‡Ð°Ñ Ð½Ð° ДеÑÑтую Международную Конференцию по Unicode, ÐºÐ¾Ñ‚Ð¾Ñ€Ð°Ñ ÑоÑтоитÑÑ 10-12 марта 1997 года в Майнце в Германии. ÐšÐ¾Ð½Ñ„ÐµÑ€ÐµÐ½Ñ†Ð¸Ñ Ñоберет широкий круг ÑкÑпертов по вопроÑам глобального Интернета и Unicode, локализации и интернационализации, воплощению и применению Unicode в различных операционных ÑиÑтемах и программных приложениÑÑ…, шрифтах, верÑтке и многоÑзычных компьютерных ÑиÑтемах. Thai (UCS Level 2): Excerpt from a poetry on The Romance of The Three Kingdoms (a Chinese classic 'San Gua'): [----------------------------|------------------------] ๠à¹à¸œà¹ˆà¸™à¸”ินฮั่นเสื่à¸à¸¡à¹‚ทรมà¹à¸ªà¸™à¸ªà¸±à¸‡à¹€à¸§à¸Š พระปà¸à¹€à¸à¸¨à¸à¸à¸‡à¸šà¸¹à¹Šà¸à¸¹à¹‰à¸‚ึ้นใหม่ สิบสà¸à¸‡à¸à¸©à¸±à¸•ริย์à¸à¹ˆà¸à¸™à¸«à¸™à¹‰à¸²à¹à¸¥à¸–ัดไป สà¸à¸‡à¸à¸‡à¸„์ไซร้โง่เขลาเบาปัà¸à¸à¸² ทรงนับถืà¸à¸‚ันทีเป็นที่พึ่ง บ้านเมืà¸à¸‡à¸ˆà¸¶à¸‡à¸§à¸´à¸›à¸£à¸´à¸•เป็นนัà¸à¸«à¸™à¸² โฮจิ๋นเรียà¸à¸—ัพทั่วหัวเมืà¸à¸‡à¸¡à¸² หมายจะฆ่ามดชั่วตัวสำคัภเหมืà¸à¸™à¸‚ับไสไล่เสืà¸à¸ˆà¸²à¸à¹€à¸„หา รับหมาป่าเข้ามาเลยà¸à¸²à¸ªà¸±à¸ à¸à¹ˆà¸²à¸¢à¸à¹‰à¸à¸‡à¸à¸¸à¹‰à¸™à¸¢à¸¸à¹à¸¢à¸à¹ƒà¸«à¹‰à¹à¸•à¸à¸à¸±à¸™ ใช้สาวนั้นเป็นชนวนชื่นชวนใจ พลันลิฉุยà¸à¸¸à¸¢à¸à¸µà¸à¸¥à¸±à¸šà¸à¹ˆà¸à¹€à¸«à¸•ุ ช่างà¸à¸²à¹€à¸žà¸¨à¸ˆà¸£à¸´à¸‡à¸«à¸™à¸²à¸Ÿà¹‰à¸²à¸£à¹‰à¸à¸‡à¹„ห้ ต้à¸à¸‡à¸£à¸šà¸£à¸²à¸†à¹ˆà¸²à¸Ÿà¸±à¸™à¸ˆà¸™à¸šà¸£à¸£à¸¥à¸±à¸¢ ฤๅหาใครค้ำชูà¸à¸¹à¹‰à¸šà¸£à¸£à¸¥à¸±à¸‡à¸à¹Œ ฯ (The above is a two-column text. If combining characters are handled correctly, the lines of the second column should be aligned with the | character above.) Ethiopian: Proverbs in the Amharic language: ሰማዠአá‹á‰³áˆ¨áˆµ ንጉሥ አá‹áŠ¨áˆ°áˆµá¢ á‰¥áˆ‹ ካለአእንደአባቴ በቆመጠáŠá¢ ጌጥ ያለቤቱ á‰áˆáŒ¥áŠ“ áŠá‹á¢ ደሀ በሕáˆáˆ™ ቅቤ ባá‹áŒ ጣ ንጣት በገደለá‹á¢ የአá ወለáˆá‰³ በቅቤ አá‹á‰³áˆ½áˆá¢ አá‹áŒ¥ በበላ ዳዋ ተመታᢠሲተረጉሙ á‹á‹°áˆ¨áŒáˆ™á¢ ቀስ በቀስᥠዕንá‰áˆ‹áˆ በእáŒáˆ© á‹áˆ„ዳáˆá¢ ድሠቢያብሠአንበሳ ያስáˆá¢ ሰዠእንደቤቱ እንጅ እንደ ጉረቤቱ አá‹á‰°á‹³á‹°áˆáˆá¢ እáŒá‹œáˆ የከáˆá‰°á‹áŠ• ጉሮሮ ሳá‹á‹˜áŒ‹á‹ አá‹á‹µáˆáˆá¢ የጎረቤት ሌባᥠቢያዩት á‹áˆµá‰… ባያዩት ያጠáˆá‰…ᢠሥራ ከመáታት áˆáŒ„ን ላá‹á‰³á‰µá¢ ዓባዠማደሪያ የለá‹á¥ áŒáŠ•á‹µ á‹á‹ž á‹á‹žáˆ«áˆá¢ የእስላሠአገሩ መካ የአሞራ አገሩ á‹‹áˆáŠ«á¢ á‰°áŠ•áŒ‹áˆŽ ቢተበተመáˆáˆ¶ ባá‰á¢ ወዳጅህ ማሠቢሆን ጨáˆáˆµáˆ… አትላሰá‹á¢ እáŒáˆáˆ…ን በáራሽህ áˆáŠ á‹˜áˆáŒ‹á¢ Runes: ᚻᛖ ᚳᚹᚫᚦ ᚦᚫᛠᚻᛖ ᛒᚢᛞᛖ ᚩᚾ ᚦᚫᛗ ᛚᚪᚾᛞᛖ ᚾᚩᚱᚦᚹᛖᚪᚱᛞᚢᛗ áš¹á›áš¦ ᚦᚪ ᚹᛖᛥᚫ (Old English, which transcribed into Latin reads 'He cwaeth that he bude thaem lande northweardum with tha Westsae.' and means 'He said that he lived in the northern land near the Western Sea.') Braille: ⡌â â §â ‘ â ¼â â ’ â¡â œâ ‡â ‘⠹⠰⠎ ⡣⠕⠌ â¡â œâ ‡â ‘â ¹ â ºâ â Ž ⠙⠑â ⠙⠒ â žâ • ⠃⠑⠛⠔ ⠺⠊⠹⠲ ⡹⠻⠑ â Šâ Ž â â • ⠙⠳⠃⠞ â ±â â žâ ‘â §â » â ⠃⠳⠞ â ¹â â žâ ² ⡹⠑ ⠗⠑⠛⠊⠌⠻ â •â ‹ ⠙⠊⠎ ⠃⠥⠗⠊â â ‡ â ºâ â Ž â Žâ Šâ ›â â « ⠃⠹ ⠹⠑ ⠊⠇⠻⠛⠹â â â â ‚ ⠹⠑ â Šâ ‡â »â …â ‚ ⠹⠑ â ¥â ⠙⠻⠞â â …â »â ‚ â â â ™ ⠹⠑ â ¡â Šâ ‘â ‹ â ⠳⠗â ⠻⠲ ⡎⠊⠗⠕⠕⠛⠑ â Žâ Šâ ›â â « â Šâ žâ ² â¡â â ™ ⡎⠊⠗⠕⠕⠛⠑⠰⠎ â â â â ‘ â ºâ â Ž ⠛⠕⠕⠙ â ¥â â •â â °â¡¡â â ⠛⠑⠂ â ‹â •â — â â ⠹⠹⠔⠛ ⠙⠑ â ¡â •â Žâ ‘ â žâ • â ⠥⠞ ⠙⠊⠎ â ™â â â ™ â žâ •â ² ⡕⠇⠙ â¡â œâ ‡â ‘â ¹ â ºâ â Ž â â Ž ⠙⠑â â ™ â â Ž â ⠙⠕⠕⠗⠤â â ⠊⠇⠲ â¡â ”⠙⠖ ⡊ ⠙⠕â â °â ž â â ‘â â â žâ • â Žâ â ¹ â ¹â â ž ⡊ â …â ⠪⠂ â •â ‹ â â ¹ â ªâ â …â ⠪⠇⠫⠛⠑⠂ â ±â â ž ⠹⠻⠑ â Šâ Ž â ⠜⠞⠊⠊⠥⠇⠜⠇⠹ ⠙⠑â â ™ â ⠃⠳⠞ â ⠙⠕⠕⠗⠤â â ⠊⠇⠲ ⡊ â ⠊⠣⠞ â ™â â §â ‘ ⠃⠑⠲ ⠔⠊⠇⠔⠫⠂ â ⠹⠎⠑⠇⠋⠂ â žâ • ⠗⠑⠛⠜⠙ â ⠊⠕⠋⠋⠔⠤â â â Šâ ‡ â â Ž ⠹⠑ ⠙⠑â ⠙⠑⠌ â â Šâ ‘â Šâ ‘ â •â ‹ â Šâ —â •â â â •â ⠛⠻⠹ â ” ⠹⠑ â žâ —â ⠙⠑⠲ ⡃⠥⠞ ⠹⠑ ⠺⠊⠎⠙⠕â â •â ‹ ⠳⠗ â â ⠊⠑⠌⠕⠗⠎ â Šâ Ž â ” ⠹⠑ â Žâ Šâ ⠊⠇⠑⠆ â â â ™ â â ¹ â ¥â â ™â ⠇⠇⠪⠫ â ™â â ⠙⠎ â ©â ⠇⠇ â â •â ž ⠙⠊⠌⠥⠗⠃ â Šâ žâ ‚ â •â — ⠹⠑ ⡊⠳â ⠞⠗⠹⠰⠎ ⠙⠕â â ‘ â ‹â •â —â ² ⡹⠳ ⠺⠊⠇⠇ ⠹⠻⠑⠋⠕⠗⠑ â â »â â Šâ ž â â ‘ â žâ • â —â ‘â â ‘â â žâ ‚ â ‘â â â ™â â žâ Šâ Šâ ⠇⠇⠹⠂ â ¹â â ž â¡â œâ ‡â ‘â ¹ â ºâ â Ž â â Ž ⠙⠑â â ™ â â Ž â ⠙⠕⠕⠗⠤â â ⠊⠇⠲ (The first couple of paragraphs of "A Christmas Carol" by Dickens) Compact font selection example text: ABCDEFGHIJKLMNOPQRSTUVWXYZ /0123456789 abcdefghijklmnopqrstuvwxyz £©µÀÆÖÞßéöÿ –—‘“â€â€žâ€ •…‰™œŠŸž€ ΑΒΓΔΩαβγδω ÐБВГДабвгд ∀∂∈â„∧∪≡∞ ↑↗↨↻⇣ â”┼╔╘░►☺♀ ï¬ï¿½â‘€â‚‚ἠḂӥẄÉËâŽ×ԱრGreetings in various languages: Hello world, ΚαλημέÏα κόσμε, コンニãƒãƒ Box drawing alignment tests: â–ˆ â–‰ â•”â•â•╦â•â•â•— ┌──┬──┠â•──┬──╮ â•──┬──╮ â”â”â”┳â”â”┓ ┎┒â”┑ â•· â•» â”┯┓ ┌┰┠▊ ╱╲╱╲╳╳╳ ║┌─╨─â”â•‘ │╔â•â•§â•╗│ │╒â•╪â•╕│ │╓─â•─╖│ ┃┌─╂─â”┃ ┗╃╄┙ ╶┼╴╺╋╸┠┼┨ â”╋┥ â–‹ ╲╱╲╱╳╳╳ ║│╲ ╱│║ │║ ║│ ││ │ ││ │║ ┃ ║│ ┃│ â•¿ │┃ â”╅╆┓ ╵ ╹ â”—â”·â”› └┸┘ â–Œ ╱╲╱╲╳╳╳ â• â•¡ ╳ ╞╣ ├╢ ╟┤ ├┼─┼─┼┤ ├╫─╂─╫┤ ┣┿╾┼╼┿┫ ┕┛┖┚ ┌┄┄┠╎ â”┅┅┓ ┋ ■╲╱╲╱╳╳╳ ║│╱ ╲│║ │║ ║│ ││ │ ││ │║ ┃ ║│ ┃│ ╽ │┃ ░░▒▒▓▓██ ┊ ┆ ╎ ╠┇ ┋ â–Ž ║└─╥─┘║ │╚â•╤â•â•│ │╘â•╪â•╛│ │╙─╀─╜│ ┃└─╂─┘┃ ░░▒▒▓▓██ ┊ ┆ ╎ ╠┇ ┋ ■╚â•â•â•©â•â•╠└──┴──┘ ╰──┴──╯ ╰──┴──╯ â”—â”â”â”»â”â”â”› ▗▄▖▛▀▜ └╌╌┘ ╎ â”—â•â•â”› ┋ â–▂▃▄▅▆▇█ â–▀▘▙▄▟ Surrogates: 𠜎 𠜱 ð ¹ ð ±“ 𠱸 ð ²– ð ³ ð ³• ð ´• ð µ¼ 𠵿 𠸎 ð ¸ ð ¹· ð º ð º¢ ð »— ð »¹ 𠻺 ð ¼ ð ¼® 𠽌 ð ¾´ ð ¾¼ 𠿪 𡜠𡯠𡵠𡶠𡻠𡃠𡃉 𡇙 𢃇 𢞵 𢫕 𢃠𢯊 𢱑 𢱕 𢳂 𢴈 𢵌 𢵧 𢺳 𣲷 𤓓 𤶸 𤷪 𥄫 𦉘 𦟌 𦧲 𦧺 𧨾 𨅠𨈇 𨋢 𨳊 𨳠𨳒 𩶘 apollo-server-demo/node_modules/@protobufjs/float/ 0000755 0001750 0000144 00000000000 14067647701 022045 5 ustar andreh users apollo-server-demo/node_modules/@protobufjs/float/index.js 0000644 0001750 0000144 00000026270 13067732227 023516 0 ustar andreh users "use strict"; module.exports = factory(factory); /** * Reads / writes floats / doubles from / to buffers. * @name util.float * @namespace */ /** * Writes a 32 bit float to a buffer using little endian byte order. * @name util.float.writeFloatLE * @function * @param {number} val Value to write * @param {Uint8Array} buf Target buffer * @param {number} pos Target buffer offset * @returns {undefined} */ /** * Writes a 32 bit float to a buffer using big endian byte order. * @name util.float.writeFloatBE * @function * @param {number} val Value to write * @param {Uint8Array} buf Target buffer * @param {number} pos Target buffer offset * @returns {undefined} */ /** * Reads a 32 bit float from a buffer using little endian byte order. * @name util.float.readFloatLE * @function * @param {Uint8Array} buf Source buffer * @param {number} pos Source buffer offset * @returns {number} Value read */ /** * Reads a 32 bit float from a buffer using big endian byte order. * @name util.float.readFloatBE * @function * @param {Uint8Array} buf Source buffer * @param {number} pos Source buffer offset * @returns {number} Value read */ /** * Writes a 64 bit double to a buffer using little endian byte order. * @name util.float.writeDoubleLE * @function * @param {number} val Value to write * @param {Uint8Array} buf Target buffer * @param {number} pos Target buffer offset * @returns {undefined} */ /** * Writes a 64 bit double to a buffer using big endian byte order. * @name util.float.writeDoubleBE * @function * @param {number} val Value to write * @param {Uint8Array} buf Target buffer * @param {number} pos Target buffer offset * @returns {undefined} */ /** * Reads a 64 bit double from a buffer using little endian byte order. * @name util.float.readDoubleLE * @function * @param {Uint8Array} buf Source buffer * @param {number} pos Source buffer offset * @returns {number} Value read */ /** * Reads a 64 bit double from a buffer using big endian byte order. * @name util.float.readDoubleBE * @function * @param {Uint8Array} buf Source buffer * @param {number} pos Source buffer offset * @returns {number} Value read */ // Factory function for the purpose of node-based testing in modified global environments function factory(exports) { // float: typed array if (typeof Float32Array !== "undefined") (function() { var f32 = new Float32Array([ -0 ]), f8b = new Uint8Array(f32.buffer), le = f8b[3] === 128; function writeFloat_f32_cpy(val, buf, pos) { f32[0] = val; buf[pos ] = f8b[0]; buf[pos + 1] = f8b[1]; buf[pos + 2] = f8b[2]; buf[pos + 3] = f8b[3]; } function writeFloat_f32_rev(val, buf, pos) { f32[0] = val; buf[pos ] = f8b[3]; buf[pos + 1] = f8b[2]; buf[pos + 2] = f8b[1]; buf[pos + 3] = f8b[0]; } /* istanbul ignore next */ exports.writeFloatLE = le ? writeFloat_f32_cpy : writeFloat_f32_rev; /* istanbul ignore next */ exports.writeFloatBE = le ? writeFloat_f32_rev : writeFloat_f32_cpy; function readFloat_f32_cpy(buf, pos) { f8b[0] = buf[pos ]; f8b[1] = buf[pos + 1]; f8b[2] = buf[pos + 2]; f8b[3] = buf[pos + 3]; return f32[0]; } function readFloat_f32_rev(buf, pos) { f8b[3] = buf[pos ]; f8b[2] = buf[pos + 1]; f8b[1] = buf[pos + 2]; f8b[0] = buf[pos + 3]; return f32[0]; } /* istanbul ignore next */ exports.readFloatLE = le ? readFloat_f32_cpy : readFloat_f32_rev; /* istanbul ignore next */ exports.readFloatBE = le ? readFloat_f32_rev : readFloat_f32_cpy; // float: ieee754 })(); else (function() { function writeFloat_ieee754(writeUint, val, buf, pos) { var sign = val < 0 ? 1 : 0; if (sign) val = -val; if (val === 0) writeUint(1 / val > 0 ? /* positive */ 0 : /* negative 0 */ 2147483648, buf, pos); else if (isNaN(val)) writeUint(2143289344, buf, pos); else if (val > 3.4028234663852886e+38) // +-Infinity writeUint((sign << 31 | 2139095040) >>> 0, buf, pos); else if (val < 1.1754943508222875e-38) // denormal writeUint((sign << 31 | Math.round(val / 1.401298464324817e-45)) >>> 0, buf, pos); else { var exponent = Math.floor(Math.log(val) / Math.LN2), mantissa = Math.round(val * Math.pow(2, -exponent) * 8388608) & 8388607; writeUint((sign << 31 | exponent + 127 << 23 | mantissa) >>> 0, buf, pos); } } exports.writeFloatLE = writeFloat_ieee754.bind(null, writeUintLE); exports.writeFloatBE = writeFloat_ieee754.bind(null, writeUintBE); function readFloat_ieee754(readUint, buf, pos) { var uint = readUint(buf, pos), sign = (uint >> 31) * 2 + 1, exponent = uint >>> 23 & 255, mantissa = uint & 8388607; return exponent === 255 ? mantissa ? NaN : sign * Infinity : exponent === 0 // denormal ? sign * 1.401298464324817e-45 * mantissa : sign * Math.pow(2, exponent - 150) * (mantissa + 8388608); } exports.readFloatLE = readFloat_ieee754.bind(null, readUintLE); exports.readFloatBE = readFloat_ieee754.bind(null, readUintBE); })(); // double: typed array if (typeof Float64Array !== "undefined") (function() { var f64 = new Float64Array([-0]), f8b = new Uint8Array(f64.buffer), le = f8b[7] === 128; function writeDouble_f64_cpy(val, buf, pos) { f64[0] = val; buf[pos ] = f8b[0]; buf[pos + 1] = f8b[1]; buf[pos + 2] = f8b[2]; buf[pos + 3] = f8b[3]; buf[pos + 4] = f8b[4]; buf[pos + 5] = f8b[5]; buf[pos + 6] = f8b[6]; buf[pos + 7] = f8b[7]; } function writeDouble_f64_rev(val, buf, pos) { f64[0] = val; buf[pos ] = f8b[7]; buf[pos + 1] = f8b[6]; buf[pos + 2] = f8b[5]; buf[pos + 3] = f8b[4]; buf[pos + 4] = f8b[3]; buf[pos + 5] = f8b[2]; buf[pos + 6] = f8b[1]; buf[pos + 7] = f8b[0]; } /* istanbul ignore next */ exports.writeDoubleLE = le ? writeDouble_f64_cpy : writeDouble_f64_rev; /* istanbul ignore next */ exports.writeDoubleBE = le ? writeDouble_f64_rev : writeDouble_f64_cpy; function readDouble_f64_cpy(buf, pos) { f8b[0] = buf[pos ]; f8b[1] = buf[pos + 1]; f8b[2] = buf[pos + 2]; f8b[3] = buf[pos + 3]; f8b[4] = buf[pos + 4]; f8b[5] = buf[pos + 5]; f8b[6] = buf[pos + 6]; f8b[7] = buf[pos + 7]; return f64[0]; } function readDouble_f64_rev(buf, pos) { f8b[7] = buf[pos ]; f8b[6] = buf[pos + 1]; f8b[5] = buf[pos + 2]; f8b[4] = buf[pos + 3]; f8b[3] = buf[pos + 4]; f8b[2] = buf[pos + 5]; f8b[1] = buf[pos + 6]; f8b[0] = buf[pos + 7]; return f64[0]; } /* istanbul ignore next */ exports.readDoubleLE = le ? readDouble_f64_cpy : readDouble_f64_rev; /* istanbul ignore next */ exports.readDoubleBE = le ? readDouble_f64_rev : readDouble_f64_cpy; // double: ieee754 })(); else (function() { function writeDouble_ieee754(writeUint, off0, off1, val, buf, pos) { var sign = val < 0 ? 1 : 0; if (sign) val = -val; if (val === 0) { writeUint(0, buf, pos + off0); writeUint(1 / val > 0 ? /* positive */ 0 : /* negative 0 */ 2147483648, buf, pos + off1); } else if (isNaN(val)) { writeUint(0, buf, pos + off0); writeUint(2146959360, buf, pos + off1); } else if (val > 1.7976931348623157e+308) { // +-Infinity writeUint(0, buf, pos + off0); writeUint((sign << 31 | 2146435072) >>> 0, buf, pos + off1); } else { var mantissa; if (val < 2.2250738585072014e-308) { // denormal mantissa = val / 5e-324; writeUint(mantissa >>> 0, buf, pos + off0); writeUint((sign << 31 | mantissa / 4294967296) >>> 0, buf, pos + off1); } else { var exponent = Math.floor(Math.log(val) / Math.LN2); if (exponent === 1024) exponent = 1023; mantissa = val * Math.pow(2, -exponent); writeUint(mantissa * 4503599627370496 >>> 0, buf, pos + off0); writeUint((sign << 31 | exponent + 1023 << 20 | mantissa * 1048576 & 1048575) >>> 0, buf, pos + off1); } } } exports.writeDoubleLE = writeDouble_ieee754.bind(null, writeUintLE, 0, 4); exports.writeDoubleBE = writeDouble_ieee754.bind(null, writeUintBE, 4, 0); function readDouble_ieee754(readUint, off0, off1, buf, pos) { var lo = readUint(buf, pos + off0), hi = readUint(buf, pos + off1); var sign = (hi >> 31) * 2 + 1, exponent = hi >>> 20 & 2047, mantissa = 4294967296 * (hi & 1048575) + lo; return exponent === 2047 ? mantissa ? NaN : sign * Infinity : exponent === 0 // denormal ? sign * 5e-324 * mantissa : sign * Math.pow(2, exponent - 1075) * (mantissa + 4503599627370496); } exports.readDoubleLE = readDouble_ieee754.bind(null, readUintLE, 0, 4); exports.readDoubleBE = readDouble_ieee754.bind(null, readUintBE, 4, 0); })(); return exports; } // uint helpers function writeUintLE(val, buf, pos) { buf[pos ] = val & 255; buf[pos + 1] = val >>> 8 & 255; buf[pos + 2] = val >>> 16 & 255; buf[pos + 3] = val >>> 24; } function writeUintBE(val, buf, pos) { buf[pos ] = val >>> 24; buf[pos + 1] = val >>> 16 & 255; buf[pos + 2] = val >>> 8 & 255; buf[pos + 3] = val & 255; } function readUintLE(buf, pos) { return (buf[pos ] | buf[pos + 1] << 8 | buf[pos + 2] << 16 | buf[pos + 3] << 24) >>> 0; } function readUintBE(buf, pos) { return (buf[pos ] << 24 | buf[pos + 1] << 16 | buf[pos + 2] << 8 | buf[pos + 3]) >>> 0; } apollo-server-demo/node_modules/@protobufjs/float/LICENSE 0000644 0001750 0000144 00000002741 13067721766 023061 0 ustar andreh users Copyright (c) 2016, Daniel Wirtz All rights reserved. Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met: * Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer. * Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution. * Neither the name of its author, nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission. THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. apollo-server-demo/node_modules/@protobufjs/float/index.d.ts 0000644 0001750 0000144 00000004757 13067726410 023755 0 ustar andreh users /** * Writes a 32 bit float to a buffer using little endian byte order. * @name writeFloatLE * @function * @param {number} val Value to write * @param {Uint8Array} buf Target buffer * @param {number} pos Target buffer offset * @returns {undefined} */ export function writeFloatLE(val: number, buf: Uint8Array, pos: number): void; /** * Writes a 32 bit float to a buffer using big endian byte order. * @name writeFloatBE * @function * @param {number} val Value to write * @param {Uint8Array} buf Target buffer * @param {number} pos Target buffer offset * @returns {undefined} */ export function writeFloatBE(val: number, buf: Uint8Array, pos: number): void; /** * Reads a 32 bit float from a buffer using little endian byte order. * @name readFloatLE * @function * @param {Uint8Array} buf Source buffer * @param {number} pos Source buffer offset * @returns {number} Value read */ export function readFloatLE(buf: Uint8Array, pos: number): number; /** * Reads a 32 bit float from a buffer using big endian byte order. * @name readFloatBE * @function * @param {Uint8Array} buf Source buffer * @param {number} pos Source buffer offset * @returns {number} Value read */ export function readFloatBE(buf: Uint8Array, pos: number): number; /** * Writes a 64 bit double to a buffer using little endian byte order. * @name writeDoubleLE * @function * @param {number} val Value to write * @param {Uint8Array} buf Target buffer * @param {number} pos Target buffer offset * @returns {undefined} */ export function writeDoubleLE(val: number, buf: Uint8Array, pos: number): void; /** * Writes a 64 bit double to a buffer using big endian byte order. * @name writeDoubleBE * @function * @param {number} val Value to write * @param {Uint8Array} buf Target buffer * @param {number} pos Target buffer offset * @returns {undefined} */ export function writeDoubleBE(val: number, buf: Uint8Array, pos: number): void; /** * Reads a 64 bit double from a buffer using little endian byte order. * @name readDoubleLE * @function * @param {Uint8Array} buf Source buffer * @param {number} pos Source buffer offset * @returns {number} Value read */ export function readDoubleLE(buf: Uint8Array, pos: number): number; /** * Reads a 64 bit double from a buffer using big endian byte order. * @name readDoubleBE * @function * @param {Uint8Array} buf Source buffer * @param {number} pos Source buffer offset * @returns {number} Value read */ export function readDoubleBE(buf: Uint8Array, pos: number): number; apollo-server-demo/node_modules/@protobufjs/float/README.md 0000644 0001750 0000144 00000010060 13070152713 023304 0 ustar andreh users @protobufjs/float ================= [](https://www.npmjs.com/package/@protobufjs/float) Reads / writes floats / doubles from / to buffers in both modern and ancient browsers. Fast. API --- * **writeFloatLE(val: `number`, buf: `Uint8Array`, pos: `number`)**<br /> Writes a 32 bit float to a buffer using little endian byte order. * **writeFloatBE(val: `number`, buf: `Uint8Array`, pos: `number`)**<br /> Writes a 32 bit float to a buffer using big endian byte order. * **readFloatLE(buf: `Uint8Array`, pos: `number`): `number`**<br /> Reads a 32 bit float from a buffer using little endian byte order. * **readFloatBE(buf: `Uint8Array`, pos: `number`): `number`**<br /> Reads a 32 bit float from a buffer using big endian byte order. * **writeDoubleLE(val: `number`, buf: `Uint8Array`, pos: `number`)**<br /> Writes a 64 bit double to a buffer using little endian byte order. * **writeDoubleBE(val: `number`, buf: `Uint8Array`, pos: `number`)**<br /> Writes a 64 bit double to a buffer using big endian byte order. * **readDoubleLE(buf: `Uint8Array`, pos: `number`): `number`**<br /> Reads a 64 bit double from a buffer using little endian byte order. * **readDoubleBE(buf: `Uint8Array`, pos: `number`): `number`**<br /> Reads a 64 bit double from a buffer using big endian byte order. Performance ----------- There is a simple benchmark included comparing raw read/write performance of this library (float), float's fallback for old browsers, the [ieee754](https://www.npmjs.com/package/ieee754) module and node's [buffer](https://nodejs.org/api/buffer.html). On an i7-2600k running node 6.9.1 it yields: ``` benchmarking writeFloat performance ... float x 42,741,625 ops/sec ±1.75% (81 runs sampled) float (fallback) x 11,272,532 ops/sec ±1.12% (85 runs sampled) ieee754 x 8,653,337 ops/sec ±1.18% (84 runs sampled) buffer x 12,412,414 ops/sec ±1.41% (83 runs sampled) buffer (noAssert) x 13,471,149 ops/sec ±1.09% (84 runs sampled) float was fastest float (fallback) was 73.5% slower ieee754 was 79.6% slower buffer was 70.9% slower buffer (noAssert) was 68.3% slower benchmarking readFloat performance ... float x 44,382,729 ops/sec ±1.70% (84 runs sampled) float (fallback) x 20,925,938 ops/sec ±0.86% (87 runs sampled) ieee754 x 17,189,009 ops/sec ±1.01% (87 runs sampled) buffer x 10,518,437 ops/sec ±1.04% (83 runs sampled) buffer (noAssert) x 11,031,636 ops/sec ±1.15% (87 runs sampled) float was fastest float (fallback) was 52.5% slower ieee754 was 61.0% slower buffer was 76.1% slower buffer (noAssert) was 75.0% slower benchmarking writeDouble performance ... float x 38,624,906 ops/sec ±0.93% (83 runs sampled) float (fallback) x 10,457,811 ops/sec ±1.54% (85 runs sampled) ieee754 x 7,681,130 ops/sec ±1.11% (83 runs sampled) buffer x 12,657,876 ops/sec ±1.03% (83 runs sampled) buffer (noAssert) x 13,372,795 ops/sec ±0.84% (85 runs sampled) float was fastest float (fallback) was 73.1% slower ieee754 was 80.1% slower buffer was 67.3% slower buffer (noAssert) was 65.3% slower benchmarking readDouble performance ... float x 40,527,888 ops/sec ±1.05% (84 runs sampled) float (fallback) x 18,696,480 ops/sec ±0.84% (86 runs sampled) ieee754 x 14,074,028 ops/sec ±1.04% (87 runs sampled) buffer x 10,092,367 ops/sec ±1.15% (84 runs sampled) buffer (noAssert) x 10,623,793 ops/sec ±0.96% (84 runs sampled) float was fastest float (fallback) was 53.8% slower ieee754 was 65.3% slower buffer was 75.1% slower buffer (noAssert) was 73.8% slower ``` To run it yourself: ``` $> npm run bench ``` **License:** [BSD 3-Clause License](https://opensource.org/licenses/BSD-3-Clause) apollo-server-demo/node_modules/@protobufjs/float/package.json 0000644 0001750 0000144 00000001611 13070153065 024316 0 ustar andreh users { "name": "@protobufjs/float", "description": "Reads / writes floats / doubles from / to buffers in both modern and ancient browsers.", "version": "1.0.2", "author": "Daniel Wirtz <dcode+protobufjs@dcode.io>", "repository": { "type": "git", "url": "https://github.com/dcodeIO/protobuf.js.git" }, "dependencies": {}, "license": "BSD-3-Clause", "main": "index.js", "types": "index.d.ts", "devDependencies": { "benchmark": "^2.1.4", "chalk": "^1.1.3", "ieee754": "^1.1.8", "istanbul": "^0.4.5", "tape": "^4.6.3" }, "scripts": { "test": "tape tests/*.js", "coverage": "istanbul cover node_modules/tape/bin/tape tests/*.js", "bench": "node bench" } ,"_resolved": "https://registry.npmjs.org/@protobufjs/float/-/float-1.0.2.tgz" ,"_integrity": "sha1-Xp4avctz/Ap8uLKR33jIy9l7h9E=" ,"_from": "@protobufjs/float@1.0.2" } apollo-server-demo/node_modules/@protobufjs/float/bench/ 0000755 0001750 0000144 00000000000 14067647701 023124 5 ustar andreh users apollo-server-demo/node_modules/@protobufjs/float/bench/index.js 0000644 0001750 0000144 00000003656 13070152512 024563 0 ustar andreh users "use strict"; var float = require(".."), ieee754 = require("ieee754"), newSuite = require("./suite"); var F32 = Float32Array; var F64 = Float64Array; delete global.Float32Array; delete global.Float64Array; var floatFallback = float({}); global.Float32Array = F32; global.Float64Array = F64; var buf = new Buffer(8); newSuite("writeFloat") .add("float", function() { float.writeFloatLE(0.1, buf, 0); }) .add("float (fallback)", function() { floatFallback.writeFloatLE(0.1, buf, 0); }) .add("ieee754", function() { ieee754.write(buf, 0.1, 0, true, 23, 4); }) .add("buffer", function() { buf.writeFloatLE(0.1, 0); }) .add("buffer (noAssert)", function() { buf.writeFloatLE(0.1, 0, true); }) .run(); newSuite("readFloat") .add("float", function() { float.readFloatLE(buf, 0); }) .add("float (fallback)", function() { floatFallback.readFloatLE(buf, 0); }) .add("ieee754", function() { ieee754.read(buf, 0, true, 23, 4); }) .add("buffer", function() { buf.readFloatLE(0); }) .add("buffer (noAssert)", function() { buf.readFloatLE(0, true); }) .run(); newSuite("writeDouble") .add("float", function() { float.writeDoubleLE(0.1, buf, 0); }) .add("float (fallback)", function() { floatFallback.writeDoubleLE(0.1, buf, 0); }) .add("ieee754", function() { ieee754.write(buf, 0.1, 0, true, 52, 8); }) .add("buffer", function() { buf.writeDoubleLE(0.1, 0); }) .add("buffer (noAssert)", function() { buf.writeDoubleLE(0.1, 0, true); }) .run(); newSuite("readDouble") .add("float", function() { float.readDoubleLE(buf, 0); }) .add("float (fallback)", function() { floatFallback.readDoubleLE(buf, 0); }) .add("ieee754", function() { ieee754.read(buf, 0, true, 52, 8); }) .add("buffer", function() { buf.readDoubleLE(0); }) .add("buffer (noAssert)", function() { buf.readDoubleLE(0, true); }) .run(); apollo-server-demo/node_modules/@protobufjs/float/bench/suite.js 0000644 0001750 0000144 00000002762 13070150151 024577 0 ustar andreh users "use strict"; module.exports = newSuite; var benchmark = require("benchmark"), chalk = require("chalk"); var padSize = 27; function newSuite(name) { var benches = []; return new benchmark.Suite(name) .on("add", function(event) { benches.push(event.target); }) .on("start", function() { process.stdout.write("benchmarking " + name + " performance ...\n\n"); }) .on("cycle", function(event) { process.stdout.write(String(event.target) + "\n"); }) .on("complete", function() { if (benches.length > 1) { var fastest = this.filter("fastest"), // eslint-disable-line no-invalid-this fastestHz = getHz(fastest[0]); process.stdout.write("\n" + chalk.white(pad(fastest[0].name, padSize)) + " was " + chalk.green("fastest") + "\n"); benches.forEach(function(bench) { if (fastest.indexOf(bench) === 0) return; var hz = hz = getHz(bench); var percent = (1 - hz / fastestHz) * 100; process.stdout.write(chalk.white(pad(bench.name, padSize)) + " was " + chalk.red(percent.toFixed(1) + "% slower") + "\n"); }); } process.stdout.write("\n"); }); } function getHz(bench) { return 1 / (bench.stats.mean + bench.stats.moe); } function pad(str, len, l) { while (str.length < len) str = l ? str + " " : " " + str; return str; } apollo-server-demo/node_modules/@protobufjs/float/tests/ 0000755 0001750 0000144 00000000000 14067647701 023207 5 ustar andreh users apollo-server-demo/node_modules/@protobufjs/float/tests/index.js 0000644 0001750 0000144 00000006036 13067726257 024664 0 ustar andreh users var tape = require("tape"); var float = require(".."); tape.test("float", function(test) { // default test.test(test.name + " - typed array", function(test) { runTest(float, test); }); // ieee754 test.test(test.name + " - fallback", function(test) { var F32 = global.Float32Array, F64 = global.Float64Array; delete global.Float32Array; delete global.Float64Array; runTest(float({}), test); global.Float32Array = F32; global.Float64Array = F64; }); }); function runTest(float, test) { var common = [ 0, -0, Infinity, -Infinity, 0.125, 1024.5, -4096.5, NaN ]; test.test(test.name + " - using 32 bits", function(test) { common.concat([ 3.4028234663852886e+38, 1.1754943508222875e-38, 1.1754946310819804e-39 ]) .forEach(function(value) { var strval = value === 0 && 1 / value < 0 ? "-0" : value.toString(); test.ok( checkValue(value, 4, float.readFloatLE, float.writeFloatLE, Buffer.prototype.writeFloatLE), "should write and read back " + strval + " (32 bit LE)" ); test.ok( checkValue(value, 4, float.readFloatBE, float.writeFloatBE, Buffer.prototype.writeFloatBE), "should write and read back " + strval + " (32 bit BE)" ); }); test.end(); }); test.test(test.name + " - using 64 bits", function(test) { common.concat([ 1.7976931348623157e+308, 2.2250738585072014e-308, 2.2250738585072014e-309 ]) .forEach(function(value) { var strval = value === 0 && 1 / value < 0 ? "-0" : value.toString(); test.ok( checkValue(value, 8, float.readDoubleLE, float.writeDoubleLE, Buffer.prototype.writeDoubleLE), "should write and read back " + strval + " (64 bit LE)" ); test.ok( checkValue(value, 8, float.readDoubleBE, float.writeDoubleBE, Buffer.prototype.writeDoubleBE), "should write and read back " + strval + " (64 bit BE)" ); }); test.end(); }); test.end(); } function checkValue(value, size, read, write, write_comp) { var buffer = new Buffer(size); write(value, buffer, 0); var value_comp = read(buffer, 0); var strval = value === 0 && 1 / value < 0 ? "-0" : value.toString(); if (value !== value) { if (value_comp === value_comp) return false; } else if (value_comp !== value) return false; var buffer_comp = new Buffer(size); write_comp.call(buffer_comp, value, 0); for (var i = 0; i < size; ++i) if (buffer[i] !== buffer_comp[i]) { console.error(">", buffer, buffer_comp); return false; } return true; } apollo-server-demo/node_modules/@protobufjs/aspromise/ 0000755 0001750 0000144 00000000000 14067647701 022742 5 ustar andreh users apollo-server-demo/node_modules/@protobufjs/aspromise/index.js 0000644 0001750 0000144 00000003070 13077352365 024406 0 ustar andreh users "use strict"; module.exports = asPromise; /** * Callback as used by {@link util.asPromise}. * @typedef asPromiseCallback * @type {function} * @param {Error|null} error Error, if any * @param {...*} params Additional arguments * @returns {undefined} */ /** * Returns a promise from a node-style callback function. * @memberof util * @param {asPromiseCallback} fn Function to call * @param {*} ctx Function context * @param {...*} params Function arguments * @returns {Promise<*>} Promisified function */ function asPromise(fn, ctx/*, varargs */) { var params = new Array(arguments.length - 1), offset = 0, index = 2, pending = true; while (index < arguments.length) params[offset++] = arguments[index++]; return new Promise(function executor(resolve, reject) { params[offset] = function callback(err/*, varargs */) { if (pending) { pending = false; if (err) reject(err); else { var params = new Array(arguments.length - 1), offset = 0; while (offset < params.length) params[offset++] = arguments[offset]; resolve.apply(null, params); } } }; try { fn.apply(ctx || null, params); } catch (err) { if (pending) { pending = false; reject(err); } } }); } apollo-server-demo/node_modules/@protobufjs/aspromise/LICENSE 0000644 0001750 0000144 00000002741 13026320441 023732 0 ustar andreh users Copyright (c) 2016, Daniel Wirtz All rights reserved. Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met: * Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer. * Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution. * Neither the name of its author, nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission. THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. apollo-server-demo/node_modules/@protobufjs/aspromise/index.d.ts 0000644 0001750 0000144 00000000712 13072666543 024643 0 ustar andreh users export = asPromise; type asPromiseCallback = (error: Error | null, ...params: any[]) => {}; /** * Returns a promise from a node-style callback function. * @memberof util * @param {asPromiseCallback} fn Function to call * @param {*} ctx Function context * @param {...*} params Function arguments * @returns {Promise<*>} Promisified function */ declare function asPromise(fn: asPromiseCallback, ctx: any, ...params: any[]): Promise<any>; apollo-server-demo/node_modules/@protobufjs/aspromise/README.md 0000644 0001750 0000144 00000000720 13026321555 024206 0 ustar andreh users @protobufjs/aspromise ===================== [](https://www.npmjs.com/package/@protobufjs/aspromise) Returns a promise from a node-style callback function. API --- * **asPromise(fn: `function`, ctx: `Object`, ...params: `*`): `Promise<*>`**<br /> Returns a promise from a node-style callback function. **License:** [BSD 3-Clause License](https://opensource.org/licenses/BSD-3-Clause) apollo-server-demo/node_modules/@protobufjs/aspromise/package.json 0000644 0001750 0000144 00000001370 13077352551 025225 0 ustar andreh users { "name": "@protobufjs/aspromise", "description": "Returns a promise from a node-style callback function.", "version": "1.1.2", "author": "Daniel Wirtz <dcode+protobufjs@dcode.io>", "repository": { "type": "git", "url": "https://github.com/dcodeIO/protobuf.js.git" }, "license": "BSD-3-Clause", "main": "index.js", "types": "index.d.ts", "devDependencies": { "istanbul": "^0.4.5", "tape": "^4.6.3" }, "scripts": { "test": "tape tests/*.js", "coverage": "istanbul cover node_modules/tape/bin/tape tests/*.js" } ,"_resolved": "https://registry.npmjs.org/@protobufjs/aspromise/-/aspromise-1.1.2.tgz" ,"_integrity": "sha1-m4sMxmPWaafY9vXQiToU00jzD78=" ,"_from": "@protobufjs/aspromise@1.1.2" } apollo-server-demo/node_modules/@protobufjs/aspromise/tests/ 0000755 0001750 0000144 00000000000 14067647701 024104 5 ustar andreh users apollo-server-demo/node_modules/@protobufjs/aspromise/tests/index.js 0000644 0001750 0000144 00000010547 13042167400 025541 0 ustar andreh users var tape = require("tape"); var asPromise = require(".."); tape.test("aspromise", function(test) { test.test(this.name + " - resolve", function(test) { function fn(arg1, arg2, callback) { test.equal(this, ctx, "function should be called with this = ctx"); test.equal(arg1, 1, "function should be called with arg1 = 1"); test.equal(arg2, 2, "function should be called with arg2 = 2"); callback(null, arg2); } var ctx = {}; var promise = asPromise(fn, ctx, 1, 2); promise.then(function(arg2) { test.equal(arg2, 2, "promise should be resolved with arg2 = 2"); test.end(); }).catch(function(err) { test.fail("promise should not be rejected (" + err + ")"); }); }); test.test(this.name + " - reject", function(test) { function fn(arg1, arg2, callback) { test.equal(this, ctx, "function should be called with this = ctx"); test.equal(arg1, 1, "function should be called with arg1 = 1"); test.equal(arg2, 2, "function should be called with arg2 = 2"); callback(arg1); } var ctx = {}; var promise = asPromise(fn, ctx, 1, 2); promise.then(function() { test.fail("promise should not be resolved"); }).catch(function(err) { test.equal(err, 1, "promise should be rejected with err = 1"); test.end(); }); }); test.test(this.name + " - resolve twice", function(test) { function fn(arg1, arg2, callback) { test.equal(this, ctx, "function should be called with this = ctx"); test.equal(arg1, 1, "function should be called with arg1 = 1"); test.equal(arg2, 2, "function should be called with arg2 = 2"); callback(null, arg2); callback(null, arg1); } var ctx = {}; var count = 0; var promise = asPromise(fn, ctx, 1, 2); promise.then(function(arg2) { test.equal(arg2, 2, "promise should be resolved with arg2 = 2"); if (++count > 1) test.fail("promise should not be resolved twice"); test.end(); }).catch(function(err) { test.fail("promise should not be rejected (" + err + ")"); }); }); test.test(this.name + " - reject twice", function(test) { function fn(arg1, arg2, callback) { test.equal(this, ctx, "function should be called with this = ctx"); test.equal(arg1, 1, "function should be called with arg1 = 1"); test.equal(arg2, 2, "function should be called with arg2 = 2"); callback(arg1); callback(arg2); } var ctx = {}; var count = 0; var promise = asPromise(fn, ctx, 1, 2); promise.then(function() { test.fail("promise should not be resolved"); }).catch(function(err) { test.equal(err, 1, "promise should be rejected with err = 1"); if (++count > 1) test.fail("promise should not be rejected twice"); test.end(); }); }); test.test(this.name + " - reject error", function(test) { function fn(callback) { test.ok(arguments.length === 1 && typeof callback === "function", "function should be called with just a callback"); throw 3; } var promise = asPromise(fn, null); promise.then(function() { test.fail("promise should not be resolved"); }).catch(function(err) { test.equal(err, 3, "promise should be rejected with err = 3"); test.end(); }); }); test.test(this.name + " - reject and error", function(test) { function fn(callback) { callback(3); throw 4; } var count = 0; var promise = asPromise(fn, null); promise.then(function() { test.fail("promise should not be resolved"); }).catch(function(err) { test.equal(err, 3, "promise should be rejected with err = 3"); if (++count > 1) test.fail("promise should not be rejected twice"); test.end(); }); }); }); apollo-server-demo/node_modules/@protobufjs/eventemitter/ 0000755 0001750 0000144 00000000000 14067647701 023453 5 ustar andreh users apollo-server-demo/node_modules/@protobufjs/eventemitter/index.js 0000644 0001750 0000144 00000004050 13041011000 025057 0 ustar andreh users "use strict"; module.exports = EventEmitter; /** * Constructs a new event emitter instance. * @classdesc A minimal event emitter. * @memberof util * @constructor */ function EventEmitter() { /** * Registered listeners. * @type {Object.<string,*>} * @private */ this._listeners = {}; } /** * Registers an event listener. * @param {string} evt Event name * @param {function} fn Listener * @param {*} [ctx] Listener context * @returns {util.EventEmitter} `this` */ EventEmitter.prototype.on = function on(evt, fn, ctx) { (this._listeners[evt] || (this._listeners[evt] = [])).push({ fn : fn, ctx : ctx || this }); return this; }; /** * Removes an event listener or any matching listeners if arguments are omitted. * @param {string} [evt] Event name. Removes all listeners if omitted. * @param {function} [fn] Listener to remove. Removes all listeners of `evt` if omitted. * @returns {util.EventEmitter} `this` */ EventEmitter.prototype.off = function off(evt, fn) { if (evt === undefined) this._listeners = {}; else { if (fn === undefined) this._listeners[evt] = []; else { var listeners = this._listeners[evt]; for (var i = 0; i < listeners.length;) if (listeners[i].fn === fn) listeners.splice(i, 1); else ++i; } } return this; }; /** * Emits an event by calling its listeners with the specified arguments. * @param {string} evt Event name * @param {...*} args Arguments * @returns {util.EventEmitter} `this` */ EventEmitter.prototype.emit = function emit(evt) { var listeners = this._listeners[evt]; if (listeners) { var args = [], i = 1; for (; i < arguments.length;) args.push(arguments[i++]); for (i = 0; i < listeners.length;) listeners[i].fn.apply(listeners[i++].ctx, args); } return this; }; apollo-server-demo/node_modules/@protobufjs/eventemitter/LICENSE 0000644 0001750 0000144 00000002741 13026320544 024447 0 ustar andreh users Copyright (c) 2016, Daniel Wirtz All rights reserved. Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met: * Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer. * Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution. * Neither the name of its author, nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission. THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. apollo-server-demo/node_modules/@protobufjs/eventemitter/index.d.ts 0000644 0001750 0000144 00000002417 13031543411 025337 0 ustar andreh users export = EventEmitter; /** * Constructs a new event emitter instance. * @classdesc A minimal event emitter. * @memberof util * @constructor */ declare class EventEmitter { /** * Constructs a new event emitter instance. * @classdesc A minimal event emitter. * @memberof util * @constructor */ constructor(); /** * Registers an event listener. * @param {string} evt Event name * @param {function} fn Listener * @param {*} [ctx] Listener context * @returns {util.EventEmitter} `this` */ on(evt: string, fn: () => any, ctx?: any): EventEmitter; /** * Removes an event listener or any matching listeners if arguments are omitted. * @param {string} [evt] Event name. Removes all listeners if omitted. * @param {function} [fn] Listener to remove. Removes all listeners of `evt` if omitted. * @returns {util.EventEmitter} `this` */ off(evt?: string, fn?: () => any): EventEmitter; /** * Emits an event by calling its listeners with the specified arguments. * @param {string} evt Event name * @param {...*} args Arguments * @returns {util.EventEmitter} `this` */ emit(evt: string, ...args: any[]): EventEmitter; } apollo-server-demo/node_modules/@protobufjs/eventemitter/README.md 0000644 0001750 0000144 00000001466 13026321571 024725 0 ustar andreh users @protobufjs/eventemitter ======================== [](https://www.npmjs.com/package/@protobufjs/eventemitter) A minimal event emitter. API --- * **new EventEmitter()**<br /> Constructs a new event emitter instance. * **EventEmitter#on(evt: `string`, fn: `function`, [ctx: `Object`]): `EventEmitter`**<br /> Registers an event listener. * **EventEmitter#off([evt: `string`], [fn: `function`]): `EventEmitter`**<br /> Removes an event listener or any matching listeners if arguments are omitted. * **EventEmitter#emit(evt: `string`, ...args: `*`): `EventEmitter`**<br /> Emits an event by calling its listeners with the specified arguments. **License:** [BSD 3-Clause License](https://opensource.org/licenses/BSD-3-Clause) apollo-server-demo/node_modules/@protobufjs/eventemitter/package.json 0000644 0001750 0000144 00000001346 13042164765 025741 0 ustar andreh users { "name": "@protobufjs/eventemitter", "description": "A minimal event emitter.", "version": "1.1.0", "author": "Daniel Wirtz <dcode+protobufjs@dcode.io>", "repository": { "type": "git", "url": "https://github.com/dcodeIO/protobuf.js.git" }, "license": "BSD-3-Clause", "main": "index.js", "types": "index.d.ts", "devDependencies": { "istanbul": "^0.4.5", "tape": "^4.6.3" }, "scripts": { "test": "tape tests/*.js", "coverage": "istanbul cover node_modules/tape/bin/tape tests/*.js" } ,"_resolved": "https://registry.npmjs.org/@protobufjs/eventemitter/-/eventemitter-1.1.0.tgz" ,"_integrity": "sha1-NVy8mLr61ZePntCV85diHx0Ga3A=" ,"_from": "@protobufjs/eventemitter@1.1.0" } apollo-server-demo/node_modules/@protobufjs/eventemitter/tests/ 0000755 0001750 0000144 00000000000 14067647701 024615 5 ustar andreh users apollo-server-demo/node_modules/@protobufjs/eventemitter/tests/index.js 0000644 0001750 0000144 00000002720 13042164564 026254 0 ustar andreh users var tape = require("tape"); var EventEmitter = require(".."); tape.test("eventemitter", function(test) { var ee = new EventEmitter(); var fn; var ctx = {}; test.doesNotThrow(function() { ee.emit("a", 1); ee.off(); ee.off("a"); ee.off("a", function() {}); }, "should not throw if no listeners are registered"); test.equal(ee.on("a", function(arg1) { test.equal(this, ctx, "should be called with this = ctx"); test.equal(arg1, 1, "should be called with arg1 = 1"); }, ctx), ee, "should return itself when registering events"); ee.emit("a", 1); ee.off("a"); test.same(ee._listeners, { a: [] }, "should remove all listeners of the respective event when calling off(evt)"); ee.off(); test.same(ee._listeners, {}, "should remove all listeners when just calling off()"); ee.on("a", fn = function(arg1) { test.equal(this, ctx, "should be called with this = ctx"); test.equal(arg1, 1, "should be called with arg1 = 1"); }, ctx).emit("a", 1); ee.off("a", fn); test.same(ee._listeners, { a: [] }, "should remove the exact listener when calling off(evt, fn)"); ee.on("a", function() { test.equal(this, ee, "should be called with this = ee"); }).emit("a"); test.doesNotThrow(function() { ee.off("a", fn); }, "should not throw if no such listener is found"); test.end(); }); apollo-server-demo/node_modules/@protobufjs/fetch/ 0000755 0001750 0000144 00000000000 14067647701 022031 5 ustar andreh users apollo-server-demo/node_modules/@protobufjs/fetch/index.js 0000644 0001750 0000144 00000007653 13042655463 023505 0 ustar andreh users "use strict"; module.exports = fetch; var asPromise = require("@protobufjs/aspromise"), inquire = require("@protobufjs/inquire"); var fs = inquire("fs"); /** * Node-style callback as used by {@link util.fetch}. * @typedef FetchCallback * @type {function} * @param {?Error} error Error, if any, otherwise `null` * @param {string} [contents] File contents, if there hasn't been an error * @returns {undefined} */ /** * Options as used by {@link util.fetch}. * @typedef FetchOptions * @type {Object} * @property {boolean} [binary=false] Whether expecting a binary response * @property {boolean} [xhr=false] If `true`, forces the use of XMLHttpRequest */ /** * Fetches the contents of a file. * @memberof util * @param {string} filename File path or url * @param {FetchOptions} options Fetch options * @param {FetchCallback} callback Callback function * @returns {undefined} */ function fetch(filename, options, callback) { if (typeof options === "function") { callback = options; options = {}; } else if (!options) options = {}; if (!callback) return asPromise(fetch, this, filename, options); // eslint-disable-line no-invalid-this // if a node-like filesystem is present, try it first but fall back to XHR if nothing is found. if (!options.xhr && fs && fs.readFile) return fs.readFile(filename, function fetchReadFileCallback(err, contents) { return err && typeof XMLHttpRequest !== "undefined" ? fetch.xhr(filename, options, callback) : err ? callback(err) : callback(null, options.binary ? contents : contents.toString("utf8")); }); // use the XHR version otherwise. return fetch.xhr(filename, options, callback); } /** * Fetches the contents of a file. * @name util.fetch * @function * @param {string} path File path or url * @param {FetchCallback} callback Callback function * @returns {undefined} * @variation 2 */ /** * Fetches the contents of a file. * @name util.fetch * @function * @param {string} path File path or url * @param {FetchOptions} [options] Fetch options * @returns {Promise<string|Uint8Array>} Promise * @variation 3 */ /**/ fetch.xhr = function fetch_xhr(filename, options, callback) { var xhr = new XMLHttpRequest(); xhr.onreadystatechange /* works everywhere */ = function fetchOnReadyStateChange() { if (xhr.readyState !== 4) return undefined; // local cors security errors return status 0 / empty string, too. afaik this cannot be // reliably distinguished from an actually empty file for security reasons. feel free // to send a pull request if you are aware of a solution. if (xhr.status !== 0 && xhr.status !== 200) return callback(Error("status " + xhr.status)); // if binary data is expected, make sure that some sort of array is returned, even if // ArrayBuffers are not supported. the binary string fallback, however, is unsafe. if (options.binary) { var buffer = xhr.response; if (!buffer) { buffer = []; for (var i = 0; i < xhr.responseText.length; ++i) buffer.push(xhr.responseText.charCodeAt(i) & 255); } return callback(null, typeof Uint8Array !== "undefined" ? new Uint8Array(buffer) : buffer); } return callback(null, xhr.responseText); }; if (options.binary) { // ref: https://developer.mozilla.org/en-US/docs/Web/API/XMLHttpRequest/Sending_and_Receiving_Binary_Data#Receiving_binary_data_in_older_browsers if ("overrideMimeType" in xhr) xhr.overrideMimeType("text/plain; charset=x-user-defined"); xhr.responseType = "arraybuffer"; } xhr.open("GET", filename); xhr.send(); }; apollo-server-demo/node_modules/@protobufjs/fetch/LICENSE 0000644 0001750 0000144 00000002741 13026320606 023024 0 ustar andreh users Copyright (c) 2016, Daniel Wirtz All rights reserved. Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met: * Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer. * Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution. * Neither the name of its author, nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission. THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. apollo-server-demo/node_modules/@protobufjs/fetch/index.d.ts 0000644 0001750 0000144 00000003157 13042655457 023737 0 ustar andreh users export = fetch; /** * Node-style callback as used by {@link util.fetch}. * @typedef FetchCallback * @type {function} * @param {?Error} error Error, if any, otherwise `null` * @param {string} [contents] File contents, if there hasn't been an error * @returns {undefined} */ type FetchCallback = (error: Error, contents?: string) => void; /** * Options as used by {@link util.fetch}. * @typedef FetchOptions * @type {Object} * @property {boolean} [binary=false] Whether expecting a binary response * @property {boolean} [xhr=false] If `true`, forces the use of XMLHttpRequest */ interface FetchOptions { binary?: boolean; xhr?: boolean } /** * Fetches the contents of a file. * @memberof util * @param {string} filename File path or url * @param {FetchOptions} options Fetch options * @param {FetchCallback} callback Callback function * @returns {undefined} */ declare function fetch(filename: string, options: FetchOptions, callback: FetchCallback): void; /** * Fetches the contents of a file. * @name util.fetch * @function * @param {string} path File path or url * @param {FetchCallback} callback Callback function * @returns {undefined} * @variation 2 */ declare function fetch(path: string, callback: FetchCallback): void; /** * Fetches the contents of a file. * @name util.fetch * @function * @param {string} path File path or url * @param {FetchOptions} [options] Fetch options * @returns {Promise<string|Uint8Array>} Promise * @variation 3 */ declare function fetch(path: string, options?: FetchOptions): Promise<(string|Uint8Array)>; apollo-server-demo/node_modules/@protobufjs/fetch/README.md 0000644 0001750 0000144 00000000771 13042513461 023300 0 ustar andreh users @protobufjs/fetch ================= [](https://www.npmjs.com/package/@protobufjs/fetch) Fetches the contents of a file accross node and browsers. API --- * **fetch(path: `string`, [options: { binary: boolean } ], [callback: `function(error: ?Error, [contents: string])`]): `Promise<string|Uint8Array>|undefined`** Fetches the contents of a file. **License:** [BSD 3-Clause License](https://opensource.org/licenses/BSD-3-Clause) apollo-server-demo/node_modules/@protobufjs/fetch/package.json 0000644 0001750 0000144 00000001523 13042666117 024312 0 ustar andreh users { "name": "@protobufjs/fetch", "description": "Fetches the contents of a file accross node and browsers.", "version": "1.1.0", "author": "Daniel Wirtz <dcode+protobufjs@dcode.io>", "repository": { "type": "git", "url": "https://github.com/dcodeIO/protobuf.js.git" }, "dependencies": { "@protobufjs/aspromise": "^1.1.1", "@protobufjs/inquire": "^1.1.0" }, "license": "BSD-3-Clause", "main": "index.js", "types": "index.d.ts", "devDependencies": { "istanbul": "^0.4.5", "tape": "^4.6.3" }, "scripts": { "test": "tape tests/*.js", "coverage": "istanbul cover node_modules/tape/bin/tape tests/*.js" } ,"_resolved": "https://registry.npmjs.org/@protobufjs/fetch/-/fetch-1.1.0.tgz" ,"_integrity": "sha1-upn7WYYUr2VwDBYZ/wbUVLDYTEU=" ,"_from": "@protobufjs/fetch@1.1.0" } apollo-server-demo/node_modules/@protobufjs/fetch/tests/ 0000755 0001750 0000144 00000000000 14067647701 023173 5 ustar andreh users apollo-server-demo/node_modules/@protobufjs/fetch/tests/index.js 0000644 0001750 0000144 00000000632 13042655244 024632 0 ustar andreh users var tape = require("tape"); var fetch = require(".."); tape.test("fetch", function(test) { if (typeof Promise !== "undefined") { var promise = fetch("NOTFOUND"); promise.catch(function() {}); test.ok(promise instanceof Promise, "should return a promise if callback has been omitted"); } // TODO - some way to test this properly? test.end(); }); apollo-server-demo/node_modules/@protobufjs/pool/ 0000755 0001750 0000144 00000000000 14067647701 021711 5 ustar andreh users apollo-server-demo/node_modules/@protobufjs/pool/index.js 0000644 0001750 0000144 00000002330 13042002172 023327 0 ustar andreh users "use strict"; module.exports = pool; /** * An allocator as used by {@link util.pool}. * @typedef PoolAllocator * @type {function} * @param {number} size Buffer size * @returns {Uint8Array} Buffer */ /** * A slicer as used by {@link util.pool}. * @typedef PoolSlicer * @type {function} * @param {number} start Start offset * @param {number} end End offset * @returns {Uint8Array} Buffer slice * @this {Uint8Array} */ /** * A general purpose buffer pool. * @memberof util * @function * @param {PoolAllocator} alloc Allocator * @param {PoolSlicer} slice Slicer * @param {number} [size=8192] Slab size * @returns {PoolAllocator} Pooled allocator */ function pool(alloc, slice, size) { var SIZE = size || 8192; var MAX = SIZE >>> 1; var slab = null; var offset = SIZE; return function pool_alloc(size) { if (size < 1 || size > MAX) return alloc(size); if (offset + size > SIZE) { slab = alloc(SIZE); offset = 0; } var buf = slice.call(slab, offset, offset += size); if (offset & 7) // align to 32 bit offset = (offset | 7) + 1; return buf; }; } apollo-server-demo/node_modules/@protobufjs/pool/LICENSE 0000644 0001750 0000144 00000002741 13026320671 022706 0 ustar andreh users Copyright (c) 2016, Daniel Wirtz All rights reserved. Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met: * Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer. * Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution. * Neither the name of its author, nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission. THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. apollo-server-demo/node_modules/@protobufjs/pool/index.d.ts 0000644 0001750 0000144 00000001611 13042002773 023573 0 ustar andreh users export = pool; /** * An allocator as used by {@link util.pool}. * @typedef PoolAllocator * @type {function} * @param {number} size Buffer size * @returns {Uint8Array} Buffer */ type PoolAllocator = (size: number) => Uint8Array; /** * A slicer as used by {@link util.pool}. * @typedef PoolSlicer * @type {function} * @param {number} start Start offset * @param {number} end End offset * @returns {Uint8Array} Buffer slice * @this {Uint8Array} */ type PoolSlicer = (this: Uint8Array, start: number, end: number) => Uint8Array; /** * A general purpose buffer pool. * @memberof util * @function * @param {PoolAllocator} alloc Allocator * @param {PoolSlicer} slice Slicer * @param {number} [size=8192] Slab size * @returns {PoolAllocator} Pooled allocator */ declare function pool(alloc: PoolAllocator, slice: PoolSlicer, size?: number): PoolAllocator; apollo-server-demo/node_modules/@protobufjs/pool/README.md 0000644 0001750 0000144 00000000776 13026321615 023165 0 ustar andreh users @protobufjs/pool ================ [](https://www.npmjs.com/package/@protobufjs/pool) A general purpose buffer pool. API --- * **pool(alloc: `function(size: number): Uint8Array`, slice: `function(this: Uint8Array, start: number, end: number): Uint8Array`, [size=8192: `number`]): `function(size: number): Uint8Array`**<br /> Creates a pooled allocator. **License:** [BSD 3-Clause License](https://opensource.org/licenses/BSD-3-Clause) apollo-server-demo/node_modules/@protobufjs/pool/package.json 0000644 0001750 0000144 00000001270 13042165036 024163 0 ustar andreh users { "name": "@protobufjs/pool", "description": "A general purpose buffer pool.", "version": "1.1.0", "author": "Daniel Wirtz <dcode+protobufjs@dcode.io>", "repository": { "type": "git", "url": "https://github.com/dcodeIO/protobuf.js.git" }, "license": "BSD-3-Clause", "main": "index.js", "types": "index.d.ts", "devDependencies": { "istanbul": "^0.4.5", "tape": "^4.6.3" }, "scripts": { "test": "tape tests/*.js", "coverage": "istanbul cover node_modules/tape/bin/tape tests/*.js" } ,"_resolved": "https://registry.npmjs.org/@protobufjs/pool/-/pool-1.1.0.tgz" ,"_integrity": "sha1-Cf0V8tbTq/qbZbw2ZQbWrXhG/1Q=" ,"_from": "@protobufjs/pool@1.1.0" } apollo-server-demo/node_modules/@protobufjs/pool/.npmignore 0000644 0001750 0000144 00000000047 13042002573 023671 0 ustar andreh users npm-debug.* node_modules/ coverage/ apollo-server-demo/node_modules/@protobufjs/pool/tests/ 0000755 0001750 0000144 00000000000 14067647701 023053 5 ustar andreh users apollo-server-demo/node_modules/@protobufjs/pool/tests/index.js 0000644 0001750 0000144 00000002755 13042161670 024515 0 ustar andreh users var tape = require("tape"); var pool = require(".."); if (typeof Uint8Array !== "undefined") tape.test("pool", function(test) { var alloc = pool(function(size) { return new Uint8Array(size); }, Uint8Array.prototype.subarray); var buf1 = alloc(0); test.equal(buf1.length, 0, "should allocate a buffer of size 0"); var buf2 = alloc(1); test.equal(buf2.length, 1, "should allocate a buffer of size 1 (initializes slab)"); test.notEqual(buf2.buffer, buf1.buffer, "should not reference the same backing buffer if previous buffer had size 0"); test.equal(buf2.byteOffset, 0, "should allocate at byteOffset 0 when using a new slab"); buf1 = alloc(1); test.equal(buf1.buffer, buf2.buffer, "should reference the same backing buffer when allocating a chunk fitting into the slab"); test.equal(buf1.byteOffset, 8, "should align slices to 32 bit and this allocate at byteOffset 8"); var buf3 = alloc(4097); test.notEqual(buf3.buffer, buf2.buffer, "should not reference the same backing buffer when allocating a buffer larger than half the backing buffer's size"); buf2 = alloc(4096); test.equal(buf2.buffer, buf1.buffer, "should reference the same backing buffer when allocating a buffer smaller or equal than half the backing buffer's size"); buf1 = alloc(4096); test.notEqual(buf1.buffer, buf2.buffer, "should not reference the same backing buffer when the slab is exhausted (initializes new slab)"); test.end(); }); apollo-server-demo/node_modules/@protobufjs/base64/ 0000755 0001750 0000144 00000000000 14067647701 022024 5 ustar andreh users apollo-server-demo/node_modules/@protobufjs/base64/index.js 0000644 0001750 0000144 00000007547 13116465255 023502 0 ustar andreh users "use strict"; /** * A minimal base64 implementation for number arrays. * @memberof util * @namespace */ var base64 = exports; /** * Calculates the byte length of a base64 encoded string. * @param {string} string Base64 encoded string * @returns {number} Byte length */ base64.length = function length(string) { var p = string.length; if (!p) return 0; var n = 0; while (--p % 4 > 1 && string.charAt(p) === "=") ++n; return Math.ceil(string.length * 3) / 4 - n; }; // Base64 encoding table var b64 = new Array(64); // Base64 decoding table var s64 = new Array(123); // 65..90, 97..122, 48..57, 43, 47 for (var i = 0; i < 64;) s64[b64[i] = i < 26 ? i + 65 : i < 52 ? i + 71 : i < 62 ? i - 4 : i - 59 | 43] = i++; /** * Encodes a buffer to a base64 encoded string. * @param {Uint8Array} buffer Source buffer * @param {number} start Source start * @param {number} end Source end * @returns {string} Base64 encoded string */ base64.encode = function encode(buffer, start, end) { var parts = null, chunk = []; var i = 0, // output index j = 0, // goto index t; // temporary while (start < end) { var b = buffer[start++]; switch (j) { case 0: chunk[i++] = b64[b >> 2]; t = (b & 3) << 4; j = 1; break; case 1: chunk[i++] = b64[t | b >> 4]; t = (b & 15) << 2; j = 2; break; case 2: chunk[i++] = b64[t | b >> 6]; chunk[i++] = b64[b & 63]; j = 0; break; } if (i > 8191) { (parts || (parts = [])).push(String.fromCharCode.apply(String, chunk)); i = 0; } } if (j) { chunk[i++] = b64[t]; chunk[i++] = 61; if (j === 1) chunk[i++] = 61; } if (parts) { if (i) parts.push(String.fromCharCode.apply(String, chunk.slice(0, i))); return parts.join(""); } return String.fromCharCode.apply(String, chunk.slice(0, i)); }; var invalidEncoding = "invalid encoding"; /** * Decodes a base64 encoded string to a buffer. * @param {string} string Source string * @param {Uint8Array} buffer Destination buffer * @param {number} offset Destination offset * @returns {number} Number of bytes written * @throws {Error} If encoding is invalid */ base64.decode = function decode(string, buffer, offset) { var start = offset; var j = 0, // goto index t; // temporary for (var i = 0; i < string.length;) { var c = string.charCodeAt(i++); if (c === 61 && j > 1) break; if ((c = s64[c]) === undefined) throw Error(invalidEncoding); switch (j) { case 0: t = c; j = 1; break; case 1: buffer[offset++] = t << 2 | (c & 48) >> 4; t = c; j = 2; break; case 2: buffer[offset++] = (t & 15) << 4 | (c & 60) >> 2; t = c; j = 3; break; case 3: buffer[offset++] = (t & 3) << 6 | c; j = 0; break; } } if (j === 1) throw Error(invalidEncoding); return offset - start; }; /** * Tests if the specified string appears to be base64 encoded. * @param {string} string String to test * @returns {boolean} `true` if probably base64 encoded, otherwise false */ base64.test = function test(string) { return /^(?:[A-Za-z0-9+/]{4})*(?:[A-Za-z0-9+/]{2}==|[A-Za-z0-9+/]{3}=)?$/.test(string); }; apollo-server-demo/node_modules/@protobufjs/base64/LICENSE 0000644 0001750 0000144 00000002741 13026320474 023022 0 ustar andreh users Copyright (c) 2016, Daniel Wirtz All rights reserved. Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met: * Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer. * Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution. * Neither the name of its author, nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission. THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. apollo-server-demo/node_modules/@protobufjs/base64/index.d.ts 0000644 0001750 0000144 00000002146 13042165351 023714 0 ustar andreh users /** * Calculates the byte length of a base64 encoded string. * @param {string} string Base64 encoded string * @returns {number} Byte length */ export function length(string: string): number; /** * Encodes a buffer to a base64 encoded string. * @param {Uint8Array} buffer Source buffer * @param {number} start Source start * @param {number} end Source end * @returns {string} Base64 encoded string */ export function encode(buffer: Uint8Array, start: number, end: number): string; /** * Decodes a base64 encoded string to a buffer. * @param {string} string Source string * @param {Uint8Array} buffer Destination buffer * @param {number} offset Destination offset * @returns {number} Number of bytes written * @throws {Error} If encoding is invalid */ export function decode(string: string, buffer: Uint8Array, offset: number): number; /** * Tests if the specified string appears to be base64 encoded. * @param {string} string String to test * @returns {boolean} `true` if it appears to be base64 encoded, otherwise false */ export function test(string: string): boolean; apollo-server-demo/node_modules/@protobufjs/base64/README.md 0000644 0001750 0000144 00000001301 13026321561 023261 0 ustar andreh users @protobufjs/base64 ================== [](https://www.npmjs.com/package/@protobufjs/base64) A minimal base64 implementation for number arrays. API --- * **base64.length(string: `string`): `number`**<br /> Calculates the byte length of a base64 encoded string. * **base64.encode(buffer: `Uint8Array`, start: `number`, end: `number`): `string`**<br /> Encodes a buffer to a base64 encoded string. * **base64.decode(string: `string`, buffer: `Uint8Array`, offset: `number`): `number`**<br /> Decodes a base64 encoded string to a buffer. **License:** [BSD 3-Clause License](https://opensource.org/licenses/BSD-3-Clause) apollo-server-demo/node_modules/@protobufjs/base64/package.json 0000644 0001750 0000144 00000001446 13116465303 024305 0 ustar andreh users { "name": "@protobufjs/base64", "description": "A minimal base64 implementation for number arrays.", "version": "1.1.2", "author": "Daniel Wirtz <dcode+protobufjs@dcode.io>", "repository": { "type": "git", "url": "https://github.com/dcodeIO/protobuf.js.git" }, "license": "BSD-3-Clause", "main": "index.js", "types": "index.d.ts", "devDependencies": { "istanbul": "^0.4.5", "tape": "^4.6.3" }, "scripts": { "test": "tape tests/*.js", "coverage": "istanbul cover node_modules/tape/bin/tape tests/*.js" } ,"_resolved": "https://registry.npmjs.org/@protobufjs/base64/-/base64-1.1.2.tgz" ,"_integrity": "sha512-AZkcAA5vnN/v4PDqKyMR5lx7hZttPDgClv83E//FMNhR2TMcLUhfRUBHCmSl0oi9zMgDDqRUJkSxO3wm85+XLg==" ,"_from": "@protobufjs/base64@1.1.2" } apollo-server-demo/node_modules/@protobufjs/base64/tests/ 0000755 0001750 0000144 00000000000 14067647701 023166 5 ustar andreh users apollo-server-demo/node_modules/@protobufjs/base64/tests/index.js 0000644 0001750 0000144 00000002533 13042005757 024625 0 ustar andreh users var tape = require("tape"); var base64 = require(".."); var strings = { "": "", "a": "YQ==", "ab": "YWI=", "abcdefg": "YWJjZGVmZw==", "abcdefgh": "YWJjZGVmZ2g=", "abcdefghi": "YWJjZGVmZ2hp" }; tape.test("base64", function(test) { Object.keys(strings).forEach(function(str) { var enc = strings[str]; test.equal(base64.test(enc), true, "should detect '" + enc + "' to be base64 encoded"); var len = base64.length(enc); test.equal(len, str.length, "should calculate '" + enc + "' as " + str.length + " bytes"); var buf = new Array(len); var len2 = base64.decode(enc, buf, 0); test.equal(len2, len, "should decode '" + enc + "' to " + len + " bytes"); test.equal(String.fromCharCode.apply(String, buf), str, "should decode '" + enc + "' to '" + str + "'"); var enc2 = base64.encode(buf, 0, buf.length); test.equal(enc2, enc, "should encode '" + str + "' to '" + enc + "'"); }); test.throws(function() { var buf = new Array(10); base64.decode("YQ!", buf, 0); }, Error, "should throw if encoding is invalid"); test.throws(function() { var buf = new Array(10); base64.decode("Y", buf, 0); }, Error, "should throw if string is truncated"); test.end(); }); apollo-server-demo/node_modules/cookie/ 0000755 0001750 0000144 00000000000 14067647700 017713 5 ustar andreh users apollo-server-demo/node_modules/cookie/index.js 0000644 0001750 0000144 00000007705 03560116604 021357 0 ustar andreh users /*! * cookie * Copyright(c) 2012-2014 Roman Shtylman * Copyright(c) 2015 Douglas Christopher Wilson * MIT Licensed */ 'use strict'; /** * Module exports. * @public */ exports.parse = parse; exports.serialize = serialize; /** * Module variables. * @private */ var decode = decodeURIComponent; var encode = encodeURIComponent; var pairSplitRegExp = /; */; /** * RegExp to match field-content in RFC 7230 sec 3.2 * * field-content = field-vchar [ 1*( SP / HTAB ) field-vchar ] * field-vchar = VCHAR / obs-text * obs-text = %x80-FF */ var fieldContentRegExp = /^[\u0009\u0020-\u007e\u0080-\u00ff]+$/; /** * Parse a cookie header. * * Parse the given cookie header string into an object * The object has the various cookies as keys(names) => values * * @param {string} str * @param {object} [options] * @return {object} * @public */ function parse(str, options) { if (typeof str !== 'string') { throw new TypeError('argument str must be a string'); } var obj = {} var opt = options || {}; var pairs = str.split(pairSplitRegExp); var dec = opt.decode || decode; for (var i = 0; i < pairs.length; i++) { var pair = pairs[i]; var eq_idx = pair.indexOf('='); // skip things that don't look like key=value if (eq_idx < 0) { continue; } var key = pair.substr(0, eq_idx).trim() var val = pair.substr(++eq_idx, pair.length).trim(); // quoted values if ('"' == val[0]) { val = val.slice(1, -1); } // only assign once if (undefined == obj[key]) { obj[key] = tryDecode(val, dec); } } return obj; } /** * Serialize data into a cookie header. * * Serialize the a name value pair into a cookie string suitable for * http headers. An optional options object specified cookie parameters. * * serialize('foo', 'bar', { httpOnly: true }) * => "foo=bar; httpOnly" * * @param {string} name * @param {string} val * @param {object} [options] * @return {string} * @public */ function serialize(name, val, options) { var opt = options || {}; var enc = opt.encode || encode; if (typeof enc !== 'function') { throw new TypeError('option encode is invalid'); } if (!fieldContentRegExp.test(name)) { throw new TypeError('argument name is invalid'); } var value = enc(val); if (value && !fieldContentRegExp.test(value)) { throw new TypeError('argument val is invalid'); } var str = name + '=' + value; if (null != opt.maxAge) { var maxAge = opt.maxAge - 0; if (isNaN(maxAge)) throw new Error('maxAge should be a Number'); str += '; Max-Age=' + Math.floor(maxAge); } if (opt.domain) { if (!fieldContentRegExp.test(opt.domain)) { throw new TypeError('option domain is invalid'); } str += '; Domain=' + opt.domain; } if (opt.path) { if (!fieldContentRegExp.test(opt.path)) { throw new TypeError('option path is invalid'); } str += '; Path=' + opt.path; } if (opt.expires) { if (typeof opt.expires.toUTCString !== 'function') { throw new TypeError('option expires is invalid'); } str += '; Expires=' + opt.expires.toUTCString(); } if (opt.httpOnly) { str += '; HttpOnly'; } if (opt.secure) { str += '; Secure'; } if (opt.sameSite) { var sameSite = typeof opt.sameSite === 'string' ? opt.sameSite.toLowerCase() : opt.sameSite; switch (sameSite) { case true: str += '; SameSite=Strict'; break; case 'lax': str += '; SameSite=Lax'; break; case 'strict': str += '; SameSite=Strict'; break; case 'none': str += '; SameSite=None'; break; default: throw new TypeError('option sameSite is invalid'); } } return str; } /** * Try decoding a string using a decoding function. * * @param {string} str * @param {function} decode * @private */ function tryDecode(str, decode) { try { return decode(str); } catch (e) { return str; } } apollo-server-demo/node_modules/cookie/LICENSE 0000644 0001750 0000144 00000002227 03560116604 020711 0 ustar andreh users (The MIT License) Copyright (c) 2012-2014 Roman Shtylman <shtylman@gmail.com> Copyright (c) 2015 Douglas Christopher Wilson <doug@somethingdoug.com> Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the 'Software'), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/cookie/HISTORY.md 0000644 0001750 0000144 00000005212 03560116604 021364 0 ustar andreh users 0.4.0 / 2019-05-15 ================== * Add `SameSite=None` support 0.3.1 / 2016-05-26 ================== * Fix `sameSite: true` to work with draft-7 clients - `true` now sends `SameSite=Strict` instead of `SameSite` 0.3.0 / 2016-05-26 ================== * Add `sameSite` option - Replaces `firstPartyOnly` option, never implemented by browsers * Improve error message when `encode` is not a function * Improve error message when `expires` is not a `Date` 0.2.4 / 2016-05-20 ================== * perf: enable strict mode * perf: use for loop in parse * perf: use string concatination for serialization 0.2.3 / 2015-10-25 ================== * Fix cookie `Max-Age` to never be a floating point number 0.2.2 / 2015-09-17 ================== * Fix regression when setting empty cookie value - Ease the new restriction, which is just basic header-level validation * Fix typo in invalid value errors 0.2.1 / 2015-09-17 ================== * Throw on invalid values provided to `serialize` - Ensures the resulting string is a valid HTTP header value 0.2.0 / 2015-08-13 ================== * Add `firstPartyOnly` option * Throw better error for invalid argument to parse * perf: hoist regular expression 0.1.5 / 2015-09-17 ================== * Fix regression when setting empty cookie value - Ease the new restriction, which is just basic header-level validation * Fix typo in invalid value errors 0.1.4 / 2015-09-17 ================== * Throw better error for invalid argument to parse * Throw on invalid values provided to `serialize` - Ensures the resulting string is a valid HTTP header value 0.1.3 / 2015-05-19 ================== * Reduce the scope of try-catch deopt * Remove argument reassignments 0.1.2 / 2014-04-16 ================== * Remove unnecessary files from npm package 0.1.1 / 2014-02-23 ================== * Fix bad parse when cookie value contained a comma * Fix support for `maxAge` of `0` 0.1.0 / 2013-05-01 ================== * Add `decode` option * Add `encode` option 0.0.6 / 2013-04-08 ================== * Ignore cookie parts missing `=` 0.0.5 / 2012-10-29 ================== * Return raw cookie value if value unescape errors 0.0.4 / 2012-06-21 ================== * Use encode/decodeURIComponent for cookie encoding/decoding - Improve server/client interoperability 0.0.3 / 2012-06-06 ================== * Only escape special characters per the cookie RFC 0.0.2 / 2012-06-01 ================== * Fix `maxAge` option to not throw error 0.0.1 / 2012-05-28 ================== * Add more tests 0.0.0 / 2012-05-28 ================== * Initial release apollo-server-demo/node_modules/cookie/README.md 0000644 0001750 0000144 00000021151 03560116604 021160 0 ustar andreh users # cookie [![NPM Version][npm-version-image]][npm-url] [![NPM Downloads][npm-downloads-image]][npm-url] [![Node.js Version][node-version-image]][node-version-url] [![Build Status][travis-image]][travis-url] [![Test Coverage][coveralls-image]][coveralls-url] Basic HTTP cookie parser and serializer for HTTP servers. ## Installation ```sh $ npm install cookie ``` ## API ```js var cookie = require('cookie'); ``` ### cookie.parse(str, options) Parse an HTTP `Cookie` header string and returning an object of all cookie name-value pairs. The `str` argument is the string representing a `Cookie` header value and `options` is an optional object containing additional parsing options. ```js var cookies = cookie.parse('foo=bar; equation=E%3Dmc%5E2'); // { foo: 'bar', equation: 'E=mc^2' } ``` #### Options `cookie.parse` accepts these properties in the options object. ##### decode Specifies a function that will be used to decode a cookie's value. Since the value of a cookie has a limited character set (and must be a simple string), this function can be used to decode a previously-encoded cookie value into a JavaScript string or other object. The default function is the global `decodeURIComponent`, which will decode any URL-encoded sequences into their byte representations. **note** if an error is thrown from this function, the original, non-decoded cookie value will be returned as the cookie's value. ### cookie.serialize(name, value, options) Serialize a cookie name-value pair into a `Set-Cookie` header string. The `name` argument is the name for the cookie, the `value` argument is the value to set the cookie to, and the `options` argument is an optional object containing additional serialization options. ```js var setCookie = cookie.serialize('foo', 'bar'); // foo=bar ``` #### Options `cookie.serialize` accepts these properties in the options object. ##### domain Specifies the value for the [`Domain` `Set-Cookie` attribute][rfc-6265-5.2.3]. By default, no domain is set, and most clients will consider the cookie to apply to only the current domain. ##### encode Specifies a function that will be used to encode a cookie's value. Since value of a cookie has a limited character set (and must be a simple string), this function can be used to encode a value into a string suited for a cookie's value. The default function is the global `encodeURIComponent`, which will encode a JavaScript string into UTF-8 byte sequences and then URL-encode any that fall outside of the cookie range. ##### expires Specifies the `Date` object to be the value for the [`Expires` `Set-Cookie` attribute][rfc-6265-5.2.1]. By default, no expiration is set, and most clients will consider this a "non-persistent cookie" and will delete it on a condition like exiting a web browser application. **note** the [cookie storage model specification][rfc-6265-5.3] states that if both `expires` and `maxAge` are set, then `maxAge` takes precedence, but it is possible not all clients by obey this, so if both are set, they should point to the same date and time. ##### httpOnly Specifies the `boolean` value for the [`HttpOnly` `Set-Cookie` attribute][rfc-6265-5.2.6]. When truthy, the `HttpOnly` attribute is set, otherwise it is not. By default, the `HttpOnly` attribute is not set. **note** be careful when setting this to `true`, as compliant clients will not allow client-side JavaScript to see the cookie in `document.cookie`. ##### maxAge Specifies the `number` (in seconds) to be the value for the [`Max-Age` `Set-Cookie` attribute][rfc-6265-5.2.2]. The given number will be converted to an integer by rounding down. By default, no maximum age is set. **note** the [cookie storage model specification][rfc-6265-5.3] states that if both `expires` and `maxAge` are set, then `maxAge` takes precedence, but it is possible not all clients by obey this, so if both are set, they should point to the same date and time. ##### path Specifies the value for the [`Path` `Set-Cookie` attribute][rfc-6265-5.2.4]. By default, the path is considered the ["default path"][rfc-6265-5.1.4]. ##### sameSite Specifies the `boolean` or `string` to be the value for the [`SameSite` `Set-Cookie` attribute][rfc-6265bis-03-4.1.2.7]. - `true` will set the `SameSite` attribute to `Strict` for strict same site enforcement. - `false` will not set the `SameSite` attribute. - `'lax'` will set the `SameSite` attribute to `Lax` for lax same site enforcement. - `'none'` will set the `SameSite` attribute to `None` for an explicit cross-site cookie. - `'strict'` will set the `SameSite` attribute to `Strict` for strict same site enforcement. More information about the different enforcement levels can be found in [the specification][rfc-6265bis-03-4.1.2.7]. **note** This is an attribute that has not yet been fully standardized, and may change in the future. This also means many clients may ignore this attribute until they understand it. ##### secure Specifies the `boolean` value for the [`Secure` `Set-Cookie` attribute][rfc-6265-5.2.5]. When truthy, the `Secure` attribute is set, otherwise it is not. By default, the `Secure` attribute is not set. **note** be careful when setting this to `true`, as compliant clients will not send the cookie back to the server in the future if the browser does not have an HTTPS connection. ## Example The following example uses this module in conjunction with the Node.js core HTTP server to prompt a user for their name and display it back on future visits. ```js var cookie = require('cookie'); var escapeHtml = require('escape-html'); var http = require('http'); var url = require('url'); function onRequest(req, res) { // Parse the query string var query = url.parse(req.url, true, true).query; if (query && query.name) { // Set a new cookie with the name res.setHeader('Set-Cookie', cookie.serialize('name', String(query.name), { httpOnly: true, maxAge: 60 * 60 * 24 * 7 // 1 week })); // Redirect back after setting cookie res.statusCode = 302; res.setHeader('Location', req.headers.referer || '/'); res.end(); return; } // Parse the cookies on the request var cookies = cookie.parse(req.headers.cookie || ''); // Get the visitor name set in the cookie var name = cookies.name; res.setHeader('Content-Type', 'text/html; charset=UTF-8'); if (name) { res.write('<p>Welcome back, <b>' + escapeHtml(name) + '</b>!</p>'); } else { res.write('<p>Hello, new visitor!</p>'); } res.write('<form method="GET">'); res.write('<input placeholder="enter your name" name="name"> <input type="submit" value="Set Name">'); res.end('</form>'); } http.createServer(onRequest).listen(3000); ``` ## Testing ```sh $ npm test ``` ## Benchmark ``` $ npm run bench > cookie@0.3.1 bench cookie > node benchmark/index.js http_parser@2.8.0 node@6.14.2 v8@5.1.281.111 uv@1.16.1 zlib@1.2.11 ares@1.10.1-DEV icu@58.2 modules@48 napi@3 openssl@1.0.2o > node benchmark/parse.js cookie.parse 6 tests completed. simple x 1,200,691 ops/sec ±1.12% (189 runs sampled) decode x 1,012,994 ops/sec ±0.97% (186 runs sampled) unquote x 1,074,174 ops/sec ±2.43% (186 runs sampled) duplicates x 438,424 ops/sec ±2.17% (184 runs sampled) 10 cookies x 147,154 ops/sec ±1.01% (186 runs sampled) 100 cookies x 14,274 ops/sec ±1.07% (187 runs sampled) ``` ## References - [RFC 6265: HTTP State Management Mechanism][rfc-6265] - [Same-site Cookies][rfc-6265bis-03-4.1.2.7] [rfc-6265bis-03-4.1.2.7]: https://tools.ietf.org/html/draft-ietf-httpbis-rfc6265bis-03#section-4.1.2.7 [rfc-6265]: https://tools.ietf.org/html/rfc6265 [rfc-6265-5.1.4]: https://tools.ietf.org/html/rfc6265#section-5.1.4 [rfc-6265-5.2.1]: https://tools.ietf.org/html/rfc6265#section-5.2.1 [rfc-6265-5.2.2]: https://tools.ietf.org/html/rfc6265#section-5.2.2 [rfc-6265-5.2.3]: https://tools.ietf.org/html/rfc6265#section-5.2.3 [rfc-6265-5.2.4]: https://tools.ietf.org/html/rfc6265#section-5.2.4 [rfc-6265-5.2.5]: https://tools.ietf.org/html/rfc6265#section-5.2.5 [rfc-6265-5.2.6]: https://tools.ietf.org/html/rfc6265#section-5.2.6 [rfc-6265-5.3]: https://tools.ietf.org/html/rfc6265#section-5.3 ## License [MIT](LICENSE) [coveralls-image]: https://badgen.net/coveralls/c/github/jshttp/cookie/master [coveralls-url]: https://coveralls.io/r/jshttp/cookie?branch=master [node-version-image]: https://badgen.net/npm/node/cookie [node-version-url]: https://nodejs.org/en/download [npm-downloads-image]: https://badgen.net/npm/dm/cookie [npm-url]: https://npmjs.org/package/cookie [npm-version-image]: https://badgen.net/npm/v/cookie [travis-image]: https://badgen.net/travis/jshttp/cookie/master [travis-url]: https://travis-ci.org/jshttp/cookie apollo-server-demo/node_modules/cookie/package.json 0000644 0001750 0000144 00000002502 03560116604 022166 0 ustar andreh users { "name": "cookie", "description": "HTTP server cookie parsing and serialization", "version": "0.4.0", "author": "Roman Shtylman <shtylman@gmail.com>", "contributors": [ "Douglas Christopher Wilson <doug@somethingdoug.com>" ], "license": "MIT", "keywords": [ "cookie", "cookies" ], "repository": "jshttp/cookie", "devDependencies": { "beautify-benchmark": "0.2.4", "benchmark": "2.1.4", "eslint": "5.16.0", "eslint-plugin-markdown": "1.0.0", "istanbul": "0.4.5", "mocha": "6.1.4" }, "files": [ "HISTORY.md", "LICENSE", "README.md", "index.js" ], "engines": { "node": ">= 0.6" }, "scripts": { "bench": "node benchmark/index.js", "lint": "eslint --plugin markdown --ext js,md .", "test": "mocha --reporter spec --bail --check-leaks test/", "test-ci": "istanbul cover node_modules/mocha/bin/_mocha --report lcovonly -- --reporter spec --check-leaks test/", "test-cov": "istanbul cover node_modules/mocha/bin/_mocha -- --reporter dot --check-leaks test/", "version": "node scripts/version-history.js && git add HISTORY.md" } ,"_resolved": "https://registry.npmjs.org/cookie/-/cookie-0.4.0.tgz" ,"_integrity": "sha512-+Hp8fLp57wnUSt0tY0tHEXh4voZRDnoIrZPqlo3DPiI4y9lwg/jqx+1Om94/W6ZaPDOUbnjOt/99w66zk+l1Xg==" ,"_from": "cookie@0.4.0" } apollo-server-demo/node_modules/finalhandler/ 0000755 0001750 0000144 00000000000 14067647700 021071 5 ustar andreh users apollo-server-demo/node_modules/finalhandler/index.js 0000644 0001750 0000144 00000014566 03560116604 022540 0 ustar andreh users /*! * finalhandler * Copyright(c) 2014-2017 Douglas Christopher Wilson * MIT Licensed */ 'use strict' /** * Module dependencies. * @private */ var debug = require('debug')('finalhandler') var encodeUrl = require('encodeurl') var escapeHtml = require('escape-html') var onFinished = require('on-finished') var parseUrl = require('parseurl') var statuses = require('statuses') var unpipe = require('unpipe') /** * Module variables. * @private */ var DOUBLE_SPACE_REGEXP = /\x20{2}/g var NEWLINE_REGEXP = /\n/g /* istanbul ignore next */ var defer = typeof setImmediate === 'function' ? setImmediate : function (fn) { process.nextTick(fn.bind.apply(fn, arguments)) } var isFinished = onFinished.isFinished /** * Create a minimal HTML document. * * @param {string} message * @private */ function createHtmlDocument (message) { var body = escapeHtml(message) .replace(NEWLINE_REGEXP, '<br>') .replace(DOUBLE_SPACE_REGEXP, ' ') return '<!DOCTYPE html>\n' + '<html lang="en">\n' + '<head>\n' + '<meta charset="utf-8">\n' + '<title>Error</title>\n' + '</head>\n' + '<body>\n' + '<pre>' + body + '</pre>\n' + '</body>\n' + '</html>\n' } /** * Module exports. * @public */ module.exports = finalhandler /** * Create a function to handle the final response. * * @param {Request} req * @param {Response} res * @param {Object} [options] * @return {Function} * @public */ function finalhandler (req, res, options) { var opts = options || {} // get environment var env = opts.env || process.env.NODE_ENV || 'development' // get error callback var onerror = opts.onerror return function (err) { var headers var msg var status // ignore 404 on in-flight response if (!err && headersSent(res)) { debug('cannot 404 after headers sent') return } // unhandled error if (err) { // respect status code from error status = getErrorStatusCode(err) if (status === undefined) { // fallback to status code on response status = getResponseStatusCode(res) } else { // respect headers from error headers = getErrorHeaders(err) } // get error message msg = getErrorMessage(err, status, env) } else { // not found status = 404 msg = 'Cannot ' + req.method + ' ' + encodeUrl(getResourceName(req)) } debug('default %s', status) // schedule onerror callback if (err && onerror) { defer(onerror, err, req, res) } // cannot actually respond if (headersSent(res)) { debug('cannot %d after headers sent', status) req.socket.destroy() return } // send response send(req, res, status, headers, msg) } } /** * Get headers from Error object. * * @param {Error} err * @return {object} * @private */ function getErrorHeaders (err) { if (!err.headers || typeof err.headers !== 'object') { return undefined } var headers = Object.create(null) var keys = Object.keys(err.headers) for (var i = 0; i < keys.length; i++) { var key = keys[i] headers[key] = err.headers[key] } return headers } /** * Get message from Error object, fallback to status message. * * @param {Error} err * @param {number} status * @param {string} env * @return {string} * @private */ function getErrorMessage (err, status, env) { var msg if (env !== 'production') { // use err.stack, which typically includes err.message msg = err.stack // fallback to err.toString() when possible if (!msg && typeof err.toString === 'function') { msg = err.toString() } } return msg || statuses[status] } /** * Get status code from Error object. * * @param {Error} err * @return {number} * @private */ function getErrorStatusCode (err) { // check err.status if (typeof err.status === 'number' && err.status >= 400 && err.status < 600) { return err.status } // check err.statusCode if (typeof err.statusCode === 'number' && err.statusCode >= 400 && err.statusCode < 600) { return err.statusCode } return undefined } /** * Get resource name for the request. * * This is typically just the original pathname of the request * but will fallback to "resource" is that cannot be determined. * * @param {IncomingMessage} req * @return {string} * @private */ function getResourceName (req) { try { return parseUrl.original(req).pathname } catch (e) { return 'resource' } } /** * Get status code from response. * * @param {OutgoingMessage} res * @return {number} * @private */ function getResponseStatusCode (res) { var status = res.statusCode // default status code to 500 if outside valid range if (typeof status !== 'number' || status < 400 || status > 599) { status = 500 } return status } /** * Determine if the response headers have been sent. * * @param {object} res * @returns {boolean} * @private */ function headersSent (res) { return typeof res.headersSent !== 'boolean' ? Boolean(res._header) : res.headersSent } /** * Send response. * * @param {IncomingMessage} req * @param {OutgoingMessage} res * @param {number} status * @param {object} headers * @param {string} message * @private */ function send (req, res, status, headers, message) { function write () { // response body var body = createHtmlDocument(message) // response status res.statusCode = status res.statusMessage = statuses[status] // response headers setHeaders(res, headers) // security headers res.setHeader('Content-Security-Policy', "default-src 'none'") res.setHeader('X-Content-Type-Options', 'nosniff') // standard headers res.setHeader('Content-Type', 'text/html; charset=utf-8') res.setHeader('Content-Length', Buffer.byteLength(body, 'utf8')) if (req.method === 'HEAD') { res.end() return } res.end(body, 'utf8') } if (isFinished(req)) { write() return } // unpipe everything from the request unpipe(req) // flush the request onFinished(req, write) req.resume() } /** * Set response headers from an object. * * @param {OutgoingMessage} res * @param {object} headers * @private */ function setHeaders (res, headers) { if (!headers) { return } var keys = Object.keys(headers) for (var i = 0; i < keys.length; i++) { var key = keys[i] res.setHeader(key, headers[key]) } } apollo-server-demo/node_modules/finalhandler/LICENSE 0000644 0001750 0000144 00000002137 03560116604 022067 0 ustar andreh users (The MIT License) Copyright (c) 2014-2017 Douglas Christopher Wilson <doug@somethingdoug.com> Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the 'Software'), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/finalhandler/HISTORY.md 0000644 0001750 0000144 00000007760 03560116604 022554 0 ustar andreh users 1.1.2 / 2019-05-09 ================== * Set stricter `Content-Security-Policy` header * deps: parseurl@~1.3.3 * deps: statuses@~1.5.0 1.1.1 / 2018-03-06 ================== * Fix 404 output for bad / missing pathnames * deps: encodeurl@~1.0.2 - Fix encoding `%` as last character * deps: statuses@~1.4.0 1.1.0 / 2017-09-24 ================== * Use `res.headersSent` when available 1.0.6 / 2017-09-22 ================== * deps: debug@2.6.9 1.0.5 / 2017-09-15 ================== * deps: parseurl@~1.3.2 - perf: reduce overhead for full URLs - perf: unroll the "fast-path" `RegExp` 1.0.4 / 2017-08-03 ================== * deps: debug@2.6.8 1.0.3 / 2017-05-16 ================== * deps: debug@2.6.7 - deps: ms@2.0.0 1.0.2 / 2017-04-22 ================== * deps: debug@2.6.4 - deps: ms@0.7.3 1.0.1 / 2017-03-21 ================== * Fix missing `</html>` in HTML document * deps: debug@2.6.3 - Fix: `DEBUG_MAX_ARRAY_LENGTH` 1.0.0 / 2017-02-15 ================== * Fix exception when `err` cannot be converted to a string * Fully URL-encode the pathname in the 404 message * Only include the pathname in the 404 message * Send complete HTML document * Set `Content-Security-Policy: default-src 'self'` header * deps: debug@2.6.1 - Allow colors in workers - Deprecated `DEBUG_FD` environment variable set to `3` or higher - Fix error when running under React Native - Use same color for same namespace - deps: ms@0.7.2 0.5.1 / 2016-11-12 ================== * Fix exception when `err.headers` is not an object * deps: statuses@~1.3.1 * perf: hoist regular expressions * perf: remove duplicate validation path 0.5.0 / 2016-06-15 ================== * Change invalid or non-numeric status code to 500 * Overwrite status message to match set status code * Prefer `err.statusCode` if `err.status` is invalid * Set response headers from `err.headers` object * Use `statuses` instead of `http` module for status messages - Includes all defined status messages 0.4.1 / 2015-12-02 ================== * deps: escape-html@~1.0.3 - perf: enable strict mode - perf: optimize string replacement - perf: use faster string coercion 0.4.0 / 2015-06-14 ================== * Fix a false-positive when unpiping in Node.js 0.8 * Support `statusCode` property on `Error` objects * Use `unpipe` module for unpiping requests * deps: escape-html@1.0.2 * deps: on-finished@~2.3.0 - Add defined behavior for HTTP `CONNECT` requests - Add defined behavior for HTTP `Upgrade` requests - deps: ee-first@1.1.1 * perf: enable strict mode * perf: remove argument reassignment 0.3.6 / 2015-05-11 ================== * deps: debug@~2.2.0 - deps: ms@0.7.1 0.3.5 / 2015-04-22 ================== * deps: on-finished@~2.2.1 - Fix `isFinished(req)` when data buffered 0.3.4 / 2015-03-15 ================== * deps: debug@~2.1.3 - Fix high intensity foreground color for bold - deps: ms@0.7.0 0.3.3 / 2015-01-01 ================== * deps: debug@~2.1.1 * deps: on-finished@~2.2.0 0.3.2 / 2014-10-22 ================== * deps: on-finished@~2.1.1 - Fix handling of pipelined requests 0.3.1 / 2014-10-16 ================== * deps: debug@~2.1.0 - Implement `DEBUG_FD` env variable support 0.3.0 / 2014-09-17 ================== * Terminate in progress response only on error * Use `on-finished` to determine request status 0.2.0 / 2014-09-03 ================== * Set `X-Content-Type-Options: nosniff` header * deps: debug@~2.0.0 0.1.0 / 2014-07-16 ================== * Respond after request fully read - prevents hung responses and socket hang ups * deps: debug@1.0.4 0.0.3 / 2014-07-11 ================== * deps: debug@1.0.3 - Add support for multiple wildcards in namespaces 0.0.2 / 2014-06-19 ================== * Handle invalid status codes 0.0.1 / 2014-06-05 ================== * deps: debug@1.0.2 0.0.0 / 2014-06-05 ================== * Extracted from connect/express apollo-server-demo/node_modules/finalhandler/README.md 0000644 0001750 0000144 00000007654 03560116604 022352 0 ustar andreh users # finalhandler [![NPM Version][npm-image]][npm-url] [![NPM Downloads][downloads-image]][downloads-url] [![Node.js Version][node-image]][node-url] [![Build Status][travis-image]][travis-url] [![Test Coverage][coveralls-image]][coveralls-url] Node.js function to invoke as the final step to respond to HTTP request. ## Installation This is a [Node.js](https://nodejs.org/en/) module available through the [npm registry](https://www.npmjs.com/). Installation is done using the [`npm install` command](https://docs.npmjs.com/getting-started/installing-npm-packages-locally): ```sh $ npm install finalhandler ``` ## API <!-- eslint-disable no-unused-vars --> ```js var finalhandler = require('finalhandler') ``` ### finalhandler(req, res, [options]) Returns function to be invoked as the final step for the given `req` and `res`. This function is to be invoked as `fn(err)`. If `err` is falsy, the handler will write out a 404 response to the `res`. If it is truthy, an error response will be written out to the `res`. When an error is written, the following information is added to the response: * The `res.statusCode` is set from `err.status` (or `err.statusCode`). If this value is outside the 4xx or 5xx range, it will be set to 500. * The `res.statusMessage` is set according to the status code. * The body will be the HTML of the status code message if `env` is `'production'`, otherwise will be `err.stack`. * Any headers specified in an `err.headers` object. The final handler will also unpipe anything from `req` when it is invoked. #### options.env By default, the environment is determined by `NODE_ENV` variable, but it can be overridden by this option. #### options.onerror Provide a function to be called with the `err` when it exists. Can be used for writing errors to a central location without excessive function generation. Called as `onerror(err, req, res)`. ## Examples ### always 404 ```js var finalhandler = require('finalhandler') var http = require('http') var server = http.createServer(function (req, res) { var done = finalhandler(req, res) done() }) server.listen(3000) ``` ### perform simple action ```js var finalhandler = require('finalhandler') var fs = require('fs') var http = require('http') var server = http.createServer(function (req, res) { var done = finalhandler(req, res) fs.readFile('index.html', function (err, buf) { if (err) return done(err) res.setHeader('Content-Type', 'text/html') res.end(buf) }) }) server.listen(3000) ``` ### use with middleware-style functions ```js var finalhandler = require('finalhandler') var http = require('http') var serveStatic = require('serve-static') var serve = serveStatic('public') var server = http.createServer(function (req, res) { var done = finalhandler(req, res) serve(req, res, done) }) server.listen(3000) ``` ### keep log of all errors ```js var finalhandler = require('finalhandler') var fs = require('fs') var http = require('http') var server = http.createServer(function (req, res) { var done = finalhandler(req, res, { onerror: logerror }) fs.readFile('index.html', function (err, buf) { if (err) return done(err) res.setHeader('Content-Type', 'text/html') res.end(buf) }) }) server.listen(3000) function logerror (err) { console.error(err.stack || err.toString()) } ``` ## License [MIT](LICENSE) [npm-image]: https://img.shields.io/npm/v/finalhandler.svg [npm-url]: https://npmjs.org/package/finalhandler [node-image]: https://img.shields.io/node/v/finalhandler.svg [node-url]: https://nodejs.org/en/download [travis-image]: https://img.shields.io/travis/pillarjs/finalhandler.svg [travis-url]: https://travis-ci.org/pillarjs/finalhandler [coveralls-image]: https://img.shields.io/coveralls/pillarjs/finalhandler.svg [coveralls-url]: https://coveralls.io/r/pillarjs/finalhandler?branch=master [downloads-image]: https://img.shields.io/npm/dm/finalhandler.svg [downloads-url]: https://npmjs.org/package/finalhandler apollo-server-demo/node_modules/finalhandler/package.json 0000644 0001750 0000144 00000003003 03560116604 023341 0 ustar andreh users { "name": "finalhandler", "description": "Node.js final http responder", "version": "1.1.2", "author": "Douglas Christopher Wilson <doug@somethingdoug.com>", "license": "MIT", "repository": "pillarjs/finalhandler", "dependencies": { "debug": "2.6.9", "encodeurl": "~1.0.2", "escape-html": "~1.0.3", "on-finished": "~2.3.0", "parseurl": "~1.3.3", "statuses": "~1.5.0", "unpipe": "~1.0.0" }, "devDependencies": { "eslint": "5.16.0", "eslint-config-standard": "12.0.0", "eslint-plugin-import": "2.17.2", "eslint-plugin-markdown": "1.0.0", "eslint-plugin-node": "8.0.1", "eslint-plugin-promise": "4.1.1", "eslint-plugin-standard": "4.0.0", "istanbul": "0.4.5", "mocha": "6.1.4", "readable-stream": "2.3.6", "safe-buffer": "5.1.2", "supertest": "4.0.2" }, "files": [ "LICENSE", "HISTORY.md", "index.js" ], "engines": { "node": ">= 0.8" }, "scripts": { "lint": "eslint --plugin markdown --ext js,md .", "test": "mocha --reporter spec --bail --check-leaks test/", "test-ci": "istanbul cover node_modules/mocha/bin/_mocha --report lcovonly -- --reporter spec --check-leaks test/", "test-cov": "istanbul cover node_modules/mocha/bin/_mocha -- --reporter dot --check-leaks test/" } ,"_resolved": "https://registry.npmjs.org/finalhandler/-/finalhandler-1.1.2.tgz" ,"_integrity": "sha512-aAWcW57uxVNrQZqFXjITpW3sIUQmHGG3qSb9mUah9MgMC4NeWhNOlNjXEYq3HjRAvL6arUviZGGJsBg6z0zsWA==" ,"_from": "finalhandler@1.1.2" } apollo-server-demo/node_modules/inherits/ 0000755 0001750 0000144 00000000000 14067647700 020267 5 ustar andreh users apollo-server-demo/node_modules/inherits/LICENSE 0000644 0001750 0000144 00000001355 12204513634 021265 0 ustar andreh users The ISC License Copyright (c) Isaac Z. Schlueter Permission to use, copy, modify, and/or distribute this software for any purpose with or without fee is hereby granted, provided that the above copyright notice and this permission notice appear in all copies. THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE. apollo-server-demo/node_modules/inherits/README.md 0000644 0001750 0000144 00000003131 12145166046 021536 0 ustar andreh users Browser-friendly inheritance fully compatible with standard node.js [inherits](http://nodejs.org/api/util.html#util_util_inherits_constructor_superconstructor). This package exports standard `inherits` from node.js `util` module in node environment, but also provides alternative browser-friendly implementation through [browser field](https://gist.github.com/shtylman/4339901). Alternative implementation is a literal copy of standard one located in standalone module to avoid requiring of `util`. It also has a shim for old browsers with no `Object.create` support. While keeping you sure you are using standard `inherits` implementation in node.js environment, it allows bundlers such as [browserify](https://github.com/substack/node-browserify) to not include full `util` package to your client code if all you need is just `inherits` function. It worth, because browser shim for `util` package is large and `inherits` is often the single function you need from it. It's recommended to use this package instead of `require('util').inherits` for any code that has chances to be used not only in node.js but in browser too. ## usage ```js var inherits = require('inherits'); // then use exactly as the standard one ``` ## note on version ~1.0 Version ~1.0 had completely different motivation and is not compatible neither with 2.0 nor with standard node.js `inherits`. If you are using version ~1.0 and planning to switch to ~2.0, be careful: * new version uses `super_` instead of `super` for referencing superclass * new version overwrites current prototype while old one preserves any existing fields on it apollo-server-demo/node_modules/inherits/package.json 0000644 0001750 0000144 00000001341 12764132631 022546 0 ustar andreh users { "name": "inherits", "description": "Browser-friendly inheritance fully compatible with standard node.js inherits()", "version": "2.0.3", "keywords": [ "inheritance", "class", "klass", "oop", "object-oriented", "inherits", "browser", "browserify" ], "main": "./inherits.js", "browser": "./inherits_browser.js", "repository": "git://github.com/isaacs/inherits", "license": "ISC", "scripts": { "test": "node test" }, "devDependencies": { "tap": "^7.1.0" }, "files": [ "inherits.js", "inherits_browser.js" ] ,"_resolved": "https://registry.npmjs.org/inherits/-/inherits-2.0.3.tgz" ,"_integrity": "sha1-Yzwsg+PaQqUC9SRmAiSA9CCCYd4=" ,"_from": "inherits@2.0.3" } apollo-server-demo/node_modules/inherits/inherits_browser.js 0000644 0001750 0000144 00000001240 12145167676 024216 0 ustar andreh users if (typeof Object.create === 'function') { // implementation from standard node.js 'util' module module.exports = function inherits(ctor, superCtor) { ctor.super_ = superCtor ctor.prototype = Object.create(superCtor.prototype, { constructor: { value: ctor, enumerable: false, writable: true, configurable: true } }); }; } else { // old school shim for old browsers module.exports = function inherits(ctor, superCtor) { ctor.super_ = superCtor var TempCtor = function () {} TempCtor.prototype = superCtor.prototype ctor.prototype = new TempCtor() ctor.prototype.constructor = ctor } } apollo-server-demo/node_modules/inherits/inherits.js 0000644 0001750 0000144 00000000300 12764074241 022437 0 ustar andreh users try { var util = require('util'); if (typeof util.inherits !== 'function') throw ''; module.exports = util.inherits; } catch (e) { module.exports = require('./inherits_browser.js'); } apollo-server-demo/node_modules/yallist/ 0000755 0001750 0000144 00000000000 14067647700 020123 5 ustar andreh users apollo-server-demo/node_modules/yallist/iterator.js 0000644 0001750 0000144 00000000317 03560116604 022301 0 ustar andreh users 'use strict' module.exports = function (Yallist) { Yallist.prototype[Symbol.iterator] = function* () { for (let walker = this.head; walker; walker = walker.next) { yield walker.value } } } apollo-server-demo/node_modules/yallist/yallist.js 0000644 0001750 0000144 00000020333 03560116604 022131 0 ustar andreh users 'use strict' module.exports = Yallist Yallist.Node = Node Yallist.create = Yallist function Yallist (list) { var self = this if (!(self instanceof Yallist)) { self = new Yallist() } self.tail = null self.head = null self.length = 0 if (list && typeof list.forEach === 'function') { list.forEach(function (item) { self.push(item) }) } else if (arguments.length > 0) { for (var i = 0, l = arguments.length; i < l; i++) { self.push(arguments[i]) } } return self } Yallist.prototype.removeNode = function (node) { if (node.list !== this) { throw new Error('removing node which does not belong to this list') } var next = node.next var prev = node.prev if (next) { next.prev = prev } if (prev) { prev.next = next } if (node === this.head) { this.head = next } if (node === this.tail) { this.tail = prev } node.list.length-- node.next = null node.prev = null node.list = null return next } Yallist.prototype.unshiftNode = function (node) { if (node === this.head) { return } if (node.list) { node.list.removeNode(node) } var head = this.head node.list = this node.next = head if (head) { head.prev = node } this.head = node if (!this.tail) { this.tail = node } this.length++ } Yallist.prototype.pushNode = function (node) { if (node === this.tail) { return } if (node.list) { node.list.removeNode(node) } var tail = this.tail node.list = this node.prev = tail if (tail) { tail.next = node } this.tail = node if (!this.head) { this.head = node } this.length++ } Yallist.prototype.push = function () { for (var i = 0, l = arguments.length; i < l; i++) { push(this, arguments[i]) } return this.length } Yallist.prototype.unshift = function () { for (var i = 0, l = arguments.length; i < l; i++) { unshift(this, arguments[i]) } return this.length } Yallist.prototype.pop = function () { if (!this.tail) { return undefined } var res = this.tail.value this.tail = this.tail.prev if (this.tail) { this.tail.next = null } else { this.head = null } this.length-- return res } Yallist.prototype.shift = function () { if (!this.head) { return undefined } var res = this.head.value this.head = this.head.next if (this.head) { this.head.prev = null } else { this.tail = null } this.length-- return res } Yallist.prototype.forEach = function (fn, thisp) { thisp = thisp || this for (var walker = this.head, i = 0; walker !== null; i++) { fn.call(thisp, walker.value, i, this) walker = walker.next } } Yallist.prototype.forEachReverse = function (fn, thisp) { thisp = thisp || this for (var walker = this.tail, i = this.length - 1; walker !== null; i--) { fn.call(thisp, walker.value, i, this) walker = walker.prev } } Yallist.prototype.get = function (n) { for (var i = 0, walker = this.head; walker !== null && i < n; i++) { // abort out of the list early if we hit a cycle walker = walker.next } if (i === n && walker !== null) { return walker.value } } Yallist.prototype.getReverse = function (n) { for (var i = 0, walker = this.tail; walker !== null && i < n; i++) { // abort out of the list early if we hit a cycle walker = walker.prev } if (i === n && walker !== null) { return walker.value } } Yallist.prototype.map = function (fn, thisp) { thisp = thisp || this var res = new Yallist() for (var walker = this.head; walker !== null;) { res.push(fn.call(thisp, walker.value, this)) walker = walker.next } return res } Yallist.prototype.mapReverse = function (fn, thisp) { thisp = thisp || this var res = new Yallist() for (var walker = this.tail; walker !== null;) { res.push(fn.call(thisp, walker.value, this)) walker = walker.prev } return res } Yallist.prototype.reduce = function (fn, initial) { var acc var walker = this.head if (arguments.length > 1) { acc = initial } else if (this.head) { walker = this.head.next acc = this.head.value } else { throw new TypeError('Reduce of empty list with no initial value') } for (var i = 0; walker !== null; i++) { acc = fn(acc, walker.value, i) walker = walker.next } return acc } Yallist.prototype.reduceReverse = function (fn, initial) { var acc var walker = this.tail if (arguments.length > 1) { acc = initial } else if (this.tail) { walker = this.tail.prev acc = this.tail.value } else { throw new TypeError('Reduce of empty list with no initial value') } for (var i = this.length - 1; walker !== null; i--) { acc = fn(acc, walker.value, i) walker = walker.prev } return acc } Yallist.prototype.toArray = function () { var arr = new Array(this.length) for (var i = 0, walker = this.head; walker !== null; i++) { arr[i] = walker.value walker = walker.next } return arr } Yallist.prototype.toArrayReverse = function () { var arr = new Array(this.length) for (var i = 0, walker = this.tail; walker !== null; i++) { arr[i] = walker.value walker = walker.prev } return arr } Yallist.prototype.slice = function (from, to) { to = to || this.length if (to < 0) { to += this.length } from = from || 0 if (from < 0) { from += this.length } var ret = new Yallist() if (to < from || to < 0) { return ret } if (from < 0) { from = 0 } if (to > this.length) { to = this.length } for (var i = 0, walker = this.head; walker !== null && i < from; i++) { walker = walker.next } for (; walker !== null && i < to; i++, walker = walker.next) { ret.push(walker.value) } return ret } Yallist.prototype.sliceReverse = function (from, to) { to = to || this.length if (to < 0) { to += this.length } from = from || 0 if (from < 0) { from += this.length } var ret = new Yallist() if (to < from || to < 0) { return ret } if (from < 0) { from = 0 } if (to > this.length) { to = this.length } for (var i = this.length, walker = this.tail; walker !== null && i > to; i--) { walker = walker.prev } for (; walker !== null && i > from; i--, walker = walker.prev) { ret.push(walker.value) } return ret } Yallist.prototype.splice = function (start, deleteCount, ...nodes) { if (start > this.length) { start = this.length - 1 } if (start < 0) { start = this.length + start; } for (var i = 0, walker = this.head; walker !== null && i < start; i++) { walker = walker.next } var ret = [] for (var i = 0; walker && i < deleteCount; i++) { ret.push(walker.value) walker = this.removeNode(walker) } if (walker === null) { walker = this.tail } if (walker !== this.head && walker !== this.tail) { walker = walker.prev } for (var i = 0; i < nodes.length; i++) { walker = insert(this, walker, nodes[i]) } return ret; } Yallist.prototype.reverse = function () { var head = this.head var tail = this.tail for (var walker = head; walker !== null; walker = walker.prev) { var p = walker.prev walker.prev = walker.next walker.next = p } this.head = tail this.tail = head return this } function insert (self, node, value) { var inserted = node === self.head ? new Node(value, null, node, self) : new Node(value, node, node.next, self) if (inserted.next === null) { self.tail = inserted } if (inserted.prev === null) { self.head = inserted } self.length++ return inserted } function push (self, item) { self.tail = new Node(item, self.tail, null, self) if (!self.head) { self.head = self.tail } self.length++ } function unshift (self, item) { self.head = new Node(item, null, self.head, self) if (!self.tail) { self.tail = self.head } self.length++ } function Node (value, prev, next, list) { if (!(this instanceof Node)) { return new Node(value, prev, next, list) } this.list = list this.value = value if (prev) { prev.next = this this.prev = prev } else { this.prev = null } if (next) { next.prev = this this.next = next } else { this.next = null } } try { // add if support for Symbol.iterator is present require('./iterator.js')(Yallist) } catch (er) {} apollo-server-demo/node_modules/yallist/LICENSE 0000644 0001750 0000144 00000001375 03560116604 021124 0 ustar andreh users The ISC License Copyright (c) Isaac Z. Schlueter and Contributors Permission to use, copy, modify, and/or distribute this software for any purpose with or without fee is hereby granted, provided that the above copyright notice and this permission notice appear in all copies. THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE. apollo-server-demo/node_modules/yallist/README.md 0000644 0001750 0000144 00000011155 03560116604 021373 0 ustar andreh users # yallist Yet Another Linked List There are many doubly-linked list implementations like it, but this one is mine. For when an array would be too big, and a Map can't be iterated in reverse order. [](https://travis-ci.org/isaacs/yallist) [](https://coveralls.io/github/isaacs/yallist) ## basic usage ```javascript var yallist = require('yallist') var myList = yallist.create([1, 2, 3]) myList.push('foo') myList.unshift('bar') // of course pop() and shift() are there, too console.log(myList.toArray()) // ['bar', 1, 2, 3, 'foo'] myList.forEach(function (k) { // walk the list head to tail }) myList.forEachReverse(function (k, index, list) { // walk the list tail to head }) var myDoubledList = myList.map(function (k) { return k + k }) // now myDoubledList contains ['barbar', 2, 4, 6, 'foofoo'] // mapReverse is also a thing var myDoubledListReverse = myList.mapReverse(function (k) { return k + k }) // ['foofoo', 6, 4, 2, 'barbar'] var reduced = myList.reduce(function (set, entry) { set += entry return set }, 'start') console.log(reduced) // 'startfoo123bar' ``` ## api The whole API is considered "public". Functions with the same name as an Array method work more or less the same way. There's reverse versions of most things because that's the point. ### Yallist Default export, the class that holds and manages a list. Call it with either a forEach-able (like an array) or a set of arguments, to initialize the list. The Array-ish methods all act like you'd expect. No magic length, though, so if you change that it won't automatically prune or add empty spots. ### Yallist.create(..) Alias for Yallist function. Some people like factories. #### yallist.head The first node in the list #### yallist.tail The last node in the list #### yallist.length The number of nodes in the list. (Change this at your peril. It is not magic like Array length.) #### yallist.toArray() Convert the list to an array. #### yallist.forEach(fn, [thisp]) Call a function on each item in the list. #### yallist.forEachReverse(fn, [thisp]) Call a function on each item in the list, in reverse order. #### yallist.get(n) Get the data at position `n` in the list. If you use this a lot, probably better off just using an Array. #### yallist.getReverse(n) Get the data at position `n`, counting from the tail. #### yallist.map(fn, thisp) Create a new Yallist with the result of calling the function on each item. #### yallist.mapReverse(fn, thisp) Same as `map`, but in reverse. #### yallist.pop() Get the data from the list tail, and remove the tail from the list. #### yallist.push(item, ...) Insert one or more items to the tail of the list. #### yallist.reduce(fn, initialValue) Like Array.reduce. #### yallist.reduceReverse Like Array.reduce, but in reverse. #### yallist.reverse Reverse the list in place. #### yallist.shift() Get the data from the list head, and remove the head from the list. #### yallist.slice([from], [to]) Just like Array.slice, but returns a new Yallist. #### yallist.sliceReverse([from], [to]) Just like yallist.slice, but the result is returned in reverse. #### yallist.toArray() Create an array representation of the list. #### yallist.toArrayReverse() Create a reversed array representation of the list. #### yallist.unshift(item, ...) Insert one or more items to the head of the list. #### yallist.unshiftNode(node) Move a Node object to the front of the list. (That is, pull it out of wherever it lives, and make it the new head.) If the node belongs to a different list, then that list will remove it first. #### yallist.pushNode(node) Move a Node object to the end of the list. (That is, pull it out of wherever it lives, and make it the new tail.) If the node belongs to a list already, then that list will remove it first. #### yallist.removeNode(node) Remove a node from the list, preserving referential integrity of head and tail and other nodes. Will throw an error if you try to have a list remove a node that doesn't belong to it. ### Yallist.Node The class that holds the data and is actually the list. Call with `var n = new Node(value, previousNode, nextNode)` Note that if you do direct operations on Nodes themselves, it's very easy to get into weird states where the list is broken. Be careful :) #### node.next The next node in the list. #### node.prev The previous node in the list. #### node.value The data the node contains. #### node.list The list to which this node belongs. (Null if it does not belong to any list.) apollo-server-demo/node_modules/yallist/package.json 0000644 0001750 0000144 00000001536 03560116604 022404 0 ustar andreh users { "name": "yallist", "version": "4.0.0", "description": "Yet Another Linked List", "main": "yallist.js", "directories": { "test": "test" }, "files": [ "yallist.js", "iterator.js" ], "dependencies": {}, "devDependencies": { "tap": "^12.1.0" }, "scripts": { "test": "tap test/*.js --100", "preversion": "npm test", "postversion": "npm publish", "postpublish": "git push origin --all; git push origin --tags" }, "repository": { "type": "git", "url": "git+https://github.com/isaacs/yallist.git" }, "author": "Isaac Z. Schlueter <i@izs.me> (http://blog.izs.me/)", "license": "ISC" ,"_resolved": "https://registry.npmjs.org/yallist/-/yallist-4.0.0.tgz" ,"_integrity": "sha512-3wdGidZyq5PB084XLES5TpOSRA3wjXAlIWMhum2kRcv/41Sn2emQ0dycQW4uZXLejwKvg6EsvbdlVL+FYEct7A==" ,"_from": "yallist@4.0.0" } apollo-server-demo/node_modules/content-type/ 0000755 0001750 0000144 00000000000 14067647700 021073 5 ustar andreh users apollo-server-demo/node_modules/content-type/index.js 0000644 0001750 0000144 00000011311 13155600302 022515 0 ustar andreh users /*! * content-type * Copyright(c) 2015 Douglas Christopher Wilson * MIT Licensed */ 'use strict' /** * RegExp to match *( ";" parameter ) in RFC 7231 sec 3.1.1.1 * * parameter = token "=" ( token / quoted-string ) * token = 1*tchar * tchar = "!" / "#" / "$" / "%" / "&" / "'" / "*" * / "+" / "-" / "." / "^" / "_" / "`" / "|" / "~" * / DIGIT / ALPHA * ; any VCHAR, except delimiters * quoted-string = DQUOTE *( qdtext / quoted-pair ) DQUOTE * qdtext = HTAB / SP / %x21 / %x23-5B / %x5D-7E / obs-text * obs-text = %x80-FF * quoted-pair = "\" ( HTAB / SP / VCHAR / obs-text ) */ var PARAM_REGEXP = /; *([!#$%&'*+.^_`|~0-9A-Za-z-]+) *= *("(?:[\u000b\u0020\u0021\u0023-\u005b\u005d-\u007e\u0080-\u00ff]|\\[\u000b\u0020-\u00ff])*"|[!#$%&'*+.^_`|~0-9A-Za-z-]+) */g var TEXT_REGEXP = /^[\u000b\u0020-\u007e\u0080-\u00ff]+$/ var TOKEN_REGEXP = /^[!#$%&'*+.^_`|~0-9A-Za-z-]+$/ /** * RegExp to match quoted-pair in RFC 7230 sec 3.2.6 * * quoted-pair = "\" ( HTAB / SP / VCHAR / obs-text ) * obs-text = %x80-FF */ var QESC_REGEXP = /\\([\u000b\u0020-\u00ff])/g /** * RegExp to match chars that must be quoted-pair in RFC 7230 sec 3.2.6 */ var QUOTE_REGEXP = /([\\"])/g /** * RegExp to match type in RFC 7231 sec 3.1.1.1 * * media-type = type "/" subtype * type = token * subtype = token */ var TYPE_REGEXP = /^[!#$%&'*+.^_`|~0-9A-Za-z-]+\/[!#$%&'*+.^_`|~0-9A-Za-z-]+$/ /** * Module exports. * @public */ exports.format = format exports.parse = parse /** * Format object to media type. * * @param {object} obj * @return {string} * @public */ function format (obj) { if (!obj || typeof obj !== 'object') { throw new TypeError('argument obj is required') } var parameters = obj.parameters var type = obj.type if (!type || !TYPE_REGEXP.test(type)) { throw new TypeError('invalid type') } var string = type // append parameters if (parameters && typeof parameters === 'object') { var param var params = Object.keys(parameters).sort() for (var i = 0; i < params.length; i++) { param = params[i] if (!TOKEN_REGEXP.test(param)) { throw new TypeError('invalid parameter name') } string += '; ' + param + '=' + qstring(parameters[param]) } } return string } /** * Parse media type to object. * * @param {string|object} string * @return {Object} * @public */ function parse (string) { if (!string) { throw new TypeError('argument string is required') } // support req/res-like objects as argument var header = typeof string === 'object' ? getcontenttype(string) : string if (typeof header !== 'string') { throw new TypeError('argument string is required to be a string') } var index = header.indexOf(';') var type = index !== -1 ? header.substr(0, index).trim() : header.trim() if (!TYPE_REGEXP.test(type)) { throw new TypeError('invalid media type') } var obj = new ContentType(type.toLowerCase()) // parse parameters if (index !== -1) { var key var match var value PARAM_REGEXP.lastIndex = index while ((match = PARAM_REGEXP.exec(header))) { if (match.index !== index) { throw new TypeError('invalid parameter format') } index += match[0].length key = match[1].toLowerCase() value = match[2] if (value[0] === '"') { // remove quotes and escapes value = value .substr(1, value.length - 2) .replace(QESC_REGEXP, '$1') } obj.parameters[key] = value } if (index !== header.length) { throw new TypeError('invalid parameter format') } } return obj } /** * Get content-type from req/res objects. * * @param {object} * @return {Object} * @private */ function getcontenttype (obj) { var header if (typeof obj.getHeader === 'function') { // res-like header = obj.getHeader('content-type') } else if (typeof obj.headers === 'object') { // req-like header = obj.headers && obj.headers['content-type'] } if (typeof header !== 'string') { throw new TypeError('content-type header is missing from object') } return header } /** * Quote a string if necessary. * * @param {string} val * @return {string} * @private */ function qstring (val) { var str = String(val) // no need to quote tokens if (TOKEN_REGEXP.test(str)) { return str } if (str.length > 0 && !TEXT_REGEXP.test(str)) { throw new TypeError('invalid parameter value') } return '"' + str.replace(QUOTE_REGEXP, '\\$1') + '"' } /** * Class to represent a content type. * @private */ function ContentType (type) { this.parameters = Object.create(null) this.type = type } apollo-server-demo/node_modules/content-type/LICENSE 0000644 0001750 0000144 00000002101 13155540217 022062 0 ustar andreh users (The MIT License) Copyright (c) 2015 Douglas Christopher Wilson Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the 'Software'), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/content-type/HISTORY.md 0000644 0001750 0000144 00000000664 13155600517 022554 0 ustar andreh users 1.0.4 / 2017-09-11 ================== * perf: skip parameter parsing when no parameters 1.0.3 / 2017-09-10 ================== * perf: remove argument reassignment 1.0.2 / 2016-05-09 ================== * perf: enable strict mode 1.0.1 / 2015-02-13 ================== * Improve missing `Content-Type` header error message 1.0.0 / 2015-02-01 ================== * Initial implementation, derived from `media-typer@0.3.0` apollo-server-demo/node_modules/content-type/README.md 0000644 0001750 0000144 00000005354 13155540217 022351 0 ustar andreh users # content-type [![NPM Version][npm-image]][npm-url] [![NPM Downloads][downloads-image]][downloads-url] [![Node.js Version][node-version-image]][node-version-url] [![Build Status][travis-image]][travis-url] [![Test Coverage][coveralls-image]][coveralls-url] Create and parse HTTP Content-Type header according to RFC 7231 ## Installation ```sh $ npm install content-type ``` ## API ```js var contentType = require('content-type') ``` ### contentType.parse(string) ```js var obj = contentType.parse('image/svg+xml; charset=utf-8') ``` Parse a content type string. This will return an object with the following properties (examples are shown for the string `'image/svg+xml; charset=utf-8'`): - `type`: The media type (the type and subtype, always lower case). Example: `'image/svg+xml'` - `parameters`: An object of the parameters in the media type (name of parameter always lower case). Example: `{charset: 'utf-8'}` Throws a `TypeError` if the string is missing or invalid. ### contentType.parse(req) ```js var obj = contentType.parse(req) ``` Parse the `content-type` header from the given `req`. Short-cut for `contentType.parse(req.headers['content-type'])`. Throws a `TypeError` if the `Content-Type` header is missing or invalid. ### contentType.parse(res) ```js var obj = contentType.parse(res) ``` Parse the `content-type` header set on the given `res`. Short-cut for `contentType.parse(res.getHeader('content-type'))`. Throws a `TypeError` if the `Content-Type` header is missing or invalid. ### contentType.format(obj) ```js var str = contentType.format({type: 'image/svg+xml'}) ``` Format an object into a content type string. This will return a string of the content type for the given object with the following properties (examples are shown that produce the string `'image/svg+xml; charset=utf-8'`): - `type`: The media type (will be lower-cased). Example: `'image/svg+xml'` - `parameters`: An object of the parameters in the media type (name of the parameter will be lower-cased). Example: `{charset: 'utf-8'}` Throws a `TypeError` if the object contains an invalid type or parameter names. ## License [MIT](LICENSE) [npm-image]: https://img.shields.io/npm/v/content-type.svg [npm-url]: https://npmjs.org/package/content-type [node-version-image]: https://img.shields.io/node/v/content-type.svg [node-version-url]: http://nodejs.org/download/ [travis-image]: https://img.shields.io/travis/jshttp/content-type/master.svg [travis-url]: https://travis-ci.org/jshttp/content-type [coveralls-image]: https://img.shields.io/coveralls/jshttp/content-type/master.svg [coveralls-url]: https://coveralls.io/r/jshttp/content-type [downloads-image]: https://img.shields.io/npm/dm/content-type.svg [downloads-url]: https://npmjs.org/package/content-type apollo-server-demo/node_modules/content-type/package.json 0000644 0001750 0000144 00000002417 13155600337 023355 0 ustar andreh users { "name": "content-type", "description": "Create and parse HTTP Content-Type header", "version": "1.0.4", "author": "Douglas Christopher Wilson <doug@somethingdoug.com>", "license": "MIT", "keywords": [ "content-type", "http", "req", "res", "rfc7231" ], "repository": "jshttp/content-type", "devDependencies": { "eslint": "3.19.0", "eslint-config-standard": "10.2.1", "eslint-plugin-import": "2.7.0", "eslint-plugin-node": "5.1.1", "eslint-plugin-promise": "3.5.0", "eslint-plugin-standard": "3.0.1", "istanbul": "0.4.5", "mocha": "~1.21.5" }, "files": [ "LICENSE", "HISTORY.md", "README.md", "index.js" ], "engines": { "node": ">= 0.6" }, "scripts": { "lint": "eslint .", "test": "mocha --reporter spec --check-leaks --bail test/", "test-ci": "istanbul cover node_modules/mocha/bin/_mocha --report lcovonly -- --reporter spec --check-leaks test/", "test-cov": "istanbul cover node_modules/mocha/bin/_mocha -- --reporter dot --check-leaks test/" } ,"_resolved": "https://registry.npmjs.org/content-type/-/content-type-1.0.4.tgz" ,"_integrity": "sha512-hIP3EEPs8tB9AT1L+NUqtwOAps4mk2Zob89MWXMHjHWg9milF/j4osnnQLXBCBFBk/tvIG/tUc9mOUJiPBhPXA==" ,"_from": "content-type@1.0.4" } apollo-server-demo/node_modules/apollo-server-plugin-base/ 0000755 0001750 0000144 00000000000 14067647700 023440 5 ustar andreh users apollo-server-demo/node_modules/apollo-server-plugin-base/dist/ 0000755 0001750 0000144 00000000000 14067647700 024403 5 ustar andreh users apollo-server-demo/node_modules/apollo-server-plugin-base/dist/index.js 0000644 0001750 0000144 00000000156 03560116604 026040 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); //# sourceMappingURL=index.js.map apollo-server-demo/node_modules/apollo-server-plugin-base/dist/index.d.ts 0000644 0001750 0000144 00000006267 03560116604 026305 0 ustar andreh users import { AnyFunctionMap, BaseContext, GraphQLServiceContext, GraphQLRequestContext, GraphQLRequest, GraphQLResponse, ValueOrPromise, WithRequired, GraphQLFieldResolverParams, GraphQLRequestContextDidResolveSource, GraphQLRequestContextParsingDidStart, GraphQLRequestContextValidationDidStart, GraphQLRequestContextDidResolveOperation, GraphQLRequestContextDidEncounterErrors, GraphQLRequestContextResponseForOperation, GraphQLRequestContextExecutionDidStart, GraphQLRequestContextWillSendResponse } from 'apollo-server-types'; export { BaseContext, GraphQLServiceContext, GraphQLRequestContext, GraphQLRequest, GraphQLResponse, ValueOrPromise, WithRequired, GraphQLFieldResolverParams, GraphQLRequestContextDidResolveSource, GraphQLRequestContextParsingDidStart, GraphQLRequestContextValidationDidStart, GraphQLRequestContextDidResolveOperation, GraphQLRequestContextDidEncounterErrors, GraphQLRequestContextResponseForOperation, GraphQLRequestContextExecutionDidStart, GraphQLRequestContextWillSendResponse, }; export interface ApolloServerPlugin<TContext extends BaseContext = BaseContext> { serverWillStart?(service: GraphQLServiceContext): ValueOrPromise<GraphQLServerListener | void>; requestDidStart?(requestContext: GraphQLRequestContext<TContext>): GraphQLRequestListener<TContext> | void; } export interface GraphQLServerListener { serverWillStop?(): ValueOrPromise<void>; } export declare type GraphQLRequestListenerParsingDidEnd = (err?: Error) => void; export declare type GraphQLRequestListenerValidationDidEnd = ((err?: ReadonlyArray<Error>) => void); export declare type GraphQLRequestListenerExecutionDidEnd = ((err?: Error) => void); export declare type GraphQLRequestListenerDidResolveField = ((error: Error | null, result?: any) => void); export interface GraphQLRequestListener<TContext extends BaseContext = BaseContext> extends AnyFunctionMap { didResolveSource?(requestContext: GraphQLRequestContextDidResolveSource<TContext>): ValueOrPromise<void>; parsingDidStart?(requestContext: GraphQLRequestContextParsingDidStart<TContext>): GraphQLRequestListenerParsingDidEnd | void; validationDidStart?(requestContext: GraphQLRequestContextValidationDidStart<TContext>): GraphQLRequestListenerValidationDidEnd | void; didResolveOperation?(requestContext: GraphQLRequestContextDidResolveOperation<TContext>): ValueOrPromise<void>; didEncounterErrors?(requestContext: GraphQLRequestContextDidEncounterErrors<TContext>): ValueOrPromise<void>; responseForOperation?(requestContext: GraphQLRequestContextResponseForOperation<TContext>): ValueOrPromise<GraphQLResponse | null>; executionDidStart?(requestContext: GraphQLRequestContextExecutionDidStart<TContext>): GraphQLRequestExecutionListener | GraphQLRequestListenerExecutionDidEnd | void; willSendResponse?(requestContext: GraphQLRequestContextWillSendResponse<TContext>): ValueOrPromise<void>; } export interface GraphQLRequestExecutionListener<TContext extends BaseContext = BaseContext> extends AnyFunctionMap { executionDidEnd?: GraphQLRequestListenerExecutionDidEnd; willResolveField?(fieldResolverParams: GraphQLFieldResolverParams<any, TContext>): GraphQLRequestListenerDidResolveField | void; } //# sourceMappingURL=index.d.ts.map apollo-server-demo/node_modules/apollo-server-plugin-base/dist/index.d.ts.map 0000644 0001750 0000144 00000004202 03560116604 027044 0 ustar andreh users {"version":3,"file":"index.d.ts","sourceRoot":"","sources":["../src/index.ts"],"names":[],"mappings":"AAAA,OAAO,EACL,cAAc,EACd,WAAW,EACX,qBAAqB,EACrB,qBAAqB,EACrB,cAAc,EACd,eAAe,EACf,cAAc,EACd,YAAY,EACZ,0BAA0B,EAC1B,qCAAqC,EACrC,oCAAoC,EACpC,uCAAuC,EACvC,wCAAwC,EACxC,uCAAuC,EACvC,yCAAyC,EACzC,sCAAsC,EACtC,qCAAqC,EACtC,MAAM,qBAAqB,CAAC;AAU7B,OAAO,EACL,WAAW,EACX,qBAAqB,EACrB,qBAAqB,EACrB,cAAc,EACd,eAAe,EACf,cAAc,EACd,YAAY,EACZ,0BAA0B,EAC1B,qCAAqC,EACrC,oCAAoC,EACpC,uCAAuC,EACvC,wCAAwC,EACxC,uCAAuC,EACvC,yCAAyC,EACzC,sCAAsC,EACtC,qCAAqC,GACtC,CAAC;AAUF,MAAM,WAAW,kBAAkB,CACjC,QAAQ,SAAS,WAAW,GAAG,WAAW;IAE1C,eAAe,CAAC,CACd,OAAO,EAAE,qBAAqB,GAC7B,cAAc,CAAC,qBAAqB,GAAG,IAAI,CAAC,CAAC;IAChD,eAAe,CAAC,CACd,cAAc,EAAE,qBAAqB,CAAC,QAAQ,CAAC,GAC9C,sBAAsB,CAAC,QAAQ,CAAC,GAAG,IAAI,CAAC;CAC5C;AAED,MAAM,WAAW,qBAAqB;IACpC,cAAc,CAAC,IAAI,cAAc,CAAC,IAAI,CAAC,CAAC;CACzC;AAED,oBAAY,mCAAmC,GAAG,CAAC,GAAG,CAAC,EAAE,KAAK,KAAK,IAAI,CAAC;AACxE,oBAAY,sCAAsC,GAChD,CAAC,CAAC,GAAG,CAAC,EAAE,aAAa,CAAC,KAAK,CAAC,KAAK,IAAI,CAAC,CAAC;AACzC,oBAAY,qCAAqC,GAAG,CAAC,CAAC,GAAG,CAAC,EAAE,KAAK,KAAK,IAAI,CAAC,CAAC;AAC5E,oBAAY,qCAAqC,GAC/C,CAAC,CAAC,KAAK,EAAE,KAAK,GAAG,IAAI,EAAE,MAAM,CAAC,EAAE,GAAG,KAAK,IAAI,CAAC,CAAC;AAEhD,MAAM,WAAW,sBAAsB,CACrC,QAAQ,SAAS,WAAW,GAAG,WAAW,CAC1C,SAAQ,cAAc;IACtB,gBAAgB,CAAC,CACf,cAAc,EAAE,qCAAqC,CAAC,QAAQ,CAAC,GAC9D,cAAc,CAAC,IAAI,CAAC,CAAC;IACxB,eAAe,CAAC,CACd,cAAc,EAAE,oCAAoC,CAAC,QAAQ,CAAC,GAC7D,mCAAmC,GAAG,IAAI,CAAC;IAC9C,kBAAkB,CAAC,CACjB,cAAc,EAAE,uCAAuC,CAAC,QAAQ,CAAC,GAChE,sCAAsC,GAAG,IAAI,CAAC;IACjD,mBAAmB,CAAC,CAClB,cAAc,EAAE,wCAAwC,CAAC,QAAQ,CAAC,GACjE,cAAc,CAAC,IAAI,CAAC,CAAC;IACxB,kBAAkB,CAAC,CACjB,cAAc,EAAE,uCAAuC,CAAC,QAAQ,CAAC,GAChE,cAAc,CAAC,IAAI,CAAC,CAAC;IAMxB,oBAAoB,CAAC,CACnB,cAAc,EAAE,yCAAyC,CAAC,QAAQ,CAAC,GAClE,cAAc,CAAC,eAAe,GAAG,IAAI,CAAC,CAAC;IAC1C,iBAAiB,CAAC,CAChB,cAAc,EAAE,sCAAsC,CAAC,QAAQ,CAAC,GAE9D,+BAA+B,GAC/B,qCAAqC,GACrC,IAAI,CAAC;IACT,gBAAgB,CAAC,CACf,cAAc,EAAE,qCAAqC,CAAC,QAAQ,CAAC,GAC9D,cAAc,CAAC,IAAI,CAAC,CAAC;CACzB;AAED,MAAM,WAAW,+BAA+B,CAC9C,QAAQ,SAAS,WAAW,GAAG,WAAW,CAC1C,SAAQ,cAAc;IACtB,eAAe,CAAC,EAAE,qCAAqC,CAAC;IACxD,gBAAgB,CAAC,CACf,mBAAmB,EAAE,0BAA0B,CAAC,GAAG,EAAE,QAAQ,CAAC,GAC7D,qCAAqC,GAAG,IAAI,CAAC;CACjD"} apollo-server-demo/node_modules/apollo-server-plugin-base/dist/index.js.map 0000644 0001750 0000144 00000000146 03560116604 026613 0 ustar andreh users {"version":3,"file":"index.js","sourceRoot":"","sources":["../src/index.ts"],"names":[],"mappings":""} apollo-server-demo/node_modules/apollo-server-plugin-base/LICENSE 0000644 0001750 0000144 00000002154 03560116604 024435 0 ustar andreh users The MIT License (MIT) Copyright (c) 2016-2020 Apollo Graph, Inc. (Formerly Meteor Development Group, Inc.) Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/apollo-server-plugin-base/src/ 0000755 0001750 0000144 00000000000 14067647700 024227 5 ustar andreh users apollo-server-demo/node_modules/apollo-server-plugin-base/src/index.ts 0000644 0001750 0000144 00000011126 03560116604 025675 0 ustar andreh users import { AnyFunctionMap, BaseContext, GraphQLServiceContext, GraphQLRequestContext, GraphQLRequest, GraphQLResponse, ValueOrPromise, WithRequired, GraphQLFieldResolverParams, GraphQLRequestContextDidResolveSource, GraphQLRequestContextParsingDidStart, GraphQLRequestContextValidationDidStart, GraphQLRequestContextDidResolveOperation, GraphQLRequestContextDidEncounterErrors, GraphQLRequestContextResponseForOperation, GraphQLRequestContextExecutionDidStart, GraphQLRequestContextWillSendResponse, } from 'apollo-server-types'; // We re-export all of these so plugin authors only need to depend on a single // package. The overall concept of `apollo-server-types` and this package // is that they not depend directly on "core", in order to avoid close // coupling of plugin support with server versions. They are duplicated // concepts right now where one package is intended to be for public plugin // exposure, while the other (`-types`) is meant to be used internally. // In the future, `apollo-server-types` and `apollo-server-plugin-base` will // probably roll into the same "types" package, but that is not today! export { BaseContext, GraphQLServiceContext, GraphQLRequestContext, GraphQLRequest, GraphQLResponse, ValueOrPromise, WithRequired, GraphQLFieldResolverParams, GraphQLRequestContextDidResolveSource, GraphQLRequestContextParsingDidStart, GraphQLRequestContextValidationDidStart, GraphQLRequestContextDidResolveOperation, GraphQLRequestContextDidEncounterErrors, GraphQLRequestContextResponseForOperation, GraphQLRequestContextExecutionDidStart, GraphQLRequestContextWillSendResponse, }; // Typings Note! (Fix in AS3?) // // There are a number of types in this module which are specifying `void` as // their return type, despite the fact that we _are_ observing the value. // It's possible those should instead be `undefined`. For more details, see // the issue that was logged as a result of this discovery during (unrelated) PR // review: https://github.com/apollographql/apollo-server/issues/4103 export interface ApolloServerPlugin< TContext extends BaseContext = BaseContext > { serverWillStart?( service: GraphQLServiceContext, ): ValueOrPromise<GraphQLServerListener | void>; requestDidStart?( requestContext: GraphQLRequestContext<TContext>, ): GraphQLRequestListener<TContext> | void; } export interface GraphQLServerListener { serverWillStop?(): ValueOrPromise<void>; } export type GraphQLRequestListenerParsingDidEnd = (err?: Error) => void; export type GraphQLRequestListenerValidationDidEnd = ((err?: ReadonlyArray<Error>) => void); export type GraphQLRequestListenerExecutionDidEnd = ((err?: Error) => void); export type GraphQLRequestListenerDidResolveField = ((error: Error | null, result?: any) => void); export interface GraphQLRequestListener< TContext extends BaseContext = BaseContext > extends AnyFunctionMap { didResolveSource?( requestContext: GraphQLRequestContextDidResolveSource<TContext>, ): ValueOrPromise<void>; parsingDidStart?( requestContext: GraphQLRequestContextParsingDidStart<TContext>, ): GraphQLRequestListenerParsingDidEnd | void; validationDidStart?( requestContext: GraphQLRequestContextValidationDidStart<TContext>, ): GraphQLRequestListenerValidationDidEnd | void; didResolveOperation?( requestContext: GraphQLRequestContextDidResolveOperation<TContext>, ): ValueOrPromise<void>; didEncounterErrors?( requestContext: GraphQLRequestContextDidEncounterErrors<TContext>, ): ValueOrPromise<void>; // If this hook is defined, it is invoked immediately before GraphQL execution // would take place. If its return value resolves to a non-null // GraphQLResponse, that result is used instead of executing the query. // Hooks from different plugins are invoked in series and the first non-null // response is used. responseForOperation?( requestContext: GraphQLRequestContextResponseForOperation<TContext>, ): ValueOrPromise<GraphQLResponse | null>; executionDidStart?( requestContext: GraphQLRequestContextExecutionDidStart<TContext>, ): | GraphQLRequestExecutionListener | GraphQLRequestListenerExecutionDidEnd | void; willSendResponse?( requestContext: GraphQLRequestContextWillSendResponse<TContext>, ): ValueOrPromise<void>; } export interface GraphQLRequestExecutionListener< TContext extends BaseContext = BaseContext > extends AnyFunctionMap { executionDidEnd?: GraphQLRequestListenerExecutionDidEnd; willResolveField?( fieldResolverParams: GraphQLFieldResolverParams<any, TContext> ): GraphQLRequestListenerDidResolveField | void; } apollo-server-demo/node_modules/apollo-server-plugin-base/README.md 0000644 0001750 0000144 00000000036 03560116604 024704 0 ustar andreh users # `apollo-server-plugin-base` apollo-server-demo/node_modules/apollo-server-plugin-base/package.json 0000644 0001750 0000144 00000001410 03560116604 025710 0 ustar andreh users { "name": "apollo-server-plugin-base", "version": "0.10.4", "description": "Apollo Server plugin base classes", "main": "dist/index.js", "types": "dist/index.d.ts", "keywords": [], "author": "Apollo <opensource@apollographql.com>", "license": "MIT", "engines": { "node": ">=6" }, "dependencies": { "apollo-server-types": "^0.6.3" }, "peerDependencies": { "graphql": "^0.12.0 || ^0.13.0 || ^14.0.0 || ^15.0.0" }, "gitHead": "c212627be591cd2c2469321b58b15f1b7909778d" ,"_resolved": "https://registry.npmjs.org/apollo-server-plugin-base/-/apollo-server-plugin-base-0.10.4.tgz" ,"_integrity": "sha512-HRhbyHgHFTLP0ImubQObYhSgpmVH4Rk1BinnceZmwudIVLKrqayIVOELdyext/QnSmmzg5W7vF3NLGBcVGMqDg==" ,"_from": "apollo-server-plugin-base@0.10.4" } apollo-server-demo/node_modules/apollo-server-plugin-base/CHANGELOG.md 0000644 0001750 0000144 00000000031 03560116604 025231 0 ustar andreh users # Change Log ### vNEXT apollo-server-demo/node_modules/graphql-extensions/ 0000755 0001750 0000144 00000000000 14067647700 022275 5 ustar andreh users apollo-server-demo/node_modules/graphql-extensions/dist/ 0000755 0001750 0000144 00000000000 14067647700 023240 5 ustar andreh users apollo-server-demo/node_modules/graphql-extensions/dist/index.js 0000644 0001750 0000144 00000014060 03560116604 024674 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); exports.enableGraphQLExtensions = exports.GraphQLExtensionStack = exports.GraphQLExtension = void 0; const graphql_1 = require("graphql"); class GraphQLExtension { } exports.GraphQLExtension = GraphQLExtension; class GraphQLExtensionStack { constructor(extensions) { this.extensions = extensions; } requestDidStart(o) { return this.handleDidStart(ext => ext.requestDidStart && ext.requestDidStart(o)); } parsingDidStart(o) { return this.handleDidStart(ext => ext.parsingDidStart && ext.parsingDidStart(o)); } validationDidStart() { return this.handleDidStart(ext => ext.validationDidStart && ext.validationDidStart()); } executionDidStart(o) { if (o.executionArgs.fieldResolver) { this.fieldResolver = o.executionArgs.fieldResolver; } return this.handleDidStart(ext => ext.executionDidStart && ext.executionDidStart(o)); } didEncounterErrors(errors) { this.extensions.forEach(extension => { if (extension.didEncounterErrors) { extension.didEncounterErrors(errors); } }); } willSendResponse(o) { let reference = o; [...this.extensions].reverse().forEach(extension => { if (extension.willSendResponse) { const result = extension.willSendResponse(reference); if (result) { reference = result; } } }); return reference; } willResolveField(source, args, context, info) { const handlers = this.extensions .map(extension => extension.willResolveField && extension.willResolveField(source, args, context, info)) .filter(x => x) .reverse(); return (error, result) => { for (const handler of handlers) { handler(error, result); } }; } format() { return this.extensions .map(extension => extension.format && extension.format()) .filter(x => x).reduce((extensions, [key, value]) => Object.assign(extensions, { [key]: value }), {}); } handleDidStart(startInvoker) { const endHandlers = []; this.extensions.forEach(extension => { try { const endHandler = startInvoker(extension); if (endHandler) { endHandlers.push(endHandler); } } catch (error) { console.error(error); } }); return (...errors) => { endHandlers.reverse(); for (const endHandler of endHandlers) { try { endHandler(...errors); } catch (error) { console.error(error); } } }; } } exports.GraphQLExtensionStack = GraphQLExtensionStack; function enableGraphQLExtensions(schema) { if (schema._extensionsEnabled) { return schema; } schema._extensionsEnabled = true; forEachField(schema, wrapField); return schema; } exports.enableGraphQLExtensions = enableGraphQLExtensions; function wrapField(field) { const fieldResolver = field.resolve; field.resolve = (source, args, context, info) => { const parentPath = info.path.prev; const extensionStack = context && context._extensionStack; const handler = (extensionStack && extensionStack.willResolveField(source, args, context, info)) || ((_err, _result) => { }); const resolveObject = info.parentType.resolveObject; let whenObjectResolved; if (parentPath && resolveObject) { if (!parentPath.__fields) { parentPath.__fields = {}; } parentPath.__fields[info.fieldName] = info.fieldNodes; whenObjectResolved = parentPath.__whenObjectResolved; if (!whenObjectResolved) { whenObjectResolved = Promise.resolve().then(() => { return resolveObject(source, parentPath.__fields, context, info); }); parentPath.__whenObjectResolved = whenObjectResolved; } } try { const actualFieldResolver = fieldResolver || (extensionStack && extensionStack.fieldResolver) || graphql_1.defaultFieldResolver; let result; if (whenObjectResolved) { result = whenObjectResolved.then((resolvedObject) => { return actualFieldResolver(resolvedObject, args, context, info); }); } else { result = actualFieldResolver(source, args, context, info); } whenResultIsFinished(result, handler); return result; } catch (error) { handler(error); throw error; } }; } function isPromise(x) { return x && typeof x.then === 'function'; } function whenResultIsFinished(result, callback) { if (isPromise(result)) { result.then((r) => callback(null, r), (err) => callback(err)); } else if (Array.isArray(result)) { if (result.some(isPromise)) { Promise.all(result).then((r) => callback(null, r), (err) => callback(err)); } else { callback(null, result); } } else { callback(null, result); } } function forEachField(schema, fn) { const typeMap = schema.getTypeMap(); Object.keys(typeMap).forEach(typeName => { const type = typeMap[typeName]; if (!graphql_1.getNamedType(type).name.startsWith('__') && type instanceof graphql_1.GraphQLObjectType) { const fields = type.getFields(); Object.keys(fields).forEach(fieldName => { const field = fields[fieldName]; fn(field, typeName, fieldName); }); } }); } //# sourceMappingURL=index.js.map apollo-server-demo/node_modules/graphql-extensions/dist/index.d.ts 0000644 0001750 0000144 00000006235 03560116604 025135 0 ustar andreh users import { GraphQLSchema, GraphQLField, GraphQLFieldResolver, GraphQLResolveInfo, ExecutionArgs, DocumentNode, GraphQLError } from 'graphql'; import { Request } from 'apollo-server-env'; import { GraphQLResponse, GraphQLRequestContext } from 'apollo-server-types'; export { GraphQLResponse }; export declare type EndHandler = (...errors: Array<Error>) => void; export declare class GraphQLExtension<TContext = any> { requestDidStart?(o: { request: Pick<Request, 'url' | 'method' | 'headers'>; queryString?: string; parsedQuery?: DocumentNode; operationName?: string; variables?: { [key: string]: any; }; persistedQueryHit?: boolean; persistedQueryRegister?: boolean; context: TContext; requestContext: GraphQLRequestContext<TContext>; }): EndHandler | void; parsingDidStart?(o: { queryString: string; }): EndHandler | void; validationDidStart?(): EndHandler | void; executionDidStart?(o: { executionArgs: ExecutionArgs; }): EndHandler | void; didEncounterErrors?(errors: ReadonlyArray<GraphQLError>): void; willSendResponse?(o: { graphqlResponse: GraphQLResponse; context: TContext; }): void | { graphqlResponse: GraphQLResponse; context: TContext; }; willResolveField?(source: any, args: { [argName: string]: any; }, context: TContext, info: GraphQLResolveInfo): ((error: Error | null, result?: any) => void) | void; format?(): [string, any] | undefined; } export declare class GraphQLExtensionStack<TContext = any> { fieldResolver?: GraphQLFieldResolver<any, any>; private extensions; constructor(extensions: GraphQLExtension<TContext>[]); requestDidStart(o: { request: Pick<Request, 'url' | 'method' | 'headers'>; queryString?: string; parsedQuery?: DocumentNode; operationName?: string; variables?: { [key: string]: any; }; persistedQueryHit?: boolean; persistedQueryRegister?: boolean; context: TContext; extensions?: Record<string, any>; requestContext: GraphQLRequestContext<TContext>; }): EndHandler; parsingDidStart(o: { queryString: string; }): EndHandler; validationDidStart(): EndHandler; executionDidStart(o: { executionArgs: ExecutionArgs; }): EndHandler; didEncounterErrors(errors: ReadonlyArray<GraphQLError>): void; willSendResponse(o: { graphqlResponse: GraphQLResponse; context: TContext; }): { graphqlResponse: GraphQLResponse; context: TContext; }; willResolveField(source: any, args: { [argName: string]: any; }, context: TContext, info: GraphQLResolveInfo): (error: Error | null, result?: any) => void; format(): {}; private handleDidStart; } export declare function enableGraphQLExtensions(schema: GraphQLSchema & { _extensionsEnabled?: boolean; }): GraphQLSchema & { _extensionsEnabled?: boolean | undefined; }; export declare type FieldIteratorFn = (fieldDef: GraphQLField<any, any>, typeName: string, fieldName: string) => void; //# sourceMappingURL=index.d.ts.map apollo-server-demo/node_modules/graphql-extensions/dist/index.d.ts.map 0000644 0001750 0000144 00000005700 03560116604 025705 0 ustar andreh users {"version":3,"file":"index.d.ts","sourceRoot":"","sources":["../src/index.ts"],"names":[],"mappings":"AAAA,OAAO,EACL,aAAa,EAGb,YAAY,EAEZ,oBAAoB,EACpB,kBAAkB,EAClB,aAAa,EACb,YAAY,EAGZ,YAAY,EACb,MAAM,SAAS,CAAC;AAEjB,OAAO,EAAE,OAAO,EAAE,MAAM,mBAAmB,CAAC;AAE5C,OAAO,EAAE,eAAe,EAAE,qBAAqB,EAAE,MAAM,qBAAqB,CAAC;AAC7E,OAAO,EAAE,eAAe,EAAE,CAAC;AAI3B,oBAAY,UAAU,GAAG,CAAC,GAAG,MAAM,EAAE,KAAK,CAAC,KAAK,CAAC,KAAK,IAAI,CAAC;AAQ3D,qBAAa,gBAAgB,CAAC,QAAQ,GAAG,GAAG;IACnC,eAAe,CAAC,CAAC,CAAC,EAAE;QACzB,OAAO,EAAE,IAAI,CAAC,OAAO,EAAE,KAAK,GAAG,QAAQ,GAAG,SAAS,CAAC,CAAC;QACrD,WAAW,CAAC,EAAE,MAAM,CAAC;QACrB,WAAW,CAAC,EAAE,YAAY,CAAC;QAC3B,aAAa,CAAC,EAAE,MAAM,CAAC;QACvB,SAAS,CAAC,EAAE;YAAE,CAAC,GAAG,EAAE,MAAM,GAAG,GAAG,CAAA;SAAE,CAAC;QACnC,iBAAiB,CAAC,EAAE,OAAO,CAAC;QAC5B,sBAAsB,CAAC,EAAE,OAAO,CAAC;QACjC,OAAO,EAAE,QAAQ,CAAC;QAClB,cAAc,EAAE,qBAAqB,CAAC,QAAQ,CAAC,CAAC;KACjD,GAAG,UAAU,GAAG,IAAI;IACd,eAAe,CAAC,CAAC,CAAC,EAAE;QAAE,WAAW,EAAE,MAAM,CAAA;KAAE,GAAG,UAAU,GAAG,IAAI;IAC/D,kBAAkB,CAAC,IAAI,UAAU,GAAG,IAAI;IACxC,iBAAiB,CAAC,CAAC,CAAC,EAAE;QAC3B,aAAa,EAAE,aAAa,CAAC;KAC9B,GAAG,UAAU,GAAG,IAAI;IAEd,kBAAkB,CAAC,CAAC,MAAM,EAAE,aAAa,CAAC,YAAY,CAAC,GAAG,IAAI;IAE9D,gBAAgB,CAAC,CAAC,CAAC,EAAE;QAC1B,eAAe,EAAE,eAAe,CAAC;QACjC,OAAO,EAAE,QAAQ,CAAC;KACnB,GAAG,IAAI,GAAG;QAAE,eAAe,EAAE,eAAe,CAAC;QAAC,OAAO,EAAE,QAAQ,CAAA;KAAE;IAE3D,gBAAgB,CAAC,CACtB,MAAM,EAAE,GAAG,EACX,IAAI,EAAE;QAAE,CAAC,OAAO,EAAE,MAAM,GAAG,GAAG,CAAA;KAAE,EAChC,OAAO,EAAE,QAAQ,EACjB,IAAI,EAAE,kBAAkB,GACvB,CAAC,CAAC,KAAK,EAAE,KAAK,GAAG,IAAI,EAAE,MAAM,CAAC,EAAE,GAAG,KAAK,IAAI,CAAC,GAAG,IAAI;IAEhD,MAAM,CAAC,IAAI,CAAC,MAAM,EAAE,GAAG,CAAC,GAAG,SAAS;CAC5C;AAED,qBAAa,qBAAqB,CAAC,QAAQ,GAAG,GAAG;IACxC,aAAa,CAAC,EAAE,oBAAoB,CAAC,GAAG,EAAE,GAAG,CAAC,CAAC;IAEtD,OAAO,CAAC,UAAU,CAA+B;gBAErC,UAAU,EAAE,gBAAgB,CAAC,QAAQ,CAAC,EAAE;IAI7C,eAAe,CAAC,CAAC,EAAE;QACxB,OAAO,EAAE,IAAI,CAAC,OAAO,EAAE,KAAK,GAAG,QAAQ,GAAG,SAAS,CAAC,CAAC;QACrD,WAAW,CAAC,EAAE,MAAM,CAAC;QACrB,WAAW,CAAC,EAAE,YAAY,CAAC;QAC3B,aAAa,CAAC,EAAE,MAAM,CAAC;QACvB,SAAS,CAAC,EAAE;YAAE,CAAC,GAAG,EAAE,MAAM,GAAG,GAAG,CAAA;SAAE,CAAC;QACnC,iBAAiB,CAAC,EAAE,OAAO,CAAC;QAC5B,sBAAsB,CAAC,EAAE,OAAO,CAAC;QACjC,OAAO,EAAE,QAAQ,CAAC;QAClB,UAAU,CAAC,EAAE,MAAM,CAAC,MAAM,EAAE,GAAG,CAAC,CAAC;QACjC,cAAc,EAAE,qBAAqB,CAAC,QAAQ,CAAC,CAAC;KACjD,GAAG,UAAU;IAKP,eAAe,CAAC,CAAC,EAAE;QAAE,WAAW,EAAE,MAAM,CAAA;KAAE,GAAG,UAAU;IAKvD,kBAAkB,IAAI,UAAU;IAKhC,iBAAiB,CAAC,CAAC,EAAE;QAAE,aAAa,EAAE,aAAa,CAAA;KAAE,GAAG,UAAU;IASlE,kBAAkB,CAAC,MAAM,EAAE,aAAa,CAAC,YAAY,CAAC;IAQtD,gBAAgB,CAAC,CAAC,EAAE;QACzB,eAAe,EAAE,eAAe,CAAC;QACjC,OAAO,EAAE,QAAQ,CAAC;KACnB,GAAG;QAAE,eAAe,EAAE,eAAe,CAAC;QAAC,OAAO,EAAE,QAAQ,CAAA;KAAE;IAcpD,gBAAgB,CACrB,MAAM,EAAE,GAAG,EACX,IAAI,EAAE;QAAE,CAAC,OAAO,EAAE,MAAM,GAAG,GAAG,CAAA;KAAE,EAChC,OAAO,EAAE,QAAQ,EACjB,IAAI,EAAE,kBAAkB,WAYT,KAAK,GAAG,IAAI,WAAW,GAAG;IAOpC,MAAM;IASb,OAAO,CAAC,cAAc;CA2BvB;AAED,wBAAgB,uBAAuB,CACrC,MAAM,EAAE,aAAa,GAAG;IAAE,kBAAkB,CAAC,EAAE,OAAO,CAAA;CAAE;;EAUzD;AAkID,oBAAY,eAAe,GAAG,CAC5B,QAAQ,EAAE,YAAY,CAAC,GAAG,EAAE,GAAG,CAAC,EAChC,QAAQ,EAAE,MAAM,EAChB,SAAS,EAAE,MAAM,KACd,IAAI,CAAC"} apollo-server-demo/node_modules/graphql-extensions/dist/index.js.map 0000644 0001750 0000144 00000013205 03560116604 025450 0 ustar andreh users {"version":3,"file":"index.js","sourceRoot":"","sources":["../src/index.ts"],"names":[],"mappings":";;;AAAA,qCAaiB;AAiBjB,MAAa,gBAAgB;CAiC5B;AAjCD,4CAiCC;AAED,MAAa,qBAAqB;IAKhC,YAAY,UAAwC;QAClD,IAAI,CAAC,UAAU,GAAG,UAAU,CAAC;IAC/B,CAAC;IAEM,eAAe,CAAC,CAWtB;QACC,OAAO,IAAI,CAAC,cAAc,CACxB,GAAG,CAAC,EAAE,CAAC,GAAG,CAAC,eAAe,IAAI,GAAG,CAAC,eAAe,CAAC,CAAC,CAAC,CACrD,CAAC;IACJ,CAAC;IACM,eAAe,CAAC,CAA0B;QAC/C,OAAO,IAAI,CAAC,cAAc,CACxB,GAAG,CAAC,EAAE,CAAC,GAAG,CAAC,eAAe,IAAI,GAAG,CAAC,eAAe,CAAC,CAAC,CAAC,CACrD,CAAC;IACJ,CAAC;IACM,kBAAkB;QACvB,OAAO,IAAI,CAAC,cAAc,CACxB,GAAG,CAAC,EAAE,CAAC,GAAG,CAAC,kBAAkB,IAAI,GAAG,CAAC,kBAAkB,EAAE,CAC1D,CAAC;IACJ,CAAC;IACM,iBAAiB,CAAC,CAAmC;QAC1D,IAAI,CAAC,CAAC,aAAa,CAAC,aAAa,EAAE;YACjC,IAAI,CAAC,aAAa,GAAG,CAAC,CAAC,aAAa,CAAC,aAAa,CAAC;SACpD;QACD,OAAO,IAAI,CAAC,cAAc,CACxB,GAAG,CAAC,EAAE,CAAC,GAAG,CAAC,iBAAiB,IAAI,GAAG,CAAC,iBAAiB,CAAC,CAAC,CAAC,CACzD,CAAC;IACJ,CAAC;IAEM,kBAAkB,CAAC,MAAmC;QAC3D,IAAI,CAAC,UAAU,CAAC,OAAO,CAAC,SAAS,CAAC,EAAE;YAClC,IAAI,SAAS,CAAC,kBAAkB,EAAE;gBAChC,SAAS,CAAC,kBAAkB,CAAC,MAAM,CAAC,CAAC;aACtC;QACH,CAAC,CAAC,CAAC;IACL,CAAC;IAEM,gBAAgB,CAAC,CAGvB;QACC,IAAI,SAAS,GAAG,CAAC,CAAC;QAElB,CAAC,GAAG,IAAI,CAAC,UAAU,CAAC,CAAC,OAAO,EAAE,CAAC,OAAO,CAAC,SAAS,CAAC,EAAE;YACjD,IAAI,SAAS,CAAC,gBAAgB,EAAE;gBAC9B,MAAM,MAAM,GAAG,SAAS,CAAC,gBAAgB,CAAC,SAAS,CAAC,CAAC;gBACrD,IAAI,MAAM,EAAE;oBACV,SAAS,GAAG,MAAM,CAAC;iBACpB;aACF;QACH,CAAC,CAAC,CAAC;QACH,OAAO,SAAS,CAAC;IACnB,CAAC;IAEM,gBAAgB,CACrB,MAAW,EACX,IAAgC,EAChC,OAAiB,EACjB,IAAwB;QAExB,MAAM,QAAQ,GAAG,IAAI,CAAC,UAAU;aAC7B,GAAG,CACF,SAAS,CAAC,EAAE,CACV,SAAS,CAAC,gBAAgB;YAC1B,SAAS,CAAC,gBAAgB,CAAC,MAAM,EAAE,IAAI,EAAE,OAAO,EAAE,IAAI,CAAC,CAC1D;aACA,MAAM,CAAC,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC;aAEd,OAAO,EAAqD,CAAC;QAEhE,OAAO,CAAC,KAAmB,EAAE,MAAY,EAAE,EAAE;YAC3C,KAAK,MAAM,OAAO,IAAI,QAAQ,EAAE;gBAC9B,OAAO,CAAC,KAAK,EAAE,MAAM,CAAC,CAAC;aACxB;QACH,CAAC,CAAC;IACJ,CAAC;IAEM,MAAM;QACX,OAAQ,IAAI,CAAC,UAAU;aACpB,GAAG,CAAC,SAAS,CAAC,EAAE,CAAC,SAAS,CAAC,MAAM,IAAI,SAAS,CAAC,MAAM,EAAE,CAAC;aACxD,MAAM,CAAC,CAAC,CAAC,EAAE,CAAC,CAAC,CAAqB,CAAC,MAAM,CAC1C,CAAC,UAAU,EAAE,CAAC,GAAG,EAAE,KAAK,CAAC,EAAE,EAAE,CAAC,MAAM,CAAC,MAAM,CAAC,UAAU,EAAE,EAAE,CAAC,GAAG,CAAC,EAAE,KAAK,EAAE,CAAC,EACzE,EAAE,CACH,CAAC;IACJ,CAAC;IAEO,cAAc,CAAC,YAAiC;QACtD,MAAM,WAAW,GAAiB,EAAE,CAAC;QACrC,IAAI,CAAC,UAAU,CAAC,OAAO,CAAC,SAAS,CAAC,EAAE;YAElC,IAAI;gBACF,MAAM,UAAU,GAAG,YAAY,CAAC,SAAS,CAAC,CAAC;gBAC3C,IAAI,UAAU,EAAE;oBACd,WAAW,CAAC,IAAI,CAAC,UAAU,CAAC,CAAC;iBAC9B;aACF;YAAC,OAAO,KAAK,EAAE;gBACd,OAAO,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC;aACtB;QACH,CAAC,CAAC,CAAC;QACH,OAAO,CAAC,GAAG,MAAoB,EAAE,EAAE;YAIjC,WAAW,CAAC,OAAO,EAAE,CAAC;YACtB,KAAK,MAAM,UAAU,IAAI,WAAW,EAAE;gBACpC,IAAI;oBACF,UAAU,CAAC,GAAG,MAAM,CAAC,CAAC;iBACvB;gBAAC,OAAO,KAAK,EAAE;oBACd,OAAO,CAAC,KAAK,CAAC,KAAK,CAAC,CAAC;iBACtB;aACF;QACH,CAAC,CAAC;IACJ,CAAC;CACF;AAhID,sDAgIC;AAED,SAAgB,uBAAuB,CACrC,MAAwD;IAExD,IAAI,MAAM,CAAC,kBAAkB,EAAE;QAC7B,OAAO,MAAM,CAAC;KACf;IACD,MAAM,CAAC,kBAAkB,GAAG,IAAI,CAAC;IAEjC,YAAY,CAAC,MAAM,EAAE,SAAS,CAAC,CAAC;IAEhC,OAAO,MAAM,CAAC;AAChB,CAAC;AAXD,0DAWC;AAED,SAAS,SAAS,CAAC,KAA6B;IAC9C,MAAM,aAAa,GAAG,KAAK,CAAC,OAAO,CAAC;IAEpC,KAAK,CAAC,OAAO,GAAG,CAAC,MAAM,EAAE,IAAI,EAAE,OAAO,EAAE,IAAI,EAAE,EAAE;QAK9C,MAAM,UAAU,GAAG,IAAI,CAAC,IAAI,CAAC,IAG5B,CAAC;QAEF,MAAM,cAAc,GAAG,OAAO,IAAI,OAAO,CAAC,eAAe,CAAC;QAC1D,MAAM,OAAO,GACX,CAAC,cAAc;YACb,cAAc,CAAC,gBAAgB,CAAC,MAAM,EAAE,IAAI,EAAE,OAAO,EAAE,IAAI,CAAC,CAAC;YAC/D,CAAC,CAAC,IAAkB,EAAE,OAAa,EAAE,EAAE;YAEvC,CAAC,CAAC,CAAC;QAEL,MAAM,aAAa,GAGd,IAAI,CAAC,UAAkB,CAAC,aAAa,CAAC;QAE3C,IAAI,kBAA4C,CAAC;QAEjD,IAAI,UAAU,IAAI,aAAa,EAAE;YAC/B,IAAI,CAAC,UAAU,CAAC,QAAQ,EAAE;gBACxB,UAAU,CAAC,QAAQ,GAAG,EAAE,CAAC;aAC1B;YAED,UAAU,CAAC,QAAQ,CAAC,IAAI,CAAC,SAAS,CAAC,GAAG,IAAI,CAAC,UAAU,CAAC;YAEtD,kBAAkB,GAAG,UAAU,CAAC,oBAAoB,CAAC;YACrD,IAAI,CAAC,kBAAkB,EAAE;gBAGvB,kBAAkB,GAAG,OAAO,CAAC,OAAO,EAAE,CAAC,IAAI,CAAC,GAAG,EAAE;oBAC/C,OAAO,aAAa,CAAC,MAAM,EAAE,UAAU,CAAC,QAAS,EAAE,OAAO,EAAE,IAAI,CAAC,CAAC;gBACpE,CAAC,CAAC,CAAC;gBACH,UAAU,CAAC,oBAAoB,GAAG,kBAAkB,CAAC;aACtD;SACF;QAED,IAAI;YAQF,MAAM,mBAAmB,GACvB,aAAa;gBACb,CAAC,cAAc,IAAI,cAAc,CAAC,aAAa,CAAC;gBAChD,8BAAoB,CAAC;YAEvB,IAAI,MAAW,CAAC;YAChB,IAAI,kBAAkB,EAAE;gBACtB,MAAM,GAAG,kBAAkB,CAAC,IAAI,CAAC,CAAC,cAAmB,EAAE,EAAE;oBACvD,OAAO,mBAAmB,CAAC,cAAc,EAAE,IAAI,EAAE,OAAO,EAAE,IAAI,CAAC,CAAC;gBAClE,CAAC,CAAC,CAAC;aACJ;iBAAM;gBACL,MAAM,GAAG,mBAAmB,CAAC,MAAM,EAAE,IAAI,EAAE,OAAO,EAAE,IAAI,CAAC,CAAC;aAC3D;YAKD,oBAAoB,CAAC,MAAM,EAAE,OAAO,CAAC,CAAC;YACtC,OAAO,MAAM,CAAC;SACf;QAAC,OAAO,KAAK,EAAE;YAId,OAAO,CAAC,KAAK,CAAC,CAAC;YACf,MAAM,KAAK,CAAC;SACb;IACH,CAAC,CAAC;AACJ,CAAC;AAED,SAAS,SAAS,CAAC,CAAM;IACvB,OAAO,CAAC,IAAI,OAAO,CAAC,CAAC,IAAI,KAAK,UAAU,CAAC;AAC3C,CAAC;AAKD,SAAS,oBAAoB,CAC3B,MAAW,EACX,QAAmD;IAEnD,IAAI,SAAS,CAAC,MAAM,CAAC,EAAE;QACrB,MAAM,CAAC,IAAI,CAAC,CAAC,CAAM,EAAE,EAAE,CAAC,QAAQ,CAAC,IAAI,EAAE,CAAC,CAAC,EAAE,CAAC,GAAU,EAAE,EAAE,CAAC,QAAQ,CAAC,GAAG,CAAC,CAAC,CAAC;KAC3E;SAAM,IAAI,KAAK,CAAC,OAAO,CAAC,MAAM,CAAC,EAAE;QAChC,IAAI,MAAM,CAAC,IAAI,CAAC,SAAS,CAAC,EAAE;YAC1B,OAAO,CAAC,GAAG,CAAC,MAAM,CAAC,CAAC,IAAI,CACtB,CAAC,CAAM,EAAE,EAAE,CAAC,QAAQ,CAAC,IAAI,EAAE,CAAC,CAAC,EAC7B,CAAC,GAAU,EAAE,EAAE,CAAC,QAAQ,CAAC,GAAG,CAAC,CAC9B,CAAC;SACH;aAAM;YACL,QAAQ,CAAC,IAAI,EAAE,MAAM,CAAC,CAAC;SACxB;KACF;SAAM;QACL,QAAQ,CAAC,IAAI,EAAE,MAAM,CAAC,CAAC;KACxB;AACH,CAAC;AAED,SAAS,YAAY,CAAC,MAAqB,EAAE,EAAmB;IAC9D,MAAM,OAAO,GAAG,MAAM,CAAC,UAAU,EAAE,CAAC;IACpC,MAAM,CAAC,IAAI,CAAC,OAAO,CAAC,CAAC,OAAO,CAAC,QAAQ,CAAC,EAAE;QACtC,MAAM,IAAI,GAAG,OAAO,CAAC,QAAQ,CAAC,CAAC;QAE/B,IACE,CAAC,sBAAY,CAAC,IAAI,CAAC,CAAC,IAAI,CAAC,UAAU,CAAC,IAAI,CAAC;YACzC,IAAI,YAAY,2BAAiB,EACjC;YACA,MAAM,MAAM,GAAG,IAAI,CAAC,SAAS,EAAE,CAAC;YAChC,MAAM,CAAC,IAAI,CAAC,MAAM,CAAC,CAAC,OAAO,CAAC,SAAS,CAAC,EAAE;gBACtC,MAAM,KAAK,GAAG,MAAM,CAAC,SAAS,CAAC,CAAC;gBAChC,EAAE,CAAC,KAAK,EAAE,QAAQ,EAAE,SAAS,CAAC,CAAC;YACjC,CAAC,CAAC,CAAC;SACJ;IACH,CAAC,CAAC,CAAC;AACL,CAAC"} apollo-server-demo/node_modules/graphql-extensions/LICENSE 0000644 0001750 0000144 00000002154 03560116604 023272 0 ustar andreh users The MIT License (MIT) Copyright (c) 2016-2020 Apollo Graph, Inc. (Formerly Meteor Development Group, Inc.) Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/graphql-extensions/src/ 0000755 0001750 0000144 00000000000 14067647700 023064 5 ustar andreh users apollo-server-demo/node_modules/graphql-extensions/src/index.ts 0000644 0001750 0000144 00000024433 03560116604 024537 0 ustar andreh users import { GraphQLSchema, GraphQLObjectType, getNamedType, GraphQLField, defaultFieldResolver, GraphQLFieldResolver, GraphQLResolveInfo, ExecutionArgs, DocumentNode, ResponsePath, FieldNode, GraphQLError, } from 'graphql'; import { Request } from 'apollo-server-env'; import { GraphQLResponse, GraphQLRequestContext } from 'apollo-server-types'; export { GraphQLResponse }; import { GraphQLObjectResolver } from '@apollographql/apollo-tools'; export type EndHandler = (...errors: Array<Error>) => void; // A StartHandlerInvoker is a function that, given a specific GraphQLExtension, // finds a specific StartHandler on that extension and calls it with appropriate // arguments. type StartHandlerInvoker<TContext = any> = ( ext: GraphQLExtension<TContext>, ) => EndHandler | void; export class GraphQLExtension<TContext = any> { public requestDidStart?(o: { request: Pick<Request, 'url' | 'method' | 'headers'>; queryString?: string; parsedQuery?: DocumentNode; operationName?: string; variables?: { [key: string]: any }; persistedQueryHit?: boolean; persistedQueryRegister?: boolean; context: TContext; requestContext: GraphQLRequestContext<TContext>; }): EndHandler | void; public parsingDidStart?(o: { queryString: string }): EndHandler | void; public validationDidStart?(): EndHandler | void; public executionDidStart?(o: { executionArgs: ExecutionArgs; }): EndHandler | void; public didEncounterErrors?(errors: ReadonlyArray<GraphQLError>): void; public willSendResponse?(o: { graphqlResponse: GraphQLResponse; context: TContext; }): void | { graphqlResponse: GraphQLResponse; context: TContext }; public willResolveField?( source: any, args: { [argName: string]: any }, context: TContext, info: GraphQLResolveInfo, ): ((error: Error | null, result?: any) => void) | void; public format?(): [string, any] | undefined; } export class GraphQLExtensionStack<TContext = any> { public fieldResolver?: GraphQLFieldResolver<any, any>; private extensions: GraphQLExtension<TContext>[]; constructor(extensions: GraphQLExtension<TContext>[]) { this.extensions = extensions; } public requestDidStart(o: { request: Pick<Request, 'url' | 'method' | 'headers'>; queryString?: string; parsedQuery?: DocumentNode; operationName?: string; variables?: { [key: string]: any }; persistedQueryHit?: boolean; persistedQueryRegister?: boolean; context: TContext; extensions?: Record<string, any>; requestContext: GraphQLRequestContext<TContext>; }): EndHandler { return this.handleDidStart( ext => ext.requestDidStart && ext.requestDidStart(o), ); } public parsingDidStart(o: { queryString: string }): EndHandler { return this.handleDidStart( ext => ext.parsingDidStart && ext.parsingDidStart(o), ); } public validationDidStart(): EndHandler { return this.handleDidStart( ext => ext.validationDidStart && ext.validationDidStart(), ); } public executionDidStart(o: { executionArgs: ExecutionArgs }): EndHandler { if (o.executionArgs.fieldResolver) { this.fieldResolver = o.executionArgs.fieldResolver; } return this.handleDidStart( ext => ext.executionDidStart && ext.executionDidStart(o), ); } public didEncounterErrors(errors: ReadonlyArray<GraphQLError>) { this.extensions.forEach(extension => { if (extension.didEncounterErrors) { extension.didEncounterErrors(errors); } }); } public willSendResponse(o: { graphqlResponse: GraphQLResponse; context: TContext; }): { graphqlResponse: GraphQLResponse; context: TContext } { let reference = o; // Reverse the array, since this is functions as an end handler [...this.extensions].reverse().forEach(extension => { if (extension.willSendResponse) { const result = extension.willSendResponse(reference); if (result) { reference = result; } } }); return reference; } public willResolveField( source: any, args: { [argName: string]: any }, context: TContext, info: GraphQLResolveInfo, ) { const handlers = this.extensions .map( extension => extension.willResolveField && extension.willResolveField(source, args, context, info), ) .filter(x => x) // Reverse list so that handlers "nest", like in handleDidStart. .reverse() as ((error: Error | null, result?: any) => void)[]; return (error: Error | null, result?: any) => { for (const handler of handlers) { handler(error, result); } }; } public format() { return (this.extensions .map(extension => extension.format && extension.format()) .filter(x => x) as [string, any][]).reduce( (extensions, [key, value]) => Object.assign(extensions, { [key]: value }), {}, ); } private handleDidStart(startInvoker: StartHandlerInvoker): EndHandler { const endHandlers: EndHandler[] = []; this.extensions.forEach(extension => { // Invoke the start handler, which may return an end handler. try { const endHandler = startInvoker(extension); if (endHandler) { endHandlers.push(endHandler); } } catch (error) { console.error(error); } }); return (...errors: Array<Error>) => { // We run end handlers in reverse order of start handlers. That way, the // first handler in the stack "surrounds" the entire event's process // (helpful for tracing/reporting!) endHandlers.reverse(); for (const endHandler of endHandlers) { try { endHandler(...errors); } catch (error) { console.error(error); } } }; } } export function enableGraphQLExtensions( schema: GraphQLSchema & { _extensionsEnabled?: boolean }, ) { if (schema._extensionsEnabled) { return schema; } schema._extensionsEnabled = true; forEachField(schema, wrapField); return schema; } function wrapField(field: GraphQLField<any, any>): void { const fieldResolver = field.resolve; field.resolve = (source, args, context, info) => { // This is a bit of a hack, but since `ResponsePath` is a linked list, // a new object gets created every time a path segment is added. // So we can use that to share our `whenObjectResolved` promise across // all field resolvers for the same object. const parentPath = info.path.prev as ResponsePath & { __fields?: Record<string, ReadonlyArray<FieldNode>>; __whenObjectResolved?: Promise<any>; }; const extensionStack = context && context._extensionStack; const handler = (extensionStack && extensionStack.willResolveField(source, args, context, info)) || ((_err: Error | null, _result?: any) => { /* do nothing */ }); const resolveObject: GraphQLObjectResolver< any, any > = (info.parentType as any).resolveObject; let whenObjectResolved: Promise<any> | undefined; if (parentPath && resolveObject) { if (!parentPath.__fields) { parentPath.__fields = {}; } parentPath.__fields[info.fieldName] = info.fieldNodes; whenObjectResolved = parentPath.__whenObjectResolved; if (!whenObjectResolved) { // Use `Promise.resolve().then()` to delay executing // `resolveObject()` so we can collect all the fields first. whenObjectResolved = Promise.resolve().then(() => { return resolveObject(source, parentPath.__fields!, context, info); }); parentPath.__whenObjectResolved = whenObjectResolved; } } try { // If no resolver has been defined for a field, use either the configured // field resolver or the default field resolver // (which matches the behavior of graphql-js when there is no explicit // resolve function defined). // XXX: Can't this be pulled up to the top of `wrapField` and only // assigned once? It seems `extensionStack.fieldResolver` isn't set // anywhere? const actualFieldResolver = fieldResolver || (extensionStack && extensionStack.fieldResolver) || defaultFieldResolver; let result: any; if (whenObjectResolved) { result = whenObjectResolved.then((resolvedObject: any) => { return actualFieldResolver(resolvedObject, args, context, info); }); } else { result = actualFieldResolver(source, args, context, info); } // Call the stack's handlers either immediately (if result is not a // Promise) or once the Promise is done. Then return that same // maybe-Promise value. whenResultIsFinished(result, handler); return result; } catch (error) { // Normally it's a bad sign to see an error both handled and // re-thrown. But it is useful to allow extensions to track errors while // still handling them in the normal GraphQL way. handler(error); throw error; } }; } function isPromise(x: any): boolean { return x && typeof x.then === 'function'; } // Given result (which may be a Promise or an array some of whose elements are // promises) Promises, set up 'callback' to be invoked when result is fully // resolved. function whenResultIsFinished( result: any, callback: (err: Error | null, result?: any) => void, ) { if (isPromise(result)) { result.then((r: any) => callback(null, r), (err: Error) => callback(err)); } else if (Array.isArray(result)) { if (result.some(isPromise)) { Promise.all(result).then( (r: any) => callback(null, r), (err: Error) => callback(err), ); } else { callback(null, result); } } else { callback(null, result); } } function forEachField(schema: GraphQLSchema, fn: FieldIteratorFn): void { const typeMap = schema.getTypeMap(); Object.keys(typeMap).forEach(typeName => { const type = typeMap[typeName]; if ( !getNamedType(type).name.startsWith('__') && type instanceof GraphQLObjectType ) { const fields = type.getFields(); Object.keys(fields).forEach(fieldName => { const field = fields[fieldName]; fn(field, typeName, fieldName); }); } }); } export type FieldIteratorFn = ( fieldDef: GraphQLField<any, any>, typeName: string, fieldName: string, ) => void; apollo-server-demo/node_modules/graphql-extensions/README.md 0000644 0001750 0000144 00000000431 03560116604 023540 0 ustar andreh users # graphql-extensions [](https://badge.fury.io/js/graphql-extensions) [](https://circleci.com/gh/apollographql/apollo-server) apollo-server-demo/node_modules/graphql-extensions/package.json 0000644 0001750 0000144 00000001663 03560116604 024557 0 ustar andreh users { "name": "graphql-extensions", "version": "0.12.8", "description": "Add extensions to GraphQL servers", "main": "./dist/index.js", "types": "./dist/index.d.ts", "repository": { "type": "git", "url": "apollographql/apollo-server", "directory": "packages/graphql-extensions" }, "author": "Apollo <opensource@apollographql.com>", "license": "MIT", "engines": { "node": ">=6.0" }, "dependencies": { "@apollographql/apollo-tools": "^0.4.3", "apollo-server-env": "^3.0.0", "apollo-server-types": "^0.6.3" }, "peerDependencies": { "graphql": "^0.12.0 || ^0.13.0 || ^14.0.0 || ^15.0.0" }, "gitHead": "c212627be591cd2c2469321b58b15f1b7909778d" ,"_resolved": "https://registry.npmjs.org/graphql-extensions/-/graphql-extensions-0.12.8.tgz" ,"_integrity": "sha512-xjsSaB6yKt9jarFNNdivl2VOx52WySYhxPgf8Y16g6GKZyAzBoIFiwyGw5PJDlOSUa6cpmzn6o7z8fVMbSAbkg==" ,"_from": "graphql-extensions@0.12.8" } apollo-server-demo/node_modules/graphql-extensions/CHANGELOG.md 0000644 0001750 0000144 00000001636 03560116604 024102 0 ustar andreh users # Changelog > This package is deprecated. Please use the [Apollo Server Plugin API](https://www.apollographql.com/docs/apollo-server/integrations/plugins/) (specified on the `plugins` property, rather than `extensions`), which provides the same functionality (and more). ### 0.1.0-beta - *Backwards-incompatible change*: `fooDidStart` handlers (where foo is `request`, `parsing`, `validation`, and `execution`) now return their end handler; the `fooDidEnd` handlers no longer exist. The end handlers now take errors. There is a new `willSendResponse` handler. The `fooDidStart` handlers take extra options (eg, the `ExecutionArgs` for `executionDidStart`). - *Backwards-incompatible change*: Previously, the `GraphQLExtensionStack` constructor took either `GraphQLExtension` objects or their constructors. Now you may only pass in `GraphQLExtension` objects. ### 0.0.10 - Fix lifecycle method invocations on extensions apollo-server-demo/node_modules/@types/ 0000755 0001750 0000144 00000000000 14067647701 017707 5 ustar andreh users apollo-server-demo/node_modules/@types/body-parser/ 0000755 0001750 0000144 00000000000 14067647700 022135 5 ustar andreh users apollo-server-demo/node_modules/@types/body-parser/LICENSE 0000644 0001750 0000144 00000002237 13620344310 023127 0 ustar andreh users MIT License Copyright (c) Microsoft Corporation. All rights reserved. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE apollo-server-demo/node_modules/@types/body-parser/index.d.ts 0000644 0001750 0000144 00000010227 13620344310 024021 0 ustar andreh users // Type definitions for body-parser 1.19 // Project: https://github.com/expressjs/body-parser // Definitions by: Santi Albo <https://github.com/santialbo> // Vilic Vane <https://github.com/vilic> // Jonathan Häberle <https://github.com/dreampulse> // Gevik Babakhani <https://github.com/blendsdk> // Tomasz Åaziuk <https://github.com/tlaziuk> // Jason Walton <https://github.com/jwalton> // Piotr BÅ‚ażejewicz <https://github.com/peterblazejewicz> // Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped /// <reference types="node" /> import { NextHandleFunction } from 'connect'; import * as http from 'http'; // for docs go to https://github.com/expressjs/body-parser/tree/1.19.0#body-parser /** @deprecated */ declare function bodyParser( options?: bodyParser.OptionsJson & bodyParser.OptionsText & bodyParser.OptionsUrlencoded, ): NextHandleFunction; declare namespace bodyParser { interface Options { /** When set to true, then deflated (compressed) bodies will be inflated; when false, deflated bodies are rejected. Defaults to true. */ inflate?: boolean; /** * Controls the maximum request body size. If this is a number, * then the value specifies the number of bytes; if it is a string, * the value is passed to the bytes library for parsing. Defaults to '100kb'. */ limit?: number | string; /** * The type option is used to determine what media type the middleware will parse */ type?: string | string[] | ((req: http.IncomingMessage) => any); /** * The verify option, if supplied, is called as verify(req, res, buf, encoding), * where buf is a Buffer of the raw request body and encoding is the encoding of the request. */ verify?(req: http.IncomingMessage, res: http.ServerResponse, buf: Buffer, encoding: string): void; } interface OptionsJson extends Options { /** * * The reviver option is passed directly to JSON.parse as the second argument. */ reviver?(key: string, value: any): any; /** * When set to `true`, will only accept arrays and objects; * when `false` will accept anything JSON.parse accepts. Defaults to `true`. */ strict?: boolean; } interface OptionsText extends Options { /** * Specify the default character set for the text content if the charset * is not specified in the Content-Type header of the request. * Defaults to `utf-8`. */ defaultCharset?: string; } interface OptionsUrlencoded extends Options { /** * The extended option allows to choose between parsing the URL-encoded data * with the querystring library (when `false`) or the qs library (when `true`). */ extended?: boolean; /** * The parameterLimit option controls the maximum number of parameters * that are allowed in the URL-encoded data. If a request contains more parameters than this value, * a 413 will be returned to the client. Defaults to 1000. */ parameterLimit?: number; } /** * Returns middleware that only parses json and only looks at requests * where the Content-Type header matches the type option. */ function json(options?: OptionsJson): NextHandleFunction; /** * Returns middleware that parses all bodies as a Buffer and only looks at requests * where the Content-Type header matches the type option. */ function raw(options?: Options): NextHandleFunction; /** * Returns middleware that parses all bodies as a string and only looks at requests * where the Content-Type header matches the type option. */ function text(options?: OptionsText): NextHandleFunction; /** * Returns middleware that only parses urlencoded bodies and only looks at requests * where the Content-Type header matches the type option */ function urlencoded(options?: OptionsUrlencoded): NextHandleFunction; } export = bodyParser; apollo-server-demo/node_modules/@types/body-parser/README.md 0000644 0001750 0000144 00000001605 13620344310 023377 0 ustar andreh users # Installation > `npm install --save @types/body-parser` # Summary This package contains type definitions for body-parser (https://github.com/expressjs/body-parser). # Details Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/body-parser. ### Additional Details * Last updated: Mon, 10 Feb 2020 21:19:04 GMT * Dependencies: [@types/connect](https://npmjs.com/package/@types/connect), [@types/node](https://npmjs.com/package/@types/node) * Global values: none # Credits These definitions were written by Santi Albo (https://github.com/santialbo), Vilic Vane (https://github.com/vilic), Jonathan Häberle (https://github.com/dreampulse), Gevik Babakhani (https://github.com/blendsdk), Tomasz Åaziuk (https://github.com/tlaziuk), Jason Walton (https://github.com/jwalton), and Piotr BÅ‚ażejewicz (https://github.com/peterblazejewicz). apollo-server-demo/node_modules/@types/body-parser/package.json 0000644 0001750 0000144 00000003576 13620344310 024417 0 ustar andreh users { "name": "@types/body-parser", "version": "1.19.0", "description": "TypeScript definitions for body-parser", "license": "MIT", "contributors": [ { "name": "Santi Albo", "url": "https://github.com/santialbo", "githubUsername": "santialbo" }, { "name": "Vilic Vane", "url": "https://github.com/vilic", "githubUsername": "vilic" }, { "name": "Jonathan Häberle", "url": "https://github.com/dreampulse", "githubUsername": "dreampulse" }, { "name": "Gevik Babakhani", "url": "https://github.com/blendsdk", "githubUsername": "blendsdk" }, { "name": "Tomasz Åaziuk", "url": "https://github.com/tlaziuk", "githubUsername": "tlaziuk" }, { "name": "Jason Walton", "url": "https://github.com/jwalton", "githubUsername": "jwalton" }, { "name": "Piotr BÅ‚ażejewicz", "url": "https://github.com/peterblazejewicz", "githubUsername": "peterblazejewicz" } ], "main": "", "types": "index.d.ts", "repository": { "type": "git", "url": "https://github.com/DefinitelyTyped/DefinitelyTyped.git", "directory": "types/body-parser" }, "scripts": {}, "dependencies": { "@types/connect": "*", "@types/node": "*" }, "typesPublisherContentHash": "4257cff3580f6064eb283c690c28aa3a5347cd3cae2a2e208b8f23c61705724a", "typeScriptVersion": "2.8" ,"_resolved": "https://registry.npmjs.org/@types/body-parser/-/body-parser-1.19.0.tgz" ,"_integrity": "sha512-W98JrE0j2K78swW4ukqMleo8R7h/pFETjM2DQ90MF6XK2i4LO4W3gQ71Lt4w3bfm2EvVSyWHplECvB5sK22yFQ==" ,"_from": "@types/body-parser@1.19.0" } apollo-server-demo/node_modules/@types/express/ 0000755 0001750 0000144 00000000000 14067647700 021377 5 ustar andreh users apollo-server-demo/node_modules/@types/express/LICENSE 0000644 0001750 0000144 00000002165 13777413717 022416 0 ustar andreh users MIT License Copyright (c) Microsoft Corporation. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE apollo-server-demo/node_modules/@types/express/index.d.ts 0000644 0001750 0000144 00000011176 13777413717 023314 0 ustar andreh users // Type definitions for Express 4.17 // Project: http://expressjs.com // Definitions by: Boris Yankov <https://github.com/borisyankov> // China Medical University Hospital <https://github.com/CMUH> // Puneet Arora <https://github.com/puneetar> // Dylan Frankland <https://github.com/dfrankland> // Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped /* =================== USAGE =================== import express = require("express"); var app = express(); =============================================== */ /// <reference types="express-serve-static-core" /> /// <reference types="serve-static" /> import * as bodyParser from 'body-parser'; import * as serveStatic from 'serve-static'; import * as core from 'express-serve-static-core'; import * as qs from 'qs'; /** * Creates an Express application. The express() function is a top-level function exported by the express module. */ declare function e(): core.Express; declare namespace e { /** * This is a built-in middleware function in Express. It parses incoming requests with JSON payloads and is based on body-parser. * @since 4.16.0 */ var json: typeof bodyParser.json; /** * This is a built-in middleware function in Express. It parses incoming requests with Buffer payloads and is based on body-parser. * @since 4.17.0 */ var raw: typeof bodyParser.raw; /** * This is a built-in middleware function in Express. It parses incoming requests with text payloads and is based on body-parser. * @since 4.17.0 */ var text: typeof bodyParser.text; /** * These are the exposed prototypes. */ var application: Application; var request: Request; var response: Response; /** * This is a built-in middleware function in Express. It serves static files and is based on serve-static. */ var static: serveStatic.RequestHandlerConstructor<Response>; /** * This is a built-in middleware function in Express. It parses incoming requests with urlencoded payloads and is based on body-parser. * @since 4.16.0 */ var urlencoded: typeof bodyParser.urlencoded; /** * This is a built-in middleware function in Express. It parses incoming request query parameters. */ export function query(options: qs.IParseOptions | typeof qs.parse): Handler; export function Router(options?: RouterOptions): core.Router; interface RouterOptions { /** * Enable case sensitivity. */ caseSensitive?: boolean; /** * Preserve the req.params values from the parent router. * If the parent and the child have conflicting param names, the child’s value take precedence. * * @default false * @since 4.5.0 */ mergeParams?: boolean; /** * Enable strict routing. */ strict?: boolean; } interface Application extends core.Application {} interface CookieOptions extends core.CookieOptions {} interface Errback extends core.Errback {} interface ErrorRequestHandler< P = core.ParamsDictionary, ResBody = any, ReqBody = any, ReqQuery = core.Query, Locals extends Record<string, any> = Record<string, any> > extends core.ErrorRequestHandler<P, ResBody, ReqBody, ReqQuery, Locals> {} interface Express extends core.Express {} interface Handler extends core.Handler {} interface IRoute extends core.IRoute {} interface IRouter extends core.IRouter {} interface IRouterHandler<T> extends core.IRouterHandler<T> {} interface IRouterMatcher<T> extends core.IRouterMatcher<T> {} interface MediaType extends core.MediaType {} interface NextFunction extends core.NextFunction {} interface Request< P = core.ParamsDictionary, ResBody = any, ReqBody = any, ReqQuery = core.Query, Locals extends Record<string, any> = Record<string, any> > extends core.Request<P, ResBody, ReqBody, ReqQuery, Locals> {} interface RequestHandler< P = core.ParamsDictionary, ResBody = any, ReqBody = any, ReqQuery = core.Query, Locals extends Record<string, any> = Record<string, any> > extends core.RequestHandler<P, ResBody, ReqBody, ReqQuery, Locals> {} interface RequestParamHandler extends core.RequestParamHandler {} export interface Response<ResBody = any, Locals extends Record<string, any> = Record<string, any>> extends core.Response<ResBody, Locals> {} interface Router extends core.Router {} interface Send extends core.Send {} } export = e; apollo-server-demo/node_modules/@types/express/README.md 0000644 0001750 0000144 00000001625 13777413717 022670 0 ustar andreh users # Installation > `npm install --save @types/express` # Summary This package contains type definitions for Express (http://expressjs.com). # Details Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/express. ### Additional Details * Last updated: Tue, 12 Jan 2021 21:42:39 GMT * Dependencies: [@types/body-parser](https://npmjs.com/package/@types/body-parser), [@types/serve-static](https://npmjs.com/package/@types/serve-static), [@types/express-serve-static-core](https://npmjs.com/package/@types/express-serve-static-core), [@types/qs](https://npmjs.com/package/@types/qs) * Global values: none # Credits These definitions were written by [Boris Yankov](https://github.com/borisyankov), [China Medical University Hospital](https://github.com/CMUH), [Puneet Arora](https://github.com/puneetar), and [Dylan Frankland](https://github.com/dfrankland). apollo-server-demo/node_modules/@types/express/package.json 0000644 0001750 0000144 00000003014 13777413717 023671 0 ustar andreh users { "name": "@types/express", "version": "4.17.11", "description": "TypeScript definitions for Express", "license": "MIT", "contributors": [ { "name": "Boris Yankov", "url": "https://github.com/borisyankov", "githubUsername": "borisyankov" }, { "name": "China Medical University Hospital", "url": "https://github.com/CMUH", "githubUsername": "CMUH" }, { "name": "Puneet Arora", "url": "https://github.com/puneetar", "githubUsername": "puneetar" }, { "name": "Dylan Frankland", "url": "https://github.com/dfrankland", "githubUsername": "dfrankland" } ], "main": "", "types": "index.d.ts", "repository": { "type": "git", "url": "https://github.com/DefinitelyTyped/DefinitelyTyped.git", "directory": "types/express" }, "scripts": {}, "dependencies": { "@types/body-parser": "*", "@types/express-serve-static-core": "^4.17.18", "@types/qs": "*", "@types/serve-static": "*" }, "typesPublisherContentHash": "51b7ca65fbad2f43fbcff8d74c47db7b8f36b31f71458c1fb328511d0075ac5a", "typeScriptVersion": "3.4" ,"_resolved": "https://registry.npmjs.org/@types/express/-/express-4.17.11.tgz" ,"_integrity": "sha512-no+R6rW60JEc59977wIxreQVsIEOAYwgCqldrA/vkpCnbD7MqTefO97lmoBe4WE0F156bC4uLSP1XHDOySnChg==" ,"_from": "@types/express@4.17.11" } apollo-server-demo/node_modules/@types/cors/ 0000755 0001750 0000144 00000000000 14067647700 020654 5 ustar andreh users apollo-server-demo/node_modules/@types/cors/LICENSE 0000644 0001750 0000144 00000002165 13740242362 021655 0 ustar andreh users MIT License Copyright (c) Microsoft Corporation. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE apollo-server-demo/node_modules/@types/cors/index.d.ts 0000644 0001750 0000144 00000002476 13740242363 022557 0 ustar andreh users // Type definitions for cors 2.8 // Project: https://github.com/expressjs/cors/ // Definitions by: Alan Plum <https://github.com/pluma> // Gaurav Sharma <https://github.com/gtpan77> // Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped // TypeScript Version: 2.3 import express = require('express'); type CustomOrigin = ( requestOrigin: string | undefined, callback: (err: Error | null, allow?: boolean) => void ) => void; declare namespace e { interface CorsOptions { /** * @default '*'' */ origin?: boolean | string | RegExp | (string | RegExp)[] | CustomOrigin; /** * @default 'GET,HEAD,PUT,PATCH,POST,DELETE' */ methods?: string | string[]; allowedHeaders?: string | string[]; exposedHeaders?: string | string[]; credentials?: boolean; maxAge?: number; /** * @default false */ preflightContinue?: boolean; /** * @default 204 */ optionsSuccessStatus?: number; } type CorsOptionsDelegate = ( req: express.Request, callback: (err: Error | null, options?: CorsOptions) => void ) => void; } declare function e( options?: e.CorsOptions | e.CorsOptionsDelegate ): express.RequestHandler; export = e; apollo-server-demo/node_modules/@types/cors/README.md 0000644 0001750 0000144 00000001101 13740242362 022114 0 ustar andreh users # Installation > `npm install --save @types/cors` # Summary This package contains type definitions for cors (https://github.com/expressjs/cors/). # Details Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/cors. ### Additional Details * Last updated: Sat, 10 Oct 2020 05:21:54 GMT * Dependencies: [@types/express](https://npmjs.com/package/@types/express) * Global values: none # Credits These definitions were written by [Alan Plum](https://github.com/pluma), and [Gaurav Sharma](https://github.com/gtpan77). apollo-server-demo/node_modules/@types/cors/package.json 0000644 0001750 0000144 00000002056 13740242362 023135 0 ustar andreh users { "name": "@types/cors", "version": "2.8.8", "description": "TypeScript definitions for cors", "license": "MIT", "contributors": [ { "name": "Alan Plum", "url": "https://github.com/pluma", "githubUsername": "pluma" }, { "name": "Gaurav Sharma", "url": "https://github.com/gtpan77", "githubUsername": "gtpan77" } ], "main": "", "types": "index.d.ts", "repository": { "type": "git", "url": "https://github.com/DefinitelyTyped/DefinitelyTyped.git", "directory": "types/cors" }, "scripts": {}, "dependencies": { "@types/express": "*" }, "typesPublisherContentHash": "b24d14934ef66476c80faf7287ca41764d0c8dffa662ea161164704a1eae48e6", "typeScriptVersion": "3.2" ,"_resolved": "https://registry.npmjs.org/@types/cors/-/cors-2.8.8.tgz" ,"_integrity": "sha512-fO3gf3DxU2Trcbr75O7obVndW/X5k8rJNZkLXlQWStTHhP71PkRqjwPIEI0yMnJdg9R9OasjU+Bsr+Hr1xy/0w==" ,"_from": "@types/cors@2.8.8" } apollo-server-demo/node_modules/@types/http-assert/ 0000755 0001750 0000144 00000000000 14067647701 022165 5 ustar andreh users apollo-server-demo/node_modules/@types/http-assert/LICENSE 0000644 0001750 0000144 00000002237 13526371204 023165 0 ustar andreh users MIT License Copyright (c) Microsoft Corporation. All rights reserved. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE apollo-server-demo/node_modules/@types/http-assert/index.d.ts 0000644 0001750 0000144 00000003535 13526371204 024063 0 ustar andreh users // Type definitions for http-assert 1.5 // Project: https://github.com/jshttp/http-assert // Definitions by: jKey Lu <https://github.com/jkeylu> // Peter Squicciarini <https://github.com/stripedpajamas> // Alex Bulanov <https://github.com/sapfear> // Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped // TypeScript Version: 2.3 /** * @param status the status code * @param msg the message of the error, defaulting to node's text for that status code * @param opts custom properties to attach to the error object */ declare function assert(value: any, status?: number, msg?: string, opts?: {}): void; declare function assert(value: any, status?: number, opts?: {}): void; declare namespace assert { /** * @param status the status code * @param msg the message of the error, defaulting to node's text for that status code * @param opts custom properties to attach to the error object */ type Assert = <T>(a: T, b: T, status?: number, msg?: string, opts?: {}) => void; /** * @param status the status code * @param msg the message of the error, defaulting to node's text for that status code * @param opts custom properties to attach to the error object */ type AssertOK = (a: any, status?: number, msg?: string, opts?: {}) => void; /** * @param status the status code * @param msg the message of the error, defaulting to node's text for that status code * @param opts custom properties to attach to the error object */ type AssertEqual = (a: any, b: any, status?: number, msg?: string, opts?: {}) => void; const equal: Assert; const notEqual: Assert; const ok: AssertOK; const strictEqual: AssertEqual; const notStrictEqual: AssertEqual; const deepEqual: AssertEqual; const notDeepEqual: AssertEqual; } export = assert; apollo-server-demo/node_modules/@types/http-assert/README.md 0000644 0001750 0000144 00000001122 13526371204 023427 0 ustar andreh users # Installation > `npm install --save @types/http-assert` # Summary This package contains type definitions for http-assert (https://github.com/jshttp/http-assert). # Details Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/http-assert Additional Details * Last updated: Mon, 19 Aug 2019 00:51:16 GMT * Dependencies: none * Global values: none # Credits These definitions were written by jKey Lu <https://github.com/jkeylu>, Peter Squicciarini <https://github.com/stripedpajamas>, and Alex Bulanov <https://github.com/sapfear>. apollo-server-demo/node_modules/@types/http-assert/package.json 0000644 0001750 0000144 00000002325 13526371204 024444 0 ustar andreh users { "name": "@types/http-assert", "version": "1.5.1", "description": "TypeScript definitions for http-assert", "license": "MIT", "contributors": [ { "name": "jKey Lu", "url": "https://github.com/jkeylu", "githubUsername": "jkeylu" }, { "name": "Peter Squicciarini", "url": "https://github.com/stripedpajamas", "githubUsername": "stripedpajamas" }, { "name": "Alex Bulanov", "url": "https://github.com/sapfear", "githubUsername": "sapfear" } ], "main": "", "types": "index", "repository": { "type": "git", "url": "https://github.com/DefinitelyTyped/DefinitelyTyped.git", "directory": "types/http-assert" }, "scripts": {}, "dependencies": {}, "typesPublisherContentHash": "25e5ec6bb6a8c0e3ba83fc4a67c444744defd0d4d2707b09649fc09dbb271083", "typeScriptVersion": "2.3" ,"_resolved": "https://registry.npmjs.org/@types/http-assert/-/http-assert-1.5.1.tgz" ,"_integrity": "sha512-PGAK759pxyfXE78NbKxyfRcWYA/KwW17X290cNev/qAsn9eQIxkH4shoNBafH37wewhDG/0p1cHPbK6+SzZjWQ==" ,"_from": "@types/http-assert@1.5.1" } apollo-server-demo/node_modules/@types/cookies/ 0000755 0001750 0000144 00000000000 14067647701 021343 5 ustar andreh users apollo-server-demo/node_modules/@types/cookies/LICENSE 0000644 0001750 0000144 00000002165 13770463207 022351 0 ustar andreh users MIT License Copyright (c) Microsoft Corporation. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE apollo-server-demo/node_modules/@types/cookies/index.d.ts 0000644 0001750 0000144 00000013131 13770463207 023240 0 ustar andreh users // Type definitions for cookies 0.7 // Project: https://github.com/pillarjs/cookies // Definitions by: Wang Zishi <https://github.com/WangZishi> // jKey Lu <https://github.com/jkeylu> // BendingBender <https://github.com/BendingBender> // Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped // TypeScript Version: 2.3 /// <reference types="node" /> import { IncomingMessage, ServerResponse } from 'http'; import * as Keygrip from 'keygrip'; import * as express from 'express'; import * as connect from 'connect'; interface Cookies { secure: boolean; request: IncomingMessage; response: ServerResponse; /** * This extracts the cookie with the given name from the * Cookie header in the request. If such a cookie exists, * its value is returned. Otherwise, nothing is returned. */ get(name: string, opts?: Cookies.GetOption): string | undefined; /** * This sets the given cookie in the response and returns * the current context to allow chaining.If the value is omitted, * an outbound header with an expired date is used to delete the cookie. */ set(name: string, value?: string | null, opts?: Cookies.SetOption): this; } declare namespace Cookies { /** * for backward-compatibility */ type ICookies = Cookies; /** * for backward-compatibility */ type IOptions = SetOption; interface Option { keys?: string[] | Keygrip; secure?: boolean; } interface GetOption { signed: boolean; } interface SetOption { /** * a number representing the milliseconds from Date.now() for expiry */ maxAge?: number; /** * a Date object indicating the cookie's expiration * date (expires at the end of session by default). */ expires?: Date; /** * a string indicating the path of the cookie (/ by default). */ path?: string; /** * a string indicating the domain of the cookie (no default). */ domain?: string; /** * a boolean indicating whether the cookie is only to be sent * over HTTPS (false by default for HTTP, true by default for HTTPS). */ secure?: boolean; /** * "secureProxy" option is deprecated; use "secure" option, provide "secure" to constructor if needed */ secureProxy?: boolean; /** * a boolean indicating whether the cookie is only to be sent over HTTP(S), * and not made available to client JavaScript (true by default). */ httpOnly?: boolean; /** * a boolean or string indicating whether the cookie is a "same site" cookie (false by default). * This can be set to 'strict', 'lax', or true (which maps to 'strict'). */ sameSite?: 'strict' | 'lax' | 'none' | boolean; /** * a boolean indicating whether the cookie is to be signed (false by default). * If this is true, another cookie of the same name with the .sig suffix * appended will also be sent, with a 27-byte url-safe base64 SHA1 value * representing the hash of cookie-name=cookie-value against the first Keygrip key. * This signature key is used to detect tampering the next time a cookie is received. */ signed?: boolean; /** * a boolean indicating whether to overwrite previously set * cookies of the same name (false by default). If this is true, * all cookies set during the same request with the same * name (regardless of path or domain) are filtered out of * the Set-Cookie header when setting this cookie. */ overwrite?: boolean; } type CookieAttr = SetOption; interface Cookie { name: string; value: string; /** * "maxage" is deprecated, use "maxAge" instead */ maxage: number; maxAge: number; expires: Date; path: string; domain: string; secure: boolean; httpOnly: boolean; sameSite: boolean; overwrite: boolean; toString(): string; toHeader(): string; } } interface CookiesFunction { (request: IncomingMessage, response: ServerResponse, options?: Cookies.Option): Cookies; /** * "options" array of key strings is deprecated, provide using options {"keys": keygrip} */ (request: IncomingMessage, response: ServerResponse, options: string[]): Cookies; /** * "options" instance of Keygrip is deprecated, provide using options {"keys": keygrip} */ // tslint:disable-next-line:unified-signatures (request: IncomingMessage, response: ServerResponse, options: Keygrip): Cookies; new (request: IncomingMessage, response: ServerResponse, options?: Cookies.Option): Cookies; /** * "options" array of key strings is deprecated, provide using options {"keys": keygrip} */ new (request: IncomingMessage, response: ServerResponse, options: string[]): Cookies; /** * "options" instance of Keygrip is deprecated, provide using options {"keys": keygrip} */ // tslint:disable-next-line:unified-signatures new (request: IncomingMessage, response: ServerResponse, options: Keygrip): Cookies; Cookie: { new (name: string, value?: string, attrs?: Cookies.CookieAttr): Cookies.Cookie; }; express(keys: string[] | Keygrip): express.Handler; connect(keys: string[] | Keygrip): connect.NextHandleFunction; } declare const Cookies: CookiesFunction; export = Cookies; apollo-server-demo/node_modules/@types/cookies/README.md 0000644 0001750 0000144 00000001452 13770463207 022621 0 ustar andreh users # Installation > `npm install --save @types/cookies` # Summary This package contains type definitions for cookies (https://github.com/pillarjs/cookies). # Details Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/cookies. ### Additional Details * Last updated: Tue, 22 Dec 2020 21:35:03 GMT * Dependencies: [@types/keygrip](https://npmjs.com/package/@types/keygrip), [@types/express](https://npmjs.com/package/@types/express), [@types/connect](https://npmjs.com/package/@types/connect), [@types/node](https://npmjs.com/package/@types/node) * Global values: none # Credits These definitions were written by [Wang Zishi](https://github.com/WangZishi), [jKey Lu](https://github.com/jkeylu), and [BendingBender](https://github.com/BendingBender). apollo-server-demo/node_modules/@types/cookies/package.json 0000644 0001750 0000144 00000002472 13770463207 023633 0 ustar andreh users { "name": "@types/cookies", "version": "0.7.6", "description": "TypeScript definitions for cookies", "license": "MIT", "contributors": [ { "name": "Wang Zishi", "url": "https://github.com/WangZishi", "githubUsername": "WangZishi" }, { "name": "jKey Lu", "url": "https://github.com/jkeylu", "githubUsername": "jkeylu" }, { "name": "BendingBender", "url": "https://github.com/BendingBender", "githubUsername": "BendingBender" } ], "main": "", "types": "index.d.ts", "repository": { "type": "git", "url": "https://github.com/DefinitelyTyped/DefinitelyTyped.git", "directory": "types/cookies" }, "scripts": {}, "dependencies": { "@types/connect": "*", "@types/express": "*", "@types/keygrip": "*", "@types/node": "*" }, "typesPublisherContentHash": "09a4522e334d7a4d84019482a9f1b00d7b41792842dc6238a27404c92cde2a72", "typeScriptVersion": "3.3" ,"_resolved": "https://registry.npmjs.org/@types/cookies/-/cookies-0.7.6.tgz" ,"_integrity": "sha512-FK4U5Qyn7/Sc5ih233OuHO0qAkOpEcD/eG6584yEiLKizTFRny86qHLe/rej3HFQrkBuUjF4whFliAdODbVN/w==" ,"_from": "@types/cookies@0.7.6" } apollo-server-demo/node_modules/@types/ws/ 0000755 0001750 0000144 00000000000 14067647700 020337 5 ustar andreh users apollo-server-demo/node_modules/@types/ws/LICENSE 0000644 0001750 0000144 00000002165 13752352623 021345 0 ustar andreh users MIT License Copyright (c) Microsoft Corporation. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE apollo-server-demo/node_modules/@types/ws/index.d.ts 0000644 0001750 0000144 00000027720 13752352623 022245 0 ustar andreh users // Type definitions for ws 7.4 // Project: https://github.com/websockets/ws // Definitions by: Paul Loyd <https://github.com/loyd> // Matt Silverlock <https://github.com/elithrar> // Margus Lamp <https://github.com/mlamp> // Philippe D'Alva <https://github.com/TitaneBoy> // reduckted <https://github.com/reduckted> // teidesu <https://github.com/teidesu> // Bartosz Wojtkowiak <https://github.com/wojtkowiak> // Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped /// <reference types="node" /> import * as events from 'events'; import * as http from 'http'; import * as https from 'https'; import * as net from 'net'; import * as url from 'url'; import * as zlib from 'zlib'; import * as stream from 'stream'; import { SecureContextOptions } from 'tls'; // WebSocket socket. declare class WebSocket extends events.EventEmitter { static CONNECTING: number; static OPEN: number; static CLOSING: number; static CLOSED: number; binaryType: string; bufferedAmount: number; extensions: string; protocol: string; readyState: number; url: string; CONNECTING: number; OPEN: number; CLOSING: number; CLOSED: number; onopen: (event: WebSocket.OpenEvent) => void; onerror: (event: WebSocket.ErrorEvent) => void; onclose: (event: WebSocket.CloseEvent) => void; onmessage: (event: WebSocket.MessageEvent) => void; constructor(address: string | url.URL, options?: WebSocket.ClientOptions | http.ClientRequestArgs); constructor(address: string | url.URL, protocols?: string | string[], options?: WebSocket.ClientOptions | http.ClientRequestArgs); close(code?: number, data?: string): void; ping(data?: any, mask?: boolean, cb?: (err: Error) => void): void; pong(data?: any, mask?: boolean, cb?: (err: Error) => void): void; send(data: any, cb?: (err?: Error) => void): void; send(data: any, options: { mask?: boolean; binary?: boolean; compress?: boolean; fin?: boolean }, cb?: (err?: Error) => void): void; terminate(): void; // HTML5 WebSocket events addEventListener(method: 'message', cb: (event: { data: any; type: string; target: WebSocket }) => void, options?: WebSocket.EventListenerOptions): void; addEventListener(method: 'close', cb: (event: { wasClean: boolean; code: number; reason: string; target: WebSocket }) => void, options?: WebSocket.EventListenerOptions): void; addEventListener(method: 'error', cb: (event: { error: any, message: any, type: string, target: WebSocket }) => void, options?: WebSocket.EventListenerOptions): void; addEventListener(method: 'open', cb: (event: { target: WebSocket }) => void, options?: WebSocket.EventListenerOptions): void; addEventListener(method: string, listener: () => void, options?: WebSocket.EventListenerOptions): void; removeEventListener(method: 'message', cb?: (event: { data: any; type: string; target: WebSocket }) => void): void; removeEventListener(method: 'close', cb?: (event: { wasClean: boolean; code: number; reason: string; target: WebSocket }) => void): void; removeEventListener(method: 'error', cb?: (event: {error: any, message: any, type: string, target: WebSocket }) => void): void; removeEventListener(method: 'open', cb?: (event: { target: WebSocket }) => void): void; removeEventListener(method: string, listener?: () => void): void; // Events on(event: 'close', listener: (this: WebSocket, code: number, reason: string) => void): this; on(event: 'error', listener: (this: WebSocket, err: Error) => void): this; on(event: 'upgrade', listener: (this: WebSocket, request: http.IncomingMessage) => void): this; on(event: 'message', listener: (this: WebSocket, data: WebSocket.Data) => void): this; on(event: 'open' , listener: (this: WebSocket) => void): this; on(event: 'ping' | 'pong', listener: (this: WebSocket, data: Buffer) => void): this; on(event: 'unexpected-response', listener: (this: WebSocket, request: http.ClientRequest, response: http.IncomingMessage) => void): this; on(event: string | symbol, listener: (this: WebSocket, ...args: any[]) => void): this; addListener(event: 'close', listener: (code: number, message: string) => void): this; addListener(event: 'error', listener: (err: Error) => void): this; addListener(event: 'upgrade', listener: (request: http.IncomingMessage) => void): this; addListener(event: 'message', listener: (data: WebSocket.Data) => void): this; addListener(event: 'open' , listener: () => void): this; addListener(event: 'ping' | 'pong', listener: (data: Buffer) => void): this; addListener(event: 'unexpected-response', listener: (request: http.ClientRequest, response: http.IncomingMessage) => void): this; addListener(event: string | symbol, listener: (...args: any[]) => void): this; removeListener(event: 'close', listener: (code: number, message: string) => void): this; removeListener(event: 'error', listener: (err: Error) => void): this; removeListener(event: 'upgrade', listener: (request: http.IncomingMessage) => void): this; removeListener(event: 'message', listener: (data: WebSocket.Data) => void): this; removeListener(event: 'open' , listener: () => void): this; removeListener(event: 'ping' | 'pong', listener: (data: Buffer) => void): this; removeListener(event: 'unexpected-response', listener: (request: http.ClientRequest, response: http.IncomingMessage) => void): this; removeListener(event: string | symbol, listener: (...args: any[]) => void): this; } declare namespace WebSocket { /** * Data represents the message payload received over the WebSocket. */ type Data = string | Buffer | ArrayBuffer | Buffer[]; /** * CertMeta represents the accepted types for certificate & key data. */ type CertMeta = string | string[] | Buffer | Buffer[]; /** * VerifyClientCallbackSync is a synchronous callback used to inspect the * incoming message. The return value (boolean) of the function determines * whether or not to accept the handshake. */ type VerifyClientCallbackSync = (info: { origin: string; secure: boolean; req: http.IncomingMessage }) => boolean; /** * VerifyClientCallbackAsync is an asynchronous callback used to inspect the * incoming message. The return value (boolean) of the function determines * whether or not to accept the handshake. */ type VerifyClientCallbackAsync = (info: { origin: string; secure: boolean; req: http.IncomingMessage } , callback: (res: boolean, code?: number, message?: string, headers?: http.OutgoingHttpHeaders) => void) => void; interface ClientOptions extends SecureContextOptions { protocol?: string; followRedirects?: boolean; handshakeTimeout?: number; maxRedirects?: number; perMessageDeflate?: boolean | PerMessageDeflateOptions; localAddress?: string; protocolVersion?: number; headers?: { [key: string]: string }; origin?: string; agent?: http.Agent; host?: string; family?: number; checkServerIdentity?(servername: string, cert: CertMeta): boolean; rejectUnauthorized?: boolean; maxPayload?: number; } interface PerMessageDeflateOptions { serverNoContextTakeover?: boolean; clientNoContextTakeover?: boolean; serverMaxWindowBits?: number; clientMaxWindowBits?: number; zlibDeflateOptions?: { flush?: number; finishFlush?: number; chunkSize?: number; windowBits?: number; level?: number; memLevel?: number; strategy?: number; dictionary?: Buffer | Buffer[] | DataView; info?: boolean; }; zlibInflateOptions?: zlib.ZlibOptions; threshold?: number; concurrencyLimit?: number; } interface OpenEvent { target: WebSocket; } interface ErrorEvent { error: any; message: string; type: string; target: WebSocket; } interface CloseEvent { wasClean: boolean; code: number; reason: string; target: WebSocket; } interface MessageEvent { data: Data; type: string; target: WebSocket; } interface EventListenerOptions { once?: boolean; } interface ServerOptions { host?: string; port?: number; backlog?: number; server?: http.Server | https.Server; verifyClient?: VerifyClientCallbackAsync | VerifyClientCallbackSync; handleProtocols?: any; path?: string; noServer?: boolean; clientTracking?: boolean; perMessageDeflate?: boolean | PerMessageDeflateOptions; maxPayload?: number; } interface AddressInfo { address: string; family: string; port: number; } // WebSocket Server class Server extends events.EventEmitter { options: ServerOptions; path: string; clients: Set<WebSocket>; constructor(options?: ServerOptions, callback?: () => void); address(): AddressInfo | string; close(cb?: (err?: Error) => void): void; handleUpgrade(request: http.IncomingMessage, socket: net.Socket, upgradeHead: Buffer, callback: (client: WebSocket, request: http.IncomingMessage) => void): void; shouldHandle(request: http.IncomingMessage): boolean | Promise<boolean>; // Events on(event: 'connection', cb: (this: Server, socket: WebSocket, request: http.IncomingMessage) => void): this; on(event: 'error', cb: (this: Server, error: Error) => void): this; on(event: 'headers', cb: (this: Server, headers: string[], request: http.IncomingMessage) => void): this; on(event: 'close' | 'listening', cb: (this: Server) => void): this; on(event: string | symbol, listener: (this: Server, ...args: any[]) => void): this; once(event: 'connection', cb: (this: Server, socket: WebSocket, request: http.IncomingMessage) => void): this; once(event: 'error', cb: (this: Server, error: Error) => void): this; once(event: 'headers', cb: (this: Server, headers: string[], request: http.IncomingMessage) => void): this; once(event: 'close' | 'listening', cb: (this: Server) => void): this; once(event: string | symbol, listener: (...args: any[]) => void): this; off(event: 'connection', cb: (this: Server, socket: WebSocket, request: http.IncomingMessage) => void): this; off(event: 'error', cb: (this: Server, error: Error) => void): this; off(event: 'headers', cb: (this: Server, headers: string[], request: http.IncomingMessage) => void): this; off(event: 'close' | 'listening', cb: (this: Server) => void): this; off(event: string | symbol, listener: (this: Server, ...args: any[]) => void): this; addListener(event: 'connection', cb: (client: WebSocket) => void): this; addListener(event: 'error', cb: (err: Error) => void): this; addListener(event: 'headers', cb: (headers: string[], request: http.IncomingMessage) => void): this; addListener(event: 'close' | 'listening', cb: () => void): this; addListener(event: string | symbol, listener: (...args: any[]) => void): this; removeListener(event: 'connection', cb: (client: WebSocket) => void): this; removeListener(event: 'error', cb: (err: Error) => void): this; removeListener(event: 'headers', cb: (headers: string[], request: http.IncomingMessage) => void): this; removeListener(event: 'close' | 'listening', cb: () => void): this; removeListener(event: string | symbol, listener: (...args: any[]) => void): this; } // WebSocket stream function createWebSocketStream(websocket: WebSocket, options?: stream.DuplexOptions): stream.Duplex; } export = WebSocket; apollo-server-demo/node_modules/@types/ws/README.md 0000644 0001750 0000144 00000001426 13752352623 021616 0 ustar andreh users # Installation > `npm install --save @types/ws` # Summary This package contains type definitions for ws (https://github.com/websockets/ws). # Details Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/ws. ### Additional Details * Last updated: Mon, 09 Nov 2020 23:49:39 GMT * Dependencies: [@types/node](https://npmjs.com/package/@types/node) * Global values: none # Credits These definitions were written by [Paul Loyd](https://github.com/loyd), [Matt Silverlock](https://github.com/elithrar), [Margus Lamp](https://github.com/mlamp), [Philippe D'Alva](https://github.com/TitaneBoy), [reduckted](https://github.com/reduckted), [teidesu](https://github.com/teidesu), and [Bartosz Wojtkowiak](https://github.com/wojtkowiak). apollo-server-demo/node_modules/@types/ws/package.json 0000644 0001750 0000144 00000003405 13752352623 022624 0 ustar andreh users { "name": "@types/ws", "version": "7.4.0", "description": "TypeScript definitions for ws", "license": "MIT", "contributors": [ { "name": "Paul Loyd", "url": "https://github.com/loyd", "githubUsername": "loyd" }, { "name": "Matt Silverlock", "url": "https://github.com/elithrar", "githubUsername": "elithrar" }, { "name": "Margus Lamp", "url": "https://github.com/mlamp", "githubUsername": "mlamp" }, { "name": "Philippe D'Alva", "url": "https://github.com/TitaneBoy", "githubUsername": "TitaneBoy" }, { "name": "reduckted", "url": "https://github.com/reduckted", "githubUsername": "reduckted" }, { "name": "teidesu", "url": "https://github.com/teidesu", "githubUsername": "teidesu" }, { "name": "Bartosz Wojtkowiak", "url": "https://github.com/wojtkowiak", "githubUsername": "wojtkowiak" } ], "main": "", "types": "index.d.ts", "repository": { "type": "git", "url": "https://github.com/DefinitelyTyped/DefinitelyTyped.git", "directory": "types/ws" }, "scripts": {}, "dependencies": { "@types/node": "*" }, "typesPublisherContentHash": "77b3e10cf373ea323f596b45785b79dd2b23f671bdbc08a581245f57a083fd1b", "typeScriptVersion": "3.2" ,"_resolved": "https://registry.npmjs.org/@types/ws/-/ws-7.4.0.tgz" ,"_integrity": "sha512-Y29uQ3Uy+58bZrFLhX36hcI3Np37nqWE7ky5tjiDoy1GDZnIwVxS0CgF+s+1bXMzjKBFy+fqaRfb708iNzdinw==" ,"_from": "@types/ws@7.4.0" } apollo-server-demo/node_modules/@types/fs-capacitor/ 0000755 0001750 0000144 00000000000 14067647701 022262 5 ustar andreh users apollo-server-demo/node_modules/@types/fs-capacitor/LICENSE 0000644 0001750 0000144 00000002237 13465067524 023273 0 ustar andreh users MIT License Copyright (c) Microsoft Corporation. All rights reserved. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE apollo-server-demo/node_modules/@types/fs-capacitor/index.d.ts 0000644 0001750 0000144 00000001127 13465067524 024164 0 ustar andreh users // Type definitions for fs-capacitor 2.0 // Project: https://github.com/mike-marcacci/fs-capacitor#readme // Definitions by: Mike Marcacci <https://github.com/mike-marcacci> // Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped // TypeScript Version: 3.3 /// <reference types="node" /> import { ReadStream as FSReadStream, WriteStream as FSWriteStream } from "fs"; export class ReadAfterDestroyedError extends Error {} export class ReadStream extends FSReadStream {} export class WriteStream extends FSWriteStream { constructor() createReadStream(name?: string): ReadStream; } apollo-server-demo/node_modules/@types/fs-capacitor/README.md 0000644 0001750 0000144 00000001023 13465067524 023535 0 ustar andreh users # Installation > `npm install --save @types/fs-capacitor` # Summary This package contains type definitions for fs-capacitor ( https://github.com/mike-marcacci/fs-capacitor#readme ). # Details Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/fs-capacitor Additional Details * Last updated: Thu, 09 May 2019 18:20:03 GMT * Dependencies: @types/node * Global values: none # Credits These definitions were written by Mike Marcacci <https://github.com/mike-marcacci>. apollo-server-demo/node_modules/@types/fs-capacitor/package.json 0000644 0001750 0000144 00000001727 13465067524 024557 0 ustar andreh users { "name": "@types/fs-capacitor", "version": "2.0.0", "description": "TypeScript definitions for fs-capacitor", "license": "MIT", "contributors": [ { "name": "Mike Marcacci", "url": "https://github.com/mike-marcacci", "githubUsername": "mike-marcacci" } ], "main": "", "types": "index", "repository": { "type": "git", "url": "https://github.com/DefinitelyTyped/DefinitelyTyped.git", "directory": "types/fs-capacitor" }, "scripts": {}, "dependencies": { "@types/node": "*" }, "typesPublisherContentHash": "3e4d6805865fc141224964e6de2778191082475da98fdef3f81e0a9d2d6b12bf", "typeScriptVersion": "3.3" ,"_resolved": "https://registry.npmjs.org/@types/fs-capacitor/-/fs-capacitor-2.0.0.tgz" ,"_integrity": "sha512-FKVPOCFbhCvZxpVAMhdBdTfVfXUpsh15wFHgqOKxh9N9vzWZVuWCSijZ5T4U34XYNnuj2oduh6xcs1i+LPI+BQ==" ,"_from": "@types/fs-capacitor@2.0.0" } apollo-server-demo/node_modules/@types/connect/ 0000755 0001750 0000144 00000000000 14067647700 021337 5 ustar andreh users apollo-server-demo/node_modules/@types/connect/LICENSE 0000644 0001750 0000144 00000002165 13763762713 022354 0 ustar andreh users MIT License Copyright (c) Microsoft Corporation. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE apollo-server-demo/node_modules/@types/connect/index.d.ts 0000644 0001750 0000144 00000006551 13763762713 023253 0 ustar andreh users // Type definitions for connect v3.4.0 // Project: https://github.com/senchalabs/connect // Definitions by: Maxime LUCE <https://github.com/SomaticIT> // Evan Hahn <https://github.com/EvanHahn> // Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped /// <reference types="node" /> import * as http from "http"; /** * Create a new connect server. */ declare function createServer(): createServer.Server; declare namespace createServer { export type ServerHandle = HandleFunction | http.Server; export class IncomingMessage extends http.IncomingMessage { originalUrl?: http.IncomingMessage["url"]; } type NextFunction = (err?: any) => void; export type SimpleHandleFunction = (req: IncomingMessage, res: http.ServerResponse) => void; export type NextHandleFunction = (req: IncomingMessage, res: http.ServerResponse, next: NextFunction) => void; export type ErrorHandleFunction = (err: any, req: IncomingMessage, res: http.ServerResponse, next: NextFunction) => void; export type HandleFunction = SimpleHandleFunction | NextHandleFunction | ErrorHandleFunction; export interface ServerStackItem { route: string; handle: ServerHandle; } export interface Server extends NodeJS.EventEmitter { (req: http.IncomingMessage, res: http.ServerResponse, next?: Function): void; route: string; stack: ServerStackItem[]; /** * Utilize the given middleware `handle` to the given `route`, * defaulting to _/_. This "route" is the mount-point for the * middleware, when given a value other than _/_ the middleware * is only effective when that segment is present in the request's * pathname. * * For example if we were to mount a function at _/admin_, it would * be invoked on _/admin_, and _/admin/settings_, however it would * not be invoked for _/_, or _/posts_. */ use(fn: NextHandleFunction): Server; use(fn: HandleFunction): Server; use(route: string, fn: NextHandleFunction): Server; use(route: string, fn: HandleFunction): Server; /** * Handle server requests, punting them down * the middleware stack. */ handle(req: http.IncomingMessage, res: http.ServerResponse, next: Function): void; /** * Listen for connections. * * This method takes the same arguments * as node's `http.Server#listen()`. * * HTTP and HTTPS: * * If you run your application both as HTTP * and HTTPS you may wrap them individually, * since your Connect "server" is really just * a JavaScript `Function`. * * var connect = require('connect') * , http = require('http') * , https = require('https'); * * var app = connect(); * * http.createServer(app).listen(80); * https.createServer(options, app).listen(443); */ listen(port: number, hostname?: string, backlog?: number, callback?: Function): http.Server; listen(port: number, hostname?: string, callback?: Function): http.Server; listen(path: string, callback?: Function): http.Server; listen(handle: any, listeningListener?: Function): http.Server; } } export = createServer; apollo-server-demo/node_modules/@types/connect/README.md 0000644 0001750 0000144 00000001112 13763762713 022615 0 ustar andreh users # Installation > `npm install --save @types/connect` # Summary This package contains type definitions for connect (https://github.com/senchalabs/connect). # Details Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/connect. ### Additional Details * Last updated: Tue, 08 Dec 2020 20:44:59 GMT * Dependencies: [@types/node](https://npmjs.com/package/@types/node) * Global values: none # Credits These definitions were written by [Maxime LUCE](https://github.com/SomaticIT), and [Evan Hahn](https://github.com/EvanHahn). apollo-server-demo/node_modules/@types/connect/package.json 0000644 0001750 0000144 00000002110 13763762713 023623 0 ustar andreh users { "name": "@types/connect", "version": "3.4.34", "description": "TypeScript definitions for connect", "license": "MIT", "contributors": [ { "name": "Maxime LUCE", "url": "https://github.com/SomaticIT", "githubUsername": "SomaticIT" }, { "name": "Evan Hahn", "url": "https://github.com/EvanHahn", "githubUsername": "EvanHahn" } ], "main": "", "types": "index.d.ts", "repository": { "type": "git", "url": "https://github.com/DefinitelyTyped/DefinitelyTyped.git", "directory": "types/connect" }, "scripts": {}, "dependencies": { "@types/node": "*" }, "typesPublisherContentHash": "2fd2f6a2f7b9371cdb60b971639b4d26989e6035dc30fb0ad12e72645dcb002d", "typeScriptVersion": "3.3" ,"_resolved": "https://registry.npmjs.org/@types/connect/-/connect-3.4.34.tgz" ,"_integrity": "sha512-ePPA/JuI+X0vb+gSWlPKOY0NdNAie/rPUqX2GUPpbZwiKTkSPhjXWuee47E4MtE54QVzGCQMQkAL6JhV2E1+cQ==" ,"_from": "@types/connect@3.4.34" } apollo-server-demo/node_modules/@types/express-serve-static-core/ 0000755 0001750 0000144 00000000000 14067647700 024734 5 ustar andreh users apollo-server-demo/node_modules/@types/express-serve-static-core/LICENSE 0000644 0001750 0000144 00000002165 13777146606 025753 0 ustar andreh users MIT License Copyright (c) Microsoft Corporation. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE apollo-server-demo/node_modules/@types/express-serve-static-core/index.d.ts 0000644 0001750 0000144 00000110600 13777146606 026641 0 ustar andreh users // Type definitions for Express 4.17 // Project: http://expressjs.com // Definitions by: Boris Yankov <https://github.com/borisyankov> // MichaÅ‚ Lytek <https://github.com/19majkel94> // Kacper Polak <https://github.com/kacepe> // Satana Charuwichitratana <https://github.com/micksatana> // Sami Jaber <https://github.com/samijaber> // Jose Luis Leon <https://github.com/JoseLion> // David Stephens <https://github.com/dwrss> // Shin Ando <https://github.com/andoshin11> // Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped // TypeScript Version: 2.3 // This extracts the core definitions from express to prevent a circular dependency between express and serve-static /// <reference types="node" /> declare global { namespace Express { // These open interfaces may be extended in an application-specific manner via declaration merging. // See for example method-override.d.ts (https://github.com/DefinitelyTyped/DefinitelyTyped/blob/master/types/method-override/index.d.ts) interface Request {} interface Response {} interface Application {} } } import * as http from 'http'; import { EventEmitter } from 'events'; import { Options as RangeParserOptions, Result as RangeParserResult, Ranges as RangeParserRanges } from 'range-parser'; import { ParsedQs } from 'qs'; export type Query = ParsedQs; export interface NextFunction { (err?: any): void; /** * "Break-out" of a router by calling {next('router')}; * @see {https://expressjs.com/en/guide/using-middleware.html#middleware.router} */ (deferToNext: 'router'): void; } export interface Dictionary<T> { [key: string]: T; } export interface ParamsDictionary { [key: string]: string; } export type ParamsArray = string[]; export type Params = ParamsDictionary | ParamsArray; export interface RequestHandler< P = ParamsDictionary, ResBody = any, ReqBody = any, ReqQuery = ParsedQs, Locals extends Record<string, any> = Record<string, any> > { // tslint:disable-next-line callable-types (This is extended from and can't extend from a type alias in ts<2.2) ( req: Request<P, ResBody, ReqBody, ReqQuery, Locals>, res: Response<ResBody, Locals>, next: NextFunction, ): void; } export type ErrorRequestHandler< P = ParamsDictionary, ResBody = any, ReqBody = any, ReqQuery = ParsedQs, Locals extends Record<string, any> = Record<string, any> > = ( err: any, req: Request<P, ResBody, ReqBody, ReqQuery, Locals>, res: Response<ResBody, Locals>, next: NextFunction, ) => void; export type PathParams = string | RegExp | Array<string | RegExp>; export type RequestHandlerParams< P = ParamsDictionary, ResBody = any, ReqBody = any, ReqQuery = ParsedQs, Locals extends Record<string, any> = Record<string, any> > = | RequestHandler<P, ResBody, ReqBody, ReqQuery, Locals> | ErrorRequestHandler<P, ResBody, ReqBody, ReqQuery, Locals> | Array<RequestHandler<P> | ErrorRequestHandler<P>>; export interface IRouterMatcher< T, Method extends 'all' | 'get' | 'post' | 'put' | 'delete' | 'patch' | 'options' | 'head' = any > { < P = ParamsDictionary, ResBody = any, ReqBody = any, ReqQuery = ParsedQs, Locals extends Record<string, any> = Record<string, any> >( path: PathParams, // tslint:disable-next-line no-unnecessary-generics (This generic is meant to be passed explicitly.) ...handlers: Array<RequestHandler<P, ResBody, ReqBody, ReqQuery, Locals>> ): T; < P = ParamsDictionary, ResBody = any, ReqBody = any, ReqQuery = ParsedQs, Locals extends Record<string, any> = Record<string, any> >( path: PathParams, // tslint:disable-next-line no-unnecessary-generics (This generic is meant to be passed explicitly.) ...handlers: Array<RequestHandlerParams<P, ResBody, ReqBody, ReqQuery, Locals>> ): T; (path: PathParams, subApplication: Application): T; } export interface IRouterHandler<T> { (...handlers: RequestHandler[]): T; (...handlers: RequestHandlerParams[]): T; < P = ParamsDictionary, ResBody = any, ReqBody = any, ReqQuery = ParsedQs, Locals extends Record<string, any> = Record<string, any> >( // tslint:disable-next-line no-unnecessary-generics (This generic is meant to be passed explicitly.) ...handlers: Array<RequestHandler<P, ResBody, ReqBody, ReqQuery, Locals>> ): T; < P = ParamsDictionary, ResBody = any, ReqBody = any, ReqQuery = ParsedQs, Locals extends Record<string, any> = Record<string, any> >( // tslint:disable-next-line no-unnecessary-generics (This generic is meant to be passed explicitly.) ...handlers: Array<RequestHandlerParams<P, ResBody, ReqBody, ReqQuery, Locals>> ): T; } export interface IRouter extends RequestHandler { /** * Map the given param placeholder `name`(s) to the given callback(s). * * Parameter mapping is used to provide pre-conditions to routes * which use normalized placeholders. For example a _:user_id_ parameter * could automatically load a user's information from the database without * any additional code, * * The callback uses the samesignature as middleware, the only differencing * being that the value of the placeholder is passed, in this case the _id_ * of the user. Once the `next()` function is invoked, just like middleware * it will continue on to execute the route, or subsequent parameter functions. * * app.param('user_id', function(req, res, next, id){ * User.find(id, function(err, user){ * if (err) { * next(err); * } else if (user) { * req.user = user; * next(); * } else { * next(new Error('failed to load user')); * } * }); * }); */ param(name: string, handler: RequestParamHandler): this; /** * Alternatively, you can pass only a callback, in which case you have the opportunity to alter the app.param() * * @deprecated since version 4.11 */ param(callback: (name: string, matcher: RegExp) => RequestParamHandler): this; /** * Special-cased "all" method, applying the given route `path`, * middleware, and callback to _every_ HTTP method. */ all: IRouterMatcher<this, 'all'>; get: IRouterMatcher<this, 'get'>; post: IRouterMatcher<this, 'post'>; put: IRouterMatcher<this, 'put'>; delete: IRouterMatcher<this, 'delete'>; patch: IRouterMatcher<this, 'patch'>; options: IRouterMatcher<this, 'options'>; head: IRouterMatcher<this, 'head'>; checkout: IRouterMatcher<this>; connect: IRouterMatcher<this>; copy: IRouterMatcher<this>; lock: IRouterMatcher<this>; merge: IRouterMatcher<this>; mkactivity: IRouterMatcher<this>; mkcol: IRouterMatcher<this>; move: IRouterMatcher<this>; 'm-search': IRouterMatcher<this>; notify: IRouterMatcher<this>; propfind: IRouterMatcher<this>; proppatch: IRouterMatcher<this>; purge: IRouterMatcher<this>; report: IRouterMatcher<this>; search: IRouterMatcher<this>; subscribe: IRouterMatcher<this>; trace: IRouterMatcher<this>; unlock: IRouterMatcher<this>; unsubscribe: IRouterMatcher<this>; use: IRouterHandler<this> & IRouterMatcher<this>; route(prefix: PathParams): IRoute; /** * Stack of configured routes */ stack: any[]; } export interface IRoute { path: string; stack: any; all: IRouterHandler<this>; get: IRouterHandler<this>; post: IRouterHandler<this>; put: IRouterHandler<this>; delete: IRouterHandler<this>; patch: IRouterHandler<this>; options: IRouterHandler<this>; head: IRouterHandler<this>; checkout: IRouterHandler<this>; copy: IRouterHandler<this>; lock: IRouterHandler<this>; merge: IRouterHandler<this>; mkactivity: IRouterHandler<this>; mkcol: IRouterHandler<this>; move: IRouterHandler<this>; 'm-search': IRouterHandler<this>; notify: IRouterHandler<this>; purge: IRouterHandler<this>; report: IRouterHandler<this>; search: IRouterHandler<this>; subscribe: IRouterHandler<this>; trace: IRouterHandler<this>; unlock: IRouterHandler<this>; unsubscribe: IRouterHandler<this>; } export interface Router extends IRouter {} export interface CookieOptions { maxAge?: number; signed?: boolean; expires?: Date; httpOnly?: boolean; path?: string; domain?: string; secure?: boolean; encode?: (val: string) => string; sameSite?: boolean | 'lax' | 'strict' | 'none'; } export interface ByteRange { start: number; end: number; } export interface RequestRanges extends RangeParserRanges {} export type Errback = (err: Error) => void; /** * @param P For most requests, this should be `ParamsDictionary`, but if you're * using this in a route handler for a route that uses a `RegExp` or a wildcard * `string` path (e.g. `'/user/*'`), then `req.params` will be an array, in * which case you should use `ParamsArray` instead. * * @see https://expressjs.com/en/api.html#req.params * * @example * app.get('/user/:id', (req, res) => res.send(req.params.id)); // implicitly `ParamsDictionary` * app.get<ParamsArray>(/user\/(.*)/, (req, res) => res.send(req.params[0])); * app.get<ParamsArray>('/user/*', (req, res) => res.send(req.params[0])); */ export interface Request< P = ParamsDictionary, ResBody = any, ReqBody = any, ReqQuery = ParsedQs, Locals extends Record<string, any> = Record<string, any> > extends http.IncomingMessage, Express.Request { /** * Return request header. * * The `Referrer` header field is special-cased, * both `Referrer` and `Referer` are interchangeable. * * Examples: * * req.get('Content-Type'); * // => "text/plain" * * req.get('content-type'); * // => "text/plain" * * req.get('Something'); * // => undefined * * Aliased as `req.header()`. */ get(name: 'set-cookie'): string[] | undefined; get(name: string): string | undefined; header(name: 'set-cookie'): string[] | undefined; header(name: string): string | undefined; /** * Check if the given `type(s)` is acceptable, returning * the best match when true, otherwise `undefined`, in which * case you should respond with 406 "Not Acceptable". * * The `type` value may be a single mime type string * such as "application/json", the extension name * such as "json", a comma-delimted list such as "json, html, text/plain", * or an array `["json", "html", "text/plain"]`. When a list * or array is given the _best_ match, if any is returned. * * Examples: * * // Accept: text/html * req.accepts('html'); * // => "html" * * // Accept: text/*, application/json * req.accepts('html'); * // => "html" * req.accepts('text/html'); * // => "text/html" * req.accepts('json, text'); * // => "json" * req.accepts('application/json'); * // => "application/json" * * // Accept: text/*, application/json * req.accepts('image/png'); * req.accepts('png'); * // => undefined * * // Accept: text/*;q=.5, application/json * req.accepts(['html', 'json']); * req.accepts('html, json'); * // => "json" */ accepts(): string[]; accepts(type: string): string | false; accepts(type: string[]): string | false; accepts(...type: string[]): string | false; /** * Returns the first accepted charset of the specified character sets, * based on the request's Accept-Charset HTTP header field. * If none of the specified charsets is accepted, returns false. * * For more information, or if you have issues or concerns, see accepts. */ acceptsCharsets(): string[]; acceptsCharsets(charset: string): string | false; acceptsCharsets(charset: string[]): string | false; acceptsCharsets(...charset: string[]): string | false; /** * Returns the first accepted encoding of the specified encodings, * based on the request's Accept-Encoding HTTP header field. * If none of the specified encodings is accepted, returns false. * * For more information, or if you have issues or concerns, see accepts. */ acceptsEncodings(): string[]; acceptsEncodings(encoding: string): string | false; acceptsEncodings(encoding: string[]): string | false; acceptsEncodings(...encoding: string[]): string | false; /** * Returns the first accepted language of the specified languages, * based on the request's Accept-Language HTTP header field. * If none of the specified languages is accepted, returns false. * * For more information, or if you have issues or concerns, see accepts. */ acceptsLanguages(): string[]; acceptsLanguages(lang: string): string | false; acceptsLanguages(lang: string[]): string | false; acceptsLanguages(...lang: string[]): string | false; /** * Parse Range header field, capping to the given `size`. * * Unspecified ranges such as "0-" require knowledge of your resource length. In * the case of a byte range this is of course the total number of bytes. * If the Range header field is not given `undefined` is returned. * If the Range header field is given, return value is a result of range-parser. * See more ./types/range-parser/index.d.ts * * NOTE: remember that ranges are inclusive, so for example "Range: users=0-3" * should respond with 4 users when available, not 3. * */ range(size: number, options?: RangeParserOptions): RangeParserRanges | RangeParserResult | undefined; /** * Return an array of Accepted media types * ordered from highest quality to lowest. */ accepted: MediaType[]; /** * @deprecated since 4.11 Use either req.params, req.body or req.query, as applicable. * * Return the value of param `name` when present or `defaultValue`. * * - Checks route placeholders, ex: _/user/:id_ * - Checks body params, ex: id=12, {"id":12} * - Checks query string params, ex: ?id=12 * * To utilize request bodies, `req.body` * should be an object. This can be done by using * the `connect.bodyParser()` middleware. */ param(name: string, defaultValue?: any): string; /** * Check if the incoming request contains the "Content-Type" * header field, and it contains the give mime `type`. * * Examples: * * // With Content-Type: text/html; charset=utf-8 * req.is('html'); * req.is('text/html'); * req.is('text/*'); * // => true * * // When Content-Type is application/json * req.is('json'); * req.is('application/json'); * req.is('application/*'); * // => true * * req.is('html'); * // => false */ is(type: string | string[]): string | false | null; /** * Return the protocol string "http" or "https" * when requested with TLS. When the "trust proxy" * setting is enabled the "X-Forwarded-Proto" header * field will be trusted. If you're running behind * a reverse proxy that supplies https for you this * may be enabled. */ protocol: string; /** * Short-hand for: * * req.protocol == 'https' */ secure: boolean; /** * Return the remote address, or when * "trust proxy" is `true` return * the upstream addr. */ ip: string; /** * When "trust proxy" is `true`, parse * the "X-Forwarded-For" ip address list. * * For example if the value were "client, proxy1, proxy2" * you would receive the array `["client", "proxy1", "proxy2"]` * where "proxy2" is the furthest down-stream. */ ips: string[]; /** * Return subdomains as an array. * * Subdomains are the dot-separated parts of the host before the main domain of * the app. By default, the domain of the app is assumed to be the last two * parts of the host. This can be changed by setting "subdomain offset". * * For example, if the domain is "tobi.ferrets.example.com": * If "subdomain offset" is not set, req.subdomains is `["ferrets", "tobi"]`. * If "subdomain offset" is 3, req.subdomains is `["tobi"]`. */ subdomains: string[]; /** * Short-hand for `url.parse(req.url).pathname`. */ path: string; /** * Parse the "Host" header field hostname. */ hostname: string; /** * @deprecated Use hostname instead. */ host: string; /** * Check if the request is fresh, aka * Last-Modified and/or the ETag * still match. */ fresh: boolean; /** * Check if the request is stale, aka * "Last-Modified" and / or the "ETag" for the * resource has changed. */ stale: boolean; /** * Check if the request was an _XMLHttpRequest_. */ xhr: boolean; //body: { username: string; password: string; remember: boolean; title: string; }; body: ReqBody; //cookies: { string; remember: boolean; }; cookies: any; method: string; params: P; query: ReqQuery; route: any; signedCookies: any; originalUrl: string; url: string; baseUrl: string; app: Application; /** * After middleware.init executed, Request will contain res and next properties * See: express/lib/middleware/init.js */ res?: Response<ResBody, Locals>; next?: NextFunction; } export interface MediaType { value: string; quality: number; type: string; subtype: string; } export type Send<ResBody = any, T = Response<ResBody>> = (body?: ResBody) => T; export interface Response< ResBody = any, Locals extends Record<string, any> = Record<string, any>, StatusCode extends number = number > extends http.ServerResponse, Express.Response { /** * Set status `code`. */ status(code: StatusCode): this; /** * Set the response HTTP status code to `statusCode` and send its string representation as the response body. * @link http://expressjs.com/4x/api.html#res.sendStatus * * Examples: * * res.sendStatus(200); // equivalent to res.status(200).send('OK') * res.sendStatus(403); // equivalent to res.status(403).send('Forbidden') * res.sendStatus(404); // equivalent to res.status(404).send('Not Found') * res.sendStatus(500); // equivalent to res.status(500).send('Internal Server Error') */ sendStatus(code: StatusCode): this; /** * Set Link header field with the given `links`. * * Examples: * * res.links({ * next: 'http://api.example.com/users?page=2', * last: 'http://api.example.com/users?page=5' * }); */ links(links: any): this; /** * Send a response. * * Examples: * * res.send(new Buffer('wahoo')); * res.send({ some: 'json' }); * res.send('<p>some html</p>'); * res.status(404).send('Sorry, cant find that'); */ send: Send<ResBody, this>; /** * Send JSON response. * * Examples: * * res.json(null); * res.json({ user: 'tj' }); * res.status(500).json('oh noes!'); * res.status(404).json('I dont have that'); */ json: Send<ResBody, this>; /** * Send JSON response with JSONP callback support. * * Examples: * * res.jsonp(null); * res.jsonp({ user: 'tj' }); * res.status(500).jsonp('oh noes!'); * res.status(404).jsonp('I dont have that'); */ jsonp: Send<ResBody, this>; /** * Transfer the file at the given `path`. * * Automatically sets the _Content-Type_ response header field. * The callback `fn(err)` is invoked when the transfer is complete * or when an error occurs. Be sure to check `res.headersSent` * if you wish to attempt responding, as the header and some data * may have already been transferred. * * Options: * * - `maxAge` defaulting to 0 (can be string converted by `ms`) * - `root` root directory for relative filenames * - `headers` object of headers to serve with file * - `dotfiles` serve dotfiles, defaulting to false; can be `"allow"` to send them * * Other options are passed along to `send`. * * Examples: * * The following example illustrates how `res.sendFile()` may * be used as an alternative for the `static()` middleware for * dynamic situations. The code backing `res.sendFile()` is actually * the same code, so HTTP cache support etc is identical. * * app.get('/user/:uid/photos/:file', function(req, res){ * var uid = req.params.uid * , file = req.params.file; * * req.user.mayViewFilesFrom(uid, function(yes){ * if (yes) { * res.sendFile('/uploads/' + uid + '/' + file); * } else { * res.send(403, 'Sorry! you cant see that.'); * } * }); * }); * * @api public */ sendFile(path: string, fn?: Errback): void; sendFile(path: string, options: any, fn?: Errback): void; /** * @deprecated Use sendFile instead. */ sendfile(path: string): void; /** * @deprecated Use sendFile instead. */ sendfile(path: string, options: any): void; /** * @deprecated Use sendFile instead. */ sendfile(path: string, fn: Errback): void; /** * @deprecated Use sendFile instead. */ sendfile(path: string, options: any, fn: Errback): void; /** * Transfer the file at the given `path` as an attachment. * * Optionally providing an alternate attachment `filename`, * and optional callback `fn(err)`. The callback is invoked * when the data transfer is complete, or when an error has * ocurred. Be sure to check `res.headersSent` if you plan to respond. * * The optional options argument passes through to the underlying * res.sendFile() call, and takes the exact same parameters. * * This method uses `res.sendfile()`. */ download(path: string, fn?: Errback): void; download(path: string, filename: string, fn?: Errback): void; download(path: string, filename: string, options: any, fn?: Errback): void; /** * Set _Content-Type_ response header with `type` through `mime.lookup()` * when it does not contain "/", or set the Content-Type to `type` otherwise. * * Examples: * * res.type('.html'); * res.type('html'); * res.type('json'); * res.type('application/json'); * res.type('png'); */ contentType(type: string): this; /** * Set _Content-Type_ response header with `type` through `mime.lookup()` * when it does not contain "/", or set the Content-Type to `type` otherwise. * * Examples: * * res.type('.html'); * res.type('html'); * res.type('json'); * res.type('application/json'); * res.type('png'); */ type(type: string): this; /** * Respond to the Acceptable formats using an `obj` * of mime-type callbacks. * * This method uses `req.accepted`, an array of * acceptable types ordered by their quality values. * When "Accept" is not present the _first_ callback * is invoked, otherwise the first match is used. When * no match is performed the server responds with * 406 "Not Acceptable". * * Content-Type is set for you, however if you choose * you may alter this within the callback using `res.type()` * or `res.set('Content-Type', ...)`. * * res.format({ * 'text/plain': function(){ * res.send('hey'); * }, * * 'text/html': function(){ * res.send('<p>hey</p>'); * }, * * 'appliation/json': function(){ * res.send({ message: 'hey' }); * } * }); * * In addition to canonicalized MIME types you may * also use extnames mapped to these types: * * res.format({ * text: function(){ * res.send('hey'); * }, * * html: function(){ * res.send('<p>hey</p>'); * }, * * json: function(){ * res.send({ message: 'hey' }); * } * }); * * By default Express passes an `Error` * with a `.status` of 406 to `next(err)` * if a match is not made. If you provide * a `.default` callback it will be invoked * instead. */ format(obj: any): this; /** * Set _Content-Disposition_ header to _attachment_ with optional `filename`. */ attachment(filename?: string): this; /** * Set header `field` to `val`, or pass * an object of header fields. * * Examples: * * res.set('Foo', ['bar', 'baz']); * res.set('Accept', 'application/json'); * res.set({ Accept: 'text/plain', 'X-API-Key': 'tobi' }); * * Aliased as `res.header()`. */ set(field: any): this; set(field: string, value?: string | string[]): this; header(field: any): this; header(field: string, value?: string | string[]): this; // Property indicating if HTTP headers has been sent for the response. headersSent: boolean; /** Get value for header `field`. */ get(field: string): string; /** Clear cookie `name`. */ clearCookie(name: string, options?: any): this; /** * Set cookie `name` to `val`, with the given `options`. * * Options: * * - `maxAge` max-age in milliseconds, converted to `expires` * - `signed` sign the cookie * - `path` defaults to "/" * * Examples: * * // "Remember Me" for 15 minutes * res.cookie('rememberme', '1', { expires: new Date(Date.now() + 900000), httpOnly: true }); * * // save as above * res.cookie('rememberme', '1', { maxAge: 900000, httpOnly: true }) */ cookie(name: string, val: string, options: CookieOptions): this; cookie(name: string, val: any, options: CookieOptions): this; cookie(name: string, val: any): this; /** * Set the location header to `url`. * * The given `url` can also be the name of a mapped url, for * example by default express supports "back" which redirects * to the _Referrer_ or _Referer_ headers or "/". * * Examples: * * res.location('/foo/bar').; * res.location('http://example.com'); * res.location('../login'); // /blog/post/1 -> /blog/login * * Mounting: * * When an application is mounted and `res.location()` * is given a path that does _not_ lead with "/" it becomes * relative to the mount-point. For example if the application * is mounted at "/blog", the following would become "/blog/login". * * res.location('login'); * * While the leading slash would result in a location of "/login": * * res.location('/login'); */ location(url: string): this; /** * Redirect to the given `url` with optional response `status` * defaulting to 302. * * The resulting `url` is determined by `res.location()`, so * it will play nicely with mounted apps, relative paths, * `"back"` etc. * * Examples: * * res.redirect('/foo/bar'); * res.redirect('http://example.com'); * res.redirect(301, 'http://example.com'); * res.redirect('http://example.com', 301); * res.redirect('../login'); // /blog/post/1 -> /blog/login */ redirect(url: string): void; redirect(status: number, url: string): void; redirect(url: string, status: number): void; /** * Render `view` with the given `options` and optional callback `fn`. * When a callback function is given a response will _not_ be made * automatically, otherwise a response of _200_ and _text/html_ is given. * * Options: * * - `cache` boolean hinting to the engine it should cache * - `filename` filename of the view being rendered */ render(view: string, options?: object, callback?: (err: Error, html: string) => void): void; render(view: string, callback?: (err: Error, html: string) => void): void; locals: Locals; charset: string; /** * Adds the field to the Vary response header, if it is not there already. * Examples: * * res.vary('User-Agent').render('docs'); * */ vary(field: string): this; app: Application; /** * Appends the specified value to the HTTP response header field. * If the header is not already set, it creates the header with the specified value. * The value parameter can be a string or an array. * * Note: calling res.set() after res.append() will reset the previously-set header value. * * @since 4.11.0 */ append(field: string, value?: string[] | string): this; /** * After middleware.init executed, Response will contain req property * See: express/lib/middleware/init.js */ req?: Request; } export interface Handler extends RequestHandler {} export type RequestParamHandler = (req: Request, res: Response, next: NextFunction, value: any, name: string) => any; export type ApplicationRequestHandler<T> = IRouterHandler<T> & IRouterMatcher<T> & ((...handlers: RequestHandlerParams[]) => T); export interface Application extends EventEmitter, IRouter, Express.Application { /** * Express instance itself is a request handler, which could be invoked without * third argument. */ (req: Request | http.IncomingMessage, res: Response | http.ServerResponse): any; /** * Initialize the server. * * - setup default configuration * - setup default middleware * - setup route reflection methods */ init(): void; /** * Initialize application configuration. */ defaultConfiguration(): void; /** * Register the given template engine callback `fn` * as `ext`. * * By default will `require()` the engine based on the * file extension. For example if you try to render * a "foo.jade" file Express will invoke the following internally: * * app.engine('jade', require('jade').__express); * * For engines that do not provide `.__express` out of the box, * or if you wish to "map" a different extension to the template engine * you may use this method. For example mapping the EJS template engine to * ".html" files: * * app.engine('html', require('ejs').renderFile); * * In this case EJS provides a `.renderFile()` method with * the same signature that Express expects: `(path, options, callback)`, * though note that it aliases this method as `ejs.__express` internally * so if you're using ".ejs" extensions you dont need to do anything. * * Some template engines do not follow this convention, the * [Consolidate.js](https://github.com/visionmedia/consolidate.js) * library was created to map all of node's popular template * engines to follow this convention, thus allowing them to * work seamlessly within Express. */ engine( ext: string, fn: (path: string, options: object, callback: (e: any, rendered?: string) => void) => void, ): this; /** * Assign `setting` to `val`, or return `setting`'s value. * * app.set('foo', 'bar'); * app.get('foo'); * // => "bar" * app.set('foo', ['bar', 'baz']); * app.get('foo'); * // => ["bar", "baz"] * * Mounted servers inherit their parent server's settings. */ set(setting: string, val: any): this; get: ((name: string) => any) & IRouterMatcher<this>; param(name: string | string[], handler: RequestParamHandler): this; /** * Alternatively, you can pass only a callback, in which case you have the opportunity to alter the app.param() * * @deprecated since version 4.11 */ param(callback: (name: string, matcher: RegExp) => RequestParamHandler): this; /** * Return the app's absolute pathname * based on the parent(s) that have * mounted it. * * For example if the application was * mounted as "/admin", which itself * was mounted as "/blog" then the * return value would be "/blog/admin". */ path(): string; /** * Check if `setting` is enabled (truthy). * * app.enabled('foo') * // => false * * app.enable('foo') * app.enabled('foo') * // => true */ enabled(setting: string): boolean; /** * Check if `setting` is disabled. * * app.disabled('foo') * // => true * * app.enable('foo') * app.disabled('foo') * // => false */ disabled(setting: string): boolean; /** Enable `setting`. */ enable(setting: string): this; /** Disable `setting`. */ disable(setting: string): this; /** * Render the given view `name` name with `options` * and a callback accepting an error and the * rendered template string. * * Example: * * app.render('email', { name: 'Tobi' }, function(err, html){ * // ... * }) */ render(name: string, options?: object, callback?: (err: Error, html: string) => void): void; render(name: string, callback: (err: Error, html: string) => void): void; /** * Listen for connections. * * A node `http.Server` is returned, with this * application (which is a `Function`) as its * callback. If you wish to create both an HTTP * and HTTPS server you may do so with the "http" * and "https" modules as shown here: * * var http = require('http') * , https = require('https') * , express = require('express') * , app = express(); * * http.createServer(app).listen(80); * https.createServer({ ... }, app).listen(443); */ listen(port: number, hostname: string, backlog: number, callback?: () => void): http.Server; listen(port: number, hostname: string, callback?: () => void): http.Server; listen(port: number, callback?: () => void): http.Server; listen(callback?: () => void): http.Server; listen(path: string, callback?: () => void): http.Server; listen(handle: any, listeningListener?: () => void): http.Server; router: string; settings: any; resource: any; map: any; locals: Record<string, any>; /** * The app.routes object houses all of the routes defined mapped by the * associated HTTP verb. This object may be used for introspection * capabilities, for example Express uses this internally not only for * routing but to provide default OPTIONS behaviour unless app.options() * is used. Your application or framework may also remove routes by * simply by removing them from this object. */ routes: any; /** * Used to get all registered routes in Express Application */ _router: any; use: ApplicationRequestHandler<this>; /** * The mount event is fired on a sub-app, when it is mounted on a parent app. * The parent app is passed to the callback function. * * NOTE: * Sub-apps will: * - Not inherit the value of settings that have a default value. You must set the value in the sub-app. * - Inherit the value of settings with no default value. */ on: (event: string, callback: (parent: Application) => void) => this; /** * The app.mountpath property contains one or more path patterns on which a sub-app was mounted. */ mountpath: string | string[]; } export interface Express extends Application { request: Request; response: Response; } apollo-server-demo/node_modules/@types/express-serve-static-core/README.md 0000644 0001750 0000144 00000001767 13777146606 026234 0 ustar andreh users # Installation > `npm install --save @types/express-serve-static-core` # Summary This package contains type definitions for Express (http://expressjs.com). # Details Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/express-serve-static-core. ### Additional Details * Last updated: Mon, 11 Jan 2021 22:13:26 GMT * Dependencies: [@types/range-parser](https://npmjs.com/package/@types/range-parser), [@types/qs](https://npmjs.com/package/@types/qs), [@types/node](https://npmjs.com/package/@types/node) * Global values: none # Credits These definitions were written by [Boris Yankov](https://github.com/borisyankov), [MichaÅ‚ Lytek](https://github.com/19majkel94), [Kacper Polak](https://github.com/kacepe), [Satana Charuwichitratana](https://github.com/micksatana), [Sami Jaber](https://github.com/samijaber), [Jose Luis Leon](https://github.com/JoseLion), [David Stephens](https://github.com/dwrss), and [Shin Ando](https://github.com/andoshin11). apollo-server-demo/node_modules/@types/express-serve-static-core/package.json 0000644 0001750 0000144 00000004163 13777146606 027234 0 ustar andreh users { "name": "@types/express-serve-static-core", "version": "4.17.18", "description": "TypeScript definitions for Express", "license": "MIT", "contributors": [ { "name": "Boris Yankov", "url": "https://github.com/borisyankov", "githubUsername": "borisyankov" }, { "name": "MichaÅ‚ Lytek", "url": "https://github.com/19majkel94", "githubUsername": "19majkel94" }, { "name": "Kacper Polak", "url": "https://github.com/kacepe", "githubUsername": "kacepe" }, { "name": "Satana Charuwichitratana", "url": "https://github.com/micksatana", "githubUsername": "micksatana" }, { "name": "Sami Jaber", "url": "https://github.com/samijaber", "githubUsername": "samijaber" }, { "name": "Jose Luis Leon", "url": "https://github.com/JoseLion", "githubUsername": "JoseLion" }, { "name": "David Stephens", "url": "https://github.com/dwrss", "githubUsername": "dwrss" }, { "name": "Shin Ando", "url": "https://github.com/andoshin11", "githubUsername": "andoshin11" } ], "main": "", "types": "index.d.ts", "repository": { "type": "git", "url": "https://github.com/DefinitelyTyped/DefinitelyTyped.git", "directory": "types/express-serve-static-core" }, "scripts": {}, "dependencies": { "@types/node": "*", "@types/qs": "*", "@types/range-parser": "*" }, "typesPublisherContentHash": "60873d177898f88d23027366f6d52aec9d27759ca997fea4f7c6b9729356fc20", "typeScriptVersion": "3.3" ,"_resolved": "https://registry.npmjs.org/@types/express-serve-static-core/-/express-serve-static-core-4.17.18.tgz" ,"_integrity": "sha512-m4JTwx5RUBNZvky/JJ8swEJPKFd8si08pPF2PfizYjGZOKr/svUWPcoUmLow6MmPzhasphB7gSTINY67xn3JNA==" ,"_from": "@types/express-serve-static-core@4.17.18" } apollo-server-demo/node_modules/@types/long/ 0000755 0001750 0000144 00000000000 14067647701 020646 5 ustar andreh users apollo-server-demo/node_modules/@types/long/LICENSE 0000644 0001750 0000144 00000002237 13612120122 021631 0 ustar andreh users MIT License Copyright (c) Microsoft Corporation. All rights reserved. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE apollo-server-demo/node_modules/@types/long/index.d.ts 0000644 0001750 0000144 00000025076 13612120122 022533 0 ustar andreh users // Type definitions for long.js 4.0.0 // Project: https://github.com/dcodeIO/long.js // Definitions by: Peter Kooijmans <https://github.com/peterkooijmans> // Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped // Definitions by: Denis Cappellin <https://github.com/cappellin> export = Long; export as namespace Long; declare const Long: Long.LongConstructor; type Long = Long.Long; declare namespace Long { interface LongConstructor { /** * Constructs a 64 bit two's-complement integer, given its low and high 32 bit values as signed integers. See the from* functions below for more convenient ways of constructing Longs. */ new( low: number, high?: number, unsigned?: boolean ): Long; prototype: Long; /** * Maximum unsigned value. */ MAX_UNSIGNED_VALUE: Long; /** * Maximum signed value. */ MAX_VALUE: Long; /** * Minimum signed value. */ MIN_VALUE: Long; /** * Signed negative one. */ NEG_ONE: Long; /** * Signed one. */ ONE: Long; /** * Unsigned one. */ UONE: Long; /** * Unsigned zero. */ UZERO: Long; /** * Signed zero */ ZERO: Long; /** * Returns a Long representing the 64 bit integer that comes by concatenating the given low and high bits. Each is assumed to use 32 bits. */ fromBits( lowBits:number, highBits:number, unsigned?:boolean ): Long; /** * Returns a Long representing the given 32 bit integer value. */ fromInt( value: number, unsigned?: boolean ): Long; /** * Returns a Long representing the given value, provided that it is a finite number. Otherwise, zero is returned. */ fromNumber( value: number, unsigned?: boolean ): Long; /** * Returns a Long representation of the given string, written using the specified radix. */ fromString( str: string, unsigned?: boolean | number, radix?: number ): Long; /** * Creates a Long from its byte representation. */ fromBytes( bytes: number[], unsigned?: boolean, le?: boolean ): Long; /** * Creates a Long from its little endian byte representation. */ fromBytesLE( bytes: number[], unsigned?: boolean ): Long; /** * Creates a Long from its little endian byte representation. */ fromBytesBE( bytes: number[], unsigned?: boolean ): Long; /** * Tests if the specified object is a Long. */ isLong( obj: any ): obj is Long; /** * Converts the specified value to a Long. */ fromValue( val: Long | number | string | {low: number, high: number, unsigned: boolean} ): Long; } interface Long { /** * The high 32 bits as a signed value. */ high: number; /** * The low 32 bits as a signed value. */ low: number; /** * Whether unsigned or not. */ unsigned: boolean; /** * Returns the sum of this and the specified Long. */ add( addend: number | Long | string ): Long; /** * Returns the bitwise AND of this Long and the specified. */ and( other: Long | number | string ): Long; /** * Compares this Long's value with the specified's. */ compare( other: Long | number | string ): number; /** * Compares this Long's value with the specified's. */ comp( other: Long | number | string ): number; /** * Returns this Long divided by the specified. */ divide( divisor: Long | number | string ): Long; /** * Returns this Long divided by the specified. */ div( divisor: Long | number | string ): Long; /** * Tests if this Long's value equals the specified's. */ equals( other: Long | number | string ): boolean; /** * Tests if this Long's value equals the specified's. */ eq( other: Long | number | string ): boolean; /** * Gets the high 32 bits as a signed integer. */ getHighBits(): number; /** * Gets the high 32 bits as an unsigned integer. */ getHighBitsUnsigned(): number; /** * Gets the low 32 bits as a signed integer. */ getLowBits(): number; /** * Gets the low 32 bits as an unsigned integer. */ getLowBitsUnsigned(): number; /** * Gets the number of bits needed to represent the absolute value of this Long. */ getNumBitsAbs(): number; /** * Tests if this Long's value is greater than the specified's. */ greaterThan( other: Long | number | string ): boolean; /** * Tests if this Long's value is greater than the specified's. */ gt( other: Long | number | string ): boolean; /** * Tests if this Long's value is greater than or equal the specified's. */ greaterThanOrEqual( other: Long | number | string ): boolean; /** * Tests if this Long's value is greater than or equal the specified's. */ gte( other: Long | number | string ): boolean; /** * Tests if this Long's value is even. */ isEven(): boolean; /** * Tests if this Long's value is negative. */ isNegative(): boolean; /** * Tests if this Long's value is odd. */ isOdd(): boolean; /** * Tests if this Long's value is positive. */ isPositive(): boolean; /** * Tests if this Long's value equals zero. */ isZero(): boolean; /** * Tests if this Long's value is less than the specified's. */ lessThan( other: Long | number | string ): boolean; /** * Tests if this Long's value is less than the specified's. */ lt( other: Long | number | string ): boolean; /** * Tests if this Long's value is less than or equal the specified's. */ lessThanOrEqual( other: Long | number | string ): boolean; /** * Tests if this Long's value is less than or equal the specified's. */ lte( other: Long | number | string ): boolean; /** * Returns this Long modulo the specified. */ modulo( other: Long | number | string ): Long; /** * Returns this Long modulo the specified. */ mod( other: Long | number | string ): Long; /** * Returns the product of this and the specified Long. */ multiply( multiplier: Long | number | string ): Long; /** * Returns the product of this and the specified Long. */ mul( multiplier: Long | number | string ): Long; /** * Negates this Long's value. */ negate(): Long; /** * Negates this Long's value. */ neg(): Long; /** * Returns the bitwise NOT of this Long. */ not(): Long; /** * Tests if this Long's value differs from the specified's. */ notEquals( other: Long | number | string ): boolean; /** * Tests if this Long's value differs from the specified's. */ neq( other: Long | number | string ): boolean; /** * Returns the bitwise OR of this Long and the specified. */ or( other: Long | number | string ): Long; /** * Returns this Long with bits shifted to the left by the given amount. */ shiftLeft( numBits: number | Long ): Long; /** * Returns this Long with bits shifted to the left by the given amount. */ shl( numBits: number | Long ): Long; /** * Returns this Long with bits arithmetically shifted to the right by the given amount. */ shiftRight( numBits: number | Long ): Long; /** * Returns this Long with bits arithmetically shifted to the right by the given amount. */ shr( numBits: number | Long ): Long; /** * Returns this Long with bits logically shifted to the right by the given amount. */ shiftRightUnsigned( numBits: number | Long ): Long; /** * Returns this Long with bits logically shifted to the right by the given amount. */ shru( numBits: number | Long ): Long; /** * Returns the difference of this and the specified Long. */ subtract( subtrahend: number | Long | string ): Long; /** * Returns the difference of this and the specified Long. */ sub( subtrahend: number | Long |string ): Long; /** * Converts the Long to a 32 bit integer, assuming it is a 32 bit integer. */ toInt(): number; /** * Converts the Long to a the nearest floating-point representation of this value (double, 53 bit mantissa). */ toNumber(): number; /** * Converts this Long to its byte representation. */ toBytes( le?: boolean ): number[]; /** * Converts this Long to its little endian byte representation. */ toBytesLE(): number[]; /** * Converts this Long to its big endian byte representation. */ toBytesBE(): number[]; /** * Converts this Long to signed. */ toSigned(): Long; /** * Converts the Long to a string written in the specified radix. */ toString( radix?: number ): string; /** * Converts this Long to unsigned. */ toUnsigned(): Long; /** * Returns the bitwise XOR of this Long and the given one. */ xor( other: Long | number | string ): Long; } } apollo-server-demo/node_modules/@types/long/README.md 0000644 0001750 0000144 00000000755 13612120122 022106 0 ustar andreh users # Installation > `npm install --save @types/long` # Summary This package contains type definitions for long.js (https://github.com/dcodeIO/long.js). # Details Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/long. ### Additional Details * Last updated: Wed, 22 Jan 2020 19:19:46 GMT * Dependencies: none * Global values: `Long` # Credits These definitions were written by Peter Kooijmans (https://github.com/peterkooijmans). apollo-server-demo/node_modules/@types/long/package.json 0000644 0001750 0000144 00000001623 13612120122 023110 0 ustar andreh users { "name": "@types/long", "version": "4.0.1", "description": "TypeScript definitions for long.js", "license": "MIT", "contributors": [ { "name": "Peter Kooijmans", "url": "https://github.com/peterkooijmans", "githubUsername": "peterkooijmans" } ], "main": "", "types": "index.d.ts", "repository": { "type": "git", "url": "https://github.com/DefinitelyTyped/DefinitelyTyped.git", "directory": "types/long" }, "scripts": {}, "dependencies": {}, "typesPublisherContentHash": "5a2ae1424989c49d7303e1f5cc510288bfab1e71e0e2143cdcb9d24ff1c3dc8e", "typeScriptVersion": "2.8" ,"_resolved": "https://registry.npmjs.org/@types/long/-/long-4.0.1.tgz" ,"_integrity": "sha512-5tXH6Bx/kNGd3MgffdmP4dy2Z+G4eaXw0SE81Tq3BNadtnMR5/ySMzX4SLEzHJzSmPNn4HIdpQsBvXMUykr58w==" ,"_from": "@types/long@4.0.1" } apollo-server-demo/node_modules/@types/range-parser/ 0000755 0001750 0000144 00000000000 14067647700 022274 5 ustar andreh users apollo-server-demo/node_modules/@types/range-parser/LICENSE 0000644 0001750 0000144 00000002237 13402544120 023265 0 ustar andreh users MIT License Copyright (c) Microsoft Corporation. All rights reserved. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE apollo-server-demo/node_modules/@types/range-parser/index.d.ts 0000644 0001750 0000144 00000002310 13402544120 024151 0 ustar andreh users // Type definitions for range-parser 1.2 // Project: https://github.com/jshttp/range-parser // Definitions by: Tomek Åaziuk <https://github.com/tlaziuk> // Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped /** * When ranges are returned, the array has a "type" property which is the type of * range that is required (most commonly, "bytes"). Each array element is an object * with a "start" and "end" property for the portion of the range. * * @returns `-1` when unsatisfiable and `-2` when syntactically invalid, ranges otherwise. */ declare function RangeParser(size: number, str: string, options?: RangeParser.Options): RangeParser.Result | RangeParser.Ranges; declare namespace RangeParser { interface Ranges extends Array<Range> { type: string; } interface Range { start: number; end: number; } interface Options { /** * The "combine" option can be set to `true` and overlapping & adjacent ranges * will be combined into a single range. */ combine?: boolean; } type ResultUnsatisfiable = -1; type ResultInvalid = -2; type Result = ResultUnsatisfiable | ResultInvalid; } export = RangeParser; apollo-server-demo/node_modules/@types/range-parser/README.md 0000644 0001750 0000144 00000000766 13402544120 023544 0 ustar andreh users # Installation > `npm install --save @types/range-parser` # Summary This package contains type definitions for range-parser (https://github.com/jshttp/range-parser). # Details Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/range-parser Additional Details * Last updated: Fri, 07 Dec 2018 19:21:52 GMT * Dependencies: none * Global values: none # Credits These definitions were written by Tomek Åaziuk <https://github.com/tlaziuk>. apollo-server-demo/node_modules/@types/range-parser/package.json 0000644 0001750 0000144 00000001600 13402544120 024537 0 ustar andreh users { "name": "@types/range-parser", "version": "1.2.3", "description": "TypeScript definitions for range-parser", "license": "MIT", "contributors": [ { "name": "Tomek Åaziuk", "url": "https://github.com/tlaziuk", "githubUsername": "tlaziuk" } ], "main": "", "types": "index", "repository": { "type": "git", "url": "https://github.com/DefinitelyTyped/DefinitelyTyped.git" }, "scripts": {}, "dependencies": {}, "typesPublisherContentHash": "71bf3049d2d484657017f768fe0c54c4e9f03ee340b5a62a56523455925a00ae", "typeScriptVersion": "2.0" ,"_resolved": "https://registry.npmjs.org/@types/range-parser/-/range-parser-1.2.3.tgz" ,"_integrity": "sha512-ewFXqrQHlFsgc09MK5jP5iR7vumV/BYayNC6PgJO2LPe8vrnNFyjQjSppfEngITi0qvfKtzFvgKymGheFM9UOA==" ,"_from": "@types/range-parser@1.2.3" } apollo-server-demo/node_modules/@types/content-disposition/ 0000755 0001750 0000144 00000000000 14067647701 023723 5 ustar andreh users apollo-server-demo/node_modules/@types/content-disposition/LICENSE 0000644 0001750 0000144 00000002237 13640741422 024723 0 ustar andreh users MIT License Copyright (c) Microsoft Corporation. All rights reserved. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE apollo-server-demo/node_modules/@types/content-disposition/index.d.ts 0000644 0001750 0000144 00000004042 13640741422 025613 0 ustar andreh users // Type definitions for content-disposition 0.5 // Project: https://github.com/jshttp/content-disposition // Definitions by: Stefan Reichel <https://github.com/bomret> // Piotr BÅ‚ażejewicz <https://github.com/peterblazejewicz> // Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped declare namespace contentDisposition { /** * Class for parsed Content-Disposition header for v8 optimization */ interface ContentDisposition { /** * The disposition type (always lower case) */ type: 'attachment' | 'inline' | string; /** * An object of the parameters in the disposition * (name of parameter always lower case and extended versions replace non-extended versions) */ parameters: any; } interface Options { /** * Specifies the disposition type. * This can also be "inline", or any other value (all values except `inline` are treated like attachment, * but can convey additional information if both parties agree to it). * The `type` is normalized to lower-case. * @default 'attachment' */ type?: 'attachment' | 'inline' | string; /** * If the filename option is outside ISO-8859-1, * then the file name is actually stored in a supplemental field for clients * that support Unicode file names and a ISO-8859-1 version of the file name is automatically generated * @default true */ fallback?: string | boolean; } /** * Parse a Content-Disposition header string */ function parse(contentDispositionHeader: string): ContentDisposition; } /** * Create an attachment `Content-Disposition` header value using the given file name, if supplied. * The `filename` is optional and if no file name is desired, but you want to specify options, set `filename` to undefined. */ declare function contentDisposition(filename?: string, options?: contentDisposition.Options): string; export = contentDisposition; apollo-server-demo/node_modules/@types/content-disposition/README.md 0000644 0001750 0000144 00000001130 13640741422 025164 0 ustar andreh users # Installation > `npm install --save @types/content-disposition` # Summary This package contains type definitions for content-disposition (https://github.com/jshttp/content-disposition). # Details Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/content-disposition. ### Additional Details * Last updated: Tue, 31 Mar 2020 22:24:18 GMT * Dependencies: none * Global values: none # Credits These definitions were written by [Stefan Reichel](https://github.com/bomret), and [Piotr BÅ‚ażejewicz](https://github.com/peterblazejewicz). apollo-server-demo/node_modules/@types/content-disposition/package.json 0000644 0001750 0000144 00000002204 13640741422 026176 0 ustar andreh users { "name": "@types/content-disposition", "version": "0.5.3", "description": "TypeScript definitions for content-disposition", "license": "MIT", "contributors": [ { "name": "Stefan Reichel", "url": "https://github.com/bomret", "githubUsername": "bomret" }, { "name": "Piotr BÅ‚ażejewicz", "url": "https://github.com/peterblazejewicz", "githubUsername": "peterblazejewicz" } ], "main": "", "types": "index.d.ts", "repository": { "type": "git", "url": "https://github.com/DefinitelyTyped/DefinitelyTyped.git", "directory": "types/content-disposition" }, "scripts": {}, "dependencies": {}, "typesPublisherContentHash": "713abf3c213aa73aac34682a2a9f500cc7cdf72fdd23e8c027d63e42fbc3e494", "typeScriptVersion": "2.8" ,"_resolved": "https://registry.npmjs.org/@types/content-disposition/-/content-disposition-0.5.3.tgz" ,"_integrity": "sha512-P1bffQfhD3O4LW0ioENXUhZ9OIa0Zn+P7M+pWgkCKaT53wVLSq0mrKksCID/FGHpFhRSxRGhgrQmfhRuzwtKdg==" ,"_from": "@types/content-disposition@0.5.3" } apollo-server-demo/node_modules/@types/node-fetch/ 0000755 0001750 0000144 00000000000 14067647701 021723 5 ustar andreh users apollo-server-demo/node_modules/@types/node-fetch/LICENSE 0000644 0001750 0000144 00000002165 13650366362 022732 0 ustar andreh users MIT License Copyright (c) Microsoft Corporation. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE apollo-server-demo/node_modules/@types/node-fetch/externals.d.ts 0000644 0001750 0000144 00000001322 13650366362 024516 0 ustar andreh users // `AbortSignal` is defined here to prevent a dependency on a particular // implementation like the `abort-controller` package, and to avoid requiring // the `dom` library in `tsconfig.json`. export interface AbortSignal { aborted: boolean; addEventListener: (type: "abort", listener: ((this: AbortSignal, event: any) => any), options?: boolean | { capture?: boolean, once?: boolean, passive?: boolean }) => void; removeEventListener: (type: "abort", listener: ((this: AbortSignal, event: any) => any), options?: boolean | { capture?: boolean }) => void; dispatchEvent: (event: any) => boolean; onabort?: null | ((this: AbortSignal, event: any) => void); } apollo-server-demo/node_modules/@types/node-fetch/index.d.ts 0000644 0001750 0000144 00000014016 13650366362 023624 0 ustar andreh users // Type definitions for node-fetch 2.5 // Project: https://github.com/bitinn/node-fetch // Definitions by: Torsten Werner <https://github.com/torstenwerner> // Niklas Lindgren <https://github.com/nikcorg> // Vinay Bedre <https://github.com/vinaybedre> // Antonio Román <https://github.com/kyranet> // Andrew Leedham <https://github.com/AndrewLeedham> // Jason Li <https://github.com/JasonLi914> // Brandon Wilson <https://github.com/wilsonianb> // Steve Faulkner <https://github.com/southpolesteve> // ExE Boss <https://github.com/ExE-Boss> // Alex Savin <https://github.com/alexandrusavin> // Alexis Tyler <https://github.com/OmgImAlexis> // Jakub Kisielewski <https://github.com/kbkk> // Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped /// <reference types="node" /> import FormData = require('form-data'); import { Agent } from "http"; import { URLSearchParams, URL } from "url"; import { AbortSignal } from "./externals"; export class Request extends Body { constructor(input: RequestInfo, init?: RequestInit); clone(): Request; context: RequestContext; headers: Headers; method: string; redirect: RequestRedirect; referrer: string; url: string; // node-fetch extensions to the whatwg/fetch spec agent?: Agent | ((parsedUrl: URL) => Agent); compress: boolean; counter: number; follow: number; hostname: string; port?: number; protocol: string; size: number; timeout: number; } export interface RequestInit { // whatwg/fetch standard options body?: BodyInit; headers?: HeadersInit; method?: string; redirect?: RequestRedirect; signal?: AbortSignal | null; // node-fetch extensions agent?: Agent | ((parsedUrl: URL) => Agent); // =null http.Agent instance, allows custom proxy, certificate etc. compress?: boolean; // =true support gzip/deflate content encoding. false to disable follow?: number; // =20 maximum redirect count. 0 to not follow redirect size?: number; // =0 maximum response body size in bytes. 0 to disable timeout?: number; // =0 req/res timeout in ms, it resets on redirect. 0 to disable (OS limit applies) // node-fetch does not support mode, cache or credentials options } export type RequestContext = "audio" | "beacon" | "cspreport" | "download" | "embed" | "eventsource" | "favicon" | "fetch" | "font" | "form" | "frame" | "hyperlink" | "iframe" | "image" | "imageset" | "import" | "internal" | "location" | "manifest" | "object" | "ping" | "plugin" | "prefetch" | "script" | "serviceworker" | "sharedworker" | "style" | "subresource" | "track" | "video" | "worker" | "xmlhttprequest" | "xslt"; export type RequestMode = "cors" | "no-cors" | "same-origin"; export type RequestRedirect = "error" | "follow" | "manual"; export type RequestCredentials = "omit" | "include" | "same-origin"; export type RequestCache = "default" | "force-cache" | "no-cache" | "no-store" | "only-if-cached" | "reload"; export class Headers implements Iterable<[string, string]> { constructor(init?: HeadersInit); forEach(callback: (value: string, name: string) => void): void; append(name: string, value: string): void; delete(name: string): void; get(name: string): string | null; has(name: string): boolean; raw(): { [k: string]: string[] }; set(name: string, value: string): void; // Iterable methods entries(): IterableIterator<[string, string]>; keys(): IterableIterator<string>; values(): IterableIterator<[string]>; [Symbol.iterator](): Iterator<[string, string]>; } type BlobPart = ArrayBuffer | ArrayBufferView | Blob | string; interface BlobOptions { type?: string; endings?: "transparent" | "native"; } export class Blob { constructor(blobParts?: BlobPart[], options?: BlobOptions); readonly type: string; readonly size: number; slice(start?: number, end?: number): Blob; } export class Body { constructor(body?: any, opts?: { size?: number; timeout?: number }); arrayBuffer(): Promise<ArrayBuffer>; blob(): Promise<Blob>; body: NodeJS.ReadableStream; bodyUsed: boolean; buffer(): Promise<Buffer>; json(): Promise<any>; size: number; text(): Promise<string>; textConverted(): Promise<string>; timeout: number; } interface SystemError extends Error { code?: string; } export class FetchError extends Error { name: "FetchError"; constructor(message: string, type: string, systemError?: SystemError); type: string; code?: string; errno?: string; } export class Response extends Body { constructor(body?: BodyInit, init?: ResponseInit); static error(): Response; static redirect(url: string, status: number): Response; clone(): Response; headers: Headers; ok: boolean; redirected: boolean; status: number; statusText: string; type: ResponseType; url: string; } export type ResponseType = "basic" | "cors" | "default" | "error" | "opaque" | "opaqueredirect"; export interface ResponseInit { headers?: HeadersInit; size?: number; status?: number; statusText?: string; timeout?: number; url?: string; } interface URLLike { href: string; } export type HeadersInit = Headers | string[][] | { [key: string]: string }; // HeaderInit is exported to support backwards compatibility. See PR #34382 export type HeaderInit = HeadersInit; export type BodyInit = ArrayBuffer | ArrayBufferView | NodeJS.ReadableStream | string | URLSearchParams | FormData; export type RequestInfo = string | URLLike | Request; declare function fetch( url: RequestInfo, init?: RequestInit ): Promise<Response>; declare namespace fetch { function isRedirect(code: number): boolean; } export default fetch; apollo-server-demo/node_modules/@types/node-fetch/README.md 0000644 0001750 0000144 00000002167 13650366362 023206 0 ustar andreh users # Installation > `npm install --save @types/node-fetch` # Summary This package contains type definitions for node-fetch (https://github.com/bitinn/node-fetch). # Details Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/node-fetch. ### Additional Details * Last updated: Thu, 23 Apr 2020 19:30:58 GMT * Dependencies: [@types/form-data](https://npmjs.com/package/@types/form-data), [@types/node](https://npmjs.com/package/@types/node) * Global values: none # Credits These definitions were written by [Torsten Werner](https://github.com/torstenwerner), [Niklas Lindgren](https://github.com/nikcorg), [Vinay Bedre](https://github.com/vinaybedre), [Antonio Román](https://github.com/kyranet), [Andrew Leedham](https://github.com/AndrewLeedham), [Jason Li](https://github.com/JasonLi914), [Brandon Wilson](https://github.com/wilsonianb), [Steve Faulkner](https://github.com/southpolesteve), [ExE Boss](https://github.com/ExE-Boss), [Alex Savin](https://github.com/alexandrusavin), [Alexis Tyler](https://github.com/OmgImAlexis), and [Jakub Kisielewski](https://github.com/kbkk). apollo-server-demo/node_modules/@types/node-fetch/package.json 0000644 0001750 0000144 00000005171 13650366362 024213 0 ustar andreh users { "name": "@types/node-fetch", "version": "2.5.7", "description": "TypeScript definitions for node-fetch", "license": "MIT", "contributors": [ { "name": "Torsten Werner", "url": "https://github.com/torstenwerner", "githubUsername": "torstenwerner" }, { "name": "Niklas Lindgren", "url": "https://github.com/nikcorg", "githubUsername": "nikcorg" }, { "name": "Vinay Bedre", "url": "https://github.com/vinaybedre", "githubUsername": "vinaybedre" }, { "name": "Antonio Román", "url": "https://github.com/kyranet", "githubUsername": "kyranet" }, { "name": "Andrew Leedham", "url": "https://github.com/AndrewLeedham", "githubUsername": "AndrewLeedham" }, { "name": "Jason Li", "url": "https://github.com/JasonLi914", "githubUsername": "JasonLi914" }, { "name": "Brandon Wilson", "url": "https://github.com/wilsonianb", "githubUsername": "wilsonianb" }, { "name": "Steve Faulkner", "url": "https://github.com/southpolesteve", "githubUsername": "southpolesteve" }, { "name": "ExE Boss", "url": "https://github.com/ExE-Boss", "githubUsername": "ExE-Boss" }, { "name": "Alex Savin", "url": "https://github.com/alexandrusavin", "githubUsername": "alexandrusavin" }, { "name": "Alexis Tyler", "url": "https://github.com/OmgImAlexis", "githubUsername": "OmgImAlexis" }, { "name": "Jakub Kisielewski", "url": "https://github.com/kbkk", "githubUsername": "kbkk" } ], "main": "", "types": "index.d.ts", "repository": { "type": "git", "url": "https://github.com/DefinitelyTyped/DefinitelyTyped.git", "directory": "types/node-fetch" }, "scripts": {}, "dependencies": { "@types/node": "*", "form-data": "^3.0.0" }, "typesPublisherContentHash": "108c44e190ea37e4618fa6c4d6835aedecb20ddd8433a81984ee5cdfdd915b01", "typeScriptVersion": "2.8" ,"_resolved": "https://registry.npmjs.org/@types/node-fetch/-/node-fetch-2.5.7.tgz" ,"_integrity": "sha512-o2WVNf5UhWRkxlf6eq+jMZDu7kjgpgJfl4xVNlvryc95O/6F2ld8ztKX+qu+Rjyet93WAWm5LjeX9H5FGkODvw==" ,"_from": "@types/node-fetch@2.5.7" } apollo-server-demo/node_modules/@types/mime/ 0000755 0001750 0000144 00000000000 14067647700 020635 5 ustar andreh users apollo-server-demo/node_modules/@types/mime/LICENSE 0000644 0001750 0000144 00000002165 14001315757 021636 0 ustar andreh users MIT License Copyright (c) Microsoft Corporation. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE apollo-server-demo/node_modules/@types/mime/index.d.ts 0000644 0001750 0000144 00000002073 14001315757 022530 0 ustar andreh users // Type definitions for mime 1.3 // Project: https://github.com/broofa/node-mime // Definitions by: Jeff Goddard <https://github.com/jedigo> // Daniel Hritzkiv <https://github.com/dhritzkiv> // Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped // Originally imported from: https://github.com/soywiz/typescript-node-definitions/mime.d.ts export as namespace mime; export interface TypeMap { [key: string]: string[]; } /** * Look up a mime type based on extension. * * If not found, uses the fallback argument if provided, and otherwise * uses `default_type`. */ export function lookup(path: string, fallback?: string): string; /** * Return a file extensions associated with a mime type. */ export function extension(mime: string): string | undefined; /** * Load an Apache2-style ".types" file. */ export function load(filepath: string): void; export function define(mimes: TypeMap): void; export interface Charsets { lookup(mime: string, fallback: string): string; } export const charsets: Charsets; export const default_type: string; apollo-server-demo/node_modules/@types/mime/README.md 0000644 0001750 0000144 00000001045 14001315757 022104 0 ustar andreh users # Installation > `npm install --save @types/mime` # Summary This package contains type definitions for mime (https://github.com/broofa/node-mime). # Details Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/mime/v1. ### Additional Details * Last updated: Mon, 18 Jan 2021 14:32:15 GMT * Dependencies: none * Global values: `mime`, `mimelite` # Credits These definitions were written by [Jeff Goddard](https://github.com/jedigo), and [Daniel Hritzkiv](https://github.com/dhritzkiv). apollo-server-demo/node_modules/@types/mime/lite.d.ts 0000644 0001750 0000144 00000000174 14001315757 022356 0 ustar andreh users import { default as Mime } from "./Mime"; declare const mimelite: Mime; export as namespace mimelite; export = mimelite; apollo-server-demo/node_modules/@types/mime/package.json 0000644 0001750 0000144 00000002026 14001315757 023113 0 ustar andreh users { "name": "@types/mime", "version": "1.3.2", "description": "TypeScript definitions for mime", "license": "MIT", "contributors": [ { "name": "Jeff Goddard", "url": "https://github.com/jedigo", "githubUsername": "jedigo" }, { "name": "Daniel Hritzkiv", "url": "https://github.com/dhritzkiv", "githubUsername": "dhritzkiv" } ], "main": "", "types": "index.d.ts", "repository": { "type": "git", "url": "https://github.com/DefinitelyTyped/DefinitelyTyped.git", "directory": "types/mime" }, "scripts": {}, "dependencies": {}, "typesPublisherContentHash": "529a2ee85950b588c079bd053520c5b3c2bbff1886870bc08188284265324348", "typeScriptVersion": "3.4" ,"_resolved": "https://registry.npmjs.org/@types/mime/-/mime-1.3.2.tgz" ,"_integrity": "sha512-YATxVxgRqNH6nHEIsvg6k2Boc1JHI9ZbH5iWFFv/MTkchz3b1ieGDa5T0a9RznNdI0KhVbdbWSN+KWWrQZRxTw==" ,"_from": "@types/mime@1.3.2" } apollo-server-demo/node_modules/@types/mime/Mime.d.ts 0000644 0001750 0000144 00000000416 14001315757 022307 0 ustar andreh users import { TypeMap } from "./index"; export default class Mime { constructor(mimes: TypeMap); lookup(path: string, fallback?: string): string; extension(mime: string): string | undefined; load(filepath: string): void; define(mimes: TypeMap): void; } apollo-server-demo/node_modules/@types/http-errors/ 0000755 0001750 0000144 00000000000 14067647701 022200 5 ustar andreh users apollo-server-demo/node_modules/@types/http-errors/LICENSE 0000644 0001750 0000144 00000002165 13702074057 023202 0 ustar andreh users MIT License Copyright (c) Microsoft Corporation. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE apollo-server-demo/node_modules/@types/http-errors/index.d.ts 0000644 0001750 0000144 00000005703 13702074057 024077 0 ustar andreh users // Type definitions for http-errors 1.8 // Project: https://github.com/jshttp/http-errors // Definitions by: Tanguy Krotoff <https://github.com/tkrotoff> // BendingBender <https://github.com/BendingBender> // Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped // TypeScript Version: 2.3 export = createHttpError; declare const createHttpError: createHttpError.CreateHttpError & createHttpError.NamedConstructors & { isHttpError: createHttpError.IsHttpError }; declare namespace createHttpError { interface HttpError extends Error { status: number; statusCode: number; expose: boolean; headers?: { [key: string]: string; }; [key: string]: any; } type UnknownError = Error | string | number | { [key: string]: any }; type HttpErrorConstructor = new (msg?: string) => HttpError; type CreateHttpError = (...args: UnknownError[]) => HttpError; type IsHttpError = (error: unknown) => error is HttpError; type NamedConstructors = { [code: string]: HttpErrorConstructor; HttpError: HttpErrorConstructor; } & Record<'BadRequest' | 'Unauthorized' | 'PaymentRequired' | 'Forbidden' | 'NotFound' | 'MethodNotAllowed' | 'NotAcceptable' | 'ProxyAuthenticationRequired' | 'RequestTimeout' | 'Conflict' | 'Gone' | 'LengthRequired' | 'PreconditionFailed' | 'PayloadTooLarge' | 'URITooLong' | 'UnsupportedMediaType' | 'RangeNotSatisfiable' | 'ExpectationFailed' | 'ImATeapot' | 'MisdirectedRequest' | 'UnprocessableEntity' | 'Locked' | 'FailedDependency' | 'UnorderedCollection' | 'UpgradeRequired' | 'PreconditionRequired' | 'TooManyRequests' | 'RequestHeaderFieldsTooLarge' | 'UnavailableForLegalReasons' | 'InternalServerError' | 'NotImplemented' | 'BadGateway' | 'ServiceUnavailable' | 'GatewayTimeout' | 'HTTPVersionNotSupported' | 'VariantAlsoNegotiates' | 'InsufficientStorage' | 'LoopDetected' | 'BandwidthLimitExceeded' | 'NotExtended' | 'NetworkAuthenticationRequire' | '400' | '401' | '402' | '403' | '404' | '405' | '406' | '407' | '408' | '409' | '410' | '411' | '412' | '413' | '414' | '415' | '416' | '417' | '418' | '421' | '422' | '423' | '424' | '425' | '426' | '428' | '429' | '431' | '451' | '500' | '501' | '502' | '503' | '504' | '505' | '506' | '507' | '508' | '509' | '510' | '511', HttpErrorConstructor>; } apollo-server-demo/node_modules/@types/http-errors/README.md 0000644 0001750 0000144 00000001061 13702074057 023446 0 ustar andreh users # Installation > `npm install --save @types/http-errors` # Summary This package contains type definitions for http-errors (https://github.com/jshttp/http-errors). # Details Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/http-errors. ### Additional Details * Last updated: Fri, 10 Jul 2020 14:16:15 GMT * Dependencies: none * Global values: none # Credits These definitions were written by [Tanguy Krotoff](https://github.com/tkrotoff), and [BendingBender](https://github.com/BendingBender). apollo-server-demo/node_modules/@types/http-errors/package.json 0000644 0001750 0000144 00000002114 13702074057 024455 0 ustar andreh users { "name": "@types/http-errors", "version": "1.8.0", "description": "TypeScript definitions for http-errors", "license": "MIT", "contributors": [ { "name": "Tanguy Krotoff", "url": "https://github.com/tkrotoff", "githubUsername": "tkrotoff" }, { "name": "BendingBender", "url": "https://github.com/BendingBender", "githubUsername": "BendingBender" } ], "main": "", "types": "index.d.ts", "repository": { "type": "git", "url": "https://github.com/DefinitelyTyped/DefinitelyTyped.git", "directory": "types/http-errors" }, "scripts": {}, "dependencies": {}, "typesPublisherContentHash": "61c68cc213bf1a21d07bfac01c18754adea53b40a66a043c89fb6a35957ac0c9", "typeScriptVersion": "3.0" ,"_resolved": "https://registry.npmjs.org/@types/http-errors/-/http-errors-1.8.0.tgz" ,"_integrity": "sha512-2aoSC4UUbHDj2uCsCxcG/vRMXey/m17bC7UwitVm5hn22nI8O8Y9iDpA76Orc+DWkQ4zZrOKEshCqR/jSuXAHA==" ,"_from": "@types/http-errors@1.8.0" } apollo-server-demo/node_modules/@types/koa/ 0000755 0001750 0000144 00000000000 14067647701 020461 5 ustar andreh users apollo-server-demo/node_modules/@types/koa/LICENSE 0000644 0001750 0000144 00000002165 13743574742 021476 0 ustar andreh users MIT License Copyright (c) Microsoft Corporation. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE apollo-server-demo/node_modules/@types/koa/index.d.ts 0000644 0001750 0000144 00000047211 13743574742 022373 0 ustar andreh users // Type definitions for Koa 2.11.0 // Project: http://koajs.com // Definitions by: DavidCai1993 <https://github.com/DavidCai1993> // jKey Lu <https://github.com/jkeylu> // Brice Bernard <https://github.com/brikou> // harryparkdotio <https://github.com/harryparkdotio> // Wooram Jun <https://github.com/chatoo2412> // Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped // TypeScript Version: 2.3 /* =================== USAGE =================== import * as Koa from "koa" const app = new Koa() async function (ctx: Koa.Context, next: Koa.Next) { // ... } =============================================== */ /// <reference types="node" /> import * as accepts from 'accepts'; import * as Cookies from 'cookies'; import { EventEmitter } from 'events'; import { IncomingMessage, ServerResponse, Server } from 'http'; import { Http2ServerRequest, Http2ServerResponse } from 'http2'; import httpAssert = require('http-assert'); import * as HttpErrors from 'http-errors'; import * as Keygrip from 'keygrip'; import * as compose from 'koa-compose'; import { Socket, ListenOptions } from 'net'; import * as url from 'url'; import * as contentDisposition from 'content-disposition'; declare interface ContextDelegatedRequest { /** * Return request header. */ header: any; /** * Return request header, alias as request.header */ headers: any; /** * Get/Set request URL. */ url: string; /** * Get origin of URL. */ origin: string; /** * Get full request URL. */ href: string; /** * Get/Set request method. */ method: string; /** * Get request pathname. * Set pathname, retaining the query-string when present. */ path: string; /** * Get parsed query-string. * Set query-string as an object. */ query: any; /** * Get/Set query string. */ querystring: string; /** * Get the search string. Same as the querystring * except it includes the leading ?. * * Set the search string. Same as * response.querystring= but included for ubiquity. */ search: string; /** * Parse the "Host" header field host * and support X-Forwarded-Host when a * proxy is enabled. */ host: string; /** * Parse the "Host" header field hostname * and support X-Forwarded-Host when a * proxy is enabled. */ hostname: string; /** * Get WHATWG parsed URL object. */ URL: url.URL; /** * Check if the request is fresh, aka * Last-Modified and/or the ETag * still match. */ fresh: boolean; /** * Check if the request is stale, aka * "Last-Modified" and / or the "ETag" for the * resource has changed. */ stale: boolean; /** * Check if the request is idempotent. */ idempotent: boolean; /** * Return the request socket. */ socket: Socket; /** * Return the protocol string "http" or "https" * when requested with TLS. When the proxy setting * is enabled the "X-Forwarded-Proto" header * field will be trusted. If you're running behind * a reverse proxy that supplies https for you this * may be enabled. */ protocol: string; /** * Short-hand for: * * this.protocol == 'https' */ secure: boolean; /** * Request remote address. Supports X-Forwarded-For when app.proxy is true. */ ip: string; /** * When `app.proxy` is `true`, parse * the "X-Forwarded-For" ip address list. * * For example if the value were "client, proxy1, proxy2" * you would receive the array `["client", "proxy1", "proxy2"]` * where "proxy2" is the furthest down-stream. */ ips: string[]; /** * Return subdomains as an array. * * Subdomains are the dot-separated parts of the host before the main domain * of the app. By default, the domain of the app is assumed to be the last two * parts of the host. This can be changed by setting `app.subdomainOffset`. * * For example, if the domain is "tobi.ferrets.example.com": * If `app.subdomainOffset` is not set, this.subdomains is * `["ferrets", "tobi"]`. * If `app.subdomainOffset` is 3, this.subdomains is `["tobi"]`. */ subdomains: string[]; /** * Check if the given `type(s)` is acceptable, returning * the best match when true, otherwise `undefined`, in which * case you should respond with 406 "Not Acceptable". * * The `type` value may be a single mime type string * such as "application/json", the extension name * such as "json" or an array `["json", "html", "text/plain"]`. When a list * or array is given the _best_ match, if any is returned. * * Examples: * * // Accept: text/html * this.accepts('html'); * // => "html" * * // Accept: text/*, application/json * this.accepts('html'); * // => "html" * this.accepts('text/html'); * // => "text/html" * this.accepts('json', 'text'); * // => "json" * this.accepts('application/json'); * // => "application/json" * * // Accept: text/*, application/json * this.accepts('image/png'); * this.accepts('png'); * // => undefined * * // Accept: text/*;q=.5, application/json * this.accepts(['html', 'json']); * this.accepts('html', 'json'); * // => "json" */ accepts(): string[] | boolean; accepts(...types: string[]): string | boolean; accepts(types: string[]): string | boolean; /** * Return accepted encodings or best fit based on `encodings`. * * Given `Accept-Encoding: gzip, deflate` * an array sorted by quality is returned: * * ['gzip', 'deflate'] */ acceptsEncodings(): string[] | boolean; acceptsEncodings(...encodings: string[]): string | boolean; acceptsEncodings(encodings: string[]): string | boolean; /** * Return accepted charsets or best fit based on `charsets`. * * Given `Accept-Charset: utf-8, iso-8859-1;q=0.2, utf-7;q=0.5` * an array sorted by quality is returned: * * ['utf-8', 'utf-7', 'iso-8859-1'] */ acceptsCharsets(): string[] | boolean; acceptsCharsets(...charsets: string[]): string | boolean; acceptsCharsets(charsets: string[]): string | boolean; /** * Return accepted languages or best fit based on `langs`. * * Given `Accept-Language: en;q=0.8, es, pt` * an array sorted by quality is returned: * * ['es', 'pt', 'en'] */ acceptsLanguages(): string[] | boolean; acceptsLanguages(...langs: string[]): string | boolean; acceptsLanguages(langs: string[]): string | boolean; /** * Check if the incoming request contains the "Content-Type" * header field, and it contains any of the give mime `type`s. * If there is no request body, `null` is returned. * If there is no content type, `false` is returned. * Otherwise, it returns the first `type` that matches. * * Examples: * * // With Content-Type: text/html; charset=utf-8 * this.is('html'); // => 'html' * this.is('text/html'); // => 'text/html' * this.is('text/*', 'application/json'); // => 'text/html' * * // When Content-Type is application/json * this.is('json', 'urlencoded'); // => 'json' * this.is('application/json'); // => 'application/json' * this.is('html', 'application/*'); // => 'application/json' * * this.is('html'); // => false */ // is(): string | boolean; is(...types: string[]): string | boolean; is(types: string[]): string | boolean; /** * Return request header. If the header is not set, will return an empty * string. * * The `Referrer` header field is special-cased, both `Referrer` and * `Referer` are interchangeable. * * Examples: * * this.get('Content-Type'); * // => "text/plain" * * this.get('content-type'); * // => "text/plain" * * this.get('Something'); * // => '' */ get(field: string): string; } declare interface ContextDelegatedResponse { /** * Get/Set response status code. */ status: number; /** * Get response status message */ message: string; /** * Get/Set response body. */ body: any; /** * Return parsed response Content-Length when present. * Set Content-Length field to `n`. */ length: number; /** * Check if a header has been written to the socket. */ headerSent: boolean; /** * Vary on `field`. */ vary(field: string): void; /** * Perform a 302 redirect to `url`. * * The string "back" is special-cased * to provide Referrer support, when Referrer * is not present `alt` or "/" is used. * * Examples: * * this.redirect('back'); * this.redirect('back', '/index.html'); * this.redirect('/login'); * this.redirect('http://google.com'); */ redirect(url: string, alt?: string): void; /** * Set Content-Disposition to "attachment" to signal the client to prompt for download. * Optionally specify the filename of the download and some options. */ attachment(filename?: string, options?: contentDisposition.Options): void; /** * Return the response mime type void of * parameters such as "charset". * * Set Content-Type response header with `type` through `mime.lookup()` * when it does not contain a charset. * * Examples: * * this.type = '.html'; * this.type = 'html'; * this.type = 'json'; * this.type = 'application/json'; * this.type = 'png'; */ type: string; /** * Get the Last-Modified date in Date form, if it exists. * Set the Last-Modified date using a string or a Date. * * this.response.lastModified = new Date(); * this.response.lastModified = '2013-09-13'; */ lastModified: Date; /** * Get/Set the ETag of a response. * This will normalize the quotes if necessary. * * this.response.etag = 'md5hashsum'; * this.response.etag = '"md5hashsum"'; * this.response.etag = 'W/"123456789"'; * * @param {String} etag * @api public */ etag: string; /** * Set header `field` to `val`, or pass * an object of header fields. * * Examples: * * this.set('Foo', ['bar', 'baz']); * this.set('Accept', 'application/json'); * this.set({ Accept: 'text/plain', 'X-API-Key': 'tobi' }); */ set(field: { [key: string]: string | string[] }): void; set(field: string, val: string | string[]): void; /** * Append additional header `field` with value `val`. * * Examples: * * ``` * this.append('Link', ['<http://localhost/>', '<http://localhost:3000/>']); * this.append('Set-Cookie', 'foo=bar; Path=/; HttpOnly'); * this.append('Warning', '199 Miscellaneous warning'); * ``` */ append(field: string, val: string | string[]): void; /** * Remove header `field`. */ remove(field: string): void; /** * Checks if the request is writable. * Tests for the existence of the socket * as node sometimes does not set it. */ writable: boolean; /** * Flush any set headers, and begin the body */ flushHeaders(): void; } declare class Application< StateT = Application.DefaultState, CustomT = Application.DefaultContext > extends EventEmitter { proxy: boolean; proxyIpHeader: string; maxIpsCount: number; middleware: Application.Middleware<StateT, CustomT>[]; subdomainOffset: number; env: string; context: Application.BaseContext & CustomT; request: Application.BaseRequest; response: Application.BaseResponse; silent: boolean; keys: Keygrip | string[]; constructor(); /** * Shorthand for: * * http.createServer(app.callback()).listen(...) */ listen(port?: number, hostname?: string, backlog?: number, listeningListener?: () => void): Server; listen(port: number, hostname?: string, listeningListener?: () => void): Server; listen(port: number, backlog?: number, listeningListener?: () => void): Server; listen(port: number, listeningListener?: () => void): Server; listen(path: string, backlog?: number, listeningListener?: () => void): Server; listen(path: string, listeningListener?: () => void): Server; listen(options: ListenOptions, listeningListener?: () => void): Server; listen(handle: any, backlog?: number, listeningListener?: () => void): Server; listen(handle: any, listeningListener?: () => void): Server; /** * Return JSON representation. * We only bother showing settings. */ inspect(): any; /** * Return JSON representation. * We only bother showing settings. */ toJSON(): any; /** * Use the given middleware `fn`. * * Old-style middleware will be converted. */ use<NewStateT = {}, NewCustomT = {}>( middleware: Application.Middleware<StateT & NewStateT, CustomT & NewCustomT>, ): Application<StateT & NewStateT, CustomT & NewCustomT>; /** * Return a request handler callback * for node's native http/http2 server. */ callback(): (req: IncomingMessage | Http2ServerRequest, res: ServerResponse | Http2ServerResponse) => void; /** * Initialize a new context. * * @api private */ createContext<StateT = Application.DefaultState>( req: IncomingMessage, res: ServerResponse, ): Application.ParameterizedContext<StateT>; /** * Default error handler. * * @api private */ onerror(err: Error): void; } declare namespace Application { type DefaultStateExtends = any; /** * This interface can be augmented by users to add types to Koa's default state */ interface DefaultState extends DefaultStateExtends {} type DefaultContextExtends = {}; /** * This interface can be augmented by users to add types to Koa's default context */ interface DefaultContext extends DefaultContextExtends { /** * Custom properties. */ [key: string]: any; } type Middleware<StateT = DefaultState, CustomT = DefaultContext> = compose.Middleware< ParameterizedContext<StateT, CustomT> >; interface BaseRequest extends ContextDelegatedRequest { /** * Get the charset when present or undefined. */ charset: string; /** * Return parsed Content-Length when present. */ length: number; /** * Return the request mime type void of * parameters such as "charset". */ type: string; /** * Inspect implementation. */ inspect(): any; /** * Return JSON representation. */ toJSON(): any; } interface BaseResponse extends ContextDelegatedResponse { /** * Return the request socket. * * @return {Connection} * @api public */ socket: Socket; /** * Return response header. */ header: any; /** * Return response header, alias as response.header */ headers: any; /** * Check whether the response is one of the listed types. * Pretty much the same as `this.request.is()`. * * @param {String|Array} types... * @return {String|false} * @api public */ // is(): string; is(...types: string[]): string; is(types: string[]): string; /** * Return response header. If the header is not set, will return an empty * string. * * The `Referrer` header field is special-cased, both `Referrer` and * `Referer` are interchangeable. * * Examples: * * this.get('Content-Type'); * // => "text/plain" * * this.get('content-type'); * // => "text/plain" * * this.get('Something'); * // => '' */ get(field: string): string; /** * Inspect implementation. */ inspect(): any; /** * Return JSON representation. */ toJSON(): any; } interface BaseContext extends ContextDelegatedRequest, ContextDelegatedResponse { /** * util.inspect() implementation, which * just returns the JSON output. */ inspect(): any; /** * Return JSON representation. * * Here we explicitly invoke .toJSON() on each * object, as iteration will otherwise fail due * to the getters and cause utilities such as * clone() to fail. */ toJSON(): any; /** * Similar to .throw(), adds assertion. * * this.assert(this.user, 401, 'Please login!'); * * See: https://github.com/jshttp/http-assert */ assert: typeof httpAssert; /** * Throw an error with `msg` and optional `status` * defaulting to 500. Note that these are user-level * errors, and the message may be exposed to the client. * * this.throw(403) * this.throw('name required', 400) * this.throw(400, 'name required') * this.throw('something exploded') * this.throw(new Error('invalid'), 400); * this.throw(400, new Error('invalid')); * * See: https://github.com/jshttp/http-errors */ throw(message: string, code?: number, properties?: {}): never; throw(status: number): never; throw(...properties: Array<number | string | {}>): never; /** * Default error handling. */ onerror(err: Error): void; } interface Request extends BaseRequest { app: Application; req: IncomingMessage; res: ServerResponse; ctx: Context; response: Response; originalUrl: string; ip: string; accept: accepts.Accepts; } interface Response extends BaseResponse { app: Application; req: IncomingMessage; res: ServerResponse; ctx: Context; request: Request; } interface ExtendableContext extends BaseContext { app: Application; request: Request; response: Response; req: IncomingMessage; res: ServerResponse; originalUrl: string; cookies: Cookies; accept: accepts.Accepts; /** * To bypass Koa's built-in response handling, you may explicitly set `ctx.respond = false;` */ respond?: boolean; } type ParameterizedContext<StateT = DefaultState, CustomT = DefaultContext> = ExtendableContext & { state: StateT; } & CustomT; interface Context extends ParameterizedContext {} type Next = () => Promise<any>; /** * A re-export of `HttpError` from the `http-assert` package. * * This is the error type that is thrown by `ctx.assert()` and `ctx.throw()`. */ const HttpError: typeof HttpErrors.HttpError; } export = Application; apollo-server-demo/node_modules/@types/koa/README.md 0000644 0001750 0000144 00000002213 13743574742 021742 0 ustar andreh users # Installation > `npm install --save @types/koa` # Summary This package contains type definitions for Koa (http://koajs.com). # Details Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/koa. ### Additional Details * Last updated: Tue, 20 Oct 2020 14:53:22 GMT * Dependencies: [@types/accepts](https://npmjs.com/package/@types/accepts), [@types/cookies](https://npmjs.com/package/@types/cookies), [@types/http-assert](https://npmjs.com/package/@types/http-assert), [@types/http-errors](https://npmjs.com/package/@types/http-errors), [@types/keygrip](https://npmjs.com/package/@types/keygrip), [@types/koa-compose](https://npmjs.com/package/@types/koa-compose), [@types/content-disposition](https://npmjs.com/package/@types/content-disposition), [@types/node](https://npmjs.com/package/@types/node) * Global values: none # Credits These definitions were written by [DavidCai1993](https://github.com/DavidCai1993), [jKey Lu](https://github.com/jkeylu), [Brice Bernard](https://github.com/brikou), [harryparkdotio](https://github.com/harryparkdotio), and [Wooram Jun](https://github.com/chatoo2412). apollo-server-demo/node_modules/@types/koa/package.json 0000644 0001750 0000144 00000003353 13743574742 022757 0 ustar andreh users { "name": "@types/koa", "version": "2.11.6", "description": "TypeScript definitions for Koa", "license": "MIT", "contributors": [ { "name": "DavidCai1993", "url": "https://github.com/DavidCai1993", "githubUsername": "DavidCai1993" }, { "name": "jKey Lu", "url": "https://github.com/jkeylu", "githubUsername": "jkeylu" }, { "name": "Brice Bernard", "url": "https://github.com/brikou", "githubUsername": "brikou" }, { "name": "harryparkdotio", "url": "https://github.com/harryparkdotio", "githubUsername": "harryparkdotio" }, { "name": "Wooram Jun", "url": "https://github.com/chatoo2412", "githubUsername": "chatoo2412" } ], "main": "", "types": "index.d.ts", "repository": { "type": "git", "url": "https://github.com/DefinitelyTyped/DefinitelyTyped.git", "directory": "types/koa" }, "scripts": {}, "dependencies": { "@types/accepts": "*", "@types/content-disposition": "*", "@types/cookies": "*", "@types/http-assert": "*", "@types/http-errors": "*", "@types/keygrip": "*", "@types/koa-compose": "*", "@types/node": "*" }, "typesPublisherContentHash": "557190fbae4bd7927b9ac54a1ff3a370ea131d37defaacc6f8e5e03a4cd10e86", "typeScriptVersion": "3.2" ,"_resolved": "https://registry.npmjs.org/@types/koa/-/koa-2.11.6.tgz" ,"_integrity": "sha512-BhyrMj06eQkk04C97fovEDQMpLpd2IxCB4ecitaXwOKGq78Wi2tooaDOWOFGajPk8IkQOAtMppApgSVkYe1F/A==" ,"_from": "@types/koa@2.11.6" } apollo-server-demo/node_modules/@types/qs/ 0000755 0001750 0000144 00000000000 14067647700 020331 5 ustar andreh users apollo-server-demo/node_modules/@types/qs/LICENSE 0000644 0001750 0000144 00000002165 13730515101 021323 0 ustar andreh users MIT License Copyright (c) Microsoft Corporation. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE apollo-server-demo/node_modules/@types/qs/index.d.ts 0000644 0001750 0000144 00000005157 13730515101 022223 0 ustar andreh users // Type definitions for qs 6.9 // Project: https://github.com/ljharb/qs // Definitions by: Roman Korneev <https://github.com/RWander> // Leon Yu <https://github.com/leonyu> // Belinda Teh <https://github.com/tehbelinda> // Melvin Lee <https://github.com/zyml> // Arturs Vonda <https://github.com/artursvonda> // Carlos Bonetti <https://github.com/CarlosBonetti> // Dan Smith <https://github.com/dpsmith3> // Hunter Perrin <https://github.com/hperrin> // Jordan Harband <https://github.com/ljharb> // Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped export = QueryString; export as namespace qs; declare namespace QueryString { type defaultEncoder = (str: any, defaultEncoder?: any, charset?: string) => string; type defaultDecoder = (str: string, decoder?: any, charset?: string) => string; interface IStringifyOptions { delimiter?: string; strictNullHandling?: boolean; skipNulls?: boolean; encode?: boolean; encoder?: (str: any, defaultEncoder: defaultEncoder, charset: string, type: 'key' | 'value') => string; filter?: Array<string | number> | ((prefix: string, value: any) => any); arrayFormat?: 'indices' | 'brackets' | 'repeat' | 'comma'; indices?: boolean; sort?: (a: any, b: any) => number; serializeDate?: (d: Date) => string; format?: 'RFC1738' | 'RFC3986'; encodeValuesOnly?: boolean; addQueryPrefix?: boolean; allowDots?: boolean; charset?: 'utf-8' | 'iso-8859-1'; charsetSentinel?: boolean; } interface IParseOptions { comma?: boolean; delimiter?: string | RegExp; depth?: number | false; decoder?: (str: string, defaultDecoder: defaultDecoder, charset: string, type: 'key' | 'value') => any; arrayLimit?: number; parseArrays?: boolean; allowDots?: boolean; plainObjects?: boolean; allowPrototypes?: boolean; parameterLimit?: number; strictNullHandling?: boolean; ignoreQueryPrefix?: boolean; charset?: 'utf-8' | 'iso-8859-1'; charsetSentinel?: boolean; interpretNumericEntities?: boolean; } interface ParsedQs { [key: string]: undefined | string | string[] | ParsedQs | ParsedQs[] } function stringify(obj: any, options?: IStringifyOptions): string; function parse(str: string, options?: IParseOptions & { decoder?: never }): ParsedQs; function parse(str: string, options?: IParseOptions): { [key: string]: unknown }; } apollo-server-demo/node_modules/@types/qs/README.md 0000644 0001750 0000144 00000001473 13730515101 021576 0 ustar andreh users # Installation > `npm install --save @types/qs` # Summary This package contains type definitions for qs (https://github.com/ljharb/qs). # Details Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/qs. ### Additional Details * Last updated: Wed, 16 Sep 2020 23:05:37 GMT * Dependencies: none * Global values: `qs` # Credits These definitions were written by [Roman Korneev](https://github.com/RWander), [Leon Yu](https://github.com/leonyu), [Belinda Teh](https://github.com/tehbelinda), [Melvin Lee](https://github.com/zyml), [Arturs Vonda](https://github.com/artursvonda), [Carlos Bonetti](https://github.com/CarlosBonetti), [Dan Smith](https://github.com/dpsmith3), [Hunter Perrin](https://github.com/hperrin), and [Jordan Harband](https://github.com/ljharb). apollo-server-demo/node_modules/@types/qs/package.json 0000644 0001750 0000144 00000004020 13730515101 022574 0 ustar andreh users { "name": "@types/qs", "version": "6.9.5", "description": "TypeScript definitions for qs", "license": "MIT", "contributors": [ { "name": "Roman Korneev", "url": "https://github.com/RWander", "githubUsername": "RWander" }, { "name": "Leon Yu", "url": "https://github.com/leonyu", "githubUsername": "leonyu" }, { "name": "Belinda Teh", "url": "https://github.com/tehbelinda", "githubUsername": "tehbelinda" }, { "name": "Melvin Lee", "url": "https://github.com/zyml", "githubUsername": "zyml" }, { "name": "Arturs Vonda", "url": "https://github.com/artursvonda", "githubUsername": "artursvonda" }, { "name": "Carlos Bonetti", "url": "https://github.com/CarlosBonetti", "githubUsername": "CarlosBonetti" }, { "name": "Dan Smith", "url": "https://github.com/dpsmith3", "githubUsername": "dpsmith3" }, { "name": "Hunter Perrin", "url": "https://github.com/hperrin", "githubUsername": "hperrin" }, { "name": "Jordan Harband", "url": "https://github.com/ljharb", "githubUsername": "ljharb" } ], "main": "", "types": "index.d.ts", "repository": { "type": "git", "url": "https://github.com/DefinitelyTyped/DefinitelyTyped.git", "directory": "types/qs" }, "scripts": {}, "dependencies": {}, "typesPublisherContentHash": "1a8820a6aece2344fa333148c105b71a132db5e68f839c47934a78889cd44574", "typeScriptVersion": "3.2" ,"_resolved": "https://registry.npmjs.org/@types/qs/-/qs-6.9.5.tgz" ,"_integrity": "sha512-/JHkVHtx/REVG0VVToGRGH2+23hsYLHdyG+GrvoUGlGAd0ErauXDyvHtRI/7H7mzLm+tBCKA7pfcpkQ1lf58iQ==" ,"_from": "@types/qs@6.9.5" } apollo-server-demo/node_modules/@types/graphql-upload/ 0000755 0001750 0000144 00000000000 14067647700 022626 5 ustar andreh users apollo-server-demo/node_modules/@types/graphql-upload/LICENSE 0000644 0001750 0000144 00000002165 13724235746 023641 0 ustar andreh users MIT License Copyright (c) Microsoft Corporation. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE apollo-server-demo/node_modules/@types/graphql-upload/index.d.ts 0000644 0001750 0000144 00000002457 13724235746 024541 0 ustar andreh users // Type definitions for graphql-upload 8.0 // Project: https://github.com/jaydenseric/graphql-upload#readme // Definitions by: Mike Marcacci <https://github.com/mike-marcacci> // Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped // TypeScript Version: 3.3 /* tslint:disable:no-unnecessary-generics */ import { IncomingMessage, ServerResponse } from "http"; import { GraphQLScalarType } from "graphql"; import { RequestHandler } from "express"; import { Middleware } from "koa"; import { ReadStream } from "fs-capacitor"; export interface UploadOptions { maxFieldSize?: number; maxFileSize?: number; maxFiles?: number; } export interface GraphQLOperation { query: string; operationName?: null | string; variables?: null | unknown; } export function processRequest( request: IncomingMessage, response: ServerResponse, uploadOptions?: UploadOptions ): Promise<GraphQLOperation | GraphQLOperation[]>; export function graphqlUploadExpress( uploadOptions?: UploadOptions ): RequestHandler; export function graphqlUploadKoa <StateT = any, CustomT = {}>( uploadOptions?: UploadOptions ): Middleware<StateT, CustomT>; export const GraphQLUpload: GraphQLScalarType; export interface FileUpload { filename: string; mimetype: string; encoding: string; createReadStream(): ReadStream; } apollo-server-demo/node_modules/@types/graphql-upload/README.md 0000644 0001750 0000144 00000001402 13724235746 024104 0 ustar andreh users # Installation > `npm install --save @types/graphql-upload` # Summary This package contains type definitions for graphql-upload (https://github.com/jaydenseric/graphql-upload#readme). # Details Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/graphql-upload. ### Additional Details * Last updated: Thu, 03 Sep 2020 18:54:30 GMT * Dependencies: [@types/graphql](https://npmjs.com/package/@types/graphql), [@types/express](https://npmjs.com/package/@types/express), [@types/koa](https://npmjs.com/package/@types/koa), [@types/fs-capacitor](https://npmjs.com/package/@types/fs-capacitor) * Global values: none # Credits These definitions were written by [Mike Marcacci](https://github.com/mike-marcacci). apollo-server-demo/node_modules/@types/graphql-upload/package.json 0000644 0001750 0000144 00000002110 13724235746 025110 0 ustar andreh users { "name": "@types/graphql-upload", "version": "8.0.4", "description": "TypeScript definitions for graphql-upload", "license": "MIT", "contributors": [ { "name": "Mike Marcacci", "url": "https://github.com/mike-marcacci", "githubUsername": "mike-marcacci" } ], "main": "", "types": "index.d.ts", "repository": { "type": "git", "url": "https://github.com/DefinitelyTyped/DefinitelyTyped.git", "directory": "types/graphql-upload" }, "scripts": {}, "dependencies": { "@types/express": "*", "@types/fs-capacitor": "*", "@types/koa": "*", "graphql": "^15.3.0" }, "typesPublisherContentHash": "c998722b32f0659e19e9562ed4dbabe114288107ed4696f50b968fa2d1eacb8d", "typeScriptVersion": "3.3" ,"_resolved": "https://registry.npmjs.org/@types/graphql-upload/-/graphql-upload-8.0.4.tgz" ,"_integrity": "sha512-0TRyJD2o8vbkmJF8InppFcPVcXKk+Rvlg/xvpHBIndSJYpmDWfmtx/ZAtl4f3jR2vfarpTqYgj8MZuJssSoU7Q==" ,"_from": "@types/graphql-upload@8.0.4" } apollo-server-demo/node_modules/@types/keygrip/ 0000755 0001750 0000144 00000000000 14067647701 021361 5 ustar andreh users apollo-server-demo/node_modules/@types/keygrip/LICENSE 0000644 0001750 0000144 00000002237 13601201453 022351 0 ustar andreh users MIT License Copyright (c) Microsoft Corporation. All rights reserved. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE apollo-server-demo/node_modules/@types/keygrip/index.d.ts 0000644 0001750 0000144 00000001151 13601201453 023237 0 ustar andreh users // Type definitions for keygrip 1.0 // Project: https://github.com/crypto-utils/keygrip // Definitions by: jKey Lu <https://github.com/jkeylu> // Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped interface Keygrip { sign(data: any): string; verify(data: any, digest: string): boolean; index(data: any, digest: string): number; } interface KeygripFunction { new (keys: ReadonlyArray<string>, algorithm?: string, encoding?: string): Keygrip; (keys: ReadonlyArray<string>, algorithm?: string, encoding?: string): Keygrip; } declare const Keygrip: KeygripFunction; export = Keygrip; apollo-server-demo/node_modules/@types/keygrip/README.md 0000644 0001750 0000144 00000000746 13601201453 022626 0 ustar andreh users # Installation > `npm install --save @types/keygrip` # Summary This package contains type definitions for keygrip (https://github.com/crypto-utils/keygrip). # Details Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/keygrip. ### Additional Details * Last updated: Thu, 26 Dec 2019 18:59:55 GMT * Dependencies: none * Global values: none # Credits These definitions were written by jKey Lu (https://github.com/jkeylu). apollo-server-demo/node_modules/@types/keygrip/package.json 0000644 0001750 0000144 00000001612 13601201453 023626 0 ustar andreh users { "name": "@types/keygrip", "version": "1.0.2", "description": "TypeScript definitions for keygrip", "license": "MIT", "contributors": [ { "name": "jKey Lu", "url": "https://github.com/jkeylu", "githubUsername": "jkeylu" } ], "main": "", "types": "index.d.ts", "repository": { "type": "git", "url": "https://github.com/DefinitelyTyped/DefinitelyTyped.git", "directory": "types/keygrip" }, "scripts": {}, "dependencies": {}, "typesPublisherContentHash": "85791e9272f5401dc3416aead8d95149b11fbcc20d9d5b22ef8f75aef9021382", "typeScriptVersion": "2.8" ,"_resolved": "https://registry.npmjs.org/@types/keygrip/-/keygrip-1.0.2.tgz" ,"_integrity": "sha512-GJhpTepz2udxGexqos8wgaBx4I/zWIDPh/KOGEwAqtuGDkOUJu5eFvwmdBX4AmB8Odsr+9pHCQqiAqDL/yKMKw==" ,"_from": "@types/keygrip@1.0.2" } apollo-server-demo/node_modules/@types/accepts/ 0000755 0001750 0000144 00000000000 14067647700 021330 5 ustar andreh users apollo-server-demo/node_modules/@types/accepts/LICENSE 0000644 0001750 0000144 00000002212 13240451532 022316 0 ustar andreh users MIT License Copyright (c) Microsoft Corporation. All rights reserved. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE apollo-server-demo/node_modules/@types/accepts/index.d.ts 0000644 0001750 0000144 00000011011 13240451532 023207 0 ustar andreh users // Type definitions for accepts 1.3 // Project: https://github.com/jshttp/accepts // Definitions by: Stefan Reichel <https://github.com/bomret> // Brice BERNARD <https://github.com/brikou> // Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped /// <reference types="node" /> import { IncomingMessage } from "http"; declare namespace accepts { interface Accepts { /** * Return the first accepted charset. If nothing in `charsets` is accepted, then `false` is returned. * If no charsets are supplied, all accepted charsets are returned, in the order of the client's preference * (most preferred first). */ charset(): string[]; charset(charsets: string[]): string | false; charset(...charsets: string[]): string | false; /** * Return the first accepted charset. If nothing in `charsets` is accepted, then `false` is returned. * If no charsets are supplied, all accepted charsets are returned, in the order of the client's preference * (most preferred first). */ charsets(): string[]; charsets(charsets: string[]): string | false; charsets(...charsets: string[]): string | false; /** * Return the first accepted encoding. If nothing in `encodings` is accepted, then `false` is returned. * If no encodings are supplied, all accepted encodings are returned, in the order of the client's preference * (most preferred first). */ encoding(): string[]; encoding(encodings: string[]): string | false; encoding(...encodings: string[]): string | false; /** * Return the first accepted encoding. If nothing in `encodings` is accepted, then `false` is returned. * If no encodings are supplied, all accepted encodings are returned, in the order of the client's preference * (most preferred first). */ encodings(): string[]; encodings(encodings: string[]): string | false; encodings(...encodings: string[]): string | false; /** * Return the first accepted language. If nothing in `languages` is accepted, then `false` is returned. * If no languaes are supplied, all accepted languages are returned, in the order of the client's preference * (most preferred first). */ language(): string[]; language(languages: string[]): string | false; language(...languages: string[]): string | false; /** * Return the first accepted language. If nothing in `languages` is accepted, then `false` is returned. * If no languaes are supplied, all accepted languages are returned, in the order of the client's preference * (most preferred first). */ languages(): string[]; languages(languages: string[]): string | false; languages(...languages: string[]): string | false; /** * Return the first accepted language. If nothing in `languages` is accepted, then `false` is returned. * If no languaes are supplied, all accepted languages are returned, in the order of the client's preference * (most preferred first). */ lang(): string[]; lang(languages: string[]): string | false; lang(...languages: string[]): string | false; /** * Return the first accepted language. If nothing in `languages` is accepted, then `false` is returned. * If no languaes are supplied, all accepted languages are returned, in the order of the client's preference * (most preferred first). */ langs(): string[]; langs(languages: string[]): string | false; langs(...languages: string[]): string | false; /** * Return the first accepted type (and it is returned as the same text as what appears in the `types` array). If nothing in `types` is accepted, then `false` is returned. * If no types are supplied, return the entire set of acceptable types. * * The `types` array can contain full MIME types or file extensions. Any value that is not a full MIME types is passed to `require('mime-types').lookup`. */ type(types: string[]): string[] | string | false; type(...types: string[]): string[] | string | false; types(types: string[]): string[] | string | false; types(...types: string[]): string[] | string | false; } } declare function accepts(req: IncomingMessage): accepts.Accepts; export = accepts; apollo-server-demo/node_modules/@types/accepts/README.md 0000644 0001750 0000144 00000001027 13240451532 022573 0 ustar andreh users # Installation > `npm install --save @types/accepts` # Summary This package contains type definitions for accepts (https://github.com/jshttp/accepts). # Details Files were exported from https://www.github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/accepts Additional Details * Last updated: Tue, 13 Feb 2018 02:54:18 GMT * Dependencies: http, node * Global values: none # Credits These definitions were written by Stefan Reichel <https://github.com/bomret>, Brice BERNARD <https://github.com/brikou>. apollo-server-demo/node_modules/@types/accepts/package.json 0000644 0001750 0000144 00000002005 13240451532 023577 0 ustar andreh users { "name": "@types/accepts", "version": "1.3.5", "description": "TypeScript definitions for accepts", "license": "MIT", "contributors": [ { "name": "Stefan Reichel", "url": "https://github.com/bomret", "githubUsername": "bomret" }, { "name": "Brice BERNARD", "url": "https://github.com/brikou", "githubUsername": "brikou" } ], "main": "", "repository": { "type": "git", "url": "https://www.github.com/DefinitelyTyped/DefinitelyTyped.git" }, "scripts": {}, "dependencies": { "@types/node": "*" }, "typesPublisherContentHash": "0c1b369f923466e74bec6da148c76a05cc138923a142ea737eff8cf2b29b559f", "typeScriptVersion": "2.0" ,"_resolved": "https://registry.npmjs.org/@types/accepts/-/accepts-1.3.5.tgz" ,"_integrity": "sha512-jOdnI/3qTpHABjM5cx1Hc0sKsPoYCp+DP/GJRGtDlPd7fiV9oXGGIcjW/ZOxLIvjGz8MA+uMZI9metHlgqbgwQ==" ,"_from": "@types/accepts@1.3.5" } apollo-server-demo/node_modules/@types/serve-static/ 0000755 0001750 0000144 00000000000 14067647700 022317 5 ustar andreh users apollo-server-demo/node_modules/@types/serve-static/LICENSE 0000644 0001750 0000144 00000002165 14001315761 023313 0 ustar andreh users MIT License Copyright (c) Microsoft Corporation. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE apollo-server-demo/node_modules/@types/serve-static/index.d.ts 0000644 0001750 0000144 00000010776 14001315761 024216 0 ustar andreh users // Type definitions for serve-static 1.13 // Project: https://github.com/expressjs/serve-static // Definitions by: Uros Smolnik <https://github.com/urossmolnik> // Linus Unnebäck <https://github.com/LinusU> // Devansh Jethmalani <https://github.com/devanshj> // Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped // TypeScript Version: 2.3 /// <reference types="node" /> import * as m from "mime"; import * as http from "http"; /** * Create a new middleware function to serve files from within a given root directory. * The file to serve will be determined by combining req.url with the provided root directory. * When a file is not found, instead of sending a 404 response, this module will instead call next() to move on to the next middleware, allowing for stacking and fall-backs. */ declare function serveStatic<R extends http.ServerResponse>( root: string, options?: serveStatic.ServeStaticOptions<R> ): serveStatic.RequestHandler<R>; declare namespace serveStatic { var mime: typeof m; interface ServeStaticOptions<R extends http.ServerResponse = http.ServerResponse> { /** * Enable or disable setting Cache-Control response header, defaults to true. * Disabling this will ignore the immutable and maxAge options. */ cacheControl?: boolean; /** * Set how "dotfiles" are treated when encountered. A dotfile is a file or directory that begins with a dot ("."). * Note this check is done on the path itself without checking if the path actually exists on the disk. * If root is specified, only the dotfiles above the root are checked (i.e. the root itself can be within a dotfile when when set to "deny"). * The default value is 'ignore'. * 'allow' No special treatment for dotfiles * 'deny' Send a 403 for any request for a dotfile * 'ignore' Pretend like the dotfile does not exist and call next() */ dotfiles?: string; /** * Enable or disable etag generation, defaults to true. */ etag?: boolean; /** * Set file extension fallbacks. When set, if a file is not found, the given extensions will be added to the file name and search for. * The first that exists will be served. Example: ['html', 'htm']. * The default value is false. */ extensions?: string[] | false; /** * Let client errors fall-through as unhandled requests, otherwise forward a client error. * The default value is true. */ fallthrough?: boolean; /** * Enable or disable the immutable directive in the Cache-Control response header. * If enabled, the maxAge option should also be specified to enable caching. The immutable directive will prevent supported clients from making conditional requests during the life of the maxAge option to check if the file has changed. */ immutable?: boolean; /** * By default this module will send "index.html" files in response to a request on a directory. * To disable this set false or to supply a new index pass a string or an array in preferred order. */ index?: boolean | string | string[]; /** * Enable or disable Last-Modified header, defaults to true. Uses the file system's last modified value. */ lastModified?: boolean; /** * Provide a max-age in milliseconds for http caching, defaults to 0. This can also be a string accepted by the ms module. */ maxAge?: number | string; /** * Redirect to trailing "/" when the pathname is a dir. Defaults to true. */ redirect?: boolean; /** * Function to set custom headers on response. Alterations to the headers need to occur synchronously. * The function is called as fn(res, path, stat), where the arguments are: * res the response object * path the file path that is being sent * stat the stat object of the file that is being sent */ setHeaders?: (res: R, path: string, stat: any) => any; } interface RequestHandler<R extends http.ServerResponse> { (request: http.IncomingMessage, response: R, next: () => void): any; } interface RequestHandlerConstructor<R extends http.ServerResponse> { (root: string, options?: ServeStaticOptions<R>): RequestHandler<R>; mime: typeof m; } } export = serveStatic; apollo-server-demo/node_modules/@types/serve-static/README.md 0000644 0001750 0000144 00000001315 14001315761 023561 0 ustar andreh users # Installation > `npm install --save @types/serve-static` # Summary This package contains type definitions for serve-static (https://github.com/expressjs/serve-static). # Details Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/serve-static. ### Additional Details * Last updated: Mon, 18 Jan 2021 14:32:17 GMT * Dependencies: [@types/mime](https://npmjs.com/package/@types/mime), [@types/node](https://npmjs.com/package/@types/node) * Global values: none # Credits These definitions were written by [Uros Smolnik](https://github.com/urossmolnik), [Linus Unnebäck](https://github.com/LinusU), and [Devansh Jethmalani](https://github.com/devanshj). apollo-server-demo/node_modules/@types/serve-static/package.json 0000644 0001750 0000144 00000002444 14001315761 024574 0 ustar andreh users { "name": "@types/serve-static", "version": "1.13.9", "description": "TypeScript definitions for serve-static", "license": "MIT", "contributors": [ { "name": "Uros Smolnik", "url": "https://github.com/urossmolnik", "githubUsername": "urossmolnik" }, { "name": "Linus Unnebäck", "url": "https://github.com/LinusU", "githubUsername": "LinusU" }, { "name": "Devansh Jethmalani", "url": "https://github.com/devanshj", "githubUsername": "devanshj" } ], "main": "", "types": "index.d.ts", "repository": { "type": "git", "url": "https://github.com/DefinitelyTyped/DefinitelyTyped.git", "directory": "types/serve-static" }, "scripts": {}, "dependencies": { "@types/mime": "^1", "@types/node": "*" }, "typesPublisherContentHash": "128f8f2571ac1e49c45cb7906b8de2eca4e5c41dbcc05194d377a3183a964abd", "typeScriptVersion": "3.4" ,"_resolved": "https://registry.npmjs.org/@types/serve-static/-/serve-static-1.13.9.tgz" ,"_integrity": "sha512-ZFqF6qa48XsPdjXV5Gsz0Zqmux2PerNd3a/ktL45mHpa19cuMi/cL8tcxdAx497yRh+QtYPuofjT9oWw9P7nkA==" ,"_from": "@types/serve-static@1.13.9" } apollo-server-demo/node_modules/@types/koa-compose/ 0000755 0001750 0000144 00000000000 14067647701 022124 5 ustar andreh users apollo-server-demo/node_modules/@types/koa-compose/LICENSE 0000644 0001750 0000144 00000002237 13561055211 023120 0 ustar andreh users MIT License Copyright (c) Microsoft Corporation. All rights reserved. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE apollo-server-demo/node_modules/@types/koa-compose/index.d.ts 0000644 0001750 0000144 00000005060 13561055211 024011 0 ustar andreh users // Type definitions for koa-compose 3.2 // Project: https://github.com/koajs/compose // Definitions by: jKey Lu <https://github.com/jkeylu> // Anton Astashov <https://github.com/astashov> // Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped // TypeScript Version: 2.3 import * as Koa from "koa"; declare function compose<T1, U1, T2, U2>( middleware: [Koa.Middleware<T1, U1>, Koa.Middleware<T2, U2>] ): Koa.Middleware<T1 & T2, U1 & U2>; declare function compose<T1, U1, T2, U2, T3, U3>( middleware: [Koa.Middleware<T1, U1>, Koa.Middleware<T2, U2>, Koa.Middleware<T3, U3>] ): Koa.Middleware<T1 & T2 & T3, U1 & U2 & U3>; declare function compose<T1, U1, T2, U2, T3, U3, T4, U4>( middleware: [Koa.Middleware<T1, U1>, Koa.Middleware<T2, U2>, Koa.Middleware<T3, U3>, Koa.Middleware<T4, U4>] ): Koa.Middleware<T1 & T2 & T3 & T4, U1 & U2 & U3 & U4>; declare function compose<T1, U1, T2, U2, T3, U3, T4, U4, T5, U5>( middleware: [ Koa.Middleware<T1, U1>, Koa.Middleware<T2, U2>, Koa.Middleware<T3, U3>, Koa.Middleware<T4, U4>, Koa.Middleware<T5, U5> ] ): Koa.Middleware<T1 & T2 & T3 & T4 & T5, U1 & U2 & U3 & U4 & U5>; declare function compose<T1, U1, T2, U2, T3, U3, T4, U4, T5, U5, T6, U6>( middleware: [ Koa.Middleware<T1, U1>, Koa.Middleware<T2, U2>, Koa.Middleware<T3, U3>, Koa.Middleware<T4, U4>, Koa.Middleware<T5, U5>, Koa.Middleware<T6, U6> ] ): Koa.Middleware<T1 & T2 & T3 & T4 & T5 & T6, U1 & U2 & U3 & U4 & U5 & U6>; declare function compose<T1, U1, T2, U2, T3, U3, T4, U4, T5, U5, T6, U6, T7, U7>( middleware: [ Koa.Middleware<T1, U1>, Koa.Middleware<T2, U2>, Koa.Middleware<T3, U3>, Koa.Middleware<T4, U4>, Koa.Middleware<T5, U5>, Koa.Middleware<T6, U6>, Koa.Middleware<T7, U7> ] ): Koa.Middleware<T1 & T2 & T3 & T4 & T5 & T6 & T7, U1 & U2 & U3 & U4 & U5 & U6 & U7>; declare function compose<T1, U1, T2, U2, T3, U3, T4, U4, T5, U5, T6, U6, T7, U7, T8, U8>( middleware: [ Koa.Middleware<T1, U1>, Koa.Middleware<T2, U2>, Koa.Middleware<T3, U3>, Koa.Middleware<T4, U4>, Koa.Middleware<T5, U5>, Koa.Middleware<T6, U6>, Koa.Middleware<T7, U7>, Koa.Middleware<T8, U8> ] ): Koa.Middleware<T1 & T2 & T3 & T4 & T5 & T6 & T7 & T8, U1 & U2 & U3 & U4 & U5 & U6 & U7 & U8>; declare function compose<T>(middleware: Array<compose.Middleware<T>>): compose.ComposedMiddleware<T>; declare namespace compose { type Middleware<T> = (context: T, next: Koa.Next) => any; type ComposedMiddleware<T> = (context: T, next?: Koa.Next) => Promise<void>; } export = compose; apollo-server-demo/node_modules/@types/koa-compose/README.md 0000644 0001750 0000144 00000001036 13561055211 023366 0 ustar andreh users # Installation > `npm install --save @types/koa-compose` # Summary This package contains type definitions for koa-compose (https://github.com/koajs/compose). # Details Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/koa-compose Additional Details * Last updated: Thu, 07 Nov 2019 17:55:21 GMT * Dependencies: @types/koa * Global values: none # Credits These definitions were written by jKey Lu <https://github.com/jkeylu>, and Anton Astashov <https://github.com/astashov>. apollo-server-demo/node_modules/@types/koa-compose/package.json 0000644 0001750 0000144 00000002127 13561055211 024377 0 ustar andreh users { "name": "@types/koa-compose", "version": "3.2.5", "description": "TypeScript definitions for koa-compose", "license": "MIT", "contributors": [ { "name": "jKey Lu", "url": "https://github.com/jkeylu", "githubUsername": "jkeylu" }, { "name": "Anton Astashov", "url": "https://github.com/astashov", "githubUsername": "astashov" } ], "main": "", "types": "index.d.ts", "repository": { "type": "git", "url": "https://github.com/DefinitelyTyped/DefinitelyTyped.git", "directory": "types/koa-compose" }, "scripts": {}, "dependencies": { "@types/koa": "*" }, "typesPublisherContentHash": "a5fb98541fe6f08d4799ff1ca630573727c2aaf2ecaeabcdef672cde7eb1c72d", "typeScriptVersion": "2.8" ,"_resolved": "https://registry.npmjs.org/@types/koa-compose/-/koa-compose-3.2.5.tgz" ,"_integrity": "sha512-B8nG/OoE1ORZqCkBVsup/AKcvjdgoHnfi4pZMn5UwAPCbhk/96xyv284eBYW8JlQbQ7zDmnpFr68I/40mFoIBQ==" ,"_from": "@types/koa-compose@3.2.5" } apollo-server-demo/node_modules/@types/node/ 0000755 0001750 0000144 00000000000 14067647700 020633 5 ustar andreh users apollo-server-demo/node_modules/@types/node/http.d.ts 0000644 0001750 0000144 00000044667 14000133700 022376 0 ustar andreh users declare module "http" { import * as stream from "stream"; import { URL } from "url"; import { Socket, Server as NetServer } from "net"; // incoming headers will never contain number interface IncomingHttpHeaders extends NodeJS.Dict<string | string[]> { 'accept'?: string; 'accept-language'?: string; 'accept-patch'?: string; 'accept-ranges'?: string; 'access-control-allow-credentials'?: string; 'access-control-allow-headers'?: string; 'access-control-allow-methods'?: string; 'access-control-allow-origin'?: string; 'access-control-expose-headers'?: string; 'access-control-max-age'?: string; 'access-control-request-headers'?: string; 'access-control-request-method'?: string; 'age'?: string; 'allow'?: string; 'alt-svc'?: string; 'authorization'?: string; 'cache-control'?: string; 'connection'?: string; 'content-disposition'?: string; 'content-encoding'?: string; 'content-language'?: string; 'content-length'?: string; 'content-location'?: string; 'content-range'?: string; 'content-type'?: string; 'cookie'?: string; 'date'?: string; 'expect'?: string; 'expires'?: string; 'forwarded'?: string; 'from'?: string; 'host'?: string; 'if-match'?: string; 'if-modified-since'?: string; 'if-none-match'?: string; 'if-unmodified-since'?: string; 'last-modified'?: string; 'location'?: string; 'origin'?: string; 'pragma'?: string; 'proxy-authenticate'?: string; 'proxy-authorization'?: string; 'public-key-pins'?: string; 'range'?: string; 'referer'?: string; 'retry-after'?: string; 'sec-websocket-accept'?: string; 'sec-websocket-extensions'?: string; 'sec-websocket-key'?: string; 'sec-websocket-protocol'?: string; 'sec-websocket-version'?: string; 'set-cookie'?: string[]; 'strict-transport-security'?: string; 'tk'?: string; 'trailer'?: string; 'transfer-encoding'?: string; 'upgrade'?: string; 'user-agent'?: string; 'vary'?: string; 'via'?: string; 'warning'?: string; 'www-authenticate'?: string; } // outgoing headers allows numbers (as they are converted internally to strings) type OutgoingHttpHeader = number | string | string[]; interface OutgoingHttpHeaders extends NodeJS.Dict<OutgoingHttpHeader> { } interface ClientRequestArgs { protocol?: string | null; host?: string | null; hostname?: string | null; family?: number; port?: number | string | null; defaultPort?: number | string; localAddress?: string; socketPath?: string; /** * @default 8192 */ maxHeaderSize?: number; method?: string; path?: string | null; headers?: OutgoingHttpHeaders; auth?: string | null; agent?: Agent | boolean; _defaultAgent?: Agent; timeout?: number; setHost?: boolean; // https://github.com/nodejs/node/blob/master/lib/_http_client.js#L278 createConnection?: (options: ClientRequestArgs, oncreate: (err: Error, socket: Socket) => void) => Socket; } interface ServerOptions { IncomingMessage?: typeof IncomingMessage; ServerResponse?: typeof ServerResponse; /** * Optionally overrides the value of * [`--max-http-header-size`][] for requests received by this server, i.e. * the maximum length of request headers in bytes. * @default 8192 */ maxHeaderSize?: number; /** * Use an insecure HTTP parser that accepts invalid HTTP headers when true. * Using the insecure parser should be avoided. * See --insecure-http-parser for more information. * @default false */ insecureHTTPParser?: boolean; } type RequestListener = (req: IncomingMessage, res: ServerResponse) => void; interface HttpBase { setTimeout(msecs?: number, callback?: () => void): this; setTimeout(callback: () => void): this; /** * Limits maximum incoming headers count. If set to 0, no limit will be applied. * @default 2000 * {@link https://nodejs.org/api/http.html#http_server_maxheaderscount} */ maxHeadersCount: number | null; timeout: number; /** * Limit the amount of time the parser will wait to receive the complete HTTP headers. * @default 60000 * {@link https://nodejs.org/api/http.html#http_server_headerstimeout} */ headersTimeout: number; keepAliveTimeout: number; /** * Sets the timeout value in milliseconds for receiving the entire request from the client. * @default 0 * {@link https://nodejs.org/api/http.html#http_server_requesttimeout} */ requestTimeout: number; } interface Server extends HttpBase {} class Server extends NetServer { constructor(requestListener?: RequestListener); constructor(options: ServerOptions, requestListener?: RequestListener); } // https://github.com/nodejs/node/blob/master/lib/_http_outgoing.js class OutgoingMessage extends stream.Writable { upgrading: boolean; chunkedEncoding: boolean; shouldKeepAlive: boolean; useChunkedEncodingByDefault: boolean; sendDate: boolean; /** * @deprecated Use `writableEnded` instead. */ finished: boolean; headersSent: boolean; /** * @deprecate Use `socket` instead. */ connection: Socket | null; socket: Socket | null; constructor(); setTimeout(msecs: number, callback?: () => void): this; setHeader(name: string, value: number | string | ReadonlyArray<string>): void; getHeader(name: string): number | string | string[] | undefined; getHeaders(): OutgoingHttpHeaders; getHeaderNames(): string[]; hasHeader(name: string): boolean; removeHeader(name: string): void; addTrailers(headers: OutgoingHttpHeaders | ReadonlyArray<[string, string]>): void; flushHeaders(): void; } // https://github.com/nodejs/node/blob/master/lib/_http_server.js#L108-L256 class ServerResponse extends OutgoingMessage { statusCode: number; statusMessage: string; constructor(req: IncomingMessage); assignSocket(socket: Socket): void; detachSocket(socket: Socket): void; // https://github.com/nodejs/node/blob/master/test/parallel/test-http-write-callbacks.js#L53 // no args in writeContinue callback writeContinue(callback?: () => void): void; writeHead(statusCode: number, reasonPhrase?: string, headers?: OutgoingHttpHeaders | OutgoingHttpHeader[]): this; writeHead(statusCode: number, headers?: OutgoingHttpHeaders | OutgoingHttpHeader[]): this; writeProcessing(): void; } interface InformationEvent { statusCode: number; statusMessage: string; httpVersion: string; httpVersionMajor: number; httpVersionMinor: number; headers: IncomingHttpHeaders; rawHeaders: string[]; } // https://github.com/nodejs/node/blob/master/lib/_http_client.js#L77 class ClientRequest extends OutgoingMessage { aborted: boolean; host: string; protocol: string; constructor(url: string | URL | ClientRequestArgs, cb?: (res: IncomingMessage) => void); method: string; path: string; /** @deprecated since v14.1.0 Use `request.destroy()` instead. */ abort(): void; onSocket(socket: Socket): void; setTimeout(timeout: number, callback?: () => void): this; setNoDelay(noDelay?: boolean): void; setSocketKeepAlive(enable?: boolean, initialDelay?: number): void; addListener(event: 'abort', listener: () => void): this; addListener(event: 'connect', listener: (response: IncomingMessage, socket: Socket, head: Buffer) => void): this; addListener(event: 'continue', listener: () => void): this; addListener(event: 'information', listener: (info: InformationEvent) => void): this; addListener(event: 'response', listener: (response: IncomingMessage) => void): this; addListener(event: 'socket', listener: (socket: Socket) => void): this; addListener(event: 'timeout', listener: () => void): this; addListener(event: 'upgrade', listener: (response: IncomingMessage, socket: Socket, head: Buffer) => void): this; addListener(event: 'close', listener: () => void): this; addListener(event: 'drain', listener: () => void): this; addListener(event: 'error', listener: (err: Error) => void): this; addListener(event: 'finish', listener: () => void): this; addListener(event: 'pipe', listener: (src: stream.Readable) => void): this; addListener(event: 'unpipe', listener: (src: stream.Readable) => void): this; addListener(event: string | symbol, listener: (...args: any[]) => void): this; on(event: 'abort', listener: () => void): this; on(event: 'connect', listener: (response: IncomingMessage, socket: Socket, head: Buffer) => void): this; on(event: 'continue', listener: () => void): this; on(event: 'information', listener: (info: InformationEvent) => void): this; on(event: 'response', listener: (response: IncomingMessage) => void): this; on(event: 'socket', listener: (socket: Socket) => void): this; on(event: 'timeout', listener: () => void): this; on(event: 'upgrade', listener: (response: IncomingMessage, socket: Socket, head: Buffer) => void): this; on(event: 'close', listener: () => void): this; on(event: 'drain', listener: () => void): this; on(event: 'error', listener: (err: Error) => void): this; on(event: 'finish', listener: () => void): this; on(event: 'pipe', listener: (src: stream.Readable) => void): this; on(event: 'unpipe', listener: (src: stream.Readable) => void): this; on(event: string | symbol, listener: (...args: any[]) => void): this; once(event: 'abort', listener: () => void): this; once(event: 'connect', listener: (response: IncomingMessage, socket: Socket, head: Buffer) => void): this; once(event: 'continue', listener: () => void): this; once(event: 'information', listener: (info: InformationEvent) => void): this; once(event: 'response', listener: (response: IncomingMessage) => void): this; once(event: 'socket', listener: (socket: Socket) => void): this; once(event: 'timeout', listener: () => void): this; once(event: 'upgrade', listener: (response: IncomingMessage, socket: Socket, head: Buffer) => void): this; once(event: 'close', listener: () => void): this; once(event: 'drain', listener: () => void): this; once(event: 'error', listener: (err: Error) => void): this; once(event: 'finish', listener: () => void): this; once(event: 'pipe', listener: (src: stream.Readable) => void): this; once(event: 'unpipe', listener: (src: stream.Readable) => void): this; once(event: string | symbol, listener: (...args: any[]) => void): this; prependListener(event: 'abort', listener: () => void): this; prependListener(event: 'connect', listener: (response: IncomingMessage, socket: Socket, head: Buffer) => void): this; prependListener(event: 'continue', listener: () => void): this; prependListener(event: 'information', listener: (info: InformationEvent) => void): this; prependListener(event: 'response', listener: (response: IncomingMessage) => void): this; prependListener(event: 'socket', listener: (socket: Socket) => void): this; prependListener(event: 'timeout', listener: () => void): this; prependListener(event: 'upgrade', listener: (response: IncomingMessage, socket: Socket, head: Buffer) => void): this; prependListener(event: 'close', listener: () => void): this; prependListener(event: 'drain', listener: () => void): this; prependListener(event: 'error', listener: (err: Error) => void): this; prependListener(event: 'finish', listener: () => void): this; prependListener(event: 'pipe', listener: (src: stream.Readable) => void): this; prependListener(event: 'unpipe', listener: (src: stream.Readable) => void): this; prependListener(event: string | symbol, listener: (...args: any[]) => void): this; prependOnceListener(event: 'abort', listener: () => void): this; prependOnceListener(event: 'connect', listener: (response: IncomingMessage, socket: Socket, head: Buffer) => void): this; prependOnceListener(event: 'continue', listener: () => void): this; prependOnceListener(event: 'information', listener: (info: InformationEvent) => void): this; prependOnceListener(event: 'response', listener: (response: IncomingMessage) => void): this; prependOnceListener(event: 'socket', listener: (socket: Socket) => void): this; prependOnceListener(event: 'timeout', listener: () => void): this; prependOnceListener(event: 'upgrade', listener: (response: IncomingMessage, socket: Socket, head: Buffer) => void): this; prependOnceListener(event: 'close', listener: () => void): this; prependOnceListener(event: 'drain', listener: () => void): this; prependOnceListener(event: 'error', listener: (err: Error) => void): this; prependOnceListener(event: 'finish', listener: () => void): this; prependOnceListener(event: 'pipe', listener: (src: stream.Readable) => void): this; prependOnceListener(event: 'unpipe', listener: (src: stream.Readable) => void): this; prependOnceListener(event: string | symbol, listener: (...args: any[]) => void): this; } class IncomingMessage extends stream.Readable { constructor(socket: Socket); aborted: boolean; httpVersion: string; httpVersionMajor: number; httpVersionMinor: number; complete: boolean; /** * @deprecated since v13.0.0 - Use `socket` instead. */ connection: Socket; socket: Socket; headers: IncomingHttpHeaders; rawHeaders: string[]; trailers: NodeJS.Dict<string>; rawTrailers: string[]; setTimeout(msecs: number, callback?: () => void): this; /** * Only valid for request obtained from http.Server. */ method?: string; /** * Only valid for request obtained from http.Server. */ url?: string; /** * Only valid for response obtained from http.ClientRequest. */ statusCode?: number; /** * Only valid for response obtained from http.ClientRequest. */ statusMessage?: string; destroy(error?: Error): void; } interface AgentOptions { /** * Keep sockets around in a pool to be used by other requests in the future. Default = false */ keepAlive?: boolean; /** * When using HTTP KeepAlive, how often to send TCP KeepAlive packets over sockets being kept alive. Default = 1000. * Only relevant if keepAlive is set to true. */ keepAliveMsecs?: number; /** * Maximum number of sockets to allow per host. Default for Node 0.10 is 5, default for Node 0.12 is Infinity */ maxSockets?: number; /** * Maximum number of sockets allowed for all hosts in total. Each request will use a new socket until the maximum is reached. Default: Infinity. */ maxTotalSockets?: number; /** * Maximum number of sockets to leave open in a free state. Only relevant if keepAlive is set to true. Default = 256. */ maxFreeSockets?: number; /** * Socket timeout in milliseconds. This will set the timeout after the socket is connected. */ timeout?: number; /** * Scheduling strategy to apply when picking the next free socket to use. Default: 'fifo'. */ scheduling?: 'fifo' | 'lifo'; } class Agent { maxFreeSockets: number; maxSockets: number; maxTotalSockets: number; readonly freeSockets: NodeJS.ReadOnlyDict<Socket[]>; readonly sockets: NodeJS.ReadOnlyDict<Socket[]>; readonly requests: NodeJS.ReadOnlyDict<IncomingMessage[]>; constructor(opts?: AgentOptions); /** * Destroy any sockets that are currently in use by the agent. * It is usually not necessary to do this. However, if you are using an agent with KeepAlive enabled, * then it is best to explicitly shut down the agent when you know that it will no longer be used. Otherwise, * sockets may hang open for quite a long time before the server terminates them. */ destroy(): void; } const METHODS: string[]; const STATUS_CODES: { [errorCode: number]: string | undefined; [errorCode: string]: string | undefined; }; function createServer(requestListener?: RequestListener): Server; function createServer(options: ServerOptions, requestListener?: RequestListener): Server; // although RequestOptions are passed as ClientRequestArgs to ClientRequest directly, // create interface RequestOptions would make the naming more clear to developers interface RequestOptions extends ClientRequestArgs { } function request(options: RequestOptions | string | URL, callback?: (res: IncomingMessage) => void): ClientRequest; function request(url: string | URL, options: RequestOptions, callback?: (res: IncomingMessage) => void): ClientRequest; function get(options: RequestOptions | string | URL, callback?: (res: IncomingMessage) => void): ClientRequest; function get(url: string | URL, options: RequestOptions, callback?: (res: IncomingMessage) => void): ClientRequest; let globalAgent: Agent; /** * Read-only property specifying the maximum allowed size of HTTP headers in bytes. * Defaults to 16KB. Configurable using the [`--max-http-header-size`][] CLI option. */ const maxHeaderSize: number; } apollo-server-demo/node_modules/@types/node/https.d.ts 0000644 0001750 0000144 00000003224 14000133700 022541 0 ustar andreh users declare module "https" { import * as tls from "tls"; import * as events from "events"; import * as http from "http"; import { URL } from "url"; type ServerOptions = tls.SecureContextOptions & tls.TlsOptions & http.ServerOptions; type RequestOptions = http.RequestOptions & tls.SecureContextOptions & { rejectUnauthorized?: boolean; // Defaults to true servername?: string; // SNI TLS Extension }; interface AgentOptions extends http.AgentOptions, tls.ConnectionOptions { rejectUnauthorized?: boolean; maxCachedSessions?: number; } class Agent extends http.Agent { constructor(options?: AgentOptions); options: AgentOptions; } interface Server extends http.HttpBase {} class Server extends tls.Server { constructor(requestListener?: http.RequestListener); constructor(options: ServerOptions, requestListener?: http.RequestListener); } function createServer(requestListener?: http.RequestListener): Server; function createServer(options: ServerOptions, requestListener?: http.RequestListener): Server; function request(options: RequestOptions | string | URL, callback?: (res: http.IncomingMessage) => void): http.ClientRequest; function request(url: string | URL, options: RequestOptions, callback?: (res: http.IncomingMessage) => void): http.ClientRequest; function get(options: RequestOptions | string | URL, callback?: (res: http.IncomingMessage) => void): http.ClientRequest; function get(url: string | URL, options: RequestOptions, callback?: (res: http.IncomingMessage) => void): http.ClientRequest; let globalAgent: Agent; } apollo-server-demo/node_modules/@types/node/ts3.4/ 0000755 0001750 0000144 00000000000 14067647700 021506 5 ustar andreh users apollo-server-demo/node_modules/@types/node/ts3.4/base.d.ts 0000644 0001750 0000144 00000004564 14000133701 023175 0 ustar andreh users // NOTE: These definitions support NodeJS and TypeScript 3.2 - 3.4. // NOTE: TypeScript version-specific augmentations can be found in the following paths: // - ~/base.d.ts - Shared definitions common to all TypeScript versions // - ~/index.d.ts - Definitions specific to TypeScript 2.1 // - ~/ts3.2/base.d.ts - Definitions specific to TypeScript 3.2 // - ~/ts3.2/index.d.ts - Definitions specific to TypeScript 3.2 with global and assert pulled in // Reference required types from the default lib: /// <reference lib="es2018" /> /// <reference lib="esnext.asynciterable" /> /// <reference lib="esnext.intl" /> /// <reference lib="esnext.bigint" /> // Base definitions for all NodeJS modules that are not specific to any version of TypeScript: /// <reference path="../globals.d.ts" /> /// <reference path="../async_hooks.d.ts" /> /// <reference path="../buffer.d.ts" /> /// <reference path="../child_process.d.ts" /> /// <reference path="../cluster.d.ts" /> /// <reference path="../console.d.ts" /> /// <reference path="../constants.d.ts" /> /// <reference path="../crypto.d.ts" /> /// <reference path="../dgram.d.ts" /> /// <reference path="../dns.d.ts" /> /// <reference path="../domain.d.ts" /> /// <reference path="../events.d.ts" /> /// <reference path="../fs.d.ts" /> /// <reference path="../fs/promises.d.ts" /> /// <reference path="../http.d.ts" /> /// <reference path="../http2.d.ts" /> /// <reference path="../https.d.ts" /> /// <reference path="../inspector.d.ts" /> /// <reference path="../module.d.ts" /> /// <reference path="../net.d.ts" /> /// <reference path="../os.d.ts" /> /// <reference path="../path.d.ts" /> /// <reference path="../perf_hooks.d.ts" /> /// <reference path="../process.d.ts" /> /// <reference path="../punycode.d.ts" /> /// <reference path="../querystring.d.ts" /> /// <reference path="../readline.d.ts" /> /// <reference path="../repl.d.ts" /> /// <reference path="../stream.d.ts" /> /// <reference path="../string_decoder.d.ts" /> /// <reference path="../timers.d.ts" /> /// <reference path="../tls.d.ts" /> /// <reference path="../trace_events.d.ts" /> /// <reference path="../tty.d.ts" /> /// <reference path="../url.d.ts" /> /// <reference path="../util.d.ts" /> /// <reference path="../v8.d.ts" /> /// <reference path="../vm.d.ts" /> /// <reference path="../worker_threads.d.ts" /> /// <reference path="../zlib.d.ts" /> apollo-server-demo/node_modules/@types/node/ts3.4/index.d.ts 0000644 0001750 0000144 00000000655 14000133700 023366 0 ustar andreh users // NOTE: These definitions support NodeJS and TypeScript 3.2 - 3.4. // This is required to enable globalThis support for global in ts3.5 without causing errors // This is required to enable typing assert in ts3.7 without causing errors // Typically type modifiations should be made in base.d.ts instead of here /// <reference path="base.d.ts" /> /// <reference path="assert.d.ts" /> /// <reference path="globals.global.d.ts" /> apollo-server-demo/node_modules/@types/node/ts3.4/globals.global.d.ts 0000644 0001750 0000144 00000000043 14000133700 025130 0 ustar andreh users declare var global: NodeJS.Global; apollo-server-demo/node_modules/@types/node/ts3.4/assert.d.ts 0000644 0001750 0000144 00000011050 14000133701 023550 0 ustar andreh users declare module 'assert' { /** An alias of `assert.ok()`. */ function assert(value: any, message?: string | Error): void; namespace assert { class AssertionError implements Error { name: string; message: string; actual: any; expected: any; operator: string; generatedMessage: boolean; code: 'ERR_ASSERTION'; constructor(options?: { /** If provided, the error message is set to this value. */ message?: string; /** The `actual` property on the error instance. */ actual?: any; /** The `expected` property on the error instance. */ expected?: any; /** The `operator` property on the error instance. */ operator?: string; /** If provided, the generated stack trace omits frames before this function. */ // tslint:disable-next-line:ban-types stackStartFn?: Function; }); } class CallTracker { calls(exact?: number): () => void; calls<Func extends (...args: any[]) => any>(fn?: Func, exact?: number): Func; report(): CallTrackerReportInformation[]; verify(): void; } interface CallTrackerReportInformation { message: string; /** The actual number of times the function was called. */ actual: number; /** The number of times the function was expected to be called. */ expected: number; /** The name of the function that is wrapped. */ operator: string; /** A stack trace of the function. */ stack: object; } type AssertPredicate = RegExp | (new () => object) | ((thrown: any) => boolean) | object | Error; function fail(message?: string | Error): never; /** @deprecated since v10.0.0 - use fail([message]) or other assert functions instead. */ function fail( actual: any, expected: any, message?: string | Error, operator?: string, // tslint:disable-next-line:ban-types stackStartFn?: Function, ): never; function ok(value: any, message?: string | Error): void; /** @deprecated since v9.9.0 - use strictEqual() instead. */ function equal(actual: any, expected: any, message?: string | Error): void; /** @deprecated since v9.9.0 - use notStrictEqual() instead. */ function notEqual(actual: any, expected: any, message?: string | Error): void; /** @deprecated since v9.9.0 - use deepStrictEqual() instead. */ function deepEqual(actual: any, expected: any, message?: string | Error): void; /** @deprecated since v9.9.0 - use notDeepStrictEqual() instead. */ function notDeepEqual(actual: any, expected: any, message?: string | Error): void; function strictEqual(actual: any, expected: any, message?: string | Error): void; function notStrictEqual(actual: any, expected: any, message?: string | Error): void; function deepStrictEqual(actual: any, expected: any, message?: string | Error): void; function notDeepStrictEqual(actual: any, expected: any, message?: string | Error): void; function throws(block: () => any, message?: string | Error): void; function throws(block: () => any, error: AssertPredicate, message?: string | Error): void; function doesNotThrow(block: () => any, message?: string | Error): void; function doesNotThrow(block: () => any, error: AssertPredicate, message?: string | Error): void; function ifError(value: any): void; function rejects(block: (() => Promise<any>) | Promise<any>, message?: string | Error): Promise<void>; function rejects( block: (() => Promise<any>) | Promise<any>, error: AssertPredicate, message?: string | Error, ): Promise<void>; function doesNotReject(block: (() => Promise<any>) | Promise<any>, message?: string | Error): Promise<void>; function doesNotReject( block: (() => Promise<any>) | Promise<any>, error: AssertPredicate, message?: string | Error, ): Promise<void>; function match(value: string, regExp: RegExp, message?: string | Error): void; function doesNotMatch(value: string, regExp: RegExp, message?: string | Error): void; const strict: typeof assert; } export = assert; } apollo-server-demo/node_modules/@types/node/buffer.d.ts 0000644 0001750 0000144 00000001304 14000133701 022646 0 ustar andreh users declare module "buffer" { export const INSPECT_MAX_BYTES: number; export const kMaxLength: number; export const kStringMaxLength: number; export const constants: { MAX_LENGTH: number; MAX_STRING_LENGTH: number; }; const BuffType: typeof Buffer; export type TranscodeEncoding = "ascii" | "utf8" | "utf16le" | "ucs2" | "latin1" | "binary"; export function transcode(source: Uint8Array, fromEnc: TranscodeEncoding, toEnc: TranscodeEncoding): Buffer; export const SlowBuffer: { /** @deprecated since v6.0.0, use `Buffer.allocUnsafeSlow()` */ new(size: number): Buffer; prototype: Buffer; }; export { BuffType as Buffer }; } apollo-server-demo/node_modules/@types/node/path.d.ts 0000644 0001750 0000144 00000014245 14000133700 022340 0 ustar andreh users declare module "path" { namespace path { /** * A parsed path object generated by path.parse() or consumed by path.format(). */ interface ParsedPath { /** * The root of the path such as '/' or 'c:\' */ root: string; /** * The full directory path such as '/home/user/dir' or 'c:\path\dir' */ dir: string; /** * The file name including extension (if any) such as 'index.html' */ base: string; /** * The file extension (if any) such as '.html' */ ext: string; /** * The file name without extension (if any) such as 'index' */ name: string; } interface FormatInputPathObject { /** * The root of the path such as '/' or 'c:\' */ root?: string; /** * The full directory path such as '/home/user/dir' or 'c:\path\dir' */ dir?: string; /** * The file name including extension (if any) such as 'index.html' */ base?: string; /** * The file extension (if any) such as '.html' */ ext?: string; /** * The file name without extension (if any) such as 'index' */ name?: string; } interface PlatformPath { /** * Normalize a string path, reducing '..' and '.' parts. * When multiple slashes are found, they're replaced by a single one; when the path contains a trailing slash, it is preserved. On Windows backslashes are used. * * @param p string path to normalize. */ normalize(p: string): string; /** * Join all arguments together and normalize the resulting path. * Arguments must be strings. In v0.8, non-string arguments were silently ignored. In v0.10 and up, an exception is thrown. * * @param paths paths to join. */ join(...paths: string[]): string; /** * The right-most parameter is considered {to}. Other parameters are considered an array of {from}. * * Starting from leftmost {from} parameter, resolves {to} to an absolute path. * * If {to} isn't already absolute, {from} arguments are prepended in right to left order, * until an absolute path is found. If after using all {from} paths still no absolute path is found, * the current working directory is used as well. The resulting path is normalized, * and trailing slashes are removed unless the path gets resolved to the root directory. * * @param pathSegments string paths to join. Non-string arguments are ignored. */ resolve(...pathSegments: string[]): string; /** * Determines whether {path} is an absolute path. An absolute path will always resolve to the same location, regardless of the working directory. * * @param path path to test. */ isAbsolute(p: string): boolean; /** * Solve the relative path from {from} to {to}. * At times we have two absolute paths, and we need to derive the relative path from one to the other. This is actually the reverse transform of path.resolve. */ relative(from: string, to: string): string; /** * Return the directory name of a path. Similar to the Unix dirname command. * * @param p the path to evaluate. */ dirname(p: string): string; /** * Return the last portion of a path. Similar to the Unix basename command. * Often used to extract the file name from a fully qualified path. * * @param p the path to evaluate. * @param ext optionally, an extension to remove from the result. */ basename(p: string, ext?: string): string; /** * Return the extension of the path, from the last '.' to end of string in the last portion of the path. * If there is no '.' in the last portion of the path or the first character of it is '.', then it returns an empty string * * @param p the path to evaluate. */ extname(p: string): string; /** * The platform-specific file separator. '\\' or '/'. */ readonly sep: string; /** * The platform-specific file delimiter. ';' or ':'. */ readonly delimiter: string; /** * Returns an object from a path string - the opposite of format(). * * @param pathString path to evaluate. */ parse(p: string): ParsedPath; /** * Returns a path string from an object - the opposite of parse(). * * @param pathString path to evaluate. */ format(pP: FormatInputPathObject): string; /** * On Windows systems only, returns an equivalent namespace-prefixed path for the given path. * If path is not a string, path will be returned without modifications. * This method is meaningful only on Windows system. * On POSIX systems, the method is non-operational and always returns path without modifications. */ toNamespacedPath(path: string): string; /** * Posix specific pathing. * Same as parent object on posix. */ readonly posix: PlatformPath; /** * Windows specific pathing. * Same as parent object on windows */ readonly win32: PlatformPath; } } const path: path.PlatformPath; export = path; } apollo-server-demo/node_modules/@types/node/LICENSE 0000644 0001750 0000144 00000002165 14000133675 021630 0 ustar andreh users MIT License Copyright (c) Microsoft Corporation. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE apollo-server-demo/node_modules/@types/node/punycode.d.ts 0000644 0001750 0000144 00000006127 14000133700 023232 0 ustar andreh users declare module "punycode" { /** * @deprecated since v7.0.0 * The version of the punycode module bundled in Node.js is being deprecated. * In a future major version of Node.js this module will be removed. * Users currently depending on the punycode module should switch to using * the userland-provided Punycode.js module instead. */ function decode(string: string): string; /** * @deprecated since v7.0.0 * The version of the punycode module bundled in Node.js is being deprecated. * In a future major version of Node.js this module will be removed. * Users currently depending on the punycode module should switch to using * the userland-provided Punycode.js module instead. */ function encode(string: string): string; /** * @deprecated since v7.0.0 * The version of the punycode module bundled in Node.js is being deprecated. * In a future major version of Node.js this module will be removed. * Users currently depending on the punycode module should switch to using * the userland-provided Punycode.js module instead. */ function toUnicode(domain: string): string; /** * @deprecated since v7.0.0 * The version of the punycode module bundled in Node.js is being deprecated. * In a future major version of Node.js this module will be removed. * Users currently depending on the punycode module should switch to using * the userland-provided Punycode.js module instead. */ function toASCII(domain: string): string; /** * @deprecated since v7.0.0 * The version of the punycode module bundled in Node.js is being deprecated. * In a future major version of Node.js this module will be removed. * Users currently depending on the punycode module should switch to using * the userland-provided Punycode.js module instead. */ const ucs2: ucs2; interface ucs2 { /** * @deprecated since v7.0.0 * The version of the punycode module bundled in Node.js is being deprecated. * In a future major version of Node.js this module will be removed. * Users currently depending on the punycode module should switch to using * the userland-provided Punycode.js module instead. */ decode(string: string): number[]; /** * @deprecated since v7.0.0 * The version of the punycode module bundled in Node.js is being deprecated. * In a future major version of Node.js this module will be removed. * Users currently depending on the punycode module should switch to using * the userland-provided Punycode.js module instead. */ encode(codePoints: ReadonlyArray<number>): string; } /** * @deprecated since v7.0.0 * The version of the punycode module bundled in Node.js is being deprecated. * In a future major version of Node.js this module will be removed. * Users currently depending on the punycode module should switch to using * the userland-provided Punycode.js module instead. */ const version: string; } apollo-server-demo/node_modules/@types/node/tty.d.ts 0000644 0001750 0000144 00000004612 14000133701 022222 0 ustar andreh users declare module "tty" { import * as net from "net"; function isatty(fd: number): boolean; class ReadStream extends net.Socket { constructor(fd: number, options?: net.SocketConstructorOpts); isRaw: boolean; setRawMode(mode: boolean): this; isTTY: boolean; } /** * -1 - to the left from cursor * 0 - the entire line * 1 - to the right from cursor */ type Direction = -1 | 0 | 1; class WriteStream extends net.Socket { constructor(fd: number); addListener(event: string, listener: (...args: any[]) => void): this; addListener(event: "resize", listener: () => void): this; emit(event: string | symbol, ...args: any[]): boolean; emit(event: "resize"): boolean; on(event: string, listener: (...args: any[]) => void): this; on(event: "resize", listener: () => void): this; once(event: string, listener: (...args: any[]) => void): this; once(event: "resize", listener: () => void): this; prependListener(event: string, listener: (...args: any[]) => void): this; prependListener(event: "resize", listener: () => void): this; prependOnceListener(event: string, listener: (...args: any[]) => void): this; prependOnceListener(event: "resize", listener: () => void): this; /** * Clears the current line of this WriteStream in a direction identified by `dir`. */ clearLine(dir: Direction, callback?: () => void): boolean; /** * Clears this `WriteStream` from the current cursor down. */ clearScreenDown(callback?: () => void): boolean; /** * Moves this WriteStream's cursor to the specified position. */ cursorTo(x: number, y?: number, callback?: () => void): boolean; cursorTo(x: number, callback: () => void): boolean; /** * Moves this WriteStream's cursor relative to its current position. */ moveCursor(dx: number, dy: number, callback?: () => void): boolean; /** * @default `process.env` */ getColorDepth(env?: {}): number; hasColors(depth?: number): boolean; hasColors(env?: {}): boolean; hasColors(depth: number, env?: {}): boolean; getWindowSize(): [number, number]; columns: number; rows: number; isTTY: boolean; } } apollo-server-demo/node_modules/@types/node/worker_threads.d.ts 0000644 0001750 0000144 00000026266 14000133701 024436 0 ustar andreh users declare module "worker_threads" { import { Context } from "vm"; import { EventEmitter } from "events"; import { Readable, Writable } from "stream"; import { URL } from "url"; import { FileHandle } from "fs/promises"; const isMainThread: boolean; const parentPort: null | MessagePort; const resourceLimits: ResourceLimits; const SHARE_ENV: unique symbol; const threadId: number; const workerData: any; class MessageChannel { readonly port1: MessagePort; readonly port2: MessagePort; } type TransferListItem = ArrayBuffer | MessagePort | FileHandle; class MessagePort extends EventEmitter { close(): void; postMessage(value: any, transferList?: ReadonlyArray<TransferListItem>): void; ref(): void; unref(): void; start(): void; addListener(event: "close", listener: () => void): this; addListener(event: "message", listener: (value: any) => void): this; addListener(event: "messageerror", listener: (error: Error) => void): this; addListener(event: string | symbol, listener: (...args: any[]) => void): this; emit(event: "close"): boolean; emit(event: "message", value: any): boolean; emit(event: "messageerror", error: Error): boolean; emit(event: string | symbol, ...args: any[]): boolean; on(event: "close", listener: () => void): this; on(event: "message", listener: (value: any) => void): this; on(event: "messageerror", listener: (error: Error) => void): this; on(event: string | symbol, listener: (...args: any[]) => void): this; once(event: "close", listener: () => void): this; once(event: "message", listener: (value: any) => void): this; once(event: "messageerror", listener: (error: Error) => void): this; once(event: string | symbol, listener: (...args: any[]) => void): this; prependListener(event: "close", listener: () => void): this; prependListener(event: "message", listener: (value: any) => void): this; prependListener(event: "messageerror", listener: (error: Error) => void): this; prependListener(event: string | symbol, listener: (...args: any[]) => void): this; prependOnceListener(event: "close", listener: () => void): this; prependOnceListener(event: "message", listener: (value: any) => void): this; prependOnceListener(event: "messageerror", listener: (error: Error) => void): this; prependOnceListener(event: string | symbol, listener: (...args: any[]) => void): this; removeListener(event: "close", listener: () => void): this; removeListener(event: "message", listener: (value: any) => void): this; removeListener(event: "messageerror", listener: (error: Error) => void): this; removeListener(event: string | symbol, listener: (...args: any[]) => void): this; off(event: "close", listener: () => void): this; off(event: "message", listener: (value: any) => void): this; off(event: "messageerror", listener: (error: Error) => void): this; off(event: string | symbol, listener: (...args: any[]) => void): this; } interface WorkerOptions { /** * List of arguments which would be stringified and appended to * `process.argv` in the worker. This is mostly similar to the `workerData` * but the values will be available on the global `process.argv` as if they * were passed as CLI options to the script. */ argv?: any[]; env?: NodeJS.Dict<string> | typeof SHARE_ENV; eval?: boolean; workerData?: any; stdin?: boolean; stdout?: boolean; stderr?: boolean; execArgv?: string[]; resourceLimits?: ResourceLimits; /** * Additional data to send in the first worker message. */ transferList?: TransferListItem[]; trackUnmanagedFds?: boolean; } interface ResourceLimits { /** * The maximum size of a heap space for recently created objects. */ maxYoungGenerationSizeMb?: number; /** * The maximum size of the main heap in MB. */ maxOldGenerationSizeMb?: number; /** * The size of a pre-allocated memory range used for generated code. */ codeRangeSizeMb?: number; /** * The default maximum stack size for the thread. Small values may lead to unusable Worker instances. * @default 4 */ stackSizeMb?: number; } class Worker extends EventEmitter { readonly stdin: Writable | null; readonly stdout: Readable; readonly stderr: Readable; readonly threadId: number; readonly resourceLimits?: ResourceLimits; /** * @param filename The path to the Worker’s main script or module. * Must be either an absolute path or a relative path (i.e. relative to the current working directory) starting with ./ or ../, * or a WHATWG URL object using file: protocol. If options.eval is true, this is a string containing JavaScript code rather than a path. */ constructor(filename: string | URL, options?: WorkerOptions); postMessage(value: any, transferList?: ReadonlyArray<TransferListItem>): void; ref(): void; unref(): void; /** * Stop all JavaScript execution in the worker thread as soon as possible. * Returns a Promise for the exit code that is fulfilled when the `exit` event is emitted. */ terminate(): Promise<number>; /** * Returns a readable stream for a V8 snapshot of the current state of the Worker. * See [`v8.getHeapSnapshot()`][] for more details. * * If the Worker thread is no longer running, which may occur before the * [`'exit'` event][] is emitted, the returned `Promise` will be rejected * immediately with an [`ERR_WORKER_NOT_RUNNING`][] error */ getHeapSnapshot(): Promise<Readable>; addListener(event: "error", listener: (err: Error) => void): this; addListener(event: "exit", listener: (exitCode: number) => void): this; addListener(event: "message", listener: (value: any) => void): this; addListener(event: "messageerror", listener: (error: Error) => void): this; addListener(event: "online", listener: () => void): this; addListener(event: string | symbol, listener: (...args: any[]) => void): this; emit(event: "error", err: Error): boolean; emit(event: "exit", exitCode: number): boolean; emit(event: "message", value: any): boolean; emit(event: "messageerror", error: Error): boolean; emit(event: "online"): boolean; emit(event: string | symbol, ...args: any[]): boolean; on(event: "error", listener: (err: Error) => void): this; on(event: "exit", listener: (exitCode: number) => void): this; on(event: "message", listener: (value: any) => void): this; on(event: "messageerror", listener: (error: Error) => void): this; on(event: "online", listener: () => void): this; on(event: string | symbol, listener: (...args: any[]) => void): this; once(event: "error", listener: (err: Error) => void): this; once(event: "exit", listener: (exitCode: number) => void): this; once(event: "message", listener: (value: any) => void): this; once(event: "messageerror", listener: (error: Error) => void): this; once(event: "online", listener: () => void): this; once(event: string | symbol, listener: (...args: any[]) => void): this; prependListener(event: "error", listener: (err: Error) => void): this; prependListener(event: "exit", listener: (exitCode: number) => void): this; prependListener(event: "message", listener: (value: any) => void): this; prependListener(event: "messageerror", listener: (error: Error) => void): this; prependListener(event: "online", listener: () => void): this; prependListener(event: string | symbol, listener: (...args: any[]) => void): this; prependOnceListener(event: "error", listener: (err: Error) => void): this; prependOnceListener(event: "exit", listener: (exitCode: number) => void): this; prependOnceListener(event: "message", listener: (value: any) => void): this; prependOnceListener(event: "messageerror", listener: (error: Error) => void): this; prependOnceListener(event: "online", listener: () => void): this; prependOnceListener(event: string | symbol, listener: (...args: any[]) => void): this; removeListener(event: "error", listener: (err: Error) => void): this; removeListener(event: "exit", listener: (exitCode: number) => void): this; removeListener(event: "message", listener: (value: any) => void): this; removeListener(event: "messageerror", listener: (error: Error) => void): this; removeListener(event: "online", listener: () => void): this; removeListener(event: string | symbol, listener: (...args: any[]) => void): this; off(event: "error", listener: (err: Error) => void): this; off(event: "exit", listener: (exitCode: number) => void): this; off(event: "message", listener: (value: any) => void): this; off(event: "messageerror", listener: (error: Error) => void): this; off(event: "online", listener: () => void): this; off(event: string | symbol, listener: (...args: any[]) => void): this; } /** * Mark an object as not transferable. * If `object` occurs in the transfer list of a `port.postMessage()` call, it will be ignored. * * In particular, this makes sense for objects that can be cloned, rather than transferred, * and which are used by other objects on the sending side. For example, Node.js marks * the `ArrayBuffer`s it uses for its Buffer pool with this. * * This operation cannot be undone. */ function markAsUntransferable(object: object): void; /** * Transfer a `MessagePort` to a different `vm` Context. The original `port` * object will be rendered unusable, and the returned `MessagePort` instance will * take its place. * * The returned `MessagePort` will be an object in the target context, and will * inherit from its global `Object` class. Objects passed to the * `port.onmessage()` listener will also be created in the target context * and inherit from its global `Object` class. * * However, the created `MessagePort` will no longer inherit from * `EventEmitter`, and only `port.onmessage()` can be used to receive * events using it. */ function moveMessagePortToContext(port: MessagePort, context: Context): MessagePort; /** * Receive a single message from a given `MessagePort`. If no message is available, * `undefined` is returned, otherwise an object with a single `message` property * that contains the message payload, corresponding to the oldest message in the * `MessagePort`’s queue. */ function receiveMessageOnPort(port: MessagePort): { message: any } | undefined; } apollo-server-demo/node_modules/@types/node/base.d.ts 0000644 0001750 0000144 00000001611 14000133700 022307 0 ustar andreh users // NOTE: These definitions support NodeJS and TypeScript 3.7. // NOTE: TypeScript version-specific augmentations can be found in the following paths: // - ~/base.d.ts - Shared definitions common to all TypeScript versions // - ~/index.d.ts - Definitions specific to TypeScript 2.1 // - ~/ts3.7/base.d.ts - Definitions specific to TypeScript 3.7 // - ~/ts3.7/index.d.ts - Definitions specific to TypeScript 3.7 with assert pulled in // Reference required types from the default lib: /// <reference lib="es2018" /> /// <reference lib="esnext.asynciterable" /> /// <reference lib="esnext.intl" /> /// <reference lib="esnext.bigint" /> // Base definitions for all NodeJS modules that are not specific to any version of TypeScript: /// <reference path="ts3.6/base.d.ts" /> // TypeScript 3.7-specific augmentations: /// <reference path="assert.d.ts" /> apollo-server-demo/node_modules/@types/node/index.d.ts 0000644 0001750 0000144 00000007361 14000133700 022514 0 ustar andreh users // Type definitions for non-npm package Node.js 14.14 // Project: http://nodejs.org/ // Definitions by: Microsoft TypeScript <https://github.com/Microsoft> // DefinitelyTyped <https://github.com/DefinitelyTyped> // Alberto Schiabel <https://github.com/jkomyno> // Alexander T. <https://github.com/a-tarasyuk> // Alvis HT Tang <https://github.com/alvis> // Andrew Makarov <https://github.com/r3nya> // Benjamin Toueg <https://github.com/btoueg> // Bruno Scheufler <https://github.com/brunoscheufler> // Chigozirim C. <https://github.com/smac89> // David Junger <https://github.com/touffy> // Deividas Bakanas <https://github.com/DeividasBakanas> // Eugene Y. Q. Shen <https://github.com/eyqs> // Flarna <https://github.com/Flarna> // Hannes Magnusson <https://github.com/Hannes-Magnusson-CK> // Hoà ng Văn Khải <https://github.com/KSXGitHub> // Huw <https://github.com/hoo29> // Kelvin Jin <https://github.com/kjin> // Klaus Meinhardt <https://github.com/ajafff> // Lishude <https://github.com/islishude> // Mariusz Wiktorczyk <https://github.com/mwiktorczyk> // Mohsen Azimi <https://github.com/mohsen1> // Nicolas Even <https://github.com/n-e> // Nikita Galkin <https://github.com/galkin> // Parambir Singh <https://github.com/parambirs> // Sebastian Silbermann <https://github.com/eps1lon> // Simon Schick <https://github.com/SimonSchick> // Thomas den Hollander <https://github.com/ThomasdenH> // Wilco Bakker <https://github.com/WilcoBakker> // wwwy3y3 <https://github.com/wwwy3y3> // Samuel Ainsworth <https://github.com/samuela> // Kyle Uehlein <https://github.com/kuehlein> // Jordi Oliveras Rovira <https://github.com/j-oliveras> // Thanik Bhongbhibhat <https://github.com/bhongy> // Marcin Kopacz <https://github.com/chyzwar> // Trivikram Kamat <https://github.com/trivikr> // Minh Son Nguyen <https://github.com/nguymin4> // Junxiao Shi <https://github.com/yoursunny> // Ilia Baryshnikov <https://github.com/qwelias> // ExE Boss <https://github.com/ExE-Boss> // Surasak Chaisurin <https://github.com/Ryan-Willpower> // Piotr Błażejewicz <https://github.com/peterblazejewicz> // Anna Henningsen <https://github.com/addaleax> // Jason Kwok <https://github.com/JasonHK> // Victor Perin <https://github.com/victorperin> // Yongsheng Zhang <https://github.com/ZYSzys> // Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped // NOTE: These definitions support NodeJS and TypeScript 3.7. // Typically type modifications should be made in base.d.ts instead of here /// <reference path="base.d.ts" /> // NOTE: TypeScript version-specific augmentations can be found in the following paths: // - ~/base.d.ts - Shared definitions common to all TypeScript versions // - ~/index.d.ts - Definitions specific to TypeScript 2.8 // - ~/ts3.5/index.d.ts - Definitions specific to TypeScript 3.5 // NOTE: Augmentations for TypeScript 3.5 and later should use individual files for overrides // within the respective ~/ts3.5 (or later) folder. However, this is disallowed for versions // prior to TypeScript 3.5, so the older definitions will be found here. apollo-server-demo/node_modules/@types/node/README.md 0000644 0001750 0000144 00000005317 14000133675 022104 0 ustar andreh users # Installation > `npm install --save @types/node` # Summary This package contains type definitions for Node.js (http://nodejs.org/). # Details Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/node. ### Additional Details * Last updated: Thu, 14 Jan 2021 21:29:33 GMT * Dependencies: none * Global values: `Buffer`, `__dirname`, `__filename`, `clearImmediate`, `clearInterval`, `clearTimeout`, `console`, `exports`, `global`, `module`, `process`, `queueMicrotask`, `require`, `setImmediate`, `setInterval`, `setTimeout` # Credits These definitions were written by [Microsoft TypeScript](https://github.com/Microsoft), [DefinitelyTyped](https://github.com/DefinitelyTyped), [Alberto Schiabel](https://github.com/jkomyno), [Alexander T.](https://github.com/a-tarasyuk), [Alvis HT Tang](https://github.com/alvis), [Andrew Makarov](https://github.com/r3nya), [Benjamin Toueg](https://github.com/btoueg), [Bruno Scheufler](https://github.com/brunoscheufler), [Chigozirim C.](https://github.com/smac89), [David Junger](https://github.com/touffy), [Deividas Bakanas](https://github.com/DeividasBakanas), [Eugene Y. Q. Shen](https://github.com/eyqs), [Flarna](https://github.com/Flarna), [Hannes Magnusson](https://github.com/Hannes-Magnusson-CK), [Hoà ng Văn Khải](https://github.com/KSXGitHub), [Huw](https://github.com/hoo29), [Kelvin Jin](https://github.com/kjin), [Klaus Meinhardt](https://github.com/ajafff), [Lishude](https://github.com/islishude), [Mariusz Wiktorczyk](https://github.com/mwiktorczyk), [Mohsen Azimi](https://github.com/mohsen1), [Nicolas Even](https://github.com/n-e), [Nikita Galkin](https://github.com/galkin), [Parambir Singh](https://github.com/parambirs), [Sebastian Silbermann](https://github.com/eps1lon), [Simon Schick](https://github.com/SimonSchick), [Thomas den Hollander](https://github.com/ThomasdenH), [Wilco Bakker](https://github.com/WilcoBakker), [wwwy3y3](https://github.com/wwwy3y3), [Samuel Ainsworth](https://github.com/samuela), [Kyle Uehlein](https://github.com/kuehlein), [Jordi Oliveras Rovira](https://github.com/j-oliveras), [Thanik Bhongbhibhat](https://github.com/bhongy), [Marcin Kopacz](https://github.com/chyzwar), [Trivikram Kamat](https://github.com/trivikr), [Minh Son Nguyen](https://github.com/nguymin4), [Junxiao Shi](https://github.com/yoursunny), [Ilia Baryshnikov](https://github.com/qwelias), [ExE Boss](https://github.com/ExE-Boss), [Surasak Chaisurin](https://github.com/Ryan-Willpower), [Piotr Błażejewicz](https://github.com/peterblazejewicz), [Anna Henningsen](https://github.com/addaleax), [Jason Kwok](https://github.com/JasonHK), [Victor Perin](https://github.com/victorperin), and [Yongsheng Zhang](https://github.com/ZYSzys). apollo-server-demo/node_modules/@types/node/util.d.ts 0000644 0001750 0000144 00000030721 14000133701 022357 0 ustar andreh users declare module "util" { interface InspectOptions extends NodeJS.InspectOptions { } type Style = 'special' | 'number' | 'bigint' | 'boolean' | 'undefined' | 'null' | 'string' | 'symbol' | 'date' | 'regexp' | 'module'; type CustomInspectFunction = (depth: number, options: InspectOptionsStylized) => string; interface InspectOptionsStylized extends InspectOptions { stylize(text: string, styleType: Style): string; } function format(format?: any, ...param: any[]): string; function formatWithOptions(inspectOptions: InspectOptions, format?: any, ...param: any[]): string; /** @deprecated since v0.11.3 - use a third party module instead. */ function log(string: string): void; function inspect(object: any, showHidden?: boolean, depth?: number | null, color?: boolean): string; function inspect(object: any, options: InspectOptions): string; namespace inspect { let colors: NodeJS.Dict<[number, number]>; let styles: { [K in Style]: string }; let defaultOptions: InspectOptions; /** * Allows changing inspect settings from the repl. */ let replDefaults: InspectOptions; const custom: unique symbol; } /** @deprecated since v4.0.0 - use `Array.isArray()` instead. */ function isArray(object: any): object is any[]; /** @deprecated since v4.0.0 - use `util.types.isRegExp()` instead. */ function isRegExp(object: any): object is RegExp; /** @deprecated since v4.0.0 - use `util.types.isDate()` instead. */ function isDate(object: any): object is Date; /** @deprecated since v4.0.0 - use `util.types.isNativeError()` instead. */ function isError(object: any): object is Error; function inherits(constructor: any, superConstructor: any): void; function debuglog(key: string): (msg: string, ...param: any[]) => void; /** @deprecated since v4.0.0 - use `typeof value === 'boolean'` instead. */ function isBoolean(object: any): object is boolean; /** @deprecated since v4.0.0 - use `Buffer.isBuffer()` instead. */ function isBuffer(object: any): object is Buffer; /** @deprecated since v4.0.0 - use `typeof value === 'function'` instead. */ function isFunction(object: any): boolean; /** @deprecated since v4.0.0 - use `value === null` instead. */ function isNull(object: any): object is null; /** @deprecated since v4.0.0 - use `value === null || value === undefined` instead. */ function isNullOrUndefined(object: any): object is null | undefined; /** @deprecated since v4.0.0 - use `typeof value === 'number'` instead. */ function isNumber(object: any): object is number; /** @deprecated since v4.0.0 - use `value !== null && typeof value === 'object'` instead. */ function isObject(object: any): boolean; /** @deprecated since v4.0.0 - use `(typeof value !== 'object' && typeof value !== 'function') || value === null` instead. */ function isPrimitive(object: any): boolean; /** @deprecated since v4.0.0 - use `typeof value === 'string'` instead. */ function isString(object: any): object is string; /** @deprecated since v4.0.0 - use `typeof value === 'symbol'` instead. */ function isSymbol(object: any): object is symbol; /** @deprecated since v4.0.0 - use `value === undefined` instead. */ function isUndefined(object: any): object is undefined; function deprecate<T extends Function>(fn: T, message: string, code?: string): T; function isDeepStrictEqual(val1: any, val2: any): boolean; function callbackify(fn: () => Promise<void>): (callback: (err: NodeJS.ErrnoException) => void) => void; function callbackify<TResult>(fn: () => Promise<TResult>): (callback: (err: NodeJS.ErrnoException, result: TResult) => void) => void; function callbackify<T1>(fn: (arg1: T1) => Promise<void>): (arg1: T1, callback: (err: NodeJS.ErrnoException) => void) => void; function callbackify<T1, TResult>(fn: (arg1: T1) => Promise<TResult>): (arg1: T1, callback: (err: NodeJS.ErrnoException, result: TResult) => void) => void; function callbackify<T1, T2>(fn: (arg1: T1, arg2: T2) => Promise<void>): (arg1: T1, arg2: T2, callback: (err: NodeJS.ErrnoException) => void) => void; function callbackify<T1, T2, TResult>(fn: (arg1: T1, arg2: T2) => Promise<TResult>): (arg1: T1, arg2: T2, callback: (err: NodeJS.ErrnoException | null, result: TResult) => void) => void; function callbackify<T1, T2, T3>(fn: (arg1: T1, arg2: T2, arg3: T3) => Promise<void>): (arg1: T1, arg2: T2, arg3: T3, callback: (err: NodeJS.ErrnoException) => void) => void; function callbackify<T1, T2, T3, TResult>( fn: (arg1: T1, arg2: T2, arg3: T3) => Promise<TResult>): (arg1: T1, arg2: T2, arg3: T3, callback: (err: NodeJS.ErrnoException | null, result: TResult) => void) => void; function callbackify<T1, T2, T3, T4>( fn: (arg1: T1, arg2: T2, arg3: T3, arg4: T4) => Promise<void>): (arg1: T1, arg2: T2, arg3: T3, arg4: T4, callback: (err: NodeJS.ErrnoException) => void) => void; function callbackify<T1, T2, T3, T4, TResult>( fn: (arg1: T1, arg2: T2, arg3: T3, arg4: T4) => Promise<TResult>): (arg1: T1, arg2: T2, arg3: T3, arg4: T4, callback: (err: NodeJS.ErrnoException | null, result: TResult) => void) => void; function callbackify<T1, T2, T3, T4, T5>( fn: (arg1: T1, arg2: T2, arg3: T3, arg4: T4, arg5: T5) => Promise<void>): (arg1: T1, arg2: T2, arg3: T3, arg4: T4, arg5: T5, callback: (err: NodeJS.ErrnoException) => void) => void; function callbackify<T1, T2, T3, T4, T5, TResult>( fn: (arg1: T1, arg2: T2, arg3: T3, arg4: T4, arg5: T5) => Promise<TResult>, ): (arg1: T1, arg2: T2, arg3: T3, arg4: T4, arg5: T5, callback: (err: NodeJS.ErrnoException | null, result: TResult) => void) => void; function callbackify<T1, T2, T3, T4, T5, T6>( fn: (arg1: T1, arg2: T2, arg3: T3, arg4: T4, arg5: T5, arg6: T6) => Promise<void>, ): (arg1: T1, arg2: T2, arg3: T3, arg4: T4, arg5: T5, arg6: T6, callback: (err: NodeJS.ErrnoException) => void) => void; function callbackify<T1, T2, T3, T4, T5, T6, TResult>( fn: (arg1: T1, arg2: T2, arg3: T3, arg4: T4, arg5: T5, arg6: T6) => Promise<TResult> ): (arg1: T1, arg2: T2, arg3: T3, arg4: T4, arg5: T5, arg6: T6, callback: (err: NodeJS.ErrnoException | null, result: TResult) => void) => void; interface CustomPromisifyLegacy<TCustom extends Function> extends Function { __promisify__: TCustom; } interface CustomPromisifySymbol<TCustom extends Function> extends Function { [promisify.custom]: TCustom; } type CustomPromisify<TCustom extends Function> = CustomPromisifySymbol<TCustom> | CustomPromisifyLegacy<TCustom>; function promisify<TCustom extends Function>(fn: CustomPromisify<TCustom>): TCustom; function promisify<TResult>(fn: (callback: (err: any, result: TResult) => void) => void): () => Promise<TResult>; function promisify(fn: (callback: (err?: any) => void) => void): () => Promise<void>; function promisify<T1, TResult>(fn: (arg1: T1, callback: (err: any, result: TResult) => void) => void): (arg1: T1) => Promise<TResult>; function promisify<T1>(fn: (arg1: T1, callback: (err?: any) => void) => void): (arg1: T1) => Promise<void>; function promisify<T1, T2, TResult>(fn: (arg1: T1, arg2: T2, callback: (err: any, result: TResult) => void) => void): (arg1: T1, arg2: T2) => Promise<TResult>; function promisify<T1, T2>(fn: (arg1: T1, arg2: T2, callback: (err?: any) => void) => void): (arg1: T1, arg2: T2) => Promise<void>; function promisify<T1, T2, T3, TResult>(fn: (arg1: T1, arg2: T2, arg3: T3, callback: (err: any, result: TResult) => void) => void): (arg1: T1, arg2: T2, arg3: T3) => Promise<TResult>; function promisify<T1, T2, T3>(fn: (arg1: T1, arg2: T2, arg3: T3, callback: (err?: any) => void) => void): (arg1: T1, arg2: T2, arg3: T3) => Promise<void>; function promisify<T1, T2, T3, T4, TResult>( fn: (arg1: T1, arg2: T2, arg3: T3, arg4: T4, callback: (err: any, result: TResult) => void) => void, ): (arg1: T1, arg2: T2, arg3: T3, arg4: T4) => Promise<TResult>; function promisify<T1, T2, T3, T4>(fn: (arg1: T1, arg2: T2, arg3: T3, arg4: T4, callback: (err?: any) => void) => void): (arg1: T1, arg2: T2, arg3: T3, arg4: T4) => Promise<void>; function promisify<T1, T2, T3, T4, T5, TResult>( fn: (arg1: T1, arg2: T2, arg3: T3, arg4: T4, arg5: T5, callback: (err: any, result: TResult) => void) => void, ): (arg1: T1, arg2: T2, arg3: T3, arg4: T4, arg5: T5) => Promise<TResult>; function promisify<T1, T2, T3, T4, T5>( fn: (arg1: T1, arg2: T2, arg3: T3, arg4: T4, arg5: T5, callback: (err?: any) => void) => void, ): (arg1: T1, arg2: T2, arg3: T3, arg4: T4, arg5: T5) => Promise<void>; function promisify(fn: Function): Function; namespace promisify { const custom: unique symbol; } namespace types { function isAnyArrayBuffer(object: any): object is ArrayBufferLike; function isArgumentsObject(object: any): object is IArguments; function isArrayBuffer(object: any): object is ArrayBuffer; function isArrayBufferView(object: any): object is NodeJS.ArrayBufferView; function isAsyncFunction(object: any): boolean; function isBigInt64Array(value: any): value is BigInt64Array; function isBigUint64Array(value: any): value is BigUint64Array; function isBooleanObject(object: any): object is Boolean; function isBoxedPrimitive(object: any): object is String | Number | BigInt | Boolean | Symbol; function isDataView(object: any): object is DataView; function isDate(object: any): object is Date; function isExternal(object: any): boolean; function isFloat32Array(object: any): object is Float32Array; function isFloat64Array(object: any): object is Float64Array; function isGeneratorFunction(object: any): object is GeneratorFunction; function isGeneratorObject(object: any): object is Generator; function isInt8Array(object: any): object is Int8Array; function isInt16Array(object: any): object is Int16Array; function isInt32Array(object: any): object is Int32Array; function isMap<T>( object: T | {}, ): object is T extends ReadonlyMap<any, any> ? unknown extends T ? never : ReadonlyMap<any, any> : Map<any, any>; function isMapIterator(object: any): boolean; function isModuleNamespaceObject(value: any): boolean; function isNativeError(object: any): object is Error; function isNumberObject(object: any): object is Number; function isPromise(object: any): object is Promise<any>; function isProxy(object: any): boolean; function isRegExp(object: any): object is RegExp; function isSet<T>( object: T | {}, ): object is T extends ReadonlySet<any> ? unknown extends T ? never : ReadonlySet<any> : Set<any>; function isSetIterator(object: any): boolean; function isSharedArrayBuffer(object: any): object is SharedArrayBuffer; function isStringObject(object: any): object is String; function isSymbolObject(object: any): object is Symbol; function isTypedArray(object: any): object is NodeJS.TypedArray; function isUint8Array(object: any): object is Uint8Array; function isUint8ClampedArray(object: any): object is Uint8ClampedArray; function isUint16Array(object: any): object is Uint16Array; function isUint32Array(object: any): object is Uint32Array; function isWeakMap(object: any): object is WeakMap<any, any>; function isWeakSet(object: any): object is WeakSet<any>; } class TextDecoder { readonly encoding: string; readonly fatal: boolean; readonly ignoreBOM: boolean; constructor( encoding?: string, options?: { fatal?: boolean; ignoreBOM?: boolean } ); decode( input?: NodeJS.ArrayBufferView | ArrayBuffer | null, options?: { stream?: boolean } ): string; } interface EncodeIntoResult { /** * The read Unicode code units of input. */ read: number; /** * The written UTF-8 bytes of output. */ written: number; } class TextEncoder { readonly encoding: string; encode(input?: string): Uint8Array; encodeInto(input: string, output: Uint8Array): EncodeIntoResult; } } apollo-server-demo/node_modules/@types/node/ts3.6/ 0000755 0001750 0000144 00000000000 14067647700 021510 5 ustar andreh users apollo-server-demo/node_modules/@types/node/ts3.6/base.d.ts 0000644 0001750 0000144 00000001750 14000133701 023171 0 ustar andreh users // NOTE: These definitions support NodeJS and TypeScript 3.5. // NOTE: TypeScript version-specific augmentations can be found in the following paths: // - ~/base.d.ts - Shared definitions common to all TypeScript versions // - ~/index.d.ts - Definitions specific to TypeScript 2.1 // - ~/ts3.5/base.d.ts - Definitions specific to TypeScript 3.5 // - ~/ts3.5/index.d.ts - Definitions specific to TypeScript 3.5 with assert pulled in // Reference required types from the default lib: /// <reference lib="es2018" /> /// <reference lib="esnext.asynciterable" /> /// <reference lib="esnext.intl" /> /// <reference lib="esnext.bigint" /> // Base definitions for all NodeJS modules that are not specific to any version of TypeScript: /// <reference path="../ts3.4/base.d.ts" /> // TypeScript 3.5-specific augmentations: /// <reference path="../globals.global.d.ts" /> // TypeScript 3.5-specific augmentations: /// <reference path="../wasi.d.ts" /> apollo-server-demo/node_modules/@types/node/ts3.6/index.d.ts 0000644 0001750 0000144 00000000457 14000133701 023371 0 ustar andreh users // NOTE: These definitions support NodeJS and TypeScript 3.5 - 3.6. // This is required to enable typing assert in ts3.7 without causing errors // Typically type modifications should be made in base.d.ts instead of here /// <reference path="base.d.ts" /> /// <reference path="../ts3.4/assert.d.ts" /> apollo-server-demo/node_modules/@types/node/stream.d.ts 0000644 0001750 0000144 00000045656 14000133700 022711 0 ustar andreh users declare module 'stream' { import EventEmitter = require('events'); class internal extends EventEmitter { pipe<T extends NodeJS.WritableStream>(destination: T, options?: { end?: boolean; }): T; } namespace internal { class Stream extends internal { constructor(opts?: ReadableOptions); } interface ReadableOptions { highWaterMark?: number; encoding?: BufferEncoding; objectMode?: boolean; read?(this: Readable, size: number): void; destroy?(this: Readable, error: Error | null, callback: (error: Error | null) => void): void; autoDestroy?: boolean; } class Readable extends Stream implements NodeJS.ReadableStream { /** * A utility method for creating Readable Streams out of iterators. */ static from(iterable: Iterable<any> | AsyncIterable<any>, options?: ReadableOptions): Readable; readable: boolean; readonly readableEncoding: BufferEncoding | null; readonly readableEnded: boolean; readonly readableFlowing: boolean | null; readonly readableHighWaterMark: number; readonly readableLength: number; readonly readableObjectMode: boolean; destroyed: boolean; constructor(opts?: ReadableOptions); _read(size: number): void; read(size?: number): any; setEncoding(encoding: BufferEncoding): this; pause(): this; resume(): this; isPaused(): boolean; unpipe(destination?: NodeJS.WritableStream): this; unshift(chunk: any, encoding?: BufferEncoding): void; wrap(oldStream: NodeJS.ReadableStream): this; push(chunk: any, encoding?: BufferEncoding): boolean; _destroy(error: Error | null, callback: (error?: Error | null) => void): void; destroy(error?: Error): void; /** * Event emitter * The defined events on documents including: * 1. close * 2. data * 3. end * 4. error * 5. pause * 6. readable * 7. resume */ addListener(event: "close", listener: () => void): this; addListener(event: "data", listener: (chunk: any) => void): this; addListener(event: "end", listener: () => void): this; addListener(event: "error", listener: (err: Error) => void): this; addListener(event: "pause", listener: () => void): this; addListener(event: "readable", listener: () => void): this; addListener(event: "resume", listener: () => void): this; addListener(event: string | symbol, listener: (...args: any[]) => void): this; emit(event: "close"): boolean; emit(event: "data", chunk: any): boolean; emit(event: "end"): boolean; emit(event: "error", err: Error): boolean; emit(event: "pause"): boolean; emit(event: "readable"): boolean; emit(event: "resume"): boolean; emit(event: string | symbol, ...args: any[]): boolean; on(event: "close", listener: () => void): this; on(event: "data", listener: (chunk: any) => void): this; on(event: "end", listener: () => void): this; on(event: "error", listener: (err: Error) => void): this; on(event: "pause", listener: () => void): this; on(event: "readable", listener: () => void): this; on(event: "resume", listener: () => void): this; on(event: string | symbol, listener: (...args: any[]) => void): this; once(event: "close", listener: () => void): this; once(event: "data", listener: (chunk: any) => void): this; once(event: "end", listener: () => void): this; once(event: "error", listener: (err: Error) => void): this; once(event: "pause", listener: () => void): this; once(event: "readable", listener: () => void): this; once(event: "resume", listener: () => void): this; once(event: string | symbol, listener: (...args: any[]) => void): this; prependListener(event: "close", listener: () => void): this; prependListener(event: "data", listener: (chunk: any) => void): this; prependListener(event: "end", listener: () => void): this; prependListener(event: "error", listener: (err: Error) => void): this; prependListener(event: "pause", listener: () => void): this; prependListener(event: "readable", listener: () => void): this; prependListener(event: "resume", listener: () => void): this; prependListener(event: string | symbol, listener: (...args: any[]) => void): this; prependOnceListener(event: "close", listener: () => void): this; prependOnceListener(event: "data", listener: (chunk: any) => void): this; prependOnceListener(event: "end", listener: () => void): this; prependOnceListener(event: "error", listener: (err: Error) => void): this; prependOnceListener(event: "pause", listener: () => void): this; prependOnceListener(event: "readable", listener: () => void): this; prependOnceListener(event: "resume", listener: () => void): this; prependOnceListener(event: string | symbol, listener: (...args: any[]) => void): this; removeListener(event: "close", listener: () => void): this; removeListener(event: "data", listener: (chunk: any) => void): this; removeListener(event: "end", listener: () => void): this; removeListener(event: "error", listener: (err: Error) => void): this; removeListener(event: "pause", listener: () => void): this; removeListener(event: "readable", listener: () => void): this; removeListener(event: "resume", listener: () => void): this; removeListener(event: string | symbol, listener: (...args: any[]) => void): this; [Symbol.asyncIterator](): AsyncIterableIterator<any>; } interface WritableOptions { highWaterMark?: number; decodeStrings?: boolean; defaultEncoding?: BufferEncoding; objectMode?: boolean; emitClose?: boolean; write?(this: Writable, chunk: any, encoding: BufferEncoding, callback: (error?: Error | null) => void): void; writev?(this: Writable, chunks: Array<{ chunk: any, encoding: BufferEncoding }>, callback: (error?: Error | null) => void): void; destroy?(this: Writable, error: Error | null, callback: (error: Error | null) => void): void; final?(this: Writable, callback: (error?: Error | null) => void): void; autoDestroy?: boolean; } class Writable extends Stream implements NodeJS.WritableStream { readonly writable: boolean; readonly writableEnded: boolean; readonly writableFinished: boolean; readonly writableHighWaterMark: number; readonly writableLength: number; readonly writableObjectMode: boolean; readonly writableCorked: number; destroyed: boolean; constructor(opts?: WritableOptions); _write(chunk: any, encoding: BufferEncoding, callback: (error?: Error | null) => void): void; _writev?(chunks: Array<{ chunk: any, encoding: BufferEncoding }>, callback: (error?: Error | null) => void): void; _destroy(error: Error | null, callback: (error?: Error | null) => void): void; _final(callback: (error?: Error | null) => void): void; write(chunk: any, cb?: (error: Error | null | undefined) => void): boolean; write(chunk: any, encoding: BufferEncoding, cb?: (error: Error | null | undefined) => void): boolean; setDefaultEncoding(encoding: BufferEncoding): this; end(cb?: () => void): void; end(chunk: any, cb?: () => void): void; end(chunk: any, encoding: BufferEncoding, cb?: () => void): void; cork(): void; uncork(): void; destroy(error?: Error): void; /** * Event emitter * The defined events on documents including: * 1. close * 2. drain * 3. error * 4. finish * 5. pipe * 6. unpipe */ addListener(event: "close", listener: () => void): this; addListener(event: "drain", listener: () => void): this; addListener(event: "error", listener: (err: Error) => void): this; addListener(event: "finish", listener: () => void): this; addListener(event: "pipe", listener: (src: Readable) => void): this; addListener(event: "unpipe", listener: (src: Readable) => void): this; addListener(event: string | symbol, listener: (...args: any[]) => void): this; emit(event: "close"): boolean; emit(event: "drain"): boolean; emit(event: "error", err: Error): boolean; emit(event: "finish"): boolean; emit(event: "pipe", src: Readable): boolean; emit(event: "unpipe", src: Readable): boolean; emit(event: string | symbol, ...args: any[]): boolean; on(event: "close", listener: () => void): this; on(event: "drain", listener: () => void): this; on(event: "error", listener: (err: Error) => void): this; on(event: "finish", listener: () => void): this; on(event: "pipe", listener: (src: Readable) => void): this; on(event: "unpipe", listener: (src: Readable) => void): this; on(event: string | symbol, listener: (...args: any[]) => void): this; once(event: "close", listener: () => void): this; once(event: "drain", listener: () => void): this; once(event: "error", listener: (err: Error) => void): this; once(event: "finish", listener: () => void): this; once(event: "pipe", listener: (src: Readable) => void): this; once(event: "unpipe", listener: (src: Readable) => void): this; once(event: string | symbol, listener: (...args: any[]) => void): this; prependListener(event: "close", listener: () => void): this; prependListener(event: "drain", listener: () => void): this; prependListener(event: "error", listener: (err: Error) => void): this; prependListener(event: "finish", listener: () => void): this; prependListener(event: "pipe", listener: (src: Readable) => void): this; prependListener(event: "unpipe", listener: (src: Readable) => void): this; prependListener(event: string | symbol, listener: (...args: any[]) => void): this; prependOnceListener(event: "close", listener: () => void): this; prependOnceListener(event: "drain", listener: () => void): this; prependOnceListener(event: "error", listener: (err: Error) => void): this; prependOnceListener(event: "finish", listener: () => void): this; prependOnceListener(event: "pipe", listener: (src: Readable) => void): this; prependOnceListener(event: "unpipe", listener: (src: Readable) => void): this; prependOnceListener(event: string | symbol, listener: (...args: any[]) => void): this; removeListener(event: "close", listener: () => void): this; removeListener(event: "drain", listener: () => void): this; removeListener(event: "error", listener: (err: Error) => void): this; removeListener(event: "finish", listener: () => void): this; removeListener(event: "pipe", listener: (src: Readable) => void): this; removeListener(event: "unpipe", listener: (src: Readable) => void): this; removeListener(event: string | symbol, listener: (...args: any[]) => void): this; } interface DuplexOptions extends ReadableOptions, WritableOptions { allowHalfOpen?: boolean; readableObjectMode?: boolean; writableObjectMode?: boolean; readableHighWaterMark?: number; writableHighWaterMark?: number; writableCorked?: number; read?(this: Duplex, size: number): void; write?(this: Duplex, chunk: any, encoding: BufferEncoding, callback: (error?: Error | null) => void): void; writev?(this: Duplex, chunks: Array<{ chunk: any, encoding: BufferEncoding }>, callback: (error?: Error | null) => void): void; final?(this: Duplex, callback: (error?: Error | null) => void): void; destroy?(this: Duplex, error: Error | null, callback: (error: Error | null) => void): void; } // Note: Duplex extends both Readable and Writable. class Duplex extends Readable implements Writable { readonly writable: boolean; readonly writableEnded: boolean; readonly writableFinished: boolean; readonly writableHighWaterMark: number; readonly writableLength: number; readonly writableObjectMode: boolean; readonly writableCorked: number; constructor(opts?: DuplexOptions); _write(chunk: any, encoding: BufferEncoding, callback: (error?: Error | null) => void): void; _writev?(chunks: Array<{ chunk: any, encoding: BufferEncoding }>, callback: (error?: Error | null) => void): void; _destroy(error: Error | null, callback: (error: Error | null) => void): void; _final(callback: (error?: Error | null) => void): void; write(chunk: any, encoding?: BufferEncoding, cb?: (error: Error | null | undefined) => void): boolean; write(chunk: any, cb?: (error: Error | null | undefined) => void): boolean; setDefaultEncoding(encoding: BufferEncoding): this; end(cb?: () => void): void; end(chunk: any, cb?: () => void): void; end(chunk: any, encoding?: BufferEncoding, cb?: () => void): void; cork(): void; uncork(): void; } type TransformCallback = (error?: Error | null, data?: any) => void; interface TransformOptions extends DuplexOptions { read?(this: Transform, size: number): void; write?(this: Transform, chunk: any, encoding: BufferEncoding, callback: (error?: Error | null) => void): void; writev?(this: Transform, chunks: Array<{ chunk: any, encoding: BufferEncoding }>, callback: (error?: Error | null) => void): void; final?(this: Transform, callback: (error?: Error | null) => void): void; destroy?(this: Transform, error: Error | null, callback: (error: Error | null) => void): void; transform?(this: Transform, chunk: any, encoding: BufferEncoding, callback: TransformCallback): void; flush?(this: Transform, callback: TransformCallback): void; } class Transform extends Duplex { constructor(opts?: TransformOptions); _transform(chunk: any, encoding: BufferEncoding, callback: TransformCallback): void; _flush(callback: TransformCallback): void; } class PassThrough extends Transform { } interface FinishedOptions { error?: boolean; readable?: boolean; writable?: boolean; } function finished(stream: NodeJS.ReadableStream | NodeJS.WritableStream | NodeJS.ReadWriteStream, options: FinishedOptions, callback: (err?: NodeJS.ErrnoException | null) => void): () => void; function finished(stream: NodeJS.ReadableStream | NodeJS.WritableStream | NodeJS.ReadWriteStream, callback: (err?: NodeJS.ErrnoException | null) => void): () => void; namespace finished { function __promisify__(stream: NodeJS.ReadableStream | NodeJS.WritableStream | NodeJS.ReadWriteStream, options?: FinishedOptions): Promise<void>; } function pipeline<T extends NodeJS.WritableStream>(stream1: NodeJS.ReadableStream, stream2: T, callback?: (err: NodeJS.ErrnoException | null) => void): T; function pipeline<T extends NodeJS.WritableStream>(stream1: NodeJS.ReadableStream, stream2: NodeJS.ReadWriteStream, stream3: T, callback?: (err: NodeJS.ErrnoException | null) => void): T; function pipeline<T extends NodeJS.WritableStream>( stream1: NodeJS.ReadableStream, stream2: NodeJS.ReadWriteStream, stream3: NodeJS.ReadWriteStream, stream4: T, callback?: (err: NodeJS.ErrnoException | null) => void, ): T; function pipeline<T extends NodeJS.WritableStream>( stream1: NodeJS.ReadableStream, stream2: NodeJS.ReadWriteStream, stream3: NodeJS.ReadWriteStream, stream4: NodeJS.ReadWriteStream, stream5: T, callback?: (err: NodeJS.ErrnoException | null) => void, ): T; function pipeline( streams: ReadonlyArray<NodeJS.ReadableStream | NodeJS.WritableStream | NodeJS.ReadWriteStream>, callback?: (err: NodeJS.ErrnoException | null) => void, ): NodeJS.WritableStream; function pipeline( stream1: NodeJS.ReadableStream, stream2: NodeJS.ReadWriteStream | NodeJS.WritableStream, ...streams: Array<NodeJS.ReadWriteStream | NodeJS.WritableStream | ((err: NodeJS.ErrnoException | null) => void)>, ): NodeJS.WritableStream; namespace pipeline { function __promisify__(stream1: NodeJS.ReadableStream, stream2: NodeJS.WritableStream): Promise<void>; function __promisify__(stream1: NodeJS.ReadableStream, stream2: NodeJS.ReadWriteStream, stream3: NodeJS.WritableStream): Promise<void>; function __promisify__(stream1: NodeJS.ReadableStream, stream2: NodeJS.ReadWriteStream, stream3: NodeJS.ReadWriteStream, stream4: NodeJS.WritableStream): Promise<void>; function __promisify__( stream1: NodeJS.ReadableStream, stream2: NodeJS.ReadWriteStream, stream3: NodeJS.ReadWriteStream, stream4: NodeJS.ReadWriteStream, stream5: NodeJS.WritableStream, ): Promise<void>; function __promisify__(streams: ReadonlyArray<NodeJS.ReadableStream | NodeJS.WritableStream | NodeJS.ReadWriteStream>): Promise<void>; function __promisify__( stream1: NodeJS.ReadableStream, stream2: NodeJS.ReadWriteStream | NodeJS.WritableStream, ...streams: Array<NodeJS.ReadWriteStream | NodeJS.WritableStream>, ): Promise<void>; } interface Pipe { close(): void; hasRef(): boolean; ref(): void; unref(): void; } } export = internal; } apollo-server-demo/node_modules/@types/node/wasi.d.ts 0000644 0001750 0000144 00000006463 14000133701 022353 0 ustar andreh users declare module 'wasi' { interface WASIOptions { /** * An array of strings that the WebAssembly application will * see as command line arguments. The first argument is the virtual path to the * WASI command itself. */ args?: string[]; /** * An object similar to `process.env` that the WebAssembly * application will see as its environment. */ env?: object; /** * This object represents the WebAssembly application's * sandbox directory structure. The string keys of `preopens` are treated as * directories within the sandbox. The corresponding values in `preopens` are * the real paths to those directories on the host machine. */ preopens?: NodeJS.Dict<string>; /** * By default, WASI applications terminate the Node.js * process via the `__wasi_proc_exit()` function. Setting this option to `true` * causes `wasi.start()` to return the exit code rather than terminate the * process. * @default false */ returnOnExit?: boolean; /** * The file descriptor used as standard input in the WebAssembly application. * @default 0 */ stdin?: number; /** * The file descriptor used as standard output in the WebAssembly application. * @default 1 */ stdout?: number; /** * The file descriptor used as standard error in the WebAssembly application. * @default 2 */ stderr?: number; } class WASI { constructor(options?: WASIOptions); /** * * Attempt to begin execution of `instance` by invoking its `_start()` export. * If `instance` does not contain a `_start()` export, then `start()` attempts to * invoke the `__wasi_unstable_reactor_start()` export. If neither of those exports * is present on `instance`, then `start()` does nothing. * * `start()` requires that `instance` exports a [`WebAssembly.Memory`][] named * `memory`. If `instance` does not have a `memory` export an exception is thrown. * * If `start()` is called more than once, an exception is thrown. */ start(instance: object): void; // TODO: avoid DOM dependency until WASM moved to own lib. /** * Attempt to initialize `instance` as a WASI reactor by invoking its `_initialize()` export, if it is present. * If `instance` contains a `_start()` export, then an exception is thrown. * * `start()` requires that `instance` exports a [`WebAssembly.Memory`][] named * `memory`. If `instance` does not have a `memory` export an exception is thrown. * * If `initialize()` is called more than once, an exception is thrown. */ initialize(instance: object): void; // TODO: avoid DOM dependency until WASM moved to own lib. /** * Is an object that implements the WASI system call API. This object * should be passed as the `wasi_snapshot_preview1` import during the instantiation of a * [`WebAssembly.Instance`][]. */ readonly wasiImport: NodeJS.Dict<any>; // TODO: Narrow to DOM types } } apollo-server-demo/node_modules/@types/node/url.d.ts 0000644 0001750 0000144 00000010161 14000133701 022200 0 ustar andreh users declare module "url" { import { ParsedUrlQuery, ParsedUrlQueryInput } from 'querystring'; // Input to `url.format` interface UrlObject { auth?: string | null; hash?: string | null; host?: string | null; hostname?: string | null; href?: string | null; pathname?: string | null; protocol?: string | null; search?: string | null; slashes?: boolean | null; port?: string | number | null; query?: string | null | ParsedUrlQueryInput; } // Output of `url.parse` interface Url { auth: string | null; hash: string | null; host: string | null; hostname: string | null; href: string; path: string | null; pathname: string | null; protocol: string | null; search: string | null; slashes: boolean | null; port: string | null; query: string | null | ParsedUrlQuery; } interface UrlWithParsedQuery extends Url { query: ParsedUrlQuery; } interface UrlWithStringQuery extends Url { query: string | null; } /** @deprecated since v11.0.0 - Use the WHATWG URL API. */ function parse(urlStr: string): UrlWithStringQuery; /** @deprecated since v11.0.0 - Use the WHATWG URL API. */ function parse(urlStr: string, parseQueryString: false | undefined, slashesDenoteHost?: boolean): UrlWithStringQuery; /** @deprecated since v11.0.0 - Use the WHATWG URL API. */ function parse(urlStr: string, parseQueryString: true, slashesDenoteHost?: boolean): UrlWithParsedQuery; /** @deprecated since v11.0.0 - Use the WHATWG URL API. */ function parse(urlStr: string, parseQueryString: boolean, slashesDenoteHost?: boolean): Url; function format(URL: URL, options?: URLFormatOptions): string; /** @deprecated since v11.0.0 - Use the WHATWG URL API. */ function format(urlObject: UrlObject | string): string; /** @deprecated since v11.0.0 - Use the WHATWG URL API. */ function resolve(from: string, to: string): string; function domainToASCII(domain: string): string; function domainToUnicode(domain: string): string; /** * This function ensures the correct decodings of percent-encoded characters as * well as ensuring a cross-platform valid absolute path string. * @param url The file URL string or URL object to convert to a path. */ function fileURLToPath(url: string | URL): string; /** * This function ensures that path is resolved absolutely, and that the URL * control characters are correctly encoded when converting into a File URL. * @param url The path to convert to a File URL. */ function pathToFileURL(url: string): URL; interface URLFormatOptions { auth?: boolean; fragment?: boolean; search?: boolean; unicode?: boolean; } class URL { constructor(input: string, base?: string | URL); hash: string; host: string; hostname: string; href: string; readonly origin: string; password: string; pathname: string; port: string; protocol: string; search: string; readonly searchParams: URLSearchParams; username: string; toString(): string; toJSON(): string; } class URLSearchParams implements Iterable<[string, string]> { constructor(init?: URLSearchParams | string | NodeJS.Dict<string | ReadonlyArray<string>> | Iterable<[string, string]> | ReadonlyArray<[string, string]>); append(name: string, value: string): void; delete(name: string): void; entries(): IterableIterator<[string, string]>; forEach(callback: (value: string, name: string, searchParams: this) => void): void; get(name: string): string | null; getAll(name: string): string[]; has(name: string): boolean; keys(): IterableIterator<string>; set(name: string, value: string): void; sort(): void; toString(): string; values(): IterableIterator<string>; [Symbol.iterator](): IterableIterator<[string, string]>; } } apollo-server-demo/node_modules/@types/node/package.json 0000644 0001750 0000144 00000017114 14000133675 023111 0 ustar andreh users { "name": "@types/node", "version": "14.14.21", "description": "TypeScript definitions for Node.js", "license": "MIT", "contributors": [ { "name": "Microsoft TypeScript", "url": "https://github.com/Microsoft", "githubUsername": "Microsoft" }, { "name": "DefinitelyTyped", "url": "https://github.com/DefinitelyTyped", "githubUsername": "DefinitelyTyped" }, { "name": "Alberto Schiabel", "url": "https://github.com/jkomyno", "githubUsername": "jkomyno" }, { "name": "Alexander T.", "url": "https://github.com/a-tarasyuk", "githubUsername": "a-tarasyuk" }, { "name": "Alvis HT Tang", "url": "https://github.com/alvis", "githubUsername": "alvis" }, { "name": "Andrew Makarov", "url": "https://github.com/r3nya", "githubUsername": "r3nya" }, { "name": "Benjamin Toueg", "url": "https://github.com/btoueg", "githubUsername": "btoueg" }, { "name": "Bruno Scheufler", "url": "https://github.com/brunoscheufler", "githubUsername": "brunoscheufler" }, { "name": "Chigozirim C.", "url": "https://github.com/smac89", "githubUsername": "smac89" }, { "name": "David Junger", "url": "https://github.com/touffy", "githubUsername": "touffy" }, { "name": "Deividas Bakanas", "url": "https://github.com/DeividasBakanas", "githubUsername": "DeividasBakanas" }, { "name": "Eugene Y. Q. Shen", "url": "https://github.com/eyqs", "githubUsername": "eyqs" }, { "name": "Flarna", "url": "https://github.com/Flarna", "githubUsername": "Flarna" }, { "name": "Hannes Magnusson", "url": "https://github.com/Hannes-Magnusson-CK", "githubUsername": "Hannes-Magnusson-CK" }, { "name": "Hoà ng Văn Khải", "url": "https://github.com/KSXGitHub", "githubUsername": "KSXGitHub" }, { "name": "Huw", "url": "https://github.com/hoo29", "githubUsername": "hoo29" }, { "name": "Kelvin Jin", "url": "https://github.com/kjin", "githubUsername": "kjin" }, { "name": "Klaus Meinhardt", "url": "https://github.com/ajafff", "githubUsername": "ajafff" }, { "name": "Lishude", "url": "https://github.com/islishude", "githubUsername": "islishude" }, { "name": "Mariusz Wiktorczyk", "url": "https://github.com/mwiktorczyk", "githubUsername": "mwiktorczyk" }, { "name": "Mohsen Azimi", "url": "https://github.com/mohsen1", "githubUsername": "mohsen1" }, { "name": "Nicolas Even", "url": "https://github.com/n-e", "githubUsername": "n-e" }, { "name": "Nikita Galkin", "url": "https://github.com/galkin", "githubUsername": "galkin" }, { "name": "Parambir Singh", "url": "https://github.com/parambirs", "githubUsername": "parambirs" }, { "name": "Sebastian Silbermann", "url": "https://github.com/eps1lon", "githubUsername": "eps1lon" }, { "name": "Simon Schick", "url": "https://github.com/SimonSchick", "githubUsername": "SimonSchick" }, { "name": "Thomas den Hollander", "url": "https://github.com/ThomasdenH", "githubUsername": "ThomasdenH" }, { "name": "Wilco Bakker", "url": "https://github.com/WilcoBakker", "githubUsername": "WilcoBakker" }, { "name": "wwwy3y3", "url": "https://github.com/wwwy3y3", "githubUsername": "wwwy3y3" }, { "name": "Samuel Ainsworth", "url": "https://github.com/samuela", "githubUsername": "samuela" }, { "name": "Kyle Uehlein", "url": "https://github.com/kuehlein", "githubUsername": "kuehlein" }, { "name": "Jordi Oliveras Rovira", "url": "https://github.com/j-oliveras", "githubUsername": "j-oliveras" }, { "name": "Thanik Bhongbhibhat", "url": "https://github.com/bhongy", "githubUsername": "bhongy" }, { "name": "Marcin Kopacz", "url": "https://github.com/chyzwar", "githubUsername": "chyzwar" }, { "name": "Trivikram Kamat", "url": "https://github.com/trivikr", "githubUsername": "trivikr" }, { "name": "Minh Son Nguyen", "url": "https://github.com/nguymin4", "githubUsername": "nguymin4" }, { "name": "Junxiao Shi", "url": "https://github.com/yoursunny", "githubUsername": "yoursunny" }, { "name": "Ilia Baryshnikov", "url": "https://github.com/qwelias", "githubUsername": "qwelias" }, { "name": "ExE Boss", "url": "https://github.com/ExE-Boss", "githubUsername": "ExE-Boss" }, { "name": "Surasak Chaisurin", "url": "https://github.com/Ryan-Willpower", "githubUsername": "Ryan-Willpower" }, { "name": "Piotr Błażejewicz", "url": "https://github.com/peterblazejewicz", "githubUsername": "peterblazejewicz" }, { "name": "Anna Henningsen", "url": "https://github.com/addaleax", "githubUsername": "addaleax" }, { "name": "Jason Kwok", "url": "https://github.com/JasonHK", "githubUsername": "JasonHK" }, { "name": "Victor Perin", "url": "https://github.com/victorperin", "githubUsername": "victorperin" }, { "name": "Yongsheng Zhang", "url": "https://github.com/ZYSzys", "githubUsername": "ZYSzys" } ], "main": "", "types": "index.d.ts", "typesVersions": { "<=3.4": { "*": [ "ts3.4/*" ] }, "<=3.6": { "*": [ "ts3.6/*" ] } }, "repository": { "type": "git", "url": "https://github.com/DefinitelyTyped/DefinitelyTyped.git", "directory": "types/node" }, "scripts": {}, "dependencies": {}, "typesPublisherContentHash": "1a40e0df056d33fd040f811ee3fd362ffee55f73924d333e10c98bbfd01438ed", "typeScriptVersion": "3.4" ,"_resolved": "https://registry.npmjs.org/@types/node/-/node-14.14.21.tgz" ,"_integrity": "sha512-cHYfKsnwllYhjOzuC5q1VpguABBeecUp24yFluHpn/BQaVxB1CuQ1FSRZCzrPxrkIfWISXV2LbeoBthLWg0+0A==" ,"_from": "@types/node@14.14.21" } apollo-server-demo/node_modules/@types/node/http2.d.ts 0000644 0001750 0000144 00000156423 14000133700 022452 0 ustar andreh users declare module "http2" { import * as events from "events"; import * as fs from "fs"; import * as net from "net"; import * as stream from "stream"; import * as tls from "tls"; import * as url from "url"; import { IncomingHttpHeaders as Http1IncomingHttpHeaders, OutgoingHttpHeaders, IncomingMessage, ServerResponse } from "http"; export { OutgoingHttpHeaders } from "http"; export interface IncomingHttpStatusHeader { ":status"?: number; } export interface IncomingHttpHeaders extends Http1IncomingHttpHeaders { ":path"?: string; ":method"?: string; ":authority"?: string; ":scheme"?: string; } // Http2Stream export interface StreamPriorityOptions { exclusive?: boolean; parent?: number; weight?: number; silent?: boolean; } export interface StreamState { localWindowSize?: number; state?: number; localClose?: number; remoteClose?: number; sumDependencyWeight?: number; weight?: number; } export interface ServerStreamResponseOptions { endStream?: boolean; waitForTrailers?: boolean; } export interface StatOptions { offset: number; length: number; } export interface ServerStreamFileResponseOptions { statCheck?(stats: fs.Stats, headers: OutgoingHttpHeaders, statOptions: StatOptions): void | boolean; waitForTrailers?: boolean; offset?: number; length?: number; } export interface ServerStreamFileResponseOptionsWithError extends ServerStreamFileResponseOptions { onError?(err: NodeJS.ErrnoException): void; } export interface Http2Stream extends stream.Duplex { readonly aborted: boolean; readonly bufferSize: number; readonly closed: boolean; readonly destroyed: boolean; /** * Set the true if the END_STREAM flag was set in the request or response HEADERS frame received, * indicating that no additional data should be received and the readable side of the Http2Stream will be closed. */ readonly endAfterHeaders: boolean; readonly id?: number; readonly pending: boolean; readonly rstCode: number; readonly sentHeaders: OutgoingHttpHeaders; readonly sentInfoHeaders?: OutgoingHttpHeaders[]; readonly sentTrailers?: OutgoingHttpHeaders; readonly session: Http2Session; readonly state: StreamState; close(code?: number, callback?: () => void): void; priority(options: StreamPriorityOptions): void; setTimeout(msecs: number, callback?: () => void): void; sendTrailers(headers: OutgoingHttpHeaders): void; addListener(event: "aborted", listener: () => void): this; addListener(event: "close", listener: () => void): this; addListener(event: "data", listener: (chunk: Buffer | string) => void): this; addListener(event: "drain", listener: () => void): this; addListener(event: "end", listener: () => void): this; addListener(event: "error", listener: (err: Error) => void): this; addListener(event: "finish", listener: () => void): this; addListener(event: "frameError", listener: (frameType: number, errorCode: number) => void): this; addListener(event: "pipe", listener: (src: stream.Readable) => void): this; addListener(event: "unpipe", listener: (src: stream.Readable) => void): this; addListener(event: "streamClosed", listener: (code: number) => void): this; addListener(event: "timeout", listener: () => void): this; addListener(event: "trailers", listener: (trailers: IncomingHttpHeaders, flags: number) => void): this; addListener(event: "wantTrailers", listener: () => void): this; addListener(event: string | symbol, listener: (...args: any[]) => void): this; emit(event: "aborted"): boolean; emit(event: "close"): boolean; emit(event: "data", chunk: Buffer | string): boolean; emit(event: "drain"): boolean; emit(event: "end"): boolean; emit(event: "error", err: Error): boolean; emit(event: "finish"): boolean; emit(event: "frameError", frameType: number, errorCode: number): boolean; emit(event: "pipe", src: stream.Readable): boolean; emit(event: "unpipe", src: stream.Readable): boolean; emit(event: "streamClosed", code: number): boolean; emit(event: "timeout"): boolean; emit(event: "trailers", trailers: IncomingHttpHeaders, flags: number): boolean; emit(event: "wantTrailers"): boolean; emit(event: string | symbol, ...args: any[]): boolean; on(event: "aborted", listener: () => void): this; on(event: "close", listener: () => void): this; on(event: "data", listener: (chunk: Buffer | string) => void): this; on(event: "drain", listener: () => void): this; on(event: "end", listener: () => void): this; on(event: "error", listener: (err: Error) => void): this; on(event: "finish", listener: () => void): this; on(event: "frameError", listener: (frameType: number, errorCode: number) => void): this; on(event: "pipe", listener: (src: stream.Readable) => void): this; on(event: "unpipe", listener: (src: stream.Readable) => void): this; on(event: "streamClosed", listener: (code: number) => void): this; on(event: "timeout", listener: () => void): this; on(event: "trailers", listener: (trailers: IncomingHttpHeaders, flags: number) => void): this; on(event: "wantTrailers", listener: () => void): this; on(event: string | symbol, listener: (...args: any[]) => void): this; once(event: "aborted", listener: () => void): this; once(event: "close", listener: () => void): this; once(event: "data", listener: (chunk: Buffer | string) => void): this; once(event: "drain", listener: () => void): this; once(event: "end", listener: () => void): this; once(event: "error", listener: (err: Error) => void): this; once(event: "finish", listener: () => void): this; once(event: "frameError", listener: (frameType: number, errorCode: number) => void): this; once(event: "pipe", listener: (src: stream.Readable) => void): this; once(event: "unpipe", listener: (src: stream.Readable) => void): this; once(event: "streamClosed", listener: (code: number) => void): this; once(event: "timeout", listener: () => void): this; once(event: "trailers", listener: (trailers: IncomingHttpHeaders, flags: number) => void): this; once(event: "wantTrailers", listener: () => void): this; once(event: string | symbol, listener: (...args: any[]) => void): this; prependListener(event: "aborted", listener: () => void): this; prependListener(event: "close", listener: () => void): this; prependListener(event: "data", listener: (chunk: Buffer | string) => void): this; prependListener(event: "drain", listener: () => void): this; prependListener(event: "end", listener: () => void): this; prependListener(event: "error", listener: (err: Error) => void): this; prependListener(event: "finish", listener: () => void): this; prependListener(event: "frameError", listener: (frameType: number, errorCode: number) => void): this; prependListener(event: "pipe", listener: (src: stream.Readable) => void): this; prependListener(event: "unpipe", listener: (src: stream.Readable) => void): this; prependListener(event: "streamClosed", listener: (code: number) => void): this; prependListener(event: "timeout", listener: () => void): this; prependListener(event: "trailers", listener: (trailers: IncomingHttpHeaders, flags: number) => void): this; prependListener(event: "wantTrailers", listener: () => void): this; prependListener(event: string | symbol, listener: (...args: any[]) => void): this; prependOnceListener(event: "aborted", listener: () => void): this; prependOnceListener(event: "close", listener: () => void): this; prependOnceListener(event: "data", listener: (chunk: Buffer | string) => void): this; prependOnceListener(event: "drain", listener: () => void): this; prependOnceListener(event: "end", listener: () => void): this; prependOnceListener(event: "error", listener: (err: Error) => void): this; prependOnceListener(event: "finish", listener: () => void): this; prependOnceListener(event: "frameError", listener: (frameType: number, errorCode: number) => void): this; prependOnceListener(event: "pipe", listener: (src: stream.Readable) => void): this; prependOnceListener(event: "unpipe", listener: (src: stream.Readable) => void): this; prependOnceListener(event: "streamClosed", listener: (code: number) => void): this; prependOnceListener(event: "timeout", listener: () => void): this; prependOnceListener(event: "trailers", listener: (trailers: IncomingHttpHeaders, flags: number) => void): this; prependOnceListener(event: "wantTrailers", listener: () => void): this; prependOnceListener(event: string | symbol, listener: (...args: any[]) => void): this; } export interface ClientHttp2Stream extends Http2Stream { addListener(event: "continue", listener: () => {}): this; addListener(event: "headers", listener: (headers: IncomingHttpHeaders & IncomingHttpStatusHeader, flags: number) => void): this; addListener(event: "push", listener: (headers: IncomingHttpHeaders, flags: number) => void): this; addListener(event: "response", listener: (headers: IncomingHttpHeaders & IncomingHttpStatusHeader, flags: number) => void): this; addListener(event: string | symbol, listener: (...args: any[]) => void): this; emit(event: "continue"): boolean; emit(event: "headers", headers: IncomingHttpHeaders & IncomingHttpStatusHeader, flags: number): boolean; emit(event: "push", headers: IncomingHttpHeaders, flags: number): boolean; emit(event: "response", headers: IncomingHttpHeaders & IncomingHttpStatusHeader, flags: number): boolean; emit(event: string | symbol, ...args: any[]): boolean; on(event: "continue", listener: () => {}): this; on(event: "headers", listener: (headers: IncomingHttpHeaders & IncomingHttpStatusHeader, flags: number) => void): this; on(event: "push", listener: (headers: IncomingHttpHeaders, flags: number) => void): this; on(event: "response", listener: (headers: IncomingHttpHeaders & IncomingHttpStatusHeader, flags: number) => void): this; on(event: string | symbol, listener: (...args: any[]) => void): this; once(event: "continue", listener: () => {}): this; once(event: "headers", listener: (headers: IncomingHttpHeaders & IncomingHttpStatusHeader, flags: number) => void): this; once(event: "push", listener: (headers: IncomingHttpHeaders, flags: number) => void): this; once(event: "response", listener: (headers: IncomingHttpHeaders & IncomingHttpStatusHeader, flags: number) => void): this; once(event: string | symbol, listener: (...args: any[]) => void): this; prependListener(event: "continue", listener: () => {}): this; prependListener(event: "headers", listener: (headers: IncomingHttpHeaders & IncomingHttpStatusHeader, flags: number) => void): this; prependListener(event: "push", listener: (headers: IncomingHttpHeaders, flags: number) => void): this; prependListener(event: "response", listener: (headers: IncomingHttpHeaders & IncomingHttpStatusHeader, flags: number) => void): this; prependListener(event: string | symbol, listener: (...args: any[]) => void): this; prependOnceListener(event: "continue", listener: () => {}): this; prependOnceListener(event: "headers", listener: (headers: IncomingHttpHeaders & IncomingHttpStatusHeader, flags: number) => void): this; prependOnceListener(event: "push", listener: (headers: IncomingHttpHeaders, flags: number) => void): this; prependOnceListener(event: "response", listener: (headers: IncomingHttpHeaders & IncomingHttpStatusHeader, flags: number) => void): this; prependOnceListener(event: string | symbol, listener: (...args: any[]) => void): this; } export interface ServerHttp2Stream extends Http2Stream { readonly headersSent: boolean; readonly pushAllowed: boolean; additionalHeaders(headers: OutgoingHttpHeaders): void; pushStream(headers: OutgoingHttpHeaders, callback?: (err: Error | null, pushStream: ServerHttp2Stream, headers: OutgoingHttpHeaders) => void): void; pushStream(headers: OutgoingHttpHeaders, options?: StreamPriorityOptions, callback?: (err: Error | null, pushStream: ServerHttp2Stream, headers: OutgoingHttpHeaders) => void): void; respond(headers?: OutgoingHttpHeaders, options?: ServerStreamResponseOptions): void; respondWithFD(fd: number | fs.promises.FileHandle, headers?: OutgoingHttpHeaders, options?: ServerStreamFileResponseOptions): void; respondWithFile(path: string, headers?: OutgoingHttpHeaders, options?: ServerStreamFileResponseOptionsWithError): void; } // Http2Session export interface Settings { headerTableSize?: number; enablePush?: boolean; initialWindowSize?: number; maxFrameSize?: number; maxConcurrentStreams?: number; maxHeaderListSize?: number; enableConnectProtocol?: boolean; } export interface ClientSessionRequestOptions { endStream?: boolean; exclusive?: boolean; parent?: number; weight?: number; waitForTrailers?: boolean; } export interface SessionState { effectiveLocalWindowSize?: number; effectiveRecvDataLength?: number; nextStreamID?: number; localWindowSize?: number; lastProcStreamID?: number; remoteWindowSize?: number; outboundQueueSize?: number; deflateDynamicTableSize?: number; inflateDynamicTableSize?: number; } export interface Http2Session extends events.EventEmitter { readonly alpnProtocol?: string; readonly closed: boolean; readonly connecting: boolean; readonly destroyed: boolean; readonly encrypted?: boolean; readonly localSettings: Settings; readonly originSet?: string[]; readonly pendingSettingsAck: boolean; readonly remoteSettings: Settings; readonly socket: net.Socket | tls.TLSSocket; readonly state: SessionState; readonly type: number; close(callback?: () => void): void; destroy(error?: Error, code?: number): void; goaway(code?: number, lastStreamID?: number, opaqueData?: NodeJS.ArrayBufferView): void; ping(callback: (err: Error | null, duration: number, payload: Buffer) => void): boolean; ping(payload: NodeJS.ArrayBufferView, callback: (err: Error | null, duration: number, payload: Buffer) => void): boolean; ref(): void; setLocalWindowSize(windowSize: number): void; setTimeout(msecs: number, callback?: () => void): void; settings(settings: Settings): void; unref(): void; addListener(event: "close", listener: () => void): this; addListener(event: "error", listener: (err: Error) => void): this; addListener(event: "frameError", listener: (frameType: number, errorCode: number, streamID: number) => void): this; addListener(event: "goaway", listener: (errorCode: number, lastStreamID: number, opaqueData: Buffer) => void): this; addListener(event: "localSettings", listener: (settings: Settings) => void): this; addListener(event: "ping", listener: () => void): this; addListener(event: "remoteSettings", listener: (settings: Settings) => void): this; addListener(event: "timeout", listener: () => void): this; addListener(event: string | symbol, listener: (...args: any[]) => void): this; emit(event: "close"): boolean; emit(event: "error", err: Error): boolean; emit(event: "frameError", frameType: number, errorCode: number, streamID: number): boolean; emit(event: "goaway", errorCode: number, lastStreamID: number, opaqueData: Buffer): boolean; emit(event: "localSettings", settings: Settings): boolean; emit(event: "ping"): boolean; emit(event: "remoteSettings", settings: Settings): boolean; emit(event: "timeout"): boolean; emit(event: string | symbol, ...args: any[]): boolean; on(event: "close", listener: () => void): this; on(event: "error", listener: (err: Error) => void): this; on(event: "frameError", listener: (frameType: number, errorCode: number, streamID: number) => void): this; on(event: "goaway", listener: (errorCode: number, lastStreamID: number, opaqueData: Buffer) => void): this; on(event: "localSettings", listener: (settings: Settings) => void): this; on(event: "ping", listener: () => void): this; on(event: "remoteSettings", listener: (settings: Settings) => void): this; on(event: "timeout", listener: () => void): this; on(event: string | symbol, listener: (...args: any[]) => void): this; once(event: "close", listener: () => void): this; once(event: "error", listener: (err: Error) => void): this; once(event: "frameError", listener: (frameType: number, errorCode: number, streamID: number) => void): this; once(event: "goaway", listener: (errorCode: number, lastStreamID: number, opaqueData: Buffer) => void): this; once(event: "localSettings", listener: (settings: Settings) => void): this; once(event: "ping", listener: () => void): this; once(event: "remoteSettings", listener: (settings: Settings) => void): this; once(event: "timeout", listener: () => void): this; once(event: string | symbol, listener: (...args: any[]) => void): this; prependListener(event: "close", listener: () => void): this; prependListener(event: "error", listener: (err: Error) => void): this; prependListener(event: "frameError", listener: (frameType: number, errorCode: number, streamID: number) => void): this; prependListener(event: "goaway", listener: (errorCode: number, lastStreamID: number, opaqueData: Buffer) => void): this; prependListener(event: "localSettings", listener: (settings: Settings) => void): this; prependListener(event: "ping", listener: () => void): this; prependListener(event: "remoteSettings", listener: (settings: Settings) => void): this; prependListener(event: "timeout", listener: () => void): this; prependListener(event: string | symbol, listener: (...args: any[]) => void): this; prependOnceListener(event: "close", listener: () => void): this; prependOnceListener(event: "error", listener: (err: Error) => void): this; prependOnceListener(event: "frameError", listener: (frameType: number, errorCode: number, streamID: number) => void): this; prependOnceListener(event: "goaway", listener: (errorCode: number, lastStreamID: number, opaqueData: Buffer) => void): this; prependOnceListener(event: "localSettings", listener: (settings: Settings) => void): this; prependOnceListener(event: "ping", listener: () => void): this; prependOnceListener(event: "remoteSettings", listener: (settings: Settings) => void): this; prependOnceListener(event: "timeout", listener: () => void): this; prependOnceListener(event: string | symbol, listener: (...args: any[]) => void): this; } export interface ClientHttp2Session extends Http2Session { request(headers?: OutgoingHttpHeaders, options?: ClientSessionRequestOptions): ClientHttp2Stream; addListener(event: "altsvc", listener: (alt: string, origin: string, stream: number) => void): this; addListener(event: "origin", listener: (origins: string[]) => void): this; addListener(event: "connect", listener: (session: ClientHttp2Session, socket: net.Socket | tls.TLSSocket) => void): this; addListener(event: "stream", listener: (stream: ClientHttp2Stream, headers: IncomingHttpHeaders & IncomingHttpStatusHeader, flags: number) => void): this; addListener(event: string | symbol, listener: (...args: any[]) => void): this; emit(event: "altsvc", alt: string, origin: string, stream: number): boolean; emit(event: "origin", origins: ReadonlyArray<string>): boolean; emit(event: "connect", session: ClientHttp2Session, socket: net.Socket | tls.TLSSocket): boolean; emit(event: "stream", stream: ClientHttp2Stream, headers: IncomingHttpHeaders & IncomingHttpStatusHeader, flags: number): boolean; emit(event: string | symbol, ...args: any[]): boolean; on(event: "altsvc", listener: (alt: string, origin: string, stream: number) => void): this; on(event: "origin", listener: (origins: string[]) => void): this; on(event: "connect", listener: (session: ClientHttp2Session, socket: net.Socket | tls.TLSSocket) => void): this; on(event: "stream", listener: (stream: ClientHttp2Stream, headers: IncomingHttpHeaders & IncomingHttpStatusHeader, flags: number) => void): this; on(event: string | symbol, listener: (...args: any[]) => void): this; once(event: "altsvc", listener: (alt: string, origin: string, stream: number) => void): this; once(event: "origin", listener: (origins: string[]) => void): this; once(event: "connect", listener: (session: ClientHttp2Session, socket: net.Socket | tls.TLSSocket) => void): this; once(event: "stream", listener: (stream: ClientHttp2Stream, headers: IncomingHttpHeaders & IncomingHttpStatusHeader, flags: number) => void): this; once(event: string | symbol, listener: (...args: any[]) => void): this; prependListener(event: "altsvc", listener: (alt: string, origin: string, stream: number) => void): this; prependListener(event: "origin", listener: (origins: string[]) => void): this; prependListener(event: "connect", listener: (session: ClientHttp2Session, socket: net.Socket | tls.TLSSocket) => void): this; prependListener(event: "stream", listener: (stream: ClientHttp2Stream, headers: IncomingHttpHeaders & IncomingHttpStatusHeader, flags: number) => void): this; prependListener(event: string | symbol, listener: (...args: any[]) => void): this; prependOnceListener(event: "altsvc", listener: (alt: string, origin: string, stream: number) => void): this; prependOnceListener(event: "origin", listener: (origins: string[]) => void): this; prependOnceListener(event: "connect", listener: (session: ClientHttp2Session, socket: net.Socket | tls.TLSSocket) => void): this; prependOnceListener(event: "stream", listener: (stream: ClientHttp2Stream, headers: IncomingHttpHeaders & IncomingHttpStatusHeader, flags: number) => void): this; prependOnceListener(event: string | symbol, listener: (...args: any[]) => void): this; } export interface AlternativeServiceOptions { origin: number | string | url.URL; } export interface ServerHttp2Session extends Http2Session { readonly server: Http2Server | Http2SecureServer; altsvc(alt: string, originOrStream: number | string | url.URL | AlternativeServiceOptions): void; origin(...args: Array<string | url.URL | { origin: string }>): void; addListener(event: "connect", listener: (session: ServerHttp2Session, socket: net.Socket | tls.TLSSocket) => void): this; addListener(event: "stream", listener: (stream: ServerHttp2Stream, headers: IncomingHttpHeaders, flags: number) => void): this; addListener(event: string | symbol, listener: (...args: any[]) => void): this; emit(event: "connect", session: ServerHttp2Session, socket: net.Socket | tls.TLSSocket): boolean; emit(event: "stream", stream: ServerHttp2Stream, headers: IncomingHttpHeaders, flags: number): boolean; emit(event: string | symbol, ...args: any[]): boolean; on(event: "connect", listener: (session: ServerHttp2Session, socket: net.Socket | tls.TLSSocket) => void): this; on(event: "stream", listener: (stream: ServerHttp2Stream, headers: IncomingHttpHeaders, flags: number) => void): this; on(event: string | symbol, listener: (...args: any[]) => void): this; once(event: "connect", listener: (session: ServerHttp2Session, socket: net.Socket | tls.TLSSocket) => void): this; once(event: "stream", listener: (stream: ServerHttp2Stream, headers: IncomingHttpHeaders, flags: number) => void): this; once(event: string | symbol, listener: (...args: any[]) => void): this; prependListener(event: "connect", listener: (session: ServerHttp2Session, socket: net.Socket | tls.TLSSocket) => void): this; prependListener(event: "stream", listener: (stream: ServerHttp2Stream, headers: IncomingHttpHeaders, flags: number) => void): this; prependListener(event: string | symbol, listener: (...args: any[]) => void): this; prependOnceListener(event: "connect", listener: (session: ServerHttp2Session, socket: net.Socket | tls.TLSSocket) => void): this; prependOnceListener(event: "stream", listener: (stream: ServerHttp2Stream, headers: IncomingHttpHeaders, flags: number) => void): this; prependOnceListener(event: string | symbol, listener: (...args: any[]) => void): this; } // Http2Server export interface SessionOptions { maxDeflateDynamicTableSize?: number; maxSessionMemory?: number; maxHeaderListPairs?: number; maxOutstandingPings?: number; maxSendHeaderBlockLength?: number; paddingStrategy?: number; peerMaxConcurrentStreams?: number; settings?: Settings; selectPadding?(frameLen: number, maxFrameLen: number): number; createConnection?(authority: url.URL, option: SessionOptions): stream.Duplex; } export interface ClientSessionOptions extends SessionOptions { maxReservedRemoteStreams?: number; createConnection?: (authority: url.URL, option: SessionOptions) => stream.Duplex; protocol?: 'http:' | 'https:'; } export interface ServerSessionOptions extends SessionOptions { Http1IncomingMessage?: typeof IncomingMessage; Http1ServerResponse?: typeof ServerResponse; Http2ServerRequest?: typeof Http2ServerRequest; Http2ServerResponse?: typeof Http2ServerResponse; } export interface SecureClientSessionOptions extends ClientSessionOptions, tls.ConnectionOptions { } export interface SecureServerSessionOptions extends ServerSessionOptions, tls.TlsOptions { } export interface ServerOptions extends ServerSessionOptions { } export interface SecureServerOptions extends SecureServerSessionOptions { allowHTTP1?: boolean; origins?: string[]; } export interface Http2Server extends net.Server { addListener(event: "checkContinue", listener: (request: Http2ServerRequest, response: Http2ServerResponse) => void): this; addListener(event: "request", listener: (request: Http2ServerRequest, response: Http2ServerResponse) => void): this; addListener(event: "session", listener: (session: ServerHttp2Session) => void): this; addListener(event: "sessionError", listener: (err: Error) => void): this; addListener(event: "stream", listener: (stream: ServerHttp2Stream, headers: IncomingHttpHeaders, flags: number) => void): this; addListener(event: "timeout", listener: () => void): this; addListener(event: string | symbol, listener: (...args: any[]) => void): this; emit(event: "checkContinue", request: Http2ServerRequest, response: Http2ServerResponse): boolean; emit(event: "request", request: Http2ServerRequest, response: Http2ServerResponse): boolean; emit(event: "session", session: ServerHttp2Session): boolean; emit(event: "sessionError", err: Error): boolean; emit(event: "stream", stream: ServerHttp2Stream, headers: IncomingHttpHeaders, flags: number): boolean; emit(event: "timeout"): boolean; emit(event: string | symbol, ...args: any[]): boolean; on(event: "checkContinue", listener: (request: Http2ServerRequest, response: Http2ServerResponse) => void): this; on(event: "request", listener: (request: Http2ServerRequest, response: Http2ServerResponse) => void): this; on(event: "session", listener: (session: ServerHttp2Session) => void): this; on(event: "sessionError", listener: (err: Error) => void): this; on(event: "stream", listener: (stream: ServerHttp2Stream, headers: IncomingHttpHeaders, flags: number) => void): this; on(event: "timeout", listener: () => void): this; on(event: string | symbol, listener: (...args: any[]) => void): this; once(event: "checkContinue", listener: (request: Http2ServerRequest, response: Http2ServerResponse) => void): this; once(event: "request", listener: (request: Http2ServerRequest, response: Http2ServerResponse) => void): this; once(event: "session", listener: (session: ServerHttp2Session) => void): this; once(event: "sessionError", listener: (err: Error) => void): this; once(event: "stream", listener: (stream: ServerHttp2Stream, headers: IncomingHttpHeaders, flags: number) => void): this; once(event: "timeout", listener: () => void): this; once(event: string | symbol, listener: (...args: any[]) => void): this; prependListener(event: "checkContinue", listener: (request: Http2ServerRequest, response: Http2ServerResponse) => void): this; prependListener(event: "request", listener: (request: Http2ServerRequest, response: Http2ServerResponse) => void): this; prependListener(event: "session", listener: (session: ServerHttp2Session) => void): this; prependListener(event: "sessionError", listener: (err: Error) => void): this; prependListener(event: "stream", listener: (stream: ServerHttp2Stream, headers: IncomingHttpHeaders, flags: number) => void): this; prependListener(event: "timeout", listener: () => void): this; prependListener(event: string | symbol, listener: (...args: any[]) => void): this; prependOnceListener(event: "checkContinue", listener: (request: Http2ServerRequest, response: Http2ServerResponse) => void): this; prependOnceListener(event: "request", listener: (request: Http2ServerRequest, response: Http2ServerResponse) => void): this; prependOnceListener(event: "session", listener: (session: ServerHttp2Session) => void): this; prependOnceListener(event: "sessionError", listener: (err: Error) => void): this; prependOnceListener(event: "stream", listener: (stream: ServerHttp2Stream, headers: IncomingHttpHeaders, flags: number) => void): this; prependOnceListener(event: "timeout", listener: () => void): this; prependOnceListener(event: string | symbol, listener: (...args: any[]) => void): this; setTimeout(msec?: number, callback?: () => void): this; } export interface Http2SecureServer extends tls.Server { addListener(event: "checkContinue", listener: (request: Http2ServerRequest, response: Http2ServerResponse) => void): this; addListener(event: "request", listener: (request: Http2ServerRequest, response: Http2ServerResponse) => void): this; addListener(event: "session", listener: (session: ServerHttp2Session) => void): this; addListener(event: "sessionError", listener: (err: Error) => void): this; addListener(event: "stream", listener: (stream: ServerHttp2Stream, headers: IncomingHttpHeaders, flags: number) => void): this; addListener(event: "timeout", listener: () => void): this; addListener(event: "unknownProtocol", listener: (socket: tls.TLSSocket) => void): this; addListener(event: string | symbol, listener: (...args: any[]) => void): this; emit(event: "checkContinue", request: Http2ServerRequest, response: Http2ServerResponse): boolean; emit(event: "request", request: Http2ServerRequest, response: Http2ServerResponse): boolean; emit(event: "session", session: ServerHttp2Session): boolean; emit(event: "sessionError", err: Error): boolean; emit(event: "stream", stream: ServerHttp2Stream, headers: IncomingHttpHeaders, flags: number): boolean; emit(event: "timeout"): boolean; emit(event: "unknownProtocol", socket: tls.TLSSocket): boolean; emit(event: string | symbol, ...args: any[]): boolean; on(event: "checkContinue", listener: (request: Http2ServerRequest, response: Http2ServerResponse) => void): this; on(event: "request", listener: (request: Http2ServerRequest, response: Http2ServerResponse) => void): this; on(event: "session", listener: (session: ServerHttp2Session) => void): this; on(event: "sessionError", listener: (err: Error) => void): this; on(event: "stream", listener: (stream: ServerHttp2Stream, headers: IncomingHttpHeaders, flags: number) => void): this; on(event: "timeout", listener: () => void): this; on(event: "unknownProtocol", listener: (socket: tls.TLSSocket) => void): this; on(event: string | symbol, listener: (...args: any[]) => void): this; once(event: "checkContinue", listener: (request: Http2ServerRequest, response: Http2ServerResponse) => void): this; once(event: "request", listener: (request: Http2ServerRequest, response: Http2ServerResponse) => void): this; once(event: "session", listener: (session: ServerHttp2Session) => void): this; once(event: "sessionError", listener: (err: Error) => void): this; once(event: "stream", listener: (stream: ServerHttp2Stream, headers: IncomingHttpHeaders, flags: number) => void): this; once(event: "timeout", listener: () => void): this; once(event: "unknownProtocol", listener: (socket: tls.TLSSocket) => void): this; once(event: string | symbol, listener: (...args: any[]) => void): this; prependListener(event: "checkContinue", listener: (request: Http2ServerRequest, response: Http2ServerResponse) => void): this; prependListener(event: "request", listener: (request: Http2ServerRequest, response: Http2ServerResponse) => void): this; prependListener(event: "session", listener: (session: ServerHttp2Session) => void): this; prependListener(event: "sessionError", listener: (err: Error) => void): this; prependListener(event: "stream", listener: (stream: ServerHttp2Stream, headers: IncomingHttpHeaders, flags: number) => void): this; prependListener(event: "timeout", listener: () => void): this; prependListener(event: "unknownProtocol", listener: (socket: tls.TLSSocket) => void): this; prependListener(event: string | symbol, listener: (...args: any[]) => void): this; prependOnceListener(event: "checkContinue", listener: (request: Http2ServerRequest, response: Http2ServerResponse) => void): this; prependOnceListener(event: "request", listener: (request: Http2ServerRequest, response: Http2ServerResponse) => void): this; prependOnceListener(event: "session", listener: (session: ServerHttp2Session) => void): this; prependOnceListener(event: "sessionError", listener: (err: Error) => void): this; prependOnceListener(event: "stream", listener: (stream: ServerHttp2Stream, headers: IncomingHttpHeaders, flags: number) => void): this; prependOnceListener(event: "timeout", listener: () => void): this; prependOnceListener(event: "unknownProtocol", listener: (socket: tls.TLSSocket) => void): this; prependOnceListener(event: string | symbol, listener: (...args: any[]) => void): this; setTimeout(msec?: number, callback?: () => void): this; } export class Http2ServerRequest extends stream.Readable { constructor(stream: ServerHttp2Stream, headers: IncomingHttpHeaders, options: stream.ReadableOptions, rawHeaders: ReadonlyArray<string>); readonly aborted: boolean; readonly authority: string; readonly connection: net.Socket | tls.TLSSocket; readonly complete: boolean; readonly headers: IncomingHttpHeaders; readonly httpVersion: string; readonly httpVersionMinor: number; readonly httpVersionMajor: number; readonly method: string; readonly rawHeaders: string[]; readonly rawTrailers: string[]; readonly scheme: string; readonly socket: net.Socket | tls.TLSSocket; readonly stream: ServerHttp2Stream; readonly trailers: IncomingHttpHeaders; readonly url: string; setTimeout(msecs: number, callback?: () => void): void; read(size?: number): Buffer | string | null; addListener(event: "aborted", listener: (hadError: boolean, code: number) => void): this; addListener(event: "close", listener: () => void): this; addListener(event: "data", listener: (chunk: Buffer | string) => void): this; addListener(event: "end", listener: () => void): this; addListener(event: "readable", listener: () => void): this; addListener(event: "error", listener: (err: Error) => void): this; addListener(event: string | symbol, listener: (...args: any[]) => void): this; emit(event: "aborted", hadError: boolean, code: number): boolean; emit(event: "close"): boolean; emit(event: "data", chunk: Buffer | string): boolean; emit(event: "end"): boolean; emit(event: "readable"): boolean; emit(event: "error", err: Error): boolean; emit(event: string | symbol, ...args: any[]): boolean; on(event: "aborted", listener: (hadError: boolean, code: number) => void): this; on(event: "close", listener: () => void): this; on(event: "data", listener: (chunk: Buffer | string) => void): this; on(event: "end", listener: () => void): this; on(event: "readable", listener: () => void): this; on(event: "error", listener: (err: Error) => void): this; on(event: string | symbol, listener: (...args: any[]) => void): this; once(event: "aborted", listener: (hadError: boolean, code: number) => void): this; once(event: "close", listener: () => void): this; once(event: "data", listener: (chunk: Buffer | string) => void): this; once(event: "end", listener: () => void): this; once(event: "readable", listener: () => void): this; once(event: "error", listener: (err: Error) => void): this; once(event: string | symbol, listener: (...args: any[]) => void): this; prependListener(event: "aborted", listener: (hadError: boolean, code: number) => void): this; prependListener(event: "close", listener: () => void): this; prependListener(event: "data", listener: (chunk: Buffer | string) => void): this; prependListener(event: "end", listener: () => void): this; prependListener(event: "readable", listener: () => void): this; prependListener(event: "error", listener: (err: Error) => void): this; prependListener(event: string | symbol, listener: (...args: any[]) => void): this; prependOnceListener(event: "aborted", listener: (hadError: boolean, code: number) => void): this; prependOnceListener(event: "close", listener: () => void): this; prependOnceListener(event: "data", listener: (chunk: Buffer | string) => void): this; prependOnceListener(event: "end", listener: () => void): this; prependOnceListener(event: "readable", listener: () => void): this; prependOnceListener(event: "error", listener: (err: Error) => void): this; prependOnceListener(event: string | symbol, listener: (...args: any[]) => void): this; } export class Http2ServerResponse extends stream.Stream { constructor(stream: ServerHttp2Stream); readonly connection: net.Socket | tls.TLSSocket; readonly finished: boolean; readonly headersSent: boolean; readonly socket: net.Socket | tls.TLSSocket; readonly stream: ServerHttp2Stream; sendDate: boolean; statusCode: number; statusMessage: ''; addTrailers(trailers: OutgoingHttpHeaders): void; end(callback?: () => void): void; end(data: string | Uint8Array, callback?: () => void): void; end(data: string | Uint8Array, encoding: BufferEncoding, callback?: () => void): void; getHeader(name: string): string; getHeaderNames(): string[]; getHeaders(): OutgoingHttpHeaders; hasHeader(name: string): boolean; removeHeader(name: string): void; setHeader(name: string, value: number | string | ReadonlyArray<string>): void; setTimeout(msecs: number, callback?: () => void): void; write(chunk: string | Uint8Array, callback?: (err: Error) => void): boolean; write(chunk: string | Uint8Array, encoding: BufferEncoding, callback?: (err: Error) => void): boolean; writeContinue(): void; writeHead(statusCode: number, headers?: OutgoingHttpHeaders): this; writeHead(statusCode: number, statusMessage: string, headers?: OutgoingHttpHeaders): this; createPushResponse(headers: OutgoingHttpHeaders, callback: (err: Error | null, res: Http2ServerResponse) => void): void; addListener(event: "close", listener: () => void): this; addListener(event: "drain", listener: () => void): this; addListener(event: "error", listener: (error: Error) => void): this; addListener(event: "finish", listener: () => void): this; addListener(event: "pipe", listener: (src: stream.Readable) => void): this; addListener(event: "unpipe", listener: (src: stream.Readable) => void): this; addListener(event: string | symbol, listener: (...args: any[]) => void): this; emit(event: "close"): boolean; emit(event: "drain"): boolean; emit(event: "error", error: Error): boolean; emit(event: "finish"): boolean; emit(event: "pipe", src: stream.Readable): boolean; emit(event: "unpipe", src: stream.Readable): boolean; emit(event: string | symbol, ...args: any[]): boolean; on(event: "close", listener: () => void): this; on(event: "drain", listener: () => void): this; on(event: "error", listener: (error: Error) => void): this; on(event: "finish", listener: () => void): this; on(event: "pipe", listener: (src: stream.Readable) => void): this; on(event: "unpipe", listener: (src: stream.Readable) => void): this; on(event: string | symbol, listener: (...args: any[]) => void): this; once(event: "close", listener: () => void): this; once(event: "drain", listener: () => void): this; once(event: "error", listener: (error: Error) => void): this; once(event: "finish", listener: () => void): this; once(event: "pipe", listener: (src: stream.Readable) => void): this; once(event: "unpipe", listener: (src: stream.Readable) => void): this; once(event: string | symbol, listener: (...args: any[]) => void): this; prependListener(event: "close", listener: () => void): this; prependListener(event: "drain", listener: () => void): this; prependListener(event: "error", listener: (error: Error) => void): this; prependListener(event: "finish", listener: () => void): this; prependListener(event: "pipe", listener: (src: stream.Readable) => void): this; prependListener(event: "unpipe", listener: (src: stream.Readable) => void): this; prependListener(event: string | symbol, listener: (...args: any[]) => void): this; prependOnceListener(event: "close", listener: () => void): this; prependOnceListener(event: "drain", listener: () => void): this; prependOnceListener(event: "error", listener: (error: Error) => void): this; prependOnceListener(event: "finish", listener: () => void): this; prependOnceListener(event: "pipe", listener: (src: stream.Readable) => void): this; prependOnceListener(event: "unpipe", listener: (src: stream.Readable) => void): this; prependOnceListener(event: string | symbol, listener: (...args: any[]) => void): this; } // Public API export namespace constants { const NGHTTP2_SESSION_SERVER: number; const NGHTTP2_SESSION_CLIENT: number; const NGHTTP2_STREAM_STATE_IDLE: number; const NGHTTP2_STREAM_STATE_OPEN: number; const NGHTTP2_STREAM_STATE_RESERVED_LOCAL: number; const NGHTTP2_STREAM_STATE_RESERVED_REMOTE: number; const NGHTTP2_STREAM_STATE_HALF_CLOSED_LOCAL: number; const NGHTTP2_STREAM_STATE_HALF_CLOSED_REMOTE: number; const NGHTTP2_STREAM_STATE_CLOSED: number; const NGHTTP2_NO_ERROR: number; const NGHTTP2_PROTOCOL_ERROR: number; const NGHTTP2_INTERNAL_ERROR: number; const NGHTTP2_FLOW_CONTROL_ERROR: number; const NGHTTP2_SETTINGS_TIMEOUT: number; const NGHTTP2_STREAM_CLOSED: number; const NGHTTP2_FRAME_SIZE_ERROR: number; const NGHTTP2_REFUSED_STREAM: number; const NGHTTP2_CANCEL: number; const NGHTTP2_COMPRESSION_ERROR: number; const NGHTTP2_CONNECT_ERROR: number; const NGHTTP2_ENHANCE_YOUR_CALM: number; const NGHTTP2_INADEQUATE_SECURITY: number; const NGHTTP2_HTTP_1_1_REQUIRED: number; const NGHTTP2_ERR_FRAME_SIZE_ERROR: number; const NGHTTP2_FLAG_NONE: number; const NGHTTP2_FLAG_END_STREAM: number; const NGHTTP2_FLAG_END_HEADERS: number; const NGHTTP2_FLAG_ACK: number; const NGHTTP2_FLAG_PADDED: number; const NGHTTP2_FLAG_PRIORITY: number; const DEFAULT_SETTINGS_HEADER_TABLE_SIZE: number; const DEFAULT_SETTINGS_ENABLE_PUSH: number; const DEFAULT_SETTINGS_INITIAL_WINDOW_SIZE: number; const DEFAULT_SETTINGS_MAX_FRAME_SIZE: number; const MAX_MAX_FRAME_SIZE: number; const MIN_MAX_FRAME_SIZE: number; const MAX_INITIAL_WINDOW_SIZE: number; const NGHTTP2_DEFAULT_WEIGHT: number; const NGHTTP2_SETTINGS_HEADER_TABLE_SIZE: number; const NGHTTP2_SETTINGS_ENABLE_PUSH: number; const NGHTTP2_SETTINGS_MAX_CONCURRENT_STREAMS: number; const NGHTTP2_SETTINGS_INITIAL_WINDOW_SIZE: number; const NGHTTP2_SETTINGS_MAX_FRAME_SIZE: number; const NGHTTP2_SETTINGS_MAX_HEADER_LIST_SIZE: number; const PADDING_STRATEGY_NONE: number; const PADDING_STRATEGY_MAX: number; const PADDING_STRATEGY_CALLBACK: number; const HTTP2_HEADER_STATUS: string; const HTTP2_HEADER_METHOD: string; const HTTP2_HEADER_AUTHORITY: string; const HTTP2_HEADER_SCHEME: string; const HTTP2_HEADER_PATH: string; const HTTP2_HEADER_ACCEPT_CHARSET: string; const HTTP2_HEADER_ACCEPT_ENCODING: string; const HTTP2_HEADER_ACCEPT_LANGUAGE: string; const HTTP2_HEADER_ACCEPT_RANGES: string; const HTTP2_HEADER_ACCEPT: string; const HTTP2_HEADER_ACCESS_CONTROL_ALLOW_ORIGIN: string; const HTTP2_HEADER_AGE: string; const HTTP2_HEADER_ALLOW: string; const HTTP2_HEADER_AUTHORIZATION: string; const HTTP2_HEADER_CACHE_CONTROL: string; const HTTP2_HEADER_CONNECTION: string; const HTTP2_HEADER_CONTENT_DISPOSITION: string; const HTTP2_HEADER_CONTENT_ENCODING: string; const HTTP2_HEADER_CONTENT_LANGUAGE: string; const HTTP2_HEADER_CONTENT_LENGTH: string; const HTTP2_HEADER_CONTENT_LOCATION: string; const HTTP2_HEADER_CONTENT_MD5: string; const HTTP2_HEADER_CONTENT_RANGE: string; const HTTP2_HEADER_CONTENT_TYPE: string; const HTTP2_HEADER_COOKIE: string; const HTTP2_HEADER_DATE: string; const HTTP2_HEADER_ETAG: string; const HTTP2_HEADER_EXPECT: string; const HTTP2_HEADER_EXPIRES: string; const HTTP2_HEADER_FROM: string; const HTTP2_HEADER_HOST: string; const HTTP2_HEADER_IF_MATCH: string; const HTTP2_HEADER_IF_MODIFIED_SINCE: string; const HTTP2_HEADER_IF_NONE_MATCH: string; const HTTP2_HEADER_IF_RANGE: string; const HTTP2_HEADER_IF_UNMODIFIED_SINCE: string; const HTTP2_HEADER_LAST_MODIFIED: string; const HTTP2_HEADER_LINK: string; const HTTP2_HEADER_LOCATION: string; const HTTP2_HEADER_MAX_FORWARDS: string; const HTTP2_HEADER_PREFER: string; const HTTP2_HEADER_PROXY_AUTHENTICATE: string; const HTTP2_HEADER_PROXY_AUTHORIZATION: string; const HTTP2_HEADER_RANGE: string; const HTTP2_HEADER_REFERER: string; const HTTP2_HEADER_REFRESH: string; const HTTP2_HEADER_RETRY_AFTER: string; const HTTP2_HEADER_SERVER: string; const HTTP2_HEADER_SET_COOKIE: string; const HTTP2_HEADER_STRICT_TRANSPORT_SECURITY: string; const HTTP2_HEADER_TRANSFER_ENCODING: string; const HTTP2_HEADER_TE: string; const HTTP2_HEADER_UPGRADE: string; const HTTP2_HEADER_USER_AGENT: string; const HTTP2_HEADER_VARY: string; const HTTP2_HEADER_VIA: string; const HTTP2_HEADER_WWW_AUTHENTICATE: string; const HTTP2_HEADER_HTTP2_SETTINGS: string; const HTTP2_HEADER_KEEP_ALIVE: string; const HTTP2_HEADER_PROXY_CONNECTION: string; const HTTP2_METHOD_ACL: string; const HTTP2_METHOD_BASELINE_CONTROL: string; const HTTP2_METHOD_BIND: string; const HTTP2_METHOD_CHECKIN: string; const HTTP2_METHOD_CHECKOUT: string; const HTTP2_METHOD_CONNECT: string; const HTTP2_METHOD_COPY: string; const HTTP2_METHOD_DELETE: string; const HTTP2_METHOD_GET: string; const HTTP2_METHOD_HEAD: string; const HTTP2_METHOD_LABEL: string; const HTTP2_METHOD_LINK: string; const HTTP2_METHOD_LOCK: string; const HTTP2_METHOD_MERGE: string; const HTTP2_METHOD_MKACTIVITY: string; const HTTP2_METHOD_MKCALENDAR: string; const HTTP2_METHOD_MKCOL: string; const HTTP2_METHOD_MKREDIRECTREF: string; const HTTP2_METHOD_MKWORKSPACE: string; const HTTP2_METHOD_MOVE: string; const HTTP2_METHOD_OPTIONS: string; const HTTP2_METHOD_ORDERPATCH: string; const HTTP2_METHOD_PATCH: string; const HTTP2_METHOD_POST: string; const HTTP2_METHOD_PRI: string; const HTTP2_METHOD_PROPFIND: string; const HTTP2_METHOD_PROPPATCH: string; const HTTP2_METHOD_PUT: string; const HTTP2_METHOD_REBIND: string; const HTTP2_METHOD_REPORT: string; const HTTP2_METHOD_SEARCH: string; const HTTP2_METHOD_TRACE: string; const HTTP2_METHOD_UNBIND: string; const HTTP2_METHOD_UNCHECKOUT: string; const HTTP2_METHOD_UNLINK: string; const HTTP2_METHOD_UNLOCK: string; const HTTP2_METHOD_UPDATE: string; const HTTP2_METHOD_UPDATEREDIRECTREF: string; const HTTP2_METHOD_VERSION_CONTROL: string; const HTTP_STATUS_CONTINUE: number; const HTTP_STATUS_SWITCHING_PROTOCOLS: number; const HTTP_STATUS_PROCESSING: number; const HTTP_STATUS_OK: number; const HTTP_STATUS_CREATED: number; const HTTP_STATUS_ACCEPTED: number; const HTTP_STATUS_NON_AUTHORITATIVE_INFORMATION: number; const HTTP_STATUS_NO_CONTENT: number; const HTTP_STATUS_RESET_CONTENT: number; const HTTP_STATUS_PARTIAL_CONTENT: number; const HTTP_STATUS_MULTI_STATUS: number; const HTTP_STATUS_ALREADY_REPORTED: number; const HTTP_STATUS_IM_USED: number; const HTTP_STATUS_MULTIPLE_CHOICES: number; const HTTP_STATUS_MOVED_PERMANENTLY: number; const HTTP_STATUS_FOUND: number; const HTTP_STATUS_SEE_OTHER: number; const HTTP_STATUS_NOT_MODIFIED: number; const HTTP_STATUS_USE_PROXY: number; const HTTP_STATUS_TEMPORARY_REDIRECT: number; const HTTP_STATUS_PERMANENT_REDIRECT: number; const HTTP_STATUS_BAD_REQUEST: number; const HTTP_STATUS_UNAUTHORIZED: number; const HTTP_STATUS_PAYMENT_REQUIRED: number; const HTTP_STATUS_FORBIDDEN: number; const HTTP_STATUS_NOT_FOUND: number; const HTTP_STATUS_METHOD_NOT_ALLOWED: number; const HTTP_STATUS_NOT_ACCEPTABLE: number; const HTTP_STATUS_PROXY_AUTHENTICATION_REQUIRED: number; const HTTP_STATUS_REQUEST_TIMEOUT: number; const HTTP_STATUS_CONFLICT: number; const HTTP_STATUS_GONE: number; const HTTP_STATUS_LENGTH_REQUIRED: number; const HTTP_STATUS_PRECONDITION_FAILED: number; const HTTP_STATUS_PAYLOAD_TOO_LARGE: number; const HTTP_STATUS_URI_TOO_LONG: number; const HTTP_STATUS_UNSUPPORTED_MEDIA_TYPE: number; const HTTP_STATUS_RANGE_NOT_SATISFIABLE: number; const HTTP_STATUS_EXPECTATION_FAILED: number; const HTTP_STATUS_TEAPOT: number; const HTTP_STATUS_MISDIRECTED_REQUEST: number; const HTTP_STATUS_UNPROCESSABLE_ENTITY: number; const HTTP_STATUS_LOCKED: number; const HTTP_STATUS_FAILED_DEPENDENCY: number; const HTTP_STATUS_UNORDERED_COLLECTION: number; const HTTP_STATUS_UPGRADE_REQUIRED: number; const HTTP_STATUS_PRECONDITION_REQUIRED: number; const HTTP_STATUS_TOO_MANY_REQUESTS: number; const HTTP_STATUS_REQUEST_HEADER_FIELDS_TOO_LARGE: number; const HTTP_STATUS_UNAVAILABLE_FOR_LEGAL_REASONS: number; const HTTP_STATUS_INTERNAL_SERVER_ERROR: number; const HTTP_STATUS_NOT_IMPLEMENTED: number; const HTTP_STATUS_BAD_GATEWAY: number; const HTTP_STATUS_SERVICE_UNAVAILABLE: number; const HTTP_STATUS_GATEWAY_TIMEOUT: number; const HTTP_STATUS_HTTP_VERSION_NOT_SUPPORTED: number; const HTTP_STATUS_VARIANT_ALSO_NEGOTIATES: number; const HTTP_STATUS_INSUFFICIENT_STORAGE: number; const HTTP_STATUS_LOOP_DETECTED: number; const HTTP_STATUS_BANDWIDTH_LIMIT_EXCEEDED: number; const HTTP_STATUS_NOT_EXTENDED: number; const HTTP_STATUS_NETWORK_AUTHENTICATION_REQUIRED: number; } export function getDefaultSettings(): Settings; export function getPackedSettings(settings: Settings): Buffer; export function getUnpackedSettings(buf: Uint8Array): Settings; export function createServer(onRequestHandler?: (request: Http2ServerRequest, response: Http2ServerResponse) => void): Http2Server; export function createServer(options: ServerOptions, onRequestHandler?: (request: Http2ServerRequest, response: Http2ServerResponse) => void): Http2Server; export function createSecureServer(onRequestHandler?: (request: Http2ServerRequest, response: Http2ServerResponse) => void): Http2SecureServer; export function createSecureServer(options: SecureServerOptions, onRequestHandler?: (request: Http2ServerRequest, response: Http2ServerResponse) => void): Http2SecureServer; export function connect(authority: string | url.URL, listener: (session: ClientHttp2Session, socket: net.Socket | tls.TLSSocket) => void): ClientHttp2Session; export function connect( authority: string | url.URL, options?: ClientSessionOptions | SecureClientSessionOptions, listener?: (session: ClientHttp2Session, socket: net.Socket | tls.TLSSocket) => void ): ClientHttp2Session; } apollo-server-demo/node_modules/@types/node/trace_events.d.ts 0000644 0001750 0000144 00000004101 14000133701 024055 0 ustar andreh users declare module "trace_events" { /** * The `Tracing` object is used to enable or disable tracing for sets of * categories. Instances are created using the * `trace_events.createTracing()` method. * * When created, the `Tracing` object is disabled. Calling the * `tracing.enable()` method adds the categories to the set of enabled trace * event categories. Calling `tracing.disable()` will remove the categories * from the set of enabled trace event categories. */ interface Tracing { /** * A comma-separated list of the trace event categories covered by this * `Tracing` object. */ readonly categories: string; /** * Disables this `Tracing` object. * * Only trace event categories _not_ covered by other enabled `Tracing` * objects and _not_ specified by the `--trace-event-categories` flag * will be disabled. */ disable(): void; /** * Enables this `Tracing` object for the set of categories covered by * the `Tracing` object. */ enable(): void; /** * `true` only if the `Tracing` object has been enabled. */ readonly enabled: boolean; } interface CreateTracingOptions { /** * An array of trace category names. Values included in the array are * coerced to a string when possible. An error will be thrown if the * value cannot be coerced. */ categories: string[]; } /** * Creates and returns a Tracing object for the given set of categories. */ function createTracing(options: CreateTracingOptions): Tracing; /** * Returns a comma-separated list of all currently-enabled trace event * categories. The current set of enabled trace event categories is * determined by the union of all currently-enabled `Tracing` objects and * any categories enabled using the `--trace-event-categories` flag. */ function getEnabledCategories(): string | undefined; } apollo-server-demo/node_modules/@types/node/zlib.d.ts 0000644 0001750 0000144 00000033767 14000133701 022357 0 ustar andreh users declare module "zlib" { import * as stream from "stream"; interface ZlibOptions { /** * @default constants.Z_NO_FLUSH */ flush?: number; /** * @default constants.Z_FINISH */ finishFlush?: number; /** * @default 16*1024 */ chunkSize?: number; windowBits?: number; level?: number; // compression only memLevel?: number; // compression only strategy?: number; // compression only dictionary?: NodeJS.ArrayBufferView | ArrayBuffer; // deflate/inflate only, empty dictionary by default info?: boolean; maxOutputLength?: number; } interface BrotliOptions { /** * @default constants.BROTLI_OPERATION_PROCESS */ flush?: number; /** * @default constants.BROTLI_OPERATION_FINISH */ finishFlush?: number; /** * @default 16*1024 */ chunkSize?: number; params?: { /** * Each key is a `constants.BROTLI_*` constant. */ [key: number]: boolean | number; }; maxOutputLength?: number; } interface Zlib { /** @deprecated Use bytesWritten instead. */ readonly bytesRead: number; readonly bytesWritten: number; shell?: boolean | string; close(callback?: () => void): void; flush(kind?: number, callback?: () => void): void; flush(callback?: () => void): void; } interface ZlibParams { params(level: number, strategy: number, callback: () => void): void; } interface ZlibReset { reset(): void; } interface BrotliCompress extends stream.Transform, Zlib { } interface BrotliDecompress extends stream.Transform, Zlib { } interface Gzip extends stream.Transform, Zlib { } interface Gunzip extends stream.Transform, Zlib { } interface Deflate extends stream.Transform, Zlib, ZlibReset, ZlibParams { } interface Inflate extends stream.Transform, Zlib, ZlibReset { } interface DeflateRaw extends stream.Transform, Zlib, ZlibReset, ZlibParams { } interface InflateRaw extends stream.Transform, Zlib, ZlibReset { } interface Unzip extends stream.Transform, Zlib { } function createBrotliCompress(options?: BrotliOptions): BrotliCompress; function createBrotliDecompress(options?: BrotliOptions): BrotliDecompress; function createGzip(options?: ZlibOptions): Gzip; function createGunzip(options?: ZlibOptions): Gunzip; function createDeflate(options?: ZlibOptions): Deflate; function createInflate(options?: ZlibOptions): Inflate; function createDeflateRaw(options?: ZlibOptions): DeflateRaw; function createInflateRaw(options?: ZlibOptions): InflateRaw; function createUnzip(options?: ZlibOptions): Unzip; type InputType = string | ArrayBuffer | NodeJS.ArrayBufferView; type CompressCallback = (error: Error | null, result: Buffer) => void; function brotliCompress(buf: InputType, options: BrotliOptions, callback: CompressCallback): void; function brotliCompress(buf: InputType, callback: CompressCallback): void; namespace brotliCompress { function __promisify__(buffer: InputType, options?: BrotliOptions): Promise<Buffer>; } function brotliCompressSync(buf: InputType, options?: BrotliOptions): Buffer; function brotliDecompress(buf: InputType, options: BrotliOptions, callback: CompressCallback): void; function brotliDecompress(buf: InputType, callback: CompressCallback): void; namespace brotliDecompress { function __promisify__(buffer: InputType, options?: BrotliOptions): Promise<Buffer>; } function brotliDecompressSync(buf: InputType, options?: BrotliOptions): Buffer; function deflate(buf: InputType, callback: CompressCallback): void; function deflate(buf: InputType, options: ZlibOptions, callback: CompressCallback): void; namespace deflate { function __promisify__(buffer: InputType, options?: ZlibOptions): Promise<Buffer>; } function deflateSync(buf: InputType, options?: ZlibOptions): Buffer; function deflateRaw(buf: InputType, callback: CompressCallback): void; function deflateRaw(buf: InputType, options: ZlibOptions, callback: CompressCallback): void; namespace deflateRaw { function __promisify__(buffer: InputType, options?: ZlibOptions): Promise<Buffer>; } function deflateRawSync(buf: InputType, options?: ZlibOptions): Buffer; function gzip(buf: InputType, callback: CompressCallback): void; function gzip(buf: InputType, options: ZlibOptions, callback: CompressCallback): void; namespace gzip { function __promisify__(buffer: InputType, options?: ZlibOptions): Promise<Buffer>; } function gzipSync(buf: InputType, options?: ZlibOptions): Buffer; function gunzip(buf: InputType, callback: CompressCallback): void; function gunzip(buf: InputType, options: ZlibOptions, callback: CompressCallback): void; namespace gunzip { function __promisify__(buffer: InputType, options?: ZlibOptions): Promise<Buffer>; } function gunzipSync(buf: InputType, options?: ZlibOptions): Buffer; function inflate(buf: InputType, callback: CompressCallback): void; function inflate(buf: InputType, options: ZlibOptions, callback: CompressCallback): void; namespace inflate { function __promisify__(buffer: InputType, options?: ZlibOptions): Promise<Buffer>; } function inflateSync(buf: InputType, options?: ZlibOptions): Buffer; function inflateRaw(buf: InputType, callback: CompressCallback): void; function inflateRaw(buf: InputType, options: ZlibOptions, callback: CompressCallback): void; namespace inflateRaw { function __promisify__(buffer: InputType, options?: ZlibOptions): Promise<Buffer>; } function inflateRawSync(buf: InputType, options?: ZlibOptions): Buffer; function unzip(buf: InputType, callback: CompressCallback): void; function unzip(buf: InputType, options: ZlibOptions, callback: CompressCallback): void; namespace unzip { function __promisify__(buffer: InputType, options?: ZlibOptions): Promise<Buffer>; } function unzipSync(buf: InputType, options?: ZlibOptions): Buffer; namespace constants { const BROTLI_DECODE: number; const BROTLI_DECODER_ERROR_ALLOC_BLOCK_TYPE_TREES: number; const BROTLI_DECODER_ERROR_ALLOC_CONTEXT_MAP: number; const BROTLI_DECODER_ERROR_ALLOC_CONTEXT_MODES: number; const BROTLI_DECODER_ERROR_ALLOC_RING_BUFFER_1: number; const BROTLI_DECODER_ERROR_ALLOC_RING_BUFFER_2: number; const BROTLI_DECODER_ERROR_ALLOC_TREE_GROUPS: number; const BROTLI_DECODER_ERROR_DICTIONARY_NOT_SET: number; const BROTLI_DECODER_ERROR_FORMAT_BLOCK_LENGTH_1: number; const BROTLI_DECODER_ERROR_FORMAT_BLOCK_LENGTH_2: number; const BROTLI_DECODER_ERROR_FORMAT_CL_SPACE: number; const BROTLI_DECODER_ERROR_FORMAT_CONTEXT_MAP_REPEAT: number; const BROTLI_DECODER_ERROR_FORMAT_DICTIONARY: number; const BROTLI_DECODER_ERROR_FORMAT_DISTANCE: number; const BROTLI_DECODER_ERROR_FORMAT_EXUBERANT_META_NIBBLE: number; const BROTLI_DECODER_ERROR_FORMAT_EXUBERANT_NIBBLE: number; const BROTLI_DECODER_ERROR_FORMAT_HUFFMAN_SPACE: number; const BROTLI_DECODER_ERROR_FORMAT_PADDING_1: number; const BROTLI_DECODER_ERROR_FORMAT_PADDING_2: number; const BROTLI_DECODER_ERROR_FORMAT_RESERVED: number; const BROTLI_DECODER_ERROR_FORMAT_SIMPLE_HUFFMAN_ALPHABET: number; const BROTLI_DECODER_ERROR_FORMAT_SIMPLE_HUFFMAN_SAME: number; const BROTLI_DECODER_ERROR_FORMAT_TRANSFORM: number; const BROTLI_DECODER_ERROR_FORMAT_WINDOW_BITS: number; const BROTLI_DECODER_ERROR_INVALID_ARGUMENTS: number; const BROTLI_DECODER_ERROR_UNREACHABLE: number; const BROTLI_DECODER_NEEDS_MORE_INPUT: number; const BROTLI_DECODER_NEEDS_MORE_OUTPUT: number; const BROTLI_DECODER_NO_ERROR: number; const BROTLI_DECODER_PARAM_DISABLE_RING_BUFFER_REALLOCATION: number; const BROTLI_DECODER_PARAM_LARGE_WINDOW: number; const BROTLI_DECODER_RESULT_ERROR: number; const BROTLI_DECODER_RESULT_NEEDS_MORE_INPUT: number; const BROTLI_DECODER_RESULT_NEEDS_MORE_OUTPUT: number; const BROTLI_DECODER_RESULT_SUCCESS: number; const BROTLI_DECODER_SUCCESS: number; const BROTLI_DEFAULT_MODE: number; const BROTLI_DEFAULT_QUALITY: number; const BROTLI_DEFAULT_WINDOW: number; const BROTLI_ENCODE: number; const BROTLI_LARGE_MAX_WINDOW_BITS: number; const BROTLI_MAX_INPUT_BLOCK_BITS: number; const BROTLI_MAX_QUALITY: number; const BROTLI_MAX_WINDOW_BITS: number; const BROTLI_MIN_INPUT_BLOCK_BITS: number; const BROTLI_MIN_QUALITY: number; const BROTLI_MIN_WINDOW_BITS: number; const BROTLI_MODE_FONT: number; const BROTLI_MODE_GENERIC: number; const BROTLI_MODE_TEXT: number; const BROTLI_OPERATION_EMIT_METADATA: number; const BROTLI_OPERATION_FINISH: number; const BROTLI_OPERATION_FLUSH: number; const BROTLI_OPERATION_PROCESS: number; const BROTLI_PARAM_DISABLE_LITERAL_CONTEXT_MODELING: number; const BROTLI_PARAM_LARGE_WINDOW: number; const BROTLI_PARAM_LGBLOCK: number; const BROTLI_PARAM_LGWIN: number; const BROTLI_PARAM_MODE: number; const BROTLI_PARAM_NDIRECT: number; const BROTLI_PARAM_NPOSTFIX: number; const BROTLI_PARAM_QUALITY: number; const BROTLI_PARAM_SIZE_HINT: number; const DEFLATE: number; const DEFLATERAW: number; const GUNZIP: number; const GZIP: number; const INFLATE: number; const INFLATERAW: number; const UNZIP: number; // Allowed flush values. const Z_NO_FLUSH: number; const Z_PARTIAL_FLUSH: number; const Z_SYNC_FLUSH: number; const Z_FULL_FLUSH: number; const Z_FINISH: number; const Z_BLOCK: number; const Z_TREES: number; // Return codes for the compression/decompression functions. // Negative values are errors, positive values are used for special but normal events. const Z_OK: number; const Z_STREAM_END: number; const Z_NEED_DICT: number; const Z_ERRNO: number; const Z_STREAM_ERROR: number; const Z_DATA_ERROR: number; const Z_MEM_ERROR: number; const Z_BUF_ERROR: number; const Z_VERSION_ERROR: number; // Compression levels. const Z_NO_COMPRESSION: number; const Z_BEST_SPEED: number; const Z_BEST_COMPRESSION: number; const Z_DEFAULT_COMPRESSION: number; // Compression strategy. const Z_FILTERED: number; const Z_HUFFMAN_ONLY: number; const Z_RLE: number; const Z_FIXED: number; const Z_DEFAULT_STRATEGY: number; const Z_DEFAULT_WINDOWBITS: number; const Z_MIN_WINDOWBITS: number; const Z_MAX_WINDOWBITS: number; const Z_MIN_CHUNK: number; const Z_MAX_CHUNK: number; const Z_DEFAULT_CHUNK: number; const Z_MIN_MEMLEVEL: number; const Z_MAX_MEMLEVEL: number; const Z_DEFAULT_MEMLEVEL: number; const Z_MIN_LEVEL: number; const Z_MAX_LEVEL: number; const Z_DEFAULT_LEVEL: number; const ZLIB_VERNUM: number; } // Allowed flush values. /** @deprecated Use `constants.Z_NO_FLUSH` */ const Z_NO_FLUSH: number; /** @deprecated Use `constants.Z_PARTIAL_FLUSH` */ const Z_PARTIAL_FLUSH: number; /** @deprecated Use `constants.Z_SYNC_FLUSH` */ const Z_SYNC_FLUSH: number; /** @deprecated Use `constants.Z_FULL_FLUSH` */ const Z_FULL_FLUSH: number; /** @deprecated Use `constants.Z_FINISH` */ const Z_FINISH: number; /** @deprecated Use `constants.Z_BLOCK` */ const Z_BLOCK: number; /** @deprecated Use `constants.Z_TREES` */ const Z_TREES: number; // Return codes for the compression/decompression functions. // Negative values are errors, positive values are used for special but normal events. /** @deprecated Use `constants.Z_OK` */ const Z_OK: number; /** @deprecated Use `constants.Z_STREAM_END` */ const Z_STREAM_END: number; /** @deprecated Use `constants.Z_NEED_DICT` */ const Z_NEED_DICT: number; /** @deprecated Use `constants.Z_ERRNO` */ const Z_ERRNO: number; /** @deprecated Use `constants.Z_STREAM_ERROR` */ const Z_STREAM_ERROR: number; /** @deprecated Use `constants.Z_DATA_ERROR` */ const Z_DATA_ERROR: number; /** @deprecated Use `constants.Z_MEM_ERROR` */ const Z_MEM_ERROR: number; /** @deprecated Use `constants.Z_BUF_ERROR` */ const Z_BUF_ERROR: number; /** @deprecated Use `constants.Z_VERSION_ERROR` */ const Z_VERSION_ERROR: number; // Compression levels. /** @deprecated Use `constants.Z_NO_COMPRESSION` */ const Z_NO_COMPRESSION: number; /** @deprecated Use `constants.Z_BEST_SPEED` */ const Z_BEST_SPEED: number; /** @deprecated Use `constants.Z_BEST_COMPRESSION` */ const Z_BEST_COMPRESSION: number; /** @deprecated Use `constants.Z_DEFAULT_COMPRESSION` */ const Z_DEFAULT_COMPRESSION: number; // Compression strategy. /** @deprecated Use `constants.Z_FILTERED` */ const Z_FILTERED: number; /** @deprecated Use `constants.Z_HUFFMAN_ONLY` */ const Z_HUFFMAN_ONLY: number; /** @deprecated Use `constants.Z_RLE` */ const Z_RLE: number; /** @deprecated Use `constants.Z_FIXED` */ const Z_FIXED: number; /** @deprecated Use `constants.Z_DEFAULT_STRATEGY` */ const Z_DEFAULT_STRATEGY: number; /** @deprecated */ const Z_BINARY: number; /** @deprecated */ const Z_TEXT: number; /** @deprecated */ const Z_ASCII: number; /** @deprecated */ const Z_UNKNOWN: number; /** @deprecated */ const Z_DEFLATED: number; } apollo-server-demo/node_modules/@types/node/tls.d.ts 0000644 0001750 0000144 00000111600 14000133701 022200 0 ustar andreh users declare module "tls" { import * as crypto from "crypto"; import * as dns from "dns"; import * as net from "net"; import * as stream from "stream"; const CLIENT_RENEG_LIMIT: number; const CLIENT_RENEG_WINDOW: number; interface Certificate { /** * Country code. */ C: string; /** * Street. */ ST: string; /** * Locality. */ L: string; /** * Organization. */ O: string; /** * Organizational unit. */ OU: string; /** * Common name. */ CN: string; } interface PeerCertificate { subject: Certificate; issuer: Certificate; subjectaltname: string; infoAccess: NodeJS.Dict<string[]>; modulus: string; exponent: string; valid_from: string; valid_to: string; fingerprint: string; fingerprint256: string; ext_key_usage: string[]; serialNumber: string; raw: Buffer; } interface DetailedPeerCertificate extends PeerCertificate { issuerCertificate: DetailedPeerCertificate; } interface CipherNameAndProtocol { /** * The cipher name. */ name: string; /** * SSL/TLS protocol version. */ version: string; /** * IETF name for the cipher suite. */ standardName: string; } interface EphemeralKeyInfo { /** * The supported types are 'DH' and 'ECDH'. */ type: string; /** * The name property is available only when type is 'ECDH'. */ name?: string; /** * The size of parameter of an ephemeral key exchange. */ size: number; } interface KeyObject { /** * Private keys in PEM format. */ pem: string | Buffer; /** * Optional passphrase. */ passphrase?: string; } interface PxfObject { /** * PFX or PKCS12 encoded private key and certificate chain. */ buf: string | Buffer; /** * Optional passphrase. */ passphrase?: string; } interface TLSSocketOptions extends SecureContextOptions, CommonConnectionOptions { /** * If true the TLS socket will be instantiated in server-mode. * Defaults to false. */ isServer?: boolean; /** * An optional net.Server instance. */ server?: net.Server; /** * An optional Buffer instance containing a TLS session. */ session?: Buffer; /** * If true, specifies that the OCSP status request extension will be * added to the client hello and an 'OCSPResponse' event will be * emitted on the socket before establishing a secure communication */ requestOCSP?: boolean; } class TLSSocket extends net.Socket { /** * Construct a new tls.TLSSocket object from an existing TCP socket. */ constructor(socket: net.Socket, options?: TLSSocketOptions); /** * A boolean that is true if the peer certificate was signed by one of the specified CAs, otherwise false. */ authorized: boolean; /** * The reason why the peer's certificate has not been verified. * This property becomes available only when tlsSocket.authorized === false. */ authorizationError: Error; /** * Static boolean value, always true. * May be used to distinguish TLS sockets from regular ones. */ encrypted: boolean; /** * String containing the selected ALPN protocol. * When ALPN has no selected protocol, tlsSocket.alpnProtocol equals false. */ alpnProtocol?: string; /** * Returns an object representing the local certificate. The returned * object has some properties corresponding to the fields of the * certificate. * * See tls.TLSSocket.getPeerCertificate() for an example of the * certificate structure. * * If there is no local certificate, an empty object will be returned. * If the socket has been destroyed, null will be returned. */ getCertificate(): PeerCertificate | object | null; /** * Returns an object representing the cipher name and the SSL/TLS protocol version of the current connection. * @returns Returns an object representing the cipher name * and the SSL/TLS protocol version of the current connection. */ getCipher(): CipherNameAndProtocol; /** * Returns an object representing the type, name, and size of parameter * of an ephemeral key exchange in Perfect Forward Secrecy on a client * connection. It returns an empty object when the key exchange is not * ephemeral. As this is only supported on a client socket; null is * returned if called on a server socket. The supported types are 'DH' * and 'ECDH'. The name property is available only when type is 'ECDH'. * * For example: { type: 'ECDH', name: 'prime256v1', size: 256 }. */ getEphemeralKeyInfo(): EphemeralKeyInfo | object | null; /** * Returns the latest Finished message that has * been sent to the socket as part of a SSL/TLS handshake, or undefined * if no Finished message has been sent yet. * * As the Finished messages are message digests of the complete * handshake (with a total of 192 bits for TLS 1.0 and more for SSL * 3.0), they can be used for external authentication procedures when * the authentication provided by SSL/TLS is not desired or is not * enough. * * Corresponds to the SSL_get_finished routine in OpenSSL and may be * used to implement the tls-unique channel binding from RFC 5929. */ getFinished(): Buffer | undefined; /** * Returns an object representing the peer's certificate. * The returned object has some properties corresponding to the field of the certificate. * If detailed argument is true the full chain with issuer property will be returned, * if false only the top certificate without issuer property. * If the peer does not provide a certificate, it returns null or an empty object. * @param detailed - If true; the full chain with issuer property will be returned. * @returns An object representing the peer's certificate. */ getPeerCertificate(detailed: true): DetailedPeerCertificate; getPeerCertificate(detailed?: false): PeerCertificate; getPeerCertificate(detailed?: boolean): PeerCertificate | DetailedPeerCertificate; /** * Returns the latest Finished message that is expected or has actually * been received from the socket as part of a SSL/TLS handshake, or * undefined if there is no Finished message so far. * * As the Finished messages are message digests of the complete * handshake (with a total of 192 bits for TLS 1.0 and more for SSL * 3.0), they can be used for external authentication procedures when * the authentication provided by SSL/TLS is not desired or is not * enough. * * Corresponds to the SSL_get_peer_finished routine in OpenSSL and may * be used to implement the tls-unique channel binding from RFC 5929. */ getPeerFinished(): Buffer | undefined; /** * Returns a string containing the negotiated SSL/TLS protocol version of the current connection. * The value `'unknown'` will be returned for connected sockets that have not completed the handshaking process. * The value `null` will be returned for server sockets or disconnected client sockets. * See https://www.openssl.org/docs/man1.0.2/ssl/SSL_get_version.html for more information. * @returns negotiated SSL/TLS protocol version of the current connection */ getProtocol(): string | null; /** * Could be used to speed up handshake establishment when reconnecting to the server. * @returns ASN.1 encoded TLS session or undefined if none was negotiated. */ getSession(): Buffer | undefined; /** * Returns a list of signature algorithms shared between the server and * the client in the order of decreasing preference. */ getSharedSigalgs(): string[]; /** * NOTE: Works only with client TLS sockets. * Useful only for debugging, for session reuse provide session option to tls.connect(). * @returns TLS session ticket or undefined if none was negotiated. */ getTLSTicket(): Buffer | undefined; /** * Returns true if the session was reused, false otherwise. */ isSessionReused(): boolean; /** * Initiate TLS renegotiation process. * * NOTE: Can be used to request peer's certificate after the secure connection has been established. * ANOTHER NOTE: When running as the server, socket will be destroyed with an error after handshakeTimeout timeout. * @param options - The options may contain the following fields: rejectUnauthorized, * requestCert (See tls.createServer() for details). * @param callback - callback(err) will be executed with null as err, once the renegotiation * is successfully completed. * @return `undefined` when socket is destroy, `false` if negotiaion can't be initiated. */ renegotiate(options: { rejectUnauthorized?: boolean, requestCert?: boolean }, callback: (err: Error | null) => void): undefined | boolean; /** * Set maximum TLS fragment size (default and maximum value is: 16384, minimum is: 512). * Smaller fragment size decreases buffering latency on the client: large fragments are buffered by * the TLS layer until the entire fragment is received and its integrity is verified; * large fragments can span multiple roundtrips, and their processing can be delayed due to packet * loss or reordering. However, smaller fragments add extra TLS framing bytes and CPU overhead, * which may decrease overall server throughput. * @param size - TLS fragment size (default and maximum value is: 16384, minimum is: 512). * @returns Returns true on success, false otherwise. */ setMaxSendFragment(size: number): boolean; /** * Disables TLS renegotiation for this TLSSocket instance. Once called, * attempts to renegotiate will trigger an 'error' event on the * TLSSocket. */ disableRenegotiation(): void; /** * When enabled, TLS packet trace information is written to `stderr`. This can be * used to debug TLS connection problems. * * Note: The format of the output is identical to the output of `openssl s_client * -trace` or `openssl s_server -trace`. While it is produced by OpenSSL's * `SSL_trace()` function, the format is undocumented, can change without notice, * and should not be relied on. */ enableTrace(): void; /** * @param length number of bytes to retrieve from keying material * @param label an application specific label, typically this will be a value from the * [IANA Exporter Label Registry](https://www.iana.org/assignments/tls-parameters/tls-parameters.xhtml#exporter-labels). * @param context optionally provide a context. */ exportKeyingMaterial(length: number, label: string, context: Buffer): Buffer; addListener(event: string, listener: (...args: any[]) => void): this; addListener(event: "OCSPResponse", listener: (response: Buffer) => void): this; addListener(event: "secureConnect", listener: () => void): this; addListener(event: "session", listener: (session: Buffer) => void): this; addListener(event: "keylog", listener: (line: Buffer) => void): this; emit(event: string | symbol, ...args: any[]): boolean; emit(event: "OCSPResponse", response: Buffer): boolean; emit(event: "secureConnect"): boolean; emit(event: "session", session: Buffer): boolean; emit(event: "keylog", line: Buffer): boolean; on(event: string, listener: (...args: any[]) => void): this; on(event: "OCSPResponse", listener: (response: Buffer) => void): this; on(event: "secureConnect", listener: () => void): this; on(event: "session", listener: (session: Buffer) => void): this; on(event: "keylog", listener: (line: Buffer) => void): this; once(event: string, listener: (...args: any[]) => void): this; once(event: "OCSPResponse", listener: (response: Buffer) => void): this; once(event: "secureConnect", listener: () => void): this; once(event: "session", listener: (session: Buffer) => void): this; once(event: "keylog", listener: (line: Buffer) => void): this; prependListener(event: string, listener: (...args: any[]) => void): this; prependListener(event: "OCSPResponse", listener: (response: Buffer) => void): this; prependListener(event: "secureConnect", listener: () => void): this; prependListener(event: "session", listener: (session: Buffer) => void): this; prependListener(event: "keylog", listener: (line: Buffer) => void): this; prependOnceListener(event: string, listener: (...args: any[]) => void): this; prependOnceListener(event: "OCSPResponse", listener: (response: Buffer) => void): this; prependOnceListener(event: "secureConnect", listener: () => void): this; prependOnceListener(event: "session", listener: (session: Buffer) => void): this; prependOnceListener(event: "keylog", listener: (line: Buffer) => void): this; } interface CommonConnectionOptions { /** * An optional TLS context object from tls.createSecureContext() */ secureContext?: SecureContext; /** * When enabled, TLS packet trace information is written to `stderr`. This can be * used to debug TLS connection problems. * @default false */ enableTrace?: boolean; /** * If true the server will request a certificate from clients that * connect and attempt to verify that certificate. Defaults to * false. */ requestCert?: boolean; /** * An array of strings or a Buffer naming possible ALPN protocols. * (Protocols should be ordered by their priority.) */ ALPNProtocols?: string[] | Uint8Array[] | Uint8Array; /** * SNICallback(servername, cb) <Function> A function that will be * called if the client supports SNI TLS extension. Two arguments * will be passed when called: servername and cb. SNICallback should * invoke cb(null, ctx), where ctx is a SecureContext instance. * (tls.createSecureContext(...) can be used to get a proper * SecureContext.) If SNICallback wasn't provided the default callback * with high-level API will be used (see below). */ SNICallback?: (servername: string, cb: (err: Error | null, ctx: SecureContext) => void) => void; /** * If true the server will reject any connection which is not * authorized with the list of supplied CAs. This option only has an * effect if requestCert is true. * @default true */ rejectUnauthorized?: boolean; } interface TlsOptions extends SecureContextOptions, CommonConnectionOptions { /** * Abort the connection if the SSL/TLS handshake does not finish in the * specified number of milliseconds. A 'tlsClientError' is emitted on * the tls.Server object whenever a handshake times out. Default: * 120000 (120 seconds). */ handshakeTimeout?: number; /** * The number of seconds after which a TLS session created by the * server will no longer be resumable. See Session Resumption for more * information. Default: 300. */ sessionTimeout?: number; /** * 48-bytes of cryptographically strong pseudo-random data. */ ticketKeys?: Buffer; /** * * @param socket * @param identity identity parameter sent from the client. * @return pre-shared key that must either be * a buffer or `null` to stop the negotiation process. Returned PSK must be * compatible with the selected cipher's digest. * * When negotiating TLS-PSK (pre-shared keys), this function is called * with the identity provided by the client. * If the return value is `null` the negotiation process will stop and an * "unknown_psk_identity" alert message will be sent to the other party. * If the server wishes to hide the fact that the PSK identity was not known, * the callback must provide some random data as `psk` to make the connection * fail with "decrypt_error" before negotiation is finished. * PSK ciphers are disabled by default, and using TLS-PSK thus * requires explicitly specifying a cipher suite with the `ciphers` option. * More information can be found in the RFC 4279. */ pskCallback?(socket: TLSSocket, identity: string): DataView | NodeJS.TypedArray | null; /** * hint to send to a client to help * with selecting the identity during TLS-PSK negotiation. Will be ignored * in TLS 1.3. Upon failing to set pskIdentityHint `tlsClientError` will be * emitted with `ERR_TLS_PSK_SET_IDENTIY_HINT_FAILED` code. */ pskIdentityHint?: string; } interface PSKCallbackNegotation { psk: DataView | NodeJS.TypedArray; identity: string; } interface ConnectionOptions extends SecureContextOptions, CommonConnectionOptions { host?: string; port?: number; path?: string; // Creates unix socket connection to path. If this option is specified, `host` and `port` are ignored. socket?: net.Socket; // Establish secure connection on a given socket rather than creating a new socket checkServerIdentity?: typeof checkServerIdentity; servername?: string; // SNI TLS Extension session?: Buffer; minDHSize?: number; lookup?: net.LookupFunction; timeout?: number; /** * When negotiating TLS-PSK (pre-shared keys), this function is called * with optional identity `hint` provided by the server or `null` * in case of TLS 1.3 where `hint` was removed. * It will be necessary to provide a custom `tls.checkServerIdentity()` * for the connection as the default one will try to check hostname/IP * of the server against the certificate but that's not applicable for PSK * because there won't be a certificate present. * More information can be found in the RFC 4279. * * @param hint message sent from the server to help client * decide which identity to use during negotiation. * Always `null` if TLS 1.3 is used. * @returns Return `null` to stop the negotiation process. `psk` must be * compatible with the selected cipher's digest. * `identity` must use UTF-8 encoding. */ pskCallback?(hint: string | null): PSKCallbackNegotation | null; } class Server extends net.Server { /** * The server.addContext() method adds a secure context that will be * used if the client request's SNI name matches the supplied hostname * (or wildcard). */ addContext(hostName: string, credentials: SecureContextOptions): void; /** * Returns the session ticket keys. */ getTicketKeys(): Buffer; /** * * The server.setSecureContext() method replaces the * secure context of an existing server. Existing connections to the * server are not interrupted. */ setSecureContext(details: SecureContextOptions): void; /** * The server.setSecureContext() method replaces the secure context of * an existing server. Existing connections to the server are not * interrupted. */ setTicketKeys(keys: Buffer): void; /** * events.EventEmitter * 1. tlsClientError * 2. newSession * 3. OCSPRequest * 4. resumeSession * 5. secureConnection * 6. keylog */ addListener(event: string, listener: (...args: any[]) => void): this; addListener(event: "tlsClientError", listener: (err: Error, tlsSocket: TLSSocket) => void): this; addListener(event: "newSession", listener: (sessionId: Buffer, sessionData: Buffer, callback: (err: Error, resp: Buffer) => void) => void): this; addListener(event: "OCSPRequest", listener: (certificate: Buffer, issuer: Buffer, callback: (err: Error | null, resp: Buffer) => void) => void): this; addListener(event: "resumeSession", listener: (sessionId: Buffer, callback: (err: Error, sessionData: Buffer) => void) => void): this; addListener(event: "secureConnection", listener: (tlsSocket: TLSSocket) => void): this; addListener(event: "keylog", listener: (line: Buffer, tlsSocket: TLSSocket) => void): this; emit(event: string | symbol, ...args: any[]): boolean; emit(event: "tlsClientError", err: Error, tlsSocket: TLSSocket): boolean; emit(event: "newSession", sessionId: Buffer, sessionData: Buffer, callback: (err: Error, resp: Buffer) => void): boolean; emit(event: "OCSPRequest", certificate: Buffer, issuer: Buffer, callback: (err: Error | null, resp: Buffer) => void): boolean; emit(event: "resumeSession", sessionId: Buffer, callback: (err: Error, sessionData: Buffer) => void): boolean; emit(event: "secureConnection", tlsSocket: TLSSocket): boolean; emit(event: "keylog", line: Buffer, tlsSocket: TLSSocket): boolean; on(event: string, listener: (...args: any[]) => void): this; on(event: "tlsClientError", listener: (err: Error, tlsSocket: TLSSocket) => void): this; on(event: "newSession", listener: (sessionId: Buffer, sessionData: Buffer, callback: (err: Error, resp: Buffer) => void) => void): this; on(event: "OCSPRequest", listener: (certificate: Buffer, issuer: Buffer, callback: (err: Error | null, resp: Buffer) => void) => void): this; on(event: "resumeSession", listener: (sessionId: Buffer, callback: (err: Error, sessionData: Buffer) => void) => void): this; on(event: "secureConnection", listener: (tlsSocket: TLSSocket) => void): this; on(event: "keylog", listener: (line: Buffer, tlsSocket: TLSSocket) => void): this; once(event: string, listener: (...args: any[]) => void): this; once(event: "tlsClientError", listener: (err: Error, tlsSocket: TLSSocket) => void): this; once(event: "newSession", listener: (sessionId: Buffer, sessionData: Buffer, callback: (err: Error, resp: Buffer) => void) => void): this; once(event: "OCSPRequest", listener: (certificate: Buffer, issuer: Buffer, callback: (err: Error | null, resp: Buffer) => void) => void): this; once(event: "resumeSession", listener: (sessionId: Buffer, callback: (err: Error, sessionData: Buffer) => void) => void): this; once(event: "secureConnection", listener: (tlsSocket: TLSSocket) => void): this; once(event: "keylog", listener: (line: Buffer, tlsSocket: TLSSocket) => void): this; prependListener(event: string, listener: (...args: any[]) => void): this; prependListener(event: "tlsClientError", listener: (err: Error, tlsSocket: TLSSocket) => void): this; prependListener(event: "newSession", listener: (sessionId: Buffer, sessionData: Buffer, callback: (err: Error, resp: Buffer) => void) => void): this; prependListener(event: "OCSPRequest", listener: (certificate: Buffer, issuer: Buffer, callback: (err: Error | null, resp: Buffer) => void) => void): this; prependListener(event: "resumeSession", listener: (sessionId: Buffer, callback: (err: Error, sessionData: Buffer) => void) => void): this; prependListener(event: "secureConnection", listener: (tlsSocket: TLSSocket) => void): this; prependListener(event: "keylog", listener: (line: Buffer, tlsSocket: TLSSocket) => void): this; prependOnceListener(event: string, listener: (...args: any[]) => void): this; prependOnceListener(event: "tlsClientError", listener: (err: Error, tlsSocket: TLSSocket) => void): this; prependOnceListener(event: "newSession", listener: (sessionId: Buffer, sessionData: Buffer, callback: (err: Error, resp: Buffer) => void) => void): this; prependOnceListener(event: "OCSPRequest", listener: (certificate: Buffer, issuer: Buffer, callback: (err: Error | null, resp: Buffer) => void) => void): this; prependOnceListener(event: "resumeSession", listener: (sessionId: Buffer, callback: (err: Error, sessionData: Buffer) => void) => void): this; prependOnceListener(event: "secureConnection", listener: (tlsSocket: TLSSocket) => void): this; prependOnceListener(event: "keylog", listener: (line: Buffer, tlsSocket: TLSSocket) => void): this; } interface SecurePair { encrypted: TLSSocket; cleartext: TLSSocket; } type SecureVersion = 'TLSv1.3' | 'TLSv1.2' | 'TLSv1.1' | 'TLSv1'; interface SecureContextOptions { /** * Optionally override the trusted CA certificates. Default is to trust * the well-known CAs curated by Mozilla. Mozilla's CAs are completely * replaced when CAs are explicitly specified using this option. */ ca?: string | Buffer | Array<string | Buffer>; /** * Cert chains in PEM format. One cert chain should be provided per * private key. Each cert chain should consist of the PEM formatted * certificate for a provided private key, followed by the PEM * formatted intermediate certificates (if any), in order, and not * including the root CA (the root CA must be pre-known to the peer, * see ca). When providing multiple cert chains, they do not have to * be in the same order as their private keys in key. If the * intermediate certificates are not provided, the peer will not be * able to validate the certificate, and the handshake will fail. */ cert?: string | Buffer | Array<string | Buffer>; /** * Colon-separated list of supported signature algorithms. The list * can contain digest algorithms (SHA256, MD5 etc.), public key * algorithms (RSA-PSS, ECDSA etc.), combination of both (e.g * 'RSA+SHA384') or TLS v1.3 scheme names (e.g. rsa_pss_pss_sha512). */ sigalgs?: string; /** * Cipher suite specification, replacing the default. For more * information, see modifying the default cipher suite. Permitted * ciphers can be obtained via tls.getCiphers(). Cipher names must be * uppercased in order for OpenSSL to accept them. */ ciphers?: string; /** * Name of an OpenSSL engine which can provide the client certificate. */ clientCertEngine?: string; /** * PEM formatted CRLs (Certificate Revocation Lists). */ crl?: string | Buffer | Array<string | Buffer>; /** * Diffie Hellman parameters, required for Perfect Forward Secrecy. Use * openssl dhparam to create the parameters. The key length must be * greater than or equal to 1024 bits or else an error will be thrown. * Although 1024 bits is permissible, use 2048 bits or larger for * stronger security. If omitted or invalid, the parameters are * silently discarded and DHE ciphers will not be available. */ dhparam?: string | Buffer; /** * A string describing a named curve or a colon separated list of curve * NIDs or names, for example P-521:P-384:P-256, to use for ECDH key * agreement. Set to auto to select the curve automatically. Use * crypto.getCurves() to obtain a list of available curve names. On * recent releases, openssl ecparam -list_curves will also display the * name and description of each available elliptic curve. Default: * tls.DEFAULT_ECDH_CURVE. */ ecdhCurve?: string; /** * Attempt to use the server's cipher suite preferences instead of the * client's. When true, causes SSL_OP_CIPHER_SERVER_PREFERENCE to be * set in secureOptions */ honorCipherOrder?: boolean; /** * Private keys in PEM format. PEM allows the option of private keys * being encrypted. Encrypted keys will be decrypted with * options.passphrase. Multiple keys using different algorithms can be * provided either as an array of unencrypted key strings or buffers, * or an array of objects in the form {pem: <string|buffer>[, * passphrase: <string>]}. The object form can only occur in an array. * object.passphrase is optional. Encrypted keys will be decrypted with * object.passphrase if provided, or options.passphrase if it is not. */ key?: string | Buffer | Array<Buffer | KeyObject>; /** * Name of an OpenSSL engine to get private key from. Should be used * together with privateKeyIdentifier. */ privateKeyEngine?: string; /** * Identifier of a private key managed by an OpenSSL engine. Should be * used together with privateKeyEngine. Should not be set together with * key, because both options define a private key in different ways. */ privateKeyIdentifier?: string; /** * Optionally set the maximum TLS version to allow. One * of `'TLSv1.3'`, `'TLSv1.2'`, `'TLSv1.1'`, or `'TLSv1'`. Cannot be specified along with the * `secureProtocol` option, use one or the other. * **Default:** `'TLSv1.3'`, unless changed using CLI options. Using * `--tls-max-v1.2` sets the default to `'TLSv1.2'`. Using `--tls-max-v1.3` sets the default to * `'TLSv1.3'`. If multiple of the options are provided, the highest maximum is used. */ maxVersion?: SecureVersion; /** * Optionally set the minimum TLS version to allow. One * of `'TLSv1.3'`, `'TLSv1.2'`, `'TLSv1.1'`, or `'TLSv1'`. Cannot be specified along with the * `secureProtocol` option, use one or the other. It is not recommended to use * less than TLSv1.2, but it may be required for interoperability. * **Default:** `'TLSv1.2'`, unless changed using CLI options. Using * `--tls-v1.0` sets the default to `'TLSv1'`. Using `--tls-v1.1` sets the default to * `'TLSv1.1'`. Using `--tls-min-v1.3` sets the default to * 'TLSv1.3'. If multiple of the options are provided, the lowest minimum is used. */ minVersion?: SecureVersion; /** * Shared passphrase used for a single private key and/or a PFX. */ passphrase?: string; /** * PFX or PKCS12 encoded private key and certificate chain. pfx is an * alternative to providing key and cert individually. PFX is usually * encrypted, if it is, passphrase will be used to decrypt it. Multiple * PFX can be provided either as an array of unencrypted PFX buffers, * or an array of objects in the form {buf: <string|buffer>[, * passphrase: <string>]}. The object form can only occur in an array. * object.passphrase is optional. Encrypted PFX will be decrypted with * object.passphrase if provided, or options.passphrase if it is not. */ pfx?: string | Buffer | Array<string | Buffer | PxfObject>; /** * Optionally affect the OpenSSL protocol behavior, which is not * usually necessary. This should be used carefully if at all! Value is * a numeric bitmask of the SSL_OP_* options from OpenSSL Options */ secureOptions?: number; // Value is a numeric bitmask of the `SSL_OP_*` options /** * Legacy mechanism to select the TLS protocol version to use, it does * not support independent control of the minimum and maximum version, * and does not support limiting the protocol to TLSv1.3. Use * minVersion and maxVersion instead. The possible values are listed as * SSL_METHODS, use the function names as strings. For example, use * 'TLSv1_1_method' to force TLS version 1.1, or 'TLS_method' to allow * any TLS protocol version up to TLSv1.3. It is not recommended to use * TLS versions less than 1.2, but it may be required for * interoperability. Default: none, see minVersion. */ secureProtocol?: string; /** * Opaque identifier used by servers to ensure session state is not * shared between applications. Unused by clients. */ sessionIdContext?: string; /** * 48-bytes of cryptographically strong pseudo-random data. * See Session Resumption for more information. */ ticketKeys?: Buffer; /** * The number of seconds after which a TLS session created by the * server will no longer be resumable. See Session Resumption for more * information. Default: 300. */ sessionTimeout?: number; } interface SecureContext { context: any; } /* * Verifies the certificate `cert` is issued to host `host`. * @host The hostname to verify the certificate against * @cert PeerCertificate representing the peer's certificate * * Returns Error object, populating it with the reason, host and cert on failure. On success, returns undefined. */ function checkServerIdentity(host: string, cert: PeerCertificate): Error | undefined; function createServer(secureConnectionListener?: (socket: TLSSocket) => void): Server; function createServer(options: TlsOptions, secureConnectionListener?: (socket: TLSSocket) => void): Server; function connect(options: ConnectionOptions, secureConnectListener?: () => void): TLSSocket; function connect(port: number, host?: string, options?: ConnectionOptions, secureConnectListener?: () => void): TLSSocket; function connect(port: number, options?: ConnectionOptions, secureConnectListener?: () => void): TLSSocket; /** * @deprecated since v0.11.3 Use `tls.TLSSocket` instead. */ function createSecurePair(credentials?: SecureContext, isServer?: boolean, requestCert?: boolean, rejectUnauthorized?: boolean): SecurePair; function createSecureContext(options?: SecureContextOptions): SecureContext; function getCiphers(): string[]; /** * The default curve name to use for ECDH key agreement in a tls server. * The default value is 'auto'. See tls.createSecureContext() for further * information. */ let DEFAULT_ECDH_CURVE: string; /** * The default value of the maxVersion option of * tls.createSecureContext(). It can be assigned any of the supported TLS * protocol versions, 'TLSv1.3', 'TLSv1.2', 'TLSv1.1', or 'TLSv1'. Default: * 'TLSv1.3', unless changed using CLI options. Using --tls-max-v1.2 sets * the default to 'TLSv1.2'. Using --tls-max-v1.3 sets the default to * 'TLSv1.3'. If multiple of the options are provided, the highest maximum * is used. */ let DEFAULT_MAX_VERSION: SecureVersion; /** * The default value of the minVersion option of tls.createSecureContext(). * It can be assigned any of the supported TLS protocol versions, * 'TLSv1.3', 'TLSv1.2', 'TLSv1.1', or 'TLSv1'. Default: 'TLSv1.2', unless * changed using CLI options. Using --tls-min-v1.0 sets the default to * 'TLSv1'. Using --tls-min-v1.1 sets the default to 'TLSv1.1'. Using * --tls-min-v1.3 sets the default to 'TLSv1.3'. If multiple of the options * are provided, the lowest minimum is used. */ let DEFAULT_MIN_VERSION: SecureVersion; /** * An immutable array of strings representing the root certificates (in PEM * format) used for verifying peer certificates. This is the default value * of the ca option to tls.createSecureContext(). */ const rootCertificates: ReadonlyArray<string>; } apollo-server-demo/node_modules/@types/node/domain.d.ts 0000644 0001750 0000144 00000001315 14000133700 022645 0 ustar andreh users declare module 'domain' { import EventEmitter = require('events'); global { namespace NodeJS { interface Domain extends EventEmitter { run<T>(fn: (...args: any[]) => T, ...args: any[]): T; add(emitter: EventEmitter | Timer): void; remove(emitter: EventEmitter | Timer): void; bind<T extends Function>(cb: T): T; intercept<T extends Function>(cb: T): T; } } } interface Domain extends NodeJS.Domain {} class Domain extends EventEmitter { members: Array<EventEmitter | NodeJS.Timer>; enter(): void; exit(): void; } function create(): Domain; } apollo-server-demo/node_modules/@types/node/dns.d.ts 0000644 0001750 0000144 00000037673 14000133700 022202 0 ustar andreh users declare module "dns" { // Supported getaddrinfo flags. const ADDRCONFIG: number; const V4MAPPED: number; /** * If `dns.V4MAPPED` is specified, return resolved IPv6 addresses as * well as IPv4 mapped IPv6 addresses. */ const ALL: number; interface LookupOptions { family?: number; hints?: number; all?: boolean; verbatim?: boolean; } interface LookupOneOptions extends LookupOptions { all?: false; } interface LookupAllOptions extends LookupOptions { all: true; } interface LookupAddress { address: string; family: number; } function lookup(hostname: string, family: number, callback: (err: NodeJS.ErrnoException | null, address: string, family: number) => void): void; function lookup(hostname: string, options: LookupOneOptions, callback: (err: NodeJS.ErrnoException | null, address: string, family: number) => void): void; function lookup(hostname: string, options: LookupAllOptions, callback: (err: NodeJS.ErrnoException | null, addresses: LookupAddress[]) => void): void; function lookup(hostname: string, options: LookupOptions, callback: (err: NodeJS.ErrnoException | null, address: string | LookupAddress[], family: number) => void): void; function lookup(hostname: string, callback: (err: NodeJS.ErrnoException | null, address: string, family: number) => void): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. namespace lookup { function __promisify__(hostname: string, options: LookupAllOptions): Promise<LookupAddress[]>; function __promisify__(hostname: string, options?: LookupOneOptions | number): Promise<LookupAddress>; function __promisify__(hostname: string, options: LookupOptions): Promise<LookupAddress | LookupAddress[]>; } function lookupService(address: string, port: number, callback: (err: NodeJS.ErrnoException | null, hostname: string, service: string) => void): void; namespace lookupService { function __promisify__(address: string, port: number): Promise<{ hostname: string, service: string }>; } interface ResolveOptions { ttl: boolean; } interface ResolveWithTtlOptions extends ResolveOptions { ttl: true; } interface RecordWithTtl { address: string; ttl: number; } /** @deprecated Use `AnyARecord` or `AnyAaaaRecord` instead. */ type AnyRecordWithTtl = AnyARecord | AnyAaaaRecord; interface AnyARecord extends RecordWithTtl { type: "A"; } interface AnyAaaaRecord extends RecordWithTtl { type: "AAAA"; } interface MxRecord { priority: number; exchange: string; } interface AnyMxRecord extends MxRecord { type: "MX"; } interface NaptrRecord { flags: string; service: string; regexp: string; replacement: string; order: number; preference: number; } interface AnyNaptrRecord extends NaptrRecord { type: "NAPTR"; } interface SoaRecord { nsname: string; hostmaster: string; serial: number; refresh: number; retry: number; expire: number; minttl: number; } interface AnySoaRecord extends SoaRecord { type: "SOA"; } interface SrvRecord { priority: number; weight: number; port: number; name: string; } interface AnySrvRecord extends SrvRecord { type: "SRV"; } interface AnyTxtRecord { type: "TXT"; entries: string[]; } interface AnyNsRecord { type: "NS"; value: string; } interface AnyPtrRecord { type: "PTR"; value: string; } interface AnyCnameRecord { type: "CNAME"; value: string; } type AnyRecord = AnyARecord | AnyAaaaRecord | AnyCnameRecord | AnyMxRecord | AnyNaptrRecord | AnyNsRecord | AnyPtrRecord | AnySoaRecord | AnySrvRecord | AnyTxtRecord; function resolve(hostname: string, callback: (err: NodeJS.ErrnoException | null, addresses: string[]) => void): void; function resolve(hostname: string, rrtype: "A", callback: (err: NodeJS.ErrnoException | null, addresses: string[]) => void): void; function resolve(hostname: string, rrtype: "AAAA", callback: (err: NodeJS.ErrnoException | null, addresses: string[]) => void): void; function resolve(hostname: string, rrtype: "ANY", callback: (err: NodeJS.ErrnoException | null, addresses: AnyRecord[]) => void): void; function resolve(hostname: string, rrtype: "CNAME", callback: (err: NodeJS.ErrnoException | null, addresses: string[]) => void): void; function resolve(hostname: string, rrtype: "MX", callback: (err: NodeJS.ErrnoException | null, addresses: MxRecord[]) => void): void; function resolve(hostname: string, rrtype: "NAPTR", callback: (err: NodeJS.ErrnoException | null, addresses: NaptrRecord[]) => void): void; function resolve(hostname: string, rrtype: "NS", callback: (err: NodeJS.ErrnoException | null, addresses: string[]) => void): void; function resolve(hostname: string, rrtype: "PTR", callback: (err: NodeJS.ErrnoException | null, addresses: string[]) => void): void; function resolve(hostname: string, rrtype: "SOA", callback: (err: NodeJS.ErrnoException | null, addresses: SoaRecord) => void): void; function resolve(hostname: string, rrtype: "SRV", callback: (err: NodeJS.ErrnoException | null, addresses: SrvRecord[]) => void): void; function resolve(hostname: string, rrtype: "TXT", callback: (err: NodeJS.ErrnoException | null, addresses: string[][]) => void): void; function resolve( hostname: string, rrtype: string, callback: (err: NodeJS.ErrnoException | null, addresses: string[] | MxRecord[] | NaptrRecord[] | SoaRecord | SrvRecord[] | string[][] | AnyRecord[]) => void, ): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. namespace resolve { function __promisify__(hostname: string, rrtype?: "A" | "AAAA" | "CNAME" | "NS" | "PTR"): Promise<string[]>; function __promisify__(hostname: string, rrtype: "ANY"): Promise<AnyRecord[]>; function __promisify__(hostname: string, rrtype: "MX"): Promise<MxRecord[]>; function __promisify__(hostname: string, rrtype: "NAPTR"): Promise<NaptrRecord[]>; function __promisify__(hostname: string, rrtype: "SOA"): Promise<SoaRecord>; function __promisify__(hostname: string, rrtype: "SRV"): Promise<SrvRecord[]>; function __promisify__(hostname: string, rrtype: "TXT"): Promise<string[][]>; function __promisify__(hostname: string, rrtype: string): Promise<string[] | MxRecord[] | NaptrRecord[] | SoaRecord | SrvRecord[] | string[][] | AnyRecord[]>; } function resolve4(hostname: string, callback: (err: NodeJS.ErrnoException | null, addresses: string[]) => void): void; function resolve4(hostname: string, options: ResolveWithTtlOptions, callback: (err: NodeJS.ErrnoException | null, addresses: RecordWithTtl[]) => void): void; function resolve4(hostname: string, options: ResolveOptions, callback: (err: NodeJS.ErrnoException | null, addresses: string[] | RecordWithTtl[]) => void): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. namespace resolve4 { function __promisify__(hostname: string): Promise<string[]>; function __promisify__(hostname: string, options: ResolveWithTtlOptions): Promise<RecordWithTtl[]>; function __promisify__(hostname: string, options?: ResolveOptions): Promise<string[] | RecordWithTtl[]>; } function resolve6(hostname: string, callback: (err: NodeJS.ErrnoException | null, addresses: string[]) => void): void; function resolve6(hostname: string, options: ResolveWithTtlOptions, callback: (err: NodeJS.ErrnoException | null, addresses: RecordWithTtl[]) => void): void; function resolve6(hostname: string, options: ResolveOptions, callback: (err: NodeJS.ErrnoException | null, addresses: string[] | RecordWithTtl[]) => void): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. namespace resolve6 { function __promisify__(hostname: string): Promise<string[]>; function __promisify__(hostname: string, options: ResolveWithTtlOptions): Promise<RecordWithTtl[]>; function __promisify__(hostname: string, options?: ResolveOptions): Promise<string[] | RecordWithTtl[]>; } function resolveCname(hostname: string, callback: (err: NodeJS.ErrnoException | null, addresses: string[]) => void): void; namespace resolveCname { function __promisify__(hostname: string): Promise<string[]>; } function resolveMx(hostname: string, callback: (err: NodeJS.ErrnoException | null, addresses: MxRecord[]) => void): void; namespace resolveMx { function __promisify__(hostname: string): Promise<MxRecord[]>; } function resolveNaptr(hostname: string, callback: (err: NodeJS.ErrnoException | null, addresses: NaptrRecord[]) => void): void; namespace resolveNaptr { function __promisify__(hostname: string): Promise<NaptrRecord[]>; } function resolveNs(hostname: string, callback: (err: NodeJS.ErrnoException | null, addresses: string[]) => void): void; namespace resolveNs { function __promisify__(hostname: string): Promise<string[]>; } function resolvePtr(hostname: string, callback: (err: NodeJS.ErrnoException | null, addresses: string[]) => void): void; namespace resolvePtr { function __promisify__(hostname: string): Promise<string[]>; } function resolveSoa(hostname: string, callback: (err: NodeJS.ErrnoException | null, address: SoaRecord) => void): void; namespace resolveSoa { function __promisify__(hostname: string): Promise<SoaRecord>; } function resolveSrv(hostname: string, callback: (err: NodeJS.ErrnoException | null, addresses: SrvRecord[]) => void): void; namespace resolveSrv { function __promisify__(hostname: string): Promise<SrvRecord[]>; } function resolveTxt(hostname: string, callback: (err: NodeJS.ErrnoException | null, addresses: string[][]) => void): void; namespace resolveTxt { function __promisify__(hostname: string): Promise<string[][]>; } function resolveAny(hostname: string, callback: (err: NodeJS.ErrnoException | null, addresses: AnyRecord[]) => void): void; namespace resolveAny { function __promisify__(hostname: string): Promise<AnyRecord[]>; } function reverse(ip: string, callback: (err: NodeJS.ErrnoException | null, hostnames: string[]) => void): void; function setServers(servers: ReadonlyArray<string>): void; function getServers(): string[]; // Error codes const NODATA: string; const FORMERR: string; const SERVFAIL: string; const NOTFOUND: string; const NOTIMP: string; const REFUSED: string; const BADQUERY: string; const BADNAME: string; const BADFAMILY: string; const BADRESP: string; const CONNREFUSED: string; const TIMEOUT: string; const EOF: string; const FILE: string; const NOMEM: string; const DESTRUCTION: string; const BADSTR: string; const BADFLAGS: string; const NONAME: string; const BADHINTS: string; const NOTINITIALIZED: string; const LOADIPHLPAPI: string; const ADDRGETNETWORKPARAMS: string; const CANCELLED: string; class Resolver { cancel(): void; getServers: typeof getServers; resolve: typeof resolve; resolve4: typeof resolve4; resolve6: typeof resolve6; resolveAny: typeof resolveAny; resolveCname: typeof resolveCname; resolveMx: typeof resolveMx; resolveNaptr: typeof resolveNaptr; resolveNs: typeof resolveNs; resolvePtr: typeof resolvePtr; resolveSoa: typeof resolveSoa; resolveSrv: typeof resolveSrv; resolveTxt: typeof resolveTxt; reverse: typeof reverse; setLocalAddress(ipv4?: string, ipv6?: string): void; setServers: typeof setServers; } namespace promises { function getServers(): string[]; function lookup(hostname: string, family: number): Promise<LookupAddress>; function lookup(hostname: string, options: LookupOneOptions): Promise<LookupAddress>; function lookup(hostname: string, options: LookupAllOptions): Promise<LookupAddress[]>; function lookup(hostname: string, options: LookupOptions): Promise<LookupAddress | LookupAddress[]>; function lookup(hostname: string): Promise<LookupAddress>; function lookupService(address: string, port: number): Promise<{ hostname: string, service: string }>; function resolve(hostname: string): Promise<string[]>; function resolve(hostname: string, rrtype: "A"): Promise<string[]>; function resolve(hostname: string, rrtype: "AAAA"): Promise<string[]>; function resolve(hostname: string, rrtype: "ANY"): Promise<AnyRecord[]>; function resolve(hostname: string, rrtype: "CNAME"): Promise<string[]>; function resolve(hostname: string, rrtype: "MX"): Promise<MxRecord[]>; function resolve(hostname: string, rrtype: "NAPTR"): Promise<NaptrRecord[]>; function resolve(hostname: string, rrtype: "NS"): Promise<string[]>; function resolve(hostname: string, rrtype: "PTR"): Promise<string[]>; function resolve(hostname: string, rrtype: "SOA"): Promise<SoaRecord>; function resolve(hostname: string, rrtype: "SRV"): Promise<SrvRecord[]>; function resolve(hostname: string, rrtype: "TXT"): Promise<string[][]>; function resolve(hostname: string, rrtype: string): Promise<string[] | MxRecord[] | NaptrRecord[] | SoaRecord | SrvRecord[] | string[][] | AnyRecord[]>; function resolve4(hostname: string): Promise<string[]>; function resolve4(hostname: string, options: ResolveWithTtlOptions): Promise<RecordWithTtl[]>; function resolve4(hostname: string, options: ResolveOptions): Promise<string[] | RecordWithTtl[]>; function resolve6(hostname: string): Promise<string[]>; function resolve6(hostname: string, options: ResolveWithTtlOptions): Promise<RecordWithTtl[]>; function resolve6(hostname: string, options: ResolveOptions): Promise<string[] | RecordWithTtl[]>; function resolveAny(hostname: string): Promise<AnyRecord[]>; function resolveCname(hostname: string): Promise<string[]>; function resolveMx(hostname: string): Promise<MxRecord[]>; function resolveNaptr(hostname: string): Promise<NaptrRecord[]>; function resolveNs(hostname: string): Promise<string[]>; function resolvePtr(hostname: string): Promise<string[]>; function resolveSoa(hostname: string): Promise<SoaRecord>; function resolveSrv(hostname: string): Promise<SrvRecord[]>; function resolveTxt(hostname: string): Promise<string[][]>; function reverse(ip: string): Promise<string[]>; function setServers(servers: ReadonlyArray<string>): void; class Resolver { cancel(): void; getServers: typeof getServers; resolve: typeof resolve; resolve4: typeof resolve4; resolve6: typeof resolve6; resolveAny: typeof resolveAny; resolveCname: typeof resolveCname; resolveMx: typeof resolveMx; resolveNaptr: typeof resolveNaptr; resolveNs: typeof resolveNs; resolvePtr: typeof resolvePtr; resolveSoa: typeof resolveSoa; resolveSrv: typeof resolveSrv; resolveTxt: typeof resolveTxt; reverse: typeof reverse; setLocalAddress(ipv4?: string, ipv6?: string): void; setServers: typeof setServers; } } } apollo-server-demo/node_modules/@types/node/constants.d.ts 0000644 0001750 0000144 00000000713 14000133700 023413 0 ustar andreh users /** @deprecated since v6.3.0 - use constants property exposed by the relevant module instead. */ declare module "constants" { import { constants as osConstants, SignalConstants } from 'os'; import { constants as cryptoConstants } from 'crypto'; import { constants as fsConstants } from 'fs'; const exp: typeof osConstants.errno & typeof osConstants.priority & SignalConstants & typeof cryptoConstants & typeof fsConstants; export = exp; } apollo-server-demo/node_modules/@types/node/globals.global.d.ts 0000644 0001750 0000144 00000000067 14000133700 024263 0 ustar andreh users declare var global: NodeJS.Global & typeof globalThis; apollo-server-demo/node_modules/@types/node/console.d.ts 0000644 0001750 0000144 00000013443 14000133700 023045 0 ustar andreh users declare module "console" { import { InspectOptions } from 'util'; global { // This needs to be global to avoid TS2403 in case lib.dom.d.ts is present in the same build interface Console { Console: NodeJS.ConsoleConstructor; /** * A simple assertion test that verifies whether `value` is truthy. * If it is not, an `AssertionError` is thrown. * If provided, the error `message` is formatted using `util.format()` and used as the error message. */ assert(value: any, message?: string, ...optionalParams: any[]): void; /** * When `stdout` is a TTY, calling `console.clear()` will attempt to clear the TTY. * When `stdout` is not a TTY, this method does nothing. */ clear(): void; /** * Maintains an internal counter specific to `label` and outputs to `stdout` the number of times `console.count()` has been called with the given `label`. */ count(label?: string): void; /** * Resets the internal counter specific to `label`. */ countReset(label?: string): void; /** * The `console.debug()` function is an alias for {@link console.log()}. */ debug(message?: any, ...optionalParams: any[]): void; /** * Uses {@link util.inspect()} on `obj` and prints the resulting string to `stdout`. * This function bypasses any custom `inspect()` function defined on `obj`. */ dir(obj: any, options?: InspectOptions): void; /** * This method calls {@link console.log()} passing it the arguments received. Please note that this method does not produce any XML formatting */ dirxml(...data: any[]): void; /** * Prints to `stderr` with newline. */ error(message?: any, ...optionalParams: any[]): void; /** * Increases indentation of subsequent lines by two spaces. * If one or more `label`s are provided, those are printed first without the additional indentation. */ group(...label: any[]): void; /** * The `console.groupCollapsed()` function is an alias for {@link console.group()}. */ groupCollapsed(...label: any[]): void; /** * Decreases indentation of subsequent lines by two spaces. */ groupEnd(): void; /** * The {@link console.info()} function is an alias for {@link console.log()}. */ info(message?: any, ...optionalParams: any[]): void; /** * Prints to `stdout` with newline. */ log(message?: any, ...optionalParams: any[]): void; /** * This method does not display anything unless used in the inspector. * Prints to `stdout` the array `array` formatted as a table. */ table(tabularData: any, properties?: ReadonlyArray<string>): void; /** * Starts a timer that can be used to compute the duration of an operation. Timers are identified by a unique `label`. */ time(label?: string): void; /** * Stops a timer that was previously started by calling {@link console.time()} and prints the result to `stdout`. */ timeEnd(label?: string): void; /** * For a timer that was previously started by calling {@link console.time()}, prints the elapsed time and other `data` arguments to `stdout`. */ timeLog(label?: string, ...data: any[]): void; /** * Prints to `stderr` the string 'Trace :', followed by the {@link util.format()} formatted message and stack trace to the current position in the code. */ trace(message?: any, ...optionalParams: any[]): void; /** * The {@link console.warn()} function is an alias for {@link console.error()}. */ warn(message?: any, ...optionalParams: any[]): void; // --- Inspector mode only --- /** * This method does not display anything unless used in the inspector. * Starts a JavaScript CPU profile with an optional label. */ profile(label?: string): void; /** * This method does not display anything unless used in the inspector. * Stops the current JavaScript CPU profiling session if one has been started and prints the report to the Profiles panel of the inspector. */ profileEnd(label?: string): void; /** * This method does not display anything unless used in the inspector. * Adds an event with the label `label` to the Timeline panel of the inspector. */ timeStamp(label?: string): void; } var console: Console; namespace NodeJS { interface ConsoleConstructorOptions { stdout: WritableStream; stderr?: WritableStream; ignoreErrors?: boolean; colorMode?: boolean | 'auto'; inspectOptions?: InspectOptions; } interface ConsoleConstructor { prototype: Console; new(stdout: WritableStream, stderr?: WritableStream, ignoreErrors?: boolean): Console; new(options: ConsoleConstructorOptions): Console; } interface Global { console: typeof console; } } } export = console; } apollo-server-demo/node_modules/@types/node/inspector.d.ts 0000644 0001750 0000144 00000356115 14000133700 023417 0 ustar andreh users // tslint:disable-next-line:dt-header // Type definitions for inspector // These definitions are auto-generated. // Please see https://github.com/DefinitelyTyped/DefinitelyTyped/pull/19330 // for more information. // tslint:disable:max-line-length /** * The inspector module provides an API for interacting with the V8 inspector. */ declare module "inspector" { import { EventEmitter } from 'events'; interface InspectorNotification<T> { method: string; params: T; } namespace Schema { /** * Description of the protocol domain. */ interface Domain { /** * Domain name. */ name: string; /** * Domain version. */ version: string; } interface GetDomainsReturnType { /** * List of supported domains. */ domains: Domain[]; } } namespace Runtime { /** * Unique script identifier. */ type ScriptId = string; /** * Unique object identifier. */ type RemoteObjectId = string; /** * Primitive value which cannot be JSON-stringified. */ type UnserializableValue = string; /** * Mirror object referencing original JavaScript object. */ interface RemoteObject { /** * Object type. */ type: string; /** * Object subtype hint. Specified for <code>object</code> type values only. */ subtype?: string; /** * Object class (constructor) name. Specified for <code>object</code> type values only. */ className?: string; /** * Remote object value in case of primitive values or JSON values (if it was requested). */ value?: any; /** * Primitive value which can not be JSON-stringified does not have <code>value</code>, but gets this property. */ unserializableValue?: UnserializableValue; /** * String representation of the object. */ description?: string; /** * Unique object identifier (for non-primitive values). */ objectId?: RemoteObjectId; /** * Preview containing abbreviated property values. Specified for <code>object</code> type values only. * @experimental */ preview?: ObjectPreview; /** * @experimental */ customPreview?: CustomPreview; } /** * @experimental */ interface CustomPreview { header: string; hasBody: boolean; formatterObjectId: RemoteObjectId; bindRemoteObjectFunctionId: RemoteObjectId; configObjectId?: RemoteObjectId; } /** * Object containing abbreviated remote object value. * @experimental */ interface ObjectPreview { /** * Object type. */ type: string; /** * Object subtype hint. Specified for <code>object</code> type values only. */ subtype?: string; /** * String representation of the object. */ description?: string; /** * True iff some of the properties or entries of the original object did not fit. */ overflow: boolean; /** * List of the properties. */ properties: PropertyPreview[]; /** * List of the entries. Specified for <code>map</code> and <code>set</code> subtype values only. */ entries?: EntryPreview[]; } /** * @experimental */ interface PropertyPreview { /** * Property name. */ name: string; /** * Object type. Accessor means that the property itself is an accessor property. */ type: string; /** * User-friendly property value string. */ value?: string; /** * Nested value preview. */ valuePreview?: ObjectPreview; /** * Object subtype hint. Specified for <code>object</code> type values only. */ subtype?: string; } /** * @experimental */ interface EntryPreview { /** * Preview of the key. Specified for map-like collection entries. */ key?: ObjectPreview; /** * Preview of the value. */ value: ObjectPreview; } /** * Object property descriptor. */ interface PropertyDescriptor { /** * Property name or symbol description. */ name: string; /** * The value associated with the property. */ value?: RemoteObject; /** * True if the value associated with the property may be changed (data descriptors only). */ writable?: boolean; /** * A function which serves as a getter for the property, or <code>undefined</code> if there is no getter (accessor descriptors only). */ get?: RemoteObject; /** * A function which serves as a setter for the property, or <code>undefined</code> if there is no setter (accessor descriptors only). */ set?: RemoteObject; /** * True if the type of this property descriptor may be changed and if the property may be deleted from the corresponding object. */ configurable: boolean; /** * True if this property shows up during enumeration of the properties on the corresponding object. */ enumerable: boolean; /** * True if the result was thrown during the evaluation. */ wasThrown?: boolean; /** * True if the property is owned for the object. */ isOwn?: boolean; /** * Property symbol object, if the property is of the <code>symbol</code> type. */ symbol?: RemoteObject; } /** * Object internal property descriptor. This property isn't normally visible in JavaScript code. */ interface InternalPropertyDescriptor { /** * Conventional property name. */ name: string; /** * The value associated with the property. */ value?: RemoteObject; } /** * Represents function call argument. Either remote object id <code>objectId</code>, primitive <code>value</code>, unserializable primitive value or neither of (for undefined) them should be specified. */ interface CallArgument { /** * Primitive value or serializable javascript object. */ value?: any; /** * Primitive value which can not be JSON-stringified. */ unserializableValue?: UnserializableValue; /** * Remote object handle. */ objectId?: RemoteObjectId; } /** * Id of an execution context. */ type ExecutionContextId = number; /** * Description of an isolated world. */ interface ExecutionContextDescription { /** * Unique id of the execution context. It can be used to specify in which execution context script evaluation should be performed. */ id: ExecutionContextId; /** * Execution context origin. */ origin: string; /** * Human readable name describing given context. */ name: string; /** * Embedder-specific auxiliary data. */ auxData?: {}; } /** * Detailed information about exception (or error) that was thrown during script compilation or execution. */ interface ExceptionDetails { /** * Exception id. */ exceptionId: number; /** * Exception text, which should be used together with exception object when available. */ text: string; /** * Line number of the exception location (0-based). */ lineNumber: number; /** * Column number of the exception location (0-based). */ columnNumber: number; /** * Script ID of the exception location. */ scriptId?: ScriptId; /** * URL of the exception location, to be used when the script was not reported. */ url?: string; /** * JavaScript stack trace if available. */ stackTrace?: StackTrace; /** * Exception object if available. */ exception?: RemoteObject; /** * Identifier of the context where exception happened. */ executionContextId?: ExecutionContextId; } /** * Number of milliseconds since epoch. */ type Timestamp = number; /** * Stack entry for runtime errors and assertions. */ interface CallFrame { /** * JavaScript function name. */ functionName: string; /** * JavaScript script id. */ scriptId: ScriptId; /** * JavaScript script name or url. */ url: string; /** * JavaScript script line number (0-based). */ lineNumber: number; /** * JavaScript script column number (0-based). */ columnNumber: number; } /** * Call frames for assertions or error messages. */ interface StackTrace { /** * String label of this stack trace. For async traces this may be a name of the function that initiated the async call. */ description?: string; /** * JavaScript function name. */ callFrames: CallFrame[]; /** * Asynchronous JavaScript stack trace that preceded this stack, if available. */ parent?: StackTrace; /** * Asynchronous JavaScript stack trace that preceded this stack, if available. * @experimental */ parentId?: StackTraceId; } /** * Unique identifier of current debugger. * @experimental */ type UniqueDebuggerId = string; /** * If <code>debuggerId</code> is set stack trace comes from another debugger and can be resolved there. This allows to track cross-debugger calls. See <code>Runtime.StackTrace</code> and <code>Debugger.paused</code> for usages. * @experimental */ interface StackTraceId { id: string; debuggerId?: UniqueDebuggerId; } interface EvaluateParameterType { /** * Expression to evaluate. */ expression: string; /** * Symbolic group name that can be used to release multiple objects. */ objectGroup?: string; /** * Determines whether Command Line API should be available during the evaluation. */ includeCommandLineAPI?: boolean; /** * In silent mode exceptions thrown during evaluation are not reported and do not pause execution. Overrides <code>setPauseOnException</code> state. */ silent?: boolean; /** * Specifies in which execution context to perform evaluation. If the parameter is omitted the evaluation will be performed in the context of the inspected page. */ contextId?: ExecutionContextId; /** * Whether the result is expected to be a JSON object that should be sent by value. */ returnByValue?: boolean; /** * Whether preview should be generated for the result. * @experimental */ generatePreview?: boolean; /** * Whether execution should be treated as initiated by user in the UI. */ userGesture?: boolean; /** * Whether execution should <code>await</code> for resulting value and return once awaited promise is resolved. */ awaitPromise?: boolean; } interface AwaitPromiseParameterType { /** * Identifier of the promise. */ promiseObjectId: RemoteObjectId; /** * Whether the result is expected to be a JSON object that should be sent by value. */ returnByValue?: boolean; /** * Whether preview should be generated for the result. */ generatePreview?: boolean; } interface CallFunctionOnParameterType { /** * Declaration of the function to call. */ functionDeclaration: string; /** * Identifier of the object to call function on. Either objectId or executionContextId should be specified. */ objectId?: RemoteObjectId; /** * Call arguments. All call arguments must belong to the same JavaScript world as the target object. */ arguments?: CallArgument[]; /** * In silent mode exceptions thrown during evaluation are not reported and do not pause execution. Overrides <code>setPauseOnException</code> state. */ silent?: boolean; /** * Whether the result is expected to be a JSON object which should be sent by value. */ returnByValue?: boolean; /** * Whether preview should be generated for the result. * @experimental */ generatePreview?: boolean; /** * Whether execution should be treated as initiated by user in the UI. */ userGesture?: boolean; /** * Whether execution should <code>await</code> for resulting value and return once awaited promise is resolved. */ awaitPromise?: boolean; /** * Specifies execution context which global object will be used to call function on. Either executionContextId or objectId should be specified. */ executionContextId?: ExecutionContextId; /** * Symbolic group name that can be used to release multiple objects. If objectGroup is not specified and objectId is, objectGroup will be inherited from object. */ objectGroup?: string; } interface GetPropertiesParameterType { /** * Identifier of the object to return properties for. */ objectId: RemoteObjectId; /** * If true, returns properties belonging only to the element itself, not to its prototype chain. */ ownProperties?: boolean; /** * If true, returns accessor properties (with getter/setter) only; internal properties are not returned either. * @experimental */ accessorPropertiesOnly?: boolean; /** * Whether preview should be generated for the results. * @experimental */ generatePreview?: boolean; } interface ReleaseObjectParameterType { /** * Identifier of the object to release. */ objectId: RemoteObjectId; } interface ReleaseObjectGroupParameterType { /** * Symbolic object group name. */ objectGroup: string; } interface SetCustomObjectFormatterEnabledParameterType { enabled: boolean; } interface CompileScriptParameterType { /** * Expression to compile. */ expression: string; /** * Source url to be set for the script. */ sourceURL: string; /** * Specifies whether the compiled script should be persisted. */ persistScript: boolean; /** * Specifies in which execution context to perform script run. If the parameter is omitted the evaluation will be performed in the context of the inspected page. */ executionContextId?: ExecutionContextId; } interface RunScriptParameterType { /** * Id of the script to run. */ scriptId: ScriptId; /** * Specifies in which execution context to perform script run. If the parameter is omitted the evaluation will be performed in the context of the inspected page. */ executionContextId?: ExecutionContextId; /** * Symbolic group name that can be used to release multiple objects. */ objectGroup?: string; /** * In silent mode exceptions thrown during evaluation are not reported and do not pause execution. Overrides <code>setPauseOnException</code> state. */ silent?: boolean; /** * Determines whether Command Line API should be available during the evaluation. */ includeCommandLineAPI?: boolean; /** * Whether the result is expected to be a JSON object which should be sent by value. */ returnByValue?: boolean; /** * Whether preview should be generated for the result. */ generatePreview?: boolean; /** * Whether execution should <code>await</code> for resulting value and return once awaited promise is resolved. */ awaitPromise?: boolean; } interface QueryObjectsParameterType { /** * Identifier of the prototype to return objects for. */ prototypeObjectId: RemoteObjectId; } interface GlobalLexicalScopeNamesParameterType { /** * Specifies in which execution context to lookup global scope variables. */ executionContextId?: ExecutionContextId; } interface EvaluateReturnType { /** * Evaluation result. */ result: RemoteObject; /** * Exception details. */ exceptionDetails?: ExceptionDetails; } interface AwaitPromiseReturnType { /** * Promise result. Will contain rejected value if promise was rejected. */ result: RemoteObject; /** * Exception details if stack strace is available. */ exceptionDetails?: ExceptionDetails; } interface CallFunctionOnReturnType { /** * Call result. */ result: RemoteObject; /** * Exception details. */ exceptionDetails?: ExceptionDetails; } interface GetPropertiesReturnType { /** * Object properties. */ result: PropertyDescriptor[]; /** * Internal object properties (only of the element itself). */ internalProperties?: InternalPropertyDescriptor[]; /** * Exception details. */ exceptionDetails?: ExceptionDetails; } interface CompileScriptReturnType { /** * Id of the script. */ scriptId?: ScriptId; /** * Exception details. */ exceptionDetails?: ExceptionDetails; } interface RunScriptReturnType { /** * Run result. */ result: RemoteObject; /** * Exception details. */ exceptionDetails?: ExceptionDetails; } interface QueryObjectsReturnType { /** * Array with objects. */ objects: RemoteObject; } interface GlobalLexicalScopeNamesReturnType { names: string[]; } interface ExecutionContextCreatedEventDataType { /** * A newly created execution context. */ context: ExecutionContextDescription; } interface ExecutionContextDestroyedEventDataType { /** * Id of the destroyed context */ executionContextId: ExecutionContextId; } interface ExceptionThrownEventDataType { /** * Timestamp of the exception. */ timestamp: Timestamp; exceptionDetails: ExceptionDetails; } interface ExceptionRevokedEventDataType { /** * Reason describing why exception was revoked. */ reason: string; /** * The id of revoked exception, as reported in <code>exceptionThrown</code>. */ exceptionId: number; } interface ConsoleAPICalledEventDataType { /** * Type of the call. */ type: string; /** * Call arguments. */ args: RemoteObject[]; /** * Identifier of the context where the call was made. */ executionContextId: ExecutionContextId; /** * Call timestamp. */ timestamp: Timestamp; /** * Stack trace captured when the call was made. */ stackTrace?: StackTrace; /** * Console context descriptor for calls on non-default console context (not console.*): 'anonymous#unique-logger-id' for call on unnamed context, 'name#unique-logger-id' for call on named context. * @experimental */ context?: string; } interface InspectRequestedEventDataType { object: RemoteObject; hints: {}; } } namespace Debugger { /** * Breakpoint identifier. */ type BreakpointId = string; /** * Call frame identifier. */ type CallFrameId = string; /** * Location in the source code. */ interface Location { /** * Script identifier as reported in the <code>Debugger.scriptParsed</code>. */ scriptId: Runtime.ScriptId; /** * Line number in the script (0-based). */ lineNumber: number; /** * Column number in the script (0-based). */ columnNumber?: number; } /** * Location in the source code. * @experimental */ interface ScriptPosition { lineNumber: number; columnNumber: number; } /** * JavaScript call frame. Array of call frames form the call stack. */ interface CallFrame { /** * Call frame identifier. This identifier is only valid while the virtual machine is paused. */ callFrameId: CallFrameId; /** * Name of the JavaScript function called on this call frame. */ functionName: string; /** * Location in the source code. */ functionLocation?: Location; /** * Location in the source code. */ location: Location; /** * JavaScript script name or url. */ url: string; /** * Scope chain for this call frame. */ scopeChain: Scope[]; /** * <code>this</code> object for this call frame. */ this: Runtime.RemoteObject; /** * The value being returned, if the function is at return point. */ returnValue?: Runtime.RemoteObject; } /** * Scope description. */ interface Scope { /** * Scope type. */ type: string; /** * Object representing the scope. For <code>global</code> and <code>with</code> scopes it represents the actual object; for the rest of the scopes, it is artificial transient object enumerating scope variables as its properties. */ object: Runtime.RemoteObject; name?: string; /** * Location in the source code where scope starts */ startLocation?: Location; /** * Location in the source code where scope ends */ endLocation?: Location; } /** * Search match for resource. */ interface SearchMatch { /** * Line number in resource content. */ lineNumber: number; /** * Line with match content. */ lineContent: string; } interface BreakLocation { /** * Script identifier as reported in the <code>Debugger.scriptParsed</code>. */ scriptId: Runtime.ScriptId; /** * Line number in the script (0-based). */ lineNumber: number; /** * Column number in the script (0-based). */ columnNumber?: number; type?: string; } interface SetBreakpointsActiveParameterType { /** * New value for breakpoints active state. */ active: boolean; } interface SetSkipAllPausesParameterType { /** * New value for skip pauses state. */ skip: boolean; } interface SetBreakpointByUrlParameterType { /** * Line number to set breakpoint at. */ lineNumber: number; /** * URL of the resources to set breakpoint on. */ url?: string; /** * Regex pattern for the URLs of the resources to set breakpoints on. Either <code>url</code> or <code>urlRegex</code> must be specified. */ urlRegex?: string; /** * Script hash of the resources to set breakpoint on. */ scriptHash?: string; /** * Offset in the line to set breakpoint at. */ columnNumber?: number; /** * Expression to use as a breakpoint condition. When specified, debugger will only stop on the breakpoint if this expression evaluates to true. */ condition?: string; } interface SetBreakpointParameterType { /** * Location to set breakpoint in. */ location: Location; /** * Expression to use as a breakpoint condition. When specified, debugger will only stop on the breakpoint if this expression evaluates to true. */ condition?: string; } interface RemoveBreakpointParameterType { breakpointId: BreakpointId; } interface GetPossibleBreakpointsParameterType { /** * Start of range to search possible breakpoint locations in. */ start: Location; /** * End of range to search possible breakpoint locations in (excluding). When not specified, end of scripts is used as end of range. */ end?: Location; /** * Only consider locations which are in the same (non-nested) function as start. */ restrictToFunction?: boolean; } interface ContinueToLocationParameterType { /** * Location to continue to. */ location: Location; targetCallFrames?: string; } interface PauseOnAsyncCallParameterType { /** * Debugger will pause when async call with given stack trace is started. */ parentStackTraceId: Runtime.StackTraceId; } interface StepIntoParameterType { /** * Debugger will issue additional Debugger.paused notification if any async task is scheduled before next pause. * @experimental */ breakOnAsyncCall?: boolean; } interface GetStackTraceParameterType { stackTraceId: Runtime.StackTraceId; } interface SearchInContentParameterType { /** * Id of the script to search in. */ scriptId: Runtime.ScriptId; /** * String to search for. */ query: string; /** * If true, search is case sensitive. */ caseSensitive?: boolean; /** * If true, treats string parameter as regex. */ isRegex?: boolean; } interface SetScriptSourceParameterType { /** * Id of the script to edit. */ scriptId: Runtime.ScriptId; /** * New content of the script. */ scriptSource: string; /** * If true the change will not actually be applied. Dry run may be used to get result description without actually modifying the code. */ dryRun?: boolean; } interface RestartFrameParameterType { /** * Call frame identifier to evaluate on. */ callFrameId: CallFrameId; } interface GetScriptSourceParameterType { /** * Id of the script to get source for. */ scriptId: Runtime.ScriptId; } interface SetPauseOnExceptionsParameterType { /** * Pause on exceptions mode. */ state: string; } interface EvaluateOnCallFrameParameterType { /** * Call frame identifier to evaluate on. */ callFrameId: CallFrameId; /** * Expression to evaluate. */ expression: string; /** * String object group name to put result into (allows rapid releasing resulting object handles using <code>releaseObjectGroup</code>). */ objectGroup?: string; /** * Specifies whether command line API should be available to the evaluated expression, defaults to false. */ includeCommandLineAPI?: boolean; /** * In silent mode exceptions thrown during evaluation are not reported and do not pause execution. Overrides <code>setPauseOnException</code> state. */ silent?: boolean; /** * Whether the result is expected to be a JSON object that should be sent by value. */ returnByValue?: boolean; /** * Whether preview should be generated for the result. * @experimental */ generatePreview?: boolean; /** * Whether to throw an exception if side effect cannot be ruled out during evaluation. */ throwOnSideEffect?: boolean; } interface SetVariableValueParameterType { /** * 0-based number of scope as was listed in scope chain. Only 'local', 'closure' and 'catch' scope types are allowed. Other scopes could be manipulated manually. */ scopeNumber: number; /** * Variable name. */ variableName: string; /** * New variable value. */ newValue: Runtime.CallArgument; /** * Id of callframe that holds variable. */ callFrameId: CallFrameId; } interface SetReturnValueParameterType { /** * New return value. */ newValue: Runtime.CallArgument; } interface SetAsyncCallStackDepthParameterType { /** * Maximum depth of async call stacks. Setting to <code>0</code> will effectively disable collecting async call stacks (default). */ maxDepth: number; } interface SetBlackboxPatternsParameterType { /** * Array of regexps that will be used to check script url for blackbox state. */ patterns: string[]; } interface SetBlackboxedRangesParameterType { /** * Id of the script. */ scriptId: Runtime.ScriptId; positions: ScriptPosition[]; } interface EnableReturnType { /** * Unique identifier of the debugger. * @experimental */ debuggerId: Runtime.UniqueDebuggerId; } interface SetBreakpointByUrlReturnType { /** * Id of the created breakpoint for further reference. */ breakpointId: BreakpointId; /** * List of the locations this breakpoint resolved into upon addition. */ locations: Location[]; } interface SetBreakpointReturnType { /** * Id of the created breakpoint for further reference. */ breakpointId: BreakpointId; /** * Location this breakpoint resolved into. */ actualLocation: Location; } interface GetPossibleBreakpointsReturnType { /** * List of the possible breakpoint locations. */ locations: BreakLocation[]; } interface GetStackTraceReturnType { stackTrace: Runtime.StackTrace; } interface SearchInContentReturnType { /** * List of search matches. */ result: SearchMatch[]; } interface SetScriptSourceReturnType { /** * New stack trace in case editing has happened while VM was stopped. */ callFrames?: CallFrame[]; /** * Whether current call stack was modified after applying the changes. */ stackChanged?: boolean; /** * Async stack trace, if any. */ asyncStackTrace?: Runtime.StackTrace; /** * Async stack trace, if any. * @experimental */ asyncStackTraceId?: Runtime.StackTraceId; /** * Exception details if any. */ exceptionDetails?: Runtime.ExceptionDetails; } interface RestartFrameReturnType { /** * New stack trace. */ callFrames: CallFrame[]; /** * Async stack trace, if any. */ asyncStackTrace?: Runtime.StackTrace; /** * Async stack trace, if any. * @experimental */ asyncStackTraceId?: Runtime.StackTraceId; } interface GetScriptSourceReturnType { /** * Script source. */ scriptSource: string; } interface EvaluateOnCallFrameReturnType { /** * Object wrapper for the evaluation result. */ result: Runtime.RemoteObject; /** * Exception details. */ exceptionDetails?: Runtime.ExceptionDetails; } interface ScriptParsedEventDataType { /** * Identifier of the script parsed. */ scriptId: Runtime.ScriptId; /** * URL or name of the script parsed (if any). */ url: string; /** * Line offset of the script within the resource with given URL (for script tags). */ startLine: number; /** * Column offset of the script within the resource with given URL. */ startColumn: number; /** * Last line of the script. */ endLine: number; /** * Length of the last line of the script. */ endColumn: number; /** * Specifies script creation context. */ executionContextId: Runtime.ExecutionContextId; /** * Content hash of the script. */ hash: string; /** * Embedder-specific auxiliary data. */ executionContextAuxData?: {}; /** * True, if this script is generated as a result of the live edit operation. * @experimental */ isLiveEdit?: boolean; /** * URL of source map associated with script (if any). */ sourceMapURL?: string; /** * True, if this script has sourceURL. */ hasSourceURL?: boolean; /** * True, if this script is ES6 module. */ isModule?: boolean; /** * This script length. */ length?: number; /** * JavaScript top stack frame of where the script parsed event was triggered if available. * @experimental */ stackTrace?: Runtime.StackTrace; } interface ScriptFailedToParseEventDataType { /** * Identifier of the script parsed. */ scriptId: Runtime.ScriptId; /** * URL or name of the script parsed (if any). */ url: string; /** * Line offset of the script within the resource with given URL (for script tags). */ startLine: number; /** * Column offset of the script within the resource with given URL. */ startColumn: number; /** * Last line of the script. */ endLine: number; /** * Length of the last line of the script. */ endColumn: number; /** * Specifies script creation context. */ executionContextId: Runtime.ExecutionContextId; /** * Content hash of the script. */ hash: string; /** * Embedder-specific auxiliary data. */ executionContextAuxData?: {}; /** * URL of source map associated with script (if any). */ sourceMapURL?: string; /** * True, if this script has sourceURL. */ hasSourceURL?: boolean; /** * True, if this script is ES6 module. */ isModule?: boolean; /** * This script length. */ length?: number; /** * JavaScript top stack frame of where the script parsed event was triggered if available. * @experimental */ stackTrace?: Runtime.StackTrace; } interface BreakpointResolvedEventDataType { /** * Breakpoint unique identifier. */ breakpointId: BreakpointId; /** * Actual breakpoint location. */ location: Location; } interface PausedEventDataType { /** * Call stack the virtual machine stopped on. */ callFrames: CallFrame[]; /** * Pause reason. */ reason: string; /** * Object containing break-specific auxiliary properties. */ data?: {}; /** * Hit breakpoints IDs */ hitBreakpoints?: string[]; /** * Async stack trace, if any. */ asyncStackTrace?: Runtime.StackTrace; /** * Async stack trace, if any. * @experimental */ asyncStackTraceId?: Runtime.StackTraceId; /** * Just scheduled async call will have this stack trace as parent stack during async execution. This field is available only after <code>Debugger.stepInto</code> call with <code>breakOnAsynCall</code> flag. * @experimental */ asyncCallStackTraceId?: Runtime.StackTraceId; } } namespace Console { /** * Console message. */ interface ConsoleMessage { /** * Message source. */ source: string; /** * Message severity. */ level: string; /** * Message text. */ text: string; /** * URL of the message origin. */ url?: string; /** * Line number in the resource that generated this message (1-based). */ line?: number; /** * Column number in the resource that generated this message (1-based). */ column?: number; } interface MessageAddedEventDataType { /** * Console message that has been added. */ message: ConsoleMessage; } } namespace Profiler { /** * Profile node. Holds callsite information, execution statistics and child nodes. */ interface ProfileNode { /** * Unique id of the node. */ id: number; /** * Function location. */ callFrame: Runtime.CallFrame; /** * Number of samples where this node was on top of the call stack. */ hitCount?: number; /** * Child node ids. */ children?: number[]; /** * The reason of being not optimized. The function may be deoptimized or marked as don't optimize. */ deoptReason?: string; /** * An array of source position ticks. */ positionTicks?: PositionTickInfo[]; } /** * Profile. */ interface Profile { /** * The list of profile nodes. First item is the root node. */ nodes: ProfileNode[]; /** * Profiling start timestamp in microseconds. */ startTime: number; /** * Profiling end timestamp in microseconds. */ endTime: number; /** * Ids of samples top nodes. */ samples?: number[]; /** * Time intervals between adjacent samples in microseconds. The first delta is relative to the profile startTime. */ timeDeltas?: number[]; } /** * Specifies a number of samples attributed to a certain source position. */ interface PositionTickInfo { /** * Source line number (1-based). */ line: number; /** * Number of samples attributed to the source line. */ ticks: number; } /** * Coverage data for a source range. */ interface CoverageRange { /** * JavaScript script source offset for the range start. */ startOffset: number; /** * JavaScript script source offset for the range end. */ endOffset: number; /** * Collected execution count of the source range. */ count: number; } /** * Coverage data for a JavaScript function. */ interface FunctionCoverage { /** * JavaScript function name. */ functionName: string; /** * Source ranges inside the function with coverage data. */ ranges: CoverageRange[]; /** * Whether coverage data for this function has block granularity. */ isBlockCoverage: boolean; } /** * Coverage data for a JavaScript script. */ interface ScriptCoverage { /** * JavaScript script id. */ scriptId: Runtime.ScriptId; /** * JavaScript script name or url. */ url: string; /** * Functions contained in the script that has coverage data. */ functions: FunctionCoverage[]; } /** * Describes a type collected during runtime. * @experimental */ interface TypeObject { /** * Name of a type collected with type profiling. */ name: string; } /** * Source offset and types for a parameter or return value. * @experimental */ interface TypeProfileEntry { /** * Source offset of the parameter or end of function for return values. */ offset: number; /** * The types for this parameter or return value. */ types: TypeObject[]; } /** * Type profile data collected during runtime for a JavaScript script. * @experimental */ interface ScriptTypeProfile { /** * JavaScript script id. */ scriptId: Runtime.ScriptId; /** * JavaScript script name or url. */ url: string; /** * Type profile entries for parameters and return values of the functions in the script. */ entries: TypeProfileEntry[]; } interface SetSamplingIntervalParameterType { /** * New sampling interval in microseconds. */ interval: number; } interface StartPreciseCoverageParameterType { /** * Collect accurate call counts beyond simple 'covered' or 'not covered'. */ callCount?: boolean; /** * Collect block-based coverage. */ detailed?: boolean; } interface StopReturnType { /** * Recorded profile. */ profile: Profile; } interface TakePreciseCoverageReturnType { /** * Coverage data for the current isolate. */ result: ScriptCoverage[]; } interface GetBestEffortCoverageReturnType { /** * Coverage data for the current isolate. */ result: ScriptCoverage[]; } interface TakeTypeProfileReturnType { /** * Type profile for all scripts since startTypeProfile() was turned on. */ result: ScriptTypeProfile[]; } interface ConsoleProfileStartedEventDataType { id: string; /** * Location of console.profile(). */ location: Debugger.Location; /** * Profile title passed as an argument to console.profile(). */ title?: string; } interface ConsoleProfileFinishedEventDataType { id: string; /** * Location of console.profileEnd(). */ location: Debugger.Location; profile: Profile; /** * Profile title passed as an argument to console.profile(). */ title?: string; } } namespace HeapProfiler { /** * Heap snapshot object id. */ type HeapSnapshotObjectId = string; /** * Sampling Heap Profile node. Holds callsite information, allocation statistics and child nodes. */ interface SamplingHeapProfileNode { /** * Function location. */ callFrame: Runtime.CallFrame; /** * Allocations size in bytes for the node excluding children. */ selfSize: number; /** * Child nodes. */ children: SamplingHeapProfileNode[]; } /** * Profile. */ interface SamplingHeapProfile { head: SamplingHeapProfileNode; } interface StartTrackingHeapObjectsParameterType { trackAllocations?: boolean; } interface StopTrackingHeapObjectsParameterType { /** * If true 'reportHeapSnapshotProgress' events will be generated while snapshot is being taken when the tracking is stopped. */ reportProgress?: boolean; } interface TakeHeapSnapshotParameterType { /** * If true 'reportHeapSnapshotProgress' events will be generated while snapshot is being taken. */ reportProgress?: boolean; } interface GetObjectByHeapObjectIdParameterType { objectId: HeapSnapshotObjectId; /** * Symbolic group name that can be used to release multiple objects. */ objectGroup?: string; } interface AddInspectedHeapObjectParameterType { /** * Heap snapshot object id to be accessible by means of $x command line API. */ heapObjectId: HeapSnapshotObjectId; } interface GetHeapObjectIdParameterType { /** * Identifier of the object to get heap object id for. */ objectId: Runtime.RemoteObjectId; } interface StartSamplingParameterType { /** * Average sample interval in bytes. Poisson distribution is used for the intervals. The default value is 32768 bytes. */ samplingInterval?: number; } interface GetObjectByHeapObjectIdReturnType { /** * Evaluation result. */ result: Runtime.RemoteObject; } interface GetHeapObjectIdReturnType { /** * Id of the heap snapshot object corresponding to the passed remote object id. */ heapSnapshotObjectId: HeapSnapshotObjectId; } interface StopSamplingReturnType { /** * Recorded sampling heap profile. */ profile: SamplingHeapProfile; } interface GetSamplingProfileReturnType { /** * Return the sampling profile being collected. */ profile: SamplingHeapProfile; } interface AddHeapSnapshotChunkEventDataType { chunk: string; } interface ReportHeapSnapshotProgressEventDataType { done: number; total: number; finished?: boolean; } interface LastSeenObjectIdEventDataType { lastSeenObjectId: number; timestamp: number; } interface HeapStatsUpdateEventDataType { /** * An array of triplets. Each triplet describes a fragment. The first integer is the fragment index, the second integer is a total count of objects for the fragment, the third integer is a total size of the objects for the fragment. */ statsUpdate: number[]; } } namespace NodeTracing { interface TraceConfig { /** * Controls how the trace buffer stores data. */ recordMode?: string; /** * Included category filters. */ includedCategories: string[]; } interface StartParameterType { traceConfig: TraceConfig; } interface GetCategoriesReturnType { /** * A list of supported tracing categories. */ categories: string[]; } interface DataCollectedEventDataType { value: Array<{}>; } } namespace NodeWorker { type WorkerID = string; /** * Unique identifier of attached debugging session. */ type SessionID = string; interface WorkerInfo { workerId: WorkerID; type: string; title: string; url: string; } interface SendMessageToWorkerParameterType { message: string; /** * Identifier of the session. */ sessionId: SessionID; } interface EnableParameterType { /** * Whether to new workers should be paused until the frontend sends `Runtime.runIfWaitingForDebugger` * message to run them. */ waitForDebuggerOnStart: boolean; } interface DetachParameterType { sessionId: SessionID; } interface AttachedToWorkerEventDataType { /** * Identifier assigned to the session used to send/receive messages. */ sessionId: SessionID; workerInfo: WorkerInfo; waitingForDebugger: boolean; } interface DetachedFromWorkerEventDataType { /** * Detached session identifier. */ sessionId: SessionID; } interface ReceivedMessageFromWorkerEventDataType { /** * Identifier of a session which sends a message. */ sessionId: SessionID; message: string; } } namespace NodeRuntime { interface NotifyWhenWaitingForDisconnectParameterType { enabled: boolean; } } /** * The inspector.Session is used for dispatching messages to the V8 inspector back-end and receiving message responses and notifications. */ class Session extends EventEmitter { /** * Create a new instance of the inspector.Session class. * The inspector session needs to be connected through session.connect() before the messages can be dispatched to the inspector backend. */ constructor(); /** * Connects a session to the inspector back-end. * An exception will be thrown if there is already a connected session established either * through the API or by a front-end connected to the Inspector WebSocket port. */ connect(): void; /** * Immediately close the session. All pending message callbacks will be called with an error. * session.connect() will need to be called to be able to send messages again. * Reconnected session will lose all inspector state, such as enabled agents or configured breakpoints. */ disconnect(): void; /** * Posts a message to the inspector back-end. callback will be notified when a response is received. * callback is a function that accepts two optional arguments - error and message-specific result. */ post(method: string, params?: {}, callback?: (err: Error | null, params?: {}) => void): void; post(method: string, callback?: (err: Error | null, params?: {}) => void): void; /** * Returns supported domains. */ post(method: "Schema.getDomains", callback?: (err: Error | null, params: Schema.GetDomainsReturnType) => void): void; /** * Evaluates expression on global object. */ post(method: "Runtime.evaluate", params?: Runtime.EvaluateParameterType, callback?: (err: Error | null, params: Runtime.EvaluateReturnType) => void): void; post(method: "Runtime.evaluate", callback?: (err: Error | null, params: Runtime.EvaluateReturnType) => void): void; /** * Add handler to promise with given promise object id. */ post(method: "Runtime.awaitPromise", params?: Runtime.AwaitPromiseParameterType, callback?: (err: Error | null, params: Runtime.AwaitPromiseReturnType) => void): void; post(method: "Runtime.awaitPromise", callback?: (err: Error | null, params: Runtime.AwaitPromiseReturnType) => void): void; /** * Calls function with given declaration on the given object. Object group of the result is inherited from the target object. */ post(method: "Runtime.callFunctionOn", params?: Runtime.CallFunctionOnParameterType, callback?: (err: Error | null, params: Runtime.CallFunctionOnReturnType) => void): void; post(method: "Runtime.callFunctionOn", callback?: (err: Error | null, params: Runtime.CallFunctionOnReturnType) => void): void; /** * Returns properties of a given object. Object group of the result is inherited from the target object. */ post(method: "Runtime.getProperties", params?: Runtime.GetPropertiesParameterType, callback?: (err: Error | null, params: Runtime.GetPropertiesReturnType) => void): void; post(method: "Runtime.getProperties", callback?: (err: Error | null, params: Runtime.GetPropertiesReturnType) => void): void; /** * Releases remote object with given id. */ post(method: "Runtime.releaseObject", params?: Runtime.ReleaseObjectParameterType, callback?: (err: Error | null) => void): void; post(method: "Runtime.releaseObject", callback?: (err: Error | null) => void): void; /** * Releases all remote objects that belong to a given group. */ post(method: "Runtime.releaseObjectGroup", params?: Runtime.ReleaseObjectGroupParameterType, callback?: (err: Error | null) => void): void; post(method: "Runtime.releaseObjectGroup", callback?: (err: Error | null) => void): void; /** * Tells inspected instance to run if it was waiting for debugger to attach. */ post(method: "Runtime.runIfWaitingForDebugger", callback?: (err: Error | null) => void): void; /** * Enables reporting of execution contexts creation by means of <code>executionContextCreated</code> event. When the reporting gets enabled the event will be sent immediately for each existing execution context. */ post(method: "Runtime.enable", callback?: (err: Error | null) => void): void; /** * Disables reporting of execution contexts creation. */ post(method: "Runtime.disable", callback?: (err: Error | null) => void): void; /** * Discards collected exceptions and console API calls. */ post(method: "Runtime.discardConsoleEntries", callback?: (err: Error | null) => void): void; /** * @experimental */ post(method: "Runtime.setCustomObjectFormatterEnabled", params?: Runtime.SetCustomObjectFormatterEnabledParameterType, callback?: (err: Error | null) => void): void; post(method: "Runtime.setCustomObjectFormatterEnabled", callback?: (err: Error | null) => void): void; /** * Compiles expression. */ post(method: "Runtime.compileScript", params?: Runtime.CompileScriptParameterType, callback?: (err: Error | null, params: Runtime.CompileScriptReturnType) => void): void; post(method: "Runtime.compileScript", callback?: (err: Error | null, params: Runtime.CompileScriptReturnType) => void): void; /** * Runs script with given id in a given context. */ post(method: "Runtime.runScript", params?: Runtime.RunScriptParameterType, callback?: (err: Error | null, params: Runtime.RunScriptReturnType) => void): void; post(method: "Runtime.runScript", callback?: (err: Error | null, params: Runtime.RunScriptReturnType) => void): void; post(method: "Runtime.queryObjects", params?: Runtime.QueryObjectsParameterType, callback?: (err: Error | null, params: Runtime.QueryObjectsReturnType) => void): void; post(method: "Runtime.queryObjects", callback?: (err: Error | null, params: Runtime.QueryObjectsReturnType) => void): void; /** * Returns all let, const and class variables from global scope. */ post( method: "Runtime.globalLexicalScopeNames", params?: Runtime.GlobalLexicalScopeNamesParameterType, callback?: (err: Error | null, params: Runtime.GlobalLexicalScopeNamesReturnType) => void ): void; post(method: "Runtime.globalLexicalScopeNames", callback?: (err: Error | null, params: Runtime.GlobalLexicalScopeNamesReturnType) => void): void; /** * Enables debugger for the given page. Clients should not assume that the debugging has been enabled until the result for this command is received. */ post(method: "Debugger.enable", callback?: (err: Error | null, params: Debugger.EnableReturnType) => void): void; /** * Disables debugger for given page. */ post(method: "Debugger.disable", callback?: (err: Error | null) => void): void; /** * Activates / deactivates all breakpoints on the page. */ post(method: "Debugger.setBreakpointsActive", params?: Debugger.SetBreakpointsActiveParameterType, callback?: (err: Error | null) => void): void; post(method: "Debugger.setBreakpointsActive", callback?: (err: Error | null) => void): void; /** * Makes page not interrupt on any pauses (breakpoint, exception, dom exception etc). */ post(method: "Debugger.setSkipAllPauses", params?: Debugger.SetSkipAllPausesParameterType, callback?: (err: Error | null) => void): void; post(method: "Debugger.setSkipAllPauses", callback?: (err: Error | null) => void): void; /** * Sets JavaScript breakpoint at given location specified either by URL or URL regex. Once this command is issued, all existing parsed scripts will have breakpoints resolved and returned in <code>locations</code> property. Further matching script parsing will result in subsequent <code>breakpointResolved</code> events issued. This logical breakpoint will survive page reloads. */ post(method: "Debugger.setBreakpointByUrl", params?: Debugger.SetBreakpointByUrlParameterType, callback?: (err: Error | null, params: Debugger.SetBreakpointByUrlReturnType) => void): void; post(method: "Debugger.setBreakpointByUrl", callback?: (err: Error | null, params: Debugger.SetBreakpointByUrlReturnType) => void): void; /** * Sets JavaScript breakpoint at a given location. */ post(method: "Debugger.setBreakpoint", params?: Debugger.SetBreakpointParameterType, callback?: (err: Error | null, params: Debugger.SetBreakpointReturnType) => void): void; post(method: "Debugger.setBreakpoint", callback?: (err: Error | null, params: Debugger.SetBreakpointReturnType) => void): void; /** * Removes JavaScript breakpoint. */ post(method: "Debugger.removeBreakpoint", params?: Debugger.RemoveBreakpointParameterType, callback?: (err: Error | null) => void): void; post(method: "Debugger.removeBreakpoint", callback?: (err: Error | null) => void): void; /** * Returns possible locations for breakpoint. scriptId in start and end range locations should be the same. */ post( method: "Debugger.getPossibleBreakpoints", params?: Debugger.GetPossibleBreakpointsParameterType, callback?: (err: Error | null, params: Debugger.GetPossibleBreakpointsReturnType) => void ): void; post(method: "Debugger.getPossibleBreakpoints", callback?: (err: Error | null, params: Debugger.GetPossibleBreakpointsReturnType) => void): void; /** * Continues execution until specific location is reached. */ post(method: "Debugger.continueToLocation", params?: Debugger.ContinueToLocationParameterType, callback?: (err: Error | null) => void): void; post(method: "Debugger.continueToLocation", callback?: (err: Error | null) => void): void; /** * @experimental */ post(method: "Debugger.pauseOnAsyncCall", params?: Debugger.PauseOnAsyncCallParameterType, callback?: (err: Error | null) => void): void; post(method: "Debugger.pauseOnAsyncCall", callback?: (err: Error | null) => void): void; /** * Steps over the statement. */ post(method: "Debugger.stepOver", callback?: (err: Error | null) => void): void; /** * Steps into the function call. */ post(method: "Debugger.stepInto", params?: Debugger.StepIntoParameterType, callback?: (err: Error | null) => void): void; post(method: "Debugger.stepInto", callback?: (err: Error | null) => void): void; /** * Steps out of the function call. */ post(method: "Debugger.stepOut", callback?: (err: Error | null) => void): void; /** * Stops on the next JavaScript statement. */ post(method: "Debugger.pause", callback?: (err: Error | null) => void): void; /** * This method is deprecated - use Debugger.stepInto with breakOnAsyncCall and Debugger.pauseOnAsyncTask instead. Steps into next scheduled async task if any is scheduled before next pause. Returns success when async task is actually scheduled, returns error if no task were scheduled or another scheduleStepIntoAsync was called. * @experimental */ post(method: "Debugger.scheduleStepIntoAsync", callback?: (err: Error | null) => void): void; /** * Resumes JavaScript execution. */ post(method: "Debugger.resume", callback?: (err: Error | null) => void): void; /** * Returns stack trace with given <code>stackTraceId</code>. * @experimental */ post(method: "Debugger.getStackTrace", params?: Debugger.GetStackTraceParameterType, callback?: (err: Error | null, params: Debugger.GetStackTraceReturnType) => void): void; post(method: "Debugger.getStackTrace", callback?: (err: Error | null, params: Debugger.GetStackTraceReturnType) => void): void; /** * Searches for given string in script content. */ post(method: "Debugger.searchInContent", params?: Debugger.SearchInContentParameterType, callback?: (err: Error | null, params: Debugger.SearchInContentReturnType) => void): void; post(method: "Debugger.searchInContent", callback?: (err: Error | null, params: Debugger.SearchInContentReturnType) => void): void; /** * Edits JavaScript source live. */ post(method: "Debugger.setScriptSource", params?: Debugger.SetScriptSourceParameterType, callback?: (err: Error | null, params: Debugger.SetScriptSourceReturnType) => void): void; post(method: "Debugger.setScriptSource", callback?: (err: Error | null, params: Debugger.SetScriptSourceReturnType) => void): void; /** * Restarts particular call frame from the beginning. */ post(method: "Debugger.restartFrame", params?: Debugger.RestartFrameParameterType, callback?: (err: Error | null, params: Debugger.RestartFrameReturnType) => void): void; post(method: "Debugger.restartFrame", callback?: (err: Error | null, params: Debugger.RestartFrameReturnType) => void): void; /** * Returns source for the script with given id. */ post(method: "Debugger.getScriptSource", params?: Debugger.GetScriptSourceParameterType, callback?: (err: Error | null, params: Debugger.GetScriptSourceReturnType) => void): void; post(method: "Debugger.getScriptSource", callback?: (err: Error | null, params: Debugger.GetScriptSourceReturnType) => void): void; /** * Defines pause on exceptions state. Can be set to stop on all exceptions, uncaught exceptions or no exceptions. Initial pause on exceptions state is <code>none</code>. */ post(method: "Debugger.setPauseOnExceptions", params?: Debugger.SetPauseOnExceptionsParameterType, callback?: (err: Error | null) => void): void; post(method: "Debugger.setPauseOnExceptions", callback?: (err: Error | null) => void): void; /** * Evaluates expression on a given call frame. */ post(method: "Debugger.evaluateOnCallFrame", params?: Debugger.EvaluateOnCallFrameParameterType, callback?: (err: Error | null, params: Debugger.EvaluateOnCallFrameReturnType) => void): void; post(method: "Debugger.evaluateOnCallFrame", callback?: (err: Error | null, params: Debugger.EvaluateOnCallFrameReturnType) => void): void; /** * Changes value of variable in a callframe. Object-based scopes are not supported and must be mutated manually. */ post(method: "Debugger.setVariableValue", params?: Debugger.SetVariableValueParameterType, callback?: (err: Error | null) => void): void; post(method: "Debugger.setVariableValue", callback?: (err: Error | null) => void): void; /** * Changes return value in top frame. Available only at return break position. * @experimental */ post(method: "Debugger.setReturnValue", params?: Debugger.SetReturnValueParameterType, callback?: (err: Error | null) => void): void; post(method: "Debugger.setReturnValue", callback?: (err: Error | null) => void): void; /** * Enables or disables async call stacks tracking. */ post(method: "Debugger.setAsyncCallStackDepth", params?: Debugger.SetAsyncCallStackDepthParameterType, callback?: (err: Error | null) => void): void; post(method: "Debugger.setAsyncCallStackDepth", callback?: (err: Error | null) => void): void; /** * Replace previous blackbox patterns with passed ones. Forces backend to skip stepping/pausing in scripts with url matching one of the patterns. VM will try to leave blackboxed script by performing 'step in' several times, finally resorting to 'step out' if unsuccessful. * @experimental */ post(method: "Debugger.setBlackboxPatterns", params?: Debugger.SetBlackboxPatternsParameterType, callback?: (err: Error | null) => void): void; post(method: "Debugger.setBlackboxPatterns", callback?: (err: Error | null) => void): void; /** * Makes backend skip steps in the script in blackboxed ranges. VM will try leave blacklisted scripts by performing 'step in' several times, finally resorting to 'step out' if unsuccessful. Positions array contains positions where blackbox state is changed. First interval isn't blackboxed. Array should be sorted. * @experimental */ post(method: "Debugger.setBlackboxedRanges", params?: Debugger.SetBlackboxedRangesParameterType, callback?: (err: Error | null) => void): void; post(method: "Debugger.setBlackboxedRanges", callback?: (err: Error | null) => void): void; /** * Enables console domain, sends the messages collected so far to the client by means of the <code>messageAdded</code> notification. */ post(method: "Console.enable", callback?: (err: Error | null) => void): void; /** * Disables console domain, prevents further console messages from being reported to the client. */ post(method: "Console.disable", callback?: (err: Error | null) => void): void; /** * Does nothing. */ post(method: "Console.clearMessages", callback?: (err: Error | null) => void): void; post(method: "Profiler.enable", callback?: (err: Error | null) => void): void; post(method: "Profiler.disable", callback?: (err: Error | null) => void): void; /** * Changes CPU profiler sampling interval. Must be called before CPU profiles recording started. */ post(method: "Profiler.setSamplingInterval", params?: Profiler.SetSamplingIntervalParameterType, callback?: (err: Error | null) => void): void; post(method: "Profiler.setSamplingInterval", callback?: (err: Error | null) => void): void; post(method: "Profiler.start", callback?: (err: Error | null) => void): void; post(method: "Profiler.stop", callback?: (err: Error | null, params: Profiler.StopReturnType) => void): void; /** * Enable precise code coverage. Coverage data for JavaScript executed before enabling precise code coverage may be incomplete. Enabling prevents running optimized code and resets execution counters. */ post(method: "Profiler.startPreciseCoverage", params?: Profiler.StartPreciseCoverageParameterType, callback?: (err: Error | null) => void): void; post(method: "Profiler.startPreciseCoverage", callback?: (err: Error | null) => void): void; /** * Disable precise code coverage. Disabling releases unnecessary execution count records and allows executing optimized code. */ post(method: "Profiler.stopPreciseCoverage", callback?: (err: Error | null) => void): void; /** * Collect coverage data for the current isolate, and resets execution counters. Precise code coverage needs to have started. */ post(method: "Profiler.takePreciseCoverage", callback?: (err: Error | null, params: Profiler.TakePreciseCoverageReturnType) => void): void; /** * Collect coverage data for the current isolate. The coverage data may be incomplete due to garbage collection. */ post(method: "Profiler.getBestEffortCoverage", callback?: (err: Error | null, params: Profiler.GetBestEffortCoverageReturnType) => void): void; /** * Enable type profile. * @experimental */ post(method: "Profiler.startTypeProfile", callback?: (err: Error | null) => void): void; /** * Disable type profile. Disabling releases type profile data collected so far. * @experimental */ post(method: "Profiler.stopTypeProfile", callback?: (err: Error | null) => void): void; /** * Collect type profile. * @experimental */ post(method: "Profiler.takeTypeProfile", callback?: (err: Error | null, params: Profiler.TakeTypeProfileReturnType) => void): void; post(method: "HeapProfiler.enable", callback?: (err: Error | null) => void): void; post(method: "HeapProfiler.disable", callback?: (err: Error | null) => void): void; post(method: "HeapProfiler.startTrackingHeapObjects", params?: HeapProfiler.StartTrackingHeapObjectsParameterType, callback?: (err: Error | null) => void): void; post(method: "HeapProfiler.startTrackingHeapObjects", callback?: (err: Error | null) => void): void; post(method: "HeapProfiler.stopTrackingHeapObjects", params?: HeapProfiler.StopTrackingHeapObjectsParameterType, callback?: (err: Error | null) => void): void; post(method: "HeapProfiler.stopTrackingHeapObjects", callback?: (err: Error | null) => void): void; post(method: "HeapProfiler.takeHeapSnapshot", params?: HeapProfiler.TakeHeapSnapshotParameterType, callback?: (err: Error | null) => void): void; post(method: "HeapProfiler.takeHeapSnapshot", callback?: (err: Error | null) => void): void; post(method: "HeapProfiler.collectGarbage", callback?: (err: Error | null) => void): void; post( method: "HeapProfiler.getObjectByHeapObjectId", params?: HeapProfiler.GetObjectByHeapObjectIdParameterType, callback?: (err: Error | null, params: HeapProfiler.GetObjectByHeapObjectIdReturnType) => void ): void; post(method: "HeapProfiler.getObjectByHeapObjectId", callback?: (err: Error | null, params: HeapProfiler.GetObjectByHeapObjectIdReturnType) => void): void; /** * Enables console to refer to the node with given id via $x (see Command Line API for more details $x functions). */ post(method: "HeapProfiler.addInspectedHeapObject", params?: HeapProfiler.AddInspectedHeapObjectParameterType, callback?: (err: Error | null) => void): void; post(method: "HeapProfiler.addInspectedHeapObject", callback?: (err: Error | null) => void): void; post(method: "HeapProfiler.getHeapObjectId", params?: HeapProfiler.GetHeapObjectIdParameterType, callback?: (err: Error | null, params: HeapProfiler.GetHeapObjectIdReturnType) => void): void; post(method: "HeapProfiler.getHeapObjectId", callback?: (err: Error | null, params: HeapProfiler.GetHeapObjectIdReturnType) => void): void; post(method: "HeapProfiler.startSampling", params?: HeapProfiler.StartSamplingParameterType, callback?: (err: Error | null) => void): void; post(method: "HeapProfiler.startSampling", callback?: (err: Error | null) => void): void; post(method: "HeapProfiler.stopSampling", callback?: (err: Error | null, params: HeapProfiler.StopSamplingReturnType) => void): void; post(method: "HeapProfiler.getSamplingProfile", callback?: (err: Error | null, params: HeapProfiler.GetSamplingProfileReturnType) => void): void; /** * Gets supported tracing categories. */ post(method: "NodeTracing.getCategories", callback?: (err: Error | null, params: NodeTracing.GetCategoriesReturnType) => void): void; /** * Start trace events collection. */ post(method: "NodeTracing.start", params?: NodeTracing.StartParameterType, callback?: (err: Error | null) => void): void; post(method: "NodeTracing.start", callback?: (err: Error | null) => void): void; /** * Stop trace events collection. Remaining collected events will be sent as a sequence of * dataCollected events followed by tracingComplete event. */ post(method: "NodeTracing.stop", callback?: (err: Error | null) => void): void; /** * Sends protocol message over session with given id. */ post(method: "NodeWorker.sendMessageToWorker", params?: NodeWorker.SendMessageToWorkerParameterType, callback?: (err: Error | null) => void): void; post(method: "NodeWorker.sendMessageToWorker", callback?: (err: Error | null) => void): void; /** * Instructs the inspector to attach to running workers. Will also attach to new workers * as they start */ post(method: "NodeWorker.enable", params?: NodeWorker.EnableParameterType, callback?: (err: Error | null) => void): void; post(method: "NodeWorker.enable", callback?: (err: Error | null) => void): void; /** * Detaches from all running workers and disables attaching to new workers as they are started. */ post(method: "NodeWorker.disable", callback?: (err: Error | null) => void): void; /** * Detached from the worker with given sessionId. */ post(method: "NodeWorker.detach", params?: NodeWorker.DetachParameterType, callback?: (err: Error | null) => void): void; post(method: "NodeWorker.detach", callback?: (err: Error | null) => void): void; /** * Enable the `NodeRuntime.waitingForDisconnect`. */ post(method: "NodeRuntime.notifyWhenWaitingForDisconnect", params?: NodeRuntime.NotifyWhenWaitingForDisconnectParameterType, callback?: (err: Error | null) => void): void; post(method: "NodeRuntime.notifyWhenWaitingForDisconnect", callback?: (err: Error | null) => void): void; // Events addListener(event: string, listener: (...args: any[]) => void): this; /** * Emitted when any notification from the V8 Inspector is received. */ addListener(event: "inspectorNotification", listener: (message: InspectorNotification<{}>) => void): this; /** * Issued when new execution context is created. */ addListener(event: "Runtime.executionContextCreated", listener: (message: InspectorNotification<Runtime.ExecutionContextCreatedEventDataType>) => void): this; /** * Issued when execution context is destroyed. */ addListener(event: "Runtime.executionContextDestroyed", listener: (message: InspectorNotification<Runtime.ExecutionContextDestroyedEventDataType>) => void): this; /** * Issued when all executionContexts were cleared in browser */ addListener(event: "Runtime.executionContextsCleared", listener: () => void): this; /** * Issued when exception was thrown and unhandled. */ addListener(event: "Runtime.exceptionThrown", listener: (message: InspectorNotification<Runtime.ExceptionThrownEventDataType>) => void): this; /** * Issued when unhandled exception was revoked. */ addListener(event: "Runtime.exceptionRevoked", listener: (message: InspectorNotification<Runtime.ExceptionRevokedEventDataType>) => void): this; /** * Issued when console API was called. */ addListener(event: "Runtime.consoleAPICalled", listener: (message: InspectorNotification<Runtime.ConsoleAPICalledEventDataType>) => void): this; /** * Issued when object should be inspected (for example, as a result of inspect() command line API call). */ addListener(event: "Runtime.inspectRequested", listener: (message: InspectorNotification<Runtime.InspectRequestedEventDataType>) => void): this; /** * Fired when virtual machine parses script. This event is also fired for all known and uncollected scripts upon enabling debugger. */ addListener(event: "Debugger.scriptParsed", listener: (message: InspectorNotification<Debugger.ScriptParsedEventDataType>) => void): this; /** * Fired when virtual machine fails to parse the script. */ addListener(event: "Debugger.scriptFailedToParse", listener: (message: InspectorNotification<Debugger.ScriptFailedToParseEventDataType>) => void): this; /** * Fired when breakpoint is resolved to an actual script and location. */ addListener(event: "Debugger.breakpointResolved", listener: (message: InspectorNotification<Debugger.BreakpointResolvedEventDataType>) => void): this; /** * Fired when the virtual machine stopped on breakpoint or exception or any other stop criteria. */ addListener(event: "Debugger.paused", listener: (message: InspectorNotification<Debugger.PausedEventDataType>) => void): this; /** * Fired when the virtual machine resumed execution. */ addListener(event: "Debugger.resumed", listener: () => void): this; /** * Issued when new console message is added. */ addListener(event: "Console.messageAdded", listener: (message: InspectorNotification<Console.MessageAddedEventDataType>) => void): this; /** * Sent when new profile recording is started using console.profile() call. */ addListener(event: "Profiler.consoleProfileStarted", listener: (message: InspectorNotification<Profiler.ConsoleProfileStartedEventDataType>) => void): this; addListener(event: "Profiler.consoleProfileFinished", listener: (message: InspectorNotification<Profiler.ConsoleProfileFinishedEventDataType>) => void): this; addListener(event: "HeapProfiler.addHeapSnapshotChunk", listener: (message: InspectorNotification<HeapProfiler.AddHeapSnapshotChunkEventDataType>) => void): this; addListener(event: "HeapProfiler.resetProfiles", listener: () => void): this; addListener(event: "HeapProfiler.reportHeapSnapshotProgress", listener: (message: InspectorNotification<HeapProfiler.ReportHeapSnapshotProgressEventDataType>) => void): this; /** * If heap objects tracking has been started then backend regularly sends a current value for last seen object id and corresponding timestamp. If the were changes in the heap since last event then one or more heapStatsUpdate events will be sent before a new lastSeenObjectId event. */ addListener(event: "HeapProfiler.lastSeenObjectId", listener: (message: InspectorNotification<HeapProfiler.LastSeenObjectIdEventDataType>) => void): this; /** * If heap objects tracking has been started then backend may send update for one or more fragments */ addListener(event: "HeapProfiler.heapStatsUpdate", listener: (message: InspectorNotification<HeapProfiler.HeapStatsUpdateEventDataType>) => void): this; /** * Contains an bucket of collected trace events. */ addListener(event: "NodeTracing.dataCollected", listener: (message: InspectorNotification<NodeTracing.DataCollectedEventDataType>) => void): this; /** * Signals that tracing is stopped and there is no trace buffers pending flush, all data were * delivered via dataCollected events. */ addListener(event: "NodeTracing.tracingComplete", listener: () => void): this; /** * Issued when attached to a worker. */ addListener(event: "NodeWorker.attachedToWorker", listener: (message: InspectorNotification<NodeWorker.AttachedToWorkerEventDataType>) => void): this; /** * Issued when detached from the worker. */ addListener(event: "NodeWorker.detachedFromWorker", listener: (message: InspectorNotification<NodeWorker.DetachedFromWorkerEventDataType>) => void): this; /** * Notifies about a new protocol message received from the session * (session ID is provided in attachedToWorker notification). */ addListener(event: "NodeWorker.receivedMessageFromWorker", listener: (message: InspectorNotification<NodeWorker.ReceivedMessageFromWorkerEventDataType>) => void): this; /** * This event is fired instead of `Runtime.executionContextDestroyed` when * enabled. * It is fired when the Node process finished all code execution and is * waiting for all frontends to disconnect. */ addListener(event: "NodeRuntime.waitingForDisconnect", listener: () => void): this; emit(event: string | symbol, ...args: any[]): boolean; emit(event: "inspectorNotification", message: InspectorNotification<{}>): boolean; emit(event: "Runtime.executionContextCreated", message: InspectorNotification<Runtime.ExecutionContextCreatedEventDataType>): boolean; emit(event: "Runtime.executionContextDestroyed", message: InspectorNotification<Runtime.ExecutionContextDestroyedEventDataType>): boolean; emit(event: "Runtime.executionContextsCleared"): boolean; emit(event: "Runtime.exceptionThrown", message: InspectorNotification<Runtime.ExceptionThrownEventDataType>): boolean; emit(event: "Runtime.exceptionRevoked", message: InspectorNotification<Runtime.ExceptionRevokedEventDataType>): boolean; emit(event: "Runtime.consoleAPICalled", message: InspectorNotification<Runtime.ConsoleAPICalledEventDataType>): boolean; emit(event: "Runtime.inspectRequested", message: InspectorNotification<Runtime.InspectRequestedEventDataType>): boolean; emit(event: "Debugger.scriptParsed", message: InspectorNotification<Debugger.ScriptParsedEventDataType>): boolean; emit(event: "Debugger.scriptFailedToParse", message: InspectorNotification<Debugger.ScriptFailedToParseEventDataType>): boolean; emit(event: "Debugger.breakpointResolved", message: InspectorNotification<Debugger.BreakpointResolvedEventDataType>): boolean; emit(event: "Debugger.paused", message: InspectorNotification<Debugger.PausedEventDataType>): boolean; emit(event: "Debugger.resumed"): boolean; emit(event: "Console.messageAdded", message: InspectorNotification<Console.MessageAddedEventDataType>): boolean; emit(event: "Profiler.consoleProfileStarted", message: InspectorNotification<Profiler.ConsoleProfileStartedEventDataType>): boolean; emit(event: "Profiler.consoleProfileFinished", message: InspectorNotification<Profiler.ConsoleProfileFinishedEventDataType>): boolean; emit(event: "HeapProfiler.addHeapSnapshotChunk", message: InspectorNotification<HeapProfiler.AddHeapSnapshotChunkEventDataType>): boolean; emit(event: "HeapProfiler.resetProfiles"): boolean; emit(event: "HeapProfiler.reportHeapSnapshotProgress", message: InspectorNotification<HeapProfiler.ReportHeapSnapshotProgressEventDataType>): boolean; emit(event: "HeapProfiler.lastSeenObjectId", message: InspectorNotification<HeapProfiler.LastSeenObjectIdEventDataType>): boolean; emit(event: "HeapProfiler.heapStatsUpdate", message: InspectorNotification<HeapProfiler.HeapStatsUpdateEventDataType>): boolean; emit(event: "NodeTracing.dataCollected", message: InspectorNotification<NodeTracing.DataCollectedEventDataType>): boolean; emit(event: "NodeTracing.tracingComplete"): boolean; emit(event: "NodeWorker.attachedToWorker", message: InspectorNotification<NodeWorker.AttachedToWorkerEventDataType>): boolean; emit(event: "NodeWorker.detachedFromWorker", message: InspectorNotification<NodeWorker.DetachedFromWorkerEventDataType>): boolean; emit(event: "NodeWorker.receivedMessageFromWorker", message: InspectorNotification<NodeWorker.ReceivedMessageFromWorkerEventDataType>): boolean; emit(event: "NodeRuntime.waitingForDisconnect"): boolean; on(event: string, listener: (...args: any[]) => void): this; /** * Emitted when any notification from the V8 Inspector is received. */ on(event: "inspectorNotification", listener: (message: InspectorNotification<{}>) => void): this; /** * Issued when new execution context is created. */ on(event: "Runtime.executionContextCreated", listener: (message: InspectorNotification<Runtime.ExecutionContextCreatedEventDataType>) => void): this; /** * Issued when execution context is destroyed. */ on(event: "Runtime.executionContextDestroyed", listener: (message: InspectorNotification<Runtime.ExecutionContextDestroyedEventDataType>) => void): this; /** * Issued when all executionContexts were cleared in browser */ on(event: "Runtime.executionContextsCleared", listener: () => void): this; /** * Issued when exception was thrown and unhandled. */ on(event: "Runtime.exceptionThrown", listener: (message: InspectorNotification<Runtime.ExceptionThrownEventDataType>) => void): this; /** * Issued when unhandled exception was revoked. */ on(event: "Runtime.exceptionRevoked", listener: (message: InspectorNotification<Runtime.ExceptionRevokedEventDataType>) => void): this; /** * Issued when console API was called. */ on(event: "Runtime.consoleAPICalled", listener: (message: InspectorNotification<Runtime.ConsoleAPICalledEventDataType>) => void): this; /** * Issued when object should be inspected (for example, as a result of inspect() command line API call). */ on(event: "Runtime.inspectRequested", listener: (message: InspectorNotification<Runtime.InspectRequestedEventDataType>) => void): this; /** * Fired when virtual machine parses script. This event is also fired for all known and uncollected scripts upon enabling debugger. */ on(event: "Debugger.scriptParsed", listener: (message: InspectorNotification<Debugger.ScriptParsedEventDataType>) => void): this; /** * Fired when virtual machine fails to parse the script. */ on(event: "Debugger.scriptFailedToParse", listener: (message: InspectorNotification<Debugger.ScriptFailedToParseEventDataType>) => void): this; /** * Fired when breakpoint is resolved to an actual script and location. */ on(event: "Debugger.breakpointResolved", listener: (message: InspectorNotification<Debugger.BreakpointResolvedEventDataType>) => void): this; /** * Fired when the virtual machine stopped on breakpoint or exception or any other stop criteria. */ on(event: "Debugger.paused", listener: (message: InspectorNotification<Debugger.PausedEventDataType>) => void): this; /** * Fired when the virtual machine resumed execution. */ on(event: "Debugger.resumed", listener: () => void): this; /** * Issued when new console message is added. */ on(event: "Console.messageAdded", listener: (message: InspectorNotification<Console.MessageAddedEventDataType>) => void): this; /** * Sent when new profile recording is started using console.profile() call. */ on(event: "Profiler.consoleProfileStarted", listener: (message: InspectorNotification<Profiler.ConsoleProfileStartedEventDataType>) => void): this; on(event: "Profiler.consoleProfileFinished", listener: (message: InspectorNotification<Profiler.ConsoleProfileFinishedEventDataType>) => void): this; on(event: "HeapProfiler.addHeapSnapshotChunk", listener: (message: InspectorNotification<HeapProfiler.AddHeapSnapshotChunkEventDataType>) => void): this; on(event: "HeapProfiler.resetProfiles", listener: () => void): this; on(event: "HeapProfiler.reportHeapSnapshotProgress", listener: (message: InspectorNotification<HeapProfiler.ReportHeapSnapshotProgressEventDataType>) => void): this; /** * If heap objects tracking has been started then backend regularly sends a current value for last seen object id and corresponding timestamp. If the were changes in the heap since last event then one or more heapStatsUpdate events will be sent before a new lastSeenObjectId event. */ on(event: "HeapProfiler.lastSeenObjectId", listener: (message: InspectorNotification<HeapProfiler.LastSeenObjectIdEventDataType>) => void): this; /** * If heap objects tracking has been started then backend may send update for one or more fragments */ on(event: "HeapProfiler.heapStatsUpdate", listener: (message: InspectorNotification<HeapProfiler.HeapStatsUpdateEventDataType>) => void): this; /** * Contains an bucket of collected trace events. */ on(event: "NodeTracing.dataCollected", listener: (message: InspectorNotification<NodeTracing.DataCollectedEventDataType>) => void): this; /** * Signals that tracing is stopped and there is no trace buffers pending flush, all data were * delivered via dataCollected events. */ on(event: "NodeTracing.tracingComplete", listener: () => void): this; /** * Issued when attached to a worker. */ on(event: "NodeWorker.attachedToWorker", listener: (message: InspectorNotification<NodeWorker.AttachedToWorkerEventDataType>) => void): this; /** * Issued when detached from the worker. */ on(event: "NodeWorker.detachedFromWorker", listener: (message: InspectorNotification<NodeWorker.DetachedFromWorkerEventDataType>) => void): this; /** * Notifies about a new protocol message received from the session * (session ID is provided in attachedToWorker notification). */ on(event: "NodeWorker.receivedMessageFromWorker", listener: (message: InspectorNotification<NodeWorker.ReceivedMessageFromWorkerEventDataType>) => void): this; /** * This event is fired instead of `Runtime.executionContextDestroyed` when * enabled. * It is fired when the Node process finished all code execution and is * waiting for all frontends to disconnect. */ on(event: "NodeRuntime.waitingForDisconnect", listener: () => void): this; once(event: string, listener: (...args: any[]) => void): this; /** * Emitted when any notification from the V8 Inspector is received. */ once(event: "inspectorNotification", listener: (message: InspectorNotification<{}>) => void): this; /** * Issued when new execution context is created. */ once(event: "Runtime.executionContextCreated", listener: (message: InspectorNotification<Runtime.ExecutionContextCreatedEventDataType>) => void): this; /** * Issued when execution context is destroyed. */ once(event: "Runtime.executionContextDestroyed", listener: (message: InspectorNotification<Runtime.ExecutionContextDestroyedEventDataType>) => void): this; /** * Issued when all executionContexts were cleared in browser */ once(event: "Runtime.executionContextsCleared", listener: () => void): this; /** * Issued when exception was thrown and unhandled. */ once(event: "Runtime.exceptionThrown", listener: (message: InspectorNotification<Runtime.ExceptionThrownEventDataType>) => void): this; /** * Issued when unhandled exception was revoked. */ once(event: "Runtime.exceptionRevoked", listener: (message: InspectorNotification<Runtime.ExceptionRevokedEventDataType>) => void): this; /** * Issued when console API was called. */ once(event: "Runtime.consoleAPICalled", listener: (message: InspectorNotification<Runtime.ConsoleAPICalledEventDataType>) => void): this; /** * Issued when object should be inspected (for example, as a result of inspect() command line API call). */ once(event: "Runtime.inspectRequested", listener: (message: InspectorNotification<Runtime.InspectRequestedEventDataType>) => void): this; /** * Fired when virtual machine parses script. This event is also fired for all known and uncollected scripts upon enabling debugger. */ once(event: "Debugger.scriptParsed", listener: (message: InspectorNotification<Debugger.ScriptParsedEventDataType>) => void): this; /** * Fired when virtual machine fails to parse the script. */ once(event: "Debugger.scriptFailedToParse", listener: (message: InspectorNotification<Debugger.ScriptFailedToParseEventDataType>) => void): this; /** * Fired when breakpoint is resolved to an actual script and location. */ once(event: "Debugger.breakpointResolved", listener: (message: InspectorNotification<Debugger.BreakpointResolvedEventDataType>) => void): this; /** * Fired when the virtual machine stopped on breakpoint or exception or any other stop criteria. */ once(event: "Debugger.paused", listener: (message: InspectorNotification<Debugger.PausedEventDataType>) => void): this; /** * Fired when the virtual machine resumed execution. */ once(event: "Debugger.resumed", listener: () => void): this; /** * Issued when new console message is added. */ once(event: "Console.messageAdded", listener: (message: InspectorNotification<Console.MessageAddedEventDataType>) => void): this; /** * Sent when new profile recording is started using console.profile() call. */ once(event: "Profiler.consoleProfileStarted", listener: (message: InspectorNotification<Profiler.ConsoleProfileStartedEventDataType>) => void): this; once(event: "Profiler.consoleProfileFinished", listener: (message: InspectorNotification<Profiler.ConsoleProfileFinishedEventDataType>) => void): this; once(event: "HeapProfiler.addHeapSnapshotChunk", listener: (message: InspectorNotification<HeapProfiler.AddHeapSnapshotChunkEventDataType>) => void): this; once(event: "HeapProfiler.resetProfiles", listener: () => void): this; once(event: "HeapProfiler.reportHeapSnapshotProgress", listener: (message: InspectorNotification<HeapProfiler.ReportHeapSnapshotProgressEventDataType>) => void): this; /** * If heap objects tracking has been started then backend regularly sends a current value for last seen object id and corresponding timestamp. If the were changes in the heap since last event then one or more heapStatsUpdate events will be sent before a new lastSeenObjectId event. */ once(event: "HeapProfiler.lastSeenObjectId", listener: (message: InspectorNotification<HeapProfiler.LastSeenObjectIdEventDataType>) => void): this; /** * If heap objects tracking has been started then backend may send update for one or more fragments */ once(event: "HeapProfiler.heapStatsUpdate", listener: (message: InspectorNotification<HeapProfiler.HeapStatsUpdateEventDataType>) => void): this; /** * Contains an bucket of collected trace events. */ once(event: "NodeTracing.dataCollected", listener: (message: InspectorNotification<NodeTracing.DataCollectedEventDataType>) => void): this; /** * Signals that tracing is stopped and there is no trace buffers pending flush, all data were * delivered via dataCollected events. */ once(event: "NodeTracing.tracingComplete", listener: () => void): this; /** * Issued when attached to a worker. */ once(event: "NodeWorker.attachedToWorker", listener: (message: InspectorNotification<NodeWorker.AttachedToWorkerEventDataType>) => void): this; /** * Issued when detached from the worker. */ once(event: "NodeWorker.detachedFromWorker", listener: (message: InspectorNotification<NodeWorker.DetachedFromWorkerEventDataType>) => void): this; /** * Notifies about a new protocol message received from the session * (session ID is provided in attachedToWorker notification). */ once(event: "NodeWorker.receivedMessageFromWorker", listener: (message: InspectorNotification<NodeWorker.ReceivedMessageFromWorkerEventDataType>) => void): this; /** * This event is fired instead of `Runtime.executionContextDestroyed` when * enabled. * It is fired when the Node process finished all code execution and is * waiting for all frontends to disconnect. */ once(event: "NodeRuntime.waitingForDisconnect", listener: () => void): this; prependListener(event: string, listener: (...args: any[]) => void): this; /** * Emitted when any notification from the V8 Inspector is received. */ prependListener(event: "inspectorNotification", listener: (message: InspectorNotification<{}>) => void): this; /** * Issued when new execution context is created. */ prependListener(event: "Runtime.executionContextCreated", listener: (message: InspectorNotification<Runtime.ExecutionContextCreatedEventDataType>) => void): this; /** * Issued when execution context is destroyed. */ prependListener(event: "Runtime.executionContextDestroyed", listener: (message: InspectorNotification<Runtime.ExecutionContextDestroyedEventDataType>) => void): this; /** * Issued when all executionContexts were cleared in browser */ prependListener(event: "Runtime.executionContextsCleared", listener: () => void): this; /** * Issued when exception was thrown and unhandled. */ prependListener(event: "Runtime.exceptionThrown", listener: (message: InspectorNotification<Runtime.ExceptionThrownEventDataType>) => void): this; /** * Issued when unhandled exception was revoked. */ prependListener(event: "Runtime.exceptionRevoked", listener: (message: InspectorNotification<Runtime.ExceptionRevokedEventDataType>) => void): this; /** * Issued when console API was called. */ prependListener(event: "Runtime.consoleAPICalled", listener: (message: InspectorNotification<Runtime.ConsoleAPICalledEventDataType>) => void): this; /** * Issued when object should be inspected (for example, as a result of inspect() command line API call). */ prependListener(event: "Runtime.inspectRequested", listener: (message: InspectorNotification<Runtime.InspectRequestedEventDataType>) => void): this; /** * Fired when virtual machine parses script. This event is also fired for all known and uncollected scripts upon enabling debugger. */ prependListener(event: "Debugger.scriptParsed", listener: (message: InspectorNotification<Debugger.ScriptParsedEventDataType>) => void): this; /** * Fired when virtual machine fails to parse the script. */ prependListener(event: "Debugger.scriptFailedToParse", listener: (message: InspectorNotification<Debugger.ScriptFailedToParseEventDataType>) => void): this; /** * Fired when breakpoint is resolved to an actual script and location. */ prependListener(event: "Debugger.breakpointResolved", listener: (message: InspectorNotification<Debugger.BreakpointResolvedEventDataType>) => void): this; /** * Fired when the virtual machine stopped on breakpoint or exception or any other stop criteria. */ prependListener(event: "Debugger.paused", listener: (message: InspectorNotification<Debugger.PausedEventDataType>) => void): this; /** * Fired when the virtual machine resumed execution. */ prependListener(event: "Debugger.resumed", listener: () => void): this; /** * Issued when new console message is added. */ prependListener(event: "Console.messageAdded", listener: (message: InspectorNotification<Console.MessageAddedEventDataType>) => void): this; /** * Sent when new profile recording is started using console.profile() call. */ prependListener(event: "Profiler.consoleProfileStarted", listener: (message: InspectorNotification<Profiler.ConsoleProfileStartedEventDataType>) => void): this; prependListener(event: "Profiler.consoleProfileFinished", listener: (message: InspectorNotification<Profiler.ConsoleProfileFinishedEventDataType>) => void): this; prependListener(event: "HeapProfiler.addHeapSnapshotChunk", listener: (message: InspectorNotification<HeapProfiler.AddHeapSnapshotChunkEventDataType>) => void): this; prependListener(event: "HeapProfiler.resetProfiles", listener: () => void): this; prependListener(event: "HeapProfiler.reportHeapSnapshotProgress", listener: (message: InspectorNotification<HeapProfiler.ReportHeapSnapshotProgressEventDataType>) => void): this; /** * If heap objects tracking has been started then backend regularly sends a current value for last seen object id and corresponding timestamp. If the were changes in the heap since last event then one or more heapStatsUpdate events will be sent before a new lastSeenObjectId event. */ prependListener(event: "HeapProfiler.lastSeenObjectId", listener: (message: InspectorNotification<HeapProfiler.LastSeenObjectIdEventDataType>) => void): this; /** * If heap objects tracking has been started then backend may send update for one or more fragments */ prependListener(event: "HeapProfiler.heapStatsUpdate", listener: (message: InspectorNotification<HeapProfiler.HeapStatsUpdateEventDataType>) => void): this; /** * Contains an bucket of collected trace events. */ prependListener(event: "NodeTracing.dataCollected", listener: (message: InspectorNotification<NodeTracing.DataCollectedEventDataType>) => void): this; /** * Signals that tracing is stopped and there is no trace buffers pending flush, all data were * delivered via dataCollected events. */ prependListener(event: "NodeTracing.tracingComplete", listener: () => void): this; /** * Issued when attached to a worker. */ prependListener(event: "NodeWorker.attachedToWorker", listener: (message: InspectorNotification<NodeWorker.AttachedToWorkerEventDataType>) => void): this; /** * Issued when detached from the worker. */ prependListener(event: "NodeWorker.detachedFromWorker", listener: (message: InspectorNotification<NodeWorker.DetachedFromWorkerEventDataType>) => void): this; /** * Notifies about a new protocol message received from the session * (session ID is provided in attachedToWorker notification). */ prependListener(event: "NodeWorker.receivedMessageFromWorker", listener: (message: InspectorNotification<NodeWorker.ReceivedMessageFromWorkerEventDataType>) => void): this; /** * This event is fired instead of `Runtime.executionContextDestroyed` when * enabled. * It is fired when the Node process finished all code execution and is * waiting for all frontends to disconnect. */ prependListener(event: "NodeRuntime.waitingForDisconnect", listener: () => void): this; prependOnceListener(event: string, listener: (...args: any[]) => void): this; /** * Emitted when any notification from the V8 Inspector is received. */ prependOnceListener(event: "inspectorNotification", listener: (message: InspectorNotification<{}>) => void): this; /** * Issued when new execution context is created. */ prependOnceListener(event: "Runtime.executionContextCreated", listener: (message: InspectorNotification<Runtime.ExecutionContextCreatedEventDataType>) => void): this; /** * Issued when execution context is destroyed. */ prependOnceListener(event: "Runtime.executionContextDestroyed", listener: (message: InspectorNotification<Runtime.ExecutionContextDestroyedEventDataType>) => void): this; /** * Issued when all executionContexts were cleared in browser */ prependOnceListener(event: "Runtime.executionContextsCleared", listener: () => void): this; /** * Issued when exception was thrown and unhandled. */ prependOnceListener(event: "Runtime.exceptionThrown", listener: (message: InspectorNotification<Runtime.ExceptionThrownEventDataType>) => void): this; /** * Issued when unhandled exception was revoked. */ prependOnceListener(event: "Runtime.exceptionRevoked", listener: (message: InspectorNotification<Runtime.ExceptionRevokedEventDataType>) => void): this; /** * Issued when console API was called. */ prependOnceListener(event: "Runtime.consoleAPICalled", listener: (message: InspectorNotification<Runtime.ConsoleAPICalledEventDataType>) => void): this; /** * Issued when object should be inspected (for example, as a result of inspect() command line API call). */ prependOnceListener(event: "Runtime.inspectRequested", listener: (message: InspectorNotification<Runtime.InspectRequestedEventDataType>) => void): this; /** * Fired when virtual machine parses script. This event is also fired for all known and uncollected scripts upon enabling debugger. */ prependOnceListener(event: "Debugger.scriptParsed", listener: (message: InspectorNotification<Debugger.ScriptParsedEventDataType>) => void): this; /** * Fired when virtual machine fails to parse the script. */ prependOnceListener(event: "Debugger.scriptFailedToParse", listener: (message: InspectorNotification<Debugger.ScriptFailedToParseEventDataType>) => void): this; /** * Fired when breakpoint is resolved to an actual script and location. */ prependOnceListener(event: "Debugger.breakpointResolved", listener: (message: InspectorNotification<Debugger.BreakpointResolvedEventDataType>) => void): this; /** * Fired when the virtual machine stopped on breakpoint or exception or any other stop criteria. */ prependOnceListener(event: "Debugger.paused", listener: (message: InspectorNotification<Debugger.PausedEventDataType>) => void): this; /** * Fired when the virtual machine resumed execution. */ prependOnceListener(event: "Debugger.resumed", listener: () => void): this; /** * Issued when new console message is added. */ prependOnceListener(event: "Console.messageAdded", listener: (message: InspectorNotification<Console.MessageAddedEventDataType>) => void): this; /** * Sent when new profile recording is started using console.profile() call. */ prependOnceListener(event: "Profiler.consoleProfileStarted", listener: (message: InspectorNotification<Profiler.ConsoleProfileStartedEventDataType>) => void): this; prependOnceListener(event: "Profiler.consoleProfileFinished", listener: (message: InspectorNotification<Profiler.ConsoleProfileFinishedEventDataType>) => void): this; prependOnceListener(event: "HeapProfiler.addHeapSnapshotChunk", listener: (message: InspectorNotification<HeapProfiler.AddHeapSnapshotChunkEventDataType>) => void): this; prependOnceListener(event: "HeapProfiler.resetProfiles", listener: () => void): this; prependOnceListener(event: "HeapProfiler.reportHeapSnapshotProgress", listener: (message: InspectorNotification<HeapProfiler.ReportHeapSnapshotProgressEventDataType>) => void): this; /** * If heap objects tracking has been started then backend regularly sends a current value for last seen object id and corresponding timestamp. If the were changes in the heap since last event then one or more heapStatsUpdate events will be sent before a new lastSeenObjectId event. */ prependOnceListener(event: "HeapProfiler.lastSeenObjectId", listener: (message: InspectorNotification<HeapProfiler.LastSeenObjectIdEventDataType>) => void): this; /** * If heap objects tracking has been started then backend may send update for one or more fragments */ prependOnceListener(event: "HeapProfiler.heapStatsUpdate", listener: (message: InspectorNotification<HeapProfiler.HeapStatsUpdateEventDataType>) => void): this; /** * Contains an bucket of collected trace events. */ prependOnceListener(event: "NodeTracing.dataCollected", listener: (message: InspectorNotification<NodeTracing.DataCollectedEventDataType>) => void): this; /** * Signals that tracing is stopped and there is no trace buffers pending flush, all data were * delivered via dataCollected events. */ prependOnceListener(event: "NodeTracing.tracingComplete", listener: () => void): this; /** * Issued when attached to a worker. */ prependOnceListener(event: "NodeWorker.attachedToWorker", listener: (message: InspectorNotification<NodeWorker.AttachedToWorkerEventDataType>) => void): this; /** * Issued when detached from the worker. */ prependOnceListener(event: "NodeWorker.detachedFromWorker", listener: (message: InspectorNotification<NodeWorker.DetachedFromWorkerEventDataType>) => void): this; /** * Notifies about a new protocol message received from the session * (session ID is provided in attachedToWorker notification). */ prependOnceListener(event: "NodeWorker.receivedMessageFromWorker", listener: (message: InspectorNotification<NodeWorker.ReceivedMessageFromWorkerEventDataType>) => void): this; /** * This event is fired instead of `Runtime.executionContextDestroyed` when * enabled. * It is fired when the Node process finished all code execution and is * waiting for all frontends to disconnect. */ prependOnceListener(event: "NodeRuntime.waitingForDisconnect", listener: () => void): this; } // Top Level API /** * Activate inspector on host and port. Equivalent to node --inspect=[[host:]port], but can be done programatically after node has started. * If wait is true, will block until a client has connected to the inspect port and flow control has been passed to the debugger client. * @param port Port to listen on for inspector connections. Optional, defaults to what was specified on the CLI. * @param host Host to listen on for inspector connections. Optional, defaults to what was specified on the CLI. * @param wait Block until a client has connected. Optional, defaults to false. */ function open(port?: number, host?: string, wait?: boolean): void; /** * Deactivate the inspector. Blocks until there are no active connections. */ function close(): void; /** * Return the URL of the active inspector, or `undefined` if there is none. */ function url(): string | undefined; /** * Blocks until a client (existing or connected later) has sent * `Runtime.runIfWaitingForDebugger` command. * An exception will be thrown if there is no active inspector. */ function waitForDebugger(): void; } apollo-server-demo/node_modules/@types/node/perf_hooks.d.ts 0000644 0001750 0000144 00000024570 14000133700 023545 0 ustar andreh users declare module 'perf_hooks' { import { AsyncResource } from 'async_hooks'; type EntryType = 'node' | 'mark' | 'measure' | 'gc' | 'function' | 'http2' | 'http'; interface PerformanceEntry { /** * The total number of milliseconds elapsed for this entry. * This value will not be meaningful for all Performance Entry types. */ readonly duration: number; /** * The name of the performance entry. */ readonly name: string; /** * The high resolution millisecond timestamp marking the starting time of the Performance Entry. */ readonly startTime: number; /** * The type of the performance entry. * Currently it may be one of: 'node', 'mark', 'measure', 'gc', or 'function'. */ readonly entryType: EntryType; /** * When `performanceEntry.entryType` is equal to 'gc', `the performance.kind` property identifies * the type of garbage collection operation that occurred. * See perf_hooks.constants for valid values. */ readonly kind?: number; /** * When `performanceEntry.entryType` is equal to 'gc', the `performance.flags` * property contains additional information about garbage collection operation. * See perf_hooks.constants for valid values. */ readonly flags?: number; } interface PerformanceNodeTiming extends PerformanceEntry { /** * The high resolution millisecond timestamp at which the Node.js process completed bootstrap. */ readonly bootstrapComplete: number; /** * The high resolution millisecond timestamp at which the Node.js process completed bootstrapping. * If bootstrapping has not yet finished, the property has the value of -1. */ readonly environment: number; /** * The high resolution millisecond timestamp at which the Node.js environment was initialized. */ readonly idleTime: number; /** * The high resolution millisecond timestamp of the amount of time the event loop has been idle * within the event loop's event provider (e.g. `epoll_wait`). This does not take CPU usage * into consideration. If the event loop has not yet started (e.g., in the first tick of the main script), * the property has the value of 0. */ readonly loopExit: number; /** * The high resolution millisecond timestamp at which the Node.js event loop started. * If the event loop has not yet started (e.g., in the first tick of the main script), the property has the value of -1. */ readonly loopStart: number; /** * The high resolution millisecond timestamp at which the V8 platform was initialized. */ readonly v8Start: number; } interface EventLoopUtilization { idle: number; active: number; utilization: number; } interface Performance { /** * If name is not provided, removes all PerformanceMark objects from the Performance Timeline. * If name is provided, removes only the named mark. * @param name */ clearMarks(name?: string): void; /** * Creates a new PerformanceMark entry in the Performance Timeline. * A PerformanceMark is a subclass of PerformanceEntry whose performanceEntry.entryType is always 'mark', * and whose performanceEntry.duration is always 0. * Performance marks are used to mark specific significant moments in the Performance Timeline. * @param name */ mark(name?: string): void; /** * Creates a new PerformanceMeasure entry in the Performance Timeline. * A PerformanceMeasure is a subclass of PerformanceEntry whose performanceEntry.entryType is always 'measure', * and whose performanceEntry.duration measures the number of milliseconds elapsed since startMark and endMark. * * The startMark argument may identify any existing PerformanceMark in the the Performance Timeline, or may identify * any of the timestamp properties provided by the PerformanceNodeTiming class. If the named startMark does not exist, * then startMark is set to timeOrigin by default. * * The endMark argument must identify any existing PerformanceMark in the the Performance Timeline or any of the timestamp * properties provided by the PerformanceNodeTiming class. If the named endMark does not exist, an error will be thrown. * @param name * @param startMark * @param endMark */ measure(name: string, startMark: string, endMark: string): void; /** * An instance of the PerformanceNodeTiming class that provides performance metrics for specific Node.js operational milestones. */ readonly nodeTiming: PerformanceNodeTiming; /** * @return the current high resolution millisecond timestamp */ now(): number; /** * The timeOrigin specifies the high resolution millisecond timestamp from which all performance metric durations are measured. */ readonly timeOrigin: number; /** * Wraps a function within a new function that measures the running time of the wrapped function. * A PerformanceObserver must be subscribed to the 'function' event type in order for the timing details to be accessed. * @param fn */ timerify<T extends (...optionalParams: any[]) => any>(fn: T): T; /** * eventLoopUtilization is similar to CPU utilization except that it is calculated using high precision wall-clock time. * It represents the percentage of time the event loop has spent outside the event loop's event provider (e.g. epoll_wait). * No other CPU idle time is taken into consideration. * * @param util1 The result of a previous call to eventLoopUtilization() * @param util2 The result of a previous call to eventLoopUtilization() prior to util1 */ eventLoopUtilization(util1?: EventLoopUtilization, util2?: EventLoopUtilization): EventLoopUtilization; } interface PerformanceObserverEntryList { /** * @return a list of PerformanceEntry objects in chronological order with respect to performanceEntry.startTime. */ getEntries(): PerformanceEntry[]; /** * @return a list of PerformanceEntry objects in chronological order with respect to performanceEntry.startTime * whose performanceEntry.name is equal to name, and optionally, whose performanceEntry.entryType is equal to type. */ getEntriesByName(name: string, type?: EntryType): PerformanceEntry[]; /** * @return Returns a list of PerformanceEntry objects in chronological order with respect to performanceEntry.startTime * whose performanceEntry.entryType is equal to type. */ getEntriesByType(type: EntryType): PerformanceEntry[]; } type PerformanceObserverCallback = (list: PerformanceObserverEntryList, observer: PerformanceObserver) => void; class PerformanceObserver extends AsyncResource { constructor(callback: PerformanceObserverCallback); /** * Disconnects the PerformanceObserver instance from all notifications. */ disconnect(): void; /** * Subscribes the PerformanceObserver instance to notifications of new PerformanceEntry instances identified by options.entryTypes. * When options.buffered is false, the callback will be invoked once for every PerformanceEntry instance. * Property buffered defaults to false. * @param options */ observe(options: { entryTypes: ReadonlyArray<EntryType>; buffered?: boolean }): void; } namespace constants { const NODE_PERFORMANCE_GC_MAJOR: number; const NODE_PERFORMANCE_GC_MINOR: number; const NODE_PERFORMANCE_GC_INCREMENTAL: number; const NODE_PERFORMANCE_GC_WEAKCB: number; const NODE_PERFORMANCE_GC_FLAGS_NO: number; const NODE_PERFORMANCE_GC_FLAGS_CONSTRUCT_RETAINED: number; const NODE_PERFORMANCE_GC_FLAGS_FORCED: number; const NODE_PERFORMANCE_GC_FLAGS_SYNCHRONOUS_PHANTOM_PROCESSING: number; const NODE_PERFORMANCE_GC_FLAGS_ALL_AVAILABLE_GARBAGE: number; const NODE_PERFORMANCE_GC_FLAGS_ALL_EXTERNAL_MEMORY: number; const NODE_PERFORMANCE_GC_FLAGS_SCHEDULE_IDLE: number; } const performance: Performance; interface EventLoopMonitorOptions { /** * The sampling rate in milliseconds. * Must be greater than zero. * @default 10 */ resolution?: number; } interface EventLoopDelayMonitor { /** * Enables the event loop delay sample timer. Returns `true` if the timer was started, `false` if it was already started. */ enable(): boolean; /** * Disables the event loop delay sample timer. Returns `true` if the timer was stopped, `false` if it was already stopped. */ disable(): boolean; /** * Resets the collected histogram data. */ reset(): void; /** * Returns the value at the given percentile. * @param percentile A percentile value between 1 and 100. */ percentile(percentile: number): number; /** * A `Map` object detailing the accumulated percentile distribution. */ readonly percentiles: Map<number, number>; /** * The number of times the event loop delay exceeded the maximum 1 hour eventloop delay threshold. */ readonly exceeds: number; /** * The minimum recorded event loop delay. */ readonly min: number; /** * The maximum recorded event loop delay. */ readonly max: number; /** * The mean of the recorded event loop delays. */ readonly mean: number; /** * The standard deviation of the recorded event loop delays. */ readonly stddev: number; } function monitorEventLoopDelay(options?: EventLoopMonitorOptions): EventLoopDelayMonitor; } apollo-server-demo/node_modules/@types/node/net.d.ts 0000644 0001750 0000144 00000031141 14000133700 022164 0 ustar andreh users declare module "net" { import * as stream from "stream"; import * as events from "events"; import * as dns from "dns"; type LookupFunction = (hostname: string, options: dns.LookupOneOptions, callback: (err: NodeJS.ErrnoException | null, address: string, family: number) => void) => void; interface AddressInfo { address: string; family: string; port: number; } interface SocketConstructorOpts { fd?: number; allowHalfOpen?: boolean; readable?: boolean; writable?: boolean; } interface OnReadOpts { buffer: Uint8Array | (() => Uint8Array); /** * This function is called for every chunk of incoming data. * Two arguments are passed to it: the number of bytes written to buffer and a reference to buffer. * Return false from this function to implicitly pause() the socket. */ callback(bytesWritten: number, buf: Uint8Array): boolean; } interface ConnectOpts { /** * If specified, incoming data is stored in a single buffer and passed to the supplied callback when data arrives on the socket. * Note: this will cause the streaming functionality to not provide any data, however events like 'error', 'end', and 'close' will * still be emitted as normal and methods like pause() and resume() will also behave as expected. */ onread?: OnReadOpts; } interface TcpSocketConnectOpts extends ConnectOpts { port: number; host?: string; localAddress?: string; localPort?: number; hints?: number; family?: number; lookup?: LookupFunction; } interface IpcSocketConnectOpts extends ConnectOpts { path: string; } type SocketConnectOpts = TcpSocketConnectOpts | IpcSocketConnectOpts; class Socket extends stream.Duplex { constructor(options?: SocketConstructorOpts); // Extended base methods write(buffer: Uint8Array | string, cb?: (err?: Error) => void): boolean; write(str: Uint8Array | string, encoding?: BufferEncoding, cb?: (err?: Error) => void): boolean; connect(options: SocketConnectOpts, connectionListener?: () => void): this; connect(port: number, host: string, connectionListener?: () => void): this; connect(port: number, connectionListener?: () => void): this; connect(path: string, connectionListener?: () => void): this; setEncoding(encoding?: BufferEncoding): this; pause(): this; resume(): this; setTimeout(timeout: number, callback?: () => void): this; setNoDelay(noDelay?: boolean): this; setKeepAlive(enable?: boolean, initialDelay?: number): this; address(): AddressInfo | {}; unref(): this; ref(): this; /** @deprecated since v14.6.0 - Use `writableLength` instead. */ readonly bufferSize: number; readonly bytesRead: number; readonly bytesWritten: number; readonly connecting: boolean; readonly destroyed: boolean; readonly localAddress: string; readonly localPort: number; readonly remoteAddress?: string; readonly remoteFamily?: string; readonly remotePort?: number; // Extended base methods end(cb?: () => void): void; end(buffer: Uint8Array | string, cb?: () => void): void; end(str: Uint8Array | string, encoding?: BufferEncoding, cb?: () => void): void; /** * events.EventEmitter * 1. close * 2. connect * 3. data * 4. drain * 5. end * 6. error * 7. lookup * 8. timeout */ addListener(event: string, listener: (...args: any[]) => void): this; addListener(event: "close", listener: (had_error: boolean) => void): this; addListener(event: "connect", listener: () => void): this; addListener(event: "data", listener: (data: Buffer) => void): this; addListener(event: "drain", listener: () => void): this; addListener(event: "end", listener: () => void): this; addListener(event: "error", listener: (err: Error) => void): this; addListener(event: "lookup", listener: (err: Error, address: string, family: string | number, host: string) => void): this; addListener(event: "timeout", listener: () => void): this; emit(event: string | symbol, ...args: any[]): boolean; emit(event: "close", had_error: boolean): boolean; emit(event: "connect"): boolean; emit(event: "data", data: Buffer): boolean; emit(event: "drain"): boolean; emit(event: "end"): boolean; emit(event: "error", err: Error): boolean; emit(event: "lookup", err: Error, address: string, family: string | number, host: string): boolean; emit(event: "timeout"): boolean; on(event: string, listener: (...args: any[]) => void): this; on(event: "close", listener: (had_error: boolean) => void): this; on(event: "connect", listener: () => void): this; on(event: "data", listener: (data: Buffer) => void): this; on(event: "drain", listener: () => void): this; on(event: "end", listener: () => void): this; on(event: "error", listener: (err: Error) => void): this; on(event: "lookup", listener: (err: Error, address: string, family: string | number, host: string) => void): this; on(event: "timeout", listener: () => void): this; once(event: string, listener: (...args: any[]) => void): this; once(event: "close", listener: (had_error: boolean) => void): this; once(event: "connect", listener: () => void): this; once(event: "data", listener: (data: Buffer) => void): this; once(event: "drain", listener: () => void): this; once(event: "end", listener: () => void): this; once(event: "error", listener: (err: Error) => void): this; once(event: "lookup", listener: (err: Error, address: string, family: string | number, host: string) => void): this; once(event: "timeout", listener: () => void): this; prependListener(event: string, listener: (...args: any[]) => void): this; prependListener(event: "close", listener: (had_error: boolean) => void): this; prependListener(event: "connect", listener: () => void): this; prependListener(event: "data", listener: (data: Buffer) => void): this; prependListener(event: "drain", listener: () => void): this; prependListener(event: "end", listener: () => void): this; prependListener(event: "error", listener: (err: Error) => void): this; prependListener(event: "lookup", listener: (err: Error, address: string, family: string | number, host: string) => void): this; prependListener(event: "timeout", listener: () => void): this; prependOnceListener(event: string, listener: (...args: any[]) => void): this; prependOnceListener(event: "close", listener: (had_error: boolean) => void): this; prependOnceListener(event: "connect", listener: () => void): this; prependOnceListener(event: "data", listener: (data: Buffer) => void): this; prependOnceListener(event: "drain", listener: () => void): this; prependOnceListener(event: "end", listener: () => void): this; prependOnceListener(event: "error", listener: (err: Error) => void): this; prependOnceListener(event: "lookup", listener: (err: Error, address: string, family: string | number, host: string) => void): this; prependOnceListener(event: "timeout", listener: () => void): this; } interface ListenOptions { port?: number; host?: string; backlog?: number; path?: string; exclusive?: boolean; readableAll?: boolean; writableAll?: boolean; /** * @default false */ ipv6Only?: boolean; } // https://github.com/nodejs/node/blob/master/lib/net.js class Server extends events.EventEmitter { constructor(connectionListener?: (socket: Socket) => void); constructor(options?: { allowHalfOpen?: boolean, pauseOnConnect?: boolean }, connectionListener?: (socket: Socket) => void); listen(port?: number, hostname?: string, backlog?: number, listeningListener?: () => void): this; listen(port?: number, hostname?: string, listeningListener?: () => void): this; listen(port?: number, backlog?: number, listeningListener?: () => void): this; listen(port?: number, listeningListener?: () => void): this; listen(path: string, backlog?: number, listeningListener?: () => void): this; listen(path: string, listeningListener?: () => void): this; listen(options: ListenOptions, listeningListener?: () => void): this; listen(handle: any, backlog?: number, listeningListener?: () => void): this; listen(handle: any, listeningListener?: () => void): this; close(callback?: (err?: Error) => void): this; address(): AddressInfo | string | null; getConnections(cb: (error: Error | null, count: number) => void): void; ref(): this; unref(): this; maxConnections: number; connections: number; listening: boolean; /** * events.EventEmitter * 1. close * 2. connection * 3. error * 4. listening */ addListener(event: string, listener: (...args: any[]) => void): this; addListener(event: "close", listener: () => void): this; addListener(event: "connection", listener: (socket: Socket) => void): this; addListener(event: "error", listener: (err: Error) => void): this; addListener(event: "listening", listener: () => void): this; emit(event: string | symbol, ...args: any[]): boolean; emit(event: "close"): boolean; emit(event: "connection", socket: Socket): boolean; emit(event: "error", err: Error): boolean; emit(event: "listening"): boolean; on(event: string, listener: (...args: any[]) => void): this; on(event: "close", listener: () => void): this; on(event: "connection", listener: (socket: Socket) => void): this; on(event: "error", listener: (err: Error) => void): this; on(event: "listening", listener: () => void): this; once(event: string, listener: (...args: any[]) => void): this; once(event: "close", listener: () => void): this; once(event: "connection", listener: (socket: Socket) => void): this; once(event: "error", listener: (err: Error) => void): this; once(event: "listening", listener: () => void): this; prependListener(event: string, listener: (...args: any[]) => void): this; prependListener(event: "close", listener: () => void): this; prependListener(event: "connection", listener: (socket: Socket) => void): this; prependListener(event: "error", listener: (err: Error) => void): this; prependListener(event: "listening", listener: () => void): this; prependOnceListener(event: string, listener: (...args: any[]) => void): this; prependOnceListener(event: "close", listener: () => void): this; prependOnceListener(event: "connection", listener: (socket: Socket) => void): this; prependOnceListener(event: "error", listener: (err: Error) => void): this; prependOnceListener(event: "listening", listener: () => void): this; } interface TcpNetConnectOpts extends TcpSocketConnectOpts, SocketConstructorOpts { timeout?: number; } interface IpcNetConnectOpts extends IpcSocketConnectOpts, SocketConstructorOpts { timeout?: number; } type NetConnectOpts = TcpNetConnectOpts | IpcNetConnectOpts; function createServer(connectionListener?: (socket: Socket) => void): Server; function createServer(options?: { allowHalfOpen?: boolean, pauseOnConnect?: boolean }, connectionListener?: (socket: Socket) => void): Server; function connect(options: NetConnectOpts, connectionListener?: () => void): Socket; function connect(port: number, host?: string, connectionListener?: () => void): Socket; function connect(path: string, connectionListener?: () => void): Socket; function createConnection(options: NetConnectOpts, connectionListener?: () => void): Socket; function createConnection(port: number, host?: string, connectionListener?: () => void): Socket; function createConnection(path: string, connectionListener?: () => void): Socket; function isIP(input: string): number; function isIPv4(input: string): boolean; function isIPv6(input: string): boolean; } apollo-server-demo/node_modules/@types/node/timers.d.ts 0000644 0001750 0000144 00000001472 14000133701 022706 0 ustar andreh users declare module "timers" { function setTimeout(callback: (...args: any[]) => void, ms: number, ...args: any[]): NodeJS.Timeout; namespace setTimeout { function __promisify__(ms: number): Promise<void>; function __promisify__<T>(ms: number, value: T): Promise<T>; } function clearTimeout(timeoutId: NodeJS.Timeout): void; function setInterval(callback: (...args: any[]) => void, ms: number, ...args: any[]): NodeJS.Timeout; function clearInterval(intervalId: NodeJS.Timeout): void; function setImmediate(callback: (...args: any[]) => void, ...args: any[]): NodeJS.Immediate; namespace setImmediate { function __promisify__(): Promise<void>; function __promisify__<T>(value: T): Promise<T>; } function clearImmediate(immediateId: NodeJS.Immediate): void; } apollo-server-demo/node_modules/@types/node/globals.d.ts 0000644 0001750 0000144 00000056225 14000133700 023033 0 ustar andreh users // Declare "static" methods in Error interface ErrorConstructor { /** Create .stack property on a target object */ captureStackTrace(targetObject: object, constructorOpt?: Function): void; /** * Optional override for formatting stack traces * * @see https://github.com/v8/v8/wiki/Stack%20Trace%20API#customizing-stack-traces */ prepareStackTrace?: (err: Error, stackTraces: NodeJS.CallSite[]) => any; stackTraceLimit: number; } // Node.js ESNEXT support interface String { /** Removes whitespace from the left end of a string. */ trimLeft(): string; /** Removes whitespace from the right end of a string. */ trimRight(): string; /** Returns a copy with leading whitespace removed. */ trimStart(): string; /** Returns a copy with trailing whitespace removed. */ trimEnd(): string; } interface ImportMeta { url: string; } /*-----------------------------------------------* * * * GLOBAL * * * ------------------------------------------------*/ // For backwards compability interface NodeRequire extends NodeJS.Require {} interface RequireResolve extends NodeJS.RequireResolve {} interface NodeModule extends NodeJS.Module {} declare var process: NodeJS.Process; declare var console: Console; declare var __filename: string; declare var __dirname: string; declare function setTimeout(callback: (...args: any[]) => void, ms: number, ...args: any[]): NodeJS.Timeout; declare namespace setTimeout { function __promisify__(ms: number): Promise<void>; function __promisify__<T>(ms: number, value: T): Promise<T>; } declare function clearTimeout(timeoutId: NodeJS.Timeout): void; declare function setInterval(callback: (...args: any[]) => void, ms: number, ...args: any[]): NodeJS.Timeout; declare function clearInterval(intervalId: NodeJS.Timeout): void; declare function setImmediate(callback: (...args: any[]) => void, ...args: any[]): NodeJS.Immediate; declare namespace setImmediate { function __promisify__(): Promise<void>; function __promisify__<T>(value: T): Promise<T>; } declare function clearImmediate(immediateId: NodeJS.Immediate): void; declare function queueMicrotask(callback: () => void): void; declare var require: NodeRequire; declare var module: NodeModule; // Same as module.exports declare var exports: any; // Buffer class type BufferEncoding = "ascii" | "utf8" | "utf-8" | "utf16le" | "ucs2" | "ucs-2" | "base64" | "latin1" | "binary" | "hex"; type WithImplicitCoercion<T> = T | { valueOf(): T }; /** * Raw data is stored in instances of the Buffer class. * A Buffer is similar to an array of integers but corresponds to a raw memory allocation outside the V8 heap. A Buffer cannot be resized. * Valid string encodings: 'ascii'|'utf8'|'utf16le'|'ucs2'(alias of 'utf16le')|'base64'|'binary'(deprecated)|'hex' */ declare class Buffer extends Uint8Array { /** * Allocates a new buffer containing the given {str}. * * @param str String to store in buffer. * @param encoding encoding to use, optional. Default is 'utf8' * @deprecated since v10.0.0 - Use `Buffer.from(string[, encoding])` instead. */ constructor(str: string, encoding?: BufferEncoding); /** * Allocates a new buffer of {size} octets. * * @param size count of octets to allocate. * @deprecated since v10.0.0 - Use `Buffer.alloc()` instead (also see `Buffer.allocUnsafe()`). */ constructor(size: number); /** * Allocates a new buffer containing the given {array} of octets. * * @param array The octets to store. * @deprecated since v10.0.0 - Use `Buffer.from(array)` instead. */ constructor(array: Uint8Array); /** * Produces a Buffer backed by the same allocated memory as * the given {ArrayBuffer}/{SharedArrayBuffer}. * * * @param arrayBuffer The ArrayBuffer with which to share memory. * @deprecated since v10.0.0 - Use `Buffer.from(arrayBuffer[, byteOffset[, length]])` instead. */ constructor(arrayBuffer: ArrayBuffer | SharedArrayBuffer); /** * Allocates a new buffer containing the given {array} of octets. * * @param array The octets to store. * @deprecated since v10.0.0 - Use `Buffer.from(array)` instead. */ constructor(array: ReadonlyArray<any>); /** * Copies the passed {buffer} data onto a new {Buffer} instance. * * @param buffer The buffer to copy. * @deprecated since v10.0.0 - Use `Buffer.from(buffer)` instead. */ constructor(buffer: Buffer); /** * When passed a reference to the .buffer property of a TypedArray instance, * the newly created Buffer will share the same allocated memory as the TypedArray. * The optional {byteOffset} and {length} arguments specify a memory range * within the {arrayBuffer} that will be shared by the Buffer. * * @param arrayBuffer The .buffer property of any TypedArray or a new ArrayBuffer() */ static from(arrayBuffer: WithImplicitCoercion<ArrayBuffer | SharedArrayBuffer>, byteOffset?: number, length?: number): Buffer; /** * Creates a new Buffer using the passed {data} * @param data data to create a new Buffer */ static from(data: Uint8Array | ReadonlyArray<number>): Buffer; static from(data: WithImplicitCoercion<Uint8Array | ReadonlyArray<number> | string>): Buffer; /** * Creates a new Buffer containing the given JavaScript string {str}. * If provided, the {encoding} parameter identifies the character encoding. * If not provided, {encoding} defaults to 'utf8'. */ static from(str: WithImplicitCoercion<string> | { [Symbol.toPrimitive](hint: 'string'): string }, encoding?: BufferEncoding): Buffer; /** * Creates a new Buffer using the passed {data} * @param values to create a new Buffer */ static of(...items: number[]): Buffer; /** * Returns true if {obj} is a Buffer * * @param obj object to test. */ static isBuffer(obj: any): obj is Buffer; /** * Returns true if {encoding} is a valid encoding argument. * Valid string encodings in Node 0.12: 'ascii'|'utf8'|'utf16le'|'ucs2'(alias of 'utf16le')|'base64'|'binary'(deprecated)|'hex' * * @param encoding string to test. */ static isEncoding(encoding: string): encoding is BufferEncoding; /** * Gives the actual byte length of a string. encoding defaults to 'utf8'. * This is not the same as String.prototype.length since that returns the number of characters in a string. * * @param string string to test. * @param encoding encoding used to evaluate (defaults to 'utf8') */ static byteLength( string: string | NodeJS.ArrayBufferView | ArrayBuffer | SharedArrayBuffer, encoding?: BufferEncoding ): number; /** * Returns a buffer which is the result of concatenating all the buffers in the list together. * * If the list has no items, or if the totalLength is 0, then it returns a zero-length buffer. * If the list has exactly one item, then the first item of the list is returned. * If the list has more than one item, then a new Buffer is created. * * @param list An array of Buffer objects to concatenate * @param totalLength Total length of the buffers when concatenated. * If totalLength is not provided, it is read from the buffers in the list. However, this adds an additional loop to the function, so it is faster to provide the length explicitly. */ static concat(list: ReadonlyArray<Uint8Array>, totalLength?: number): Buffer; /** * The same as buf1.compare(buf2). */ static compare(buf1: Uint8Array, buf2: Uint8Array): number; /** * Allocates a new buffer of {size} octets. * * @param size count of octets to allocate. * @param fill if specified, buffer will be initialized by calling buf.fill(fill). * If parameter is omitted, buffer will be filled with zeros. * @param encoding encoding used for call to buf.fill while initalizing */ static alloc(size: number, fill?: string | Buffer | number, encoding?: BufferEncoding): Buffer; /** * Allocates a new buffer of {size} octets, leaving memory not initialized, so the contents * of the newly created Buffer are unknown and may contain sensitive data. * * @param size count of octets to allocate */ static allocUnsafe(size: number): Buffer; /** * Allocates a new non-pooled buffer of {size} octets, leaving memory not initialized, so the contents * of the newly created Buffer are unknown and may contain sensitive data. * * @param size count of octets to allocate */ static allocUnsafeSlow(size: number): Buffer; /** * This is the number of bytes used to determine the size of pre-allocated, internal Buffer instances used for pooling. This value may be modified. */ static poolSize: number; write(string: string, encoding?: BufferEncoding): number; write(string: string, offset: number, encoding?: BufferEncoding): number; write(string: string, offset: number, length: number, encoding?: BufferEncoding): number; toString(encoding?: BufferEncoding, start?: number, end?: number): string; toJSON(): { type: 'Buffer'; data: number[] }; equals(otherBuffer: Uint8Array): boolean; compare( otherBuffer: Uint8Array, targetStart?: number, targetEnd?: number, sourceStart?: number, sourceEnd?: number ): number; copy(targetBuffer: Uint8Array, targetStart?: number, sourceStart?: number, sourceEnd?: number): number; /** * Returns a new `Buffer` that references **the same memory as the original**, but offset and cropped by the start and end indices. * * This method is incompatible with `Uint8Array#slice()`, which returns a copy of the original memory. * * @param begin Where the new `Buffer` will start. Default: `0`. * @param end Where the new `Buffer` will end (not inclusive). Default: `buf.length`. */ slice(begin?: number, end?: number): Buffer; /** * Returns a new `Buffer` that references **the same memory as the original**, but offset and cropped by the start and end indices. * * This method is compatible with `Uint8Array#subarray()`. * * @param begin Where the new `Buffer` will start. Default: `0`. * @param end Where the new `Buffer` will end (not inclusive). Default: `buf.length`. */ subarray(begin?: number, end?: number): Buffer; writeBigInt64BE(value: bigint, offset?: number): number; writeBigInt64LE(value: bigint, offset?: number): number; writeBigUInt64BE(value: bigint, offset?: number): number; writeBigUInt64LE(value: bigint, offset?: number): number; writeUIntLE(value: number, offset: number, byteLength: number): number; writeUIntBE(value: number, offset: number, byteLength: number): number; writeIntLE(value: number, offset: number, byteLength: number): number; writeIntBE(value: number, offset: number, byteLength: number): number; readBigUInt64BE(offset?: number): bigint; readBigUInt64LE(offset?: number): bigint; readBigInt64BE(offset?: number): bigint; readBigInt64LE(offset?: number): bigint; readUIntLE(offset: number, byteLength: number): number; readUIntBE(offset: number, byteLength: number): number; readIntLE(offset: number, byteLength: number): number; readIntBE(offset: number, byteLength: number): number; readUInt8(offset?: number): number; readUInt16LE(offset?: number): number; readUInt16BE(offset?: number): number; readUInt32LE(offset?: number): number; readUInt32BE(offset?: number): number; readInt8(offset?: number): number; readInt16LE(offset?: number): number; readInt16BE(offset?: number): number; readInt32LE(offset?: number): number; readInt32BE(offset?: number): number; readFloatLE(offset?: number): number; readFloatBE(offset?: number): number; readDoubleLE(offset?: number): number; readDoubleBE(offset?: number): number; reverse(): this; swap16(): Buffer; swap32(): Buffer; swap64(): Buffer; writeUInt8(value: number, offset?: number): number; writeUInt16LE(value: number, offset?: number): number; writeUInt16BE(value: number, offset?: number): number; writeUInt32LE(value: number, offset?: number): number; writeUInt32BE(value: number, offset?: number): number; writeInt8(value: number, offset?: number): number; writeInt16LE(value: number, offset?: number): number; writeInt16BE(value: number, offset?: number): number; writeInt32LE(value: number, offset?: number): number; writeInt32BE(value: number, offset?: number): number; writeFloatLE(value: number, offset?: number): number; writeFloatBE(value: number, offset?: number): number; writeDoubleLE(value: number, offset?: number): number; writeDoubleBE(value: number, offset?: number): number; fill(value: string | Uint8Array | number, offset?: number, end?: number, encoding?: BufferEncoding): this; indexOf(value: string | number | Uint8Array, byteOffset?: number, encoding?: BufferEncoding): number; lastIndexOf(value: string | number | Uint8Array, byteOffset?: number, encoding?: BufferEncoding): number; entries(): IterableIterator<[number, number]>; includes(value: string | number | Buffer, byteOffset?: number, encoding?: BufferEncoding): boolean; keys(): IterableIterator<number>; values(): IterableIterator<number>; } /*----------------------------------------------* * * * GLOBAL INTERFACES * * * *-----------------------------------------------*/ declare namespace NodeJS { interface InspectOptions { /** * If set to `true`, getters are going to be * inspected as well. If set to `'get'` only getters without setter are going * to be inspected. If set to `'set'` only getters having a corresponding * setter are going to be inspected. This might cause side effects depending on * the getter function. * @default `false` */ getters?: 'get' | 'set' | boolean; showHidden?: boolean; /** * @default 2 */ depth?: number | null; colors?: boolean; customInspect?: boolean; showProxy?: boolean; maxArrayLength?: number | null; /** * Specifies the maximum number of characters to * include when formatting. Set to `null` or `Infinity` to show all elements. * Set to `0` or negative to show no characters. * @default Infinity */ maxStringLength?: number | null; breakLength?: number; /** * Setting this to `false` causes each object key * to be displayed on a new line. It will also add new lines to text that is * longer than `breakLength`. If set to a number, the most `n` inner elements * are united on a single line as long as all properties fit into * `breakLength`. Short array elements are also grouped together. Note that no * text will be reduced below 16 characters, no matter the `breakLength` size. * For more information, see the example below. * @default `true` */ compact?: boolean | number; sorted?: boolean | ((a: string, b: string) => number); } interface CallSite { /** * Value of "this" */ getThis(): any; /** * Type of "this" as a string. * This is the name of the function stored in the constructor field of * "this", if available. Otherwise the object's [[Class]] internal * property. */ getTypeName(): string | null; /** * Current function */ getFunction(): Function | undefined; /** * Name of the current function, typically its name property. * If a name property is not available an attempt will be made to try * to infer a name from the function's context. */ getFunctionName(): string | null; /** * Name of the property [of "this" or one of its prototypes] that holds * the current function */ getMethodName(): string | null; /** * Name of the script [if this function was defined in a script] */ getFileName(): string | null; /** * Current line number [if this function was defined in a script] */ getLineNumber(): number | null; /** * Current column number [if this function was defined in a script] */ getColumnNumber(): number | null; /** * A call site object representing the location where eval was called * [if this function was created using a call to eval] */ getEvalOrigin(): string | undefined; /** * Is this a toplevel invocation, that is, is "this" the global object? */ isToplevel(): boolean; /** * Does this call take place in code defined by a call to eval? */ isEval(): boolean; /** * Is this call in native V8 code? */ isNative(): boolean; /** * Is this a constructor call? */ isConstructor(): boolean; } interface ErrnoException extends Error { errno?: number; code?: string; path?: string; syscall?: string; stack?: string; } interface ReadableStream extends EventEmitter { readable: boolean; read(size?: number): string | Buffer; setEncoding(encoding: BufferEncoding): this; pause(): this; resume(): this; isPaused(): boolean; pipe<T extends WritableStream>(destination: T, options?: { end?: boolean; }): T; unpipe(destination?: WritableStream): this; unshift(chunk: string | Uint8Array, encoding?: BufferEncoding): void; wrap(oldStream: ReadableStream): this; [Symbol.asyncIterator](): AsyncIterableIterator<string | Buffer>; } interface WritableStream extends EventEmitter { writable: boolean; write(buffer: Uint8Array | string, cb?: (err?: Error | null) => void): boolean; write(str: string, encoding?: BufferEncoding, cb?: (err?: Error | null) => void): boolean; end(cb?: () => void): void; end(data: string | Uint8Array, cb?: () => void): void; end(str: string, encoding?: BufferEncoding, cb?: () => void): void; } interface ReadWriteStream extends ReadableStream, WritableStream { } interface Global { Array: typeof Array; ArrayBuffer: typeof ArrayBuffer; Boolean: typeof Boolean; Buffer: typeof Buffer; DataView: typeof DataView; Date: typeof Date; Error: typeof Error; EvalError: typeof EvalError; Float32Array: typeof Float32Array; Float64Array: typeof Float64Array; Function: typeof Function; Infinity: typeof Infinity; Int16Array: typeof Int16Array; Int32Array: typeof Int32Array; Int8Array: typeof Int8Array; Intl: typeof Intl; JSON: typeof JSON; Map: MapConstructor; Math: typeof Math; NaN: typeof NaN; Number: typeof Number; Object: typeof Object; Promise: typeof Promise; RangeError: typeof RangeError; ReferenceError: typeof ReferenceError; RegExp: typeof RegExp; Set: SetConstructor; String: typeof String; Symbol: Function; SyntaxError: typeof SyntaxError; TypeError: typeof TypeError; URIError: typeof URIError; Uint16Array: typeof Uint16Array; Uint32Array: typeof Uint32Array; Uint8Array: typeof Uint8Array; Uint8ClampedArray: typeof Uint8ClampedArray; WeakMap: WeakMapConstructor; WeakSet: WeakSetConstructor; clearImmediate: (immediateId: Immediate) => void; clearInterval: (intervalId: Timeout) => void; clearTimeout: (timeoutId: Timeout) => void; decodeURI: typeof decodeURI; decodeURIComponent: typeof decodeURIComponent; encodeURI: typeof encodeURI; encodeURIComponent: typeof encodeURIComponent; escape: (str: string) => string; eval: typeof eval; global: Global; isFinite: typeof isFinite; isNaN: typeof isNaN; parseFloat: typeof parseFloat; parseInt: typeof parseInt; setImmediate: (callback: (...args: any[]) => void, ...args: any[]) => Immediate; setInterval: (callback: (...args: any[]) => void, ms: number, ...args: any[]) => Timeout; setTimeout: (callback: (...args: any[]) => void, ms: number, ...args: any[]) => Timeout; queueMicrotask: typeof queueMicrotask; undefined: typeof undefined; unescape: (str: string) => string; gc: () => void; v8debug?: any; } interface RefCounted { ref(): this; unref(): this; } // compatibility with older typings interface Timer extends RefCounted { hasRef(): boolean; refresh(): this; [Symbol.toPrimitive](): number; } interface Immediate extends RefCounted { hasRef(): boolean; _onImmediate: Function; // to distinguish it from the Timeout class } interface Timeout extends Timer { hasRef(): boolean; refresh(): this; [Symbol.toPrimitive](): number; } type TypedArray = | Uint8Array | Uint8ClampedArray | Uint16Array | Uint32Array | Int8Array | Int16Array | Int32Array | BigUint64Array | BigInt64Array | Float32Array | Float64Array; type ArrayBufferView = TypedArray | DataView; interface Require { (id: string): any; resolve: RequireResolve; cache: Dict<NodeModule>; /** * @deprecated */ extensions: RequireExtensions; main: Module | undefined; } interface RequireResolve { (id: string, options?: { paths?: string[]; }): string; paths(request: string): string[] | null; } interface RequireExtensions extends Dict<(m: Module, filename: string) => any> { '.js': (m: Module, filename: string) => any; '.json': (m: Module, filename: string) => any; '.node': (m: Module, filename: string) => any; } interface Module { exports: any; require: Require; id: string; filename: string; loaded: boolean; /** @deprecated since 14.6.0 Please use `require.main` and `module.children` instead. */ parent: Module | null | undefined; children: Module[]; /** * @since 11.14.0 * * The directory name of the module. This is usually the same as the path.dirname() of the module.id. */ path: string; paths: string[]; } interface Dict<T> { [key: string]: T | undefined; } interface ReadOnlyDict<T> { readonly [key: string]: T | undefined; } } apollo-server-demo/node_modules/@types/node/vm.d.ts 0000644 0001750 0000144 00000013414 14000133701 022024 0 ustar andreh users declare module "vm" { interface Context extends NodeJS.Dict<any> { } interface BaseOptions { /** * Specifies the filename used in stack traces produced by this script. * Default: `''`. */ filename?: string; /** * Specifies the line number offset that is displayed in stack traces produced by this script. * Default: `0`. */ lineOffset?: number; /** * Specifies the column number offset that is displayed in stack traces produced by this script. * Default: `0` */ columnOffset?: number; } interface ScriptOptions extends BaseOptions { displayErrors?: boolean; timeout?: number; cachedData?: Buffer; produceCachedData?: boolean; } interface RunningScriptOptions extends BaseOptions { /** * When `true`, if an `Error` occurs while compiling the `code`, the line of code causing the error is attached to the stack trace. * Default: `true`. */ displayErrors?: boolean; /** * Specifies the number of milliseconds to execute code before terminating execution. * If execution is terminated, an `Error` will be thrown. This value must be a strictly positive integer. */ timeout?: number; /** * If `true`, the execution will be terminated when `SIGINT` (Ctrl+C) is received. * Existing handlers for the event that have been attached via `process.on('SIGINT')` will be disabled during script execution, but will continue to work after that. * If execution is terminated, an `Error` will be thrown. * Default: `false`. */ breakOnSigint?: boolean; /** * If set to `afterEvaluate`, microtasks will be run immediately after the script has run. */ microtaskMode?: 'afterEvaluate'; } interface CompileFunctionOptions extends BaseOptions { /** * Provides an optional data with V8's code cache data for the supplied source. */ cachedData?: Buffer; /** * Specifies whether to produce new cache data. * Default: `false`, */ produceCachedData?: boolean; /** * The sandbox/context in which the said function should be compiled in. */ parsingContext?: Context; /** * An array containing a collection of context extensions (objects wrapping the current scope) to be applied while compiling */ contextExtensions?: Object[]; } interface CreateContextOptions { /** * Human-readable name of the newly created context. * @default 'VM Context i' Where i is an ascending numerical index of the created context. */ name?: string; /** * Corresponds to the newly created context for display purposes. * The origin should be formatted like a `URL`, but with only the scheme, host, and port (if necessary), * like the value of the `url.origin` property of a URL object. * Most notably, this string should omit the trailing slash, as that denotes a path. * @default '' */ origin?: string; codeGeneration?: { /** * If set to false any calls to eval or function constructors (Function, GeneratorFunction, etc) * will throw an EvalError. * @default true */ strings?: boolean; /** * If set to false any attempt to compile a WebAssembly module will throw a WebAssembly.CompileError. * @default true */ wasm?: boolean; }; } type MeasureMemoryMode = 'summary' | 'detailed'; interface MeasureMemoryOptions { /** * @default 'summary' */ mode?: MeasureMemoryMode; context?: Context; } interface MemoryMeasurement { total: { jsMemoryEstimate: number; jsMemoryRange: [number, number]; }; } class Script { constructor(code: string, options?: ScriptOptions); runInContext(contextifiedSandbox: Context, options?: RunningScriptOptions): any; runInNewContext(sandbox?: Context, options?: RunningScriptOptions): any; runInThisContext(options?: RunningScriptOptions): any; createCachedData(): Buffer; } function createContext(sandbox?: Context, options?: CreateContextOptions): Context; function isContext(sandbox: Context): boolean; function runInContext(code: string, contextifiedSandbox: Context, options?: RunningScriptOptions | string): any; function runInNewContext(code: string, sandbox?: Context, options?: RunningScriptOptions | string): any; function runInThisContext(code: string, options?: RunningScriptOptions | string): any; function compileFunction(code: string, params?: ReadonlyArray<string>, options?: CompileFunctionOptions): Function; /** * Measure the memory known to V8 and used by the current execution context or a specified context. * * The format of the object that the returned Promise may resolve with is * specific to the V8 engine and may change from one version of V8 to the next. * * The returned result is different from the statistics returned by * `v8.getHeapSpaceStatistics()` in that `vm.measureMemory()` measures * the memory reachable by V8 from a specific context, while * `v8.getHeapSpaceStatistics()` measures the memory used by an instance * of V8 engine, which can switch among multiple contexts that reference * objects in the heap of one engine. * * @experimental */ function measureMemory(options?: MeasureMemoryOptions): Promise<MemoryMeasurement>; } apollo-server-demo/node_modules/@types/node/assert.d.ts 0000644 0001750 0000144 00000012733 14000133700 022705 0 ustar andreh users declare module 'assert' { /** An alias of `assert.ok()`. */ function assert(value: any, message?: string | Error): asserts value; namespace assert { class AssertionError extends Error { actual: any; expected: any; operator: string; generatedMessage: boolean; code: 'ERR_ASSERTION'; constructor(options?: { /** If provided, the error message is set to this value. */ message?: string; /** The `actual` property on the error instance. */ actual?: any; /** The `expected` property on the error instance. */ expected?: any; /** The `operator` property on the error instance. */ operator?: string; /** If provided, the generated stack trace omits frames before this function. */ // tslint:disable-next-line:ban-types stackStartFn?: Function; }); } class CallTracker { calls(exact?: number): () => void; calls<Func extends (...args: any[]) => any>(fn?: Func, exact?: number): Func; report(): CallTrackerReportInformation[]; verify(): void; } interface CallTrackerReportInformation { message: string; /** The actual number of times the function was called. */ actual: number; /** The number of times the function was expected to be called. */ expected: number; /** The name of the function that is wrapped. */ operator: string; /** A stack trace of the function. */ stack: object; } type AssertPredicate = RegExp | (new () => object) | ((thrown: any) => boolean) | object | Error; function fail(message?: string | Error): never; /** @deprecated since v10.0.0 - use fail([message]) or other assert functions instead. */ function fail( actual: any, expected: any, message?: string | Error, operator?: string, // tslint:disable-next-line:ban-types stackStartFn?: Function, ): never; function ok(value: any, message?: string | Error): asserts value; /** @deprecated since v9.9.0 - use strictEqual() instead. */ function equal(actual: any, expected: any, message?: string | Error): void; /** @deprecated since v9.9.0 - use notStrictEqual() instead. */ function notEqual(actual: any, expected: any, message?: string | Error): void; /** @deprecated since v9.9.0 - use deepStrictEqual() instead. */ function deepEqual(actual: any, expected: any, message?: string | Error): void; /** @deprecated since v9.9.0 - use notDeepStrictEqual() instead. */ function notDeepEqual(actual: any, expected: any, message?: string | Error): void; function strictEqual<T>(actual: any, expected: T, message?: string | Error): asserts actual is T; function notStrictEqual(actual: any, expected: any, message?: string | Error): void; function deepStrictEqual<T>(actual: any, expected: T, message?: string | Error): asserts actual is T; function notDeepStrictEqual(actual: any, expected: any, message?: string | Error): void; function throws(block: () => any, message?: string | Error): void; function throws(block: () => any, error: AssertPredicate, message?: string | Error): void; function doesNotThrow(block: () => any, message?: string | Error): void; function doesNotThrow(block: () => any, error: AssertPredicate, message?: string | Error): void; function ifError(value: any): asserts value is null | undefined; function rejects(block: (() => Promise<any>) | Promise<any>, message?: string | Error): Promise<void>; function rejects( block: (() => Promise<any>) | Promise<any>, error: AssertPredicate, message?: string | Error, ): Promise<void>; function doesNotReject(block: (() => Promise<any>) | Promise<any>, message?: string | Error): Promise<void>; function doesNotReject( block: (() => Promise<any>) | Promise<any>, error: AssertPredicate, message?: string | Error, ): Promise<void>; function match(value: string, regExp: RegExp, message?: string | Error): void; function doesNotMatch(value: string, regExp: RegExp, message?: string | Error): void; const strict: Omit< typeof assert, | 'equal' | 'notEqual' | 'deepEqual' | 'notDeepEqual' | 'ok' | 'strictEqual' | 'deepStrictEqual' | 'ifError' | 'strict' > & { (value: any, message?: string | Error): asserts value; equal: typeof strictEqual; notEqual: typeof notStrictEqual; deepEqual: typeof deepStrictEqual; notDeepEqual: typeof notDeepStrictEqual; // Mapped types and assertion functions are incompatible? // TS2775: Assertions require every name in the call target // to be declared with an explicit type annotation. ok: typeof ok; strictEqual: typeof strictEqual; deepStrictEqual: typeof deepStrictEqual; ifError: typeof ifError; strict: typeof strict; }; } export = assert; } apollo-server-demo/node_modules/@types/node/readline.d.ts 0000644 0001750 0000144 00000016242 14000133700 023166 0 ustar andreh users declare module "readline" { import * as events from "events"; import * as stream from "stream"; interface Key { sequence?: string; name?: string; ctrl?: boolean; meta?: boolean; shift?: boolean; } class Interface extends events.EventEmitter { readonly terminal: boolean; // Need direct access to line/cursor data, for use in external processes // see: https://github.com/nodejs/node/issues/30347 /** The current input data */ readonly line: string; /** The current cursor position in the input line */ readonly cursor: number; /** * NOTE: According to the documentation: * * > Instances of the `readline.Interface` class are constructed using the * > `readline.createInterface()` method. * * @see https://nodejs.org/dist/latest-v10.x/docs/api/readline.html#readline_class_interface */ protected constructor(input: NodeJS.ReadableStream, output?: NodeJS.WritableStream, completer?: Completer | AsyncCompleter, terminal?: boolean); /** * NOTE: According to the documentation: * * > Instances of the `readline.Interface` class are constructed using the * > `readline.createInterface()` method. * * @see https://nodejs.org/dist/latest-v10.x/docs/api/readline.html#readline_class_interface */ protected constructor(options: ReadLineOptions); setPrompt(prompt: string): void; prompt(preserveCursor?: boolean): void; question(query: string, callback: (answer: string) => void): void; pause(): this; resume(): this; close(): void; write(data: string | Buffer, key?: Key): void; /** * Returns the real position of the cursor in relation to the input * prompt + string. Long input (wrapping) strings, as well as multiple * line prompts are included in the calculations. */ getCursorPos(): CursorPos; /** * events.EventEmitter * 1. close * 2. line * 3. pause * 4. resume * 5. SIGCONT * 6. SIGINT * 7. SIGTSTP */ addListener(event: string, listener: (...args: any[]) => void): this; addListener(event: "close", listener: () => void): this; addListener(event: "line", listener: (input: string) => void): this; addListener(event: "pause", listener: () => void): this; addListener(event: "resume", listener: () => void): this; addListener(event: "SIGCONT", listener: () => void): this; addListener(event: "SIGINT", listener: () => void): this; addListener(event: "SIGTSTP", listener: () => void): this; emit(event: string | symbol, ...args: any[]): boolean; emit(event: "close"): boolean; emit(event: "line", input: string): boolean; emit(event: "pause"): boolean; emit(event: "resume"): boolean; emit(event: "SIGCONT"): boolean; emit(event: "SIGINT"): boolean; emit(event: "SIGTSTP"): boolean; on(event: string, listener: (...args: any[]) => void): this; on(event: "close", listener: () => void): this; on(event: "line", listener: (input: string) => void): this; on(event: "pause", listener: () => void): this; on(event: "resume", listener: () => void): this; on(event: "SIGCONT", listener: () => void): this; on(event: "SIGINT", listener: () => void): this; on(event: "SIGTSTP", listener: () => void): this; once(event: string, listener: (...args: any[]) => void): this; once(event: "close", listener: () => void): this; once(event: "line", listener: (input: string) => void): this; once(event: "pause", listener: () => void): this; once(event: "resume", listener: () => void): this; once(event: "SIGCONT", listener: () => void): this; once(event: "SIGINT", listener: () => void): this; once(event: "SIGTSTP", listener: () => void): this; prependListener(event: string, listener: (...args: any[]) => void): this; prependListener(event: "close", listener: () => void): this; prependListener(event: "line", listener: (input: string) => void): this; prependListener(event: "pause", listener: () => void): this; prependListener(event: "resume", listener: () => void): this; prependListener(event: "SIGCONT", listener: () => void): this; prependListener(event: "SIGINT", listener: () => void): this; prependListener(event: "SIGTSTP", listener: () => void): this; prependOnceListener(event: string, listener: (...args: any[]) => void): this; prependOnceListener(event: "close", listener: () => void): this; prependOnceListener(event: "line", listener: (input: string) => void): this; prependOnceListener(event: "pause", listener: () => void): this; prependOnceListener(event: "resume", listener: () => void): this; prependOnceListener(event: "SIGCONT", listener: () => void): this; prependOnceListener(event: "SIGINT", listener: () => void): this; prependOnceListener(event: "SIGTSTP", listener: () => void): this; [Symbol.asyncIterator](): AsyncIterableIterator<string>; } type ReadLine = Interface; // type forwarded for backwards compatiblity type Completer = (line: string) => CompleterResult; type AsyncCompleter = (line: string, callback: (err?: null | Error, result?: CompleterResult) => void) => any; type CompleterResult = [string[], string]; interface ReadLineOptions { input: NodeJS.ReadableStream; output?: NodeJS.WritableStream; completer?: Completer | AsyncCompleter; terminal?: boolean; historySize?: number; prompt?: string; crlfDelay?: number; removeHistoryDuplicates?: boolean; escapeCodeTimeout?: number; tabSize?: number; } function createInterface(input: NodeJS.ReadableStream, output?: NodeJS.WritableStream, completer?: Completer | AsyncCompleter, terminal?: boolean): Interface; function createInterface(options: ReadLineOptions): Interface; function emitKeypressEvents(stream: NodeJS.ReadableStream, readlineInterface?: Interface): void; type Direction = -1 | 0 | 1; interface CursorPos { rows: number; cols: number; } /** * Clears the current line of this WriteStream in a direction identified by `dir`. */ function clearLine(stream: NodeJS.WritableStream, dir: Direction, callback?: () => void): boolean; /** * Clears this `WriteStream` from the current cursor down. */ function clearScreenDown(stream: NodeJS.WritableStream, callback?: () => void): boolean; /** * Moves this WriteStream's cursor to the specified position. */ function cursorTo(stream: NodeJS.WritableStream, x: number, y?: number, callback?: () => void): boolean; /** * Moves this WriteStream's cursor relative to its current position. */ function moveCursor(stream: NodeJS.WritableStream, dx: number, dy: number, callback?: () => void): boolean; } apollo-server-demo/node_modules/@types/node/os.d.ts 0000644 0001750 0000144 00000017762 14000133700 022034 0 ustar andreh users declare module "os" { interface CpuInfo { model: string; speed: number; times: { user: number; nice: number; sys: number; idle: number; irq: number; }; } interface NetworkInterfaceBase { address: string; netmask: string; mac: string; internal: boolean; cidr: string | null; } interface NetworkInterfaceInfoIPv4 extends NetworkInterfaceBase { family: "IPv4"; } interface NetworkInterfaceInfoIPv6 extends NetworkInterfaceBase { family: "IPv6"; scopeid: number; } interface UserInfo<T> { username: T; uid: number; gid: number; shell: T; homedir: T; } type NetworkInterfaceInfo = NetworkInterfaceInfoIPv4 | NetworkInterfaceInfoIPv6; function hostname(): string; function loadavg(): number[]; function uptime(): number; function freemem(): number; function totalmem(): number; function cpus(): CpuInfo[]; function type(): string; function release(): string; function networkInterfaces(): NodeJS.Dict<NetworkInterfaceInfo[]>; function homedir(): string; function userInfo(options: { encoding: 'buffer' }): UserInfo<Buffer>; function userInfo(options?: { encoding: BufferEncoding }): UserInfo<string>; type SignalConstants = { [key in NodeJS.Signals]: number; }; namespace constants { const UV_UDP_REUSEADDR: number; namespace signals {} const signals: SignalConstants; namespace errno { const E2BIG: number; const EACCES: number; const EADDRINUSE: number; const EADDRNOTAVAIL: number; const EAFNOSUPPORT: number; const EAGAIN: number; const EALREADY: number; const EBADF: number; const EBADMSG: number; const EBUSY: number; const ECANCELED: number; const ECHILD: number; const ECONNABORTED: number; const ECONNREFUSED: number; const ECONNRESET: number; const EDEADLK: number; const EDESTADDRREQ: number; const EDOM: number; const EDQUOT: number; const EEXIST: number; const EFAULT: number; const EFBIG: number; const EHOSTUNREACH: number; const EIDRM: number; const EILSEQ: number; const EINPROGRESS: number; const EINTR: number; const EINVAL: number; const EIO: number; const EISCONN: number; const EISDIR: number; const ELOOP: number; const EMFILE: number; const EMLINK: number; const EMSGSIZE: number; const EMULTIHOP: number; const ENAMETOOLONG: number; const ENETDOWN: number; const ENETRESET: number; const ENETUNREACH: number; const ENFILE: number; const ENOBUFS: number; const ENODATA: number; const ENODEV: number; const ENOENT: number; const ENOEXEC: number; const ENOLCK: number; const ENOLINK: number; const ENOMEM: number; const ENOMSG: number; const ENOPROTOOPT: number; const ENOSPC: number; const ENOSR: number; const ENOSTR: number; const ENOSYS: number; const ENOTCONN: number; const ENOTDIR: number; const ENOTEMPTY: number; const ENOTSOCK: number; const ENOTSUP: number; const ENOTTY: number; const ENXIO: number; const EOPNOTSUPP: number; const EOVERFLOW: number; const EPERM: number; const EPIPE: number; const EPROTO: number; const EPROTONOSUPPORT: number; const EPROTOTYPE: number; const ERANGE: number; const EROFS: number; const ESPIPE: number; const ESRCH: number; const ESTALE: number; const ETIME: number; const ETIMEDOUT: number; const ETXTBSY: number; const EWOULDBLOCK: number; const EXDEV: number; const WSAEINTR: number; const WSAEBADF: number; const WSAEACCES: number; const WSAEFAULT: number; const WSAEINVAL: number; const WSAEMFILE: number; const WSAEWOULDBLOCK: number; const WSAEINPROGRESS: number; const WSAEALREADY: number; const WSAENOTSOCK: number; const WSAEDESTADDRREQ: number; const WSAEMSGSIZE: number; const WSAEPROTOTYPE: number; const WSAENOPROTOOPT: number; const WSAEPROTONOSUPPORT: number; const WSAESOCKTNOSUPPORT: number; const WSAEOPNOTSUPP: number; const WSAEPFNOSUPPORT: number; const WSAEAFNOSUPPORT: number; const WSAEADDRINUSE: number; const WSAEADDRNOTAVAIL: number; const WSAENETDOWN: number; const WSAENETUNREACH: number; const WSAENETRESET: number; const WSAECONNABORTED: number; const WSAECONNRESET: number; const WSAENOBUFS: number; const WSAEISCONN: number; const WSAENOTCONN: number; const WSAESHUTDOWN: number; const WSAETOOMANYREFS: number; const WSAETIMEDOUT: number; const WSAECONNREFUSED: number; const WSAELOOP: number; const WSAENAMETOOLONG: number; const WSAEHOSTDOWN: number; const WSAEHOSTUNREACH: number; const WSAENOTEMPTY: number; const WSAEPROCLIM: number; const WSAEUSERS: number; const WSAEDQUOT: number; const WSAESTALE: number; const WSAEREMOTE: number; const WSASYSNOTREADY: number; const WSAVERNOTSUPPORTED: number; const WSANOTINITIALISED: number; const WSAEDISCON: number; const WSAENOMORE: number; const WSAECANCELLED: number; const WSAEINVALIDPROCTABLE: number; const WSAEINVALIDPROVIDER: number; const WSAEPROVIDERFAILEDINIT: number; const WSASYSCALLFAILURE: number; const WSASERVICE_NOT_FOUND: number; const WSATYPE_NOT_FOUND: number; const WSA_E_NO_MORE: number; const WSA_E_CANCELLED: number; const WSAEREFUSED: number; } namespace priority { const PRIORITY_LOW: number; const PRIORITY_BELOW_NORMAL: number; const PRIORITY_NORMAL: number; const PRIORITY_ABOVE_NORMAL: number; const PRIORITY_HIGH: number; const PRIORITY_HIGHEST: number; } } function arch(): string; /** * Returns a string identifying the kernel version. * On POSIX systems, the operating system release is determined by calling * [uname(3)][]. On Windows, `pRtlGetVersion` is used, and if it is not available, * `GetVersionExW()` will be used. See * https://en.wikipedia.org/wiki/Uname#Examples for more information. */ function version(): string; function platform(): NodeJS.Platform; function tmpdir(): string; const EOL: string; function endianness(): "BE" | "LE"; /** * Gets the priority of a process. * Defaults to current process. */ function getPriority(pid?: number): number; /** * Sets the priority of the current process. * @param priority Must be in range of -20 to 19 */ function setPriority(priority: number): void; /** * Sets the priority of the process specified process. * @param priority Must be in range of -20 to 19 */ function setPriority(pid: number, priority: number): void; } apollo-server-demo/node_modules/@types/node/process.d.ts 0000644 0001750 0000144 00000047554 14000133700 023073 0 ustar andreh users declare module "process" { import * as tty from "tty"; global { var process: NodeJS.Process; namespace NodeJS { // this namespace merge is here because these are specifically used // as the type for process.stdin, process.stdout, and process.stderr. // they can't live in tty.d.ts because we need to disambiguate the imported name. interface ReadStream extends tty.ReadStream {} interface WriteStream extends tty.WriteStream {} interface MemoryUsage { rss: number; heapTotal: number; heapUsed: number; external: number; arrayBuffers: number; } interface CpuUsage { user: number; system: number; } interface ProcessRelease { name: string; sourceUrl?: string; headersUrl?: string; libUrl?: string; lts?: string; } interface ProcessVersions extends Dict<string> { http_parser: string; node: string; v8: string; ares: string; uv: string; zlib: string; modules: string; openssl: string; } type Platform = 'aix' | 'android' | 'darwin' | 'freebsd' | 'linux' | 'openbsd' | 'sunos' | 'win32' | 'cygwin' | 'netbsd'; type Signals = "SIGABRT" | "SIGALRM" | "SIGBUS" | "SIGCHLD" | "SIGCONT" | "SIGFPE" | "SIGHUP" | "SIGILL" | "SIGINT" | "SIGIO" | "SIGIOT" | "SIGKILL" | "SIGPIPE" | "SIGPOLL" | "SIGPROF" | "SIGPWR" | "SIGQUIT" | "SIGSEGV" | "SIGSTKFLT" | "SIGSTOP" | "SIGSYS" | "SIGTERM" | "SIGTRAP" | "SIGTSTP" | "SIGTTIN" | "SIGTTOU" | "SIGUNUSED" | "SIGURG" | "SIGUSR1" | "SIGUSR2" | "SIGVTALRM" | "SIGWINCH" | "SIGXCPU" | "SIGXFSZ" | "SIGBREAK" | "SIGLOST" | "SIGINFO"; type MultipleResolveType = 'resolve' | 'reject'; type BeforeExitListener = (code: number) => void; type DisconnectListener = () => void; type ExitListener = (code: number) => void; type RejectionHandledListener = (promise: Promise<any>) => void; type UncaughtExceptionListener = (error: Error) => void; type UnhandledRejectionListener = (reason: {} | null | undefined, promise: Promise<any>) => void; type WarningListener = (warning: Error) => void; type MessageListener = (message: any, sendHandle: any) => void; type SignalsListener = (signal: Signals) => void; type NewListenerListener = (type: string | symbol, listener: (...args: any[]) => void) => void; type RemoveListenerListener = (type: string | symbol, listener: (...args: any[]) => void) => void; type MultipleResolveListener = (type: MultipleResolveType, promise: Promise<any>, value: any) => void; interface Socket extends ReadWriteStream { isTTY?: true; } // Alias for compatibility interface ProcessEnv extends Dict<string> {} interface HRTime { (time?: [number, number]): [number, number]; bigint(): bigint; } interface ProcessReport { /** * Directory where the report is written. * working directory of the Node.js process. * @default '' indicating that reports are written to the current */ directory: string; /** * Filename where the report is written. * The default value is the empty string. * @default '' the output filename will be comprised of a timestamp, * PID, and sequence number. */ filename: string; /** * Returns a JSON-formatted diagnostic report for the running process. * The report's JavaScript stack trace is taken from err, if present. */ getReport(err?: Error): string; /** * If true, a diagnostic report is generated on fatal errors, * such as out of memory errors or failed C++ assertions. * @default false */ reportOnFatalError: boolean; /** * If true, a diagnostic report is generated when the process * receives the signal specified by process.report.signal. * @defaul false */ reportOnSignal: boolean; /** * If true, a diagnostic report is generated on uncaught exception. * @default false */ reportOnUncaughtException: boolean; /** * The signal used to trigger the creation of a diagnostic report. * @default 'SIGUSR2' */ signal: Signals; /** * Writes a diagnostic report to a file. If filename is not provided, the default filename * includes the date, time, PID, and a sequence number. * The report's JavaScript stack trace is taken from err, if present. * * @param fileName Name of the file where the report is written. * This should be a relative path, that will be appended to the directory specified in * `process.report.directory`, or the current working directory of the Node.js process, * if unspecified. * @param error A custom error used for reporting the JavaScript stack. * @return Filename of the generated report. */ writeReport(fileName?: string): string; writeReport(error?: Error): string; writeReport(fileName?: string, err?: Error): string; } interface ResourceUsage { fsRead: number; fsWrite: number; involuntaryContextSwitches: number; ipcReceived: number; ipcSent: number; majorPageFault: number; maxRSS: number; minorPageFault: number; sharedMemorySize: number; signalsCount: number; swappedOut: number; systemCPUTime: number; unsharedDataSize: number; unsharedStackSize: number; userCPUTime: number; voluntaryContextSwitches: number; } interface Process extends EventEmitter { /** * Can also be a tty.WriteStream, not typed due to limitations. */ stdout: WriteStream & { fd: 1; }; /** * Can also be a tty.WriteStream, not typed due to limitations. */ stderr: WriteStream & { fd: 2; }; stdin: ReadStream & { fd: 0; }; openStdin(): Socket; argv: string[]; argv0: string; execArgv: string[]; execPath: string; abort(): never; chdir(directory: string): void; cwd(): string; debugPort: number; emitWarning(warning: string | Error, name?: string, ctor?: Function): void; env: ProcessEnv; exit(code?: number): never; exitCode?: number; getgid(): number; setgid(id: number | string): void; getuid(): number; setuid(id: number | string): void; geteuid(): number; seteuid(id: number | string): void; getegid(): number; setegid(id: number | string): void; getgroups(): number[]; setgroups(groups: ReadonlyArray<string | number>): void; setUncaughtExceptionCaptureCallback(cb: ((err: Error) => void) | null): void; hasUncaughtExceptionCaptureCallback(): boolean; version: string; versions: ProcessVersions; config: { target_defaults: { cflags: any[]; default_configuration: string; defines: string[]; include_dirs: string[]; libraries: string[]; }; variables: { clang: number; host_arch: string; node_install_npm: boolean; node_install_waf: boolean; node_prefix: string; node_shared_openssl: boolean; node_shared_v8: boolean; node_shared_zlib: boolean; node_use_dtrace: boolean; node_use_etw: boolean; node_use_openssl: boolean; target_arch: string; v8_no_strict_aliasing: number; v8_use_snapshot: boolean; visibility: string; }; }; kill(pid: number, signal?: string | number): true; pid: number; ppid: number; title: string; arch: string; platform: Platform; /** @deprecated since v14.0.0 - use `require.main` instead. */ mainModule?: Module; memoryUsage(): MemoryUsage; cpuUsage(previousValue?: CpuUsage): CpuUsage; nextTick(callback: Function, ...args: any[]): void; release: ProcessRelease; features: { inspector: boolean; debug: boolean; uv: boolean; ipv6: boolean; tls_alpn: boolean; tls_sni: boolean; tls_ocsp: boolean; tls: boolean; }; /** * @deprecated since v14.0.0 - Calling process.umask() with no argument causes * the process-wide umask to be written twice. This introduces a race condition between threads, * and is a potential security vulnerability. There is no safe, cross-platform alternative API. */ umask(): number; /** * Can only be set if not in worker thread. */ umask(mask: string | number): number; uptime(): number; hrtime: HRTime; domain: Domain; // Worker send?(message: any, sendHandle?: any, options?: { swallowErrors?: boolean}, callback?: (error: Error | null) => void): boolean; disconnect(): void; connected: boolean; /** * The `process.allowedNodeEnvironmentFlags` property is a special, * read-only `Set` of flags allowable within the [`NODE_OPTIONS`][] * environment variable. */ allowedNodeEnvironmentFlags: ReadonlySet<string>; /** * Only available with `--experimental-report` */ report?: ProcessReport; resourceUsage(): ResourceUsage; traceDeprecation: boolean; /* EventEmitter */ addListener(event: "beforeExit", listener: BeforeExitListener): this; addListener(event: "disconnect", listener: DisconnectListener): this; addListener(event: "exit", listener: ExitListener): this; addListener(event: "rejectionHandled", listener: RejectionHandledListener): this; addListener(event: "uncaughtException", listener: UncaughtExceptionListener): this; addListener(event: "uncaughtExceptionMonitor", listener: UncaughtExceptionListener): this; addListener(event: "unhandledRejection", listener: UnhandledRejectionListener): this; addListener(event: "warning", listener: WarningListener): this; addListener(event: "message", listener: MessageListener): this; addListener(event: Signals, listener: SignalsListener): this; addListener(event: "newListener", listener: NewListenerListener): this; addListener(event: "removeListener", listener: RemoveListenerListener): this; addListener(event: "multipleResolves", listener: MultipleResolveListener): this; emit(event: "beforeExit", code: number): boolean; emit(event: "disconnect"): boolean; emit(event: "exit", code: number): boolean; emit(event: "rejectionHandled", promise: Promise<any>): boolean; emit(event: "uncaughtException", error: Error): boolean; emit(event: "uncaughtExceptionMonitor", error: Error): boolean; emit(event: "unhandledRejection", reason: any, promise: Promise<any>): boolean; emit(event: "warning", warning: Error): boolean; emit(event: "message", message: any, sendHandle: any): this; emit(event: Signals, signal: Signals): boolean; emit(event: "newListener", eventName: string | symbol, listener: (...args: any[]) => void): this; emit(event: "removeListener", eventName: string, listener: (...args: any[]) => void): this; emit(event: "multipleResolves", listener: MultipleResolveListener): this; on(event: "beforeExit", listener: BeforeExitListener): this; on(event: "disconnect", listener: DisconnectListener): this; on(event: "exit", listener: ExitListener): this; on(event: "rejectionHandled", listener: RejectionHandledListener): this; on(event: "uncaughtException", listener: UncaughtExceptionListener): this; on(event: "uncaughtExceptionMonitor", listener: UncaughtExceptionListener): this; on(event: "unhandledRejection", listener: UnhandledRejectionListener): this; on(event: "warning", listener: WarningListener): this; on(event: "message", listener: MessageListener): this; on(event: Signals, listener: SignalsListener): this; on(event: "newListener", listener: NewListenerListener): this; on(event: "removeListener", listener: RemoveListenerListener): this; on(event: "multipleResolves", listener: MultipleResolveListener): this; on(event: string | symbol, listener: (...args: any[]) => void): this; once(event: "beforeExit", listener: BeforeExitListener): this; once(event: "disconnect", listener: DisconnectListener): this; once(event: "exit", listener: ExitListener): this; once(event: "rejectionHandled", listener: RejectionHandledListener): this; once(event: "uncaughtException", listener: UncaughtExceptionListener): this; once(event: "uncaughtExceptionMonitor", listener: UncaughtExceptionListener): this; once(event: "unhandledRejection", listener: UnhandledRejectionListener): this; once(event: "warning", listener: WarningListener): this; once(event: "message", listener: MessageListener): this; once(event: Signals, listener: SignalsListener): this; once(event: "newListener", listener: NewListenerListener): this; once(event: "removeListener", listener: RemoveListenerListener): this; once(event: "multipleResolves", listener: MultipleResolveListener): this; prependListener(event: "beforeExit", listener: BeforeExitListener): this; prependListener(event: "disconnect", listener: DisconnectListener): this; prependListener(event: "exit", listener: ExitListener): this; prependListener(event: "rejectionHandled", listener: RejectionHandledListener): this; prependListener(event: "uncaughtException", listener: UncaughtExceptionListener): this; prependListener(event: "uncaughtExceptionMonitor", listener: UncaughtExceptionListener): this; prependListener(event: "unhandledRejection", listener: UnhandledRejectionListener): this; prependListener(event: "warning", listener: WarningListener): this; prependListener(event: "message", listener: MessageListener): this; prependListener(event: Signals, listener: SignalsListener): this; prependListener(event: "newListener", listener: NewListenerListener): this; prependListener(event: "removeListener", listener: RemoveListenerListener): this; prependListener(event: "multipleResolves", listener: MultipleResolveListener): this; prependOnceListener(event: "beforeExit", listener: BeforeExitListener): this; prependOnceListener(event: "disconnect", listener: DisconnectListener): this; prependOnceListener(event: "exit", listener: ExitListener): this; prependOnceListener(event: "rejectionHandled", listener: RejectionHandledListener): this; prependOnceListener(event: "uncaughtException", listener: UncaughtExceptionListener): this; prependOnceListener(event: "uncaughtExceptionMonitor", listener: UncaughtExceptionListener): this; prependOnceListener(event: "unhandledRejection", listener: UnhandledRejectionListener): this; prependOnceListener(event: "warning", listener: WarningListener): this; prependOnceListener(event: "message", listener: MessageListener): this; prependOnceListener(event: Signals, listener: SignalsListener): this; prependOnceListener(event: "newListener", listener: NewListenerListener): this; prependOnceListener(event: "removeListener", listener: RemoveListenerListener): this; prependOnceListener(event: "multipleResolves", listener: MultipleResolveListener): this; listeners(event: "beforeExit"): BeforeExitListener[]; listeners(event: "disconnect"): DisconnectListener[]; listeners(event: "exit"): ExitListener[]; listeners(event: "rejectionHandled"): RejectionHandledListener[]; listeners(event: "uncaughtException"): UncaughtExceptionListener[]; listeners(event: "uncaughtExceptionMonitor"): UncaughtExceptionListener[]; listeners(event: "unhandledRejection"): UnhandledRejectionListener[]; listeners(event: "warning"): WarningListener[]; listeners(event: "message"): MessageListener[]; listeners(event: Signals): SignalsListener[]; listeners(event: "newListener"): NewListenerListener[]; listeners(event: "removeListener"): RemoveListenerListener[]; listeners(event: "multipleResolves"): MultipleResolveListener[]; } interface Global { process: Process; } } } export = process; } apollo-server-demo/node_modules/@types/node/events.d.ts 0000644 0001750 0000144 00000006454 14000133700 022713 0 ustar andreh users declare module 'events' { interface EventEmitterOptions { /** * Enables automatic capturing of promise rejection. */ captureRejections?: boolean; } interface NodeEventTarget { once(event: string | symbol, listener: (...args: any[]) => void): this; } interface DOMEventTarget { addEventListener(event: string, listener: (...args: any[]) => void, opts?: { once: boolean }): any; } interface EventEmitter extends NodeJS.EventEmitter {} class EventEmitter { constructor(options?: EventEmitterOptions); static once(emitter: NodeEventTarget, event: string | symbol): Promise<any[]>; static once(emitter: DOMEventTarget, event: string): Promise<any[]>; static on(emitter: NodeJS.EventEmitter, event: string): AsyncIterableIterator<any>; /** @deprecated since v4.0.0 */ static listenerCount(emitter: NodeJS.EventEmitter, event: string | symbol): number; /** * This symbol shall be used to install a listener for only monitoring `'error'` * events. Listeners installed using this symbol are called before the regular * `'error'` listeners are called. * * Installing a listener using this symbol does not change the behavior once an * `'error'` event is emitted, therefore the process will still crash if no * regular `'error'` listener is installed. */ static readonly errorMonitor: unique symbol; static readonly captureRejectionSymbol: unique symbol; /** * Sets or gets the default captureRejection value for all emitters. */ // TODO: These should be described using static getter/setter pairs: static captureRejections: boolean; static defaultMaxListeners: number; } import internal = require('events'); namespace EventEmitter { // Should just be `export { EventEmitter }`, but that doesn't work in TypeScript 3.4 export { internal as EventEmitter }; } global { namespace NodeJS { interface EventEmitter { addListener(event: string | symbol, listener: (...args: any[]) => void): this; on(event: string | symbol, listener: (...args: any[]) => void): this; once(event: string | symbol, listener: (...args: any[]) => void): this; removeListener(event: string | symbol, listener: (...args: any[]) => void): this; off(event: string | symbol, listener: (...args: any[]) => void): this; removeAllListeners(event?: string | symbol): this; setMaxListeners(n: number): this; getMaxListeners(): number; listeners(event: string | symbol): Function[]; rawListeners(event: string | symbol): Function[]; emit(event: string | symbol, ...args: any[]): boolean; listenerCount(event: string | symbol): number; // Added in Node 6... prependListener(event: string | symbol, listener: (...args: any[]) => void): this; prependOnceListener(event: string | symbol, listener: (...args: any[]) => void): this; eventNames(): Array<string | symbol>; } } } export = EventEmitter; } apollo-server-demo/node_modules/@types/node/dgram.d.ts 0000644 0001750 0000144 00000016033 14000133700 022473 0 ustar andreh users declare module "dgram" { import { AddressInfo } from "net"; import * as dns from "dns"; import * as events from "events"; interface RemoteInfo { address: string; family: 'IPv4' | 'IPv6'; port: number; size: number; } interface BindOptions { port?: number; address?: string; exclusive?: boolean; fd?: number; } type SocketType = "udp4" | "udp6"; interface SocketOptions { type: SocketType; reuseAddr?: boolean; /** * @default false */ ipv6Only?: boolean; recvBufferSize?: number; sendBufferSize?: number; lookup?: (hostname: string, options: dns.LookupOneOptions, callback: (err: NodeJS.ErrnoException | null, address: string, family: number) => void) => void; } function createSocket(type: SocketType, callback?: (msg: Buffer, rinfo: RemoteInfo) => void): Socket; function createSocket(options: SocketOptions, callback?: (msg: Buffer, rinfo: RemoteInfo) => void): Socket; class Socket extends events.EventEmitter { addMembership(multicastAddress: string, multicastInterface?: string): void; address(): AddressInfo; bind(port?: number, address?: string, callback?: () => void): void; bind(port?: number, callback?: () => void): void; bind(callback?: () => void): void; bind(options: BindOptions, callback?: () => void): void; close(callback?: () => void): void; connect(port: number, address?: string, callback?: () => void): void; connect(port: number, callback: () => void): void; disconnect(): void; dropMembership(multicastAddress: string, multicastInterface?: string): void; getRecvBufferSize(): number; getSendBufferSize(): number; ref(): this; remoteAddress(): AddressInfo; send(msg: string | Uint8Array | ReadonlyArray<any>, port?: number, address?: string, callback?: (error: Error | null, bytes: number) => void): void; send(msg: string | Uint8Array | ReadonlyArray<any>, port?: number, callback?: (error: Error | null, bytes: number) => void): void; send(msg: string | Uint8Array | ReadonlyArray<any>, callback?: (error: Error | null, bytes: number) => void): void; send(msg: string | Uint8Array, offset: number, length: number, port?: number, address?: string, callback?: (error: Error | null, bytes: number) => void): void; send(msg: string | Uint8Array, offset: number, length: number, port?: number, callback?: (error: Error | null, bytes: number) => void): void; send(msg: string | Uint8Array, offset: number, length: number, callback?: (error: Error | null, bytes: number) => void): void; setBroadcast(flag: boolean): void; setMulticastInterface(multicastInterface: string): void; setMulticastLoopback(flag: boolean): void; setMulticastTTL(ttl: number): void; setRecvBufferSize(size: number): void; setSendBufferSize(size: number): void; setTTL(ttl: number): void; unref(): this; /** * Tells the kernel to join a source-specific multicast channel at the given * `sourceAddress` and `groupAddress`, using the `multicastInterface` with the * `IP_ADD_SOURCE_MEMBERSHIP` socket option. * If the `multicastInterface` argument * is not specified, the operating system will choose one interface and will add * membership to it. * To add membership to every available interface, call * `socket.addSourceSpecificMembership()` multiple times, once per interface. */ addSourceSpecificMembership(sourceAddress: string, groupAddress: string, multicastInterface?: string): void; /** * Instructs the kernel to leave a source-specific multicast channel at the given * `sourceAddress` and `groupAddress` using the `IP_DROP_SOURCE_MEMBERSHIP` * socket option. This method is automatically called by the kernel when the * socket is closed or the process terminates, so most apps will never have * reason to call this. * * If `multicastInterface` is not specified, the operating system will attempt to * drop membership on all valid interfaces. */ dropSourceSpecificMembership(sourceAddress: string, groupAddress: string, multicastInterface?: string): void; /** * events.EventEmitter * 1. close * 2. connect * 3. error * 4. listening * 5. message */ addListener(event: string, listener: (...args: any[]) => void): this; addListener(event: "close", listener: () => void): this; addListener(event: "connect", listener: () => void): this; addListener(event: "error", listener: (err: Error) => void): this; addListener(event: "listening", listener: () => void): this; addListener(event: "message", listener: (msg: Buffer, rinfo: RemoteInfo) => void): this; emit(event: string | symbol, ...args: any[]): boolean; emit(event: "close"): boolean; emit(event: "connect"): boolean; emit(event: "error", err: Error): boolean; emit(event: "listening"): boolean; emit(event: "message", msg: Buffer, rinfo: RemoteInfo): boolean; on(event: string, listener: (...args: any[]) => void): this; on(event: "close", listener: () => void): this; on(event: "connect", listener: () => void): this; on(event: "error", listener: (err: Error) => void): this; on(event: "listening", listener: () => void): this; on(event: "message", listener: (msg: Buffer, rinfo: RemoteInfo) => void): this; once(event: string, listener: (...args: any[]) => void): this; once(event: "close", listener: () => void): this; once(event: "connect", listener: () => void): this; once(event: "error", listener: (err: Error) => void): this; once(event: "listening", listener: () => void): this; once(event: "message", listener: (msg: Buffer, rinfo: RemoteInfo) => void): this; prependListener(event: string, listener: (...args: any[]) => void): this; prependListener(event: "close", listener: () => void): this; prependListener(event: "connect", listener: () => void): this; prependListener(event: "error", listener: (err: Error) => void): this; prependListener(event: "listening", listener: () => void): this; prependListener(event: "message", listener: (msg: Buffer, rinfo: RemoteInfo) => void): this; prependOnceListener(event: string, listener: (...args: any[]) => void): this; prependOnceListener(event: "close", listener: () => void): this; prependOnceListener(event: "connect", listener: () => void): this; prependOnceListener(event: "error", listener: (err: Error) => void): this; prependOnceListener(event: "listening", listener: () => void): this; prependOnceListener(event: "message", listener: (msg: Buffer, rinfo: RemoteInfo) => void): this; } } apollo-server-demo/node_modules/@types/node/crypto.d.ts 0000644 0001750 0000144 00000132376 14000133700 022732 0 ustar andreh users declare module 'crypto' { import * as stream from 'stream'; interface Certificate { /** * @param spkac * @returns The challenge component of the `spkac` data structure, * which includes a public key and a challenge. */ exportChallenge(spkac: BinaryLike): Buffer; /** * @param spkac * @param encoding The encoding of the spkac string. * @returns The public key component of the `spkac` data structure, * which includes a public key and a challenge. */ exportPublicKey(spkac: BinaryLike, encoding?: string): Buffer; /** * @param spkac * @returns `true` if the given `spkac` data structure is valid, * `false` otherwise. */ verifySpkac(spkac: NodeJS.ArrayBufferView): boolean; } const Certificate: Certificate & { /** @deprecated since v14.9.0 - Use static methods of `crypto.Certificate` instead. */ new (): Certificate; /** @deprecated since v14.9.0 - Use static methods of `crypto.Certificate` instead. */ (): Certificate; }; namespace constants { // https://nodejs.org/dist/latest-v10.x/docs/api/crypto.html#crypto_crypto_constants const OPENSSL_VERSION_NUMBER: number; /** Applies multiple bug workarounds within OpenSSL. See https://www.openssl.org/docs/man1.0.2/ssl/SSL_CTX_set_options.html for detail. */ const SSL_OP_ALL: number; /** Allows legacy insecure renegotiation between OpenSSL and unpatched clients or servers. See https://www.openssl.org/docs/man1.0.2/ssl/SSL_CTX_set_options.html. */ const SSL_OP_ALLOW_UNSAFE_LEGACY_RENEGOTIATION: number; /** Attempts to use the server's preferences instead of the client's when selecting a cipher. See https://www.openssl.org/docs/man1.0.2/ssl/SSL_CTX_set_options.html. */ const SSL_OP_CIPHER_SERVER_PREFERENCE: number; /** Instructs OpenSSL to use Cisco's "speshul" version of DTLS_BAD_VER. */ const SSL_OP_CISCO_ANYCONNECT: number; /** Instructs OpenSSL to turn on cookie exchange. */ const SSL_OP_COOKIE_EXCHANGE: number; /** Instructs OpenSSL to add server-hello extension from an early version of the cryptopro draft. */ const SSL_OP_CRYPTOPRO_TLSEXT_BUG: number; /** Instructs OpenSSL to disable a SSL 3.0/TLS 1.0 vulnerability workaround added in OpenSSL 0.9.6d. */ const SSL_OP_DONT_INSERT_EMPTY_FRAGMENTS: number; /** Instructs OpenSSL to always use the tmp_rsa key when performing RSA operations. */ const SSL_OP_EPHEMERAL_RSA: number; /** Allows initial connection to servers that do not support RI. */ const SSL_OP_LEGACY_SERVER_CONNECT: number; const SSL_OP_MICROSOFT_BIG_SSLV3_BUFFER: number; const SSL_OP_MICROSOFT_SESS_ID_BUG: number; /** Instructs OpenSSL to disable the workaround for a man-in-the-middle protocol-version vulnerability in the SSL 2.0 server implementation. */ const SSL_OP_MSIE_SSLV2_RSA_PADDING: number; const SSL_OP_NETSCAPE_CA_DN_BUG: number; const SSL_OP_NETSCAPE_CHALLENGE_BUG: number; const SSL_OP_NETSCAPE_DEMO_CIPHER_CHANGE_BUG: number; const SSL_OP_NETSCAPE_REUSE_CIPHER_CHANGE_BUG: number; /** Instructs OpenSSL to disable support for SSL/TLS compression. */ const SSL_OP_NO_COMPRESSION: number; const SSL_OP_NO_QUERY_MTU: number; /** Instructs OpenSSL to always start a new session when performing renegotiation. */ const SSL_OP_NO_SESSION_RESUMPTION_ON_RENEGOTIATION: number; const SSL_OP_NO_SSLv2: number; const SSL_OP_NO_SSLv3: number; const SSL_OP_NO_TICKET: number; const SSL_OP_NO_TLSv1: number; const SSL_OP_NO_TLSv1_1: number; const SSL_OP_NO_TLSv1_2: number; const SSL_OP_PKCS1_CHECK_1: number; const SSL_OP_PKCS1_CHECK_2: number; /** Instructs OpenSSL to always create a new key when using temporary/ephemeral DH parameters. */ const SSL_OP_SINGLE_DH_USE: number; /** Instructs OpenSSL to always create a new key when using temporary/ephemeral ECDH parameters. */ const SSL_OP_SINGLE_ECDH_USE: number; const SSL_OP_SSLEAY_080_CLIENT_DH_BUG: number; const SSL_OP_SSLREF2_REUSE_CERT_TYPE_BUG: number; const SSL_OP_TLS_BLOCK_PADDING_BUG: number; const SSL_OP_TLS_D5_BUG: number; /** Instructs OpenSSL to disable version rollback attack detection. */ const SSL_OP_TLS_ROLLBACK_BUG: number; const ENGINE_METHOD_RSA: number; const ENGINE_METHOD_DSA: number; const ENGINE_METHOD_DH: number; const ENGINE_METHOD_RAND: number; const ENGINE_METHOD_EC: number; const ENGINE_METHOD_CIPHERS: number; const ENGINE_METHOD_DIGESTS: number; const ENGINE_METHOD_PKEY_METHS: number; const ENGINE_METHOD_PKEY_ASN1_METHS: number; const ENGINE_METHOD_ALL: number; const ENGINE_METHOD_NONE: number; const DH_CHECK_P_NOT_SAFE_PRIME: number; const DH_CHECK_P_NOT_PRIME: number; const DH_UNABLE_TO_CHECK_GENERATOR: number; const DH_NOT_SUITABLE_GENERATOR: number; const ALPN_ENABLED: number; const RSA_PKCS1_PADDING: number; const RSA_SSLV23_PADDING: number; const RSA_NO_PADDING: number; const RSA_PKCS1_OAEP_PADDING: number; const RSA_X931_PADDING: number; const RSA_PKCS1_PSS_PADDING: number; /** Sets the salt length for RSA_PKCS1_PSS_PADDING to the digest size when signing or verifying. */ const RSA_PSS_SALTLEN_DIGEST: number; /** Sets the salt length for RSA_PKCS1_PSS_PADDING to the maximum permissible value when signing data. */ const RSA_PSS_SALTLEN_MAX_SIGN: number; /** Causes the salt length for RSA_PKCS1_PSS_PADDING to be determined automatically when verifying a signature. */ const RSA_PSS_SALTLEN_AUTO: number; const POINT_CONVERSION_COMPRESSED: number; const POINT_CONVERSION_UNCOMPRESSED: number; const POINT_CONVERSION_HYBRID: number; /** Specifies the built-in default cipher list used by Node.js (colon-separated values). */ const defaultCoreCipherList: string; /** Specifies the active default cipher list used by the current Node.js process (colon-separated values). */ const defaultCipherList: string; } interface HashOptions extends stream.TransformOptions { /** * For XOF hash functions such as `shake256`, the * outputLength option can be used to specify the desired output length in bytes. */ outputLength?: number; } /** @deprecated since v10.0.0 */ const fips: boolean; function createHash(algorithm: string, options?: HashOptions): Hash; function createHmac(algorithm: string, key: BinaryLike | KeyObject, options?: stream.TransformOptions): Hmac; // https://nodejs.org/api/buffer.html#buffer_buffers_and_character_encodings type BinaryToTextEncoding = 'base64' | 'hex'; type CharacterEncoding = 'utf8' | 'utf-8' | 'utf16le' | 'latin1'; type LegacyCharacterEncoding = 'ascii' | 'binary' | 'ucs2' | 'ucs-2'; type Encoding = BinaryToTextEncoding | CharacterEncoding | LegacyCharacterEncoding; type ECDHKeyFormat = 'compressed' | 'uncompressed' | 'hybrid'; class Hash extends stream.Transform { private constructor(); copy(): Hash; update(data: BinaryLike): Hash; update(data: string, input_encoding: Encoding): Hash; digest(): Buffer; digest(encoding: BinaryToTextEncoding): string; } class Hmac extends stream.Transform { private constructor(); update(data: BinaryLike): Hmac; update(data: string, input_encoding: Encoding): Hmac; digest(): Buffer; digest(encoding: BinaryToTextEncoding): string; } type KeyObjectType = 'secret' | 'public' | 'private'; interface KeyExportOptions<T extends KeyFormat> { type: 'pkcs1' | 'spki' | 'pkcs8' | 'sec1'; format: T; cipher?: string; passphrase?: string | Buffer; } class KeyObject { private constructor(); asymmetricKeyType?: KeyType; /** * For asymmetric keys, this property represents the size of the embedded key in * bytes. This property is `undefined` for symmetric keys. */ asymmetricKeySize?: number; export(options: KeyExportOptions<'pem'>): string | Buffer; export(options?: KeyExportOptions<'der'>): Buffer; symmetricKeySize?: number; type: KeyObjectType; } type CipherCCMTypes = 'aes-128-ccm' | 'aes-192-ccm' | 'aes-256-ccm' | 'chacha20-poly1305'; type CipherGCMTypes = 'aes-128-gcm' | 'aes-192-gcm' | 'aes-256-gcm'; type BinaryLike = string | NodeJS.ArrayBufferView; type CipherKey = BinaryLike | KeyObject; interface CipherCCMOptions extends stream.TransformOptions { authTagLength: number; } interface CipherGCMOptions extends stream.TransformOptions { authTagLength?: number; } /** @deprecated since v10.0.0 use `createCipheriv()` */ function createCipher(algorithm: CipherCCMTypes, password: BinaryLike, options: CipherCCMOptions): CipherCCM; /** @deprecated since v10.0.0 use `createCipheriv()` */ function createCipher(algorithm: CipherGCMTypes, password: BinaryLike, options?: CipherGCMOptions): CipherGCM; /** @deprecated since v10.0.0 use `createCipheriv()` */ function createCipher(algorithm: string, password: BinaryLike, options?: stream.TransformOptions): Cipher; function createCipheriv( algorithm: CipherCCMTypes, key: CipherKey, iv: BinaryLike | null, options: CipherCCMOptions, ): CipherCCM; function createCipheriv( algorithm: CipherGCMTypes, key: CipherKey, iv: BinaryLike | null, options?: CipherGCMOptions, ): CipherGCM; function createCipheriv( algorithm: string, key: CipherKey, iv: BinaryLike | null, options?: stream.TransformOptions, ): Cipher; class Cipher extends stream.Transform { private constructor(); update(data: BinaryLike): Buffer; update(data: string, input_encoding: Encoding): Buffer; update(data: NodeJS.ArrayBufferView, input_encoding: undefined, output_encoding: BinaryToTextEncoding): string; update(data: string, input_encoding: Encoding | undefined, output_encoding: BinaryToTextEncoding): string; final(): Buffer; final(output_encoding: BufferEncoding): string; setAutoPadding(auto_padding?: boolean): this; // getAuthTag(): Buffer; // setAAD(buffer: NodeJS.ArrayBufferView): this; } interface CipherCCM extends Cipher { setAAD(buffer: NodeJS.ArrayBufferView, options: { plaintextLength: number }): this; getAuthTag(): Buffer; } interface CipherGCM extends Cipher { setAAD(buffer: NodeJS.ArrayBufferView, options?: { plaintextLength: number }): this; getAuthTag(): Buffer; } /** @deprecated since v10.0.0 use `createDecipheriv()` */ function createDecipher(algorithm: CipherCCMTypes, password: BinaryLike, options: CipherCCMOptions): DecipherCCM; /** @deprecated since v10.0.0 use `createDecipheriv()` */ function createDecipher(algorithm: CipherGCMTypes, password: BinaryLike, options?: CipherGCMOptions): DecipherGCM; /** @deprecated since v10.0.0 use `createDecipheriv()` */ function createDecipher(algorithm: string, password: BinaryLike, options?: stream.TransformOptions): Decipher; function createDecipheriv( algorithm: CipherCCMTypes, key: CipherKey, iv: BinaryLike | null, options: CipherCCMOptions, ): DecipherCCM; function createDecipheriv( algorithm: CipherGCMTypes, key: CipherKey, iv: BinaryLike | null, options?: CipherGCMOptions, ): DecipherGCM; function createDecipheriv( algorithm: string, key: CipherKey, iv: BinaryLike | null, options?: stream.TransformOptions, ): Decipher; class Decipher extends stream.Transform { private constructor(); update(data: NodeJS.ArrayBufferView): Buffer; update(data: string, input_encoding: BinaryToTextEncoding): Buffer; update(data: NodeJS.ArrayBufferView, input_encoding: undefined, output_encoding: Encoding): string; update(data: string, input_encoding: BinaryToTextEncoding | undefined, output_encoding: Encoding): string; final(): Buffer; final(output_encoding: BufferEncoding): string; setAutoPadding(auto_padding?: boolean): this; // setAuthTag(tag: NodeJS.ArrayBufferView): this; // setAAD(buffer: NodeJS.ArrayBufferView): this; } interface DecipherCCM extends Decipher { setAuthTag(buffer: NodeJS.ArrayBufferView): this; setAAD(buffer: NodeJS.ArrayBufferView, options: { plaintextLength: number }): this; } interface DecipherGCM extends Decipher { setAuthTag(buffer: NodeJS.ArrayBufferView): this; setAAD(buffer: NodeJS.ArrayBufferView, options?: { plaintextLength: number }): this; } interface PrivateKeyInput { key: string | Buffer; format?: KeyFormat; type?: 'pkcs1' | 'pkcs8' | 'sec1'; passphrase?: string | Buffer; } interface PublicKeyInput { key: string | Buffer; format?: KeyFormat; type?: 'pkcs1' | 'spki'; } function createPrivateKey(key: PrivateKeyInput | string | Buffer): KeyObject; function createPublicKey(key: PublicKeyInput | string | Buffer | KeyObject): KeyObject; function createSecretKey(key: NodeJS.ArrayBufferView): KeyObject; function createSign(algorithm: string, options?: stream.WritableOptions): Signer; type DSAEncoding = 'der' | 'ieee-p1363'; interface SigningOptions { /** * @See crypto.constants.RSA_PKCS1_PADDING */ padding?: number; saltLength?: number; dsaEncoding?: DSAEncoding; } interface SignPrivateKeyInput extends PrivateKeyInput, SigningOptions {} interface SignKeyObjectInput extends SigningOptions { key: KeyObject; } interface VerifyPublicKeyInput extends PublicKeyInput, SigningOptions {} interface VerifyKeyObjectInput extends SigningOptions { key: KeyObject; } type KeyLike = string | Buffer | KeyObject; class Signer extends stream.Writable { private constructor(); update(data: BinaryLike): Signer; update(data: string, input_encoding: Encoding): Signer; sign(private_key: KeyLike | SignKeyObjectInput | SignPrivateKeyInput): Buffer; sign( private_key: KeyLike | SignKeyObjectInput | SignPrivateKeyInput, output_format: BinaryToTextEncoding, ): string; } function createVerify(algorithm: string, options?: stream.WritableOptions): Verify; class Verify extends stream.Writable { private constructor(); update(data: BinaryLike): Verify; update(data: string, input_encoding: Encoding): Verify; verify( object: KeyLike | VerifyKeyObjectInput | VerifyPublicKeyInput, signature: NodeJS.ArrayBufferView, ): boolean; verify( object: KeyLike | VerifyKeyObjectInput | VerifyPublicKeyInput, signature: string, signature_format?: BinaryToTextEncoding, ): boolean; // https://nodejs.org/api/crypto.html#crypto_verifier_verify_object_signature_signature_format // The signature field accepts a TypedArray type, but it is only available starting ES2017 } function createDiffieHellman(prime_length: number, generator?: number | NodeJS.ArrayBufferView): DiffieHellman; function createDiffieHellman(prime: NodeJS.ArrayBufferView): DiffieHellman; function createDiffieHellman(prime: string, prime_encoding: BinaryToTextEncoding): DiffieHellman; function createDiffieHellman( prime: string, prime_encoding: BinaryToTextEncoding, generator: number | NodeJS.ArrayBufferView, ): DiffieHellman; function createDiffieHellman( prime: string, prime_encoding: BinaryToTextEncoding, generator: string, generator_encoding: BinaryToTextEncoding, ): DiffieHellman; class DiffieHellman { private constructor(); generateKeys(): Buffer; generateKeys(encoding: BinaryToTextEncoding): string; computeSecret(other_public_key: NodeJS.ArrayBufferView): Buffer; computeSecret(other_public_key: string, input_encoding: BinaryToTextEncoding): Buffer; computeSecret(other_public_key: NodeJS.ArrayBufferView, output_encoding: BinaryToTextEncoding): string; computeSecret( other_public_key: string, input_encoding: BinaryToTextEncoding, output_encoding: BinaryToTextEncoding, ): string; getPrime(): Buffer; getPrime(encoding: BinaryToTextEncoding): string; getGenerator(): Buffer; getGenerator(encoding: BinaryToTextEncoding): string; getPublicKey(): Buffer; getPublicKey(encoding: BinaryToTextEncoding): string; getPrivateKey(): Buffer; getPrivateKey(encoding: BinaryToTextEncoding): string; setPublicKey(public_key: NodeJS.ArrayBufferView): void; setPublicKey(public_key: string, encoding: BufferEncoding): void; setPrivateKey(private_key: NodeJS.ArrayBufferView): void; setPrivateKey(private_key: string, encoding: BufferEncoding): void; verifyError: number; } function getDiffieHellman(group_name: string): DiffieHellman; function pbkdf2( password: BinaryLike, salt: BinaryLike, iterations: number, keylen: number, digest: string, callback: (err: Error | null, derivedKey: Buffer) => any, ): void; function pbkdf2Sync( password: BinaryLike, salt: BinaryLike, iterations: number, keylen: number, digest: string, ): Buffer; function randomBytes(size: number): Buffer; function randomBytes(size: number, callback: (err: Error | null, buf: Buffer) => void): void; function pseudoRandomBytes(size: number): Buffer; function pseudoRandomBytes(size: number, callback: (err: Error | null, buf: Buffer) => void): void; function randomInt(max: number): number; function randomInt(min: number, max: number): number; function randomInt(max: number, callback: (err: Error | null, value: number) => void): void; function randomInt(min: number, max: number, callback: (err: Error | null, value: number) => void): void; function randomFillSync<T extends NodeJS.ArrayBufferView>(buffer: T, offset?: number, size?: number): T; function randomFill<T extends NodeJS.ArrayBufferView>( buffer: T, callback: (err: Error | null, buf: T) => void, ): void; function randomFill<T extends NodeJS.ArrayBufferView>( buffer: T, offset: number, callback: (err: Error | null, buf: T) => void, ): void; function randomFill<T extends NodeJS.ArrayBufferView>( buffer: T, offset: number, size: number, callback: (err: Error | null, buf: T) => void, ): void; interface ScryptOptions { cost?: number; blockSize?: number; parallelization?: number; N?: number; r?: number; p?: number; maxmem?: number; } function scrypt( password: BinaryLike, salt: BinaryLike, keylen: number, callback: (err: Error | null, derivedKey: Buffer) => void, ): void; function scrypt( password: BinaryLike, salt: BinaryLike, keylen: number, options: ScryptOptions, callback: (err: Error | null, derivedKey: Buffer) => void, ): void; function scryptSync(password: BinaryLike, salt: BinaryLike, keylen: number, options?: ScryptOptions): Buffer; interface RsaPublicKey { key: KeyLike; padding?: number; } interface RsaPrivateKey { key: KeyLike; passphrase?: string; /** * @default 'sha1' */ oaepHash?: string; oaepLabel?: NodeJS.TypedArray; padding?: number; } function publicEncrypt(key: RsaPublicKey | RsaPrivateKey | KeyLike, buffer: NodeJS.ArrayBufferView): Buffer; function publicDecrypt(key: RsaPublicKey | RsaPrivateKey | KeyLike, buffer: NodeJS.ArrayBufferView): Buffer; function privateDecrypt(private_key: RsaPrivateKey | KeyLike, buffer: NodeJS.ArrayBufferView): Buffer; function privateEncrypt(private_key: RsaPrivateKey | KeyLike, buffer: NodeJS.ArrayBufferView): Buffer; function getCiphers(): string[]; function getCurves(): string[]; function getFips(): 1 | 0; function getHashes(): string[]; class ECDH { private constructor(); static convertKey( key: BinaryLike, curve: string, inputEncoding?: BinaryToTextEncoding, outputEncoding?: 'latin1' | 'hex' | 'base64', format?: 'uncompressed' | 'compressed' | 'hybrid', ): Buffer | string; generateKeys(): Buffer; generateKeys(encoding: BinaryToTextEncoding, format?: ECDHKeyFormat): string; computeSecret(other_public_key: NodeJS.ArrayBufferView): Buffer; computeSecret(other_public_key: string, input_encoding: BinaryToTextEncoding): Buffer; computeSecret(other_public_key: NodeJS.ArrayBufferView, output_encoding: BinaryToTextEncoding): string; computeSecret( other_public_key: string, input_encoding: BinaryToTextEncoding, output_encoding: BinaryToTextEncoding, ): string; getPrivateKey(): Buffer; getPrivateKey(encoding: BinaryToTextEncoding): string; getPublicKey(): Buffer; getPublicKey(encoding: BinaryToTextEncoding, format?: ECDHKeyFormat): string; setPrivateKey(private_key: NodeJS.ArrayBufferView): void; setPrivateKey(private_key: string, encoding: BinaryToTextEncoding): void; } function createECDH(curve_name: string): ECDH; function timingSafeEqual(a: NodeJS.ArrayBufferView, b: NodeJS.ArrayBufferView): boolean; /** @deprecated since v10.0.0 */ const DEFAULT_ENCODING: BufferEncoding; type KeyType = 'rsa' | 'dsa' | 'ec' | 'ed25519' | 'ed448' | 'x25519' | 'x448'; type KeyFormat = 'pem' | 'der'; interface BasePrivateKeyEncodingOptions<T extends KeyFormat> { format: T; cipher?: string; passphrase?: string; } interface KeyPairKeyObjectResult { publicKey: KeyObject; privateKey: KeyObject; } interface ED25519KeyPairKeyObjectOptions { /** * No options. */ } interface ED448KeyPairKeyObjectOptions { /** * No options. */ } interface X25519KeyPairKeyObjectOptions { /** * No options. */ } interface X448KeyPairKeyObjectOptions { /** * No options. */ } interface ECKeyPairKeyObjectOptions { /** * Name of the curve to use. */ namedCurve: string; } interface RSAKeyPairKeyObjectOptions { /** * Key size in bits */ modulusLength: number; /** * @default 0x10001 */ publicExponent?: number; } interface DSAKeyPairKeyObjectOptions { /** * Key size in bits */ modulusLength: number; /** * Size of q in bits */ divisorLength: number; } interface RSAKeyPairOptions<PubF extends KeyFormat, PrivF extends KeyFormat> { /** * Key size in bits */ modulusLength: number; /** * @default 0x10001 */ publicExponent?: number; publicKeyEncoding: { type: 'pkcs1' | 'spki'; format: PubF; }; privateKeyEncoding: BasePrivateKeyEncodingOptions<PrivF> & { type: 'pkcs1' | 'pkcs8'; }; } interface DSAKeyPairOptions<PubF extends KeyFormat, PrivF extends KeyFormat> { /** * Key size in bits */ modulusLength: number; /** * Size of q in bits */ divisorLength: number; publicKeyEncoding: { type: 'spki'; format: PubF; }; privateKeyEncoding: BasePrivateKeyEncodingOptions<PrivF> & { type: 'pkcs8'; }; } interface ECKeyPairOptions<PubF extends KeyFormat, PrivF extends KeyFormat> { /** * Name of the curve to use. */ namedCurve: string; publicKeyEncoding: { type: 'pkcs1' | 'spki'; format: PubF; }; privateKeyEncoding: BasePrivateKeyEncodingOptions<PrivF> & { type: 'sec1' | 'pkcs8'; }; } interface ED25519KeyPairOptions<PubF extends KeyFormat, PrivF extends KeyFormat> { publicKeyEncoding: { type: 'spki'; format: PubF; }; privateKeyEncoding: BasePrivateKeyEncodingOptions<PrivF> & { type: 'pkcs8'; }; } interface ED448KeyPairOptions<PubF extends KeyFormat, PrivF extends KeyFormat> { publicKeyEncoding: { type: 'spki'; format: PubF; }; privateKeyEncoding: BasePrivateKeyEncodingOptions<PrivF> & { type: 'pkcs8'; }; } interface X25519KeyPairOptions<PubF extends KeyFormat, PrivF extends KeyFormat> { publicKeyEncoding: { type: 'spki'; format: PubF; }; privateKeyEncoding: BasePrivateKeyEncodingOptions<PrivF> & { type: 'pkcs8'; }; } interface X448KeyPairOptions<PubF extends KeyFormat, PrivF extends KeyFormat> { publicKeyEncoding: { type: 'spki'; format: PubF; }; privateKeyEncoding: BasePrivateKeyEncodingOptions<PrivF> & { type: 'pkcs8'; }; } interface KeyPairSyncResult<T1 extends string | Buffer, T2 extends string | Buffer> { publicKey: T1; privateKey: T2; } function generateKeyPairSync( type: 'rsa', options: RSAKeyPairOptions<'pem', 'pem'>, ): KeyPairSyncResult<string, string>; function generateKeyPairSync( type: 'rsa', options: RSAKeyPairOptions<'pem', 'der'>, ): KeyPairSyncResult<string, Buffer>; function generateKeyPairSync( type: 'rsa', options: RSAKeyPairOptions<'der', 'pem'>, ): KeyPairSyncResult<Buffer, string>; function generateKeyPairSync( type: 'rsa', options: RSAKeyPairOptions<'der', 'der'>, ): KeyPairSyncResult<Buffer, Buffer>; function generateKeyPairSync(type: 'rsa', options: RSAKeyPairKeyObjectOptions): KeyPairKeyObjectResult; function generateKeyPairSync( type: 'dsa', options: DSAKeyPairOptions<'pem', 'pem'>, ): KeyPairSyncResult<string, string>; function generateKeyPairSync( type: 'dsa', options: DSAKeyPairOptions<'pem', 'der'>, ): KeyPairSyncResult<string, Buffer>; function generateKeyPairSync( type: 'dsa', options: DSAKeyPairOptions<'der', 'pem'>, ): KeyPairSyncResult<Buffer, string>; function generateKeyPairSync( type: 'dsa', options: DSAKeyPairOptions<'der', 'der'>, ): KeyPairSyncResult<Buffer, Buffer>; function generateKeyPairSync(type: 'dsa', options: DSAKeyPairKeyObjectOptions): KeyPairKeyObjectResult; function generateKeyPairSync( type: 'ec', options: ECKeyPairOptions<'pem', 'pem'>, ): KeyPairSyncResult<string, string>; function generateKeyPairSync( type: 'ec', options: ECKeyPairOptions<'pem', 'der'>, ): KeyPairSyncResult<string, Buffer>; function generateKeyPairSync( type: 'ec', options: ECKeyPairOptions<'der', 'pem'>, ): KeyPairSyncResult<Buffer, string>; function generateKeyPairSync( type: 'ec', options: ECKeyPairOptions<'der', 'der'>, ): KeyPairSyncResult<Buffer, Buffer>; function generateKeyPairSync(type: 'ec', options: ECKeyPairKeyObjectOptions): KeyPairKeyObjectResult; function generateKeyPairSync( type: 'ed25519', options: ED25519KeyPairOptions<'pem', 'pem'>, ): KeyPairSyncResult<string, string>; function generateKeyPairSync( type: 'ed25519', options: ED25519KeyPairOptions<'pem', 'der'>, ): KeyPairSyncResult<string, Buffer>; function generateKeyPairSync( type: 'ed25519', options: ED25519KeyPairOptions<'der', 'pem'>, ): KeyPairSyncResult<Buffer, string>; function generateKeyPairSync( type: 'ed25519', options: ED25519KeyPairOptions<'der', 'der'>, ): KeyPairSyncResult<Buffer, Buffer>; function generateKeyPairSync(type: 'ed25519', options?: ED25519KeyPairKeyObjectOptions): KeyPairKeyObjectResult; function generateKeyPairSync( type: 'ed448', options: ED448KeyPairOptions<'pem', 'pem'>, ): KeyPairSyncResult<string, string>; function generateKeyPairSync( type: 'ed448', options: ED448KeyPairOptions<'pem', 'der'>, ): KeyPairSyncResult<string, Buffer>; function generateKeyPairSync( type: 'ed448', options: ED448KeyPairOptions<'der', 'pem'>, ): KeyPairSyncResult<Buffer, string>; function generateKeyPairSync( type: 'ed448', options: ED448KeyPairOptions<'der', 'der'>, ): KeyPairSyncResult<Buffer, Buffer>; function generateKeyPairSync(type: 'ed448', options?: ED448KeyPairKeyObjectOptions): KeyPairKeyObjectResult; function generateKeyPairSync( type: 'x25519', options: X25519KeyPairOptions<'pem', 'pem'>, ): KeyPairSyncResult<string, string>; function generateKeyPairSync( type: 'x25519', options: X25519KeyPairOptions<'pem', 'der'>, ): KeyPairSyncResult<string, Buffer>; function generateKeyPairSync( type: 'x25519', options: X25519KeyPairOptions<'der', 'pem'>, ): KeyPairSyncResult<Buffer, string>; function generateKeyPairSync( type: 'x25519', options: X25519KeyPairOptions<'der', 'der'>, ): KeyPairSyncResult<Buffer, Buffer>; function generateKeyPairSync(type: 'x25519', options?: X25519KeyPairKeyObjectOptions): KeyPairKeyObjectResult; function generateKeyPairSync( type: 'x448', options: X448KeyPairOptions<'pem', 'pem'>, ): KeyPairSyncResult<string, string>; function generateKeyPairSync( type: 'x448', options: X448KeyPairOptions<'pem', 'der'>, ): KeyPairSyncResult<string, Buffer>; function generateKeyPairSync( type: 'x448', options: X448KeyPairOptions<'der', 'pem'>, ): KeyPairSyncResult<Buffer, string>; function generateKeyPairSync( type: 'x448', options: X448KeyPairOptions<'der', 'der'>, ): KeyPairSyncResult<Buffer, Buffer>; function generateKeyPairSync(type: 'x448', options?: X448KeyPairKeyObjectOptions): KeyPairKeyObjectResult; function generateKeyPair( type: 'rsa', options: RSAKeyPairOptions<'pem', 'pem'>, callback: (err: Error | null, publicKey: string, privateKey: string) => void, ): void; function generateKeyPair( type: 'rsa', options: RSAKeyPairOptions<'pem', 'der'>, callback: (err: Error | null, publicKey: string, privateKey: Buffer) => void, ): void; function generateKeyPair( type: 'rsa', options: RSAKeyPairOptions<'der', 'pem'>, callback: (err: Error | null, publicKey: Buffer, privateKey: string) => void, ): void; function generateKeyPair( type: 'rsa', options: RSAKeyPairOptions<'der', 'der'>, callback: (err: Error | null, publicKey: Buffer, privateKey: Buffer) => void, ): void; function generateKeyPair( type: 'rsa', options: RSAKeyPairKeyObjectOptions, callback: (err: Error | null, publicKey: KeyObject, privateKey: KeyObject) => void, ): void; function generateKeyPair( type: 'dsa', options: DSAKeyPairOptions<'pem', 'pem'>, callback: (err: Error | null, publicKey: string, privateKey: string) => void, ): void; function generateKeyPair( type: 'dsa', options: DSAKeyPairOptions<'pem', 'der'>, callback: (err: Error | null, publicKey: string, privateKey: Buffer) => void, ): void; function generateKeyPair( type: 'dsa', options: DSAKeyPairOptions<'der', 'pem'>, callback: (err: Error | null, publicKey: Buffer, privateKey: string) => void, ): void; function generateKeyPair( type: 'dsa', options: DSAKeyPairOptions<'der', 'der'>, callback: (err: Error | null, publicKey: Buffer, privateKey: Buffer) => void, ): void; function generateKeyPair( type: 'dsa', options: DSAKeyPairKeyObjectOptions, callback: (err: Error | null, publicKey: KeyObject, privateKey: KeyObject) => void, ): void; function generateKeyPair( type: 'ec', options: ECKeyPairOptions<'pem', 'pem'>, callback: (err: Error | null, publicKey: string, privateKey: string) => void, ): void; function generateKeyPair( type: 'ec', options: ECKeyPairOptions<'pem', 'der'>, callback: (err: Error | null, publicKey: string, privateKey: Buffer) => void, ): void; function generateKeyPair( type: 'ec', options: ECKeyPairOptions<'der', 'pem'>, callback: (err: Error | null, publicKey: Buffer, privateKey: string) => void, ): void; function generateKeyPair( type: 'ec', options: ECKeyPairOptions<'der', 'der'>, callback: (err: Error | null, publicKey: Buffer, privateKey: Buffer) => void, ): void; function generateKeyPair( type: 'ec', options: ECKeyPairKeyObjectOptions, callback: (err: Error | null, publicKey: KeyObject, privateKey: KeyObject) => void, ): void; function generateKeyPair( type: 'ed25519', options: ED25519KeyPairOptions<'pem', 'pem'>, callback: (err: Error | null, publicKey: string, privateKey: string) => void, ): void; function generateKeyPair( type: 'ed25519', options: ED25519KeyPairOptions<'pem', 'der'>, callback: (err: Error | null, publicKey: string, privateKey: Buffer) => void, ): void; function generateKeyPair( type: 'ed25519', options: ED25519KeyPairOptions<'der', 'pem'>, callback: (err: Error | null, publicKey: Buffer, privateKey: string) => void, ): void; function generateKeyPair( type: 'ed25519', options: ED25519KeyPairOptions<'der', 'der'>, callback: (err: Error | null, publicKey: Buffer, privateKey: Buffer) => void, ): void; function generateKeyPair( type: 'ed25519', options: ED25519KeyPairKeyObjectOptions | undefined, callback: (err: Error | null, publicKey: KeyObject, privateKey: KeyObject) => void, ): void; function generateKeyPair( type: 'ed448', options: ED448KeyPairOptions<'pem', 'pem'>, callback: (err: Error | null, publicKey: string, privateKey: string) => void, ): void; function generateKeyPair( type: 'ed448', options: ED448KeyPairOptions<'pem', 'der'>, callback: (err: Error | null, publicKey: string, privateKey: Buffer) => void, ): void; function generateKeyPair( type: 'ed448', options: ED448KeyPairOptions<'der', 'pem'>, callback: (err: Error | null, publicKey: Buffer, privateKey: string) => void, ): void; function generateKeyPair( type: 'ed448', options: ED448KeyPairOptions<'der', 'der'>, callback: (err: Error | null, publicKey: Buffer, privateKey: Buffer) => void, ): void; function generateKeyPair( type: 'ed448', options: ED448KeyPairKeyObjectOptions | undefined, callback: (err: Error | null, publicKey: KeyObject, privateKey: KeyObject) => void, ): void; function generateKeyPair( type: 'x25519', options: X25519KeyPairOptions<'pem', 'pem'>, callback: (err: Error | null, publicKey: string, privateKey: string) => void, ): void; function generateKeyPair( type: 'x25519', options: X25519KeyPairOptions<'pem', 'der'>, callback: (err: Error | null, publicKey: string, privateKey: Buffer) => void, ): void; function generateKeyPair( type: 'x25519', options: X25519KeyPairOptions<'der', 'pem'>, callback: (err: Error | null, publicKey: Buffer, privateKey: string) => void, ): void; function generateKeyPair( type: 'x25519', options: X25519KeyPairOptions<'der', 'der'>, callback: (err: Error | null, publicKey: Buffer, privateKey: Buffer) => void, ): void; function generateKeyPair( type: 'x25519', options: X25519KeyPairKeyObjectOptions | undefined, callback: (err: Error | null, publicKey: KeyObject, privateKey: KeyObject) => void, ): void; function generateKeyPair( type: 'x448', options: X448KeyPairOptions<'pem', 'pem'>, callback: (err: Error | null, publicKey: string, privateKey: string) => void, ): void; function generateKeyPair( type: 'x448', options: X448KeyPairOptions<'pem', 'der'>, callback: (err: Error | null, publicKey: string, privateKey: Buffer) => void, ): void; function generateKeyPair( type: 'x448', options: X448KeyPairOptions<'der', 'pem'>, callback: (err: Error | null, publicKey: Buffer, privateKey: string) => void, ): void; function generateKeyPair( type: 'x448', options: X448KeyPairOptions<'der', 'der'>, callback: (err: Error | null, publicKey: Buffer, privateKey: Buffer) => void, ): void; function generateKeyPair( type: 'x448', options: X448KeyPairKeyObjectOptions | undefined, callback: (err: Error | null, publicKey: KeyObject, privateKey: KeyObject) => void, ): void; namespace generateKeyPair { function __promisify__( type: 'rsa', options: RSAKeyPairOptions<'pem', 'pem'>, ): Promise<{ publicKey: string; privateKey: string }>; function __promisify__( type: 'rsa', options: RSAKeyPairOptions<'pem', 'der'>, ): Promise<{ publicKey: string; privateKey: Buffer }>; function __promisify__( type: 'rsa', options: RSAKeyPairOptions<'der', 'pem'>, ): Promise<{ publicKey: Buffer; privateKey: string }>; function __promisify__( type: 'rsa', options: RSAKeyPairOptions<'der', 'der'>, ): Promise<{ publicKey: Buffer; privateKey: Buffer }>; function __promisify__(type: 'rsa', options: RSAKeyPairKeyObjectOptions): Promise<KeyPairKeyObjectResult>; function __promisify__( type: 'dsa', options: DSAKeyPairOptions<'pem', 'pem'>, ): Promise<{ publicKey: string; privateKey: string }>; function __promisify__( type: 'dsa', options: DSAKeyPairOptions<'pem', 'der'>, ): Promise<{ publicKey: string; privateKey: Buffer }>; function __promisify__( type: 'dsa', options: DSAKeyPairOptions<'der', 'pem'>, ): Promise<{ publicKey: Buffer; privateKey: string }>; function __promisify__( type: 'dsa', options: DSAKeyPairOptions<'der', 'der'>, ): Promise<{ publicKey: Buffer; privateKey: Buffer }>; function __promisify__(type: 'dsa', options: DSAKeyPairKeyObjectOptions): Promise<KeyPairKeyObjectResult>; function __promisify__( type: 'ec', options: ECKeyPairOptions<'pem', 'pem'>, ): Promise<{ publicKey: string; privateKey: string }>; function __promisify__( type: 'ec', options: ECKeyPairOptions<'pem', 'der'>, ): Promise<{ publicKey: string; privateKey: Buffer }>; function __promisify__( type: 'ec', options: ECKeyPairOptions<'der', 'pem'>, ): Promise<{ publicKey: Buffer; privateKey: string }>; function __promisify__( type: 'ec', options: ECKeyPairOptions<'der', 'der'>, ): Promise<{ publicKey: Buffer; privateKey: Buffer }>; function __promisify__(type: 'ec', options: ECKeyPairKeyObjectOptions): Promise<KeyPairKeyObjectResult>; function __promisify__( type: 'ed25519', options: ED25519KeyPairOptions<'pem', 'pem'>, ): Promise<{ publicKey: string; privateKey: string }>; function __promisify__( type: 'ed25519', options: ED25519KeyPairOptions<'pem', 'der'>, ): Promise<{ publicKey: string; privateKey: Buffer }>; function __promisify__( type: 'ed25519', options: ED25519KeyPairOptions<'der', 'pem'>, ): Promise<{ publicKey: Buffer; privateKey: string }>; function __promisify__( type: 'ed25519', options: ED25519KeyPairOptions<'der', 'der'>, ): Promise<{ publicKey: Buffer; privateKey: Buffer }>; function __promisify__( type: 'ed25519', options?: ED25519KeyPairKeyObjectOptions, ): Promise<KeyPairKeyObjectResult>; function __promisify__( type: 'ed448', options: ED448KeyPairOptions<'pem', 'pem'>, ): Promise<{ publicKey: string; privateKey: string }>; function __promisify__( type: 'ed448', options: ED448KeyPairOptions<'pem', 'der'>, ): Promise<{ publicKey: string; privateKey: Buffer }>; function __promisify__( type: 'ed448', options: ED448KeyPairOptions<'der', 'pem'>, ): Promise<{ publicKey: Buffer; privateKey: string }>; function __promisify__( type: 'ed448', options: ED448KeyPairOptions<'der', 'der'>, ): Promise<{ publicKey: Buffer; privateKey: Buffer }>; function __promisify__(type: 'ed448', options?: ED448KeyPairKeyObjectOptions): Promise<KeyPairKeyObjectResult>; function __promisify__( type: 'x25519', options: X25519KeyPairOptions<'pem', 'pem'>, ): Promise<{ publicKey: string; privateKey: string }>; function __promisify__( type: 'x25519', options: X25519KeyPairOptions<'pem', 'der'>, ): Promise<{ publicKey: string; privateKey: Buffer }>; function __promisify__( type: 'x25519', options: X25519KeyPairOptions<'der', 'pem'>, ): Promise<{ publicKey: Buffer; privateKey: string }>; function __promisify__( type: 'x25519', options: X25519KeyPairOptions<'der', 'der'>, ): Promise<{ publicKey: Buffer; privateKey: Buffer }>; function __promisify__( type: 'x25519', options?: X25519KeyPairKeyObjectOptions, ): Promise<KeyPairKeyObjectResult>; function __promisify__( type: 'x448', options: X448KeyPairOptions<'pem', 'pem'>, ): Promise<{ publicKey: string; privateKey: string }>; function __promisify__( type: 'x448', options: X448KeyPairOptions<'pem', 'der'>, ): Promise<{ publicKey: string; privateKey: Buffer }>; function __promisify__( type: 'x448', options: X448KeyPairOptions<'der', 'pem'>, ): Promise<{ publicKey: Buffer; privateKey: string }>; function __promisify__( type: 'x448', options: X448KeyPairOptions<'der', 'der'>, ): Promise<{ publicKey: Buffer; privateKey: Buffer }>; function __promisify__(type: 'x448', options?: X448KeyPairKeyObjectOptions): Promise<KeyPairKeyObjectResult>; } /** * Calculates and returns the signature for `data` using the given private key and * algorithm. If `algorithm` is `null` or `undefined`, then the algorithm is * dependent upon the key type (especially Ed25519 and Ed448). * * If `key` is not a [`KeyObject`][], this function behaves as if `key` had been * passed to [`crypto.createPrivateKey()`][]. */ function sign( algorithm: string | null | undefined, data: NodeJS.ArrayBufferView, key: KeyLike | SignKeyObjectInput | SignPrivateKeyInput, ): Buffer; /** * Calculates and returns the signature for `data` using the given private key and * algorithm. If `algorithm` is `null` or `undefined`, then the algorithm is * dependent upon the key type (especially Ed25519 and Ed448). * * If `key` is not a [`KeyObject`][], this function behaves as if `key` had been * passed to [`crypto.createPublicKey()`][]. */ function verify( algorithm: string | null | undefined, data: NodeJS.ArrayBufferView, key: KeyLike | VerifyKeyObjectInput | VerifyPublicKeyInput, signature: NodeJS.ArrayBufferView, ): boolean; /** * Computes the Diffie-Hellman secret based on a privateKey and a publicKey. * Both keys must have the same asymmetricKeyType, which must be one of * 'dh' (for Diffie-Hellman), 'ec' (for ECDH), 'x448', or 'x25519' (for ECDH-ES). */ function diffieHellman(options: { privateKey: KeyObject; publicKey: KeyObject }): Buffer; } apollo-server-demo/node_modules/@types/node/cluster.d.ts 0000644 0001750 0000144 00000037375 14000133700 023076 0 ustar andreh users declare module "cluster" { import * as child from "child_process"; import * as events from "events"; import * as net from "net"; // interfaces interface ClusterSettings { execArgv?: string[]; // default: process.execArgv exec?: string; args?: string[]; silent?: boolean; stdio?: any[]; uid?: number; gid?: number; inspectPort?: number | (() => number); } interface Address { address: string; port: number; addressType: number | "udp4" | "udp6"; // 4, 6, -1, "udp4", "udp6" } class Worker extends events.EventEmitter { id: number; process: child.ChildProcess; send(message: child.Serializable, sendHandle?: child.SendHandle, callback?: (error: Error | null) => void): boolean; kill(signal?: string): void; destroy(signal?: string): void; disconnect(): void; isConnected(): boolean; isDead(): boolean; exitedAfterDisconnect: boolean; /** * events.EventEmitter * 1. disconnect * 2. error * 3. exit * 4. listening * 5. message * 6. online */ addListener(event: string, listener: (...args: any[]) => void): this; addListener(event: "disconnect", listener: () => void): this; addListener(event: "error", listener: (error: Error) => void): this; addListener(event: "exit", listener: (code: number, signal: string) => void): this; addListener(event: "listening", listener: (address: Address) => void): this; addListener(event: "message", listener: (message: any, handle: net.Socket | net.Server) => void): this; // the handle is a net.Socket or net.Server object, or undefined. addListener(event: "online", listener: () => void): this; emit(event: string | symbol, ...args: any[]): boolean; emit(event: "disconnect"): boolean; emit(event: "error", error: Error): boolean; emit(event: "exit", code: number, signal: string): boolean; emit(event: "listening", address: Address): boolean; emit(event: "message", message: any, handle: net.Socket | net.Server): boolean; emit(event: "online"): boolean; on(event: string, listener: (...args: any[]) => void): this; on(event: "disconnect", listener: () => void): this; on(event: "error", listener: (error: Error) => void): this; on(event: "exit", listener: (code: number, signal: string) => void): this; on(event: "listening", listener: (address: Address) => void): this; on(event: "message", listener: (message: any, handle: net.Socket | net.Server) => void): this; // the handle is a net.Socket or net.Server object, or undefined. on(event: "online", listener: () => void): this; once(event: string, listener: (...args: any[]) => void): this; once(event: "disconnect", listener: () => void): this; once(event: "error", listener: (error: Error) => void): this; once(event: "exit", listener: (code: number, signal: string) => void): this; once(event: "listening", listener: (address: Address) => void): this; once(event: "message", listener: (message: any, handle: net.Socket | net.Server) => void): this; // the handle is a net.Socket or net.Server object, or undefined. once(event: "online", listener: () => void): this; prependListener(event: string, listener: (...args: any[]) => void): this; prependListener(event: "disconnect", listener: () => void): this; prependListener(event: "error", listener: (error: Error) => void): this; prependListener(event: "exit", listener: (code: number, signal: string) => void): this; prependListener(event: "listening", listener: (address: Address) => void): this; prependListener(event: "message", listener: (message: any, handle: net.Socket | net.Server) => void): this; // the handle is a net.Socket or net.Server object, or undefined. prependListener(event: "online", listener: () => void): this; prependOnceListener(event: string, listener: (...args: any[]) => void): this; prependOnceListener(event: "disconnect", listener: () => void): this; prependOnceListener(event: "error", listener: (error: Error) => void): this; prependOnceListener(event: "exit", listener: (code: number, signal: string) => void): this; prependOnceListener(event: "listening", listener: (address: Address) => void): this; prependOnceListener(event: "message", listener: (message: any, handle: net.Socket | net.Server) => void): this; // the handle is a net.Socket or net.Server object, or undefined. prependOnceListener(event: "online", listener: () => void): this; } interface Cluster extends events.EventEmitter { Worker: Worker; disconnect(callback?: () => void): void; fork(env?: any): Worker; isMaster: boolean; isWorker: boolean; schedulingPolicy: number; settings: ClusterSettings; setupMaster(settings?: ClusterSettings): void; worker?: Worker; workers?: NodeJS.Dict<Worker>; readonly SCHED_NONE: number; readonly SCHED_RR: number; /** * events.EventEmitter * 1. disconnect * 2. exit * 3. fork * 4. listening * 5. message * 6. online * 7. setup */ addListener(event: string, listener: (...args: any[]) => void): this; addListener(event: "disconnect", listener: (worker: Worker) => void): this; addListener(event: "exit", listener: (worker: Worker, code: number, signal: string) => void): this; addListener(event: "fork", listener: (worker: Worker) => void): this; addListener(event: "listening", listener: (worker: Worker, address: Address) => void): this; addListener(event: "message", listener: (worker: Worker, message: any, handle: net.Socket | net.Server) => void): this; // the handle is a net.Socket or net.Server object, or undefined. addListener(event: "online", listener: (worker: Worker) => void): this; addListener(event: "setup", listener: (settings: ClusterSettings) => void): this; emit(event: string | symbol, ...args: any[]): boolean; emit(event: "disconnect", worker: Worker): boolean; emit(event: "exit", worker: Worker, code: number, signal: string): boolean; emit(event: "fork", worker: Worker): boolean; emit(event: "listening", worker: Worker, address: Address): boolean; emit(event: "message", worker: Worker, message: any, handle: net.Socket | net.Server): boolean; emit(event: "online", worker: Worker): boolean; emit(event: "setup", settings: ClusterSettings): boolean; on(event: string, listener: (...args: any[]) => void): this; on(event: "disconnect", listener: (worker: Worker) => void): this; on(event: "exit", listener: (worker: Worker, code: number, signal: string) => void): this; on(event: "fork", listener: (worker: Worker) => void): this; on(event: "listening", listener: (worker: Worker, address: Address) => void): this; on(event: "message", listener: (worker: Worker, message: any, handle: net.Socket | net.Server) => void): this; // the handle is a net.Socket or net.Server object, or undefined. on(event: "online", listener: (worker: Worker) => void): this; on(event: "setup", listener: (settings: ClusterSettings) => void): this; once(event: string, listener: (...args: any[]) => void): this; once(event: "disconnect", listener: (worker: Worker) => void): this; once(event: "exit", listener: (worker: Worker, code: number, signal: string) => void): this; once(event: "fork", listener: (worker: Worker) => void): this; once(event: "listening", listener: (worker: Worker, address: Address) => void): this; once(event: "message", listener: (worker: Worker, message: any, handle: net.Socket | net.Server) => void): this; // the handle is a net.Socket or net.Server object, or undefined. once(event: "online", listener: (worker: Worker) => void): this; once(event: "setup", listener: (settings: ClusterSettings) => void): this; prependListener(event: string, listener: (...args: any[]) => void): this; prependListener(event: "disconnect", listener: (worker: Worker) => void): this; prependListener(event: "exit", listener: (worker: Worker, code: number, signal: string) => void): this; prependListener(event: "fork", listener: (worker: Worker) => void): this; prependListener(event: "listening", listener: (worker: Worker, address: Address) => void): this; prependListener(event: "message", listener: (worker: Worker, message: any, handle: net.Socket | net.Server) => void): this; // the handle is a net.Socket or net.Server object, or undefined. prependListener(event: "online", listener: (worker: Worker) => void): this; prependListener(event: "setup", listener: (settings: ClusterSettings) => void): this; prependOnceListener(event: string, listener: (...args: any[]) => void): this; prependOnceListener(event: "disconnect", listener: (worker: Worker) => void): this; prependOnceListener(event: "exit", listener: (worker: Worker, code: number, signal: string) => void): this; prependOnceListener(event: "fork", listener: (worker: Worker) => void): this; prependOnceListener(event: "listening", listener: (worker: Worker, address: Address) => void): this; // the handle is a net.Socket or net.Server object, or undefined. prependOnceListener(event: "message", listener: (worker: Worker, message: any, handle: net.Socket | net.Server) => void): this; prependOnceListener(event: "online", listener: (worker: Worker) => void): this; prependOnceListener(event: "setup", listener: (settings: ClusterSettings) => void): this; } const SCHED_NONE: number; const SCHED_RR: number; function disconnect(callback?: () => void): void; function fork(env?: any): Worker; const isMaster: boolean; const isWorker: boolean; let schedulingPolicy: number; const settings: ClusterSettings; function setupMaster(settings?: ClusterSettings): void; const worker: Worker; const workers: NodeJS.Dict<Worker>; /** * events.EventEmitter * 1. disconnect * 2. exit * 3. fork * 4. listening * 5. message * 6. online * 7. setup */ function addListener(event: string, listener: (...args: any[]) => void): Cluster; function addListener(event: "disconnect", listener: (worker: Worker) => void): Cluster; function addListener(event: "exit", listener: (worker: Worker, code: number, signal: string) => void): Cluster; function addListener(event: "fork", listener: (worker: Worker) => void): Cluster; function addListener(event: "listening", listener: (worker: Worker, address: Address) => void): Cluster; // the handle is a net.Socket or net.Server object, or undefined. function addListener(event: "message", listener: (worker: Worker, message: any, handle: net.Socket | net.Server) => void): Cluster; function addListener(event: "online", listener: (worker: Worker) => void): Cluster; function addListener(event: "setup", listener: (settings: ClusterSettings) => void): Cluster; function emit(event: string | symbol, ...args: any[]): boolean; function emit(event: "disconnect", worker: Worker): boolean; function emit(event: "exit", worker: Worker, code: number, signal: string): boolean; function emit(event: "fork", worker: Worker): boolean; function emit(event: "listening", worker: Worker, address: Address): boolean; function emit(event: "message", worker: Worker, message: any, handle: net.Socket | net.Server): boolean; function emit(event: "online", worker: Worker): boolean; function emit(event: "setup", settings: ClusterSettings): boolean; function on(event: string, listener: (...args: any[]) => void): Cluster; function on(event: "disconnect", listener: (worker: Worker) => void): Cluster; function on(event: "exit", listener: (worker: Worker, code: number, signal: string) => void): Cluster; function on(event: "fork", listener: (worker: Worker) => void): Cluster; function on(event: "listening", listener: (worker: Worker, address: Address) => void): Cluster; function on(event: "message", listener: (worker: Worker, message: any, handle: net.Socket | net.Server) => void): Cluster; // the handle is a net.Socket or net.Server object, or undefined. function on(event: "online", listener: (worker: Worker) => void): Cluster; function on(event: "setup", listener: (settings: ClusterSettings) => void): Cluster; function once(event: string, listener: (...args: any[]) => void): Cluster; function once(event: "disconnect", listener: (worker: Worker) => void): Cluster; function once(event: "exit", listener: (worker: Worker, code: number, signal: string) => void): Cluster; function once(event: "fork", listener: (worker: Worker) => void): Cluster; function once(event: "listening", listener: (worker: Worker, address: Address) => void): Cluster; function once(event: "message", listener: (worker: Worker, message: any, handle: net.Socket | net.Server) => void): Cluster; // the handle is a net.Socket or net.Server object, or undefined. function once(event: "online", listener: (worker: Worker) => void): Cluster; function once(event: "setup", listener: (settings: ClusterSettings) => void): Cluster; function removeListener(event: string, listener: (...args: any[]) => void): Cluster; function removeAllListeners(event?: string): Cluster; function setMaxListeners(n: number): Cluster; function getMaxListeners(): number; function listeners(event: string): Function[]; function listenerCount(type: string): number; function prependListener(event: string, listener: (...args: any[]) => void): Cluster; function prependListener(event: "disconnect", listener: (worker: Worker) => void): Cluster; function prependListener(event: "exit", listener: (worker: Worker, code: number, signal: string) => void): Cluster; function prependListener(event: "fork", listener: (worker: Worker) => void): Cluster; function prependListener(event: "listening", listener: (worker: Worker, address: Address) => void): Cluster; // the handle is a net.Socket or net.Server object, or undefined. function prependListener(event: "message", listener: (worker: Worker, message: any, handle: net.Socket | net.Server) => void): Cluster; function prependListener(event: "online", listener: (worker: Worker) => void): Cluster; function prependListener(event: "setup", listener: (settings: ClusterSettings) => void): Cluster; function prependOnceListener(event: string, listener: (...args: any[]) => void): Cluster; function prependOnceListener(event: "disconnect", listener: (worker: Worker) => void): Cluster; function prependOnceListener(event: "exit", listener: (worker: Worker, code: number, signal: string) => void): Cluster; function prependOnceListener(event: "fork", listener: (worker: Worker) => void): Cluster; function prependOnceListener(event: "listening", listener: (worker: Worker, address: Address) => void): Cluster; // the handle is a net.Socket or net.Server object, or undefined. function prependOnceListener(event: "message", listener: (worker: Worker, message: any, handle: net.Socket | net.Server) => void): Cluster; function prependOnceListener(event: "online", listener: (worker: Worker) => void): Cluster; function prependOnceListener(event: "setup", listener: (settings: ClusterSettings) => void): Cluster; function eventNames(): string[]; } apollo-server-demo/node_modules/@types/node/fs.d.ts 0000644 0001750 0000144 00000346120 14000133700 022014 0 ustar andreh users declare module "fs" { import * as stream from "stream"; import * as events from "events"; import { URL } from "url"; import * as promises from 'fs/promises'; export { promises }; /** * Valid types for path values in "fs". */ export type PathLike = string | Buffer | URL; export type NoParamCallback = (err: NodeJS.ErrnoException | null) => void; export type BufferEncodingOption = 'buffer' | { encoding: 'buffer' }; export interface BaseEncodingOptions { encoding?: BufferEncoding | null; } export type OpenMode = number | string; export type Mode = number | string; export interface StatsBase<T> { isFile(): boolean; isDirectory(): boolean; isBlockDevice(): boolean; isCharacterDevice(): boolean; isSymbolicLink(): boolean; isFIFO(): boolean; isSocket(): boolean; dev: T; ino: T; mode: T; nlink: T; uid: T; gid: T; rdev: T; size: T; blksize: T; blocks: T; atimeMs: T; mtimeMs: T; ctimeMs: T; birthtimeMs: T; atime: Date; mtime: Date; ctime: Date; birthtime: Date; } export interface Stats extends StatsBase<number> { } export class Stats { } export class Dirent { isFile(): boolean; isDirectory(): boolean; isBlockDevice(): boolean; isCharacterDevice(): boolean; isSymbolicLink(): boolean; isFIFO(): boolean; isSocket(): boolean; name: string; } /** * A class representing a directory stream. */ export class Dir { readonly path: string; /** * Asynchronously iterates over the directory via `readdir(3)` until all entries have been read. */ [Symbol.asyncIterator](): AsyncIterableIterator<Dirent>; /** * Asynchronously close the directory's underlying resource handle. * Subsequent reads will result in errors. */ close(): Promise<void>; close(cb: NoParamCallback): void; /** * Synchronously close the directory's underlying resource handle. * Subsequent reads will result in errors. */ closeSync(): void; /** * Asynchronously read the next directory entry via `readdir(3)` as an `Dirent`. * After the read is completed, a value is returned that will be resolved with an `Dirent`, or `null` if there are no more directory entries to read. * Directory entries returned by this function are in no particular order as provided by the operating system's underlying directory mechanisms. */ read(): Promise<Dirent | null>; read(cb: (err: NodeJS.ErrnoException | null, dirEnt: Dirent | null) => void): void; /** * Synchronously read the next directory entry via `readdir(3)` as a `Dirent`. * If there are no more directory entries to read, null will be returned. * Directory entries returned by this function are in no particular order as provided by the operating system's underlying directory mechanisms. */ readSync(): Dirent | null; } export interface FSWatcher extends events.EventEmitter { close(): void; /** * events.EventEmitter * 1. change * 2. error */ addListener(event: string, listener: (...args: any[]) => void): this; addListener(event: "change", listener: (eventType: string, filename: string | Buffer) => void): this; addListener(event: "error", listener: (error: Error) => void): this; addListener(event: "close", listener: () => void): this; on(event: string, listener: (...args: any[]) => void): this; on(event: "change", listener: (eventType: string, filename: string | Buffer) => void): this; on(event: "error", listener: (error: Error) => void): this; on(event: "close", listener: () => void): this; once(event: string, listener: (...args: any[]) => void): this; once(event: "change", listener: (eventType: string, filename: string | Buffer) => void): this; once(event: "error", listener: (error: Error) => void): this; once(event: "close", listener: () => void): this; prependListener(event: string, listener: (...args: any[]) => void): this; prependListener(event: "change", listener: (eventType: string, filename: string | Buffer) => void): this; prependListener(event: "error", listener: (error: Error) => void): this; prependListener(event: "close", listener: () => void): this; prependOnceListener(event: string, listener: (...args: any[]) => void): this; prependOnceListener(event: "change", listener: (eventType: string, filename: string | Buffer) => void): this; prependOnceListener(event: "error", listener: (error: Error) => void): this; prependOnceListener(event: "close", listener: () => void): this; } export class ReadStream extends stream.Readable { close(): void; bytesRead: number; path: string | Buffer; pending: boolean; /** * events.EventEmitter * 1. open * 2. close * 3. ready */ addListener(event: "close", listener: () => void): this; addListener(event: "data", listener: (chunk: Buffer | string) => void): this; addListener(event: "end", listener: () => void): this; addListener(event: "error", listener: (err: Error) => void): this; addListener(event: "open", listener: (fd: number) => void): this; addListener(event: "pause", listener: () => void): this; addListener(event: "readable", listener: () => void): this; addListener(event: "ready", listener: () => void): this; addListener(event: "resume", listener: () => void): this; addListener(event: string | symbol, listener: (...args: any[]) => void): this; on(event: "close", listener: () => void): this; on(event: "data", listener: (chunk: Buffer | string) => void): this; on(event: "end", listener: () => void): this; on(event: "error", listener: (err: Error) => void): this; on(event: "open", listener: (fd: number) => void): this; on(event: "pause", listener: () => void): this; on(event: "readable", listener: () => void): this; on(event: "ready", listener: () => void): this; on(event: "resume", listener: () => void): this; on(event: string | symbol, listener: (...args: any[]) => void): this; once(event: "close", listener: () => void): this; once(event: "data", listener: (chunk: Buffer | string) => void): this; once(event: "end", listener: () => void): this; once(event: "error", listener: (err: Error) => void): this; once(event: "open", listener: (fd: number) => void): this; once(event: "pause", listener: () => void): this; once(event: "readable", listener: () => void): this; once(event: "ready", listener: () => void): this; once(event: "resume", listener: () => void): this; once(event: string | symbol, listener: (...args: any[]) => void): this; prependListener(event: "close", listener: () => void): this; prependListener(event: "data", listener: (chunk: Buffer | string) => void): this; prependListener(event: "end", listener: () => void): this; prependListener(event: "error", listener: (err: Error) => void): this; prependListener(event: "open", listener: (fd: number) => void): this; prependListener(event: "pause", listener: () => void): this; prependListener(event: "readable", listener: () => void): this; prependListener(event: "ready", listener: () => void): this; prependListener(event: "resume", listener: () => void): this; prependListener(event: string | symbol, listener: (...args: any[]) => void): this; prependOnceListener(event: "close", listener: () => void): this; prependOnceListener(event: "data", listener: (chunk: Buffer | string) => void): this; prependOnceListener(event: "end", listener: () => void): this; prependOnceListener(event: "error", listener: (err: Error) => void): this; prependOnceListener(event: "open", listener: (fd: number) => void): this; prependOnceListener(event: "pause", listener: () => void): this; prependOnceListener(event: "readable", listener: () => void): this; prependOnceListener(event: "ready", listener: () => void): this; prependOnceListener(event: "resume", listener: () => void): this; prependOnceListener(event: string | symbol, listener: (...args: any[]) => void): this; } export class WriteStream extends stream.Writable { close(): void; bytesWritten: number; path: string | Buffer; pending: boolean; /** * events.EventEmitter * 1. open * 2. close * 3. ready */ addListener(event: "close", listener: () => void): this; addListener(event: "drain", listener: () => void): this; addListener(event: "error", listener: (err: Error) => void): this; addListener(event: "finish", listener: () => void): this; addListener(event: "open", listener: (fd: number) => void): this; addListener(event: "pipe", listener: (src: stream.Readable) => void): this; addListener(event: "ready", listener: () => void): this; addListener(event: "unpipe", listener: (src: stream.Readable) => void): this; addListener(event: string | symbol, listener: (...args: any[]) => void): this; on(event: "close", listener: () => void): this; on(event: "drain", listener: () => void): this; on(event: "error", listener: (err: Error) => void): this; on(event: "finish", listener: () => void): this; on(event: "open", listener: (fd: number) => void): this; on(event: "pipe", listener: (src: stream.Readable) => void): this; on(event: "ready", listener: () => void): this; on(event: "unpipe", listener: (src: stream.Readable) => void): this; on(event: string | symbol, listener: (...args: any[]) => void): this; once(event: "close", listener: () => void): this; once(event: "drain", listener: () => void): this; once(event: "error", listener: (err: Error) => void): this; once(event: "finish", listener: () => void): this; once(event: "open", listener: (fd: number) => void): this; once(event: "pipe", listener: (src: stream.Readable) => void): this; once(event: "ready", listener: () => void): this; once(event: "unpipe", listener: (src: stream.Readable) => void): this; once(event: string | symbol, listener: (...args: any[]) => void): this; prependListener(event: "close", listener: () => void): this; prependListener(event: "drain", listener: () => void): this; prependListener(event: "error", listener: (err: Error) => void): this; prependListener(event: "finish", listener: () => void): this; prependListener(event: "open", listener: (fd: number) => void): this; prependListener(event: "pipe", listener: (src: stream.Readable) => void): this; prependListener(event: "ready", listener: () => void): this; prependListener(event: "unpipe", listener: (src: stream.Readable) => void): this; prependListener(event: string | symbol, listener: (...args: any[]) => void): this; prependOnceListener(event: "close", listener: () => void): this; prependOnceListener(event: "drain", listener: () => void): this; prependOnceListener(event: "error", listener: (err: Error) => void): this; prependOnceListener(event: "finish", listener: () => void): this; prependOnceListener(event: "open", listener: (fd: number) => void): this; prependOnceListener(event: "pipe", listener: (src: stream.Readable) => void): this; prependOnceListener(event: "ready", listener: () => void): this; prependOnceListener(event: "unpipe", listener: (src: stream.Readable) => void): this; prependOnceListener(event: string | symbol, listener: (...args: any[]) => void): this; } /** * Asynchronous rename(2) - Change the name or location of a file or directory. * @param oldPath A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. * @param newPath A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. */ export function rename(oldPath: PathLike, newPath: PathLike, callback: NoParamCallback): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace rename { /** * Asynchronous rename(2) - Change the name or location of a file or directory. * @param oldPath A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. * @param newPath A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. */ function __promisify__(oldPath: PathLike, newPath: PathLike): Promise<void>; } /** * Synchronous rename(2) - Change the name or location of a file or directory. * @param oldPath A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. * @param newPath A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. */ export function renameSync(oldPath: PathLike, newPath: PathLike): void; /** * Asynchronous truncate(2) - Truncate a file to a specified length. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param len If not specified, defaults to `0`. */ export function truncate(path: PathLike, len: number | undefined | null, callback: NoParamCallback): void; /** * Asynchronous truncate(2) - Truncate a file to a specified length. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. */ export function truncate(path: PathLike, callback: NoParamCallback): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace truncate { /** * Asynchronous truncate(2) - Truncate a file to a specified length. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param len If not specified, defaults to `0`. */ function __promisify__(path: PathLike, len?: number | null): Promise<void>; } /** * Synchronous truncate(2) - Truncate a file to a specified length. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param len If not specified, defaults to `0`. */ export function truncateSync(path: PathLike, len?: number | null): void; /** * Asynchronous ftruncate(2) - Truncate a file to a specified length. * @param fd A file descriptor. * @param len If not specified, defaults to `0`. */ export function ftruncate(fd: number, len: number | undefined | null, callback: NoParamCallback): void; /** * Asynchronous ftruncate(2) - Truncate a file to a specified length. * @param fd A file descriptor. */ export function ftruncate(fd: number, callback: NoParamCallback): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace ftruncate { /** * Asynchronous ftruncate(2) - Truncate a file to a specified length. * @param fd A file descriptor. * @param len If not specified, defaults to `0`. */ function __promisify__(fd: number, len?: number | null): Promise<void>; } /** * Synchronous ftruncate(2) - Truncate a file to a specified length. * @param fd A file descriptor. * @param len If not specified, defaults to `0`. */ export function ftruncateSync(fd: number, len?: number | null): void; /** * Asynchronous chown(2) - Change ownership of a file. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ export function chown(path: PathLike, uid: number, gid: number, callback: NoParamCallback): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace chown { /** * Asynchronous chown(2) - Change ownership of a file. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ function __promisify__(path: PathLike, uid: number, gid: number): Promise<void>; } /** * Synchronous chown(2) - Change ownership of a file. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ export function chownSync(path: PathLike, uid: number, gid: number): void; /** * Asynchronous fchown(2) - Change ownership of a file. * @param fd A file descriptor. */ export function fchown(fd: number, uid: number, gid: number, callback: NoParamCallback): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace fchown { /** * Asynchronous fchown(2) - Change ownership of a file. * @param fd A file descriptor. */ function __promisify__(fd: number, uid: number, gid: number): Promise<void>; } /** * Synchronous fchown(2) - Change ownership of a file. * @param fd A file descriptor. */ export function fchownSync(fd: number, uid: number, gid: number): void; /** * Asynchronous lchown(2) - Change ownership of a file. Does not dereference symbolic links. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ export function lchown(path: PathLike, uid: number, gid: number, callback: NoParamCallback): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace lchown { /** * Asynchronous lchown(2) - Change ownership of a file. Does not dereference symbolic links. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ function __promisify__(path: PathLike, uid: number, gid: number): Promise<void>; } /** * Synchronous lchown(2) - Change ownership of a file. Does not dereference symbolic links. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ export function lchownSync(path: PathLike, uid: number, gid: number): void; /** * Changes the access and modification times of a file in the same way as `fs.utimes()`, * with the difference that if the path refers to a symbolic link, then the link is not * dereferenced: instead, the timestamps of the symbolic link itself are changed. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param atime The last access time. If a string is provided, it will be coerced to number. * @param mtime The last modified time. If a string is provided, it will be coerced to number. */ export function lutimes(path: PathLike, atime: string | number | Date, mtime: string | number | Date, callback: NoParamCallback): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace lutimes { /** * Changes the access and modification times of a file in the same way as `fsPromises.utimes()`, * with the difference that if the path refers to a symbolic link, then the link is not * dereferenced: instead, the timestamps of the symbolic link itself are changed. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param atime The last access time. If a string is provided, it will be coerced to number. * @param mtime The last modified time. If a string is provided, it will be coerced to number. */ function __promisify__(path: PathLike, atime: string | number | Date, mtime: string | number | Date): Promise<void>; } /** * Change the file system timestamps of the symbolic link referenced by `path`. Returns `undefined`, * or throws an exception when parameters are incorrect or the operation fails. * This is the synchronous version of `fs.lutimes()`. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param atime The last access time. If a string is provided, it will be coerced to number. * @param mtime The last modified time. If a string is provided, it will be coerced to number. */ export function lutimesSync(path: PathLike, atime: string | number | Date, mtime: string | number | Date): void; /** * Asynchronous chmod(2) - Change permissions of a file. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param mode A file mode. If a string is passed, it is parsed as an octal integer. */ export function chmod(path: PathLike, mode: Mode, callback: NoParamCallback): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace chmod { /** * Asynchronous chmod(2) - Change permissions of a file. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param mode A file mode. If a string is passed, it is parsed as an octal integer. */ function __promisify__(path: PathLike, mode: Mode): Promise<void>; } /** * Synchronous chmod(2) - Change permissions of a file. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param mode A file mode. If a string is passed, it is parsed as an octal integer. */ export function chmodSync(path: PathLike, mode: Mode): void; /** * Asynchronous fchmod(2) - Change permissions of a file. * @param fd A file descriptor. * @param mode A file mode. If a string is passed, it is parsed as an octal integer. */ export function fchmod(fd: number, mode: Mode, callback: NoParamCallback): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace fchmod { /** * Asynchronous fchmod(2) - Change permissions of a file. * @param fd A file descriptor. * @param mode A file mode. If a string is passed, it is parsed as an octal integer. */ function __promisify__(fd: number, mode: Mode): Promise<void>; } /** * Synchronous fchmod(2) - Change permissions of a file. * @param fd A file descriptor. * @param mode A file mode. If a string is passed, it is parsed as an octal integer. */ export function fchmodSync(fd: number, mode: Mode): void; /** * Asynchronous lchmod(2) - Change permissions of a file. Does not dereference symbolic links. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param mode A file mode. If a string is passed, it is parsed as an octal integer. */ export function lchmod(path: PathLike, mode: Mode, callback: NoParamCallback): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace lchmod { /** * Asynchronous lchmod(2) - Change permissions of a file. Does not dereference symbolic links. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param mode A file mode. If a string is passed, it is parsed as an octal integer. */ function __promisify__(path: PathLike, mode: Mode): Promise<void>; } /** * Synchronous lchmod(2) - Change permissions of a file. Does not dereference symbolic links. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param mode A file mode. If a string is passed, it is parsed as an octal integer. */ export function lchmodSync(path: PathLike, mode: Mode): void; /** * Asynchronous stat(2) - Get file status. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ export function stat(path: PathLike, options: BigIntOptions, callback: (err: NodeJS.ErrnoException | null, stats: BigIntStats) => void): void; export function stat(path: PathLike, options: StatOptions, callback: (err: NodeJS.ErrnoException | null, stats: Stats | BigIntStats) => void): void; export function stat(path: PathLike, callback: (err: NodeJS.ErrnoException | null, stats: Stats) => void): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace stat { /** * Asynchronous stat(2) - Get file status. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ function __promisify__(path: PathLike, options: BigIntOptions): Promise<BigIntStats>; function __promisify__(path: PathLike, options: StatOptions): Promise<Stats | BigIntStats>; function __promisify__(path: PathLike): Promise<Stats>; } /** * Synchronous stat(2) - Get file status. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ export function statSync(path: PathLike, options: BigIntOptions): BigIntStats; export function statSync(path: PathLike, options: StatOptions): Stats | BigIntStats; export function statSync(path: PathLike): Stats; /** * Asynchronous fstat(2) - Get file status. * @param fd A file descriptor. */ export function fstat(fd: number, callback: (err: NodeJS.ErrnoException | null, stats: Stats) => void): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace fstat { /** * Asynchronous fstat(2) - Get file status. * @param fd A file descriptor. */ function __promisify__(fd: number): Promise<Stats>; } /** * Synchronous fstat(2) - Get file status. * @param fd A file descriptor. */ export function fstatSync(fd: number): Stats; /** * Asynchronous lstat(2) - Get file status. Does not dereference symbolic links. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ export function lstat(path: PathLike, callback: (err: NodeJS.ErrnoException | null, stats: Stats) => void): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace lstat { /** * Asynchronous lstat(2) - Get file status. Does not dereference symbolic links. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ function __promisify__(path: PathLike): Promise<Stats>; } /** * Synchronous lstat(2) - Get file status. Does not dereference symbolic links. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ export function lstatSync(path: PathLike): Stats; /** * Asynchronous link(2) - Create a new link (also known as a hard link) to an existing file. * @param existingPath A path to a file. If a URL is provided, it must use the `file:` protocol. * @param newPath A path to a file. If a URL is provided, it must use the `file:` protocol. */ export function link(existingPath: PathLike, newPath: PathLike, callback: NoParamCallback): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace link { /** * Asynchronous link(2) - Create a new link (also known as a hard link) to an existing file. * @param existingPath A path to a file. If a URL is provided, it must use the `file:` protocol. * @param newPath A path to a file. If a URL is provided, it must use the `file:` protocol. */ function __promisify__(existingPath: PathLike, newPath: PathLike): Promise<void>; } /** * Synchronous link(2) - Create a new link (also known as a hard link) to an existing file. * @param existingPath A path to a file. If a URL is provided, it must use the `file:` protocol. * @param newPath A path to a file. If a URL is provided, it must use the `file:` protocol. */ export function linkSync(existingPath: PathLike, newPath: PathLike): void; /** * Asynchronous symlink(2) - Create a new symbolic link to an existing file. * @param target A path to an existing file. If a URL is provided, it must use the `file:` protocol. * @param path A path to the new symlink. If a URL is provided, it must use the `file:` protocol. * @param type May be set to `'dir'`, `'file'`, or `'junction'` (default is `'file'`) and is only available on Windows (ignored on other platforms). * When using `'junction'`, the `target` argument will automatically be normalized to an absolute path. */ export function symlink(target: PathLike, path: PathLike, type: symlink.Type | undefined | null, callback: NoParamCallback): void; /** * Asynchronous symlink(2) - Create a new symbolic link to an existing file. * @param target A path to an existing file. If a URL is provided, it must use the `file:` protocol. * @param path A path to the new symlink. If a URL is provided, it must use the `file:` protocol. */ export function symlink(target: PathLike, path: PathLike, callback: NoParamCallback): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace symlink { /** * Asynchronous symlink(2) - Create a new symbolic link to an existing file. * @param target A path to an existing file. If a URL is provided, it must use the `file:` protocol. * @param path A path to the new symlink. If a URL is provided, it must use the `file:` protocol. * @param type May be set to `'dir'`, `'file'`, or `'junction'` (default is `'file'`) and is only available on Windows (ignored on other platforms). * When using `'junction'`, the `target` argument will automatically be normalized to an absolute path. */ function __promisify__(target: PathLike, path: PathLike, type?: string | null): Promise<void>; type Type = "dir" | "file" | "junction"; } /** * Synchronous symlink(2) - Create a new symbolic link to an existing file. * @param target A path to an existing file. If a URL is provided, it must use the `file:` protocol. * @param path A path to the new symlink. If a URL is provided, it must use the `file:` protocol. * @param type May be set to `'dir'`, `'file'`, or `'junction'` (default is `'file'`) and is only available on Windows (ignored on other platforms). * When using `'junction'`, the `target` argument will automatically be normalized to an absolute path. */ export function symlinkSync(target: PathLike, path: PathLike, type?: symlink.Type | null): void; /** * Asynchronous readlink(2) - read value of a symbolic link. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ export function readlink( path: PathLike, options: BaseEncodingOptions | BufferEncoding | undefined | null, callback: (err: NodeJS.ErrnoException | null, linkString: string) => void ): void; /** * Asynchronous readlink(2) - read value of a symbolic link. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ export function readlink(path: PathLike, options: BufferEncodingOption, callback: (err: NodeJS.ErrnoException | null, linkString: Buffer) => void): void; /** * Asynchronous readlink(2) - read value of a symbolic link. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ export function readlink(path: PathLike, options: BaseEncodingOptions | string | undefined | null, callback: (err: NodeJS.ErrnoException | null, linkString: string | Buffer) => void): void; /** * Asynchronous readlink(2) - read value of a symbolic link. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ export function readlink(path: PathLike, callback: (err: NodeJS.ErrnoException | null, linkString: string) => void): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace readlink { /** * Asynchronous readlink(2) - read value of a symbolic link. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ function __promisify__(path: PathLike, options?: BaseEncodingOptions | BufferEncoding | null): Promise<string>; /** * Asynchronous readlink(2) - read value of a symbolic link. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ function __promisify__(path: PathLike, options: BufferEncodingOption): Promise<Buffer>; /** * Asynchronous readlink(2) - read value of a symbolic link. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ function __promisify__(path: PathLike, options?: BaseEncodingOptions | string | null): Promise<string | Buffer>; } /** * Synchronous readlink(2) - read value of a symbolic link. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ export function readlinkSync(path: PathLike, options?: BaseEncodingOptions | BufferEncoding | null): string; /** * Synchronous readlink(2) - read value of a symbolic link. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ export function readlinkSync(path: PathLike, options: BufferEncodingOption): Buffer; /** * Synchronous readlink(2) - read value of a symbolic link. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ export function readlinkSync(path: PathLike, options?: BaseEncodingOptions | string | null): string | Buffer; /** * Asynchronous realpath(3) - return the canonicalized absolute pathname. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ export function realpath( path: PathLike, options: BaseEncodingOptions | BufferEncoding | undefined | null, callback: (err: NodeJS.ErrnoException | null, resolvedPath: string) => void ): void; /** * Asynchronous realpath(3) - return the canonicalized absolute pathname. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ export function realpath(path: PathLike, options: BufferEncodingOption, callback: (err: NodeJS.ErrnoException | null, resolvedPath: Buffer) => void): void; /** * Asynchronous realpath(3) - return the canonicalized absolute pathname. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ export function realpath(path: PathLike, options: BaseEncodingOptions | string | undefined | null, callback: (err: NodeJS.ErrnoException | null, resolvedPath: string | Buffer) => void): void; /** * Asynchronous realpath(3) - return the canonicalized absolute pathname. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ export function realpath(path: PathLike, callback: (err: NodeJS.ErrnoException | null, resolvedPath: string) => void): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace realpath { /** * Asynchronous realpath(3) - return the canonicalized absolute pathname. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ function __promisify__(path: PathLike, options?: BaseEncodingOptions | BufferEncoding | null): Promise<string>; /** * Asynchronous realpath(3) - return the canonicalized absolute pathname. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ function __promisify__(path: PathLike, options: BufferEncodingOption): Promise<Buffer>; /** * Asynchronous realpath(3) - return the canonicalized absolute pathname. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ function __promisify__(path: PathLike, options?: BaseEncodingOptions | string | null): Promise<string | Buffer>; function native( path: PathLike, options: BaseEncodingOptions | BufferEncoding | undefined | null, callback: (err: NodeJS.ErrnoException | null, resolvedPath: string) => void ): void; function native(path: PathLike, options: BufferEncodingOption, callback: (err: NodeJS.ErrnoException | null, resolvedPath: Buffer) => void): void; function native(path: PathLike, options: BaseEncodingOptions | string | undefined | null, callback: (err: NodeJS.ErrnoException | null, resolvedPath: string | Buffer) => void): void; function native(path: PathLike, callback: (err: NodeJS.ErrnoException | null, resolvedPath: string) => void): void; } /** * Synchronous realpath(3) - return the canonicalized absolute pathname. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ export function realpathSync(path: PathLike, options?: BaseEncodingOptions | BufferEncoding | null): string; /** * Synchronous realpath(3) - return the canonicalized absolute pathname. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ export function realpathSync(path: PathLike, options: BufferEncodingOption): Buffer; /** * Synchronous realpath(3) - return the canonicalized absolute pathname. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ export function realpathSync(path: PathLike, options?: BaseEncodingOptions | string | null): string | Buffer; export namespace realpathSync { function native(path: PathLike, options?: BaseEncodingOptions | BufferEncoding | null): string; function native(path: PathLike, options: BufferEncodingOption): Buffer; function native(path: PathLike, options?: BaseEncodingOptions | string | null): string | Buffer; } /** * Asynchronous unlink(2) - delete a name and possibly the file it refers to. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ export function unlink(path: PathLike, callback: NoParamCallback): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace unlink { /** * Asynchronous unlink(2) - delete a name and possibly the file it refers to. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ function __promisify__(path: PathLike): Promise<void>; } /** * Synchronous unlink(2) - delete a name and possibly the file it refers to. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ export function unlinkSync(path: PathLike): void; export interface RmDirOptions { /** * If an `EBUSY`, `EMFILE`, `ENFILE`, `ENOTEMPTY`, or * `EPERM` error is encountered, Node.js will retry the operation with a linear * backoff wait of `retryDelay` ms longer on each try. This option represents the * number of retries. This option is ignored if the `recursive` option is not * `true`. * @default 0 */ maxRetries?: number; /** * @deprecated since v14.14.0 In future versions of Node.js, * `fs.rmdir(path, { recursive: true })` will throw on nonexistent * paths, or when given a file as a target. * Use `fs.rm(path, { recursive: true, force: true })` instead. * * If `true`, perform a recursive directory removal. In * recursive mode, errors are not reported if `path` does not exist, and * operations are retried on failure. * @default false */ recursive?: boolean; /** * The amount of time in milliseconds to wait between retries. * This option is ignored if the `recursive` option is not `true`. * @default 100 */ retryDelay?: number; } /** * Asynchronous rmdir(2) - delete a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ export function rmdir(path: PathLike, callback: NoParamCallback): void; export function rmdir(path: PathLike, options: RmDirOptions, callback: NoParamCallback): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace rmdir { /** * Asynchronous rmdir(2) - delete a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ function __promisify__(path: PathLike, options?: RmDirOptions): Promise<void>; } /** * Synchronous rmdir(2) - delete a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ export function rmdirSync(path: PathLike, options?: RmDirOptions): void; export interface RmOptions { /** * When `true`, exceptions will be ignored if `path` does not exist. * @default false */ force?: boolean; /** * If an `EBUSY`, `EMFILE`, `ENFILE`, `ENOTEMPTY`, or * `EPERM` error is encountered, Node.js will retry the operation with a linear * backoff wait of `retryDelay` ms longer on each try. This option represents the * number of retries. This option is ignored if the `recursive` option is not * `true`. * @default 0 */ maxRetries?: number; /** * If `true`, perform a recursive directory removal. In * recursive mode, errors are not reported if `path` does not exist, and * operations are retried on failure. * @default false */ recursive?: boolean; /** * The amount of time in milliseconds to wait between retries. * This option is ignored if the `recursive` option is not `true`. * @default 100 */ retryDelay?: number; } /** * Asynchronously removes files and directories (modeled on the standard POSIX `rm` utility). */ export function rm(path: PathLike, callback: NoParamCallback): void; export function rm(path: PathLike, options: RmOptions, callback: NoParamCallback): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace rm { /** * Asynchronously removes files and directories (modeled on the standard POSIX `rm` utility). */ function __promisify__(path: PathLike, options?: RmOptions): Promise<void>; } /** * Synchronously removes files and directories (modeled on the standard POSIX `rm` utility). */ export function rmSync(path: PathLike, options?: RmOptions): void; export interface MakeDirectoryOptions { /** * Indicates whether parent folders should be created. * If a folder was created, the path to the first created folder will be returned. * @default false */ recursive?: boolean; /** * A file mode. If a string is passed, it is parsed as an octal integer. If not specified * @default 0o777 */ mode?: Mode; } /** * Asynchronous mkdir(2) - create a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options Either the file mode, or an object optionally specifying the file mode and whether parent folders * should be created. If a string is passed, it is parsed as an octal integer. If not specified, defaults to `0o777`. */ export function mkdir(path: PathLike, options: MakeDirectoryOptions & { recursive: true }, callback: (err: NodeJS.ErrnoException | null, path: string) => void): void; /** * Asynchronous mkdir(2) - create a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options Either the file mode, or an object optionally specifying the file mode and whether parent folders * should be created. If a string is passed, it is parsed as an octal integer. If not specified, defaults to `0o777`. */ export function mkdir(path: PathLike, options: Mode | (MakeDirectoryOptions & { recursive?: false; }) | null | undefined, callback: NoParamCallback): void; /** * Asynchronous mkdir(2) - create a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options Either the file mode, or an object optionally specifying the file mode and whether parent folders * should be created. If a string is passed, it is parsed as an octal integer. If not specified, defaults to `0o777`. */ export function mkdir(path: PathLike, options: Mode | MakeDirectoryOptions | null | undefined, callback: (err: NodeJS.ErrnoException | null, path: string | undefined) => void): void; /** * Asynchronous mkdir(2) - create a directory with a mode of `0o777`. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ export function mkdir(path: PathLike, callback: NoParamCallback): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace mkdir { /** * Asynchronous mkdir(2) - create a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options Either the file mode, or an object optionally specifying the file mode and whether parent folders * should be created. If a string is passed, it is parsed as an octal integer. If not specified, defaults to `0o777`. */ function __promisify__(path: PathLike, options: MakeDirectoryOptions & { recursive: true; }): Promise<string>; /** * Asynchronous mkdir(2) - create a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options Either the file mode, or an object optionally specifying the file mode and whether parent folders * should be created. If a string is passed, it is parsed as an octal integer. If not specified, defaults to `0o777`. */ function __promisify__(path: PathLike, options?: Mode | (MakeDirectoryOptions & { recursive?: false; }) | null): Promise<void>; /** * Asynchronous mkdir(2) - create a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options Either the file mode, or an object optionally specifying the file mode and whether parent folders * should be created. If a string is passed, it is parsed as an octal integer. If not specified, defaults to `0o777`. */ function __promisify__(path: PathLike, options?: Mode | MakeDirectoryOptions | null): Promise<string | undefined>; } /** * Synchronous mkdir(2) - create a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options Either the file mode, or an object optionally specifying the file mode and whether parent folders * should be created. If a string is passed, it is parsed as an octal integer. If not specified, defaults to `0o777`. */ export function mkdirSync(path: PathLike, options: MakeDirectoryOptions & { recursive: true; }): string; /** * Synchronous mkdir(2) - create a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options Either the file mode, or an object optionally specifying the file mode and whether parent folders * should be created. If a string is passed, it is parsed as an octal integer. If not specified, defaults to `0o777`. */ export function mkdirSync(path: PathLike, options?: Mode | (MakeDirectoryOptions & { recursive?: false; }) | null): void; /** * Synchronous mkdir(2) - create a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options Either the file mode, or an object optionally specifying the file mode and whether parent folders * should be created. If a string is passed, it is parsed as an octal integer. If not specified, defaults to `0o777`. */ export function mkdirSync(path: PathLike, options?: Mode | MakeDirectoryOptions | null): string | undefined; /** * Asynchronously creates a unique temporary directory. * Generates six random characters to be appended behind a required prefix to create a unique temporary directory. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ export function mkdtemp(prefix: string, options: BaseEncodingOptions | BufferEncoding | undefined | null, callback: (err: NodeJS.ErrnoException | null, folder: string) => void): void; /** * Asynchronously creates a unique temporary directory. * Generates six random characters to be appended behind a required prefix to create a unique temporary directory. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ export function mkdtemp(prefix: string, options: "buffer" | { encoding: "buffer" }, callback: (err: NodeJS.ErrnoException | null, folder: Buffer) => void): void; /** * Asynchronously creates a unique temporary directory. * Generates six random characters to be appended behind a required prefix to create a unique temporary directory. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ export function mkdtemp(prefix: string, options: BaseEncodingOptions | string | undefined | null, callback: (err: NodeJS.ErrnoException | null, folder: string | Buffer) => void): void; /** * Asynchronously creates a unique temporary directory. * Generates six random characters to be appended behind a required prefix to create a unique temporary directory. */ export function mkdtemp(prefix: string, callback: (err: NodeJS.ErrnoException | null, folder: string) => void): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace mkdtemp { /** * Asynchronously creates a unique temporary directory. * Generates six random characters to be appended behind a required prefix to create a unique temporary directory. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ function __promisify__(prefix: string, options?: BaseEncodingOptions | BufferEncoding | null): Promise<string>; /** * Asynchronously creates a unique temporary directory. * Generates six random characters to be appended behind a required prefix to create a unique temporary directory. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ function __promisify__(prefix: string, options: BufferEncodingOption): Promise<Buffer>; /** * Asynchronously creates a unique temporary directory. * Generates six random characters to be appended behind a required prefix to create a unique temporary directory. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ function __promisify__(prefix: string, options?: BaseEncodingOptions | string | null): Promise<string | Buffer>; } /** * Synchronously creates a unique temporary directory. * Generates six random characters to be appended behind a required prefix to create a unique temporary directory. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ export function mkdtempSync(prefix: string, options?: BaseEncodingOptions | BufferEncoding | null): string; /** * Synchronously creates a unique temporary directory. * Generates six random characters to be appended behind a required prefix to create a unique temporary directory. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ export function mkdtempSync(prefix: string, options: BufferEncodingOption): Buffer; /** * Synchronously creates a unique temporary directory. * Generates six random characters to be appended behind a required prefix to create a unique temporary directory. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ export function mkdtempSync(prefix: string, options?: BaseEncodingOptions | string | null): string | Buffer; /** * Asynchronous readdir(3) - read a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ export function readdir( path: PathLike, options: { encoding: BufferEncoding | null; withFileTypes?: false } | BufferEncoding | undefined | null, callback: (err: NodeJS.ErrnoException | null, files: string[]) => void, ): void; /** * Asynchronous readdir(3) - read a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ export function readdir(path: PathLike, options: { encoding: "buffer"; withFileTypes?: false } | "buffer", callback: (err: NodeJS.ErrnoException | null, files: Buffer[]) => void): void; /** * Asynchronous readdir(3) - read a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ export function readdir( path: PathLike, options: BaseEncodingOptions & { withFileTypes?: false } | BufferEncoding | undefined | null, callback: (err: NodeJS.ErrnoException | null, files: string[] | Buffer[]) => void, ): void; /** * Asynchronous readdir(3) - read a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ export function readdir(path: PathLike, callback: (err: NodeJS.ErrnoException | null, files: string[]) => void): void; /** * Asynchronous readdir(3) - read a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options If called with `withFileTypes: true` the result data will be an array of Dirent. */ export function readdir(path: PathLike, options: BaseEncodingOptions & { withFileTypes: true }, callback: (err: NodeJS.ErrnoException | null, files: Dirent[]) => void): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace readdir { /** * Asynchronous readdir(3) - read a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ function __promisify__(path: PathLike, options?: { encoding: BufferEncoding | null; withFileTypes?: false } | BufferEncoding | null): Promise<string[]>; /** * Asynchronous readdir(3) - read a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ function __promisify__(path: PathLike, options: "buffer" | { encoding: "buffer"; withFileTypes?: false }): Promise<Buffer[]>; /** * Asynchronous readdir(3) - read a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ function __promisify__(path: PathLike, options?: BaseEncodingOptions & { withFileTypes?: false } | BufferEncoding | null): Promise<string[] | Buffer[]>; /** * Asynchronous readdir(3) - read a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options If called with `withFileTypes: true` the result data will be an array of Dirent */ function __promisify__(path: PathLike, options: BaseEncodingOptions & { withFileTypes: true }): Promise<Dirent[]>; } /** * Synchronous readdir(3) - read a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ export function readdirSync(path: PathLike, options?: { encoding: BufferEncoding | null; withFileTypes?: false } | BufferEncoding | null): string[]; /** * Synchronous readdir(3) - read a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ export function readdirSync(path: PathLike, options: { encoding: "buffer"; withFileTypes?: false } | "buffer"): Buffer[]; /** * Synchronous readdir(3) - read a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ export function readdirSync(path: PathLike, options?: BaseEncodingOptions & { withFileTypes?: false } | BufferEncoding | null): string[] | Buffer[]; /** * Synchronous readdir(3) - read a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options If called with `withFileTypes: true` the result data will be an array of Dirent. */ export function readdirSync(path: PathLike, options: BaseEncodingOptions & { withFileTypes: true }): Dirent[]; /** * Asynchronous close(2) - close a file descriptor. * @param fd A file descriptor. */ export function close(fd: number, callback: NoParamCallback): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace close { /** * Asynchronous close(2) - close a file descriptor. * @param fd A file descriptor. */ function __promisify__(fd: number): Promise<void>; } /** * Synchronous close(2) - close a file descriptor. * @param fd A file descriptor. */ export function closeSync(fd: number): void; /** * Asynchronous open(2) - open and possibly create a file. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param mode A file mode. If a string is passed, it is parsed as an octal integer. If not supplied, defaults to `0o666`. */ export function open(path: PathLike, flags: OpenMode, mode: Mode | undefined | null, callback: (err: NodeJS.ErrnoException | null, fd: number) => void): void; /** * Asynchronous open(2) - open and possibly create a file. If the file is created, its mode will be `0o666`. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ export function open(path: PathLike, flags: OpenMode, callback: (err: NodeJS.ErrnoException | null, fd: number) => void): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace open { /** * Asynchronous open(2) - open and possibly create a file. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param mode A file mode. If a string is passed, it is parsed as an octal integer. If not supplied, defaults to `0o666`. */ function __promisify__(path: PathLike, flags: OpenMode, mode?: Mode | null): Promise<number>; } /** * Synchronous open(2) - open and possibly create a file, returning a file descriptor.. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param mode A file mode. If a string is passed, it is parsed as an octal integer. If not supplied, defaults to `0o666`. */ export function openSync(path: PathLike, flags: OpenMode, mode?: Mode | null): number; /** * Asynchronously change file timestamps of the file referenced by the supplied path. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param atime The last access time. If a string is provided, it will be coerced to number. * @param mtime The last modified time. If a string is provided, it will be coerced to number. */ export function utimes(path: PathLike, atime: string | number | Date, mtime: string | number | Date, callback: NoParamCallback): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace utimes { /** * Asynchronously change file timestamps of the file referenced by the supplied path. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param atime The last access time. If a string is provided, it will be coerced to number. * @param mtime The last modified time. If a string is provided, it will be coerced to number. */ function __promisify__(path: PathLike, atime: string | number | Date, mtime: string | number | Date): Promise<void>; } /** * Synchronously change file timestamps of the file referenced by the supplied path. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param atime The last access time. If a string is provided, it will be coerced to number. * @param mtime The last modified time. If a string is provided, it will be coerced to number. */ export function utimesSync(path: PathLike, atime: string | number | Date, mtime: string | number | Date): void; /** * Asynchronously change file timestamps of the file referenced by the supplied file descriptor. * @param fd A file descriptor. * @param atime The last access time. If a string is provided, it will be coerced to number. * @param mtime The last modified time. If a string is provided, it will be coerced to number. */ export function futimes(fd: number, atime: string | number | Date, mtime: string | number | Date, callback: NoParamCallback): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace futimes { /** * Asynchronously change file timestamps of the file referenced by the supplied file descriptor. * @param fd A file descriptor. * @param atime The last access time. If a string is provided, it will be coerced to number. * @param mtime The last modified time. If a string is provided, it will be coerced to number. */ function __promisify__(fd: number, atime: string | number | Date, mtime: string | number | Date): Promise<void>; } /** * Synchronously change file timestamps of the file referenced by the supplied file descriptor. * @param fd A file descriptor. * @param atime The last access time. If a string is provided, it will be coerced to number. * @param mtime The last modified time. If a string is provided, it will be coerced to number. */ export function futimesSync(fd: number, atime: string | number | Date, mtime: string | number | Date): void; /** * Asynchronous fsync(2) - synchronize a file's in-core state with the underlying storage device. * @param fd A file descriptor. */ export function fsync(fd: number, callback: NoParamCallback): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace fsync { /** * Asynchronous fsync(2) - synchronize a file's in-core state with the underlying storage device. * @param fd A file descriptor. */ function __promisify__(fd: number): Promise<void>; } /** * Synchronous fsync(2) - synchronize a file's in-core state with the underlying storage device. * @param fd A file descriptor. */ export function fsyncSync(fd: number): void; /** * Asynchronously writes `buffer` to the file referenced by the supplied file descriptor. * @param fd A file descriptor. * @param offset The part of the buffer to be written. If not supplied, defaults to `0`. * @param length The number of bytes to write. If not supplied, defaults to `buffer.length - offset`. * @param position The offset from the beginning of the file where this data should be written. If not supplied, defaults to the current position. */ export function write<TBuffer extends NodeJS.ArrayBufferView>( fd: number, buffer: TBuffer, offset: number | undefined | null, length: number | undefined | null, position: number | undefined | null, callback: (err: NodeJS.ErrnoException | null, written: number, buffer: TBuffer) => void, ): void; /** * Asynchronously writes `buffer` to the file referenced by the supplied file descriptor. * @param fd A file descriptor. * @param offset The part of the buffer to be written. If not supplied, defaults to `0`. * @param length The number of bytes to write. If not supplied, defaults to `buffer.length - offset`. */ export function write<TBuffer extends NodeJS.ArrayBufferView>( fd: number, buffer: TBuffer, offset: number | undefined | null, length: number | undefined | null, callback: (err: NodeJS.ErrnoException | null, written: number, buffer: TBuffer) => void, ): void; /** * Asynchronously writes `buffer` to the file referenced by the supplied file descriptor. * @param fd A file descriptor. * @param offset The part of the buffer to be written. If not supplied, defaults to `0`. */ export function write<TBuffer extends NodeJS.ArrayBufferView>( fd: number, buffer: TBuffer, offset: number | undefined | null, callback: (err: NodeJS.ErrnoException | null, written: number, buffer: TBuffer) => void ): void; /** * Asynchronously writes `buffer` to the file referenced by the supplied file descriptor. * @param fd A file descriptor. */ export function write<TBuffer extends NodeJS.ArrayBufferView>(fd: number, buffer: TBuffer, callback: (err: NodeJS.ErrnoException | null, written: number, buffer: TBuffer) => void): void; /** * Asynchronously writes `string` to the file referenced by the supplied file descriptor. * @param fd A file descriptor. * @param string A string to write. * @param position The offset from the beginning of the file where this data should be written. If not supplied, defaults to the current position. * @param encoding The expected string encoding. */ export function write( fd: number, string: string, position: number | undefined | null, encoding: BufferEncoding | undefined | null, callback: (err: NodeJS.ErrnoException | null, written: number, str: string) => void, ): void; /** * Asynchronously writes `string` to the file referenced by the supplied file descriptor. * @param fd A file descriptor. * @param string A string to write. * @param position The offset from the beginning of the file where this data should be written. If not supplied, defaults to the current position. */ export function write(fd: number, string: string, position: number | undefined | null, callback: (err: NodeJS.ErrnoException | null, written: number, str: string) => void): void; /** * Asynchronously writes `string` to the file referenced by the supplied file descriptor. * @param fd A file descriptor. * @param string A string to write. */ export function write(fd: number, string: string, callback: (err: NodeJS.ErrnoException | null, written: number, str: string) => void): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace write { /** * Asynchronously writes `buffer` to the file referenced by the supplied file descriptor. * @param fd A file descriptor. * @param offset The part of the buffer to be written. If not supplied, defaults to `0`. * @param length The number of bytes to write. If not supplied, defaults to `buffer.length - offset`. * @param position The offset from the beginning of the file where this data should be written. If not supplied, defaults to the current position. */ function __promisify__<TBuffer extends NodeJS.ArrayBufferView>( fd: number, buffer?: TBuffer, offset?: number, length?: number, position?: number | null, ): Promise<{ bytesWritten: number, buffer: TBuffer }>; /** * Asynchronously writes `string` to the file referenced by the supplied file descriptor. * @param fd A file descriptor. * @param string A string to write. * @param position The offset from the beginning of the file where this data should be written. If not supplied, defaults to the current position. * @param encoding The expected string encoding. */ function __promisify__(fd: number, string: string, position?: number | null, encoding?: BufferEncoding | null): Promise<{ bytesWritten: number, buffer: string }>; } /** * Synchronously writes `buffer` to the file referenced by the supplied file descriptor, returning the number of bytes written. * @param fd A file descriptor. * @param offset The part of the buffer to be written. If not supplied, defaults to `0`. * @param length The number of bytes to write. If not supplied, defaults to `buffer.length - offset`. * @param position The offset from the beginning of the file where this data should be written. If not supplied, defaults to the current position. */ export function writeSync(fd: number, buffer: NodeJS.ArrayBufferView, offset?: number | null, length?: number | null, position?: number | null): number; /** * Synchronously writes `string` to the file referenced by the supplied file descriptor, returning the number of bytes written. * @param fd A file descriptor. * @param string A string to write. * @param position The offset from the beginning of the file where this data should be written. If not supplied, defaults to the current position. * @param encoding The expected string encoding. */ export function writeSync(fd: number, string: string, position?: number | null, encoding?: BufferEncoding | null): number; /** * Asynchronously reads data from the file referenced by the supplied file descriptor. * @param fd A file descriptor. * @param buffer The buffer that the data will be written to. * @param offset The offset in the buffer at which to start writing. * @param length The number of bytes to read. * @param position The offset from the beginning of the file from which data should be read. If `null`, data will be read from the current position. */ export function read<TBuffer extends NodeJS.ArrayBufferView>( fd: number, buffer: TBuffer, offset: number, length: number, position: number | null, callback: (err: NodeJS.ErrnoException | null, bytesRead: number, buffer: TBuffer) => void, ): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace read { /** * @param fd A file descriptor. * @param buffer The buffer that the data will be written to. * @param offset The offset in the buffer at which to start writing. * @param length The number of bytes to read. * @param position The offset from the beginning of the file from which data should be read. If `null`, data will be read from the current position. */ function __promisify__<TBuffer extends NodeJS.ArrayBufferView>( fd: number, buffer: TBuffer, offset: number, length: number, position: number | null ): Promise<{ bytesRead: number, buffer: TBuffer }>; } export interface ReadSyncOptions { /** * @default 0 */ offset?: number; /** * @default `length of buffer` */ length?: number; /** * @default null */ position?: number | null; } /** * Synchronously reads data from the file referenced by the supplied file descriptor, returning the number of bytes read. * @param fd A file descriptor. * @param buffer The buffer that the data will be written to. * @param offset The offset in the buffer at which to start writing. * @param length The number of bytes to read. * @param position The offset from the beginning of the file from which data should be read. If `null`, data will be read from the current position. */ export function readSync(fd: number, buffer: NodeJS.ArrayBufferView, offset: number, length: number, position: number | null): number; /** * Similar to the above `fs.readSync` function, this version takes an optional `options` object. * If no `options` object is specified, it will default with the above values. */ export function readSync(fd: number, buffer: NodeJS.ArrayBufferView, opts?: ReadSyncOptions): number; /** * Asynchronously reads the entire contents of a file. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * If a file descriptor is provided, the underlying file will _not_ be closed automatically. * @param options An object that may contain an optional flag. * If a flag is not provided, it defaults to `'r'`. */ export function readFile(path: PathLike | number, options: { encoding?: null; flag?: string; } | undefined | null, callback: (err: NodeJS.ErrnoException | null, data: Buffer) => void): void; /** * Asynchronously reads the entire contents of a file. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. * If a file descriptor is provided, the underlying file will _not_ be closed automatically. * @param options Either the encoding for the result, or an object that contains the encoding and an optional flag. * If a flag is not provided, it defaults to `'r'`. */ export function readFile(path: PathLike | number, options: { encoding: BufferEncoding; flag?: string; } | string, callback: (err: NodeJS.ErrnoException | null, data: string) => void): void; /** * Asynchronously reads the entire contents of a file. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. * If a file descriptor is provided, the underlying file will _not_ be closed automatically. * @param options Either the encoding for the result, or an object that contains the encoding and an optional flag. * If a flag is not provided, it defaults to `'r'`. */ export function readFile( path: PathLike | number, options: BaseEncodingOptions & { flag?: string; } | string | undefined | null, callback: (err: NodeJS.ErrnoException | null, data: string | Buffer) => void, ): void; /** * Asynchronously reads the entire contents of a file. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * If a file descriptor is provided, the underlying file will _not_ be closed automatically. */ export function readFile(path: PathLike | number, callback: (err: NodeJS.ErrnoException | null, data: Buffer) => void): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace readFile { /** * Asynchronously reads the entire contents of a file. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * If a file descriptor is provided, the underlying file will _not_ be closed automatically. * @param options An object that may contain an optional flag. * If a flag is not provided, it defaults to `'r'`. */ function __promisify__(path: PathLike | number, options?: { encoding?: null; flag?: string; } | null): Promise<Buffer>; /** * Asynchronously reads the entire contents of a file. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. * If a file descriptor is provided, the underlying file will _not_ be closed automatically. * @param options Either the encoding for the result, or an object that contains the encoding and an optional flag. * If a flag is not provided, it defaults to `'r'`. */ function __promisify__(path: PathLike | number, options: { encoding: BufferEncoding; flag?: string; } | string): Promise<string>; /** * Asynchronously reads the entire contents of a file. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. * If a file descriptor is provided, the underlying file will _not_ be closed automatically. * @param options Either the encoding for the result, or an object that contains the encoding and an optional flag. * If a flag is not provided, it defaults to `'r'`. */ function __promisify__(path: PathLike | number, options?: BaseEncodingOptions & { flag?: string; } | string | null): Promise<string | Buffer>; } /** * Synchronously reads the entire contents of a file. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. * If a file descriptor is provided, the underlying file will _not_ be closed automatically. * @param options An object that may contain an optional flag. If a flag is not provided, it defaults to `'r'`. */ export function readFileSync(path: PathLike | number, options?: { encoding?: null; flag?: string; } | null): Buffer; /** * Synchronously reads the entire contents of a file. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. * If a file descriptor is provided, the underlying file will _not_ be closed automatically. * @param options Either the encoding for the result, or an object that contains the encoding and an optional flag. * If a flag is not provided, it defaults to `'r'`. */ export function readFileSync(path: PathLike | number, options: { encoding: BufferEncoding; flag?: string; } | BufferEncoding): string; /** * Synchronously reads the entire contents of a file. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. * If a file descriptor is provided, the underlying file will _not_ be closed automatically. * @param options Either the encoding for the result, or an object that contains the encoding and an optional flag. * If a flag is not provided, it defaults to `'r'`. */ export function readFileSync(path: PathLike | number, options?: BaseEncodingOptions & { flag?: string; } | BufferEncoding | null): string | Buffer; export type WriteFileOptions = BaseEncodingOptions & { mode?: Mode; flag?: string; } | string | null; /** * Asynchronously writes data to a file, replacing the file if it already exists. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. * If a file descriptor is provided, the underlying file will _not_ be closed automatically. * @param data The data to write. If something other than a Buffer or Uint8Array is provided, the value is coerced to a string. * @param options Either the encoding for the file, or an object optionally specifying the encoding, file mode, and flag. * If `encoding` is not supplied, the default of `'utf8'` is used. * If `mode` is not supplied, the default of `0o666` is used. * If `mode` is a string, it is parsed as an octal integer. * If `flag` is not supplied, the default of `'w'` is used. */ export function writeFile(path: PathLike | number, data: string | NodeJS.ArrayBufferView, options: WriteFileOptions, callback: NoParamCallback): void; /** * Asynchronously writes data to a file, replacing the file if it already exists. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. * If a file descriptor is provided, the underlying file will _not_ be closed automatically. * @param data The data to write. If something other than a Buffer or Uint8Array is provided, the value is coerced to a string. */ export function writeFile(path: PathLike | number, data: string | NodeJS.ArrayBufferView, callback: NoParamCallback): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace writeFile { /** * Asynchronously writes data to a file, replacing the file if it already exists. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. * If a file descriptor is provided, the underlying file will _not_ be closed automatically. * @param data The data to write. If something other than a Buffer or Uint8Array is provided, the value is coerced to a string. * @param options Either the encoding for the file, or an object optionally specifying the encoding, file mode, and flag. * If `encoding` is not supplied, the default of `'utf8'` is used. * If `mode` is not supplied, the default of `0o666` is used. * If `mode` is a string, it is parsed as an octal integer. * If `flag` is not supplied, the default of `'w'` is used. */ function __promisify__(path: PathLike | number, data: string | NodeJS.ArrayBufferView, options?: WriteFileOptions): Promise<void>; } /** * Synchronously writes data to a file, replacing the file if it already exists. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. * If a file descriptor is provided, the underlying file will _not_ be closed automatically. * @param data The data to write. If something other than a Buffer or Uint8Array is provided, the value is coerced to a string. * @param options Either the encoding for the file, or an object optionally specifying the encoding, file mode, and flag. * If `encoding` is not supplied, the default of `'utf8'` is used. * If `mode` is not supplied, the default of `0o666` is used. * If `mode` is a string, it is parsed as an octal integer. * If `flag` is not supplied, the default of `'w'` is used. */ export function writeFileSync(path: PathLike | number, data: string | NodeJS.ArrayBufferView, options?: WriteFileOptions): void; /** * Asynchronously append data to a file, creating the file if it does not exist. * @param file A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. * If a file descriptor is provided, the underlying file will _not_ be closed automatically. * @param data The data to write. If something other than a Buffer or Uint8Array is provided, the value is coerced to a string. * @param options Either the encoding for the file, or an object optionally specifying the encoding, file mode, and flag. * If `encoding` is not supplied, the default of `'utf8'` is used. * If `mode` is not supplied, the default of `0o666` is used. * If `mode` is a string, it is parsed as an octal integer. * If `flag` is not supplied, the default of `'a'` is used. */ export function appendFile(file: PathLike | number, data: string | Uint8Array, options: WriteFileOptions, callback: NoParamCallback): void; /** * Asynchronously append data to a file, creating the file if it does not exist. * @param file A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. * If a file descriptor is provided, the underlying file will _not_ be closed automatically. * @param data The data to write. If something other than a Buffer or Uint8Array is provided, the value is coerced to a string. */ export function appendFile(file: PathLike | number, data: string | Uint8Array, callback: NoParamCallback): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace appendFile { /** * Asynchronously append data to a file, creating the file if it does not exist. * @param file A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. * If a file descriptor is provided, the underlying file will _not_ be closed automatically. * @param data The data to write. If something other than a Buffer or Uint8Array is provided, the value is coerced to a string. * @param options Either the encoding for the file, or an object optionally specifying the encoding, file mode, and flag. * If `encoding` is not supplied, the default of `'utf8'` is used. * If `mode` is not supplied, the default of `0o666` is used. * If `mode` is a string, it is parsed as an octal integer. * If `flag` is not supplied, the default of `'a'` is used. */ function __promisify__(file: PathLike | number, data: string | Uint8Array, options?: WriteFileOptions): Promise<void>; } /** * Synchronously append data to a file, creating the file if it does not exist. * @param file A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. * If a file descriptor is provided, the underlying file will _not_ be closed automatically. * @param data The data to write. If something other than a Buffer or Uint8Array is provided, the value is coerced to a string. * @param options Either the encoding for the file, or an object optionally specifying the encoding, file mode, and flag. * If `encoding` is not supplied, the default of `'utf8'` is used. * If `mode` is not supplied, the default of `0o666` is used. * If `mode` is a string, it is parsed as an octal integer. * If `flag` is not supplied, the default of `'a'` is used. */ export function appendFileSync(file: PathLike | number, data: string | Uint8Array, options?: WriteFileOptions): void; /** * Watch for changes on `filename`. The callback `listener` will be called each time the file is accessed. */ export function watchFile(filename: PathLike, options: { persistent?: boolean; interval?: number; } | undefined, listener: (curr: Stats, prev: Stats) => void): void; /** * Watch for changes on `filename`. The callback `listener` will be called each time the file is accessed. * @param filename A path to a file or directory. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. */ export function watchFile(filename: PathLike, listener: (curr: Stats, prev: Stats) => void): void; /** * Stop watching for changes on `filename`. * @param filename A path to a file or directory. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. */ export function unwatchFile(filename: PathLike, listener?: (curr: Stats, prev: Stats) => void): void; /** * Watch for changes on `filename`, where `filename` is either a file or a directory, returning an `FSWatcher`. * @param filename A path to a file or directory. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. * @param options Either the encoding for the filename provided to the listener, or an object optionally specifying encoding, persistent, and recursive options. * If `encoding` is not supplied, the default of `'utf8'` is used. * If `persistent` is not supplied, the default of `true` is used. * If `recursive` is not supplied, the default of `false` is used. */ export function watch( filename: PathLike, options: { encoding?: BufferEncoding | null, persistent?: boolean, recursive?: boolean } | BufferEncoding | undefined | null, listener?: (event: string, filename: string) => void, ): FSWatcher; /** * Watch for changes on `filename`, where `filename` is either a file or a directory, returning an `FSWatcher`. * @param filename A path to a file or directory. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. * @param options Either the encoding for the filename provided to the listener, or an object optionally specifying encoding, persistent, and recursive options. * If `encoding` is not supplied, the default of `'utf8'` is used. * If `persistent` is not supplied, the default of `true` is used. * If `recursive` is not supplied, the default of `false` is used. */ export function watch(filename: PathLike, options: { encoding: "buffer", persistent?: boolean, recursive?: boolean } | "buffer", listener?: (event: string, filename: Buffer) => void): FSWatcher; /** * Watch for changes on `filename`, where `filename` is either a file or a directory, returning an `FSWatcher`. * @param filename A path to a file or directory. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. * @param options Either the encoding for the filename provided to the listener, or an object optionally specifying encoding, persistent, and recursive options. * If `encoding` is not supplied, the default of `'utf8'` is used. * If `persistent` is not supplied, the default of `true` is used. * If `recursive` is not supplied, the default of `false` is used. */ export function watch( filename: PathLike, options: { encoding?: BufferEncoding | null, persistent?: boolean, recursive?: boolean } | string | null, listener?: (event: string, filename: string | Buffer) => void, ): FSWatcher; /** * Watch for changes on `filename`, where `filename` is either a file or a directory, returning an `FSWatcher`. * @param filename A path to a file or directory. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. */ export function watch(filename: PathLike, listener?: (event: string, filename: string) => any): FSWatcher; /** * Asynchronously tests whether or not the given path exists by checking with the file system. * @deprecated since v1.0.0 Use `fs.stat()` or `fs.access()` instead * @param path A path to a file or directory. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. */ export function exists(path: PathLike, callback: (exists: boolean) => void): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace exists { /** * @param path A path to a file or directory. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. */ function __promisify__(path: PathLike): Promise<boolean>; } /** * Synchronously tests whether or not the given path exists by checking with the file system. * @param path A path to a file or directory. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. */ export function existsSync(path: PathLike): boolean; export namespace constants { // File Access Constants /** Constant for fs.access(). File is visible to the calling process. */ const F_OK: number; /** Constant for fs.access(). File can be read by the calling process. */ const R_OK: number; /** Constant for fs.access(). File can be written by the calling process. */ const W_OK: number; /** Constant for fs.access(). File can be executed by the calling process. */ const X_OK: number; // File Copy Constants /** Constant for fs.copyFile. Flag indicating the destination file should not be overwritten if it already exists. */ const COPYFILE_EXCL: number; /** * Constant for fs.copyFile. copy operation will attempt to create a copy-on-write reflink. * If the underlying platform does not support copy-on-write, then a fallback copy mechanism is used. */ const COPYFILE_FICLONE: number; /** * Constant for fs.copyFile. Copy operation will attempt to create a copy-on-write reflink. * If the underlying platform does not support copy-on-write, then the operation will fail with an error. */ const COPYFILE_FICLONE_FORCE: number; // File Open Constants /** Constant for fs.open(). Flag indicating to open a file for read-only access. */ const O_RDONLY: number; /** Constant for fs.open(). Flag indicating to open a file for write-only access. */ const O_WRONLY: number; /** Constant for fs.open(). Flag indicating to open a file for read-write access. */ const O_RDWR: number; /** Constant for fs.open(). Flag indicating to create the file if it does not already exist. */ const O_CREAT: number; /** Constant for fs.open(). Flag indicating that opening a file should fail if the O_CREAT flag is set and the file already exists. */ const O_EXCL: number; /** * Constant for fs.open(). Flag indicating that if path identifies a terminal device, * opening the path shall not cause that terminal to become the controlling terminal for the process * (if the process does not already have one). */ const O_NOCTTY: number; /** Constant for fs.open(). Flag indicating that if the file exists and is a regular file, and the file is opened successfully for write access, its length shall be truncated to zero. */ const O_TRUNC: number; /** Constant for fs.open(). Flag indicating that data will be appended to the end of the file. */ const O_APPEND: number; /** Constant for fs.open(). Flag indicating that the open should fail if the path is not a directory. */ const O_DIRECTORY: number; /** * constant for fs.open(). * Flag indicating reading accesses to the file system will no longer result in * an update to the atime information associated with the file. * This flag is available on Linux operating systems only. */ const O_NOATIME: number; /** Constant for fs.open(). Flag indicating that the open should fail if the path is a symbolic link. */ const O_NOFOLLOW: number; /** Constant for fs.open(). Flag indicating that the file is opened for synchronous I/O. */ const O_SYNC: number; /** Constant for fs.open(). Flag indicating that the file is opened for synchronous I/O with write operations waiting for data integrity. */ const O_DSYNC: number; /** Constant for fs.open(). Flag indicating to open the symbolic link itself rather than the resource it is pointing to. */ const O_SYMLINK: number; /** Constant for fs.open(). When set, an attempt will be made to minimize caching effects of file I/O. */ const O_DIRECT: number; /** Constant for fs.open(). Flag indicating to open the file in nonblocking mode when possible. */ const O_NONBLOCK: number; // File Type Constants /** Constant for fs.Stats mode property for determining a file's type. Bit mask used to extract the file type code. */ const S_IFMT: number; /** Constant for fs.Stats mode property for determining a file's type. File type constant for a regular file. */ const S_IFREG: number; /** Constant for fs.Stats mode property for determining a file's type. File type constant for a directory. */ const S_IFDIR: number; /** Constant for fs.Stats mode property for determining a file's type. File type constant for a character-oriented device file. */ const S_IFCHR: number; /** Constant for fs.Stats mode property for determining a file's type. File type constant for a block-oriented device file. */ const S_IFBLK: number; /** Constant for fs.Stats mode property for determining a file's type. File type constant for a FIFO/pipe. */ const S_IFIFO: number; /** Constant for fs.Stats mode property for determining a file's type. File type constant for a symbolic link. */ const S_IFLNK: number; /** Constant for fs.Stats mode property for determining a file's type. File type constant for a socket. */ const S_IFSOCK: number; // File Mode Constants /** Constant for fs.Stats mode property for determining access permissions for a file. File mode indicating readable, writable and executable by owner. */ const S_IRWXU: number; /** Constant for fs.Stats mode property for determining access permissions for a file. File mode indicating readable by owner. */ const S_IRUSR: number; /** Constant for fs.Stats mode property for determining access permissions for a file. File mode indicating writable by owner. */ const S_IWUSR: number; /** Constant for fs.Stats mode property for determining access permissions for a file. File mode indicating executable by owner. */ const S_IXUSR: number; /** Constant for fs.Stats mode property for determining access permissions for a file. File mode indicating readable, writable and executable by group. */ const S_IRWXG: number; /** Constant for fs.Stats mode property for determining access permissions for a file. File mode indicating readable by group. */ const S_IRGRP: number; /** Constant for fs.Stats mode property for determining access permissions for a file. File mode indicating writable by group. */ const S_IWGRP: number; /** Constant for fs.Stats mode property for determining access permissions for a file. File mode indicating executable by group. */ const S_IXGRP: number; /** Constant for fs.Stats mode property for determining access permissions for a file. File mode indicating readable, writable and executable by others. */ const S_IRWXO: number; /** Constant for fs.Stats mode property for determining access permissions for a file. File mode indicating readable by others. */ const S_IROTH: number; /** Constant for fs.Stats mode property for determining access permissions for a file. File mode indicating writable by others. */ const S_IWOTH: number; /** Constant for fs.Stats mode property for determining access permissions for a file. File mode indicating executable by others. */ const S_IXOTH: number; /** * When set, a memory file mapping is used to access the file. This flag * is available on Windows operating systems only. On other operating systems, * this flag is ignored. */ const UV_FS_O_FILEMAP: number; } /** * Asynchronously tests a user's permissions for the file specified by path. * @param path A path to a file or directory. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. */ export function access(path: PathLike, mode: number | undefined, callback: NoParamCallback): void; /** * Asynchronously tests a user's permissions for the file specified by path. * @param path A path to a file or directory. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. */ export function access(path: PathLike, callback: NoParamCallback): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace access { /** * Asynchronously tests a user's permissions for the file specified by path. * @param path A path to a file or directory. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. */ function __promisify__(path: PathLike, mode?: number): Promise<void>; } /** * Synchronously tests a user's permissions for the file specified by path. * @param path A path to a file or directory. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. */ export function accessSync(path: PathLike, mode?: number): void; /** * Returns a new `ReadStream` object. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. */ export function createReadStream(path: PathLike, options?: string | { flags?: string; encoding?: BufferEncoding; fd?: number; mode?: number; autoClose?: boolean; /** * @default false */ emitClose?: boolean; start?: number; end?: number; highWaterMark?: number; }): ReadStream; /** * Returns a new `WriteStream` object. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. */ export function createWriteStream(path: PathLike, options?: string | { flags?: string; encoding?: BufferEncoding; fd?: number; mode?: number; autoClose?: boolean; emitClose?: boolean; start?: number; highWaterMark?: number; }): WriteStream; /** * Asynchronous fdatasync(2) - synchronize a file's in-core state with storage device. * @param fd A file descriptor. */ export function fdatasync(fd: number, callback: NoParamCallback): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace fdatasync { /** * Asynchronous fdatasync(2) - synchronize a file's in-core state with storage device. * @param fd A file descriptor. */ function __promisify__(fd: number): Promise<void>; } /** * Synchronous fdatasync(2) - synchronize a file's in-core state with storage device. * @param fd A file descriptor. */ export function fdatasyncSync(fd: number): void; /** * Asynchronously copies src to dest. By default, dest is overwritten if it already exists. * No arguments other than a possible exception are given to the callback function. * Node.js makes no guarantees about the atomicity of the copy operation. * If an error occurs after the destination file has been opened for writing, Node.js will attempt * to remove the destination. * @param src A path to the source file. * @param dest A path to the destination file. */ export function copyFile(src: PathLike, dest: PathLike, callback: NoParamCallback): void; /** * Asynchronously copies src to dest. By default, dest is overwritten if it already exists. * No arguments other than a possible exception are given to the callback function. * Node.js makes no guarantees about the atomicity of the copy operation. * If an error occurs after the destination file has been opened for writing, Node.js will attempt * to remove the destination. * @param src A path to the source file. * @param dest A path to the destination file. * @param flags An integer that specifies the behavior of the copy operation. The only supported flag is fs.constants.COPYFILE_EXCL, which causes the copy operation to fail if dest already exists. */ export function copyFile(src: PathLike, dest: PathLike, flags: number, callback: NoParamCallback): void; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. export namespace copyFile { /** * Asynchronously copies src to dest. By default, dest is overwritten if it already exists. * No arguments other than a possible exception are given to the callback function. * Node.js makes no guarantees about the atomicity of the copy operation. * If an error occurs after the destination file has been opened for writing, Node.js will attempt * to remove the destination. * @param src A path to the source file. * @param dest A path to the destination file. * @param flags An optional integer that specifies the behavior of the copy operation. * The only supported flag is fs.constants.COPYFILE_EXCL, * which causes the copy operation to fail if dest already exists. */ function __promisify__(src: PathLike, dst: PathLike, flags?: number): Promise<void>; } /** * Synchronously copies src to dest. By default, dest is overwritten if it already exists. * Node.js makes no guarantees about the atomicity of the copy operation. * If an error occurs after the destination file has been opened for writing, Node.js will attempt * to remove the destination. * @param src A path to the source file. * @param dest A path to the destination file. * @param flags An optional integer that specifies the behavior of the copy operation. * The only supported flag is fs.constants.COPYFILE_EXCL, which causes the copy operation to fail if dest already exists. */ export function copyFileSync(src: PathLike, dest: PathLike, flags?: number): void; /** * Write an array of ArrayBufferViews to the file specified by fd using writev(). * position is the offset from the beginning of the file where this data should be written. * It is unsafe to use fs.writev() multiple times on the same file without waiting for the callback. For this scenario, use fs.createWriteStream(). * On Linux, positional writes don't work when the file is opened in append mode. * The kernel ignores the position argument and always appends the data to the end of the file. */ export function writev( fd: number, buffers: ReadonlyArray<NodeJS.ArrayBufferView>, cb: (err: NodeJS.ErrnoException | null, bytesWritten: number, buffers: NodeJS.ArrayBufferView[]) => void ): void; export function writev( fd: number, buffers: ReadonlyArray<NodeJS.ArrayBufferView>, position: number, cb: (err: NodeJS.ErrnoException | null, bytesWritten: number, buffers: NodeJS.ArrayBufferView[]) => void ): void; export interface WriteVResult { bytesWritten: number; buffers: NodeJS.ArrayBufferView[]; } export namespace writev { function __promisify__(fd: number, buffers: ReadonlyArray<NodeJS.ArrayBufferView>, position?: number): Promise<WriteVResult>; } /** * See `writev`. */ export function writevSync(fd: number, buffers: ReadonlyArray<NodeJS.ArrayBufferView>, position?: number): number; export function readv( fd: number, buffers: ReadonlyArray<NodeJS.ArrayBufferView>, cb: (err: NodeJS.ErrnoException | null, bytesRead: number, buffers: NodeJS.ArrayBufferView[]) => void ): void; export function readv( fd: number, buffers: ReadonlyArray<NodeJS.ArrayBufferView>, position: number, cb: (err: NodeJS.ErrnoException | null, bytesRead: number, buffers: NodeJS.ArrayBufferView[]) => void ): void; export interface ReadVResult { bytesRead: number; buffers: NodeJS.ArrayBufferView[]; } export namespace readv { function __promisify__(fd: number, buffers: ReadonlyArray<NodeJS.ArrayBufferView>, position?: number): Promise<ReadVResult>; } /** * See `readv`. */ export function readvSync(fd: number, buffers: ReadonlyArray<NodeJS.ArrayBufferView>, position?: number): number; export interface OpenDirOptions { encoding?: BufferEncoding; /** * Number of directory entries that are buffered * internally when reading from the directory. Higher values lead to better * performance but higher memory usage. * @default 32 */ bufferSize?: number; } export function opendirSync(path: string, options?: OpenDirOptions): Dir; export function opendir(path: string, cb: (err: NodeJS.ErrnoException | null, dir: Dir) => void): void; export function opendir(path: string, options: OpenDirOptions, cb: (err: NodeJS.ErrnoException | null, dir: Dir) => void): void; export namespace opendir { function __promisify__(path: string, options?: OpenDirOptions): Promise<Dir>; } export interface BigIntStats extends StatsBase<bigint> { } export class BigIntStats { atimeNs: bigint; mtimeNs: bigint; ctimeNs: bigint; birthtimeNs: bigint; } export interface BigIntOptions { bigint: true; } export interface StatOptions { bigint: boolean; } } apollo-server-demo/node_modules/@types/node/string_decoder.d.ts 0000644 0001750 0000144 00000000301 14000133701 024364 0 ustar andreh users declare module "string_decoder" { class StringDecoder { constructor(encoding?: BufferEncoding); write(buffer: Buffer): string; end(buffer?: Buffer): string; } } apollo-server-demo/node_modules/@types/node/querystring.d.ts 0000644 0001750 0000144 00000002026 14000133700 023772 0 ustar andreh users declare module "querystring" { interface StringifyOptions { encodeURIComponent?: (str: string) => string; } interface ParseOptions { maxKeys?: number; decodeURIComponent?: (str: string) => string; } interface ParsedUrlQuery extends NodeJS.Dict<string | string[]> { } interface ParsedUrlQueryInput extends NodeJS.Dict<string | number | boolean | ReadonlyArray<string> | ReadonlyArray<number> | ReadonlyArray<boolean> | null> { } function stringify(obj?: ParsedUrlQueryInput, sep?: string, eq?: string, options?: StringifyOptions): string; function parse(str: string, sep?: string, eq?: string, options?: ParseOptions): ParsedUrlQuery; /** * The querystring.encode() function is an alias for querystring.stringify(). */ const encode: typeof stringify; /** * The querystring.decode() function is an alias for querystring.parse(). */ const decode: typeof parse; function escape(str: string): string; function unescape(str: string): string; } apollo-server-demo/node_modules/@types/node/module.d.ts 0000644 0001750 0000144 00000003153 14000133700 022665 0 ustar andreh users declare module "module" { import { URL } from "url"; namespace Module { /** * Updates all the live bindings for builtin ES Modules to match the properties of the CommonJS exports. * It does not add or remove exported names from the ES Modules. */ function syncBuiltinESMExports(): void; function findSourceMap(path: string, error?: Error): SourceMap; interface SourceMapPayload { file: string; version: number; sources: string[]; sourcesContent: string[]; names: string[]; mappings: string; sourceRoot: string; } interface SourceMapping { generatedLine: number; generatedColumn: number; originalSource: string; originalLine: number; originalColumn: number; } class SourceMap { readonly payload: SourceMapPayload; constructor(payload: SourceMapPayload); findEntry(line: number, column: number): SourceMapping; } } interface Module extends NodeModule {} class Module { static runMain(): void; static wrap(code: string): string; /** * @deprecated Deprecated since: v12.2.0. Please use createRequire() instead. */ static createRequireFromPath(path: string): NodeRequire; static createRequire(path: string | URL): NodeRequire; static builtinModules: string[]; static Module: typeof Module; constructor(id: string, parent?: Module); } export = Module; } apollo-server-demo/node_modules/@types/node/fs/ 0000755 0001750 0000144 00000000000 14067647700 021243 5 ustar andreh users apollo-server-demo/node_modules/@types/node/fs/promises.d.ts 0000644 0001750 0000144 00000072625 14000133700 023663 0 ustar andreh users declare module 'fs/promises' { import { Stats, WriteVResult, ReadVResult, PathLike, RmDirOptions, RmOptions, MakeDirectoryOptions, Dirent, OpenDirOptions, Dir, BaseEncodingOptions, BufferEncodingOption, OpenMode, Mode, } from 'fs'; interface FileHandle { /** * Gets the file descriptor for this file handle. */ readonly fd: number; /** * Asynchronously append data to a file, creating the file if it does not exist. The underlying file will _not_ be closed automatically. * The `FileHandle` must have been opened for appending. * @param data The data to write. If something other than a `Buffer` or `Uint8Array` is provided, the value is coerced to a string. * @param options Either the encoding for the file, or an object optionally specifying the encoding, file mode, and flag. * If `encoding` is not supplied, the default of `'utf8'` is used. * If `mode` is not supplied, the default of `0o666` is used. * If `mode` is a string, it is parsed as an octal integer. * If `flag` is not supplied, the default of `'a'` is used. */ appendFile(data: string | Uint8Array, options?: BaseEncodingOptions & { mode?: Mode, flag?: OpenMode } | BufferEncoding | null): Promise<void>; /** * Asynchronous fchown(2) - Change ownership of a file. */ chown(uid: number, gid: number): Promise<void>; /** * Asynchronous fchmod(2) - Change permissions of a file. * @param mode A file mode. If a string is passed, it is parsed as an octal integer. */ chmod(mode: Mode): Promise<void>; /** * Asynchronous fdatasync(2) - synchronize a file's in-core state with storage device. */ datasync(): Promise<void>; /** * Asynchronous fsync(2) - synchronize a file's in-core state with the underlying storage device. */ sync(): Promise<void>; /** * Asynchronously reads data from the file. * The `FileHandle` must have been opened for reading. * @param buffer The buffer that the data will be written to. * @param offset The offset in the buffer at which to start writing. * @param length The number of bytes to read. * @param position The offset from the beginning of the file from which data should be read. If `null`, data will be read from the current position. */ read<TBuffer extends Uint8Array>(buffer: TBuffer, offset?: number | null, length?: number | null, position?: number | null): Promise<{ bytesRead: number, buffer: TBuffer }>; /** * Asynchronously reads the entire contents of a file. The underlying file will _not_ be closed automatically. * The `FileHandle` must have been opened for reading. * @param options An object that may contain an optional flag. * If a flag is not provided, it defaults to `'r'`. */ readFile(options?: { encoding?: null, flag?: OpenMode } | null): Promise<Buffer>; /** * Asynchronously reads the entire contents of a file. The underlying file will _not_ be closed automatically. * The `FileHandle` must have been opened for reading. * @param options An object that may contain an optional flag. * If a flag is not provided, it defaults to `'r'`. */ readFile(options: { encoding: BufferEncoding, flag?: OpenMode } | BufferEncoding): Promise<string>; /** * Asynchronously reads the entire contents of a file. The underlying file will _not_ be closed automatically. * The `FileHandle` must have been opened for reading. * @param options An object that may contain an optional flag. * If a flag is not provided, it defaults to `'r'`. */ readFile(options?: BaseEncodingOptions & { flag?: OpenMode } | BufferEncoding | null): Promise<string | Buffer>; /** * Asynchronous fstat(2) - Get file status. */ stat(): Promise<Stats>; /** * Asynchronous ftruncate(2) - Truncate a file to a specified length. * @param len If not specified, defaults to `0`. */ truncate(len?: number): Promise<void>; /** * Asynchronously change file timestamps of the file. * @param atime The last access time. If a string is provided, it will be coerced to number. * @param mtime The last modified time. If a string is provided, it will be coerced to number. */ utimes(atime: string | number | Date, mtime: string | number | Date): Promise<void>; /** * Asynchronously writes `buffer` to the file. * The `FileHandle` must have been opened for writing. * @param buffer The buffer that the data will be written to. * @param offset The part of the buffer to be written. If not supplied, defaults to `0`. * @param length The number of bytes to write. If not supplied, defaults to `buffer.length - offset`. * @param position The offset from the beginning of the file where this data should be written. If not supplied, defaults to the current position. */ write<TBuffer extends Uint8Array>(buffer: TBuffer, offset?: number | null, length?: number | null, position?: number | null): Promise<{ bytesWritten: number, buffer: TBuffer }>; /** * Asynchronously writes `string` to the file. * The `FileHandle` must have been opened for writing. * It is unsafe to call `write()` multiple times on the same file without waiting for the `Promise` * to be resolved (or rejected). For this scenario, `fs.createWriteStream` is strongly recommended. * @param string A string to write. * @param position The offset from the beginning of the file where this data should be written. If not supplied, defaults to the current position. * @param encoding The expected string encoding. */ write(data: string | Uint8Array, position?: number | null, encoding?: BufferEncoding | null): Promise<{ bytesWritten: number, buffer: string }>; /** * Asynchronously writes data to a file, replacing the file if it already exists. The underlying file will _not_ be closed automatically. * The `FileHandle` must have been opened for writing. * It is unsafe to call `writeFile()` multiple times on the same file without waiting for the `Promise` to be resolved (or rejected). * @param data The data to write. If something other than a `Buffer` or `Uint8Array` is provided, the value is coerced to a string. * @param options Either the encoding for the file, or an object optionally specifying the encoding, file mode, and flag. * If `encoding` is not supplied, the default of `'utf8'` is used. * If `mode` is not supplied, the default of `0o666` is used. * If `mode` is a string, it is parsed as an octal integer. * If `flag` is not supplied, the default of `'w'` is used. */ writeFile(data: string | Uint8Array, options?: BaseEncodingOptions & { mode?: Mode, flag?: OpenMode } | BufferEncoding | null): Promise<void>; /** * See `fs.writev` promisified version. */ writev(buffers: ReadonlyArray<NodeJS.ArrayBufferView>, position?: number): Promise<WriteVResult>; /** * See `fs.readv` promisified version. */ readv(buffers: ReadonlyArray<NodeJS.ArrayBufferView>, position?: number): Promise<ReadVResult>; /** * Asynchronous close(2) - close a `FileHandle`. */ close(): Promise<void>; } /** * Asynchronously tests a user's permissions for the file specified by path. * @param path A path to a file or directory. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. */ function access(path: PathLike, mode?: number): Promise<void>; /** * Asynchronously copies `src` to `dest`. By default, `dest` is overwritten if it already exists. * Node.js makes no guarantees about the atomicity of the copy operation. * If an error occurs after the destination file has been opened for writing, Node.js will attempt * to remove the destination. * @param src A path to the source file. * @param dest A path to the destination file. * @param flags An optional integer that specifies the behavior of the copy operation. The only * supported flag is `fs.constants.COPYFILE_EXCL`, which causes the copy operation to fail if * `dest` already exists. */ function copyFile(src: PathLike, dest: PathLike, flags?: number): Promise<void>; /** * Asynchronous open(2) - open and possibly create a file. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param mode A file mode. If a string is passed, it is parsed as an octal integer. If not * supplied, defaults to `0o666`. */ function open(path: PathLike, flags: string | number, mode?: Mode): Promise<FileHandle>; /** * Asynchronously reads data from the file referenced by the supplied `FileHandle`. * @param handle A `FileHandle`. * @param buffer The buffer that the data will be written to. * @param offset The offset in the buffer at which to start writing. * @param length The number of bytes to read. * @param position The offset from the beginning of the file from which data should be read. If * `null`, data will be read from the current position. */ function read<TBuffer extends Uint8Array>( handle: FileHandle, buffer: TBuffer, offset?: number | null, length?: number | null, position?: number | null, ): Promise<{ bytesRead: number, buffer: TBuffer }>; /** * Asynchronously writes `buffer` to the file referenced by the supplied `FileHandle`. * It is unsafe to call `fsPromises.write()` multiple times on the same file without waiting for the `Promise` * to be resolved (or rejected). For this scenario, `fs.createWriteStream` is strongly recommended. * @param handle A `FileHandle`. * @param buffer The buffer that the data will be written to. * @param offset The part of the buffer to be written. If not supplied, defaults to `0`. * @param length The number of bytes to write. If not supplied, defaults to `buffer.length - offset`. * @param position The offset from the beginning of the file where this data should be written. If not supplied, defaults to the current position. */ function write<TBuffer extends Uint8Array>( handle: FileHandle, buffer: TBuffer, offset?: number | null, length?: number | null, position?: number | null): Promise<{ bytesWritten: number, buffer: TBuffer }>; /** * Asynchronously writes `string` to the file referenced by the supplied `FileHandle`. * It is unsafe to call `fsPromises.write()` multiple times on the same file without waiting for the `Promise` * to be resolved (or rejected). For this scenario, `fs.createWriteStream` is strongly recommended. * @param handle A `FileHandle`. * @param string A string to write. * @param position The offset from the beginning of the file where this data should be written. If not supplied, defaults to the current position. * @param encoding The expected string encoding. */ function write(handle: FileHandle, string: string, position?: number | null, encoding?: BufferEncoding | null): Promise<{ bytesWritten: number, buffer: string }>; /** * Asynchronous rename(2) - Change the name or location of a file or directory. * @param oldPath A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. * @param newPath A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. */ function rename(oldPath: PathLike, newPath: PathLike): Promise<void>; /** * Asynchronous truncate(2) - Truncate a file to a specified length. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param len If not specified, defaults to `0`. */ function truncate(path: PathLike, len?: number): Promise<void>; /** * Asynchronous ftruncate(2) - Truncate a file to a specified length. * @param handle A `FileHandle`. * @param len If not specified, defaults to `0`. */ function ftruncate(handle: FileHandle, len?: number): Promise<void>; /** * Asynchronous rmdir(2) - delete a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ function rmdir(path: PathLike, options?: RmDirOptions): Promise<void>; /** * Asynchronously removes files and directories (modeled on the standard POSIX `rm` utility). */ function rm(path: PathLike, options?: RmOptions): Promise<void>; /** * Asynchronous fdatasync(2) - synchronize a file's in-core state with storage device. * @param handle A `FileHandle`. */ function fdatasync(handle: FileHandle): Promise<void>; /** * Asynchronous fsync(2) - synchronize a file's in-core state with the underlying storage device. * @param handle A `FileHandle`. */ function fsync(handle: FileHandle): Promise<void>; /** * Asynchronous mkdir(2) - create a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options Either the file mode, or an object optionally specifying the file mode and whether parent folders * should be created. If a string is passed, it is parsed as an octal integer. If not specified, defaults to `0o777`. */ function mkdir(path: PathLike, options: MakeDirectoryOptions & { recursive: true; }): Promise<string>; /** * Asynchronous mkdir(2) - create a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options Either the file mode, or an object optionally specifying the file mode and whether parent folders * should be created. If a string is passed, it is parsed as an octal integer. If not specified, defaults to `0o777`. */ function mkdir(path: PathLike, options?: Mode | (MakeDirectoryOptions & { recursive?: false; }) | null): Promise<void>; /** * Asynchronous mkdir(2) - create a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options Either the file mode, or an object optionally specifying the file mode and whether parent folders * should be created. If a string is passed, it is parsed as an octal integer. If not specified, defaults to `0o777`. */ function mkdir(path: PathLike, options?: Mode | MakeDirectoryOptions | null): Promise<string | undefined>; /** * Asynchronous readdir(3) - read a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ function readdir(path: PathLike, options?: BaseEncodingOptions & { withFileTypes?: false } | BufferEncoding | null): Promise<string[]>; /** * Asynchronous readdir(3) - read a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ function readdir(path: PathLike, options: { encoding: "buffer"; withFileTypes?: false } | "buffer"): Promise<Buffer[]>; /** * Asynchronous readdir(3) - read a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ function readdir(path: PathLike, options?: BaseEncodingOptions & { withFileTypes?: false } | BufferEncoding | null): Promise<string[] | Buffer[]>; /** * Asynchronous readdir(3) - read a directory. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options If called with `withFileTypes: true` the result data will be an array of Dirent. */ function readdir(path: PathLike, options: BaseEncodingOptions & { withFileTypes: true }): Promise<Dirent[]>; /** * Asynchronous readlink(2) - read value of a symbolic link. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ function readlink(path: PathLike, options?: BaseEncodingOptions | BufferEncoding | null): Promise<string>; /** * Asynchronous readlink(2) - read value of a symbolic link. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ function readlink(path: PathLike, options: BufferEncodingOption): Promise<Buffer>; /** * Asynchronous readlink(2) - read value of a symbolic link. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ function readlink(path: PathLike, options?: BaseEncodingOptions | string | null): Promise<string | Buffer>; /** * Asynchronous symlink(2) - Create a new symbolic link to an existing file. * @param target A path to an existing file. If a URL is provided, it must use the `file:` protocol. * @param path A path to the new symlink. If a URL is provided, it must use the `file:` protocol. * @param type May be set to `'dir'`, `'file'`, or `'junction'` (default is `'file'`) and is only available on Windows (ignored on other platforms). * When using `'junction'`, the `target` argument will automatically be normalized to an absolute path. */ function symlink(target: PathLike, path: PathLike, type?: string | null): Promise<void>; /** * Asynchronous fstat(2) - Get file status. * @param handle A `FileHandle`. */ function fstat(handle: FileHandle): Promise<Stats>; /** * Asynchronous lstat(2) - Get file status. Does not dereference symbolic links. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ function lstat(path: PathLike): Promise<Stats>; /** * Asynchronous stat(2) - Get file status. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ function stat(path: PathLike): Promise<Stats>; /** * Asynchronous link(2) - Create a new link (also known as a hard link) to an existing file. * @param existingPath A path to a file. If a URL is provided, it must use the `file:` protocol. * @param newPath A path to a file. If a URL is provided, it must use the `file:` protocol. */ function link(existingPath: PathLike, newPath: PathLike): Promise<void>; /** * Asynchronous unlink(2) - delete a name and possibly the file it refers to. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ function unlink(path: PathLike): Promise<void>; /** * Asynchronous fchmod(2) - Change permissions of a file. * @param handle A `FileHandle`. * @param mode A file mode. If a string is passed, it is parsed as an octal integer. */ function fchmod(handle: FileHandle, mode: Mode): Promise<void>; /** * Asynchronous chmod(2) - Change permissions of a file. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param mode A file mode. If a string is passed, it is parsed as an octal integer. */ function chmod(path: PathLike, mode: Mode): Promise<void>; /** * Asynchronous lchmod(2) - Change permissions of a file. Does not dereference symbolic links. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param mode A file mode. If a string is passed, it is parsed as an octal integer. */ function lchmod(path: PathLike, mode: Mode): Promise<void>; /** * Asynchronous lchown(2) - Change ownership of a file. Does not dereference symbolic links. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ function lchown(path: PathLike, uid: number, gid: number): Promise<void>; /** * Changes the access and modification times of a file in the same way as `fsPromises.utimes()`, * with the difference that if the path refers to a symbolic link, then the link is not * dereferenced: instead, the timestamps of the symbolic link itself are changed. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param atime The last access time. If a string is provided, it will be coerced to number. * @param mtime The last modified time. If a string is provided, it will be coerced to number. */ function lutimes(path: PathLike, atime: string | number | Date, mtime: string | number | Date): Promise<void>; /** * Asynchronous fchown(2) - Change ownership of a file. * @param handle A `FileHandle`. */ function fchown(handle: FileHandle, uid: number, gid: number): Promise<void>; /** * Asynchronous chown(2) - Change ownership of a file. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. */ function chown(path: PathLike, uid: number, gid: number): Promise<void>; /** * Asynchronously change file timestamps of the file referenced by the supplied path. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param atime The last access time. If a string is provided, it will be coerced to number. * @param mtime The last modified time. If a string is provided, it will be coerced to number. */ function utimes(path: PathLike, atime: string | number | Date, mtime: string | number | Date): Promise<void>; /** * Asynchronously change file timestamps of the file referenced by the supplied `FileHandle`. * @param handle A `FileHandle`. * @param atime The last access time. If a string is provided, it will be coerced to number. * @param mtime The last modified time. If a string is provided, it will be coerced to number. */ function futimes(handle: FileHandle, atime: string | number | Date, mtime: string | number | Date): Promise<void>; /** * Asynchronous realpath(3) - return the canonicalized absolute pathname. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ function realpath(path: PathLike, options?: BaseEncodingOptions | BufferEncoding | null): Promise<string>; /** * Asynchronous realpath(3) - return the canonicalized absolute pathname. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ function realpath(path: PathLike, options: BufferEncodingOption): Promise<Buffer>; /** * Asynchronous realpath(3) - return the canonicalized absolute pathname. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ function realpath(path: PathLike, options?: BaseEncodingOptions | BufferEncoding | null): Promise<string | Buffer>; /** * Asynchronously creates a unique temporary directory. * Generates six random characters to be appended behind a required `prefix` to create a unique temporary directory. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ function mkdtemp(prefix: string, options?: BaseEncodingOptions | BufferEncoding | null): Promise<string>; /** * Asynchronously creates a unique temporary directory. * Generates six random characters to be appended behind a required `prefix` to create a unique temporary directory. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ function mkdtemp(prefix: string, options: BufferEncodingOption): Promise<Buffer>; /** * Asynchronously creates a unique temporary directory. * Generates six random characters to be appended behind a required `prefix` to create a unique temporary directory. * @param options The encoding (or an object specifying the encoding), used as the encoding of the result. If not provided, `'utf8'` is used. */ function mkdtemp(prefix: string, options?: BaseEncodingOptions | BufferEncoding | null): Promise<string | Buffer>; /** * Asynchronously writes data to a file, replacing the file if it already exists. * It is unsafe to call `fsPromises.writeFile()` multiple times on the same file without waiting for the `Promise` to be resolved (or rejected). * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. * If a `FileHandle` is provided, the underlying file will _not_ be closed automatically. * @param data The data to write. If something other than a `Buffer` or `Uint8Array` is provided, the value is coerced to a string. * @param options Either the encoding for the file, or an object optionally specifying the encoding, file mode, and flag. * If `encoding` is not supplied, the default of `'utf8'` is used. * If `mode` is not supplied, the default of `0o666` is used. * If `mode` is a string, it is parsed as an octal integer. * If `flag` is not supplied, the default of `'w'` is used. */ function writeFile(path: PathLike | FileHandle, data: string | Uint8Array, options?: BaseEncodingOptions & { mode?: Mode, flag?: OpenMode } | BufferEncoding | null): Promise<void>; /** * Asynchronously append data to a file, creating the file if it does not exist. * @param file A path to a file. If a URL is provided, it must use the `file:` protocol. * URL support is _experimental_. * If a `FileHandle` is provided, the underlying file will _not_ be closed automatically. * @param data The data to write. If something other than a `Buffer` or `Uint8Array` is provided, the value is coerced to a string. * @param options Either the encoding for the file, or an object optionally specifying the encoding, file mode, and flag. * If `encoding` is not supplied, the default of `'utf8'` is used. * If `mode` is not supplied, the default of `0o666` is used. * If `mode` is a string, it is parsed as an octal integer. * If `flag` is not supplied, the default of `'a'` is used. */ function appendFile(path: PathLike | FileHandle, data: string | Uint8Array, options?: BaseEncodingOptions & { mode?: Mode, flag?: OpenMode } | BufferEncoding | null): Promise<void>; /** * Asynchronously reads the entire contents of a file. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * If a `FileHandle` is provided, the underlying file will _not_ be closed automatically. * @param options An object that may contain an optional flag. * If a flag is not provided, it defaults to `'r'`. */ function readFile(path: PathLike | FileHandle, options?: { encoding?: null, flag?: OpenMode } | null): Promise<Buffer>; /** * Asynchronously reads the entire contents of a file. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * If a `FileHandle` is provided, the underlying file will _not_ be closed automatically. * @param options An object that may contain an optional flag. * If a flag is not provided, it defaults to `'r'`. */ function readFile(path: PathLike | FileHandle, options: { encoding: BufferEncoding, flag?: OpenMode } | BufferEncoding): Promise<string>; /** * Asynchronously reads the entire contents of a file. * @param path A path to a file. If a URL is provided, it must use the `file:` protocol. * If a `FileHandle` is provided, the underlying file will _not_ be closed automatically. * @param options An object that may contain an optional flag. * If a flag is not provided, it defaults to `'r'`. */ function readFile(path: PathLike | FileHandle, options?: BaseEncodingOptions & { flag?: OpenMode } | BufferEncoding | null): Promise<string | Buffer>; function opendir(path: string, options?: OpenDirOptions): Promise<Dir>; } apollo-server-demo/node_modules/@types/node/v8.d.ts 0000644 0001750 0000144 00000015161 14000133701 021740 0 ustar andreh users declare module "v8" { import { Readable } from "stream"; interface HeapSpaceInfo { space_name: string; space_size: number; space_used_size: number; space_available_size: number; physical_space_size: number; } // ** Signifies if the --zap_code_space option is enabled or not. 1 == enabled, 0 == disabled. */ type DoesZapCodeSpaceFlag = 0 | 1; interface HeapInfo { total_heap_size: number; total_heap_size_executable: number; total_physical_size: number; total_available_size: number; used_heap_size: number; heap_size_limit: number; malloced_memory: number; peak_malloced_memory: number; does_zap_garbage: DoesZapCodeSpaceFlag; number_of_native_contexts: number; number_of_detached_contexts: number; } interface HeapCodeStatistics { code_and_metadata_size: number; bytecode_and_metadata_size: number; external_script_source_size: number; } /** * Returns an integer representing a "version tag" derived from the V8 version, command line flags and detected CPU features. * This is useful for determining whether a vm.Script cachedData buffer is compatible with this instance of V8. */ function cachedDataVersionTag(): number; function getHeapStatistics(): HeapInfo; function getHeapSpaceStatistics(): HeapSpaceInfo[]; function setFlagsFromString(flags: string): void; /** * Generates a snapshot of the current V8 heap and returns a Readable * Stream that may be used to read the JSON serialized representation. * This conversation was marked as resolved by joyeecheung * This JSON stream format is intended to be used with tools such as * Chrome DevTools. The JSON schema is undocumented and specific to the * V8 engine, and may change from one version of V8 to the next. */ function getHeapSnapshot(): Readable; /** * * @param fileName The file path where the V8 heap snapshot is to be * saved. If not specified, a file name with the pattern * `'Heap-${yyyymmdd}-${hhmmss}-${pid}-${thread_id}.heapsnapshot'` will be * generated, where `{pid}` will be the PID of the Node.js process, * `{thread_id}` will be `0` when `writeHeapSnapshot()` is called from * the main Node.js thread or the id of a worker thread. */ function writeHeapSnapshot(fileName?: string): string; function getHeapCodeStatistics(): HeapCodeStatistics; class Serializer { /** * Writes out a header, which includes the serialization format version. */ writeHeader(): void; /** * Serializes a JavaScript value and adds the serialized representation to the internal buffer. * This throws an error if value cannot be serialized. */ writeValue(val: any): boolean; /** * Returns the stored internal buffer. * This serializer should not be used once the buffer is released. * Calling this method results in undefined behavior if a previous write has failed. */ releaseBuffer(): Buffer; /** * Marks an ArrayBuffer as having its contents transferred out of band.\ * Pass the corresponding ArrayBuffer in the deserializing context to deserializer.transferArrayBuffer(). */ transferArrayBuffer(id: number, arrayBuffer: ArrayBuffer): void; /** * Write a raw 32-bit unsigned integer. */ writeUint32(value: number): void; /** * Write a raw 64-bit unsigned integer, split into high and low 32-bit parts. */ writeUint64(hi: number, lo: number): void; /** * Write a JS number value. */ writeDouble(value: number): void; /** * Write raw bytes into the serializer’s internal buffer. * The deserializer will require a way to compute the length of the buffer. */ writeRawBytes(buffer: NodeJS.TypedArray): void; } /** * A subclass of `Serializer` that serializes `TypedArray` (in particular `Buffer`) and `DataView` objects as host objects, * and only stores the part of their underlying `ArrayBuffers` that they are referring to. */ class DefaultSerializer extends Serializer { } class Deserializer { constructor(data: NodeJS.TypedArray); /** * Reads and validates a header (including the format version). * May, for example, reject an invalid or unsupported wire format. * In that case, an Error is thrown. */ readHeader(): boolean; /** * Deserializes a JavaScript value from the buffer and returns it. */ readValue(): any; /** * Marks an ArrayBuffer as having its contents transferred out of band. * Pass the corresponding `ArrayBuffer` in the serializing context to serializer.transferArrayBuffer() * (or return the id from serializer._getSharedArrayBufferId() in the case of SharedArrayBuffers). */ transferArrayBuffer(id: number, arrayBuffer: ArrayBuffer): void; /** * Reads the underlying wire format version. * Likely mostly to be useful to legacy code reading old wire format versions. * May not be called before .readHeader(). */ getWireFormatVersion(): number; /** * Read a raw 32-bit unsigned integer and return it. */ readUint32(): number; /** * Read a raw 64-bit unsigned integer and return it as an array [hi, lo] with two 32-bit unsigned integer entries. */ readUint64(): [number, number]; /** * Read a JS number value. */ readDouble(): number; /** * Read raw bytes from the deserializer’s internal buffer. * The length parameter must correspond to the length of the buffer that was passed to serializer.writeRawBytes(). */ readRawBytes(length: number): Buffer; } /** * A subclass of `Serializer` that serializes `TypedArray` (in particular `Buffer`) and `DataView` objects as host objects, * and only stores the part of their underlying `ArrayBuffers` that they are referring to. */ class DefaultDeserializer extends Deserializer { } /** * Uses a `DefaultSerializer` to serialize value into a buffer. */ function serialize(value: any): Buffer; /** * Uses a `DefaultDeserializer` with default options to read a JS value from a buffer. */ function deserialize(data: NodeJS.TypedArray): any; } apollo-server-demo/node_modules/@types/node/async_hooks.d.ts 0000644 0001750 0000144 00000022735 14000133701 023730 0 ustar andreh users /** * Async Hooks module: https://nodejs.org/api/async_hooks.html */ declare module "async_hooks" { /** * Returns the asyncId of the current execution context. */ function executionAsyncId(): number; /** * The resource representing the current execution. * Useful to store data within the resource. * * Resource objects returned by `executionAsyncResource()` are most often internal * Node.js handle objects with undocumented APIs. Using any functions or properties * on the object is likely to crash your application and should be avoided. * * Using `executionAsyncResource()` in the top-level execution context will * return an empty object as there is no handle or request object to use, * but having an object representing the top-level can be helpful. */ function executionAsyncResource(): object; /** * Returns the ID of the resource responsible for calling the callback that is currently being executed. */ function triggerAsyncId(): number; interface HookCallbacks { /** * Called when a class is constructed that has the possibility to emit an asynchronous event. * @param asyncId a unique ID for the async resource * @param type the type of the async resource * @param triggerAsyncId the unique ID of the async resource in whose execution context this async resource was created * @param resource reference to the resource representing the async operation, needs to be released during destroy */ init?(asyncId: number, type: string, triggerAsyncId: number, resource: object): void; /** * When an asynchronous operation is initiated or completes a callback is called to notify the user. * The before callback is called just before said callback is executed. * @param asyncId the unique identifier assigned to the resource about to execute the callback. */ before?(asyncId: number): void; /** * Called immediately after the callback specified in before is completed. * @param asyncId the unique identifier assigned to the resource which has executed the callback. */ after?(asyncId: number): void; /** * Called when a promise has resolve() called. This may not be in the same execution id * as the promise itself. * @param asyncId the unique id for the promise that was resolve()d. */ promiseResolve?(asyncId: number): void; /** * Called after the resource corresponding to asyncId is destroyed * @param asyncId a unique ID for the async resource */ destroy?(asyncId: number): void; } interface AsyncHook { /** * Enable the callbacks for a given AsyncHook instance. If no callbacks are provided enabling is a noop. */ enable(): this; /** * Disable the callbacks for a given AsyncHook instance from the global pool of AsyncHook callbacks to be executed. Once a hook has been disabled it will not be called again until enabled. */ disable(): this; } /** * Registers functions to be called for different lifetime events of each async operation. * @param options the callbacks to register * @return an AsyncHooks instance used for disabling and enabling hooks */ function createHook(options: HookCallbacks): AsyncHook; interface AsyncResourceOptions { /** * The ID of the execution context that created this async event. * Default: `executionAsyncId()` */ triggerAsyncId?: number; /** * Disables automatic `emitDestroy` when the object is garbage collected. * This usually does not need to be set (even if `emitDestroy` is called * manually), unless the resource's `asyncId` is retrieved and the * sensitive API's `emitDestroy` is called with it. * Default: `false` */ requireManualDestroy?: boolean; } /** * The class AsyncResource was designed to be extended by the embedder's async resources. * Using this users can easily trigger the lifetime events of their own resources. */ class AsyncResource { /** * AsyncResource() is meant to be extended. Instantiating a * new AsyncResource() also triggers init. If triggerAsyncId is omitted then * async_hook.executionAsyncId() is used. * @param type The type of async event. * @param triggerAsyncId The ID of the execution context that created * this async event (default: `executionAsyncId()`), or an * AsyncResourceOptions object (since 9.3) */ constructor(type: string, triggerAsyncId?: number|AsyncResourceOptions); /** * Binds the given function to the current execution context. * @param fn The function to bind to the current execution context. * @param type An optional name to associate with the underlying `AsyncResource`. */ static bind<Func extends (...args: any[]) => any>(fn: Func, type?: string): Func & { asyncResource: AsyncResource }; /** * Binds the given function to execute to this `AsyncResource`'s scope. * @param fn The function to bind to the current `AsyncResource`. */ bind<Func extends (...args: any[]) => any>(fn: Func): Func & { asyncResource: AsyncResource }; /** * Call the provided function with the provided arguments in the * execution context of the async resource. This will establish the * context, trigger the AsyncHooks before callbacks, call the function, * trigger the AsyncHooks after callbacks, and then restore the original * execution context. * @param fn The function to call in the execution context of this * async resource. * @param thisArg The receiver to be used for the function call. * @param args Optional arguments to pass to the function. */ runInAsyncScope<This, Result>(fn: (this: This, ...args: any[]) => Result, thisArg?: This, ...args: any[]): Result; /** * Call AsyncHooks destroy callbacks. */ emitDestroy(): void; /** * @return the unique ID assigned to this AsyncResource instance. */ asyncId(): number; /** * @return the trigger ID for this AsyncResource instance. */ triggerAsyncId(): number; } /** * When having multiple instances of `AsyncLocalStorage`, they are independent * from each other. It is safe to instantiate this class multiple times. */ class AsyncLocalStorage<T> { /** * This method disables the instance of `AsyncLocalStorage`. All subsequent calls * to `asyncLocalStorage.getStore()` will return `undefined` until * `asyncLocalStorage.run()` is called again. * * When calling `asyncLocalStorage.disable()`, all current contexts linked to the * instance will be exited. * * Calling `asyncLocalStorage.disable()` is required before the * `asyncLocalStorage` can be garbage collected. This does not apply to stores * provided by the `asyncLocalStorage`, as those objects are garbage collected * along with the corresponding async resources. * * This method is to be used when the `asyncLocalStorage` is not in use anymore * in the current process. */ disable(): void; /** * This method returns the current store. If this method is called outside of an * asynchronous context initialized by calling `asyncLocalStorage.run`, it will * return `undefined`. */ getStore(): T | undefined; /** * This methods runs a function synchronously within a context and return its * return value. The store is not accessible outside of the callback function or * the asynchronous operations created within the callback. * * Optionally, arguments can be passed to the function. They will be passed to the * callback function. * * I the callback function throws an error, it will be thrown by `run` too. The * stacktrace will not be impacted by this call and the context will be exited. */ // TODO: Apply generic vararg once available run<R>(store: T, callback: (...args: any[]) => R, ...args: any[]): R; /** * This methods runs a function synchronously outside of a context and return its * return value. The store is not accessible within the callback function or the * asynchronous operations created within the callback. * * Optionally, arguments can be passed to the function. They will be passed to the * callback function. * * If the callback function throws an error, it will be thrown by `exit` too. The * stacktrace will not be impacted by this call and the context will be * re-entered. */ // TODO: Apply generic vararg once available exit<R>(callback: (...args: any[]) => R, ...args: any[]): R; /** * Calling `asyncLocalStorage.enterWith(store)` will transition into the context * for the remainder of the current synchronous execution and will persist * through any following asynchronous calls. */ enterWith(store: T): void; } } apollo-server-demo/node_modules/@types/node/repl.d.ts 0000644 0001750 0000144 00000043306 14000133700 022346 0 ustar andreh users declare module "repl" { import { Interface, Completer, AsyncCompleter } from "readline"; import { Context } from "vm"; import { InspectOptions } from "util"; interface ReplOptions { /** * The input prompt to display. * Default: `"> "` */ prompt?: string; /** * The `Readable` stream from which REPL input will be read. * Default: `process.stdin` */ input?: NodeJS.ReadableStream; /** * The `Writable` stream to which REPL output will be written. * Default: `process.stdout` */ output?: NodeJS.WritableStream; /** * If `true`, specifies that the output should be treated as a TTY terminal, and have * ANSI/VT100 escape codes written to it. * Default: checking the value of the `isTTY` property on the output stream upon * instantiation. */ terminal?: boolean; /** * The function to be used when evaluating each given line of input. * Default: an async wrapper for the JavaScript `eval()` function. An `eval` function can * error with `repl.Recoverable` to indicate the input was incomplete and prompt for * additional lines. * * @see https://nodejs.org/dist/latest-v10.x/docs/api/repl.html#repl_default_evaluation * @see https://nodejs.org/dist/latest-v10.x/docs/api/repl.html#repl_custom_evaluation_functions */ eval?: REPLEval; /** * Defines if the repl prints output previews or not. * @default `true` Always `false` in case `terminal` is falsy. */ preview?: boolean; /** * If `true`, specifies that the default `writer` function should include ANSI color * styling to REPL output. If a custom `writer` function is provided then this has no * effect. * Default: the REPL instance's `terminal` value. */ useColors?: boolean; /** * If `true`, specifies that the default evaluation function will use the JavaScript * `global` as the context as opposed to creating a new separate context for the REPL * instance. The node CLI REPL sets this value to `true`. * Default: `false`. */ useGlobal?: boolean; /** * If `true`, specifies that the default writer will not output the return value of a * command if it evaluates to `undefined`. * Default: `false`. */ ignoreUndefined?: boolean; /** * The function to invoke to format the output of each command before writing to `output`. * Default: a wrapper for `util.inspect`. * * @see https://nodejs.org/dist/latest-v10.x/docs/api/repl.html#repl_customizing_repl_output */ writer?: REPLWriter; /** * An optional function used for custom Tab auto completion. * * @see https://nodejs.org/dist/latest-v11.x/docs/api/readline.html#readline_use_of_the_completer_function */ completer?: Completer | AsyncCompleter; /** * A flag that specifies whether the default evaluator executes all JavaScript commands in * strict mode or default (sloppy) mode. * Accepted values are: * - `repl.REPL_MODE_SLOPPY` - evaluates expressions in sloppy mode. * - `repl.REPL_MODE_STRICT` - evaluates expressions in strict mode. This is equivalent to * prefacing every repl statement with `'use strict'`. */ replMode?: typeof REPL_MODE_SLOPPY | typeof REPL_MODE_STRICT; /** * Stop evaluating the current piece of code when `SIGINT` is received, i.e. `Ctrl+C` is * pressed. This cannot be used together with a custom `eval` function. * Default: `false`. */ breakEvalOnSigint?: boolean; } type REPLEval = (this: REPLServer, evalCmd: string, context: Context, file: string, cb: (err: Error | null, result: any) => void) => void; type REPLWriter = (this: REPLServer, obj: any) => string; /** * This is the default "writer" value, if none is passed in the REPL options, * and it can be overridden by custom print functions. */ const writer: REPLWriter & { options: InspectOptions }; type REPLCommandAction = (this: REPLServer, text: string) => void; interface REPLCommand { /** * Help text to be displayed when `.help` is entered. */ help?: string; /** * The function to execute, optionally accepting a single string argument. */ action: REPLCommandAction; } /** * Provides a customizable Read-Eval-Print-Loop (REPL). * * Instances of `repl.REPLServer` will accept individual lines of user input, evaluate those * according to a user-defined evaluation function, then output the result. Input and output * may be from `stdin` and `stdout`, respectively, or may be connected to any Node.js `stream`. * * Instances of `repl.REPLServer` support automatic completion of inputs, simplistic Emacs-style * line editing, multi-line inputs, ANSI-styled output, saving and restoring current REPL session * state, error recovery, and customizable evaluation functions. * * Instances of `repl.REPLServer` are created using the `repl.start()` method and _should not_ * be created directly using the JavaScript `new` keyword. * * @see https://nodejs.org/dist/latest-v10.x/docs/api/repl.html#repl_repl */ class REPLServer extends Interface { /** * The `vm.Context` provided to the `eval` function to be used for JavaScript * evaluation. */ readonly context: Context; /** * @deprecated since v14.3.0 - Use `input` instead. */ readonly inputStream: NodeJS.ReadableStream; /** * @deprecated since v14.3.0 - Use `output` instead. */ readonly outputStream: NodeJS.WritableStream; /** * The `Readable` stream from which REPL input will be read. */ readonly input: NodeJS.ReadableStream; /** * The `Writable` stream to which REPL output will be written. */ readonly output: NodeJS.WritableStream; /** * The commands registered via `replServer.defineCommand()`. */ readonly commands: NodeJS.ReadOnlyDict<REPLCommand>; /** * A value indicating whether the REPL is currently in "editor mode". * * @see https://nodejs.org/dist/latest-v10.x/docs/api/repl.html#repl_commands_and_special_keys */ readonly editorMode: boolean; /** * A value indicating whether the `_` variable has been assigned. * * @see https://nodejs.org/dist/latest-v10.x/docs/api/repl.html#repl_assignment_of_the_underscore_variable */ readonly underscoreAssigned: boolean; /** * The last evaluation result from the REPL (assigned to the `_` variable inside of the REPL). * * @see https://nodejs.org/dist/latest-v10.x/docs/api/repl.html#repl_assignment_of_the_underscore_variable */ readonly last: any; /** * A value indicating whether the `_error` variable has been assigned. * * @since v9.8.0 * @see https://nodejs.org/dist/latest-v10.x/docs/api/repl.html#repl_assignment_of_the_underscore_variable */ readonly underscoreErrAssigned: boolean; /** * The last error raised inside the REPL (assigned to the `_error` variable inside of the REPL). * * @since v9.8.0 * @see https://nodejs.org/dist/latest-v10.x/docs/api/repl.html#repl_assignment_of_the_underscore_variable */ readonly lastError: any; /** * Specified in the REPL options, this is the function to be used when evaluating each * given line of input. If not specified in the REPL options, this is an async wrapper * for the JavaScript `eval()` function. */ readonly eval: REPLEval; /** * Specified in the REPL options, this is a value indicating whether the default * `writer` function should include ANSI color styling to REPL output. */ readonly useColors: boolean; /** * Specified in the REPL options, this is a value indicating whether the default `eval` * function will use the JavaScript `global` as the context as opposed to creating a new * separate context for the REPL instance. */ readonly useGlobal: boolean; /** * Specified in the REPL options, this is a value indicating whether the default `writer` * function should output the result of a command if it evaluates to `undefined`. */ readonly ignoreUndefined: boolean; /** * Specified in the REPL options, this is the function to invoke to format the output of * each command before writing to `outputStream`. If not specified in the REPL options, * this will be a wrapper for `util.inspect`. */ readonly writer: REPLWriter; /** * Specified in the REPL options, this is the function to use for custom Tab auto-completion. */ readonly completer: Completer | AsyncCompleter; /** * Specified in the REPL options, this is a flag that specifies whether the default `eval` * function should execute all JavaScript commands in strict mode or default (sloppy) mode. * Possible values are: * - `repl.REPL_MODE_SLOPPY` - evaluates expressions in sloppy mode. * - `repl.REPL_MODE_STRICT` - evaluates expressions in strict mode. This is equivalent to * prefacing every repl statement with `'use strict'`. */ readonly replMode: typeof REPL_MODE_SLOPPY | typeof REPL_MODE_STRICT; /** * NOTE: According to the documentation: * * > Instances of `repl.REPLServer` are created using the `repl.start()` method and * > _should not_ be created directly using the JavaScript `new` keyword. * * `REPLServer` cannot be subclassed due to implementation specifics in NodeJS. * * @see https://nodejs.org/dist/latest-v10.x/docs/api/repl.html#repl_class_replserver */ private constructor(); /** * Used to add new `.`-prefixed commands to the REPL instance. Such commands are invoked * by typing a `.` followed by the `keyword`. * * @param keyword The command keyword (_without_ a leading `.` character). * @param cmd The function to invoke when the command is processed. * * @see https://nodejs.org/dist/latest-v10.x/docs/api/repl.html#repl_replserver_definecommand_keyword_cmd */ defineCommand(keyword: string, cmd: REPLCommandAction | REPLCommand): void; /** * Readies the REPL instance for input from the user, printing the configured `prompt` to a * new line in the `output` and resuming the `input` to accept new input. * * When multi-line input is being entered, an ellipsis is printed rather than the 'prompt'. * * This method is primarily intended to be called from within the action function for * commands registered using the `replServer.defineCommand()` method. * * @param preserveCursor When `true`, the cursor placement will not be reset to `0`. */ displayPrompt(preserveCursor?: boolean): void; /** * Clears any command that has been buffered but not yet executed. * * This method is primarily intended to be called from within the action function for * commands registered using the `replServer.defineCommand()` method. * * @since v9.0.0 */ clearBufferedCommand(): void; /** * Initializes a history log file for the REPL instance. When executing the * Node.js binary and using the command line REPL, a history file is initialized * by default. However, this is not the case when creating a REPL * programmatically. Use this method to initialize a history log file when working * with REPL instances programmatically. * @param path The path to the history file */ setupHistory(path: string, cb: (err: Error | null, repl: this) => void): void; /** * events.EventEmitter * 1. close - inherited from `readline.Interface` * 2. line - inherited from `readline.Interface` * 3. pause - inherited from `readline.Interface` * 4. resume - inherited from `readline.Interface` * 5. SIGCONT - inherited from `readline.Interface` * 6. SIGINT - inherited from `readline.Interface` * 7. SIGTSTP - inherited from `readline.Interface` * 8. exit * 9. reset */ addListener(event: string, listener: (...args: any[]) => void): this; addListener(event: "close", listener: () => void): this; addListener(event: "line", listener: (input: string) => void): this; addListener(event: "pause", listener: () => void): this; addListener(event: "resume", listener: () => void): this; addListener(event: "SIGCONT", listener: () => void): this; addListener(event: "SIGINT", listener: () => void): this; addListener(event: "SIGTSTP", listener: () => void): this; addListener(event: "exit", listener: () => void): this; addListener(event: "reset", listener: (context: Context) => void): this; emit(event: string | symbol, ...args: any[]): boolean; emit(event: "close"): boolean; emit(event: "line", input: string): boolean; emit(event: "pause"): boolean; emit(event: "resume"): boolean; emit(event: "SIGCONT"): boolean; emit(event: "SIGINT"): boolean; emit(event: "SIGTSTP"): boolean; emit(event: "exit"): boolean; emit(event: "reset", context: Context): boolean; on(event: string, listener: (...args: any[]) => void): this; on(event: "close", listener: () => void): this; on(event: "line", listener: (input: string) => void): this; on(event: "pause", listener: () => void): this; on(event: "resume", listener: () => void): this; on(event: "SIGCONT", listener: () => void): this; on(event: "SIGINT", listener: () => void): this; on(event: "SIGTSTP", listener: () => void): this; on(event: "exit", listener: () => void): this; on(event: "reset", listener: (context: Context) => void): this; once(event: string, listener: (...args: any[]) => void): this; once(event: "close", listener: () => void): this; once(event: "line", listener: (input: string) => void): this; once(event: "pause", listener: () => void): this; once(event: "resume", listener: () => void): this; once(event: "SIGCONT", listener: () => void): this; once(event: "SIGINT", listener: () => void): this; once(event: "SIGTSTP", listener: () => void): this; once(event: "exit", listener: () => void): this; once(event: "reset", listener: (context: Context) => void): this; prependListener(event: string, listener: (...args: any[]) => void): this; prependListener(event: "close", listener: () => void): this; prependListener(event: "line", listener: (input: string) => void): this; prependListener(event: "pause", listener: () => void): this; prependListener(event: "resume", listener: () => void): this; prependListener(event: "SIGCONT", listener: () => void): this; prependListener(event: "SIGINT", listener: () => void): this; prependListener(event: "SIGTSTP", listener: () => void): this; prependListener(event: "exit", listener: () => void): this; prependListener(event: "reset", listener: (context: Context) => void): this; prependOnceListener(event: string, listener: (...args: any[]) => void): this; prependOnceListener(event: "close", listener: () => void): this; prependOnceListener(event: "line", listener: (input: string) => void): this; prependOnceListener(event: "pause", listener: () => void): this; prependOnceListener(event: "resume", listener: () => void): this; prependOnceListener(event: "SIGCONT", listener: () => void): this; prependOnceListener(event: "SIGINT", listener: () => void): this; prependOnceListener(event: "SIGTSTP", listener: () => void): this; prependOnceListener(event: "exit", listener: () => void): this; prependOnceListener(event: "reset", listener: (context: Context) => void): this; } /** * A flag passed in the REPL options. Evaluates expressions in sloppy mode. */ const REPL_MODE_SLOPPY: unique symbol; /** * A flag passed in the REPL options. Evaluates expressions in strict mode. * This is equivalent to prefacing every repl statement with `'use strict'`. */ const REPL_MODE_STRICT: unique symbol; /** * Creates and starts a `repl.REPLServer` instance. * * @param options The options for the `REPLServer`. If `options` is a string, then it specifies * the input prompt. */ function start(options?: string | ReplOptions): REPLServer; /** * Indicates a recoverable error that a `REPLServer` can use to support multi-line input. * * @see https://nodejs.org/dist/latest-v10.x/docs/api/repl.html#repl_recoverable_errors */ class Recoverable extends SyntaxError { err: Error; constructor(err: Error); } } apollo-server-demo/node_modules/@types/node/child_process.d.ts 0000644 0001750 0000144 00000060774 14000133700 024235 0 ustar andreh users declare module "child_process" { import { BaseEncodingOptions } from 'fs'; import * as events from "events"; import * as net from "net"; import { Writable, Readable, Stream, Pipe } from "stream"; type Serializable = string | object | number | boolean; type SendHandle = net.Socket | net.Server; interface ChildProcess extends events.EventEmitter { stdin: Writable | null; stdout: Readable | null; stderr: Readable | null; readonly channel?: Pipe | null; readonly stdio: [ Writable | null, // stdin Readable | null, // stdout Readable | null, // stderr Readable | Writable | null | undefined, // extra Readable | Writable | null | undefined // extra ]; readonly killed: boolean; readonly pid: number; readonly connected: boolean; readonly exitCode: number | null; readonly signalCode: NodeJS.Signals | null; readonly spawnargs: string[]; readonly spawnfile: string; kill(signal?: NodeJS.Signals | number): boolean; send(message: Serializable, callback?: (error: Error | null) => void): boolean; send(message: Serializable, sendHandle?: SendHandle, callback?: (error: Error | null) => void): boolean; send(message: Serializable, sendHandle?: SendHandle, options?: MessageOptions, callback?: (error: Error | null) => void): boolean; disconnect(): void; unref(): void; ref(): void; /** * events.EventEmitter * 1. close * 2. disconnect * 3. error * 4. exit * 5. message */ addListener(event: string, listener: (...args: any[]) => void): this; addListener(event: "close", listener: (code: number | null, signal: NodeJS.Signals | null) => void): this; addListener(event: "disconnect", listener: () => void): this; addListener(event: "error", listener: (err: Error) => void): this; addListener(event: "exit", listener: (code: number | null, signal: NodeJS.Signals | null) => void): this; addListener(event: "message", listener: (message: Serializable, sendHandle: SendHandle) => void): this; emit(event: string | symbol, ...args: any[]): boolean; emit(event: "close", code: number | null, signal: NodeJS.Signals | null): boolean; emit(event: "disconnect"): boolean; emit(event: "error", err: Error): boolean; emit(event: "exit", code: number | null, signal: NodeJS.Signals | null): boolean; emit(event: "message", message: Serializable, sendHandle: SendHandle): boolean; on(event: string, listener: (...args: any[]) => void): this; on(event: "close", listener: (code: number | null, signal: NodeJS.Signals | null) => void): this; on(event: "disconnect", listener: () => void): this; on(event: "error", listener: (err: Error) => void): this; on(event: "exit", listener: (code: number | null, signal: NodeJS.Signals | null) => void): this; on(event: "message", listener: (message: Serializable, sendHandle: SendHandle) => void): this; once(event: string, listener: (...args: any[]) => void): this; once(event: "close", listener: (code: number | null, signal: NodeJS.Signals | null) => void): this; once(event: "disconnect", listener: () => void): this; once(event: "error", listener: (err: Error) => void): this; once(event: "exit", listener: (code: number | null, signal: NodeJS.Signals | null) => void): this; once(event: "message", listener: (message: Serializable, sendHandle: SendHandle) => void): this; prependListener(event: string, listener: (...args: any[]) => void): this; prependListener(event: "close", listener: (code: number | null, signal: NodeJS.Signals | null) => void): this; prependListener(event: "disconnect", listener: () => void): this; prependListener(event: "error", listener: (err: Error) => void): this; prependListener(event: "exit", listener: (code: number | null, signal: NodeJS.Signals | null) => void): this; prependListener(event: "message", listener: (message: Serializable, sendHandle: SendHandle) => void): this; prependOnceListener(event: string, listener: (...args: any[]) => void): this; prependOnceListener(event: "close", listener: (code: number | null, signal: NodeJS.Signals | null) => void): this; prependOnceListener(event: "disconnect", listener: () => void): this; prependOnceListener(event: "error", listener: (err: Error) => void): this; prependOnceListener(event: "exit", listener: (code: number | null, signal: NodeJS.Signals | null) => void): this; prependOnceListener(event: "message", listener: (message: Serializable, sendHandle: SendHandle) => void): this; } // return this object when stdio option is undefined or not specified interface ChildProcessWithoutNullStreams extends ChildProcess { stdin: Writable; stdout: Readable; stderr: Readable; readonly stdio: [ Writable, // stdin Readable, // stdout Readable, // stderr Readable | Writable | null | undefined, // extra, no modification Readable | Writable | null | undefined // extra, no modification ]; } // return this object when stdio option is a tuple of 3 interface ChildProcessByStdio< I extends null | Writable, O extends null | Readable, E extends null | Readable, > extends ChildProcess { stdin: I; stdout: O; stderr: E; readonly stdio: [ I, O, E, Readable | Writable | null | undefined, // extra, no modification Readable | Writable | null | undefined // extra, no modification ]; } interface MessageOptions { keepOpen?: boolean; } type StdioOptions = "pipe" | "ignore" | "inherit" | Array<("pipe" | "ipc" | "ignore" | "inherit" | Stream | number | null | undefined)>; type SerializationType = 'json' | 'advanced'; interface MessagingOptions { /** * Specify the kind of serialization used for sending messages between processes. * @default 'json' */ serialization?: SerializationType; } interface ProcessEnvOptions { uid?: number; gid?: number; cwd?: string; env?: NodeJS.ProcessEnv; } interface CommonOptions extends ProcessEnvOptions { /** * @default true */ windowsHide?: boolean; /** * @default 0 */ timeout?: number; } interface CommonSpawnOptions extends CommonOptions, MessagingOptions { argv0?: string; stdio?: StdioOptions; shell?: boolean | string; windowsVerbatimArguments?: boolean; } interface SpawnOptions extends CommonSpawnOptions { detached?: boolean; } interface SpawnOptionsWithoutStdio extends SpawnOptions { stdio?: 'pipe' | Array<null | undefined | 'pipe'>; } type StdioNull = 'inherit' | 'ignore' | Stream; type StdioPipe = undefined | null | 'pipe'; interface SpawnOptionsWithStdioTuple< Stdin extends StdioNull | StdioPipe, Stdout extends StdioNull | StdioPipe, Stderr extends StdioNull | StdioPipe, > extends SpawnOptions { stdio: [Stdin, Stdout, Stderr]; } // overloads of spawn without 'args' function spawn(command: string, options?: SpawnOptionsWithoutStdio): ChildProcessWithoutNullStreams; function spawn( command: string, options: SpawnOptionsWithStdioTuple<StdioPipe, StdioPipe, StdioPipe>, ): ChildProcessByStdio<Writable, Readable, Readable>; function spawn( command: string, options: SpawnOptionsWithStdioTuple<StdioPipe, StdioPipe, StdioNull>, ): ChildProcessByStdio<Writable, Readable, null>; function spawn( command: string, options: SpawnOptionsWithStdioTuple<StdioPipe, StdioNull, StdioPipe>, ): ChildProcessByStdio<Writable, null, Readable>; function spawn( command: string, options: SpawnOptionsWithStdioTuple<StdioNull, StdioPipe, StdioPipe>, ): ChildProcessByStdio<null, Readable, Readable>; function spawn( command: string, options: SpawnOptionsWithStdioTuple<StdioPipe, StdioNull, StdioNull>, ): ChildProcessByStdio<Writable, null, null>; function spawn( command: string, options: SpawnOptionsWithStdioTuple<StdioNull, StdioPipe, StdioNull>, ): ChildProcessByStdio<null, Readable, null>; function spawn( command: string, options: SpawnOptionsWithStdioTuple<StdioNull, StdioNull, StdioPipe>, ): ChildProcessByStdio<null, null, Readable>; function spawn( command: string, options: SpawnOptionsWithStdioTuple<StdioNull, StdioNull, StdioNull>, ): ChildProcessByStdio<null, null, null>; function spawn(command: string, options: SpawnOptions): ChildProcess; // overloads of spawn with 'args' function spawn(command: string, args?: ReadonlyArray<string>, options?: SpawnOptionsWithoutStdio): ChildProcessWithoutNullStreams; function spawn( command: string, args: ReadonlyArray<string>, options: SpawnOptionsWithStdioTuple<StdioPipe, StdioPipe, StdioPipe>, ): ChildProcessByStdio<Writable, Readable, Readable>; function spawn( command: string, args: ReadonlyArray<string>, options: SpawnOptionsWithStdioTuple<StdioPipe, StdioPipe, StdioNull>, ): ChildProcessByStdio<Writable, Readable, null>; function spawn( command: string, args: ReadonlyArray<string>, options: SpawnOptionsWithStdioTuple<StdioPipe, StdioNull, StdioPipe>, ): ChildProcessByStdio<Writable, null, Readable>; function spawn( command: string, args: ReadonlyArray<string>, options: SpawnOptionsWithStdioTuple<StdioNull, StdioPipe, StdioPipe>, ): ChildProcessByStdio<null, Readable, Readable>; function spawn( command: string, args: ReadonlyArray<string>, options: SpawnOptionsWithStdioTuple<StdioPipe, StdioNull, StdioNull>, ): ChildProcessByStdio<Writable, null, null>; function spawn( command: string, args: ReadonlyArray<string>, options: SpawnOptionsWithStdioTuple<StdioNull, StdioPipe, StdioNull>, ): ChildProcessByStdio<null, Readable, null>; function spawn( command: string, args: ReadonlyArray<string>, options: SpawnOptionsWithStdioTuple<StdioNull, StdioNull, StdioPipe>, ): ChildProcessByStdio<null, null, Readable>; function spawn( command: string, args: ReadonlyArray<string>, options: SpawnOptionsWithStdioTuple<StdioNull, StdioNull, StdioNull>, ): ChildProcessByStdio<null, null, null>; function spawn(command: string, args: ReadonlyArray<string>, options: SpawnOptions): ChildProcess; interface ExecOptions extends CommonOptions { shell?: string; maxBuffer?: number; killSignal?: NodeJS.Signals | number; } interface ExecOptionsWithStringEncoding extends ExecOptions { encoding: BufferEncoding; } interface ExecOptionsWithBufferEncoding extends ExecOptions { encoding: BufferEncoding | null; // specify `null`. } interface ExecException extends Error { cmd?: string; killed?: boolean; code?: number; signal?: NodeJS.Signals; } // no `options` definitely means stdout/stderr are `string`. function exec(command: string, callback?: (error: ExecException | null, stdout: string, stderr: string) => void): ChildProcess; // `options` with `"buffer"` or `null` for `encoding` means stdout/stderr are definitely `Buffer`. function exec(command: string, options: { encoding: "buffer" | null } & ExecOptions, callback?: (error: ExecException | null, stdout: Buffer, stderr: Buffer) => void): ChildProcess; // `options` with well known `encoding` means stdout/stderr are definitely `string`. function exec(command: string, options: { encoding: BufferEncoding } & ExecOptions, callback?: (error: ExecException | null, stdout: string, stderr: string) => void): ChildProcess; // `options` with an `encoding` whose type is `string` means stdout/stderr could either be `Buffer` or `string`. // There is no guarantee the `encoding` is unknown as `string` is a superset of `BufferEncoding`. function exec( command: string, options: { encoding: BufferEncoding } & ExecOptions, callback?: (error: ExecException | null, stdout: string | Buffer, stderr: string | Buffer) => void, ): ChildProcess; // `options` without an `encoding` means stdout/stderr are definitely `string`. function exec(command: string, options: ExecOptions, callback?: (error: ExecException | null, stdout: string, stderr: string) => void): ChildProcess; // fallback if nothing else matches. Worst case is always `string | Buffer`. function exec( command: string, options: (BaseEncodingOptions & ExecOptions) | undefined | null, callback?: (error: ExecException | null, stdout: string | Buffer, stderr: string | Buffer) => void, ): ChildProcess; interface PromiseWithChild<T> extends Promise<T> { child: ChildProcess; } // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. namespace exec { function __promisify__(command: string): PromiseWithChild<{ stdout: string, stderr: string }>; function __promisify__(command: string, options: { encoding: "buffer" | null } & ExecOptions): PromiseWithChild<{ stdout: Buffer, stderr: Buffer }>; function __promisify__(command: string, options: { encoding: BufferEncoding } & ExecOptions): PromiseWithChild<{ stdout: string, stderr: string }>; function __promisify__(command: string, options: ExecOptions): PromiseWithChild<{ stdout: string, stderr: string }>; function __promisify__(command: string, options?: (BaseEncodingOptions & ExecOptions) | null): PromiseWithChild<{ stdout: string | Buffer, stderr: string | Buffer }>; } interface ExecFileOptions extends CommonOptions { maxBuffer?: number; killSignal?: NodeJS.Signals | number; windowsVerbatimArguments?: boolean; shell?: boolean | string; } interface ExecFileOptionsWithStringEncoding extends ExecFileOptions { encoding: BufferEncoding; } interface ExecFileOptionsWithBufferEncoding extends ExecFileOptions { encoding: 'buffer' | null; } interface ExecFileOptionsWithOtherEncoding extends ExecFileOptions { encoding: BufferEncoding; } function execFile(file: string): ChildProcess; function execFile(file: string, options: (BaseEncodingOptions & ExecFileOptions) | undefined | null): ChildProcess; function execFile(file: string, args?: ReadonlyArray<string> | null): ChildProcess; function execFile(file: string, args: ReadonlyArray<string> | undefined | null, options: (BaseEncodingOptions & ExecFileOptions) | undefined | null): ChildProcess; // no `options` definitely means stdout/stderr are `string`. function execFile(file: string, callback: (error: ExecException | null, stdout: string, stderr: string) => void): ChildProcess; function execFile(file: string, args: ReadonlyArray<string> | undefined | null, callback: (error: ExecException | null, stdout: string, stderr: string) => void): ChildProcess; // `options` with `"buffer"` or `null` for `encoding` means stdout/stderr are definitely `Buffer`. function execFile(file: string, options: ExecFileOptionsWithBufferEncoding, callback: (error: ExecException | null, stdout: Buffer, stderr: Buffer) => void): ChildProcess; function execFile( file: string, args: ReadonlyArray<string> | undefined | null, options: ExecFileOptionsWithBufferEncoding, callback: (error: ExecException | null, stdout: Buffer, stderr: Buffer) => void, ): ChildProcess; // `options` with well known `encoding` means stdout/stderr are definitely `string`. function execFile(file: string, options: ExecFileOptionsWithStringEncoding, callback: (error: ExecException | null, stdout: string, stderr: string) => void): ChildProcess; function execFile( file: string, args: ReadonlyArray<string> | undefined | null, options: ExecFileOptionsWithStringEncoding, callback: (error: ExecException | null, stdout: string, stderr: string) => void, ): ChildProcess; // `options` with an `encoding` whose type is `string` means stdout/stderr could either be `Buffer` or `string`. // There is no guarantee the `encoding` is unknown as `string` is a superset of `BufferEncoding`. function execFile( file: string, options: ExecFileOptionsWithOtherEncoding, callback: (error: ExecException | null, stdout: string | Buffer, stderr: string | Buffer) => void, ): ChildProcess; function execFile( file: string, args: ReadonlyArray<string> | undefined | null, options: ExecFileOptionsWithOtherEncoding, callback: (error: ExecException | null, stdout: string | Buffer, stderr: string | Buffer) => void, ): ChildProcess; // `options` without an `encoding` means stdout/stderr are definitely `string`. function execFile(file: string, options: ExecFileOptions, callback: (error: ExecException | null, stdout: string, stderr: string) => void): ChildProcess; function execFile( file: string, args: ReadonlyArray<string> | undefined | null, options: ExecFileOptions, callback: (error: ExecException | null, stdout: string, stderr: string) => void ): ChildProcess; // fallback if nothing else matches. Worst case is always `string | Buffer`. function execFile( file: string, options: (BaseEncodingOptions & ExecFileOptions) | undefined | null, callback: ((error: ExecException | null, stdout: string | Buffer, stderr: string | Buffer) => void) | undefined | null, ): ChildProcess; function execFile( file: string, args: ReadonlyArray<string> | undefined | null, options: (BaseEncodingOptions & ExecFileOptions) | undefined | null, callback: ((error: ExecException | null, stdout: string | Buffer, stderr: string | Buffer) => void) | undefined | null, ): ChildProcess; // NOTE: This namespace provides design-time support for util.promisify. Exported members do not exist at runtime. namespace execFile { function __promisify__(file: string): PromiseWithChild<{ stdout: string, stderr: string }>; function __promisify__(file: string, args: ReadonlyArray<string> | undefined | null): PromiseWithChild<{ stdout: string, stderr: string }>; function __promisify__(file: string, options: ExecFileOptionsWithBufferEncoding): PromiseWithChild<{ stdout: Buffer, stderr: Buffer }>; function __promisify__(file: string, args: ReadonlyArray<string> | undefined | null, options: ExecFileOptionsWithBufferEncoding): PromiseWithChild<{ stdout: Buffer, stderr: Buffer }>; function __promisify__(file: string, options: ExecFileOptionsWithStringEncoding): PromiseWithChild<{ stdout: string, stderr: string }>; function __promisify__(file: string, args: ReadonlyArray<string> | undefined | null, options: ExecFileOptionsWithStringEncoding): PromiseWithChild<{ stdout: string, stderr: string }>; function __promisify__(file: string, options: ExecFileOptionsWithOtherEncoding): PromiseWithChild<{ stdout: string | Buffer, stderr: string | Buffer }>; function __promisify__( file: string, args: ReadonlyArray<string> | undefined | null, options: ExecFileOptionsWithOtherEncoding, ): PromiseWithChild<{ stdout: string | Buffer, stderr: string | Buffer }>; function __promisify__(file: string, options: ExecFileOptions): PromiseWithChild<{ stdout: string, stderr: string }>; function __promisify__(file: string, args: ReadonlyArray<string> | undefined | null, options: ExecFileOptions): PromiseWithChild<{ stdout: string, stderr: string }>; function __promisify__(file: string, options: (BaseEncodingOptions & ExecFileOptions) | undefined | null): PromiseWithChild<{ stdout: string | Buffer, stderr: string | Buffer }>; function __promisify__( file: string, args: ReadonlyArray<string> | undefined | null, options: (BaseEncodingOptions & ExecFileOptions) | undefined | null, ): PromiseWithChild<{ stdout: string | Buffer, stderr: string | Buffer }>; } interface ForkOptions extends ProcessEnvOptions, MessagingOptions { execPath?: string; execArgv?: string[]; silent?: boolean; stdio?: StdioOptions; detached?: boolean; windowsVerbatimArguments?: boolean; } function fork(modulePath: string, options?: ForkOptions): ChildProcess; function fork(modulePath: string, args?: ReadonlyArray<string>, options?: ForkOptions): ChildProcess; interface SpawnSyncOptions extends CommonSpawnOptions { input?: string | NodeJS.ArrayBufferView; killSignal?: NodeJS.Signals | number; maxBuffer?: number; encoding?: BufferEncoding | 'buffer' | null; } interface SpawnSyncOptionsWithStringEncoding extends SpawnSyncOptions { encoding: BufferEncoding; } interface SpawnSyncOptionsWithBufferEncoding extends SpawnSyncOptions { encoding?: 'buffer' | null; } interface SpawnSyncReturns<T> { pid: number; output: string[]; stdout: T; stderr: T; status: number | null; signal: NodeJS.Signals | null; error?: Error; } function spawnSync(command: string): SpawnSyncReturns<Buffer>; function spawnSync(command: string, options?: SpawnSyncOptionsWithStringEncoding): SpawnSyncReturns<string>; function spawnSync(command: string, options?: SpawnSyncOptionsWithBufferEncoding): SpawnSyncReturns<Buffer>; function spawnSync(command: string, options?: SpawnSyncOptions): SpawnSyncReturns<Buffer>; function spawnSync(command: string, args?: ReadonlyArray<string>, options?: SpawnSyncOptionsWithStringEncoding): SpawnSyncReturns<string>; function spawnSync(command: string, args?: ReadonlyArray<string>, options?: SpawnSyncOptionsWithBufferEncoding): SpawnSyncReturns<Buffer>; function spawnSync(command: string, args?: ReadonlyArray<string>, options?: SpawnSyncOptions): SpawnSyncReturns<Buffer>; interface ExecSyncOptions extends CommonOptions { input?: string | Uint8Array; stdio?: StdioOptions; shell?: string; killSignal?: NodeJS.Signals | number; maxBuffer?: number; encoding?: BufferEncoding | 'buffer' | null; } interface ExecSyncOptionsWithStringEncoding extends ExecSyncOptions { encoding: BufferEncoding; } interface ExecSyncOptionsWithBufferEncoding extends ExecSyncOptions { encoding?: 'buffer' | null; } function execSync(command: string): Buffer; function execSync(command: string, options?: ExecSyncOptionsWithStringEncoding): string; function execSync(command: string, options?: ExecSyncOptionsWithBufferEncoding): Buffer; function execSync(command: string, options?: ExecSyncOptions): Buffer; interface ExecFileSyncOptions extends CommonOptions { input?: string | NodeJS.ArrayBufferView; stdio?: StdioOptions; killSignal?: NodeJS.Signals | number; maxBuffer?: number; encoding?: BufferEncoding; shell?: boolean | string; } interface ExecFileSyncOptionsWithStringEncoding extends ExecFileSyncOptions { encoding: BufferEncoding; } interface ExecFileSyncOptionsWithBufferEncoding extends ExecFileSyncOptions { encoding: BufferEncoding; // specify `null`. } function execFileSync(command: string): Buffer; function execFileSync(command: string, options?: ExecFileSyncOptionsWithStringEncoding): string; function execFileSync(command: string, options?: ExecFileSyncOptionsWithBufferEncoding): Buffer; function execFileSync(command: string, options?: ExecFileSyncOptions): Buffer; function execFileSync(command: string, args?: ReadonlyArray<string>, options?: ExecFileSyncOptionsWithStringEncoding): string; function execFileSync(command: string, args?: ReadonlyArray<string>, options?: ExecFileSyncOptionsWithBufferEncoding): Buffer; function execFileSync(command: string, args?: ReadonlyArray<string>, options?: ExecFileSyncOptions): Buffer; } apollo-server-demo/node_modules/async-retry/ 0000755 0001750 0000144 00000000000 14067647700 020722 5 ustar andreh users apollo-server-demo/node_modules/async-retry/LICENSE.md 0000644 0001750 0000144 00000002053 03560116604 022314 0 ustar andreh users MIT License Copyright (c) 2017 ZEIT, Inc. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/async-retry/README.md 0000644 0001750 0000144 00000003650 03560116604 022173 0 ustar andreh users # async-retry [](https://github.com/airbnb/javascript) [](https://spectrum.chat/zeit) Retrying made simple, easy, and async. ## Usage ```js // Packages const retry = require('async-retry') const fetch = require('node-fetch') await retry(async bail => { // if anything throws, we retry const res = await fetch('https://google.com') if (403 === res.status) { // don't retry upon 403 bail(new Error('Unauthorized')) return } const data = await res.text() return data.substr(0, 500) }, { retries: 5 }) ``` ### API ```js retry(retrier : Function, opts : Object) => Promise ``` - The supplied function can be `async` or not. In other words, it can be a function that returns a `Promise` or a value. - The supplied function receives two parameters 1. A `Function` you can invoke to abort the retrying (bail) 2. A `Number` identifying the attempt. The absolute first attempt (before any retries) is `1`. - The `opts` are passed to `node-retry`. Read [its docs](https://github.com/tim-kos/node-retry) * `retries`: The maximum amount of times to retry the operation. Default is `10`. * `factor`: The exponential factor to use. Default is `2`. * `minTimeout`: The number of milliseconds before starting the first retry. Default is `1000`. * `maxTimeout`: The maximum number of milliseconds between two retries. Default is `Infinity`. * `randomize`: Randomizes the timeouts by multiplying with a factor between `1` to `2`. Default is `true`. * `onRetry`: an optional `Function` that is invoked after a new retry is performed. It's passed the `Error` that triggered it as a parameter. ## Authors - Guillermo Rauch ([@rauchg](https://twitter.com/rauchg)) - [ZEIT](https://zeit.co) - Leo Lamprecht ([@notquiteleo](https://twitter.com/notquiteleo)) - [ZEIT](https://zeit.co) apollo-server-demo/node_modules/async-retry/package.json 0000644 0001750 0000144 00000002553 03560116604 023203 0 ustar andreh users { "name": "async-retry", "version": "1.3.1", "description": "Retrying made simple, easy and async", "main": "./lib/index.js", "scripts": { "test": "yarn run test-lint && yarn run test-unit", "test-lint": "eslint .", "test-unit": "ava", "lint:staged": "lint-staged" }, "files": [ "lib" ], "license": "MIT", "repository": "zeit/async-retry", "ava": { "failFast": true }, "dependencies": { "retry": "0.12.0" }, "pre-commit": "lint:staged", "lint-staged": { "*.js": [ "eslint", "prettier --write --single-quote", "git add" ] }, "eslintConfig": { "extends": [ "airbnb", "prettier" ], "rules": { "no-var": 0, "prefer-arrow-callback": 0 } }, "devDependencies": { "ava": "0.25.0", "eslint": "5.5.0", "eslint-config-airbnb": "17.1.0", "eslint-config-prettier": "3.0.1", "eslint-plugin-import": "2.14.0", "eslint-plugin-jsx-a11y": "6.1.1", "eslint-plugin-react": "7.11.1", "lint-staged": "7.2.2", "node-fetch": "2.2.0", "pre-commit": "1.2.2", "prettier": "1.14.2", "then-sleep": "1.0.1" } ,"_resolved": "https://registry.npmjs.org/async-retry/-/async-retry-1.3.1.tgz" ,"_integrity": "sha512-aiieFW/7h3hY0Bq5d+ktDBejxuwR78vRu9hDUdR8rNhSaQ29VzPL4AoIRG7D/c7tdenwOcKvgPM6tIxB3cB6HA==" ,"_from": "async-retry@1.3.1" } apollo-server-demo/node_modules/async-retry/lib/ 0000755 0001750 0000144 00000000000 14067647700 021470 5 ustar andreh users apollo-server-demo/node_modules/async-retry/lib/index.js 0000644 0001750 0000144 00000002221 03560116604 023120 0 ustar andreh users // Packages var retrier = require('retry'); function retry(fn, opts) { function run(resolve, reject) { var options = opts || {}; // Default `randomize` to true if (!('randomize' in options)) { options.randomize = true; } var op = retrier.operation(options); // We allow the user to abort retrying // this makes sense in the cases where // knowledge is obtained that retrying // would be futile (e.g.: auth errors) function bail(err) { reject(err || new Error('Aborted')); } function onError(err, num) { if (err.bail) { bail(err); return; } if (!op.retry(err)) { reject(op.mainError()); } else if (options.onRetry) { options.onRetry(err, num); } } function runAttempt(num) { var val; try { val = fn(bail, num); } catch (err) { onError(err, num); return; } Promise.resolve(val) .then(resolve) .catch(function catchIt(err) { onError(err, num); }); } op.attempt(runAttempt); } return new Promise(run); } module.exports = retry; apollo-server-demo/node_modules/has-symbols/ 0000755 0001750 0000144 00000000000 14067647700 020703 5 ustar andreh users apollo-server-demo/node_modules/has-symbols/index.js 0000644 0001750 0000144 00000000612 03560116604 022335 0 ustar andreh users 'use strict'; var origSymbol = global.Symbol; var hasSymbolSham = require('./shams'); module.exports = function hasNativeSymbols() { if (typeof origSymbol !== 'function') { return false; } if (typeof Symbol !== 'function') { return false; } if (typeof origSymbol('foo') !== 'symbol') { return false; } if (typeof Symbol('bar') !== 'symbol') { return false; } return hasSymbolSham(); }; apollo-server-demo/node_modules/has-symbols/LICENSE 0000644 0001750 0000144 00000002057 03560116604 021702 0 ustar andreh users MIT License Copyright (c) 2016 Jordan Harband Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/has-symbols/.eslintrc 0000644 0001750 0000144 00000000244 03560116604 022515 0 ustar andreh users { "root": true, "extends": "@ljharb", "rules": { "max-statements-per-line": [2, { "max": 2 }], "no-magic-numbers": 0, "multiline-comment-style": 0, } } apollo-server-demo/node_modules/has-symbols/.github/ 0000755 0001750 0000144 00000000000 14067647700 022243 5 ustar andreh users apollo-server-demo/node_modules/has-symbols/.github/FUNDING.yml 0000644 0001750 0000144 00000001106 03560116604 024044 0 ustar andreh users # These are supported funding model platforms github: [ljharb] patreon: # Replace with a single Patreon username open_collective: # Replace with a single Open Collective username ko_fi: # Replace with a single Ko-fi username tidelift: npm/has-symbols community_bridge: # Replace with a single Community Bridge project-name e.g., cloud-foundry liberapay: # Replace with a single Liberapay username issuehunt: # Replace with a single IssueHunt username otechie: # Replace with a single Otechie username custom: # Replace with up to 4 custom sponsorship URLs e.g., ['link1', 'link2'] apollo-server-demo/node_modules/has-symbols/.github/workflows/ 0000755 0001750 0000144 00000000000 14067647700 024300 5 ustar andreh users apollo-server-demo/node_modules/has-symbols/.github/workflows/rebase.yml 0000644 0001750 0000144 00000000372 03560116604 026254 0 ustar andreh users name: Automatic Rebase on: [pull_request] jobs: _: name: "Automatic Rebase" runs-on: ubuntu-latest steps: - uses: actions/checkout@v1 - uses: ljharb/rebase@master env: GITHUB_TOKEN: ${{ secrets.GITHUB_TOKEN }} apollo-server-demo/node_modules/has-symbols/README.md 0000644 0001750 0000144 00000003412 03560116604 022150 0 ustar andreh users # has-symbols <sup>[![Version Badge][2]][1]</sup> [![Build Status][3]][4] [![dependency status][5]][6] [![dev dependency status][7]][8] [![License][license-image]][license-url] [![Downloads][downloads-image]][downloads-url] [![npm badge][11]][1] Determine if the JS environment has Symbol support. Supports spec, or shams. ## Example ```js var hasSymbols = require('has-symbols'); hasSymbols() === true; // if the environment has native Symbol support. Not polyfillable, not forgeable. var hasSymbolsKinda = require('has-symbols/shams'); hasSymbolsKinda() === true; // if the environment has a Symbol sham that mostly follows the spec. ``` ## Supported Symbol shams - get-own-property-symbols [npm](https://www.npmjs.com/package/get-own-property-symbols) | [github](https://github.com/WebReflection/get-own-property-symbols) - core-js [npm](https://www.npmjs.com/package/core-js) | [github](https://github.com/zloirock/core-js) ## Tests Simply clone the repo, `npm install`, and run `npm test` [1]: https://npmjs.org/package/has-symbols [2]: http://versionbadg.es/ljharb/has-symbols.svg [3]: https://travis-ci.org/ljharb/has-symbols.svg [4]: https://travis-ci.org/ljharb/has-symbols [5]: https://david-dm.org/ljharb/has-symbols.svg [6]: https://david-dm.org/ljharb/has-symbols [7]: https://david-dm.org/ljharb/has-symbols/dev-status.svg [8]: https://david-dm.org/ljharb/has-symbols#info=devDependencies [9]: https://ci.testling.com/ljharb/has-symbols.png [10]: https://ci.testling.com/ljharb/has-symbols [11]: https://nodei.co/npm/has-symbols.png?downloads=true&stars=true [license-image]: http://img.shields.io/npm/l/has-symbols.svg [license-url]: LICENSE [downloads-image]: http://img.shields.io/npm/dm/has-symbols.svg [downloads-url]: http://npm-stat.com/charts.html?package=has-symbols apollo-server-demo/node_modules/has-symbols/package.json 0000644 0001750 0000144 00000005024 03560116604 023160 0 ustar andreh users { "name": "has-symbols", "version": "1.0.1", "author": { "name": "Jordan Harband", "email": "ljharb@gmail.com", "url": "http://ljharb.codes" }, "funding": { "url": "https://github.com/sponsors/ljharb" }, "contributors": [ { "name": "Jordan Harband", "email": "ljharb@gmail.com", "url": "http://ljharb.codes" } ], "description": "Determine if the JS environment has Symbol support. Supports spec, or shams.", "license": "MIT", "main": "index.js", "scripts": { "prepublish": "safe-publish-latest", "pretest": "npm run --silent lint", "test": "npm run --silent tests-only", "posttest": "npx aud", "tests-only": "npm run --silent test:stock && npm run --silent test:staging && npm run --silent test:shams", "test:stock": "node test", "test:staging": "node --harmony --es-staging test", "test:shams": "npm run --silent test:shams:getownpropertysymbols && npm run --silent test:shams:corejs", "test:shams:corejs": "node test/shams/core-js.js", "test:shams:getownpropertysymbols": "node test/shams/get-own-property-symbols.js", "lint": "eslint *.js", "version": "auto-changelog && git add CHANGELOG.md", "postversion": "auto-changelog && git add CHANGELOG.md && git commit --no-edit --amend && git tag -f \"v$(node -e \"console.log(require('./package.json').version)\")\"" }, "repository": { "type": "git", "url": "git://github.com/ljharb/has-symbols.git" }, "keywords": [ "Symbol", "symbols", "typeof", "sham", "polyfill", "native", "core-js", "ES6" ], "dependencies": {}, "devDependencies": { "@ljharb/eslint-config": "^15.0.1", "auto-changelog": "^1.16.2", "core-js": "^2.6.10", "eslint": "^6.6.0", "get-own-property-symbols": "^0.9.4", "safe-publish-latest": "^1.1.4", "tape": "^4.11.0" }, "testling": { "files": "test/index.js", "browsers": [ "iexplore/6.0..latest", "firefox/3.0..6.0", "firefox/15.0..latest", "firefox/nightly", "chrome/4.0..10.0", "chrome/20.0..latest", "chrome/canary", "opera/10.0..latest", "opera/next", "safari/4.0..latest", "ipad/6.0..latest", "iphone/6.0..latest", "android-browser/4.2" ] }, "engines": { "node": ">= 0.4" }, "auto-changelog": { "output": "CHANGELOG.md", "template": "keepachangelog", "unreleased": false, "commitLimit": false, "backfillLimit": false } ,"_resolved": "https://registry.npmjs.org/has-symbols/-/has-symbols-1.0.1.tgz" ,"_integrity": "sha512-PLcsoqu++dmEIZB+6totNFKq/7Do+Z0u4oT0zKOJNl3lYK6vGwwu2hjHs+68OEZbTjiUE9bgOABXbP/GvrS0Kg==" ,"_from": "has-symbols@1.0.1" } apollo-server-demo/node_modules/has-symbols/test/ 0000755 0001750 0000144 00000000000 14067647700 021662 5 ustar andreh users apollo-server-demo/node_modules/has-symbols/test/index.js 0000644 0001750 0000144 00000001217 03560116604 023316 0 ustar andreh users 'use strict'; var test = require('tape'); var hasSymbols = require('../'); var runSymbolTests = require('./tests'); test('interface', function (t) { t.equal(typeof hasSymbols, 'function', 'is a function'); t.equal(typeof hasSymbols(), 'boolean', 'returns a boolean'); t.end(); }); test('Symbols are supported', { skip: !hasSymbols() }, function (t) { runSymbolTests(t); t.end(); }); test('Symbols are not supported', { skip: hasSymbols() }, function (t) { t.equal(typeof Symbol, 'undefined', 'global Symbol is undefined'); t.equal(typeof Object.getOwnPropertySymbols, 'undefined', 'Object.getOwnPropertySymbols does not exist'); t.end(); }); apollo-server-demo/node_modules/has-symbols/test/tests.js 0000644 0001750 0000144 00000003605 03560116604 023354 0 ustar andreh users 'use strict'; module.exports = function runSymbolTests(t) { t.equal(typeof Symbol, 'function', 'global Symbol is a function'); if (typeof Symbol !== 'function') { return false }; t.notEqual(Symbol(), Symbol(), 'two symbols are not equal'); /* t.equal( Symbol.prototype.toString.call(Symbol('foo')), Symbol.prototype.toString.call(Symbol('foo')), 'two symbols with the same description stringify the same' ); */ var foo = Symbol('foo'); /* t.notEqual( String(foo), String(Symbol('bar')), 'two symbols with different descriptions do not stringify the same' ); */ t.equal(typeof Symbol.prototype.toString, 'function', 'Symbol#toString is a function'); // t.equal(String(foo), Symbol.prototype.toString.call(foo), 'Symbol#toString equals String of the same symbol'); t.equal(typeof Object.getOwnPropertySymbols, 'function', 'Object.getOwnPropertySymbols is a function'); var obj = {}; var sym = Symbol('test'); var symObj = Object(sym); t.notEqual(typeof sym, 'string', 'Symbol is not a string'); t.equal(Object.prototype.toString.call(sym), '[object Symbol]', 'symbol primitive Object#toStrings properly'); t.equal(Object.prototype.toString.call(symObj), '[object Symbol]', 'symbol primitive Object#toStrings properly'); var symVal = 42; obj[sym] = symVal; for (sym in obj) { t.fail('symbol property key was found in for..in of object'); } t.deepEqual(Object.keys(obj), [], 'no enumerable own keys on symbol-valued object'); t.deepEqual(Object.getOwnPropertyNames(obj), [], 'no own names on symbol-valued object'); t.deepEqual(Object.getOwnPropertySymbols(obj), [sym], 'one own symbol on symbol-valued object'); t.equal(Object.prototype.propertyIsEnumerable.call(obj, sym), true, 'symbol is enumerable'); t.deepEqual(Object.getOwnPropertyDescriptor(obj, sym), { configurable: true, enumerable: true, value: 42, writable: true }, 'property descriptor is correct'); }; apollo-server-demo/node_modules/has-symbols/test/shams/ 0000755 0001750 0000144 00000000000 14067647700 022775 5 ustar andreh users apollo-server-demo/node_modules/has-symbols/test/shams/core-js.js 0000644 0001750 0000144 00000001323 03560116604 024662 0 ustar andreh users 'use strict'; var test = require('tape'); if (typeof Symbol === 'function' && typeof Symbol() === 'symbol') { test('has native Symbol support', function (t) { t.equal(typeof Symbol, 'function'); t.equal(typeof Symbol(), 'symbol'); t.end(); }); return; } var hasSymbols = require('../../shams'); test('polyfilled Symbols', function (t) { /* eslint-disable global-require */ t.equal(hasSymbols(), false, 'hasSymbols is false before polyfilling'); require('core-js/fn/symbol'); require('core-js/fn/symbol/to-string-tag'); require('../tests')(t); var hasSymbolsAfter = hasSymbols(); t.equal(hasSymbolsAfter, true, 'hasSymbols is true after polyfilling'); /* eslint-enable global-require */ t.end(); }); apollo-server-demo/node_modules/has-symbols/test/shams/get-own-property-symbols.js 0000644 0001750 0000144 00000001256 03560116604 030255 0 ustar andreh users 'use strict'; var test = require('tape'); if (typeof Symbol === 'function' && typeof Symbol() === 'symbol') { test('has native Symbol support', function (t) { t.equal(typeof Symbol, 'function'); t.equal(typeof Symbol(), 'symbol'); t.end(); }); return; } var hasSymbols = require('../../shams'); test('polyfilled Symbols', function (t) { /* eslint-disable global-require */ t.equal(hasSymbols(), false, 'hasSymbols is false before polyfilling'); require('get-own-property-symbols'); require('../tests')(t); var hasSymbolsAfter = hasSymbols(); t.equal(hasSymbolsAfter, true, 'hasSymbols is true after polyfilling'); /* eslint-enable global-require */ t.end(); }); apollo-server-demo/node_modules/has-symbols/CHANGELOG.md 0000644 0001750 0000144 00000005470 03560116604 022510 0 ustar andreh users # Changelog All notable changes to this project will be documented in this file. The format is based on [Keep a Changelog](https://keepachangelog.com/en/1.0.0/) and this project adheres to [Semantic Versioning](https://semver.org/spec/v2.0.0.html). Generated by [`auto-changelog`](https://github.com/CookPete/auto-changelog). ## [v1.0.1](https://github.com/inspect-js/has-symbols/compare/v1.0.0...v1.0.1) - 2019-11-17 ### Commits - [Tests] use shared travis-ci configs [`ce396c9`](https://github.com/inspect-js/has-symbols/commit/ce396c9419ff11c43d0da5d05cdbb79f7fb42229) - [Tests] up to `node` `v12.4`, `v11.15`, `v10.15`, `v9.11`, `v8.15`, `v7.10`, `v6.17`, `v4.9`; use `nvm install-latest-npm` [`0690732`](https://github.com/inspect-js/has-symbols/commit/0690732801f47ab429f39ba1962f522d5c462d6b) - [meta] add `auto-changelog` [`2163d0b`](https://github.com/inspect-js/has-symbols/commit/2163d0b7f36343076b8f947cd1667dd1750f26fc) - [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `core-js`, `safe-publish-latest`, `tape` [`8e0951f`](https://github.com/inspect-js/has-symbols/commit/8e0951f1a7a2e52068222b7bb73511761e6e4d9c) - [actions] add automatic rebasing / merge commit blocking [`b09cdb7`](https://github.com/inspect-js/has-symbols/commit/b09cdb7cd7ee39e7a769878f56e2d6066f5ccd1d) - [Dev Deps] update `eslint`, `@ljharb/eslint-config`, `safe-publish-latest`, `core-js`, `get-own-property-symbols`, `tape` [`1dd42cd`](https://github.com/inspect-js/has-symbols/commit/1dd42cd86183ed0c50f99b1062345c458babca91) - [meta] create FUNDING.yml [`aa57a17`](https://github.com/inspect-js/has-symbols/commit/aa57a17b19708906d1927f821ea8e73394d84ca4) - Only apps should have lockfiles [`a2d8bea`](https://github.com/inspect-js/has-symbols/commit/a2d8bea23a97d15c09eaf60f5b107fcf9a4d57aa) - [Tests] use `npx aud` instead of `nsp` or `npm audit` with hoops [`9e96cb7`](https://github.com/inspect-js/has-symbols/commit/9e96cb783746cbed0c10ef78e599a8eaa7ebe193) - [meta] add `funding` field [`a0b32cf`](https://github.com/inspect-js/has-symbols/commit/a0b32cf68e803f963c1639b6d47b0a9d6440bab0) - [Dev Deps] update `safe-publish-latest` [`cb9f0a5`](https://github.com/inspect-js/has-symbols/commit/cb9f0a521a3a1790f1064d437edd33bb6c3d6af0) ## v1.0.0 - 2016-09-19 ### Commits - Tests. [`ecb6eb9`](https://github.com/inspect-js/has-symbols/commit/ecb6eb934e4883137f3f93b965ba5e0a98df430d) - package.json [`88a337c`](https://github.com/inspect-js/has-symbols/commit/88a337cee0864a0da35f5d19e69ff0ef0150e46a) - Initial commit [`42e1e55`](https://github.com/inspect-js/has-symbols/commit/42e1e5502536a2b8ac529c9443984acd14836b1c) - Initial implementation. [`33f5cc6`](https://github.com/inspect-js/has-symbols/commit/33f5cc6cdff86e2194b081ee842bfdc63caf43fb) - read me [`01f1170`](https://github.com/inspect-js/has-symbols/commit/01f1170188ff7cb1558aa297f6ba5b516c6d7b0c) apollo-server-demo/node_modules/has-symbols/shams.js 0000644 0001750 0000144 00000003314 03560116604 022343 0 ustar andreh users 'use strict'; /* eslint complexity: [2, 18], max-statements: [2, 33] */ module.exports = function hasSymbols() { if (typeof Symbol !== 'function' || typeof Object.getOwnPropertySymbols !== 'function') { return false; } if (typeof Symbol.iterator === 'symbol') { return true; } var obj = {}; var sym = Symbol('test'); var symObj = Object(sym); if (typeof sym === 'string') { return false; } if (Object.prototype.toString.call(sym) !== '[object Symbol]') { return false; } if (Object.prototype.toString.call(symObj) !== '[object Symbol]') { return false; } // temp disabled per https://github.com/ljharb/object.assign/issues/17 // if (sym instanceof Symbol) { return false; } // temp disabled per https://github.com/WebReflection/get-own-property-symbols/issues/4 // if (!(symObj instanceof Symbol)) { return false; } // if (typeof Symbol.prototype.toString !== 'function') { return false; } // if (String(sym) !== Symbol.prototype.toString.call(sym)) { return false; } var symVal = 42; obj[sym] = symVal; for (sym in obj) { return false; } // eslint-disable-line no-restricted-syntax if (typeof Object.keys === 'function' && Object.keys(obj).length !== 0) { return false; } if (typeof Object.getOwnPropertyNames === 'function' && Object.getOwnPropertyNames(obj).length !== 0) { return false; } var syms = Object.getOwnPropertySymbols(obj); if (syms.length !== 1 || syms[0] !== sym) { return false; } if (!Object.prototype.propertyIsEnumerable.call(obj, sym)) { return false; } if (typeof Object.getOwnPropertyDescriptor === 'function') { var descriptor = Object.getOwnPropertyDescriptor(obj, sym); if (descriptor.value !== symVal || descriptor.enumerable !== true) { return false; } } return true; }; apollo-server-demo/node_modules/has-symbols/.travis.yml 0000644 0001750 0000144 00000000374 03560116604 023006 0 ustar andreh users version: ~> 1.0 language: node_js os: - linux import: - ljharb/travis-ci:node/all.yml - ljharb/travis-ci:node/pretest.yml - ljharb/travis-ci:node/posttest.yml - ljharb/travis-ci:node/coverage.yml matrix: allow_failures: - env: COVERAGE=true apollo-server-demo/node_modules/long/ 0000755 0001750 0000144 00000000000 14067647701 017402 5 ustar andreh users apollo-server-demo/node_modules/long/index.js 0000644 0001750 0000144 00000000051 13235275472 021041 0 ustar andreh users module.exports = require("./src/long"); apollo-server-demo/node_modules/long/dist/ 0000755 0001750 0000144 00000000000 14067647701 020345 5 ustar andreh users apollo-server-demo/node_modules/long/dist/long.js.map 0000644 0001750 0000144 00000315522 13235424637 022423 0 ustar andreh users {"version":3,"sources":["webpack:///webpack/universalModuleDefinition","webpack:///long.js","webpack:///webpack/bootstrap d8921b8c3ad0790b3cc1","webpack:///./src/long.js"],"names":["root","factory","exports","module","define","amd","self","this","modules","__webpack_require__","moduleId","installedModules","i","l","call","m","c","d","name","getter","o","Object","defineProperty","configurable","enumerable","get","n","__esModule","object","property","prototype","hasOwnProperty","p","s","Long","low","high","unsigned","isLong","obj","fromInt","value","cachedObj","cache","UINT_CACHE","fromBits","INT_CACHE","fromNumber","isNaN","UZERO","ZERO","TWO_PWR_64_DBL","MAX_UNSIGNED_VALUE","TWO_PWR_63_DBL","MIN_VALUE","MAX_VALUE","neg","TWO_PWR_32_DBL","lowBits","highBits","fromString","str","radix","length","Error","RangeError","indexOf","substring","radixToPower","pow_dbl","result","size","Math","min","parseInt","power","mul","add","fromValue","val","wasm","WebAssembly","Instance","Module","Uint8Array","e","__isLong__","pow","TWO_PWR_16_DBL","TWO_PWR_24","ONE","UONE","NEG_ONE","LongPrototype","toInt","toNumber","toString","isZero","isNegative","eq","radixLong","div","rem1","sub","rem","remDiv","intval","digits","getHighBits","getHighBitsUnsigned","getLowBits","getLowBitsUnsigned","getNumBitsAbs","bit","eqz","isPositive","isOdd","isEven","equals","other","notEquals","neq","ne","lessThan","comp","lt","lessThanOrEqual","lte","le","greaterThan","gt","greaterThanOrEqual","gte","ge","compare","thisNeg","otherNeg","negate","not","addend","a48","a32","a16","a00","b48","b32","b16","b00","c48","c32","c16","c00","subtract","subtrahend","multiply","multiplier","get_high","divide","divisor","div_u","div_s","approx","res","toUnsigned","shru","shr","shl","max","floor","log2","ceil","log","LN2","delta","approxRes","approxRem","modulo","rem_u","rem_s","mod","and","or","xor","shiftLeft","numBits","shiftRight","shiftRightUnsigned","shr_u","toSigned","toBytes","toBytesLE","toBytesBE","hi","lo","fromBytes","bytes","fromBytesLE","fromBytesBE"],"mappings":"CAAA,SAAAA,EAAAC,GACA,gBAAAC,UAAA,gBAAAC,QACAA,OAAAD,QAAAD,IACA,kBAAAG,gBAAAC,IACAD,UAAAH,GACA,gBAAAC,SACAA,QAAA,KAAAD,IAEAD,EAAA,KAAAC,KACC,mBAAAK,WAAAC,KAAA,WACD,MCAgB,UAAUC,GCN1B,QAAAC,GAAAC,GAGA,GAAAC,EAAAD,GACA,MAAAC,GAAAD,GAAAR,OAGA,IAAAC,GAAAQ,EAAAD,IACAE,EAAAF,EACAG,GAAA,EACAX,WAUA,OANAM,GAAAE,GAAAI,KAAAX,EAAAD,QAAAC,IAAAD,QAAAO,GAGAN,EAAAU,GAAA,EAGAV,EAAAD,QAvBA,GAAAS,KA4DA,OAhCAF,GAAAM,EAAAP,EAGAC,EAAAO,EAAAL,EAGAF,EAAAQ,EAAA,SAAAf,EAAAgB,EAAAC,GACAV,EAAAW,EAAAlB,EAAAgB,IACAG,OAAAC,eAAApB,EAAAgB,GACAK,cAAA,EACAC,YAAA,EACAC,IAAAN,KAMAV,EAAAiB,EAAA,SAAAvB,GACA,GAAAgB,GAAAhB,KAAAwB,WACA,WAA2B,MAAAxB,GAAA,SAC3B,WAAiC,MAAAA,GAEjC,OADAM,GAAAQ,EAAAE,EAAA,IAAAA,GACAA,GAIAV,EAAAW,EAAA,SAAAQ,EAAAC,GAAsD,MAAAR,QAAAS,UAAAC,eAAAjB,KAAAc,EAAAC,IAGtDpB,EAAAuB,EAAA,GAGAvB,IAAAwB,EAAA,KDgBM,SAAU9B,EAAQD,GEpDxB,QAAAgC,GAAAC,EAAAC,EAAAC,GAMA9B,KAAA4B,IAAA,EAAAA,EAMA5B,KAAA6B,KAAA,EAAAA,EAMA7B,KAAA8B,aAoCA,QAAAC,GAAAC,GACA,YAAAA,KAAA,YA+BA,QAAAC,GAAAC,EAAAJ,GACA,GAAAE,GAAAG,EAAAC,CACA,OAAAN,IACAI,KAAA,GACAE,EAAA,GAAAF,KAAA,OACAC,EAAAE,EAAAH,IAEAC,GAEAH,EAAAM,EAAAJ,GAAA,EAAAA,GAAA,WACAE,IACAC,EAAAH,GAAAF,GACAA,KAEAE,GAAA,GACAE,GAAA,KAAAF,KAAA,OACAC,EAAAI,EAAAL,IAEAC,GAEAH,EAAAM,EAAAJ,IAAA,WACAE,IACAG,EAAAL,GAAAF,GACAA,IAmBA,QAAAQ,GAAAN,EAAAJ,GACA,GAAAW,MAAAP,GACA,MAAAJ,GAAAY,EAAAC,CACA,IAAAb,EAAA,CACA,GAAAI,EAAA,EACA,MAAAQ,EACA,IAAAR,GAAAU,EACA,MAAAC,OACK,CACL,GAAAX,IAAAY,EACA,MAAAC,EACA,IAAAb,EAAA,GAAAY,EACA,MAAAE,GAEA,MAAAd,GAAA,EACAM,GAAAN,EAAAJ,GAAAmB,MACAX,EAAAJ,EAAAgB,EAAA,EAAAhB,EAAAgB,EAAA,EAAApB,GAmBA,QAAAQ,GAAAa,EAAAC,EAAAtB,GACA,UAAAH,GAAAwB,EAAAC,EAAAtB,GA8BA,QAAAuB,GAAAC,EAAAxB,EAAAyB,GACA,OAAAD,EAAAE,OACA,KAAAC,OAAA,eACA,YAAAH,GAAA,aAAAA,GAAA,cAAAA,GAAA,cAAAA,EACA,MAAAX,EASA,IARA,gBAAAb,IAEAyB,EAAAzB,EACAA,GAAA,GAEAA,OAEAyB,KAAA,IACA,MAAAA,EACA,KAAAG,YAAA,QAEA,IAAAjC,EACA,KAAAA,EAAA6B,EAAAK,QAAA,QACA,KAAAF,OAAA,kBACA,QAAAhC,EACA,MAAA4B,GAAAC,EAAAM,UAAA,GAAA9B,EAAAyB,GAAAN,KAQA,QAHAY,GAAArB,EAAAsB,EAAAP,EAAA,IAEAQ,EAAApB,EACAtC,EAAA,EAAmBA,EAAAiD,EAAAE,OAAgBnD,GAAA,GACnC,GAAA2D,GAAAC,KAAAC,IAAA,EAAAZ,EAAAE,OAAAnD,GACA6B,EAAAiC,SAAAb,EAAAM,UAAAvD,IAAA2D,GAAAT,EACA,IAAAS,EAAA,GACA,GAAAI,GAAA5B,EAAAsB,EAAAP,EAAAS,GACAD,KAAAM,IAAAD,GAAAE,IAAA9B,EAAAN,QAEA6B,KAAAM,IAAAR,GACAE,IAAAO,IAAA9B,EAAAN,IAIA,MADA6B,GAAAjC,WACAiC,EAoBA,QAAAQ,GAAAC,EAAA1C,GACA,sBAAA0C,GACAhC,EAAAgC,EAAA1C,GACA,gBAAA0C,GACAnB,EAAAmB,EAAA1C,GAEAQ,EAAAkC,EAAA5C,IAAA4C,EAAA3C,KAAA,iBAAAC,KAAA0C,EAAA1C,UA7RAlC,EAAAD,QAAAgC,CAKA,IAAA8C,GAAA,IAEA,KACAA,EAAA,GAAAC,aAAAC,SAAA,GAAAD,aAAAE,OAAA,GAAAC,aACA,u2BACSlF,QACR,MAAAmF,IA0DDnD,EAAAJ,UAAAwD,WAEAjE,OAAAC,eAAAY,EAAAJ,UAAA,cAAqDW,OAAA,IAkBrDP,EAAAI,QAOA,IAAAQ,MAOAF,IA0CAV,GAAAM,UAkCAN,EAAAa,aAsBAb,EAAAW,UASA,IAAAwB,GAAAG,KAAAe,GA4DArD,GAAA0B,aAyBA1B,EAAA4C,WAUA,IAcArB,GAAA+B,WAOArC,EAAAM,IAOAJ,EAAAF,EAAA,EAOAsC,EAAAjD,EA5BA,OAkCAU,EAAAV,EAAA,EAMAN,GAAAgB,MAMA,IAAAD,GAAAT,EAAA,KAMAN,GAAAe,OAMA,IAAAyC,GAAAlD,EAAA,EAMAN,GAAAwD,KAMA,IAAAC,GAAAnD,EAAA,KAMAN,GAAAyD,MAMA,IAAAC,GAAApD,GAAA,EAMAN,GAAA0D,SAMA,IAAArC,GAAAV,GAAA,gBAMAX,GAAAqB,WAMA,IAAAH,GAAAP,GAAA,QAMAX,GAAAkB,oBAMA,IAAAE,GAAAT,EAAA,iBAMAX,GAAAoB,WAMA,IAAAuC,GAAA3D,EAAAJ,SAMA+D,GAAAC,MAAA,WACA,MAAAvF,MAAA8B,SAAA9B,KAAA4B,MAAA,EAAA5B,KAAA4B,KAOA0D,EAAAE,SAAA,WACA,MAAAxF,MAAA8B,UACA9B,KAAA6B,OAAA,GAAAqB,GAAAlD,KAAA4B,MAAA,GACA5B,KAAA6B,KAAAqB,GAAAlD,KAAA4B,MAAA,IAUA0D,EAAAG,SAAA,SAAAlC,GAEA,IADAA,KAAA,IACA,MAAAA,EACA,KAAAG,YAAA,QACA,IAAA1D,KAAA0F,SACA,SACA,IAAA1F,KAAA2F,aAAA,CACA,GAAA3F,KAAA4F,GAAA7C,GAAA,CAGA,GAAA8C,GAAArD,EAAAe,GACAuC,EAAA9F,KAAA8F,IAAAD,GACAE,EAAAD,EAAAzB,IAAAwB,GAAAG,IAAAhG,KACA,OAAA8F,GAAAL,SAAAlC,GAAAwC,EAAAR,QAAAE,SAAAlC,GAEA,UAAAvD,KAAAiD,MAAAwC,SAAAlC,GAQA,IAHA,GAAAM,GAAArB,EAAAsB,EAAAP,EAAA,GAAAvD,KAAA8B,UACAmE,EAAAjG,KACA+D,EAAA,KACA,CACA,GAAAmC,GAAAD,EAAAH,IAAAjC,GACAsC,EAAAF,EAAAD,IAAAE,EAAA7B,IAAAR,IAAA0B,UAAA,EACAa,EAAAD,EAAAV,SAAAlC,EAEA,IADA0C,EAAAC,EACAD,EAAAP,SACA,MAAAU,GAAArC,CAEA,MAAAqC,EAAA5C,OAAA,GACA4C,EAAA,IAAAA,CACArC,GAAA,GAAAqC,EAAArC,IASAuB,EAAAe,YAAA,WACA,MAAArG,MAAA6B,MAOAyD,EAAAgB,oBAAA,WACA,MAAAtG,MAAA6B,OAAA,GAOAyD,EAAAiB,WAAA,WACA,MAAAvG,MAAA4B,KAOA0D,EAAAkB,mBAAA,WACA,MAAAxG,MAAA4B,MAAA,GAOA0D,EAAAmB,cAAA,WACA,GAAAzG,KAAA2F,aACA,MAAA3F,MAAA4F,GAAA7C,GAAA,GAAA/C,KAAAiD,MAAAwD,eAEA,QADAjC,GAAA,GAAAxE,KAAA6B,KAAA7B,KAAA6B,KAAA7B,KAAA4B,IACA8E,EAAA,GAAsBA,EAAA,GACtB,IAAAlC,EAAA,GAAAkC,GAD+BA,KAG/B,UAAA1G,KAAA6B,KAAA6E,EAAA,GAAAA,EAAA,GAOApB,EAAAI,OAAA,WACA,WAAA1F,KAAA6B,MAAA,IAAA7B,KAAA4B,KAOA0D,EAAAqB,IAAArB,EAAAI,OAMAJ,EAAAK,WAAA,WACA,OAAA3F,KAAA8B,UAAA9B,KAAA6B,KAAA,GAOAyD,EAAAsB,WAAA,WACA,MAAA5G,MAAA8B,UAAA9B,KAAA6B,MAAA,GAOAyD,EAAAuB,MAAA,WACA,aAAA7G,KAAA4B,MAOA0D,EAAAwB,OAAA,WACA,aAAA9G,KAAA4B,MAQA0D,EAAAyB,OAAA,SAAAC,GAGA,MAFAjF,GAAAiF,KACAA,EAAAzC,EAAAyC,KACAhH,KAAA8B,WAAAkF,EAAAlF,UAAA9B,KAAA6B,OAAA,OAAAmF,EAAAnF,OAAA,SAEA7B,KAAA6B,OAAAmF,EAAAnF,MAAA7B,KAAA4B,MAAAoF,EAAApF,MASA0D,EAAAM,GAAAN,EAAAyB,OAOAzB,EAAA2B,UAAA,SAAAD,GACA,OAAAhH,KAAA4F,GAAAoB,IASA1B,EAAA4B,IAAA5B,EAAA2B,UAQA3B,EAAA6B,GAAA7B,EAAA2B,UAOA3B,EAAA8B,SAAA,SAAAJ,GACA,MAAAhH,MAAAqH,KAAAL,GAAA,GASA1B,EAAAgC,GAAAhC,EAAA8B,SAOA9B,EAAAiC,gBAAA,SAAAP,GACA,MAAAhH,MAAAqH,KAAAL,IAAA,GASA1B,EAAAkC,IAAAlC,EAAAiC,gBAQAjC,EAAAmC,GAAAnC,EAAAiC,gBAOAjC,EAAAoC,YAAA,SAAAV,GACA,MAAAhH,MAAAqH,KAAAL,GAAA,GASA1B,EAAAqC,GAAArC,EAAAoC,YAOApC,EAAAsC,mBAAA,SAAAZ,GACA,MAAAhH,MAAAqH,KAAAL,IAAA,GASA1B,EAAAuC,IAAAvC,EAAAsC,mBAQAtC,EAAAwC,GAAAxC,EAAAsC,mBAQAtC,EAAAyC,QAAA,SAAAf,GAGA,GAFAjF,EAAAiF,KACAA,EAAAzC,EAAAyC,IACAhH,KAAA4F,GAAAoB,GACA,QACA,IAAAgB,GAAAhI,KAAA2F,aACAsC,EAAAjB,EAAArB,YACA,OAAAqC,KAAAC,GACA,GACAD,GAAAC,EACA,EAEAjI,KAAA8B,SAGAkF,EAAAnF,OAAA,EAAA7B,KAAA6B,OAAA,GAAAmF,EAAAnF,OAAA7B,KAAA6B,MAAAmF,EAAApF,MAAA,EAAA5B,KAAA4B,MAAA,OAFA5B,KAAAgG,IAAAgB,GAAArB,cAAA,KAYAL,EAAA+B,KAAA/B,EAAAyC,QAMAzC,EAAA4C,OAAA,WACA,OAAAlI,KAAA8B,UAAA9B,KAAA4F,GAAA7C,GACAA,EACA/C,KAAAmI,MAAA7D,IAAAa,IAQAG,EAAArC,IAAAqC,EAAA4C,OAOA5C,EAAAhB,IAAA,SAAA8D,GACArG,EAAAqG,KACAA,EAAA7D,EAAA6D,GAIA,IAAAC,GAAArI,KAAA6B,OAAA,GACAyG,EAAA,MAAAtI,KAAA6B,KACA0G,EAAAvI,KAAA4B,MAAA,GACA4G,EAAA,MAAAxI,KAAA4B,IAEA6G,EAAAL,EAAAvG,OAAA,GACA6G,EAAA,MAAAN,EAAAvG,KACA8G,EAAAP,EAAAxG,MAAA,GACAgH,EAAA,MAAAR,EAAAxG,IAEAiH,EAAA,EAAAC,EAAA,EAAAC,EAAA,EAAAC,EAAA,CAYA,OAXAA,IAAAR,EAAAI,EACAG,GAAAC,IAAA,GACAA,GAAA,MACAD,GAAAR,EAAAI,EACAG,GAAAC,IAAA,GACAA,GAAA,MACAD,GAAAR,EAAAI,EACAG,GAAAC,IAAA,GACAA,GAAA,MACAD,GAAAR,EAAAI,EACAI,GAAA,MACAvG,EAAAyG,GAAA,GAAAC,EAAAH,GAAA,GAAAC,EAAA9I,KAAA8B,WAQAwD,EAAA2D,SAAA,SAAAC,GAGA,MAFAnH,GAAAmH,KACAA,EAAA3E,EAAA2E,IACAlJ,KAAAsE,IAAA4E,EAAAjG,QASAqC,EAAAU,IAAAV,EAAA2D,SAOA3D,EAAA6D,SAAA,SAAAC,GACA,GAAApJ,KAAA0F,SACA,MAAA/C,EAKA,IAJAZ,EAAAqH,KACAA,EAAA7E,EAAA6E,IAGA3E,EAAA,CAKA,MAAAnC,GAJAmC,EAAAJ,IAAArE,KAAA4B,IACA5B,KAAA6B,KACAuH,EAAAxH,IACAwH,EAAAvH,MACA4C,EAAA4E,WAAArJ,KAAA8B,UAGA,GAAAsH,EAAA1D,SACA,MAAA/C,EACA,IAAA3C,KAAA4F,GAAA7C,GACA,MAAAqG,GAAAvC,QAAA9D,EAAAJ,CACA,IAAAyG,EAAAxD,GAAA7C,GACA,MAAA/C,MAAA6G,QAAA9D,EAAAJ,CAEA,IAAA3C,KAAA2F,aACA,MAAAyD,GAAAzD,aACA3F,KAAAiD,MAAAoB,IAAA+E,EAAAnG,OAEAjD,KAAAiD,MAAAoB,IAAA+E,GAAAnG,KACK,IAAAmG,EAAAzD,aACL,MAAA3F,MAAAqE,IAAA+E,EAAAnG,YAGA,IAAAjD,KAAAsH,GAAApC,IAAAkE,EAAA9B,GAAApC,GACA,MAAA1C,GAAAxC,KAAAwF,WAAA4D,EAAA5D,WAAAxF,KAAA8B,SAKA,IAAAuG,GAAArI,KAAA6B,OAAA,GACAyG,EAAA,MAAAtI,KAAA6B,KACA0G,EAAAvI,KAAA4B,MAAA,GACA4G,EAAA,MAAAxI,KAAA4B,IAEA6G,EAAAW,EAAAvH,OAAA,GACA6G,EAAA,MAAAU,EAAAvH,KACA8G,EAAAS,EAAAxH,MAAA,GACAgH,EAAA,MAAAQ,EAAAxH,IAEAiH,EAAA,EAAAC,EAAA,EAAAC,EAAA,EAAAC,EAAA,CAqBA,OApBAA,IAAAR,EAAAI,EACAG,GAAAC,IAAA,GACAA,GAAA,MACAD,GAAAR,EAAAK,EACAE,GAAAC,IAAA,GACAA,GAAA,MACAA,GAAAP,EAAAG,EACAG,GAAAC,IAAA,GACAA,GAAA,MACAD,GAAAR,EAAAM,EACAC,GAAAC,IAAA,GACAA,GAAA,MACAA,GAAAP,EAAAI,EACAE,GAAAC,IAAA,GACAA,GAAA,MACAA,GAAAN,EAAAE,EACAG,GAAAC,IAAA,GACAA,GAAA,MACAD,GAAAR,EAAAO,EAAAN,EAAAK,EAAAJ,EAAAG,EAAAF,EAAAC,EACAI,GAAA,MACAvG,EAAAyG,GAAA,GAAAC,EAAAH,GAAA,GAAAC,EAAA9I,KAAA8B,WASAwD,EAAAjB,IAAAiB,EAAA6D,SAQA7D,EAAAgE,OAAA,SAAAC,GAGA,GAFAxH,EAAAwH,KACAA,EAAAhF,EAAAgF,IACAA,EAAA7D,SACA,KAAAjC,OAAA,mBAGA,IAAAgB,EAAA,CAIA,IAAAzE,KAAA8B,WACA,aAAA9B,KAAA6B,OACA,IAAA0H,EAAA3H,MAAA,IAAA2H,EAAA1H,KAEA,MAAA7B,KAQA,OAAAsC,IANAtC,KAAA8B,SAAA2C,EAAA+E,MAAA/E,EAAAgF,OACAzJ,KAAA4B,IACA5B,KAAA6B,KACA0H,EAAA3H,IACA2H,EAAA1H,MAEA4C,EAAA4E,WAAArJ,KAAA8B,UAGA,GAAA9B,KAAA0F,SACA,MAAA1F,MAAA8B,SAAAY,EAAAC,CACA,IAAA+G,GAAAzD,EAAA0D,CACA,IAAA3J,KAAA8B,SA6BK,CAKL,GAFAyH,EAAAzH,WACAyH,IAAAK,cACAL,EAAA5B,GAAA3H,MACA,MAAA0C,EACA,IAAA6G,EAAA5B,GAAA3H,KAAA6J,KAAA,IACA,MAAAzE,EACAuE,GAAAjH,MAtCA,CAGA,GAAA1C,KAAA4F,GAAA7C,GAAA,CACA,GAAAwG,EAAA3D,GAAAT,IAAAoE,EAAA3D,GAAAP,GACA,MAAAtC,EACA,IAAAwG,EAAA3D,GAAA7C,GACA,MAAAoC,EAKA,OADAuE,GADA1J,KAAA8J,IAAA,GACAhE,IAAAyD,GAAAQ,IAAA,GACAL,EAAA9D,GAAAjD,GACA4G,EAAA5D,aAAAR,EAAAE,GAEAY,EAAAjG,KAAAgG,IAAAuD,EAAAlF,IAAAqF,IACAC,EAAAD,EAAApF,IAAA2B,EAAAH,IAAAyD,KAIS,GAAAA,EAAA3D,GAAA7C,GACT,MAAA/C,MAAA8B,SAAAY,EAAAC,CACA,IAAA3C,KAAA2F,aACA,MAAA4D,GAAA5D,aACA3F,KAAAiD,MAAA6C,IAAAyD,EAAAtG,OACAjD,KAAAiD,MAAA6C,IAAAyD,GAAAtG,KACS,IAAAsG,EAAA5D,aACT,MAAA3F,MAAA8F,IAAAyD,EAAAtG,YACA0G,GAAAhH,EAmBA,IADAsD,EAAAjG,KACAiG,EAAA4B,IAAA0B,IAAA,CAGAG,EAAAzF,KAAA+F,IAAA,EAAA/F,KAAAgG,MAAAhE,EAAAT,WAAA+D,EAAA/D,YAWA,KAPA,GAAA0E,GAAAjG,KAAAkG,KAAAlG,KAAAmG,IAAAV,GAAAzF,KAAAoG,KACAC,EAAAJ,GAAA,KAAApG,EAAA,EAAAoG,EAAA,IAIAK,EAAA/H,EAAAkH,GACAc,EAAAD,EAAAlG,IAAAkF,GACAiB,EAAA7E,cAAA6E,EAAA7C,GAAA1B,IACAyD,GAAAY,EACAC,EAAA/H,EAAAkH,EAAA1J,KAAA8B,UACA0I,EAAAD,EAAAlG,IAAAkF,EAKAgB,GAAA7E,WACA6E,EAAApF,GAEAwE,IAAArF,IAAAiG,GACAtE,IAAAD,IAAAwE,GAEA,MAAAb,IASArE,EAAAQ,IAAAR,EAAAgE,OAOAhE,EAAAmF,OAAA,SAAAlB,GAKA,GAJAxH,EAAAwH,KACAA,EAAAhF,EAAAgF,IAGA9E,EAAA,CAOA,MAAAnC,IANAtC,KAAA8B,SAAA2C,EAAAiG,MAAAjG,EAAAkG,OACA3K,KAAA4B,IACA5B,KAAA6B,KACA0H,EAAA3H,IACA2H,EAAA1H,MAEA4C,EAAA4E,WAAArJ,KAAA8B,UAGA,MAAA9B,MAAAgG,IAAAhG,KAAA8F,IAAAyD,GAAAlF,IAAAkF,KASAjE,EAAAsF,IAAAtF,EAAAmF,OAQAnF,EAAAW,IAAAX,EAAAmF,OAMAnF,EAAA6C,IAAA,WACA,MAAA7F,IAAAtC,KAAA4B,KAAA5B,KAAA6B,KAAA7B,KAAA8B,WAQAwD,EAAAuF,IAAA,SAAA7D,GAGA,MAFAjF,GAAAiF,KACAA,EAAAzC,EAAAyC,IACA1E,EAAAtC,KAAA4B,IAAAoF,EAAApF,IAAA5B,KAAA6B,KAAAmF,EAAAnF,KAAA7B,KAAA8B,WAQAwD,EAAAwF,GAAA,SAAA9D,GAGA,MAFAjF,GAAAiF,KACAA,EAAAzC,EAAAyC,IACA1E,EAAAtC,KAAA4B,IAAAoF,EAAApF,IAAA5B,KAAA6B,KAAAmF,EAAAnF,KAAA7B,KAAA8B,WAQAwD,EAAAyF,IAAA,SAAA/D,GAGA,MAFAjF,GAAAiF,KACAA,EAAAzC,EAAAyC,IACA1E,EAAAtC,KAAA4B,IAAAoF,EAAApF,IAAA5B,KAAA6B,KAAAmF,EAAAnF,KAAA7B,KAAA8B,WAQAwD,EAAA0F,UAAA,SAAAC,GAGA,MAFAlJ,GAAAkJ,KACAA,IAAA1F,SACA,IAAA0F,GAAA,IACAjL,KACAiL,EAAA,GACA3I,EAAAtC,KAAA4B,KAAAqJ,EAAAjL,KAAA6B,MAAAoJ,EAAAjL,KAAA4B,MAAA,GAAAqJ,EAAAjL,KAAA8B,UAEAQ,EAAA,EAAAtC,KAAA4B,KAAAqJ,EAAA,GAAAjL,KAAA8B,WASAwD,EAAAyE,IAAAzE,EAAA0F,UAOA1F,EAAA4F,WAAA,SAAAD,GAGA,MAFAlJ,GAAAkJ,KACAA,IAAA1F,SACA,IAAA0F,GAAA,IACAjL,KACAiL,EAAA,GACA3I,EAAAtC,KAAA4B,MAAAqJ,EAAAjL,KAAA6B,MAAA,GAAAoJ,EAAAjL,KAAA6B,MAAAoJ,EAAAjL,KAAA8B,UAEAQ,EAAAtC,KAAA6B,MAAAoJ,EAAA,GAAAjL,KAAA6B,MAAA,OAAA7B,KAAA8B,WASAwD,EAAAwE,IAAAxE,EAAA4F,WAOA5F,EAAA6F,mBAAA,SAAAF,GAIA,GAHAlJ,EAAAkJ,KACAA,IAAA1F,SAEA,KADA0F,GAAA,IAEA,MAAAjL,KAEA,IAAA6B,GAAA7B,KAAA6B,IACA,IAAAoJ,EAAA,IAEA,MAAA3I,GADAtC,KAAA4B,MACAqJ,EAAApJ,GAAA,GAAAoJ,EAAApJ,IAAAoJ,EAAAjL,KAAA8B,UACS,YAAAmJ,EACT3I,EAAAT,EAAA,EAAA7B,KAAA8B,UAEAQ,EAAAT,IAAAoJ,EAAA,KAAAjL,KAAA8B,WAUAwD,EAAAuE,KAAAvE,EAAA6F,mBAQA7F,EAAA8F,MAAA9F,EAAA6F,mBAMA7F,EAAA+F,SAAA,WACA,MAAArL,MAAA8B,SAEAQ,EAAAtC,KAAA4B,IAAA5B,KAAA6B,MAAA,GADA7B,MAQAsF,EAAAsE,WAAA,WACA,MAAA5J,MAAA8B,SACA9B,KACAsC,EAAAtC,KAAA4B,IAAA5B,KAAA6B,MAAA,IAQAyD,EAAAgG,QAAA,SAAA7D,GACA,MAAAA,GAAAzH,KAAAuL,YAAAvL,KAAAwL,aAOAlG,EAAAiG,UAAA,WACA,GAAAE,GAAAzL,KAAA6B,KACA6J,EAAA1L,KAAA4B,GACA,QACA,IAAA8J,EACAA,IAAA,MACAA,IAAA,OACAA,IAAA,GACA,IAAAD,EACAA,IAAA,MACAA,IAAA,OACAA,IAAA,KAQAnG,EAAAkG,UAAA,WACA,GAAAC,GAAAzL,KAAA6B,KACA6J,EAAA1L,KAAA4B,GACA,QACA6J,IAAA,GACAA,IAAA,OACAA,IAAA,MACA,IAAAA,EACAC,IAAA,GACAA,IAAA,OACAA,IAAA,MACA,IAAAA,IAWA/J,EAAAgK,UAAA,SAAAC,EAAA9J,EAAA2F,GACA,MAAAA,GAAA9F,EAAAkK,YAAAD,EAAA9J,GAAAH,EAAAmK,YAAAF,EAAA9J,IASAH,EAAAkK,YAAA,SAAAD,EAAA9J,GACA,UAAAH,GACAiK,EAAA,GACAA,EAAA,MACAA,EAAA,OACAA,EAAA,OACAA,EAAA,GACAA,EAAA,MACAA,EAAA,OACAA,EAAA,OACA9J,IAUAH,EAAAmK,YAAA,SAAAF,EAAA9J,GACA,UAAAH,GACAiK,EAAA,OACAA,EAAA,OACAA,EAAA,MACAA,EAAA,GACAA,EAAA,OACAA,EAAA,OACAA,EAAA,MACAA,EAAA,GACA9J","file":"long.js","sourcesContent":["(function webpackUniversalModuleDefinition(root, factory) {\n\tif(typeof exports === 'object' && typeof module === 'object')\n\t\tmodule.exports = factory();\n\telse if(typeof define === 'function' && define.amd)\n\t\tdefine([], factory);\n\telse if(typeof exports === 'object')\n\t\texports[\"Long\"] = factory();\n\telse\n\t\troot[\"Long\"] = factory();\n})(typeof self !== 'undefined' ? self : this, function() {\nreturn \n\n\n// WEBPACK FOOTER //\n// webpack/universalModuleDefinition","(function webpackUniversalModuleDefinition(root, factory) {\n\tif(typeof exports === 'object' && typeof module === 'object')\n\t\tmodule.exports = factory();\n\telse if(typeof define === 'function' && define.amd)\n\t\tdefine([], factory);\n\telse if(typeof exports === 'object')\n\t\texports[\"Long\"] = factory();\n\telse\n\t\troot[\"Long\"] = factory();\n})(typeof self !== 'undefined' ? self : this, function() {\nreturn /******/ (function(modules) { // webpackBootstrap\n/******/ \t// The module cache\n/******/ \tvar installedModules = {};\n/******/\n/******/ \t// The require function\n/******/ \tfunction __webpack_require__(moduleId) {\n/******/\n/******/ \t\t// Check if module is in cache\n/******/ \t\tif(installedModules[moduleId]) {\n/******/ \t\t\treturn installedModules[moduleId].exports;\n/******/ \t\t}\n/******/ \t\t// Create a new module (and put it into the cache)\n/******/ \t\tvar module = installedModules[moduleId] = {\n/******/ \t\t\ti: moduleId,\n/******/ \t\t\tl: false,\n/******/ \t\t\texports: {}\n/******/ \t\t};\n/******/\n/******/ \t\t// Execute the module function\n/******/ \t\tmodules[moduleId].call(module.exports, module, module.exports, __webpack_require__);\n/******/\n/******/ \t\t// Flag the module as loaded\n/******/ \t\tmodule.l = true;\n/******/\n/******/ \t\t// Return the exports of the module\n/******/ \t\treturn module.exports;\n/******/ \t}\n/******/\n/******/\n/******/ \t// expose the modules object (__webpack_modules__)\n/******/ \t__webpack_require__.m = modules;\n/******/\n/******/ \t// expose the module cache\n/******/ \t__webpack_require__.c = installedModules;\n/******/\n/******/ \t// define getter function for harmony exports\n/******/ \t__webpack_require__.d = function(exports, name, getter) {\n/******/ \t\tif(!__webpack_require__.o(exports, name)) {\n/******/ \t\t\tObject.defineProperty(exports, name, {\n/******/ \t\t\t\tconfigurable: false,\n/******/ \t\t\t\tenumerable: true,\n/******/ \t\t\t\tget: getter\n/******/ \t\t\t});\n/******/ \t\t}\n/******/ \t};\n/******/\n/******/ \t// getDefaultExport function for compatibility with non-harmony modules\n/******/ \t__webpack_require__.n = function(module) {\n/******/ \t\tvar getter = module && module.__esModule ?\n/******/ \t\t\tfunction getDefault() { return module['default']; } :\n/******/ \t\t\tfunction getModuleExports() { return module; };\n/******/ \t\t__webpack_require__.d(getter, 'a', getter);\n/******/ \t\treturn getter;\n/******/ \t};\n/******/\n/******/ \t// Object.prototype.hasOwnProperty.call\n/******/ \t__webpack_require__.o = function(object, property) { return Object.prototype.hasOwnProperty.call(object, property); };\n/******/\n/******/ \t// __webpack_public_path__\n/******/ \t__webpack_require__.p = \"\";\n/******/\n/******/ \t// Load entry module and return exports\n/******/ \treturn __webpack_require__(__webpack_require__.s = 0);\n/******/ })\n/************************************************************************/\n/******/ ([\n/* 0 */\n/***/ (function(module, exports) {\n\nmodule.exports = Long;\r\n\r\n/**\r\n * wasm optimizations, to do native i64 multiplication and divide\r\n */\r\nvar wasm = null;\r\n\r\ntry {\r\n wasm = new WebAssembly.Instance(new WebAssembly.Module(new Uint8Array([\r\n 0, 97, 115, 109, 1, 0, 0, 0, 1, 13, 2, 96, 0, 1, 127, 96, 4, 127, 127, 127, 127, 1, 127, 3, 7, 6, 0, 1, 1, 1, 1, 1, 6, 6, 1, 127, 1, 65, 0, 11, 7, 50, 6, 3, 109, 117, 108, 0, 1, 5, 100, 105, 118, 95, 115, 0, 2, 5, 100, 105, 118, 95, 117, 0, 3, 5, 114, 101, 109, 95, 115, 0, 4, 5, 114, 101, 109, 95, 117, 0, 5, 8, 103, 101, 116, 95, 104, 105, 103, 104, 0, 0, 10, 191, 1, 6, 4, 0, 35, 0, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 126, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 127, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 128, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 129, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 130, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11\r\n ])), {}).exports;\r\n} catch (e) {\r\n // no wasm support :(\r\n}\r\n\r\n/**\r\n * Constructs a 64 bit two's-complement integer, given its low and high 32 bit values as *signed* integers.\r\n * See the from* functions below for more convenient ways of constructing Longs.\r\n * @exports Long\r\n * @class A Long class for representing a 64 bit two's-complement integer value.\r\n * @param {number} low The low (signed) 32 bits of the long\r\n * @param {number} high The high (signed) 32 bits of the long\r\n * @param {boolean=} unsigned Whether unsigned or not, defaults to signed\r\n * @constructor\r\n */\r\nfunction Long(low, high, unsigned) {\r\n\r\n /**\r\n * The low 32 bits as a signed value.\r\n * @type {number}\r\n */\r\n this.low = low | 0;\r\n\r\n /**\r\n * The high 32 bits as a signed value.\r\n * @type {number}\r\n */\r\n this.high = high | 0;\r\n\r\n /**\r\n * Whether unsigned or not.\r\n * @type {boolean}\r\n */\r\n this.unsigned = !!unsigned;\r\n}\r\n\r\n// The internal representation of a long is the two given signed, 32-bit values.\r\n// We use 32-bit pieces because these are the size of integers on which\r\n// Javascript performs bit-operations. For operations like addition and\r\n// multiplication, we split each number into 16 bit pieces, which can easily be\r\n// multiplied within Javascript's floating-point representation without overflow\r\n// or change in sign.\r\n//\r\n// In the algorithms below, we frequently reduce the negative case to the\r\n// positive case by negating the input(s) and then post-processing the result.\r\n// Note that we must ALWAYS check specially whether those values are MIN_VALUE\r\n// (-2^63) because -MIN_VALUE == MIN_VALUE (since 2^63 cannot be represented as\r\n// a positive number, it overflows back into a negative). Not handling this\r\n// case would often result in infinite recursion.\r\n//\r\n// Common constant values ZERO, ONE, NEG_ONE, etc. are defined below the from*\r\n// methods on which they depend.\r\n\r\n/**\r\n * An indicator used to reliably determine if an object is a Long or not.\r\n * @type {boolean}\r\n * @const\r\n * @private\r\n */\r\nLong.prototype.__isLong__;\r\n\r\nObject.defineProperty(Long.prototype, \"__isLong__\", { value: true });\r\n\r\n/**\r\n * @function\r\n * @param {*} obj Object\r\n * @returns {boolean}\r\n * @inner\r\n */\r\nfunction isLong(obj) {\r\n return (obj && obj[\"__isLong__\"]) === true;\r\n}\r\n\r\n/**\r\n * Tests if the specified object is a Long.\r\n * @function\r\n * @param {*} obj Object\r\n * @returns {boolean}\r\n */\r\nLong.isLong = isLong;\r\n\r\n/**\r\n * A cache of the Long representations of small integer values.\r\n * @type {!Object}\r\n * @inner\r\n */\r\nvar INT_CACHE = {};\r\n\r\n/**\r\n * A cache of the Long representations of small unsigned integer values.\r\n * @type {!Object}\r\n * @inner\r\n */\r\nvar UINT_CACHE = {};\r\n\r\n/**\r\n * @param {number} value\r\n * @param {boolean=} unsigned\r\n * @returns {!Long}\r\n * @inner\r\n */\r\nfunction fromInt(value, unsigned) {\r\n var obj, cachedObj, cache;\r\n if (unsigned) {\r\n value >>>= 0;\r\n if (cache = (0 <= value && value < 256)) {\r\n cachedObj = UINT_CACHE[value];\r\n if (cachedObj)\r\n return cachedObj;\r\n }\r\n obj = fromBits(value, (value | 0) < 0 ? -1 : 0, true);\r\n if (cache)\r\n UINT_CACHE[value] = obj;\r\n return obj;\r\n } else {\r\n value |= 0;\r\n if (cache = (-128 <= value && value < 128)) {\r\n cachedObj = INT_CACHE[value];\r\n if (cachedObj)\r\n return cachedObj;\r\n }\r\n obj = fromBits(value, value < 0 ? -1 : 0, false);\r\n if (cache)\r\n INT_CACHE[value] = obj;\r\n return obj;\r\n }\r\n}\r\n\r\n/**\r\n * Returns a Long representing the given 32 bit integer value.\r\n * @function\r\n * @param {number} value The 32 bit integer in question\r\n * @param {boolean=} unsigned Whether unsigned or not, defaults to signed\r\n * @returns {!Long} The corresponding Long value\r\n */\r\nLong.fromInt = fromInt;\r\n\r\n/**\r\n * @param {number} value\r\n * @param {boolean=} unsigned\r\n * @returns {!Long}\r\n * @inner\r\n */\r\nfunction fromNumber(value, unsigned) {\r\n if (isNaN(value))\r\n return unsigned ? UZERO : ZERO;\r\n if (unsigned) {\r\n if (value < 0)\r\n return UZERO;\r\n if (value >= TWO_PWR_64_DBL)\r\n return MAX_UNSIGNED_VALUE;\r\n } else {\r\n if (value <= -TWO_PWR_63_DBL)\r\n return MIN_VALUE;\r\n if (value + 1 >= TWO_PWR_63_DBL)\r\n return MAX_VALUE;\r\n }\r\n if (value < 0)\r\n return fromNumber(-value, unsigned).neg();\r\n return fromBits((value % TWO_PWR_32_DBL) | 0, (value / TWO_PWR_32_DBL) | 0, unsigned);\r\n}\r\n\r\n/**\r\n * Returns a Long representing the given value, provided that it is a finite number. Otherwise, zero is returned.\r\n * @function\r\n * @param {number} value The number in question\r\n * @param {boolean=} unsigned Whether unsigned or not, defaults to signed\r\n * @returns {!Long} The corresponding Long value\r\n */\r\nLong.fromNumber = fromNumber;\r\n\r\n/**\r\n * @param {number} lowBits\r\n * @param {number} highBits\r\n * @param {boolean=} unsigned\r\n * @returns {!Long}\r\n * @inner\r\n */\r\nfunction fromBits(lowBits, highBits, unsigned) {\r\n return new Long(lowBits, highBits, unsigned);\r\n}\r\n\r\n/**\r\n * Returns a Long representing the 64 bit integer that comes by concatenating the given low and high bits. Each is\r\n * assumed to use 32 bits.\r\n * @function\r\n * @param {number} lowBits The low 32 bits\r\n * @param {number} highBits The high 32 bits\r\n * @param {boolean=} unsigned Whether unsigned or not, defaults to signed\r\n * @returns {!Long} The corresponding Long value\r\n */\r\nLong.fromBits = fromBits;\r\n\r\n/**\r\n * @function\r\n * @param {number} base\r\n * @param {number} exponent\r\n * @returns {number}\r\n * @inner\r\n */\r\nvar pow_dbl = Math.pow; // Used 4 times (4*8 to 15+4)\r\n\r\n/**\r\n * @param {string} str\r\n * @param {(boolean|number)=} unsigned\r\n * @param {number=} radix\r\n * @returns {!Long}\r\n * @inner\r\n */\r\nfunction fromString(str, unsigned, radix) {\r\n if (str.length === 0)\r\n throw Error('empty string');\r\n if (str === \"NaN\" || str === \"Infinity\" || str === \"+Infinity\" || str === \"-Infinity\")\r\n return ZERO;\r\n if (typeof unsigned === 'number') {\r\n // For goog.math.long compatibility\r\n radix = unsigned,\r\n unsigned = false;\r\n } else {\r\n unsigned = !! unsigned;\r\n }\r\n radix = radix || 10;\r\n if (radix < 2 || 36 < radix)\r\n throw RangeError('radix');\r\n\r\n var p;\r\n if ((p = str.indexOf('-')) > 0)\r\n throw Error('interior hyphen');\r\n else if (p === 0) {\r\n return fromString(str.substring(1), unsigned, radix).neg();\r\n }\r\n\r\n // Do several (8) digits each time through the loop, so as to\r\n // minimize the calls to the very expensive emulated div.\r\n var radixToPower = fromNumber(pow_dbl(radix, 8));\r\n\r\n var result = ZERO;\r\n for (var i = 0; i < str.length; i += 8) {\r\n var size = Math.min(8, str.length - i),\r\n value = parseInt(str.substring(i, i + size), radix);\r\n if (size < 8) {\r\n var power = fromNumber(pow_dbl(radix, size));\r\n result = result.mul(power).add(fromNumber(value));\r\n } else {\r\n result = result.mul(radixToPower);\r\n result = result.add(fromNumber(value));\r\n }\r\n }\r\n result.unsigned = unsigned;\r\n return result;\r\n}\r\n\r\n/**\r\n * Returns a Long representation of the given string, written using the specified radix.\r\n * @function\r\n * @param {string} str The textual representation of the Long\r\n * @param {(boolean|number)=} unsigned Whether unsigned or not, defaults to signed\r\n * @param {number=} radix The radix in which the text is written (2-36), defaults to 10\r\n * @returns {!Long} The corresponding Long value\r\n */\r\nLong.fromString = fromString;\r\n\r\n/**\r\n * @function\r\n * @param {!Long|number|string|!{low: number, high: number, unsigned: boolean}} val\r\n * @param {boolean=} unsigned\r\n * @returns {!Long}\r\n * @inner\r\n */\r\nfunction fromValue(val, unsigned) {\r\n if (typeof val === 'number')\r\n return fromNumber(val, unsigned);\r\n if (typeof val === 'string')\r\n return fromString(val, unsigned);\r\n // Throws for non-objects, converts non-instanceof Long:\r\n return fromBits(val.low, val.high, typeof unsigned === 'boolean' ? unsigned : val.unsigned);\r\n}\r\n\r\n/**\r\n * Converts the specified value to a Long using the appropriate from* function for its type.\r\n * @function\r\n * @param {!Long|number|string|!{low: number, high: number, unsigned: boolean}} val Value\r\n * @param {boolean=} unsigned Whether unsigned or not, defaults to signed\r\n * @returns {!Long}\r\n */\r\nLong.fromValue = fromValue;\r\n\r\n// NOTE: the compiler should inline these constant values below and then remove these variables, so there should be\r\n// no runtime penalty for these.\r\n\r\n/**\r\n * @type {number}\r\n * @const\r\n * @inner\r\n */\r\nvar TWO_PWR_16_DBL = 1 << 16;\r\n\r\n/**\r\n * @type {number}\r\n * @const\r\n * @inner\r\n */\r\nvar TWO_PWR_24_DBL = 1 << 24;\r\n\r\n/**\r\n * @type {number}\r\n * @const\r\n * @inner\r\n */\r\nvar TWO_PWR_32_DBL = TWO_PWR_16_DBL * TWO_PWR_16_DBL;\r\n\r\n/**\r\n * @type {number}\r\n * @const\r\n * @inner\r\n */\r\nvar TWO_PWR_64_DBL = TWO_PWR_32_DBL * TWO_PWR_32_DBL;\r\n\r\n/**\r\n * @type {number}\r\n * @const\r\n * @inner\r\n */\r\nvar TWO_PWR_63_DBL = TWO_PWR_64_DBL / 2;\r\n\r\n/**\r\n * @type {!Long}\r\n * @const\r\n * @inner\r\n */\r\nvar TWO_PWR_24 = fromInt(TWO_PWR_24_DBL);\r\n\r\n/**\r\n * @type {!Long}\r\n * @inner\r\n */\r\nvar ZERO = fromInt(0);\r\n\r\n/**\r\n * Signed zero.\r\n * @type {!Long}\r\n */\r\nLong.ZERO = ZERO;\r\n\r\n/**\r\n * @type {!Long}\r\n * @inner\r\n */\r\nvar UZERO = fromInt(0, true);\r\n\r\n/**\r\n * Unsigned zero.\r\n * @type {!Long}\r\n */\r\nLong.UZERO = UZERO;\r\n\r\n/**\r\n * @type {!Long}\r\n * @inner\r\n */\r\nvar ONE = fromInt(1);\r\n\r\n/**\r\n * Signed one.\r\n * @type {!Long}\r\n */\r\nLong.ONE = ONE;\r\n\r\n/**\r\n * @type {!Long}\r\n * @inner\r\n */\r\nvar UONE = fromInt(1, true);\r\n\r\n/**\r\n * Unsigned one.\r\n * @type {!Long}\r\n */\r\nLong.UONE = UONE;\r\n\r\n/**\r\n * @type {!Long}\r\n * @inner\r\n */\r\nvar NEG_ONE = fromInt(-1);\r\n\r\n/**\r\n * Signed negative one.\r\n * @type {!Long}\r\n */\r\nLong.NEG_ONE = NEG_ONE;\r\n\r\n/**\r\n * @type {!Long}\r\n * @inner\r\n */\r\nvar MAX_VALUE = fromBits(0xFFFFFFFF|0, 0x7FFFFFFF|0, false);\r\n\r\n/**\r\n * Maximum signed value.\r\n * @type {!Long}\r\n */\r\nLong.MAX_VALUE = MAX_VALUE;\r\n\r\n/**\r\n * @type {!Long}\r\n * @inner\r\n */\r\nvar MAX_UNSIGNED_VALUE = fromBits(0xFFFFFFFF|0, 0xFFFFFFFF|0, true);\r\n\r\n/**\r\n * Maximum unsigned value.\r\n * @type {!Long}\r\n */\r\nLong.MAX_UNSIGNED_VALUE = MAX_UNSIGNED_VALUE;\r\n\r\n/**\r\n * @type {!Long}\r\n * @inner\r\n */\r\nvar MIN_VALUE = fromBits(0, 0x80000000|0, false);\r\n\r\n/**\r\n * Minimum signed value.\r\n * @type {!Long}\r\n */\r\nLong.MIN_VALUE = MIN_VALUE;\r\n\r\n/**\r\n * @alias Long.prototype\r\n * @inner\r\n */\r\nvar LongPrototype = Long.prototype;\r\n\r\n/**\r\n * Converts the Long to a 32 bit integer, assuming it is a 32 bit integer.\r\n * @returns {number}\r\n */\r\nLongPrototype.toInt = function toInt() {\r\n return this.unsigned ? this.low >>> 0 : this.low;\r\n};\r\n\r\n/**\r\n * Converts the Long to a the nearest floating-point representation of this value (double, 53 bit mantissa).\r\n * @returns {number}\r\n */\r\nLongPrototype.toNumber = function toNumber() {\r\n if (this.unsigned)\r\n return ((this.high >>> 0) * TWO_PWR_32_DBL) + (this.low >>> 0);\r\n return this.high * TWO_PWR_32_DBL + (this.low >>> 0);\r\n};\r\n\r\n/**\r\n * Converts the Long to a string written in the specified radix.\r\n * @param {number=} radix Radix (2-36), defaults to 10\r\n * @returns {string}\r\n * @override\r\n * @throws {RangeError} If `radix` is out of range\r\n */\r\nLongPrototype.toString = function toString(radix) {\r\n radix = radix || 10;\r\n if (radix < 2 || 36 < radix)\r\n throw RangeError('radix');\r\n if (this.isZero())\r\n return '0';\r\n if (this.isNegative()) { // Unsigned Longs are never negative\r\n if (this.eq(MIN_VALUE)) {\r\n // We need to change the Long value before it can be negated, so we remove\r\n // the bottom-most digit in this base and then recurse to do the rest.\r\n var radixLong = fromNumber(radix),\r\n div = this.div(radixLong),\r\n rem1 = div.mul(radixLong).sub(this);\r\n return div.toString(radix) + rem1.toInt().toString(radix);\r\n } else\r\n return '-' + this.neg().toString(radix);\r\n }\r\n\r\n // Do several (6) digits each time through the loop, so as to\r\n // minimize the calls to the very expensive emulated div.\r\n var radixToPower = fromNumber(pow_dbl(radix, 6), this.unsigned),\r\n rem = this;\r\n var result = '';\r\n while (true) {\r\n var remDiv = rem.div(radixToPower),\r\n intval = rem.sub(remDiv.mul(radixToPower)).toInt() >>> 0,\r\n digits = intval.toString(radix);\r\n rem = remDiv;\r\n if (rem.isZero())\r\n return digits + result;\r\n else {\r\n while (digits.length < 6)\r\n digits = '0' + digits;\r\n result = '' + digits + result;\r\n }\r\n }\r\n};\r\n\r\n/**\r\n * Gets the high 32 bits as a signed integer.\r\n * @returns {number} Signed high bits\r\n */\r\nLongPrototype.getHighBits = function getHighBits() {\r\n return this.high;\r\n};\r\n\r\n/**\r\n * Gets the high 32 bits as an unsigned integer.\r\n * @returns {number} Unsigned high bits\r\n */\r\nLongPrototype.getHighBitsUnsigned = function getHighBitsUnsigned() {\r\n return this.high >>> 0;\r\n};\r\n\r\n/**\r\n * Gets the low 32 bits as a signed integer.\r\n * @returns {number} Signed low bits\r\n */\r\nLongPrototype.getLowBits = function getLowBits() {\r\n return this.low;\r\n};\r\n\r\n/**\r\n * Gets the low 32 bits as an unsigned integer.\r\n * @returns {number} Unsigned low bits\r\n */\r\nLongPrototype.getLowBitsUnsigned = function getLowBitsUnsigned() {\r\n return this.low >>> 0;\r\n};\r\n\r\n/**\r\n * Gets the number of bits needed to represent the absolute value of this Long.\r\n * @returns {number}\r\n */\r\nLongPrototype.getNumBitsAbs = function getNumBitsAbs() {\r\n if (this.isNegative()) // Unsigned Longs are never negative\r\n return this.eq(MIN_VALUE) ? 64 : this.neg().getNumBitsAbs();\r\n var val = this.high != 0 ? this.high : this.low;\r\n for (var bit = 31; bit > 0; bit--)\r\n if ((val & (1 << bit)) != 0)\r\n break;\r\n return this.high != 0 ? bit + 33 : bit + 1;\r\n};\r\n\r\n/**\r\n * Tests if this Long's value equals zero.\r\n * @returns {boolean}\r\n */\r\nLongPrototype.isZero = function isZero() {\r\n return this.high === 0 && this.low === 0;\r\n};\r\n\r\n/**\r\n * Tests if this Long's value equals zero. This is an alias of {@link Long#isZero}.\r\n * @returns {boolean}\r\n */\r\nLongPrototype.eqz = LongPrototype.isZero;\r\n\r\n/**\r\n * Tests if this Long's value is negative.\r\n * @returns {boolean}\r\n */\r\nLongPrototype.isNegative = function isNegative() {\r\n return !this.unsigned && this.high < 0;\r\n};\r\n\r\n/**\r\n * Tests if this Long's value is positive.\r\n * @returns {boolean}\r\n */\r\nLongPrototype.isPositive = function isPositive() {\r\n return this.unsigned || this.high >= 0;\r\n};\r\n\r\n/**\r\n * Tests if this Long's value is odd.\r\n * @returns {boolean}\r\n */\r\nLongPrototype.isOdd = function isOdd() {\r\n return (this.low & 1) === 1;\r\n};\r\n\r\n/**\r\n * Tests if this Long's value is even.\r\n * @returns {boolean}\r\n */\r\nLongPrototype.isEven = function isEven() {\r\n return (this.low & 1) === 0;\r\n};\r\n\r\n/**\r\n * Tests if this Long's value equals the specified's.\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.equals = function equals(other) {\r\n if (!isLong(other))\r\n other = fromValue(other);\r\n if (this.unsigned !== other.unsigned && (this.high >>> 31) === 1 && (other.high >>> 31) === 1)\r\n return false;\r\n return this.high === other.high && this.low === other.low;\r\n};\r\n\r\n/**\r\n * Tests if this Long's value equals the specified's. This is an alias of {@link Long#equals}.\r\n * @function\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.eq = LongPrototype.equals;\r\n\r\n/**\r\n * Tests if this Long's value differs from the specified's.\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.notEquals = function notEquals(other) {\r\n return !this.eq(/* validates */ other);\r\n};\r\n\r\n/**\r\n * Tests if this Long's value differs from the specified's. This is an alias of {@link Long#notEquals}.\r\n * @function\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.neq = LongPrototype.notEquals;\r\n\r\n/**\r\n * Tests if this Long's value differs from the specified's. This is an alias of {@link Long#notEquals}.\r\n * @function\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.ne = LongPrototype.notEquals;\r\n\r\n/**\r\n * Tests if this Long's value is less than the specified's.\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.lessThan = function lessThan(other) {\r\n return this.comp(/* validates */ other) < 0;\r\n};\r\n\r\n/**\r\n * Tests if this Long's value is less than the specified's. This is an alias of {@link Long#lessThan}.\r\n * @function\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.lt = LongPrototype.lessThan;\r\n\r\n/**\r\n * Tests if this Long's value is less than or equal the specified's.\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.lessThanOrEqual = function lessThanOrEqual(other) {\r\n return this.comp(/* validates */ other) <= 0;\r\n};\r\n\r\n/**\r\n * Tests if this Long's value is less than or equal the specified's. This is an alias of {@link Long#lessThanOrEqual}.\r\n * @function\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.lte = LongPrototype.lessThanOrEqual;\r\n\r\n/**\r\n * Tests if this Long's value is less than or equal the specified's. This is an alias of {@link Long#lessThanOrEqual}.\r\n * @function\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.le = LongPrototype.lessThanOrEqual;\r\n\r\n/**\r\n * Tests if this Long's value is greater than the specified's.\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.greaterThan = function greaterThan(other) {\r\n return this.comp(/* validates */ other) > 0;\r\n};\r\n\r\n/**\r\n * Tests if this Long's value is greater than the specified's. This is an alias of {@link Long#greaterThan}.\r\n * @function\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.gt = LongPrototype.greaterThan;\r\n\r\n/**\r\n * Tests if this Long's value is greater than or equal the specified's.\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.greaterThanOrEqual = function greaterThanOrEqual(other) {\r\n return this.comp(/* validates */ other) >= 0;\r\n};\r\n\r\n/**\r\n * Tests if this Long's value is greater than or equal the specified's. This is an alias of {@link Long#greaterThanOrEqual}.\r\n * @function\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.gte = LongPrototype.greaterThanOrEqual;\r\n\r\n/**\r\n * Tests if this Long's value is greater than or equal the specified's. This is an alias of {@link Long#greaterThanOrEqual}.\r\n * @function\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.ge = LongPrototype.greaterThanOrEqual;\r\n\r\n/**\r\n * Compares this Long's value with the specified's.\r\n * @param {!Long|number|string} other Other value\r\n * @returns {number} 0 if they are the same, 1 if the this is greater and -1\r\n * if the given one is greater\r\n */\r\nLongPrototype.compare = function compare(other) {\r\n if (!isLong(other))\r\n other = fromValue(other);\r\n if (this.eq(other))\r\n return 0;\r\n var thisNeg = this.isNegative(),\r\n otherNeg = other.isNegative();\r\n if (thisNeg && !otherNeg)\r\n return -1;\r\n if (!thisNeg && otherNeg)\r\n return 1;\r\n // At this point the sign bits are the same\r\n if (!this.unsigned)\r\n return this.sub(other).isNegative() ? -1 : 1;\r\n // Both are positive if at least one is unsigned\r\n return (other.high >>> 0) > (this.high >>> 0) || (other.high === this.high && (other.low >>> 0) > (this.low >>> 0)) ? -1 : 1;\r\n};\r\n\r\n/**\r\n * Compares this Long's value with the specified's. This is an alias of {@link Long#compare}.\r\n * @function\r\n * @param {!Long|number|string} other Other value\r\n * @returns {number} 0 if they are the same, 1 if the this is greater and -1\r\n * if the given one is greater\r\n */\r\nLongPrototype.comp = LongPrototype.compare;\r\n\r\n/**\r\n * Negates this Long's value.\r\n * @returns {!Long} Negated Long\r\n */\r\nLongPrototype.negate = function negate() {\r\n if (!this.unsigned && this.eq(MIN_VALUE))\r\n return MIN_VALUE;\r\n return this.not().add(ONE);\r\n};\r\n\r\n/**\r\n * Negates this Long's value. This is an alias of {@link Long#negate}.\r\n * @function\r\n * @returns {!Long} Negated Long\r\n */\r\nLongPrototype.neg = LongPrototype.negate;\r\n\r\n/**\r\n * Returns the sum of this and the specified Long.\r\n * @param {!Long|number|string} addend Addend\r\n * @returns {!Long} Sum\r\n */\r\nLongPrototype.add = function add(addend) {\r\n if (!isLong(addend))\r\n addend = fromValue(addend);\r\n\r\n // Divide each number into 4 chunks of 16 bits, and then sum the chunks.\r\n\r\n var a48 = this.high >>> 16;\r\n var a32 = this.high & 0xFFFF;\r\n var a16 = this.low >>> 16;\r\n var a00 = this.low & 0xFFFF;\r\n\r\n var b48 = addend.high >>> 16;\r\n var b32 = addend.high & 0xFFFF;\r\n var b16 = addend.low >>> 16;\r\n var b00 = addend.low & 0xFFFF;\r\n\r\n var c48 = 0, c32 = 0, c16 = 0, c00 = 0;\r\n c00 += a00 + b00;\r\n c16 += c00 >>> 16;\r\n c00 &= 0xFFFF;\r\n c16 += a16 + b16;\r\n c32 += c16 >>> 16;\r\n c16 &= 0xFFFF;\r\n c32 += a32 + b32;\r\n c48 += c32 >>> 16;\r\n c32 &= 0xFFFF;\r\n c48 += a48 + b48;\r\n c48 &= 0xFFFF;\r\n return fromBits((c16 << 16) | c00, (c48 << 16) | c32, this.unsigned);\r\n};\r\n\r\n/**\r\n * Returns the difference of this and the specified Long.\r\n * @param {!Long|number|string} subtrahend Subtrahend\r\n * @returns {!Long} Difference\r\n */\r\nLongPrototype.subtract = function subtract(subtrahend) {\r\n if (!isLong(subtrahend))\r\n subtrahend = fromValue(subtrahend);\r\n return this.add(subtrahend.neg());\r\n};\r\n\r\n/**\r\n * Returns the difference of this and the specified Long. This is an alias of {@link Long#subtract}.\r\n * @function\r\n * @param {!Long|number|string} subtrahend Subtrahend\r\n * @returns {!Long} Difference\r\n */\r\nLongPrototype.sub = LongPrototype.subtract;\r\n\r\n/**\r\n * Returns the product of this and the specified Long.\r\n * @param {!Long|number|string} multiplier Multiplier\r\n * @returns {!Long} Product\r\n */\r\nLongPrototype.multiply = function multiply(multiplier) {\r\n if (this.isZero())\r\n return ZERO;\r\n if (!isLong(multiplier))\r\n multiplier = fromValue(multiplier);\r\n\r\n // use wasm support if present\r\n if (wasm) {\r\n var low = wasm.mul(this.low,\r\n this.high,\r\n multiplier.low,\r\n multiplier.high);\r\n return fromBits(low, wasm.get_high(), this.unsigned);\r\n }\r\n\r\n if (multiplier.isZero())\r\n return ZERO;\r\n if (this.eq(MIN_VALUE))\r\n return multiplier.isOdd() ? MIN_VALUE : ZERO;\r\n if (multiplier.eq(MIN_VALUE))\r\n return this.isOdd() ? MIN_VALUE : ZERO;\r\n\r\n if (this.isNegative()) {\r\n if (multiplier.isNegative())\r\n return this.neg().mul(multiplier.neg());\r\n else\r\n return this.neg().mul(multiplier).neg();\r\n } else if (multiplier.isNegative())\r\n return this.mul(multiplier.neg()).neg();\r\n\r\n // If both longs are small, use float multiplication\r\n if (this.lt(TWO_PWR_24) && multiplier.lt(TWO_PWR_24))\r\n return fromNumber(this.toNumber() * multiplier.toNumber(), this.unsigned);\r\n\r\n // Divide each long into 4 chunks of 16 bits, and then add up 4x4 products.\r\n // We can skip products that would overflow.\r\n\r\n var a48 = this.high >>> 16;\r\n var a32 = this.high & 0xFFFF;\r\n var a16 = this.low >>> 16;\r\n var a00 = this.low & 0xFFFF;\r\n\r\n var b48 = multiplier.high >>> 16;\r\n var b32 = multiplier.high & 0xFFFF;\r\n var b16 = multiplier.low >>> 16;\r\n var b00 = multiplier.low & 0xFFFF;\r\n\r\n var c48 = 0, c32 = 0, c16 = 0, c00 = 0;\r\n c00 += a00 * b00;\r\n c16 += c00 >>> 16;\r\n c00 &= 0xFFFF;\r\n c16 += a16 * b00;\r\n c32 += c16 >>> 16;\r\n c16 &= 0xFFFF;\r\n c16 += a00 * b16;\r\n c32 += c16 >>> 16;\r\n c16 &= 0xFFFF;\r\n c32 += a32 * b00;\r\n c48 += c32 >>> 16;\r\n c32 &= 0xFFFF;\r\n c32 += a16 * b16;\r\n c48 += c32 >>> 16;\r\n c32 &= 0xFFFF;\r\n c32 += a00 * b32;\r\n c48 += c32 >>> 16;\r\n c32 &= 0xFFFF;\r\n c48 += a48 * b00 + a32 * b16 + a16 * b32 + a00 * b48;\r\n c48 &= 0xFFFF;\r\n return fromBits((c16 << 16) | c00, (c48 << 16) | c32, this.unsigned);\r\n};\r\n\r\n/**\r\n * Returns the product of this and the specified Long. This is an alias of {@link Long#multiply}.\r\n * @function\r\n * @param {!Long|number|string} multiplier Multiplier\r\n * @returns {!Long} Product\r\n */\r\nLongPrototype.mul = LongPrototype.multiply;\r\n\r\n/**\r\n * Returns this Long divided by the specified. The result is signed if this Long is signed or\r\n * unsigned if this Long is unsigned.\r\n * @param {!Long|number|string} divisor Divisor\r\n * @returns {!Long} Quotient\r\n */\r\nLongPrototype.divide = function divide(divisor) {\r\n if (!isLong(divisor))\r\n divisor = fromValue(divisor);\r\n if (divisor.isZero())\r\n throw Error('division by zero');\r\n\r\n // use wasm support if present\r\n if (wasm) {\r\n // guard against signed division overflow: the largest\r\n // negative number / -1 would be 1 larger than the largest\r\n // positive number, due to two's complement.\r\n if (!this.unsigned &&\r\n this.high === -0x80000000 &&\r\n divisor.low === -1 && divisor.high === -1) {\r\n // be consistent with non-wasm code path\r\n return this;\r\n }\r\n var low = (this.unsigned ? wasm.div_u : wasm.div_s)(\r\n this.low,\r\n this.high,\r\n divisor.low,\r\n divisor.high\r\n );\r\n return fromBits(low, wasm.get_high(), this.unsigned);\r\n }\r\n\r\n if (this.isZero())\r\n return this.unsigned ? UZERO : ZERO;\r\n var approx, rem, res;\r\n if (!this.unsigned) {\r\n // This section is only relevant for signed longs and is derived from the\r\n // closure library as a whole.\r\n if (this.eq(MIN_VALUE)) {\r\n if (divisor.eq(ONE) || divisor.eq(NEG_ONE))\r\n return MIN_VALUE; // recall that -MIN_VALUE == MIN_VALUE\r\n else if (divisor.eq(MIN_VALUE))\r\n return ONE;\r\n else {\r\n // At this point, we have |other| >= 2, so |this/other| < |MIN_VALUE|.\r\n var halfThis = this.shr(1);\r\n approx = halfThis.div(divisor).shl(1);\r\n if (approx.eq(ZERO)) {\r\n return divisor.isNegative() ? ONE : NEG_ONE;\r\n } else {\r\n rem = this.sub(divisor.mul(approx));\r\n res = approx.add(rem.div(divisor));\r\n return res;\r\n }\r\n }\r\n } else if (divisor.eq(MIN_VALUE))\r\n return this.unsigned ? UZERO : ZERO;\r\n if (this.isNegative()) {\r\n if (divisor.isNegative())\r\n return this.neg().div(divisor.neg());\r\n return this.neg().div(divisor).neg();\r\n } else if (divisor.isNegative())\r\n return this.div(divisor.neg()).neg();\r\n res = ZERO;\r\n } else {\r\n // The algorithm below has not been made for unsigned longs. It's therefore\r\n // required to take special care of the MSB prior to running it.\r\n if (!divisor.unsigned)\r\n divisor = divisor.toUnsigned();\r\n if (divisor.gt(this))\r\n return UZERO;\r\n if (divisor.gt(this.shru(1))) // 15 >>> 1 = 7 ; with divisor = 8 ; true\r\n return UONE;\r\n res = UZERO;\r\n }\r\n\r\n // Repeat the following until the remainder is less than other: find a\r\n // floating-point that approximates remainder / other *from below*, add this\r\n // into the result, and subtract it from the remainder. It is critical that\r\n // the approximate value is less than or equal to the real value so that the\r\n // remainder never becomes negative.\r\n rem = this;\r\n while (rem.gte(divisor)) {\r\n // Approximate the result of division. This may be a little greater or\r\n // smaller than the actual value.\r\n approx = Math.max(1, Math.floor(rem.toNumber() / divisor.toNumber()));\r\n\r\n // We will tweak the approximate result by changing it in the 48-th digit or\r\n // the smallest non-fractional digit, whichever is larger.\r\n var log2 = Math.ceil(Math.log(approx) / Math.LN2),\r\n delta = (log2 <= 48) ? 1 : pow_dbl(2, log2 - 48),\r\n\r\n // Decrease the approximation until it is smaller than the remainder. Note\r\n // that if it is too large, the product overflows and is negative.\r\n approxRes = fromNumber(approx),\r\n approxRem = approxRes.mul(divisor);\r\n while (approxRem.isNegative() || approxRem.gt(rem)) {\r\n approx -= delta;\r\n approxRes = fromNumber(approx, this.unsigned);\r\n approxRem = approxRes.mul(divisor);\r\n }\r\n\r\n // We know the answer can't be zero... and actually, zero would cause\r\n // infinite recursion since we would make no progress.\r\n if (approxRes.isZero())\r\n approxRes = ONE;\r\n\r\n res = res.add(approxRes);\r\n rem = rem.sub(approxRem);\r\n }\r\n return res;\r\n};\r\n\r\n/**\r\n * Returns this Long divided by the specified. This is an alias of {@link Long#divide}.\r\n * @function\r\n * @param {!Long|number|string} divisor Divisor\r\n * @returns {!Long} Quotient\r\n */\r\nLongPrototype.div = LongPrototype.divide;\r\n\r\n/**\r\n * Returns this Long modulo the specified.\r\n * @param {!Long|number|string} divisor Divisor\r\n * @returns {!Long} Remainder\r\n */\r\nLongPrototype.modulo = function modulo(divisor) {\r\n if (!isLong(divisor))\r\n divisor = fromValue(divisor);\r\n\r\n // use wasm support if present\r\n if (wasm) {\r\n var low = (this.unsigned ? wasm.rem_u : wasm.rem_s)(\r\n this.low,\r\n this.high,\r\n divisor.low,\r\n divisor.high\r\n );\r\n return fromBits(low, wasm.get_high(), this.unsigned);\r\n }\r\n\r\n return this.sub(this.div(divisor).mul(divisor));\r\n};\r\n\r\n/**\r\n * Returns this Long modulo the specified. This is an alias of {@link Long#modulo}.\r\n * @function\r\n * @param {!Long|number|string} divisor Divisor\r\n * @returns {!Long} Remainder\r\n */\r\nLongPrototype.mod = LongPrototype.modulo;\r\n\r\n/**\r\n * Returns this Long modulo the specified. This is an alias of {@link Long#modulo}.\r\n * @function\r\n * @param {!Long|number|string} divisor Divisor\r\n * @returns {!Long} Remainder\r\n */\r\nLongPrototype.rem = LongPrototype.modulo;\r\n\r\n/**\r\n * Returns the bitwise NOT of this Long.\r\n * @returns {!Long}\r\n */\r\nLongPrototype.not = function not() {\r\n return fromBits(~this.low, ~this.high, this.unsigned);\r\n};\r\n\r\n/**\r\n * Returns the bitwise AND of this Long and the specified.\r\n * @param {!Long|number|string} other Other Long\r\n * @returns {!Long}\r\n */\r\nLongPrototype.and = function and(other) {\r\n if (!isLong(other))\r\n other = fromValue(other);\r\n return fromBits(this.low & other.low, this.high & other.high, this.unsigned);\r\n};\r\n\r\n/**\r\n * Returns the bitwise OR of this Long and the specified.\r\n * @param {!Long|number|string} other Other Long\r\n * @returns {!Long}\r\n */\r\nLongPrototype.or = function or(other) {\r\n if (!isLong(other))\r\n other = fromValue(other);\r\n return fromBits(this.low | other.low, this.high | other.high, this.unsigned);\r\n};\r\n\r\n/**\r\n * Returns the bitwise XOR of this Long and the given one.\r\n * @param {!Long|number|string} other Other Long\r\n * @returns {!Long}\r\n */\r\nLongPrototype.xor = function xor(other) {\r\n if (!isLong(other))\r\n other = fromValue(other);\r\n return fromBits(this.low ^ other.low, this.high ^ other.high, this.unsigned);\r\n};\r\n\r\n/**\r\n * Returns this Long with bits shifted to the left by the given amount.\r\n * @param {number|!Long} numBits Number of bits\r\n * @returns {!Long} Shifted Long\r\n */\r\nLongPrototype.shiftLeft = function shiftLeft(numBits) {\r\n if (isLong(numBits))\r\n numBits = numBits.toInt();\r\n if ((numBits &= 63) === 0)\r\n return this;\r\n else if (numBits < 32)\r\n return fromBits(this.low << numBits, (this.high << numBits) | (this.low >>> (32 - numBits)), this.unsigned);\r\n else\r\n return fromBits(0, this.low << (numBits - 32), this.unsigned);\r\n};\r\n\r\n/**\r\n * Returns this Long with bits shifted to the left by the given amount. This is an alias of {@link Long#shiftLeft}.\r\n * @function\r\n * @param {number|!Long} numBits Number of bits\r\n * @returns {!Long} Shifted Long\r\n */\r\nLongPrototype.shl = LongPrototype.shiftLeft;\r\n\r\n/**\r\n * Returns this Long with bits arithmetically shifted to the right by the given amount.\r\n * @param {number|!Long} numBits Number of bits\r\n * @returns {!Long} Shifted Long\r\n */\r\nLongPrototype.shiftRight = function shiftRight(numBits) {\r\n if (isLong(numBits))\r\n numBits = numBits.toInt();\r\n if ((numBits &= 63) === 0)\r\n return this;\r\n else if (numBits < 32)\r\n return fromBits((this.low >>> numBits) | (this.high << (32 - numBits)), this.high >> numBits, this.unsigned);\r\n else\r\n return fromBits(this.high >> (numBits - 32), this.high >= 0 ? 0 : -1, this.unsigned);\r\n};\r\n\r\n/**\r\n * Returns this Long with bits arithmetically shifted to the right by the given amount. This is an alias of {@link Long#shiftRight}.\r\n * @function\r\n * @param {number|!Long} numBits Number of bits\r\n * @returns {!Long} Shifted Long\r\n */\r\nLongPrototype.shr = LongPrototype.shiftRight;\r\n\r\n/**\r\n * Returns this Long with bits logically shifted to the right by the given amount.\r\n * @param {number|!Long} numBits Number of bits\r\n * @returns {!Long} Shifted Long\r\n */\r\nLongPrototype.shiftRightUnsigned = function shiftRightUnsigned(numBits) {\r\n if (isLong(numBits))\r\n numBits = numBits.toInt();\r\n numBits &= 63;\r\n if (numBits === 0)\r\n return this;\r\n else {\r\n var high = this.high;\r\n if (numBits < 32) {\r\n var low = this.low;\r\n return fromBits((low >>> numBits) | (high << (32 - numBits)), high >>> numBits, this.unsigned);\r\n } else if (numBits === 32)\r\n return fromBits(high, 0, this.unsigned);\r\n else\r\n return fromBits(high >>> (numBits - 32), 0, this.unsigned);\r\n }\r\n};\r\n\r\n/**\r\n * Returns this Long with bits logically shifted to the right by the given amount. This is an alias of {@link Long#shiftRightUnsigned}.\r\n * @function\r\n * @param {number|!Long} numBits Number of bits\r\n * @returns {!Long} Shifted Long\r\n */\r\nLongPrototype.shru = LongPrototype.shiftRightUnsigned;\r\n\r\n/**\r\n * Returns this Long with bits logically shifted to the right by the given amount. This is an alias of {@link Long#shiftRightUnsigned}.\r\n * @function\r\n * @param {number|!Long} numBits Number of bits\r\n * @returns {!Long} Shifted Long\r\n */\r\nLongPrototype.shr_u = LongPrototype.shiftRightUnsigned;\r\n\r\n/**\r\n * Converts this Long to signed.\r\n * @returns {!Long} Signed long\r\n */\r\nLongPrototype.toSigned = function toSigned() {\r\n if (!this.unsigned)\r\n return this;\r\n return fromBits(this.low, this.high, false);\r\n};\r\n\r\n/**\r\n * Converts this Long to unsigned.\r\n * @returns {!Long} Unsigned long\r\n */\r\nLongPrototype.toUnsigned = function toUnsigned() {\r\n if (this.unsigned)\r\n return this;\r\n return fromBits(this.low, this.high, true);\r\n};\r\n\r\n/**\r\n * Converts this Long to its byte representation.\r\n * @param {boolean=} le Whether little or big endian, defaults to big endian\r\n * @returns {!Array.<number>} Byte representation\r\n */\r\nLongPrototype.toBytes = function toBytes(le) {\r\n return le ? this.toBytesLE() : this.toBytesBE();\r\n};\r\n\r\n/**\r\n * Converts this Long to its little endian byte representation.\r\n * @returns {!Array.<number>} Little endian byte representation\r\n */\r\nLongPrototype.toBytesLE = function toBytesLE() {\r\n var hi = this.high,\r\n lo = this.low;\r\n return [\r\n lo & 0xff,\r\n lo >>> 8 & 0xff,\r\n lo >>> 16 & 0xff,\r\n lo >>> 24 ,\r\n hi & 0xff,\r\n hi >>> 8 & 0xff,\r\n hi >>> 16 & 0xff,\r\n hi >>> 24\r\n ];\r\n};\r\n\r\n/**\r\n * Converts this Long to its big endian byte representation.\r\n * @returns {!Array.<number>} Big endian byte representation\r\n */\r\nLongPrototype.toBytesBE = function toBytesBE() {\r\n var hi = this.high,\r\n lo = this.low;\r\n return [\r\n hi >>> 24 ,\r\n hi >>> 16 & 0xff,\r\n hi >>> 8 & 0xff,\r\n hi & 0xff,\r\n lo >>> 24 ,\r\n lo >>> 16 & 0xff,\r\n lo >>> 8 & 0xff,\r\n lo & 0xff\r\n ];\r\n};\r\n\r\n/**\r\n * Creates a Long from its byte representation.\r\n * @param {!Array.<number>} bytes Byte representation\r\n * @param {boolean=} unsigned Whether unsigned or not, defaults to signed\r\n * @param {boolean=} le Whether little or big endian, defaults to big endian\r\n * @returns {Long} The corresponding Long value\r\n */\r\nLong.fromBytes = function fromBytes(bytes, unsigned, le) {\r\n return le ? Long.fromBytesLE(bytes, unsigned) : Long.fromBytesBE(bytes, unsigned);\r\n};\r\n\r\n/**\r\n * Creates a Long from its little endian byte representation.\r\n * @param {!Array.<number>} bytes Little endian byte representation\r\n * @param {boolean=} unsigned Whether unsigned or not, defaults to signed\r\n * @returns {Long} The corresponding Long value\r\n */\r\nLong.fromBytesLE = function fromBytesLE(bytes, unsigned) {\r\n return new Long(\r\n bytes[0] |\r\n bytes[1] << 8 |\r\n bytes[2] << 16 |\r\n bytes[3] << 24,\r\n bytes[4] |\r\n bytes[5] << 8 |\r\n bytes[6] << 16 |\r\n bytes[7] << 24,\r\n unsigned\r\n );\r\n};\r\n\r\n/**\r\n * Creates a Long from its big endian byte representation.\r\n * @param {!Array.<number>} bytes Big endian byte representation\r\n * @param {boolean=} unsigned Whether unsigned or not, defaults to signed\r\n * @returns {Long} The corresponding Long value\r\n */\r\nLong.fromBytesBE = function fromBytesBE(bytes, unsigned) {\r\n return new Long(\r\n bytes[4] << 24 |\r\n bytes[5] << 16 |\r\n bytes[6] << 8 |\r\n bytes[7],\r\n bytes[0] << 24 |\r\n bytes[1] << 16 |\r\n bytes[2] << 8 |\r\n bytes[3],\r\n unsigned\r\n );\r\n};\r\n\n\n/***/ })\n/******/ ]);\n});\n\n\n// WEBPACK FOOTER //\n// long.js"," \t// The module cache\n \tvar installedModules = {};\n\n \t// The require function\n \tfunction __webpack_require__(moduleId) {\n\n \t\t// Check if module is in cache\n \t\tif(installedModules[moduleId]) {\n \t\t\treturn installedModules[moduleId].exports;\n \t\t}\n \t\t// Create a new module (and put it into the cache)\n \t\tvar module = installedModules[moduleId] = {\n \t\t\ti: moduleId,\n \t\t\tl: false,\n \t\t\texports: {}\n \t\t};\n\n \t\t// Execute the module function\n \t\tmodules[moduleId].call(module.exports, module, module.exports, __webpack_require__);\n\n \t\t// Flag the module as loaded\n \t\tmodule.l = true;\n\n \t\t// Return the exports of the module\n \t\treturn module.exports;\n \t}\n\n\n \t// expose the modules object (__webpack_modules__)\n \t__webpack_require__.m = modules;\n\n \t// expose the module cache\n \t__webpack_require__.c = installedModules;\n\n \t// define getter function for harmony exports\n \t__webpack_require__.d = function(exports, name, getter) {\n \t\tif(!__webpack_require__.o(exports, name)) {\n \t\t\tObject.defineProperty(exports, name, {\n \t\t\t\tconfigurable: false,\n \t\t\t\tenumerable: true,\n \t\t\t\tget: getter\n \t\t\t});\n \t\t}\n \t};\n\n \t// getDefaultExport function for compatibility with non-harmony modules\n \t__webpack_require__.n = function(module) {\n \t\tvar getter = module && module.__esModule ?\n \t\t\tfunction getDefault() { return module['default']; } :\n \t\t\tfunction getModuleExports() { return module; };\n \t\t__webpack_require__.d(getter, 'a', getter);\n \t\treturn getter;\n \t};\n\n \t// Object.prototype.hasOwnProperty.call\n \t__webpack_require__.o = function(object, property) { return Object.prototype.hasOwnProperty.call(object, property); };\n\n \t// __webpack_public_path__\n \t__webpack_require__.p = \"\";\n\n \t// Load entry module and return exports\n \treturn __webpack_require__(__webpack_require__.s = 0);\n\n\n\n// WEBPACK FOOTER //\n// webpack/bootstrap d8921b8c3ad0790b3cc1","module.exports = Long;\r\n\r\n/**\r\n * wasm optimizations, to do native i64 multiplication and divide\r\n */\r\nvar wasm = null;\r\n\r\ntry {\r\n wasm = new WebAssembly.Instance(new WebAssembly.Module(new Uint8Array([\r\n 0, 97, 115, 109, 1, 0, 0, 0, 1, 13, 2, 96, 0, 1, 127, 96, 4, 127, 127, 127, 127, 1, 127, 3, 7, 6, 0, 1, 1, 1, 1, 1, 6, 6, 1, 127, 1, 65, 0, 11, 7, 50, 6, 3, 109, 117, 108, 0, 1, 5, 100, 105, 118, 95, 115, 0, 2, 5, 100, 105, 118, 95, 117, 0, 3, 5, 114, 101, 109, 95, 115, 0, 4, 5, 114, 101, 109, 95, 117, 0, 5, 8, 103, 101, 116, 95, 104, 105, 103, 104, 0, 0, 10, 191, 1, 6, 4, 0, 35, 0, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 126, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 127, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 128, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 129, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 130, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11\r\n ])), {}).exports;\r\n} catch (e) {\r\n // no wasm support :(\r\n}\r\n\r\n/**\r\n * Constructs a 64 bit two's-complement integer, given its low and high 32 bit values as *signed* integers.\r\n * See the from* functions below for more convenient ways of constructing Longs.\r\n * @exports Long\r\n * @class A Long class for representing a 64 bit two's-complement integer value.\r\n * @param {number} low The low (signed) 32 bits of the long\r\n * @param {number} high The high (signed) 32 bits of the long\r\n * @param {boolean=} unsigned Whether unsigned or not, defaults to signed\r\n * @constructor\r\n */\r\nfunction Long(low, high, unsigned) {\r\n\r\n /**\r\n * The low 32 bits as a signed value.\r\n * @type {number}\r\n */\r\n this.low = low | 0;\r\n\r\n /**\r\n * The high 32 bits as a signed value.\r\n * @type {number}\r\n */\r\n this.high = high | 0;\r\n\r\n /**\r\n * Whether unsigned or not.\r\n * @type {boolean}\r\n */\r\n this.unsigned = !!unsigned;\r\n}\r\n\r\n// The internal representation of a long is the two given signed, 32-bit values.\r\n// We use 32-bit pieces because these are the size of integers on which\r\n// Javascript performs bit-operations. For operations like addition and\r\n// multiplication, we split each number into 16 bit pieces, which can easily be\r\n// multiplied within Javascript's floating-point representation without overflow\r\n// or change in sign.\r\n//\r\n// In the algorithms below, we frequently reduce the negative case to the\r\n// positive case by negating the input(s) and then post-processing the result.\r\n// Note that we must ALWAYS check specially whether those values are MIN_VALUE\r\n// (-2^63) because -MIN_VALUE == MIN_VALUE (since 2^63 cannot be represented as\r\n// a positive number, it overflows back into a negative). Not handling this\r\n// case would often result in infinite recursion.\r\n//\r\n// Common constant values ZERO, ONE, NEG_ONE, etc. are defined below the from*\r\n// methods on which they depend.\r\n\r\n/**\r\n * An indicator used to reliably determine if an object is a Long or not.\r\n * @type {boolean}\r\n * @const\r\n * @private\r\n */\r\nLong.prototype.__isLong__;\r\n\r\nObject.defineProperty(Long.prototype, \"__isLong__\", { value: true });\r\n\r\n/**\r\n * @function\r\n * @param {*} obj Object\r\n * @returns {boolean}\r\n * @inner\r\n */\r\nfunction isLong(obj) {\r\n return (obj && obj[\"__isLong__\"]) === true;\r\n}\r\n\r\n/**\r\n * Tests if the specified object is a Long.\r\n * @function\r\n * @param {*} obj Object\r\n * @returns {boolean}\r\n */\r\nLong.isLong = isLong;\r\n\r\n/**\r\n * A cache of the Long representations of small integer values.\r\n * @type {!Object}\r\n * @inner\r\n */\r\nvar INT_CACHE = {};\r\n\r\n/**\r\n * A cache of the Long representations of small unsigned integer values.\r\n * @type {!Object}\r\n * @inner\r\n */\r\nvar UINT_CACHE = {};\r\n\r\n/**\r\n * @param {number} value\r\n * @param {boolean=} unsigned\r\n * @returns {!Long}\r\n * @inner\r\n */\r\nfunction fromInt(value, unsigned) {\r\n var obj, cachedObj, cache;\r\n if (unsigned) {\r\n value >>>= 0;\r\n if (cache = (0 <= value && value < 256)) {\r\n cachedObj = UINT_CACHE[value];\r\n if (cachedObj)\r\n return cachedObj;\r\n }\r\n obj = fromBits(value, (value | 0) < 0 ? -1 : 0, true);\r\n if (cache)\r\n UINT_CACHE[value] = obj;\r\n return obj;\r\n } else {\r\n value |= 0;\r\n if (cache = (-128 <= value && value < 128)) {\r\n cachedObj = INT_CACHE[value];\r\n if (cachedObj)\r\n return cachedObj;\r\n }\r\n obj = fromBits(value, value < 0 ? -1 : 0, false);\r\n if (cache)\r\n INT_CACHE[value] = obj;\r\n return obj;\r\n }\r\n}\r\n\r\n/**\r\n * Returns a Long representing the given 32 bit integer value.\r\n * @function\r\n * @param {number} value The 32 bit integer in question\r\n * @param {boolean=} unsigned Whether unsigned or not, defaults to signed\r\n * @returns {!Long} The corresponding Long value\r\n */\r\nLong.fromInt = fromInt;\r\n\r\n/**\r\n * @param {number} value\r\n * @param {boolean=} unsigned\r\n * @returns {!Long}\r\n * @inner\r\n */\r\nfunction fromNumber(value, unsigned) {\r\n if (isNaN(value))\r\n return unsigned ? UZERO : ZERO;\r\n if (unsigned) {\r\n if (value < 0)\r\n return UZERO;\r\n if (value >= TWO_PWR_64_DBL)\r\n return MAX_UNSIGNED_VALUE;\r\n } else {\r\n if (value <= -TWO_PWR_63_DBL)\r\n return MIN_VALUE;\r\n if (value + 1 >= TWO_PWR_63_DBL)\r\n return MAX_VALUE;\r\n }\r\n if (value < 0)\r\n return fromNumber(-value, unsigned).neg();\r\n return fromBits((value % TWO_PWR_32_DBL) | 0, (value / TWO_PWR_32_DBL) | 0, unsigned);\r\n}\r\n\r\n/**\r\n * Returns a Long representing the given value, provided that it is a finite number. Otherwise, zero is returned.\r\n * @function\r\n * @param {number} value The number in question\r\n * @param {boolean=} unsigned Whether unsigned or not, defaults to signed\r\n * @returns {!Long} The corresponding Long value\r\n */\r\nLong.fromNumber = fromNumber;\r\n\r\n/**\r\n * @param {number} lowBits\r\n * @param {number} highBits\r\n * @param {boolean=} unsigned\r\n * @returns {!Long}\r\n * @inner\r\n */\r\nfunction fromBits(lowBits, highBits, unsigned) {\r\n return new Long(lowBits, highBits, unsigned);\r\n}\r\n\r\n/**\r\n * Returns a Long representing the 64 bit integer that comes by concatenating the given low and high bits. Each is\r\n * assumed to use 32 bits.\r\n * @function\r\n * @param {number} lowBits The low 32 bits\r\n * @param {number} highBits The high 32 bits\r\n * @param {boolean=} unsigned Whether unsigned or not, defaults to signed\r\n * @returns {!Long} The corresponding Long value\r\n */\r\nLong.fromBits = fromBits;\r\n\r\n/**\r\n * @function\r\n * @param {number} base\r\n * @param {number} exponent\r\n * @returns {number}\r\n * @inner\r\n */\r\nvar pow_dbl = Math.pow; // Used 4 times (4*8 to 15+4)\r\n\r\n/**\r\n * @param {string} str\r\n * @param {(boolean|number)=} unsigned\r\n * @param {number=} radix\r\n * @returns {!Long}\r\n * @inner\r\n */\r\nfunction fromString(str, unsigned, radix) {\r\n if (str.length === 0)\r\n throw Error('empty string');\r\n if (str === \"NaN\" || str === \"Infinity\" || str === \"+Infinity\" || str === \"-Infinity\")\r\n return ZERO;\r\n if (typeof unsigned === 'number') {\r\n // For goog.math.long compatibility\r\n radix = unsigned,\r\n unsigned = false;\r\n } else {\r\n unsigned = !! unsigned;\r\n }\r\n radix = radix || 10;\r\n if (radix < 2 || 36 < radix)\r\n throw RangeError('radix');\r\n\r\n var p;\r\n if ((p = str.indexOf('-')) > 0)\r\n throw Error('interior hyphen');\r\n else if (p === 0) {\r\n return fromString(str.substring(1), unsigned, radix).neg();\r\n }\r\n\r\n // Do several (8) digits each time through the loop, so as to\r\n // minimize the calls to the very expensive emulated div.\r\n var radixToPower = fromNumber(pow_dbl(radix, 8));\r\n\r\n var result = ZERO;\r\n for (var i = 0; i < str.length; i += 8) {\r\n var size = Math.min(8, str.length - i),\r\n value = parseInt(str.substring(i, i + size), radix);\r\n if (size < 8) {\r\n var power = fromNumber(pow_dbl(radix, size));\r\n result = result.mul(power).add(fromNumber(value));\r\n } else {\r\n result = result.mul(radixToPower);\r\n result = result.add(fromNumber(value));\r\n }\r\n }\r\n result.unsigned = unsigned;\r\n return result;\r\n}\r\n\r\n/**\r\n * Returns a Long representation of the given string, written using the specified radix.\r\n * @function\r\n * @param {string} str The textual representation of the Long\r\n * @param {(boolean|number)=} unsigned Whether unsigned or not, defaults to signed\r\n * @param {number=} radix The radix in which the text is written (2-36), defaults to 10\r\n * @returns {!Long} The corresponding Long value\r\n */\r\nLong.fromString = fromString;\r\n\r\n/**\r\n * @function\r\n * @param {!Long|number|string|!{low: number, high: number, unsigned: boolean}} val\r\n * @param {boolean=} unsigned\r\n * @returns {!Long}\r\n * @inner\r\n */\r\nfunction fromValue(val, unsigned) {\r\n if (typeof val === 'number')\r\n return fromNumber(val, unsigned);\r\n if (typeof val === 'string')\r\n return fromString(val, unsigned);\r\n // Throws for non-objects, converts non-instanceof Long:\r\n return fromBits(val.low, val.high, typeof unsigned === 'boolean' ? unsigned : val.unsigned);\r\n}\r\n\r\n/**\r\n * Converts the specified value to a Long using the appropriate from* function for its type.\r\n * @function\r\n * @param {!Long|number|string|!{low: number, high: number, unsigned: boolean}} val Value\r\n * @param {boolean=} unsigned Whether unsigned or not, defaults to signed\r\n * @returns {!Long}\r\n */\r\nLong.fromValue = fromValue;\r\n\r\n// NOTE: the compiler should inline these constant values below and then remove these variables, so there should be\r\n// no runtime penalty for these.\r\n\r\n/**\r\n * @type {number}\r\n * @const\r\n * @inner\r\n */\r\nvar TWO_PWR_16_DBL = 1 << 16;\r\n\r\n/**\r\n * @type {number}\r\n * @const\r\n * @inner\r\n */\r\nvar TWO_PWR_24_DBL = 1 << 24;\r\n\r\n/**\r\n * @type {number}\r\n * @const\r\n * @inner\r\n */\r\nvar TWO_PWR_32_DBL = TWO_PWR_16_DBL * TWO_PWR_16_DBL;\r\n\r\n/**\r\n * @type {number}\r\n * @const\r\n * @inner\r\n */\r\nvar TWO_PWR_64_DBL = TWO_PWR_32_DBL * TWO_PWR_32_DBL;\r\n\r\n/**\r\n * @type {number}\r\n * @const\r\n * @inner\r\n */\r\nvar TWO_PWR_63_DBL = TWO_PWR_64_DBL / 2;\r\n\r\n/**\r\n * @type {!Long}\r\n * @const\r\n * @inner\r\n */\r\nvar TWO_PWR_24 = fromInt(TWO_PWR_24_DBL);\r\n\r\n/**\r\n * @type {!Long}\r\n * @inner\r\n */\r\nvar ZERO = fromInt(0);\r\n\r\n/**\r\n * Signed zero.\r\n * @type {!Long}\r\n */\r\nLong.ZERO = ZERO;\r\n\r\n/**\r\n * @type {!Long}\r\n * @inner\r\n */\r\nvar UZERO = fromInt(0, true);\r\n\r\n/**\r\n * Unsigned zero.\r\n * @type {!Long}\r\n */\r\nLong.UZERO = UZERO;\r\n\r\n/**\r\n * @type {!Long}\r\n * @inner\r\n */\r\nvar ONE = fromInt(1);\r\n\r\n/**\r\n * Signed one.\r\n * @type {!Long}\r\n */\r\nLong.ONE = ONE;\r\n\r\n/**\r\n * @type {!Long}\r\n * @inner\r\n */\r\nvar UONE = fromInt(1, true);\r\n\r\n/**\r\n * Unsigned one.\r\n * @type {!Long}\r\n */\r\nLong.UONE = UONE;\r\n\r\n/**\r\n * @type {!Long}\r\n * @inner\r\n */\r\nvar NEG_ONE = fromInt(-1);\r\n\r\n/**\r\n * Signed negative one.\r\n * @type {!Long}\r\n */\r\nLong.NEG_ONE = NEG_ONE;\r\n\r\n/**\r\n * @type {!Long}\r\n * @inner\r\n */\r\nvar MAX_VALUE = fromBits(0xFFFFFFFF|0, 0x7FFFFFFF|0, false);\r\n\r\n/**\r\n * Maximum signed value.\r\n * @type {!Long}\r\n */\r\nLong.MAX_VALUE = MAX_VALUE;\r\n\r\n/**\r\n * @type {!Long}\r\n * @inner\r\n */\r\nvar MAX_UNSIGNED_VALUE = fromBits(0xFFFFFFFF|0, 0xFFFFFFFF|0, true);\r\n\r\n/**\r\n * Maximum unsigned value.\r\n * @type {!Long}\r\n */\r\nLong.MAX_UNSIGNED_VALUE = MAX_UNSIGNED_VALUE;\r\n\r\n/**\r\n * @type {!Long}\r\n * @inner\r\n */\r\nvar MIN_VALUE = fromBits(0, 0x80000000|0, false);\r\n\r\n/**\r\n * Minimum signed value.\r\n * @type {!Long}\r\n */\r\nLong.MIN_VALUE = MIN_VALUE;\r\n\r\n/**\r\n * @alias Long.prototype\r\n * @inner\r\n */\r\nvar LongPrototype = Long.prototype;\r\n\r\n/**\r\n * Converts the Long to a 32 bit integer, assuming it is a 32 bit integer.\r\n * @returns {number}\r\n */\r\nLongPrototype.toInt = function toInt() {\r\n return this.unsigned ? this.low >>> 0 : this.low;\r\n};\r\n\r\n/**\r\n * Converts the Long to a the nearest floating-point representation of this value (double, 53 bit mantissa).\r\n * @returns {number}\r\n */\r\nLongPrototype.toNumber = function toNumber() {\r\n if (this.unsigned)\r\n return ((this.high >>> 0) * TWO_PWR_32_DBL) + (this.low >>> 0);\r\n return this.high * TWO_PWR_32_DBL + (this.low >>> 0);\r\n};\r\n\r\n/**\r\n * Converts the Long to a string written in the specified radix.\r\n * @param {number=} radix Radix (2-36), defaults to 10\r\n * @returns {string}\r\n * @override\r\n * @throws {RangeError} If `radix` is out of range\r\n */\r\nLongPrototype.toString = function toString(radix) {\r\n radix = radix || 10;\r\n if (radix < 2 || 36 < radix)\r\n throw RangeError('radix');\r\n if (this.isZero())\r\n return '0';\r\n if (this.isNegative()) { // Unsigned Longs are never negative\r\n if (this.eq(MIN_VALUE)) {\r\n // We need to change the Long value before it can be negated, so we remove\r\n // the bottom-most digit in this base and then recurse to do the rest.\r\n var radixLong = fromNumber(radix),\r\n div = this.div(radixLong),\r\n rem1 = div.mul(radixLong).sub(this);\r\n return div.toString(radix) + rem1.toInt().toString(radix);\r\n } else\r\n return '-' + this.neg().toString(radix);\r\n }\r\n\r\n // Do several (6) digits each time through the loop, so as to\r\n // minimize the calls to the very expensive emulated div.\r\n var radixToPower = fromNumber(pow_dbl(radix, 6), this.unsigned),\r\n rem = this;\r\n var result = '';\r\n while (true) {\r\n var remDiv = rem.div(radixToPower),\r\n intval = rem.sub(remDiv.mul(radixToPower)).toInt() >>> 0,\r\n digits = intval.toString(radix);\r\n rem = remDiv;\r\n if (rem.isZero())\r\n return digits + result;\r\n else {\r\n while (digits.length < 6)\r\n digits = '0' + digits;\r\n result = '' + digits + result;\r\n }\r\n }\r\n};\r\n\r\n/**\r\n * Gets the high 32 bits as a signed integer.\r\n * @returns {number} Signed high bits\r\n */\r\nLongPrototype.getHighBits = function getHighBits() {\r\n return this.high;\r\n};\r\n\r\n/**\r\n * Gets the high 32 bits as an unsigned integer.\r\n * @returns {number} Unsigned high bits\r\n */\r\nLongPrototype.getHighBitsUnsigned = function getHighBitsUnsigned() {\r\n return this.high >>> 0;\r\n};\r\n\r\n/**\r\n * Gets the low 32 bits as a signed integer.\r\n * @returns {number} Signed low bits\r\n */\r\nLongPrototype.getLowBits = function getLowBits() {\r\n return this.low;\r\n};\r\n\r\n/**\r\n * Gets the low 32 bits as an unsigned integer.\r\n * @returns {number} Unsigned low bits\r\n */\r\nLongPrototype.getLowBitsUnsigned = function getLowBitsUnsigned() {\r\n return this.low >>> 0;\r\n};\r\n\r\n/**\r\n * Gets the number of bits needed to represent the absolute value of this Long.\r\n * @returns {number}\r\n */\r\nLongPrototype.getNumBitsAbs = function getNumBitsAbs() {\r\n if (this.isNegative()) // Unsigned Longs are never negative\r\n return this.eq(MIN_VALUE) ? 64 : this.neg().getNumBitsAbs();\r\n var val = this.high != 0 ? this.high : this.low;\r\n for (var bit = 31; bit > 0; bit--)\r\n if ((val & (1 << bit)) != 0)\r\n break;\r\n return this.high != 0 ? bit + 33 : bit + 1;\r\n};\r\n\r\n/**\r\n * Tests if this Long's value equals zero.\r\n * @returns {boolean}\r\n */\r\nLongPrototype.isZero = function isZero() {\r\n return this.high === 0 && this.low === 0;\r\n};\r\n\r\n/**\r\n * Tests if this Long's value equals zero. This is an alias of {@link Long#isZero}.\r\n * @returns {boolean}\r\n */\r\nLongPrototype.eqz = LongPrototype.isZero;\r\n\r\n/**\r\n * Tests if this Long's value is negative.\r\n * @returns {boolean}\r\n */\r\nLongPrototype.isNegative = function isNegative() {\r\n return !this.unsigned && this.high < 0;\r\n};\r\n\r\n/**\r\n * Tests if this Long's value is positive.\r\n * @returns {boolean}\r\n */\r\nLongPrototype.isPositive = function isPositive() {\r\n return this.unsigned || this.high >= 0;\r\n};\r\n\r\n/**\r\n * Tests if this Long's value is odd.\r\n * @returns {boolean}\r\n */\r\nLongPrototype.isOdd = function isOdd() {\r\n return (this.low & 1) === 1;\r\n};\r\n\r\n/**\r\n * Tests if this Long's value is even.\r\n * @returns {boolean}\r\n */\r\nLongPrototype.isEven = function isEven() {\r\n return (this.low & 1) === 0;\r\n};\r\n\r\n/**\r\n * Tests if this Long's value equals the specified's.\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.equals = function equals(other) {\r\n if (!isLong(other))\r\n other = fromValue(other);\r\n if (this.unsigned !== other.unsigned && (this.high >>> 31) === 1 && (other.high >>> 31) === 1)\r\n return false;\r\n return this.high === other.high && this.low === other.low;\r\n};\r\n\r\n/**\r\n * Tests if this Long's value equals the specified's. This is an alias of {@link Long#equals}.\r\n * @function\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.eq = LongPrototype.equals;\r\n\r\n/**\r\n * Tests if this Long's value differs from the specified's.\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.notEquals = function notEquals(other) {\r\n return !this.eq(/* validates */ other);\r\n};\r\n\r\n/**\r\n * Tests if this Long's value differs from the specified's. This is an alias of {@link Long#notEquals}.\r\n * @function\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.neq = LongPrototype.notEquals;\r\n\r\n/**\r\n * Tests if this Long's value differs from the specified's. This is an alias of {@link Long#notEquals}.\r\n * @function\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.ne = LongPrototype.notEquals;\r\n\r\n/**\r\n * Tests if this Long's value is less than the specified's.\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.lessThan = function lessThan(other) {\r\n return this.comp(/* validates */ other) < 0;\r\n};\r\n\r\n/**\r\n * Tests if this Long's value is less than the specified's. This is an alias of {@link Long#lessThan}.\r\n * @function\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.lt = LongPrototype.lessThan;\r\n\r\n/**\r\n * Tests if this Long's value is less than or equal the specified's.\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.lessThanOrEqual = function lessThanOrEqual(other) {\r\n return this.comp(/* validates */ other) <= 0;\r\n};\r\n\r\n/**\r\n * Tests if this Long's value is less than or equal the specified's. This is an alias of {@link Long#lessThanOrEqual}.\r\n * @function\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.lte = LongPrototype.lessThanOrEqual;\r\n\r\n/**\r\n * Tests if this Long's value is less than or equal the specified's. This is an alias of {@link Long#lessThanOrEqual}.\r\n * @function\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.le = LongPrototype.lessThanOrEqual;\r\n\r\n/**\r\n * Tests if this Long's value is greater than the specified's.\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.greaterThan = function greaterThan(other) {\r\n return this.comp(/* validates */ other) > 0;\r\n};\r\n\r\n/**\r\n * Tests if this Long's value is greater than the specified's. This is an alias of {@link Long#greaterThan}.\r\n * @function\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.gt = LongPrototype.greaterThan;\r\n\r\n/**\r\n * Tests if this Long's value is greater than or equal the specified's.\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.greaterThanOrEqual = function greaterThanOrEqual(other) {\r\n return this.comp(/* validates */ other) >= 0;\r\n};\r\n\r\n/**\r\n * Tests if this Long's value is greater than or equal the specified's. This is an alias of {@link Long#greaterThanOrEqual}.\r\n * @function\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.gte = LongPrototype.greaterThanOrEqual;\r\n\r\n/**\r\n * Tests if this Long's value is greater than or equal the specified's. This is an alias of {@link Long#greaterThanOrEqual}.\r\n * @function\r\n * @param {!Long|number|string} other Other value\r\n * @returns {boolean}\r\n */\r\nLongPrototype.ge = LongPrototype.greaterThanOrEqual;\r\n\r\n/**\r\n * Compares this Long's value with the specified's.\r\n * @param {!Long|number|string} other Other value\r\n * @returns {number} 0 if they are the same, 1 if the this is greater and -1\r\n * if the given one is greater\r\n */\r\nLongPrototype.compare = function compare(other) {\r\n if (!isLong(other))\r\n other = fromValue(other);\r\n if (this.eq(other))\r\n return 0;\r\n var thisNeg = this.isNegative(),\r\n otherNeg = other.isNegative();\r\n if (thisNeg && !otherNeg)\r\n return -1;\r\n if (!thisNeg && otherNeg)\r\n return 1;\r\n // At this point the sign bits are the same\r\n if (!this.unsigned)\r\n return this.sub(other).isNegative() ? -1 : 1;\r\n // Both are positive if at least one is unsigned\r\n return (other.high >>> 0) > (this.high >>> 0) || (other.high === this.high && (other.low >>> 0) > (this.low >>> 0)) ? -1 : 1;\r\n};\r\n\r\n/**\r\n * Compares this Long's value with the specified's. This is an alias of {@link Long#compare}.\r\n * @function\r\n * @param {!Long|number|string} other Other value\r\n * @returns {number} 0 if they are the same, 1 if the this is greater and -1\r\n * if the given one is greater\r\n */\r\nLongPrototype.comp = LongPrototype.compare;\r\n\r\n/**\r\n * Negates this Long's value.\r\n * @returns {!Long} Negated Long\r\n */\r\nLongPrototype.negate = function negate() {\r\n if (!this.unsigned && this.eq(MIN_VALUE))\r\n return MIN_VALUE;\r\n return this.not().add(ONE);\r\n};\r\n\r\n/**\r\n * Negates this Long's value. This is an alias of {@link Long#negate}.\r\n * @function\r\n * @returns {!Long} Negated Long\r\n */\r\nLongPrototype.neg = LongPrototype.negate;\r\n\r\n/**\r\n * Returns the sum of this and the specified Long.\r\n * @param {!Long|number|string} addend Addend\r\n * @returns {!Long} Sum\r\n */\r\nLongPrototype.add = function add(addend) {\r\n if (!isLong(addend))\r\n addend = fromValue(addend);\r\n\r\n // Divide each number into 4 chunks of 16 bits, and then sum the chunks.\r\n\r\n var a48 = this.high >>> 16;\r\n var a32 = this.high & 0xFFFF;\r\n var a16 = this.low >>> 16;\r\n var a00 = this.low & 0xFFFF;\r\n\r\n var b48 = addend.high >>> 16;\r\n var b32 = addend.high & 0xFFFF;\r\n var b16 = addend.low >>> 16;\r\n var b00 = addend.low & 0xFFFF;\r\n\r\n var c48 = 0, c32 = 0, c16 = 0, c00 = 0;\r\n c00 += a00 + b00;\r\n c16 += c00 >>> 16;\r\n c00 &= 0xFFFF;\r\n c16 += a16 + b16;\r\n c32 += c16 >>> 16;\r\n c16 &= 0xFFFF;\r\n c32 += a32 + b32;\r\n c48 += c32 >>> 16;\r\n c32 &= 0xFFFF;\r\n c48 += a48 + b48;\r\n c48 &= 0xFFFF;\r\n return fromBits((c16 << 16) | c00, (c48 << 16) | c32, this.unsigned);\r\n};\r\n\r\n/**\r\n * Returns the difference of this and the specified Long.\r\n * @param {!Long|number|string} subtrahend Subtrahend\r\n * @returns {!Long} Difference\r\n */\r\nLongPrototype.subtract = function subtract(subtrahend) {\r\n if (!isLong(subtrahend))\r\n subtrahend = fromValue(subtrahend);\r\n return this.add(subtrahend.neg());\r\n};\r\n\r\n/**\r\n * Returns the difference of this and the specified Long. This is an alias of {@link Long#subtract}.\r\n * @function\r\n * @param {!Long|number|string} subtrahend Subtrahend\r\n * @returns {!Long} Difference\r\n */\r\nLongPrototype.sub = LongPrototype.subtract;\r\n\r\n/**\r\n * Returns the product of this and the specified Long.\r\n * @param {!Long|number|string} multiplier Multiplier\r\n * @returns {!Long} Product\r\n */\r\nLongPrototype.multiply = function multiply(multiplier) {\r\n if (this.isZero())\r\n return ZERO;\r\n if (!isLong(multiplier))\r\n multiplier = fromValue(multiplier);\r\n\r\n // use wasm support if present\r\n if (wasm) {\r\n var low = wasm.mul(this.low,\r\n this.high,\r\n multiplier.low,\r\n multiplier.high);\r\n return fromBits(low, wasm.get_high(), this.unsigned);\r\n }\r\n\r\n if (multiplier.isZero())\r\n return ZERO;\r\n if (this.eq(MIN_VALUE))\r\n return multiplier.isOdd() ? MIN_VALUE : ZERO;\r\n if (multiplier.eq(MIN_VALUE))\r\n return this.isOdd() ? MIN_VALUE : ZERO;\r\n\r\n if (this.isNegative()) {\r\n if (multiplier.isNegative())\r\n return this.neg().mul(multiplier.neg());\r\n else\r\n return this.neg().mul(multiplier).neg();\r\n } else if (multiplier.isNegative())\r\n return this.mul(multiplier.neg()).neg();\r\n\r\n // If both longs are small, use float multiplication\r\n if (this.lt(TWO_PWR_24) && multiplier.lt(TWO_PWR_24))\r\n return fromNumber(this.toNumber() * multiplier.toNumber(), this.unsigned);\r\n\r\n // Divide each long into 4 chunks of 16 bits, and then add up 4x4 products.\r\n // We can skip products that would overflow.\r\n\r\n var a48 = this.high >>> 16;\r\n var a32 = this.high & 0xFFFF;\r\n var a16 = this.low >>> 16;\r\n var a00 = this.low & 0xFFFF;\r\n\r\n var b48 = multiplier.high >>> 16;\r\n var b32 = multiplier.high & 0xFFFF;\r\n var b16 = multiplier.low >>> 16;\r\n var b00 = multiplier.low & 0xFFFF;\r\n\r\n var c48 = 0, c32 = 0, c16 = 0, c00 = 0;\r\n c00 += a00 * b00;\r\n c16 += c00 >>> 16;\r\n c00 &= 0xFFFF;\r\n c16 += a16 * b00;\r\n c32 += c16 >>> 16;\r\n c16 &= 0xFFFF;\r\n c16 += a00 * b16;\r\n c32 += c16 >>> 16;\r\n c16 &= 0xFFFF;\r\n c32 += a32 * b00;\r\n c48 += c32 >>> 16;\r\n c32 &= 0xFFFF;\r\n c32 += a16 * b16;\r\n c48 += c32 >>> 16;\r\n c32 &= 0xFFFF;\r\n c32 += a00 * b32;\r\n c48 += c32 >>> 16;\r\n c32 &= 0xFFFF;\r\n c48 += a48 * b00 + a32 * b16 + a16 * b32 + a00 * b48;\r\n c48 &= 0xFFFF;\r\n return fromBits((c16 << 16) | c00, (c48 << 16) | c32, this.unsigned);\r\n};\r\n\r\n/**\r\n * Returns the product of this and the specified Long. This is an alias of {@link Long#multiply}.\r\n * @function\r\n * @param {!Long|number|string} multiplier Multiplier\r\n * @returns {!Long} Product\r\n */\r\nLongPrototype.mul = LongPrototype.multiply;\r\n\r\n/**\r\n * Returns this Long divided by the specified. The result is signed if this Long is signed or\r\n * unsigned if this Long is unsigned.\r\n * @param {!Long|number|string} divisor Divisor\r\n * @returns {!Long} Quotient\r\n */\r\nLongPrototype.divide = function divide(divisor) {\r\n if (!isLong(divisor))\r\n divisor = fromValue(divisor);\r\n if (divisor.isZero())\r\n throw Error('division by zero');\r\n\r\n // use wasm support if present\r\n if (wasm) {\r\n // guard against signed division overflow: the largest\r\n // negative number / -1 would be 1 larger than the largest\r\n // positive number, due to two's complement.\r\n if (!this.unsigned &&\r\n this.high === -0x80000000 &&\r\n divisor.low === -1 && divisor.high === -1) {\r\n // be consistent with non-wasm code path\r\n return this;\r\n }\r\n var low = (this.unsigned ? wasm.div_u : wasm.div_s)(\r\n this.low,\r\n this.high,\r\n divisor.low,\r\n divisor.high\r\n );\r\n return fromBits(low, wasm.get_high(), this.unsigned);\r\n }\r\n\r\n if (this.isZero())\r\n return this.unsigned ? UZERO : ZERO;\r\n var approx, rem, res;\r\n if (!this.unsigned) {\r\n // This section is only relevant for signed longs and is derived from the\r\n // closure library as a whole.\r\n if (this.eq(MIN_VALUE)) {\r\n if (divisor.eq(ONE) || divisor.eq(NEG_ONE))\r\n return MIN_VALUE; // recall that -MIN_VALUE == MIN_VALUE\r\n else if (divisor.eq(MIN_VALUE))\r\n return ONE;\r\n else {\r\n // At this point, we have |other| >= 2, so |this/other| < |MIN_VALUE|.\r\n var halfThis = this.shr(1);\r\n approx = halfThis.div(divisor).shl(1);\r\n if (approx.eq(ZERO)) {\r\n return divisor.isNegative() ? ONE : NEG_ONE;\r\n } else {\r\n rem = this.sub(divisor.mul(approx));\r\n res = approx.add(rem.div(divisor));\r\n return res;\r\n }\r\n }\r\n } else if (divisor.eq(MIN_VALUE))\r\n return this.unsigned ? UZERO : ZERO;\r\n if (this.isNegative()) {\r\n if (divisor.isNegative())\r\n return this.neg().div(divisor.neg());\r\n return this.neg().div(divisor).neg();\r\n } else if (divisor.isNegative())\r\n return this.div(divisor.neg()).neg();\r\n res = ZERO;\r\n } else {\r\n // The algorithm below has not been made for unsigned longs. It's therefore\r\n // required to take special care of the MSB prior to running it.\r\n if (!divisor.unsigned)\r\n divisor = divisor.toUnsigned();\r\n if (divisor.gt(this))\r\n return UZERO;\r\n if (divisor.gt(this.shru(1))) // 15 >>> 1 = 7 ; with divisor = 8 ; true\r\n return UONE;\r\n res = UZERO;\r\n }\r\n\r\n // Repeat the following until the remainder is less than other: find a\r\n // floating-point that approximates remainder / other *from below*, add this\r\n // into the result, and subtract it from the remainder. It is critical that\r\n // the approximate value is less than or equal to the real value so that the\r\n // remainder never becomes negative.\r\n rem = this;\r\n while (rem.gte(divisor)) {\r\n // Approximate the result of division. This may be a little greater or\r\n // smaller than the actual value.\r\n approx = Math.max(1, Math.floor(rem.toNumber() / divisor.toNumber()));\r\n\r\n // We will tweak the approximate result by changing it in the 48-th digit or\r\n // the smallest non-fractional digit, whichever is larger.\r\n var log2 = Math.ceil(Math.log(approx) / Math.LN2),\r\n delta = (log2 <= 48) ? 1 : pow_dbl(2, log2 - 48),\r\n\r\n // Decrease the approximation until it is smaller than the remainder. Note\r\n // that if it is too large, the product overflows and is negative.\r\n approxRes = fromNumber(approx),\r\n approxRem = approxRes.mul(divisor);\r\n while (approxRem.isNegative() || approxRem.gt(rem)) {\r\n approx -= delta;\r\n approxRes = fromNumber(approx, this.unsigned);\r\n approxRem = approxRes.mul(divisor);\r\n }\r\n\r\n // We know the answer can't be zero... and actually, zero would cause\r\n // infinite recursion since we would make no progress.\r\n if (approxRes.isZero())\r\n approxRes = ONE;\r\n\r\n res = res.add(approxRes);\r\n rem = rem.sub(approxRem);\r\n }\r\n return res;\r\n};\r\n\r\n/**\r\n * Returns this Long divided by the specified. This is an alias of {@link Long#divide}.\r\n * @function\r\n * @param {!Long|number|string} divisor Divisor\r\n * @returns {!Long} Quotient\r\n */\r\nLongPrototype.div = LongPrototype.divide;\r\n\r\n/**\r\n * Returns this Long modulo the specified.\r\n * @param {!Long|number|string} divisor Divisor\r\n * @returns {!Long} Remainder\r\n */\r\nLongPrototype.modulo = function modulo(divisor) {\r\n if (!isLong(divisor))\r\n divisor = fromValue(divisor);\r\n\r\n // use wasm support if present\r\n if (wasm) {\r\n var low = (this.unsigned ? wasm.rem_u : wasm.rem_s)(\r\n this.low,\r\n this.high,\r\n divisor.low,\r\n divisor.high\r\n );\r\n return fromBits(low, wasm.get_high(), this.unsigned);\r\n }\r\n\r\n return this.sub(this.div(divisor).mul(divisor));\r\n};\r\n\r\n/**\r\n * Returns this Long modulo the specified. This is an alias of {@link Long#modulo}.\r\n * @function\r\n * @param {!Long|number|string} divisor Divisor\r\n * @returns {!Long} Remainder\r\n */\r\nLongPrototype.mod = LongPrototype.modulo;\r\n\r\n/**\r\n * Returns this Long modulo the specified. This is an alias of {@link Long#modulo}.\r\n * @function\r\n * @param {!Long|number|string} divisor Divisor\r\n * @returns {!Long} Remainder\r\n */\r\nLongPrototype.rem = LongPrototype.modulo;\r\n\r\n/**\r\n * Returns the bitwise NOT of this Long.\r\n * @returns {!Long}\r\n */\r\nLongPrototype.not = function not() {\r\n return fromBits(~this.low, ~this.high, this.unsigned);\r\n};\r\n\r\n/**\r\n * Returns the bitwise AND of this Long and the specified.\r\n * @param {!Long|number|string} other Other Long\r\n * @returns {!Long}\r\n */\r\nLongPrototype.and = function and(other) {\r\n if (!isLong(other))\r\n other = fromValue(other);\r\n return fromBits(this.low & other.low, this.high & other.high, this.unsigned);\r\n};\r\n\r\n/**\r\n * Returns the bitwise OR of this Long and the specified.\r\n * @param {!Long|number|string} other Other Long\r\n * @returns {!Long}\r\n */\r\nLongPrototype.or = function or(other) {\r\n if (!isLong(other))\r\n other = fromValue(other);\r\n return fromBits(this.low | other.low, this.high | other.high, this.unsigned);\r\n};\r\n\r\n/**\r\n * Returns the bitwise XOR of this Long and the given one.\r\n * @param {!Long|number|string} other Other Long\r\n * @returns {!Long}\r\n */\r\nLongPrototype.xor = function xor(other) {\r\n if (!isLong(other))\r\n other = fromValue(other);\r\n return fromBits(this.low ^ other.low, this.high ^ other.high, this.unsigned);\r\n};\r\n\r\n/**\r\n * Returns this Long with bits shifted to the left by the given amount.\r\n * @param {number|!Long} numBits Number of bits\r\n * @returns {!Long} Shifted Long\r\n */\r\nLongPrototype.shiftLeft = function shiftLeft(numBits) {\r\n if (isLong(numBits))\r\n numBits = numBits.toInt();\r\n if ((numBits &= 63) === 0)\r\n return this;\r\n else if (numBits < 32)\r\n return fromBits(this.low << numBits, (this.high << numBits) | (this.low >>> (32 - numBits)), this.unsigned);\r\n else\r\n return fromBits(0, this.low << (numBits - 32), this.unsigned);\r\n};\r\n\r\n/**\r\n * Returns this Long with bits shifted to the left by the given amount. This is an alias of {@link Long#shiftLeft}.\r\n * @function\r\n * @param {number|!Long} numBits Number of bits\r\n * @returns {!Long} Shifted Long\r\n */\r\nLongPrototype.shl = LongPrototype.shiftLeft;\r\n\r\n/**\r\n * Returns this Long with bits arithmetically shifted to the right by the given amount.\r\n * @param {number|!Long} numBits Number of bits\r\n * @returns {!Long} Shifted Long\r\n */\r\nLongPrototype.shiftRight = function shiftRight(numBits) {\r\n if (isLong(numBits))\r\n numBits = numBits.toInt();\r\n if ((numBits &= 63) === 0)\r\n return this;\r\n else if (numBits < 32)\r\n return fromBits((this.low >>> numBits) | (this.high << (32 - numBits)), this.high >> numBits, this.unsigned);\r\n else\r\n return fromBits(this.high >> (numBits - 32), this.high >= 0 ? 0 : -1, this.unsigned);\r\n};\r\n\r\n/**\r\n * Returns this Long with bits arithmetically shifted to the right by the given amount. This is an alias of {@link Long#shiftRight}.\r\n * @function\r\n * @param {number|!Long} numBits Number of bits\r\n * @returns {!Long} Shifted Long\r\n */\r\nLongPrototype.shr = LongPrototype.shiftRight;\r\n\r\n/**\r\n * Returns this Long with bits logically shifted to the right by the given amount.\r\n * @param {number|!Long} numBits Number of bits\r\n * @returns {!Long} Shifted Long\r\n */\r\nLongPrototype.shiftRightUnsigned = function shiftRightUnsigned(numBits) {\r\n if (isLong(numBits))\r\n numBits = numBits.toInt();\r\n numBits &= 63;\r\n if (numBits === 0)\r\n return this;\r\n else {\r\n var high = this.high;\r\n if (numBits < 32) {\r\n var low = this.low;\r\n return fromBits((low >>> numBits) | (high << (32 - numBits)), high >>> numBits, this.unsigned);\r\n } else if (numBits === 32)\r\n return fromBits(high, 0, this.unsigned);\r\n else\r\n return fromBits(high >>> (numBits - 32), 0, this.unsigned);\r\n }\r\n};\r\n\r\n/**\r\n * Returns this Long with bits logically shifted to the right by the given amount. This is an alias of {@link Long#shiftRightUnsigned}.\r\n * @function\r\n * @param {number|!Long} numBits Number of bits\r\n * @returns {!Long} Shifted Long\r\n */\r\nLongPrototype.shru = LongPrototype.shiftRightUnsigned;\r\n\r\n/**\r\n * Returns this Long with bits logically shifted to the right by the given amount. This is an alias of {@link Long#shiftRightUnsigned}.\r\n * @function\r\n * @param {number|!Long} numBits Number of bits\r\n * @returns {!Long} Shifted Long\r\n */\r\nLongPrototype.shr_u = LongPrototype.shiftRightUnsigned;\r\n\r\n/**\r\n * Converts this Long to signed.\r\n * @returns {!Long} Signed long\r\n */\r\nLongPrototype.toSigned = function toSigned() {\r\n if (!this.unsigned)\r\n return this;\r\n return fromBits(this.low, this.high, false);\r\n};\r\n\r\n/**\r\n * Converts this Long to unsigned.\r\n * @returns {!Long} Unsigned long\r\n */\r\nLongPrototype.toUnsigned = function toUnsigned() {\r\n if (this.unsigned)\r\n return this;\r\n return fromBits(this.low, this.high, true);\r\n};\r\n\r\n/**\r\n * Converts this Long to its byte representation.\r\n * @param {boolean=} le Whether little or big endian, defaults to big endian\r\n * @returns {!Array.<number>} Byte representation\r\n */\r\nLongPrototype.toBytes = function toBytes(le) {\r\n return le ? this.toBytesLE() : this.toBytesBE();\r\n};\r\n\r\n/**\r\n * Converts this Long to its little endian byte representation.\r\n * @returns {!Array.<number>} Little endian byte representation\r\n */\r\nLongPrototype.toBytesLE = function toBytesLE() {\r\n var hi = this.high,\r\n lo = this.low;\r\n return [\r\n lo & 0xff,\r\n lo >>> 8 & 0xff,\r\n lo >>> 16 & 0xff,\r\n lo >>> 24 ,\r\n hi & 0xff,\r\n hi >>> 8 & 0xff,\r\n hi >>> 16 & 0xff,\r\n hi >>> 24\r\n ];\r\n};\r\n\r\n/**\r\n * Converts this Long to its big endian byte representation.\r\n * @returns {!Array.<number>} Big endian byte representation\r\n */\r\nLongPrototype.toBytesBE = function toBytesBE() {\r\n var hi = this.high,\r\n lo = this.low;\r\n return [\r\n hi >>> 24 ,\r\n hi >>> 16 & 0xff,\r\n hi >>> 8 & 0xff,\r\n hi & 0xff,\r\n lo >>> 24 ,\r\n lo >>> 16 & 0xff,\r\n lo >>> 8 & 0xff,\r\n lo & 0xff\r\n ];\r\n};\r\n\r\n/**\r\n * Creates a Long from its byte representation.\r\n * @param {!Array.<number>} bytes Byte representation\r\n * @param {boolean=} unsigned Whether unsigned or not, defaults to signed\r\n * @param {boolean=} le Whether little or big endian, defaults to big endian\r\n * @returns {Long} The corresponding Long value\r\n */\r\nLong.fromBytes = function fromBytes(bytes, unsigned, le) {\r\n return le ? Long.fromBytesLE(bytes, unsigned) : Long.fromBytesBE(bytes, unsigned);\r\n};\r\n\r\n/**\r\n * Creates a Long from its little endian byte representation.\r\n * @param {!Array.<number>} bytes Little endian byte representation\r\n * @param {boolean=} unsigned Whether unsigned or not, defaults to signed\r\n * @returns {Long} The corresponding Long value\r\n */\r\nLong.fromBytesLE = function fromBytesLE(bytes, unsigned) {\r\n return new Long(\r\n bytes[0] |\r\n bytes[1] << 8 |\r\n bytes[2] << 16 |\r\n bytes[3] << 24,\r\n bytes[4] |\r\n bytes[5] << 8 |\r\n bytes[6] << 16 |\r\n bytes[7] << 24,\r\n unsigned\r\n );\r\n};\r\n\r\n/**\r\n * Creates a Long from its big endian byte representation.\r\n * @param {!Array.<number>} bytes Big endian byte representation\r\n * @param {boolean=} unsigned Whether unsigned or not, defaults to signed\r\n * @returns {Long} The corresponding Long value\r\n */\r\nLong.fromBytesBE = function fromBytesBE(bytes, unsigned) {\r\n return new Long(\r\n bytes[4] << 24 |\r\n bytes[5] << 16 |\r\n bytes[6] << 8 |\r\n bytes[7],\r\n bytes[0] << 24 |\r\n bytes[1] << 16 |\r\n bytes[2] << 8 |\r\n bytes[3],\r\n unsigned\r\n );\r\n};\r\n\n\n\n//////////////////\n// WEBPACK FOOTER\n// ./src/long.js\n// module id = 0\n// module chunks = 0"],"sourceRoot":""} apollo-server-demo/node_modules/long/dist/long.js 0000644 0001750 0000144 00000023127 13235424637 021644 0 ustar andreh users !function(t,i){"object"==typeof exports&&"object"==typeof module?module.exports=i():"function"==typeof define&&define.amd?define([],i):"object"==typeof exports?exports.Long=i():t.Long=i()}("undefined"!=typeof self?self:this,function(){return function(t){function i(e){if(n[e])return n[e].exports;var r=n[e]={i:e,l:!1,exports:{}};return t[e].call(r.exports,r,r.exports,i),r.l=!0,r.exports}var n={};return i.m=t,i.c=n,i.d=function(t,n,e){i.o(t,n)||Object.defineProperty(t,n,{configurable:!1,enumerable:!0,get:e})},i.n=function(t){var n=t&&t.__esModule?function(){return t.default}:function(){return t};return i.d(n,"a",n),n},i.o=function(t,i){return Object.prototype.hasOwnProperty.call(t,i)},i.p="",i(i.s=0)}([function(t,i){function n(t,i,n){this.low=0|t,this.high=0|i,this.unsigned=!!n}function e(t){return!0===(t&&t.__isLong__)}function r(t,i){var n,e,r;return i?(t>>>=0,(r=0<=t&&t<256)&&(e=l[t])?e:(n=h(t,(0|t)<0?-1:0,!0),r&&(l[t]=n),n)):(t|=0,(r=-128<=t&&t<128)&&(e=f[t])?e:(n=h(t,t<0?-1:0,!1),r&&(f[t]=n),n))}function s(t,i){if(isNaN(t))return i?p:m;if(i){if(t<0)return p;if(t>=c)return q}else{if(t<=-v)return _;if(t+1>=v)return E}return t<0?s(-t,i).neg():h(t%d|0,t/d|0,i)}function h(t,i,e){return new n(t,i,e)}function u(t,i,n){if(0===t.length)throw Error("empty string");if("NaN"===t||"Infinity"===t||"+Infinity"===t||"-Infinity"===t)return m;if("number"==typeof i?(n=i,i=!1):i=!!i,(n=n||10)<2||36<n)throw RangeError("radix");var e;if((e=t.indexOf("-"))>0)throw Error("interior hyphen");if(0===e)return u(t.substring(1),i,n).neg();for(var r=s(a(n,8)),h=m,o=0;o<t.length;o+=8){var g=Math.min(8,t.length-o),f=parseInt(t.substring(o,o+g),n);if(g<8){var l=s(a(n,g));h=h.mul(l).add(s(f))}else h=h.mul(r),h=h.add(s(f))}return h.unsigned=i,h}function o(t,i){return"number"==typeof t?s(t,i):"string"==typeof t?u(t,i):h(t.low,t.high,"boolean"==typeof i?i:t.unsigned)}t.exports=n;var g=null;try{g=new WebAssembly.Instance(new WebAssembly.Module(new Uint8Array([0,97,115,109,1,0,0,0,1,13,2,96,0,1,127,96,4,127,127,127,127,1,127,3,7,6,0,1,1,1,1,1,6,6,1,127,1,65,0,11,7,50,6,3,109,117,108,0,1,5,100,105,118,95,115,0,2,5,100,105,118,95,117,0,3,5,114,101,109,95,115,0,4,5,114,101,109,95,117,0,5,8,103,101,116,95,104,105,103,104,0,0,10,191,1,6,4,0,35,0,11,36,1,1,126,32,0,173,32,1,173,66,32,134,132,32,2,173,32,3,173,66,32,134,132,126,34,4,66,32,135,167,36,0,32,4,167,11,36,1,1,126,32,0,173,32,1,173,66,32,134,132,32,2,173,32,3,173,66,32,134,132,127,34,4,66,32,135,167,36,0,32,4,167,11,36,1,1,126,32,0,173,32,1,173,66,32,134,132,32,2,173,32,3,173,66,32,134,132,128,34,4,66,32,135,167,36,0,32,4,167,11,36,1,1,126,32,0,173,32,1,173,66,32,134,132,32,2,173,32,3,173,66,32,134,132,129,34,4,66,32,135,167,36,0,32,4,167,11,36,1,1,126,32,0,173,32,1,173,66,32,134,132,32,2,173,32,3,173,66,32,134,132,130,34,4,66,32,135,167,36,0,32,4,167,11])),{}).exports}catch(t){}n.prototype.__isLong__,Object.defineProperty(n.prototype,"__isLong__",{value:!0}),n.isLong=e;var f={},l={};n.fromInt=r,n.fromNumber=s,n.fromBits=h;var a=Math.pow;n.fromString=u,n.fromValue=o;var d=4294967296,c=d*d,v=c/2,w=r(1<<24),m=r(0);n.ZERO=m;var p=r(0,!0);n.UZERO=p;var y=r(1);n.ONE=y;var b=r(1,!0);n.UONE=b;var N=r(-1);n.NEG_ONE=N;var E=h(-1,2147483647,!1);n.MAX_VALUE=E;var q=h(-1,-1,!0);n.MAX_UNSIGNED_VALUE=q;var _=h(0,-2147483648,!1);n.MIN_VALUE=_;var B=n.prototype;B.toInt=function(){return this.unsigned?this.low>>>0:this.low},B.toNumber=function(){return this.unsigned?(this.high>>>0)*d+(this.low>>>0):this.high*d+(this.low>>>0)},B.toString=function(t){if((t=t||10)<2||36<t)throw RangeError("radix");if(this.isZero())return"0";if(this.isNegative()){if(this.eq(_)){var i=s(t),n=this.div(i),e=n.mul(i).sub(this);return n.toString(t)+e.toInt().toString(t)}return"-"+this.neg().toString(t)}for(var r=s(a(t,6),this.unsigned),h=this,u="";;){var o=h.div(r),g=h.sub(o.mul(r)).toInt()>>>0,f=g.toString(t);if(h=o,h.isZero())return f+u;for(;f.length<6;)f="0"+f;u=""+f+u}},B.getHighBits=function(){return this.high},B.getHighBitsUnsigned=function(){return this.high>>>0},B.getLowBits=function(){return this.low},B.getLowBitsUnsigned=function(){return this.low>>>0},B.getNumBitsAbs=function(){if(this.isNegative())return this.eq(_)?64:this.neg().getNumBitsAbs();for(var t=0!=this.high?this.high:this.low,i=31;i>0&&0==(t&1<<i);i--);return 0!=this.high?i+33:i+1},B.isZero=function(){return 0===this.high&&0===this.low},B.eqz=B.isZero,B.isNegative=function(){return!this.unsigned&&this.high<0},B.isPositive=function(){return this.unsigned||this.high>=0},B.isOdd=function(){return 1==(1&this.low)},B.isEven=function(){return 0==(1&this.low)},B.equals=function(t){return e(t)||(t=o(t)),(this.unsigned===t.unsigned||this.high>>>31!=1||t.high>>>31!=1)&&(this.high===t.high&&this.low===t.low)},B.eq=B.equals,B.notEquals=function(t){return!this.eq(t)},B.neq=B.notEquals,B.ne=B.notEquals,B.lessThan=function(t){return this.comp(t)<0},B.lt=B.lessThan,B.lessThanOrEqual=function(t){return this.comp(t)<=0},B.lte=B.lessThanOrEqual,B.le=B.lessThanOrEqual,B.greaterThan=function(t){return this.comp(t)>0},B.gt=B.greaterThan,B.greaterThanOrEqual=function(t){return this.comp(t)>=0},B.gte=B.greaterThanOrEqual,B.ge=B.greaterThanOrEqual,B.compare=function(t){if(e(t)||(t=o(t)),this.eq(t))return 0;var i=this.isNegative(),n=t.isNegative();return i&&!n?-1:!i&&n?1:this.unsigned?t.high>>>0>this.high>>>0||t.high===this.high&&t.low>>>0>this.low>>>0?-1:1:this.sub(t).isNegative()?-1:1},B.comp=B.compare,B.negate=function(){return!this.unsigned&&this.eq(_)?_:this.not().add(y)},B.neg=B.negate,B.add=function(t){e(t)||(t=o(t));var i=this.high>>>16,n=65535&this.high,r=this.low>>>16,s=65535&this.low,u=t.high>>>16,g=65535&t.high,f=t.low>>>16,l=65535&t.low,a=0,d=0,c=0,v=0;return v+=s+l,c+=v>>>16,v&=65535,c+=r+f,d+=c>>>16,c&=65535,d+=n+g,a+=d>>>16,d&=65535,a+=i+u,a&=65535,h(c<<16|v,a<<16|d,this.unsigned)},B.subtract=function(t){return e(t)||(t=o(t)),this.add(t.neg())},B.sub=B.subtract,B.multiply=function(t){if(this.isZero())return m;if(e(t)||(t=o(t)),g){return h(g.mul(this.low,this.high,t.low,t.high),g.get_high(),this.unsigned)}if(t.isZero())return m;if(this.eq(_))return t.isOdd()?_:m;if(t.eq(_))return this.isOdd()?_:m;if(this.isNegative())return t.isNegative()?this.neg().mul(t.neg()):this.neg().mul(t).neg();if(t.isNegative())return this.mul(t.neg()).neg();if(this.lt(w)&&t.lt(w))return s(this.toNumber()*t.toNumber(),this.unsigned);var i=this.high>>>16,n=65535&this.high,r=this.low>>>16,u=65535&this.low,f=t.high>>>16,l=65535&t.high,a=t.low>>>16,d=65535&t.low,c=0,v=0,p=0,y=0;return y+=u*d,p+=y>>>16,y&=65535,p+=r*d,v+=p>>>16,p&=65535,p+=u*a,v+=p>>>16,p&=65535,v+=n*d,c+=v>>>16,v&=65535,v+=r*a,c+=v>>>16,v&=65535,v+=u*l,c+=v>>>16,v&=65535,c+=i*d+n*a+r*l+u*f,c&=65535,h(p<<16|y,c<<16|v,this.unsigned)},B.mul=B.multiply,B.divide=function(t){if(e(t)||(t=o(t)),t.isZero())throw Error("division by zero");if(g){if(!this.unsigned&&-2147483648===this.high&&-1===t.low&&-1===t.high)return this;return h((this.unsigned?g.div_u:g.div_s)(this.low,this.high,t.low,t.high),g.get_high(),this.unsigned)}if(this.isZero())return this.unsigned?p:m;var i,n,r;if(this.unsigned){if(t.unsigned||(t=t.toUnsigned()),t.gt(this))return p;if(t.gt(this.shru(1)))return b;r=p}else{if(this.eq(_)){if(t.eq(y)||t.eq(N))return _;if(t.eq(_))return y;return i=this.shr(1).div(t).shl(1),i.eq(m)?t.isNegative()?y:N:(n=this.sub(t.mul(i)),r=i.add(n.div(t)))}if(t.eq(_))return this.unsigned?p:m;if(this.isNegative())return t.isNegative()?this.neg().div(t.neg()):this.neg().div(t).neg();if(t.isNegative())return this.div(t.neg()).neg();r=m}for(n=this;n.gte(t);){i=Math.max(1,Math.floor(n.toNumber()/t.toNumber()));for(var u=Math.ceil(Math.log(i)/Math.LN2),f=u<=48?1:a(2,u-48),l=s(i),d=l.mul(t);d.isNegative()||d.gt(n);)i-=f,l=s(i,this.unsigned),d=l.mul(t);l.isZero()&&(l=y),r=r.add(l),n=n.sub(d)}return r},B.div=B.divide,B.modulo=function(t){if(e(t)||(t=o(t)),g){return h((this.unsigned?g.rem_u:g.rem_s)(this.low,this.high,t.low,t.high),g.get_high(),this.unsigned)}return this.sub(this.div(t).mul(t))},B.mod=B.modulo,B.rem=B.modulo,B.not=function(){return h(~this.low,~this.high,this.unsigned)},B.and=function(t){return e(t)||(t=o(t)),h(this.low&t.low,this.high&t.high,this.unsigned)},B.or=function(t){return e(t)||(t=o(t)),h(this.low|t.low,this.high|t.high,this.unsigned)},B.xor=function(t){return e(t)||(t=o(t)),h(this.low^t.low,this.high^t.high,this.unsigned)},B.shiftLeft=function(t){return e(t)&&(t=t.toInt()),0==(t&=63)?this:t<32?h(this.low<<t,this.high<<t|this.low>>>32-t,this.unsigned):h(0,this.low<<t-32,this.unsigned)},B.shl=B.shiftLeft,B.shiftRight=function(t){return e(t)&&(t=t.toInt()),0==(t&=63)?this:t<32?h(this.low>>>t|this.high<<32-t,this.high>>t,this.unsigned):h(this.high>>t-32,this.high>=0?0:-1,this.unsigned)},B.shr=B.shiftRight,B.shiftRightUnsigned=function(t){if(e(t)&&(t=t.toInt()),0===(t&=63))return this;var i=this.high;if(t<32){return h(this.low>>>t|i<<32-t,i>>>t,this.unsigned)}return 32===t?h(i,0,this.unsigned):h(i>>>t-32,0,this.unsigned)},B.shru=B.shiftRightUnsigned,B.shr_u=B.shiftRightUnsigned,B.toSigned=function(){return this.unsigned?h(this.low,this.high,!1):this},B.toUnsigned=function(){return this.unsigned?this:h(this.low,this.high,!0)},B.toBytes=function(t){return t?this.toBytesLE():this.toBytesBE()},B.toBytesLE=function(){var t=this.high,i=this.low;return[255&i,i>>>8&255,i>>>16&255,i>>>24,255&t,t>>>8&255,t>>>16&255,t>>>24]},B.toBytesBE=function(){var t=this.high,i=this.low;return[t>>>24,t>>>16&255,t>>>8&255,255&t,i>>>24,i>>>16&255,i>>>8&255,255&i]},n.fromBytes=function(t,i,e){return e?n.fromBytesLE(t,i):n.fromBytesBE(t,i)},n.fromBytesLE=function(t,i){return new n(t[0]|t[1]<<8|t[2]<<16|t[3]<<24,t[4]|t[5]<<8|t[6]<<16|t[7]<<24,i)},n.fromBytesBE=function(t,i){return new n(t[4]<<24|t[5]<<16|t[6]<<8|t[7],t[0]<<24|t[1]<<16|t[2]<<8|t[3],i)}}])}); //# sourceMappingURL=long.js.map apollo-server-demo/node_modules/long/LICENSE 0000644 0001750 0000144 00000026450 12113156054 020400 0 ustar andreh users Apache License Version 2.0, January 2004 http://www.apache.org/licenses/ TERMS AND CONDITIONS FOR USE, REPRODUCTION, AND DISTRIBUTION 1. Definitions. "License" shall mean the terms and conditions for use, reproduction, and distribution as defined by Sections 1 through 9 of this document. "Licensor" shall mean the copyright owner or entity authorized by the copyright owner that is granting the License. "Legal Entity" shall mean the union of the acting entity and all other entities that control, are controlled by, or are under common control with that entity. For the purposes of this definition, "control" means (i) the power, direct or indirect, to cause the direction or management of such entity, whether by contract or otherwise, or (ii) ownership of fifty percent (50%) or more of the outstanding shares, or (iii) beneficial ownership of such entity. "You" (or "Your") shall mean an individual or Legal Entity exercising permissions granted by this License. "Source" form shall mean the preferred form for making modifications, including but not limited to software source code, documentation source, and configuration files. "Object" form shall mean any form resulting from mechanical transformation or translation of a Source form, including but not limited to compiled object code, generated documentation, and conversions to other media types. "Work" shall mean the work of authorship, whether in Source or Object form, made available under the License, as indicated by a copyright notice that is included in or attached to the work (an example is provided in the Appendix below). "Derivative Works" shall mean any work, whether in Source or Object form, that is based on (or derived from) the Work and for which the editorial revisions, annotations, elaborations, or other modifications represent, as a whole, an original work of authorship. For the purposes of this License, Derivative Works shall not include works that remain separable from, or merely link (or bind by name) to the interfaces of, the Work and Derivative Works thereof. "Contribution" shall mean any work of authorship, including the original version of the Work and any modifications or additions to that Work or Derivative Works thereof, that is intentionally submitted to Licensor for inclusion in the Work by the copyright owner or by an individual or Legal Entity authorized to submit on behalf of the copyright owner. For the purposes of this definition, "submitted" means any form of electronic, verbal, or written communication sent to the Licensor or its representatives, including but not limited to communication on electronic mailing lists, source code control systems, and issue tracking systems that are managed by, or on behalf of, the Licensor for the purpose of discussing and improving the Work, but excluding communication that is conspicuously marked or otherwise designated in writing by the copyright owner as "Not a Contribution." "Contributor" shall mean Licensor and any individual or Legal Entity on behalf of whom a Contribution has been received by Licensor and subsequently incorporated within the Work. 2. Grant of Copyright License. Subject to the terms and conditions of this License, each Contributor hereby grants to You a perpetual, worldwide, non-exclusive, no-charge, royalty-free, irrevocable copyright license to reproduce, prepare Derivative Works of, publicly display, publicly perform, sublicense, and distribute the Work and such Derivative Works in Source or Object form. 3. Grant of Patent License. Subject to the terms and conditions of this License, each Contributor hereby grants to You a perpetual, worldwide, non-exclusive, no-charge, royalty-free, irrevocable (except as stated in this section) patent license to make, have made, use, offer to sell, sell, import, and otherwise transfer the Work, where such license applies only to those patent claims licensable by such Contributor that are necessarily infringed by their Contribution(s) alone or by combination of their Contribution(s) with the Work to which such Contribution(s) was submitted. If You institute patent litigation against any entity (including a cross-claim or counterclaim in a lawsuit) alleging that the Work or a Contribution incorporated within the Work constitutes direct or contributory patent infringement, then any patent licenses granted to You under this License for that Work shall terminate as of the date such litigation is filed. 4. Redistribution. You may reproduce and distribute copies of the Work or Derivative Works thereof in any medium, with or without modifications, and in Source or Object form, provided that You meet the following conditions: (a) You must give any other recipients of the Work or Derivative Works a copy of this License; and (b) You must cause any modified files to carry prominent notices stating that You changed the files; and (c) You must retain, in the Source form of any Derivative Works that You distribute, all copyright, patent, trademark, and attribution notices from the Source form of the Work, excluding those notices that do not pertain to any part of the Derivative Works; and (d) If the Work includes a "NOTICE" text file as part of its distribution, then any Derivative Works that You distribute must include a readable copy of the attribution notices contained within such NOTICE file, excluding those notices that do not pertain to any part of the Derivative Works, in at least one of the following places: within a NOTICE text file distributed as part of the Derivative Works; within the Source form or documentation, if provided along with the Derivative Works; or, within a display generated by the Derivative Works, if and wherever such third-party notices normally appear. The contents of the NOTICE file are for informational purposes only and do not modify the License. You may add Your own attribution notices within Derivative Works that You distribute, alongside or as an addendum to the NOTICE text from the Work, provided that such additional attribution notices cannot be construed as modifying the License. You may add Your own copyright statement to Your modifications and may provide additional or different license terms and conditions for use, reproduction, or distribution of Your modifications, or for any such Derivative Works as a whole, provided Your use, reproduction, and distribution of the Work otherwise complies with the conditions stated in this License. 5. Submission of Contributions. Unless You explicitly state otherwise, any Contribution intentionally submitted for inclusion in the Work by You to the Licensor shall be under the terms and conditions of this License, without any additional terms or conditions. Notwithstanding the above, nothing herein shall supersede or modify the terms of any separate license agreement you may have executed with Licensor regarding such Contributions. 6. Trademarks. This License does not grant permission to use the trade names, trademarks, service marks, or product names of the Licensor, except as required for reasonable and customary use in describing the origin of the Work and reproducing the content of the NOTICE file. 7. Disclaimer of Warranty. Unless required by applicable law or agreed to in writing, Licensor provides the Work (and each Contributor provides its Contributions) on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied, including, without limitation, any warranties or conditions of TITLE, NON-INFRINGEMENT, MERCHANTABILITY, or FITNESS FOR A PARTICULAR PURPOSE. You are solely responsible for determining the appropriateness of using or redistributing the Work and assume any risks associated with Your exercise of permissions under this License. 8. Limitation of Liability. In no event and under no legal theory, whether in tort (including negligence), contract, or otherwise, unless required by applicable law (such as deliberate and grossly negligent acts) or agreed to in writing, shall any Contributor be liable to You for damages, including any direct, indirect, special, incidental, or consequential damages of any character arising as a result of this License or out of the use or inability to use the Work (including but not limited to damages for loss of goodwill, work stoppage, computer failure or malfunction, or any and all other commercial damages or losses), even if such Contributor has been advised of the possibility of such damages. 9. Accepting Warranty or Additional Liability. While redistributing the Work or Derivative Works thereof, You may choose to offer, and charge a fee for, acceptance of support, warranty, indemnity, or other liability obligations and/or rights consistent with this License. However, in accepting such obligations, You may act only on Your own behalf and on Your sole responsibility, not on behalf of any other Contributor, and only if You agree to indemnify, defend, and hold each Contributor harmless for any liability incurred by, or claims asserted against, such Contributor by reason of your accepting any such warranty or additional liability. END OF TERMS AND CONDITIONS APPENDIX: How to apply the Apache License to your work. To apply the Apache License to your work, attach the following boilerplate notice, with the fields enclosed by brackets "[]" replaced with your own identifying information. (Don't include the brackets!) The text should be enclosed in the appropriate comment syntax for the file format. We also recommend that a file or class name and description of purpose be included on the same "printed page" as the copyright notice for easier identification within third-party archives. Copyright [yyyy] [name of copyright owner] Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License. You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0 Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions and limitations under the License. apollo-server-demo/node_modules/long/src/ 0000755 0001750 0000144 00000000000 14067647701 020171 5 ustar andreh users apollo-server-demo/node_modules/long/src/long.js 0000644 0001750 0000144 00000116244 13235424560 021466 0 ustar andreh users module.exports = Long; /** * wasm optimizations, to do native i64 multiplication and divide */ var wasm = null; try { wasm = new WebAssembly.Instance(new WebAssembly.Module(new Uint8Array([ 0, 97, 115, 109, 1, 0, 0, 0, 1, 13, 2, 96, 0, 1, 127, 96, 4, 127, 127, 127, 127, 1, 127, 3, 7, 6, 0, 1, 1, 1, 1, 1, 6, 6, 1, 127, 1, 65, 0, 11, 7, 50, 6, 3, 109, 117, 108, 0, 1, 5, 100, 105, 118, 95, 115, 0, 2, 5, 100, 105, 118, 95, 117, 0, 3, 5, 114, 101, 109, 95, 115, 0, 4, 5, 114, 101, 109, 95, 117, 0, 5, 8, 103, 101, 116, 95, 104, 105, 103, 104, 0, 0, 10, 191, 1, 6, 4, 0, 35, 0, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 126, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 127, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 128, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 129, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11, 36, 1, 1, 126, 32, 0, 173, 32, 1, 173, 66, 32, 134, 132, 32, 2, 173, 32, 3, 173, 66, 32, 134, 132, 130, 34, 4, 66, 32, 135, 167, 36, 0, 32, 4, 167, 11 ])), {}).exports; } catch (e) { // no wasm support :( } /** * Constructs a 64 bit two's-complement integer, given its low and high 32 bit values as *signed* integers. * See the from* functions below for more convenient ways of constructing Longs. * @exports Long * @class A Long class for representing a 64 bit two's-complement integer value. * @param {number} low The low (signed) 32 bits of the long * @param {number} high The high (signed) 32 bits of the long * @param {boolean=} unsigned Whether unsigned or not, defaults to signed * @constructor */ function Long(low, high, unsigned) { /** * The low 32 bits as a signed value. * @type {number} */ this.low = low | 0; /** * The high 32 bits as a signed value. * @type {number} */ this.high = high | 0; /** * Whether unsigned or not. * @type {boolean} */ this.unsigned = !!unsigned; } // The internal representation of a long is the two given signed, 32-bit values. // We use 32-bit pieces because these are the size of integers on which // Javascript performs bit-operations. For operations like addition and // multiplication, we split each number into 16 bit pieces, which can easily be // multiplied within Javascript's floating-point representation without overflow // or change in sign. // // In the algorithms below, we frequently reduce the negative case to the // positive case by negating the input(s) and then post-processing the result. // Note that we must ALWAYS check specially whether those values are MIN_VALUE // (-2^63) because -MIN_VALUE == MIN_VALUE (since 2^63 cannot be represented as // a positive number, it overflows back into a negative). Not handling this // case would often result in infinite recursion. // // Common constant values ZERO, ONE, NEG_ONE, etc. are defined below the from* // methods on which they depend. /** * An indicator used to reliably determine if an object is a Long or not. * @type {boolean} * @const * @private */ Long.prototype.__isLong__; Object.defineProperty(Long.prototype, "__isLong__", { value: true }); /** * @function * @param {*} obj Object * @returns {boolean} * @inner */ function isLong(obj) { return (obj && obj["__isLong__"]) === true; } /** * Tests if the specified object is a Long. * @function * @param {*} obj Object * @returns {boolean} */ Long.isLong = isLong; /** * A cache of the Long representations of small integer values. * @type {!Object} * @inner */ var INT_CACHE = {}; /** * A cache of the Long representations of small unsigned integer values. * @type {!Object} * @inner */ var UINT_CACHE = {}; /** * @param {number} value * @param {boolean=} unsigned * @returns {!Long} * @inner */ function fromInt(value, unsigned) { var obj, cachedObj, cache; if (unsigned) { value >>>= 0; if (cache = (0 <= value && value < 256)) { cachedObj = UINT_CACHE[value]; if (cachedObj) return cachedObj; } obj = fromBits(value, (value | 0) < 0 ? -1 : 0, true); if (cache) UINT_CACHE[value] = obj; return obj; } else { value |= 0; if (cache = (-128 <= value && value < 128)) { cachedObj = INT_CACHE[value]; if (cachedObj) return cachedObj; } obj = fromBits(value, value < 0 ? -1 : 0, false); if (cache) INT_CACHE[value] = obj; return obj; } } /** * Returns a Long representing the given 32 bit integer value. * @function * @param {number} value The 32 bit integer in question * @param {boolean=} unsigned Whether unsigned or not, defaults to signed * @returns {!Long} The corresponding Long value */ Long.fromInt = fromInt; /** * @param {number} value * @param {boolean=} unsigned * @returns {!Long} * @inner */ function fromNumber(value, unsigned) { if (isNaN(value)) return unsigned ? UZERO : ZERO; if (unsigned) { if (value < 0) return UZERO; if (value >= TWO_PWR_64_DBL) return MAX_UNSIGNED_VALUE; } else { if (value <= -TWO_PWR_63_DBL) return MIN_VALUE; if (value + 1 >= TWO_PWR_63_DBL) return MAX_VALUE; } if (value < 0) return fromNumber(-value, unsigned).neg(); return fromBits((value % TWO_PWR_32_DBL) | 0, (value / TWO_PWR_32_DBL) | 0, unsigned); } /** * Returns a Long representing the given value, provided that it is a finite number. Otherwise, zero is returned. * @function * @param {number} value The number in question * @param {boolean=} unsigned Whether unsigned or not, defaults to signed * @returns {!Long} The corresponding Long value */ Long.fromNumber = fromNumber; /** * @param {number} lowBits * @param {number} highBits * @param {boolean=} unsigned * @returns {!Long} * @inner */ function fromBits(lowBits, highBits, unsigned) { return new Long(lowBits, highBits, unsigned); } /** * Returns a Long representing the 64 bit integer that comes by concatenating the given low and high bits. Each is * assumed to use 32 bits. * @function * @param {number} lowBits The low 32 bits * @param {number} highBits The high 32 bits * @param {boolean=} unsigned Whether unsigned or not, defaults to signed * @returns {!Long} The corresponding Long value */ Long.fromBits = fromBits; /** * @function * @param {number} base * @param {number} exponent * @returns {number} * @inner */ var pow_dbl = Math.pow; // Used 4 times (4*8 to 15+4) /** * @param {string} str * @param {(boolean|number)=} unsigned * @param {number=} radix * @returns {!Long} * @inner */ function fromString(str, unsigned, radix) { if (str.length === 0) throw Error('empty string'); if (str === "NaN" || str === "Infinity" || str === "+Infinity" || str === "-Infinity") return ZERO; if (typeof unsigned === 'number') { // For goog.math.long compatibility radix = unsigned, unsigned = false; } else { unsigned = !! unsigned; } radix = radix || 10; if (radix < 2 || 36 < radix) throw RangeError('radix'); var p; if ((p = str.indexOf('-')) > 0) throw Error('interior hyphen'); else if (p === 0) { return fromString(str.substring(1), unsigned, radix).neg(); } // Do several (8) digits each time through the loop, so as to // minimize the calls to the very expensive emulated div. var radixToPower = fromNumber(pow_dbl(radix, 8)); var result = ZERO; for (var i = 0; i < str.length; i += 8) { var size = Math.min(8, str.length - i), value = parseInt(str.substring(i, i + size), radix); if (size < 8) { var power = fromNumber(pow_dbl(radix, size)); result = result.mul(power).add(fromNumber(value)); } else { result = result.mul(radixToPower); result = result.add(fromNumber(value)); } } result.unsigned = unsigned; return result; } /** * Returns a Long representation of the given string, written using the specified radix. * @function * @param {string} str The textual representation of the Long * @param {(boolean|number)=} unsigned Whether unsigned or not, defaults to signed * @param {number=} radix The radix in which the text is written (2-36), defaults to 10 * @returns {!Long} The corresponding Long value */ Long.fromString = fromString; /** * @function * @param {!Long|number|string|!{low: number, high: number, unsigned: boolean}} val * @param {boolean=} unsigned * @returns {!Long} * @inner */ function fromValue(val, unsigned) { if (typeof val === 'number') return fromNumber(val, unsigned); if (typeof val === 'string') return fromString(val, unsigned); // Throws for non-objects, converts non-instanceof Long: return fromBits(val.low, val.high, typeof unsigned === 'boolean' ? unsigned : val.unsigned); } /** * Converts the specified value to a Long using the appropriate from* function for its type. * @function * @param {!Long|number|string|!{low: number, high: number, unsigned: boolean}} val Value * @param {boolean=} unsigned Whether unsigned or not, defaults to signed * @returns {!Long} */ Long.fromValue = fromValue; // NOTE: the compiler should inline these constant values below and then remove these variables, so there should be // no runtime penalty for these. /** * @type {number} * @const * @inner */ var TWO_PWR_16_DBL = 1 << 16; /** * @type {number} * @const * @inner */ var TWO_PWR_24_DBL = 1 << 24; /** * @type {number} * @const * @inner */ var TWO_PWR_32_DBL = TWO_PWR_16_DBL * TWO_PWR_16_DBL; /** * @type {number} * @const * @inner */ var TWO_PWR_64_DBL = TWO_PWR_32_DBL * TWO_PWR_32_DBL; /** * @type {number} * @const * @inner */ var TWO_PWR_63_DBL = TWO_PWR_64_DBL / 2; /** * @type {!Long} * @const * @inner */ var TWO_PWR_24 = fromInt(TWO_PWR_24_DBL); /** * @type {!Long} * @inner */ var ZERO = fromInt(0); /** * Signed zero. * @type {!Long} */ Long.ZERO = ZERO; /** * @type {!Long} * @inner */ var UZERO = fromInt(0, true); /** * Unsigned zero. * @type {!Long} */ Long.UZERO = UZERO; /** * @type {!Long} * @inner */ var ONE = fromInt(1); /** * Signed one. * @type {!Long} */ Long.ONE = ONE; /** * @type {!Long} * @inner */ var UONE = fromInt(1, true); /** * Unsigned one. * @type {!Long} */ Long.UONE = UONE; /** * @type {!Long} * @inner */ var NEG_ONE = fromInt(-1); /** * Signed negative one. * @type {!Long} */ Long.NEG_ONE = NEG_ONE; /** * @type {!Long} * @inner */ var MAX_VALUE = fromBits(0xFFFFFFFF|0, 0x7FFFFFFF|0, false); /** * Maximum signed value. * @type {!Long} */ Long.MAX_VALUE = MAX_VALUE; /** * @type {!Long} * @inner */ var MAX_UNSIGNED_VALUE = fromBits(0xFFFFFFFF|0, 0xFFFFFFFF|0, true); /** * Maximum unsigned value. * @type {!Long} */ Long.MAX_UNSIGNED_VALUE = MAX_UNSIGNED_VALUE; /** * @type {!Long} * @inner */ var MIN_VALUE = fromBits(0, 0x80000000|0, false); /** * Minimum signed value. * @type {!Long} */ Long.MIN_VALUE = MIN_VALUE; /** * @alias Long.prototype * @inner */ var LongPrototype = Long.prototype; /** * Converts the Long to a 32 bit integer, assuming it is a 32 bit integer. * @returns {number} */ LongPrototype.toInt = function toInt() { return this.unsigned ? this.low >>> 0 : this.low; }; /** * Converts the Long to a the nearest floating-point representation of this value (double, 53 bit mantissa). * @returns {number} */ LongPrototype.toNumber = function toNumber() { if (this.unsigned) return ((this.high >>> 0) * TWO_PWR_32_DBL) + (this.low >>> 0); return this.high * TWO_PWR_32_DBL + (this.low >>> 0); }; /** * Converts the Long to a string written in the specified radix. * @param {number=} radix Radix (2-36), defaults to 10 * @returns {string} * @override * @throws {RangeError} If `radix` is out of range */ LongPrototype.toString = function toString(radix) { radix = radix || 10; if (radix < 2 || 36 < radix) throw RangeError('radix'); if (this.isZero()) return '0'; if (this.isNegative()) { // Unsigned Longs are never negative if (this.eq(MIN_VALUE)) { // We need to change the Long value before it can be negated, so we remove // the bottom-most digit in this base and then recurse to do the rest. var radixLong = fromNumber(radix), div = this.div(radixLong), rem1 = div.mul(radixLong).sub(this); return div.toString(radix) + rem1.toInt().toString(radix); } else return '-' + this.neg().toString(radix); } // Do several (6) digits each time through the loop, so as to // minimize the calls to the very expensive emulated div. var radixToPower = fromNumber(pow_dbl(radix, 6), this.unsigned), rem = this; var result = ''; while (true) { var remDiv = rem.div(radixToPower), intval = rem.sub(remDiv.mul(radixToPower)).toInt() >>> 0, digits = intval.toString(radix); rem = remDiv; if (rem.isZero()) return digits + result; else { while (digits.length < 6) digits = '0' + digits; result = '' + digits + result; } } }; /** * Gets the high 32 bits as a signed integer. * @returns {number} Signed high bits */ LongPrototype.getHighBits = function getHighBits() { return this.high; }; /** * Gets the high 32 bits as an unsigned integer. * @returns {number} Unsigned high bits */ LongPrototype.getHighBitsUnsigned = function getHighBitsUnsigned() { return this.high >>> 0; }; /** * Gets the low 32 bits as a signed integer. * @returns {number} Signed low bits */ LongPrototype.getLowBits = function getLowBits() { return this.low; }; /** * Gets the low 32 bits as an unsigned integer. * @returns {number} Unsigned low bits */ LongPrototype.getLowBitsUnsigned = function getLowBitsUnsigned() { return this.low >>> 0; }; /** * Gets the number of bits needed to represent the absolute value of this Long. * @returns {number} */ LongPrototype.getNumBitsAbs = function getNumBitsAbs() { if (this.isNegative()) // Unsigned Longs are never negative return this.eq(MIN_VALUE) ? 64 : this.neg().getNumBitsAbs(); var val = this.high != 0 ? this.high : this.low; for (var bit = 31; bit > 0; bit--) if ((val & (1 << bit)) != 0) break; return this.high != 0 ? bit + 33 : bit + 1; }; /** * Tests if this Long's value equals zero. * @returns {boolean} */ LongPrototype.isZero = function isZero() { return this.high === 0 && this.low === 0; }; /** * Tests if this Long's value equals zero. This is an alias of {@link Long#isZero}. * @returns {boolean} */ LongPrototype.eqz = LongPrototype.isZero; /** * Tests if this Long's value is negative. * @returns {boolean} */ LongPrototype.isNegative = function isNegative() { return !this.unsigned && this.high < 0; }; /** * Tests if this Long's value is positive. * @returns {boolean} */ LongPrototype.isPositive = function isPositive() { return this.unsigned || this.high >= 0; }; /** * Tests if this Long's value is odd. * @returns {boolean} */ LongPrototype.isOdd = function isOdd() { return (this.low & 1) === 1; }; /** * Tests if this Long's value is even. * @returns {boolean} */ LongPrototype.isEven = function isEven() { return (this.low & 1) === 0; }; /** * Tests if this Long's value equals the specified's. * @param {!Long|number|string} other Other value * @returns {boolean} */ LongPrototype.equals = function equals(other) { if (!isLong(other)) other = fromValue(other); if (this.unsigned !== other.unsigned && (this.high >>> 31) === 1 && (other.high >>> 31) === 1) return false; return this.high === other.high && this.low === other.low; }; /** * Tests if this Long's value equals the specified's. This is an alias of {@link Long#equals}. * @function * @param {!Long|number|string} other Other value * @returns {boolean} */ LongPrototype.eq = LongPrototype.equals; /** * Tests if this Long's value differs from the specified's. * @param {!Long|number|string} other Other value * @returns {boolean} */ LongPrototype.notEquals = function notEquals(other) { return !this.eq(/* validates */ other); }; /** * Tests if this Long's value differs from the specified's. This is an alias of {@link Long#notEquals}. * @function * @param {!Long|number|string} other Other value * @returns {boolean} */ LongPrototype.neq = LongPrototype.notEquals; /** * Tests if this Long's value differs from the specified's. This is an alias of {@link Long#notEquals}. * @function * @param {!Long|number|string} other Other value * @returns {boolean} */ LongPrototype.ne = LongPrototype.notEquals; /** * Tests if this Long's value is less than the specified's. * @param {!Long|number|string} other Other value * @returns {boolean} */ LongPrototype.lessThan = function lessThan(other) { return this.comp(/* validates */ other) < 0; }; /** * Tests if this Long's value is less than the specified's. This is an alias of {@link Long#lessThan}. * @function * @param {!Long|number|string} other Other value * @returns {boolean} */ LongPrototype.lt = LongPrototype.lessThan; /** * Tests if this Long's value is less than or equal the specified's. * @param {!Long|number|string} other Other value * @returns {boolean} */ LongPrototype.lessThanOrEqual = function lessThanOrEqual(other) { return this.comp(/* validates */ other) <= 0; }; /** * Tests if this Long's value is less than or equal the specified's. This is an alias of {@link Long#lessThanOrEqual}. * @function * @param {!Long|number|string} other Other value * @returns {boolean} */ LongPrototype.lte = LongPrototype.lessThanOrEqual; /** * Tests if this Long's value is less than or equal the specified's. This is an alias of {@link Long#lessThanOrEqual}. * @function * @param {!Long|number|string} other Other value * @returns {boolean} */ LongPrototype.le = LongPrototype.lessThanOrEqual; /** * Tests if this Long's value is greater than the specified's. * @param {!Long|number|string} other Other value * @returns {boolean} */ LongPrototype.greaterThan = function greaterThan(other) { return this.comp(/* validates */ other) > 0; }; /** * Tests if this Long's value is greater than the specified's. This is an alias of {@link Long#greaterThan}. * @function * @param {!Long|number|string} other Other value * @returns {boolean} */ LongPrototype.gt = LongPrototype.greaterThan; /** * Tests if this Long's value is greater than or equal the specified's. * @param {!Long|number|string} other Other value * @returns {boolean} */ LongPrototype.greaterThanOrEqual = function greaterThanOrEqual(other) { return this.comp(/* validates */ other) >= 0; }; /** * Tests if this Long's value is greater than or equal the specified's. This is an alias of {@link Long#greaterThanOrEqual}. * @function * @param {!Long|number|string} other Other value * @returns {boolean} */ LongPrototype.gte = LongPrototype.greaterThanOrEqual; /** * Tests if this Long's value is greater than or equal the specified's. This is an alias of {@link Long#greaterThanOrEqual}. * @function * @param {!Long|number|string} other Other value * @returns {boolean} */ LongPrototype.ge = LongPrototype.greaterThanOrEqual; /** * Compares this Long's value with the specified's. * @param {!Long|number|string} other Other value * @returns {number} 0 if they are the same, 1 if the this is greater and -1 * if the given one is greater */ LongPrototype.compare = function compare(other) { if (!isLong(other)) other = fromValue(other); if (this.eq(other)) return 0; var thisNeg = this.isNegative(), otherNeg = other.isNegative(); if (thisNeg && !otherNeg) return -1; if (!thisNeg && otherNeg) return 1; // At this point the sign bits are the same if (!this.unsigned) return this.sub(other).isNegative() ? -1 : 1; // Both are positive if at least one is unsigned return (other.high >>> 0) > (this.high >>> 0) || (other.high === this.high && (other.low >>> 0) > (this.low >>> 0)) ? -1 : 1; }; /** * Compares this Long's value with the specified's. This is an alias of {@link Long#compare}. * @function * @param {!Long|number|string} other Other value * @returns {number} 0 if they are the same, 1 if the this is greater and -1 * if the given one is greater */ LongPrototype.comp = LongPrototype.compare; /** * Negates this Long's value. * @returns {!Long} Negated Long */ LongPrototype.negate = function negate() { if (!this.unsigned && this.eq(MIN_VALUE)) return MIN_VALUE; return this.not().add(ONE); }; /** * Negates this Long's value. This is an alias of {@link Long#negate}. * @function * @returns {!Long} Negated Long */ LongPrototype.neg = LongPrototype.negate; /** * Returns the sum of this and the specified Long. * @param {!Long|number|string} addend Addend * @returns {!Long} Sum */ LongPrototype.add = function add(addend) { if (!isLong(addend)) addend = fromValue(addend); // Divide each number into 4 chunks of 16 bits, and then sum the chunks. var a48 = this.high >>> 16; var a32 = this.high & 0xFFFF; var a16 = this.low >>> 16; var a00 = this.low & 0xFFFF; var b48 = addend.high >>> 16; var b32 = addend.high & 0xFFFF; var b16 = addend.low >>> 16; var b00 = addend.low & 0xFFFF; var c48 = 0, c32 = 0, c16 = 0, c00 = 0; c00 += a00 + b00; c16 += c00 >>> 16; c00 &= 0xFFFF; c16 += a16 + b16; c32 += c16 >>> 16; c16 &= 0xFFFF; c32 += a32 + b32; c48 += c32 >>> 16; c32 &= 0xFFFF; c48 += a48 + b48; c48 &= 0xFFFF; return fromBits((c16 << 16) | c00, (c48 << 16) | c32, this.unsigned); }; /** * Returns the difference of this and the specified Long. * @param {!Long|number|string} subtrahend Subtrahend * @returns {!Long} Difference */ LongPrototype.subtract = function subtract(subtrahend) { if (!isLong(subtrahend)) subtrahend = fromValue(subtrahend); return this.add(subtrahend.neg()); }; /** * Returns the difference of this and the specified Long. This is an alias of {@link Long#subtract}. * @function * @param {!Long|number|string} subtrahend Subtrahend * @returns {!Long} Difference */ LongPrototype.sub = LongPrototype.subtract; /** * Returns the product of this and the specified Long. * @param {!Long|number|string} multiplier Multiplier * @returns {!Long} Product */ LongPrototype.multiply = function multiply(multiplier) { if (this.isZero()) return ZERO; if (!isLong(multiplier)) multiplier = fromValue(multiplier); // use wasm support if present if (wasm) { var low = wasm.mul(this.low, this.high, multiplier.low, multiplier.high); return fromBits(low, wasm.get_high(), this.unsigned); } if (multiplier.isZero()) return ZERO; if (this.eq(MIN_VALUE)) return multiplier.isOdd() ? MIN_VALUE : ZERO; if (multiplier.eq(MIN_VALUE)) return this.isOdd() ? MIN_VALUE : ZERO; if (this.isNegative()) { if (multiplier.isNegative()) return this.neg().mul(multiplier.neg()); else return this.neg().mul(multiplier).neg(); } else if (multiplier.isNegative()) return this.mul(multiplier.neg()).neg(); // If both longs are small, use float multiplication if (this.lt(TWO_PWR_24) && multiplier.lt(TWO_PWR_24)) return fromNumber(this.toNumber() * multiplier.toNumber(), this.unsigned); // Divide each long into 4 chunks of 16 bits, and then add up 4x4 products. // We can skip products that would overflow. var a48 = this.high >>> 16; var a32 = this.high & 0xFFFF; var a16 = this.low >>> 16; var a00 = this.low & 0xFFFF; var b48 = multiplier.high >>> 16; var b32 = multiplier.high & 0xFFFF; var b16 = multiplier.low >>> 16; var b00 = multiplier.low & 0xFFFF; var c48 = 0, c32 = 0, c16 = 0, c00 = 0; c00 += a00 * b00; c16 += c00 >>> 16; c00 &= 0xFFFF; c16 += a16 * b00; c32 += c16 >>> 16; c16 &= 0xFFFF; c16 += a00 * b16; c32 += c16 >>> 16; c16 &= 0xFFFF; c32 += a32 * b00; c48 += c32 >>> 16; c32 &= 0xFFFF; c32 += a16 * b16; c48 += c32 >>> 16; c32 &= 0xFFFF; c32 += a00 * b32; c48 += c32 >>> 16; c32 &= 0xFFFF; c48 += a48 * b00 + a32 * b16 + a16 * b32 + a00 * b48; c48 &= 0xFFFF; return fromBits((c16 << 16) | c00, (c48 << 16) | c32, this.unsigned); }; /** * Returns the product of this and the specified Long. This is an alias of {@link Long#multiply}. * @function * @param {!Long|number|string} multiplier Multiplier * @returns {!Long} Product */ LongPrototype.mul = LongPrototype.multiply; /** * Returns this Long divided by the specified. The result is signed if this Long is signed or * unsigned if this Long is unsigned. * @param {!Long|number|string} divisor Divisor * @returns {!Long} Quotient */ LongPrototype.divide = function divide(divisor) { if (!isLong(divisor)) divisor = fromValue(divisor); if (divisor.isZero()) throw Error('division by zero'); // use wasm support if present if (wasm) { // guard against signed division overflow: the largest // negative number / -1 would be 1 larger than the largest // positive number, due to two's complement. if (!this.unsigned && this.high === -0x80000000 && divisor.low === -1 && divisor.high === -1) { // be consistent with non-wasm code path return this; } var low = (this.unsigned ? wasm.div_u : wasm.div_s)( this.low, this.high, divisor.low, divisor.high ); return fromBits(low, wasm.get_high(), this.unsigned); } if (this.isZero()) return this.unsigned ? UZERO : ZERO; var approx, rem, res; if (!this.unsigned) { // This section is only relevant for signed longs and is derived from the // closure library as a whole. if (this.eq(MIN_VALUE)) { if (divisor.eq(ONE) || divisor.eq(NEG_ONE)) return MIN_VALUE; // recall that -MIN_VALUE == MIN_VALUE else if (divisor.eq(MIN_VALUE)) return ONE; else { // At this point, we have |other| >= 2, so |this/other| < |MIN_VALUE|. var halfThis = this.shr(1); approx = halfThis.div(divisor).shl(1); if (approx.eq(ZERO)) { return divisor.isNegative() ? ONE : NEG_ONE; } else { rem = this.sub(divisor.mul(approx)); res = approx.add(rem.div(divisor)); return res; } } } else if (divisor.eq(MIN_VALUE)) return this.unsigned ? UZERO : ZERO; if (this.isNegative()) { if (divisor.isNegative()) return this.neg().div(divisor.neg()); return this.neg().div(divisor).neg(); } else if (divisor.isNegative()) return this.div(divisor.neg()).neg(); res = ZERO; } else { // The algorithm below has not been made for unsigned longs. It's therefore // required to take special care of the MSB prior to running it. if (!divisor.unsigned) divisor = divisor.toUnsigned(); if (divisor.gt(this)) return UZERO; if (divisor.gt(this.shru(1))) // 15 >>> 1 = 7 ; with divisor = 8 ; true return UONE; res = UZERO; } // Repeat the following until the remainder is less than other: find a // floating-point that approximates remainder / other *from below*, add this // into the result, and subtract it from the remainder. It is critical that // the approximate value is less than or equal to the real value so that the // remainder never becomes negative. rem = this; while (rem.gte(divisor)) { // Approximate the result of division. This may be a little greater or // smaller than the actual value. approx = Math.max(1, Math.floor(rem.toNumber() / divisor.toNumber())); // We will tweak the approximate result by changing it in the 48-th digit or // the smallest non-fractional digit, whichever is larger. var log2 = Math.ceil(Math.log(approx) / Math.LN2), delta = (log2 <= 48) ? 1 : pow_dbl(2, log2 - 48), // Decrease the approximation until it is smaller than the remainder. Note // that if it is too large, the product overflows and is negative. approxRes = fromNumber(approx), approxRem = approxRes.mul(divisor); while (approxRem.isNegative() || approxRem.gt(rem)) { approx -= delta; approxRes = fromNumber(approx, this.unsigned); approxRem = approxRes.mul(divisor); } // We know the answer can't be zero... and actually, zero would cause // infinite recursion since we would make no progress. if (approxRes.isZero()) approxRes = ONE; res = res.add(approxRes); rem = rem.sub(approxRem); } return res; }; /** * Returns this Long divided by the specified. This is an alias of {@link Long#divide}. * @function * @param {!Long|number|string} divisor Divisor * @returns {!Long} Quotient */ LongPrototype.div = LongPrototype.divide; /** * Returns this Long modulo the specified. * @param {!Long|number|string} divisor Divisor * @returns {!Long} Remainder */ LongPrototype.modulo = function modulo(divisor) { if (!isLong(divisor)) divisor = fromValue(divisor); // use wasm support if present if (wasm) { var low = (this.unsigned ? wasm.rem_u : wasm.rem_s)( this.low, this.high, divisor.low, divisor.high ); return fromBits(low, wasm.get_high(), this.unsigned); } return this.sub(this.div(divisor).mul(divisor)); }; /** * Returns this Long modulo the specified. This is an alias of {@link Long#modulo}. * @function * @param {!Long|number|string} divisor Divisor * @returns {!Long} Remainder */ LongPrototype.mod = LongPrototype.modulo; /** * Returns this Long modulo the specified. This is an alias of {@link Long#modulo}. * @function * @param {!Long|number|string} divisor Divisor * @returns {!Long} Remainder */ LongPrototype.rem = LongPrototype.modulo; /** * Returns the bitwise NOT of this Long. * @returns {!Long} */ LongPrototype.not = function not() { return fromBits(~this.low, ~this.high, this.unsigned); }; /** * Returns the bitwise AND of this Long and the specified. * @param {!Long|number|string} other Other Long * @returns {!Long} */ LongPrototype.and = function and(other) { if (!isLong(other)) other = fromValue(other); return fromBits(this.low & other.low, this.high & other.high, this.unsigned); }; /** * Returns the bitwise OR of this Long and the specified. * @param {!Long|number|string} other Other Long * @returns {!Long} */ LongPrototype.or = function or(other) { if (!isLong(other)) other = fromValue(other); return fromBits(this.low | other.low, this.high | other.high, this.unsigned); }; /** * Returns the bitwise XOR of this Long and the given one. * @param {!Long|number|string} other Other Long * @returns {!Long} */ LongPrototype.xor = function xor(other) { if (!isLong(other)) other = fromValue(other); return fromBits(this.low ^ other.low, this.high ^ other.high, this.unsigned); }; /** * Returns this Long with bits shifted to the left by the given amount. * @param {number|!Long} numBits Number of bits * @returns {!Long} Shifted Long */ LongPrototype.shiftLeft = function shiftLeft(numBits) { if (isLong(numBits)) numBits = numBits.toInt(); if ((numBits &= 63) === 0) return this; else if (numBits < 32) return fromBits(this.low << numBits, (this.high << numBits) | (this.low >>> (32 - numBits)), this.unsigned); else return fromBits(0, this.low << (numBits - 32), this.unsigned); }; /** * Returns this Long with bits shifted to the left by the given amount. This is an alias of {@link Long#shiftLeft}. * @function * @param {number|!Long} numBits Number of bits * @returns {!Long} Shifted Long */ LongPrototype.shl = LongPrototype.shiftLeft; /** * Returns this Long with bits arithmetically shifted to the right by the given amount. * @param {number|!Long} numBits Number of bits * @returns {!Long} Shifted Long */ LongPrototype.shiftRight = function shiftRight(numBits) { if (isLong(numBits)) numBits = numBits.toInt(); if ((numBits &= 63) === 0) return this; else if (numBits < 32) return fromBits((this.low >>> numBits) | (this.high << (32 - numBits)), this.high >> numBits, this.unsigned); else return fromBits(this.high >> (numBits - 32), this.high >= 0 ? 0 : -1, this.unsigned); }; /** * Returns this Long with bits arithmetically shifted to the right by the given amount. This is an alias of {@link Long#shiftRight}. * @function * @param {number|!Long} numBits Number of bits * @returns {!Long} Shifted Long */ LongPrototype.shr = LongPrototype.shiftRight; /** * Returns this Long with bits logically shifted to the right by the given amount. * @param {number|!Long} numBits Number of bits * @returns {!Long} Shifted Long */ LongPrototype.shiftRightUnsigned = function shiftRightUnsigned(numBits) { if (isLong(numBits)) numBits = numBits.toInt(); numBits &= 63; if (numBits === 0) return this; else { var high = this.high; if (numBits < 32) { var low = this.low; return fromBits((low >>> numBits) | (high << (32 - numBits)), high >>> numBits, this.unsigned); } else if (numBits === 32) return fromBits(high, 0, this.unsigned); else return fromBits(high >>> (numBits - 32), 0, this.unsigned); } }; /** * Returns this Long with bits logically shifted to the right by the given amount. This is an alias of {@link Long#shiftRightUnsigned}. * @function * @param {number|!Long} numBits Number of bits * @returns {!Long} Shifted Long */ LongPrototype.shru = LongPrototype.shiftRightUnsigned; /** * Returns this Long with bits logically shifted to the right by the given amount. This is an alias of {@link Long#shiftRightUnsigned}. * @function * @param {number|!Long} numBits Number of bits * @returns {!Long} Shifted Long */ LongPrototype.shr_u = LongPrototype.shiftRightUnsigned; /** * Converts this Long to signed. * @returns {!Long} Signed long */ LongPrototype.toSigned = function toSigned() { if (!this.unsigned) return this; return fromBits(this.low, this.high, false); }; /** * Converts this Long to unsigned. * @returns {!Long} Unsigned long */ LongPrototype.toUnsigned = function toUnsigned() { if (this.unsigned) return this; return fromBits(this.low, this.high, true); }; /** * Converts this Long to its byte representation. * @param {boolean=} le Whether little or big endian, defaults to big endian * @returns {!Array.<number>} Byte representation */ LongPrototype.toBytes = function toBytes(le) { return le ? this.toBytesLE() : this.toBytesBE(); }; /** * Converts this Long to its little endian byte representation. * @returns {!Array.<number>} Little endian byte representation */ LongPrototype.toBytesLE = function toBytesLE() { var hi = this.high, lo = this.low; return [ lo & 0xff, lo >>> 8 & 0xff, lo >>> 16 & 0xff, lo >>> 24 , hi & 0xff, hi >>> 8 & 0xff, hi >>> 16 & 0xff, hi >>> 24 ]; }; /** * Converts this Long to its big endian byte representation. * @returns {!Array.<number>} Big endian byte representation */ LongPrototype.toBytesBE = function toBytesBE() { var hi = this.high, lo = this.low; return [ hi >>> 24 , hi >>> 16 & 0xff, hi >>> 8 & 0xff, hi & 0xff, lo >>> 24 , lo >>> 16 & 0xff, lo >>> 8 & 0xff, lo & 0xff ]; }; /** * Creates a Long from its byte representation. * @param {!Array.<number>} bytes Byte representation * @param {boolean=} unsigned Whether unsigned or not, defaults to signed * @param {boolean=} le Whether little or big endian, defaults to big endian * @returns {Long} The corresponding Long value */ Long.fromBytes = function fromBytes(bytes, unsigned, le) { return le ? Long.fromBytesLE(bytes, unsigned) : Long.fromBytesBE(bytes, unsigned); }; /** * Creates a Long from its little endian byte representation. * @param {!Array.<number>} bytes Little endian byte representation * @param {boolean=} unsigned Whether unsigned or not, defaults to signed * @returns {Long} The corresponding Long value */ Long.fromBytesLE = function fromBytesLE(bytes, unsigned) { return new Long( bytes[0] | bytes[1] << 8 | bytes[2] << 16 | bytes[3] << 24, bytes[4] | bytes[5] << 8 | bytes[6] << 16 | bytes[7] << 24, unsigned ); }; /** * Creates a Long from its big endian byte representation. * @param {!Array.<number>} bytes Big endian byte representation * @param {boolean=} unsigned Whether unsigned or not, defaults to signed * @returns {Long} The corresponding Long value */ Long.fromBytesBE = function fromBytesBE(bytes, unsigned) { return new Long( bytes[4] << 24 | bytes[5] << 16 | bytes[6] << 8 | bytes[7], bytes[0] << 24 | bytes[1] << 16 | bytes[2] << 8 | bytes[3], unsigned ); }; apollo-server-demo/node_modules/long/README.md 0000644 0001750 0000144 00000021531 13235365346 020661 0 ustar andreh users long.js ======= A Long class for representing a 64 bit two's-complement integer value derived from the [Closure Library](https://github.com/google/closure-library) for stand-alone use and extended with unsigned support. [](https://travis-ci.org/dcodeIO/long.js) Background ---------- As of [ECMA-262 5th Edition](http://ecma262-5.com/ELS5_HTML.htm#Section_8.5), "all the positive and negative integers whose magnitude is no greater than 2<sup>53</sup> are representable in the Number type", which is "representing the doubleprecision 64-bit format IEEE 754 values as specified in the IEEE Standard for Binary Floating-Point Arithmetic". The [maximum safe integer](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Number/MAX_SAFE_INTEGER) in JavaScript is 2<sup>53</sup>-1. Example: 2<sup>64</sup>-1 is 1844674407370955**1615** but in JavaScript it evaluates to 1844674407370955**2000**. Furthermore, bitwise operators in JavaScript "deal only with integers in the range −2<sup>31</sup> through 2<sup>31</sup>−1, inclusive, or in the range 0 through 2<sup>32</sup>−1, inclusive. These operators accept any value of the Number type but first convert each such value to one of 2<sup>32</sup> integer values." In some use cases, however, it is required to be able to reliably work with and perform bitwise operations on the full 64 bits. This is where long.js comes into play. Usage ----- The class is compatible with CommonJS and AMD loaders and is exposed globally as `Long` if neither is available. ```javascript var Long = require("long"); var longVal = new Long(0xFFFFFFFF, 0x7FFFFFFF); console.log(longVal.toString()); ... ``` API --- ### Constructor * new **Long**(low: `number`, high: `number`, unsigned?: `boolean`)<br /> Constructs a 64 bit two's-complement integer, given its low and high 32 bit values as *signed* integers. See the from* functions below for more convenient ways of constructing Longs. ### Fields * Long#**low**: `number`<br /> The low 32 bits as a signed value. * Long#**high**: `number`<br /> The high 32 bits as a signed value. * Long#**unsigned**: `boolean`<br /> Whether unsigned or not. ### Constants * Long.**ZERO**: `Long`<br /> Signed zero. * Long.**ONE**: `Long`<br /> Signed one. * Long.**NEG_ONE**: `Long`<br /> Signed negative one. * Long.**UZERO**: `Long`<br /> Unsigned zero. * Long.**UONE**: `Long`<br /> Unsigned one. * Long.**MAX_VALUE**: `Long`<br /> Maximum signed value. * Long.**MIN_VALUE**: `Long`<br /> Minimum signed value. * Long.**MAX_UNSIGNED_VALUE**: `Long`<br /> Maximum unsigned value. ### Utility * Long.**isLong**(obj: `*`): `boolean`<br /> Tests if the specified object is a Long. * Long.**fromBits**(lowBits: `number`, highBits: `number`, unsigned?: `boolean`): `Long`<br /> Returns a Long representing the 64 bit integer that comes by concatenating the given low and high bits. Each is assumed to use 32 bits. * Long.**fromBytes**(bytes: `number[]`, unsigned?: `boolean`, le?: `boolean`): `Long`<br /> Creates a Long from its byte representation. * Long.**fromBytesLE**(bytes: `number[]`, unsigned?: `boolean`): `Long`<br /> Creates a Long from its little endian byte representation. * Long.**fromBytesBE**(bytes: `number[]`, unsigned?: `boolean`): `Long`<br /> Creates a Long from its big endian byte representation. * Long.**fromInt**(value: `number`, unsigned?: `boolean`): `Long`<br /> Returns a Long representing the given 32 bit integer value. * Long.**fromNumber**(value: `number`, unsigned?: `boolean`): `Long`<br /> Returns a Long representing the given value, provided that it is a finite number. Otherwise, zero is returned. * Long.**fromString**(str: `string`, unsigned?: `boolean`, radix?: `number`)<br /> Long.**fromString**(str: `string`, radix: `number`)<br /> Returns a Long representation of the given string, written using the specified radix. * Long.**fromValue**(val: `*`, unsigned?: `boolean`): `Long`<br /> Converts the specified value to a Long using the appropriate from* function for its type. ### Methods * Long#**add**(addend: `Long | number | string`): `Long`<br /> Returns the sum of this and the specified Long. * Long#**and**(other: `Long | number | string`): `Long`<br /> Returns the bitwise AND of this Long and the specified. * Long#**compare**/**comp**(other: `Long | number | string`): `number`<br /> Compares this Long's value with the specified's. Returns `0` if they are the same, `1` if the this is greater and `-1` if the given one is greater. * Long#**divide**/**div**(divisor: `Long | number | string`): `Long`<br /> Returns this Long divided by the specified. * Long#**equals**/**eq**(other: `Long | number | string`): `boolean`<br /> Tests if this Long's value equals the specified's. * Long#**getHighBits**(): `number`<br /> Gets the high 32 bits as a signed integer. * Long#**getHighBitsUnsigned**(): `number`<br /> Gets the high 32 bits as an unsigned integer. * Long#**getLowBits**(): `number`<br /> Gets the low 32 bits as a signed integer. * Long#**getLowBitsUnsigned**(): `number`<br /> Gets the low 32 bits as an unsigned integer. * Long#**getNumBitsAbs**(): `number`<br /> Gets the number of bits needed to represent the absolute value of this Long. * Long#**greaterThan**/**gt**(other: `Long | number | string`): `boolean`<br /> Tests if this Long's value is greater than the specified's. * Long#**greaterThanOrEqual**/**gte**/**ge**(other: `Long | number | string`): `boolean`<br /> Tests if this Long's value is greater than or equal the specified's. * Long#**isEven**(): `boolean`<br /> Tests if this Long's value is even. * Long#**isNegative**(): `boolean`<br /> Tests if this Long's value is negative. * Long#**isOdd**(): `boolean`<br /> Tests if this Long's value is odd. * Long#**isPositive**(): `boolean`<br /> Tests if this Long's value is positive. * Long#**isZero**/**eqz**(): `boolean`<br /> Tests if this Long's value equals zero. * Long#**lessThan**/**lt**(other: `Long | number | string`): `boolean`<br /> Tests if this Long's value is less than the specified's. * Long#**lessThanOrEqual**/**lte**/**le**(other: `Long | number | string`): `boolean`<br /> Tests if this Long's value is less than or equal the specified's. * Long#**modulo**/**mod**/**rem**(divisor: `Long | number | string`): `Long`<br /> Returns this Long modulo the specified. * Long#**multiply**/**mul**(multiplier: `Long | number | string`): `Long`<br /> Returns the product of this and the specified Long. * Long#**negate**/**neg**(): `Long`<br /> Negates this Long's value. * Long#**not**(): `Long`<br /> Returns the bitwise NOT of this Long. * Long#**notEquals**/**neq**/**ne**(other: `Long | number | string`): `boolean`<br /> Tests if this Long's value differs from the specified's. * Long#**or**(other: `Long | number | string`): `Long`<br /> Returns the bitwise OR of this Long and the specified. * Long#**shiftLeft**/**shl**(numBits: `Long | number | string`): `Long`<br /> Returns this Long with bits shifted to the left by the given amount. * Long#**shiftRight**/**shr**(numBits: `Long | number | string`): `Long`<br /> Returns this Long with bits arithmetically shifted to the right by the given amount. * Long#**shiftRightUnsigned**/**shru**/**shr_u**(numBits: `Long | number | string`): `Long`<br /> Returns this Long with bits logically shifted to the right by the given amount. * Long#**subtract**/**sub**(subtrahend: `Long | number | string`): `Long`<br /> Returns the difference of this and the specified Long. * Long#**toBytes**(le?: `boolean`): `number[]`<br /> Converts this Long to its byte representation. * Long#**toBytesLE**(): `number[]`<br /> Converts this Long to its little endian byte representation. * Long#**toBytesBE**(): `number[]`<br /> Converts this Long to its big endian byte representation. * Long#**toInt**(): `number`<br /> Converts the Long to a 32 bit integer, assuming it is a 32 bit integer. * Long#**toNumber**(): `number`<br /> Converts the Long to a the nearest floating-point representation of this value (double, 53 bit mantissa). * Long#**toSigned**(): `Long`<br /> Converts this Long to signed. * Long#**toString**(radix?: `number`): `string`<br /> Converts the Long to a string written in the specified radix. * Long#**toUnsigned**(): `Long`<br /> Converts this Long to unsigned. * Long#**xor**(other: `Long | number | string`): `Long`<br /> Returns the bitwise XOR of this Long and the given one. Building -------- To build an UMD bundle to `dist/long.js`, run: ``` $> npm install $> npm run build ``` Running the [tests](./tests): ``` $> npm test ``` apollo-server-demo/node_modules/long/package.json 0000644 0001750 0000144 00000001740 13235415742 021664 0 ustar andreh users { "name": "long", "version": "4.0.0", "author": "Daniel Wirtz <dcode@dcode.io>", "description": "A Long class for representing a 64-bit two's-complement integer value.", "main": "src/long.js", "repository": { "type": "git", "url": "https://github.com/dcodeIO/long.js.git" }, "bugs": { "url": "https://github.com/dcodeIO/long.js/issues" }, "keywords": [ "math" ], "dependencies": {}, "devDependencies": { "webpack": "^3.10.0" }, "license": "Apache-2.0", "scripts": { "build": "webpack", "test": "node tests" }, "files": [ "index.js", "LICENSE", "README.md", "src/long.js", "dist/long.js", "dist/long.js.map" ] ,"_resolved": "https://registry.npmjs.org/long/-/long-4.0.0.tgz" ,"_integrity": "sha512-XsP+KhQif4bjX1kbuSiySJFNAehNxgLb6hPRGJ9QsUr8ajHkuXGdrHmFUTUUXhDwVX2R5bY4JNZEwbUiMhV+MA==" ,"_from": "long@4.0.0" } apollo-server-demo/node_modules/sha.js/ 0000755 0001750 0000144 00000000000 14067647700 017630 5 ustar andreh users apollo-server-demo/node_modules/sha.js/bin.js 0000755 0001750 0000144 00000001737 14067647700 020751 0 ustar andreh users #! /usr/bin/env node var createHash = require('./browserify') var argv = process.argv.slice(2) function pipe (algorithm, s) { var start = Date.now() var hash = createHash(algorithm || 'sha1') s.on('data', function (data) { hash.update(data) }) s.on('end', function () { if (process.env.DEBUG) { return console.log(hash.digest('hex'), Date.now() - start) } console.log(hash.digest('hex')) }) } function usage () { console.error('sha.js [algorithm=sha1] [filename] # hash filename with algorithm') console.error('input | sha.js [algorithm=sha1] # hash stdin with algorithm') console.error('sha.js --help # display this message') } if (!process.stdin.isTTY) { pipe(argv[0], process.stdin) } else if (argv.length) { if (/--help|-h/.test(argv[0])) { usage() } else { var filename = argv.pop() var algorithm = argv.pop() pipe(algorithm, require('fs').createReadStream(filename)) } } else { usage() } apollo-server-demo/node_modules/sha.js/index.js 0000644 0001750 0000144 00000000724 14067647700 021300 0 ustar andreh users var exports = module.exports = function SHA (algorithm) { algorithm = algorithm.toLowerCase() var Algorithm = exports[algorithm] if (!Algorithm) throw new Error(algorithm + ' is not supported (we accept pull requests)') return new Algorithm() } exports.sha = require('./sha') exports.sha1 = require('./sha1') exports.sha224 = require('./sha224') exports.sha256 = require('./sha256') exports.sha384 = require('./sha384') exports.sha512 = require('./sha512') apollo-server-demo/node_modules/sha.js/LICENSE 0000644 0001750 0000144 00000004775 14067647700 020652 0 ustar andreh users Copyright (c) 2013-2018 sha.js contributors Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. Copyright (c) 1998 - 2009, Paul Johnston & Contributors All rights reserved. Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met: Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer. Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution. Neither the name of the author nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission. THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. apollo-server-demo/node_modules/sha.js/sha224.js 0000644 0001750 0000144 00000002103 14067647700 021165 0 ustar andreh users /** * A JavaScript implementation of the Secure Hash Algorithm, SHA-256, as defined * in FIPS 180-2 * Version 2.2-beta Copyright Angel Marin, Paul Johnston 2000 - 2009. * Other contributors: Greg Holt, Andrew Kepert, Ydnar, Lostinet * */ var inherits = require('inherits') var Sha256 = require('./sha256') var Hash = require('./hash') var Buffer = require('safe-buffer').Buffer var W = new Array(64) function Sha224 () { this.init() this._w = W // new Array(64) Hash.call(this, 64, 56) } inherits(Sha224, Sha256) Sha224.prototype.init = function () { this._a = 0xc1059ed8 this._b = 0x367cd507 this._c = 0x3070dd17 this._d = 0xf70e5939 this._e = 0xffc00b31 this._f = 0x68581511 this._g = 0x64f98fa7 this._h = 0xbefa4fa4 return this } Sha224.prototype._hash = function () { var H = Buffer.allocUnsafe(28) H.writeInt32BE(this._a, 0) H.writeInt32BE(this._b, 4) H.writeInt32BE(this._c, 8) H.writeInt32BE(this._d, 12) H.writeInt32BE(this._e, 16) H.writeInt32BE(this._f, 20) H.writeInt32BE(this._g, 24) return H } module.exports = Sha224 apollo-server-demo/node_modules/sha.js/sha1.js 0000644 0001750 0000144 00000003756 14067647700 021035 0 ustar andreh users /* * A JavaScript implementation of the Secure Hash Algorithm, SHA-1, as defined * in FIPS PUB 180-1 * Version 2.1a Copyright Paul Johnston 2000 - 2002. * Other contributors: Greg Holt, Andrew Kepert, Ydnar, Lostinet * Distributed under the BSD License * See http://pajhome.org.uk/crypt/md5 for details. */ var inherits = require('inherits') var Hash = require('./hash') var Buffer = require('safe-buffer').Buffer var K = [ 0x5a827999, 0x6ed9eba1, 0x8f1bbcdc | 0, 0xca62c1d6 | 0 ] var W = new Array(80) function Sha1 () { this.init() this._w = W Hash.call(this, 64, 56) } inherits(Sha1, Hash) Sha1.prototype.init = function () { this._a = 0x67452301 this._b = 0xefcdab89 this._c = 0x98badcfe this._d = 0x10325476 this._e = 0xc3d2e1f0 return this } function rotl1 (num) { return (num << 1) | (num >>> 31) } function rotl5 (num) { return (num << 5) | (num >>> 27) } function rotl30 (num) { return (num << 30) | (num >>> 2) } function ft (s, b, c, d) { if (s === 0) return (b & c) | ((~b) & d) if (s === 2) return (b & c) | (b & d) | (c & d) return b ^ c ^ d } Sha1.prototype._update = function (M) { var W = this._w var a = this._a | 0 var b = this._b | 0 var c = this._c | 0 var d = this._d | 0 var e = this._e | 0 for (var i = 0; i < 16; ++i) W[i] = M.readInt32BE(i * 4) for (; i < 80; ++i) W[i] = rotl1(W[i - 3] ^ W[i - 8] ^ W[i - 14] ^ W[i - 16]) for (var j = 0; j < 80; ++j) { var s = ~~(j / 20) var t = (rotl5(a) + ft(s, b, c, d) + e + W[j] + K[s]) | 0 e = d d = c c = rotl30(b) b = a a = t } this._a = (a + this._a) | 0 this._b = (b + this._b) | 0 this._c = (c + this._c) | 0 this._d = (d + this._d) | 0 this._e = (e + this._e) | 0 } Sha1.prototype._hash = function () { var H = Buffer.allocUnsafe(20) H.writeInt32BE(this._a | 0, 0) H.writeInt32BE(this._b | 0, 4) H.writeInt32BE(this._c | 0, 8) H.writeInt32BE(this._d | 0, 12) H.writeInt32BE(this._e | 0, 16) return H } module.exports = Sha1 apollo-server-demo/node_modules/sha.js/sha256.js 0000644 0001750 0000144 00000006313 14067647700 021201 0 ustar andreh users /** * A JavaScript implementation of the Secure Hash Algorithm, SHA-256, as defined * in FIPS 180-2 * Version 2.2-beta Copyright Angel Marin, Paul Johnston 2000 - 2009. * Other contributors: Greg Holt, Andrew Kepert, Ydnar, Lostinet * */ var inherits = require('inherits') var Hash = require('./hash') var Buffer = require('safe-buffer').Buffer var K = [ 0x428A2F98, 0x71374491, 0xB5C0FBCF, 0xE9B5DBA5, 0x3956C25B, 0x59F111F1, 0x923F82A4, 0xAB1C5ED5, 0xD807AA98, 0x12835B01, 0x243185BE, 0x550C7DC3, 0x72BE5D74, 0x80DEB1FE, 0x9BDC06A7, 0xC19BF174, 0xE49B69C1, 0xEFBE4786, 0x0FC19DC6, 0x240CA1CC, 0x2DE92C6F, 0x4A7484AA, 0x5CB0A9DC, 0x76F988DA, 0x983E5152, 0xA831C66D, 0xB00327C8, 0xBF597FC7, 0xC6E00BF3, 0xD5A79147, 0x06CA6351, 0x14292967, 0x27B70A85, 0x2E1B2138, 0x4D2C6DFC, 0x53380D13, 0x650A7354, 0x766A0ABB, 0x81C2C92E, 0x92722C85, 0xA2BFE8A1, 0xA81A664B, 0xC24B8B70, 0xC76C51A3, 0xD192E819, 0xD6990624, 0xF40E3585, 0x106AA070, 0x19A4C116, 0x1E376C08, 0x2748774C, 0x34B0BCB5, 0x391C0CB3, 0x4ED8AA4A, 0x5B9CCA4F, 0x682E6FF3, 0x748F82EE, 0x78A5636F, 0x84C87814, 0x8CC70208, 0x90BEFFFA, 0xA4506CEB, 0xBEF9A3F7, 0xC67178F2 ] var W = new Array(64) function Sha256 () { this.init() this._w = W // new Array(64) Hash.call(this, 64, 56) } inherits(Sha256, Hash) Sha256.prototype.init = function () { this._a = 0x6a09e667 this._b = 0xbb67ae85 this._c = 0x3c6ef372 this._d = 0xa54ff53a this._e = 0x510e527f this._f = 0x9b05688c this._g = 0x1f83d9ab this._h = 0x5be0cd19 return this } function ch (x, y, z) { return z ^ (x & (y ^ z)) } function maj (x, y, z) { return (x & y) | (z & (x | y)) } function sigma0 (x) { return (x >>> 2 | x << 30) ^ (x >>> 13 | x << 19) ^ (x >>> 22 | x << 10) } function sigma1 (x) { return (x >>> 6 | x << 26) ^ (x >>> 11 | x << 21) ^ (x >>> 25 | x << 7) } function gamma0 (x) { return (x >>> 7 | x << 25) ^ (x >>> 18 | x << 14) ^ (x >>> 3) } function gamma1 (x) { return (x >>> 17 | x << 15) ^ (x >>> 19 | x << 13) ^ (x >>> 10) } Sha256.prototype._update = function (M) { var W = this._w var a = this._a | 0 var b = this._b | 0 var c = this._c | 0 var d = this._d | 0 var e = this._e | 0 var f = this._f | 0 var g = this._g | 0 var h = this._h | 0 for (var i = 0; i < 16; ++i) W[i] = M.readInt32BE(i * 4) for (; i < 64; ++i) W[i] = (gamma1(W[i - 2]) + W[i - 7] + gamma0(W[i - 15]) + W[i - 16]) | 0 for (var j = 0; j < 64; ++j) { var T1 = (h + sigma1(e) + ch(e, f, g) + K[j] + W[j]) | 0 var T2 = (sigma0(a) + maj(a, b, c)) | 0 h = g g = f f = e e = (d + T1) | 0 d = c c = b b = a a = (T1 + T2) | 0 } this._a = (a + this._a) | 0 this._b = (b + this._b) | 0 this._c = (c + this._c) | 0 this._d = (d + this._d) | 0 this._e = (e + this._e) | 0 this._f = (f + this._f) | 0 this._g = (g + this._g) | 0 this._h = (h + this._h) | 0 } Sha256.prototype._hash = function () { var H = Buffer.allocUnsafe(32) H.writeInt32BE(this._a, 0) H.writeInt32BE(this._b, 4) H.writeInt32BE(this._c, 8) H.writeInt32BE(this._d, 12) H.writeInt32BE(this._e, 16) H.writeInt32BE(this._f, 20) H.writeInt32BE(this._g, 24) H.writeInt32BE(this._h, 28) return H } module.exports = Sha256 apollo-server-demo/node_modules/sha.js/README.md 0000644 0001750 0000144 00000003354 14067647700 021114 0 ustar andreh users # sha.js [](https://www.npmjs.org/package/sha.js) [](https://travis-ci.org/crypto-browserify/sha.js) [](https://david-dm.org/crypto-browserify/sha.js#info=dependencies) [](https://github.com/feross/standard) Node style `SHA` on pure JavaScript. ```js var shajs = require('sha.js') console.log(shajs('sha256').update('42').digest('hex')) // => 73475cb40a568e8da8a045ced110137e159f890ac4da883b6b17dc651b3a8049 console.log(new shajs.sha256().update('42').digest('hex')) // => 73475cb40a568e8da8a045ced110137e159f890ac4da883b6b17dc651b3a8049 var sha256stream = shajs('sha256') sha256stream.end('42') console.log(sha256stream.read().toString('hex')) // => 73475cb40a568e8da8a045ced110137e159f890ac4da883b6b17dc651b3a8049 ``` ## supported hashes `sha.js` currently implements: - SHA (SHA-0) -- **legacy, do not use in new systems** - SHA-1 -- **legacy, do not use in new systems** - SHA-224 - SHA-256 - SHA-384 - SHA-512 ## Not an actual stream Note, this doesn't actually implement a stream, but wrapping this in a stream is trivial. It does update incrementally, so you can hash things larger than RAM, as it uses a constant amount of memory (except when using base64 or utf8 encoding, see code comments). ## Acknowledgements This work is derived from Paul Johnston's [A JavaScript implementation of the Secure Hash Algorithm](http://pajhome.org.uk/crypt/md5/sha1.html). ## LICENSE [MIT](LICENSE) apollo-server-demo/node_modules/sha.js/sha.js 0000644 0001750 0000144 00000003571 14067647700 020747 0 ustar andreh users /* * A JavaScript implementation of the Secure Hash Algorithm, SHA-0, as defined * in FIPS PUB 180-1 * This source code is derived from sha1.js of the same repository. * The difference between SHA-0 and SHA-1 is just a bitwise rotate left * operation was added. */ var inherits = require('inherits') var Hash = require('./hash') var Buffer = require('safe-buffer').Buffer var K = [ 0x5a827999, 0x6ed9eba1, 0x8f1bbcdc | 0, 0xca62c1d6 | 0 ] var W = new Array(80) function Sha () { this.init() this._w = W Hash.call(this, 64, 56) } inherits(Sha, Hash) Sha.prototype.init = function () { this._a = 0x67452301 this._b = 0xefcdab89 this._c = 0x98badcfe this._d = 0x10325476 this._e = 0xc3d2e1f0 return this } function rotl5 (num) { return (num << 5) | (num >>> 27) } function rotl30 (num) { return (num << 30) | (num >>> 2) } function ft (s, b, c, d) { if (s === 0) return (b & c) | ((~b) & d) if (s === 2) return (b & c) | (b & d) | (c & d) return b ^ c ^ d } Sha.prototype._update = function (M) { var W = this._w var a = this._a | 0 var b = this._b | 0 var c = this._c | 0 var d = this._d | 0 var e = this._e | 0 for (var i = 0; i < 16; ++i) W[i] = M.readInt32BE(i * 4) for (; i < 80; ++i) W[i] = W[i - 3] ^ W[i - 8] ^ W[i - 14] ^ W[i - 16] for (var j = 0; j < 80; ++j) { var s = ~~(j / 20) var t = (rotl5(a) + ft(s, b, c, d) + e + W[j] + K[s]) | 0 e = d d = c c = rotl30(b) b = a a = t } this._a = (a + this._a) | 0 this._b = (b + this._b) | 0 this._c = (c + this._c) | 0 this._d = (d + this._d) | 0 this._e = (e + this._e) | 0 } Sha.prototype._hash = function () { var H = Buffer.allocUnsafe(20) H.writeInt32BE(this._a | 0, 0) H.writeInt32BE(this._b | 0, 4) H.writeInt32BE(this._c | 0, 8) H.writeInt32BE(this._d | 0, 12) H.writeInt32BE(this._e | 0, 16) return H } module.exports = Sha apollo-server-demo/node_modules/sha.js/sha512.js 0000644 0001750 0000144 00000016014 14067647700 021173 0 ustar andreh users var inherits = require('inherits') var Hash = require('./hash') var Buffer = require('safe-buffer').Buffer var K = [ 0x428a2f98, 0xd728ae22, 0x71374491, 0x23ef65cd, 0xb5c0fbcf, 0xec4d3b2f, 0xe9b5dba5, 0x8189dbbc, 0x3956c25b, 0xf348b538, 0x59f111f1, 0xb605d019, 0x923f82a4, 0xaf194f9b, 0xab1c5ed5, 0xda6d8118, 0xd807aa98, 0xa3030242, 0x12835b01, 0x45706fbe, 0x243185be, 0x4ee4b28c, 0x550c7dc3, 0xd5ffb4e2, 0x72be5d74, 0xf27b896f, 0x80deb1fe, 0x3b1696b1, 0x9bdc06a7, 0x25c71235, 0xc19bf174, 0xcf692694, 0xe49b69c1, 0x9ef14ad2, 0xefbe4786, 0x384f25e3, 0x0fc19dc6, 0x8b8cd5b5, 0x240ca1cc, 0x77ac9c65, 0x2de92c6f, 0x592b0275, 0x4a7484aa, 0x6ea6e483, 0x5cb0a9dc, 0xbd41fbd4, 0x76f988da, 0x831153b5, 0x983e5152, 0xee66dfab, 0xa831c66d, 0x2db43210, 0xb00327c8, 0x98fb213f, 0xbf597fc7, 0xbeef0ee4, 0xc6e00bf3, 0x3da88fc2, 0xd5a79147, 0x930aa725, 0x06ca6351, 0xe003826f, 0x14292967, 0x0a0e6e70, 0x27b70a85, 0x46d22ffc, 0x2e1b2138, 0x5c26c926, 0x4d2c6dfc, 0x5ac42aed, 0x53380d13, 0x9d95b3df, 0x650a7354, 0x8baf63de, 0x766a0abb, 0x3c77b2a8, 0x81c2c92e, 0x47edaee6, 0x92722c85, 0x1482353b, 0xa2bfe8a1, 0x4cf10364, 0xa81a664b, 0xbc423001, 0xc24b8b70, 0xd0f89791, 0xc76c51a3, 0x0654be30, 0xd192e819, 0xd6ef5218, 0xd6990624, 0x5565a910, 0xf40e3585, 0x5771202a, 0x106aa070, 0x32bbd1b8, 0x19a4c116, 0xb8d2d0c8, 0x1e376c08, 0x5141ab53, 0x2748774c, 0xdf8eeb99, 0x34b0bcb5, 0xe19b48a8, 0x391c0cb3, 0xc5c95a63, 0x4ed8aa4a, 0xe3418acb, 0x5b9cca4f, 0x7763e373, 0x682e6ff3, 0xd6b2b8a3, 0x748f82ee, 0x5defb2fc, 0x78a5636f, 0x43172f60, 0x84c87814, 0xa1f0ab72, 0x8cc70208, 0x1a6439ec, 0x90befffa, 0x23631e28, 0xa4506ceb, 0xde82bde9, 0xbef9a3f7, 0xb2c67915, 0xc67178f2, 0xe372532b, 0xca273ece, 0xea26619c, 0xd186b8c7, 0x21c0c207, 0xeada7dd6, 0xcde0eb1e, 0xf57d4f7f, 0xee6ed178, 0x06f067aa, 0x72176fba, 0x0a637dc5, 0xa2c898a6, 0x113f9804, 0xbef90dae, 0x1b710b35, 0x131c471b, 0x28db77f5, 0x23047d84, 0x32caab7b, 0x40c72493, 0x3c9ebe0a, 0x15c9bebc, 0x431d67c4, 0x9c100d4c, 0x4cc5d4be, 0xcb3e42b6, 0x597f299c, 0xfc657e2a, 0x5fcb6fab, 0x3ad6faec, 0x6c44198c, 0x4a475817 ] var W = new Array(160) function Sha512 () { this.init() this._w = W Hash.call(this, 128, 112) } inherits(Sha512, Hash) Sha512.prototype.init = function () { this._ah = 0x6a09e667 this._bh = 0xbb67ae85 this._ch = 0x3c6ef372 this._dh = 0xa54ff53a this._eh = 0x510e527f this._fh = 0x9b05688c this._gh = 0x1f83d9ab this._hh = 0x5be0cd19 this._al = 0xf3bcc908 this._bl = 0x84caa73b this._cl = 0xfe94f82b this._dl = 0x5f1d36f1 this._el = 0xade682d1 this._fl = 0x2b3e6c1f this._gl = 0xfb41bd6b this._hl = 0x137e2179 return this } function Ch (x, y, z) { return z ^ (x & (y ^ z)) } function maj (x, y, z) { return (x & y) | (z & (x | y)) } function sigma0 (x, xl) { return (x >>> 28 | xl << 4) ^ (xl >>> 2 | x << 30) ^ (xl >>> 7 | x << 25) } function sigma1 (x, xl) { return (x >>> 14 | xl << 18) ^ (x >>> 18 | xl << 14) ^ (xl >>> 9 | x << 23) } function Gamma0 (x, xl) { return (x >>> 1 | xl << 31) ^ (x >>> 8 | xl << 24) ^ (x >>> 7) } function Gamma0l (x, xl) { return (x >>> 1 | xl << 31) ^ (x >>> 8 | xl << 24) ^ (x >>> 7 | xl << 25) } function Gamma1 (x, xl) { return (x >>> 19 | xl << 13) ^ (xl >>> 29 | x << 3) ^ (x >>> 6) } function Gamma1l (x, xl) { return (x >>> 19 | xl << 13) ^ (xl >>> 29 | x << 3) ^ (x >>> 6 | xl << 26) } function getCarry (a, b) { return (a >>> 0) < (b >>> 0) ? 1 : 0 } Sha512.prototype._update = function (M) { var W = this._w var ah = this._ah | 0 var bh = this._bh | 0 var ch = this._ch | 0 var dh = this._dh | 0 var eh = this._eh | 0 var fh = this._fh | 0 var gh = this._gh | 0 var hh = this._hh | 0 var al = this._al | 0 var bl = this._bl | 0 var cl = this._cl | 0 var dl = this._dl | 0 var el = this._el | 0 var fl = this._fl | 0 var gl = this._gl | 0 var hl = this._hl | 0 for (var i = 0; i < 32; i += 2) { W[i] = M.readInt32BE(i * 4) W[i + 1] = M.readInt32BE(i * 4 + 4) } for (; i < 160; i += 2) { var xh = W[i - 15 * 2] var xl = W[i - 15 * 2 + 1] var gamma0 = Gamma0(xh, xl) var gamma0l = Gamma0l(xl, xh) xh = W[i - 2 * 2] xl = W[i - 2 * 2 + 1] var gamma1 = Gamma1(xh, xl) var gamma1l = Gamma1l(xl, xh) // W[i] = gamma0 + W[i - 7] + gamma1 + W[i - 16] var Wi7h = W[i - 7 * 2] var Wi7l = W[i - 7 * 2 + 1] var Wi16h = W[i - 16 * 2] var Wi16l = W[i - 16 * 2 + 1] var Wil = (gamma0l + Wi7l) | 0 var Wih = (gamma0 + Wi7h + getCarry(Wil, gamma0l)) | 0 Wil = (Wil + gamma1l) | 0 Wih = (Wih + gamma1 + getCarry(Wil, gamma1l)) | 0 Wil = (Wil + Wi16l) | 0 Wih = (Wih + Wi16h + getCarry(Wil, Wi16l)) | 0 W[i] = Wih W[i + 1] = Wil } for (var j = 0; j < 160; j += 2) { Wih = W[j] Wil = W[j + 1] var majh = maj(ah, bh, ch) var majl = maj(al, bl, cl) var sigma0h = sigma0(ah, al) var sigma0l = sigma0(al, ah) var sigma1h = sigma1(eh, el) var sigma1l = sigma1(el, eh) // t1 = h + sigma1 + ch + K[j] + W[j] var Kih = K[j] var Kil = K[j + 1] var chh = Ch(eh, fh, gh) var chl = Ch(el, fl, gl) var t1l = (hl + sigma1l) | 0 var t1h = (hh + sigma1h + getCarry(t1l, hl)) | 0 t1l = (t1l + chl) | 0 t1h = (t1h + chh + getCarry(t1l, chl)) | 0 t1l = (t1l + Kil) | 0 t1h = (t1h + Kih + getCarry(t1l, Kil)) | 0 t1l = (t1l + Wil) | 0 t1h = (t1h + Wih + getCarry(t1l, Wil)) | 0 // t2 = sigma0 + maj var t2l = (sigma0l + majl) | 0 var t2h = (sigma0h + majh + getCarry(t2l, sigma0l)) | 0 hh = gh hl = gl gh = fh gl = fl fh = eh fl = el el = (dl + t1l) | 0 eh = (dh + t1h + getCarry(el, dl)) | 0 dh = ch dl = cl ch = bh cl = bl bh = ah bl = al al = (t1l + t2l) | 0 ah = (t1h + t2h + getCarry(al, t1l)) | 0 } this._al = (this._al + al) | 0 this._bl = (this._bl + bl) | 0 this._cl = (this._cl + cl) | 0 this._dl = (this._dl + dl) | 0 this._el = (this._el + el) | 0 this._fl = (this._fl + fl) | 0 this._gl = (this._gl + gl) | 0 this._hl = (this._hl + hl) | 0 this._ah = (this._ah + ah + getCarry(this._al, al)) | 0 this._bh = (this._bh + bh + getCarry(this._bl, bl)) | 0 this._ch = (this._ch + ch + getCarry(this._cl, cl)) | 0 this._dh = (this._dh + dh + getCarry(this._dl, dl)) | 0 this._eh = (this._eh + eh + getCarry(this._el, el)) | 0 this._fh = (this._fh + fh + getCarry(this._fl, fl)) | 0 this._gh = (this._gh + gh + getCarry(this._gl, gl)) | 0 this._hh = (this._hh + hh + getCarry(this._hl, hl)) | 0 } Sha512.prototype._hash = function () { var H = Buffer.allocUnsafe(64) function writeInt64BE (h, l, offset) { H.writeInt32BE(h, offset) H.writeInt32BE(l, offset + 4) } writeInt64BE(this._ah, this._al, 0) writeInt64BE(this._bh, this._bl, 8) writeInt64BE(this._ch, this._cl, 16) writeInt64BE(this._dh, this._dl, 24) writeInt64BE(this._eh, this._el, 32) writeInt64BE(this._fh, this._fl, 40) writeInt64BE(this._gh, this._gl, 48) writeInt64BE(this._hh, this._hl, 56) return H } module.exports = Sha512 apollo-server-demo/node_modules/sha.js/package.json 0000644 0001750 0000144 00000002022 14067647700 022112 0 ustar andreh users { "name": "sha.js", "description": "Streamable SHA hashes in pure javascript", "version": "2.4.11", "homepage": "https://github.com/crypto-browserify/sha.js", "repository": { "type": "git", "url": "git://github.com/crypto-browserify/sha.js.git" }, "dependencies": { "inherits": "^2.0.1", "safe-buffer": "^5.0.1" }, "devDependencies": { "buffer": "~2.3.2", "hash-test-vectors": "^1.3.1", "standard": "^10.0.2", "tape": "~2.3.2", "typedarray": "0.0.6" }, "bin": "./bin.js", "scripts": { "prepublish": "npm ls && npm run unit", "lint": "standard", "test": "npm run lint && npm run unit", "unit": "set -e; for t in test/*.js; do node $t; done;" }, "author": "Dominic Tarr <dominic.tarr@gmail.com> (dominictarr.com)", "license": "(MIT AND BSD-3-Clause)" ,"_resolved": "https://registry.npmjs.org/sha.js/-/sha.js-2.4.11.tgz" ,"_integrity": "sha512-QMEp5B7cftE7APOjk5Y6xgrbWu+WkLVQwk8JNjZ8nKRciZaByEW6MubieAiToS7+dwvrjGhH8jRXz3MVd0AYqQ==" ,"_from": "sha.js@2.4.11" } apollo-server-demo/node_modules/sha.js/test/ 0000755 0001750 0000144 00000000000 14067647700 020607 5 ustar andreh users apollo-server-demo/node_modules/sha.js/test/test.js 0000644 0001750 0000144 00000004715 14067647700 022133 0 ustar andreh users var crypto = require('crypto') var tape = require('tape') var Sha1 = require('../').sha1 var inputs = [ ['', 'ascii'], ['abc', 'ascii'], ['123', 'ascii'], ['123456789abcdef123456789abcdef123456789abcdef123456789abcdef', 'ascii'], ['123456789abcdef123456789abcdef123456789abcdef123456789abc', 'ascii'], ['123456789abcdef123456789abcdef123456789abcdef123456789ab', 'ascii'], ['0123456789abcdef0123456789abcdef0123456789abcdef0123456789abcde', 'ascii'], ['0123456789abcdef0123456789abcdef0123456789abcdef0123456789abcdef', 'ascii'], ['foobarbaz', 'ascii'] ] tape("hash is the same as node's crypto", function (t) { inputs.forEach(function (v) { var a = new Sha1().update(v[0], v[1]).digest('hex') var e = crypto.createHash('sha1').update(v[0], v[1]).digest('hex') console.log(a, '==', e) t.equal(a, e) }) t.end() }) tape('call update multiple times', function (t) { inputs.forEach(function (v) { var hash = new Sha1() var _hash = crypto.createHash('sha1') for (var i = 0; i < v[0].length; i = (i + 1) * 2) { var s = v[0].substring(i, (i + 1) * 2) hash.update(s, v[1]) _hash.update(s, v[1]) } var a = hash.digest('hex') var e = _hash.digest('hex') console.log(a, '==', e) t.equal(a, e) }) t.end() }) tape('call update twice', function (t) { var _hash = crypto.createHash('sha1') var hash = new Sha1() _hash.update('foo', 'ascii') hash.update('foo', 'ascii') _hash.update('bar', 'ascii') hash.update('bar', 'ascii') _hash.update('baz', 'ascii') hash.update('baz', 'ascii') var a = hash.digest('hex') var e = _hash.digest('hex') t.equal(a, e) t.end() }) tape('hex encoding', function (t) { inputs.forEach(function (v) { var hash = new Sha1() var _hash = crypto.createHash('sha1') for (var i = 0; i < v[0].length; i = (i + 1) * 2) { var s = v[0].substring(i, (i + 1) * 2) hash.update(Buffer.from(s, 'ascii').toString('hex'), 'hex') _hash.update(Buffer.from(s, 'ascii').toString('hex'), 'hex') } var a = hash.digest('hex') var e = _hash.digest('hex') console.log(a, '==', e) t.equal(a, e) }) t.end() }) tape('call digest for more than MAX_UINT32 bits of data', function (t) { var _hash = crypto.createHash('sha1') var hash = new Sha1() var bigData = Buffer.alloc(0x1ffffffff / 8) hash.update(bigData) _hash.update(bigData) var a = hash.digest('hex') var e = _hash.digest('hex') t.equal(a, e) t.end() }) apollo-server-demo/node_modules/sha.js/test/vectors.js 0000644 0001750 0000144 00000003146 14067647700 022636 0 ustar andreh users var tape = require('tape') var vectors = require('hash-test-vectors') // var from = require('bops/typedarray/from') var Buffer = require('safe-buffer').Buffer var createHash = require('../') function makeTest (alg, i, verbose) { var v = vectors[i] tape(alg + ': NIST vector ' + i, function (t) { if (verbose) { console.log(v) console.log('VECTOR', i) console.log('INPUT', v.input) console.log(Buffer.from(v.input, 'base64').toString('hex')) } var buf = Buffer.from(v.input, 'base64') t.equal(createHash(alg).update(buf).digest('hex'), v[alg]) i = ~~(buf.length / 2) var buf1 = buf.slice(0, i) var buf2 = buf.slice(i, buf.length) console.log(buf1.length, buf2.length, buf.length) console.log(createHash(alg)._block.length) t.equal( createHash(alg) .update(buf1) .update(buf2) .digest('hex'), v[alg] ) var j, buf3 i = ~~(buf.length / 3) j = ~~(buf.length * 2 / 3) buf1 = buf.slice(0, i) buf2 = buf.slice(i, j) buf3 = buf.slice(j, buf.length) t.equal( createHash(alg) .update(buf1) .update(buf2) .update(buf3) .digest('hex'), v[alg] ) setTimeout(function () { // avoid "too much recursion" errors in tape in firefox t.end() }) }) } if (process.argv[2]) { makeTest(process.argv[2], parseInt(process.argv[3], 10), true) } else { vectors.forEach(function (v, i) { makeTest('sha', i) makeTest('sha1', i) makeTest('sha224', i) makeTest('sha256', i) makeTest('sha384', i) makeTest('sha512', i) }) } apollo-server-demo/node_modules/sha.js/test/hash.js 0000644 0001750 0000144 00000003024 14067647700 022067 0 ustar andreh users var tape = require('tape') var Hash = require('../hash') var hex = '0A1B2C3D4E5F6G7H' function equal (t, a, b) { t.equal(a.length, b.length) t.equal(a.toString('hex'), b.toString('hex')) } var hexBuf = Buffer.from('0A1B2C3D4E5F6G7H', 'utf8') var count16 = { strings: ['0A1B2C3D4E5F6G7H'], buffers: [ hexBuf, Buffer.from('80000000000000000000000000000080', 'hex') ] } var empty = { strings: [''], buffers: [ Buffer.from('80000000000000000000000000000000', 'hex') ] } var multi = { strings: ['abcd', 'efhijk', 'lmnopq'], buffers: [ Buffer.from('abcdefhijklmnopq', 'ascii'), Buffer.from('80000000000000000000000000000080', 'hex') ] } var long = { strings: [hex + hex], buffers: [ hexBuf, hexBuf, Buffer.from('80000000000000000000000000000100', 'hex') ] } function makeTest (name, data) { tape(name, function (t) { var h = new Hash(16, 8) var hash = Buffer.alloc(20) var n = 2 var expected = data.buffers.slice() // t.plan(expected.length + 1) h._update = function (block) { var e = expected.shift() equal(t, block, e) if (n < 0) { throw new Error('expecting only 2 calls to _update') } } h._hash = function () { return hash } data.strings.forEach(function (string) { h.update(string, 'ascii') }) equal(t, h.digest(), hash) t.end() }) } makeTest('Hash#update 1 in 1', count16) makeTest('empty Hash#update', empty) makeTest('Hash#update 1 in 3', multi) makeTest('Hash#update 2 in 1', long) apollo-server-demo/node_modules/sha.js/sha384.js 0000644 0001750 0000144 00000002217 14067647700 021202 0 ustar andreh users var inherits = require('inherits') var SHA512 = require('./sha512') var Hash = require('./hash') var Buffer = require('safe-buffer').Buffer var W = new Array(160) function Sha384 () { this.init() this._w = W Hash.call(this, 128, 112) } inherits(Sha384, SHA512) Sha384.prototype.init = function () { this._ah = 0xcbbb9d5d this._bh = 0x629a292a this._ch = 0x9159015a this._dh = 0x152fecd8 this._eh = 0x67332667 this._fh = 0x8eb44a87 this._gh = 0xdb0c2e0d this._hh = 0x47b5481d this._al = 0xc1059ed8 this._bl = 0x367cd507 this._cl = 0x3070dd17 this._dl = 0xf70e5939 this._el = 0xffc00b31 this._fl = 0x68581511 this._gl = 0x64f98fa7 this._hl = 0xbefa4fa4 return this } Sha384.prototype._hash = function () { var H = Buffer.allocUnsafe(48) function writeInt64BE (h, l, offset) { H.writeInt32BE(h, offset) H.writeInt32BE(l, offset + 4) } writeInt64BE(this._ah, this._al, 0) writeInt64BE(this._bh, this._bl, 8) writeInt64BE(this._ch, this._cl, 16) writeInt64BE(this._dh, this._dl, 24) writeInt64BE(this._eh, this._el, 32) writeInt64BE(this._fh, this._fl, 40) return H } module.exports = Sha384 apollo-server-demo/node_modules/sha.js/hash.js 0000644 0001750 0000144 00000003541 14067647700 021114 0 ustar andreh users var Buffer = require('safe-buffer').Buffer // prototype class for hash functions function Hash (blockSize, finalSize) { this._block = Buffer.alloc(blockSize) this._finalSize = finalSize this._blockSize = blockSize this._len = 0 } Hash.prototype.update = function (data, enc) { if (typeof data === 'string') { enc = enc || 'utf8' data = Buffer.from(data, enc) } var block = this._block var blockSize = this._blockSize var length = data.length var accum = this._len for (var offset = 0; offset < length;) { var assigned = accum % blockSize var remainder = Math.min(length - offset, blockSize - assigned) for (var i = 0; i < remainder; i++) { block[assigned + i] = data[offset + i] } accum += remainder offset += remainder if ((accum % blockSize) === 0) { this._update(block) } } this._len += length return this } Hash.prototype.digest = function (enc) { var rem = this._len % this._blockSize this._block[rem] = 0x80 // zero (rem + 1) trailing bits, where (rem + 1) is the smallest // non-negative solution to the equation (length + 1 + (rem + 1)) === finalSize mod blockSize this._block.fill(0, rem + 1) if (rem >= this._finalSize) { this._update(this._block) this._block.fill(0) } var bits = this._len * 8 // uint32 if (bits <= 0xffffffff) { this._block.writeUInt32BE(bits, this._blockSize - 4) // uint64 } else { var lowBits = (bits & 0xffffffff) >>> 0 var highBits = (bits - lowBits) / 0x100000000 this._block.writeUInt32BE(highBits, this._blockSize - 8) this._block.writeUInt32BE(lowBits, this._blockSize - 4) } this._update(this._block) var hash = this._hash() return enc ? hash.toString(enc) : hash } Hash.prototype._update = function () { throw new Error('_update must be implemented by subclass') } module.exports = Hash apollo-server-demo/node_modules/sha.js/.travis.yml 0000644 0001750 0000144 00000000327 14067647700 021743 0 ustar andreh users sudo: false os: - linux language: node_js node_js: - "4" - "5" - "6" - "7" env: matrix: - TEST_SUITE=unit matrix: include: - node_js: "7" env: TEST_SUITE=lint script: npm run $TEST_SUITE apollo-server-demo/node_modules/range-parser/ 0000755 0001750 0000144 00000000000 14067647700 021030 5 ustar andreh users apollo-server-demo/node_modules/range-parser/index.js 0000644 0001750 0000144 00000005524 03560116604 022471 0 ustar andreh users /*! * range-parser * Copyright(c) 2012-2014 TJ Holowaychuk * Copyright(c) 2015-2016 Douglas Christopher Wilson * MIT Licensed */ 'use strict' /** * Module exports. * @public */ module.exports = rangeParser /** * Parse "Range" header `str` relative to the given file `size`. * * @param {Number} size * @param {String} str * @param {Object} [options] * @return {Array} * @public */ function rangeParser (size, str, options) { if (typeof str !== 'string') { throw new TypeError('argument str must be a string') } var index = str.indexOf('=') if (index === -1) { return -2 } // split the range string var arr = str.slice(index + 1).split(',') var ranges = [] // add ranges type ranges.type = str.slice(0, index) // parse all ranges for (var i = 0; i < arr.length; i++) { var range = arr[i].split('-') var start = parseInt(range[0], 10) var end = parseInt(range[1], 10) // -nnn if (isNaN(start)) { start = size - end end = size - 1 // nnn- } else if (isNaN(end)) { end = size - 1 } // limit last-byte-pos to current length if (end > size - 1) { end = size - 1 } // invalid or unsatisifiable if (isNaN(start) || isNaN(end) || start > end || start < 0) { continue } // add range ranges.push({ start: start, end: end }) } if (ranges.length < 1) { // unsatisifiable return -1 } return options && options.combine ? combineRanges(ranges) : ranges } /** * Combine overlapping & adjacent ranges. * @private */ function combineRanges (ranges) { var ordered = ranges.map(mapWithIndex).sort(sortByRangeStart) for (var j = 0, i = 1; i < ordered.length; i++) { var range = ordered[i] var current = ordered[j] if (range.start > current.end + 1) { // next range ordered[++j] = range } else if (range.end > current.end) { // extend range current.end = range.end current.index = Math.min(current.index, range.index) } } // trim ordered array ordered.length = j + 1 // generate combined range var combined = ordered.sort(sortByRangeIndex).map(mapWithoutIndex) // copy ranges type combined.type = ranges.type return combined } /** * Map function to add index value to ranges. * @private */ function mapWithIndex (range, index) { return { start: range.start, end: range.end, index: index } } /** * Map function to remove index value from ranges. * @private */ function mapWithoutIndex (range) { return { start: range.start, end: range.end } } /** * Sort function to sort ranges by index. * @private */ function sortByRangeIndex (a, b) { return a.index - b.index } /** * Sort function to sort ranges by start position. * @private */ function sortByRangeStart (a, b) { return a.start - b.start } apollo-server-demo/node_modules/range-parser/LICENSE 0000644 0001750 0000144 00000002232 03560116604 022022 0 ustar andreh users (The MIT License) Copyright (c) 2012-2014 TJ Holowaychuk <tj@vision-media.ca> Copyright (c) 2015-2016 Douglas Christopher Wilson <doug@somethingdoug.com Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the 'Software'), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/range-parser/HISTORY.md 0000644 0001750 0000144 00000001625 03560116604 022505 0 ustar andreh users 1.2.1 / 2019-05-10 ================== * Improve error when `str` is not a string 1.2.0 / 2016-06-01 ================== * Add `combine` option to combine overlapping ranges 1.1.0 / 2016-05-13 ================== * Fix incorrectly returning -1 when there is at least one valid range * perf: remove internal function 1.0.3 / 2015-10-29 ================== * perf: enable strict mode 1.0.2 / 2014-09-08 ================== * Support Node.js 0.6 1.0.1 / 2014-09-07 ================== * Move repository to jshttp 1.0.0 / 2013-12-11 ================== * Add repository to package.json * Add MIT license 0.0.4 / 2012-06-17 ================== * Change ret -1 for unsatisfiable and -2 when invalid 0.0.3 / 2012-06-17 ================== * Fix last-byte-pos default to len - 1 0.0.2 / 2012-06-14 ================== * Add `.type` 0.0.1 / 2012-06-11 ================== * Initial release apollo-server-demo/node_modules/range-parser/README.md 0000644 0001750 0000144 00000004346 03560116604 022304 0 ustar andreh users # range-parser [![NPM Version][npm-version-image]][npm-url] [![NPM Downloads][npm-downloads-image]][npm-url] [![Node.js Version][node-image]][node-url] [![Build Status][travis-image]][travis-url] [![Test Coverage][coveralls-image]][coveralls-url] Range header field parser. ## Installation This is a [Node.js](https://nodejs.org/en/) module available through the [npm registry](https://www.npmjs.com/). Installation is done using the [`npm install` command](https://docs.npmjs.com/getting-started/installing-npm-packages-locally): ```sh $ npm install range-parser ``` ## API <!-- eslint-disable no-unused-vars --> ```js var parseRange = require('range-parser') ``` ### parseRange(size, header, options) Parse the given `header` string where `size` is the maximum size of the resource. An array of ranges will be returned or negative numbers indicating an error parsing. * `-2` signals a malformed header string * `-1` signals an unsatisfiable range <!-- eslint-disable no-undef --> ```js // parse header from request var range = parseRange(size, req.headers.range) // the type of the range if (range.type === 'bytes') { // the ranges range.forEach(function (r) { // do something with r.start and r.end }) } ``` #### Options These properties are accepted in the options object. ##### combine Specifies if overlapping & adjacent ranges should be combined, defaults to `false`. When `true`, ranges will be combined and returned as if they were specified that way in the header. <!-- eslint-disable no-undef --> ```js parseRange(100, 'bytes=50-55,0-10,5-10,56-60', { combine: true }) // => [ // { start: 0, end: 10 }, // { start: 50, end: 60 } // ] ``` ## License [MIT](LICENSE) [coveralls-image]: https://badgen.net/coveralls/c/github/jshttp/range-parser/master [coveralls-url]: https://coveralls.io/r/jshttp/range-parser?branch=master [node-image]: https://badgen.net/npm/node/range-parser [node-url]: https://nodejs.org/en/download [npm-downloads-image]: https://badgen.net/npm/dm/range-parser [npm-url]: https://npmjs.org/package/range-parser [npm-version-image]: https://badgen.net/npm/v/range-parser [travis-image]: https://badgen.net/travis/jshttp/range-parser/master [travis-url]: https://travis-ci.org/jshttp/range-parser apollo-server-demo/node_modules/range-parser/package.json 0000644 0001750 0000144 00000002601 03560116604 023303 0 ustar andreh users { "name": "range-parser", "author": "TJ Holowaychuk <tj@vision-media.ca> (http://tjholowaychuk.com)", "description": "Range header field string parser", "version": "1.2.1", "contributors": [ "Douglas Christopher Wilson <doug@somethingdoug.com>", "James Wyatt Cready <wyatt.cready@lanetix.com>", "Jonathan Ong <me@jongleberry.com> (http://jongleberry.com)" ], "license": "MIT", "keywords": [ "range", "parser", "http" ], "repository": "jshttp/range-parser", "devDependencies": { "deep-equal": "1.0.1", "eslint": "5.16.0", "eslint-config-standard": "12.0.0", "eslint-plugin-markdown": "1.0.0", "eslint-plugin-import": "2.17.2", "eslint-plugin-node": "8.0.1", "eslint-plugin-promise": "4.1.1", "eslint-plugin-standard": "4.0.0", "mocha": "6.1.4", "nyc": "14.1.1" }, "files": [ "HISTORY.md", "LICENSE", "index.js" ], "engines": { "node": ">= 0.6" }, "scripts": { "lint": "eslint --plugin markdown --ext js,md .", "test": "mocha --reporter spec", "test-cov": "nyc --reporter=html --reporter=text npm test", "test-travis": "nyc --reporter=text npm test" } ,"_resolved": "https://registry.npmjs.org/range-parser/-/range-parser-1.2.1.tgz" ,"_integrity": "sha512-Hrgsx+orqoygnmhFbKaHE6c296J+HTAQXoxEF6gNupROmmGJRoyzfG3ccAveqCBrwr/2yxQ5BVd/GTl5agOwSg==" ,"_from": "range-parser@1.2.1" } apollo-server-demo/node_modules/lodash.sortby/ 0000755 0001750 0000144 00000000000 14067647701 021236 5 ustar andreh users apollo-server-demo/node_modules/lodash.sortby/index.js 0000644 0001750 0000144 00000215640 12753655065 022715 0 ustar andreh users /** * lodash (Custom Build) <https://lodash.com/> * Build: `lodash modularize exports="npm" -o ./` * Copyright jQuery Foundation and other contributors <https://jquery.org/> * Released under MIT license <https://lodash.com/license> * Based on Underscore.js 1.8.3 <http://underscorejs.org/LICENSE> * Copyright Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors */ /** Used as the size to enable large array optimizations. */ var LARGE_ARRAY_SIZE = 200; /** Used as the `TypeError` message for "Functions" methods. */ var FUNC_ERROR_TEXT = 'Expected a function'; /** Used to stand-in for `undefined` hash values. */ var HASH_UNDEFINED = '__lodash_hash_undefined__'; /** Used to compose bitmasks for comparison styles. */ var UNORDERED_COMPARE_FLAG = 1, PARTIAL_COMPARE_FLAG = 2; /** Used as references for various `Number` constants. */ var INFINITY = 1 / 0, MAX_SAFE_INTEGER = 9007199254740991; /** `Object#toString` result references. */ var argsTag = '[object Arguments]', arrayTag = '[object Array]', boolTag = '[object Boolean]', dateTag = '[object Date]', errorTag = '[object Error]', funcTag = '[object Function]', genTag = '[object GeneratorFunction]', mapTag = '[object Map]', numberTag = '[object Number]', objectTag = '[object Object]', promiseTag = '[object Promise]', regexpTag = '[object RegExp]', setTag = '[object Set]', stringTag = '[object String]', symbolTag = '[object Symbol]', weakMapTag = '[object WeakMap]'; var arrayBufferTag = '[object ArrayBuffer]', dataViewTag = '[object DataView]', float32Tag = '[object Float32Array]', float64Tag = '[object Float64Array]', int8Tag = '[object Int8Array]', int16Tag = '[object Int16Array]', int32Tag = '[object Int32Array]', uint8Tag = '[object Uint8Array]', uint8ClampedTag = '[object Uint8ClampedArray]', uint16Tag = '[object Uint16Array]', uint32Tag = '[object Uint32Array]'; /** Used to match property names within property paths. */ var reIsDeepProp = /\.|\[(?:[^[\]]*|(["'])(?:(?!\1)[^\\]|\\.)*?\1)\]/, reIsPlainProp = /^\w*$/, reLeadingDot = /^\./, rePropName = /[^.[\]]+|\[(?:(-?\d+(?:\.\d+)?)|(["'])((?:(?!\2)[^\\]|\\.)*?)\2)\]|(?=(?:\.|\[\])(?:\.|\[\]|$))/g; /** * Used to match `RegExp` * [syntax characters](http://ecma-international.org/ecma-262/7.0/#sec-patterns). */ var reRegExpChar = /[\\^$.*+?()[\]{}|]/g; /** Used to match backslashes in property paths. */ var reEscapeChar = /\\(\\)?/g; /** Used to detect host constructors (Safari). */ var reIsHostCtor = /^\[object .+?Constructor\]$/; /** Used to detect unsigned integer values. */ var reIsUint = /^(?:0|[1-9]\d*)$/; /** Used to identify `toStringTag` values of typed arrays. */ var typedArrayTags = {}; typedArrayTags[float32Tag] = typedArrayTags[float64Tag] = typedArrayTags[int8Tag] = typedArrayTags[int16Tag] = typedArrayTags[int32Tag] = typedArrayTags[uint8Tag] = typedArrayTags[uint8ClampedTag] = typedArrayTags[uint16Tag] = typedArrayTags[uint32Tag] = true; typedArrayTags[argsTag] = typedArrayTags[arrayTag] = typedArrayTags[arrayBufferTag] = typedArrayTags[boolTag] = typedArrayTags[dataViewTag] = typedArrayTags[dateTag] = typedArrayTags[errorTag] = typedArrayTags[funcTag] = typedArrayTags[mapTag] = typedArrayTags[numberTag] = typedArrayTags[objectTag] = typedArrayTags[regexpTag] = typedArrayTags[setTag] = typedArrayTags[stringTag] = typedArrayTags[weakMapTag] = false; /** Detect free variable `global` from Node.js. */ var freeGlobal = typeof global == 'object' && global && global.Object === Object && global; /** Detect free variable `self`. */ var freeSelf = typeof self == 'object' && self && self.Object === Object && self; /** Used as a reference to the global object. */ var root = freeGlobal || freeSelf || Function('return this')(); /** Detect free variable `exports`. */ var freeExports = typeof exports == 'object' && exports && !exports.nodeType && exports; /** Detect free variable `module`. */ var freeModule = freeExports && typeof module == 'object' && module && !module.nodeType && module; /** Detect the popular CommonJS extension `module.exports`. */ var moduleExports = freeModule && freeModule.exports === freeExports; /** Detect free variable `process` from Node.js. */ var freeProcess = moduleExports && freeGlobal.process; /** Used to access faster Node.js helpers. */ var nodeUtil = (function() { try { return freeProcess && freeProcess.binding('util'); } catch (e) {} }()); /* Node.js helper references. */ var nodeIsTypedArray = nodeUtil && nodeUtil.isTypedArray; /** * A faster alternative to `Function#apply`, this function invokes `func` * with the `this` binding of `thisArg` and the arguments of `args`. * * @private * @param {Function} func The function to invoke. * @param {*} thisArg The `this` binding of `func`. * @param {Array} args The arguments to invoke `func` with. * @returns {*} Returns the result of `func`. */ function apply(func, thisArg, args) { switch (args.length) { case 0: return func.call(thisArg); case 1: return func.call(thisArg, args[0]); case 2: return func.call(thisArg, args[0], args[1]); case 3: return func.call(thisArg, args[0], args[1], args[2]); } return func.apply(thisArg, args); } /** * A specialized version of `_.map` for arrays without support for iteratee * shorthands. * * @private * @param {Array} [array] The array to iterate over. * @param {Function} iteratee The function invoked per iteration. * @returns {Array} Returns the new mapped array. */ function arrayMap(array, iteratee) { var index = -1, length = array ? array.length : 0, result = Array(length); while (++index < length) { result[index] = iteratee(array[index], index, array); } return result; } /** * Appends the elements of `values` to `array`. * * @private * @param {Array} array The array to modify. * @param {Array} values The values to append. * @returns {Array} Returns `array`. */ function arrayPush(array, values) { var index = -1, length = values.length, offset = array.length; while (++index < length) { array[offset + index] = values[index]; } return array; } /** * A specialized version of `_.some` for arrays without support for iteratee * shorthands. * * @private * @param {Array} [array] The array to iterate over. * @param {Function} predicate The function invoked per iteration. * @returns {boolean} Returns `true` if any element passes the predicate check, * else `false`. */ function arraySome(array, predicate) { var index = -1, length = array ? array.length : 0; while (++index < length) { if (predicate(array[index], index, array)) { return true; } } return false; } /** * The base implementation of `_.property` without support for deep paths. * * @private * @param {string} key The key of the property to get. * @returns {Function} Returns the new accessor function. */ function baseProperty(key) { return function(object) { return object == null ? undefined : object[key]; }; } /** * The base implementation of `_.sortBy` which uses `comparer` to define the * sort order of `array` and replaces criteria objects with their corresponding * values. * * @private * @param {Array} array The array to sort. * @param {Function} comparer The function to define sort order. * @returns {Array} Returns `array`. */ function baseSortBy(array, comparer) { var length = array.length; array.sort(comparer); while (length--) { array[length] = array[length].value; } return array; } /** * The base implementation of `_.times` without support for iteratee shorthands * or max array length checks. * * @private * @param {number} n The number of times to invoke `iteratee`. * @param {Function} iteratee The function invoked per iteration. * @returns {Array} Returns the array of results. */ function baseTimes(n, iteratee) { var index = -1, result = Array(n); while (++index < n) { result[index] = iteratee(index); } return result; } /** * The base implementation of `_.unary` without support for storing metadata. * * @private * @param {Function} func The function to cap arguments for. * @returns {Function} Returns the new capped function. */ function baseUnary(func) { return function(value) { return func(value); }; } /** * Gets the value at `key` of `object`. * * @private * @param {Object} [object] The object to query. * @param {string} key The key of the property to get. * @returns {*} Returns the property value. */ function getValue(object, key) { return object == null ? undefined : object[key]; } /** * Checks if `value` is a host object in IE < 9. * * @private * @param {*} value The value to check. * @returns {boolean} Returns `true` if `value` is a host object, else `false`. */ function isHostObject(value) { // Many host objects are `Object` objects that can coerce to strings // despite having improperly defined `toString` methods. var result = false; if (value != null && typeof value.toString != 'function') { try { result = !!(value + ''); } catch (e) {} } return result; } /** * Converts `map` to its key-value pairs. * * @private * @param {Object} map The map to convert. * @returns {Array} Returns the key-value pairs. */ function mapToArray(map) { var index = -1, result = Array(map.size); map.forEach(function(value, key) { result[++index] = [key, value]; }); return result; } /** * Creates a unary function that invokes `func` with its argument transformed. * * @private * @param {Function} func The function to wrap. * @param {Function} transform The argument transform. * @returns {Function} Returns the new function. */ function overArg(func, transform) { return function(arg) { return func(transform(arg)); }; } /** * Converts `set` to an array of its values. * * @private * @param {Object} set The set to convert. * @returns {Array} Returns the values. */ function setToArray(set) { var index = -1, result = Array(set.size); set.forEach(function(value) { result[++index] = value; }); return result; } /** Used for built-in method references. */ var arrayProto = Array.prototype, funcProto = Function.prototype, objectProto = Object.prototype; /** Used to detect overreaching core-js shims. */ var coreJsData = root['__core-js_shared__']; /** Used to detect methods masquerading as native. */ var maskSrcKey = (function() { var uid = /[^.]+$/.exec(coreJsData && coreJsData.keys && coreJsData.keys.IE_PROTO || ''); return uid ? ('Symbol(src)_1.' + uid) : ''; }()); /** Used to resolve the decompiled source of functions. */ var funcToString = funcProto.toString; /** Used to check objects for own properties. */ var hasOwnProperty = objectProto.hasOwnProperty; /** * Used to resolve the * [`toStringTag`](http://ecma-international.org/ecma-262/7.0/#sec-object.prototype.tostring) * of values. */ var objectToString = objectProto.toString; /** Used to detect if a method is native. */ var reIsNative = RegExp('^' + funcToString.call(hasOwnProperty).replace(reRegExpChar, '\\$&') .replace(/hasOwnProperty|(function).*?(?=\\\()| for .+?(?=\\\])/g, '$1.*?') + '$' ); /** Built-in value references. */ var Symbol = root.Symbol, Uint8Array = root.Uint8Array, propertyIsEnumerable = objectProto.propertyIsEnumerable, splice = arrayProto.splice, spreadableSymbol = Symbol ? Symbol.isConcatSpreadable : undefined; /* Built-in method references for those with the same name as other `lodash` methods. */ var nativeKeys = overArg(Object.keys, Object), nativeMax = Math.max; /* Built-in method references that are verified to be native. */ var DataView = getNative(root, 'DataView'), Map = getNative(root, 'Map'), Promise = getNative(root, 'Promise'), Set = getNative(root, 'Set'), WeakMap = getNative(root, 'WeakMap'), nativeCreate = getNative(Object, 'create'); /** Used to detect maps, sets, and weakmaps. */ var dataViewCtorString = toSource(DataView), mapCtorString = toSource(Map), promiseCtorString = toSource(Promise), setCtorString = toSource(Set), weakMapCtorString = toSource(WeakMap); /** Used to convert symbols to primitives and strings. */ var symbolProto = Symbol ? Symbol.prototype : undefined, symbolValueOf = symbolProto ? symbolProto.valueOf : undefined, symbolToString = symbolProto ? symbolProto.toString : undefined; /** * Creates a hash object. * * @private * @constructor * @param {Array} [entries] The key-value pairs to cache. */ function Hash(entries) { var index = -1, length = entries ? entries.length : 0; this.clear(); while (++index < length) { var entry = entries[index]; this.set(entry[0], entry[1]); } } /** * Removes all key-value entries from the hash. * * @private * @name clear * @memberOf Hash */ function hashClear() { this.__data__ = nativeCreate ? nativeCreate(null) : {}; } /** * Removes `key` and its value from the hash. * * @private * @name delete * @memberOf Hash * @param {Object} hash The hash to modify. * @param {string} key The key of the value to remove. * @returns {boolean} Returns `true` if the entry was removed, else `false`. */ function hashDelete(key) { return this.has(key) && delete this.__data__[key]; } /** * Gets the hash value for `key`. * * @private * @name get * @memberOf Hash * @param {string} key The key of the value to get. * @returns {*} Returns the entry value. */ function hashGet(key) { var data = this.__data__; if (nativeCreate) { var result = data[key]; return result === HASH_UNDEFINED ? undefined : result; } return hasOwnProperty.call(data, key) ? data[key] : undefined; } /** * Checks if a hash value for `key` exists. * * @private * @name has * @memberOf Hash * @param {string} key The key of the entry to check. * @returns {boolean} Returns `true` if an entry for `key` exists, else `false`. */ function hashHas(key) { var data = this.__data__; return nativeCreate ? data[key] !== undefined : hasOwnProperty.call(data, key); } /** * Sets the hash `key` to `value`. * * @private * @name set * @memberOf Hash * @param {string} key The key of the value to set. * @param {*} value The value to set. * @returns {Object} Returns the hash instance. */ function hashSet(key, value) { var data = this.__data__; data[key] = (nativeCreate && value === undefined) ? HASH_UNDEFINED : value; return this; } // Add methods to `Hash`. Hash.prototype.clear = hashClear; Hash.prototype['delete'] = hashDelete; Hash.prototype.get = hashGet; Hash.prototype.has = hashHas; Hash.prototype.set = hashSet; /** * Creates an list cache object. * * @private * @constructor * @param {Array} [entries] The key-value pairs to cache. */ function ListCache(entries) { var index = -1, length = entries ? entries.length : 0; this.clear(); while (++index < length) { var entry = entries[index]; this.set(entry[0], entry[1]); } } /** * Removes all key-value entries from the list cache. * * @private * @name clear * @memberOf ListCache */ function listCacheClear() { this.__data__ = []; } /** * Removes `key` and its value from the list cache. * * @private * @name delete * @memberOf ListCache * @param {string} key The key of the value to remove. * @returns {boolean} Returns `true` if the entry was removed, else `false`. */ function listCacheDelete(key) { var data = this.__data__, index = assocIndexOf(data, key); if (index < 0) { return false; } var lastIndex = data.length - 1; if (index == lastIndex) { data.pop(); } else { splice.call(data, index, 1); } return true; } /** * Gets the list cache value for `key`. * * @private * @name get * @memberOf ListCache * @param {string} key The key of the value to get. * @returns {*} Returns the entry value. */ function listCacheGet(key) { var data = this.__data__, index = assocIndexOf(data, key); return index < 0 ? undefined : data[index][1]; } /** * Checks if a list cache value for `key` exists. * * @private * @name has * @memberOf ListCache * @param {string} key The key of the entry to check. * @returns {boolean} Returns `true` if an entry for `key` exists, else `false`. */ function listCacheHas(key) { return assocIndexOf(this.__data__, key) > -1; } /** * Sets the list cache `key` to `value`. * * @private * @name set * @memberOf ListCache * @param {string} key The key of the value to set. * @param {*} value The value to set. * @returns {Object} Returns the list cache instance. */ function listCacheSet(key, value) { var data = this.__data__, index = assocIndexOf(data, key); if (index < 0) { data.push([key, value]); } else { data[index][1] = value; } return this; } // Add methods to `ListCache`. ListCache.prototype.clear = listCacheClear; ListCache.prototype['delete'] = listCacheDelete; ListCache.prototype.get = listCacheGet; ListCache.prototype.has = listCacheHas; ListCache.prototype.set = listCacheSet; /** * Creates a map cache object to store key-value pairs. * * @private * @constructor * @param {Array} [entries] The key-value pairs to cache. */ function MapCache(entries) { var index = -1, length = entries ? entries.length : 0; this.clear(); while (++index < length) { var entry = entries[index]; this.set(entry[0], entry[1]); } } /** * Removes all key-value entries from the map. * * @private * @name clear * @memberOf MapCache */ function mapCacheClear() { this.__data__ = { 'hash': new Hash, 'map': new (Map || ListCache), 'string': new Hash }; } /** * Removes `key` and its value from the map. * * @private * @name delete * @memberOf MapCache * @param {string} key The key of the value to remove. * @returns {boolean} Returns `true` if the entry was removed, else `false`. */ function mapCacheDelete(key) { return getMapData(this, key)['delete'](key); } /** * Gets the map value for `key`. * * @private * @name get * @memberOf MapCache * @param {string} key The key of the value to get. * @returns {*} Returns the entry value. */ function mapCacheGet(key) { return getMapData(this, key).get(key); } /** * Checks if a map value for `key` exists. * * @private * @name has * @memberOf MapCache * @param {string} key The key of the entry to check. * @returns {boolean} Returns `true` if an entry for `key` exists, else `false`. */ function mapCacheHas(key) { return getMapData(this, key).has(key); } /** * Sets the map `key` to `value`. * * @private * @name set * @memberOf MapCache * @param {string} key The key of the value to set. * @param {*} value The value to set. * @returns {Object} Returns the map cache instance. */ function mapCacheSet(key, value) { getMapData(this, key).set(key, value); return this; } // Add methods to `MapCache`. MapCache.prototype.clear = mapCacheClear; MapCache.prototype['delete'] = mapCacheDelete; MapCache.prototype.get = mapCacheGet; MapCache.prototype.has = mapCacheHas; MapCache.prototype.set = mapCacheSet; /** * * Creates an array cache object to store unique values. * * @private * @constructor * @param {Array} [values] The values to cache. */ function SetCache(values) { var index = -1, length = values ? values.length : 0; this.__data__ = new MapCache; while (++index < length) { this.add(values[index]); } } /** * Adds `value` to the array cache. * * @private * @name add * @memberOf SetCache * @alias push * @param {*} value The value to cache. * @returns {Object} Returns the cache instance. */ function setCacheAdd(value) { this.__data__.set(value, HASH_UNDEFINED); return this; } /** * Checks if `value` is in the array cache. * * @private * @name has * @memberOf SetCache * @param {*} value The value to search for. * @returns {number} Returns `true` if `value` is found, else `false`. */ function setCacheHas(value) { return this.__data__.has(value); } // Add methods to `SetCache`. SetCache.prototype.add = SetCache.prototype.push = setCacheAdd; SetCache.prototype.has = setCacheHas; /** * Creates a stack cache object to store key-value pairs. * * @private * @constructor * @param {Array} [entries] The key-value pairs to cache. */ function Stack(entries) { this.__data__ = new ListCache(entries); } /** * Removes all key-value entries from the stack. * * @private * @name clear * @memberOf Stack */ function stackClear() { this.__data__ = new ListCache; } /** * Removes `key` and its value from the stack. * * @private * @name delete * @memberOf Stack * @param {string} key The key of the value to remove. * @returns {boolean} Returns `true` if the entry was removed, else `false`. */ function stackDelete(key) { return this.__data__['delete'](key); } /** * Gets the stack value for `key`. * * @private * @name get * @memberOf Stack * @param {string} key The key of the value to get. * @returns {*} Returns the entry value. */ function stackGet(key) { return this.__data__.get(key); } /** * Checks if a stack value for `key` exists. * * @private * @name has * @memberOf Stack * @param {string} key The key of the entry to check. * @returns {boolean} Returns `true` if an entry for `key` exists, else `false`. */ function stackHas(key) { return this.__data__.has(key); } /** * Sets the stack `key` to `value`. * * @private * @name set * @memberOf Stack * @param {string} key The key of the value to set. * @param {*} value The value to set. * @returns {Object} Returns the stack cache instance. */ function stackSet(key, value) { var cache = this.__data__; if (cache instanceof ListCache) { var pairs = cache.__data__; if (!Map || (pairs.length < LARGE_ARRAY_SIZE - 1)) { pairs.push([key, value]); return this; } cache = this.__data__ = new MapCache(pairs); } cache.set(key, value); return this; } // Add methods to `Stack`. Stack.prototype.clear = stackClear; Stack.prototype['delete'] = stackDelete; Stack.prototype.get = stackGet; Stack.prototype.has = stackHas; Stack.prototype.set = stackSet; /** * Creates an array of the enumerable property names of the array-like `value`. * * @private * @param {*} value The value to query. * @param {boolean} inherited Specify returning inherited property names. * @returns {Array} Returns the array of property names. */ function arrayLikeKeys(value, inherited) { // Safari 8.1 makes `arguments.callee` enumerable in strict mode. // Safari 9 makes `arguments.length` enumerable in strict mode. var result = (isArray(value) || isArguments(value)) ? baseTimes(value.length, String) : []; var length = result.length, skipIndexes = !!length; for (var key in value) { if ((inherited || hasOwnProperty.call(value, key)) && !(skipIndexes && (key == 'length' || isIndex(key, length)))) { result.push(key); } } return result; } /** * Gets the index at which the `key` is found in `array` of key-value pairs. * * @private * @param {Array} array The array to inspect. * @param {*} key The key to search for. * @returns {number} Returns the index of the matched value, else `-1`. */ function assocIndexOf(array, key) { var length = array.length; while (length--) { if (eq(array[length][0], key)) { return length; } } return -1; } /** * The base implementation of `_.forEach` without support for iteratee shorthands. * * @private * @param {Array|Object} collection The collection to iterate over. * @param {Function} iteratee The function invoked per iteration. * @returns {Array|Object} Returns `collection`. */ var baseEach = createBaseEach(baseForOwn); /** * The base implementation of `_.flatten` with support for restricting flattening. * * @private * @param {Array} array The array to flatten. * @param {number} depth The maximum recursion depth. * @param {boolean} [predicate=isFlattenable] The function invoked per iteration. * @param {boolean} [isStrict] Restrict to values that pass `predicate` checks. * @param {Array} [result=[]] The initial result value. * @returns {Array} Returns the new flattened array. */ function baseFlatten(array, depth, predicate, isStrict, result) { var index = -1, length = array.length; predicate || (predicate = isFlattenable); result || (result = []); while (++index < length) { var value = array[index]; if (depth > 0 && predicate(value)) { if (depth > 1) { // Recursively flatten arrays (susceptible to call stack limits). baseFlatten(value, depth - 1, predicate, isStrict, result); } else { arrayPush(result, value); } } else if (!isStrict) { result[result.length] = value; } } return result; } /** * The base implementation of `baseForOwn` which iterates over `object` * properties returned by `keysFunc` and invokes `iteratee` for each property. * Iteratee functions may exit iteration early by explicitly returning `false`. * * @private * @param {Object} object The object to iterate over. * @param {Function} iteratee The function invoked per iteration. * @param {Function} keysFunc The function to get the keys of `object`. * @returns {Object} Returns `object`. */ var baseFor = createBaseFor(); /** * The base implementation of `_.forOwn` without support for iteratee shorthands. * * @private * @param {Object} object The object to iterate over. * @param {Function} iteratee The function invoked per iteration. * @returns {Object} Returns `object`. */ function baseForOwn(object, iteratee) { return object && baseFor(object, iteratee, keys); } /** * The base implementation of `_.get` without support for default values. * * @private * @param {Object} object The object to query. * @param {Array|string} path The path of the property to get. * @returns {*} Returns the resolved value. */ function baseGet(object, path) { path = isKey(path, object) ? [path] : castPath(path); var index = 0, length = path.length; while (object != null && index < length) { object = object[toKey(path[index++])]; } return (index && index == length) ? object : undefined; } /** * The base implementation of `getTag`. * * @private * @param {*} value The value to query. * @returns {string} Returns the `toStringTag`. */ function baseGetTag(value) { return objectToString.call(value); } /** * The base implementation of `_.hasIn` without support for deep paths. * * @private * @param {Object} [object] The object to query. * @param {Array|string} key The key to check. * @returns {boolean} Returns `true` if `key` exists, else `false`. */ function baseHasIn(object, key) { return object != null && key in Object(object); } /** * The base implementation of `_.isEqual` which supports partial comparisons * and tracks traversed objects. * * @private * @param {*} value The value to compare. * @param {*} other The other value to compare. * @param {Function} [customizer] The function to customize comparisons. * @param {boolean} [bitmask] The bitmask of comparison flags. * The bitmask may be composed of the following flags: * 1 - Unordered comparison * 2 - Partial comparison * @param {Object} [stack] Tracks traversed `value` and `other` objects. * @returns {boolean} Returns `true` if the values are equivalent, else `false`. */ function baseIsEqual(value, other, customizer, bitmask, stack) { if (value === other) { return true; } if (value == null || other == null || (!isObject(value) && !isObjectLike(other))) { return value !== value && other !== other; } return baseIsEqualDeep(value, other, baseIsEqual, customizer, bitmask, stack); } /** * A specialized version of `baseIsEqual` for arrays and objects which performs * deep comparisons and tracks traversed objects enabling objects with circular * references to be compared. * * @private * @param {Object} object The object to compare. * @param {Object} other The other object to compare. * @param {Function} equalFunc The function to determine equivalents of values. * @param {Function} [customizer] The function to customize comparisons. * @param {number} [bitmask] The bitmask of comparison flags. See `baseIsEqual` * for more details. * @param {Object} [stack] Tracks traversed `object` and `other` objects. * @returns {boolean} Returns `true` if the objects are equivalent, else `false`. */ function baseIsEqualDeep(object, other, equalFunc, customizer, bitmask, stack) { var objIsArr = isArray(object), othIsArr = isArray(other), objTag = arrayTag, othTag = arrayTag; if (!objIsArr) { objTag = getTag(object); objTag = objTag == argsTag ? objectTag : objTag; } if (!othIsArr) { othTag = getTag(other); othTag = othTag == argsTag ? objectTag : othTag; } var objIsObj = objTag == objectTag && !isHostObject(object), othIsObj = othTag == objectTag && !isHostObject(other), isSameTag = objTag == othTag; if (isSameTag && !objIsObj) { stack || (stack = new Stack); return (objIsArr || isTypedArray(object)) ? equalArrays(object, other, equalFunc, customizer, bitmask, stack) : equalByTag(object, other, objTag, equalFunc, customizer, bitmask, stack); } if (!(bitmask & PARTIAL_COMPARE_FLAG)) { var objIsWrapped = objIsObj && hasOwnProperty.call(object, '__wrapped__'), othIsWrapped = othIsObj && hasOwnProperty.call(other, '__wrapped__'); if (objIsWrapped || othIsWrapped) { var objUnwrapped = objIsWrapped ? object.value() : object, othUnwrapped = othIsWrapped ? other.value() : other; stack || (stack = new Stack); return equalFunc(objUnwrapped, othUnwrapped, customizer, bitmask, stack); } } if (!isSameTag) { return false; } stack || (stack = new Stack); return equalObjects(object, other, equalFunc, customizer, bitmask, stack); } /** * The base implementation of `_.isMatch` without support for iteratee shorthands. * * @private * @param {Object} object The object to inspect. * @param {Object} source The object of property values to match. * @param {Array} matchData The property names, values, and compare flags to match. * @param {Function} [customizer] The function to customize comparisons. * @returns {boolean} Returns `true` if `object` is a match, else `false`. */ function baseIsMatch(object, source, matchData, customizer) { var index = matchData.length, length = index, noCustomizer = !customizer; if (object == null) { return !length; } object = Object(object); while (index--) { var data = matchData[index]; if ((noCustomizer && data[2]) ? data[1] !== object[data[0]] : !(data[0] in object) ) { return false; } } while (++index < length) { data = matchData[index]; var key = data[0], objValue = object[key], srcValue = data[1]; if (noCustomizer && data[2]) { if (objValue === undefined && !(key in object)) { return false; } } else { var stack = new Stack; if (customizer) { var result = customizer(objValue, srcValue, key, object, source, stack); } if (!(result === undefined ? baseIsEqual(srcValue, objValue, customizer, UNORDERED_COMPARE_FLAG | PARTIAL_COMPARE_FLAG, stack) : result )) { return false; } } } return true; } /** * The base implementation of `_.isNative` without bad shim checks. * * @private * @param {*} value The value to check. * @returns {boolean} Returns `true` if `value` is a native function, * else `false`. */ function baseIsNative(value) { if (!isObject(value) || isMasked(value)) { return false; } var pattern = (isFunction(value) || isHostObject(value)) ? reIsNative : reIsHostCtor; return pattern.test(toSource(value)); } /** * The base implementation of `_.isTypedArray` without Node.js optimizations. * * @private * @param {*} value The value to check. * @returns {boolean} Returns `true` if `value` is a typed array, else `false`. */ function baseIsTypedArray(value) { return isObjectLike(value) && isLength(value.length) && !!typedArrayTags[objectToString.call(value)]; } /** * The base implementation of `_.iteratee`. * * @private * @param {*} [value=_.identity] The value to convert to an iteratee. * @returns {Function} Returns the iteratee. */ function baseIteratee(value) { // Don't store the `typeof` result in a variable to avoid a JIT bug in Safari 9. // See https://bugs.webkit.org/show_bug.cgi?id=156034 for more details. if (typeof value == 'function') { return value; } if (value == null) { return identity; } if (typeof value == 'object') { return isArray(value) ? baseMatchesProperty(value[0], value[1]) : baseMatches(value); } return property(value); } /** * The base implementation of `_.keys` which doesn't treat sparse arrays as dense. * * @private * @param {Object} object The object to query. * @returns {Array} Returns the array of property names. */ function baseKeys(object) { if (!isPrototype(object)) { return nativeKeys(object); } var result = []; for (var key in Object(object)) { if (hasOwnProperty.call(object, key) && key != 'constructor') { result.push(key); } } return result; } /** * The base implementation of `_.map` without support for iteratee shorthands. * * @private * @param {Array|Object} collection The collection to iterate over. * @param {Function} iteratee The function invoked per iteration. * @returns {Array} Returns the new mapped array. */ function baseMap(collection, iteratee) { var index = -1, result = isArrayLike(collection) ? Array(collection.length) : []; baseEach(collection, function(value, key, collection) { result[++index] = iteratee(value, key, collection); }); return result; } /** * The base implementation of `_.matches` which doesn't clone `source`. * * @private * @param {Object} source The object of property values to match. * @returns {Function} Returns the new spec function. */ function baseMatches(source) { var matchData = getMatchData(source); if (matchData.length == 1 && matchData[0][2]) { return matchesStrictComparable(matchData[0][0], matchData[0][1]); } return function(object) { return object === source || baseIsMatch(object, source, matchData); }; } /** * The base implementation of `_.matchesProperty` which doesn't clone `srcValue`. * * @private * @param {string} path The path of the property to get. * @param {*} srcValue The value to match. * @returns {Function} Returns the new spec function. */ function baseMatchesProperty(path, srcValue) { if (isKey(path) && isStrictComparable(srcValue)) { return matchesStrictComparable(toKey(path), srcValue); } return function(object) { var objValue = get(object, path); return (objValue === undefined && objValue === srcValue) ? hasIn(object, path) : baseIsEqual(srcValue, objValue, undefined, UNORDERED_COMPARE_FLAG | PARTIAL_COMPARE_FLAG); }; } /** * The base implementation of `_.orderBy` without param guards. * * @private * @param {Array|Object} collection The collection to iterate over. * @param {Function[]|Object[]|string[]} iteratees The iteratees to sort by. * @param {string[]} orders The sort orders of `iteratees`. * @returns {Array} Returns the new sorted array. */ function baseOrderBy(collection, iteratees, orders) { var index = -1; iteratees = arrayMap(iteratees.length ? iteratees : [identity], baseUnary(baseIteratee)); var result = baseMap(collection, function(value, key, collection) { var criteria = arrayMap(iteratees, function(iteratee) { return iteratee(value); }); return { 'criteria': criteria, 'index': ++index, 'value': value }; }); return baseSortBy(result, function(object, other) { return compareMultiple(object, other, orders); }); } /** * A specialized version of `baseProperty` which supports deep paths. * * @private * @param {Array|string} path The path of the property to get. * @returns {Function} Returns the new accessor function. */ function basePropertyDeep(path) { return function(object) { return baseGet(object, path); }; } /** * The base implementation of `_.rest` which doesn't validate or coerce arguments. * * @private * @param {Function} func The function to apply a rest parameter to. * @param {number} [start=func.length-1] The start position of the rest parameter. * @returns {Function} Returns the new function. */ function baseRest(func, start) { start = nativeMax(start === undefined ? (func.length - 1) : start, 0); return function() { var args = arguments, index = -1, length = nativeMax(args.length - start, 0), array = Array(length); while (++index < length) { array[index] = args[start + index]; } index = -1; var otherArgs = Array(start + 1); while (++index < start) { otherArgs[index] = args[index]; } otherArgs[start] = array; return apply(func, this, otherArgs); }; } /** * The base implementation of `_.toString` which doesn't convert nullish * values to empty strings. * * @private * @param {*} value The value to process. * @returns {string} Returns the string. */ function baseToString(value) { // Exit early for strings to avoid a performance hit in some environments. if (typeof value == 'string') { return value; } if (isSymbol(value)) { return symbolToString ? symbolToString.call(value) : ''; } var result = (value + ''); return (result == '0' && (1 / value) == -INFINITY) ? '-0' : result; } /** * Casts `value` to a path array if it's not one. * * @private * @param {*} value The value to inspect. * @returns {Array} Returns the cast property path array. */ function castPath(value) { return isArray(value) ? value : stringToPath(value); } /** * Compares values to sort them in ascending order. * * @private * @param {*} value The value to compare. * @param {*} other The other value to compare. * @returns {number} Returns the sort order indicator for `value`. */ function compareAscending(value, other) { if (value !== other) { var valIsDefined = value !== undefined, valIsNull = value === null, valIsReflexive = value === value, valIsSymbol = isSymbol(value); var othIsDefined = other !== undefined, othIsNull = other === null, othIsReflexive = other === other, othIsSymbol = isSymbol(other); if ((!othIsNull && !othIsSymbol && !valIsSymbol && value > other) || (valIsSymbol && othIsDefined && othIsReflexive && !othIsNull && !othIsSymbol) || (valIsNull && othIsDefined && othIsReflexive) || (!valIsDefined && othIsReflexive) || !valIsReflexive) { return 1; } if ((!valIsNull && !valIsSymbol && !othIsSymbol && value < other) || (othIsSymbol && valIsDefined && valIsReflexive && !valIsNull && !valIsSymbol) || (othIsNull && valIsDefined && valIsReflexive) || (!othIsDefined && valIsReflexive) || !othIsReflexive) { return -1; } } return 0; } /** * Used by `_.orderBy` to compare multiple properties of a value to another * and stable sort them. * * If `orders` is unspecified, all values are sorted in ascending order. Otherwise, * specify an order of "desc" for descending or "asc" for ascending sort order * of corresponding values. * * @private * @param {Object} object The object to compare. * @param {Object} other The other object to compare. * @param {boolean[]|string[]} orders The order to sort by for each property. * @returns {number} Returns the sort order indicator for `object`. */ function compareMultiple(object, other, orders) { var index = -1, objCriteria = object.criteria, othCriteria = other.criteria, length = objCriteria.length, ordersLength = orders.length; while (++index < length) { var result = compareAscending(objCriteria[index], othCriteria[index]); if (result) { if (index >= ordersLength) { return result; } var order = orders[index]; return result * (order == 'desc' ? -1 : 1); } } // Fixes an `Array#sort` bug in the JS engine embedded in Adobe applications // that causes it, under certain circumstances, to provide the same value for // `object` and `other`. See https://github.com/jashkenas/underscore/pull/1247 // for more details. // // This also ensures a stable sort in V8 and other engines. // See https://bugs.chromium.org/p/v8/issues/detail?id=90 for more details. return object.index - other.index; } /** * Creates a `baseEach` or `baseEachRight` function. * * @private * @param {Function} eachFunc The function to iterate over a collection. * @param {boolean} [fromRight] Specify iterating from right to left. * @returns {Function} Returns the new base function. */ function createBaseEach(eachFunc, fromRight) { return function(collection, iteratee) { if (collection == null) { return collection; } if (!isArrayLike(collection)) { return eachFunc(collection, iteratee); } var length = collection.length, index = fromRight ? length : -1, iterable = Object(collection); while ((fromRight ? index-- : ++index < length)) { if (iteratee(iterable[index], index, iterable) === false) { break; } } return collection; }; } /** * Creates a base function for methods like `_.forIn` and `_.forOwn`. * * @private * @param {boolean} [fromRight] Specify iterating from right to left. * @returns {Function} Returns the new base function. */ function createBaseFor(fromRight) { return function(object, iteratee, keysFunc) { var index = -1, iterable = Object(object), props = keysFunc(object), length = props.length; while (length--) { var key = props[fromRight ? length : ++index]; if (iteratee(iterable[key], key, iterable) === false) { break; } } return object; }; } /** * A specialized version of `baseIsEqualDeep` for arrays with support for * partial deep comparisons. * * @private * @param {Array} array The array to compare. * @param {Array} other The other array to compare. * @param {Function} equalFunc The function to determine equivalents of values. * @param {Function} customizer The function to customize comparisons. * @param {number} bitmask The bitmask of comparison flags. See `baseIsEqual` * for more details. * @param {Object} stack Tracks traversed `array` and `other` objects. * @returns {boolean} Returns `true` if the arrays are equivalent, else `false`. */ function equalArrays(array, other, equalFunc, customizer, bitmask, stack) { var isPartial = bitmask & PARTIAL_COMPARE_FLAG, arrLength = array.length, othLength = other.length; if (arrLength != othLength && !(isPartial && othLength > arrLength)) { return false; } // Assume cyclic values are equal. var stacked = stack.get(array); if (stacked && stack.get(other)) { return stacked == other; } var index = -1, result = true, seen = (bitmask & UNORDERED_COMPARE_FLAG) ? new SetCache : undefined; stack.set(array, other); stack.set(other, array); // Ignore non-index properties. while (++index < arrLength) { var arrValue = array[index], othValue = other[index]; if (customizer) { var compared = isPartial ? customizer(othValue, arrValue, index, other, array, stack) : customizer(arrValue, othValue, index, array, other, stack); } if (compared !== undefined) { if (compared) { continue; } result = false; break; } // Recursively compare arrays (susceptible to call stack limits). if (seen) { if (!arraySome(other, function(othValue, othIndex) { if (!seen.has(othIndex) && (arrValue === othValue || equalFunc(arrValue, othValue, customizer, bitmask, stack))) { return seen.add(othIndex); } })) { result = false; break; } } else if (!( arrValue === othValue || equalFunc(arrValue, othValue, customizer, bitmask, stack) )) { result = false; break; } } stack['delete'](array); stack['delete'](other); return result; } /** * A specialized version of `baseIsEqualDeep` for comparing objects of * the same `toStringTag`. * * **Note:** This function only supports comparing values with tags of * `Boolean`, `Date`, `Error`, `Number`, `RegExp`, or `String`. * * @private * @param {Object} object The object to compare. * @param {Object} other The other object to compare. * @param {string} tag The `toStringTag` of the objects to compare. * @param {Function} equalFunc The function to determine equivalents of values. * @param {Function} customizer The function to customize comparisons. * @param {number} bitmask The bitmask of comparison flags. See `baseIsEqual` * for more details. * @param {Object} stack Tracks traversed `object` and `other` objects. * @returns {boolean} Returns `true` if the objects are equivalent, else `false`. */ function equalByTag(object, other, tag, equalFunc, customizer, bitmask, stack) { switch (tag) { case dataViewTag: if ((object.byteLength != other.byteLength) || (object.byteOffset != other.byteOffset)) { return false; } object = object.buffer; other = other.buffer; case arrayBufferTag: if ((object.byteLength != other.byteLength) || !equalFunc(new Uint8Array(object), new Uint8Array(other))) { return false; } return true; case boolTag: case dateTag: case numberTag: // Coerce booleans to `1` or `0` and dates to milliseconds. // Invalid dates are coerced to `NaN`. return eq(+object, +other); case errorTag: return object.name == other.name && object.message == other.message; case regexpTag: case stringTag: // Coerce regexes to strings and treat strings, primitives and objects, // as equal. See http://www.ecma-international.org/ecma-262/7.0/#sec-regexp.prototype.tostring // for more details. return object == (other + ''); case mapTag: var convert = mapToArray; case setTag: var isPartial = bitmask & PARTIAL_COMPARE_FLAG; convert || (convert = setToArray); if (object.size != other.size && !isPartial) { return false; } // Assume cyclic values are equal. var stacked = stack.get(object); if (stacked) { return stacked == other; } bitmask |= UNORDERED_COMPARE_FLAG; // Recursively compare objects (susceptible to call stack limits). stack.set(object, other); var result = equalArrays(convert(object), convert(other), equalFunc, customizer, bitmask, stack); stack['delete'](object); return result; case symbolTag: if (symbolValueOf) { return symbolValueOf.call(object) == symbolValueOf.call(other); } } return false; } /** * A specialized version of `baseIsEqualDeep` for objects with support for * partial deep comparisons. * * @private * @param {Object} object The object to compare. * @param {Object} other The other object to compare. * @param {Function} equalFunc The function to determine equivalents of values. * @param {Function} customizer The function to customize comparisons. * @param {number} bitmask The bitmask of comparison flags. See `baseIsEqual` * for more details. * @param {Object} stack Tracks traversed `object` and `other` objects. * @returns {boolean} Returns `true` if the objects are equivalent, else `false`. */ function equalObjects(object, other, equalFunc, customizer, bitmask, stack) { var isPartial = bitmask & PARTIAL_COMPARE_FLAG, objProps = keys(object), objLength = objProps.length, othProps = keys(other), othLength = othProps.length; if (objLength != othLength && !isPartial) { return false; } var index = objLength; while (index--) { var key = objProps[index]; if (!(isPartial ? key in other : hasOwnProperty.call(other, key))) { return false; } } // Assume cyclic values are equal. var stacked = stack.get(object); if (stacked && stack.get(other)) { return stacked == other; } var result = true; stack.set(object, other); stack.set(other, object); var skipCtor = isPartial; while (++index < objLength) { key = objProps[index]; var objValue = object[key], othValue = other[key]; if (customizer) { var compared = isPartial ? customizer(othValue, objValue, key, other, object, stack) : customizer(objValue, othValue, key, object, other, stack); } // Recursively compare objects (susceptible to call stack limits). if (!(compared === undefined ? (objValue === othValue || equalFunc(objValue, othValue, customizer, bitmask, stack)) : compared )) { result = false; break; } skipCtor || (skipCtor = key == 'constructor'); } if (result && !skipCtor) { var objCtor = object.constructor, othCtor = other.constructor; // Non `Object` object instances with different constructors are not equal. if (objCtor != othCtor && ('constructor' in object && 'constructor' in other) && !(typeof objCtor == 'function' && objCtor instanceof objCtor && typeof othCtor == 'function' && othCtor instanceof othCtor)) { result = false; } } stack['delete'](object); stack['delete'](other); return result; } /** * Gets the data for `map`. * * @private * @param {Object} map The map to query. * @param {string} key The reference key. * @returns {*} Returns the map data. */ function getMapData(map, key) { var data = map.__data__; return isKeyable(key) ? data[typeof key == 'string' ? 'string' : 'hash'] : data.map; } /** * Gets the property names, values, and compare flags of `object`. * * @private * @param {Object} object The object to query. * @returns {Array} Returns the match data of `object`. */ function getMatchData(object) { var result = keys(object), length = result.length; while (length--) { var key = result[length], value = object[key]; result[length] = [key, value, isStrictComparable(value)]; } return result; } /** * Gets the native function at `key` of `object`. * * @private * @param {Object} object The object to query. * @param {string} key The key of the method to get. * @returns {*} Returns the function if it's native, else `undefined`. */ function getNative(object, key) { var value = getValue(object, key); return baseIsNative(value) ? value : undefined; } /** * Gets the `toStringTag` of `value`. * * @private * @param {*} value The value to query. * @returns {string} Returns the `toStringTag`. */ var getTag = baseGetTag; // Fallback for data views, maps, sets, and weak maps in IE 11, // for data views in Edge < 14, and promises in Node.js. if ((DataView && getTag(new DataView(new ArrayBuffer(1))) != dataViewTag) || (Map && getTag(new Map) != mapTag) || (Promise && getTag(Promise.resolve()) != promiseTag) || (Set && getTag(new Set) != setTag) || (WeakMap && getTag(new WeakMap) != weakMapTag)) { getTag = function(value) { var result = objectToString.call(value), Ctor = result == objectTag ? value.constructor : undefined, ctorString = Ctor ? toSource(Ctor) : undefined; if (ctorString) { switch (ctorString) { case dataViewCtorString: return dataViewTag; case mapCtorString: return mapTag; case promiseCtorString: return promiseTag; case setCtorString: return setTag; case weakMapCtorString: return weakMapTag; } } return result; }; } /** * Checks if `path` exists on `object`. * * @private * @param {Object} object The object to query. * @param {Array|string} path The path to check. * @param {Function} hasFunc The function to check properties. * @returns {boolean} Returns `true` if `path` exists, else `false`. */ function hasPath(object, path, hasFunc) { path = isKey(path, object) ? [path] : castPath(path); var result, index = -1, length = path.length; while (++index < length) { var key = toKey(path[index]); if (!(result = object != null && hasFunc(object, key))) { break; } object = object[key]; } if (result) { return result; } var length = object ? object.length : 0; return !!length && isLength(length) && isIndex(key, length) && (isArray(object) || isArguments(object)); } /** * Checks if `value` is a flattenable `arguments` object or array. * * @private * @param {*} value The value to check. * @returns {boolean} Returns `true` if `value` is flattenable, else `false`. */ function isFlattenable(value) { return isArray(value) || isArguments(value) || !!(spreadableSymbol && value && value[spreadableSymbol]); } /** * Checks if `value` is a valid array-like index. * * @private * @param {*} value The value to check. * @param {number} [length=MAX_SAFE_INTEGER] The upper bounds of a valid index. * @returns {boolean} Returns `true` if `value` is a valid index, else `false`. */ function isIndex(value, length) { length = length == null ? MAX_SAFE_INTEGER : length; return !!length && (typeof value == 'number' || reIsUint.test(value)) && (value > -1 && value % 1 == 0 && value < length); } /** * Checks if the given arguments are from an iteratee call. * * @private * @param {*} value The potential iteratee value argument. * @param {*} index The potential iteratee index or key argument. * @param {*} object The potential iteratee object argument. * @returns {boolean} Returns `true` if the arguments are from an iteratee call, * else `false`. */ function isIterateeCall(value, index, object) { if (!isObject(object)) { return false; } var type = typeof index; if (type == 'number' ? (isArrayLike(object) && isIndex(index, object.length)) : (type == 'string' && index in object) ) { return eq(object[index], value); } return false; } /** * Checks if `value` is a property name and not a property path. * * @private * @param {*} value The value to check. * @param {Object} [object] The object to query keys on. * @returns {boolean} Returns `true` if `value` is a property name, else `false`. */ function isKey(value, object) { if (isArray(value)) { return false; } var type = typeof value; if (type == 'number' || type == 'symbol' || type == 'boolean' || value == null || isSymbol(value)) { return true; } return reIsPlainProp.test(value) || !reIsDeepProp.test(value) || (object != null && value in Object(object)); } /** * Checks if `value` is suitable for use as unique object key. * * @private * @param {*} value The value to check. * @returns {boolean} Returns `true` if `value` is suitable, else `false`. */ function isKeyable(value) { var type = typeof value; return (type == 'string' || type == 'number' || type == 'symbol' || type == 'boolean') ? (value !== '__proto__') : (value === null); } /** * Checks if `func` has its source masked. * * @private * @param {Function} func The function to check. * @returns {boolean} Returns `true` if `func` is masked, else `false`. */ function isMasked(func) { return !!maskSrcKey && (maskSrcKey in func); } /** * Checks if `value` is likely a prototype object. * * @private * @param {*} value The value to check. * @returns {boolean} Returns `true` if `value` is a prototype, else `false`. */ function isPrototype(value) { var Ctor = value && value.constructor, proto = (typeof Ctor == 'function' && Ctor.prototype) || objectProto; return value === proto; } /** * Checks if `value` is suitable for strict equality comparisons, i.e. `===`. * * @private * @param {*} value The value to check. * @returns {boolean} Returns `true` if `value` if suitable for strict * equality comparisons, else `false`. */ function isStrictComparable(value) { return value === value && !isObject(value); } /** * A specialized version of `matchesProperty` for source values suitable * for strict equality comparisons, i.e. `===`. * * @private * @param {string} key The key of the property to get. * @param {*} srcValue The value to match. * @returns {Function} Returns the new spec function. */ function matchesStrictComparable(key, srcValue) { return function(object) { if (object == null) { return false; } return object[key] === srcValue && (srcValue !== undefined || (key in Object(object))); }; } /** * Converts `string` to a property path array. * * @private * @param {string} string The string to convert. * @returns {Array} Returns the property path array. */ var stringToPath = memoize(function(string) { string = toString(string); var result = []; if (reLeadingDot.test(string)) { result.push(''); } string.replace(rePropName, function(match, number, quote, string) { result.push(quote ? string.replace(reEscapeChar, '$1') : (number || match)); }); return result; }); /** * Converts `value` to a string key if it's not a string or symbol. * * @private * @param {*} value The value to inspect. * @returns {string|symbol} Returns the key. */ function toKey(value) { if (typeof value == 'string' || isSymbol(value)) { return value; } var result = (value + ''); return (result == '0' && (1 / value) == -INFINITY) ? '-0' : result; } /** * Converts `func` to its source code. * * @private * @param {Function} func The function to process. * @returns {string} Returns the source code. */ function toSource(func) { if (func != null) { try { return funcToString.call(func); } catch (e) {} try { return (func + ''); } catch (e) {} } return ''; } /** * Creates an array of elements, sorted in ascending order by the results of * running each element in a collection thru each iteratee. This method * performs a stable sort, that is, it preserves the original sort order of * equal elements. The iteratees are invoked with one argument: (value). * * @static * @memberOf _ * @since 0.1.0 * @category Collection * @param {Array|Object} collection The collection to iterate over. * @param {...(Function|Function[])} [iteratees=[_.identity]] * The iteratees to sort by. * @returns {Array} Returns the new sorted array. * @example * * var users = [ * { 'user': 'fred', 'age': 48 }, * { 'user': 'barney', 'age': 36 }, * { 'user': 'fred', 'age': 40 }, * { 'user': 'barney', 'age': 34 } * ]; * * _.sortBy(users, function(o) { return o.user; }); * // => objects for [['barney', 36], ['barney', 34], ['fred', 48], ['fred', 40]] * * _.sortBy(users, ['user', 'age']); * // => objects for [['barney', 34], ['barney', 36], ['fred', 40], ['fred', 48]] * * _.sortBy(users, 'user', function(o) { * return Math.floor(o.age / 10); * }); * // => objects for [['barney', 36], ['barney', 34], ['fred', 48], ['fred', 40]] */ var sortBy = baseRest(function(collection, iteratees) { if (collection == null) { return []; } var length = iteratees.length; if (length > 1 && isIterateeCall(collection, iteratees[0], iteratees[1])) { iteratees = []; } else if (length > 2 && isIterateeCall(iteratees[0], iteratees[1], iteratees[2])) { iteratees = [iteratees[0]]; } return baseOrderBy(collection, baseFlatten(iteratees, 1), []); }); /** * Creates a function that memoizes the result of `func`. If `resolver` is * provided, it determines the cache key for storing the result based on the * arguments provided to the memoized function. By default, the first argument * provided to the memoized function is used as the map cache key. The `func` * is invoked with the `this` binding of the memoized function. * * **Note:** The cache is exposed as the `cache` property on the memoized * function. Its creation may be customized by replacing the `_.memoize.Cache` * constructor with one whose instances implement the * [`Map`](http://ecma-international.org/ecma-262/7.0/#sec-properties-of-the-map-prototype-object) * method interface of `delete`, `get`, `has`, and `set`. * * @static * @memberOf _ * @since 0.1.0 * @category Function * @param {Function} func The function to have its output memoized. * @param {Function} [resolver] The function to resolve the cache key. * @returns {Function} Returns the new memoized function. * @example * * var object = { 'a': 1, 'b': 2 }; * var other = { 'c': 3, 'd': 4 }; * * var values = _.memoize(_.values); * values(object); * // => [1, 2] * * values(other); * // => [3, 4] * * object.a = 2; * values(object); * // => [1, 2] * * // Modify the result cache. * values.cache.set(object, ['a', 'b']); * values(object); * // => ['a', 'b'] * * // Replace `_.memoize.Cache`. * _.memoize.Cache = WeakMap; */ function memoize(func, resolver) { if (typeof func != 'function' || (resolver && typeof resolver != 'function')) { throw new TypeError(FUNC_ERROR_TEXT); } var memoized = function() { var args = arguments, key = resolver ? resolver.apply(this, args) : args[0], cache = memoized.cache; if (cache.has(key)) { return cache.get(key); } var result = func.apply(this, args); memoized.cache = cache.set(key, result); return result; }; memoized.cache = new (memoize.Cache || MapCache); return memoized; } // Assign cache to `_.memoize`. memoize.Cache = MapCache; /** * Performs a * [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero) * comparison between two values to determine if they are equivalent. * * @static * @memberOf _ * @since 4.0.0 * @category Lang * @param {*} value The value to compare. * @param {*} other The other value to compare. * @returns {boolean} Returns `true` if the values are equivalent, else `false`. * @example * * var object = { 'a': 1 }; * var other = { 'a': 1 }; * * _.eq(object, object); * // => true * * _.eq(object, other); * // => false * * _.eq('a', 'a'); * // => true * * _.eq('a', Object('a')); * // => false * * _.eq(NaN, NaN); * // => true */ function eq(value, other) { return value === other || (value !== value && other !== other); } /** * Checks if `value` is likely an `arguments` object. * * @static * @memberOf _ * @since 0.1.0 * @category Lang * @param {*} value The value to check. * @returns {boolean} Returns `true` if `value` is an `arguments` object, * else `false`. * @example * * _.isArguments(function() { return arguments; }()); * // => true * * _.isArguments([1, 2, 3]); * // => false */ function isArguments(value) { // Safari 8.1 makes `arguments.callee` enumerable in strict mode. return isArrayLikeObject(value) && hasOwnProperty.call(value, 'callee') && (!propertyIsEnumerable.call(value, 'callee') || objectToString.call(value) == argsTag); } /** * Checks if `value` is classified as an `Array` object. * * @static * @memberOf _ * @since 0.1.0 * @category Lang * @param {*} value The value to check. * @returns {boolean} Returns `true` if `value` is an array, else `false`. * @example * * _.isArray([1, 2, 3]); * // => true * * _.isArray(document.body.children); * // => false * * _.isArray('abc'); * // => false * * _.isArray(_.noop); * // => false */ var isArray = Array.isArray; /** * Checks if `value` is array-like. A value is considered array-like if it's * not a function and has a `value.length` that's an integer greater than or * equal to `0` and less than or equal to `Number.MAX_SAFE_INTEGER`. * * @static * @memberOf _ * @since 4.0.0 * @category Lang * @param {*} value The value to check. * @returns {boolean} Returns `true` if `value` is array-like, else `false`. * @example * * _.isArrayLike([1, 2, 3]); * // => true * * _.isArrayLike(document.body.children); * // => true * * _.isArrayLike('abc'); * // => true * * _.isArrayLike(_.noop); * // => false */ function isArrayLike(value) { return value != null && isLength(value.length) && !isFunction(value); } /** * This method is like `_.isArrayLike` except that it also checks if `value` * is an object. * * @static * @memberOf _ * @since 4.0.0 * @category Lang * @param {*} value The value to check. * @returns {boolean} Returns `true` if `value` is an array-like object, * else `false`. * @example * * _.isArrayLikeObject([1, 2, 3]); * // => true * * _.isArrayLikeObject(document.body.children); * // => true * * _.isArrayLikeObject('abc'); * // => false * * _.isArrayLikeObject(_.noop); * // => false */ function isArrayLikeObject(value) { return isObjectLike(value) && isArrayLike(value); } /** * Checks if `value` is classified as a `Function` object. * * @static * @memberOf _ * @since 0.1.0 * @category Lang * @param {*} value The value to check. * @returns {boolean} Returns `true` if `value` is a function, else `false`. * @example * * _.isFunction(_); * // => true * * _.isFunction(/abc/); * // => false */ function isFunction(value) { // The use of `Object#toString` avoids issues with the `typeof` operator // in Safari 8-9 which returns 'object' for typed array and other constructors. var tag = isObject(value) ? objectToString.call(value) : ''; return tag == funcTag || tag == genTag; } /** * Checks if `value` is a valid array-like length. * * **Note:** This method is loosely based on * [`ToLength`](http://ecma-international.org/ecma-262/7.0/#sec-tolength). * * @static * @memberOf _ * @since 4.0.0 * @category Lang * @param {*} value The value to check. * @returns {boolean} Returns `true` if `value` is a valid length, else `false`. * @example * * _.isLength(3); * // => true * * _.isLength(Number.MIN_VALUE); * // => false * * _.isLength(Infinity); * // => false * * _.isLength('3'); * // => false */ function isLength(value) { return typeof value == 'number' && value > -1 && value % 1 == 0 && value <= MAX_SAFE_INTEGER; } /** * Checks if `value` is the * [language type](http://www.ecma-international.org/ecma-262/7.0/#sec-ecmascript-language-types) * of `Object`. (e.g. arrays, functions, objects, regexes, `new Number(0)`, and `new String('')`) * * @static * @memberOf _ * @since 0.1.0 * @category Lang * @param {*} value The value to check. * @returns {boolean} Returns `true` if `value` is an object, else `false`. * @example * * _.isObject({}); * // => true * * _.isObject([1, 2, 3]); * // => true * * _.isObject(_.noop); * // => true * * _.isObject(null); * // => false */ function isObject(value) { var type = typeof value; return !!value && (type == 'object' || type == 'function'); } /** * Checks if `value` is object-like. A value is object-like if it's not `null` * and has a `typeof` result of "object". * * @static * @memberOf _ * @since 4.0.0 * @category Lang * @param {*} value The value to check. * @returns {boolean} Returns `true` if `value` is object-like, else `false`. * @example * * _.isObjectLike({}); * // => true * * _.isObjectLike([1, 2, 3]); * // => true * * _.isObjectLike(_.noop); * // => false * * _.isObjectLike(null); * // => false */ function isObjectLike(value) { return !!value && typeof value == 'object'; } /** * Checks if `value` is classified as a `Symbol` primitive or object. * * @static * @memberOf _ * @since 4.0.0 * @category Lang * @param {*} value The value to check. * @returns {boolean} Returns `true` if `value` is a symbol, else `false`. * @example * * _.isSymbol(Symbol.iterator); * // => true * * _.isSymbol('abc'); * // => false */ function isSymbol(value) { return typeof value == 'symbol' || (isObjectLike(value) && objectToString.call(value) == symbolTag); } /** * Checks if `value` is classified as a typed array. * * @static * @memberOf _ * @since 3.0.0 * @category Lang * @param {*} value The value to check. * @returns {boolean} Returns `true` if `value` is a typed array, else `false`. * @example * * _.isTypedArray(new Uint8Array); * // => true * * _.isTypedArray([]); * // => false */ var isTypedArray = nodeIsTypedArray ? baseUnary(nodeIsTypedArray) : baseIsTypedArray; /** * Converts `value` to a string. An empty string is returned for `null` * and `undefined` values. The sign of `-0` is preserved. * * @static * @memberOf _ * @since 4.0.0 * @category Lang * @param {*} value The value to process. * @returns {string} Returns the string. * @example * * _.toString(null); * // => '' * * _.toString(-0); * // => '-0' * * _.toString([1, 2, 3]); * // => '1,2,3' */ function toString(value) { return value == null ? '' : baseToString(value); } /** * Gets the value at `path` of `object`. If the resolved value is * `undefined`, the `defaultValue` is returned in its place. * * @static * @memberOf _ * @since 3.7.0 * @category Object * @param {Object} object The object to query. * @param {Array|string} path The path of the property to get. * @param {*} [defaultValue] The value returned for `undefined` resolved values. * @returns {*} Returns the resolved value. * @example * * var object = { 'a': [{ 'b': { 'c': 3 } }] }; * * _.get(object, 'a[0].b.c'); * // => 3 * * _.get(object, ['a', '0', 'b', 'c']); * // => 3 * * _.get(object, 'a.b.c', 'default'); * // => 'default' */ function get(object, path, defaultValue) { var result = object == null ? undefined : baseGet(object, path); return result === undefined ? defaultValue : result; } /** * Checks if `path` is a direct or inherited property of `object`. * * @static * @memberOf _ * @since 4.0.0 * @category Object * @param {Object} object The object to query. * @param {Array|string} path The path to check. * @returns {boolean} Returns `true` if `path` exists, else `false`. * @example * * var object = _.create({ 'a': _.create({ 'b': 2 }) }); * * _.hasIn(object, 'a'); * // => true * * _.hasIn(object, 'a.b'); * // => true * * _.hasIn(object, ['a', 'b']); * // => true * * _.hasIn(object, 'b'); * // => false */ function hasIn(object, path) { return object != null && hasPath(object, path, baseHasIn); } /** * Creates an array of the own enumerable property names of `object`. * * **Note:** Non-object values are coerced to objects. See the * [ES spec](http://ecma-international.org/ecma-262/7.0/#sec-object.keys) * for more details. * * @static * @since 0.1.0 * @memberOf _ * @category Object * @param {Object} object The object to query. * @returns {Array} Returns the array of property names. * @example * * function Foo() { * this.a = 1; * this.b = 2; * } * * Foo.prototype.c = 3; * * _.keys(new Foo); * // => ['a', 'b'] (iteration order is not guaranteed) * * _.keys('hi'); * // => ['0', '1'] */ function keys(object) { return isArrayLike(object) ? arrayLikeKeys(object) : baseKeys(object); } /** * This method returns the first argument it receives. * * @static * @since 0.1.0 * @memberOf _ * @category Util * @param {*} value Any value. * @returns {*} Returns `value`. * @example * * var object = { 'a': 1 }; * * console.log(_.identity(object) === object); * // => true */ function identity(value) { return value; } /** * Creates a function that returns the value at `path` of a given object. * * @static * @memberOf _ * @since 2.4.0 * @category Util * @param {Array|string} path The path of the property to get. * @returns {Function} Returns the new accessor function. * @example * * var objects = [ * { 'a': { 'b': 2 } }, * { 'a': { 'b': 1 } } * ]; * * _.map(objects, _.property('a.b')); * // => [2, 1] * * _.map(_.sortBy(objects, _.property(['a', 'b'])), 'a.b'); * // => [1, 2] */ function property(path) { return isKey(path) ? baseProperty(toKey(path)) : basePropertyDeep(path); } module.exports = sortBy; apollo-server-demo/node_modules/lodash.sortby/LICENSE 0000644 0001750 0000144 00000003637 12753654770 022260 0 ustar andreh users Copyright jQuery Foundation and other contributors <https://jquery.org/> Based on Underscore.js, copyright Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors <http://underscorejs.org/> This software consists of voluntary contributions made by many individuals. For exact contribution history, see the revision history available at https://github.com/lodash/lodash The following license applies to all parts of this software except as documented below: ==== Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. ==== Copyright and related rights for sample code are waived via CC0. Sample code is defined as all source code displayed within the prose of the documentation. CC0: http://creativecommons.org/publicdomain/zero/1.0/ ==== Files located in the node_modules and vendor directories are externally maintained libraries used by this software which have their own licenses; we recommend you read them, as their terms may differ from the terms above. apollo-server-demo/node_modules/lodash.sortby/README.md 0000644 0001750 0000144 00000000705 12753655065 022521 0 ustar andreh users # lodash.sortby v4.7.0 The [lodash](https://lodash.com/) method `_.sortBy` exported as a [Node.js](https://nodejs.org/) module. ## Installation Using npm: ```bash $ {sudo -H} npm i -g npm $ npm i --save lodash.sortby ``` In Node.js: ```js var sortBy = require('lodash.sortby'); ``` See the [documentation](https://lodash.com/docs#sortBy) or [package source](https://github.com/lodash/lodash/blob/4.7.0-npm-packages/lodash.sortby) for more details. apollo-server-demo/node_modules/lodash.sortby/package.json 0000644 0001750 0000144 00000001615 12753655065 023531 0 ustar andreh users { "name": "lodash.sortby", "version": "4.7.0", "description": "The lodash method `_.sortBy` exported as a module.", "homepage": "https://lodash.com/", "icon": "https://lodash.com/icon.svg", "license": "MIT", "keywords": "lodash-modularized, sortby", "author": "John-David Dalton <john.david.dalton@gmail.com> (http://allyoucanleet.com/)", "contributors": [ "John-David Dalton <john.david.dalton@gmail.com> (http://allyoucanleet.com/)", "Blaine Bublitz <blaine.bublitz@gmail.com> (https://github.com/phated)", "Mathias Bynens <mathias@qiwi.be> (https://mathiasbynens.be/)" ], "repository": "lodash/lodash", "scripts": { "test": "echo \"See https://travis-ci.org/lodash/lodash-cli for testing details.\"" } ,"_resolved": "https://registry.npmjs.org/lodash.sortby/-/lodash.sortby-4.7.0.tgz" ,"_integrity": "sha1-7dFMgk4sycHgsKG0K7UhBRakJDg=" ,"_from": "lodash.sortby@4.7.0" } apollo-server-demo/node_modules/deprecated-decorator/ 0000755 0001750 0000144 00000000000 14067647700 022522 5 ustar andreh users apollo-server-demo/node_modules/deprecated-decorator/README.md 0000644 0001750 0000144 00000004560 12662742175 024007 0 ustar andreh users [](https://www.npmjs.com/package/deprecated-decorator) [](https://travis-ci.org/vilic/deprecated-decorator) # Deprecated Decorator A simple decorator for deprecated properties, methods and classes. It can also wrap normal functions via the old-fashioned way. Transpilers supported: - **TypeScript** with `experimentalDecorators` option enabled. - **Babel** with [transform-decorators-legacy](https://github.com/loganfsmyth/babel-plugin-transform-decorators-legacy) for version 6.x. ## Install ```sh npm install deprecated-decorator --save ``` ## API References ```ts export declare type DeprecatedDecorator = ClassDecorator & PropertyDecorator; export interface DeprecatedOptions { alternative?: string; version?: string; url?: string; } export declare function deprecated(options?: DeprecatedOptions): DeprecatedDecorator; export declare function deprecated(alternative?: string, version?: string, url?: string): DeprecatedDecorator; export declare function deprecated<T extends Function>(fn: T): T; export declare function deprecated<T extends Function>(options: DeprecatedOptions, fn: T): T; export declare function deprecated<T extends Function>(alternative: string, fn: T): T; export declare function deprecated<T extends Function>(alternative: string, version: string, fn: T): T; export declare function deprecated<T extends Function>(alternative: string, version: string, url: string, fn: T): T; export default deprecated; ``` ## Usage Decorating a class will enable warning on constructor and static methods (including static getters and setters): ```ts import deprecated from 'deprecated-decorator'; // alternative, since version, url @deprecated('Bar', '0.1.0', 'http://vane.life/') class Foo { static method() { } } ``` Or you can decorate methods respectively: ```ts import deprecated from 'deprecated-decorator'; class Foo { @deprecated('otherMethod') method() { } @deprecated({ alternative: 'otherProperty', version: '0.1.2', url: 'http://vane.life/' }) get property() { } } ``` For functions: ```ts import deprecated from 'deprecated-decorator'; let foo = deprecated({ alternative: 'bar', version: '0.1.0' }, function foo() { // ... }); ``` ## License MIT License. apollo-server-demo/node_modules/deprecated-decorator/package.json 0000644 0001750 0000144 00000001761 12703120166 025000 0 ustar andreh users { "name": "deprecated-decorator", "version": "0.1.6", "description": "A simple decorator for deprecated methods and properties.", "main": "bld/index.js", "typings": "bld/index.d.ts", "scripts": { "build": "tsc", "test": "node node_modules/mocha/bin/_mocha" }, "repository": { "type": "git", "url": "git+https://github.com/vilic/deprecated-decorator.git" }, "keywords": ["deprecated", "decorator", "typescript", "babel", "es7"], "author": "vilicvane", "license": "MIT", "bugs": { "url": "https://github.com/vilic/deprecated-decorator/issues" }, "homepage": "https://github.com/vilic/deprecated-decorator#readme", "devDependencies": { "chai": "latest", "mocha": "latest", "sinon": "latest", "source-map-support": "latest", "typescript": "latest" } ,"_resolved": "https://registry.npmjs.org/deprecated-decorator/-/deprecated-decorator-0.1.6.tgz" ,"_integrity": "sha1-AJZjF7ehL+kvPMgx91g68ym4bDc=" ,"_from": "deprecated-decorator@0.1.6" } apollo-server-demo/node_modules/deprecated-decorator/bld/ 0000755 0001750 0000144 00000000000 14067647700 023263 5 ustar andreh users apollo-server-demo/node_modules/deprecated-decorator/bld/index.js 0000644 0001750 0000144 00000013203 12703117710 024713 0 ustar andreh users /* Deprecated Decorator v0.1 https://github.com/vilic/deprecated-decorator */ "use strict"; /** @internal */ exports.options = { getWarner: undefined }; function createWarner(type, name, alternative, version, url) { var warnedPositions = {}; return function () { var stack = (new Error()).stack || ''; var at = (stack.match(/(?:\s+at\s.+){2}\s+at\s(.+)/) || [undefined, ''])[1]; if (/\)$/.test(at)) { at = at.match(/[^(]+(?=\)$)/)[0]; } else { at = at.trim(); } if (at in warnedPositions) { return; } warnedPositions[at] = true; var message; switch (type) { case 'class': message = 'Class'; break; case 'property': message = 'Property'; break; case 'method': message = 'Method'; break; case 'function': message = 'Function'; break; } message += " `" + name + "` has been deprecated"; if (version) { message += " since version " + version; } if (alternative) { message += ", use `" + alternative + "` instead"; } message += '.'; if (at) { message += "\n at " + at; } if (url) { message += "\nCheck out " + url + " for more information."; } console.warn(message); }; } function decorateProperty(type, name, descriptor, alternative, version, url) { var warner = (exports.options.getWarner || createWarner)(type, name, alternative, version, url); descriptor = descriptor || { writable: true, enumerable: false, configurable: true }; var deprecatedDescriptor = { enumerable: descriptor.enumerable, configurable: descriptor.configurable }; if (descriptor.get || descriptor.set) { if (descriptor.get) { deprecatedDescriptor.get = function () { warner(); return descriptor.get.call(this); }; } if (descriptor.set) { deprecatedDescriptor.set = function (value) { warner(); return descriptor.set.call(this, value); }; } } else { var propertyValue_1 = descriptor.value; deprecatedDescriptor.get = function () { warner(); return propertyValue_1; }; if (descriptor.writable) { deprecatedDescriptor.set = function (value) { warner(); propertyValue_1 = value; }; } } return deprecatedDescriptor; } function decorateFunction(type, target, alternative, version, url) { var name = target.name; var warner = (exports.options.getWarner || createWarner)(type, name, alternative, version, url); var fn = function () { warner(); return target.apply(this, arguments); }; for (var _i = 0, _a = Object.getOwnPropertyNames(target); _i < _a.length; _i++) { var propertyName = _a[_i]; var descriptor = Object.getOwnPropertyDescriptor(target, propertyName); if (descriptor.writable) { fn[propertyName] = target[propertyName]; } else if (descriptor.configurable) { Object.defineProperty(fn, propertyName, descriptor); } } return fn; } function deprecated() { var args = []; for (var _i = 0; _i < arguments.length; _i++) { args[_i - 0] = arguments[_i]; } var fn = args[args.length - 1]; if (typeof fn === 'function') { fn = args.pop(); } else { fn = undefined; } var options = args[0]; var alternative; var version; var url; if (typeof options === 'string') { alternative = options; version = args[1]; url = args[2]; } else if (options) { (alternative = options.alternative, version = options.version, url = options.url, options); } if (fn) { return decorateFunction('function', fn, alternative, version, url); } return function (target, name, descriptor) { if (typeof name === 'string') { var type = descriptor && typeof descriptor.value === 'function' ? 'method' : 'property'; return decorateProperty(type, name, descriptor, alternative, version, url); } else if (typeof target === 'function') { var constructor = decorateFunction('class', target, alternative, version, url); var className = target.name; for (var _i = 0, _a = Object.getOwnPropertyNames(constructor); _i < _a.length; _i++) { var propertyName = _a[_i]; var descriptor_1 = Object.getOwnPropertyDescriptor(constructor, propertyName); descriptor_1 = decorateProperty('class', className, descriptor_1, alternative, version, url); if (descriptor_1.writable) { constructor[propertyName] = target[propertyName]; } else if (descriptor_1.configurable) { Object.defineProperty(constructor, propertyName, descriptor_1); } } return constructor; } }; } exports.deprecated = deprecated; Object.defineProperty(exports, "__esModule", { value: true }); exports.default = deprecated; //# sourceMappingURL=index.js.map apollo-server-demo/node_modules/deprecated-decorator/bld/index.d.ts 0000644 0001750 0000144 00000001571 12703117710 025154 0 ustar andreh users export declare type DeprecatedDecorator = ClassDecorator & PropertyDecorator; export interface DeprecatedOptions { alternative?: string; version?: string; url?: string; } export declare function deprecated(options?: DeprecatedOptions): DeprecatedDecorator; export declare function deprecated(alternative?: string, version?: string, url?: string): DeprecatedDecorator; export declare function deprecated<T extends Function>(fn: T): T; export declare function deprecated<T extends Function>(options: DeprecatedOptions, fn: T): T; export declare function deprecated<T extends Function>(alternative: string, fn: T): T; export declare function deprecated<T extends Function>(alternative: string, version: string, fn: T): T; export declare function deprecated<T extends Function>(alternative: string, version: string, url: string, fn: T): T; export default deprecated; apollo-server-demo/node_modules/deprecated-decorator/bld/index.js.map 0000644 0001750 0000144 00000012726 12703117710 025500 0 ustar andreh users {"version":3,"file":"index.js","sourceRoot":"c:/Projects/decorators/deprecated/src/","sources":["index.ts"],"names":[],"mappings":"AAAA;;;EAGE;;AAWF,gBAAgB;AACH,eAAO,GAAG;IACnB,SAAS,EAAE,SAAgC;CAC9C,CAAC;AAEF,sBAAsB,IAAY,EAAE,IAAY,EAAE,WAAmB,EAAE,OAAe,EAAE,GAAW;IAC/F,IAAI,eAAe,GAAY,EAAE,CAAC;IAElC,MAAM,CAAC;QACH,IAAI,KAAK,GAAW,CAAM,IAAI,KAAK,EAAE,CAAC,CAAC,KAAK,IAAI,EAAE,CAAC;QACnD,IAAI,EAAE,GAAG,CAAC,KAAK,CAAC,KAAK,CAAC,6BAA6B,CAAC,IAAI,CAAC,SAAS,EAAE,EAAE,CAAC,CAAC,CAAC,CAAC,CAAC,CAAC;QAE5E,EAAE,CAAC,CAAC,KAAK,CAAC,IAAI,CAAC,EAAE,CAAC,CAAC,CAAC,CAAC;YACjB,EAAE,GAAG,EAAE,CAAC,KAAK,CAAC,cAAc,CAAC,CAAC,CAAC,CAAC,CAAC;QACrC,CAAC;QAAC,IAAI,CAAC,CAAC;YACJ,EAAE,GAAG,EAAE,CAAC,IAAI,EAAE,CAAC;QACnB,CAAC;QAED,EAAE,CAAC,CAAC,EAAE,IAAI,eAAe,CAAC,CAAC,CAAC;YACxB,MAAM,CAAC;QACX,CAAC;QAED,eAAe,CAAC,EAAE,CAAC,GAAG,IAAI,CAAC;QAE3B,IAAI,OAAe,CAAC;QAEpB,MAAM,CAAC,CAAC,IAAI,CAAC,CAAC,CAAC;YACX,KAAK,OAAO;gBACR,OAAO,GAAG,OAAO,CAAC;gBAClB,KAAK,CAAC;YACV,KAAK,UAAU;gBACX,OAAO,GAAG,UAAU,CAAC;gBACrB,KAAK,CAAC;YACV,KAAK,QAAQ;gBACT,OAAO,GAAG,QAAQ,CAAC;gBACnB,KAAK,CAAC;YACV,KAAK,UAAU;gBACX,OAAO,GAAG,UAAU,CAAC;gBACrB,KAAK,CAAC;QACd,CAAC;QAED,OAAO,IAAI,OAAM,IAAI,0BAAwB,CAAC;QAE9C,EAAE,CAAC,CAAC,OAAO,CAAC,CAAC,CAAC;YACV,OAAO,IAAI,oBAAkB,OAAS,CAAC;QAC3C,CAAC;QAED,EAAE,CAAC,CAAC,WAAW,CAAC,CAAC,CAAC;YACd,OAAO,IAAI,YAAW,WAAW,cAAY,CAAC;QAClD,CAAC;QAED,OAAO,IAAI,GAAG,CAAC;QAEf,EAAE,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC;YACL,OAAO,IAAI,cAAY,EAAI,CAAC;QAChC,CAAC;QAED,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC;YACN,OAAO,IAAI,iBAAe,GAAG,2BAAwB,CAAC;QAC1D,CAAC;QAED,OAAO,CAAC,IAAI,CAAC,OAAO,CAAC,CAAC;IAC1B,CAAC,CAAC;AACN,CAAC;AAED,0BACI,IAAY,EACZ,IAAY,EACZ,UAA8B,EAC9B,WAAmB,EACnB,OAAe,EACf,GAAW;IAEX,IAAI,MAAM,GAAG,CAAC,eAAO,CAAC,SAAS,IAAI,YAAY,CAAC,CAAC,IAAI,EAAE,IAAI,EAAE,WAAW,EAAE,OAAO,EAAE,GAAG,CAAC,CAAC;IAExF,UAAU,GAAG,UAAU,IAAI;QACvB,QAAQ,EAAE,IAAI;QACd,UAAU,EAAE,KAAK;QACjB,YAAY,EAAE,IAAI;KACrB,CAAC;IAEF,IAAI,oBAAoB,GAAuB;QAC3C,UAAU,EAAE,UAAU,CAAC,UAAU;QACjC,YAAY,EAAE,UAAU,CAAC,YAAY;KACxC,CAAC;IAEF,EAAE,CAAC,CAAC,UAAU,CAAC,GAAG,IAAI,UAAU,CAAC,GAAG,CAAC,CAAC,CAAC;QACnC,EAAE,CAAC,CAAC,UAAU,CAAC,GAAG,CAAC,CAAC,CAAC;YACjB,oBAAoB,CAAC,GAAG,GAAG;gBACvB,MAAM,EAAE,CAAC;gBACT,MAAM,CAAC,UAAU,CAAC,GAAG,CAAC,IAAI,CAAC,IAAI,CAAC,CAAC;YACrC,CAAC,CAAC;QACN,CAAC;QAED,EAAE,CAAC,CAAC,UAAU,CAAC,GAAG,CAAC,CAAC,CAAC;YACjB,oBAAoB,CAAC,GAAG,GAAG,UAAU,KAAK;gBACtC,MAAM,EAAE,CAAC;gBACT,MAAM,CAAC,UAAU,CAAC,GAAG,CAAC,IAAI,CAAC,IAAI,EAAE,KAAK,CAAC,CAAC;YAC5C,CAAC,CAAC;QACN,CAAC;IACL,CAAC;IAAC,IAAI,CAAC,CAAC;QACJ,IAAI,eAAa,GAAG,UAAU,CAAC,KAAK,CAAC;QAErC,oBAAoB,CAAC,GAAG,GAAG;YACvB,MAAM,EAAE,CAAC;YACT,MAAM,CAAC,eAAa,CAAC;QACzB,CAAC,CAAC;QAEF,EAAE,CAAC,CAAC,UAAU,CAAC,QAAQ,CAAC,CAAC,CAAC;YACtB,oBAAoB,CAAC,GAAG,GAAG,UAAU,KAAK;gBACtC,MAAM,EAAE,CAAC;gBACT,eAAa,GAAG,KAAK,CAAC;YAC1B,CAAC,CAAC;QACN,CAAC;IACL,CAAC;IAED,MAAM,CAAC,oBAAoB,CAAC;AAChC,CAAC;AAED,0BACI,IAAY,EACZ,MAAS,EACT,WAAmB,EACnB,OAAe,EACf,GAAW;IAEX,IAAI,IAAI,GAAiB,MAAO,CAAC,IAAI,CAAC;IACtC,IAAI,MAAM,GAAG,CAAC,eAAO,CAAC,SAAS,IAAI,YAAY,CAAC,CAAC,IAAI,EAAE,IAAI,EAAE,WAAW,EAAE,OAAO,EAAE,GAAG,CAAC,CAAC;IAExF,IAAI,EAAE,GAAW;QACb,MAAM,EAAE,CAAC;QACT,MAAM,CAAC,MAAM,CAAC,KAAK,CAAC,IAAI,EAAE,SAAS,CAAC,CAAC;IACzC,CAAC,CAAC;IAEF,GAAG,CAAC,CAAqB,UAAkC,EAAlC,KAAA,MAAM,CAAC,mBAAmB,CAAC,MAAM,CAAC,EAAlC,cAAkC,EAAlC,IAAkC,CAAC;QAAvD,IAAI,YAAY,SAAA;QACjB,IAAI,UAAU,GAAG,MAAM,CAAC,wBAAwB,CAAC,MAAM,EAAE,YAAY,CAAC,CAAC;QAEvE,EAAE,CAAC,CAAC,UAAU,CAAC,QAAQ,CAAC,CAAC,CAAC;YAChB,EAAG,CAAC,YAAY,CAAC,GAAS,MAAO,CAAC,YAAY,CAAC,CAAC;QAC1D,CAAC;QAAC,IAAI,CAAC,EAAE,CAAC,CAAC,UAAU,CAAC,YAAY,CAAC,CAAC,CAAC;YACjC,MAAM,CAAC,cAAc,CAAC,EAAE,EAAE,YAAY,EAAE,UAAU,CAAC,CAAC;QACxD,CAAC;KACJ;IAED,MAAM,CAAC,EAAE,CAAC;AACd,CAAC;AAiBD;IAA2B,cAAc;SAAd,WAAc,CAAd,sBAAc,CAAd,IAAc;QAAd,6BAAc;;IACrC,IAAI,EAAE,GAAG,IAAI,CAAC,IAAI,CAAC,MAAM,GAAG,CAAC,CAAC,CAAC;IAE/B,EAAE,CAAC,CAAC,OAAO,EAAE,KAAK,UAAU,CAAC,CAAC,CAAC;QAC3B,EAAE,GAAG,IAAI,CAAC,GAAG,EAAE,CAAC;IACpB,CAAC;IAAC,IAAI,CAAC,CAAC;QACJ,EAAE,GAAG,SAAS,CAAC;IACnB,CAAC;IAED,IAAI,OAAO,GAAG,IAAI,CAAC,CAAC,CAAC,CAAC;IAEtB,IAAI,WAAmB,CAAC;IACxB,IAAI,OAAe,CAAC;IACpB,IAAI,GAAW,CAAC;IAEhB,EAAE,CAAC,CAAC,OAAO,OAAO,KAAK,QAAQ,CAAC,CAAC,CAAC;QAC9B,WAAW,GAAG,OAAO,CAAC;QACtB,OAAO,GAAG,IAAI,CAAC,CAAC,CAAC,CAAC;QAClB,GAAG,GAAG,IAAI,CAAC,CAAC,CAAC,CAAC;IAClB,CAAC;IAAC,IAAI,CAAC,EAAE,CAAC,CAAC,OAAO,CAAC,CAAC,CAAC;QACjB,CACI,iCAAW,EACX,yBAAO,EACP,iBAAG,EACH,OAAO,CAAC,CAAC;IACjB,CAAC;IAED,EAAE,CAAC,CAAC,EAAE,CAAC,CAAC,CAAC;QACL,MAAM,CAAC,gBAAgB,CAAC,UAAU,EAAE,EAAE,EAAE,WAAW,EAAE,OAAO,EAAE,GAAG,CAAC,CAAC;IACvE,CAAC;IAED,MAAM,CAAC,UAAC,MAAyB,EAAE,IAAa,EAAE,UAA+B;QAC7E,EAAE,CAAC,CAAC,OAAO,IAAI,KAAK,QAAQ,CAAC,CAAC,CAAC;YAC3B,IAAI,IAAI,GAAG,UAAU,IAAI,OAAO,UAAU,CAAC,KAAK,KAAK,UAAU;gBAC3D,QAAQ,GAAG,UAAU,CAAC;YAE1B,MAAM,CAAC,gBAAgB,CAAC,IAAI,EAAE,IAAI,EAAE,UAAU,EAAE,WAAW,EAAE,OAAO,EAAE,GAAG,CAAC,CAAC;QAC/E,CAAC;QAAC,IAAI,CAAC,EAAE,CAAC,CAAC,OAAO,MAAM,KAAK,UAAU,CAAC,CAAC,CAAC;YACtC,IAAI,WAAW,GAAG,gBAAgB,CAAC,OAAO,EAAE,MAAkB,EAAE,WAAW,EAAE,OAAO,EAAE,GAAG,CAAC,CAAC;YAC3F,IAAI,SAAS,GAAiB,MAAO,CAAC,IAAI,CAAC;YAE3C,GAAG,CAAC,CAAqB,UAAuC,EAAvC,KAAA,MAAM,CAAC,mBAAmB,CAAC,WAAW,CAAC,EAAvC,cAAuC,EAAvC,IAAuC,CAAC;gBAA5D,IAAI,YAAY,SAAA;gBACjB,IAAI,YAAU,GAAG,MAAM,CAAC,wBAAwB,CAAC,WAAW,EAAE,YAAY,CAAC,CAAC;gBAC5E,YAAU,GAAG,gBAAgB,CAAC,OAAO,EAAE,SAAS,EAAE,YAAU,EAAE,WAAW,EAAE,OAAO,EAAE,GAAG,CAAC,CAAC;gBAEzF,EAAE,CAAC,CAAC,YAAU,CAAC,QAAQ,CAAC,CAAC,CAAC;oBAChB,WAAY,CAAC,YAAY,CAAC,GAAS,MAAO,CAAC,YAAY,CAAC,CAAC;gBACnE,CAAC;gBAAC,IAAI,CAAC,EAAE,CAAC,CAAC,YAAU,CAAC,YAAY,CAAC,CAAC,CAAC;oBACjC,MAAM,CAAC,cAAc,CAAC,WAAW,EAAE,YAAY,EAAE,YAAU,CAAC,CAAC;gBACjE,CAAC;aACJ;YAED,MAAM,CAAC,WAAW,CAAC;QACvB,CAAC;IACL,CAAC,CAAC;AACN,CAAC;AAvDe,kBAAU,aAuDzB,CAAA;AAED;kBAAe,UAAU,CAAC"} apollo-server-demo/node_modules/core-js/ 0000755 0001750 0000144 00000000000 14067647701 020005 5 ustar andreh users apollo-server-demo/node_modules/core-js/modules/ 0000755 0001750 0000144 00000000000 14067647701 021455 5 ustar andreh users apollo-server-demo/node_modules/core-js/modules/es.reflect.define-property.js 0000644 0001750 0000144 00000002064 03560116604 027147 0 ustar andreh users var $ = require('../internals/export'); var DESCRIPTORS = require('../internals/descriptors'); var anObject = require('../internals/an-object'); var toPrimitive = require('../internals/to-primitive'); var definePropertyModule = require('../internals/object-define-property'); var fails = require('../internals/fails'); // MS Edge has broken Reflect.defineProperty - throwing instead of returning false var ERROR_INSTEAD_OF_FALSE = fails(function () { // eslint-disable-next-line no-undef Reflect.defineProperty(definePropertyModule.f({}, 1, { value: 1 }), 1, { value: 2 }); }); // `Reflect.defineProperty` method // https://tc39.es/ecma262/#sec-reflect.defineproperty $({ target: 'Reflect', stat: true, forced: ERROR_INSTEAD_OF_FALSE, sham: !DESCRIPTORS }, { defineProperty: function defineProperty(target, propertyKey, attributes) { anObject(target); var key = toPrimitive(propertyKey, true); anObject(attributes); try { definePropertyModule.f(target, key, attributes); return true; } catch (error) { return false; } } }); apollo-server-demo/node_modules/core-js/modules/es.array.splice.js 0000644 0001750 0000144 00000005416 03560116604 025010 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var toAbsoluteIndex = require('../internals/to-absolute-index'); var toInteger = require('../internals/to-integer'); var toLength = require('../internals/to-length'); var toObject = require('../internals/to-object'); var arraySpeciesCreate = require('../internals/array-species-create'); var createProperty = require('../internals/create-property'); var arrayMethodHasSpeciesSupport = require('../internals/array-method-has-species-support'); var arrayMethodUsesToLength = require('../internals/array-method-uses-to-length'); var HAS_SPECIES_SUPPORT = arrayMethodHasSpeciesSupport('splice'); var USES_TO_LENGTH = arrayMethodUsesToLength('splice', { ACCESSORS: true, 0: 0, 1: 2 }); var max = Math.max; var min = Math.min; var MAX_SAFE_INTEGER = 0x1FFFFFFFFFFFFF; var MAXIMUM_ALLOWED_LENGTH_EXCEEDED = 'Maximum allowed length exceeded'; // `Array.prototype.splice` method // https://tc39.es/ecma262/#sec-array.prototype.splice // with adding support of @@species $({ target: 'Array', proto: true, forced: !HAS_SPECIES_SUPPORT || !USES_TO_LENGTH }, { splice: function splice(start, deleteCount /* , ...items */) { var O = toObject(this); var len = toLength(O.length); var actualStart = toAbsoluteIndex(start, len); var argumentsLength = arguments.length; var insertCount, actualDeleteCount, A, k, from, to; if (argumentsLength === 0) { insertCount = actualDeleteCount = 0; } else if (argumentsLength === 1) { insertCount = 0; actualDeleteCount = len - actualStart; } else { insertCount = argumentsLength - 2; actualDeleteCount = min(max(toInteger(deleteCount), 0), len - actualStart); } if (len + insertCount - actualDeleteCount > MAX_SAFE_INTEGER) { throw TypeError(MAXIMUM_ALLOWED_LENGTH_EXCEEDED); } A = arraySpeciesCreate(O, actualDeleteCount); for (k = 0; k < actualDeleteCount; k++) { from = actualStart + k; if (from in O) createProperty(A, k, O[from]); } A.length = actualDeleteCount; if (insertCount < actualDeleteCount) { for (k = actualStart; k < len - actualDeleteCount; k++) { from = k + actualDeleteCount; to = k + insertCount; if (from in O) O[to] = O[from]; else delete O[to]; } for (k = len; k > len - actualDeleteCount + insertCount; k--) delete O[k - 1]; } else if (insertCount > actualDeleteCount) { for (k = len - actualDeleteCount; k > actualStart; k--) { from = k + actualDeleteCount - 1; to = k + insertCount - 1; if (from in O) O[to] = O[from]; else delete O[to]; } } for (k = 0; k < insertCount; k++) { O[k + actualStart] = arguments[k + 2]; } O.length = len - actualDeleteCount + insertCount; return A; } }); apollo-server-demo/node_modules/core-js/modules/es.typed-array.uint16-array.js 0000644 0001750 0000144 00000000520 03560116604 027105 0 ustar andreh users var createTypedArrayConstructor = require('../internals/typed-array-constructor'); // `Uint16Array` constructor // https://tc39.es/ecma262/#sec-typedarray-objects createTypedArrayConstructor('Uint16', function (init) { return function Uint16Array(data, byteOffset, length) { return init(this, data, byteOffset, length); }; }); apollo-server-demo/node_modules/core-js/modules/es.date.to-json.js 0000644 0001750 0000144 00000001330 03560116604 024710 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var fails = require('../internals/fails'); var toObject = require('../internals/to-object'); var toPrimitive = require('../internals/to-primitive'); var FORCED = fails(function () { return new Date(NaN).toJSON() !== null || Date.prototype.toJSON.call({ toISOString: function () { return 1; } }) !== 1; }); // `Date.prototype.toJSON` method // https://tc39.es/ecma262/#sec-date.prototype.tojson $({ target: 'Date', proto: true, forced: FORCED }, { // eslint-disable-next-line no-unused-vars toJSON: function toJSON(key) { var O = toObject(this); var pv = toPrimitive(O); return typeof pv == 'number' && !isFinite(pv) ? null : O.toISOString(); } }); apollo-server-demo/node_modules/core-js/modules/esnext.symbol.pattern-match.js 0000644 0001750 0000144 00000000326 03560116604 027361 0 ustar andreh users var defineWellKnownSymbol = require('../internals/define-well-known-symbol'); // `Symbol.patternMatch` well-known symbol // https://github.com/tc39/proposal-pattern-matching defineWellKnownSymbol('patternMatch'); apollo-server-demo/node_modules/core-js/modules/es.number.is-integer.js 0000644 0001750 0000144 00000000354 03560116604 025745 0 ustar andreh users var $ = require('../internals/export'); var isInteger = require('../internals/is-integer'); // `Number.isInteger` method // https://tc39.es/ecma262/#sec-number.isinteger $({ target: 'Number', stat: true }, { isInteger: isInteger }); apollo-server-demo/node_modules/core-js/modules/es.reflect.is-extensible.js 0000644 0001750 0000144 00000000630 03560116604 026603 0 ustar andreh users var $ = require('../internals/export'); var anObject = require('../internals/an-object'); var objectIsExtensible = Object.isExtensible; // `Reflect.isExtensible` method // https://tc39.es/ecma262/#sec-reflect.isextensible $({ target: 'Reflect', stat: true }, { isExtensible: function isExtensible(target) { anObject(target); return objectIsExtensible ? objectIsExtensible(target) : true; } }); apollo-server-demo/node_modules/core-js/modules/es.object.lookup-getter.js 0000644 0001750 0000144 00000001616 03560116604 026460 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var DESCRIPTORS = require('../internals/descriptors'); var FORCED = require('../internals/object-prototype-accessors-forced'); var toObject = require('../internals/to-object'); var toPrimitive = require('../internals/to-primitive'); var getPrototypeOf = require('../internals/object-get-prototype-of'); var getOwnPropertyDescriptor = require('../internals/object-get-own-property-descriptor').f; // `Object.prototype.__lookupGetter__` method // https://tc39.es/ecma262/#sec-object.prototype.__lookupGetter__ if (DESCRIPTORS) { $({ target: 'Object', proto: true, forced: FORCED }, { __lookupGetter__: function __lookupGetter__(P) { var O = toObject(this); var key = toPrimitive(P, true); var desc; do { if (desc = getOwnPropertyDescriptor(O, key)) return desc.get; } while (O = getPrototypeOf(O)); } }); } apollo-server-demo/node_modules/core-js/modules/es.math.imul.js 0000644 0001750 0000144 00000001207 03560116604 024304 0 ustar andreh users var $ = require('../internals/export'); var fails = require('../internals/fails'); var nativeImul = Math.imul; var FORCED = fails(function () { return nativeImul(0xFFFFFFFF, 5) != -5 || nativeImul.length != 2; }); // `Math.imul` method // https://tc39.es/ecma262/#sec-math.imul // some WebKit versions fails with big numbers, some has wrong arity $({ target: 'Math', stat: true, forced: FORCED }, { imul: function imul(x, y) { var UINT16 = 0xFFFF; var xn = +x; var yn = +y; var xl = UINT16 & xn; var yl = UINT16 & yn; return 0 | xl * yl + ((UINT16 & xn >>> 16) * yl + xl * (UINT16 & yn >>> 16) << 16 >>> 0); } }); apollo-server-demo/node_modules/core-js/modules/es.array.reduce.js 0000644 0001750 0000144 00000002015 03560116604 024770 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var $reduce = require('../internals/array-reduce').left; var arrayMethodIsStrict = require('../internals/array-method-is-strict'); var arrayMethodUsesToLength = require('../internals/array-method-uses-to-length'); var CHROME_VERSION = require('../internals/engine-v8-version'); var IS_NODE = require('../internals/engine-is-node'); var STRICT_METHOD = arrayMethodIsStrict('reduce'); var USES_TO_LENGTH = arrayMethodUsesToLength('reduce', { 1: 0 }); // Chrome 80-82 has a critical bug // https://bugs.chromium.org/p/chromium/issues/detail?id=1049982 var CHROME_BUG = !IS_NODE && CHROME_VERSION > 79 && CHROME_VERSION < 83; // `Array.prototype.reduce` method // https://tc39.es/ecma262/#sec-array.prototype.reduce $({ target: 'Array', proto: true, forced: !STRICT_METHOD || !USES_TO_LENGTH || CHROME_BUG }, { reduce: function reduce(callbackfn /* , initialValue */) { return $reduce(this, callbackfn, arguments.length, arguments.length > 1 ? arguments[1] : undefined); } }); apollo-server-demo/node_modules/core-js/modules/esnext.array.unique-by.js 0000644 0001750 0000144 00000002360 03560116604 026341 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var toLength = require('../internals/to-length'); var toObject = require('../internals/to-object'); var getBuiltIn = require('../internals/get-built-in'); var arraySpeciesCreate = require('../internals/array-species-create'); var addToUnscopables = require('../internals/add-to-unscopables'); var push = [].push; // `Array.prototype.uniqueBy` method // https://github.com/tc39/proposal-array-unique $({ target: 'Array', proto: true }, { uniqueBy: function uniqueBy(resolver) { var that = toObject(this); var length = toLength(that.length); var result = arraySpeciesCreate(that, 0); var Map = getBuiltIn('Map'); var map = new Map(); var resolverFunction, index, item, key; if (typeof resolver == 'function') resolverFunction = resolver; else if (resolver == null) resolverFunction = function (value) { return value; }; else throw new TypeError('Incorrect resolver!'); for (index = 0; index < length; index++) { item = that[index]; key = resolverFunction(item); if (!map.has(key)) map.set(key, item); } map.forEach(function (value) { push.call(result, value); }); return result; } }); addToUnscopables('uniqueBy'); apollo-server-demo/node_modules/core-js/modules/es.symbol.async-iterator.js 0000644 0001750 0000144 00000000330 03560116604 026652 0 ustar andreh users var defineWellKnownSymbol = require('../internals/define-well-known-symbol'); // `Symbol.asyncIterator` well-known symbol // https://tc39.es/ecma262/#sec-symbol.asynciterator defineWellKnownSymbol('asyncIterator'); apollo-server-demo/node_modules/core-js/modules/es.array.last-index-of.js 0000644 0001750 0000144 00000000471 03560116604 026177 0 ustar andreh users var $ = require('../internals/export'); var lastIndexOf = require('../internals/array-last-index-of'); // `Array.prototype.lastIndexOf` method // https://tc39.es/ecma262/#sec-array.prototype.lastindexof $({ target: 'Array', proto: true, forced: lastIndexOf !== [].lastIndexOf }, { lastIndexOf: lastIndexOf }); apollo-server-demo/node_modules/core-js/modules/esnext.math.imulh.js 0000644 0001750 0000144 00000000777 03560116604 025366 0 ustar andreh users var $ = require('../internals/export'); // `Math.imulh` method // https://gist.github.com/BrendanEich/4294d5c212a6d2254703 // TODO: Remove from `core-js@4` $({ target: 'Math', stat: true }, { imulh: function imulh(u, v) { var UINT16 = 0xFFFF; var $u = +u; var $v = +v; var u0 = $u & UINT16; var v0 = $v & UINT16; var u1 = $u >> 16; var v1 = $v >> 16; var t = (u1 * v0 >>> 0) + (u0 * v0 >>> 16); return u1 * v1 + (t >> 16) + ((u0 * v1 >>> 0) + (t & UINT16) >> 16); } }); apollo-server-demo/node_modules/core-js/modules/es.object.seal.js 0000644 0001750 0000144 00000001135 03560116604 024577 0 ustar andreh users var $ = require('../internals/export'); var isObject = require('../internals/is-object'); var onFreeze = require('../internals/internal-metadata').onFreeze; var FREEZING = require('../internals/freezing'); var fails = require('../internals/fails'); var nativeSeal = Object.seal; var FAILS_ON_PRIMITIVES = fails(function () { nativeSeal(1); }); // `Object.seal` method // https://tc39.es/ecma262/#sec-object.seal $({ target: 'Object', stat: true, forced: FAILS_ON_PRIMITIVES, sham: !FREEZING }, { seal: function seal(it) { return nativeSeal && isObject(it) ? nativeSeal(onFreeze(it)) : it; } }); apollo-server-demo/node_modules/core-js/modules/es.object.keys.js 0000644 0001750 0000144 00000000716 03560116604 024632 0 ustar andreh users var $ = require('../internals/export'); var toObject = require('../internals/to-object'); var nativeKeys = require('../internals/object-keys'); var fails = require('../internals/fails'); var FAILS_ON_PRIMITIVES = fails(function () { nativeKeys(1); }); // `Object.keys` method // https://tc39.es/ecma262/#sec-object.keys $({ target: 'Object', stat: true, forced: FAILS_ON_PRIMITIVES }, { keys: function keys(it) { return nativeKeys(toObject(it)); } }); apollo-server-demo/node_modules/core-js/modules/es.math.fround.js 0000644 0001750 0000144 00000000326 03560116604 024634 0 ustar andreh users var $ = require('../internals/export'); var fround = require('../internals/math-fround'); // `Math.fround` method // https://tc39.es/ecma262/#sec-math.fround $({ target: 'Math', stat: true }, { fround: fround }); apollo-server-demo/node_modules/core-js/modules/esnext.iterator.filter.js 0000644 0001750 0000144 00000001742 03560116604 026426 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var aFunction = require('../internals/a-function'); var anObject = require('../internals/an-object'); var createIteratorProxy = require('../internals/iterator-create-proxy'); var callWithSafeIterationClosing = require('../internals/call-with-safe-iteration-closing'); var IteratorProxy = createIteratorProxy(function (arg) { var iterator = this.iterator; var filterer = this.filterer; var next = this.next; var result, done, value; while (true) { result = anObject(next.call(iterator, arg)); done = this.done = !!result.done; if (done) return; value = result.value; if (callWithSafeIterationClosing(iterator, filterer, value)) return value; } }); $({ target: 'Iterator', proto: true, real: true }, { filter: function filter(filterer) { return new IteratorProxy({ iterator: anObject(this), filterer: aFunction(filterer) }); } }); apollo-server-demo/node_modules/core-js/modules/es.promise.js 0000644 0001750 0000144 00000032260 03560116604 024067 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var global = require('../internals/global'); var getBuiltIn = require('../internals/get-built-in'); var NativePromise = require('../internals/native-promise-constructor'); var redefine = require('../internals/redefine'); var redefineAll = require('../internals/redefine-all'); var setToStringTag = require('../internals/set-to-string-tag'); var setSpecies = require('../internals/set-species'); var isObject = require('../internals/is-object'); var aFunction = require('../internals/a-function'); var anInstance = require('../internals/an-instance'); var inspectSource = require('../internals/inspect-source'); var iterate = require('../internals/iterate'); var checkCorrectnessOfIteration = require('../internals/check-correctness-of-iteration'); var speciesConstructor = require('../internals/species-constructor'); var task = require('../internals/task').set; var microtask = require('../internals/microtask'); var promiseResolve = require('../internals/promise-resolve'); var hostReportErrors = require('../internals/host-report-errors'); var newPromiseCapabilityModule = require('../internals/new-promise-capability'); var perform = require('../internals/perform'); var InternalStateModule = require('../internals/internal-state'); var isForced = require('../internals/is-forced'); var wellKnownSymbol = require('../internals/well-known-symbol'); var IS_NODE = require('../internals/engine-is-node'); var V8_VERSION = require('../internals/engine-v8-version'); var SPECIES = wellKnownSymbol('species'); var PROMISE = 'Promise'; var getInternalState = InternalStateModule.get; var setInternalState = InternalStateModule.set; var getInternalPromiseState = InternalStateModule.getterFor(PROMISE); var PromiseConstructor = NativePromise; var TypeError = global.TypeError; var document = global.document; var process = global.process; var $fetch = getBuiltIn('fetch'); var newPromiseCapability = newPromiseCapabilityModule.f; var newGenericPromiseCapability = newPromiseCapability; var DISPATCH_EVENT = !!(document && document.createEvent && global.dispatchEvent); var NATIVE_REJECTION_EVENT = typeof PromiseRejectionEvent == 'function'; var UNHANDLED_REJECTION = 'unhandledrejection'; var REJECTION_HANDLED = 'rejectionhandled'; var PENDING = 0; var FULFILLED = 1; var REJECTED = 2; var HANDLED = 1; var UNHANDLED = 2; var Internal, OwnPromiseCapability, PromiseWrapper, nativeThen; var FORCED = isForced(PROMISE, function () { var GLOBAL_CORE_JS_PROMISE = inspectSource(PromiseConstructor) !== String(PromiseConstructor); if (!GLOBAL_CORE_JS_PROMISE) { // V8 6.6 (Node 10 and Chrome 66) have a bug with resolving custom thenables // https://bugs.chromium.org/p/chromium/issues/detail?id=830565 // We can't detect it synchronously, so just check versions if (V8_VERSION === 66) return true; // Unhandled rejections tracking support, NodeJS Promise without it fails @@species test if (!IS_NODE && !NATIVE_REJECTION_EVENT) return true; } // We need Promise#finally in the pure version for preventing prototype pollution if (IS_PURE && !PromiseConstructor.prototype['finally']) return true; // We can't use @@species feature detection in V8 since it causes // deoptimization and performance degradation // https://github.com/zloirock/core-js/issues/679 if (V8_VERSION >= 51 && /native code/.test(PromiseConstructor)) return false; // Detect correctness of subclassing with @@species support var promise = PromiseConstructor.resolve(1); var FakePromise = function (exec) { exec(function () { /* empty */ }, function () { /* empty */ }); }; var constructor = promise.constructor = {}; constructor[SPECIES] = FakePromise; return !(promise.then(function () { /* empty */ }) instanceof FakePromise); }); var INCORRECT_ITERATION = FORCED || !checkCorrectnessOfIteration(function (iterable) { PromiseConstructor.all(iterable)['catch'](function () { /* empty */ }); }); // helpers var isThenable = function (it) { var then; return isObject(it) && typeof (then = it.then) == 'function' ? then : false; }; var notify = function (state, isReject) { if (state.notified) return; state.notified = true; var chain = state.reactions; microtask(function () { var value = state.value; var ok = state.state == FULFILLED; var index = 0; // variable length - can't use forEach while (chain.length > index) { var reaction = chain[index++]; var handler = ok ? reaction.ok : reaction.fail; var resolve = reaction.resolve; var reject = reaction.reject; var domain = reaction.domain; var result, then, exited; try { if (handler) { if (!ok) { if (state.rejection === UNHANDLED) onHandleUnhandled(state); state.rejection = HANDLED; } if (handler === true) result = value; else { if (domain) domain.enter(); result = handler(value); // can throw if (domain) { domain.exit(); exited = true; } } if (result === reaction.promise) { reject(TypeError('Promise-chain cycle')); } else if (then = isThenable(result)) { then.call(result, resolve, reject); } else resolve(result); } else reject(value); } catch (error) { if (domain && !exited) domain.exit(); reject(error); } } state.reactions = []; state.notified = false; if (isReject && !state.rejection) onUnhandled(state); }); }; var dispatchEvent = function (name, promise, reason) { var event, handler; if (DISPATCH_EVENT) { event = document.createEvent('Event'); event.promise = promise; event.reason = reason; event.initEvent(name, false, true); global.dispatchEvent(event); } else event = { promise: promise, reason: reason }; if (!NATIVE_REJECTION_EVENT && (handler = global['on' + name])) handler(event); else if (name === UNHANDLED_REJECTION) hostReportErrors('Unhandled promise rejection', reason); }; var onUnhandled = function (state) { task.call(global, function () { var promise = state.facade; var value = state.value; var IS_UNHANDLED = isUnhandled(state); var result; if (IS_UNHANDLED) { result = perform(function () { if (IS_NODE) { process.emit('unhandledRejection', value, promise); } else dispatchEvent(UNHANDLED_REJECTION, promise, value); }); // Browsers should not trigger `rejectionHandled` event if it was handled here, NodeJS - should state.rejection = IS_NODE || isUnhandled(state) ? UNHANDLED : HANDLED; if (result.error) throw result.value; } }); }; var isUnhandled = function (state) { return state.rejection !== HANDLED && !state.parent; }; var onHandleUnhandled = function (state) { task.call(global, function () { var promise = state.facade; if (IS_NODE) { process.emit('rejectionHandled', promise); } else dispatchEvent(REJECTION_HANDLED, promise, state.value); }); }; var bind = function (fn, state, unwrap) { return function (value) { fn(state, value, unwrap); }; }; var internalReject = function (state, value, unwrap) { if (state.done) return; state.done = true; if (unwrap) state = unwrap; state.value = value; state.state = REJECTED; notify(state, true); }; var internalResolve = function (state, value, unwrap) { if (state.done) return; state.done = true; if (unwrap) state = unwrap; try { if (state.facade === value) throw TypeError("Promise can't be resolved itself"); var then = isThenable(value); if (then) { microtask(function () { var wrapper = { done: false }; try { then.call(value, bind(internalResolve, wrapper, state), bind(internalReject, wrapper, state) ); } catch (error) { internalReject(wrapper, error, state); } }); } else { state.value = value; state.state = FULFILLED; notify(state, false); } } catch (error) { internalReject({ done: false }, error, state); } }; // constructor polyfill if (FORCED) { // 25.4.3.1 Promise(executor) PromiseConstructor = function Promise(executor) { anInstance(this, PromiseConstructor, PROMISE); aFunction(executor); Internal.call(this); var state = getInternalState(this); try { executor(bind(internalResolve, state), bind(internalReject, state)); } catch (error) { internalReject(state, error); } }; // eslint-disable-next-line no-unused-vars Internal = function Promise(executor) { setInternalState(this, { type: PROMISE, done: false, notified: false, parent: false, reactions: [], rejection: false, state: PENDING, value: undefined }); }; Internal.prototype = redefineAll(PromiseConstructor.prototype, { // `Promise.prototype.then` method // https://tc39.es/ecma262/#sec-promise.prototype.then then: function then(onFulfilled, onRejected) { var state = getInternalPromiseState(this); var reaction = newPromiseCapability(speciesConstructor(this, PromiseConstructor)); reaction.ok = typeof onFulfilled == 'function' ? onFulfilled : true; reaction.fail = typeof onRejected == 'function' && onRejected; reaction.domain = IS_NODE ? process.domain : undefined; state.parent = true; state.reactions.push(reaction); if (state.state != PENDING) notify(state, false); return reaction.promise; }, // `Promise.prototype.catch` method // https://tc39.es/ecma262/#sec-promise.prototype.catch 'catch': function (onRejected) { return this.then(undefined, onRejected); } }); OwnPromiseCapability = function () { var promise = new Internal(); var state = getInternalState(promise); this.promise = promise; this.resolve = bind(internalResolve, state); this.reject = bind(internalReject, state); }; newPromiseCapabilityModule.f = newPromiseCapability = function (C) { return C === PromiseConstructor || C === PromiseWrapper ? new OwnPromiseCapability(C) : newGenericPromiseCapability(C); }; if (!IS_PURE && typeof NativePromise == 'function') { nativeThen = NativePromise.prototype.then; // wrap native Promise#then for native async functions redefine(NativePromise.prototype, 'then', function then(onFulfilled, onRejected) { var that = this; return new PromiseConstructor(function (resolve, reject) { nativeThen.call(that, resolve, reject); }).then(onFulfilled, onRejected); // https://github.com/zloirock/core-js/issues/640 }, { unsafe: true }); // wrap fetch result if (typeof $fetch == 'function') $({ global: true, enumerable: true, forced: true }, { // eslint-disable-next-line no-unused-vars fetch: function fetch(input /* , init */) { return promiseResolve(PromiseConstructor, $fetch.apply(global, arguments)); } }); } } $({ global: true, wrap: true, forced: FORCED }, { Promise: PromiseConstructor }); setToStringTag(PromiseConstructor, PROMISE, false, true); setSpecies(PROMISE); PromiseWrapper = getBuiltIn(PROMISE); // statics $({ target: PROMISE, stat: true, forced: FORCED }, { // `Promise.reject` method // https://tc39.es/ecma262/#sec-promise.reject reject: function reject(r) { var capability = newPromiseCapability(this); capability.reject.call(undefined, r); return capability.promise; } }); $({ target: PROMISE, stat: true, forced: IS_PURE || FORCED }, { // `Promise.resolve` method // https://tc39.es/ecma262/#sec-promise.resolve resolve: function resolve(x) { return promiseResolve(IS_PURE && this === PromiseWrapper ? PromiseConstructor : this, x); } }); $({ target: PROMISE, stat: true, forced: INCORRECT_ITERATION }, { // `Promise.all` method // https://tc39.es/ecma262/#sec-promise.all all: function all(iterable) { var C = this; var capability = newPromiseCapability(C); var resolve = capability.resolve; var reject = capability.reject; var result = perform(function () { var $promiseResolve = aFunction(C.resolve); var values = []; var counter = 0; var remaining = 1; iterate(iterable, function (promise) { var index = counter++; var alreadyCalled = false; values.push(undefined); remaining++; $promiseResolve.call(C, promise).then(function (value) { if (alreadyCalled) return; alreadyCalled = true; values[index] = value; --remaining || resolve(values); }, reject); }); --remaining || resolve(values); }); if (result.error) reject(result.value); return capability.promise; }, // `Promise.race` method // https://tc39.es/ecma262/#sec-promise.race race: function race(iterable) { var C = this; var capability = newPromiseCapability(C); var reject = capability.reject; var result = perform(function () { var $promiseResolve = aFunction(C.resolve); iterate(iterable, function (promise) { $promiseResolve.call(C, promise).then(capability.resolve, reject); }); }); if (result.error) reject(result.value); return capability.promise; } }); apollo-server-demo/node_modules/core-js/modules/es.regexp.test.js 0000644 0001750 0000144 00000001667 03560116604 024670 0 ustar andreh users 'use strict'; // TODO: Remove from `core-js@4` since it's moved to entry points require('../modules/es.regexp.exec'); var $ = require('../internals/export'); var isObject = require('../internals/is-object'); var DELEGATES_TO_EXEC = function () { var execCalled = false; var re = /[ac]/; re.exec = function () { execCalled = true; return /./.exec.apply(this, arguments); }; return re.test('abc') === true && execCalled; }(); var nativeTest = /./.test; // `RegExp.prototype.test` method // https://tc39.es/ecma262/#sec-regexp.prototype.test $({ target: 'RegExp', proto: true, forced: !DELEGATES_TO_EXEC }, { test: function (str) { if (typeof this.exec !== 'function') { return nativeTest.call(this, str); } var result = this.exec(str); if (result !== null && !isObject(result)) { throw new Error('RegExp exec method returned something other than an Object or null'); } return !!result; } }); apollo-server-demo/node_modules/core-js/modules/esnext.symbol.async-dispose.js 0000644 0001750 0000144 00000000325 03560116604 027372 0 ustar andreh users var defineWellKnownSymbol = require('../internals/define-well-known-symbol'); // `Symbol.asyncDispose` well-known symbol // https://github.com/tc39/proposal-using-statement defineWellKnownSymbol('asyncDispose'); apollo-server-demo/node_modules/core-js/modules/es.object.freeze.js 0000644 0001750 0000144 00000001157 03560116604 025137 0 ustar andreh users var $ = require('../internals/export'); var FREEZING = require('../internals/freezing'); var fails = require('../internals/fails'); var isObject = require('../internals/is-object'); var onFreeze = require('../internals/internal-metadata').onFreeze; var nativeFreeze = Object.freeze; var FAILS_ON_PRIMITIVES = fails(function () { nativeFreeze(1); }); // `Object.freeze` method // https://tc39.es/ecma262/#sec-object.freeze $({ target: 'Object', stat: true, forced: FAILS_ON_PRIMITIVES, sham: !FREEZING }, { freeze: function freeze(it) { return nativeFreeze && isObject(it) ? nativeFreeze(onFreeze(it)) : it; } }); apollo-server-demo/node_modules/core-js/modules/esnext.async-iterator.drop.js 0000644 0001750 0000144 00000002422 03560116604 027214 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var anObject = require('../internals/an-object'); var toPositiveInteger = require('../internals/to-positive-integer'); var createAsyncIteratorProxy = require('../internals/async-iterator-create-proxy'); var AsyncIteratorProxy = createAsyncIteratorProxy(function (arg, Promise) { var state = this; return new Promise(function (resolve, reject) { var loop = function () { try { Promise.resolve( anObject(state.next.call(state.iterator, state.remaining ? undefined : arg)) ).then(function (step) { try { if (anObject(step).done) { state.done = true; resolve({ done: true, value: undefined }); } else if (state.remaining) { state.remaining--; loop(); } else resolve({ done: false, value: step.value }); } catch (err) { reject(err); } }, reject); } catch (error) { reject(error); } }; loop(); }); }); $({ target: 'AsyncIterator', proto: true, real: true }, { drop: function drop(limit) { return new AsyncIteratorProxy({ iterator: anObject(this), remaining: toPositiveInteger(limit) }); } }); apollo-server-demo/node_modules/core-js/modules/esnext.math.seeded-prng.js 0000644 0001750 0000144 00000002703 03560116604 026434 0 ustar andreh users var $ = require('../internals/export'); var anObject = require('../internals/an-object'); var numberIsFinite = require('../internals/number-is-finite'); var createIteratorConstructor = require('../internals/create-iterator-constructor'); var InternalStateModule = require('../internals/internal-state'); var SEEDED_RANDOM = 'Seeded Random'; var SEEDED_RANDOM_GENERATOR = SEEDED_RANDOM + ' Generator'; var setInternalState = InternalStateModule.set; var getInternalState = InternalStateModule.getterFor(SEEDED_RANDOM_GENERATOR); var SEED_TYPE_ERROR = 'Math.seededPRNG() argument should have a "seed" field with a finite value.'; var $SeededRandomGenerator = createIteratorConstructor(function SeededRandomGenerator(seed) { setInternalState(this, { type: SEEDED_RANDOM_GENERATOR, seed: seed % 2147483647 }); }, SEEDED_RANDOM, function next() { var state = getInternalState(this); var seed = state.seed = (state.seed * 1103515245 + 12345) % 2147483647; return { value: (seed & 1073741823) / 1073741823, done: false }; }); // `Math.seededPRNG` method // https://github.com/tc39/proposal-seeded-random // based on https://github.com/tc39/proposal-seeded-random/blob/78b8258835b57fc2100d076151ab506bc3202ae6/demo.html $({ target: 'Math', stat: true, forced: true }, { seededPRNG: function seededPRNG(it) { var seed = anObject(it).seed; if (!numberIsFinite(seed)) throw TypeError(SEED_TYPE_ERROR); return new $SeededRandomGenerator(seed); } }); apollo-server-demo/node_modules/core-js/modules/esnext.set.some.js 0000644 0001750 0000144 00000001542 03560116604 025044 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var anObject = require('../internals/an-object'); var bind = require('../internals/function-bind-context'); var getSetIterator = require('../internals/get-set-iterator'); var iterate = require('../internals/iterate'); // `Set.prototype.some` method // https://github.com/tc39/proposal-collection-methods $({ target: 'Set', proto: true, real: true, forced: IS_PURE }, { some: function some(callbackfn /* , thisArg */) { var set = anObject(this); var iterator = getSetIterator(set); var boundFunction = bind(callbackfn, arguments.length > 1 ? arguments[1] : undefined, 3); return iterate(iterator, function (value, stop) { if (boundFunction(value, value, set)) return stop(); }, { IS_ITERATOR: true, INTERRUPTED: true }).stopped; } }); apollo-server-demo/node_modules/core-js/modules/esnext.array.at.js 0000644 0001750 0000144 00000001261 03560116604 025026 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var toObject = require('../internals/to-object'); var toLength = require('../internals/to-length'); var toInteger = require('../internals/to-integer'); var addToUnscopables = require('../internals/add-to-unscopables'); // `Array.prototype.at` method // https://github.com/tc39/proposal-relative-indexing-method $({ target: 'Array', proto: true }, { at: function at(index) { var O = toObject(this); var len = toLength(O.length); var relativeIndex = toInteger(index); var k = relativeIndex >= 0 ? relativeIndex : len + relativeIndex; return (k < 0 || k >= len) ? undefined : O[k]; } }); addToUnscopables('at'); apollo-server-demo/node_modules/core-js/modules/es.typed-array.to-string.js 0000644 0001750 0000144 00000001363 03560116604 026577 0 ustar andreh users 'use strict'; var exportTypedArrayMethod = require('../internals/array-buffer-view-core').exportTypedArrayMethod; var fails = require('../internals/fails'); var global = require('../internals/global'); var Uint8Array = global.Uint8Array; var Uint8ArrayPrototype = Uint8Array && Uint8Array.prototype || {}; var arrayToString = [].toString; var arrayJoin = [].join; if (fails(function () { arrayToString.call({}); })) { arrayToString = function toString() { return arrayJoin.call(this); }; } var IS_NOT_ARRAY_METHOD = Uint8ArrayPrototype.toString != arrayToString; // `%TypedArray%.prototype.toString` method // https://tc39.es/ecma262/#sec-%typedarray%.prototype.tostring exportTypedArrayMethod('toString', arrayToString, IS_NOT_ARRAY_METHOD); apollo-server-demo/node_modules/core-js/modules/es.number.is-safe-integer.js 0000644 0001750 0000144 00000000551 03560116604 026660 0 ustar andreh users var $ = require('../internals/export'); var isInteger = require('../internals/is-integer'); var abs = Math.abs; // `Number.isSafeInteger` method // https://tc39.es/ecma262/#sec-number.issafeinteger $({ target: 'Number', stat: true }, { isSafeInteger: function isSafeInteger(number) { return isInteger(number) && abs(number) <= 0x1FFFFFFFFFFFFF; } }); apollo-server-demo/node_modules/core-js/modules/esnext.set.filter.js 0000644 0001750 0000144 00000002174 03560116604 025370 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var getBuiltIn = require('../internals/get-built-in'); var anObject = require('../internals/an-object'); var aFunction = require('../internals/a-function'); var bind = require('../internals/function-bind-context'); var speciesConstructor = require('../internals/species-constructor'); var getSetIterator = require('../internals/get-set-iterator'); var iterate = require('../internals/iterate'); // `Set.prototype.filter` method // https://github.com/tc39/proposal-collection-methods $({ target: 'Set', proto: true, real: true, forced: IS_PURE }, { filter: function filter(callbackfn /* , thisArg */) { var set = anObject(this); var iterator = getSetIterator(set); var boundFunction = bind(callbackfn, arguments.length > 1 ? arguments[1] : undefined, 3); var newSet = new (speciesConstructor(set, getBuiltIn('Set')))(); var adder = aFunction(newSet.add); iterate(iterator, function (value) { if (boundFunction(value, value, set)) adder.call(newSet, value); }, { IS_ITERATOR: true }); return newSet; } }); apollo-server-demo/node_modules/core-js/modules/es.object.get-own-property-names.js 0000644 0001750 0000144 00000000752 03560116604 030222 0 ustar andreh users var $ = require('../internals/export'); var fails = require('../internals/fails'); var nativeGetOwnPropertyNames = require('../internals/object-get-own-property-names-external').f; var FAILS_ON_PRIMITIVES = fails(function () { return !Object.getOwnPropertyNames(1); }); // `Object.getOwnPropertyNames` method // https://tc39.es/ecma262/#sec-object.getownpropertynames $({ target: 'Object', stat: true, forced: FAILS_ON_PRIMITIVES }, { getOwnPropertyNames: nativeGetOwnPropertyNames }); apollo-server-demo/node_modules/core-js/modules/es.symbol.search.js 0000644 0001750 0000144 00000000303 03560116604 025153 0 ustar andreh users var defineWellKnownSymbol = require('../internals/define-well-known-symbol'); // `Symbol.search` well-known symbol // https://tc39.es/ecma262/#sec-symbol.search defineWellKnownSymbol('search'); apollo-server-demo/node_modules/core-js/modules/es.typed-array.float32-array.js 0000644 0001750 0000144 00000000523 03560116604 027234 0 ustar andreh users var createTypedArrayConstructor = require('../internals/typed-array-constructor'); // `Float32Array` constructor // https://tc39.es/ecma262/#sec-typedarray-objects createTypedArrayConstructor('Float32', function (init) { return function Float32Array(data, byteOffset, length) { return init(this, data, byteOffset, length); }; }); apollo-server-demo/node_modules/core-js/modules/web.url.to-json.js 0000644 0001750 0000144 00000000421 03560116604 024743 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); // `URL.prototype.toJSON` method // https://url.spec.whatwg.org/#dom-url-tojson $({ target: 'URL', proto: true, enumerable: true }, { toJSON: function toJSON() { return URL.prototype.toString.call(this); } }); apollo-server-demo/node_modules/core-js/modules/es.array-buffer.constructor.js 0000644 0001750 0000144 00000001054 03560116604 027357 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var global = require('../internals/global'); var arrayBufferModule = require('../internals/array-buffer'); var setSpecies = require('../internals/set-species'); var ARRAY_BUFFER = 'ArrayBuffer'; var ArrayBuffer = arrayBufferModule[ARRAY_BUFFER]; var NativeArrayBuffer = global[ARRAY_BUFFER]; // `ArrayBuffer` constructor // https://tc39.es/ecma262/#sec-arraybuffer-constructor $({ global: true, forced: NativeArrayBuffer !== ArrayBuffer }, { ArrayBuffer: ArrayBuffer }); setSpecies(ARRAY_BUFFER); apollo-server-demo/node_modules/core-js/modules/es.number.to-fixed.js 0000644 0001750 0000144 00000006470 03560116604 025423 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var toInteger = require('../internals/to-integer'); var thisNumberValue = require('../internals/this-number-value'); var repeat = require('../internals/string-repeat'); var fails = require('../internals/fails'); var nativeToFixed = 1.0.toFixed; var floor = Math.floor; var pow = function (x, n, acc) { return n === 0 ? acc : n % 2 === 1 ? pow(x, n - 1, acc * x) : pow(x * x, n / 2, acc); }; var log = function (x) { var n = 0; var x2 = x; while (x2 >= 4096) { n += 12; x2 /= 4096; } while (x2 >= 2) { n += 1; x2 /= 2; } return n; }; var FORCED = nativeToFixed && ( 0.00008.toFixed(3) !== '0.000' || 0.9.toFixed(0) !== '1' || 1.255.toFixed(2) !== '1.25' || 1000000000000000128.0.toFixed(0) !== '1000000000000000128' ) || !fails(function () { // V8 ~ Android 4.3- nativeToFixed.call({}); }); // `Number.prototype.toFixed` method // https://tc39.es/ecma262/#sec-number.prototype.tofixed $({ target: 'Number', proto: true, forced: FORCED }, { // eslint-disable-next-line max-statements toFixed: function toFixed(fractionDigits) { var number = thisNumberValue(this); var fractDigits = toInteger(fractionDigits); var data = [0, 0, 0, 0, 0, 0]; var sign = ''; var result = '0'; var e, z, j, k; var multiply = function (n, c) { var index = -1; var c2 = c; while (++index < 6) { c2 += n * data[index]; data[index] = c2 % 1e7; c2 = floor(c2 / 1e7); } }; var divide = function (n) { var index = 6; var c = 0; while (--index >= 0) { c += data[index]; data[index] = floor(c / n); c = (c % n) * 1e7; } }; var dataToString = function () { var index = 6; var s = ''; while (--index >= 0) { if (s !== '' || index === 0 || data[index] !== 0) { var t = String(data[index]); s = s === '' ? t : s + repeat.call('0', 7 - t.length) + t; } } return s; }; if (fractDigits < 0 || fractDigits > 20) throw RangeError('Incorrect fraction digits'); // eslint-disable-next-line no-self-compare if (number != number) return 'NaN'; if (number <= -1e21 || number >= 1e21) return String(number); if (number < 0) { sign = '-'; number = -number; } if (number > 1e-21) { e = log(number * pow(2, 69, 1)) - 69; z = e < 0 ? number * pow(2, -e, 1) : number / pow(2, e, 1); z *= 0x10000000000000; e = 52 - e; if (e > 0) { multiply(0, z); j = fractDigits; while (j >= 7) { multiply(1e7, 0); j -= 7; } multiply(pow(10, j, 1), 0); j = e - 1; while (j >= 23) { divide(1 << 23); j -= 23; } divide(1 << j); multiply(1, 1); divide(2); result = dataToString(); } else { multiply(0, z); multiply(1 << -e, 0); result = dataToString() + repeat.call('0', fractDigits); } } if (fractDigits > 0) { k = result.length; result = sign + (k <= fractDigits ? '0.' + repeat.call('0', fractDigits - k) + result : result.slice(0, k - fractDigits) + '.' + result.slice(k - fractDigits)); } else { result = sign + result; } return result; } }); apollo-server-demo/node_modules/core-js/modules/esnext.weak-set.of.js 0000644 0001750 0000144 00000000345 03560116604 025432 0 ustar andreh users var $ = require('../internals/export'); var of = require('../internals/collection-of'); // `WeakSet.of` method // https://tc39.github.io/proposal-setmap-offrom/#sec-weakset.of $({ target: 'WeakSet', stat: true }, { of: of }); apollo-server-demo/node_modules/core-js/modules/es.string.iterator.js 0000644 0001750 0000144 00000002016 03560116604 025543 0 ustar andreh users 'use strict'; var charAt = require('../internals/string-multibyte').charAt; var InternalStateModule = require('../internals/internal-state'); var defineIterator = require('../internals/define-iterator'); var STRING_ITERATOR = 'String Iterator'; var setInternalState = InternalStateModule.set; var getInternalState = InternalStateModule.getterFor(STRING_ITERATOR); // `String.prototype[@@iterator]` method // https://tc39.es/ecma262/#sec-string.prototype-@@iterator defineIterator(String, 'String', function (iterated) { setInternalState(this, { type: STRING_ITERATOR, string: String(iterated), index: 0 }); // `%StringIteratorPrototype%.next` method // https://tc39.es/ecma262/#sec-%stringiteratorprototype%.next }, function next() { var state = getInternalState(this); var string = state.string; var index = state.index; var point; if (index >= string.length) return { value: undefined, done: true }; point = charAt(string, index); state.index += point.length; return { value: point, done: false }; }); apollo-server-demo/node_modules/core-js/modules/es.object.create.js 0000644 0001750 0000144 00000000453 03560116604 025120 0 ustar andreh users var $ = require('../internals/export'); var DESCRIPTORS = require('../internals/descriptors'); var create = require('../internals/object-create'); // `Object.create` method // https://tc39.es/ecma262/#sec-object.create $({ target: 'Object', stat: true, sham: !DESCRIPTORS }, { create: create }); apollo-server-demo/node_modules/core-js/modules/es.string.big.js 0000644 0001750 0000144 00000000646 03560116604 024462 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var createHTML = require('../internals/create-html'); var forcedStringHTMLMethod = require('../internals/string-html-forced'); // `String.prototype.big` method // https://tc39.es/ecma262/#sec-string.prototype.big $({ target: 'String', proto: true, forced: forcedStringHTMLMethod('big') }, { big: function big() { return createHTML(this, 'big', '', ''); } }); apollo-server-demo/node_modules/core-js/modules/esnext.weak-set.from.js 0000644 0001750 0000144 00000000361 03560116604 025767 0 ustar andreh users var $ = require('../internals/export'); var from = require('../internals/collection-from'); // `WeakSet.from` method // https://tc39.github.io/proposal-setmap-offrom/#sec-weakset.from $({ target: 'WeakSet', stat: true }, { from: from }); apollo-server-demo/node_modules/core-js/modules/esnext.string.code-points.js 0000644 0001750 0000144 00000002633 03560116604 027042 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var createIteratorConstructor = require('../internals/create-iterator-constructor'); var requireObjectCoercible = require('../internals/require-object-coercible'); var InternalStateModule = require('../internals/internal-state'); var StringMultibyteModule = require('../internals/string-multibyte'); var codeAt = StringMultibyteModule.codeAt; var charAt = StringMultibyteModule.charAt; var STRING_ITERATOR = 'String Iterator'; var setInternalState = InternalStateModule.set; var getInternalState = InternalStateModule.getterFor(STRING_ITERATOR); // TODO: unify with String#@@iterator var $StringIterator = createIteratorConstructor(function StringIterator(string) { setInternalState(this, { type: STRING_ITERATOR, string: string, index: 0 }); }, 'String', function next() { var state = getInternalState(this); var string = state.string; var index = state.index; var point; if (index >= string.length) return { value: undefined, done: true }; point = charAt(string, index); state.index += point.length; return { value: { codePoint: codeAt(point, 0), position: index }, done: false }; }); // `String.prototype.codePoints` method // https://github.com/tc39/proposal-string-prototype-codepoints $({ target: 'String', proto: true }, { codePoints: function codePoints() { return new $StringIterator(String(requireObjectCoercible(this))); } }); apollo-server-demo/node_modules/core-js/modules/es.typed-array.to-locale-string.js 0000644 0001750 0000144 00000002031 03560116604 030025 0 ustar andreh users 'use strict'; var global = require('../internals/global'); var ArrayBufferViewCore = require('../internals/array-buffer-view-core'); var fails = require('../internals/fails'); var Int8Array = global.Int8Array; var aTypedArray = ArrayBufferViewCore.aTypedArray; var exportTypedArrayMethod = ArrayBufferViewCore.exportTypedArrayMethod; var $toLocaleString = [].toLocaleString; var $slice = [].slice; // iOS Safari 6.x fails here var TO_LOCALE_STRING_BUG = !!Int8Array && fails(function () { $toLocaleString.call(new Int8Array(1)); }); var FORCED = fails(function () { return [1, 2].toLocaleString() != new Int8Array([1, 2]).toLocaleString(); }) || !fails(function () { Int8Array.prototype.toLocaleString.call([1, 2]); }); // `%TypedArray%.prototype.toLocaleString` method // https://tc39.es/ecma262/#sec-%typedarray%.prototype.tolocalestring exportTypedArrayMethod('toLocaleString', function toLocaleString() { return $toLocaleString.apply(TO_LOCALE_STRING_BUG ? $slice.call(aTypedArray(this)) : aTypedArray(this), arguments); }, FORCED); apollo-server-demo/node_modules/core-js/modules/es.array.find.js 0000644 0001750 0000144 00000001534 03560116604 024446 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var $find = require('../internals/array-iteration').find; var addToUnscopables = require('../internals/add-to-unscopables'); var arrayMethodUsesToLength = require('../internals/array-method-uses-to-length'); var FIND = 'find'; var SKIPS_HOLES = true; var USES_TO_LENGTH = arrayMethodUsesToLength(FIND); // Shouldn't skip holes if (FIND in []) Array(1)[FIND](function () { SKIPS_HOLES = false; }); // `Array.prototype.find` method // https://tc39.es/ecma262/#sec-array.prototype.find $({ target: 'Array', proto: true, forced: SKIPS_HOLES || !USES_TO_LENGTH }, { find: function find(callbackfn /* , that = undefined */) { return $find(this, callbackfn, arguments.length > 1 ? arguments[1] : undefined); } }); // https://tc39.es/ecma262/#sec-array.prototype-@@unscopables addToUnscopables(FIND); apollo-server-demo/node_modules/core-js/modules/es.typed-array.int16-array.js 0000644 0001750 0000144 00000000515 03560116604 026724 0 ustar andreh users var createTypedArrayConstructor = require('../internals/typed-array-constructor'); // `Int16Array` constructor // https://tc39.es/ecma262/#sec-typedarray-objects createTypedArrayConstructor('Int16', function (init) { return function Int16Array(data, byteOffset, length) { return init(this, data, byteOffset, length); }; }); apollo-server-demo/node_modules/core-js/modules/esnext.async-iterator.every.js 0000644 0001750 0000144 00000000456 03560116604 027407 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var $every = require('../internals/async-iterator-iteration').every; $({ target: 'AsyncIterator', proto: true, real: true }, { every: function every(fn) { return $every(this, fn); } }); apollo-server-demo/node_modules/core-js/modules/esnext.symbol.observable.js 0000644 0001750 0000144 00000000314 03560116604 026733 0 ustar andreh users var defineWellKnownSymbol = require('../internals/define-well-known-symbol'); // `Symbol.observable` well-known symbol // https://github.com/tc39/proposal-observable defineWellKnownSymbol('observable'); apollo-server-demo/node_modules/core-js/modules/es.regexp.exec.js 0000644 0001750 0000144 00000000422 03560116604 024621 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var exec = require('../internals/regexp-exec'); // `RegExp.prototype.exec` method // https://tc39.es/ecma262/#sec-regexp.prototype.exec $({ target: 'RegExp', proto: true, forced: /./.exec !== exec }, { exec: exec }); apollo-server-demo/node_modules/core-js/modules/es.array.reduce-right.js 0000644 0001750 0000144 00000002251 03560116604 026105 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var $reduceRight = require('../internals/array-reduce').right; var arrayMethodIsStrict = require('../internals/array-method-is-strict'); var arrayMethodUsesToLength = require('../internals/array-method-uses-to-length'); var CHROME_VERSION = require('../internals/engine-v8-version'); var IS_NODE = require('../internals/engine-is-node'); var STRICT_METHOD = arrayMethodIsStrict('reduceRight'); // For preventing possible almost infinite loop in non-standard implementations, test the forward version of the method var USES_TO_LENGTH = arrayMethodUsesToLength('reduce', { 1: 0 }); // Chrome 80-82 has a critical bug // https://bugs.chromium.org/p/chromium/issues/detail?id=1049982 var CHROME_BUG = !IS_NODE && CHROME_VERSION > 79 && CHROME_VERSION < 83; // `Array.prototype.reduceRight` method // https://tc39.es/ecma262/#sec-array.prototype.reduceright $({ target: 'Array', proto: true, forced: !STRICT_METHOD || !USES_TO_LENGTH || CHROME_BUG }, { reduceRight: function reduceRight(callbackfn /* , initialValue */) { return $reduceRight(this, callbackfn, arguments.length, arguments.length > 1 ? arguments[1] : undefined); } }); apollo-server-demo/node_modules/core-js/modules/es.symbol.to-primitive.js 0000644 0001750 0000144 00000000322 03560116604 026337 0 ustar andreh users var defineWellKnownSymbol = require('../internals/define-well-known-symbol'); // `Symbol.toPrimitive` well-known symbol // https://tc39.es/ecma262/#sec-symbol.toprimitive defineWellKnownSymbol('toPrimitive'); apollo-server-demo/node_modules/core-js/modules/esnext.object.iterate-entries.js 0000644 0001750 0000144 00000000540 03560116604 027655 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var ObjectIterator = require('../internals/object-iterator'); // `Object.iterateEntries` method // https://github.com/tc39/proposal-object-iteration $({ target: 'Object', stat: true }, { iterateEntries: function iterateEntries(object) { return new ObjectIterator(object, 'entries'); } }); apollo-server-demo/node_modules/core-js/modules/esnext.map.filter.js 0000644 0001750 0000144 00000002230 03560116604 025343 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var getBuiltIn = require('../internals/get-built-in'); var anObject = require('../internals/an-object'); var aFunction = require('../internals/a-function'); var bind = require('../internals/function-bind-context'); var speciesConstructor = require('../internals/species-constructor'); var getMapIterator = require('../internals/get-map-iterator'); var iterate = require('../internals/iterate'); // `Map.prototype.filter` method // https://github.com/tc39/proposal-collection-methods $({ target: 'Map', proto: true, real: true, forced: IS_PURE }, { filter: function filter(callbackfn /* , thisArg */) { var map = anObject(this); var iterator = getMapIterator(map); var boundFunction = bind(callbackfn, arguments.length > 1 ? arguments[1] : undefined, 3); var newMap = new (speciesConstructor(map, getBuiltIn('Map')))(); var setter = aFunction(newMap.set); iterate(iterator, function (key, value) { if (boundFunction(value, key, map)) setter.call(newMap, key, value); }, { AS_ENTRIES: true, IS_ITERATOR: true }); return newMap; } }); apollo-server-demo/node_modules/core-js/modules/esnext.set.every.js 0000644 0001750 0000144 00000001547 03560116604 025240 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var anObject = require('../internals/an-object'); var bind = require('../internals/function-bind-context'); var getSetIterator = require('../internals/get-set-iterator'); var iterate = require('../internals/iterate'); // `Set.prototype.every` method // https://github.com/tc39/proposal-collection-methods $({ target: 'Set', proto: true, real: true, forced: IS_PURE }, { every: function every(callbackfn /* , thisArg */) { var set = anObject(this); var iterator = getSetIterator(set); var boundFunction = bind(callbackfn, arguments.length > 1 ? arguments[1] : undefined, 3); return !iterate(iterator, function (value, stop) { if (!boundFunction(value, value, set)) return stop(); }, { IS_ITERATOR: true, INTERRUPTED: true }).stopped; } }); apollo-server-demo/node_modules/core-js/modules/es.typed-array.find-index.js 0000644 0001750 0000144 00000001104 03560116604 026667 0 ustar andreh users 'use strict'; var ArrayBufferViewCore = require('../internals/array-buffer-view-core'); var $findIndex = require('../internals/array-iteration').findIndex; var aTypedArray = ArrayBufferViewCore.aTypedArray; var exportTypedArrayMethod = ArrayBufferViewCore.exportTypedArrayMethod; // `%TypedArray%.prototype.findIndex` method // https://tc39.es/ecma262/#sec-%typedarray%.prototype.findindex exportTypedArrayMethod('findIndex', function findIndex(predicate /* , thisArg */) { return $findIndex(aTypedArray(this), predicate, arguments.length > 1 ? arguments[1] : undefined); }); apollo-server-demo/node_modules/core-js/modules/es.reflect.apply.js 0000644 0001750 0000144 00000001566 03560116604 025166 0 ustar andreh users var $ = require('../internals/export'); var getBuiltIn = require('../internals/get-built-in'); var aFunction = require('../internals/a-function'); var anObject = require('../internals/an-object'); var fails = require('../internals/fails'); var nativeApply = getBuiltIn('Reflect', 'apply'); var functionApply = Function.apply; // MS Edge argumentsList argument is optional var OPTIONAL_ARGUMENTS_LIST = !fails(function () { nativeApply(function () { /* empty */ }); }); // `Reflect.apply` method // https://tc39.es/ecma262/#sec-reflect.apply $({ target: 'Reflect', stat: true, forced: OPTIONAL_ARGUMENTS_LIST }, { apply: function apply(target, thisArgument, argumentsList) { aFunction(target); anObject(argumentsList); return nativeApply ? nativeApply(target, thisArgument, argumentsList) : functionApply.call(target, thisArgument, argumentsList); } }); apollo-server-demo/node_modules/core-js/modules/es.object.define-setter.js 0000644 0001750 0000144 00000001341 03560116604 026410 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var DESCRIPTORS = require('../internals/descriptors'); var FORCED = require('../internals/object-prototype-accessors-forced'); var toObject = require('../internals/to-object'); var aFunction = require('../internals/a-function'); var definePropertyModule = require('../internals/object-define-property'); // `Object.prototype.__defineSetter__` method // https://tc39.es/ecma262/#sec-object.prototype.__defineSetter__ if (DESCRIPTORS) { $({ target: 'Object', proto: true, forced: FORCED }, { __defineSetter__: function __defineSetter__(P, setter) { definePropertyModule.f(toObject(this), P, { set: aFunction(setter), enumerable: true, configurable: true }); } }); } apollo-server-demo/node_modules/core-js/modules/esnext.string.match-all.js 0000644 0001750 0000144 00000000103 03560116604 026446 0 ustar andreh users // TODO: Remove from `core-js@4` require('./es.string.match-all'); apollo-server-demo/node_modules/core-js/modules/es.object.values.js 0000644 0001750 0000144 00000000423 03560116604 025151 0 ustar andreh users var $ = require('../internals/export'); var $values = require('../internals/object-to-array').values; // `Object.values` method // https://tc39.es/ecma262/#sec-object.values $({ target: 'Object', stat: true }, { values: function values(O) { return $values(O); } }); apollo-server-demo/node_modules/core-js/modules/esnext.array.last-item.js 0000644 0001750 0000144 00000001537 03560116604 026327 0 ustar andreh users 'use strict'; var DESCRIPTORS = require('../internals/descriptors'); var addToUnscopables = require('../internals/add-to-unscopables'); var toObject = require('../internals/to-object'); var toLength = require('../internals/to-length'); var defineProperty = require('../internals/object-define-property').f; // `Array.prototype.lastIndex` accessor // https://github.com/keithamus/proposal-array-last if (DESCRIPTORS && !('lastItem' in [])) { defineProperty(Array.prototype, 'lastItem', { configurable: true, get: function lastItem() { var O = toObject(this); var len = toLength(O.length); return len == 0 ? undefined : O[len - 1]; }, set: function lastItem(value) { var O = toObject(this); var len = toLength(O.length); return O[len == 0 ? 0 : len - 1] = value; } }); addToUnscopables('lastItem'); } apollo-server-demo/node_modules/core-js/modules/es.array.flat-map.js 0000644 0001750 0000144 00000001457 03560116604 025233 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var flattenIntoArray = require('../internals/flatten-into-array'); var toObject = require('../internals/to-object'); var toLength = require('../internals/to-length'); var aFunction = require('../internals/a-function'); var arraySpeciesCreate = require('../internals/array-species-create'); // `Array.prototype.flatMap` method // https://tc39.es/ecma262/#sec-array.prototype.flatmap $({ target: 'Array', proto: true }, { flatMap: function flatMap(callbackfn /* , thisArg */) { var O = toObject(this); var sourceLen = toLength(O.length); var A; aFunction(callbackfn); A = arraySpeciesCreate(O, 0); A.length = flattenIntoArray(A, O, O, sourceLen, 0, 1, callbackfn, arguments.length > 1 ? arguments[1] : undefined); return A; } }); apollo-server-demo/node_modules/core-js/modules/es.math.atanh.js 0000644 0001750 0000144 00000000575 03560116604 024440 0 ustar andreh users var $ = require('../internals/export'); var nativeAtanh = Math.atanh; var log = Math.log; // `Math.atanh` method // https://tc39.es/ecma262/#sec-math.atanh // Tor Browser bug: Math.atanh(-0) -> 0 $({ target: 'Math', stat: true, forced: !(nativeAtanh && 1 / nativeAtanh(-0) < 0) }, { atanh: function atanh(x) { return (x = +x) == 0 ? x : log((1 + x) / (1 - x)) / 2; } }); apollo-server-demo/node_modules/core-js/modules/es.string.fontcolor.js 0000644 0001750 0000144 00000000722 03560116604 025721 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var createHTML = require('../internals/create-html'); var forcedStringHTMLMethod = require('../internals/string-html-forced'); // `String.prototype.fontcolor` method // https://tc39.es/ecma262/#sec-string.prototype.fontcolor $({ target: 'String', proto: true, forced: forcedStringHTMLMethod('fontcolor') }, { fontcolor: function fontcolor(color) { return createHTML(this, 'font', 'color', color); } }); apollo-server-demo/node_modules/core-js/modules/es.object.define-property.js 0000644 0001750 0000144 00000000614 03560116604 026770 0 ustar andreh users var $ = require('../internals/export'); var DESCRIPTORS = require('../internals/descriptors'); var objectDefinePropertyModile = require('../internals/object-define-property'); // `Object.defineProperty` method // https://tc39.es/ecma262/#sec-object.defineproperty $({ target: 'Object', stat: true, forced: !DESCRIPTORS, sham: !DESCRIPTORS }, { defineProperty: objectDefinePropertyModile.f }); apollo-server-demo/node_modules/core-js/modules/es.reflect.set-prototype-of.js 0000644 0001750 0000144 00000001171 03560116604 027271 0 ustar andreh users var $ = require('../internals/export'); var anObject = require('../internals/an-object'); var aPossiblePrototype = require('../internals/a-possible-prototype'); var objectSetPrototypeOf = require('../internals/object-set-prototype-of'); // `Reflect.setPrototypeOf` method // https://tc39.es/ecma262/#sec-reflect.setprototypeof if (objectSetPrototypeOf) $({ target: 'Reflect', stat: true }, { setPrototypeOf: function setPrototypeOf(target, proto) { anObject(target); aPossiblePrototype(proto); try { objectSetPrototypeOf(target, proto); return true; } catch (error) { return false; } } }); apollo-server-demo/node_modules/core-js/modules/es.typed-array.includes.js 0000644 0001750 0000144 00000001106 03560116604 026452 0 ustar andreh users 'use strict'; var ArrayBufferViewCore = require('../internals/array-buffer-view-core'); var $includes = require('../internals/array-includes').includes; var aTypedArray = ArrayBufferViewCore.aTypedArray; var exportTypedArrayMethod = ArrayBufferViewCore.exportTypedArrayMethod; // `%TypedArray%.prototype.includes` method // https://tc39.es/ecma262/#sec-%typedarray%.prototype.includes exportTypedArrayMethod('includes', function includes(searchElement /* , fromIndex */) { return $includes(aTypedArray(this), searchElement, arguments.length > 1 ? arguments[1] : undefined); }); apollo-server-demo/node_modules/core-js/modules/esnext.math.degrees.js 0000644 0001750 0000144 00000000421 03560116604 025650 0 ustar andreh users var $ = require('../internals/export'); var RAD_PER_DEG = 180 / Math.PI; // `Math.degrees` method // https://rwaldron.github.io/proposal-math-extensions/ $({ target: 'Math', stat: true }, { degrees: function degrees(radians) { return radians * RAD_PER_DEG; } }); apollo-server-demo/node_modules/core-js/modules/es.number.min-safe-integer.js 0000644 0001750 0000144 00000000327 03560116604 027031 0 ustar andreh users var $ = require('../internals/export'); // `Number.MIN_SAFE_INTEGER` constant // https://tc39.es/ecma262/#sec-number.min_safe_integer $({ target: 'Number', stat: true }, { MIN_SAFE_INTEGER: -0x1FFFFFFFFFFFFF }); apollo-server-demo/node_modules/core-js/modules/esnext.map.some.js 0000644 0001750 0000144 00000001567 03560116604 025035 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var anObject = require('../internals/an-object'); var bind = require('../internals/function-bind-context'); var getMapIterator = require('../internals/get-map-iterator'); var iterate = require('../internals/iterate'); // `Set.prototype.some` method // https://github.com/tc39/proposal-collection-methods $({ target: 'Map', proto: true, real: true, forced: IS_PURE }, { some: function some(callbackfn /* , thisArg */) { var map = anObject(this); var iterator = getMapIterator(map); var boundFunction = bind(callbackfn, arguments.length > 1 ? arguments[1] : undefined, 3); return iterate(iterator, function (key, value, stop) { if (boundFunction(value, key, map)) return stop(); }, { AS_ENTRIES: true, IS_ITERATOR: true, INTERRUPTED: true }).stopped; } }); apollo-server-demo/node_modules/core-js/modules/es.string.includes.js 0000644 0001750 0000144 00000001224 03560116604 025520 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var notARegExp = require('../internals/not-a-regexp'); var requireObjectCoercible = require('../internals/require-object-coercible'); var correctIsRegExpLogic = require('../internals/correct-is-regexp-logic'); // `String.prototype.includes` method // https://tc39.es/ecma262/#sec-string.prototype.includes $({ target: 'String', proto: true, forced: !correctIsRegExpLogic('includes') }, { includes: function includes(searchString /* , position = 0 */) { return !!~String(requireObjectCoercible(this)) .indexOf(notARegExp(searchString), arguments.length > 1 ? arguments[1] : undefined); } }); apollo-server-demo/node_modules/core-js/modules/esnext.async-iterator.for-each.js 0000644 0001750 0000144 00000000470 03560116604 027735 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var $forEach = require('../internals/async-iterator-iteration').forEach; $({ target: 'AsyncIterator', proto: true, real: true }, { forEach: function forEach(fn) { return $forEach(this, fn); } }); apollo-server-demo/node_modules/core-js/modules/es.typed-array.int8-array.js 0000644 0001750 0000144 00000000512 03560116604 026642 0 ustar andreh users var createTypedArrayConstructor = require('../internals/typed-array-constructor'); // `Int8Array` constructor // https://tc39.es/ecma262/#sec-typedarray-objects createTypedArrayConstructor('Int8', function (init) { return function Int8Array(data, byteOffset, length) { return init(this, data, byteOffset, length); }; }); apollo-server-demo/node_modules/core-js/modules/es.typed-array.int32-array.js 0000644 0001750 0000144 00000000515 03560116604 026722 0 ustar andreh users var createTypedArrayConstructor = require('../internals/typed-array-constructor'); // `Int32Array` constructor // https://tc39.es/ecma262/#sec-typedarray-objects createTypedArrayConstructor('Int32', function (init) { return function Int32Array(data, byteOffset, length) { return init(this, data, byteOffset, length); }; }); apollo-server-demo/node_modules/core-js/modules/es.typed-array.float64-array.js 0000644 0001750 0000144 00000000523 03560116604 027241 0 ustar andreh users var createTypedArrayConstructor = require('../internals/typed-array-constructor'); // `Float64Array` constructor // https://tc39.es/ecma262/#sec-typedarray-objects createTypedArrayConstructor('Float64', function (init) { return function Float64Array(data, byteOffset, length) { return init(this, data, byteOffset, length); }; }); apollo-server-demo/node_modules/core-js/modules/es.reflect.own-keys.js 0000644 0001750 0000144 00000000343 03560116604 025605 0 ustar andreh users var $ = require('../internals/export'); var ownKeys = require('../internals/own-keys'); // `Reflect.ownKeys` method // https://tc39.es/ecma262/#sec-reflect.ownkeys $({ target: 'Reflect', stat: true }, { ownKeys: ownKeys }); apollo-server-demo/node_modules/core-js/modules/esnext.async-iterator.constructor.js 0000644 0001750 0000144 00000002152 03560116604 030635 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var anInstance = require('../internals/an-instance'); var createNonEnumerableProperty = require('../internals/create-non-enumerable-property'); var has = require('../internals/has'); var wellKnownSymbol = require('../internals/well-known-symbol'); var AsyncIteratorPrototype = require('../internals/async-iterator-prototype'); var IS_PURE = require('../internals/is-pure'); var TO_STRING_TAG = wellKnownSymbol('toStringTag'); var AsyncIteratorConstructor = function AsyncIterator() { anInstance(this, AsyncIteratorConstructor); }; AsyncIteratorConstructor.prototype = AsyncIteratorPrototype; if (!has(AsyncIteratorPrototype, TO_STRING_TAG)) { createNonEnumerableProperty(AsyncIteratorPrototype, TO_STRING_TAG, 'AsyncIterator'); } if (!has(AsyncIteratorPrototype, 'constructor') || AsyncIteratorPrototype.constructor === Object) { createNonEnumerableProperty(AsyncIteratorPrototype, 'constructor', AsyncIteratorConstructor); } $({ global: true, forced: IS_PURE }, { AsyncIterator: AsyncIteratorConstructor }); apollo-server-demo/node_modules/core-js/modules/es.symbol.split.js 0000644 0001750 0000144 00000000300 03560116604 025036 0 ustar andreh users var defineWellKnownSymbol = require('../internals/define-well-known-symbol'); // `Symbol.split` well-known symbol // https://tc39.es/ecma262/#sec-symbol.split defineWellKnownSymbol('split'); apollo-server-demo/node_modules/core-js/modules/es.promise.finally.js 0000644 0001750 0000144 00000003026 03560116604 025522 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var NativePromise = require('../internals/native-promise-constructor'); var fails = require('../internals/fails'); var getBuiltIn = require('../internals/get-built-in'); var speciesConstructor = require('../internals/species-constructor'); var promiseResolve = require('../internals/promise-resolve'); var redefine = require('../internals/redefine'); // Safari bug https://bugs.webkit.org/show_bug.cgi?id=200829 var NON_GENERIC = !!NativePromise && fails(function () { NativePromise.prototype['finally'].call({ then: function () { /* empty */ } }, function () { /* empty */ }); }); // `Promise.prototype.finally` method // https://tc39.es/ecma262/#sec-promise.prototype.finally $({ target: 'Promise', proto: true, real: true, forced: NON_GENERIC }, { 'finally': function (onFinally) { var C = speciesConstructor(this, getBuiltIn('Promise')); var isFunction = typeof onFinally == 'function'; return this.then( isFunction ? function (x) { return promiseResolve(C, onFinally()).then(function () { return x; }); } : onFinally, isFunction ? function (e) { return promiseResolve(C, onFinally()).then(function () { throw e; }); } : onFinally ); } }); // patch native Promise.prototype for native async functions if (!IS_PURE && typeof NativePromise == 'function' && !NativePromise.prototype['finally']) { redefine(NativePromise.prototype, 'finally', getBuiltIn('Promise').prototype['finally']); } apollo-server-demo/node_modules/core-js/modules/es.object.lookup-setter.js 0000644 0001750 0000144 00000001616 03560116604 026474 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var DESCRIPTORS = require('../internals/descriptors'); var FORCED = require('../internals/object-prototype-accessors-forced'); var toObject = require('../internals/to-object'); var toPrimitive = require('../internals/to-primitive'); var getPrototypeOf = require('../internals/object-get-prototype-of'); var getOwnPropertyDescriptor = require('../internals/object-get-own-property-descriptor').f; // `Object.prototype.__lookupSetter__` method // https://tc39.es/ecma262/#sec-object.prototype.__lookupSetter__ if (DESCRIPTORS) { $({ target: 'Object', proto: true, forced: FORCED }, { __lookupSetter__: function __lookupSetter__(P) { var O = toObject(this); var key = toPrimitive(P, true); var desc; do { if (desc = getOwnPropertyDescriptor(O, key)) return desc.set; } while (O = getPrototypeOf(O)); } }); } apollo-server-demo/node_modules/core-js/modules/es.typed-array.reverse.js 0000644 0001750 0000144 00000001222 03560116604 026316 0 ustar andreh users 'use strict'; var ArrayBufferViewCore = require('../internals/array-buffer-view-core'); var aTypedArray = ArrayBufferViewCore.aTypedArray; var exportTypedArrayMethod = ArrayBufferViewCore.exportTypedArrayMethod; var floor = Math.floor; // `%TypedArray%.prototype.reverse` method // https://tc39.es/ecma262/#sec-%typedarray%.prototype.reverse exportTypedArrayMethod('reverse', function reverse() { var that = this; var length = aTypedArray(that).length; var middle = floor(length / 2); var index = 0; var value; while (index < middle) { value = that[index]; that[index++] = that[--length]; that[length] = value; } return that; }); apollo-server-demo/node_modules/core-js/modules/esnext.string.replace-all.js 0000644 0001750 0000144 00000000105 03560116604 026767 0 ustar andreh users // TODO: Remove from `core-js@4` require('./es.string.replace-all'); apollo-server-demo/node_modules/core-js/modules/esnext.reflect.get-metadata-keys.js 0000644 0001750 0000144 00000002443 03560116604 030241 0 ustar andreh users var $ = require('../internals/export'); // TODO: in core-js@4, move /modules/ dependencies to public entries for better optimization by tools like `preset-env` var Set = require('../modules/es.set'); var ReflectMetadataModule = require('../internals/reflect-metadata'); var anObject = require('../internals/an-object'); var getPrototypeOf = require('../internals/object-get-prototype-of'); var iterate = require('../internals/iterate'); var ordinaryOwnMetadataKeys = ReflectMetadataModule.keys; var toMetadataKey = ReflectMetadataModule.toKey; var from = function (iter) { var result = []; iterate(iter, result.push, { that: result }); return result; }; var ordinaryMetadataKeys = function (O, P) { var oKeys = ordinaryOwnMetadataKeys(O, P); var parent = getPrototypeOf(O); if (parent === null) return oKeys; var pKeys = ordinaryMetadataKeys(parent, P); return pKeys.length ? oKeys.length ? from(new Set(oKeys.concat(pKeys))) : pKeys : oKeys; }; // `Reflect.getMetadataKeys` method // https://github.com/rbuckton/reflect-metadata $({ target: 'Reflect', stat: true }, { getMetadataKeys: function getMetadataKeys(target /* , targetKey */) { var targetKey = arguments.length < 2 ? undefined : toMetadataKey(arguments[1]); return ordinaryMetadataKeys(anObject(target), targetKey); } }); apollo-server-demo/node_modules/core-js/modules/es.array-buffer.slice.js 0000644 0001750 0000144 00000002733 03560116604 026076 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var fails = require('../internals/fails'); var ArrayBufferModule = require('../internals/array-buffer'); var anObject = require('../internals/an-object'); var toAbsoluteIndex = require('../internals/to-absolute-index'); var toLength = require('../internals/to-length'); var speciesConstructor = require('../internals/species-constructor'); var ArrayBuffer = ArrayBufferModule.ArrayBuffer; var DataView = ArrayBufferModule.DataView; var nativeArrayBufferSlice = ArrayBuffer.prototype.slice; var INCORRECT_SLICE = fails(function () { return !new ArrayBuffer(2).slice(1, undefined).byteLength; }); // `ArrayBuffer.prototype.slice` method // https://tc39.es/ecma262/#sec-arraybuffer.prototype.slice $({ target: 'ArrayBuffer', proto: true, unsafe: true, forced: INCORRECT_SLICE }, { slice: function slice(start, end) { if (nativeArrayBufferSlice !== undefined && end === undefined) { return nativeArrayBufferSlice.call(anObject(this), start); // FF fix } var length = anObject(this).byteLength; var first = toAbsoluteIndex(start, length); var fin = toAbsoluteIndex(end === undefined ? length : end, length); var result = new (speciesConstructor(this, ArrayBuffer))(toLength(fin - first)); var viewSource = new DataView(this); var viewTarget = new DataView(result); var index = 0; while (first < fin) { viewTarget.setUint8(index++, viewSource.getUint8(first++)); } return result; } }); apollo-server-demo/node_modules/core-js/modules/es.math.sign.js 0000644 0001750 0000144 00000000314 03560116604 024274 0 ustar andreh users var $ = require('../internals/export'); var sign = require('../internals/math-sign'); // `Math.sign` method // https://tc39.es/ecma262/#sec-math.sign $({ target: 'Math', stat: true }, { sign: sign }); apollo-server-demo/node_modules/core-js/modules/esnext.map.from.js 0000644 0001750 0000144 00000000345 03560116604 025026 0 ustar andreh users var $ = require('../internals/export'); var from = require('../internals/collection-from'); // `Map.from` method // https://tc39.github.io/proposal-setmap-offrom/#sec-map.from $({ target: 'Map', stat: true }, { from: from }); apollo-server-demo/node_modules/core-js/modules/esnext.async-iterator.from.js 0000644 0001750 0000144 00000002055 03560116604 027215 0 ustar andreh users // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var path = require('../internals/path'); var aFunction = require('../internals/a-function'); var anObject = require('../internals/an-object'); var toObject = require('../internals/to-object'); var createAsyncIteratorProxy = require('../internals/async-iterator-create-proxy'); var getAsyncIteratorMethod = require('../internals/get-async-iterator-method'); var AsyncIterator = path.AsyncIterator; var AsyncIteratorProxy = createAsyncIteratorProxy(function (arg) { return anObject(this.next.call(this.iterator, arg)); }, true); $({ target: 'AsyncIterator', stat: true }, { from: function from(O) { var object = toObject(O); var usingIterator = getAsyncIteratorMethod(object); var iterator; if (usingIterator != null) { iterator = aFunction(usingIterator).call(object); if (iterator instanceof AsyncIterator) return iterator; } else { iterator = object; } return new AsyncIteratorProxy({ iterator: iterator }); } }); apollo-server-demo/node_modules/core-js/modules/es.string.replace-all.js 0000644 0001750 0000144 00000005170 03560116604 026077 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var requireObjectCoercible = require('../internals/require-object-coercible'); var isRegExp = require('../internals/is-regexp'); var getRegExpFlags = require('../internals/regexp-flags'); var getSubstitution = require('../internals/get-substitution'); var wellKnownSymbol = require('../internals/well-known-symbol'); var IS_PURE = require('../internals/is-pure'); var REPLACE = wellKnownSymbol('replace'); var RegExpPrototype = RegExp.prototype; var max = Math.max; var stringIndexOf = function (string, searchValue, fromIndex) { if (fromIndex > string.length) return -1; if (searchValue === '') return fromIndex; return string.indexOf(searchValue, fromIndex); }; // `String.prototype.replaceAll` method // https://tc39.es/ecma262/#sec-string.prototype.replaceall $({ target: 'String', proto: true }, { replaceAll: function replaceAll(searchValue, replaceValue) { var O = requireObjectCoercible(this); var IS_REG_EXP, flags, replacer, string, searchString, functionalReplace, searchLength, advanceBy, replacement; var position = 0; var endOfLastMatch = 0; var result = ''; if (searchValue != null) { IS_REG_EXP = isRegExp(searchValue); if (IS_REG_EXP) { flags = String(requireObjectCoercible('flags' in RegExpPrototype ? searchValue.flags : getRegExpFlags.call(searchValue) )); if (!~flags.indexOf('g')) throw TypeError('`.replaceAll` does not allow non-global regexes'); } replacer = searchValue[REPLACE]; if (replacer !== undefined) { return replacer.call(searchValue, O, replaceValue); } else if (IS_PURE && IS_REG_EXP) { return String(O).replace(searchValue, replaceValue); } } string = String(O); searchString = String(searchValue); functionalReplace = typeof replaceValue === 'function'; if (!functionalReplace) replaceValue = String(replaceValue); searchLength = searchString.length; advanceBy = max(1, searchLength); position = stringIndexOf(string, searchString, 0); while (position !== -1) { if (functionalReplace) { replacement = String(replaceValue(searchString, position, string)); } else { replacement = getSubstitution(searchString, string, position, [], undefined, replaceValue); } result += string.slice(endOfLastMatch, position) + replacement; endOfLastMatch = position + searchLength; position = stringIndexOf(string, searchString, position + advanceBy); } if (endOfLastMatch < string.length) { result += string.slice(endOfLastMatch); } return result; } }); apollo-server-demo/node_modules/core-js/modules/es.math.cosh.js 0000644 0001750 0000144 00000000645 03560116604 024277 0 ustar andreh users var $ = require('../internals/export'); var expm1 = require('../internals/math-expm1'); var nativeCosh = Math.cosh; var abs = Math.abs; var E = Math.E; // `Math.cosh` method // https://tc39.es/ecma262/#sec-math.cosh $({ target: 'Math', stat: true, forced: !nativeCosh || nativeCosh(710) === Infinity }, { cosh: function cosh(x) { var t = expm1(abs(x) - 1) + 1; return (t + 1 / (t * E * E)) * (E / 2); } }); apollo-server-demo/node_modules/core-js/modules/es.typed-array.index-of.js 0000644 0001750 0000144 00000001077 03560116604 026364 0 ustar andreh users 'use strict'; var ArrayBufferViewCore = require('../internals/array-buffer-view-core'); var $indexOf = require('../internals/array-includes').indexOf; var aTypedArray = ArrayBufferViewCore.aTypedArray; var exportTypedArrayMethod = ArrayBufferViewCore.exportTypedArrayMethod; // `%TypedArray%.prototype.indexOf` method // https://tc39.es/ecma262/#sec-%typedarray%.prototype.indexof exportTypedArrayMethod('indexOf', function indexOf(searchElement /* , fromIndex */) { return $indexOf(aTypedArray(this), searchElement, arguments.length > 1 ? arguments[1] : undefined); }); apollo-server-demo/node_modules/core-js/modules/es.symbol.iterator.js 0000644 0001750 0000144 00000000311 03560116604 025536 0 ustar andreh users var defineWellKnownSymbol = require('../internals/define-well-known-symbol'); // `Symbol.iterator` well-known symbol // https://tc39.es/ecma262/#sec-symbol.iterator defineWellKnownSymbol('iterator'); apollo-server-demo/node_modules/core-js/modules/es.typed-array.fill.js 0000644 0001750 0000144 00000001026 03560116604 025573 0 ustar andreh users 'use strict'; var ArrayBufferViewCore = require('../internals/array-buffer-view-core'); var $fill = require('../internals/array-fill'); var aTypedArray = ArrayBufferViewCore.aTypedArray; var exportTypedArrayMethod = ArrayBufferViewCore.exportTypedArrayMethod; // `%TypedArray%.prototype.fill` method // https://tc39.es/ecma262/#sec-%typedarray%.prototype.fill // eslint-disable-next-line no-unused-vars exportTypedArrayMethod('fill', function fill(value /* , start, end */) { return $fill.apply(aTypedArray(this), arguments); }); apollo-server-demo/node_modules/core-js/modules/es.string.italics.js 0000644 0001750 0000144 00000000670 03560116604 025346 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var createHTML = require('../internals/create-html'); var forcedStringHTMLMethod = require('../internals/string-html-forced'); // `String.prototype.italics` method // https://tc39.es/ecma262/#sec-string.prototype.italics $({ target: 'String', proto: true, forced: forcedStringHTMLMethod('italics') }, { italics: function italics() { return createHTML(this, 'i', '', ''); } }); apollo-server-demo/node_modules/core-js/modules/es.number.parse-float.js 0000644 0001750 0000144 00000000442 03560116604 026112 0 ustar andreh users var $ = require('../internals/export'); var parseFloat = require('../internals/number-parse-float'); // `Number.parseFloat` method // https://tc39.es/ecma262/#sec-number.parseFloat $({ target: 'Number', stat: true, forced: Number.parseFloat != parseFloat }, { parseFloat: parseFloat }); apollo-server-demo/node_modules/core-js/modules/es.typed-array.slice.js 0000644 0001750 0000144 00000001734 03560116604 025752 0 ustar andreh users 'use strict'; var ArrayBufferViewCore = require('../internals/array-buffer-view-core'); var speciesConstructor = require('../internals/species-constructor'); var fails = require('../internals/fails'); var aTypedArray = ArrayBufferViewCore.aTypedArray; var aTypedArrayConstructor = ArrayBufferViewCore.aTypedArrayConstructor; var exportTypedArrayMethod = ArrayBufferViewCore.exportTypedArrayMethod; var $slice = [].slice; var FORCED = fails(function () { // eslint-disable-next-line no-undef new Int8Array(1).slice(); }); // `%TypedArray%.prototype.slice` method // https://tc39.es/ecma262/#sec-%typedarray%.prototype.slice exportTypedArrayMethod('slice', function slice(start, end) { var list = $slice.call(aTypedArray(this), start, end); var C = speciesConstructor(this, this.constructor); var index = 0; var length = list.length; var result = new (aTypedArrayConstructor(C))(length); while (length > index) result[index] = list[index++]; return result; }, FORCED); apollo-server-demo/node_modules/core-js/modules/esnext.map.find-key.js 0000644 0001750 0000144 00000001602 03560116604 025566 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var anObject = require('../internals/an-object'); var bind = require('../internals/function-bind-context'); var getMapIterator = require('../internals/get-map-iterator'); var iterate = require('../internals/iterate'); // `Map.prototype.findKey` method // https://github.com/tc39/proposal-collection-methods $({ target: 'Map', proto: true, real: true, forced: IS_PURE }, { findKey: function findKey(callbackfn /* , thisArg */) { var map = anObject(this); var iterator = getMapIterator(map); var boundFunction = bind(callbackfn, arguments.length > 1 ? arguments[1] : undefined, 3); return iterate(iterator, function (key, value, stop) { if (boundFunction(value, key, map)) return stop(key); }, { AS_ENTRIES: true, IS_ITERATOR: true, INTERRUPTED: true }).result; } }); apollo-server-demo/node_modules/core-js/modules/es.object.get-own-property-descriptor.js 0000644 0001750 0000144 00000001403 03560116604 031267 0 ustar andreh users var $ = require('../internals/export'); var fails = require('../internals/fails'); var toIndexedObject = require('../internals/to-indexed-object'); var nativeGetOwnPropertyDescriptor = require('../internals/object-get-own-property-descriptor').f; var DESCRIPTORS = require('../internals/descriptors'); var FAILS_ON_PRIMITIVES = fails(function () { nativeGetOwnPropertyDescriptor(1); }); var FORCED = !DESCRIPTORS || FAILS_ON_PRIMITIVES; // `Object.getOwnPropertyDescriptor` method // https://tc39.es/ecma262/#sec-object.getownpropertydescriptor $({ target: 'Object', stat: true, forced: FORCED, sham: !DESCRIPTORS }, { getOwnPropertyDescriptor: function getOwnPropertyDescriptor(it, key) { return nativeGetOwnPropertyDescriptor(toIndexedObject(it), key); } }); apollo-server-demo/node_modules/core-js/modules/es.array.includes.js 0000644 0001750 0000144 00000001360 03560116604 025331 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var $includes = require('../internals/array-includes').includes; var addToUnscopables = require('../internals/add-to-unscopables'); var arrayMethodUsesToLength = require('../internals/array-method-uses-to-length'); var USES_TO_LENGTH = arrayMethodUsesToLength('indexOf', { ACCESSORS: true, 1: 0 }); // `Array.prototype.includes` method // https://tc39.es/ecma262/#sec-array.prototype.includes $({ target: 'Array', proto: true, forced: !USES_TO_LENGTH }, { includes: function includes(el /* , fromIndex = 0 */) { return $includes(this, el, arguments.length > 1 ? arguments[1] : undefined); } }); // https://tc39.es/ecma262/#sec-array.prototype-@@unscopables addToUnscopables('includes'); apollo-server-demo/node_modules/core-js/modules/es.array.unscopables.flat.js 0000644 0001750 0000144 00000000423 03560116604 026765 0 ustar andreh users // this method was added to unscopables after implementation // in popular engines, so it's moved to a separate module var addToUnscopables = require('../internals/add-to-unscopables'); // https://tc39.es/ecma262/#sec-array.prototype-@@unscopables addToUnscopables('flat'); apollo-server-demo/node_modules/core-js/modules/esnext.weak-map.from.js 0000644 0001750 0000144 00000000361 03560116604 025751 0 ustar andreh users var $ = require('../internals/export'); var from = require('../internals/collection-from'); // `WeakMap.from` method // https://tc39.github.io/proposal-setmap-offrom/#sec-weakmap.from $({ target: 'WeakMap', stat: true }, { from: from }); apollo-server-demo/node_modules/core-js/modules/es.reflect.has.js 0000644 0001750 0000144 00000000351 03560116604 024603 0 ustar andreh users var $ = require('../internals/export'); // `Reflect.has` method // https://tc39.es/ecma262/#sec-reflect.has $({ target: 'Reflect', stat: true }, { has: function has(target, propertyKey) { return propertyKey in target; } }); apollo-server-demo/node_modules/core-js/modules/web.dom-collections.for-each.js 0000644 0001750 0000144 00000001263 03560116604 027334 0 ustar andreh users var global = require('../internals/global'); var DOMIterables = require('../internals/dom-iterables'); var forEach = require('../internals/array-for-each'); var createNonEnumerableProperty = require('../internals/create-non-enumerable-property'); for (var COLLECTION_NAME in DOMIterables) { var Collection = global[COLLECTION_NAME]; var CollectionPrototype = Collection && Collection.prototype; // some Chrome versions have non-configurable methods on DOMTokenList if (CollectionPrototype && CollectionPrototype.forEach !== forEach) try { createNonEnumerableProperty(CollectionPrototype, 'forEach', forEach); } catch (error) { CollectionPrototype.forEach = forEach; } } apollo-server-demo/node_modules/core-js/modules/esnext.math.signbit.js 0000644 0001750 0000144 00000000401 03560116604 025667 0 ustar andreh users var $ = require('../internals/export'); // `Math.signbit` method // https://github.com/tc39/proposal-Math.signbit $({ target: 'Math', stat: true }, { signbit: function signbit(x) { return (x = +x) == x && x == 0 ? 1 / x == -Infinity : x < 0; } }); apollo-server-demo/node_modules/core-js/modules/es.typed-array.iterator.js 0000644 0001750 0000144 00000003213 03560116604 026476 0 ustar andreh users 'use strict'; var global = require('../internals/global'); var ArrayBufferViewCore = require('../internals/array-buffer-view-core'); var ArrayIterators = require('../modules/es.array.iterator'); var wellKnownSymbol = require('../internals/well-known-symbol'); var ITERATOR = wellKnownSymbol('iterator'); var Uint8Array = global.Uint8Array; var arrayValues = ArrayIterators.values; var arrayKeys = ArrayIterators.keys; var arrayEntries = ArrayIterators.entries; var aTypedArray = ArrayBufferViewCore.aTypedArray; var exportTypedArrayMethod = ArrayBufferViewCore.exportTypedArrayMethod; var nativeTypedArrayIterator = Uint8Array && Uint8Array.prototype[ITERATOR]; var CORRECT_ITER_NAME = !!nativeTypedArrayIterator && (nativeTypedArrayIterator.name == 'values' || nativeTypedArrayIterator.name == undefined); var typedArrayValues = function values() { return arrayValues.call(aTypedArray(this)); }; // `%TypedArray%.prototype.entries` method // https://tc39.es/ecma262/#sec-%typedarray%.prototype.entries exportTypedArrayMethod('entries', function entries() { return arrayEntries.call(aTypedArray(this)); }); // `%TypedArray%.prototype.keys` method // https://tc39.es/ecma262/#sec-%typedarray%.prototype.keys exportTypedArrayMethod('keys', function keys() { return arrayKeys.call(aTypedArray(this)); }); // `%TypedArray%.prototype.values` method // https://tc39.es/ecma262/#sec-%typedarray%.prototype.values exportTypedArrayMethod('values', typedArrayValues, !CORRECT_ITER_NAME); // `%TypedArray%.prototype[@@iterator]` method // https://tc39.es/ecma262/#sec-%typedarray%.prototype-@@iterator exportTypedArrayMethod(ITERATOR, typedArrayValues, !CORRECT_ITER_NAME); apollo-server-demo/node_modules/core-js/modules/es.array.iterator.js 0000644 0001750 0000144 00000004151 03560116604 025355 0 ustar andreh users 'use strict'; var toIndexedObject = require('../internals/to-indexed-object'); var addToUnscopables = require('../internals/add-to-unscopables'); var Iterators = require('../internals/iterators'); var InternalStateModule = require('../internals/internal-state'); var defineIterator = require('../internals/define-iterator'); var ARRAY_ITERATOR = 'Array Iterator'; var setInternalState = InternalStateModule.set; var getInternalState = InternalStateModule.getterFor(ARRAY_ITERATOR); // `Array.prototype.entries` method // https://tc39.es/ecma262/#sec-array.prototype.entries // `Array.prototype.keys` method // https://tc39.es/ecma262/#sec-array.prototype.keys // `Array.prototype.values` method // https://tc39.es/ecma262/#sec-array.prototype.values // `Array.prototype[@@iterator]` method // https://tc39.es/ecma262/#sec-array.prototype-@@iterator // `CreateArrayIterator` internal method // https://tc39.es/ecma262/#sec-createarrayiterator module.exports = defineIterator(Array, 'Array', function (iterated, kind) { setInternalState(this, { type: ARRAY_ITERATOR, target: toIndexedObject(iterated), // target index: 0, // next index kind: kind // kind }); // `%ArrayIteratorPrototype%.next` method // https://tc39.es/ecma262/#sec-%arrayiteratorprototype%.next }, function () { var state = getInternalState(this); var target = state.target; var kind = state.kind; var index = state.index++; if (!target || index >= target.length) { state.target = undefined; return { value: undefined, done: true }; } if (kind == 'keys') return { value: index, done: false }; if (kind == 'values') return { value: target[index], done: false }; return { value: [index, target[index]], done: false }; }, 'values'); // argumentsList[@@iterator] is %ArrayProto_values% // https://tc39.es/ecma262/#sec-createunmappedargumentsobject // https://tc39.es/ecma262/#sec-createmappedargumentsobject Iterators.Arguments = Iterators.Array; // https://tc39.es/ecma262/#sec-array.prototype-@@unscopables addToUnscopables('keys'); addToUnscopables('values'); addToUnscopables('entries'); apollo-server-demo/node_modules/core-js/modules/es.math.acosh.js 0000644 0001750 0000144 00000001271 03560116604 024434 0 ustar andreh users var $ = require('../internals/export'); var log1p = require('../internals/math-log1p'); var nativeAcosh = Math.acosh; var log = Math.log; var sqrt = Math.sqrt; var LN2 = Math.LN2; var FORCED = !nativeAcosh // V8 bug: https://code.google.com/p/v8/issues/detail?id=3509 || Math.floor(nativeAcosh(Number.MAX_VALUE)) != 710 // Tor Browser bug: Math.acosh(Infinity) -> NaN || nativeAcosh(Infinity) != Infinity; // `Math.acosh` method // https://tc39.es/ecma262/#sec-math.acosh $({ target: 'Math', stat: true, forced: FORCED }, { acosh: function acosh(x) { return (x = +x) < 1 ? NaN : x > 94906265.62425156 ? log(x) + LN2 : log1p(x - 1 + sqrt(x - 1) * sqrt(x + 1)); } }); apollo-server-demo/node_modules/core-js/modules/es.array.flat.js 0000644 0001750 0000144 00000001453 03560116604 024454 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var flattenIntoArray = require('../internals/flatten-into-array'); var toObject = require('../internals/to-object'); var toLength = require('../internals/to-length'); var toInteger = require('../internals/to-integer'); var arraySpeciesCreate = require('../internals/array-species-create'); // `Array.prototype.flat` method // https://tc39.es/ecma262/#sec-array.prototype.flat $({ target: 'Array', proto: true }, { flat: function flat(/* depthArg = 1 */) { var depthArg = arguments.length ? arguments[0] : undefined; var O = toObject(this); var sourceLen = toLength(O.length); var A = arraySpeciesCreate(O, 0); A.length = flattenIntoArray(A, O, O, sourceLen, 0, depthArg === undefined ? 1 : toInteger(depthArg)); return A; } }); apollo-server-demo/node_modules/core-js/modules/esnext.iterator.take.js 0000644 0001750 0000144 00000001556 03560116604 026070 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var anObject = require('../internals/an-object'); var toPositiveInteger = require('../internals/to-positive-integer'); var createIteratorProxy = require('../internals/iterator-create-proxy'); var iteratorClose = require('../internals/iterator-close'); var IteratorProxy = createIteratorProxy(function (arg) { var iterator = this.iterator; if (!this.remaining--) { this.done = true; return iteratorClose(iterator); } var result = anObject(this.next.call(iterator, arg)); var done = this.done = !!result.done; if (!done) return result.value; }); $({ target: 'Iterator', proto: true, real: true }, { take: function take(limit) { return new IteratorProxy({ iterator: anObject(this), remaining: toPositiveInteger(limit) }); } }); apollo-server-demo/node_modules/core-js/modules/es.array.some.js 0000644 0001750 0000144 00000001256 03560116604 024472 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var $some = require('../internals/array-iteration').some; var arrayMethodIsStrict = require('../internals/array-method-is-strict'); var arrayMethodUsesToLength = require('../internals/array-method-uses-to-length'); var STRICT_METHOD = arrayMethodIsStrict('some'); var USES_TO_LENGTH = arrayMethodUsesToLength('some'); // `Array.prototype.some` method // https://tc39.es/ecma262/#sec-array.prototype.some $({ target: 'Array', proto: true, forced: !STRICT_METHOD || !USES_TO_LENGTH }, { some: function some(callbackfn /* , thisArg */) { return $some(this, callbackfn, arguments.length > 1 ? arguments[1] : undefined); } }); apollo-server-demo/node_modules/core-js/modules/esnext.reflect.get-own-metadata-keys.js 0000644 0001750 0000144 00000001166 03560116604 031043 0 ustar andreh users var $ = require('../internals/export'); var ReflectMetadataModule = require('../internals/reflect-metadata'); var anObject = require('../internals/an-object'); var ordinaryOwnMetadataKeys = ReflectMetadataModule.keys; var toMetadataKey = ReflectMetadataModule.toKey; // `Reflect.getOwnMetadataKeys` method // https://github.com/rbuckton/reflect-metadata $({ target: 'Reflect', stat: true }, { getOwnMetadataKeys: function getOwnMetadataKeys(target /* , targetKey */) { var targetKey = arguments.length < 2 ? undefined : toMetadataKey(arguments[1]); return ordinaryOwnMetadataKeys(anObject(target), targetKey); } }); apollo-server-demo/node_modules/core-js/modules/esnext.set.is-subset-of.js 0000644 0001750 0000144 00000001776 03560116604 026432 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var getBuiltIn = require('../internals/get-built-in'); var anObject = require('../internals/an-object'); var aFunction = require('../internals/a-function'); var getIterator = require('../internals/get-iterator'); var iterate = require('../internals/iterate'); // `Set.prototype.isSubsetOf` method // https://tc39.github.io/proposal-set-methods/#Set.prototype.isSubsetOf $({ target: 'Set', proto: true, real: true, forced: IS_PURE }, { isSubsetOf: function isSubsetOf(iterable) { var iterator = getIterator(this); var otherSet = anObject(iterable); var hasCheck = otherSet.has; if (typeof hasCheck != 'function') { otherSet = new (getBuiltIn('Set'))(iterable); hasCheck = aFunction(otherSet.has); } return !iterate(iterator, function (value, stop) { if (hasCheck.call(otherSet, value) === false) return stop(); }, { IS_ITERATOR: true, INTERRUPTED: true }).stopped; } }); apollo-server-demo/node_modules/core-js/modules/esnext.number.range.js 0000644 0001750 0000144 00000000533 03560116604 025671 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var RangeIterator = require('../internals/range-iterator'); // `Number.range` method // https://github.com/tc39/proposal-Number.range $({ target: 'Number', stat: true }, { range: function range(start, end, option) { return new RangeIterator(start, end, option, 'number', 0, 1); } }); apollo-server-demo/node_modules/core-js/modules/README.md 0000644 0001750 0000144 00000000244 03560116604 022721 0 ustar andreh users This folder contains implementations of polyfills. It's not recommended to include in your projects directly if you don't completely understand what are you doing. apollo-server-demo/node_modules/core-js/modules/es.math.tanh.js 0000644 0001750 0000144 00000000571 03560116604 024273 0 ustar andreh users var $ = require('../internals/export'); var expm1 = require('../internals/math-expm1'); var exp = Math.exp; // `Math.tanh` method // https://tc39.es/ecma262/#sec-math.tanh $({ target: 'Math', stat: true }, { tanh: function tanh(x) { var a = expm1(x = +x); var b = expm1(-x); return a == Infinity ? 1 : b == Infinity ? -1 : (a - b) / (exp(x) + exp(-x)); } }); apollo-server-demo/node_modules/core-js/modules/es.string.pad-end.js 0000644 0001750 0000144 00000000742 03560116604 025226 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var $padEnd = require('../internals/string-pad').end; var WEBKIT_BUG = require('../internals/string-pad-webkit-bug'); // `String.prototype.padEnd` method // https://tc39.es/ecma262/#sec-string.prototype.padend $({ target: 'String', proto: true, forced: WEBKIT_BUG }, { padEnd: function padEnd(maxLength /* , fillString = ' ' */) { return $padEnd(this, maxLength, arguments.length > 1 ? arguments[1] : undefined); } }); apollo-server-demo/node_modules/core-js/modules/esnext.map.update-or-insert.js 0000644 0001750 0000144 00000000630 03560116604 027262 0 ustar andreh users 'use strict'; // TODO: remove from `core-js@4` var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var $upsert = require('../internals/map-upsert'); // `Map.prototype.updateOrInsert` method (replaced by `Map.prototype.emplace`) // https://github.com/thumbsupep/proposal-upsert $({ target: 'Map', proto: true, real: true, forced: IS_PURE }, { updateOrInsert: $upsert }); apollo-server-demo/node_modules/core-js/modules/es.regexp.to-string.js 0000644 0001750 0000144 00000001722 03560116604 025627 0 ustar andreh users 'use strict'; var redefine = require('../internals/redefine'); var anObject = require('../internals/an-object'); var fails = require('../internals/fails'); var flags = require('../internals/regexp-flags'); var TO_STRING = 'toString'; var RegExpPrototype = RegExp.prototype; var nativeToString = RegExpPrototype[TO_STRING]; var NOT_GENERIC = fails(function () { return nativeToString.call({ source: 'a', flags: 'b' }) != '/a/b'; }); // FF44- RegExp#toString has a wrong name var INCORRECT_NAME = nativeToString.name != TO_STRING; // `RegExp.prototype.toString` method // https://tc39.es/ecma262/#sec-regexp.prototype.tostring if (NOT_GENERIC || INCORRECT_NAME) { redefine(RegExp.prototype, TO_STRING, function toString() { var R = anObject(this); var p = String(R.source); var rf = R.flags; var f = String(rf === undefined && R instanceof RegExp && !('flags' in RegExpPrototype) ? flags.call(R) : rf); return '/' + p + '/' + f; }, { unsafe: true }); } apollo-server-demo/node_modules/core-js/modules/es.array.of.js 0000644 0001750 0000144 00000001373 03560116604 024133 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var fails = require('../internals/fails'); var createProperty = require('../internals/create-property'); var ISNT_GENERIC = fails(function () { function F() { /* empty */ } return !(Array.of.call(F) instanceof F); }); // `Array.of` method // https://tc39.es/ecma262/#sec-array.of // WebKit Array.of isn't generic $({ target: 'Array', stat: true, forced: ISNT_GENERIC }, { of: function of(/* ...args */) { var index = 0; var argumentsLength = arguments.length; var result = new (typeof this == 'function' ? this : Array)(argumentsLength); while (argumentsLength > index) createProperty(result, index, arguments[index++]); result.length = argumentsLength; return result; } }); apollo-server-demo/node_modules/core-js/modules/esnext.composite-key.js 0000644 0001750 0000144 00000001104 03560116604 026071 0 ustar andreh users var $ = require('../internals/export'); var getCompositeKeyNode = require('../internals/composite-key'); var getBuiltIn = require('../internals/get-built-in'); var create = require('../internals/object-create'); var initializer = function () { var freeze = getBuiltIn('Object', 'freeze'); return freeze ? freeze(create(null)) : create(null); }; // https://github.com/tc39/proposal-richer-keys/tree/master/compositeKey $({ global: true }, { compositeKey: function compositeKey() { return getCompositeKeyNode.apply(Object, arguments).get('object', initializer); } }); apollo-server-demo/node_modules/core-js/modules/es.typed-array.uint8-clamped-array.js 0000644 0001750 0000144 00000000541 03560116604 030434 0 ustar andreh users var createTypedArrayConstructor = require('../internals/typed-array-constructor'); // `Uint8ClampedArray` constructor // https://tc39.es/ecma262/#sec-typedarray-objects createTypedArrayConstructor('Uint8', function (init) { return function Uint8ClampedArray(data, byteOffset, length) { return init(this, data, byteOffset, length); }; }, true); apollo-server-demo/node_modules/core-js/modules/es.reflect.prevent-extensions.js 0000644 0001750 0000144 00000001223 03560116604 027707 0 ustar andreh users var $ = require('../internals/export'); var getBuiltIn = require('../internals/get-built-in'); var anObject = require('../internals/an-object'); var FREEZING = require('../internals/freezing'); // `Reflect.preventExtensions` method // https://tc39.es/ecma262/#sec-reflect.preventextensions $({ target: 'Reflect', stat: true, sham: !FREEZING }, { preventExtensions: function preventExtensions(target) { anObject(target); try { var objectPreventExtensions = getBuiltIn('Object', 'preventExtensions'); if (objectPreventExtensions) objectPreventExtensions(target); return true; } catch (error) { return false; } } }); apollo-server-demo/node_modules/core-js/modules/esnext.array.filter-out.js 0000644 0001750 0000144 00000000776 03560116604 026526 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var $filterOut = require('../internals/array-iteration').filterOut; var addToUnscopables = require('../internals/add-to-unscopables'); // `Array.prototype.filterOut` method // https://github.com/tc39/proposal-array-filtering $({ target: 'Array', proto: true }, { filterOut: function filterOut(callbackfn /* , thisArg */) { return $filterOut(this, callbackfn, arguments.length > 1 ? arguments[1] : undefined); } }); addToUnscopables('filterOut'); apollo-server-demo/node_modules/core-js/modules/esnext.map.find.js 0000644 0001750 0000144 00000001573 03560116604 025007 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var anObject = require('../internals/an-object'); var bind = require('../internals/function-bind-context'); var getMapIterator = require('../internals/get-map-iterator'); var iterate = require('../internals/iterate'); // `Map.prototype.find` method // https://github.com/tc39/proposal-collection-methods $({ target: 'Map', proto: true, real: true, forced: IS_PURE }, { find: function find(callbackfn /* , thisArg */) { var map = anObject(this); var iterator = getMapIterator(map); var boundFunction = bind(callbackfn, arguments.length > 1 ? arguments[1] : undefined, 3); return iterate(iterator, function (key, value, stop) { if (boundFunction(value, key, map)) return stop(value); }, { AS_ENTRIES: true, IS_ITERATOR: true, INTERRUPTED: true }).result; } }); apollo-server-demo/node_modules/core-js/modules/es.reflect.to-string-tag.js 0000644 0001750 0000144 00000000507 03560116604 026532 0 ustar andreh users var $ = require('../internals/export'); var global = require('../internals/global'); var setToStringTag = require('../internals/set-to-string-tag'); $({ global: true }, { Reflect: {} }); // Reflect[@@toStringTag] property // https://tc39.es/ecma262/#sec-reflect-@@tostringtag setToStringTag(global.Reflect, 'Reflect', true); apollo-server-demo/node_modules/core-js/modules/es.date.to-iso-string.js 0000644 0001750 0000144 00000000570 03560116604 026042 0 ustar andreh users var $ = require('../internals/export'); var toISOString = require('../internals/date-to-iso-string'); // `Date.prototype.toISOString` method // https://tc39.es/ecma262/#sec-date.prototype.toisostring // PhantomJS / old WebKit has a broken implementations $({ target: 'Date', proto: true, forced: Date.prototype.toISOString !== toISOString }, { toISOString: toISOString }); apollo-server-demo/node_modules/core-js/modules/esnext.set.is-superset-of.js 0000644 0001750 0000144 00000001311 03560116604 026760 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var anObject = require('../internals/an-object'); var aFunction = require('../internals/a-function'); var iterate = require('../internals/iterate'); // `Set.prototype.isSupersetOf` method // https://tc39.github.io/proposal-set-methods/#Set.prototype.isSupersetOf $({ target: 'Set', proto: true, real: true, forced: IS_PURE }, { isSupersetOf: function isSupersetOf(iterable) { var set = anObject(this); var hasCheck = aFunction(set.has); return !iterate(iterable, function (value, stop) { if (hasCheck.call(set, value) === false) return stop(); }, { INTERRUPTED: true }).stopped; } }); apollo-server-demo/node_modules/core-js/modules/es.string.search.js 0000644 0001750 0000144 00000002617 03560116604 025166 0 ustar andreh users 'use strict'; var fixRegExpWellKnownSymbolLogic = require('../internals/fix-regexp-well-known-symbol-logic'); var anObject = require('../internals/an-object'); var requireObjectCoercible = require('../internals/require-object-coercible'); var sameValue = require('../internals/same-value'); var regExpExec = require('../internals/regexp-exec-abstract'); // @@search logic fixRegExpWellKnownSymbolLogic('search', 1, function (SEARCH, nativeSearch, maybeCallNative) { return [ // `String.prototype.search` method // https://tc39.es/ecma262/#sec-string.prototype.search function search(regexp) { var O = requireObjectCoercible(this); var searcher = regexp == undefined ? undefined : regexp[SEARCH]; return searcher !== undefined ? searcher.call(regexp, O) : new RegExp(regexp)[SEARCH](String(O)); }, // `RegExp.prototype[@@search]` method // https://tc39.es/ecma262/#sec-regexp.prototype-@@search function (regexp) { var res = maybeCallNative(nativeSearch, regexp, this); if (res.done) return res.value; var rx = anObject(regexp); var S = String(this); var previousLastIndex = rx.lastIndex; if (!sameValue(previousLastIndex, 0)) rx.lastIndex = 0; var result = regExpExec(rx, S); if (!sameValue(rx.lastIndex, previousLastIndex)) rx.lastIndex = previousLastIndex; return result === null ? -1 : result.index; } ]; }); apollo-server-demo/node_modules/core-js/modules/esnext.promise.try.js 0000644 0001750 0000144 00000001064 03560116604 025601 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var newPromiseCapabilityModule = require('../internals/new-promise-capability'); var perform = require('../internals/perform'); // `Promise.try` method // https://github.com/tc39/proposal-promise-try $({ target: 'Promise', stat: true }, { 'try': function (callbackfn) { var promiseCapability = newPromiseCapabilityModule.f(this); var result = perform(callbackfn); (result.error ? promiseCapability.reject : promiseCapability.resolve)(result.value); return promiseCapability.promise; } }); apollo-server-demo/node_modules/core-js/modules/es.string.blink.js 0000644 0001750 0000144 00000000662 03560116604 025016 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var createHTML = require('../internals/create-html'); var forcedStringHTMLMethod = require('../internals/string-html-forced'); // `String.prototype.blink` method // https://tc39.es/ecma262/#sec-string.prototype.blink $({ target: 'String', proto: true, forced: forcedStringHTMLMethod('blink') }, { blink: function blink() { return createHTML(this, 'blink', '', ''); } }); apollo-server-demo/node_modules/core-js/modules/es.array.from.js 0000644 0001750 0000144 00000000661 03560116604 024471 0 ustar andreh users var $ = require('../internals/export'); var from = require('../internals/array-from'); var checkCorrectnessOfIteration = require('../internals/check-correctness-of-iteration'); var INCORRECT_ITERATION = !checkCorrectnessOfIteration(function (iterable) { Array.from(iterable); }); // `Array.from` method // https://tc39.es/ecma262/#sec-array.from $({ target: 'Array', stat: true, forced: INCORRECT_ITERATION }, { from: from }); apollo-server-demo/node_modules/core-js/modules/esnext.map.merge.js 0000644 0001750 0000144 00000001321 03560116604 025155 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var anObject = require('../internals/an-object'); var aFunction = require('../internals/a-function'); var iterate = require('../internals/iterate'); // `Map.prototype.merge` method // https://github.com/tc39/proposal-collection-methods $({ target: 'Map', proto: true, real: true, forced: IS_PURE }, { // eslint-disable-next-line no-unused-vars merge: function merge(iterable /* ...iterbles */) { var map = anObject(this); var setter = aFunction(map.set); var i = 0; while (i < arguments.length) { iterate(arguments[i++], setter, { that: map, AS_ENTRIES: true }); } return map; } }); apollo-server-demo/node_modules/core-js/modules/es.regexp.sticky.js 0000644 0001750 0000144 00000001573 03560116604 025213 0 ustar andreh users var DESCRIPTORS = require('../internals/descriptors'); var UNSUPPORTED_Y = require('../internals/regexp-sticky-helpers').UNSUPPORTED_Y; var defineProperty = require('../internals/object-define-property').f; var getInternalState = require('../internals/internal-state').get; var RegExpPrototype = RegExp.prototype; // `RegExp.prototype.sticky` getter // https://tc39.es/ecma262/#sec-get-regexp.prototype.sticky if (DESCRIPTORS && UNSUPPORTED_Y) { defineProperty(RegExp.prototype, 'sticky', { configurable: true, get: function () { if (this === RegExpPrototype) return undefined; // We can't use InternalStateModule.getterFor because // we don't add metadata for regexps created by a literal. if (this instanceof RegExp) { return !!getInternalState(this).sticky; } throw TypeError('Incompatible receiver, RegExp required'); } }); } apollo-server-demo/node_modules/core-js/modules/esnext.set.add-all.js 0000644 0001750 0000144 00000000655 03560116604 025403 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var collectionAddAll = require('../internals/collection-add-all'); // `Set.prototype.addAll` method // https://github.com/tc39/proposal-collection-methods $({ target: 'Set', proto: true, real: true, forced: IS_PURE }, { addAll: function addAll(/* ...elements */) { return collectionAddAll.apply(this, arguments); } }); apollo-server-demo/node_modules/core-js/modules/web.dom-collections.iterator.js 0000644 0001750 0000144 00000003035 03560116604 027500 0 ustar andreh users var global = require('../internals/global'); var DOMIterables = require('../internals/dom-iterables'); var ArrayIteratorMethods = require('../modules/es.array.iterator'); var createNonEnumerableProperty = require('../internals/create-non-enumerable-property'); var wellKnownSymbol = require('../internals/well-known-symbol'); var ITERATOR = wellKnownSymbol('iterator'); var TO_STRING_TAG = wellKnownSymbol('toStringTag'); var ArrayValues = ArrayIteratorMethods.values; for (var COLLECTION_NAME in DOMIterables) { var Collection = global[COLLECTION_NAME]; var CollectionPrototype = Collection && Collection.prototype; if (CollectionPrototype) { // some Chrome versions have non-configurable methods on DOMTokenList if (CollectionPrototype[ITERATOR] !== ArrayValues) try { createNonEnumerableProperty(CollectionPrototype, ITERATOR, ArrayValues); } catch (error) { CollectionPrototype[ITERATOR] = ArrayValues; } if (!CollectionPrototype[TO_STRING_TAG]) { createNonEnumerableProperty(CollectionPrototype, TO_STRING_TAG, COLLECTION_NAME); } if (DOMIterables[COLLECTION_NAME]) for (var METHOD_NAME in ArrayIteratorMethods) { // some Chrome versions have non-configurable methods on DOMTokenList if (CollectionPrototype[METHOD_NAME] !== ArrayIteratorMethods[METHOD_NAME]) try { createNonEnumerableProperty(CollectionPrototype, METHOD_NAME, ArrayIteratorMethods[METHOD_NAME]); } catch (error) { CollectionPrototype[METHOD_NAME] = ArrayIteratorMethods[METHOD_NAME]; } } } } apollo-server-demo/node_modules/core-js/modules/esnext.weak-set.delete-all.js 0000644 0001750 0000144 00000000707 03560116604 027040 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var collectionDeleteAll = require('../internals/collection-delete-all'); // `WeakSet.prototype.deleteAll` method // https://github.com/tc39/proposal-collection-methods $({ target: 'WeakSet', proto: true, real: true, forced: IS_PURE }, { deleteAll: function deleteAll(/* ...elements */) { return collectionDeleteAll.apply(this, arguments); } }); apollo-server-demo/node_modules/core-js/modules/esnext.async-iterator.some.js 0000644 0001750 0000144 00000000451 03560116604 027213 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var $some = require('../internals/async-iterator-iteration').some; $({ target: 'AsyncIterator', proto: true, real: true }, { some: function some(fn) { return $some(this, fn); } }); apollo-server-demo/node_modules/core-js/modules/esnext.promise.all-settled.js 0000644 0001750 0000144 00000000111 03560116604 027165 0 ustar andreh users // TODO: Remove from `core-js@4` require('./es.promise.all-settled.js'); apollo-server-demo/node_modules/core-js/modules/esnext.map.reduce.js 0000644 0001750 0000144 00000002115 03560116604 025327 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var anObject = require('../internals/an-object'); var aFunction = require('../internals/a-function'); var getMapIterator = require('../internals/get-map-iterator'); var iterate = require('../internals/iterate'); // `Map.prototype.reduce` method // https://github.com/tc39/proposal-collection-methods $({ target: 'Map', proto: true, real: true, forced: IS_PURE }, { reduce: function reduce(callbackfn /* , initialValue */) { var map = anObject(this); var iterator = getMapIterator(map); var noInitial = arguments.length < 2; var accumulator = noInitial ? undefined : arguments[1]; aFunction(callbackfn); iterate(iterator, function (key, value) { if (noInitial) { noInitial = false; accumulator = value; } else { accumulator = callbackfn(accumulator, value, key, map); } }, { AS_ENTRIES: true, IS_ITERATOR: true }); if (noInitial) throw TypeError('Reduce of empty map with no initial value'); return accumulator; } }); apollo-server-demo/node_modules/core-js/modules/es.typed-array.reduce-right.js 0000644 0001750 0000144 00000001142 03560116604 027226 0 ustar andreh users 'use strict'; var ArrayBufferViewCore = require('../internals/array-buffer-view-core'); var $reduceRight = require('../internals/array-reduce').right; var aTypedArray = ArrayBufferViewCore.aTypedArray; var exportTypedArrayMethod = ArrayBufferViewCore.exportTypedArrayMethod; // `%TypedArray%.prototype.reduceRicht` method // https://tc39.es/ecma262/#sec-%typedarray%.prototype.reduceright exportTypedArrayMethod('reduceRight', function reduceRight(callbackfn /* , initialValue */) { return $reduceRight(aTypedArray(this), callbackfn, arguments.length, arguments.length > 1 ? arguments[1] : undefined); }); apollo-server-demo/node_modules/core-js/modules/web.timers.js 0000644 0001750 0000144 00000002166 03560116604 024064 0 ustar andreh users var $ = require('../internals/export'); var global = require('../internals/global'); var userAgent = require('../internals/engine-user-agent'); var slice = [].slice; var MSIE = /MSIE .\./.test(userAgent); // <- dirty ie9- check var wrap = function (scheduler) { return function (handler, timeout /* , ...arguments */) { var boundArgs = arguments.length > 2; var args = boundArgs ? slice.call(arguments, 2) : undefined; return scheduler(boundArgs ? function () { // eslint-disable-next-line no-new-func (typeof handler == 'function' ? handler : Function(handler)).apply(this, args); } : handler, timeout); }; }; // ie9- setTimeout & setInterval additional parameters fix // https://html.spec.whatwg.org/multipage/timers-and-user-prompts.html#timers $({ global: true, bind: true, forced: MSIE }, { // `setTimeout` method // https://html.spec.whatwg.org/multipage/timers-and-user-prompts.html#dom-settimeout setTimeout: wrap(global.setTimeout), // `setInterval` method // https://html.spec.whatwg.org/multipage/timers-and-user-prompts.html#dom-setinterval setInterval: wrap(global.setInterval) }); apollo-server-demo/node_modules/core-js/modules/esnext.iterator.as-indexed-pairs.js 0000644 0001750 0000144 00000001175 03560116604 030276 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var anObject = require('../internals/an-object'); var createIteratorProxy = require('../internals/iterator-create-proxy'); var IteratorProxy = createIteratorProxy(function (arg) { var result = anObject(this.next.call(this.iterator, arg)); var done = this.done = !!result.done; if (!done) return [this.index++, result.value]; }); $({ target: 'Iterator', proto: true, real: true }, { asIndexedPairs: function asIndexedPairs() { return new IteratorProxy({ iterator: anObject(this), index: 0 }); } }); apollo-server-demo/node_modules/core-js/modules/es.string.sub.js 0000644 0001750 0000144 00000000646 03560116604 024512 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var createHTML = require('../internals/create-html'); var forcedStringHTMLMethod = require('../internals/string-html-forced'); // `String.prototype.sub` method // https://tc39.es/ecma262/#sec-string.prototype.sub $({ target: 'String', proto: true, forced: forcedStringHTMLMethod('sub') }, { sub: function sub() { return createHTML(this, 'sub', '', ''); } }); apollo-server-demo/node_modules/core-js/modules/es.string.pad-start.js 0000644 0001750 0000144 00000000760 03560116604 025615 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var $padStart = require('../internals/string-pad').start; var WEBKIT_BUG = require('../internals/string-pad-webkit-bug'); // `String.prototype.padStart` method // https://tc39.es/ecma262/#sec-string.prototype.padstart $({ target: 'String', proto: true, forced: WEBKIT_BUG }, { padStart: function padStart(maxLength /* , fillString = ' ' */) { return $padStart(this, maxLength, arguments.length > 1 ? arguments[1] : undefined); } }); apollo-server-demo/node_modules/core-js/modules/esnext.bigint.range.js 0000644 0001750 0000144 00000000705 03560116604 025656 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var RangeIterator = require('../internals/range-iterator'); // `BigInt.range` method // https://github.com/tc39/proposal-Number.range if (typeof BigInt == 'function') { $({ target: 'BigInt', stat: true }, { range: function range(start, end, option) { // eslint-disable-next-line no-undef return new RangeIterator(start, end, option, 'bigint', BigInt(0), BigInt(1)); } }); } apollo-server-demo/node_modules/core-js/modules/esnext.weak-map.delete-all.js 0000644 0001750 0000144 00000000707 03560116604 027022 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var collectionDeleteAll = require('../internals/collection-delete-all'); // `WeakMap.prototype.deleteAll` method // https://github.com/tc39/proposal-collection-methods $({ target: 'WeakMap', proto: true, real: true, forced: IS_PURE }, { deleteAll: function deleteAll(/* ...elements */) { return collectionDeleteAll.apply(this, arguments); } }); apollo-server-demo/node_modules/core-js/modules/es.regexp.flags.js 0000644 0001750 0000144 00000001034 03560116604 024771 0 ustar andreh users var DESCRIPTORS = require('../internals/descriptors'); var objectDefinePropertyModule = require('../internals/object-define-property'); var regExpFlags = require('../internals/regexp-flags'); var UNSUPPORTED_Y = require('../internals/regexp-sticky-helpers').UNSUPPORTED_Y; // `RegExp.prototype.flags` getter // https://tc39.es/ecma262/#sec-get-regexp.prototype.flags if (DESCRIPTORS && (/./g.flags != 'g' || UNSUPPORTED_Y)) { objectDefinePropertyModule.f(RegExp.prototype, 'flags', { configurable: true, get: regExpFlags }); } apollo-server-demo/node_modules/core-js/modules/es.typed-array.reduce.js 0000644 0001750 0000144 00000001103 03560116604 026110 0 ustar andreh users 'use strict'; var ArrayBufferViewCore = require('../internals/array-buffer-view-core'); var $reduce = require('../internals/array-reduce').left; var aTypedArray = ArrayBufferViewCore.aTypedArray; var exportTypedArrayMethod = ArrayBufferViewCore.exportTypedArrayMethod; // `%TypedArray%.prototype.reduce` method // https://tc39.es/ecma262/#sec-%typedarray%.prototype.reduce exportTypedArrayMethod('reduce', function reduce(callbackfn /* , initialValue */) { return $reduce(aTypedArray(this), callbackfn, arguments.length, arguments.length > 1 ? arguments[1] : undefined); }); apollo-server-demo/node_modules/core-js/modules/es.string.trim.js 0000644 0001750 0000144 00000000627 03560116604 024673 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var $trim = require('../internals/string-trim').trim; var forcedStringTrimMethod = require('../internals/string-trim-forced'); // `String.prototype.trim` method // https://tc39.es/ecma262/#sec-string.prototype.trim $({ target: 'String', proto: true, forced: forcedStringTrimMethod('trim') }, { trim: function trim() { return $trim(this); } }); apollo-server-demo/node_modules/core-js/modules/es.string.replace.js 0000644 0001750 0000144 00000007725 03560116604 025341 0 ustar andreh users 'use strict'; var fixRegExpWellKnownSymbolLogic = require('../internals/fix-regexp-well-known-symbol-logic'); var anObject = require('../internals/an-object'); var toLength = require('../internals/to-length'); var toInteger = require('../internals/to-integer'); var requireObjectCoercible = require('../internals/require-object-coercible'); var advanceStringIndex = require('../internals/advance-string-index'); var getSubstitution = require('../internals/get-substitution'); var regExpExec = require('../internals/regexp-exec-abstract'); var max = Math.max; var min = Math.min; var maybeToString = function (it) { return it === undefined ? it : String(it); }; // @@replace logic fixRegExpWellKnownSymbolLogic('replace', 2, function (REPLACE, nativeReplace, maybeCallNative, reason) { var REGEXP_REPLACE_SUBSTITUTES_UNDEFINED_CAPTURE = reason.REGEXP_REPLACE_SUBSTITUTES_UNDEFINED_CAPTURE; var REPLACE_KEEPS_$0 = reason.REPLACE_KEEPS_$0; var UNSAFE_SUBSTITUTE = REGEXP_REPLACE_SUBSTITUTES_UNDEFINED_CAPTURE ? '$' : '$0'; return [ // `String.prototype.replace` method // https://tc39.es/ecma262/#sec-string.prototype.replace function replace(searchValue, replaceValue) { var O = requireObjectCoercible(this); var replacer = searchValue == undefined ? undefined : searchValue[REPLACE]; return replacer !== undefined ? replacer.call(searchValue, O, replaceValue) : nativeReplace.call(String(O), searchValue, replaceValue); }, // `RegExp.prototype[@@replace]` method // https://tc39.es/ecma262/#sec-regexp.prototype-@@replace function (regexp, replaceValue) { if ( (!REGEXP_REPLACE_SUBSTITUTES_UNDEFINED_CAPTURE && REPLACE_KEEPS_$0) || (typeof replaceValue === 'string' && replaceValue.indexOf(UNSAFE_SUBSTITUTE) === -1) ) { var res = maybeCallNative(nativeReplace, regexp, this, replaceValue); if (res.done) return res.value; } var rx = anObject(regexp); var S = String(this); var functionalReplace = typeof replaceValue === 'function'; if (!functionalReplace) replaceValue = String(replaceValue); var global = rx.global; if (global) { var fullUnicode = rx.unicode; rx.lastIndex = 0; } var results = []; while (true) { var result = regExpExec(rx, S); if (result === null) break; results.push(result); if (!global) break; var matchStr = String(result[0]); if (matchStr === '') rx.lastIndex = advanceStringIndex(S, toLength(rx.lastIndex), fullUnicode); } var accumulatedResult = ''; var nextSourcePosition = 0; for (var i = 0; i < results.length; i++) { result = results[i]; var matched = String(result[0]); var position = max(min(toInteger(result.index), S.length), 0); var captures = []; // NOTE: This is equivalent to // captures = result.slice(1).map(maybeToString) // but for some reason `nativeSlice.call(result, 1, result.length)` (called in // the slice polyfill when slicing native arrays) "doesn't work" in safari 9 and // causes a crash (https://pastebin.com/N21QzeQA) when trying to debug it. for (var j = 1; j < result.length; j++) captures.push(maybeToString(result[j])); var namedCaptures = result.groups; if (functionalReplace) { var replacerArgs = [matched].concat(captures, position, S); if (namedCaptures !== undefined) replacerArgs.push(namedCaptures); var replacement = String(replaceValue.apply(undefined, replacerArgs)); } else { replacement = getSubstitution(matched, S, position, captures, namedCaptures, replaceValue); } if (position >= nextSourcePosition) { accumulatedResult += S.slice(nextSourcePosition, position) + replacement; nextSourcePosition = position + matched.length; } } return accumulatedResult + S.slice(nextSourcePosition); } ]; }); apollo-server-demo/node_modules/core-js/modules/es.string.trim-end.js 0000644 0001750 0000144 00000001110 03560116604 025423 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var $trimEnd = require('../internals/string-trim').end; var forcedStringTrimMethod = require('../internals/string-trim-forced'); var FORCED = forcedStringTrimMethod('trimEnd'); var trimEnd = FORCED ? function trimEnd() { return $trimEnd(this); } : ''.trimEnd; // `String.prototype.{ trimEnd, trimRight }` methods // https://tc39.es/ecma262/#sec-string.prototype.trimend // https://tc39.es/ecma262/#String.prototype.trimright $({ target: 'String', proto: true, forced: FORCED }, { trimEnd: trimEnd, trimRight: trimEnd }); apollo-server-demo/node_modules/core-js/modules/es.typed-array.join.js 0000644 0001750 0000144 00000000754 03560116604 025613 0 ustar andreh users 'use strict'; var ArrayBufferViewCore = require('../internals/array-buffer-view-core'); var aTypedArray = ArrayBufferViewCore.aTypedArray; var exportTypedArrayMethod = ArrayBufferViewCore.exportTypedArrayMethod; var $join = [].join; // `%TypedArray%.prototype.join` method // https://tc39.es/ecma262/#sec-%typedarray%.prototype.join // eslint-disable-next-line no-unused-vars exportTypedArrayMethod('join', function join(separator) { return $join.apply(aTypedArray(this), arguments); }); apollo-server-demo/node_modules/core-js/modules/esnext.map.includes.js 0000644 0001750 0000144 00000001360 03560116604 025667 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var anObject = require('../internals/an-object'); var getMapIterator = require('../internals/get-map-iterator'); var sameValueZero = require('../internals/same-value-zero'); var iterate = require('../internals/iterate'); // `Map.prototype.includes` method // https://github.com/tc39/proposal-collection-methods $({ target: 'Map', proto: true, real: true, forced: IS_PURE }, { includes: function includes(searchElement) { return iterate(getMapIterator(anObject(this)), function (key, value, stop) { if (sameValueZero(value, searchElement)) return stop(); }, { AS_ENTRIES: true, IS_ITERATOR: true, INTERRUPTED: true }).stopped; } }); apollo-server-demo/node_modules/core-js/modules/es.typed-array.filter.js 0000644 0001750 0000144 00000001650 03560116604 026135 0 ustar andreh users 'use strict'; var ArrayBufferViewCore = require('../internals/array-buffer-view-core'); var $filter = require('../internals/array-iteration').filter; var speciesConstructor = require('../internals/species-constructor'); var aTypedArray = ArrayBufferViewCore.aTypedArray; var aTypedArrayConstructor = ArrayBufferViewCore.aTypedArrayConstructor; var exportTypedArrayMethod = ArrayBufferViewCore.exportTypedArrayMethod; // `%TypedArray%.prototype.filter` method // https://tc39.es/ecma262/#sec-%typedarray%.prototype.filter exportTypedArrayMethod('filter', function filter(callbackfn /* , thisArg */) { var list = $filter(aTypedArray(this), callbackfn, arguments.length > 1 ? arguments[1] : undefined); var C = speciesConstructor(this, this.constructor); var index = 0; var length = list.length; var result = new (aTypedArrayConstructor(C))(length); while (length > index) result[index] = list[index++]; return result; }); apollo-server-demo/node_modules/core-js/modules/es.string.split.js 0000644 0001750 0000144 00000012511 03560116604 025046 0 ustar andreh users 'use strict'; var fixRegExpWellKnownSymbolLogic = require('../internals/fix-regexp-well-known-symbol-logic'); var isRegExp = require('../internals/is-regexp'); var anObject = require('../internals/an-object'); var requireObjectCoercible = require('../internals/require-object-coercible'); var speciesConstructor = require('../internals/species-constructor'); var advanceStringIndex = require('../internals/advance-string-index'); var toLength = require('../internals/to-length'); var callRegExpExec = require('../internals/regexp-exec-abstract'); var regexpExec = require('../internals/regexp-exec'); var fails = require('../internals/fails'); var arrayPush = [].push; var min = Math.min; var MAX_UINT32 = 0xFFFFFFFF; // babel-minify transpiles RegExp('x', 'y') -> /x/y and it causes SyntaxError var SUPPORTS_Y = !fails(function () { return !RegExp(MAX_UINT32, 'y'); }); // @@split logic fixRegExpWellKnownSymbolLogic('split', 2, function (SPLIT, nativeSplit, maybeCallNative) { var internalSplit; if ( 'abbc'.split(/(b)*/)[1] == 'c' || 'test'.split(/(?:)/, -1).length != 4 || 'ab'.split(/(?:ab)*/).length != 2 || '.'.split(/(.?)(.?)/).length != 4 || '.'.split(/()()/).length > 1 || ''.split(/.?/).length ) { // based on es5-shim implementation, need to rework it internalSplit = function (separator, limit) { var string = String(requireObjectCoercible(this)); var lim = limit === undefined ? MAX_UINT32 : limit >>> 0; if (lim === 0) return []; if (separator === undefined) return [string]; // If `separator` is not a regex, use native split if (!isRegExp(separator)) { return nativeSplit.call(string, separator, lim); } var output = []; var flags = (separator.ignoreCase ? 'i' : '') + (separator.multiline ? 'm' : '') + (separator.unicode ? 'u' : '') + (separator.sticky ? 'y' : ''); var lastLastIndex = 0; // Make `global` and avoid `lastIndex` issues by working with a copy var separatorCopy = new RegExp(separator.source, flags + 'g'); var match, lastIndex, lastLength; while (match = regexpExec.call(separatorCopy, string)) { lastIndex = separatorCopy.lastIndex; if (lastIndex > lastLastIndex) { output.push(string.slice(lastLastIndex, match.index)); if (match.length > 1 && match.index < string.length) arrayPush.apply(output, match.slice(1)); lastLength = match[0].length; lastLastIndex = lastIndex; if (output.length >= lim) break; } if (separatorCopy.lastIndex === match.index) separatorCopy.lastIndex++; // Avoid an infinite loop } if (lastLastIndex === string.length) { if (lastLength || !separatorCopy.test('')) output.push(''); } else output.push(string.slice(lastLastIndex)); return output.length > lim ? output.slice(0, lim) : output; }; // Chakra, V8 } else if ('0'.split(undefined, 0).length) { internalSplit = function (separator, limit) { return separator === undefined && limit === 0 ? [] : nativeSplit.call(this, separator, limit); }; } else internalSplit = nativeSplit; return [ // `String.prototype.split` method // https://tc39.es/ecma262/#sec-string.prototype.split function split(separator, limit) { var O = requireObjectCoercible(this); var splitter = separator == undefined ? undefined : separator[SPLIT]; return splitter !== undefined ? splitter.call(separator, O, limit) : internalSplit.call(String(O), separator, limit); }, // `RegExp.prototype[@@split]` method // https://tc39.es/ecma262/#sec-regexp.prototype-@@split // // NOTE: This cannot be properly polyfilled in engines that don't support // the 'y' flag. function (regexp, limit) { var res = maybeCallNative(internalSplit, regexp, this, limit, internalSplit !== nativeSplit); if (res.done) return res.value; var rx = anObject(regexp); var S = String(this); var C = speciesConstructor(rx, RegExp); var unicodeMatching = rx.unicode; var flags = (rx.ignoreCase ? 'i' : '') + (rx.multiline ? 'm' : '') + (rx.unicode ? 'u' : '') + (SUPPORTS_Y ? 'y' : 'g'); // ^(? + rx + ) is needed, in combination with some S slicing, to // simulate the 'y' flag. var splitter = new C(SUPPORTS_Y ? rx : '^(?:' + rx.source + ')', flags); var lim = limit === undefined ? MAX_UINT32 : limit >>> 0; if (lim === 0) return []; if (S.length === 0) return callRegExpExec(splitter, S) === null ? [S] : []; var p = 0; var q = 0; var A = []; while (q < S.length) { splitter.lastIndex = SUPPORTS_Y ? q : 0; var z = callRegExpExec(splitter, SUPPORTS_Y ? S : S.slice(q)); var e; if ( z === null || (e = min(toLength(splitter.lastIndex + (SUPPORTS_Y ? 0 : q)), S.length)) === p ) { q = advanceStringIndex(S, q, unicodeMatching); } else { A.push(S.slice(p, q)); if (A.length === lim) return A; for (var i = 1; i <= z.length - 1; i++) { A.push(z[i]); if (A.length === lim) return A; } q = p = e; } } A.push(S.slice(p)); return A; } ]; }, !SUPPORTS_Y); apollo-server-demo/node_modules/core-js/modules/esnext.aggregate-error.js 0000644 0001750 0000144 00000000102 03560116604 026353 0 ustar andreh users // TODO: Remove from `core-js@4` require('./es.aggregate-error'); apollo-server-demo/node_modules/core-js/modules/esnext.reflect.metadata.js 0000644 0001750 0000144 00000001135 03560116604 026510 0 ustar andreh users var $ = require('../internals/export'); var ReflectMetadataModule = require('../internals/reflect-metadata'); var anObject = require('../internals/an-object'); var toMetadataKey = ReflectMetadataModule.toKey; var ordinaryDefineOwnMetadata = ReflectMetadataModule.set; // `Reflect.metadata` method // https://github.com/rbuckton/reflect-metadata $({ target: 'Reflect', stat: true }, { metadata: function metadata(metadataKey, metadataValue) { return function decorator(target, key) { ordinaryDefineOwnMetadata(metadataKey, metadataValue, anObject(target), toMetadataKey(key)); }; } }); apollo-server-demo/node_modules/core-js/modules/es.typed-array.map.js 0000644 0001750 0000144 00000001424 03560116604 025424 0 ustar andreh users 'use strict'; var ArrayBufferViewCore = require('../internals/array-buffer-view-core'); var $map = require('../internals/array-iteration').map; var speciesConstructor = require('../internals/species-constructor'); var aTypedArray = ArrayBufferViewCore.aTypedArray; var aTypedArrayConstructor = ArrayBufferViewCore.aTypedArrayConstructor; var exportTypedArrayMethod = ArrayBufferViewCore.exportTypedArrayMethod; // `%TypedArray%.prototype.map` method // https://tc39.es/ecma262/#sec-%typedarray%.prototype.map exportTypedArrayMethod('map', function map(mapfn /* , thisArg */) { return $map(aTypedArray(this), mapfn, arguments.length > 1 ? arguments[1] : undefined, function (O, length) { return new (aTypedArrayConstructor(speciesConstructor(O, O.constructor)))(length); }); }); apollo-server-demo/node_modules/core-js/modules/es.array.slice.js 0000644 0001750 0000144 00000004201 03560116604 024617 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var isObject = require('../internals/is-object'); var isArray = require('../internals/is-array'); var toAbsoluteIndex = require('../internals/to-absolute-index'); var toLength = require('../internals/to-length'); var toIndexedObject = require('../internals/to-indexed-object'); var createProperty = require('../internals/create-property'); var wellKnownSymbol = require('../internals/well-known-symbol'); var arrayMethodHasSpeciesSupport = require('../internals/array-method-has-species-support'); var arrayMethodUsesToLength = require('../internals/array-method-uses-to-length'); var HAS_SPECIES_SUPPORT = arrayMethodHasSpeciesSupport('slice'); var USES_TO_LENGTH = arrayMethodUsesToLength('slice', { ACCESSORS: true, 0: 0, 1: 2 }); var SPECIES = wellKnownSymbol('species'); var nativeSlice = [].slice; var max = Math.max; // `Array.prototype.slice` method // https://tc39.es/ecma262/#sec-array.prototype.slice // fallback for not array-like ES3 strings and DOM objects $({ target: 'Array', proto: true, forced: !HAS_SPECIES_SUPPORT || !USES_TO_LENGTH }, { slice: function slice(start, end) { var O = toIndexedObject(this); var length = toLength(O.length); var k = toAbsoluteIndex(start, length); var fin = toAbsoluteIndex(end === undefined ? length : end, length); // inline `ArraySpeciesCreate` for usage native `Array#slice` where it's possible var Constructor, result, n; if (isArray(O)) { Constructor = O.constructor; // cross-realm fallback if (typeof Constructor == 'function' && (Constructor === Array || isArray(Constructor.prototype))) { Constructor = undefined; } else if (isObject(Constructor)) { Constructor = Constructor[SPECIES]; if (Constructor === null) Constructor = undefined; } if (Constructor === Array || Constructor === undefined) { return nativeSlice.call(O, k, fin); } } result = new (Constructor === undefined ? Array : Constructor)(max(fin - k, 0)); for (n = 0; k < fin; k++, n++) if (k in O) createProperty(result, n, O[k]); result.length = n; return result; } }); apollo-server-demo/node_modules/core-js/modules/es.promise.any.js 0000644 0001750 0000144 00000003210 03560116604 024646 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var aFunction = require('../internals/a-function'); var getBuiltIn = require('../internals/get-built-in'); var newPromiseCapabilityModule = require('../internals/new-promise-capability'); var perform = require('../internals/perform'); var iterate = require('../internals/iterate'); var PROMISE_ANY_ERROR = 'No one promise resolved'; // `Promise.any` method // https://tc39.es/ecma262/#sec-promise.any $({ target: 'Promise', stat: true }, { any: function any(iterable) { var C = this; var capability = newPromiseCapabilityModule.f(C); var resolve = capability.resolve; var reject = capability.reject; var result = perform(function () { var promiseResolve = aFunction(C.resolve); var errors = []; var counter = 0; var remaining = 1; var alreadyResolved = false; iterate(iterable, function (promise) { var index = counter++; var alreadyRejected = false; errors.push(undefined); remaining++; promiseResolve.call(C, promise).then(function (value) { if (alreadyRejected || alreadyResolved) return; alreadyResolved = true; resolve(value); }, function (error) { if (alreadyRejected || alreadyResolved) return; alreadyRejected = true; errors[index] = error; --remaining || reject(new (getBuiltIn('AggregateError'))(errors, PROMISE_ANY_ERROR)); }); }); --remaining || reject(new (getBuiltIn('AggregateError'))(errors, PROMISE_ANY_ERROR)); }); if (result.error) reject(result.value); return capability.promise; } }); apollo-server-demo/node_modules/core-js/modules/esnext.symbol.dispose.js 0000644 0001750 0000144 00000000313 03560116604 026254 0 ustar andreh users var defineWellKnownSymbol = require('../internals/define-well-known-symbol'); // `Symbol.dispose` well-known symbol // https://github.com/tc39/proposal-using-statement defineWellKnownSymbol('dispose'); apollo-server-demo/node_modules/core-js/modules/es.symbol.has-instance.js 0000644 0001750 0000144 00000000322 03560116604 026264 0 ustar andreh users var defineWellKnownSymbol = require('../internals/define-well-known-symbol'); // `Symbol.hasInstance` well-known symbol // https://tc39.es/ecma262/#sec-symbol.hasinstance defineWellKnownSymbol('hasInstance'); apollo-server-demo/node_modules/core-js/modules/es.number.max-safe-integer.js 0000644 0001750 0000144 00000000326 03560116604 027032 0 ustar andreh users var $ = require('../internals/export'); // `Number.MAX_SAFE_INTEGER` constant // https://tc39.es/ecma262/#sec-number.max_safe_integer $({ target: 'Number', stat: true }, { MAX_SAFE_INTEGER: 0x1FFFFFFFFFFFFF }); apollo-server-demo/node_modules/core-js/modules/es.math.to-string-tag.js 0000644 0001750 0000144 00000000270 03560116604 026034 0 ustar andreh users var setToStringTag = require('../internals/set-to-string-tag'); // Math[@@toStringTag] property // https://tc39.es/ecma262/#sec-math-@@tostringtag setToStringTag(Math, 'Math', true); apollo-server-demo/node_modules/core-js/modules/esnext.map.group-by.js 0000644 0001750 0000144 00000001363 03560116604 025630 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var iterate = require('../internals/iterate'); var aFunction = require('../internals/a-function'); // `Map.groupBy` method // https://github.com/tc39/proposal-collection-methods $({ target: 'Map', stat: true }, { groupBy: function groupBy(iterable, keyDerivative) { var newMap = new this(); aFunction(keyDerivative); var has = aFunction(newMap.has); var get = aFunction(newMap.get); var set = aFunction(newMap.set); iterate(iterable, function (element) { var derivedKey = keyDerivative(element); if (!has.call(newMap, derivedKey)) set.call(newMap, derivedKey, [element]); else get.call(newMap, derivedKey).push(element); }); return newMap; } }); apollo-server-demo/node_modules/core-js/modules/es.reflect.set.js 0000644 0001750 0000144 00000004171 03560116604 024627 0 ustar andreh users var $ = require('../internals/export'); var anObject = require('../internals/an-object'); var isObject = require('../internals/is-object'); var has = require('../internals/has'); var fails = require('../internals/fails'); var definePropertyModule = require('../internals/object-define-property'); var getOwnPropertyDescriptorModule = require('../internals/object-get-own-property-descriptor'); var getPrototypeOf = require('../internals/object-get-prototype-of'); var createPropertyDescriptor = require('../internals/create-property-descriptor'); // `Reflect.set` method // https://tc39.es/ecma262/#sec-reflect.set function set(target, propertyKey, V /* , receiver */) { var receiver = arguments.length < 4 ? target : arguments[3]; var ownDescriptor = getOwnPropertyDescriptorModule.f(anObject(target), propertyKey); var existingDescriptor, prototype; if (!ownDescriptor) { if (isObject(prototype = getPrototypeOf(target))) { return set(prototype, propertyKey, V, receiver); } ownDescriptor = createPropertyDescriptor(0); } if (has(ownDescriptor, 'value')) { if (ownDescriptor.writable === false || !isObject(receiver)) return false; if (existingDescriptor = getOwnPropertyDescriptorModule.f(receiver, propertyKey)) { if (existingDescriptor.get || existingDescriptor.set || existingDescriptor.writable === false) return false; existingDescriptor.value = V; definePropertyModule.f(receiver, propertyKey, existingDescriptor); } else definePropertyModule.f(receiver, propertyKey, createPropertyDescriptor(0, V)); return true; } return ownDescriptor.set === undefined ? false : (ownDescriptor.set.call(receiver, V), true); } // MS Edge 17-18 Reflect.set allows setting the property to object // with non-writable property on the prototype var MS_EDGE_BUG = fails(function () { var Constructor = function () { /* empty */ }; var object = definePropertyModule.f(new Constructor(), 'a', { configurable: true }); // eslint-disable-next-line no-undef return Reflect.set(Constructor.prototype, 'a', 1, object) !== false; }); $({ target: 'Reflect', stat: true, forced: MS_EDGE_BUG }, { set: set }); apollo-server-demo/node_modules/core-js/modules/es.typed-array.every.js 0000644 0001750 0000144 00000001052 03560116604 025776 0 ustar andreh users 'use strict'; var ArrayBufferViewCore = require('../internals/array-buffer-view-core'); var $every = require('../internals/array-iteration').every; var aTypedArray = ArrayBufferViewCore.aTypedArray; var exportTypedArrayMethod = ArrayBufferViewCore.exportTypedArrayMethod; // `%TypedArray%.prototype.every` method // https://tc39.es/ecma262/#sec-%typedarray%.prototype.every exportTypedArrayMethod('every', function every(callbackfn /* , thisArg */) { return $every(aTypedArray(this), callbackfn, arguments.length > 1 ? arguments[1] : undefined); }); apollo-server-demo/node_modules/core-js/modules/es.object.to-string.js 0000644 0001750 0000144 00000000574 03560116604 025607 0 ustar andreh users var TO_STRING_TAG_SUPPORT = require('../internals/to-string-tag-support'); var redefine = require('../internals/redefine'); var toString = require('../internals/object-to-string'); // `Object.prototype.toString` method // https://tc39.es/ecma262/#sec-object.prototype.tostring if (!TO_STRING_TAG_SUPPORT) { redefine(Object.prototype, 'toString', toString, { unsafe: true }); } apollo-server-demo/node_modules/core-js/modules/esnext.set.of.js 0000644 0001750 0000144 00000000331 03560116604 024500 0 ustar andreh users var $ = require('../internals/export'); var of = require('../internals/collection-of'); // `Set.of` method // https://tc39.github.io/proposal-setmap-offrom/#sec-set.of $({ target: 'Set', stat: true }, { of: of }); apollo-server-demo/node_modules/core-js/modules/es.parse-int.js 0000644 0001750 0000144 00000000444 03560116604 024312 0 ustar andreh users var $ = require('../internals/export'); var parseIntImplementation = require('../internals/number-parse-int'); // `parseInt` method // https://tc39.es/ecma262/#sec-parseint-string-radix $({ global: true, forced: parseInt != parseIntImplementation }, { parseInt: parseIntImplementation }); apollo-server-demo/node_modules/core-js/modules/es.math.hypot.js 0000644 0001750 0000144 00000001561 03560116604 024504 0 ustar andreh users var $ = require('../internals/export'); var $hypot = Math.hypot; var abs = Math.abs; var sqrt = Math.sqrt; // Chrome 77 bug // https://bugs.chromium.org/p/v8/issues/detail?id=9546 var BUGGY = !!$hypot && $hypot(Infinity, NaN) !== Infinity; // `Math.hypot` method // https://tc39.es/ecma262/#sec-math.hypot $({ target: 'Math', stat: true, forced: BUGGY }, { hypot: function hypot(value1, value2) { // eslint-disable-line no-unused-vars var sum = 0; var i = 0; var aLen = arguments.length; var larg = 0; var arg, div; while (i < aLen) { arg = abs(arguments[i++]); if (larg < arg) { div = larg / arg; sum = sum * div * div + 1; larg = arg; } else if (arg > 0) { div = arg / larg; sum += div * div; } else sum += arg; } return larg === Infinity ? Infinity : larg * sqrt(sum); } }); apollo-server-demo/node_modules/core-js/modules/esnext.iterator.find.js 0000644 0001750 0000144 00000001025 03560116604 026053 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var iterate = require('../internals/iterate'); var aFunction = require('../internals/a-function'); var anObject = require('../internals/an-object'); $({ target: 'Iterator', proto: true, real: true }, { find: function find(fn) { anObject(this); aFunction(fn); return iterate(this, function (value, stop) { if (fn(value)) return stop(value); }, { IS_ITERATOR: true, INTERRUPTED: true }).result; } }); apollo-server-demo/node_modules/core-js/modules/es.string.bold.js 0000644 0001750 0000144 00000000651 03560116604 024635 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var createHTML = require('../internals/create-html'); var forcedStringHTMLMethod = require('../internals/string-html-forced'); // `String.prototype.bold` method // https://tc39.es/ecma262/#sec-string.prototype.bold $({ target: 'String', proto: true, forced: forcedStringHTMLMethod('bold') }, { bold: function bold() { return createHTML(this, 'b', '', ''); } }); apollo-server-demo/node_modules/core-js/modules/es.number.is-finite.js 0000644 0001750 0000144 00000000367 03560116604 025572 0 ustar andreh users var $ = require('../internals/export'); var numberIsFinite = require('../internals/number-is-finite'); // `Number.isFinite` method // https://tc39.es/ecma262/#sec-number.isfinite $({ target: 'Number', stat: true }, { isFinite: numberIsFinite }); apollo-server-demo/node_modules/core-js/modules/es.global-this.js 0000644 0001750 0000144 00000000307 03560116604 024613 0 ustar andreh users var $ = require('../internals/export'); var global = require('../internals/global'); // `globalThis` object // https://tc39.es/ecma262/#sec-globalthis $({ global: true }, { globalThis: global }); apollo-server-demo/node_modules/core-js/modules/es.symbol.description.js 0000644 0001750 0000144 00000004112 03560116604 026233 0 ustar andreh users // `Symbol.prototype.description` getter // https://tc39.es/ecma262/#sec-symbol.prototype.description 'use strict'; var $ = require('../internals/export'); var DESCRIPTORS = require('../internals/descriptors'); var global = require('../internals/global'); var has = require('../internals/has'); var isObject = require('../internals/is-object'); var defineProperty = require('../internals/object-define-property').f; var copyConstructorProperties = require('../internals/copy-constructor-properties'); var NativeSymbol = global.Symbol; if (DESCRIPTORS && typeof NativeSymbol == 'function' && (!('description' in NativeSymbol.prototype) || // Safari 12 bug NativeSymbol().description !== undefined )) { var EmptyStringDescriptionStore = {}; // wrap Symbol constructor for correct work with undefined description var SymbolWrapper = function Symbol() { var description = arguments.length < 1 || arguments[0] === undefined ? undefined : String(arguments[0]); var result = this instanceof SymbolWrapper ? new NativeSymbol(description) // in Edge 13, String(Symbol(undefined)) === 'Symbol(undefined)' : description === undefined ? NativeSymbol() : NativeSymbol(description); if (description === '') EmptyStringDescriptionStore[result] = true; return result; }; copyConstructorProperties(SymbolWrapper, NativeSymbol); var symbolPrototype = SymbolWrapper.prototype = NativeSymbol.prototype; symbolPrototype.constructor = SymbolWrapper; var symbolToString = symbolPrototype.toString; var native = String(NativeSymbol('test')) == 'Symbol(test)'; var regexp = /^Symbol\((.*)\)[^)]+$/; defineProperty(symbolPrototype, 'description', { configurable: true, get: function description() { var symbol = isObject(this) ? this.valueOf() : this; var string = symbolToString.call(symbol); if (has(EmptyStringDescriptionStore, symbol)) return ''; var desc = native ? string.slice(7, -1) : string.replace(regexp, '$1'); return desc === '' ? undefined : desc; } }); $({ global: true, forced: true }, { Symbol: SymbolWrapper }); } apollo-server-demo/node_modules/core-js/modules/esnext.iterator.some.js 0000644 0001750 0000144 00000001021 03560116604 026072 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var iterate = require('../internals/iterate'); var aFunction = require('../internals/a-function'); var anObject = require('../internals/an-object'); $({ target: 'Iterator', proto: true, real: true }, { some: function some(fn) { anObject(this); aFunction(fn); return iterate(this, function (value, stop) { if (fn(value)) return stop(); }, { IS_ITERATOR: true, INTERRUPTED: true }).stopped; } }); apollo-server-demo/node_modules/core-js/modules/esnext.math.fscale.js 0000644 0001750 0000144 00000000604 03560116604 025472 0 ustar andreh users var $ = require('../internals/export'); var scale = require('../internals/math-scale'); var fround = require('../internals/math-fround'); // `Math.fscale` method // https://rwaldron.github.io/proposal-math-extensions/ $({ target: 'Math', stat: true }, { fscale: function fscale(x, inLow, inHigh, outLow, outHigh) { return fround(scale(x, inLow, inHigh, outLow, outHigh)); } }); apollo-server-demo/node_modules/core-js/modules/es.math.log1p.js 0000644 0001750 0000144 00000000320 03560116604 024353 0 ustar andreh users var $ = require('../internals/export'); var log1p = require('../internals/math-log1p'); // `Math.log1p` method // https://tc39.es/ecma262/#sec-math.log1p $({ target: 'Math', stat: true }, { log1p: log1p }); apollo-server-demo/node_modules/core-js/modules/es.string.fontsize.js 0000644 0001750 0000144 00000000712 03560116604 025554 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var createHTML = require('../internals/create-html'); var forcedStringHTMLMethod = require('../internals/string-html-forced'); // `String.prototype.fontsize` method // https://tc39.es/ecma262/#sec-string.prototype.fontsize $({ target: 'String', proto: true, forced: forcedStringHTMLMethod('fontsize') }, { fontsize: function fontsize(size) { return createHTML(this, 'font', 'size', size); } }); apollo-server-demo/node_modules/core-js/modules/es.math.cbrt.js 0000644 0001750 0000144 00000000465 03560116604 024275 0 ustar andreh users var $ = require('../internals/export'); var sign = require('../internals/math-sign'); var abs = Math.abs; var pow = Math.pow; // `Math.cbrt` method // https://tc39.es/ecma262/#sec-math.cbrt $({ target: 'Math', stat: true }, { cbrt: function cbrt(x) { return sign(x = +x) * pow(abs(x), 1 / 3); } }); apollo-server-demo/node_modules/core-js/modules/esnext.iterator.from.js 0000644 0001750 0000144 00000002077 03560116604 026106 0 ustar andreh users // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var path = require('../internals/path'); var aFunction = require('../internals/a-function'); var anObject = require('../internals/an-object'); var toObject = require('../internals/to-object'); var createIteratorProxy = require('../internals/iterator-create-proxy'); var getIteratorMethod = require('../internals/get-iterator-method'); var Iterator = path.Iterator; var IteratorProxy = createIteratorProxy(function (arg) { var result = anObject(this.next.call(this.iterator, arg)); var done = this.done = !!result.done; if (!done) return result.value; }, true); $({ target: 'Iterator', stat: true }, { from: function from(O) { var object = toObject(O); var usingIterator = getIteratorMethod(object); var iterator; if (usingIterator != null) { iterator = aFunction(usingIterator).call(object); if (iterator instanceof Iterator) return iterator; } else { iterator = object; } return new IteratorProxy({ iterator: iterator }); } }); apollo-server-demo/node_modules/core-js/modules/es.symbol.js 0000644 0001750 0000144 00000031350 03560116604 023715 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var global = require('../internals/global'); var getBuiltIn = require('../internals/get-built-in'); var IS_PURE = require('../internals/is-pure'); var DESCRIPTORS = require('../internals/descriptors'); var NATIVE_SYMBOL = require('../internals/native-symbol'); var USE_SYMBOL_AS_UID = require('../internals/use-symbol-as-uid'); var fails = require('../internals/fails'); var has = require('../internals/has'); var isArray = require('../internals/is-array'); var isObject = require('../internals/is-object'); var anObject = require('../internals/an-object'); var toObject = require('../internals/to-object'); var toIndexedObject = require('../internals/to-indexed-object'); var toPrimitive = require('../internals/to-primitive'); var createPropertyDescriptor = require('../internals/create-property-descriptor'); var nativeObjectCreate = require('../internals/object-create'); var objectKeys = require('../internals/object-keys'); var getOwnPropertyNamesModule = require('../internals/object-get-own-property-names'); var getOwnPropertyNamesExternal = require('../internals/object-get-own-property-names-external'); var getOwnPropertySymbolsModule = require('../internals/object-get-own-property-symbols'); var getOwnPropertyDescriptorModule = require('../internals/object-get-own-property-descriptor'); var definePropertyModule = require('../internals/object-define-property'); var propertyIsEnumerableModule = require('../internals/object-property-is-enumerable'); var createNonEnumerableProperty = require('../internals/create-non-enumerable-property'); var redefine = require('../internals/redefine'); var shared = require('../internals/shared'); var sharedKey = require('../internals/shared-key'); var hiddenKeys = require('../internals/hidden-keys'); var uid = require('../internals/uid'); var wellKnownSymbol = require('../internals/well-known-symbol'); var wrappedWellKnownSymbolModule = require('../internals/well-known-symbol-wrapped'); var defineWellKnownSymbol = require('../internals/define-well-known-symbol'); var setToStringTag = require('../internals/set-to-string-tag'); var InternalStateModule = require('../internals/internal-state'); var $forEach = require('../internals/array-iteration').forEach; var HIDDEN = sharedKey('hidden'); var SYMBOL = 'Symbol'; var PROTOTYPE = 'prototype'; var TO_PRIMITIVE = wellKnownSymbol('toPrimitive'); var setInternalState = InternalStateModule.set; var getInternalState = InternalStateModule.getterFor(SYMBOL); var ObjectPrototype = Object[PROTOTYPE]; var $Symbol = global.Symbol; var $stringify = getBuiltIn('JSON', 'stringify'); var nativeGetOwnPropertyDescriptor = getOwnPropertyDescriptorModule.f; var nativeDefineProperty = definePropertyModule.f; var nativeGetOwnPropertyNames = getOwnPropertyNamesExternal.f; var nativePropertyIsEnumerable = propertyIsEnumerableModule.f; var AllSymbols = shared('symbols'); var ObjectPrototypeSymbols = shared('op-symbols'); var StringToSymbolRegistry = shared('string-to-symbol-registry'); var SymbolToStringRegistry = shared('symbol-to-string-registry'); var WellKnownSymbolsStore = shared('wks'); var QObject = global.QObject; // Don't use setters in Qt Script, https://github.com/zloirock/core-js/issues/173 var USE_SETTER = !QObject || !QObject[PROTOTYPE] || !QObject[PROTOTYPE].findChild; // fallback for old Android, https://code.google.com/p/v8/issues/detail?id=687 var setSymbolDescriptor = DESCRIPTORS && fails(function () { return nativeObjectCreate(nativeDefineProperty({}, 'a', { get: function () { return nativeDefineProperty(this, 'a', { value: 7 }).a; } })).a != 7; }) ? function (O, P, Attributes) { var ObjectPrototypeDescriptor = nativeGetOwnPropertyDescriptor(ObjectPrototype, P); if (ObjectPrototypeDescriptor) delete ObjectPrototype[P]; nativeDefineProperty(O, P, Attributes); if (ObjectPrototypeDescriptor && O !== ObjectPrototype) { nativeDefineProperty(ObjectPrototype, P, ObjectPrototypeDescriptor); } } : nativeDefineProperty; var wrap = function (tag, description) { var symbol = AllSymbols[tag] = nativeObjectCreate($Symbol[PROTOTYPE]); setInternalState(symbol, { type: SYMBOL, tag: tag, description: description }); if (!DESCRIPTORS) symbol.description = description; return symbol; }; var isSymbol = USE_SYMBOL_AS_UID ? function (it) { return typeof it == 'symbol'; } : function (it) { return Object(it) instanceof $Symbol; }; var $defineProperty = function defineProperty(O, P, Attributes) { if (O === ObjectPrototype) $defineProperty(ObjectPrototypeSymbols, P, Attributes); anObject(O); var key = toPrimitive(P, true); anObject(Attributes); if (has(AllSymbols, key)) { if (!Attributes.enumerable) { if (!has(O, HIDDEN)) nativeDefineProperty(O, HIDDEN, createPropertyDescriptor(1, {})); O[HIDDEN][key] = true; } else { if (has(O, HIDDEN) && O[HIDDEN][key]) O[HIDDEN][key] = false; Attributes = nativeObjectCreate(Attributes, { enumerable: createPropertyDescriptor(0, false) }); } return setSymbolDescriptor(O, key, Attributes); } return nativeDefineProperty(O, key, Attributes); }; var $defineProperties = function defineProperties(O, Properties) { anObject(O); var properties = toIndexedObject(Properties); var keys = objectKeys(properties).concat($getOwnPropertySymbols(properties)); $forEach(keys, function (key) { if (!DESCRIPTORS || $propertyIsEnumerable.call(properties, key)) $defineProperty(O, key, properties[key]); }); return O; }; var $create = function create(O, Properties) { return Properties === undefined ? nativeObjectCreate(O) : $defineProperties(nativeObjectCreate(O), Properties); }; var $propertyIsEnumerable = function propertyIsEnumerable(V) { var P = toPrimitive(V, true); var enumerable = nativePropertyIsEnumerable.call(this, P); if (this === ObjectPrototype && has(AllSymbols, P) && !has(ObjectPrototypeSymbols, P)) return false; return enumerable || !has(this, P) || !has(AllSymbols, P) || has(this, HIDDEN) && this[HIDDEN][P] ? enumerable : true; }; var $getOwnPropertyDescriptor = function getOwnPropertyDescriptor(O, P) { var it = toIndexedObject(O); var key = toPrimitive(P, true); if (it === ObjectPrototype && has(AllSymbols, key) && !has(ObjectPrototypeSymbols, key)) return; var descriptor = nativeGetOwnPropertyDescriptor(it, key); if (descriptor && has(AllSymbols, key) && !(has(it, HIDDEN) && it[HIDDEN][key])) { descriptor.enumerable = true; } return descriptor; }; var $getOwnPropertyNames = function getOwnPropertyNames(O) { var names = nativeGetOwnPropertyNames(toIndexedObject(O)); var result = []; $forEach(names, function (key) { if (!has(AllSymbols, key) && !has(hiddenKeys, key)) result.push(key); }); return result; }; var $getOwnPropertySymbols = function getOwnPropertySymbols(O) { var IS_OBJECT_PROTOTYPE = O === ObjectPrototype; var names = nativeGetOwnPropertyNames(IS_OBJECT_PROTOTYPE ? ObjectPrototypeSymbols : toIndexedObject(O)); var result = []; $forEach(names, function (key) { if (has(AllSymbols, key) && (!IS_OBJECT_PROTOTYPE || has(ObjectPrototype, key))) { result.push(AllSymbols[key]); } }); return result; }; // `Symbol` constructor // https://tc39.es/ecma262/#sec-symbol-constructor if (!NATIVE_SYMBOL) { $Symbol = function Symbol() { if (this instanceof $Symbol) throw TypeError('Symbol is not a constructor'); var description = !arguments.length || arguments[0] === undefined ? undefined : String(arguments[0]); var tag = uid(description); var setter = function (value) { if (this === ObjectPrototype) setter.call(ObjectPrototypeSymbols, value); if (has(this, HIDDEN) && has(this[HIDDEN], tag)) this[HIDDEN][tag] = false; setSymbolDescriptor(this, tag, createPropertyDescriptor(1, value)); }; if (DESCRIPTORS && USE_SETTER) setSymbolDescriptor(ObjectPrototype, tag, { configurable: true, set: setter }); return wrap(tag, description); }; redefine($Symbol[PROTOTYPE], 'toString', function toString() { return getInternalState(this).tag; }); redefine($Symbol, 'withoutSetter', function (description) { return wrap(uid(description), description); }); propertyIsEnumerableModule.f = $propertyIsEnumerable; definePropertyModule.f = $defineProperty; getOwnPropertyDescriptorModule.f = $getOwnPropertyDescriptor; getOwnPropertyNamesModule.f = getOwnPropertyNamesExternal.f = $getOwnPropertyNames; getOwnPropertySymbolsModule.f = $getOwnPropertySymbols; wrappedWellKnownSymbolModule.f = function (name) { return wrap(wellKnownSymbol(name), name); }; if (DESCRIPTORS) { // https://github.com/tc39/proposal-Symbol-description nativeDefineProperty($Symbol[PROTOTYPE], 'description', { configurable: true, get: function description() { return getInternalState(this).description; } }); if (!IS_PURE) { redefine(ObjectPrototype, 'propertyIsEnumerable', $propertyIsEnumerable, { unsafe: true }); } } } $({ global: true, wrap: true, forced: !NATIVE_SYMBOL, sham: !NATIVE_SYMBOL }, { Symbol: $Symbol }); $forEach(objectKeys(WellKnownSymbolsStore), function (name) { defineWellKnownSymbol(name); }); $({ target: SYMBOL, stat: true, forced: !NATIVE_SYMBOL }, { // `Symbol.for` method // https://tc39.es/ecma262/#sec-symbol.for 'for': function (key) { var string = String(key); if (has(StringToSymbolRegistry, string)) return StringToSymbolRegistry[string]; var symbol = $Symbol(string); StringToSymbolRegistry[string] = symbol; SymbolToStringRegistry[symbol] = string; return symbol; }, // `Symbol.keyFor` method // https://tc39.es/ecma262/#sec-symbol.keyfor keyFor: function keyFor(sym) { if (!isSymbol(sym)) throw TypeError(sym + ' is not a symbol'); if (has(SymbolToStringRegistry, sym)) return SymbolToStringRegistry[sym]; }, useSetter: function () { USE_SETTER = true; }, useSimple: function () { USE_SETTER = false; } }); $({ target: 'Object', stat: true, forced: !NATIVE_SYMBOL, sham: !DESCRIPTORS }, { // `Object.create` method // https://tc39.es/ecma262/#sec-object.create create: $create, // `Object.defineProperty` method // https://tc39.es/ecma262/#sec-object.defineproperty defineProperty: $defineProperty, // `Object.defineProperties` method // https://tc39.es/ecma262/#sec-object.defineproperties defineProperties: $defineProperties, // `Object.getOwnPropertyDescriptor` method // https://tc39.es/ecma262/#sec-object.getownpropertydescriptors getOwnPropertyDescriptor: $getOwnPropertyDescriptor }); $({ target: 'Object', stat: true, forced: !NATIVE_SYMBOL }, { // `Object.getOwnPropertyNames` method // https://tc39.es/ecma262/#sec-object.getownpropertynames getOwnPropertyNames: $getOwnPropertyNames, // `Object.getOwnPropertySymbols` method // https://tc39.es/ecma262/#sec-object.getownpropertysymbols getOwnPropertySymbols: $getOwnPropertySymbols }); // Chrome 38 and 39 `Object.getOwnPropertySymbols` fails on primitives // https://bugs.chromium.org/p/v8/issues/detail?id=3443 $({ target: 'Object', stat: true, forced: fails(function () { getOwnPropertySymbolsModule.f(1); }) }, { getOwnPropertySymbols: function getOwnPropertySymbols(it) { return getOwnPropertySymbolsModule.f(toObject(it)); } }); // `JSON.stringify` method behavior with symbols // https://tc39.es/ecma262/#sec-json.stringify if ($stringify) { var FORCED_JSON_STRINGIFY = !NATIVE_SYMBOL || fails(function () { var symbol = $Symbol(); // MS Edge converts symbol values to JSON as {} return $stringify([symbol]) != '[null]' // WebKit converts symbol values to JSON as null || $stringify({ a: symbol }) != '{}' // V8 throws on boxed symbols || $stringify(Object(symbol)) != '{}'; }); $({ target: 'JSON', stat: true, forced: FORCED_JSON_STRINGIFY }, { // eslint-disable-next-line no-unused-vars stringify: function stringify(it, replacer, space) { var args = [it]; var index = 1; var $replacer; while (arguments.length > index) args.push(arguments[index++]); $replacer = replacer; if (!isObject(replacer) && it === undefined || isSymbol(it)) return; // IE8 returns string on undefined if (!isArray(replacer)) replacer = function (key, value) { if (typeof $replacer == 'function') value = $replacer.call(this, key, value); if (!isSymbol(value)) return value; }; args[1] = replacer; return $stringify.apply(null, args); } }); } // `Symbol.prototype[@@toPrimitive]` method // https://tc39.es/ecma262/#sec-symbol.prototype-@@toprimitive if (!$Symbol[PROTOTYPE][TO_PRIMITIVE]) { createNonEnumerableProperty($Symbol[PROTOTYPE], TO_PRIMITIVE, $Symbol[PROTOTYPE].valueOf); } // `Symbol.prototype[@@toStringTag]` property // https://tc39.es/ecma262/#sec-symbol.prototype-@@tostringtag setToStringTag($Symbol, SYMBOL); hiddenKeys[HIDDEN] = true; apollo-server-demo/node_modules/core-js/modules/esnext.math.rad-per-deg.js 0000644 0001750 0000144 00000000305 03560116604 026322 0 ustar andreh users var $ = require('../internals/export'); // `Math.RAD_PER_DEG` constant // https://rwaldron.github.io/proposal-math-extensions/ $({ target: 'Math', stat: true }, { RAD_PER_DEG: 180 / Math.PI }); apollo-server-demo/node_modules/core-js/modules/esnext.async-iterator.take.js 0000644 0001750 0000144 00000001771 03560116604 027202 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var anObject = require('../internals/an-object'); var toPositiveInteger = require('../internals/to-positive-integer'); var createAsyncIteratorProxy = require('../internals/async-iterator-create-proxy'); var AsyncIteratorProxy = createAsyncIteratorProxy(function (arg, Promise) { var iterator = this.iterator; var returnMethod, result; if (!this.remaining--) { result = { done: true, value: undefined }; this.done = true; returnMethod = iterator['return']; if (returnMethod !== undefined) { return Promise.resolve(returnMethod.call(iterator)).then(function () { return result; }); } return result; } return this.next.call(iterator, arg); }); $({ target: 'AsyncIterator', proto: true, real: true }, { take: function take(limit) { return new AsyncIteratorProxy({ iterator: anObject(this), remaining: toPositiveInteger(limit) }); } }); apollo-server-demo/node_modules/core-js/modules/esnext.iterator.constructor.js 0000644 0001750 0000144 00000003171 03560116604 027524 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var global = require('../internals/global'); var anInstance = require('../internals/an-instance'); var createNonEnumerableProperty = require('../internals/create-non-enumerable-property'); var fails = require('../internals/fails'); var has = require('../internals/has'); var wellKnownSymbol = require('../internals/well-known-symbol'); var IteratorPrototype = require('../internals/iterators-core').IteratorPrototype; var IS_PURE = require('../internals/is-pure'); var ITERATOR = wellKnownSymbol('iterator'); var TO_STRING_TAG = wellKnownSymbol('toStringTag'); var NativeIterator = global.Iterator; // FF56- have non-standard global helper `Iterator` var FORCED = IS_PURE || typeof NativeIterator != 'function' || NativeIterator.prototype !== IteratorPrototype // FF44- non-standard `Iterator` passes previous tests || !fails(function () { NativeIterator({}); }); var IteratorConstructor = function Iterator() { anInstance(this, IteratorConstructor); }; if (IS_PURE) { IteratorPrototype = {}; createNonEnumerableProperty(IteratorPrototype, ITERATOR, function () { return this; }); } if (!has(IteratorPrototype, TO_STRING_TAG)) { createNonEnumerableProperty(IteratorPrototype, TO_STRING_TAG, 'Iterator'); } if (FORCED || !has(IteratorPrototype, 'constructor') || IteratorPrototype.constructor === Object) { createNonEnumerableProperty(IteratorPrototype, 'constructor', IteratorConstructor); } IteratorConstructor.prototype = IteratorPrototype; $({ global: true, forced: FORCED }, { Iterator: IteratorConstructor }); apollo-server-demo/node_modules/core-js/modules/es.date.to-string.js 0000644 0001750 0000144 00000001126 03560116604 025250 0 ustar andreh users var redefine = require('../internals/redefine'); var DatePrototype = Date.prototype; var INVALID_DATE = 'Invalid Date'; var TO_STRING = 'toString'; var nativeDateToString = DatePrototype[TO_STRING]; var getTime = DatePrototype.getTime; // `Date.prototype.toString` method // https://tc39.es/ecma262/#sec-date.prototype.tostring if (new Date(NaN) + '' != INVALID_DATE) { redefine(DatePrototype, TO_STRING, function toString() { var value = getTime.call(this); // eslint-disable-next-line no-self-compare return value === value ? nativeDateToString.call(this) : INVALID_DATE; }); } apollo-server-demo/node_modules/core-js/modules/es.date.to-primitive.js 0000644 0001750 0000144 00000001023 03560116604 025746 0 ustar andreh users var createNonEnumerableProperty = require('../internals/create-non-enumerable-property'); var dateToPrimitive = require('../internals/date-to-primitive'); var wellKnownSymbol = require('../internals/well-known-symbol'); var TO_PRIMITIVE = wellKnownSymbol('toPrimitive'); var DatePrototype = Date.prototype; // `Date.prototype[@@toPrimitive]` method // https://tc39.es/ecma262/#sec-date.prototype-@@toprimitive if (!(TO_PRIMITIVE in DatePrototype)) { createNonEnumerableProperty(DatePrototype, TO_PRIMITIVE, dateToPrimitive); } apollo-server-demo/node_modules/core-js/modules/esnext.iterator.drop.js 0000644 0001750 0000144 00000001625 03560116604 026105 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var anObject = require('../internals/an-object'); var toPositiveInteger = require('../internals/to-positive-integer'); var createIteratorProxy = require('../internals/iterator-create-proxy'); var IteratorProxy = createIteratorProxy(function (arg) { var iterator = this.iterator; var next = this.next; var result, done; while (this.remaining) { this.remaining--; result = anObject(next.call(iterator)); done = this.done = !!result.done; if (done) return; } result = anObject(next.call(iterator, arg)); done = this.done = !!result.done; if (!done) return result.value; }); $({ target: 'Iterator', proto: true, real: true }, { drop: function drop(limit) { return new IteratorProxy({ iterator: anObject(this), remaining: toPositiveInteger(limit) }); } }); apollo-server-demo/node_modules/core-js/modules/esnext.promise.any.js 0000644 0001750 0000144 00000000076 03560116604 025554 0 ustar andreh users // TODO: Remove from `core-js@4` require('./es.promise.any'); apollo-server-demo/node_modules/core-js/modules/es.array.index-of.js 0000644 0001750 0000144 00000001706 03560116604 025240 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var $indexOf = require('../internals/array-includes').indexOf; var arrayMethodIsStrict = require('../internals/array-method-is-strict'); var arrayMethodUsesToLength = require('../internals/array-method-uses-to-length'); var nativeIndexOf = [].indexOf; var NEGATIVE_ZERO = !!nativeIndexOf && 1 / [1].indexOf(1, -0) < 0; var STRICT_METHOD = arrayMethodIsStrict('indexOf'); var USES_TO_LENGTH = arrayMethodUsesToLength('indexOf', { ACCESSORS: true, 1: 0 }); // `Array.prototype.indexOf` method // https://tc39.es/ecma262/#sec-array.prototype.indexof $({ target: 'Array', proto: true, forced: NEGATIVE_ZERO || !STRICT_METHOD || !USES_TO_LENGTH }, { indexOf: function indexOf(searchElement /* , fromIndex = 0 */) { return NEGATIVE_ZERO // convert -0 to +0 ? nativeIndexOf.apply(this, arguments) || 0 : $indexOf(this, searchElement, arguments.length > 1 ? arguments[1] : undefined); } }); apollo-server-demo/node_modules/core-js/modules/esnext.composite-symbol.js 0000644 0001750 0000144 00000001011 03560116604 026603 0 ustar andreh users var $ = require('../internals/export'); var getCompositeKeyNode = require('../internals/composite-key'); var getBuiltIn = require('../internals/get-built-in'); // https://github.com/tc39/proposal-richer-keys/tree/master/compositeKey $({ global: true }, { compositeSymbol: function compositeSymbol() { if (arguments.length === 1 && typeof arguments[0] === 'string') return getBuiltIn('Symbol')['for'](arguments[0]); return getCompositeKeyNode.apply(null, arguments).get('symbol', getBuiltIn('Symbol')); } }); apollo-server-demo/node_modules/core-js/modules/es.number.to-precision.js 0000644 0001750 0000144 00000001365 03560116604 026315 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var fails = require('../internals/fails'); var thisNumberValue = require('../internals/this-number-value'); var nativeToPrecision = 1.0.toPrecision; var FORCED = fails(function () { // IE7- return nativeToPrecision.call(1, undefined) !== '1'; }) || !fails(function () { // V8 ~ Android 4.3- nativeToPrecision.call({}); }); // `Number.prototype.toPrecision` method // https://tc39.es/ecma262/#sec-number.prototype.toprecision $({ target: 'Number', proto: true, forced: FORCED }, { toPrecision: function toPrecision(precision) { return precision === undefined ? nativeToPrecision.call(thisNumberValue(this)) : nativeToPrecision.call(thisNumberValue(this), precision); } }); apollo-server-demo/node_modules/core-js/modules/esnext.weak-map.emplace.js 0000644 0001750 0000144 00000000510 03560116604 026410 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var $emplace = require('../internals/map-emplace'); // `WeakMap.prototype.emplace` method // https://github.com/tc39/proposal-upsert $({ target: 'WeakMap', proto: true, real: true, forced: IS_PURE }, { emplace: $emplace }); apollo-server-demo/node_modules/core-js/modules/es.math.sinh.js 0000644 0001750 0000144 00000001075 03560116604 024302 0 ustar andreh users var $ = require('../internals/export'); var fails = require('../internals/fails'); var expm1 = require('../internals/math-expm1'); var abs = Math.abs; var exp = Math.exp; var E = Math.E; var FORCED = fails(function () { return Math.sinh(-2e-17) != -2e-17; }); // `Math.sinh` method // https://tc39.es/ecma262/#sec-math.sinh // V8 near Chromium 38 has a problem with very small numbers $({ target: 'Math', stat: true, forced: FORCED }, { sinh: function sinh(x) { return abs(x = +x) < 1 ? (expm1(x) - expm1(-x)) / 2 : (exp(x - 1) - exp(-x - 1)) * (E / 2); } }); apollo-server-demo/node_modules/core-js/modules/es.weak-map.js 0000644 0001750 0000144 00000005365 03560116604 024121 0 ustar andreh users 'use strict'; var global = require('../internals/global'); var redefineAll = require('../internals/redefine-all'); var InternalMetadataModule = require('../internals/internal-metadata'); var collection = require('../internals/collection'); var collectionWeak = require('../internals/collection-weak'); var isObject = require('../internals/is-object'); var enforceIternalState = require('../internals/internal-state').enforce; var NATIVE_WEAK_MAP = require('../internals/native-weak-map'); var IS_IE11 = !global.ActiveXObject && 'ActiveXObject' in global; var isExtensible = Object.isExtensible; var InternalWeakMap; var wrapper = function (init) { return function WeakMap() { return init(this, arguments.length ? arguments[0] : undefined); }; }; // `WeakMap` constructor // https://tc39.es/ecma262/#sec-weakmap-constructor var $WeakMap = module.exports = collection('WeakMap', wrapper, collectionWeak); // IE11 WeakMap frozen keys fix // We can't use feature detection because it crash some old IE builds // https://github.com/zloirock/core-js/issues/485 if (NATIVE_WEAK_MAP && IS_IE11) { InternalWeakMap = collectionWeak.getConstructor(wrapper, 'WeakMap', true); InternalMetadataModule.REQUIRED = true; var WeakMapPrototype = $WeakMap.prototype; var nativeDelete = WeakMapPrototype['delete']; var nativeHas = WeakMapPrototype.has; var nativeGet = WeakMapPrototype.get; var nativeSet = WeakMapPrototype.set; redefineAll(WeakMapPrototype, { 'delete': function (key) { if (isObject(key) && !isExtensible(key)) { var state = enforceIternalState(this); if (!state.frozen) state.frozen = new InternalWeakMap(); return nativeDelete.call(this, key) || state.frozen['delete'](key); } return nativeDelete.call(this, key); }, has: function has(key) { if (isObject(key) && !isExtensible(key)) { var state = enforceIternalState(this); if (!state.frozen) state.frozen = new InternalWeakMap(); return nativeHas.call(this, key) || state.frozen.has(key); } return nativeHas.call(this, key); }, get: function get(key) { if (isObject(key) && !isExtensible(key)) { var state = enforceIternalState(this); if (!state.frozen) state.frozen = new InternalWeakMap(); return nativeHas.call(this, key) ? nativeGet.call(this, key) : state.frozen.get(key); } return nativeGet.call(this, key); }, set: function set(key, value) { if (isObject(key) && !isExtensible(key)) { var state = enforceIternalState(this); if (!state.frozen) state.frozen = new InternalWeakMap(); nativeHas.call(this, key) ? nativeSet.call(this, key, value) : state.frozen.set(key, value); } else nativeSet.call(this, key, value); return this; } }); } apollo-server-demo/node_modules/core-js/modules/esnext.map.update.js 0000644 0001750 0000144 00000001457 03560116604 025352 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var anObject = require('../internals/an-object'); var aFunction = require('../internals/a-function'); // `Set.prototype.update` method // https://github.com/tc39/proposal-collection-methods $({ target: 'Map', proto: true, real: true, forced: IS_PURE }, { update: function update(key, callback /* , thunk */) { var map = anObject(this); var length = arguments.length; aFunction(callback); var isPresentInMap = map.has(key); if (!isPresentInMap && length < 3) { throw TypeError('Updating absent value'); } var value = isPresentInMap ? map.get(key) : aFunction(length > 2 ? arguments[2] : undefined)(key, map); map.set(key, callback(value, key, map)); return map; } }); apollo-server-demo/node_modules/core-js/modules/es.number.constructor.js 0000644 0001750 0000144 00000006746 03560116604 026277 0 ustar andreh users 'use strict'; var DESCRIPTORS = require('../internals/descriptors'); var global = require('../internals/global'); var isForced = require('../internals/is-forced'); var redefine = require('../internals/redefine'); var has = require('../internals/has'); var classof = require('../internals/classof-raw'); var inheritIfRequired = require('../internals/inherit-if-required'); var toPrimitive = require('../internals/to-primitive'); var fails = require('../internals/fails'); var create = require('../internals/object-create'); var getOwnPropertyNames = require('../internals/object-get-own-property-names').f; var getOwnPropertyDescriptor = require('../internals/object-get-own-property-descriptor').f; var defineProperty = require('../internals/object-define-property').f; var trim = require('../internals/string-trim').trim; var NUMBER = 'Number'; var NativeNumber = global[NUMBER]; var NumberPrototype = NativeNumber.prototype; // Opera ~12 has broken Object#toString var BROKEN_CLASSOF = classof(create(NumberPrototype)) == NUMBER; // `ToNumber` abstract operation // https://tc39.es/ecma262/#sec-tonumber var toNumber = function (argument) { var it = toPrimitive(argument, false); var first, third, radix, maxCode, digits, length, index, code; if (typeof it == 'string' && it.length > 2) { it = trim(it); first = it.charCodeAt(0); if (first === 43 || first === 45) { third = it.charCodeAt(2); if (third === 88 || third === 120) return NaN; // Number('+0x1') should be NaN, old V8 fix } else if (first === 48) { switch (it.charCodeAt(1)) { case 66: case 98: radix = 2; maxCode = 49; break; // fast equal of /^0b[01]+$/i case 79: case 111: radix = 8; maxCode = 55; break; // fast equal of /^0o[0-7]+$/i default: return +it; } digits = it.slice(2); length = digits.length; for (index = 0; index < length; index++) { code = digits.charCodeAt(index); // parseInt parses a string to a first unavailable symbol // but ToNumber should return NaN if a string contains unavailable symbols if (code < 48 || code > maxCode) return NaN; } return parseInt(digits, radix); } } return +it; }; // `Number` constructor // https://tc39.es/ecma262/#sec-number-constructor if (isForced(NUMBER, !NativeNumber(' 0o1') || !NativeNumber('0b1') || NativeNumber('+0x1'))) { var NumberWrapper = function Number(value) { var it = arguments.length < 1 ? 0 : value; var dummy = this; return dummy instanceof NumberWrapper // check on 1..constructor(foo) case && (BROKEN_CLASSOF ? fails(function () { NumberPrototype.valueOf.call(dummy); }) : classof(dummy) != NUMBER) ? inheritIfRequired(new NativeNumber(toNumber(it)), dummy, NumberWrapper) : toNumber(it); }; for (var keys = DESCRIPTORS ? getOwnPropertyNames(NativeNumber) : ( // ES3: 'MAX_VALUE,MIN_VALUE,NaN,NEGATIVE_INFINITY,POSITIVE_INFINITY,' + // ES2015 (in case, if modules with ES2015 Number statics required before): 'EPSILON,isFinite,isInteger,isNaN,isSafeInteger,MAX_SAFE_INTEGER,' + 'MIN_SAFE_INTEGER,parseFloat,parseInt,isInteger,' + // ESNext 'fromString,range' ).split(','), j = 0, key; keys.length > j; j++) { if (has(NativeNumber, key = keys[j]) && !has(NumberWrapper, key)) { defineProperty(NumberWrapper, key, getOwnPropertyDescriptor(NativeNumber, key)); } } NumberWrapper.prototype = NumberPrototype; NumberPrototype.constructor = NumberWrapper; redefine(global, NUMBER, NumberWrapper); } apollo-server-demo/node_modules/core-js/modules/es.string.sup.js 0000644 0001750 0000144 00000000646 03560116604 024530 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var createHTML = require('../internals/create-html'); var forcedStringHTMLMethod = require('../internals/string-html-forced'); // `String.prototype.sup` method // https://tc39.es/ecma262/#sec-string.prototype.sup $({ target: 'String', proto: true, forced: forcedStringHTMLMethod('sup') }, { sup: function sup() { return createHTML(this, 'sup', '', ''); } }); apollo-server-demo/node_modules/core-js/modules/esnext.array.is-template-object.js 0000644 0001750 0000144 00000001610 03560116604 030110 0 ustar andreh users var $ = require('../internals/export'); var isArray = require('../internals/is-array'); var isFrozen = Object.isFrozen; var isFrozenStringArray = function (array, allowUndefined) { if (!isFrozen || !isArray(array) || !isFrozen(array)) return false; var index = 0; var length = array.length; var element; while (index < length) { element = array[index++]; if (!(typeof element === 'string' || (allowUndefined && typeof element === 'undefined'))) { return false; } } return length !== 0; }; // `Array.isTemplateObject` method // https://github.com/tc39/proposal-array-is-template-object $({ target: 'Array', stat: true }, { isTemplateObject: function isTemplateObject(value) { if (!isFrozenStringArray(value, true)) return false; var raw = value.raw; if (raw.length !== value.length || !isFrozenStringArray(raw, false)) return false; return true; } }); apollo-server-demo/node_modules/core-js/modules/es.data-view.js 0000644 0001750 0000144 00000000534 03560116604 024271 0 ustar andreh users var $ = require('../internals/export'); var ArrayBufferModule = require('../internals/array-buffer'); var NATIVE_ARRAY_BUFFER = require('../internals/array-buffer-native'); // `DataView` constructor // https://tc39.es/ecma262/#sec-dataview-constructor $({ global: true, forced: !NATIVE_ARRAY_BUFFER }, { DataView: ArrayBufferModule.DataView }); apollo-server-demo/node_modules/core-js/modules/esnext.set.intersection.js 0000644 0001750 0000144 00000001577 03560116604 026617 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var getBuiltIn = require('../internals/get-built-in'); var anObject = require('../internals/an-object'); var aFunction = require('../internals/a-function'); var speciesConstructor = require('../internals/species-constructor'); var iterate = require('../internals/iterate'); // `Set.prototype.intersection` method // https://github.com/tc39/proposal-set-methods $({ target: 'Set', proto: true, real: true, forced: IS_PURE }, { intersection: function intersection(iterable) { var set = anObject(this); var newSet = new (speciesConstructor(set, getBuiltIn('Set')))(); var hasCheck = aFunction(set.has); var adder = aFunction(newSet.add); iterate(iterable, function (value) { if (hasCheck.call(set, value)) adder.call(newSet, value); }); return newSet; } }); apollo-server-demo/node_modules/core-js/modules/es.math.trunc.js 0000644 0001750 0000144 00000000414 03560116604 024470 0 ustar andreh users var $ = require('../internals/export'); var ceil = Math.ceil; var floor = Math.floor; // `Math.trunc` method // https://tc39.es/ecma262/#sec-math.trunc $({ target: 'Math', stat: true }, { trunc: function trunc(it) { return (it > 0 ? floor : ceil)(it); } }); apollo-server-demo/node_modules/core-js/modules/esnext.array.last-index.js 0000644 0001750 0000144 00000001271 03560116604 026473 0 ustar andreh users 'use strict'; var DESCRIPTORS = require('../internals/descriptors'); var addToUnscopables = require('../internals/add-to-unscopables'); var toObject = require('../internals/to-object'); var toLength = require('../internals/to-length'); var defineProperty = require('../internals/object-define-property').f; // `Array.prototype.lastIndex` getter // https://github.com/keithamus/proposal-array-last if (DESCRIPTORS && !('lastIndex' in [])) { defineProperty(Array.prototype, 'lastIndex', { configurable: true, get: function lastIndex() { var O = toObject(this); var len = toLength(O.length); return len == 0 ? 0 : len - 1; } }); addToUnscopables('lastIndex'); } apollo-server-demo/node_modules/core-js/modules/esnext.set.reduce.js 0000644 0001750 0000144 00000002070 03560116604 025345 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var anObject = require('../internals/an-object'); var aFunction = require('../internals/a-function'); var getSetIterator = require('../internals/get-set-iterator'); var iterate = require('../internals/iterate'); // `Set.prototype.reduce` method // https://github.com/tc39/proposal-collection-methods $({ target: 'Set', proto: true, real: true, forced: IS_PURE }, { reduce: function reduce(callbackfn /* , initialValue */) { var set = anObject(this); var iterator = getSetIterator(set); var noInitial = arguments.length < 2; var accumulator = noInitial ? undefined : arguments[1]; aFunction(callbackfn); iterate(iterator, function (value) { if (noInitial) { noInitial = false; accumulator = value; } else { accumulator = callbackfn(accumulator, value, value, set); } }, { IS_ITERATOR: true }); if (noInitial) throw TypeError('Reduce of empty set with no initial value'); return accumulator; } }); apollo-server-demo/node_modules/core-js/modules/esnext.weak-set.add-all.js 0000644 0001750 0000144 00000000665 03560116604 026331 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var collectionAddAll = require('../internals/collection-add-all'); // `WeakSet.prototype.addAll` method // https://github.com/tc39/proposal-collection-methods $({ target: 'WeakSet', proto: true, real: true, forced: IS_PURE }, { addAll: function addAll(/* ...elements */) { return collectionAddAll.apply(this, arguments); } }); apollo-server-demo/node_modules/core-js/modules/es.object.define-getter.js 0000644 0001750 0000144 00000001341 03560116604 026374 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var DESCRIPTORS = require('../internals/descriptors'); var FORCED = require('../internals/object-prototype-accessors-forced'); var toObject = require('../internals/to-object'); var aFunction = require('../internals/a-function'); var definePropertyModule = require('../internals/object-define-property'); // `Object.prototype.__defineGetter__` method // https://tc39.es/ecma262/#sec-object.prototype.__defineGetter__ if (DESCRIPTORS) { $({ target: 'Object', proto: true, forced: FORCED }, { __defineGetter__: function __defineGetter__(P, getter) { definePropertyModule.f(toObject(this), P, { get: aFunction(getter), enumerable: true, configurable: true }); } }); } apollo-server-demo/node_modules/core-js/modules/esnext.string.at-alternative.js 0000644 0001750 0000144 00000001541 03560116604 027533 0 ustar andreh users // TODO: disabled by default because of the conflict with another proposal 'use strict'; var $ = require('../internals/export'); var requireObjectCoercible = require('../internals/require-object-coercible'); var toLength = require('../internals/to-length'); var toInteger = require('../internals/to-integer'); var fails = require('../internals/fails'); var FORCED = fails(function () { return 'ð ®·'.at(0) !== '\uD842'; }); // `String.prototype.at` method // https://github.com/tc39/proposal-relative-indexing-method $({ target: 'String', proto: true, forced: FORCED }, { at: function at(index) { var S = String(requireObjectCoercible(this)); var len = toLength(S.length); var relativeIndex = toInteger(index); var k = relativeIndex >= 0 ? relativeIndex : len + relativeIndex; return (k < 0 || k >= len) ? undefined : S.charAt(k); } }); apollo-server-demo/node_modules/core-js/modules/es.string.trim-start.js 0000644 0001750 0000144 00000001135 03560116604 026021 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var $trimStart = require('../internals/string-trim').start; var forcedStringTrimMethod = require('../internals/string-trim-forced'); var FORCED = forcedStringTrimMethod('trimStart'); var trimStart = FORCED ? function trimStart() { return $trimStart(this); } : ''.trimStart; // `String.prototype.{ trimStart, trimLeft }` methods // https://tc39.es/ecma262/#sec-string.prototype.trimstart // https://tc39.es/ecma262/#String.prototype.trimleft $({ target: 'String', proto: true, forced: FORCED }, { trimStart: trimStart, trimLeft: trimStart }); apollo-server-demo/node_modules/core-js/modules/esnext.iterator.map.js 0000644 0001750 0000144 00000001517 03560116604 025716 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var aFunction = require('../internals/a-function'); var anObject = require('../internals/an-object'); var createIteratorProxy = require('../internals/iterator-create-proxy'); var callWithSafeIterationClosing = require('../internals/call-with-safe-iteration-closing'); var IteratorProxy = createIteratorProxy(function (arg) { var iterator = this.iterator; var result = anObject(this.next.call(iterator, arg)); var done = this.done = !!result.done; if (!done) return callWithSafeIterationClosing(iterator, this.mapper, result.value); }); $({ target: 'Iterator', proto: true, real: true }, { map: function map(mapper) { return new IteratorProxy({ iterator: anObject(this), mapper: aFunction(mapper) }); } }); apollo-server-demo/node_modules/core-js/modules/es.math.log2.js 0000644 0001750 0000144 00000000362 03560116604 024202 0 ustar andreh users var $ = require('../internals/export'); var log = Math.log; var LN2 = Math.LN2; // `Math.log2` method // https://tc39.es/ecma262/#sec-math.log2 $({ target: 'Math', stat: true }, { log2: function log2(x) { return log(x) / LN2; } }); apollo-server-demo/node_modules/core-js/modules/es.function.name.js 0000644 0001750 0000144 00000001241 03560116604 025150 0 ustar andreh users var DESCRIPTORS = require('../internals/descriptors'); var defineProperty = require('../internals/object-define-property').f; var FunctionPrototype = Function.prototype; var FunctionPrototypeToString = FunctionPrototype.toString; var nameRE = /^\s*function ([^ (]*)/; var NAME = 'name'; // Function instances `.name` property // https://tc39.es/ecma262/#sec-function-instances-name if (DESCRIPTORS && !(NAME in FunctionPrototype)) { defineProperty(FunctionPrototype, NAME, { configurable: true, get: function () { try { return FunctionPrototypeToString.call(this).match(nameRE)[1]; } catch (error) { return ''; } } }); } apollo-server-demo/node_modules/core-js/modules/es.typed-array.subarray.js 0000644 0001750 0000144 00000001577 03560116604 026510 0 ustar andreh users 'use strict'; var ArrayBufferViewCore = require('../internals/array-buffer-view-core'); var toLength = require('../internals/to-length'); var toAbsoluteIndex = require('../internals/to-absolute-index'); var speciesConstructor = require('../internals/species-constructor'); var aTypedArray = ArrayBufferViewCore.aTypedArray; var exportTypedArrayMethod = ArrayBufferViewCore.exportTypedArrayMethod; // `%TypedArray%.prototype.subarray` method // https://tc39.es/ecma262/#sec-%typedarray%.prototype.subarray exportTypedArrayMethod('subarray', function subarray(begin, end) { var O = aTypedArray(this); var length = O.length; var beginIndex = toAbsoluteIndex(begin, length); return new (speciesConstructor(O, O.constructor))( O.buffer, O.byteOffset + beginIndex * O.BYTES_PER_ELEMENT, toLength((end === undefined ? length : toAbsoluteIndex(end, length)) - beginIndex) ); }); apollo-server-demo/node_modules/core-js/modules/esnext.map.of.js 0000644 0001750 0000144 00000000331 03560116604 024462 0 ustar andreh users var $ = require('../internals/export'); var of = require('../internals/collection-of'); // `Map.of` method // https://tc39.github.io/proposal-setmap-offrom/#sec-map.of $({ target: 'Map', stat: true }, { of: of }); apollo-server-demo/node_modules/core-js/modules/esnext.weak-map.of.js 0000644 0001750 0000144 00000000345 03560116604 025414 0 ustar andreh users var $ = require('../internals/export'); var of = require('../internals/collection-of'); // `WeakMap.of` method // https://tc39.github.io/proposal-setmap-offrom/#sec-weakmap.of $({ target: 'WeakMap', stat: true }, { of: of }); apollo-server-demo/node_modules/core-js/modules/esnext.map.key-by.js 0000644 0001750 0000144 00000001052 03560116604 025257 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var iterate = require('../internals/iterate'); var aFunction = require('../internals/a-function'); // `Map.keyBy` method // https://github.com/tc39/proposal-collection-methods $({ target: 'Map', stat: true }, { keyBy: function keyBy(iterable, keyDerivative) { var newMap = new this(); aFunction(keyDerivative); var setter = aFunction(newMap.set); iterate(iterable, function (element) { setter.call(newMap, keyDerivative(element), element); }); return newMap; } }); apollo-server-demo/node_modules/core-js/modules/es.string.match.js 0000644 0001750 0000144 00000003143 03560116604 025010 0 ustar andreh users 'use strict'; var fixRegExpWellKnownSymbolLogic = require('../internals/fix-regexp-well-known-symbol-logic'); var anObject = require('../internals/an-object'); var toLength = require('../internals/to-length'); var requireObjectCoercible = require('../internals/require-object-coercible'); var advanceStringIndex = require('../internals/advance-string-index'); var regExpExec = require('../internals/regexp-exec-abstract'); // @@match logic fixRegExpWellKnownSymbolLogic('match', 1, function (MATCH, nativeMatch, maybeCallNative) { return [ // `String.prototype.match` method // https://tc39.es/ecma262/#sec-string.prototype.match function match(regexp) { var O = requireObjectCoercible(this); var matcher = regexp == undefined ? undefined : regexp[MATCH]; return matcher !== undefined ? matcher.call(regexp, O) : new RegExp(regexp)[MATCH](String(O)); }, // `RegExp.prototype[@@match]` method // https://tc39.es/ecma262/#sec-regexp.prototype-@@match function (regexp) { var res = maybeCallNative(nativeMatch, regexp, this); if (res.done) return res.value; var rx = anObject(regexp); var S = String(this); if (!rx.global) return regExpExec(rx, S); var fullUnicode = rx.unicode; rx.lastIndex = 0; var A = []; var n = 0; var result; while ((result = regExpExec(rx, S)) !== null) { var matchStr = String(result[0]); A[n] = matchStr; if (matchStr === '') rx.lastIndex = advanceStringIndex(S, toLength(rx.lastIndex), fullUnicode); n++; } return n === 0 ? null : A; } ]; }); apollo-server-demo/node_modules/core-js/modules/es.symbol.to-string-tag.js 0000644 0001750 0000144 00000000322 03560116604 026406 0 ustar andreh users var defineWellKnownSymbol = require('../internals/define-well-known-symbol'); // `Symbol.toStringTag` well-known symbol // https://tc39.es/ecma262/#sec-symbol.tostringtag defineWellKnownSymbol('toStringTag'); apollo-server-demo/node_modules/core-js/modules/esnext.weak-map.upsert.js 0000644 0001750 0000144 00000000616 03560116604 026333 0 ustar andreh users 'use strict'; // TODO: remove from `core-js@4` var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var $upsert = require('../internals/map-upsert'); // `WeakMap.prototype.upsert` method (replaced by `WeakMap.prototype.emplace`) // https://github.com/tc39/proposal-upsert $({ target: 'WeakMap', proto: true, real: true, forced: IS_PURE }, { upsert: $upsert }); apollo-server-demo/node_modules/core-js/modules/es.array.join.js 0000644 0001750 0000144 00000001247 03560116604 024466 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IndexedObject = require('../internals/indexed-object'); var toIndexedObject = require('../internals/to-indexed-object'); var arrayMethodIsStrict = require('../internals/array-method-is-strict'); var nativeJoin = [].join; var ES3_STRINGS = IndexedObject != Object; var STRICT_METHOD = arrayMethodIsStrict('join', ','); // `Array.prototype.join` method // https://tc39.es/ecma262/#sec-array.prototype.join $({ target: 'Array', proto: true, forced: ES3_STRINGS || !STRICT_METHOD }, { join: function join(separator) { return nativeJoin.call(toIndexedObject(this), separator === undefined ? ',' : separator); } }); apollo-server-demo/node_modules/core-js/modules/es.object.get-prototype-of.js 0000644 0001750 0000144 00000001223 03560116604 027075 0 ustar andreh users var $ = require('../internals/export'); var fails = require('../internals/fails'); var toObject = require('../internals/to-object'); var nativeGetPrototypeOf = require('../internals/object-get-prototype-of'); var CORRECT_PROTOTYPE_GETTER = require('../internals/correct-prototype-getter'); var FAILS_ON_PRIMITIVES = fails(function () { nativeGetPrototypeOf(1); }); // `Object.getPrototypeOf` method // https://tc39.es/ecma262/#sec-object.getprototypeof $({ target: 'Object', stat: true, forced: FAILS_ON_PRIMITIVES, sham: !CORRECT_PROTOTYPE_GETTER }, { getPrototypeOf: function getPrototypeOf(it) { return nativeGetPrototypeOf(toObject(it)); } }); apollo-server-demo/node_modules/core-js/modules/esnext.number.from-string.js 0000644 0001750 0000144 00000002150 03560116604 027041 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var toInteger = require('../internals/to-integer'); var parseInt = require('../internals/number-parse-int'); var INVALID_NUMBER_REPRESENTATION = 'Invalid number representation'; var INVALID_RADIX = 'Invalid radix'; var valid = /^[\da-z]+$/; // `Number.fromString` method // https://github.com/tc39/proposal-number-fromstring $({ target: 'Number', stat: true }, { fromString: function fromString(string, radix) { var sign = 1; var R, mathNum; if (typeof string != 'string') throw TypeError(INVALID_NUMBER_REPRESENTATION); if (!string.length) throw SyntaxError(INVALID_NUMBER_REPRESENTATION); if (string.charAt(0) == '-') { sign = -1; string = string.slice(1); if (!string.length) throw SyntaxError(INVALID_NUMBER_REPRESENTATION); } R = radix === undefined ? 10 : toInteger(radix); if (R < 2 || R > 36) throw RangeError(INVALID_RADIX); if (!valid.test(string) || (mathNum = parseInt(string, R)).toString(R) !== string) { throw SyntaxError(INVALID_NUMBER_REPRESENTATION); } return sign * mathNum; } }); apollo-server-demo/node_modules/core-js/modules/esnext.set.find.js 0000644 0001750 0000144 00000001546 03560116604 025025 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var anObject = require('../internals/an-object'); var bind = require('../internals/function-bind-context'); var getSetIterator = require('../internals/get-set-iterator'); var iterate = require('../internals/iterate'); // `Set.prototype.find` method // https://github.com/tc39/proposal-collection-methods $({ target: 'Set', proto: true, real: true, forced: IS_PURE }, { find: function find(callbackfn /* , thisArg */) { var set = anObject(this); var iterator = getSetIterator(set); var boundFunction = bind(callbackfn, arguments.length > 1 ? arguments[1] : undefined, 3); return iterate(iterator, function (value, stop) { if (boundFunction(value, value, set)) return stop(value); }, { IS_ITERATOR: true, INTERRUPTED: true }).result; } }); apollo-server-demo/node_modules/core-js/modules/es.map.js 0000644 0001750 0000144 00000000557 03560116604 023172 0 ustar andreh users 'use strict'; var collection = require('../internals/collection'); var collectionStrong = require('../internals/collection-strong'); // `Map` constructor // https://tc39.es/ecma262/#sec-map-objects module.exports = collection('Map', function (init) { return function Map() { return init(this, arguments.length ? arguments[0] : undefined); }; }, collectionStrong); apollo-server-demo/node_modules/core-js/modules/esnext.symbol.replace-all.js 0000644 0001750 0000144 00000000225 03560116604 026771 0 ustar andreh users // TODO: remove from `core-js@4` var defineWellKnownSymbol = require('../internals/define-well-known-symbol'); defineWellKnownSymbol('replaceAll'); apollo-server-demo/node_modules/core-js/modules/es.math.asinh.js 0000644 0001750 0000144 00000000666 03560116604 024450 0 ustar andreh users var $ = require('../internals/export'); var nativeAsinh = Math.asinh; var log = Math.log; var sqrt = Math.sqrt; function asinh(x) { return !isFinite(x = +x) || x == 0 ? x : x < 0 ? -asinh(-x) : log(x + sqrt(x * x + 1)); } // `Math.asinh` method // https://tc39.es/ecma262/#sec-math.asinh // Tor Browser bug: Math.asinh(0) -> -0 $({ target: 'Math', stat: true, forced: !(nativeAsinh && 1 / nativeAsinh(0) > 0) }, { asinh: asinh }); apollo-server-demo/node_modules/core-js/modules/es.promise.all-settled.js 0000644 0001750 0000144 00000002753 03560116604 026304 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var aFunction = require('../internals/a-function'); var newPromiseCapabilityModule = require('../internals/new-promise-capability'); var perform = require('../internals/perform'); var iterate = require('../internals/iterate'); // `Promise.allSettled` method // https://tc39.es/ecma262/#sec-promise.allsettled $({ target: 'Promise', stat: true }, { allSettled: function allSettled(iterable) { var C = this; var capability = newPromiseCapabilityModule.f(C); var resolve = capability.resolve; var reject = capability.reject; var result = perform(function () { var promiseResolve = aFunction(C.resolve); var values = []; var counter = 0; var remaining = 1; iterate(iterable, function (promise) { var index = counter++; var alreadyCalled = false; values.push(undefined); remaining++; promiseResolve.call(C, promise).then(function (value) { if (alreadyCalled) return; alreadyCalled = true; values[index] = { status: 'fulfilled', value: value }; --remaining || resolve(values); }, function (error) { if (alreadyCalled) return; alreadyCalled = true; values[index] = { status: 'rejected', reason: error }; --remaining || resolve(values); }); }); --remaining || resolve(values); }); if (result.error) reject(result.value); return capability.promise; } }); apollo-server-demo/node_modules/core-js/modules/es.reflect.construct.js 0000644 0001750 0000144 00000004204 03560116604 026055 0 ustar andreh users var $ = require('../internals/export'); var getBuiltIn = require('../internals/get-built-in'); var aFunction = require('../internals/a-function'); var anObject = require('../internals/an-object'); var isObject = require('../internals/is-object'); var create = require('../internals/object-create'); var bind = require('../internals/function-bind'); var fails = require('../internals/fails'); var nativeConstruct = getBuiltIn('Reflect', 'construct'); // `Reflect.construct` method // https://tc39.es/ecma262/#sec-reflect.construct // MS Edge supports only 2 arguments and argumentsList argument is optional // FF Nightly sets third argument as `new.target`, but does not create `this` from it var NEW_TARGET_BUG = fails(function () { function F() { /* empty */ } return !(nativeConstruct(function () { /* empty */ }, [], F) instanceof F); }); var ARGS_BUG = !fails(function () { nativeConstruct(function () { /* empty */ }); }); var FORCED = NEW_TARGET_BUG || ARGS_BUG; $({ target: 'Reflect', stat: true, forced: FORCED, sham: FORCED }, { construct: function construct(Target, args /* , newTarget */) { aFunction(Target); anObject(args); var newTarget = arguments.length < 3 ? Target : aFunction(arguments[2]); if (ARGS_BUG && !NEW_TARGET_BUG) return nativeConstruct(Target, args, newTarget); if (Target == newTarget) { // w/o altered newTarget, optimization for 0-4 arguments switch (args.length) { case 0: return new Target(); case 1: return new Target(args[0]); case 2: return new Target(args[0], args[1]); case 3: return new Target(args[0], args[1], args[2]); case 4: return new Target(args[0], args[1], args[2], args[3]); } // w/o altered newTarget, lot of arguments case var $args = [null]; $args.push.apply($args, args); return new (bind.apply(Target, $args))(); } // with altered newTarget, not support built-in constructors var proto = newTarget.prototype; var instance = create(isObject(proto) ? proto : Object.prototype); var result = Function.apply.call(Target, instance, args); return isObject(result) ? result : instance; } }); apollo-server-demo/node_modules/core-js/modules/esnext.object.iterate-values.js 0000644 0001750 0000144 00000000534 03560116604 027506 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var ObjectIterator = require('../internals/object-iterator'); // `Object.iterateValues` method // https://github.com/tc39/proposal-object-iteration $({ target: 'Object', stat: true }, { iterateValues: function iterateValues(object) { return new ObjectIterator(object, 'values'); } }); apollo-server-demo/node_modules/core-js/modules/es.array.reverse.js 0000644 0001750 0000144 00000001111 03560116604 025170 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var isArray = require('../internals/is-array'); var nativeReverse = [].reverse; var test = [1, 2]; // `Array.prototype.reverse` method // https://tc39.es/ecma262/#sec-array.prototype.reverse // fix for Safari 12.0 bug // https://bugs.webkit.org/show_bug.cgi?id=188794 $({ target: 'Array', proto: true, forced: String(test) === String(test.reverse()) }, { reverse: function reverse() { // eslint-disable-next-line no-self-assign if (isArray(this)) this.length = this.length; return nativeReverse.call(this); } }); apollo-server-demo/node_modules/core-js/modules/es.function.bind.js 0000644 0001750 0000144 00000000361 03560116604 025146 0 ustar andreh users var $ = require('../internals/export'); var bind = require('../internals/function-bind'); // `Function.prototype.bind` method // https://tc39.es/ecma262/#sec-function.prototype.bind $({ target: 'Function', proto: true }, { bind: bind }); apollo-server-demo/node_modules/core-js/modules/esnext.set.from.js 0000644 0001750 0000144 00000000345 03560116604 025044 0 ustar andreh users var $ = require('../internals/export'); var from = require('../internals/collection-from'); // `Set.from` method // https://tc39.github.io/proposal-setmap-offrom/#sec-set.from $({ target: 'Set', stat: true }, { from: from }); apollo-server-demo/node_modules/core-js/modules/es.json.to-string-tag.js 0000644 0001750 0000144 00000000354 03560116604 026057 0 ustar andreh users var global = require('../internals/global'); var setToStringTag = require('../internals/set-to-string-tag'); // JSON[@@toStringTag] property // https://tc39.es/ecma262/#sec-json-@@tostringtag setToStringTag(global.JSON, 'JSON', true); apollo-server-demo/node_modules/core-js/modules/es.string.strike.js 0000644 0001750 0000144 00000000670 03560116604 025217 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var createHTML = require('../internals/create-html'); var forcedStringHTMLMethod = require('../internals/string-html-forced'); // `String.prototype.strike` method // https://tc39.es/ecma262/#sec-string.prototype.strike $({ target: 'String', proto: true, forced: forcedStringHTMLMethod('strike') }, { strike: function strike() { return createHTML(this, 'strike', '', ''); } }); apollo-server-demo/node_modules/core-js/modules/es.array.copy-within.js 0000644 0001750 0000144 00000000654 03560116604 026002 0 ustar andreh users var $ = require('../internals/export'); var copyWithin = require('../internals/array-copy-within'); var addToUnscopables = require('../internals/add-to-unscopables'); // `Array.prototype.copyWithin` method // https://tc39.es/ecma262/#sec-array.prototype.copywithin $({ target: 'Array', proto: true }, { copyWithin: copyWithin }); // https://tc39.es/ecma262/#sec-array.prototype-@@unscopables addToUnscopables('copyWithin'); apollo-server-demo/node_modules/core-js/modules/web.immediate.js 0000644 0001750 0000144 00000001014 03560116604 024506 0 ustar andreh users var $ = require('../internals/export'); var global = require('../internals/global'); var task = require('../internals/task'); var FORCED = !global.setImmediate || !global.clearImmediate; // http://w3c.github.io/setImmediate/ $({ global: true, bind: true, enumerable: true, forced: FORCED }, { // `setImmediate` method // http://w3c.github.io/setImmediate/#si-setImmediate setImmediate: task.set, // `clearImmediate` method // http://w3c.github.io/setImmediate/#si-clearImmediate clearImmediate: task.clear }); apollo-server-demo/node_modules/core-js/modules/es.reflect.get-prototype-of.js 0000644 0001750 0000144 00000001011 03560116604 027246 0 ustar andreh users var $ = require('../internals/export'); var anObject = require('../internals/an-object'); var objectGetPrototypeOf = require('../internals/object-get-prototype-of'); var CORRECT_PROTOTYPE_GETTER = require('../internals/correct-prototype-getter'); // `Reflect.getPrototypeOf` method // https://tc39.es/ecma262/#sec-reflect.getprototypeof $({ target: 'Reflect', stat: true, sham: !CORRECT_PROTOTYPE_GETTER }, { getPrototypeOf: function getPrototypeOf(target) { return objectGetPrototypeOf(anObject(target)); } }); apollo-server-demo/node_modules/core-js/modules/web.queue-microtask.js 0000644 0001750 0000144 00000001040 03560116604 025665 0 ustar andreh users var $ = require('../internals/export'); var global = require('../internals/global'); var microtask = require('../internals/microtask'); var IS_NODE = require('../internals/engine-is-node'); var process = global.process; // `queueMicrotask` method // https://html.spec.whatwg.org/multipage/timers-and-user-prompts.html#dom-queuemicrotask $({ global: true, enumerable: true, noTargetGet: true }, { queueMicrotask: function queueMicrotask(fn) { var domain = IS_NODE && process.domain; microtask(domain ? domain.bind(fn) : fn); } }); apollo-server-demo/node_modules/core-js/modules/es.string.repeat.js 0000644 0001750 0000144 00000000365 03560116604 025177 0 ustar andreh users var $ = require('../internals/export'); var repeat = require('../internals/string-repeat'); // `String.prototype.repeat` method // https://tc39.es/ecma262/#sec-string.prototype.repeat $({ target: 'String', proto: true }, { repeat: repeat }); apollo-server-demo/node_modules/core-js/modules/esnext.iterator.every.js 0000644 0001750 0000144 00000001025 03560116604 026265 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var iterate = require('../internals/iterate'); var aFunction = require('../internals/a-function'); var anObject = require('../internals/an-object'); $({ target: 'Iterator', proto: true, real: true }, { every: function every(fn) { anObject(this); aFunction(fn); return !iterate(this, function (value, stop) { if (!fn(value)) return stop(); }, { IS_ITERATOR: true, INTERRUPTED: true }).stopped; } }); apollo-server-demo/node_modules/core-js/modules/es.reflect.get-own-property-descriptor.js 0000644 0001750 0000144 00000001105 03560116604 031444 0 ustar andreh users var $ = require('../internals/export'); var DESCRIPTORS = require('../internals/descriptors'); var anObject = require('../internals/an-object'); var getOwnPropertyDescriptorModule = require('../internals/object-get-own-property-descriptor'); // `Reflect.getOwnPropertyDescriptor` method // https://tc39.es/ecma262/#sec-reflect.getownpropertydescriptor $({ target: 'Reflect', stat: true, sham: !DESCRIPTORS }, { getOwnPropertyDescriptor: function getOwnPropertyDescriptor(target, propertyKey) { return getOwnPropertyDescriptorModule.f(anObject(target), propertyKey); } }); apollo-server-demo/node_modules/core-js/modules/es.string.anchor.js 0000644 0001750 0000144 00000000675 03560116604 025175 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var createHTML = require('../internals/create-html'); var forcedStringHTMLMethod = require('../internals/string-html-forced'); // `String.prototype.anchor` method // https://tc39.es/ecma262/#sec-string.prototype.anchor $({ target: 'String', proto: true, forced: forcedStringHTMLMethod('anchor') }, { anchor: function anchor(name) { return createHTML(this, 'a', 'name', name); } }); apollo-server-demo/node_modules/core-js/modules/es.array.fill.js 0000644 0001750 0000144 00000000601 03560116604 024446 0 ustar andreh users var $ = require('../internals/export'); var fill = require('../internals/array-fill'); var addToUnscopables = require('../internals/add-to-unscopables'); // `Array.prototype.fill` method // https://tc39.es/ecma262/#sec-array.prototype.fill $({ target: 'Array', proto: true }, { fill: fill }); // https://tc39.es/ecma262/#sec-array.prototype-@@unscopables addToUnscopables('fill'); apollo-server-demo/node_modules/core-js/modules/es.object.from-entries.js 0000644 0001750 0000144 00000000724 03560116604 026270 0 ustar andreh users var $ = require('../internals/export'); var iterate = require('../internals/iterate'); var createProperty = require('../internals/create-property'); // `Object.fromEntries` method // https://github.com/tc39/proposal-object-from-entries $({ target: 'Object', stat: true }, { fromEntries: function fromEntries(iterable) { var obj = {}; iterate(iterable, function (k, v) { createProperty(obj, k, v); }, { AS_ENTRIES: true }); return obj; } }); apollo-server-demo/node_modules/core-js/modules/es.reflect.delete-property.js 0000644 0001750 0000144 00000001050 03560116604 027151 0 ustar andreh users var $ = require('../internals/export'); var anObject = require('../internals/an-object'); var getOwnPropertyDescriptor = require('../internals/object-get-own-property-descriptor').f; // `Reflect.deleteProperty` method // https://tc39.es/ecma262/#sec-reflect.deleteproperty $({ target: 'Reflect', stat: true }, { deleteProperty: function deleteProperty(target, propertyKey) { var descriptor = getOwnPropertyDescriptor(anObject(target), propertyKey); return descriptor && !descriptor.configurable ? false : delete target[propertyKey]; } }); apollo-server-demo/node_modules/core-js/modules/es.set.js 0000644 0001750 0000144 00000000557 03560116604 023210 0 ustar andreh users 'use strict'; var collection = require('../internals/collection'); var collectionStrong = require('../internals/collection-strong'); // `Set` constructor // https://tc39.es/ecma262/#sec-set-objects module.exports = collection('Set', function (init) { return function Set() { return init(this, arguments.length ? arguments[0] : undefined); }; }, collectionStrong); apollo-server-demo/node_modules/core-js/modules/es.object.is.js 0000644 0001750 0000144 00000000311 03560116604 024261 0 ustar andreh users var $ = require('../internals/export'); var is = require('../internals/same-value'); // `Object.is` method // https://tc39.es/ecma262/#sec-object.is $({ target: 'Object', stat: true }, { is: is }); apollo-server-demo/node_modules/core-js/modules/esnext.math.iaddh.js 0000644 0001750 0000144 00000000626 03560116604 025312 0 ustar andreh users var $ = require('../internals/export'); // `Math.iaddh` method // https://gist.github.com/BrendanEich/4294d5c212a6d2254703 // TODO: Remove from `core-js@4` $({ target: 'Math', stat: true }, { iaddh: function iaddh(x0, x1, y0, y1) { var $x0 = x0 >>> 0; var $x1 = x1 >>> 0; var $y0 = y0 >>> 0; return $x1 + (y1 >>> 0) + (($x0 & $y0 | ($x0 | $y0) & ~($x0 + $y0 >>> 0)) >>> 31) | 0; } }); apollo-server-demo/node_modules/core-js/modules/es.math.log10.js 0000644 0001750 0000144 00000000377 03560116604 024267 0 ustar andreh users var $ = require('../internals/export'); var log = Math.log; var LOG10E = Math.LOG10E; // `Math.log10` method // https://tc39.es/ecma262/#sec-math.log10 $({ target: 'Math', stat: true }, { log10: function log10(x) { return log(x) * LOG10E; } }); apollo-server-demo/node_modules/core-js/modules/es.array.species.js 0000644 0001750 0000144 00000000235 03560116604 025156 0 ustar andreh users var setSpecies = require('../internals/set-species'); // `Array[@@species]` getter // https://tc39.es/ecma262/#sec-get-array-@@species setSpecies('Array'); apollo-server-demo/node_modules/core-js/modules/es.math.expm1.js 0000644 0001750 0000144 00000000355 03560116604 024373 0 ustar andreh users var $ = require('../internals/export'); var expm1 = require('../internals/math-expm1'); // `Math.expm1` method // https://tc39.es/ecma262/#sec-math.expm1 $({ target: 'Math', stat: true, forced: expm1 != Math.expm1 }, { expm1: expm1 }); apollo-server-demo/node_modules/core-js/modules/es.symbol.match-all.js 0000644 0001750 0000144 00000000311 03560116604 025547 0 ustar andreh users var defineWellKnownSymbol = require('../internals/define-well-known-symbol'); // `Symbol.matchAll` well-known symbol // https://tc39.es/ecma262/#sec-symbol.matchall defineWellKnownSymbol('matchAll'); apollo-server-demo/node_modules/core-js/modules/es.typed-array.from.js 0000644 0001750 0000144 00000000746 03560116604 025620 0 ustar andreh users 'use strict'; var TYPED_ARRAYS_CONSTRUCTORS_REQUIRES_WRAPPERS = require('../internals/typed-array-constructors-require-wrappers'); var exportTypedArrayStaticMethod = require('../internals/array-buffer-view-core').exportTypedArrayStaticMethod; var typedArrayFrom = require('../internals/typed-array-from'); // `%TypedArray%.from` method // https://tc39.es/ecma262/#sec-%typedarray%.from exportTypedArrayStaticMethod('from', typedArrayFrom, TYPED_ARRAYS_CONSTRUCTORS_REQUIRES_WRAPPERS); apollo-server-demo/node_modules/core-js/modules/es.array.every.js 0000644 0001750 0000144 00000001267 03560116604 024663 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var $every = require('../internals/array-iteration').every; var arrayMethodIsStrict = require('../internals/array-method-is-strict'); var arrayMethodUsesToLength = require('../internals/array-method-uses-to-length'); var STRICT_METHOD = arrayMethodIsStrict('every'); var USES_TO_LENGTH = arrayMethodUsesToLength('every'); // `Array.prototype.every` method // https://tc39.es/ecma262/#sec-array.prototype.every $({ target: 'Array', proto: true, forced: !STRICT_METHOD || !USES_TO_LENGTH }, { every: function every(callbackfn /* , thisArg */) { return $every(this, callbackfn, arguments.length > 1 ? arguments[1] : undefined); } }); apollo-server-demo/node_modules/core-js/modules/es.string.small.js 0000644 0001750 0000144 00000000662 03560116604 025027 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var createHTML = require('../internals/create-html'); var forcedStringHTMLMethod = require('../internals/string-html-forced'); // `String.prototype.small` method // https://tc39.es/ecma262/#sec-string.prototype.small $({ target: 'String', proto: true, forced: forcedStringHTMLMethod('small') }, { small: function small() { return createHTML(this, 'small', '', ''); } }); apollo-server-demo/node_modules/core-js/modules/esnext.async-iterator.to-array.js 0000644 0001750 0000144 00000000462 03560116604 030010 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var $toArray = require('../internals/async-iterator-iteration').toArray; $({ target: 'AsyncIterator', proto: true, real: true }, { toArray: function toArray() { return $toArray(this); } }); apollo-server-demo/node_modules/core-js/modules/es.function.has-instance.js 0000644 0001750 0000144 00000001636 03560116604 026615 0 ustar andreh users 'use strict'; var isObject = require('../internals/is-object'); var definePropertyModule = require('../internals/object-define-property'); var getPrototypeOf = require('../internals/object-get-prototype-of'); var wellKnownSymbol = require('../internals/well-known-symbol'); var HAS_INSTANCE = wellKnownSymbol('hasInstance'); var FunctionPrototype = Function.prototype; // `Function.prototype[@@hasInstance]` method // https://tc39.es/ecma262/#sec-function.prototype-@@hasinstance if (!(HAS_INSTANCE in FunctionPrototype)) { definePropertyModule.f(FunctionPrototype, HAS_INSTANCE, { value: function (O) { if (typeof this != 'function' || !isObject(O)) return false; if (!isObject(this.prototype)) return O instanceof this; // for environment w/o native `@@hasInstance` logic enough `instanceof`, but add this: while (O = getPrototypeOf(O)) if (this.prototype === O) return true; return false; } }); } apollo-server-demo/node_modules/core-js/modules/es.array.concat.js 0000644 0001750 0000144 00000004532 03560116604 024776 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var fails = require('../internals/fails'); var isArray = require('../internals/is-array'); var isObject = require('../internals/is-object'); var toObject = require('../internals/to-object'); var toLength = require('../internals/to-length'); var createProperty = require('../internals/create-property'); var arraySpeciesCreate = require('../internals/array-species-create'); var arrayMethodHasSpeciesSupport = require('../internals/array-method-has-species-support'); var wellKnownSymbol = require('../internals/well-known-symbol'); var V8_VERSION = require('../internals/engine-v8-version'); var IS_CONCAT_SPREADABLE = wellKnownSymbol('isConcatSpreadable'); var MAX_SAFE_INTEGER = 0x1FFFFFFFFFFFFF; var MAXIMUM_ALLOWED_INDEX_EXCEEDED = 'Maximum allowed index exceeded'; // We can't use this feature detection in V8 since it causes // deoptimization and serious performance degradation // https://github.com/zloirock/core-js/issues/679 var IS_CONCAT_SPREADABLE_SUPPORT = V8_VERSION >= 51 || !fails(function () { var array = []; array[IS_CONCAT_SPREADABLE] = false; return array.concat()[0] !== array; }); var SPECIES_SUPPORT = arrayMethodHasSpeciesSupport('concat'); var isConcatSpreadable = function (O) { if (!isObject(O)) return false; var spreadable = O[IS_CONCAT_SPREADABLE]; return spreadable !== undefined ? !!spreadable : isArray(O); }; var FORCED = !IS_CONCAT_SPREADABLE_SUPPORT || !SPECIES_SUPPORT; // `Array.prototype.concat` method // https://tc39.es/ecma262/#sec-array.prototype.concat // with adding support of @@isConcatSpreadable and @@species $({ target: 'Array', proto: true, forced: FORCED }, { concat: function concat(arg) { // eslint-disable-line no-unused-vars var O = toObject(this); var A = arraySpeciesCreate(O, 0); var n = 0; var i, k, length, len, E; for (i = -1, length = arguments.length; i < length; i++) { E = i === -1 ? O : arguments[i]; if (isConcatSpreadable(E)) { len = toLength(E.length); if (n + len > MAX_SAFE_INTEGER) throw TypeError(MAXIMUM_ALLOWED_INDEX_EXCEEDED); for (k = 0; k < len; k++, n++) if (k in E) createProperty(A, n, E[k]); } else { if (n >= MAX_SAFE_INTEGER) throw TypeError(MAXIMUM_ALLOWED_INDEX_EXCEEDED); createProperty(A, n++, E); } } A.length = n; return A; } }); apollo-server-demo/node_modules/core-js/modules/es.array.unscopables.flat-map.js 0000644 0001750 0000144 00000000426 03560116604 027543 0 ustar andreh users // this method was added to unscopables after implementation // in popular engines, so it's moved to a separate module var addToUnscopables = require('../internals/add-to-unscopables'); // https://tc39.es/ecma262/#sec-array.prototype-@@unscopables addToUnscopables('flatMap'); apollo-server-demo/node_modules/core-js/modules/es.reflect.get.js 0000644 0001750 0000144 00000001751 03560116604 024614 0 ustar andreh users var $ = require('../internals/export'); var isObject = require('../internals/is-object'); var anObject = require('../internals/an-object'); var has = require('../internals/has'); var getOwnPropertyDescriptorModule = require('../internals/object-get-own-property-descriptor'); var getPrototypeOf = require('../internals/object-get-prototype-of'); // `Reflect.get` method // https://tc39.es/ecma262/#sec-reflect.get function get(target, propertyKey /* , receiver */) { var receiver = arguments.length < 3 ? target : arguments[2]; var descriptor, prototype; if (anObject(target) === receiver) return target[propertyKey]; if (descriptor = getOwnPropertyDescriptorModule.f(target, propertyKey)) return has(descriptor, 'value') ? descriptor.value : descriptor.get === undefined ? undefined : descriptor.get.call(receiver); if (isObject(prototype = getPrototypeOf(target))) return get(prototype, propertyKey, receiver); } $({ target: 'Reflect', stat: true }, { get: get }); apollo-server-demo/node_modules/core-js/modules/es.weak-set.js 0000644 0001750 0000144 00000000554 03560116604 024132 0 ustar andreh users 'use strict'; var collection = require('../internals/collection'); var collectionWeak = require('../internals/collection-weak'); // `WeakSet` constructor // https://tc39.es/ecma262/#sec-weakset-constructor collection('WeakSet', function (init) { return function WeakSet() { return init(this, arguments.length ? arguments[0] : undefined); }; }, collectionWeak); apollo-server-demo/node_modules/core-js/modules/esnext.reflect.has-metadata.js 0000644 0001750 0000144 00000001677 03560116604 027274 0 ustar andreh users var $ = require('../internals/export'); var ReflectMetadataModule = require('../internals/reflect-metadata'); var anObject = require('../internals/an-object'); var getPrototypeOf = require('../internals/object-get-prototype-of'); var ordinaryHasOwnMetadata = ReflectMetadataModule.has; var toMetadataKey = ReflectMetadataModule.toKey; var ordinaryHasMetadata = function (MetadataKey, O, P) { var hasOwn = ordinaryHasOwnMetadata(MetadataKey, O, P); if (hasOwn) return true; var parent = getPrototypeOf(O); return parent !== null ? ordinaryHasMetadata(MetadataKey, parent, P) : false; }; // `Reflect.hasMetadata` method // https://github.com/rbuckton/reflect-metadata $({ target: 'Reflect', stat: true }, { hasMetadata: function hasMetadata(metadataKey, target /* , targetKey */) { var targetKey = arguments.length < 3 ? undefined : toMetadataKey(arguments[2]); return ordinaryHasMetadata(metadataKey, anObject(target), targetKey); } }); apollo-server-demo/node_modules/core-js/modules/es.symbol.match.js 0000644 0001750 0000144 00000000300 03560116604 024777 0 ustar andreh users var defineWellKnownSymbol = require('../internals/define-well-known-symbol'); // `Symbol.match` well-known symbol // https://tc39.es/ecma262/#sec-symbol.match defineWellKnownSymbol('match'); apollo-server-demo/node_modules/core-js/modules/esnext.set.map.js 0000644 0001750 0000144 00000002150 03560116604 024652 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var getBuiltIn = require('../internals/get-built-in'); var anObject = require('../internals/an-object'); var aFunction = require('../internals/a-function'); var bind = require('../internals/function-bind-context'); var speciesConstructor = require('../internals/species-constructor'); var getSetIterator = require('../internals/get-set-iterator'); var iterate = require('../internals/iterate'); // `Set.prototype.map` method // https://github.com/tc39/proposal-collection-methods $({ target: 'Set', proto: true, real: true, forced: IS_PURE }, { map: function map(callbackfn /* , thisArg */) { var set = anObject(this); var iterator = getSetIterator(set); var boundFunction = bind(callbackfn, arguments.length > 1 ? arguments[1] : undefined, 3); var newSet = new (speciesConstructor(set, getBuiltIn('Set')))(); var adder = aFunction(newSet.add); iterate(iterator, function (value) { adder.call(newSet, boundFunction(value, value, set)); }, { IS_ITERATOR: true }); return newSet; } }); apollo-server-demo/node_modules/core-js/modules/es.string.fixed.js 0000644 0001750 0000144 00000000657 03560116604 025022 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var createHTML = require('../internals/create-html'); var forcedStringHTMLMethod = require('../internals/string-html-forced'); // `String.prototype.fixed` method // https://tc39.es/ecma262/#sec-string.prototype.fixed $({ target: 'String', proto: true, forced: forcedStringHTMLMethod('fixed') }, { fixed: function fixed() { return createHTML(this, 'tt', '', ''); } }); apollo-server-demo/node_modules/core-js/modules/esnext.typed-array.filter-out.js 0000644 0001750 0000144 00000001660 03560116604 027642 0 ustar andreh users 'use strict'; var ArrayBufferViewCore = require('../internals/array-buffer-view-core'); var $filterOut = require('../internals/array-iteration').filterOut; var speciesConstructor = require('../internals/species-constructor'); var aTypedArray = ArrayBufferViewCore.aTypedArray; var aTypedArrayConstructor = ArrayBufferViewCore.aTypedArrayConstructor; var exportTypedArrayMethod = ArrayBufferViewCore.exportTypedArrayMethod; // `%TypedArray%.prototype.filterOut` method // https://github.com/tc39/proposal-array-filtering exportTypedArrayMethod('filterOut', function filterOut(callbackfn /* , thisArg */) { var list = $filterOut(aTypedArray(this), callbackfn, arguments.length > 1 ? arguments[1] : undefined); var C = speciesConstructor(this, this.constructor); var index = 0; var length = list.length; var result = new (aTypedArrayConstructor(C))(length); while (length > index) result[index] = list[index++]; return result; }); apollo-server-demo/node_modules/core-js/modules/web.url-search-params.js 0000644 0001750 0000144 00000027145 03560116604 026113 0 ustar andreh users 'use strict'; // TODO: in core-js@4, move /modules/ dependencies to public entries for better optimization by tools like `preset-env` require('../modules/es.array.iterator'); var $ = require('../internals/export'); var getBuiltIn = require('../internals/get-built-in'); var USE_NATIVE_URL = require('../internals/native-url'); var redefine = require('../internals/redefine'); var redefineAll = require('../internals/redefine-all'); var setToStringTag = require('../internals/set-to-string-tag'); var createIteratorConstructor = require('../internals/create-iterator-constructor'); var InternalStateModule = require('../internals/internal-state'); var anInstance = require('../internals/an-instance'); var hasOwn = require('../internals/has'); var bind = require('../internals/function-bind-context'); var classof = require('../internals/classof'); var anObject = require('../internals/an-object'); var isObject = require('../internals/is-object'); var create = require('../internals/object-create'); var createPropertyDescriptor = require('../internals/create-property-descriptor'); var getIterator = require('../internals/get-iterator'); var getIteratorMethod = require('../internals/get-iterator-method'); var wellKnownSymbol = require('../internals/well-known-symbol'); var $fetch = getBuiltIn('fetch'); var Headers = getBuiltIn('Headers'); var ITERATOR = wellKnownSymbol('iterator'); var URL_SEARCH_PARAMS = 'URLSearchParams'; var URL_SEARCH_PARAMS_ITERATOR = URL_SEARCH_PARAMS + 'Iterator'; var setInternalState = InternalStateModule.set; var getInternalParamsState = InternalStateModule.getterFor(URL_SEARCH_PARAMS); var getInternalIteratorState = InternalStateModule.getterFor(URL_SEARCH_PARAMS_ITERATOR); var plus = /\+/g; var sequences = Array(4); var percentSequence = function (bytes) { return sequences[bytes - 1] || (sequences[bytes - 1] = RegExp('((?:%[\\da-f]{2}){' + bytes + '})', 'gi')); }; var percentDecode = function (sequence) { try { return decodeURIComponent(sequence); } catch (error) { return sequence; } }; var deserialize = function (it) { var result = it.replace(plus, ' '); var bytes = 4; try { return decodeURIComponent(result); } catch (error) { while (bytes) { result = result.replace(percentSequence(bytes--), percentDecode); } return result; } }; var find = /[!'()~]|%20/g; var replace = { '!': '%21', "'": '%27', '(': '%28', ')': '%29', '~': '%7E', '%20': '+' }; var replacer = function (match) { return replace[match]; }; var serialize = function (it) { return encodeURIComponent(it).replace(find, replacer); }; var parseSearchParams = function (result, query) { if (query) { var attributes = query.split('&'); var index = 0; var attribute, entry; while (index < attributes.length) { attribute = attributes[index++]; if (attribute.length) { entry = attribute.split('='); result.push({ key: deserialize(entry.shift()), value: deserialize(entry.join('=')) }); } } } }; var updateSearchParams = function (query) { this.entries.length = 0; parseSearchParams(this.entries, query); }; var validateArgumentsLength = function (passed, required) { if (passed < required) throw TypeError('Not enough arguments'); }; var URLSearchParamsIterator = createIteratorConstructor(function Iterator(params, kind) { setInternalState(this, { type: URL_SEARCH_PARAMS_ITERATOR, iterator: getIterator(getInternalParamsState(params).entries), kind: kind }); }, 'Iterator', function next() { var state = getInternalIteratorState(this); var kind = state.kind; var step = state.iterator.next(); var entry = step.value; if (!step.done) { step.value = kind === 'keys' ? entry.key : kind === 'values' ? entry.value : [entry.key, entry.value]; } return step; }); // `URLSearchParams` constructor // https://url.spec.whatwg.org/#interface-urlsearchparams var URLSearchParamsConstructor = function URLSearchParams(/* init */) { anInstance(this, URLSearchParamsConstructor, URL_SEARCH_PARAMS); var init = arguments.length > 0 ? arguments[0] : undefined; var that = this; var entries = []; var iteratorMethod, iterator, next, step, entryIterator, entryNext, first, second, key; setInternalState(that, { type: URL_SEARCH_PARAMS, entries: entries, updateURL: function () { /* empty */ }, updateSearchParams: updateSearchParams }); if (init !== undefined) { if (isObject(init)) { iteratorMethod = getIteratorMethod(init); if (typeof iteratorMethod === 'function') { iterator = iteratorMethod.call(init); next = iterator.next; while (!(step = next.call(iterator)).done) { entryIterator = getIterator(anObject(step.value)); entryNext = entryIterator.next; if ( (first = entryNext.call(entryIterator)).done || (second = entryNext.call(entryIterator)).done || !entryNext.call(entryIterator).done ) throw TypeError('Expected sequence with length 2'); entries.push({ key: first.value + '', value: second.value + '' }); } } else for (key in init) if (hasOwn(init, key)) entries.push({ key: key, value: init[key] + '' }); } else { parseSearchParams(entries, typeof init === 'string' ? init.charAt(0) === '?' ? init.slice(1) : init : init + ''); } } }; var URLSearchParamsPrototype = URLSearchParamsConstructor.prototype; redefineAll(URLSearchParamsPrototype, { // `URLSearchParams.prototype.append` method // https://url.spec.whatwg.org/#dom-urlsearchparams-append append: function append(name, value) { validateArgumentsLength(arguments.length, 2); var state = getInternalParamsState(this); state.entries.push({ key: name + '', value: value + '' }); state.updateURL(); }, // `URLSearchParams.prototype.delete` method // https://url.spec.whatwg.org/#dom-urlsearchparams-delete 'delete': function (name) { validateArgumentsLength(arguments.length, 1); var state = getInternalParamsState(this); var entries = state.entries; var key = name + ''; var index = 0; while (index < entries.length) { if (entries[index].key === key) entries.splice(index, 1); else index++; } state.updateURL(); }, // `URLSearchParams.prototype.get` method // https://url.spec.whatwg.org/#dom-urlsearchparams-get get: function get(name) { validateArgumentsLength(arguments.length, 1); var entries = getInternalParamsState(this).entries; var key = name + ''; var index = 0; for (; index < entries.length; index++) { if (entries[index].key === key) return entries[index].value; } return null; }, // `URLSearchParams.prototype.getAll` method // https://url.spec.whatwg.org/#dom-urlsearchparams-getall getAll: function getAll(name) { validateArgumentsLength(arguments.length, 1); var entries = getInternalParamsState(this).entries; var key = name + ''; var result = []; var index = 0; for (; index < entries.length; index++) { if (entries[index].key === key) result.push(entries[index].value); } return result; }, // `URLSearchParams.prototype.has` method // https://url.spec.whatwg.org/#dom-urlsearchparams-has has: function has(name) { validateArgumentsLength(arguments.length, 1); var entries = getInternalParamsState(this).entries; var key = name + ''; var index = 0; while (index < entries.length) { if (entries[index++].key === key) return true; } return false; }, // `URLSearchParams.prototype.set` method // https://url.spec.whatwg.org/#dom-urlsearchparams-set set: function set(name, value) { validateArgumentsLength(arguments.length, 1); var state = getInternalParamsState(this); var entries = state.entries; var found = false; var key = name + ''; var val = value + ''; var index = 0; var entry; for (; index < entries.length; index++) { entry = entries[index]; if (entry.key === key) { if (found) entries.splice(index--, 1); else { found = true; entry.value = val; } } } if (!found) entries.push({ key: key, value: val }); state.updateURL(); }, // `URLSearchParams.prototype.sort` method // https://url.spec.whatwg.org/#dom-urlsearchparams-sort sort: function sort() { var state = getInternalParamsState(this); var entries = state.entries; // Array#sort is not stable in some engines var slice = entries.slice(); var entry, entriesIndex, sliceIndex; entries.length = 0; for (sliceIndex = 0; sliceIndex < slice.length; sliceIndex++) { entry = slice[sliceIndex]; for (entriesIndex = 0; entriesIndex < sliceIndex; entriesIndex++) { if (entries[entriesIndex].key > entry.key) { entries.splice(entriesIndex, 0, entry); break; } } if (entriesIndex === sliceIndex) entries.push(entry); } state.updateURL(); }, // `URLSearchParams.prototype.forEach` method forEach: function forEach(callback /* , thisArg */) { var entries = getInternalParamsState(this).entries; var boundFunction = bind(callback, arguments.length > 1 ? arguments[1] : undefined, 3); var index = 0; var entry; while (index < entries.length) { entry = entries[index++]; boundFunction(entry.value, entry.key, this); } }, // `URLSearchParams.prototype.keys` method keys: function keys() { return new URLSearchParamsIterator(this, 'keys'); }, // `URLSearchParams.prototype.values` method values: function values() { return new URLSearchParamsIterator(this, 'values'); }, // `URLSearchParams.prototype.entries` method entries: function entries() { return new URLSearchParamsIterator(this, 'entries'); } }, { enumerable: true }); // `URLSearchParams.prototype[@@iterator]` method redefine(URLSearchParamsPrototype, ITERATOR, URLSearchParamsPrototype.entries); // `URLSearchParams.prototype.toString` method // https://url.spec.whatwg.org/#urlsearchparams-stringification-behavior redefine(URLSearchParamsPrototype, 'toString', function toString() { var entries = getInternalParamsState(this).entries; var result = []; var index = 0; var entry; while (index < entries.length) { entry = entries[index++]; result.push(serialize(entry.key) + '=' + serialize(entry.value)); } return result.join('&'); }, { enumerable: true }); setToStringTag(URLSearchParamsConstructor, URL_SEARCH_PARAMS); $({ global: true, forced: !USE_NATIVE_URL }, { URLSearchParams: URLSearchParamsConstructor }); // Wrap `fetch` for correct work with polyfilled `URLSearchParams` // https://github.com/zloirock/core-js/issues/674 if (!USE_NATIVE_URL && typeof $fetch == 'function' && typeof Headers == 'function') { $({ global: true, enumerable: true, forced: true }, { fetch: function fetch(input /* , init */) { var args = [input]; var init, body, headers; if (arguments.length > 1) { init = arguments[1]; if (isObject(init)) { body = init.body; if (classof(body) === URL_SEARCH_PARAMS) { headers = init.headers ? new Headers(init.headers) : new Headers(); if (!headers.has('content-type')) { headers.set('content-type', 'application/x-www-form-urlencoded;charset=UTF-8'); } init = create(init, { body: createPropertyDescriptor(0, String(body)), headers: createPropertyDescriptor(0, headers) }); } } args.push(init); } return $fetch.apply(this, args); } }); } module.exports = { URLSearchParams: URLSearchParamsConstructor, getState: getInternalParamsState }; apollo-server-demo/node_modules/core-js/modules/es.typed-array.uint32-array.js 0000644 0001750 0000144 00000000520 03560116604 027103 0 ustar andreh users var createTypedArrayConstructor = require('../internals/typed-array-constructor'); // `Uint32Array` constructor // https://tc39.es/ecma262/#sec-typedarray-objects createTypedArrayConstructor('Uint32', function (init) { return function Uint32Array(data, byteOffset, length) { return init(this, data, byteOffset, length); }; }); apollo-server-demo/node_modules/core-js/modules/esnext.map.every.js 0000644 0001750 0000144 00000001574 03560116604 025222 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var anObject = require('../internals/an-object'); var bind = require('../internals/function-bind-context'); var getMapIterator = require('../internals/get-map-iterator'); var iterate = require('../internals/iterate'); // `Map.prototype.every` method // https://github.com/tc39/proposal-collection-methods $({ target: 'Map', proto: true, real: true, forced: IS_PURE }, { every: function every(callbackfn /* , thisArg */) { var map = anObject(this); var iterator = getMapIterator(map); var boundFunction = bind(callbackfn, arguments.length > 1 ? arguments[1] : undefined, 3); return !iterate(iterator, function (key, value, stop) { if (!boundFunction(value, key, map)) return stop(); }, { AS_ENTRIES: true, IS_ITERATOR: true, INTERRUPTED: true }).stopped; } }); apollo-server-demo/node_modules/core-js/modules/es.string.raw.js 0000644 0001750 0000144 00000001240 03560116604 024501 0 ustar andreh users var $ = require('../internals/export'); var toIndexedObject = require('../internals/to-indexed-object'); var toLength = require('../internals/to-length'); // `String.raw` method // https://tc39.es/ecma262/#sec-string.raw $({ target: 'String', stat: true }, { raw: function raw(template) { var rawTemplate = toIndexedObject(template.raw); var literalSegments = toLength(rawTemplate.length); var argumentsLength = arguments.length; var elements = []; var i = 0; while (literalSegments > i) { elements.push(String(rawTemplate[i++])); if (i < argumentsLength) elements.push(String(arguments[i])); } return elements.join(''); } }); apollo-server-demo/node_modules/core-js/modules/es.object.is-sealed.js 0000644 0001750 0000144 00000000773 03560116604 025530 0 ustar andreh users var $ = require('../internals/export'); var fails = require('../internals/fails'); var isObject = require('../internals/is-object'); var nativeIsSealed = Object.isSealed; var FAILS_ON_PRIMITIVES = fails(function () { nativeIsSealed(1); }); // `Object.isSealed` method // https://tc39.es/ecma262/#sec-object.issealed $({ target: 'Object', stat: true, forced: FAILS_ON_PRIMITIVES }, { isSealed: function isSealed(it) { return isObject(it) ? nativeIsSealed ? nativeIsSealed(it) : false : true; } }); apollo-server-demo/node_modules/core-js/modules/esnext.set.delete-all.js 0000644 0001750 0000144 00000000677 03560116604 026121 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var collectionDeleteAll = require('../internals/collection-delete-all'); // `Set.prototype.deleteAll` method // https://github.com/tc39/proposal-collection-methods $({ target: 'Set', proto: true, real: true, forced: IS_PURE }, { deleteAll: function deleteAll(/* ...elements */) { return collectionDeleteAll.apply(this, arguments); } }); apollo-server-demo/node_modules/core-js/modules/web.url.js 0000644 0001750 0000144 00000077631 03560116604 023374 0 ustar andreh users 'use strict'; // TODO: in core-js@4, move /modules/ dependencies to public entries for better optimization by tools like `preset-env` require('../modules/es.string.iterator'); var $ = require('../internals/export'); var DESCRIPTORS = require('../internals/descriptors'); var USE_NATIVE_URL = require('../internals/native-url'); var global = require('../internals/global'); var defineProperties = require('../internals/object-define-properties'); var redefine = require('../internals/redefine'); var anInstance = require('../internals/an-instance'); var has = require('../internals/has'); var assign = require('../internals/object-assign'); var arrayFrom = require('../internals/array-from'); var codeAt = require('../internals/string-multibyte').codeAt; var toASCII = require('../internals/string-punycode-to-ascii'); var setToStringTag = require('../internals/set-to-string-tag'); var URLSearchParamsModule = require('../modules/web.url-search-params'); var InternalStateModule = require('../internals/internal-state'); var NativeURL = global.URL; var URLSearchParams = URLSearchParamsModule.URLSearchParams; var getInternalSearchParamsState = URLSearchParamsModule.getState; var setInternalState = InternalStateModule.set; var getInternalURLState = InternalStateModule.getterFor('URL'); var floor = Math.floor; var pow = Math.pow; var INVALID_AUTHORITY = 'Invalid authority'; var INVALID_SCHEME = 'Invalid scheme'; var INVALID_HOST = 'Invalid host'; var INVALID_PORT = 'Invalid port'; var ALPHA = /[A-Za-z]/; var ALPHANUMERIC = /[\d+-.A-Za-z]/; var DIGIT = /\d/; var HEX_START = /^(0x|0X)/; var OCT = /^[0-7]+$/; var DEC = /^\d+$/; var HEX = /^[\dA-Fa-f]+$/; // eslint-disable-next-line no-control-regex var FORBIDDEN_HOST_CODE_POINT = /[\u0000\u0009\u000A\u000D #%/:?@[\\]]/; // eslint-disable-next-line no-control-regex var FORBIDDEN_HOST_CODE_POINT_EXCLUDING_PERCENT = /[\u0000\u0009\u000A\u000D #/:?@[\\]]/; // eslint-disable-next-line no-control-regex var LEADING_AND_TRAILING_C0_CONTROL_OR_SPACE = /^[\u0000-\u001F ]+|[\u0000-\u001F ]+$/g; // eslint-disable-next-line no-control-regex var TAB_AND_NEW_LINE = /[\u0009\u000A\u000D]/g; var EOF; var parseHost = function (url, input) { var result, codePoints, index; if (input.charAt(0) == '[') { if (input.charAt(input.length - 1) != ']') return INVALID_HOST; result = parseIPv6(input.slice(1, -1)); if (!result) return INVALID_HOST; url.host = result; // opaque host } else if (!isSpecial(url)) { if (FORBIDDEN_HOST_CODE_POINT_EXCLUDING_PERCENT.test(input)) return INVALID_HOST; result = ''; codePoints = arrayFrom(input); for (index = 0; index < codePoints.length; index++) { result += percentEncode(codePoints[index], C0ControlPercentEncodeSet); } url.host = result; } else { input = toASCII(input); if (FORBIDDEN_HOST_CODE_POINT.test(input)) return INVALID_HOST; result = parseIPv4(input); if (result === null) return INVALID_HOST; url.host = result; } }; var parseIPv4 = function (input) { var parts = input.split('.'); var partsLength, numbers, index, part, radix, number, ipv4; if (parts.length && parts[parts.length - 1] == '') { parts.pop(); } partsLength = parts.length; if (partsLength > 4) return input; numbers = []; for (index = 0; index < partsLength; index++) { part = parts[index]; if (part == '') return input; radix = 10; if (part.length > 1 && part.charAt(0) == '0') { radix = HEX_START.test(part) ? 16 : 8; part = part.slice(radix == 8 ? 1 : 2); } if (part === '') { number = 0; } else { if (!(radix == 10 ? DEC : radix == 8 ? OCT : HEX).test(part)) return input; number = parseInt(part, radix); } numbers.push(number); } for (index = 0; index < partsLength; index++) { number = numbers[index]; if (index == partsLength - 1) { if (number >= pow(256, 5 - partsLength)) return null; } else if (number > 255) return null; } ipv4 = numbers.pop(); for (index = 0; index < numbers.length; index++) { ipv4 += numbers[index] * pow(256, 3 - index); } return ipv4; }; // eslint-disable-next-line max-statements var parseIPv6 = function (input) { var address = [0, 0, 0, 0, 0, 0, 0, 0]; var pieceIndex = 0; var compress = null; var pointer = 0; var value, length, numbersSeen, ipv4Piece, number, swaps, swap; var char = function () { return input.charAt(pointer); }; if (char() == ':') { if (input.charAt(1) != ':') return; pointer += 2; pieceIndex++; compress = pieceIndex; } while (char()) { if (pieceIndex == 8) return; if (char() == ':') { if (compress !== null) return; pointer++; pieceIndex++; compress = pieceIndex; continue; } value = length = 0; while (length < 4 && HEX.test(char())) { value = value * 16 + parseInt(char(), 16); pointer++; length++; } if (char() == '.') { if (length == 0) return; pointer -= length; if (pieceIndex > 6) return; numbersSeen = 0; while (char()) { ipv4Piece = null; if (numbersSeen > 0) { if (char() == '.' && numbersSeen < 4) pointer++; else return; } if (!DIGIT.test(char())) return; while (DIGIT.test(char())) { number = parseInt(char(), 10); if (ipv4Piece === null) ipv4Piece = number; else if (ipv4Piece == 0) return; else ipv4Piece = ipv4Piece * 10 + number; if (ipv4Piece > 255) return; pointer++; } address[pieceIndex] = address[pieceIndex] * 256 + ipv4Piece; numbersSeen++; if (numbersSeen == 2 || numbersSeen == 4) pieceIndex++; } if (numbersSeen != 4) return; break; } else if (char() == ':') { pointer++; if (!char()) return; } else if (char()) return; address[pieceIndex++] = value; } if (compress !== null) { swaps = pieceIndex - compress; pieceIndex = 7; while (pieceIndex != 0 && swaps > 0) { swap = address[pieceIndex]; address[pieceIndex--] = address[compress + swaps - 1]; address[compress + --swaps] = swap; } } else if (pieceIndex != 8) return; return address; }; var findLongestZeroSequence = function (ipv6) { var maxIndex = null; var maxLength = 1; var currStart = null; var currLength = 0; var index = 0; for (; index < 8; index++) { if (ipv6[index] !== 0) { if (currLength > maxLength) { maxIndex = currStart; maxLength = currLength; } currStart = null; currLength = 0; } else { if (currStart === null) currStart = index; ++currLength; } } if (currLength > maxLength) { maxIndex = currStart; maxLength = currLength; } return maxIndex; }; var serializeHost = function (host) { var result, index, compress, ignore0; // ipv4 if (typeof host == 'number') { result = []; for (index = 0; index < 4; index++) { result.unshift(host % 256); host = floor(host / 256); } return result.join('.'); // ipv6 } else if (typeof host == 'object') { result = ''; compress = findLongestZeroSequence(host); for (index = 0; index < 8; index++) { if (ignore0 && host[index] === 0) continue; if (ignore0) ignore0 = false; if (compress === index) { result += index ? ':' : '::'; ignore0 = true; } else { result += host[index].toString(16); if (index < 7) result += ':'; } } return '[' + result + ']'; } return host; }; var C0ControlPercentEncodeSet = {}; var fragmentPercentEncodeSet = assign({}, C0ControlPercentEncodeSet, { ' ': 1, '"': 1, '<': 1, '>': 1, '`': 1 }); var pathPercentEncodeSet = assign({}, fragmentPercentEncodeSet, { '#': 1, '?': 1, '{': 1, '}': 1 }); var userinfoPercentEncodeSet = assign({}, pathPercentEncodeSet, { '/': 1, ':': 1, ';': 1, '=': 1, '@': 1, '[': 1, '\\': 1, ']': 1, '^': 1, '|': 1 }); var percentEncode = function (char, set) { var code = codeAt(char, 0); return code > 0x20 && code < 0x7F && !has(set, char) ? char : encodeURIComponent(char); }; var specialSchemes = { ftp: 21, file: null, http: 80, https: 443, ws: 80, wss: 443 }; var isSpecial = function (url) { return has(specialSchemes, url.scheme); }; var includesCredentials = function (url) { return url.username != '' || url.password != ''; }; var cannotHaveUsernamePasswordPort = function (url) { return !url.host || url.cannotBeABaseURL || url.scheme == 'file'; }; var isWindowsDriveLetter = function (string, normalized) { var second; return string.length == 2 && ALPHA.test(string.charAt(0)) && ((second = string.charAt(1)) == ':' || (!normalized && second == '|')); }; var startsWithWindowsDriveLetter = function (string) { var third; return string.length > 1 && isWindowsDriveLetter(string.slice(0, 2)) && ( string.length == 2 || ((third = string.charAt(2)) === '/' || third === '\\' || third === '?' || third === '#') ); }; var shortenURLsPath = function (url) { var path = url.path; var pathSize = path.length; if (pathSize && (url.scheme != 'file' || pathSize != 1 || !isWindowsDriveLetter(path[0], true))) { path.pop(); } }; var isSingleDot = function (segment) { return segment === '.' || segment.toLowerCase() === '%2e'; }; var isDoubleDot = function (segment) { segment = segment.toLowerCase(); return segment === '..' || segment === '%2e.' || segment === '.%2e' || segment === '%2e%2e'; }; // States: var SCHEME_START = {}; var SCHEME = {}; var NO_SCHEME = {}; var SPECIAL_RELATIVE_OR_AUTHORITY = {}; var PATH_OR_AUTHORITY = {}; var RELATIVE = {}; var RELATIVE_SLASH = {}; var SPECIAL_AUTHORITY_SLASHES = {}; var SPECIAL_AUTHORITY_IGNORE_SLASHES = {}; var AUTHORITY = {}; var HOST = {}; var HOSTNAME = {}; var PORT = {}; var FILE = {}; var FILE_SLASH = {}; var FILE_HOST = {}; var PATH_START = {}; var PATH = {}; var CANNOT_BE_A_BASE_URL_PATH = {}; var QUERY = {}; var FRAGMENT = {}; // eslint-disable-next-line max-statements var parseURL = function (url, input, stateOverride, base) { var state = stateOverride || SCHEME_START; var pointer = 0; var buffer = ''; var seenAt = false; var seenBracket = false; var seenPasswordToken = false; var codePoints, char, bufferCodePoints, failure; if (!stateOverride) { url.scheme = ''; url.username = ''; url.password = ''; url.host = null; url.port = null; url.path = []; url.query = null; url.fragment = null; url.cannotBeABaseURL = false; input = input.replace(LEADING_AND_TRAILING_C0_CONTROL_OR_SPACE, ''); } input = input.replace(TAB_AND_NEW_LINE, ''); codePoints = arrayFrom(input); while (pointer <= codePoints.length) { char = codePoints[pointer]; switch (state) { case SCHEME_START: if (char && ALPHA.test(char)) { buffer += char.toLowerCase(); state = SCHEME; } else if (!stateOverride) { state = NO_SCHEME; continue; } else return INVALID_SCHEME; break; case SCHEME: if (char && (ALPHANUMERIC.test(char) || char == '+' || char == '-' || char == '.')) { buffer += char.toLowerCase(); } else if (char == ':') { if (stateOverride && ( (isSpecial(url) != has(specialSchemes, buffer)) || (buffer == 'file' && (includesCredentials(url) || url.port !== null)) || (url.scheme == 'file' && !url.host) )) return; url.scheme = buffer; if (stateOverride) { if (isSpecial(url) && specialSchemes[url.scheme] == url.port) url.port = null; return; } buffer = ''; if (url.scheme == 'file') { state = FILE; } else if (isSpecial(url) && base && base.scheme == url.scheme) { state = SPECIAL_RELATIVE_OR_AUTHORITY; } else if (isSpecial(url)) { state = SPECIAL_AUTHORITY_SLASHES; } else if (codePoints[pointer + 1] == '/') { state = PATH_OR_AUTHORITY; pointer++; } else { url.cannotBeABaseURL = true; url.path.push(''); state = CANNOT_BE_A_BASE_URL_PATH; } } else if (!stateOverride) { buffer = ''; state = NO_SCHEME; pointer = 0; continue; } else return INVALID_SCHEME; break; case NO_SCHEME: if (!base || (base.cannotBeABaseURL && char != '#')) return INVALID_SCHEME; if (base.cannotBeABaseURL && char == '#') { url.scheme = base.scheme; url.path = base.path.slice(); url.query = base.query; url.fragment = ''; url.cannotBeABaseURL = true; state = FRAGMENT; break; } state = base.scheme == 'file' ? FILE : RELATIVE; continue; case SPECIAL_RELATIVE_OR_AUTHORITY: if (char == '/' && codePoints[pointer + 1] == '/') { state = SPECIAL_AUTHORITY_IGNORE_SLASHES; pointer++; } else { state = RELATIVE; continue; } break; case PATH_OR_AUTHORITY: if (char == '/') { state = AUTHORITY; break; } else { state = PATH; continue; } case RELATIVE: url.scheme = base.scheme; if (char == EOF) { url.username = base.username; url.password = base.password; url.host = base.host; url.port = base.port; url.path = base.path.slice(); url.query = base.query; } else if (char == '/' || (char == '\\' && isSpecial(url))) { state = RELATIVE_SLASH; } else if (char == '?') { url.username = base.username; url.password = base.password; url.host = base.host; url.port = base.port; url.path = base.path.slice(); url.query = ''; state = QUERY; } else if (char == '#') { url.username = base.username; url.password = base.password; url.host = base.host; url.port = base.port; url.path = base.path.slice(); url.query = base.query; url.fragment = ''; state = FRAGMENT; } else { url.username = base.username; url.password = base.password; url.host = base.host; url.port = base.port; url.path = base.path.slice(); url.path.pop(); state = PATH; continue; } break; case RELATIVE_SLASH: if (isSpecial(url) && (char == '/' || char == '\\')) { state = SPECIAL_AUTHORITY_IGNORE_SLASHES; } else if (char == '/') { state = AUTHORITY; } else { url.username = base.username; url.password = base.password; url.host = base.host; url.port = base.port; state = PATH; continue; } break; case SPECIAL_AUTHORITY_SLASHES: state = SPECIAL_AUTHORITY_IGNORE_SLASHES; if (char != '/' || buffer.charAt(pointer + 1) != '/') continue; pointer++; break; case SPECIAL_AUTHORITY_IGNORE_SLASHES: if (char != '/' && char != '\\') { state = AUTHORITY; continue; } break; case AUTHORITY: if (char == '@') { if (seenAt) buffer = '%40' + buffer; seenAt = true; bufferCodePoints = arrayFrom(buffer); for (var i = 0; i < bufferCodePoints.length; i++) { var codePoint = bufferCodePoints[i]; if (codePoint == ':' && !seenPasswordToken) { seenPasswordToken = true; continue; } var encodedCodePoints = percentEncode(codePoint, userinfoPercentEncodeSet); if (seenPasswordToken) url.password += encodedCodePoints; else url.username += encodedCodePoints; } buffer = ''; } else if ( char == EOF || char == '/' || char == '?' || char == '#' || (char == '\\' && isSpecial(url)) ) { if (seenAt && buffer == '') return INVALID_AUTHORITY; pointer -= arrayFrom(buffer).length + 1; buffer = ''; state = HOST; } else buffer += char; break; case HOST: case HOSTNAME: if (stateOverride && url.scheme == 'file') { state = FILE_HOST; continue; } else if (char == ':' && !seenBracket) { if (buffer == '') return INVALID_HOST; failure = parseHost(url, buffer); if (failure) return failure; buffer = ''; state = PORT; if (stateOverride == HOSTNAME) return; } else if ( char == EOF || char == '/' || char == '?' || char == '#' || (char == '\\' && isSpecial(url)) ) { if (isSpecial(url) && buffer == '') return INVALID_HOST; if (stateOverride && buffer == '' && (includesCredentials(url) || url.port !== null)) return; failure = parseHost(url, buffer); if (failure) return failure; buffer = ''; state = PATH_START; if (stateOverride) return; continue; } else { if (char == '[') seenBracket = true; else if (char == ']') seenBracket = false; buffer += char; } break; case PORT: if (DIGIT.test(char)) { buffer += char; } else if ( char == EOF || char == '/' || char == '?' || char == '#' || (char == '\\' && isSpecial(url)) || stateOverride ) { if (buffer != '') { var port = parseInt(buffer, 10); if (port > 0xFFFF) return INVALID_PORT; url.port = (isSpecial(url) && port === specialSchemes[url.scheme]) ? null : port; buffer = ''; } if (stateOverride) return; state = PATH_START; continue; } else return INVALID_PORT; break; case FILE: url.scheme = 'file'; if (char == '/' || char == '\\') state = FILE_SLASH; else if (base && base.scheme == 'file') { if (char == EOF) { url.host = base.host; url.path = base.path.slice(); url.query = base.query; } else if (char == '?') { url.host = base.host; url.path = base.path.slice(); url.query = ''; state = QUERY; } else if (char == '#') { url.host = base.host; url.path = base.path.slice(); url.query = base.query; url.fragment = ''; state = FRAGMENT; } else { if (!startsWithWindowsDriveLetter(codePoints.slice(pointer).join(''))) { url.host = base.host; url.path = base.path.slice(); shortenURLsPath(url); } state = PATH; continue; } } else { state = PATH; continue; } break; case FILE_SLASH: if (char == '/' || char == '\\') { state = FILE_HOST; break; } if (base && base.scheme == 'file' && !startsWithWindowsDriveLetter(codePoints.slice(pointer).join(''))) { if (isWindowsDriveLetter(base.path[0], true)) url.path.push(base.path[0]); else url.host = base.host; } state = PATH; continue; case FILE_HOST: if (char == EOF || char == '/' || char == '\\' || char == '?' || char == '#') { if (!stateOverride && isWindowsDriveLetter(buffer)) { state = PATH; } else if (buffer == '') { url.host = ''; if (stateOverride) return; state = PATH_START; } else { failure = parseHost(url, buffer); if (failure) return failure; if (url.host == 'localhost') url.host = ''; if (stateOverride) return; buffer = ''; state = PATH_START; } continue; } else buffer += char; break; case PATH_START: if (isSpecial(url)) { state = PATH; if (char != '/' && char != '\\') continue; } else if (!stateOverride && char == '?') { url.query = ''; state = QUERY; } else if (!stateOverride && char == '#') { url.fragment = ''; state = FRAGMENT; } else if (char != EOF) { state = PATH; if (char != '/') continue; } break; case PATH: if ( char == EOF || char == '/' || (char == '\\' && isSpecial(url)) || (!stateOverride && (char == '?' || char == '#')) ) { if (isDoubleDot(buffer)) { shortenURLsPath(url); if (char != '/' && !(char == '\\' && isSpecial(url))) { url.path.push(''); } } else if (isSingleDot(buffer)) { if (char != '/' && !(char == '\\' && isSpecial(url))) { url.path.push(''); } } else { if (url.scheme == 'file' && !url.path.length && isWindowsDriveLetter(buffer)) { if (url.host) url.host = ''; buffer = buffer.charAt(0) + ':'; // normalize windows drive letter } url.path.push(buffer); } buffer = ''; if (url.scheme == 'file' && (char == EOF || char == '?' || char == '#')) { while (url.path.length > 1 && url.path[0] === '') { url.path.shift(); } } if (char == '?') { url.query = ''; state = QUERY; } else if (char == '#') { url.fragment = ''; state = FRAGMENT; } } else { buffer += percentEncode(char, pathPercentEncodeSet); } break; case CANNOT_BE_A_BASE_URL_PATH: if (char == '?') { url.query = ''; state = QUERY; } else if (char == '#') { url.fragment = ''; state = FRAGMENT; } else if (char != EOF) { url.path[0] += percentEncode(char, C0ControlPercentEncodeSet); } break; case QUERY: if (!stateOverride && char == '#') { url.fragment = ''; state = FRAGMENT; } else if (char != EOF) { if (char == "'" && isSpecial(url)) url.query += '%27'; else if (char == '#') url.query += '%23'; else url.query += percentEncode(char, C0ControlPercentEncodeSet); } break; case FRAGMENT: if (char != EOF) url.fragment += percentEncode(char, fragmentPercentEncodeSet); break; } pointer++; } }; // `URL` constructor // https://url.spec.whatwg.org/#url-class var URLConstructor = function URL(url /* , base */) { var that = anInstance(this, URLConstructor, 'URL'); var base = arguments.length > 1 ? arguments[1] : undefined; var urlString = String(url); var state = setInternalState(that, { type: 'URL' }); var baseState, failure; if (base !== undefined) { if (base instanceof URLConstructor) baseState = getInternalURLState(base); else { failure = parseURL(baseState = {}, String(base)); if (failure) throw TypeError(failure); } } failure = parseURL(state, urlString, null, baseState); if (failure) throw TypeError(failure); var searchParams = state.searchParams = new URLSearchParams(); var searchParamsState = getInternalSearchParamsState(searchParams); searchParamsState.updateSearchParams(state.query); searchParamsState.updateURL = function () { state.query = String(searchParams) || null; }; if (!DESCRIPTORS) { that.href = serializeURL.call(that); that.origin = getOrigin.call(that); that.protocol = getProtocol.call(that); that.username = getUsername.call(that); that.password = getPassword.call(that); that.host = getHost.call(that); that.hostname = getHostname.call(that); that.port = getPort.call(that); that.pathname = getPathname.call(that); that.search = getSearch.call(that); that.searchParams = getSearchParams.call(that); that.hash = getHash.call(that); } }; var URLPrototype = URLConstructor.prototype; var serializeURL = function () { var url = getInternalURLState(this); var scheme = url.scheme; var username = url.username; var password = url.password; var host = url.host; var port = url.port; var path = url.path; var query = url.query; var fragment = url.fragment; var output = scheme + ':'; if (host !== null) { output += '//'; if (includesCredentials(url)) { output += username + (password ? ':' + password : '') + '@'; } output += serializeHost(host); if (port !== null) output += ':' + port; } else if (scheme == 'file') output += '//'; output += url.cannotBeABaseURL ? path[0] : path.length ? '/' + path.join('/') : ''; if (query !== null) output += '?' + query; if (fragment !== null) output += '#' + fragment; return output; }; var getOrigin = function () { var url = getInternalURLState(this); var scheme = url.scheme; var port = url.port; if (scheme == 'blob') try { return new URL(scheme.path[0]).origin; } catch (error) { return 'null'; } if (scheme == 'file' || !isSpecial(url)) return 'null'; return scheme + '://' + serializeHost(url.host) + (port !== null ? ':' + port : ''); }; var getProtocol = function () { return getInternalURLState(this).scheme + ':'; }; var getUsername = function () { return getInternalURLState(this).username; }; var getPassword = function () { return getInternalURLState(this).password; }; var getHost = function () { var url = getInternalURLState(this); var host = url.host; var port = url.port; return host === null ? '' : port === null ? serializeHost(host) : serializeHost(host) + ':' + port; }; var getHostname = function () { var host = getInternalURLState(this).host; return host === null ? '' : serializeHost(host); }; var getPort = function () { var port = getInternalURLState(this).port; return port === null ? '' : String(port); }; var getPathname = function () { var url = getInternalURLState(this); var path = url.path; return url.cannotBeABaseURL ? path[0] : path.length ? '/' + path.join('/') : ''; }; var getSearch = function () { var query = getInternalURLState(this).query; return query ? '?' + query : ''; }; var getSearchParams = function () { return getInternalURLState(this).searchParams; }; var getHash = function () { var fragment = getInternalURLState(this).fragment; return fragment ? '#' + fragment : ''; }; var accessorDescriptor = function (getter, setter) { return { get: getter, set: setter, configurable: true, enumerable: true }; }; if (DESCRIPTORS) { defineProperties(URLPrototype, { // `URL.prototype.href` accessors pair // https://url.spec.whatwg.org/#dom-url-href href: accessorDescriptor(serializeURL, function (href) { var url = getInternalURLState(this); var urlString = String(href); var failure = parseURL(url, urlString); if (failure) throw TypeError(failure); getInternalSearchParamsState(url.searchParams).updateSearchParams(url.query); }), // `URL.prototype.origin` getter // https://url.spec.whatwg.org/#dom-url-origin origin: accessorDescriptor(getOrigin), // `URL.prototype.protocol` accessors pair // https://url.spec.whatwg.org/#dom-url-protocol protocol: accessorDescriptor(getProtocol, function (protocol) { var url = getInternalURLState(this); parseURL(url, String(protocol) + ':', SCHEME_START); }), // `URL.prototype.username` accessors pair // https://url.spec.whatwg.org/#dom-url-username username: accessorDescriptor(getUsername, function (username) { var url = getInternalURLState(this); var codePoints = arrayFrom(String(username)); if (cannotHaveUsernamePasswordPort(url)) return; url.username = ''; for (var i = 0; i < codePoints.length; i++) { url.username += percentEncode(codePoints[i], userinfoPercentEncodeSet); } }), // `URL.prototype.password` accessors pair // https://url.spec.whatwg.org/#dom-url-password password: accessorDescriptor(getPassword, function (password) { var url = getInternalURLState(this); var codePoints = arrayFrom(String(password)); if (cannotHaveUsernamePasswordPort(url)) return; url.password = ''; for (var i = 0; i < codePoints.length; i++) { url.password += percentEncode(codePoints[i], userinfoPercentEncodeSet); } }), // `URL.prototype.host` accessors pair // https://url.spec.whatwg.org/#dom-url-host host: accessorDescriptor(getHost, function (host) { var url = getInternalURLState(this); if (url.cannotBeABaseURL) return; parseURL(url, String(host), HOST); }), // `URL.prototype.hostname` accessors pair // https://url.spec.whatwg.org/#dom-url-hostname hostname: accessorDescriptor(getHostname, function (hostname) { var url = getInternalURLState(this); if (url.cannotBeABaseURL) return; parseURL(url, String(hostname), HOSTNAME); }), // `URL.prototype.port` accessors pair // https://url.spec.whatwg.org/#dom-url-port port: accessorDescriptor(getPort, function (port) { var url = getInternalURLState(this); if (cannotHaveUsernamePasswordPort(url)) return; port = String(port); if (port == '') url.port = null; else parseURL(url, port, PORT); }), // `URL.prototype.pathname` accessors pair // https://url.spec.whatwg.org/#dom-url-pathname pathname: accessorDescriptor(getPathname, function (pathname) { var url = getInternalURLState(this); if (url.cannotBeABaseURL) return; url.path = []; parseURL(url, pathname + '', PATH_START); }), // `URL.prototype.search` accessors pair // https://url.spec.whatwg.org/#dom-url-search search: accessorDescriptor(getSearch, function (search) { var url = getInternalURLState(this); search = String(search); if (search == '') { url.query = null; } else { if ('?' == search.charAt(0)) search = search.slice(1); url.query = ''; parseURL(url, search, QUERY); } getInternalSearchParamsState(url.searchParams).updateSearchParams(url.query); }), // `URL.prototype.searchParams` getter // https://url.spec.whatwg.org/#dom-url-searchparams searchParams: accessorDescriptor(getSearchParams), // `URL.prototype.hash` accessors pair // https://url.spec.whatwg.org/#dom-url-hash hash: accessorDescriptor(getHash, function (hash) { var url = getInternalURLState(this); hash = String(hash); if (hash == '') { url.fragment = null; return; } if ('#' == hash.charAt(0)) hash = hash.slice(1); url.fragment = ''; parseURL(url, hash, FRAGMENT); }) }); } // `URL.prototype.toJSON` method // https://url.spec.whatwg.org/#dom-url-tojson redefine(URLPrototype, 'toJSON', function toJSON() { return serializeURL.call(this); }, { enumerable: true }); // `URL.prototype.toString` method // https://url.spec.whatwg.org/#URL-stringification-behavior redefine(URLPrototype, 'toString', function toString() { return serializeURL.call(this); }, { enumerable: true }); if (NativeURL) { var nativeCreateObjectURL = NativeURL.createObjectURL; var nativeRevokeObjectURL = NativeURL.revokeObjectURL; // `URL.createObjectURL` method // https://developer.mozilla.org/en-US/docs/Web/API/URL/createObjectURL // eslint-disable-next-line no-unused-vars if (nativeCreateObjectURL) redefine(URLConstructor, 'createObjectURL', function createObjectURL(blob) { return nativeCreateObjectURL.apply(NativeURL, arguments); }); // `URL.revokeObjectURL` method // https://developer.mozilla.org/en-US/docs/Web/API/URL/revokeObjectURL // eslint-disable-next-line no-unused-vars if (nativeRevokeObjectURL) redefine(URLConstructor, 'revokeObjectURL', function revokeObjectURL(url) { return nativeRevokeObjectURL.apply(NativeURL, arguments); }); } setToStringTag(URLConstructor, 'URL'); $({ global: true, forced: !USE_NATIVE_URL, sham: !DESCRIPTORS }, { URL: URLConstructor }); apollo-server-demo/node_modules/core-js/modules/es.object.entries.js 0000644 0001750 0000144 00000000432 03560116604 025323 0 ustar andreh users var $ = require('../internals/export'); var $entries = require('../internals/object-to-array').entries; // `Object.entries` method // https://tc39.es/ecma262/#sec-object.entries $({ target: 'Object', stat: true }, { entries: function entries(O) { return $entries(O); } }); apollo-server-demo/node_modules/core-js/modules/es.date.now.js 0000644 0001750 0000144 00000000314 03560116604 024123 0 ustar andreh users var $ = require('../internals/export'); // `Date.now` method // https://tc39.es/ecma262/#sec-date.now $({ target: 'Date', stat: true }, { now: function now() { return new Date().getTime(); } }); apollo-server-demo/node_modules/core-js/modules/esnext.typed-array.at.js 0000644 0001750 0000144 00000001257 03560116604 026156 0 ustar andreh users 'use strict'; var ArrayBufferViewCore = require('../internals/array-buffer-view-core'); var toLength = require('../internals/to-length'); var toInteger = require('../internals/to-integer'); var aTypedArray = ArrayBufferViewCore.aTypedArray; var exportTypedArrayMethod = ArrayBufferViewCore.exportTypedArrayMethod; // `%TypedArray%.prototype.at` method // https://github.com/tc39/proposal-relative-indexing-method exportTypedArrayMethod('at', function at(index) { var O = aTypedArray(this); var len = toLength(O.length); var relativeIndex = toInteger(index); var k = relativeIndex >= 0 ? relativeIndex : len + relativeIndex; return (k < 0 || k >= len) ? undefined : O[k]; }); apollo-server-demo/node_modules/core-js/modules/es.aggregate-error.js 0000644 0001750 0000144 00000002610 03560116604 025462 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var getPrototypeOf = require('../internals/object-get-prototype-of'); var setPrototypeOf = require('../internals/object-set-prototype-of'); var create = require('../internals/object-create'); var createNonEnumerableProperty = require('../internals/create-non-enumerable-property'); var createPropertyDescriptor = require('../internals/create-property-descriptor'); var iterate = require('../internals/iterate'); var $AggregateError = function AggregateError(errors, message) { var that = this; if (!(that instanceof $AggregateError)) return new $AggregateError(errors, message); if (setPrototypeOf) { // eslint-disable-next-line unicorn/error-message that = setPrototypeOf(new Error(undefined), getPrototypeOf(that)); } if (message !== undefined) createNonEnumerableProperty(that, 'message', String(message)); var errorsArray = []; iterate(errors, errorsArray.push, { that: errorsArray }); createNonEnumerableProperty(that, 'errors', errorsArray); return that; }; $AggregateError.prototype = create(Error.prototype, { constructor: createPropertyDescriptor(5, $AggregateError), message: createPropertyDescriptor(5, ''), name: createPropertyDescriptor(5, 'AggregateError') }); // `AggregateError` constructor // https://tc39.es/ecma262/#sec-aggregate-error-constructor $({ global: true }, { AggregateError: $AggregateError }); apollo-server-demo/node_modules/core-js/modules/esnext.async-iterator.find.js 0000644 0001750 0000144 00000000451 03560116604 027170 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var $find = require('../internals/async-iterator-iteration').find; $({ target: 'AsyncIterator', proto: true, real: true }, { find: function find(fn) { return $find(this, fn); } }); apollo-server-demo/node_modules/core-js/modules/es.parse-float.js 0000644 0001750 0000144 00000000456 03560116604 024630 0 ustar andreh users var $ = require('../internals/export'); var parseFloatImplementation = require('../internals/number-parse-float'); // `parseFloat` method // https://tc39.es/ecma262/#sec-parsefloat-string $({ global: true, forced: parseFloat != parseFloatImplementation }, { parseFloat: parseFloatImplementation }); apollo-server-demo/node_modules/core-js/modules/esnext.async-iterator.reduce.js 0000644 0001750 0000144 00000003043 03560116604 027517 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var aFunction = require('../internals/a-function'); var anObject = require('../internals/an-object'); var getBuiltIn = require('../internals/get-built-in'); var Promise = getBuiltIn('Promise'); $({ target: 'AsyncIterator', proto: true, real: true }, { reduce: function reduce(reducer /* , initialValue */) { var iterator = anObject(this); var next = aFunction(iterator.next); var noInitial = arguments.length < 2; var accumulator = noInitial ? undefined : arguments[1]; aFunction(reducer); return new Promise(function (resolve, reject) { var loop = function () { try { Promise.resolve(anObject(next.call(iterator))).then(function (step) { try { if (anObject(step).done) { noInitial ? reject(TypeError('Reduce of empty iterator with no initial value')) : resolve(accumulator); } else { var value = step.value; if (noInitial) { noInitial = false; accumulator = value; loop(); } else { Promise.resolve(reducer(accumulator, value)).then(function (result) { accumulator = result; loop(); }, reject); } } } catch (err) { reject(err); } }, reject); } catch (error) { reject(error); } }; loop(); }); } }); apollo-server-demo/node_modules/core-js/modules/esnext.reflect.define-metadata.js 0000644 0001750 0000144 00000001236 03560116604 027742 0 ustar andreh users var $ = require('../internals/export'); var ReflectMetadataModule = require('../internals/reflect-metadata'); var anObject = require('../internals/an-object'); var toMetadataKey = ReflectMetadataModule.toKey; var ordinaryDefineOwnMetadata = ReflectMetadataModule.set; // `Reflect.defineMetadata` method // https://github.com/rbuckton/reflect-metadata $({ target: 'Reflect', stat: true }, { defineMetadata: function defineMetadata(metadataKey, metadataValue, target /* , targetKey */) { var targetKey = arguments.length < 4 ? undefined : toMetadataKey(arguments[3]); ordinaryDefineOwnMetadata(metadataKey, metadataValue, anObject(target), targetKey); } }); apollo-server-demo/node_modules/core-js/modules/esnext.iterator.for-each.js 0000644 0001750 0000144 00000000544 03560116604 026624 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var iterate = require('../internals/iterate'); var anObject = require('../internals/an-object'); $({ target: 'Iterator', proto: true, real: true }, { forEach: function forEach(fn) { iterate(anObject(this), fn, { IS_ITERATOR: true }); } }); apollo-server-demo/node_modules/core-js/modules/esnext.observable.js 0000644 0001750 0000144 00000015644 03560116604 025443 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-observable var $ = require('../internals/export'); var DESCRIPTORS = require('../internals/descriptors'); var setSpecies = require('../internals/set-species'); var aFunction = require('../internals/a-function'); var anObject = require('../internals/an-object'); var isObject = require('../internals/is-object'); var anInstance = require('../internals/an-instance'); var defineProperty = require('../internals/object-define-property').f; var createNonEnumerableProperty = require('../internals/create-non-enumerable-property'); var redefineAll = require('../internals/redefine-all'); var getIterator = require('../internals/get-iterator'); var iterate = require('../internals/iterate'); var hostReportErrors = require('../internals/host-report-errors'); var wellKnownSymbol = require('../internals/well-known-symbol'); var InternalStateModule = require('../internals/internal-state'); var OBSERVABLE = wellKnownSymbol('observable'); var getInternalState = InternalStateModule.get; var setInternalState = InternalStateModule.set; var getMethod = function (fn) { return fn == null ? undefined : aFunction(fn); }; var cleanupSubscription = function (subscriptionState) { var cleanup = subscriptionState.cleanup; if (cleanup) { subscriptionState.cleanup = undefined; try { cleanup(); } catch (error) { hostReportErrors(error); } } }; var subscriptionClosed = function (subscriptionState) { return subscriptionState.observer === undefined; }; var close = function (subscription, subscriptionState) { if (!DESCRIPTORS) { subscription.closed = true; var subscriptionObserver = subscriptionState.subscriptionObserver; if (subscriptionObserver) subscriptionObserver.closed = true; } subscriptionState.observer = undefined; }; var Subscription = function (observer, subscriber) { var subscriptionState = setInternalState(this, { cleanup: undefined, observer: anObject(observer), subscriptionObserver: undefined }); var start; if (!DESCRIPTORS) this.closed = false; try { if (start = getMethod(observer.start)) start.call(observer, this); } catch (error) { hostReportErrors(error); } if (subscriptionClosed(subscriptionState)) return; var subscriptionObserver = subscriptionState.subscriptionObserver = new SubscriptionObserver(this); try { var cleanup = subscriber(subscriptionObserver); var subscription = cleanup; if (cleanup != null) subscriptionState.cleanup = typeof cleanup.unsubscribe === 'function' ? function () { subscription.unsubscribe(); } : aFunction(cleanup); } catch (error) { subscriptionObserver.error(error); return; } if (subscriptionClosed(subscriptionState)) cleanupSubscription(subscriptionState); }; Subscription.prototype = redefineAll({}, { unsubscribe: function unsubscribe() { var subscriptionState = getInternalState(this); if (!subscriptionClosed(subscriptionState)) { close(this, subscriptionState); cleanupSubscription(subscriptionState); } } }); if (DESCRIPTORS) defineProperty(Subscription.prototype, 'closed', { configurable: true, get: function () { return subscriptionClosed(getInternalState(this)); } }); var SubscriptionObserver = function (subscription) { setInternalState(this, { subscription: subscription }); if (!DESCRIPTORS) this.closed = false; }; SubscriptionObserver.prototype = redefineAll({}, { next: function next(value) { var subscriptionState = getInternalState(getInternalState(this).subscription); if (!subscriptionClosed(subscriptionState)) { var observer = subscriptionState.observer; try { var nextMethod = getMethod(observer.next); if (nextMethod) nextMethod.call(observer, value); } catch (error) { hostReportErrors(error); } } }, error: function error(value) { var subscription = getInternalState(this).subscription; var subscriptionState = getInternalState(subscription); if (!subscriptionClosed(subscriptionState)) { var observer = subscriptionState.observer; close(subscription, subscriptionState); try { var errorMethod = getMethod(observer.error); if (errorMethod) errorMethod.call(observer, value); else hostReportErrors(value); } catch (err) { hostReportErrors(err); } cleanupSubscription(subscriptionState); } }, complete: function complete() { var subscription = getInternalState(this).subscription; var subscriptionState = getInternalState(subscription); if (!subscriptionClosed(subscriptionState)) { var observer = subscriptionState.observer; close(subscription, subscriptionState); try { var completeMethod = getMethod(observer.complete); if (completeMethod) completeMethod.call(observer); } catch (error) { hostReportErrors(error); } cleanupSubscription(subscriptionState); } } }); if (DESCRIPTORS) defineProperty(SubscriptionObserver.prototype, 'closed', { configurable: true, get: function () { return subscriptionClosed(getInternalState(getInternalState(this).subscription)); } }); var $Observable = function Observable(subscriber) { anInstance(this, $Observable, 'Observable'); setInternalState(this, { subscriber: aFunction(subscriber) }); }; redefineAll($Observable.prototype, { subscribe: function subscribe(observer) { var length = arguments.length; return new Subscription(typeof observer === 'function' ? { next: observer, error: length > 1 ? arguments[1] : undefined, complete: length > 2 ? arguments[2] : undefined } : isObject(observer) ? observer : {}, getInternalState(this).subscriber); } }); redefineAll($Observable, { from: function from(x) { var C = typeof this === 'function' ? this : $Observable; var observableMethod = getMethod(anObject(x)[OBSERVABLE]); if (observableMethod) { var observable = anObject(observableMethod.call(x)); return observable.constructor === C ? observable : new C(function (observer) { return observable.subscribe(observer); }); } var iterator = getIterator(x); return new C(function (observer) { iterate(iterator, function (it, stop) { observer.next(it); if (observer.closed) return stop(); }, { IS_ITERATOR: true, INTERRUPTED: true }); observer.complete(); }); }, of: function of() { var C = typeof this === 'function' ? this : $Observable; var length = arguments.length; var items = new Array(length); var index = 0; while (index < length) items[index] = arguments[index++]; return new C(function (observer) { for (var i = 0; i < length; i++) { observer.next(items[i]); if (observer.closed) return; } observer.complete(); }); } }); createNonEnumerableProperty($Observable.prototype, OBSERVABLE, function () { return this; }); $({ global: true }, { Observable: $Observable }); setSpecies('Observable'); apollo-server-demo/node_modules/core-js/modules/esnext.string.at.js 0000644 0001750 0000144 00000000666 03560116604 025226 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var charAt = require('../internals/string-multibyte').charAt; var fails = require('../internals/fails'); var FORCED = fails(function () { return 'ð ®·'.at(0) !== 'ð ®·'; }); // `String.prototype.at` method // https://github.com/mathiasbynens/String.prototype.at $({ target: 'String', proto: true, forced: FORCED }, { at: function at(pos) { return charAt(this, pos); } }); apollo-server-demo/node_modules/core-js/modules/es.symbol.replace.js 0000644 0001750 0000144 00000000306 03560116604 025324 0 ustar andreh users var defineWellKnownSymbol = require('../internals/define-well-known-symbol'); // `Symbol.replace` well-known symbol // https://tc39.es/ecma262/#sec-symbol.replace defineWellKnownSymbol('replace'); apollo-server-demo/node_modules/core-js/modules/esnext.async-iterator.flat-map.js 0000644 0001750 0000144 00000004651 03560116604 027757 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var aFunction = require('../internals/a-function'); var anObject = require('../internals/an-object'); var createAsyncIteratorProxy = require('../internals/async-iterator-create-proxy'); var getAsyncIteratorMethod = require('../internals/get-async-iterator-method'); var AsyncIteratorProxy = createAsyncIteratorProxy(function (arg, Promise) { var state = this; var mapper = state.mapper; var innerIterator, iteratorMethod; return new Promise(function (resolve, reject) { var outerLoop = function () { try { Promise.resolve(anObject(state.next.call(state.iterator, arg))).then(function (step) { try { if (anObject(step).done) { state.done = true; resolve({ done: true, value: undefined }); } else { Promise.resolve(mapper(step.value)).then(function (mapped) { try { iteratorMethod = getAsyncIteratorMethod(mapped); if (iteratorMethod !== undefined) { state.innerIterator = innerIterator = anObject(iteratorMethod.call(mapped)); state.innerNext = aFunction(innerIterator.next); return innerLoop(); } reject(TypeError('.flatMap callback should return an iterable object')); } catch (error2) { reject(error2); } }, reject); } } catch (error1) { reject(error1); } }, reject); } catch (error) { reject(error); } }; var innerLoop = function () { if (innerIterator = state.innerIterator) { try { Promise.resolve(anObject(state.innerNext.call(innerIterator))).then(function (result) { try { if (anObject(result).done) { state.innerIterator = state.innerNext = null; outerLoop(); } else resolve({ done: false, value: result.value }); } catch (error1) { reject(error1); } }, reject); } catch (error) { reject(error); } } else outerLoop(); }; innerLoop(); }); }); $({ target: 'AsyncIterator', proto: true, real: true }, { flatMap: function flatMap(mapper) { return new AsyncIteratorProxy({ iterator: anObject(this), mapper: aFunction(mapper), innerIterator: null, innerNext: null }); } }); apollo-server-demo/node_modules/core-js/modules/es.array-buffer.is-view.js 0000644 0001750 0000144 00000000615 03560116604 026357 0 ustar andreh users var $ = require('../internals/export'); var ArrayBufferViewCore = require('../internals/array-buffer-view-core'); var NATIVE_ARRAY_BUFFER_VIEWS = ArrayBufferViewCore.NATIVE_ARRAY_BUFFER_VIEWS; // `ArrayBuffer.isView` method // https://tc39.es/ecma262/#sec-arraybuffer.isview $({ target: 'ArrayBuffer', stat: true, forced: !NATIVE_ARRAY_BUFFER_VIEWS }, { isView: ArrayBufferViewCore.isView }); apollo-server-demo/node_modules/core-js/modules/esnext.map.upsert.js 0000644 0001750 0000144 00000000610 03560116604 025400 0 ustar andreh users 'use strict'; // TODO: remove from `core-js@4` var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var $upsert = require('../internals/map-upsert'); // `Map.prototype.upsert` method (replaced by `Map.prototype.emplace`) // https://github.com/thumbsupep/proposal-upsert $({ target: 'Map', proto: true, real: true, forced: IS_PURE }, { upsert: $upsert }); apollo-server-demo/node_modules/core-js/modules/esnext.set.is-disjoint-from.js 0000644 0001750 0000144 00000001320 03560116604 027270 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var anObject = require('../internals/an-object'); var aFunction = require('../internals/a-function'); var iterate = require('../internals/iterate'); // `Set.prototype.isDisjointFrom` method // https://tc39.github.io/proposal-set-methods/#Set.prototype.isDisjointFrom $({ target: 'Set', proto: true, real: true, forced: IS_PURE }, { isDisjointFrom: function isDisjointFrom(iterable) { var set = anObject(this); var hasCheck = aFunction(set.has); return !iterate(iterable, function (value, stop) { if (hasCheck.call(set, value) === true) return stop(); }, { INTERRUPTED: true }).stopped; } }); apollo-server-demo/node_modules/core-js/modules/es.json.stringify.js 0000644 0001750 0000144 00000002213 03560116604 025372 0 ustar andreh users var $ = require('../internals/export'); var getBuiltIn = require('../internals/get-built-in'); var fails = require('../internals/fails'); var $stringify = getBuiltIn('JSON', 'stringify'); var re = /[\uD800-\uDFFF]/g; var low = /^[\uD800-\uDBFF]$/; var hi = /^[\uDC00-\uDFFF]$/; var fix = function (match, offset, string) { var prev = string.charAt(offset - 1); var next = string.charAt(offset + 1); if ((low.test(match) && !hi.test(next)) || (hi.test(match) && !low.test(prev))) { return '\\u' + match.charCodeAt(0).toString(16); } return match; }; var FORCED = fails(function () { return $stringify('\uDF06\uD834') !== '"\\udf06\\ud834"' || $stringify('\uDEAD') !== '"\\udead"'; }); if ($stringify) { // `JSON.stringify` method // https://tc39.es/ecma262/#sec-json.stringify // https://github.com/tc39/proposal-well-formed-stringify $({ target: 'JSON', stat: true, forced: FORCED }, { // eslint-disable-next-line no-unused-vars stringify: function stringify(it, replacer, space) { var result = $stringify.apply(null, arguments); return typeof result == 'string' ? result.replace(re, fix) : result; } }); } apollo-server-demo/node_modules/core-js/modules/esnext.map.map-keys.js 0000644 0001750 0000144 00000002222 03560116604 025605 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var getBuiltIn = require('../internals/get-built-in'); var anObject = require('../internals/an-object'); var aFunction = require('../internals/a-function'); var bind = require('../internals/function-bind-context'); var speciesConstructor = require('../internals/species-constructor'); var getMapIterator = require('../internals/get-map-iterator'); var iterate = require('../internals/iterate'); // `Map.prototype.mapKeys` method // https://github.com/tc39/proposal-collection-methods $({ target: 'Map', proto: true, real: true, forced: IS_PURE }, { mapKeys: function mapKeys(callbackfn /* , thisArg */) { var map = anObject(this); var iterator = getMapIterator(map); var boundFunction = bind(callbackfn, arguments.length > 1 ? arguments[1] : undefined, 3); var newMap = new (speciesConstructor(map, getBuiltIn('Map')))(); var setter = aFunction(newMap.set); iterate(iterator, function (key, value) { setter.call(newMap, boundFunction(value, key, map), value); }, { AS_ENTRIES: true, IS_ITERATOR: true }); return newMap; } }); apollo-server-demo/node_modules/core-js/modules/es.typed-array.last-index-of.js 0000644 0001750 0000144 00000001120 03560116604 027312 0 ustar andreh users 'use strict'; var ArrayBufferViewCore = require('../internals/array-buffer-view-core'); var $lastIndexOf = require('../internals/array-last-index-of'); var aTypedArray = ArrayBufferViewCore.aTypedArray; var exportTypedArrayMethod = ArrayBufferViewCore.exportTypedArrayMethod; // `%TypedArray%.prototype.lastIndexOf` method // https://tc39.es/ecma262/#sec-%typedarray%.prototype.lastindexof // eslint-disable-next-line no-unused-vars exportTypedArrayMethod('lastIndexOf', function lastIndexOf(searchElement /* , fromIndex */) { return $lastIndexOf.apply(aTypedArray(this), arguments); }); apollo-server-demo/node_modules/core-js/modules/esnext.global-this.js 0000644 0001750 0000144 00000000076 03560116604 025515 0 ustar andreh users // TODO: Remove from `core-js@4` require('./es.global-this'); apollo-server-demo/node_modules/core-js/modules/esnext.reflect.get-metadata.js 0000644 0001750 0000144 00000002040 03560116604 027261 0 ustar andreh users var $ = require('../internals/export'); var ReflectMetadataModule = require('../internals/reflect-metadata'); var anObject = require('../internals/an-object'); var getPrototypeOf = require('../internals/object-get-prototype-of'); var ordinaryHasOwnMetadata = ReflectMetadataModule.has; var ordinaryGetOwnMetadata = ReflectMetadataModule.get; var toMetadataKey = ReflectMetadataModule.toKey; var ordinaryGetMetadata = function (MetadataKey, O, P) { var hasOwn = ordinaryHasOwnMetadata(MetadataKey, O, P); if (hasOwn) return ordinaryGetOwnMetadata(MetadataKey, O, P); var parent = getPrototypeOf(O); return parent !== null ? ordinaryGetMetadata(MetadataKey, parent, P) : undefined; }; // `Reflect.getMetadata` method // https://github.com/rbuckton/reflect-metadata $({ target: 'Reflect', stat: true }, { getMetadata: function getMetadata(metadataKey, target /* , targetKey */) { var targetKey = arguments.length < 3 ? undefined : toMetadataKey(arguments[2]); return ordinaryGetMetadata(metadataKey, anObject(target), targetKey); } }); apollo-server-demo/node_modules/core-js/modules/es.string.ends-with.js 0000644 0001750 0000144 00000003006 03560116604 025614 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var getOwnPropertyDescriptor = require('../internals/object-get-own-property-descriptor').f; var toLength = require('../internals/to-length'); var notARegExp = require('../internals/not-a-regexp'); var requireObjectCoercible = require('../internals/require-object-coercible'); var correctIsRegExpLogic = require('../internals/correct-is-regexp-logic'); var IS_PURE = require('../internals/is-pure'); var nativeEndsWith = ''.endsWith; var min = Math.min; var CORRECT_IS_REGEXP_LOGIC = correctIsRegExpLogic('endsWith'); // https://github.com/zloirock/core-js/pull/702 var MDN_POLYFILL_BUG = !IS_PURE && !CORRECT_IS_REGEXP_LOGIC && !!function () { var descriptor = getOwnPropertyDescriptor(String.prototype, 'endsWith'); return descriptor && !descriptor.writable; }(); // `String.prototype.endsWith` method // https://tc39.es/ecma262/#sec-string.prototype.endswith $({ target: 'String', proto: true, forced: !MDN_POLYFILL_BUG && !CORRECT_IS_REGEXP_LOGIC }, { endsWith: function endsWith(searchString /* , endPosition = @length */) { var that = String(requireObjectCoercible(this)); notARegExp(searchString); var endPosition = arguments.length > 1 ? arguments[1] : undefined; var len = toLength(that.length); var end = endPosition === undefined ? len : min(toLength(endPosition), len); var search = String(searchString); return nativeEndsWith ? nativeEndsWith.call(that, search, end) : that.slice(end - search.length, end) === search; } }); apollo-server-demo/node_modules/core-js/modules/esnext.math.umulh.js 0000644 0001750 0000144 00000001003 03560116604 025361 0 ustar andreh users var $ = require('../internals/export'); // `Math.umulh` method // https://gist.github.com/BrendanEich/4294d5c212a6d2254703 // TODO: Remove from `core-js@4` $({ target: 'Math', stat: true }, { umulh: function umulh(u, v) { var UINT16 = 0xFFFF; var $u = +u; var $v = +v; var u0 = $u & UINT16; var v0 = $v & UINT16; var u1 = $u >>> 16; var v1 = $v >>> 16; var t = (u1 * v0 >>> 0) + (u0 * v0 >>> 16); return u1 * v1 + (t >>> 16) + ((u0 * v1 >>> 0) + (t & UINT16) >>> 16); } }); apollo-server-demo/node_modules/core-js/modules/es.regexp.constructor.js 0000644 0001750 0000144 00000006076 03560116604 026275 0 ustar andreh users var DESCRIPTORS = require('../internals/descriptors'); var global = require('../internals/global'); var isForced = require('../internals/is-forced'); var inheritIfRequired = require('../internals/inherit-if-required'); var defineProperty = require('../internals/object-define-property').f; var getOwnPropertyNames = require('../internals/object-get-own-property-names').f; var isRegExp = require('../internals/is-regexp'); var getFlags = require('../internals/regexp-flags'); var stickyHelpers = require('../internals/regexp-sticky-helpers'); var redefine = require('../internals/redefine'); var fails = require('../internals/fails'); var setInternalState = require('../internals/internal-state').set; var setSpecies = require('../internals/set-species'); var wellKnownSymbol = require('../internals/well-known-symbol'); var MATCH = wellKnownSymbol('match'); var NativeRegExp = global.RegExp; var RegExpPrototype = NativeRegExp.prototype; var re1 = /a/g; var re2 = /a/g; // "new" should create a new object, old webkit bug var CORRECT_NEW = new NativeRegExp(re1) !== re1; var UNSUPPORTED_Y = stickyHelpers.UNSUPPORTED_Y; var FORCED = DESCRIPTORS && isForced('RegExp', (!CORRECT_NEW || UNSUPPORTED_Y || fails(function () { re2[MATCH] = false; // RegExp constructor can alter flags and IsRegExp works correct with @@match return NativeRegExp(re1) != re1 || NativeRegExp(re2) == re2 || NativeRegExp(re1, 'i') != '/a/i'; }))); // `RegExp` constructor // https://tc39.es/ecma262/#sec-regexp-constructor if (FORCED) { var RegExpWrapper = function RegExp(pattern, flags) { var thisIsRegExp = this instanceof RegExpWrapper; var patternIsRegExp = isRegExp(pattern); var flagsAreUndefined = flags === undefined; var sticky; if (!thisIsRegExp && patternIsRegExp && pattern.constructor === RegExpWrapper && flagsAreUndefined) { return pattern; } if (CORRECT_NEW) { if (patternIsRegExp && !flagsAreUndefined) pattern = pattern.source; } else if (pattern instanceof RegExpWrapper) { if (flagsAreUndefined) flags = getFlags.call(pattern); pattern = pattern.source; } if (UNSUPPORTED_Y) { sticky = !!flags && flags.indexOf('y') > -1; if (sticky) flags = flags.replace(/y/g, ''); } var result = inheritIfRequired( CORRECT_NEW ? new NativeRegExp(pattern, flags) : NativeRegExp(pattern, flags), thisIsRegExp ? this : RegExpPrototype, RegExpWrapper ); if (UNSUPPORTED_Y && sticky) setInternalState(result, { sticky: sticky }); return result; }; var proxy = function (key) { key in RegExpWrapper || defineProperty(RegExpWrapper, key, { configurable: true, get: function () { return NativeRegExp[key]; }, set: function (it) { NativeRegExp[key] = it; } }); }; var keys = getOwnPropertyNames(NativeRegExp); var index = 0; while (keys.length > index) proxy(keys[index++]); RegExpPrototype.constructor = RegExpWrapper; RegExpWrapper.prototype = RegExpPrototype; redefine(global, 'RegExp', RegExpWrapper); } // https://tc39.es/ecma262/#sec-get-regexp-@@species setSpecies('RegExp'); apollo-server-demo/node_modules/core-js/modules/esnext.set.difference.js 0000644 0001750 0000144 00000001500 03560116604 026165 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var getBuiltIn = require('../internals/get-built-in'); var anObject = require('../internals/an-object'); var aFunction = require('../internals/a-function'); var speciesConstructor = require('../internals/species-constructor'); var iterate = require('../internals/iterate'); // `Set.prototype.difference` method // https://github.com/tc39/proposal-set-methods $({ target: 'Set', proto: true, real: true, forced: IS_PURE }, { difference: function difference(iterable) { var set = anObject(this); var newSet = new (speciesConstructor(set, getBuiltIn('Set')))(set); var remover = aFunction(newSet['delete']); iterate(iterable, function (value) { remover.call(newSet, value); }); return newSet; } }); apollo-server-demo/node_modules/core-js/modules/es.symbol.unscopables.js 0000644 0001750 0000144 00000000322 03560116604 026225 0 ustar andreh users var defineWellKnownSymbol = require('../internals/define-well-known-symbol'); // `Symbol.unscopables` well-known symbol // https://tc39.es/ecma262/#sec-symbol.unscopables defineWellKnownSymbol('unscopables'); apollo-server-demo/node_modules/core-js/modules/esnext.math.radians.js 0000644 0001750 0000144 00000000421 03560116604 025653 0 ustar andreh users var $ = require('../internals/export'); var DEG_PER_RAD = Math.PI / 180; // `Math.radians` method // https://rwaldron.github.io/proposal-math-extensions/ $({ target: 'Math', stat: true }, { radians: function radians(degrees) { return degrees * DEG_PER_RAD; } }); apollo-server-demo/node_modules/core-js/modules/es.array.find-index.js 0000644 0001750 0000144 00000001642 03560116604 025553 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var $findIndex = require('../internals/array-iteration').findIndex; var addToUnscopables = require('../internals/add-to-unscopables'); var arrayMethodUsesToLength = require('../internals/array-method-uses-to-length'); var FIND_INDEX = 'findIndex'; var SKIPS_HOLES = true; var USES_TO_LENGTH = arrayMethodUsesToLength(FIND_INDEX); // Shouldn't skip holes if (FIND_INDEX in []) Array(1)[FIND_INDEX](function () { SKIPS_HOLES = false; }); // `Array.prototype.findIndex` method // https://tc39.es/ecma262/#sec-array.prototype.findindex $({ target: 'Array', proto: true, forced: SKIPS_HOLES || !USES_TO_LENGTH }, { findIndex: function findIndex(callbackfn /* , that = undefined */) { return $findIndex(this, callbackfn, arguments.length > 1 ? arguments[1] : undefined); } }); // https://tc39.es/ecma262/#sec-array.prototype-@@unscopables addToUnscopables(FIND_INDEX); apollo-server-demo/node_modules/core-js/modules/es.typed-array.for-each.js 0000644 0001750 0000144 00000001061 03560116604 026330 0 ustar andreh users 'use strict'; var ArrayBufferViewCore = require('../internals/array-buffer-view-core'); var $forEach = require('../internals/array-iteration').forEach; var aTypedArray = ArrayBufferViewCore.aTypedArray; var exportTypedArrayMethod = ArrayBufferViewCore.exportTypedArrayMethod; // `%TypedArray%.prototype.forEach` method // https://tc39.es/ecma262/#sec-%typedarray%.prototype.foreach exportTypedArrayMethod('forEach', function forEach(callbackfn /* , thisArg */) { $forEach(aTypedArray(this), callbackfn, arguments.length > 1 ? arguments[1] : undefined); }); apollo-server-demo/node_modules/core-js/modules/es.object.prevent-extensions.js 0000644 0001750 0000144 00000001322 03560116604 027531 0 ustar andreh users var $ = require('../internals/export'); var isObject = require('../internals/is-object'); var onFreeze = require('../internals/internal-metadata').onFreeze; var FREEZING = require('../internals/freezing'); var fails = require('../internals/fails'); var nativePreventExtensions = Object.preventExtensions; var FAILS_ON_PRIMITIVES = fails(function () { nativePreventExtensions(1); }); // `Object.preventExtensions` method // https://tc39.es/ecma262/#sec-object.preventextensions $({ target: 'Object', stat: true, forced: FAILS_ON_PRIMITIVES, sham: !FREEZING }, { preventExtensions: function preventExtensions(it) { return nativePreventExtensions && isObject(it) ? nativePreventExtensions(onFreeze(it)) : it; } }); apollo-server-demo/node_modules/core-js/modules/es.array.for-each.js 0000644 0001750 0000144 00000000445 03560116604 025212 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var forEach = require('../internals/array-for-each'); // `Array.prototype.forEach` method // https://tc39.es/ecma262/#sec-array.prototype.foreach $({ target: 'Array', proto: true, forced: [].forEach != forEach }, { forEach: forEach }); apollo-server-demo/node_modules/core-js/modules/esnext.map.delete-all.js 0000644 0001750 0000144 00000000677 03560116604 026103 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var collectionDeleteAll = require('../internals/collection-delete-all'); // `Map.prototype.deleteAll` method // https://github.com/tc39/proposal-collection-methods $({ target: 'Map', proto: true, real: true, forced: IS_PURE }, { deleteAll: function deleteAll(/* ...elements */) { return collectionDeleteAll.apply(this, arguments); } }); apollo-server-demo/node_modules/core-js/modules/esnext.reflect.delete-metadata.js 0000644 0001750 0000144 00000001703 03560116604 027751 0 ustar andreh users var $ = require('../internals/export'); var ReflectMetadataModule = require('../internals/reflect-metadata'); var anObject = require('../internals/an-object'); var toMetadataKey = ReflectMetadataModule.toKey; var getOrCreateMetadataMap = ReflectMetadataModule.getMap; var store = ReflectMetadataModule.store; // `Reflect.deleteMetadata` method // https://github.com/rbuckton/reflect-metadata $({ target: 'Reflect', stat: true }, { deleteMetadata: function deleteMetadata(metadataKey, target /* , targetKey */) { var targetKey = arguments.length < 3 ? undefined : toMetadataKey(arguments[2]); var metadataMap = getOrCreateMetadataMap(anObject(target), targetKey, false); if (metadataMap === undefined || !metadataMap['delete'](metadataKey)) return false; if (metadataMap.size) return true; var targetMetadata = store.get(target); targetMetadata['delete'](targetKey); return !!targetMetadata.size || store['delete'](target); } }); apollo-server-demo/node_modules/core-js/modules/esnext.iterator.to-array.js 0000644 0001750 0000144 00000000657 03560116604 026703 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var iterate = require('../internals/iterate'); var anObject = require('../internals/an-object'); var push = [].push; $({ target: 'Iterator', proto: true, real: true }, { toArray: function toArray() { var result = []; iterate(anObject(this), push, { that: result, IS_ITERATOR: true }); return result; } }); apollo-server-demo/node_modules/core-js/modules/es.typed-array.sort.js 0000644 0001750 0000144 00000000700 03560116604 025632 0 ustar andreh users 'use strict'; var ArrayBufferViewCore = require('../internals/array-buffer-view-core'); var aTypedArray = ArrayBufferViewCore.aTypedArray; var exportTypedArrayMethod = ArrayBufferViewCore.exportTypedArrayMethod; var $sort = [].sort; // `%TypedArray%.prototype.sort` method // https://tc39.es/ecma262/#sec-%typedarray%.prototype.sort exportTypedArrayMethod('sort', function sort(comparefn) { return $sort.call(aTypedArray(this), comparefn); }); apollo-server-demo/node_modules/core-js/modules/esnext.async-iterator.as-indexed-pairs.js 0000644 0001750 0000144 00000001521 03560116604 031404 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var anObject = require('../internals/an-object'); var createAsyncIteratorProxy = require('../internals/async-iterator-create-proxy'); var AsyncIteratorProxy = createAsyncIteratorProxy(function (arg, Promise) { var state = this; var iterator = state.iterator; return Promise.resolve(anObject(state.next.call(iterator, arg))).then(function (step) { if (anObject(step).done) { state.done = true; return { done: true, value: undefined }; } return { done: false, value: [state.index++, step.value] }; }); }); $({ target: 'AsyncIterator', proto: true, real: true }, { asIndexedPairs: function asIndexedPairs() { return new AsyncIteratorProxy({ iterator: anObject(this), index: 0 }); } }); apollo-server-demo/node_modules/core-js/modules/esnext.math.scale.js 0000644 0001750 0000144 00000000337 03560116604 025327 0 ustar andreh users var $ = require('../internals/export'); var scale = require('../internals/math-scale'); // `Math.scale` method // https://rwaldron.github.io/proposal-math-extensions/ $({ target: 'Math', stat: true }, { scale: scale }); apollo-server-demo/node_modules/core-js/modules/es.typed-array.some.js 0000644 0001750 0000144 00000001043 03560116604 025607 0 ustar andreh users 'use strict'; var ArrayBufferViewCore = require('../internals/array-buffer-view-core'); var $some = require('../internals/array-iteration').some; var aTypedArray = ArrayBufferViewCore.aTypedArray; var exportTypedArrayMethod = ArrayBufferViewCore.exportTypedArrayMethod; // `%TypedArray%.prototype.some` method // https://tc39.es/ecma262/#sec-%typedarray%.prototype.some exportTypedArrayMethod('some', function some(callbackfn /* , thisArg */) { return $some(aTypedArray(this), callbackfn, arguments.length > 1 ? arguments[1] : undefined); }); apollo-server-demo/node_modules/core-js/modules/esnext.reflect.get-own-metadata.js 0000644 0001750 0000144 00000001201 03560116604 030060 0 ustar andreh users var $ = require('../internals/export'); var ReflectMetadataModule = require('../internals/reflect-metadata'); var anObject = require('../internals/an-object'); var ordinaryGetOwnMetadata = ReflectMetadataModule.get; var toMetadataKey = ReflectMetadataModule.toKey; // `Reflect.getOwnMetadata` method // https://github.com/rbuckton/reflect-metadata $({ target: 'Reflect', stat: true }, { getOwnMetadata: function getOwnMetadata(metadataKey, target /* , targetKey */) { var targetKey = arguments.length < 3 ? undefined : toMetadataKey(arguments[2]); return ordinaryGetOwnMetadata(metadataKey, anObject(target), targetKey); } }); apollo-server-demo/node_modules/core-js/modules/esnext.async-iterator.map.js 0000644 0001750 0000144 00000001703 03560116604 027026 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var aFunction = require('../internals/a-function'); var anObject = require('../internals/an-object'); var createAsyncIteratorProxy = require('../internals/async-iterator-create-proxy'); var AsyncIteratorProxy = createAsyncIteratorProxy(function (arg, Promise) { var state = this; var mapper = state.mapper; return Promise.resolve(anObject(state.next.call(state.iterator, arg))).then(function (step) { if (anObject(step).done) { state.done = true; return { done: true, value: undefined }; } return Promise.resolve(mapper(step.value)).then(function (value) { return { done: false, value: value }; }); }); }); $({ target: 'AsyncIterator', proto: true, real: true }, { map: function map(mapper) { return new AsyncIteratorProxy({ iterator: anObject(this), mapper: aFunction(mapper) }); } }); apollo-server-demo/node_modules/core-js/modules/es.array.filter.js 0000644 0001750 0000144 00000001436 03560116604 025014 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var $filter = require('../internals/array-iteration').filter; var arrayMethodHasSpeciesSupport = require('../internals/array-method-has-species-support'); var arrayMethodUsesToLength = require('../internals/array-method-uses-to-length'); var HAS_SPECIES_SUPPORT = arrayMethodHasSpeciesSupport('filter'); // Edge 14- issue var USES_TO_LENGTH = arrayMethodUsesToLength('filter'); // `Array.prototype.filter` method // https://tc39.es/ecma262/#sec-array.prototype.filter // with adding support of @@species $({ target: 'Array', proto: true, forced: !HAS_SPECIES_SUPPORT || !USES_TO_LENGTH }, { filter: function filter(callbackfn /* , thisArg */) { return $filter(this, callbackfn, arguments.length > 1 ? arguments[1] : undefined); } }); apollo-server-demo/node_modules/core-js/modules/es.array.sort.js 0000644 0001750 0000144 00000001637 03560116604 024521 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var aFunction = require('../internals/a-function'); var toObject = require('../internals/to-object'); var fails = require('../internals/fails'); var arrayMethodIsStrict = require('../internals/array-method-is-strict'); var test = []; var nativeSort = test.sort; // IE8- var FAILS_ON_UNDEFINED = fails(function () { test.sort(undefined); }); // V8 bug var FAILS_ON_NULL = fails(function () { test.sort(null); }); // Old WebKit var STRICT_METHOD = arrayMethodIsStrict('sort'); var FORCED = FAILS_ON_UNDEFINED || !FAILS_ON_NULL || !STRICT_METHOD; // `Array.prototype.sort` method // https://tc39.es/ecma262/#sec-array.prototype.sort $({ target: 'Array', proto: true, forced: FORCED }, { sort: function sort(comparefn) { return comparefn === undefined ? nativeSort.call(toObject(this)) : nativeSort.call(toObject(this), aFunction(comparefn)); } }); apollo-server-demo/node_modules/core-js/modules/esnext.async-iterator.filter.js 0000644 0001750 0000144 00000002511 03560116604 027534 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var aFunction = require('../internals/a-function'); var anObject = require('../internals/an-object'); var createAsyncIteratorProxy = require('../internals/async-iterator-create-proxy'); var AsyncIteratorProxy = createAsyncIteratorProxy(function (arg, Promise) { var state = this; var filterer = state.filterer; return new Promise(function (resolve, reject) { var loop = function () { try { Promise.resolve(anObject(state.next.call(state.iterator, arg))).then(function (step) { try { if (anObject(step).done) { state.done = true; resolve({ done: true, value: undefined }); } else { var value = step.value; Promise.resolve(filterer(value)).then(function (selected) { selected ? resolve({ done: false, value: value }) : loop(); }, reject); } } catch (err) { reject(err); } }, reject); } catch (error) { reject(error); } }; loop(); }); }); $({ target: 'AsyncIterator', proto: true, real: true }, { filter: function filter(filterer) { return new AsyncIteratorProxy({ iterator: anObject(this), filterer: aFunction(filterer) }); } }); apollo-server-demo/node_modules/core-js/modules/es.object.assign.js 0000644 0001750 0000144 00000000402 03560116604 025133 0 ustar andreh users var $ = require('../internals/export'); var assign = require('../internals/object-assign'); // `Object.assign` method // https://tc39.es/ecma262/#sec-object.assign $({ target: 'Object', stat: true, forced: Object.assign !== assign }, { assign: assign }); apollo-server-demo/node_modules/core-js/modules/es.object.is-frozen.js 0000644 0001750 0000144 00000000773 03560116604 025576 0 ustar andreh users var $ = require('../internals/export'); var fails = require('../internals/fails'); var isObject = require('../internals/is-object'); var nativeIsFrozen = Object.isFrozen; var FAILS_ON_PRIMITIVES = fails(function () { nativeIsFrozen(1); }); // `Object.isFrozen` method // https://tc39.es/ecma262/#sec-object.isfrozen $({ target: 'Object', stat: true, forced: FAILS_ON_PRIMITIVES }, { isFrozen: function isFrozen(it) { return isObject(it) ? nativeIsFrozen ? nativeIsFrozen(it) : false : true; } }); apollo-server-demo/node_modules/core-js/modules/esnext.iterator.flat-map.js 0000644 0001750 0000144 00000003131 03560116604 026634 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var aFunction = require('../internals/a-function'); var anObject = require('../internals/an-object'); var getIteratorMethod = require('../internals/get-iterator-method'); var createIteratorProxy = require('../internals/iterator-create-proxy'); var iteratorClose = require('../internals/iterator-close'); var IteratorProxy = createIteratorProxy(function (arg) { var iterator = this.iterator; var mapper = this.mapper; var result, mapped, iteratorMethod, innerIterator; while (true) { try { if (innerIterator = this.innerIterator) { result = anObject(this.innerNext.call(innerIterator)); if (!result.done) return result.value; this.innerIterator = this.innerNext = null; } result = anObject(this.next.call(iterator, arg)); if (this.done = !!result.done) return; mapped = mapper(result.value); iteratorMethod = getIteratorMethod(mapped); if (iteratorMethod === undefined) { throw TypeError('.flatMap callback should return an iterable object'); } this.innerIterator = innerIterator = anObject(iteratorMethod.call(mapped)); this.innerNext = aFunction(innerIterator.next); } catch (error) { iteratorClose(iterator); throw error; } } }); $({ target: 'Iterator', proto: true, real: true }, { flatMap: function flatMap(mapper) { return new IteratorProxy({ iterator: anObject(this), mapper: aFunction(mapper), innerIterator: null, innerNext: null }); } }); apollo-server-demo/node_modules/core-js/modules/es.number.is-nan.js 0000644 0001750 0000144 00000000414 03560116604 025061 0 ustar andreh users var $ = require('../internals/export'); // `Number.isNaN` method // https://tc39.es/ecma262/#sec-number.isnan $({ target: 'Number', stat: true }, { isNaN: function isNaN(number) { // eslint-disable-next-line no-self-compare return number != number; } }); apollo-server-demo/node_modules/core-js/modules/esnext.set.union.js 0000644 0001750 0000144 00000001356 03560116604 025234 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var getBuiltIn = require('../internals/get-built-in'); var anObject = require('../internals/an-object'); var aFunction = require('../internals/a-function'); var speciesConstructor = require('../internals/species-constructor'); var iterate = require('../internals/iterate'); // `Set.prototype.union` method // https://github.com/tc39/proposal-set-methods $({ target: 'Set', proto: true, real: true, forced: IS_PURE }, { union: function union(iterable) { var set = anObject(this); var newSet = new (speciesConstructor(set, getBuiltIn('Set')))(set); iterate(iterable, aFunction(newSet.add), { that: newSet }); return newSet; } }); apollo-server-demo/node_modules/core-js/modules/es.string.starts-with.js 0000644 0001750 0000144 00000002667 03560116604 026217 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var getOwnPropertyDescriptor = require('../internals/object-get-own-property-descriptor').f; var toLength = require('../internals/to-length'); var notARegExp = require('../internals/not-a-regexp'); var requireObjectCoercible = require('../internals/require-object-coercible'); var correctIsRegExpLogic = require('../internals/correct-is-regexp-logic'); var IS_PURE = require('../internals/is-pure'); var nativeStartsWith = ''.startsWith; var min = Math.min; var CORRECT_IS_REGEXP_LOGIC = correctIsRegExpLogic('startsWith'); // https://github.com/zloirock/core-js/pull/702 var MDN_POLYFILL_BUG = !IS_PURE && !CORRECT_IS_REGEXP_LOGIC && !!function () { var descriptor = getOwnPropertyDescriptor(String.prototype, 'startsWith'); return descriptor && !descriptor.writable; }(); // `String.prototype.startsWith` method // https://tc39.es/ecma262/#sec-string.prototype.startswith $({ target: 'String', proto: true, forced: !MDN_POLYFILL_BUG && !CORRECT_IS_REGEXP_LOGIC }, { startsWith: function startsWith(searchString /* , position = 0 */) { var that = String(requireObjectCoercible(this)); notARegExp(searchString); var index = toLength(min(arguments.length > 1 ? arguments[1] : undefined, that.length)); var search = String(searchString); return nativeStartsWith ? nativeStartsWith.call(that, search, index) : that.slice(index, index + search.length) === search; } }); apollo-server-demo/node_modules/core-js/modules/es.symbol.is-concat-spreadable.js 0000644 0001750 0000144 00000000347 03560116604 027676 0 ustar andreh users var defineWellKnownSymbol = require('../internals/define-well-known-symbol'); // `Symbol.isConcatSpreadable` well-known symbol // https://tc39.es/ecma262/#sec-symbol.isconcatspreadable defineWellKnownSymbol('isConcatSpreadable'); apollo-server-demo/node_modules/core-js/modules/esnext.reflect.has-own-metadata.js 0000644 0001750 0000144 00000001201 03560116604 030054 0 ustar andreh users var $ = require('../internals/export'); var ReflectMetadataModule = require('../internals/reflect-metadata'); var anObject = require('../internals/an-object'); var ordinaryHasOwnMetadata = ReflectMetadataModule.has; var toMetadataKey = ReflectMetadataModule.toKey; // `Reflect.hasOwnMetadata` method // https://github.com/rbuckton/reflect-metadata $({ target: 'Reflect', stat: true }, { hasOwnMetadata: function hasOwnMetadata(metadataKey, target /* , targetKey */) { var targetKey = arguments.length < 3 ? undefined : toMetadataKey(arguments[2]); return ordinaryHasOwnMetadata(metadataKey, anObject(target), targetKey); } }); apollo-server-demo/node_modules/core-js/modules/es.typed-array.of.js 0000644 0001750 0000144 00000001346 03560116604 025256 0 ustar andreh users 'use strict'; var ArrayBufferViewCore = require('../internals/array-buffer-view-core'); var TYPED_ARRAYS_CONSTRUCTORS_REQUIRES_WRAPPERS = require('../internals/typed-array-constructors-require-wrappers'); var aTypedArrayConstructor = ArrayBufferViewCore.aTypedArrayConstructor; var exportTypedArrayStaticMethod = ArrayBufferViewCore.exportTypedArrayStaticMethod; // `%TypedArray%.of` method // https://tc39.es/ecma262/#sec-%typedarray%.of exportTypedArrayStaticMethod('of', function of(/* ...items */) { var index = 0; var length = arguments.length; var result = new (aTypedArrayConstructor(this))(length); while (length > index) result[index] = arguments[index++]; return result; }, TYPED_ARRAYS_CONSTRUCTORS_REQUIRES_WRAPPERS); apollo-server-demo/node_modules/core-js/modules/es.string.match-all.js 0000644 0001750 0000144 00000010554 03560116604 025562 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var createIteratorConstructor = require('../internals/create-iterator-constructor'); var requireObjectCoercible = require('../internals/require-object-coercible'); var toLength = require('../internals/to-length'); var aFunction = require('../internals/a-function'); var anObject = require('../internals/an-object'); var classof = require('../internals/classof-raw'); var isRegExp = require('../internals/is-regexp'); var getRegExpFlags = require('../internals/regexp-flags'); var createNonEnumerableProperty = require('../internals/create-non-enumerable-property'); var fails = require('../internals/fails'); var wellKnownSymbol = require('../internals/well-known-symbol'); var speciesConstructor = require('../internals/species-constructor'); var advanceStringIndex = require('../internals/advance-string-index'); var InternalStateModule = require('../internals/internal-state'); var IS_PURE = require('../internals/is-pure'); var MATCH_ALL = wellKnownSymbol('matchAll'); var REGEXP_STRING = 'RegExp String'; var REGEXP_STRING_ITERATOR = REGEXP_STRING + ' Iterator'; var setInternalState = InternalStateModule.set; var getInternalState = InternalStateModule.getterFor(REGEXP_STRING_ITERATOR); var RegExpPrototype = RegExp.prototype; var regExpBuiltinExec = RegExpPrototype.exec; var nativeMatchAll = ''.matchAll; var WORKS_WITH_NON_GLOBAL_REGEX = !!nativeMatchAll && !fails(function () { 'a'.matchAll(/./); }); var regExpExec = function (R, S) { var exec = R.exec; var result; if (typeof exec == 'function') { result = exec.call(R, S); if (typeof result != 'object') throw TypeError('Incorrect exec result'); return result; } return regExpBuiltinExec.call(R, S); }; // eslint-disable-next-line max-len var $RegExpStringIterator = createIteratorConstructor(function RegExpStringIterator(regexp, string, global, fullUnicode) { setInternalState(this, { type: REGEXP_STRING_ITERATOR, regexp: regexp, string: string, global: global, unicode: fullUnicode, done: false }); }, REGEXP_STRING, function next() { var state = getInternalState(this); if (state.done) return { value: undefined, done: true }; var R = state.regexp; var S = state.string; var match = regExpExec(R, S); if (match === null) return { value: undefined, done: state.done = true }; if (state.global) { if (String(match[0]) == '') R.lastIndex = advanceStringIndex(S, toLength(R.lastIndex), state.unicode); return { value: match, done: false }; } state.done = true; return { value: match, done: false }; }); var $matchAll = function (string) { var R = anObject(this); var S = String(string); var C, flagsValue, flags, matcher, global, fullUnicode; C = speciesConstructor(R, RegExp); flagsValue = R.flags; if (flagsValue === undefined && R instanceof RegExp && !('flags' in RegExpPrototype)) { flagsValue = getRegExpFlags.call(R); } flags = flagsValue === undefined ? '' : String(flagsValue); matcher = new C(C === RegExp ? R.source : R, flags); global = !!~flags.indexOf('g'); fullUnicode = !!~flags.indexOf('u'); matcher.lastIndex = toLength(R.lastIndex); return new $RegExpStringIterator(matcher, S, global, fullUnicode); }; // `String.prototype.matchAll` method // https://tc39.es/ecma262/#sec-string.prototype.matchall $({ target: 'String', proto: true, forced: WORKS_WITH_NON_GLOBAL_REGEX }, { matchAll: function matchAll(regexp) { var O = requireObjectCoercible(this); var flags, S, matcher, rx; if (regexp != null) { if (isRegExp(regexp)) { flags = String(requireObjectCoercible('flags' in RegExpPrototype ? regexp.flags : getRegExpFlags.call(regexp) )); if (!~flags.indexOf('g')) throw TypeError('`.matchAll` does not allow non-global regexes'); } if (WORKS_WITH_NON_GLOBAL_REGEX) return nativeMatchAll.apply(O, arguments); matcher = regexp[MATCH_ALL]; if (matcher === undefined && IS_PURE && classof(regexp) == 'RegExp') matcher = $matchAll; if (matcher != null) return aFunction(matcher).call(regexp, O); } else if (WORKS_WITH_NON_GLOBAL_REGEX) return nativeMatchAll.apply(O, arguments); S = String(O); rx = new RegExp(regexp, 'g'); return IS_PURE ? $matchAll.call(rx, S) : rx[MATCH_ALL](S); } }); IS_PURE || MATCH_ALL in RegExpPrototype || createNonEnumerableProperty(RegExpPrototype, MATCH_ALL, $matchAll); apollo-server-demo/node_modules/core-js/modules/esnext.math.deg-per-rad.js 0000644 0001750 0000144 00000000305 03560116604 026322 0 ustar andreh users var $ = require('../internals/export'); // `Math.DEG_PER_RAD` constant // https://rwaldron.github.io/proposal-math-extensions/ $({ target: 'Math', stat: true }, { DEG_PER_RAD: Math.PI / 180 }); apollo-server-demo/node_modules/core-js/modules/es.string.code-point-at.js 0000644 0001750 0000144 00000000523 03560116604 026356 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var codeAt = require('../internals/string-multibyte').codeAt; // `String.prototype.codePointAt` method // https://tc39.es/ecma262/#sec-string.prototype.codepointat $({ target: 'String', proto: true }, { codePointAt: function codePointAt(pos) { return codeAt(this, pos); } }); apollo-server-demo/node_modules/core-js/modules/es.typed-array.set.js 0000644 0001750 0000144 00000002001 03560116604 025432 0 ustar andreh users 'use strict'; var ArrayBufferViewCore = require('../internals/array-buffer-view-core'); var toLength = require('../internals/to-length'); var toOffset = require('../internals/to-offset'); var toObject = require('../internals/to-object'); var fails = require('../internals/fails'); var aTypedArray = ArrayBufferViewCore.aTypedArray; var exportTypedArrayMethod = ArrayBufferViewCore.exportTypedArrayMethod; var FORCED = fails(function () { // eslint-disable-next-line no-undef new Int8Array(1).set({}); }); // `%TypedArray%.prototype.set` method // https://tc39.es/ecma262/#sec-%typedarray%.prototype.set exportTypedArrayMethod('set', function set(arrayLike /* , offset */) { aTypedArray(this); var offset = toOffset(arguments.length > 1 ? arguments[1] : undefined, 1); var length = this.length; var src = toObject(arrayLike); var len = toLength(src.length); var index = 0; if (len + offset > length) throw RangeError('Wrong length'); while (index < len) this[offset + index] = src[index++]; }, FORCED); apollo-server-demo/node_modules/core-js/modules/es.object.define-properties.js 0000644 0001750 0000144 00000000576 03560116604 027307 0 ustar andreh users var $ = require('../internals/export'); var DESCRIPTORS = require('../internals/descriptors'); var defineProperties = require('../internals/object-define-properties'); // `Object.defineProperties` method // https://tc39.es/ecma262/#sec-object.defineproperties $({ target: 'Object', stat: true, forced: !DESCRIPTORS, sham: !DESCRIPTORS }, { defineProperties: defineProperties }); apollo-server-demo/node_modules/core-js/modules/esnext.set.symmetric-difference.js 0000644 0001750 0000144 00000001637 03560116604 030212 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var getBuiltIn = require('../internals/get-built-in'); var anObject = require('../internals/an-object'); var aFunction = require('../internals/a-function'); var speciesConstructor = require('../internals/species-constructor'); var iterate = require('../internals/iterate'); // `Set.prototype.symmetricDifference` method // https://github.com/tc39/proposal-set-methods $({ target: 'Set', proto: true, real: true, forced: IS_PURE }, { symmetricDifference: function symmetricDifference(iterable) { var set = anObject(this); var newSet = new (speciesConstructor(set, getBuiltIn('Set')))(set); var remover = aFunction(newSet['delete']); var adder = aFunction(newSet.add); iterate(iterable, function (value) { remover.call(newSet, value) || adder.call(newSet, value); }); return newSet; } }); apollo-server-demo/node_modules/core-js/modules/es.string.link.js 0000644 0001750 0000144 00000000661 03560116604 024653 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var createHTML = require('../internals/create-html'); var forcedStringHTMLMethod = require('../internals/string-html-forced'); // `String.prototype.link` method // https://tc39.es/ecma262/#sec-string.prototype.link $({ target: 'String', proto: true, forced: forcedStringHTMLMethod('link') }, { link: function link(url) { return createHTML(this, 'a', 'href', url); } }); apollo-server-demo/node_modules/core-js/modules/esnext.map.emplace.js 0000644 0001750 0000144 00000000506 03560116604 025470 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var $emplace = require('../internals/map-emplace'); // `Map.prototype.emplace` method // https://github.com/thumbsupep/proposal-upsert $({ target: 'Map', proto: true, real: true, forced: IS_PURE }, { emplace: $emplace }); apollo-server-demo/node_modules/core-js/modules/es.symbol.species.js 0000644 0001750 0000144 00000000306 03560116604 025344 0 ustar andreh users var defineWellKnownSymbol = require('../internals/define-well-known-symbol'); // `Symbol.species` well-known symbol // https://tc39.es/ecma262/#sec-symbol.species defineWellKnownSymbol('species'); apollo-server-demo/node_modules/core-js/modules/esnext.math.clamp.js 0000644 0001750 0000144 00000000436 03560116604 025334 0 ustar andreh users var $ = require('../internals/export'); var min = Math.min; var max = Math.max; // `Math.clamp` method // https://rwaldron.github.io/proposal-math-extensions/ $({ target: 'Math', stat: true }, { clamp: function clamp(x, lower, upper) { return min(upper, max(lower, x)); } }); apollo-server-demo/node_modules/core-js/modules/esnext.math.isubh.js 0000644 0001750 0000144 00000000625 03560116604 025352 0 ustar andreh users var $ = require('../internals/export'); // `Math.isubh` method // https://gist.github.com/BrendanEich/4294d5c212a6d2254703 // TODO: Remove from `core-js@4` $({ target: 'Math', stat: true }, { isubh: function isubh(x0, x1, y0, y1) { var $x0 = x0 >>> 0; var $x1 = x1 >>> 0; var $y0 = y0 >>> 0; return $x1 - (y1 >>> 0) - ((~$x0 & $y0 | ~($x0 ^ $y0) & $x0 - $y0 >>> 0) >>> 31) | 0; } }); apollo-server-demo/node_modules/core-js/modules/es.object.set-prototype-of.js 0000644 0001750 0000144 00000000422 03560116604 027111 0 ustar andreh users var $ = require('../internals/export'); var setPrototypeOf = require('../internals/object-set-prototype-of'); // `Object.setPrototypeOf` method // https://tc39.es/ecma262/#sec-object.setprototypeof $({ target: 'Object', stat: true }, { setPrototypeOf: setPrototypeOf }); apollo-server-demo/node_modules/core-js/modules/esnext.set.join.js 0000644 0001750 0000144 00000001312 03560116604 025033 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var anObject = require('../internals/an-object'); var getSetIterator = require('../internals/get-set-iterator'); var iterate = require('../internals/iterate'); // `Set.prototype.join` method // https://github.com/tc39/proposal-collection-methods $({ target: 'Set', proto: true, real: true, forced: IS_PURE }, { join: function join(separator) { var set = anObject(this); var iterator = getSetIterator(set); var sep = separator === undefined ? ',' : String(separator); var result = []; iterate(iterator, result.push, { that: result, IS_ITERATOR: true }); return result.join(sep); } }); apollo-server-demo/node_modules/core-js/modules/esnext.object.iterate-keys.js 0000644 0001750 0000144 00000000524 03560116604 027161 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var ObjectIterator = require('../internals/object-iterator'); // `Object.iterateKeys` method // https://github.com/tc39/proposal-object-iteration $({ target: 'Object', stat: true }, { iterateKeys: function iterateKeys(object) { return new ObjectIterator(object, 'keys'); } }); apollo-server-demo/node_modules/core-js/modules/esnext.map.key-of.js 0000644 0001750 0000144 00000001243 03560116604 025253 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var anObject = require('../internals/an-object'); var getMapIterator = require('../internals/get-map-iterator'); var iterate = require('../internals/iterate'); // `Map.prototype.includes` method // https://github.com/tc39/proposal-collection-methods $({ target: 'Map', proto: true, real: true, forced: IS_PURE }, { keyOf: function keyOf(searchElement) { return iterate(getMapIterator(anObject(this)), function (key, value, stop) { if (value === searchElement) return stop(key); }, { AS_ENTRIES: true, IS_ITERATOR: true, INTERRUPTED: true }).result; } }); apollo-server-demo/node_modules/core-js/modules/es.typed-array.find.js 0000644 0001750 0000144 00000001041 03560116604 025562 0 ustar andreh users 'use strict'; var ArrayBufferViewCore = require('../internals/array-buffer-view-core'); var $find = require('../internals/array-iteration').find; var aTypedArray = ArrayBufferViewCore.aTypedArray; var exportTypedArrayMethod = ArrayBufferViewCore.exportTypedArrayMethod; // `%TypedArray%.prototype.find` method // https://tc39.es/ecma262/#sec-%typedarray%.prototype.find exportTypedArrayMethod('find', function find(predicate /* , thisArg */) { return $find(aTypedArray(this), predicate, arguments.length > 1 ? arguments[1] : undefined); }); apollo-server-demo/node_modules/core-js/modules/es.array.is-array.js 0000644 0001750 0000144 00000000335 03560116604 025253 0 ustar andreh users var $ = require('../internals/export'); var isArray = require('../internals/is-array'); // `Array.isArray` method // https://tc39.es/ecma262/#sec-array.isarray $({ target: 'Array', stat: true }, { isArray: isArray }); apollo-server-demo/node_modules/core-js/modules/es.number.epsilon.js 0000644 0001750 0000144 00000000273 03560116604 025350 0 ustar andreh users var $ = require('../internals/export'); // `Number.EPSILON` constant // https://tc39.es/ecma262/#sec-number.epsilon $({ target: 'Number', stat: true }, { EPSILON: Math.pow(2, -52) }); apollo-server-demo/node_modules/core-js/modules/esnext.iterator.reduce.js 0000644 0001750 0000144 00000001525 03560116604 026407 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-iterator-helpers var $ = require('../internals/export'); var iterate = require('../internals/iterate'); var aFunction = require('../internals/a-function'); var anObject = require('../internals/an-object'); $({ target: 'Iterator', proto: true, real: true }, { reduce: function reduce(reducer /* , initialValue */) { anObject(this); aFunction(reducer); var noInitial = arguments.length < 2; var accumulator = noInitial ? undefined : arguments[1]; iterate(this, function (value) { if (noInitial) { noInitial = false; accumulator = value; } else { accumulator = reducer(accumulator, value); } }, { IS_ITERATOR: true }); if (noInitial) throw TypeError('Reduce of empty iterator with no initial value'); return accumulator; } }); apollo-server-demo/node_modules/core-js/modules/esnext.map.map-values.js 0000644 0001750 0000144 00000002226 03560116604 026135 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var IS_PURE = require('../internals/is-pure'); var getBuiltIn = require('../internals/get-built-in'); var anObject = require('../internals/an-object'); var aFunction = require('../internals/a-function'); var bind = require('../internals/function-bind-context'); var speciesConstructor = require('../internals/species-constructor'); var getMapIterator = require('../internals/get-map-iterator'); var iterate = require('../internals/iterate'); // `Map.prototype.mapValues` method // https://github.com/tc39/proposal-collection-methods $({ target: 'Map', proto: true, real: true, forced: IS_PURE }, { mapValues: function mapValues(callbackfn /* , thisArg */) { var map = anObject(this); var iterator = getMapIterator(map); var boundFunction = bind(callbackfn, arguments.length > 1 ? arguments[1] : undefined, 3); var newMap = new (speciesConstructor(map, getBuiltIn('Map')))(); var setter = aFunction(newMap.set); iterate(iterator, function (key, value) { setter.call(newMap, key, boundFunction(value, key, map)); }, { AS_ENTRIES: true, IS_ITERATOR: true }); return newMap; } }); apollo-server-demo/node_modules/core-js/modules/es.array.map.js 0000644 0001750 0000144 00000001400 03560116604 024273 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var $map = require('../internals/array-iteration').map; var arrayMethodHasSpeciesSupport = require('../internals/array-method-has-species-support'); var arrayMethodUsesToLength = require('../internals/array-method-uses-to-length'); var HAS_SPECIES_SUPPORT = arrayMethodHasSpeciesSupport('map'); // FF49- issue var USES_TO_LENGTH = arrayMethodUsesToLength('map'); // `Array.prototype.map` method // https://tc39.es/ecma262/#sec-array.prototype.map // with adding support of @@species $({ target: 'Array', proto: true, forced: !HAS_SPECIES_SUPPORT || !USES_TO_LENGTH }, { map: function map(callbackfn /* , thisArg */) { return $map(this, callbackfn, arguments.length > 1 ? arguments[1] : undefined); } }); apollo-server-demo/node_modules/core-js/modules/es.math.clz32.js 0000644 0001750 0000144 00000000470 03560116604 024274 0 ustar andreh users var $ = require('../internals/export'); var floor = Math.floor; var log = Math.log; var LOG2E = Math.LOG2E; // `Math.clz32` method // https://tc39.es/ecma262/#sec-math.clz32 $({ target: 'Math', stat: true }, { clz32: function clz32(x) { return (x >>>= 0) ? 31 - floor(log(x + 0.5) * LOG2E) : 32; } }); apollo-server-demo/node_modules/core-js/modules/es.typed-array.copy-within.js 0000644 0001750 0000144 00000001113 03560116604 027114 0 ustar andreh users 'use strict'; var ArrayBufferViewCore = require('../internals/array-buffer-view-core'); var $copyWithin = require('../internals/array-copy-within'); var aTypedArray = ArrayBufferViewCore.aTypedArray; var exportTypedArrayMethod = ArrayBufferViewCore.exportTypedArrayMethod; // `%TypedArray%.prototype.copyWithin` method // https://tc39.es/ecma262/#sec-%typedarray%.prototype.copywithin exportTypedArrayMethod('copyWithin', function copyWithin(target, start /* , end */) { return $copyWithin.call(aTypedArray(this), target, start, arguments.length > 2 ? arguments[2] : undefined); }); apollo-server-demo/node_modules/core-js/modules/es.object.get-own-property-descriptors.js 0000644 0001750 0000144 00000001775 03560116604 031466 0 ustar andreh users var $ = require('../internals/export'); var DESCRIPTORS = require('../internals/descriptors'); var ownKeys = require('../internals/own-keys'); var toIndexedObject = require('../internals/to-indexed-object'); var getOwnPropertyDescriptorModule = require('../internals/object-get-own-property-descriptor'); var createProperty = require('../internals/create-property'); // `Object.getOwnPropertyDescriptors` method // https://tc39.es/ecma262/#sec-object.getownpropertydescriptors $({ target: 'Object', stat: true, sham: !DESCRIPTORS }, { getOwnPropertyDescriptors: function getOwnPropertyDescriptors(object) { var O = toIndexedObject(object); var getOwnPropertyDescriptor = getOwnPropertyDescriptorModule.f; var keys = ownKeys(O); var result = {}; var index = 0; var key, descriptor; while (keys.length > index) { descriptor = getOwnPropertyDescriptor(O, key = keys[index++]); if (descriptor !== undefined) createProperty(result, key, descriptor); } return result; } }); apollo-server-demo/node_modules/core-js/modules/es.number.parse-int.js 0000644 0001750 0000144 00000000422 03560116604 025575 0 ustar andreh users var $ = require('../internals/export'); var parseInt = require('../internals/number-parse-int'); // `Number.parseInt` method // https://tc39.es/ecma262/#sec-number.parseint $({ target: 'Number', stat: true, forced: Number.parseInt != parseInt }, { parseInt: parseInt }); apollo-server-demo/node_modules/core-js/modules/es.object.is-extensible.js 0000644 0001750 0000144 00000001037 03560116604 026427 0 ustar andreh users var $ = require('../internals/export'); var fails = require('../internals/fails'); var isObject = require('../internals/is-object'); var nativeIsExtensible = Object.isExtensible; var FAILS_ON_PRIMITIVES = fails(function () { nativeIsExtensible(1); }); // `Object.isExtensible` method // https://tc39.es/ecma262/#sec-object.isextensible $({ target: 'Object', stat: true, forced: FAILS_ON_PRIMITIVES }, { isExtensible: function isExtensible(it) { return isObject(it) ? nativeIsExtensible ? nativeIsExtensible(it) : true : false; } }); apollo-server-demo/node_modules/core-js/modules/es.typed-array.uint8-array.js 0000644 0001750 0000144 00000000515 03560116604 027032 0 ustar andreh users var createTypedArrayConstructor = require('../internals/typed-array-constructor'); // `Uint8Array` constructor // https://tc39.es/ecma262/#sec-typedarray-objects createTypedArrayConstructor('Uint8', function (init) { return function Uint8Array(data, byteOffset, length) { return init(this, data, byteOffset, length); }; }); apollo-server-demo/node_modules/core-js/modules/es.string.from-code-point.js 0000644 0001750 0000144 00000001740 03560116604 026717 0 ustar andreh users var $ = require('../internals/export'); var toAbsoluteIndex = require('../internals/to-absolute-index'); var fromCharCode = String.fromCharCode; var nativeFromCodePoint = String.fromCodePoint; // length should be 1, old FF problem var INCORRECT_LENGTH = !!nativeFromCodePoint && nativeFromCodePoint.length != 1; // `String.fromCodePoint` method // https://tc39.es/ecma262/#sec-string.fromcodepoint $({ target: 'String', stat: true, forced: INCORRECT_LENGTH }, { fromCodePoint: function fromCodePoint(x) { // eslint-disable-line no-unused-vars var elements = []; var length = arguments.length; var i = 0; var code; while (length > i) { code = +arguments[i++]; if (toAbsoluteIndex(code, 0x10FFFF) !== code) throw RangeError(code + ' is not a valid code point'); elements.push(code < 0x10000 ? fromCharCode(code) : fromCharCode(((code -= 0x10000) >> 10) + 0xD800, code % 0x400 + 0xDC00) ); } return elements.join(''); } }); apollo-server-demo/node_modules/core-js/index.js 0000644 0001750 0000144 00000000173 03560116604 021440 0 ustar andreh users require('./es'); require('./proposals'); require('./web'); var path = require('./internals/path'); module.exports = path; apollo-server-demo/node_modules/core-js/stable/ 0000755 0001750 0000144 00000000000 14067647701 021257 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/queue-microtask.js 0000644 0001750 0000144 00000000112 03560116604 024712 0 ustar andreh users var parent = require('../web/queue-microtask'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/weak-map/ 0000755 0001750 0000144 00000000000 14067647701 022761 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/weak-map/index.js 0000644 0001750 0000144 00000000105 03560116604 024407 0 ustar andreh users var parent = require('../../es/weak-map'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/index.js 0000644 0001750 0000144 00000000146 03560116604 022712 0 ustar andreh users require('../es'); require('../web'); var path = require('../internals/path'); module.exports = path; apollo-server-demo/node_modules/core-js/stable/array/ 0000755 0001750 0000144 00000000000 14067647701 022375 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/array/sort.js 0000644 0001750 0000144 00000000107 03560116604 023705 0 ustar andreh users var parent = require('../../es/array/sort'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/reduce.js 0000644 0001750 0000144 00000000111 03560116604 024160 0 ustar andreh users var parent = require('../../es/array/reduce'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/some.js 0000644 0001750 0000144 00000000107 03560116604 023661 0 ustar andreh users var parent = require('../../es/array/some'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/index.js 0000644 0001750 0000144 00000000102 03560116604 024020 0 ustar andreh users var parent = require('../../es/array'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/iterator.js 0000644 0001750 0000144 00000000113 03560116604 024544 0 ustar andreh users var parent = require('../../es/array/iterator'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/copy-within.js 0000644 0001750 0000144 00000000116 03560116604 025170 0 ustar andreh users var parent = require('../../es/array/copy-within'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/map.js 0000644 0001750 0000144 00000000106 03560116604 023472 0 ustar andreh users var parent = require('../../es/array/map'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/includes.js 0000644 0001750 0000144 00000000113 03560116604 024521 0 ustar andreh users var parent = require('../../es/array/includes'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/reduce-right.js 0000644 0001750 0000144 00000000117 03560116604 025301 0 ustar andreh users var parent = require('../../es/array/reduce-right'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/every.js 0000644 0001750 0000144 00000000110 03560116604 024042 0 ustar andreh users var parent = require('../../es/array/every'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/fill.js 0000644 0001750 0000144 00000000107 03560116604 023644 0 ustar andreh users var parent = require('../../es/array/fill'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/flat-map.js 0000644 0001750 0000144 00000000113 03560116604 024414 0 ustar andreh users var parent = require('../../es/array/flat-map'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/index-of.js 0000644 0001750 0000144 00000000113 03560116604 024424 0 ustar andreh users var parent = require('../../es/array/index-of'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/virtual/ 0000755 0001750 0000144 00000000000 14067647701 024063 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/array/virtual/sort.js 0000644 0001750 0000144 00000000122 03560116604 025370 0 ustar andreh users var parent = require('../../../es/array/virtual/sort'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/virtual/reduce.js 0000644 0001750 0000144 00000000124 03560116604 025652 0 ustar andreh users var parent = require('../../../es/array/virtual/reduce'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/virtual/some.js 0000644 0001750 0000144 00000000122 03560116604 025344 0 ustar andreh users var parent = require('../../../es/array/virtual/some'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/virtual/index.js 0000644 0001750 0000144 00000000115 03560116604 025512 0 ustar andreh users var parent = require('../../../es/array/virtual'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/virtual/iterator.js 0000644 0001750 0000144 00000000126 03560116604 026236 0 ustar andreh users var parent = require('../../../es/array/virtual/iterator'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/virtual/copy-within.js 0000644 0001750 0000144 00000000131 03560116604 026653 0 ustar andreh users var parent = require('../../../es/array/virtual/copy-within'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/virtual/map.js 0000644 0001750 0000144 00000000121 03560116604 025155 0 ustar andreh users var parent = require('../../../es/array/virtual/map'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/virtual/includes.js 0000644 0001750 0000144 00000000126 03560116604 026213 0 ustar andreh users var parent = require('../../../es/array/virtual/includes'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/virtual/reduce-right.js 0000644 0001750 0000144 00000000132 03560116604 026764 0 ustar andreh users var parent = require('../../../es/array/virtual/reduce-right'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/virtual/every.js 0000644 0001750 0000144 00000000123 03560116604 025534 0 ustar andreh users var parent = require('../../../es/array/virtual/every'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/virtual/fill.js 0000644 0001750 0000144 00000000122 03560116604 025327 0 ustar andreh users var parent = require('../../../es/array/virtual/fill'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/virtual/flat-map.js 0000644 0001750 0000144 00000000126 03560116604 026106 0 ustar andreh users var parent = require('../../../es/array/virtual/flat-map'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/virtual/index-of.js 0000644 0001750 0000144 00000000126 03560116604 026116 0 ustar andreh users var parent = require('../../../es/array/virtual/index-of'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/virtual/splice.js 0000644 0001750 0000144 00000000124 03560116604 025662 0 ustar andreh users var parent = require('../../../es/array/virtual/splice'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/virtual/last-index-of.js 0000644 0001750 0000144 00000000133 03560116604 027055 0 ustar andreh users var parent = require('../../../es/array/virtual/last-index-of'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/virtual/flat.js 0000644 0001750 0000144 00000000122 03560116604 025327 0 ustar andreh users var parent = require('../../../es/array/virtual/flat'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/virtual/values.js 0000644 0001750 0000144 00000000124 03560116604 025702 0 ustar andreh users var parent = require('../../../es/array/virtual/values'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/virtual/join.js 0000644 0001750 0000144 00000000122 03560116604 025340 0 ustar andreh users var parent = require('../../../es/array/virtual/join'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/virtual/entries.js 0000644 0001750 0000144 00000000125 03560116604 026055 0 ustar andreh users var parent = require('../../../es/array/virtual/entries'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/virtual/filter.js 0000644 0001750 0000144 00000000124 03560116604 025670 0 ustar andreh users var parent = require('../../../es/array/virtual/filter'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/virtual/concat.js 0000644 0001750 0000144 00000000124 03560116604 025652 0 ustar andreh users var parent = require('../../../es/array/virtual/concat'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/virtual/for-each.js 0000644 0001750 0000144 00000000126 03560116604 026071 0 ustar andreh users var parent = require('../../../es/array/virtual/for-each'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/virtual/find-index.js 0000644 0001750 0000144 00000000130 03560116604 026425 0 ustar andreh users var parent = require('../../../es/array/virtual/find-index'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/virtual/keys.js 0000644 0001750 0000144 00000000122 03560116604 025354 0 ustar andreh users var parent = require('../../../es/array/virtual/keys'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/virtual/find.js 0000644 0001750 0000144 00000000122 03560116604 025321 0 ustar andreh users var parent = require('../../../es/array/virtual/find'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/virtual/reverse.js 0000644 0001750 0000144 00000000125 03560116604 026057 0 ustar andreh users var parent = require('../../../es/array/virtual/reverse'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/virtual/slice.js 0000644 0001750 0000144 00000000123 03560116604 025501 0 ustar andreh users var parent = require('../../../es/array/virtual/slice'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/splice.js 0000644 0001750 0000144 00000000111 03560116604 024170 0 ustar andreh users var parent = require('../../es/array/splice'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/last-index-of.js 0000644 0001750 0000144 00000000120 03560116604 025363 0 ustar andreh users var parent = require('../../es/array/last-index-of'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/flat.js 0000644 0001750 0000144 00000000107 03560116604 023644 0 ustar andreh users var parent = require('../../es/array/flat'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/values.js 0000644 0001750 0000144 00000000111 03560116604 024210 0 ustar andreh users var parent = require('../../es/array/values'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/join.js 0000644 0001750 0000144 00000000107 03560116604 023655 0 ustar andreh users var parent = require('../../es/array/join'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/from.js 0000644 0001750 0000144 00000000107 03560116604 023661 0 ustar andreh users var parent = require('../../es/array/from'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/entries.js 0000644 0001750 0000144 00000000112 03560116604 024363 0 ustar andreh users var parent = require('../../es/array/entries'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/filter.js 0000644 0001750 0000144 00000000111 03560116604 024176 0 ustar andreh users var parent = require('../../es/array/filter'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/concat.js 0000644 0001750 0000144 00000000111 03560116604 024160 0 ustar andreh users var parent = require('../../es/array/concat'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/for-each.js 0000644 0001750 0000144 00000000113 03560116604 024377 0 ustar andreh users var parent = require('../../es/array/for-each'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/is-array.js 0000644 0001750 0000144 00000000113 03560116604 024442 0 ustar andreh users var parent = require('../../es/array/is-array'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/find-index.js 0000644 0001750 0000144 00000000115 03560116604 024742 0 ustar andreh users var parent = require('../../es/array/find-index'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/keys.js 0000644 0001750 0000144 00000000107 03560116604 023671 0 ustar andreh users var parent = require('../../es/array/keys'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/find.js 0000644 0001750 0000144 00000000107 03560116604 023636 0 ustar andreh users var parent = require('../../es/array/find'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/of.js 0000644 0001750 0000144 00000000105 03560116604 023320 0 ustar andreh users var parent = require('../../es/array/of'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/reverse.js 0000644 0001750 0000144 00000000112 03560116604 024365 0 ustar andreh users var parent = require('../../es/array/reverse'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array/slice.js 0000644 0001750 0000144 00000000110 03560116604 024007 0 ustar andreh users var parent = require('../../es/array/slice'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array-buffer/ 0000755 0001750 0000144 00000000000 14067647701 023644 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/array-buffer/index.js 0000644 0001750 0000144 00000000111 03560116604 025267 0 ustar andreh users var parent = require('../../es/array-buffer'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array-buffer/is-view.js 0000644 0001750 0000144 00000000121 03560116604 025544 0 ustar andreh users var parent = require('../../es/array-buffer/is-view'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array-buffer/constructor.js 0000644 0001750 0000144 00000000125 03560116604 026552 0 ustar andreh users var parent = require('../../es/array-buffer/constructor'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/array-buffer/slice.js 0000644 0001750 0000144 00000000117 03560116604 025265 0 ustar andreh users var parent = require('../../es/array-buffer/slice'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/README.md 0000644 0001750 0000144 00000000222 03560116604 022517 0 ustar andreh users This folder contains entry points for all stable `core-js` features with dependencies. It's the recommended way for usage only required features. apollo-server-demo/node_modules/core-js/stable/symbol/ 0000755 0001750 0000144 00000000000 14067647701 022564 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/symbol/index.js 0000644 0001750 0000144 00000000103 03560116604 024210 0 ustar andreh users var parent = require('../../es/symbol'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/symbol/iterator.js 0000644 0001750 0000144 00000000114 03560116604 024734 0 ustar andreh users var parent = require('../../es/symbol/iterator'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/symbol/to-string-tag.js 0000644 0001750 0000144 00000000121 03560116604 025600 0 ustar andreh users var parent = require('../../es/symbol/to-string-tag'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/symbol/match.js 0000644 0001750 0000144 00000000111 03560116604 024174 0 ustar andreh users var parent = require('../../es/symbol/match'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/symbol/unscopables.js 0000644 0001750 0000144 00000000117 03560116604 025424 0 ustar andreh users var parent = require('../../es/symbol/unscopables'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/symbol/is-concat-spreadable.js 0000644 0001750 0000144 00000000130 03560116604 027061 0 ustar andreh users var parent = require('../../es/symbol/is-concat-spreadable'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/symbol/for.js 0000644 0001750 0000144 00000000107 03560116604 023673 0 ustar andreh users var parent = require('../../es/symbol/for'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/symbol/key-for.js 0000644 0001750 0000144 00000000113 03560116604 024456 0 ustar andreh users var parent = require('../../es/symbol/key-for'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/symbol/to-primitive.js 0000644 0001750 0000144 00000000120 03560116604 025530 0 ustar andreh users var parent = require('../../es/symbol/to-primitive'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/symbol/async-iterator.js 0000644 0001750 0000144 00000000122 03560116604 026046 0 ustar andreh users var parent = require('../../es/symbol/async-iterator'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/symbol/split.js 0000644 0001750 0000144 00000000111 03560116604 024233 0 ustar andreh users var parent = require('../../es/symbol/split'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/symbol/has-instance.js 0000644 0001750 0000144 00000000120 03560116604 025455 0 ustar andreh users var parent = require('../../es/symbol/has-instance'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/symbol/species.js 0000644 0001750 0000144 00000000113 03560116604 024535 0 ustar andreh users var parent = require('../../es/symbol/species'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/symbol/search.js 0000644 0001750 0000144 00000000112 03560116604 024346 0 ustar andreh users var parent = require('../../es/symbol/search'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/symbol/replace.js 0000644 0001750 0000144 00000000113 03560116604 024515 0 ustar andreh users var parent = require('../../es/symbol/replace'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/symbol/description.js 0000644 0001750 0000144 00000000060 03560116604 025426 0 ustar andreh users require('../../modules/es.symbol.description'); apollo-server-demo/node_modules/core-js/stable/symbol/match-all.js 0000644 0001750 0000144 00000000115 03560116604 024746 0 ustar andreh users var parent = require('../../es/symbol/match-all'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/dom-collections/ 0000755 0001750 0000144 00000000000 14067647701 024352 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/dom-collections/index.js 0000644 0001750 0000144 00000000626 03560116604 026010 0 ustar andreh users require('../../modules/web.dom-collections.for-each'); require('../../modules/web.dom-collections.iterator'); var ArrayIterators = require('../../modules/es.array.iterator'); var forEach = require('../../internals/array-for-each'); module.exports = { keys: ArrayIterators.keys, values: ArrayIterators.values, entries: ArrayIterators.entries, iterator: ArrayIterators.values, forEach: forEach }; apollo-server-demo/node_modules/core-js/stable/dom-collections/iterator.js 0000644 0001750 0000144 00000000244 03560116604 026526 0 ustar andreh users require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'values'); apollo-server-demo/node_modules/core-js/stable/dom-collections/for-each.js 0000644 0001750 0000144 00000000212 03560116604 026354 0 ustar andreh users require('../../modules/web.dom-collections.for-each'); var parent = require('../../internals/array-for-each'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/ 0000755 0001750 0000144 00000000000 14067647701 023063 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/instance/sort.js 0000644 0001750 0000144 00000000112 03560116604 024367 0 ustar andreh users var parent = require('../../es/instance/sort'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/reduce.js 0000644 0001750 0000144 00000000114 03560116604 024651 0 ustar andreh users var parent = require('../../es/instance/reduce'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/some.js 0000644 0001750 0000144 00000000112 03560116604 024343 0 ustar andreh users var parent = require('../../es/instance/some'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/copy-within.js 0000644 0001750 0000144 00000000121 03560116604 025652 0 ustar andreh users var parent = require('../../es/instance/copy-within'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/repeat.js 0000644 0001750 0000144 00000000114 03560116604 024662 0 ustar andreh users var parent = require('../../es/instance/repeat'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/map.js 0000644 0001750 0000144 00000000111 03560116604 024154 0 ustar andreh users var parent = require('../../es/instance/map'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/includes.js 0000644 0001750 0000144 00000000116 03560116604 025212 0 ustar andreh users var parent = require('../../es/instance/includes'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/reduce-right.js 0000644 0001750 0000144 00000000122 03560116604 025763 0 ustar andreh users var parent = require('../../es/instance/reduce-right'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/code-point-at.js 0000644 0001750 0000144 00000000123 03560116604 026045 0 ustar andreh users var parent = require('../../es/instance/code-point-at'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/every.js 0000644 0001750 0000144 00000000113 03560116604 024533 0 ustar andreh users var parent = require('../../es/instance/every'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/fill.js 0000644 0001750 0000144 00000000112 03560116604 024326 0 ustar andreh users var parent = require('../../es/instance/fill'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/flat-map.js 0000644 0001750 0000144 00000000116 03560116604 025105 0 ustar andreh users var parent = require('../../es/instance/flat-map'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/index-of.js 0000644 0001750 0000144 00000000116 03560116604 025115 0 ustar andreh users var parent = require('../../es/instance/index-of'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/trim-left.js 0000644 0001750 0000144 00000000117 03560116604 025310 0 ustar andreh users var parent = require('../../es/instance/trim-left'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/pad-end.js 0000644 0001750 0000144 00000000115 03560116604 024713 0 ustar andreh users var parent = require('../../es/instance/pad-end'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/bind.js 0000644 0001750 0000144 00000000112 03560116604 024314 0 ustar andreh users var parent = require('../../es/instance/bind'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/splice.js 0000644 0001750 0000144 00000000114 03560116604 024661 0 ustar andreh users var parent = require('../../es/instance/splice'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/last-index-of.js 0000644 0001750 0000144 00000000123 03560116604 026054 0 ustar andreh users var parent = require('../../es/instance/last-index-of'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/flags.js 0000644 0001750 0000144 00000000113 03560116604 024475 0 ustar andreh users var parent = require('../../es/instance/flags'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/flat.js 0000644 0001750 0000144 00000000112 03560116604 024326 0 ustar andreh users var parent = require('../../es/instance/flat'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/values.js 0000644 0001750 0000144 00000001013 03560116604 024700 0 ustar andreh users require('../../modules/web.dom-collections.iterator'); var values = require('../array/virtual/values'); var classof = require('../../internals/classof'); var ArrayPrototype = Array.prototype; var DOMIterables = { DOMTokenList: true, NodeList: true }; module.exports = function (it) { var own = it.values; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.values) // eslint-disable-next-line no-prototype-builtins || DOMIterables.hasOwnProperty(classof(it)) ? values : own; }; apollo-server-demo/node_modules/core-js/stable/instance/starts-with.js 0000644 0001750 0000144 00000000121 03560116604 025671 0 ustar andreh users var parent = require('../../es/instance/starts-with'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/entries.js 0000644 0001750 0000144 00000001020 03560116604 025050 0 ustar andreh users require('../../modules/web.dom-collections.iterator'); var entries = require('../array/virtual/entries'); var classof = require('../../internals/classof'); var ArrayPrototype = Array.prototype; var DOMIterables = { DOMTokenList: true, NodeList: true }; module.exports = function (it) { var own = it.entries; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.entries) // eslint-disable-next-line no-prototype-builtins || DOMIterables.hasOwnProperty(classof(it)) ? entries : own; }; apollo-server-demo/node_modules/core-js/stable/instance/trim-end.js 0000644 0001750 0000144 00000000116 03560116604 025123 0 ustar andreh users var parent = require('../../es/instance/trim-end'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/filter.js 0000644 0001750 0000144 00000000114 03560116604 024667 0 ustar andreh users var parent = require('../../es/instance/filter'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/trim-start.js 0000644 0001750 0000144 00000000120 03560116604 025505 0 ustar andreh users var parent = require('../../es/instance/trim-start'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/concat.js 0000644 0001750 0000144 00000000114 03560116604 024651 0 ustar andreh users var parent = require('../../es/instance/concat'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/trim.js 0000644 0001750 0000144 00000000112 03560116604 024353 0 ustar andreh users var parent = require('../../es/instance/trim'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/for-each.js 0000644 0001750 0000144 00000001021 03560116604 025064 0 ustar andreh users require('../../modules/web.dom-collections.iterator'); var forEach = require('../array/virtual/for-each'); var classof = require('../../internals/classof'); var ArrayPrototype = Array.prototype; var DOMIterables = { DOMTokenList: true, NodeList: true }; module.exports = function (it) { var own = it.forEach; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.forEach) // eslint-disable-next-line no-prototype-builtins || DOMIterables.hasOwnProperty(classof(it)) ? forEach : own; }; apollo-server-demo/node_modules/core-js/stable/instance/find-index.js 0000644 0001750 0000144 00000000120 03560116604 025424 0 ustar andreh users var parent = require('../../es/instance/find-index'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/pad-start.js 0000644 0001750 0000144 00000000117 03560116604 025304 0 ustar andreh users var parent = require('../../es/instance/pad-start'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/keys.js 0000644 0001750 0000144 00000001001 03560116604 024351 0 ustar andreh users require('../../modules/web.dom-collections.iterator'); var keys = require('../array/virtual/keys'); var classof = require('../../internals/classof'); var ArrayPrototype = Array.prototype; var DOMIterables = { DOMTokenList: true, NodeList: true }; module.exports = function (it) { var own = it.keys; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.keys) // eslint-disable-next-line no-prototype-builtins || DOMIterables.hasOwnProperty(classof(it)) ? keys : own; }; apollo-server-demo/node_modules/core-js/stable/instance/find.js 0000644 0001750 0000144 00000000112 03560116604 024320 0 ustar andreh users var parent = require('../../es/instance/find'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/replace-all.js 0000644 0001750 0000144 00000000121 03560116604 025561 0 ustar andreh users var parent = require('../../es/instance/replace-all'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/match-all.js 0000644 0001750 0000144 00000000117 03560116604 025247 0 ustar andreh users var parent = require('../../es/instance/match-all'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/reverse.js 0000644 0001750 0000144 00000000115 03560116604 025056 0 ustar andreh users var parent = require('../../es/instance/reverse'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/trim-right.js 0000644 0001750 0000144 00000000120 03560116604 025465 0 ustar andreh users var parent = require('../../es/instance/trim-right'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/ends-with.js 0000644 0001750 0000144 00000000117 03560116604 025307 0 ustar andreh users var parent = require('../../es/instance/ends-with'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/instance/slice.js 0000644 0001750 0000144 00000000113 03560116604 024500 0 ustar andreh users var parent = require('../../es/instance/slice'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/map/ 0000755 0001750 0000144 00000000000 14067647701 022034 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/map/index.js 0000644 0001750 0000144 00000000100 03560116604 023455 0 ustar andreh users var parent = require('../../es/map'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/ 0000755 0001750 0000144 00000000000 14067647701 022565 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/string/index.js 0000644 0001750 0000144 00000000103 03560116604 024211 0 ustar andreh users var parent = require('../../es/string'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/iterator.js 0000644 0001750 0000144 00000000114 03560116604 024735 0 ustar andreh users var parent = require('../../es/string/iterator'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/repeat.js 0000644 0001750 0000144 00000000112 03560116604 024362 0 ustar andreh users var parent = require('../../es/string/repeat'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/includes.js 0000644 0001750 0000144 00000000114 03560116604 024712 0 ustar andreh users var parent = require('../../es/string/includes'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/italics.js 0000644 0001750 0000144 00000000113 03560116604 024533 0 ustar andreh users var parent = require('../../es/string/italics'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/sub.js 0000644 0001750 0000144 00000000107 03560116604 023677 0 ustar andreh users var parent = require('../../es/string/sub'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/fixed.js 0000644 0001750 0000144 00000000111 03560116604 024200 0 ustar andreh users var parent = require('../../es/string/fixed'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/code-point-at.js 0000644 0001750 0000144 00000000121 03560116604 025545 0 ustar andreh users var parent = require('../../es/string/code-point-at'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/match.js 0000644 0001750 0000144 00000000111 03560116604 024175 0 ustar andreh users var parent = require('../../es/string/match'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/trim-left.js 0000644 0001750 0000144 00000000115 03560116604 025010 0 ustar andreh users var parent = require('../../es/string/trim-left'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/pad-end.js 0000644 0001750 0000144 00000000113 03560116604 024413 0 ustar andreh users var parent = require('../../es/string/pad-end'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/ 0000755 0001750 0000144 00000000000 14067647701 024253 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/string/virtual/index.js 0000644 0001750 0000144 00000000116 03560116604 025703 0 ustar andreh users var parent = require('../../../es/string/virtual'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/iterator.js 0000644 0001750 0000144 00000000127 03560116604 026427 0 ustar andreh users var parent = require('../../../es/string/virtual/iterator'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/repeat.js 0000644 0001750 0000144 00000000125 03560116604 026054 0 ustar andreh users var parent = require('../../../es/string/virtual/repeat'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/includes.js 0000644 0001750 0000144 00000000127 03560116604 026404 0 ustar andreh users var parent = require('../../../es/string/virtual/includes'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/italics.js 0000644 0001750 0000144 00000000126 03560116604 026225 0 ustar andreh users var parent = require('../../../es/string/virtual/italics'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/sub.js 0000644 0001750 0000144 00000000122 03560116604 025362 0 ustar andreh users var parent = require('../../../es/string/virtual/sub'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/fixed.js 0000644 0001750 0000144 00000000124 03560116604 025672 0 ustar andreh users var parent = require('../../../es/string/virtual/fixed'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/code-point-at.js 0000644 0001750 0000144 00000000134 03560116604 027237 0 ustar andreh users var parent = require('../../../es/string/virtual/code-point-at'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/trim-left.js 0000644 0001750 0000144 00000000130 03560116604 026473 0 ustar andreh users var parent = require('../../../es/string/virtual/trim-left'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/pad-end.js 0000644 0001750 0000144 00000000126 03560116604 026105 0 ustar andreh users var parent = require('../../../es/string/virtual/pad-end'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/small.js 0000644 0001750 0000144 00000000124 03560116604 025703 0 ustar andreh users var parent = require('../../../es/string/virtual/small'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/strike.js 0000644 0001750 0000144 00000000125 03560116604 026075 0 ustar andreh users var parent = require('../../../es/string/virtual/strike'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/bold.js 0000644 0001750 0000144 00000000123 03560116604 025512 0 ustar andreh users var parent = require('../../../es/string/virtual/bold'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/link.js 0000644 0001750 0000144 00000000123 03560116604 025527 0 ustar andreh users var parent = require('../../../es/string/virtual/link'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/big.js 0000644 0001750 0000144 00000000122 03560116604 025332 0 ustar andreh users var parent = require('../../../es/string/virtual/big'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/fontcolor.js 0000644 0001750 0000144 00000000130 03560116604 026575 0 ustar andreh users var parent = require('../../../es/string/virtual/fontcolor'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/fontsize.js 0000644 0001750 0000144 00000000127 03560116604 026437 0 ustar andreh users var parent = require('../../../es/string/virtual/fontsize'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/starts-with.js 0000644 0001750 0000144 00000000132 03560116604 027063 0 ustar andreh users var parent = require('../../../es/string/virtual/starts-with'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/trim-end.js 0000644 0001750 0000144 00000000127 03560116604 026315 0 ustar andreh users var parent = require('../../../es/string/virtual/trim-end'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/trim-start.js 0000644 0001750 0000144 00000000131 03560116604 026677 0 ustar andreh users var parent = require('../../../es/string/virtual/trim-start'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/blink.js 0000644 0001750 0000144 00000000124 03560116604 025672 0 ustar andreh users var parent = require('../../../es/string/virtual/blink'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/sup.js 0000644 0001750 0000144 00000000122 03560116604 025400 0 ustar andreh users var parent = require('../../../es/string/virtual/sup'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/trim.js 0000644 0001750 0000144 00000000123 03560116604 025545 0 ustar andreh users var parent = require('../../../es/string/virtual/trim'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/pad-start.js 0000644 0001750 0000144 00000000130 03560116604 026467 0 ustar andreh users var parent = require('../../../es/string/virtual/pad-start'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/anchor.js 0000644 0001750 0000144 00000000125 03560116604 026046 0 ustar andreh users var parent = require('../../../es/string/virtual/anchor'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/replace-all.js 0000644 0001750 0000144 00000000132 03560116604 026753 0 ustar andreh users var parent = require('../../../es/string/virtual/replace-all'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/match-all.js 0000644 0001750 0000144 00000000130 03560116604 026432 0 ustar andreh users var parent = require('../../../es/string/virtual/match-all'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/trim-right.js 0000644 0001750 0000144 00000000131 03560116604 026657 0 ustar andreh users var parent = require('../../../es/string/virtual/trim-right'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/virtual/ends-with.js 0000644 0001750 0000144 00000000130 03560116604 026472 0 ustar andreh users var parent = require('../../../es/string/virtual/ends-with'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/small.js 0000644 0001750 0000144 00000000111 03560116604 024211 0 ustar andreh users var parent = require('../../es/string/small'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/strike.js 0000644 0001750 0000144 00000000112 03560116604 024403 0 ustar andreh users var parent = require('../../es/string/strike'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/bold.js 0000644 0001750 0000144 00000000110 03560116604 024020 0 ustar andreh users var parent = require('../../es/string/bold'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/link.js 0000644 0001750 0000144 00000000110 03560116604 024035 0 ustar andreh users var parent = require('../../es/string/link'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/big.js 0000644 0001750 0000144 00000000107 03560116604 023647 0 ustar andreh users var parent = require('../../es/string/big'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/fontcolor.js 0000644 0001750 0000144 00000000115 03560116604 025112 0 ustar andreh users var parent = require('../../es/string/fontcolor'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/split.js 0000644 0001750 0000144 00000000111 03560116604 024234 0 ustar andreh users var parent = require('../../es/string/split'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/fontsize.js 0000644 0001750 0000144 00000000114 03560116604 024745 0 ustar andreh users var parent = require('../../es/string/fontsize'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/starts-with.js 0000644 0001750 0000144 00000000117 03560116604 025400 0 ustar andreh users var parent = require('../../es/string/starts-with'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/trim-end.js 0000644 0001750 0000144 00000000114 03560116604 024623 0 ustar andreh users var parent = require('../../es/string/trim-end'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/search.js 0000644 0001750 0000144 00000000112 03560116604 024347 0 ustar andreh users var parent = require('../../es/string/search'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/replace.js 0000644 0001750 0000144 00000000113 03560116604 024516 0 ustar andreh users var parent = require('../../es/string/replace'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/trim-start.js 0000644 0001750 0000144 00000000116 03560116604 025214 0 ustar andreh users var parent = require('../../es/string/trim-start'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/blink.js 0000644 0001750 0000144 00000000111 03560116604 024200 0 ustar andreh users var parent = require('../../es/string/blink'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/sup.js 0000644 0001750 0000144 00000000107 03560116604 023715 0 ustar andreh users var parent = require('../../es/string/sup'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/trim.js 0000644 0001750 0000144 00000000110 03560116604 024053 0 ustar andreh users var parent = require('../../es/string/trim'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/pad-start.js 0000644 0001750 0000144 00000000115 03560116604 025004 0 ustar andreh users var parent = require('../../es/string/pad-start'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/anchor.js 0000644 0001750 0000144 00000000112 03560116604 024354 0 ustar andreh users var parent = require('../../es/string/anchor'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/replace-all.js 0000644 0001750 0000144 00000000117 03560116604 025270 0 ustar andreh users var parent = require('../../es/string/replace-all'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/match-all.js 0000644 0001750 0000144 00000000115 03560116604 024747 0 ustar andreh users var parent = require('../../es/string/match-all'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/trim-right.js 0000644 0001750 0000144 00000000116 03560116604 025174 0 ustar andreh users var parent = require('../../es/string/trim-right'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/from-code-point.js 0000644 0001750 0000144 00000000123 03560116604 026106 0 ustar andreh users var parent = require('../../es/string/from-code-point'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/ends-with.js 0000644 0001750 0000144 00000000115 03560116604 025007 0 ustar andreh users var parent = require('../../es/string/ends-with'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/string/raw.js 0000644 0001750 0000144 00000000107 03560116604 023677 0 ustar andreh users var parent = require('../../es/string/raw'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/date/ 0000755 0001750 0000144 00000000000 14067647701 022174 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/date/index.js 0000644 0001750 0000144 00000000101 03560116604 023616 0 ustar andreh users var parent = require('../../es/date'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/date/to-iso-string.js 0000644 0001750 0000144 00000000117 03560116604 025234 0 ustar andreh users var parent = require('../../es/date/to-iso-string'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/date/now.js 0000644 0001750 0000144 00000000105 03560116604 023316 0 ustar andreh users var parent = require('../../es/date/now'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/date/to-primitive.js 0000644 0001750 0000144 00000000116 03560116604 025145 0 ustar andreh users var parent = require('../../es/date/to-primitive'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/date/to-json.js 0000644 0001750 0000144 00000000111 03560116604 024101 0 ustar andreh users var parent = require('../../es/date/to-json'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/date/to-string.js 0000644 0001750 0000144 00000000113 03560116604 024440 0 ustar andreh users var parent = require('../../es/date/to-string'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/function/ 0000755 0001750 0000144 00000000000 14067647701 023104 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/function/index.js 0000644 0001750 0000144 00000000105 03560116604 024532 0 ustar andreh users var parent = require('../../es/function'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/function/virtual/ 0000755 0001750 0000144 00000000000 14067647701 024572 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/function/virtual/index.js 0000644 0001750 0000144 00000000120 03560116604 026215 0 ustar andreh users var parent = require('../../../es/function/virtual'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/function/virtual/bind.js 0000644 0001750 0000144 00000000125 03560116604 026027 0 ustar andreh users var parent = require('../../../es/function/virtual/bind'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/function/bind.js 0000644 0001750 0000144 00000000112 03560116604 024335 0 ustar andreh users var parent = require('../../es/function/bind'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/function/has-instance.js 0000644 0001750 0000144 00000000122 03560116604 025777 0 ustar andreh users var parent = require('../../es/function/has-instance'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/function/name.js 0000644 0001750 0000144 00000000112 03560116604 024341 0 ustar andreh users var parent = require('../../es/function/name'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/set-immediate.js 0000644 0001750 0000144 00000000163 03560116604 024331 0 ustar andreh users require('../modules/web.immediate'); var path = require('../internals/path'); module.exports = path.setImmediate; apollo-server-demo/node_modules/core-js/stable/global-this.js 0000644 0001750 0000144 00000000105 03560116604 024003 0 ustar andreh users var parent = require('../es/global-this'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/number/ 0000755 0001750 0000144 00000000000 14067647701 022547 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/number/index.js 0000644 0001750 0000144 00000000103 03560116604 024173 0 ustar andreh users var parent = require('../../es/number'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/number/epsilon.js 0000644 0001750 0000144 00000000113 03560116604 024536 0 ustar andreh users var parent = require('../../es/number/epsilon'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/number/to-fixed.js 0000644 0001750 0000144 00000000114 03560116604 024605 0 ustar andreh users var parent = require('../../es/number/to-fixed'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/number/virtual/ 0000755 0001750 0000144 00000000000 14067647701 024235 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/number/virtual/index.js 0000644 0001750 0000144 00000000116 03560116604 025665 0 ustar andreh users var parent = require('../../../es/number/virtual'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/number/virtual/to-fixed.js 0000644 0001750 0000144 00000000127 03560116604 026277 0 ustar andreh users var parent = require('../../../es/number/virtual/to-fixed'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/number/virtual/to-precision.js 0000644 0001750 0000144 00000000133 03560116604 027170 0 ustar andreh users var parent = require('../../../es/number/virtual/to-precision'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/number/constructor.js 0000644 0001750 0000144 00000000117 03560116604 025456 0 ustar andreh users var parent = require('../../es/number/constructor'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/number/min-safe-integer.js 0000644 0001750 0000144 00000000124 03560116604 026221 0 ustar andreh users var parent = require('../../es/number/min-safe-integer'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/number/max-safe-integer.js 0000644 0001750 0000144 00000000124 03560116604 026223 0 ustar andreh users var parent = require('../../es/number/max-safe-integer'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/number/is-integer.js 0000644 0001750 0000144 00000000116 03560116604 025136 0 ustar andreh users var parent = require('../../es/number/is-integer'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/number/to-precision.js 0000644 0001750 0000144 00000000120 03560116604 025476 0 ustar andreh users var parent = require('../../es/number/to-precision'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/number/parse-float.js 0000644 0001750 0000144 00000000117 03560116604 025306 0 ustar andreh users var parent = require('../../es/number/parse-float'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/number/parse-int.js 0000644 0001750 0000144 00000000115 03560116604 024771 0 ustar andreh users var parent = require('../../es/number/parse-int'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/number/is-nan.js 0000644 0001750 0000144 00000000112 03560116604 024251 0 ustar andreh users var parent = require('../../es/number/is-nan'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/number/is-finite.js 0000644 0001750 0000144 00000000115 03560116604 024756 0 ustar andreh users var parent = require('../../es/number/is-finite'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/number/is-safe-integer.js 0000644 0001750 0000144 00000000123 03560116604 026050 0 ustar andreh users var parent = require('../../es/number/is-safe-integer'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/parse-float.js 0000644 0001750 0000144 00000000105 03560116604 024013 0 ustar andreh users var parent = require('../es/parse-float'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/promise/ 0000755 0001750 0000144 00000000000 14067647701 022735 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/promise/index.js 0000644 0001750 0000144 00000000104 03560116604 024362 0 ustar andreh users var parent = require('../../es/promise'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/promise/finally.js 0000644 0001750 0000144 00000000114 03560116604 024712 0 ustar andreh users var parent = require('../../es/promise/finally'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/promise/all-settled.js 0000644 0001750 0000144 00000000120 03560116604 025463 0 ustar andreh users var parent = require('../../es/promise/all-settled'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/promise/any.js 0000644 0001750 0000144 00000000110 03560116604 024037 0 ustar andreh users var parent = require('../../es/promise/any'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/clear-immediate.js 0000644 0001750 0000144 00000000165 03560116604 024626 0 ustar andreh users require('../modules/web.immediate'); var path = require('../internals/path'); module.exports = path.clearImmediate; apollo-server-demo/node_modules/core-js/stable/set-interval.js 0000644 0001750 0000144 00000000157 03560116604 024222 0 ustar andreh users require('../modules/web.timers'); var path = require('../internals/path'); module.exports = path.setInterval; apollo-server-demo/node_modules/core-js/stable/aggregate-error.js 0000644 0001750 0000144 00000000231 03560116604 024653 0 ustar andreh users // TODO: remove from `core-js@4` require('../modules/esnext.aggregate-error'); var parent = require('../es/aggregate-error'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/object/ 0000755 0001750 0000144 00000000000 14067647701 022525 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/object/get-own-property-names.js 0000644 0001750 0000144 00000000132 03560116604 027407 0 ustar andreh users var parent = require('../../es/object/get-own-property-names'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/object/index.js 0000644 0001750 0000144 00000000103 03560116604 024151 0 ustar andreh users var parent = require('../../es/object'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/object/lookup-getter.js 0000644 0001750 0000144 00000000121 03560116604 025643 0 ustar andreh users var parent = require('../../es/object/lookup-getter'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/object/prevent-extensions.js 0000644 0001750 0000144 00000000126 03560116604 026727 0 ustar andreh users var parent = require('../../es/object/prevent-extensions'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/object/get-own-property-descriptor.js 0000644 0001750 0000144 00000000137 03560116604 030467 0 ustar andreh users var parent = require('../../es/object/get-own-property-descriptor'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/object/get-prototype-of.js 0000644 0001750 0000144 00000000124 03560116604 026271 0 ustar andreh users var parent = require('../../es/object/get-prototype-of'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/object/define-getter.js 0000644 0001750 0000144 00000000121 03560116604 025564 0 ustar andreh users var parent = require('../../es/object/define-getter'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/object/is-sealed.js 0000644 0001750 0000144 00000000115 03560116604 024713 0 ustar andreh users var parent = require('../../es/object/is-sealed'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/object/assign.js 0000644 0001750 0000144 00000000112 03560116604 024326 0 ustar andreh users var parent = require('../../es/object/assign'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/object/define-property.js 0000644 0001750 0000144 00000000123 03560116604 026160 0 ustar andreh users var parent = require('../../es/object/define-property'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/object/get-own-property-symbols.js 0000644 0001750 0000144 00000000134 03560116604 027776 0 ustar andreh users var parent = require('../../es/object/get-own-property-symbols'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/object/create.js 0000644 0001750 0000144 00000000112 03560116604 024305 0 ustar andreh users var parent = require('../../es/object/create'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/object/values.js 0000644 0001750 0000144 00000000112 03560116604 024341 0 ustar andreh users var parent = require('../../es/object/values'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/object/define-setter.js 0000644 0001750 0000144 00000000121 03560116604 025600 0 ustar andreh users var parent = require('../../es/object/define-setter'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/object/entries.js 0000644 0001750 0000144 00000000113 03560116604 024514 0 ustar andreh users var parent = require('../../es/object/entries'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/object/freeze.js 0000644 0001750 0000144 00000000112 03560116604 024322 0 ustar andreh users var parent = require('../../es/object/freeze'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/object/get-own-property-descriptors.js 0000644 0001750 0000144 00000000140 03560116604 030644 0 ustar andreh users var parent = require('../../es/object/get-own-property-descriptors'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/object/set-prototype-of.js 0000644 0001750 0000144 00000000124 03560116604 026305 0 ustar andreh users var parent = require('../../es/object/set-prototype-of'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/object/is.js 0000644 0001750 0000144 00000000106 03560116604 023460 0 ustar andreh users var parent = require('../../es/object/is'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/object/seal.js 0000644 0001750 0000144 00000000110 03560116604 023764 0 ustar andreh users var parent = require('../../es/object/seal'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/object/keys.js 0000644 0001750 0000144 00000000110 03560116604 024013 0 ustar andreh users var parent = require('../../es/object/keys'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/object/from-entries.js 0000644 0001750 0000144 00000000120 03560116604 025453 0 ustar andreh users var parent = require('../../es/object/from-entries'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/object/to-string.js 0000644 0001750 0000144 00000000115 03560116604 024773 0 ustar andreh users var parent = require('../../es/object/to-string'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/object/is-extensible.js 0000644 0001750 0000144 00000000121 03560116604 025615 0 ustar andreh users var parent = require('../../es/object/is-extensible'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/object/lookup-setter.js 0000644 0001750 0000144 00000000121 03560116604 025657 0 ustar andreh users var parent = require('../../es/object/lookup-setter'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/object/is-frozen.js 0000644 0001750 0000144 00000000115 03560116604 024761 0 ustar andreh users var parent = require('../../es/object/is-frozen'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/object/define-properties.js 0000644 0001750 0000144 00000000125 03560116604 026472 0 ustar andreh users var parent = require('../../es/object/define-properties'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/set-timeout.js 0000644 0001750 0000144 00000000156 03560116604 024063 0 ustar andreh users require('../modules/web.timers'); var path = require('../internals/path'); module.exports = path.setTimeout; apollo-server-demo/node_modules/core-js/stable/parse-int.js 0000644 0001750 0000144 00000000103 03560116604 023476 0 ustar andreh users var parent = require('../es/parse-int'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/set/ 0000755 0001750 0000144 00000000000 14067647701 022052 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/set/index.js 0000644 0001750 0000144 00000000100 03560116604 023473 0 ustar andreh users var parent = require('../../es/set'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/url-search-params/ 0000755 0001750 0000144 00000000000 14067647701 024605 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/url-search-params/index.js 0000644 0001750 0000144 00000000117 03560116604 026236 0 ustar andreh users var parent = require('../../web/url-search-params'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/data-view/ 0000755 0001750 0000144 00000000000 14067647701 023140 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/data-view/index.js 0000644 0001750 0000144 00000000106 03560116604 024567 0 ustar andreh users var parent = require('../../es/data-view'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/json/ 0000755 0001750 0000144 00000000000 14067647701 022230 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/json/index.js 0000644 0001750 0000144 00000000101 03560116604 023652 0 ustar andreh users var parent = require('../../es/json'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/json/to-string-tag.js 0000644 0001750 0000144 00000000117 03560116604 025251 0 ustar andreh users var parent = require('../../es/json/to-string-tag'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/json/stringify.js 0000644 0001750 0000144 00000000113 03560116604 024564 0 ustar andreh users var parent = require('../../es/json/stringify'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/math/ 0000755 0001750 0000144 00000000000 14067647701 022210 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/math/index.js 0000644 0001750 0000144 00000000101 03560116604 023632 0 ustar andreh users var parent = require('../../es/math'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/math/imul.js 0000644 0001750 0000144 00000000106 03560116604 023476 0 ustar andreh users var parent = require('../../es/math/imul'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/math/cosh.js 0000644 0001750 0000144 00000000106 03560116604 023464 0 ustar andreh users var parent = require('../../es/math/cosh'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/math/fround.js 0000644 0001750 0000144 00000000110 03560116604 024020 0 ustar andreh users var parent = require('../../es/math/fround'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/math/trunc.js 0000644 0001750 0000144 00000000107 03560116604 023664 0 ustar andreh users var parent = require('../../es/math/trunc'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/math/to-string-tag.js 0000644 0001750 0000144 00000000117 03560116604 025231 0 ustar andreh users var parent = require('../../es/math/to-string-tag'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/math/acosh.js 0000644 0001750 0000144 00000000107 03560116604 023626 0 ustar andreh users var parent = require('../../es/math/acosh'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/math/clz32.js 0000644 0001750 0000144 00000000107 03560116604 023466 0 ustar andreh users var parent = require('../../es/math/clz32'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/math/sign.js 0000644 0001750 0000144 00000000106 03560116604 023470 0 ustar andreh users var parent = require('../../es/math/sign'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/math/expm1.js 0000644 0001750 0000144 00000000107 03560116604 023563 0 ustar andreh users var parent = require('../../es/math/expm1'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/math/atanh.js 0000644 0001750 0000144 00000000107 03560116604 023624 0 ustar andreh users var parent = require('../../es/math/atanh'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/math/sinh.js 0000644 0001750 0000144 00000000106 03560116604 023471 0 ustar andreh users var parent = require('../../es/math/sinh'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/math/log1p.js 0000644 0001750 0000144 00000000107 03560116604 023553 0 ustar andreh users var parent = require('../../es/math/log1p'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/math/log10.js 0000644 0001750 0000144 00000000107 03560116604 023453 0 ustar andreh users var parent = require('../../es/math/log10'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/math/asinh.js 0000644 0001750 0000144 00000000107 03560116604 023633 0 ustar andreh users var parent = require('../../es/math/asinh'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/math/log2.js 0000644 0001750 0000144 00000000106 03560116604 023373 0 ustar andreh users var parent = require('../../es/math/log2'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/math/hypot.js 0000644 0001750 0000144 00000000107 03560116604 023674 0 ustar andreh users var parent = require('../../es/math/hypot'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/math/tanh.js 0000644 0001750 0000144 00000000106 03560116604 023462 0 ustar andreh users var parent = require('../../es/math/tanh'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/math/cbrt.js 0000644 0001750 0000144 00000000106 03560116604 023462 0 ustar andreh users var parent = require('../../es/math/cbrt'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/url/ 0000755 0001750 0000144 00000000000 14067647701 022061 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/url/index.js 0000644 0001750 0000144 00000000101 03560116604 023503 0 ustar andreh users var parent = require('../../web/url'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/url/to-json.js 0000644 0001750 0000144 00000000052 03560116604 023772 0 ustar andreh users require('../../modules/web.url.to-json'); apollo-server-demo/node_modules/core-js/stable/typed-array/ 0000755 0001750 0000144 00000000000 14067647701 023520 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/typed-array/int32-array.js 0000644 0001750 0000144 00000000124 03560116604 026113 0 ustar andreh users var parent = require('../../es/typed-array/int32-array'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/typed-array/uint8-array.js 0000644 0001750 0000144 00000000124 03560116604 026223 0 ustar andreh users var parent = require('../../es/typed-array/uint8-array'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/typed-array/sort.js 0000644 0001750 0000144 00000000056 03560116604 025033 0 ustar andreh users require('../../modules/es.typed-array.sort'); apollo-server-demo/node_modules/core-js/stable/typed-array/to-locale-string.js 0000644 0001750 0000144 00000000072 03560116604 027225 0 ustar andreh users require('../../modules/es.typed-array.to-locale-string'); apollo-server-demo/node_modules/core-js/stable/typed-array/reduce.js 0000644 0001750 0000144 00000000060 03560116604 025306 0 ustar andreh users require('../../modules/es.typed-array.reduce'); apollo-server-demo/node_modules/core-js/stable/typed-array/set.js 0000644 0001750 0000144 00000000055 03560116604 024636 0 ustar andreh users require('../../modules/es.typed-array.set'); apollo-server-demo/node_modules/core-js/stable/typed-array/some.js 0000644 0001750 0000144 00000000056 03560116604 025007 0 ustar andreh users require('../../modules/es.typed-array.some'); apollo-server-demo/node_modules/core-js/stable/typed-array/index.js 0000644 0001750 0000144 00000000110 03560116604 025142 0 ustar andreh users var parent = require('../../es/typed-array'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/typed-array/iterator.js 0000644 0001750 0000144 00000000062 03560116604 025672 0 ustar andreh users require('../../modules/es.typed-array.iterator'); apollo-server-demo/node_modules/core-js/stable/typed-array/float32-array.js 0000644 0001750 0000144 00000000126 03560116604 026430 0 ustar andreh users var parent = require('../../es/typed-array/float32-array'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/typed-array/copy-within.js 0000644 0001750 0000144 00000000065 03560116604 026316 0 ustar andreh users require('../../modules/es.typed-array.copy-within'); apollo-server-demo/node_modules/core-js/stable/typed-array/map.js 0000644 0001750 0000144 00000000055 03560116604 024620 0 ustar andreh users require('../../modules/es.typed-array.map'); apollo-server-demo/node_modules/core-js/stable/typed-array/uint16-array.js 0000644 0001750 0000144 00000000125 03560116604 026303 0 ustar andreh users var parent = require('../../es/typed-array/uint16-array'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/typed-array/includes.js 0000644 0001750 0000144 00000000062 03560116604 025647 0 ustar andreh users require('../../modules/es.typed-array.includes'); apollo-server-demo/node_modules/core-js/stable/typed-array/reduce-right.js 0000644 0001750 0000144 00000000066 03560116604 026427 0 ustar andreh users require('../../modules/es.typed-array.reduce-right'); apollo-server-demo/node_modules/core-js/stable/typed-array/every.js 0000644 0001750 0000144 00000000057 03560116604 025177 0 ustar andreh users require('../../modules/es.typed-array.every'); apollo-server-demo/node_modules/core-js/stable/typed-array/fill.js 0000644 0001750 0000144 00000000056 03560116604 024772 0 ustar andreh users require('../../modules/es.typed-array.fill'); apollo-server-demo/node_modules/core-js/stable/typed-array/index-of.js 0000644 0001750 0000144 00000000062 03560116604 025552 0 ustar andreh users require('../../modules/es.typed-array.index-of'); apollo-server-demo/node_modules/core-js/stable/typed-array/last-index-of.js 0000644 0001750 0000144 00000000067 03560116604 026520 0 ustar andreh users require('../../modules/es.typed-array.last-index-of'); apollo-server-demo/node_modules/core-js/stable/typed-array/uint32-array.js 0000644 0001750 0000144 00000000125 03560116604 026301 0 ustar andreh users var parent = require('../../es/typed-array/uint32-array'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/typed-array/values.js 0000644 0001750 0000144 00000000062 03560116604 025340 0 ustar andreh users require('../../modules/es.typed-array.iterator'); apollo-server-demo/node_modules/core-js/stable/typed-array/int8-array.js 0000644 0001750 0000144 00000000123 03560116604 026035 0 ustar andreh users var parent = require('../../es/typed-array/int8-array'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/typed-array/join.js 0000644 0001750 0000144 00000000056 03560116604 025003 0 ustar andreh users require('../../modules/es.typed-array.join'); apollo-server-demo/node_modules/core-js/stable/typed-array/from.js 0000644 0001750 0000144 00000000056 03560116604 025007 0 ustar andreh users require('../../modules/es.typed-array.from'); apollo-server-demo/node_modules/core-js/stable/typed-array/entries.js 0000644 0001750 0000144 00000000062 03560116604 025512 0 ustar andreh users require('../../modules/es.typed-array.iterator'); apollo-server-demo/node_modules/core-js/stable/typed-array/filter.js 0000644 0001750 0000144 00000000060 03560116604 025324 0 ustar andreh users require('../../modules/es.typed-array.filter'); apollo-server-demo/node_modules/core-js/stable/typed-array/for-each.js 0000644 0001750 0000144 00000000062 03560116604 025525 0 ustar andreh users require('../../modules/es.typed-array.for-each'); apollo-server-demo/node_modules/core-js/stable/typed-array/find-index.js 0000644 0001750 0000144 00000000064 03560116604 026070 0 ustar andreh users require('../../modules/es.typed-array.find-index'); apollo-server-demo/node_modules/core-js/stable/typed-array/keys.js 0000644 0001750 0000144 00000000062 03560116604 025014 0 ustar andreh users require('../../modules/es.typed-array.iterator'); apollo-server-demo/node_modules/core-js/stable/typed-array/find.js 0000644 0001750 0000144 00000000056 03560116604 024764 0 ustar andreh users require('../../modules/es.typed-array.find'); apollo-server-demo/node_modules/core-js/stable/typed-array/to-string.js 0000644 0001750 0000144 00000000063 03560116604 025770 0 ustar andreh users require('../../modules/es.typed-array.to-string'); apollo-server-demo/node_modules/core-js/stable/typed-array/int16-array.js 0000644 0001750 0000144 00000000124 03560116604 026115 0 ustar andreh users var parent = require('../../es/typed-array/int16-array'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/typed-array/of.js 0000644 0001750 0000144 00000000054 03560116604 024446 0 ustar andreh users require('../../modules/es.typed-array.of'); apollo-server-demo/node_modules/core-js/stable/typed-array/reverse.js 0000644 0001750 0000144 00000000061 03560116604 025513 0 ustar andreh users require('../../modules/es.typed-array.reverse'); apollo-server-demo/node_modules/core-js/stable/typed-array/subarray.js 0000644 0001750 0000144 00000000062 03560116604 025671 0 ustar andreh users require('../../modules/es.typed-array.subarray'); apollo-server-demo/node_modules/core-js/stable/typed-array/uint8-clamped-array.js 0000644 0001750 0000144 00000000134 03560116604 027627 0 ustar andreh users var parent = require('../../es/typed-array/uint8-clamped-array'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/typed-array/float64-array.js 0000644 0001750 0000144 00000000126 03560116604 026435 0 ustar andreh users var parent = require('../../es/typed-array/float64-array'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/typed-array/slice.js 0000644 0001750 0000144 00000000057 03560116604 025144 0 ustar andreh users require('../../modules/es.typed-array.slice'); apollo-server-demo/node_modules/core-js/stable/weak-set/ 0000755 0001750 0000144 00000000000 14067647701 022777 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/weak-set/index.js 0000644 0001750 0000144 00000000105 03560116604 024425 0 ustar andreh users var parent = require('../../es/weak-set'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/reflect/ 0000755 0001750 0000144 00000000000 14067647701 022703 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/reflect/set.js 0000644 0001750 0000144 00000000110 03560116604 024011 0 ustar andreh users var parent = require('../../es/reflect/set'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/reflect/index.js 0000644 0001750 0000144 00000000104 03560116604 024330 0 ustar andreh users var parent = require('../../es/reflect'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/reflect/prevent-extensions.js 0000644 0001750 0000144 00000000127 03560116604 027106 0 ustar andreh users var parent = require('../../es/reflect/prevent-extensions'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/reflect/get-own-property-descriptor.js 0000644 0001750 0000144 00000000140 03560116604 030637 0 ustar andreh users var parent = require('../../es/reflect/get-own-property-descriptor'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/reflect/delete-property.js 0000644 0001750 0000144 00000000124 03560116604 026347 0 ustar andreh users var parent = require('../../es/reflect/delete-property'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/reflect/get-prototype-of.js 0000644 0001750 0000144 00000000125 03560116604 026450 0 ustar andreh users var parent = require('../../es/reflect/get-prototype-of'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/reflect/to-string-tag.js 0000644 0001750 0000144 00000000120 03560116604 025716 0 ustar andreh users require('../../modules/es.reflect.to-string-tag'); module.exports = 'Reflect'; apollo-server-demo/node_modules/core-js/stable/reflect/define-property.js 0000644 0001750 0000144 00000000124 03560116604 026337 0 ustar andreh users var parent = require('../../es/reflect/define-property'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/reflect/own-keys.js 0000644 0001750 0000144 00000000115 03560116604 024777 0 ustar andreh users var parent = require('../../es/reflect/own-keys'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/reflect/apply.js 0000644 0001750 0000144 00000000112 03560116604 024345 0 ustar andreh users var parent = require('../../es/reflect/apply'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/reflect/get.js 0000644 0001750 0000144 00000000110 03560116604 023775 0 ustar andreh users var parent = require('../../es/reflect/get'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/reflect/set-prototype-of.js 0000644 0001750 0000144 00000000125 03560116604 026464 0 ustar andreh users var parent = require('../../es/reflect/set-prototype-of'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/reflect/construct.js 0000644 0001750 0000144 00000000116 03560116604 025250 0 ustar andreh users var parent = require('../../es/reflect/construct'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/reflect/is-extensible.js 0000644 0001750 0000144 00000000122 03560116604 025774 0 ustar andreh users var parent = require('../../es/reflect/is-extensible'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/reflect/has.js 0000644 0001750 0000144 00000000110 03560116604 023771 0 ustar andreh users var parent = require('../../es/reflect/has'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/regexp/ 0000755 0001750 0000144 00000000000 14067647701 022551 5 ustar andreh users apollo-server-demo/node_modules/core-js/stable/regexp/index.js 0000644 0001750 0000144 00000000103 03560116604 024175 0 ustar andreh users var parent = require('../../es/regexp'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/regexp/match.js 0000644 0001750 0000144 00000000111 03560116604 024161 0 ustar andreh users var parent = require('../../es/regexp/match'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/regexp/sticky.js 0000644 0001750 0000144 00000000112 03560116604 024374 0 ustar andreh users var parent = require('../../es/regexp/sticky'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/regexp/flags.js 0000644 0001750 0000144 00000000111 03560116604 024161 0 ustar andreh users var parent = require('../../es/regexp/flags'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/regexp/constructor.js 0000644 0001750 0000144 00000000117 03560116604 025460 0 ustar andreh users var parent = require('../../es/regexp/constructor'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/regexp/test.js 0000644 0001750 0000144 00000000110 03560116604 024043 0 ustar andreh users var parent = require('../../es/regexp/test'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/regexp/split.js 0000644 0001750 0000144 00000000111 03560116604 024220 0 ustar andreh users var parent = require('../../es/regexp/split'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/regexp/search.js 0000644 0001750 0000144 00000000112 03560116604 024333 0 ustar andreh users var parent = require('../../es/regexp/search'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/regexp/replace.js 0000644 0001750 0000144 00000000113 03560116604 024502 0 ustar andreh users var parent = require('../../es/regexp/replace'); module.exports = parent; apollo-server-demo/node_modules/core-js/stable/regexp/to-string.js 0000644 0001750 0000144 00000000115 03560116604 025017 0 ustar andreh users var parent = require('../../es/regexp/to-string'); module.exports = parent; apollo-server-demo/node_modules/core-js/LICENSE 0000644 0001750 0000144 00000002050 03560116604 020774 0 ustar andreh users Copyright (c) 2014-2021 Denis Pushkarev Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/core-js/postinstall.js 0000644 0001750 0000144 00000004132 03560116604 022704 0 ustar andreh users /* eslint-disable max-len */ var fs = require('fs'); var os = require('os'); var path = require('path'); var env = process.env; var ADBLOCK = is(env.ADBLOCK); var COLOR = is(env.npm_config_color); var DISABLE_OPENCOLLECTIVE = is(env.DISABLE_OPENCOLLECTIVE); var SILENT = ['silent', 'error', 'warn'].indexOf(env.npm_config_loglevel) !== -1; var OPEN_SOURCE_CONTRIBUTOR = is(env.OPEN_SOURCE_CONTRIBUTOR); var MINUTE = 60 * 1000; // you could add a PR with an env variable for your CI detection var CI = [ 'BUILD_NUMBER', 'CI', 'CONTINUOUS_INTEGRATION', 'DRONE', 'RUN_ID' ].some(function (it) { return is(env[it]); }); var BANNER = '\u001B[96mThank you for using core-js (\u001B[94m https://github.com/zloirock/core-js \u001B[96m) for polyfilling JavaScript standard library!\u001B[0m\n\n' + '\u001B[96mThe project needs your help! Please consider supporting of core-js on Open Collective or Patreon: \u001B[0m\n' + '\u001B[96m>\u001B[94m https://opencollective.com/core-js \u001B[0m\n' + '\u001B[96m>\u001B[94m https://www.patreon.com/zloirock \u001B[0m\n\n' + '\u001B[96mAlso, the author of core-js (\u001B[94m https://github.com/zloirock \u001B[96m) is looking for a good job -)\u001B[0m\n'; function is(it) { return !!it && it !== '0' && it !== 'false'; } function isBannerRequired() { if (ADBLOCK || CI || DISABLE_OPENCOLLECTIVE || SILENT || OPEN_SOURCE_CONTRIBUTOR) return false; var file = path.join(os.tmpdir(), 'core-js-banners'); var banners = []; try { var DELTA = Date.now() - fs.statSync(file).mtime; if (DELTA >= 0 && DELTA < MINUTE * 3) { banners = JSON.parse(fs.readFileSync(file, 'utf8')); if (banners.indexOf(BANNER) !== -1) return false; } } catch (error) { banners = []; } try { banners.push(BANNER); fs.writeFileSync(file, JSON.stringify(banners), 'utf8'); } catch (error) { /* empty */ } return true; } function showBanner() { // eslint-disable-next-line no-console,no-control-regex console.log(COLOR ? BANNER : BANNER.replace(/\u001B\[\d+m/g, '')); } if (isBannerRequired()) showBanner(); apollo-server-demo/node_modules/core-js/README.md 0000644 0001750 0000144 00000012605 03560116604 021255 0 ustar andreh users # core-js [](#sponsors) [](#backers) [](https://gitter.im/zloirock/core-js?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge) [](https://www.npmjs.com/package/core-js) [](http://npm-stat.com/charts.html?package=core-js&author=&from=2014-11-18) [](https://github.com/zloirock/core-js/actions) [](https://github.com/zloirock/core-js/actions) > Modular standard library for JavaScript. Includes polyfills for [ECMAScript up to 2021](https://github.com/zloirock/core-js#ecmascript): [promises](https://github.com/zloirock/core-js#ecmascript-promise), [symbols](https://github.com/zloirock/core-js#ecmascript-symbol), [collections](https://github.com/zloirock/core-js#ecmascript-collections), iterators, [typed arrays](https://github.com/zloirock/core-js#ecmascript-typed-arrays), many other features, [ECMAScript proposals](https://github.com/zloirock/core-js#ecmascript-proposals), [some cross-platform WHATWG / W3C features and proposals](#web-standards) like [`URL`](https://github.com/zloirock/core-js#url-and-urlsearchparams). You can load only required features or use it without global namespace pollution. ## As advertising: the author is looking for a good job -) ## [core-js@3, babel and a look into the future](https://github.com/zloirock/core-js/tree/master/docs/2019-03-19-core-js-3-babel-and-a-look-into-the-future.md) ## Raising funds `core-js` isn't backed by a company, so the future of this project depends on you. Become a sponsor or a backer [**on Open Collective**](https://opencollective.com/core-js) or [**on Patreon**](https://www.patreon.com/zloirock) if you are interested in `core-js`. --- <a href="https://opencollective.com/core-js/sponsor/0/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/0/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/1/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/1/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/2/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/2/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/3/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/3/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/4/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/4/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/5/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/5/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/6/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/6/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/7/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/7/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/8/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/8/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/9/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/9/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/10/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/10/avatar.svg"></a><a href="https://opencollective.com/core-js/sponsor/11/website" target="_blank"><img src="https://opencollective.com/core-js/sponsor/11/avatar.svg"></a> --- <a href="https://opencollective.com/core-js#backers" target="_blank"><img src="https://opencollective.com/core-js/backers.svg?width=890"></a> --- [*Example*](http://goo.gl/a2xexl): ```js import 'core-js'; // <- at the top of your entry point Array.from(new Set([1, 2, 3, 2, 1])); // => [1, 2, 3] [1, [2, 3], [4, [5]]].flat(2); // => [1, 2, 3, 4, 5] Promise.resolve(32).then(x => console.log(x)); // => 32 ``` *You can load only required features*: ```js import 'core-js/features/array/from'; // <- at the top of your entry point import 'core-js/features/array/flat'; // <- at the top of your entry point import 'core-js/features/set'; // <- at the top of your entry point import 'core-js/features/promise'; // <- at the top of your entry point Array.from(new Set([1, 2, 3, 2, 1])); // => [1, 2, 3] [1, [2, 3], [4, [5]]].flat(2); // => [1, 2, 3, 4, 5] Promise.resolve(32).then(x => console.log(x)); // => 32 ``` *Or use it without global namespace pollution*: ```js import from from 'core-js-pure/features/array/from'; import flat from 'core-js-pure/features/array/flat'; import Set from 'core-js-pure/features/set'; import Promise from 'core-js-pure/features/promise'; from(new Set([1, 2, 3, 2, 1])); // => [1, 2, 3] flat([1, [2, 3], [4, [5]]], 2); // => [1, 2, 3, 4, 5] Promise.resolve(32).then(x => console.log(x)); // => 32 ``` **It's a global version (first 2 examples), for more info see [`core-js` documentation](https://github.com/zloirock/core-js/blob/master/README.md).** apollo-server-demo/node_modules/core-js/package.json 0000644 0001750 0000144 00000002440 03560116604 022260 0 ustar andreh users { "name": "core-js", "description": "Standard library", "version": "3.8.3", "repository": { "type": "git", "url": "https://github.com/zloirock/core-js.git" }, "main": "index.js", "funding": { "type": "opencollective", "url": "https://opencollective.com/core-js" }, "license": "MIT", "keywords": [ "ES3", "ES5", "ES6", "ES7", "ES2015", "ES2016", "ES2017", "ES2018", "ES2019", "ES2020", "ECMAScript 3", "ECMAScript 5", "ECMAScript 6", "ECMAScript 7", "ECMAScript 2015", "ECMAScript 2016", "ECMAScript 2017", "ECMAScript 2018", "ECMAScript 2019", "ECMAScript 2020", "Harmony", "Strawman", "Map", "Set", "WeakMap", "WeakSet", "Promise", "Observable", "Symbol", "TypedArray", "URL", "URLSearchParams", "queueMicrotask", "setImmediate", "polyfill", "ponyfill", "shim" ], "scripts": { "postinstall": "node -e \"try{require('./postinstall')}catch(e){}\"" }, "gitHead": "a88734f1d7d8c1b5bb797e1b8ece2ec1961111c6" ,"_resolved": "https://registry.npmjs.org/core-js/-/core-js-3.8.3.tgz" ,"_integrity": "sha512-KPYXeVZYemC2TkNEkX/01I+7yd+nX3KddKwZ1Ww7SKWdI2wQprSgLmrTddT8nw92AjEklTsPBoSdQBhbI1bQ6Q==" ,"_from": "core-js@3.8.3" } apollo-server-demo/node_modules/core-js/web/ 0000755 0001750 0000144 00000000000 14067647701 020562 5 ustar andreh users apollo-server-demo/node_modules/core-js/web/queue-microtask.js 0000644 0001750 0000144 00000000173 03560116604 024224 0 ustar andreh users require('../modules/web.queue-microtask'); var path = require('../internals/path'); module.exports = path.queueMicrotask; apollo-server-demo/node_modules/core-js/web/index.js 0000644 0001750 0000144 00000000616 03560116604 022217 0 ustar andreh users require('../modules/web.dom-collections.for-each'); require('../modules/web.dom-collections.iterator'); require('../modules/web.immediate'); require('../modules/web.queue-microtask'); require('../modules/web.timers'); require('../modules/web.url'); require('../modules/web.url.to-json'); require('../modules/web.url-search-params'); var path = require('../internals/path'); module.exports = path; apollo-server-demo/node_modules/core-js/web/dom-collections.js 0000644 0001750 0000144 00000000251 03560116604 024176 0 ustar andreh users require('../modules/web.dom-collections.for-each'); require('../modules/web.dom-collections.iterator'); var path = require('../internals/path'); module.exports = path; apollo-server-demo/node_modules/core-js/web/README.md 0000644 0001750 0000144 00000000221 03560116604 022021 0 ustar andreh users This folder contains entry points for features from [WHATWG / W3C](https://github.com/zloirock/core-js/tree/v3#web-standards) with dependencies. apollo-server-demo/node_modules/core-js/web/immediate.js 0000644 0001750 0000144 00000000146 03560116604 023044 0 ustar andreh users require('../modules/web.immediate'); var path = require('../internals/path'); module.exports = path; apollo-server-demo/node_modules/core-js/web/timers.js 0000644 0001750 0000144 00000000143 03560116604 022406 0 ustar andreh users require('../modules/web.timers'); var path = require('../internals/path'); module.exports = path; apollo-server-demo/node_modules/core-js/web/url.js 0000644 0001750 0000144 00000000270 03560116604 021706 0 ustar andreh users require('../modules/web.url'); require('../modules/web.url.to-json'); require('../modules/web.url-search-params'); var path = require('../internals/path'); module.exports = path.URL; apollo-server-demo/node_modules/core-js/web/url-search-params.js 0000644 0001750 0000144 00000000176 03560116604 024437 0 ustar andreh users require('../modules/web.url-search-params'); var path = require('../internals/path'); module.exports = path.URLSearchParams; apollo-server-demo/node_modules/core-js/features/ 0000755 0001750 0000144 00000000000 14067647701 021623 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/queue-microtask.js 0000644 0001750 0000144 00000000115 03560116604 025261 0 ustar andreh users var parent = require('../stable/queue-microtask'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/weak-map/ 0000755 0001750 0000144 00000000000 14067647701 023325 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/weak-map/index.js 0000644 0001750 0000144 00000000532 03560116604 024757 0 ustar andreh users var parent = require('../../es/weak-map'); require('../../modules/esnext.weak-map.emplace'); require('../../modules/esnext.weak-map.from'); require('../../modules/esnext.weak-map.of'); require('../../modules/esnext.weak-map.delete-all'); // TODO: remove from `core-js@4` require('../../modules/esnext.weak-map.upsert'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/weak-map/upsert.js 0000644 0001750 0000144 00000000306 03560116604 025171 0 ustar andreh users require('../../modules/es.weak-map'); require('../../modules/esnext.weak-map.upsert'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('WeakMap', 'upsert'); apollo-server-demo/node_modules/core-js/features/weak-map/delete-all.js 0000644 0001750 0000144 00000000315 03560116604 025657 0 ustar andreh users require('../../modules/es.weak-map'); require('../../modules/esnext.weak-map.delete-all'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('WeakMap', 'deleteAll'); apollo-server-demo/node_modules/core-js/features/weak-map/emplace.js 0000644 0001750 0000144 00000000310 03560116604 025250 0 ustar andreh users require('../../modules/es.weak-map'); require('../../modules/esnext.weak-map.emplace'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('WeakMap', 'emplace'); apollo-server-demo/node_modules/core-js/features/weak-map/from.js 0000644 0001750 0000144 00000000715 03560116604 024616 0 ustar andreh users 'use strict'; require('../../modules/es.string.iterator'); require('../../modules/es.weak-map'); require('../../modules/esnext.weak-map.from'); require('../../modules/web.dom-collections.iterator'); var path = require('../../internals/path'); var WeakMap = path.WeakMap; var weakMapFrom = WeakMap.from; module.exports = function from(source, mapFn, thisArg) { return weakMapFrom.call(typeof this === 'function' ? this : WeakMap, source, mapFn, thisArg); }; apollo-server-demo/node_modules/core-js/features/weak-map/of.js 0000644 0001750 0000144 00000000641 03560116604 024255 0 ustar andreh users 'use strict'; require('../../modules/es.string.iterator'); require('../../modules/es.weak-map'); require('../../modules/esnext.weak-map.of'); require('../../modules/web.dom-collections.iterator'); var path = require('../../internals/path'); var WeakMap = path.WeakMap; var weakMapOf = WeakMap.of; module.exports = function of() { return weakMapOf.apply(typeof this === 'function' ? this : WeakMap, arguments); }; apollo-server-demo/node_modules/core-js/features/index.js 0000644 0001750 0000144 00000000066 03560116604 023257 0 ustar andreh users var parent = require('..'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/composite-key.js 0000644 0001750 0000144 00000000172 03560116604 024736 0 ustar andreh users require('../modules/esnext.composite-key'); var path = require('../internals/path'); module.exports = path.compositeKey; apollo-server-demo/node_modules/core-js/features/composite-symbol.js 0000644 0001750 0000144 00000000241 03560116604 025450 0 ustar andreh users require('../modules/es.symbol'); require('../modules/esnext.composite-symbol'); var path = require('../internals/path'); module.exports = path.compositeSymbol; apollo-server-demo/node_modules/core-js/features/array/ 0000755 0001750 0000144 00000000000 14067647701 022741 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/array/sort.js 0000644 0001750 0000144 00000000107 03560116604 024251 0 ustar andreh users var parent = require('../../es/array/sort'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/reduce.js 0000644 0001750 0000144 00000000111 03560116604 024524 0 ustar andreh users var parent = require('../../es/array/reduce'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/some.js 0000644 0001750 0000144 00000000107 03560116604 024225 0 ustar andreh users var parent = require('../../es/array/some'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/index.js 0000644 0001750 0000144 00000000615 03560116604 024375 0 ustar andreh users var parent = require('../../es/array'); require('../../modules/es.map'); require('../../modules/esnext.array.at'); require('../../modules/esnext.array.filter-out'); require('../../modules/esnext.array.is-template-object'); require('../../modules/esnext.array.last-item'); require('../../modules/esnext.array.last-index'); require('../../modules/esnext.array.unique-by'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/iterator.js 0000644 0001750 0000144 00000000113 03560116604 025110 0 ustar andreh users var parent = require('../../es/array/iterator'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/copy-within.js 0000644 0001750 0000144 00000000116 03560116604 025534 0 ustar andreh users var parent = require('../../es/array/copy-within'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/map.js 0000644 0001750 0000144 00000000106 03560116604 024036 0 ustar andreh users var parent = require('../../es/array/map'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/is-template-object.js 0000644 0001750 0000144 00000000225 03560116604 026753 0 ustar andreh users require('../../modules/esnext.array.is-template-object'); var path = require('../../internals/path'); module.exports = path.Array.isTemplateObject; apollo-server-demo/node_modules/core-js/features/array/includes.js 0000644 0001750 0000144 00000000113 03560116604 025065 0 ustar andreh users var parent = require('../../es/array/includes'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/reduce-right.js 0000644 0001750 0000144 00000000117 03560116604 025645 0 ustar andreh users var parent = require('../../es/array/reduce-right'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/every.js 0000644 0001750 0000144 00000000110 03560116604 024406 0 ustar andreh users var parent = require('../../es/array/every'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/fill.js 0000644 0001750 0000144 00000000107 03560116604 024210 0 ustar andreh users var parent = require('../../es/array/fill'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/flat-map.js 0000644 0001750 0000144 00000000113 03560116604 024760 0 ustar andreh users var parent = require('../../es/array/flat-map'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/last-index.js 0000644 0001750 0000144 00000000062 03560116604 025332 0 ustar andreh users require('../../modules/esnext.array.last-index'); apollo-server-demo/node_modules/core-js/features/array/index-of.js 0000644 0001750 0000144 00000000113 03560116604 024770 0 ustar andreh users var parent = require('../../es/array/index-of'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/virtual/ 0000755 0001750 0000144 00000000000 14067647701 024427 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/array/virtual/sort.js 0000644 0001750 0000144 00000000122 03560116604 025734 0 ustar andreh users var parent = require('../../../es/array/virtual/sort'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/virtual/reduce.js 0000644 0001750 0000144 00000000124 03560116604 026216 0 ustar andreh users var parent = require('../../../es/array/virtual/reduce'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/virtual/some.js 0000644 0001750 0000144 00000000122 03560116604 025710 0 ustar andreh users var parent = require('../../../es/array/virtual/some'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/virtual/index.js 0000644 0001750 0000144 00000000343 03560116604 026061 0 ustar andreh users var parent = require('../../../es/array/virtual'); require('../../../modules/esnext.array.at'); require('../../../modules/esnext.array.filter-out'); require('../../../modules/esnext.array.unique-by'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/virtual/iterator.js 0000644 0001750 0000144 00000000126 03560116604 026602 0 ustar andreh users var parent = require('../../../es/array/virtual/iterator'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/virtual/copy-within.js 0000644 0001750 0000144 00000000131 03560116604 027217 0 ustar andreh users var parent = require('../../../es/array/virtual/copy-within'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/virtual/map.js 0000644 0001750 0000144 00000000121 03560116604 025521 0 ustar andreh users var parent = require('../../../es/array/virtual/map'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/virtual/includes.js 0000644 0001750 0000144 00000000126 03560116604 026557 0 ustar andreh users var parent = require('../../../es/array/virtual/includes'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/virtual/reduce-right.js 0000644 0001750 0000144 00000000132 03560116604 027330 0 ustar andreh users var parent = require('../../../es/array/virtual/reduce-right'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/virtual/every.js 0000644 0001750 0000144 00000000123 03560116604 026100 0 ustar andreh users var parent = require('../../../es/array/virtual/every'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/virtual/fill.js 0000644 0001750 0000144 00000000122 03560116604 025673 0 ustar andreh users var parent = require('../../../es/array/virtual/fill'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/virtual/flat-map.js 0000644 0001750 0000144 00000000126 03560116604 026452 0 ustar andreh users var parent = require('../../../es/array/virtual/flat-map'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/virtual/index-of.js 0000644 0001750 0000144 00000000126 03560116604 026462 0 ustar andreh users var parent = require('../../../es/array/virtual/index-of'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/virtual/splice.js 0000644 0001750 0000144 00000000124 03560116604 026226 0 ustar andreh users var parent = require('../../../es/array/virtual/splice'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/virtual/last-index-of.js 0000644 0001750 0000144 00000000133 03560116604 027421 0 ustar andreh users var parent = require('../../../es/array/virtual/last-index-of'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/virtual/flat.js 0000644 0001750 0000144 00000000122 03560116604 025673 0 ustar andreh users var parent = require('../../../es/array/virtual/flat'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/virtual/values.js 0000644 0001750 0000144 00000000124 03560116604 026246 0 ustar andreh users var parent = require('../../../es/array/virtual/values'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/virtual/join.js 0000644 0001750 0000144 00000000122 03560116604 025704 0 ustar andreh users var parent = require('../../../es/array/virtual/join'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/virtual/entries.js 0000644 0001750 0000144 00000000125 03560116604 026421 0 ustar andreh users var parent = require('../../../es/array/virtual/entries'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/virtual/filter-out.js 0000644 0001750 0000144 00000000250 03560116604 027041 0 ustar andreh users require('../../../modules/esnext.array.filter-out'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').filterOut; apollo-server-demo/node_modules/core-js/features/array/virtual/filter.js 0000644 0001750 0000144 00000000124 03560116604 026234 0 ustar andreh users var parent = require('../../../es/array/virtual/filter'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/virtual/concat.js 0000644 0001750 0000144 00000000124 03560116604 026216 0 ustar andreh users var parent = require('../../../es/array/virtual/concat'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/virtual/at.js 0000644 0001750 0000144 00000000231 03560116604 025352 0 ustar andreh users require('../../../modules/esnext.array.at'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').at; apollo-server-demo/node_modules/core-js/features/array/virtual/unique-by.js 0000644 0001750 0000144 00000000312 03560116604 026664 0 ustar andreh users require('../../../modules/es.map'); require('../../../modules/esnext.array.unique-by'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').uniqueBy; apollo-server-demo/node_modules/core-js/features/array/virtual/for-each.js 0000644 0001750 0000144 00000000126 03560116604 026435 0 ustar andreh users var parent = require('../../../es/array/virtual/for-each'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/virtual/find-index.js 0000644 0001750 0000144 00000000130 03560116604 026771 0 ustar andreh users var parent = require('../../../es/array/virtual/find-index'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/virtual/keys.js 0000644 0001750 0000144 00000000122 03560116604 025720 0 ustar andreh users var parent = require('../../../es/array/virtual/keys'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/virtual/find.js 0000644 0001750 0000144 00000000122 03560116604 025665 0 ustar andreh users var parent = require('../../../es/array/virtual/find'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/virtual/reverse.js 0000644 0001750 0000144 00000000125 03560116604 026423 0 ustar andreh users var parent = require('../../../es/array/virtual/reverse'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/virtual/slice.js 0000644 0001750 0000144 00000000123 03560116604 026045 0 ustar andreh users var parent = require('../../../es/array/virtual/slice'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/splice.js 0000644 0001750 0000144 00000000111 03560116604 024534 0 ustar andreh users var parent = require('../../es/array/splice'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/last-index-of.js 0000644 0001750 0000144 00000000120 03560116604 025727 0 ustar andreh users var parent = require('../../es/array/last-index-of'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/flat.js 0000644 0001750 0000144 00000000107 03560116604 024210 0 ustar andreh users var parent = require('../../es/array/flat'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/values.js 0000644 0001750 0000144 00000000111 03560116604 024554 0 ustar andreh users var parent = require('../../es/array/values'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/join.js 0000644 0001750 0000144 00000000107 03560116604 024221 0 ustar andreh users var parent = require('../../es/array/join'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/from.js 0000644 0001750 0000144 00000000107 03560116604 024225 0 ustar andreh users var parent = require('../../es/array/from'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/last-item.js 0000644 0001750 0000144 00000000061 03560116604 025160 0 ustar andreh users require('../../modules/esnext.array.last-item'); apollo-server-demo/node_modules/core-js/features/array/entries.js 0000644 0001750 0000144 00000000112 03560116604 024727 0 ustar andreh users var parent = require('../../es/array/entries'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/filter-out.js 0000644 0001750 0000144 00000000242 03560116604 025354 0 ustar andreh users require('../../modules/esnext.array.filter-out'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'filterOut'); apollo-server-demo/node_modules/core-js/features/array/filter.js 0000644 0001750 0000144 00000000111 03560116604 024542 0 ustar andreh users var parent = require('../../es/array/filter'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/concat.js 0000644 0001750 0000144 00000000111 03560116604 024524 0 ustar andreh users var parent = require('../../es/array/concat'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/at.js 0000644 0001750 0000144 00000000223 03560116604 023665 0 ustar andreh users require('../../modules/esnext.array.at'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'at'); apollo-server-demo/node_modules/core-js/features/array/unique-by.js 0000644 0001750 0000144 00000000301 03560116604 025174 0 ustar andreh users require('../../modules/es.map'); require('../../modules/esnext.array.unique-by'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'uniqueBy'); apollo-server-demo/node_modules/core-js/features/array/for-each.js 0000644 0001750 0000144 00000000113 03560116604 024743 0 ustar andreh users var parent = require('../../es/array/for-each'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/is-array.js 0000644 0001750 0000144 00000000113 03560116604 025006 0 ustar andreh users var parent = require('../../es/array/is-array'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/find-index.js 0000644 0001750 0000144 00000000115 03560116604 025306 0 ustar andreh users var parent = require('../../es/array/find-index'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/keys.js 0000644 0001750 0000144 00000000107 03560116604 024235 0 ustar andreh users var parent = require('../../es/array/keys'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/find.js 0000644 0001750 0000144 00000000107 03560116604 024202 0 ustar andreh users var parent = require('../../es/array/find'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/of.js 0000644 0001750 0000144 00000000105 03560116604 023664 0 ustar andreh users var parent = require('../../es/array/of'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/reverse.js 0000644 0001750 0000144 00000000112 03560116604 024731 0 ustar andreh users var parent = require('../../es/array/reverse'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array/slice.js 0000644 0001750 0000144 00000000110 03560116604 024353 0 ustar andreh users var parent = require('../../es/array/slice'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array-buffer/ 0000755 0001750 0000144 00000000000 14067647701 024210 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/array-buffer/index.js 0000644 0001750 0000144 00000000111 03560116604 025633 0 ustar andreh users var parent = require('../../es/array-buffer'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array-buffer/is-view.js 0000644 0001750 0000144 00000000121 03560116604 026110 0 ustar andreh users var parent = require('../../es/array-buffer/is-view'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array-buffer/constructor.js 0000644 0001750 0000144 00000000125 03560116604 027116 0 ustar andreh users var parent = require('../../es/array-buffer/constructor'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/array-buffer/slice.js 0000644 0001750 0000144 00000000117 03560116604 025631 0 ustar andreh users var parent = require('../../es/array-buffer/slice'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/README.md 0000644 0001750 0000144 00000000213 03560116604 023063 0 ustar andreh users This folder contains entry points for all `core-js` features with dependencies. It's the recommended way for usage only required features. apollo-server-demo/node_modules/core-js/features/is-iterable.js 0000644 0001750 0000144 00000000262 03560116604 024346 0 ustar andreh users require('../modules/web.dom-collections.iterator'); require('../modules/es.string.iterator'); var isIterable = require('../internals/is-iterable'); module.exports = isIterable; apollo-server-demo/node_modules/core-js/features/symbol/ 0000755 0001750 0000144 00000000000 14067647701 023130 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/symbol/index.js 0000644 0001750 0000144 00000000547 03560116604 024570 0 ustar andreh users var parent = require('../../es/symbol'); require('../../modules/esnext.symbol.async-dispose'); require('../../modules/esnext.symbol.dispose'); require('../../modules/esnext.symbol.observable'); require('../../modules/esnext.symbol.pattern-match'); // TODO: Remove from `core-js@4` require('../../modules/esnext.symbol.replace-all'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/symbol/iterator.js 0000644 0001750 0000144 00000000114 03560116604 025300 0 ustar andreh users var parent = require('../../es/symbol/iterator'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/symbol/dispose.js 0000644 0001750 0000144 00000000306 03560116604 025120 0 ustar andreh users require('../../modules/esnext.symbol.dispose'); var WrappedWellKnownSymbolModule = require('../../internals/well-known-symbol-wrapped'); module.exports = WrappedWellKnownSymbolModule.f('dispose'); apollo-server-demo/node_modules/core-js/features/symbol/pattern-match.js 0000644 0001750 0000144 00000000321 03560116604 026216 0 ustar andreh users require('../../modules/esnext.symbol.pattern-match'); var WrappedWellKnownSymbolModule = require('../../internals/well-known-symbol-wrapped'); module.exports = WrappedWellKnownSymbolModule.f('patternMatch'); apollo-server-demo/node_modules/core-js/features/symbol/to-string-tag.js 0000644 0001750 0000144 00000000121 03560116604 026144 0 ustar andreh users var parent = require('../../es/symbol/to-string-tag'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/symbol/match.js 0000644 0001750 0000144 00000000111 03560116604 024540 0 ustar andreh users var parent = require('../../es/symbol/match'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/symbol/unscopables.js 0000644 0001750 0000144 00000000117 03560116604 025770 0 ustar andreh users var parent = require('../../es/symbol/unscopables'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/symbol/is-concat-spreadable.js 0000644 0001750 0000144 00000000130 03560116604 027425 0 ustar andreh users var parent = require('../../es/symbol/is-concat-spreadable'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/symbol/for.js 0000644 0001750 0000144 00000000107 03560116604 024237 0 ustar andreh users var parent = require('../../es/symbol/for'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/symbol/key-for.js 0000644 0001750 0000144 00000000113 03560116604 025022 0 ustar andreh users var parent = require('../../es/symbol/key-for'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/symbol/to-primitive.js 0000644 0001750 0000144 00000000120 03560116604 026074 0 ustar andreh users var parent = require('../../es/symbol/to-primitive'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/symbol/async-iterator.js 0000644 0001750 0000144 00000000122 03560116604 026412 0 ustar andreh users var parent = require('../../es/symbol/async-iterator'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/symbol/split.js 0000644 0001750 0000144 00000000111 03560116604 024577 0 ustar andreh users var parent = require('../../es/symbol/split'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/symbol/observable.js 0000644 0001750 0000144 00000000314 03560116604 025575 0 ustar andreh users require('../../modules/esnext.symbol.observable'); var WrappedWellKnownSymbolModule = require('../../internals/well-known-symbol-wrapped'); module.exports = WrappedWellKnownSymbolModule.f('observable'); apollo-server-demo/node_modules/core-js/features/symbol/has-instance.js 0000644 0001750 0000144 00000000120 03560116604 026021 0 ustar andreh users var parent = require('../../es/symbol/has-instance'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/symbol/species.js 0000644 0001750 0000144 00000000113 03560116604 025101 0 ustar andreh users var parent = require('../../es/symbol/species'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/symbol/search.js 0000644 0001750 0000144 00000000112 03560116604 024712 0 ustar andreh users var parent = require('../../es/symbol/search'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/symbol/replace.js 0000644 0001750 0000144 00000000113 03560116604 025061 0 ustar andreh users var parent = require('../../es/symbol/replace'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/symbol/description.js 0000644 0001750 0000144 00000000060 03560116604 025772 0 ustar andreh users require('../../modules/es.symbol.description'); apollo-server-demo/node_modules/core-js/features/symbol/replace-all.js 0000644 0001750 0000144 00000000356 03560116604 025640 0 ustar andreh users // TODO: Remove from `core-js@4` require('../../modules/esnext.symbol.replace-all'); var WrappedWellKnownSymbolModule = require('../../internals/well-known-symbol-wrapped'); module.exports = WrappedWellKnownSymbolModule.f('replaceAll'); apollo-server-demo/node_modules/core-js/features/symbol/match-all.js 0000644 0001750 0000144 00000000115 03560116604 025312 0 ustar andreh users var parent = require('../../es/symbol/match-all'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/symbol/async-dispose.js 0000644 0001750 0000144 00000000321 03560116604 026230 0 ustar andreh users require('../../modules/esnext.symbol.async-dispose'); var WrappedWellKnownSymbolModule = require('../../internals/well-known-symbol-wrapped'); module.exports = WrappedWellKnownSymbolModule.f('asyncDispose'); apollo-server-demo/node_modules/core-js/features/dom-collections/ 0000755 0001750 0000144 00000000000 14067647701 024716 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/dom-collections/index.js 0000644 0001750 0000144 00000000120 03560116604 026341 0 ustar andreh users var parent = require('../../stable/dom-collections'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/dom-collections/iterator.js 0000644 0001750 0000144 00000000131 03560116604 027065 0 ustar andreh users var parent = require('../../stable/dom-collections/iterator'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/dom-collections/for-each.js 0000644 0001750 0000144 00000000131 03560116604 026720 0 ustar andreh users var parent = require('../../stable/dom-collections/for-each'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/ 0000755 0001750 0000144 00000000000 14067647701 023427 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/instance/code-points.js 0000644 0001750 0000144 00000000466 03560116604 026204 0 ustar andreh users var codePoints = require('../string/virtual/code-points'); var StringPrototype = String.prototype; module.exports = function (it) { var own = it.codePoints; return typeof it === 'string' || it === StringPrototype || (it instanceof String && own === StringPrototype.codePoints) ? codePoints : own; }; apollo-server-demo/node_modules/core-js/features/instance/sort.js 0000644 0001750 0000144 00000000112 03560116604 024733 0 ustar andreh users var parent = require('../../es/instance/sort'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/reduce.js 0000644 0001750 0000144 00000000114 03560116604 025215 0 ustar andreh users var parent = require('../../es/instance/reduce'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/some.js 0000644 0001750 0000144 00000000112 03560116604 024707 0 ustar andreh users var parent = require('../../es/instance/some'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/copy-within.js 0000644 0001750 0000144 00000000121 03560116604 026216 0 ustar andreh users var parent = require('../../es/instance/copy-within'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/repeat.js 0000644 0001750 0000144 00000000114 03560116604 025226 0 ustar andreh users var parent = require('../../es/instance/repeat'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/map.js 0000644 0001750 0000144 00000000111 03560116604 024520 0 ustar andreh users var parent = require('../../es/instance/map'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/includes.js 0000644 0001750 0000144 00000000116 03560116604 025556 0 ustar andreh users var parent = require('../../es/instance/includes'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/reduce-right.js 0000644 0001750 0000144 00000000122 03560116604 026327 0 ustar andreh users var parent = require('../../es/instance/reduce-right'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/code-point-at.js 0000644 0001750 0000144 00000000123 03560116604 026411 0 ustar andreh users var parent = require('../../es/instance/code-point-at'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/every.js 0000644 0001750 0000144 00000000113 03560116604 025077 0 ustar andreh users var parent = require('../../es/instance/every'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/fill.js 0000644 0001750 0000144 00000000112 03560116604 024672 0 ustar andreh users var parent = require('../../es/instance/fill'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/flat-map.js 0000644 0001750 0000144 00000000116 03560116604 025451 0 ustar andreh users var parent = require('../../es/instance/flat-map'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/index-of.js 0000644 0001750 0000144 00000000116 03560116604 025461 0 ustar andreh users var parent = require('../../es/instance/index-of'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/trim-left.js 0000644 0001750 0000144 00000000117 03560116604 025654 0 ustar andreh users var parent = require('../../es/instance/trim-left'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/pad-end.js 0000644 0001750 0000144 00000000115 03560116604 025257 0 ustar andreh users var parent = require('../../es/instance/pad-end'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/bind.js 0000644 0001750 0000144 00000000112 03560116604 024660 0 ustar andreh users var parent = require('../../es/instance/bind'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/splice.js 0000644 0001750 0000144 00000000114 03560116604 025225 0 ustar andreh users var parent = require('../../es/instance/splice'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/last-index-of.js 0000644 0001750 0000144 00000000123 03560116604 026420 0 ustar andreh users var parent = require('../../es/instance/last-index-of'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/flags.js 0000644 0001750 0000144 00000000113 03560116604 025041 0 ustar andreh users var parent = require('../../es/instance/flags'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/flat.js 0000644 0001750 0000144 00000000112 03560116604 024672 0 ustar andreh users var parent = require('../../es/instance/flat'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/values.js 0000644 0001750 0000144 00000000120 03560116604 025242 0 ustar andreh users var parent = require('../../stable/instance/values'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/starts-with.js 0000644 0001750 0000144 00000000121 03560116604 026235 0 ustar andreh users var parent = require('../../es/instance/starts-with'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/entries.js 0000644 0001750 0000144 00000000121 03560116604 025415 0 ustar andreh users var parent = require('../../stable/instance/entries'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/filter-out.js 0000644 0001750 0000144 00000000415 03560116604 026044 0 ustar andreh users var filterOut = require('../array/virtual/filter-out'); var ArrayPrototype = Array.prototype; module.exports = function (it) { var own = it.filterOut; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.filterOut) ? filterOut : own; }; apollo-server-demo/node_modules/core-js/features/instance/trim-end.js 0000644 0001750 0000144 00000000116 03560116604 025467 0 ustar andreh users var parent = require('../../es/instance/trim-end'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/filter.js 0000644 0001750 0000144 00000000114 03560116604 025233 0 ustar andreh users var parent = require('../../es/instance/filter'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/trim-start.js 0000644 0001750 0000144 00000000120 03560116604 026051 0 ustar andreh users var parent = require('../../es/instance/trim-start'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/concat.js 0000644 0001750 0000144 00000000114 03560116604 025215 0 ustar andreh users var parent = require('../../es/instance/concat'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/at.js 0000644 0001750 0000144 00000000737 03560116604 024365 0 ustar andreh users var arrayAt = require('../array/virtual/at'); var stringAt = require('../string/virtual/at'); var ArrayPrototype = Array.prototype; var StringPrototype = String.prototype; module.exports = function (it) { var own = it.at; if (it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.at)) return arrayAt; if (typeof it === 'string' || it === StringPrototype || (it instanceof String && own === StringPrototype.at)) { return stringAt; } return own; }; apollo-server-demo/node_modules/core-js/features/instance/unique-by.js 0000644 0001750 0000144 00000000410 03560116604 025663 0 ustar andreh users var uniqueBy = require('../array/virtual/unique-by'); var ArrayPrototype = Array.prototype; module.exports = function (it) { var own = it.uniqueBy; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.uniqueBy) ? uniqueBy : own; }; apollo-server-demo/node_modules/core-js/features/instance/trim.js 0000644 0001750 0000144 00000000112 03560116604 024717 0 ustar andreh users var parent = require('../../es/instance/trim'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/for-each.js 0000644 0001750 0000144 00000000122 03560116604 025431 0 ustar andreh users var parent = require('../../stable/instance/for-each'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/find-index.js 0000644 0001750 0000144 00000000120 03560116604 025770 0 ustar andreh users var parent = require('../../es/instance/find-index'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/pad-start.js 0000644 0001750 0000144 00000000117 03560116604 025650 0 ustar andreh users var parent = require('../../es/instance/pad-start'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/keys.js 0000644 0001750 0000144 00000000116 03560116604 024723 0 ustar andreh users var parent = require('../../stable/instance/keys'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/find.js 0000644 0001750 0000144 00000000112 03560116604 024664 0 ustar andreh users var parent = require('../../es/instance/find'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/replace-all.js 0000644 0001750 0000144 00000000125 03560116604 026131 0 ustar andreh users var parent = require('../../stable/instance/replace-all'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/match-all.js 0000644 0001750 0000144 00000000243 03560116604 025613 0 ustar andreh users // TODO: remove from `core-js@4` require('../../modules/esnext.string.match-all'); var parent = require('../../es/instance/match-all'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/reverse.js 0000644 0001750 0000144 00000000115 03560116604 025422 0 ustar andreh users var parent = require('../../es/instance/reverse'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/trim-right.js 0000644 0001750 0000144 00000000120 03560116604 026031 0 ustar andreh users var parent = require('../../es/instance/trim-right'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/ends-with.js 0000644 0001750 0000144 00000000117 03560116604 025653 0 ustar andreh users var parent = require('../../es/instance/ends-with'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/instance/slice.js 0000644 0001750 0000144 00000000113 03560116604 025044 0 ustar andreh users var parent = require('../../es/instance/slice'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/iterator/ 0000755 0001750 0000144 00000000000 14067647701 023454 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/iterator/take.js 0000644 0001750 0000144 00000000546 03560116604 024730 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.string.iterator'); require('../../modules/esnext.iterator.constructor'); require('../../modules/esnext.iterator.take'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Iterator', 'take'); apollo-server-demo/node_modules/core-js/features/iterator/reduce.js 0000644 0001750 0000144 00000000552 03560116604 025250 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.string.iterator'); require('../../modules/esnext.iterator.constructor'); require('../../modules/esnext.iterator.reduce'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Iterator', 'reduce'); apollo-server-demo/node_modules/core-js/features/iterator/to-array.js 0000644 0001750 0000144 00000000555 03560116604 025542 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.string.iterator'); require('../../modules/esnext.iterator.constructor'); require('../../modules/esnext.iterator.to-array'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Iterator', 'toArray'); apollo-server-demo/node_modules/core-js/features/iterator/some.js 0000644 0001750 0000144 00000000546 03560116604 024747 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.string.iterator'); require('../../modules/esnext.iterator.constructor'); require('../../modules/esnext.iterator.some'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Iterator', 'some'); apollo-server-demo/node_modules/core-js/features/iterator/index.js 0000644 0001750 0000144 00000001625 03560116604 025112 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.string.iterator'); require('../../modules/esnext.iterator.constructor'); require('../../modules/esnext.iterator.as-indexed-pairs'); require('../../modules/esnext.iterator.drop'); require('../../modules/esnext.iterator.every'); require('../../modules/esnext.iterator.filter'); require('../../modules/esnext.iterator.find'); require('../../modules/esnext.iterator.flat-map'); require('../../modules/esnext.iterator.for-each'); require('../../modules/esnext.iterator.from'); require('../../modules/esnext.iterator.map'); require('../../modules/esnext.iterator.reduce'); require('../../modules/esnext.iterator.some'); require('../../modules/esnext.iterator.take'); require('../../modules/esnext.iterator.to-array'); require('../../modules/web.dom-collections.iterator'); var path = require('../../internals/path'); module.exports = path.Iterator; apollo-server-demo/node_modules/core-js/features/iterator/map.js 0000644 0001750 0000144 00000000544 03560116604 024557 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.string.iterator'); require('../../modules/esnext.iterator.constructor'); require('../../modules/esnext.iterator.map'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Iterator', 'map'); apollo-server-demo/node_modules/core-js/features/iterator/every.js 0000644 0001750 0000144 00000000550 03560116604 025131 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.string.iterator'); require('../../modules/esnext.iterator.constructor'); require('../../modules/esnext.iterator.every'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Iterator', 'every'); apollo-server-demo/node_modules/core-js/features/iterator/flat-map.js 0000644 0001750 0000144 00000000555 03560116604 025505 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.string.iterator'); require('../../modules/esnext.iterator.constructor'); require('../../modules/esnext.iterator.flat-map'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Iterator', 'flatMap'); apollo-server-demo/node_modules/core-js/features/iterator/drop.js 0000644 0001750 0000144 00000000546 03560116604 024750 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.string.iterator'); require('../../modules/esnext.iterator.constructor'); require('../../modules/esnext.iterator.drop'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Iterator', 'drop'); apollo-server-demo/node_modules/core-js/features/iterator/from.js 0000644 0001750 0000144 00000000512 03560116604 024740 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.string.iterator'); require('../../modules/esnext.iterator.constructor'); require('../../modules/esnext.iterator.from'); require('../../modules/web.dom-collections.iterator'); var path = require('../../internals/path'); module.exports = path.Iterator.from; apollo-server-demo/node_modules/core-js/features/iterator/filter.js 0000644 0001750 0000144 00000000552 03560116604 025266 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.string.iterator'); require('../../modules/esnext.iterator.constructor'); require('../../modules/esnext.iterator.filter'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Iterator', 'filter'); apollo-server-demo/node_modules/core-js/features/iterator/for-each.js 0000644 0001750 0000144 00000000555 03560116604 025470 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.string.iterator'); require('../../modules/esnext.iterator.constructor'); require('../../modules/esnext.iterator.for-each'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Iterator', 'forEach'); apollo-server-demo/node_modules/core-js/features/iterator/find.js 0000644 0001750 0000144 00000000546 03560116604 024724 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.string.iterator'); require('../../modules/esnext.iterator.constructor'); require('../../modules/esnext.iterator.find'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Iterator', 'find'); apollo-server-demo/node_modules/core-js/features/iterator/as-indexed-pairs.js 0000644 0001750 0000144 00000000575 03560116604 027143 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.string.iterator'); require('../../modules/esnext.iterator.constructor'); require('../../modules/esnext.iterator.as-indexed-pairs'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Iterator', 'asIndexedPairs'); apollo-server-demo/node_modules/core-js/features/map/ 0000755 0001750 0000144 00000000000 14067647701 022400 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/map/map-keys.js 0000644 0001750 0000144 00000000273 03560116604 024453 0 ustar andreh users require('../../modules/es.map'); require('../../modules/esnext.map.map-keys'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Map', 'mapKeys'); apollo-server-demo/node_modules/core-js/features/map/reduce.js 0000644 0001750 0000144 00000000270 03560116604 024171 0 ustar andreh users require('../../modules/es.map'); require('../../modules/esnext.map.reduce'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Map', 'reduce'); apollo-server-demo/node_modules/core-js/features/map/some.js 0000644 0001750 0000144 00000000264 03560116604 023670 0 ustar andreh users require('../../modules/es.map'); require('../../modules/esnext.map.some'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Map', 'some'); apollo-server-demo/node_modules/core-js/features/map/index.js 0000644 0001750 0000144 00000002001 03560116604 024023 0 ustar andreh users var parent = require('../../es/map'); require('../../modules/esnext.map.from'); require('../../modules/esnext.map.of'); require('../../modules/esnext.map.delete-all'); require('../../modules/esnext.map.emplace'); require('../../modules/esnext.map.every'); require('../../modules/esnext.map.filter'); require('../../modules/esnext.map.find'); require('../../modules/esnext.map.find-key'); require('../../modules/esnext.map.group-by'); require('../../modules/esnext.map.includes'); require('../../modules/esnext.map.key-by'); require('../../modules/esnext.map.key-of'); require('../../modules/esnext.map.map-keys'); require('../../modules/esnext.map.map-values'); require('../../modules/esnext.map.merge'); require('../../modules/esnext.map.reduce'); require('../../modules/esnext.map.some'); require('../../modules/esnext.map.update'); // TODO: remove from `core-js@4` require('../../modules/esnext.map.upsert'); // TODO: remove from `core-js@4` require('../../modules/esnext.map.update-or-insert'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/map/includes.js 0000644 0001750 0000144 00000000274 03560116604 024534 0 ustar andreh users require('../../modules/es.map'); require('../../modules/esnext.map.includes'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Map', 'includes'); apollo-server-demo/node_modules/core-js/features/map/upsert.js 0000644 0001750 0000144 00000000270 03560116604 024244 0 ustar andreh users require('../../modules/es.map'); require('../../modules/esnext.map.upsert'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Map', 'upsert'); apollo-server-demo/node_modules/core-js/features/map/delete-all.js 0000644 0001750 0000144 00000000277 03560116604 024741 0 ustar andreh users require('../../modules/es.map'); require('../../modules/esnext.map.delete-all'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Map', 'deleteAll'); apollo-server-demo/node_modules/core-js/features/map/update-or-insert.js 0000644 0001750 0000144 00000000353 03560116604 026126 0 ustar andreh users // TODO: remove from `core-js@4` require('../../modules/es.map'); require('../../modules/esnext.map.update-or-insert'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Map', 'updateOrInsert'); apollo-server-demo/node_modules/core-js/features/map/every.js 0000644 0001750 0000144 00000000266 03560116604 024061 0 ustar andreh users require('../../modules/es.map'); require('../../modules/esnext.map.every'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Map', 'every'); apollo-server-demo/node_modules/core-js/features/map/key-of.js 0000644 0001750 0000144 00000000267 03560116604 024122 0 ustar andreh users require('../../modules/es.map'); require('../../modules/esnext.map.key-of'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Map', 'keyOf'); apollo-server-demo/node_modules/core-js/features/map/merge.js 0000644 0001750 0000144 00000000266 03560116604 024026 0 ustar andreh users require('../../modules/es.map'); require('../../modules/esnext.map.merge'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Map', 'merge'); apollo-server-demo/node_modules/core-js/features/map/update.js 0000644 0001750 0000144 00000000270 03560116604 024204 0 ustar andreh users require('../../modules/es.map'); require('../../modules/esnext.map.update'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Map', 'update'); apollo-server-demo/node_modules/core-js/features/map/group-by.js 0000644 0001750 0000144 00000000551 03560116604 024470 0 ustar andreh users 'use strict'; require('../../modules/es.map'); require('../../modules/esnext.map.group-by'); var path = require('../../internals/path'); var Map = path.Map; var mapGroupBy = Map.groupBy; module.exports = function groupBy(source, iterable, keyDerivative) { return mapGroupBy.call(typeof this === 'function' ? this : Map, source, iterable, keyDerivative); }; apollo-server-demo/node_modules/core-js/features/map/emplace.js 0000644 0001750 0000144 00000000272 03560116604 024332 0 ustar andreh users require('../../modules/es.map'); require('../../modules/esnext.map.emplace'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Map', 'emplace'); apollo-server-demo/node_modules/core-js/features/map/from.js 0000644 0001750 0000144 00000000653 03560116604 023672 0 ustar andreh users 'use strict'; require('../../modules/es.map'); require('../../modules/es.string.iterator'); require('../../modules/esnext.map.from'); require('../../modules/web.dom-collections.iterator'); var path = require('../../internals/path'); var Map = path.Map; var mapFrom = Map.from; module.exports = function from(source, mapFn, thisArg) { return mapFrom.call(typeof this === 'function' ? this : Map, source, mapFn, thisArg); }; apollo-server-demo/node_modules/core-js/features/map/find-key.js 0000644 0001750 0000144 00000000273 03560116604 024433 0 ustar andreh users require('../../modules/es.map'); require('../../modules/esnext.map.find-key'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Map', 'findKey'); apollo-server-demo/node_modules/core-js/features/map/filter.js 0000644 0001750 0000144 00000000270 03560116604 024207 0 ustar andreh users require('../../modules/es.map'); require('../../modules/esnext.map.filter'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Map', 'filter'); apollo-server-demo/node_modules/core-js/features/map/map-values.js 0000644 0001750 0000144 00000000277 03560116604 025003 0 ustar andreh users require('../../modules/es.map'); require('../../modules/esnext.map.map-values'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Map', 'mapValues'); apollo-server-demo/node_modules/core-js/features/map/find.js 0000644 0001750 0000144 00000000264 03560116604 023645 0 ustar andreh users require('../../modules/es.map'); require('../../modules/esnext.map.find'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Map', 'find'); apollo-server-demo/node_modules/core-js/features/map/of.js 0000644 0001750 0000144 00000000577 03560116604 023340 0 ustar andreh users 'use strict'; require('../../modules/es.map'); require('../../modules/es.string.iterator'); require('../../modules/esnext.map.of'); require('../../modules/web.dom-collections.iterator'); var path = require('../../internals/path'); var Map = path.Map; var mapOf = Map.of; module.exports = function of() { return mapOf.apply(typeof this === 'function' ? this : Map, arguments); }; apollo-server-demo/node_modules/core-js/features/map/key-by.js 0000644 0001750 0000144 00000000537 03560116604 024130 0 ustar andreh users 'use strict'; require('../../modules/es.map'); require('../../modules/esnext.map.key-by'); var path = require('../../internals/path'); var Map = path.Map; var mapKeyBy = Map.keyBy; module.exports = function keyBy(source, iterable, keyDerivative) { return mapKeyBy.call(typeof this === 'function' ? this : Map, source, iterable, keyDerivative); }; apollo-server-demo/node_modules/core-js/features/string/ 0000755 0001750 0000144 00000000000 14067647701 023131 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/string/code-points.js 0000644 0001750 0000144 00000000207 03560116604 025677 0 ustar andreh users require('../../modules/esnext.string.code-points'); module.exports = require('../../internals/entry-unbind')('String', 'codePoints'); apollo-server-demo/node_modules/core-js/features/string/index.js 0000644 0001750 0000144 00000000656 03560116604 024572 0 ustar andreh users var parent = require('../../es/string'); require('../../modules/esnext.string.at'); // TODO: disabled by default because of the conflict with another proposal // require('../../modules/esnext.string.at-alternative'); require('../../modules/esnext.string.code-points'); // TODO: remove from `core-js@4` require('../../modules/esnext.string.match-all'); require('../../modules/esnext.string.replace-all'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/iterator.js 0000644 0001750 0000144 00000000114 03560116604 025301 0 ustar andreh users var parent = require('../../es/string/iterator'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/repeat.js 0000644 0001750 0000144 00000000112 03560116604 024726 0 ustar andreh users var parent = require('../../es/string/repeat'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/includes.js 0000644 0001750 0000144 00000000114 03560116604 025256 0 ustar andreh users var parent = require('../../es/string/includes'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/italics.js 0000644 0001750 0000144 00000000113 03560116604 025077 0 ustar andreh users var parent = require('../../es/string/italics'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/sub.js 0000644 0001750 0000144 00000000107 03560116604 024243 0 ustar andreh users var parent = require('../../es/string/sub'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/fixed.js 0000644 0001750 0000144 00000000111 03560116604 024544 0 ustar andreh users var parent = require('../../es/string/fixed'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/code-point-at.js 0000644 0001750 0000144 00000000121 03560116604 026111 0 ustar andreh users var parent = require('../../es/string/code-point-at'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/match.js 0000644 0001750 0000144 00000000111 03560116604 024541 0 ustar andreh users var parent = require('../../es/string/match'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/trim-left.js 0000644 0001750 0000144 00000000115 03560116604 025354 0 ustar andreh users var parent = require('../../es/string/trim-left'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/pad-end.js 0000644 0001750 0000144 00000000113 03560116604 024757 0 ustar andreh users var parent = require('../../es/string/pad-end'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/ 0000755 0001750 0000144 00000000000 14067647701 024617 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/string/virtual/code-points.js 0000644 0001750 0000144 00000000213 03560116604 027362 0 ustar andreh users require('../../../modules/esnext.string.code-points'); module.exports = require('../../../internals/entry-virtual')('String').codePoints; apollo-server-demo/node_modules/core-js/features/string/virtual/index.js 0000644 0001750 0000144 00000000710 03560116604 026247 0 ustar andreh users var parent = require('../../../es/string/virtual'); require('../../../modules/esnext.string.at'); // TODO: disabled by default because of the conflict with another proposal // require('../../../modules/esnext.string.at-alternative'); require('../../../modules/esnext.string.code-points'); // TODO: remove from `core-js@4` require('../../../modules/esnext.string.match-all'); require('../../../modules/esnext.string.replace-all'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/iterator.js 0000644 0001750 0000144 00000000127 03560116604 026773 0 ustar andreh users var parent = require('../../../es/string/virtual/iterator'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/repeat.js 0000644 0001750 0000144 00000000125 03560116604 026420 0 ustar andreh users var parent = require('../../../es/string/virtual/repeat'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/includes.js 0000644 0001750 0000144 00000000127 03560116604 026750 0 ustar andreh users var parent = require('../../../es/string/virtual/includes'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/italics.js 0000644 0001750 0000144 00000000126 03560116604 026571 0 ustar andreh users var parent = require('../../../es/string/virtual/italics'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/sub.js 0000644 0001750 0000144 00000000122 03560116604 025726 0 ustar andreh users var parent = require('../../../es/string/virtual/sub'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/fixed.js 0000644 0001750 0000144 00000000124 03560116604 026236 0 ustar andreh users var parent = require('../../../es/string/virtual/fixed'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/code-point-at.js 0000644 0001750 0000144 00000000134 03560116604 027603 0 ustar andreh users var parent = require('../../../es/string/virtual/code-point-at'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/trim-left.js 0000644 0001750 0000144 00000000130 03560116604 027037 0 ustar andreh users var parent = require('../../../es/string/virtual/trim-left'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/pad-end.js 0000644 0001750 0000144 00000000126 03560116604 026451 0 ustar andreh users var parent = require('../../../es/string/virtual/pad-end'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/small.js 0000644 0001750 0000144 00000000124 03560116604 026247 0 ustar andreh users var parent = require('../../../es/string/virtual/small'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/strike.js 0000644 0001750 0000144 00000000125 03560116604 026441 0 ustar andreh users var parent = require('../../../es/string/virtual/strike'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/bold.js 0000644 0001750 0000144 00000000123 03560116604 026056 0 ustar andreh users var parent = require('../../../es/string/virtual/bold'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/link.js 0000644 0001750 0000144 00000000123 03560116604 026073 0 ustar andreh users var parent = require('../../../es/string/virtual/link'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/big.js 0000644 0001750 0000144 00000000122 03560116604 025676 0 ustar andreh users var parent = require('../../../es/string/virtual/big'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/fontcolor.js 0000644 0001750 0000144 00000000130 03560116604 027141 0 ustar andreh users var parent = require('../../../es/string/virtual/fontcolor'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/fontsize.js 0000644 0001750 0000144 00000000127 03560116604 027003 0 ustar andreh users var parent = require('../../../es/string/virtual/fontsize'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/starts-with.js 0000644 0001750 0000144 00000000132 03560116604 027427 0 ustar andreh users var parent = require('../../../es/string/virtual/starts-with'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/trim-end.js 0000644 0001750 0000144 00000000127 03560116604 026661 0 ustar andreh users var parent = require('../../../es/string/virtual/trim-end'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/trim-start.js 0000644 0001750 0000144 00000000131 03560116604 027243 0 ustar andreh users var parent = require('../../../es/string/virtual/trim-start'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/at.js 0000644 0001750 0000144 00000000233 03560116604 025544 0 ustar andreh users require('../../../modules/esnext.string.at'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').at; apollo-server-demo/node_modules/core-js/features/string/virtual/blink.js 0000644 0001750 0000144 00000000124 03560116604 026236 0 ustar andreh users var parent = require('../../../es/string/virtual/blink'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/sup.js 0000644 0001750 0000144 00000000122 03560116604 025744 0 ustar andreh users var parent = require('../../../es/string/virtual/sup'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/trim.js 0000644 0001750 0000144 00000000123 03560116604 026111 0 ustar andreh users var parent = require('../../../es/string/virtual/trim'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/pad-start.js 0000644 0001750 0000144 00000000130 03560116604 027033 0 ustar andreh users var parent = require('../../../es/string/virtual/pad-start'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/anchor.js 0000644 0001750 0000144 00000000125 03560116604 026412 0 ustar andreh users var parent = require('../../../es/string/virtual/anchor'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/replace-all.js 0000644 0001750 0000144 00000000263 03560116604 027324 0 ustar andreh users // TODO: remove from `core-js@4` require('../../../modules/esnext.string.replace-all'); var parent = require('../../../es/string/virtual/replace-all'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/match-all.js 0000644 0001750 0000144 00000000257 03560116604 027010 0 ustar andreh users // TODO: remove from `core-js@4` require('../../../modules/esnext.string.match-all'); var parent = require('../../../es/string/virtual/match-all'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/trim-right.js 0000644 0001750 0000144 00000000131 03560116604 027223 0 ustar andreh users var parent = require('../../../es/string/virtual/trim-right'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/virtual/ends-with.js 0000644 0001750 0000144 00000000130 03560116604 027036 0 ustar andreh users var parent = require('../../../es/string/virtual/ends-with'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/small.js 0000644 0001750 0000144 00000000111 03560116604 024555 0 ustar andreh users var parent = require('../../es/string/small'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/strike.js 0000644 0001750 0000144 00000000112 03560116604 024747 0 ustar andreh users var parent = require('../../es/string/strike'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/bold.js 0000644 0001750 0000144 00000000110 03560116604 024364 0 ustar andreh users var parent = require('../../es/string/bold'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/link.js 0000644 0001750 0000144 00000000110 03560116604 024401 0 ustar andreh users var parent = require('../../es/string/link'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/big.js 0000644 0001750 0000144 00000000107 03560116604 024213 0 ustar andreh users var parent = require('../../es/string/big'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/fontcolor.js 0000644 0001750 0000144 00000000115 03560116604 025456 0 ustar andreh users var parent = require('../../es/string/fontcolor'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/split.js 0000644 0001750 0000144 00000000111 03560116604 024600 0 ustar andreh users var parent = require('../../es/string/split'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/fontsize.js 0000644 0001750 0000144 00000000114 03560116604 025311 0 ustar andreh users var parent = require('../../es/string/fontsize'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/starts-with.js 0000644 0001750 0000144 00000000117 03560116604 025744 0 ustar andreh users var parent = require('../../es/string/starts-with'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/trim-end.js 0000644 0001750 0000144 00000000114 03560116604 025167 0 ustar andreh users var parent = require('../../es/string/trim-end'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/search.js 0000644 0001750 0000144 00000000112 03560116604 024713 0 ustar andreh users var parent = require('../../es/string/search'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/replace.js 0000644 0001750 0000144 00000000113 03560116604 025062 0 ustar andreh users var parent = require('../../es/string/replace'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/trim-start.js 0000644 0001750 0000144 00000000116 03560116604 025560 0 ustar andreh users var parent = require('../../es/string/trim-start'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/at.js 0000644 0001750 0000144 00000000225 03560116604 024057 0 ustar andreh users require('../../modules/esnext.string.at'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'at'); apollo-server-demo/node_modules/core-js/features/string/blink.js 0000644 0001750 0000144 00000000111 03560116604 024544 0 ustar andreh users var parent = require('../../es/string/blink'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/sup.js 0000644 0001750 0000144 00000000107 03560116604 024261 0 ustar andreh users var parent = require('../../es/string/sup'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/trim.js 0000644 0001750 0000144 00000000110 03560116604 024417 0 ustar andreh users var parent = require('../../es/string/trim'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/pad-start.js 0000644 0001750 0000144 00000000115 03560116604 025350 0 ustar andreh users var parent = require('../../es/string/pad-start'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/anchor.js 0000644 0001750 0000144 00000000112 03560116604 024720 0 ustar andreh users var parent = require('../../es/string/anchor'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/replace-all.js 0000644 0001750 0000144 00000000245 03560116604 025636 0 ustar andreh users // TODO: remove from `core-js@4` require('../../modules/esnext.string.replace-all'); var parent = require('../../es/string/replace-all'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/match-all.js 0000644 0001750 0000144 00000000241 03560116604 025313 0 ustar andreh users // TODO: remove from `core-js@4` require('../../modules/esnext.string.match-all'); var parent = require('../../es/string/match-all'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/trim-right.js 0000644 0001750 0000144 00000000116 03560116604 025540 0 ustar andreh users var parent = require('../../es/string/trim-right'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/from-code-point.js 0000644 0001750 0000144 00000000123 03560116604 026452 0 ustar andreh users var parent = require('../../es/string/from-code-point'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/ends-with.js 0000644 0001750 0000144 00000000115 03560116604 025353 0 ustar andreh users var parent = require('../../es/string/ends-with'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/string/raw.js 0000644 0001750 0000144 00000000107 03560116604 024243 0 ustar andreh users var parent = require('../../es/string/raw'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/date/ 0000755 0001750 0000144 00000000000 14067647701 022540 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/date/index.js 0000644 0001750 0000144 00000000101 03560116604 024162 0 ustar andreh users var parent = require('../../es/date'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/date/to-iso-string.js 0000644 0001750 0000144 00000000117 03560116604 025600 0 ustar andreh users var parent = require('../../es/date/to-iso-string'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/date/now.js 0000644 0001750 0000144 00000000105 03560116604 023662 0 ustar andreh users var parent = require('../../es/date/now'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/date/to-primitive.js 0000644 0001750 0000144 00000000116 03560116604 025511 0 ustar andreh users var parent = require('../../es/date/to-primitive'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/date/to-json.js 0000644 0001750 0000144 00000000111 03560116604 024445 0 ustar andreh users var parent = require('../../es/date/to-json'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/date/to-string.js 0000644 0001750 0000144 00000000113 03560116604 025004 0 ustar andreh users var parent = require('../../es/date/to-string'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/function/ 0000755 0001750 0000144 00000000000 14067647701 023450 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/function/index.js 0000644 0001750 0000144 00000000105 03560116604 025076 0 ustar andreh users var parent = require('../../es/function'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/function/virtual/ 0000755 0001750 0000144 00000000000 14067647701 025136 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/function/virtual/index.js 0000644 0001750 0000144 00000000120 03560116604 026561 0 ustar andreh users var parent = require('../../../es/function/virtual'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/function/virtual/bind.js 0000644 0001750 0000144 00000000125 03560116604 026373 0 ustar andreh users var parent = require('../../../es/function/virtual/bind'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/function/bind.js 0000644 0001750 0000144 00000000112 03560116604 024701 0 ustar andreh users var parent = require('../../es/function/bind'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/function/has-instance.js 0000644 0001750 0000144 00000000122 03560116604 026343 0 ustar andreh users var parent = require('../../es/function/has-instance'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/function/name.js 0000644 0001750 0000144 00000000112 03560116604 024705 0 ustar andreh users var parent = require('../../es/function/name'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/set-immediate.js 0000644 0001750 0000144 00000000113 03560116604 024670 0 ustar andreh users var parent = require('../stable/set-immediate'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/global-this.js 0000644 0001750 0000144 00000000221 03560116604 024346 0 ustar andreh users // TODO: remove from `core-js@4` require('../modules/esnext.global-this'); var parent = require('../es/global-this'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/number/ 0000755 0001750 0000144 00000000000 14067647701 023113 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/number/index.js 0000644 0001750 0000144 00000000246 03560116604 024547 0 ustar andreh users var parent = require('../../es/number'); module.exports = parent; require('../../modules/esnext.number.from-string'); require('../../modules/esnext.number.range'); apollo-server-demo/node_modules/core-js/features/number/epsilon.js 0000644 0001750 0000144 00000000113 03560116604 025102 0 ustar andreh users var parent = require('../../es/number/epsilon'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/number/to-fixed.js 0000644 0001750 0000144 00000000114 03560116604 025151 0 ustar andreh users var parent = require('../../es/number/to-fixed'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/number/virtual/ 0000755 0001750 0000144 00000000000 14067647701 024601 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/number/virtual/index.js 0000644 0001750 0000144 00000000116 03560116604 026231 0 ustar andreh users var parent = require('../../../es/number/virtual'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/number/virtual/to-fixed.js 0000644 0001750 0000144 00000000127 03560116604 026643 0 ustar andreh users var parent = require('../../../es/number/virtual/to-fixed'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/number/virtual/to-precision.js 0000644 0001750 0000144 00000000133 03560116604 027534 0 ustar andreh users var parent = require('../../../es/number/virtual/to-precision'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/number/from-string.js 0000644 0001750 0000144 00000000212 03560116604 025700 0 ustar andreh users require('../../modules/esnext.number.from-string'); var path = require('../../internals/path'); module.exports = path.Number.fromString; apollo-server-demo/node_modules/core-js/features/number/constructor.js 0000644 0001750 0000144 00000000117 03560116604 026022 0 ustar andreh users var parent = require('../../es/number/constructor'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/number/min-safe-integer.js 0000644 0001750 0000144 00000000124 03560116604 026565 0 ustar andreh users var parent = require('../../es/number/min-safe-integer'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/number/max-safe-integer.js 0000644 0001750 0000144 00000000124 03560116604 026567 0 ustar andreh users var parent = require('../../es/number/max-safe-integer'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/number/is-integer.js 0000644 0001750 0000144 00000000116 03560116604 025502 0 ustar andreh users var parent = require('../../es/number/is-integer'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/number/to-precision.js 0000644 0001750 0000144 00000000120 03560116604 026042 0 ustar andreh users var parent = require('../../es/number/to-precision'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/number/parse-float.js 0000644 0001750 0000144 00000000117 03560116604 025652 0 ustar andreh users var parent = require('../../es/number/parse-float'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/number/parse-int.js 0000644 0001750 0000144 00000000115 03560116604 025335 0 ustar andreh users var parent = require('../../es/number/parse-int'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/number/is-nan.js 0000644 0001750 0000144 00000000112 03560116604 024615 0 ustar andreh users var parent = require('../../es/number/is-nan'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/number/is-finite.js 0000644 0001750 0000144 00000000115 03560116604 025322 0 ustar andreh users var parent = require('../../es/number/is-finite'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/number/is-safe-integer.js 0000644 0001750 0000144 00000000123 03560116604 026414 0 ustar andreh users var parent = require('../../es/number/is-safe-integer'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/number/range.js 0000644 0001750 0000144 00000000177 03560116604 024537 0 ustar andreh users require('../../modules/esnext.number.range'); var path = require('../../internals/path'); module.exports = path.Number.range; apollo-server-demo/node_modules/core-js/features/parse-float.js 0000644 0001750 0000144 00000000105 03560116604 024357 0 ustar andreh users var parent = require('../es/parse-float'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/promise/ 0000755 0001750 0000144 00000000000 14067647701 023301 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/promise/index.js 0000644 0001750 0000144 00000000445 03560116604 024736 0 ustar andreh users var parent = require('../../es/promise'); require('../../modules/esnext.aggregate-error'); // TODO: Remove from `core-js@4` require('../../modules/esnext.promise.all-settled'); require('../../modules/esnext.promise.try'); require('../../modules/esnext.promise.any'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/promise/finally.js 0000644 0001750 0000144 00000000114 03560116604 025256 0 ustar andreh users var parent = require('../../es/promise/finally'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/promise/try.js 0000644 0001750 0000144 00000000534 03560116604 024444 0 ustar andreh users 'use strict'; require('../../modules/es.promise'); require('../../modules/esnext.promise.try'); var path = require('../../internals/path'); var Promise = path.Promise; var promiseTry = Promise['try']; module.exports = { 'try': function (callbackfn) { return promiseTry.call(typeof this === 'function' ? this : Promise, callbackfn); } }['try']; apollo-server-demo/node_modules/core-js/features/promise/all-settled.js 0000644 0001750 0000144 00000000247 03560116604 026041 0 ustar andreh users // TODO: Remove from `core-js@4` require('../../modules/esnext.promise.all-settled'); var parent = require('../../es/promise/all-settled'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/promise/any.js 0000644 0001750 0000144 00000000310 03560116604 024405 0 ustar andreh users var parent = require('../../es/promise/any'); // TODO: Remove from `core-js@4` require('../../modules/esnext.aggregate-error'); require('../../modules/esnext.promise.any'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/clear-immediate.js 0000644 0001750 0000144 00000000115 03560116604 025165 0 ustar andreh users var parent = require('../stable/clear-immediate'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/set-interval.js 0000644 0001750 0000144 00000000112 03560116604 024555 0 ustar andreh users var parent = require('../stable/set-interval'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/aggregate-error.js 0000644 0001750 0000144 00000000235 03560116604 025223 0 ustar andreh users // TODO: remove from `core-js@4` require('../modules/esnext.aggregate-error'); var parent = require('../stable/aggregate-error'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/object/ 0000755 0001750 0000144 00000000000 14067647701 023071 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/object/get-own-property-names.js 0000644 0001750 0000144 00000000132 03560116604 027753 0 ustar andreh users var parent = require('../../es/object/get-own-property-names'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/object/index.js 0000644 0001750 0000144 00000000347 03560116604 024527 0 ustar andreh users var parent = require('../../es/object'); require('../../modules/esnext.object.iterate-entries'); require('../../modules/esnext.object.iterate-keys'); require('../../modules/esnext.object.iterate-values'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/object/lookup-getter.js 0000644 0001750 0000144 00000000121 03560116604 026207 0 ustar andreh users var parent = require('../../es/object/lookup-getter'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/object/prevent-extensions.js 0000644 0001750 0000144 00000000126 03560116604 027273 0 ustar andreh users var parent = require('../../es/object/prevent-extensions'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/object/get-own-property-descriptor.js 0000644 0001750 0000144 00000000137 03560116604 031033 0 ustar andreh users var parent = require('../../es/object/get-own-property-descriptor'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/object/iterate-keys.js 0000644 0001750 0000144 00000000214 03560116604 026017 0 ustar andreh users require('../../modules/esnext.object.iterate-keys'); var path = require('../../internals/path'); module.exports = path.Object.iterateKeys; apollo-server-demo/node_modules/core-js/features/object/get-prototype-of.js 0000644 0001750 0000144 00000000124 03560116604 026635 0 ustar andreh users var parent = require('../../es/object/get-prototype-of'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/object/define-getter.js 0000644 0001750 0000144 00000000121 03560116604 026130 0 ustar andreh users var parent = require('../../es/object/define-getter'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/object/is-sealed.js 0000644 0001750 0000144 00000000115 03560116604 025257 0 ustar andreh users var parent = require('../../es/object/is-sealed'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/object/assign.js 0000644 0001750 0000144 00000000112 03560116604 024672 0 ustar andreh users var parent = require('../../es/object/assign'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/object/define-property.js 0000644 0001750 0000144 00000000123 03560116604 026524 0 ustar andreh users var parent = require('../../es/object/define-property'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/object/get-own-property-symbols.js 0000644 0001750 0000144 00000000134 03560116604 030342 0 ustar andreh users var parent = require('../../es/object/get-own-property-symbols'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/object/create.js 0000644 0001750 0000144 00000000112 03560116604 024651 0 ustar andreh users var parent = require('../../es/object/create'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/object/values.js 0000644 0001750 0000144 00000000112 03560116604 024705 0 ustar andreh users var parent = require('../../es/object/values'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/object/iterate-entries.js 0000644 0001750 0000144 00000000222 03560116604 026514 0 ustar andreh users require('../../modules/esnext.object.iterate-entries'); var path = require('../../internals/path'); module.exports = path.Object.iterateEntries; apollo-server-demo/node_modules/core-js/features/object/define-setter.js 0000644 0001750 0000144 00000000121 03560116604 026144 0 ustar andreh users var parent = require('../../es/object/define-setter'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/object/entries.js 0000644 0001750 0000144 00000000113 03560116604 025060 0 ustar andreh users var parent = require('../../es/object/entries'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/object/iterate-values.js 0000644 0001750 0000144 00000000220 03560116604 026340 0 ustar andreh users require('../../modules/esnext.object.iterate-values'); var path = require('../../internals/path'); module.exports = path.Object.iterateValues; apollo-server-demo/node_modules/core-js/features/object/freeze.js 0000644 0001750 0000144 00000000112 03560116604 024666 0 ustar andreh users var parent = require('../../es/object/freeze'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/object/get-own-property-descriptors.js 0000644 0001750 0000144 00000000140 03560116604 031210 0 ustar andreh users var parent = require('../../es/object/get-own-property-descriptors'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/object/set-prototype-of.js 0000644 0001750 0000144 00000000124 03560116604 026651 0 ustar andreh users var parent = require('../../es/object/set-prototype-of'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/object/is.js 0000644 0001750 0000144 00000000106 03560116604 024024 0 ustar andreh users var parent = require('../../es/object/is'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/object/seal.js 0000644 0001750 0000144 00000000110 03560116604 024330 0 ustar andreh users var parent = require('../../es/object/seal'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/object/keys.js 0000644 0001750 0000144 00000000110 03560116604 024357 0 ustar andreh users var parent = require('../../es/object/keys'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/object/from-entries.js 0000644 0001750 0000144 00000000120 03560116604 026017 0 ustar andreh users var parent = require('../../es/object/from-entries'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/object/to-string.js 0000644 0001750 0000144 00000000115 03560116604 025337 0 ustar andreh users var parent = require('../../es/object/to-string'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/object/is-extensible.js 0000644 0001750 0000144 00000000121 03560116604 026161 0 ustar andreh users var parent = require('../../es/object/is-extensible'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/object/lookup-setter.js 0000644 0001750 0000144 00000000121 03560116604 026223 0 ustar andreh users var parent = require('../../es/object/lookup-setter'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/object/is-frozen.js 0000644 0001750 0000144 00000000115 03560116604 025325 0 ustar andreh users var parent = require('../../es/object/is-frozen'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/object/define-properties.js 0000644 0001750 0000144 00000000125 03560116604 027036 0 ustar andreh users var parent = require('../../es/object/define-properties'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/set-timeout.js 0000644 0001750 0000144 00000000111 03560116604 024416 0 ustar andreh users var parent = require('../stable/set-timeout'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/parse-int.js 0000644 0001750 0000144 00000000103 03560116604 024042 0 ustar andreh users var parent = require('../es/parse-int'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/async-iterator/ 0000755 0001750 0000144 00000000000 14067647701 024567 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/async-iterator/take.js 0000644 0001750 0000144 00000000634 03560116604 026041 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.promise'); require('../../modules/es.string.iterator'); require('../../modules/esnext.async-iterator.constructor'); require('../../modules/esnext.async-iterator.take'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('AsyncIterator', 'take'); apollo-server-demo/node_modules/core-js/features/async-iterator/reduce.js 0000644 0001750 0000144 00000000640 03560116604 026361 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.promise'); require('../../modules/es.string.iterator'); require('../../modules/esnext.async-iterator.constructor'); require('../../modules/esnext.async-iterator.reduce'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('AsyncIterator', 'reduce'); apollo-server-demo/node_modules/core-js/features/async-iterator/to-array.js 0000644 0001750 0000144 00000000643 03560116604 026653 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.promise'); require('../../modules/es.string.iterator'); require('../../modules/esnext.async-iterator.constructor'); require('../../modules/esnext.async-iterator.to-array'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('AsyncIterator', 'toArray'); apollo-server-demo/node_modules/core-js/features/async-iterator/some.js 0000644 0001750 0000144 00000000634 03560116604 026060 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.promise'); require('../../modules/es.string.iterator'); require('../../modules/esnext.async-iterator.constructor'); require('../../modules/esnext.async-iterator.some'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('AsyncIterator', 'some'); apollo-server-demo/node_modules/core-js/features/async-iterator/index.js 0000644 0001750 0000144 00000002023 03560116604 026216 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.promise'); require('../../modules/es.string.iterator'); require('../../modules/esnext.async-iterator.constructor'); require('../../modules/esnext.async-iterator.as-indexed-pairs'); require('../../modules/esnext.async-iterator.drop'); require('../../modules/esnext.async-iterator.every'); require('../../modules/esnext.async-iterator.filter'); require('../../modules/esnext.async-iterator.find'); require('../../modules/esnext.async-iterator.flat-map'); require('../../modules/esnext.async-iterator.for-each'); require('../../modules/esnext.async-iterator.from'); require('../../modules/esnext.async-iterator.map'); require('../../modules/esnext.async-iterator.reduce'); require('../../modules/esnext.async-iterator.some'); require('../../modules/esnext.async-iterator.take'); require('../../modules/esnext.async-iterator.to-array'); require('../../modules/web.dom-collections.iterator'); var path = require('../../internals/path'); module.exports = path.AsyncIterator; apollo-server-demo/node_modules/core-js/features/async-iterator/map.js 0000644 0001750 0000144 00000000632 03560116604 025670 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.promise'); require('../../modules/es.string.iterator'); require('../../modules/esnext.async-iterator.constructor'); require('../../modules/esnext.async-iterator.map'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('AsyncIterator', 'map'); apollo-server-demo/node_modules/core-js/features/async-iterator/every.js 0000644 0001750 0000144 00000000636 03560116604 026251 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.promise'); require('../../modules/es.string.iterator'); require('../../modules/esnext.async-iterator.constructor'); require('../../modules/esnext.async-iterator.every'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('AsyncIterator', 'every'); apollo-server-demo/node_modules/core-js/features/async-iterator/flat-map.js 0000644 0001750 0000144 00000000643 03560116604 026616 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.promise'); require('../../modules/es.string.iterator'); require('../../modules/esnext.async-iterator.constructor'); require('../../modules/esnext.async-iterator.flat-map'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('AsyncIterator', 'flatMap'); apollo-server-demo/node_modules/core-js/features/async-iterator/drop.js 0000644 0001750 0000144 00000000634 03560116604 026061 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.promise'); require('../../modules/es.string.iterator'); require('../../modules/esnext.async-iterator.constructor'); require('../../modules/esnext.async-iterator.drop'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('AsyncIterator', 'drop'); apollo-server-demo/node_modules/core-js/features/async-iterator/from.js 0000644 0001750 0000144 00000000600 03560116604 026051 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.promise'); require('../../modules/es.string.iterator'); require('../../modules/esnext.async-iterator.constructor'); require('../../modules/esnext.async-iterator.from'); require('../../modules/web.dom-collections.iterator'); var path = require('../../internals/path'); module.exports = path.AsyncIterator.from; apollo-server-demo/node_modules/core-js/features/async-iterator/filter.js 0000644 0001750 0000144 00000000640 03560116604 026377 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.promise'); require('../../modules/es.string.iterator'); require('../../modules/esnext.async-iterator.constructor'); require('../../modules/esnext.async-iterator.filter'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('AsyncIterator', 'filter'); apollo-server-demo/node_modules/core-js/features/async-iterator/for-each.js 0000644 0001750 0000144 00000000643 03560116604 026601 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.promise'); require('../../modules/es.string.iterator'); require('../../modules/esnext.async-iterator.constructor'); require('../../modules/esnext.async-iterator.for-each'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('AsyncIterator', 'forEach'); apollo-server-demo/node_modules/core-js/features/async-iterator/find.js 0000644 0001750 0000144 00000000634 03560116604 026035 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.promise'); require('../../modules/es.string.iterator'); require('../../modules/esnext.async-iterator.constructor'); require('../../modules/esnext.async-iterator.find'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('AsyncIterator', 'find'); apollo-server-demo/node_modules/core-js/features/async-iterator/as-indexed-pairs.js 0000644 0001750 0000144 00000000662 03560116604 030253 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.promise'); require('../../modules/es.string.iterator'); require('../../modules/esnext.async-iterator.constructor'); require('../../modules/esnext.async-iterator.as-indexed-pairs'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('AsyncIterator', 'asIndexedPairs'); apollo-server-demo/node_modules/core-js/features/get-iterator.js 0000644 0001750 0000144 00000000265 03560116604 024557 0 ustar andreh users require('../modules/web.dom-collections.iterator'); require('../modules/es.string.iterator'); var getIterator = require('../internals/get-iterator'); module.exports = getIterator; apollo-server-demo/node_modules/core-js/features/set/ 0000755 0001750 0000144 00000000000 14067647701 022416 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/set/reduce.js 0000644 0001750 0000144 00000000270 03560116604 024207 0 ustar andreh users require('../../modules/es.set'); require('../../modules/esnext.set.reduce'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Set', 'reduce'); apollo-server-demo/node_modules/core-js/features/set/some.js 0000644 0001750 0000144 00000000264 03560116604 023706 0 ustar andreh users require('../../modules/es.set'); require('../../modules/esnext.set.some'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Set', 'some'); apollo-server-demo/node_modules/core-js/features/set/index.js 0000644 0001750 0000144 00000001574 03560116604 024057 0 ustar andreh users var parent = require('../../es/set'); require('../../modules/esnext.set.from'); require('../../modules/esnext.set.of'); require('../../modules/esnext.set.add-all'); require('../../modules/esnext.set.delete-all'); require('../../modules/esnext.set.every'); require('../../modules/esnext.set.difference'); require('../../modules/esnext.set.filter'); require('../../modules/esnext.set.find'); require('../../modules/esnext.set.intersection'); require('../../modules/esnext.set.is-disjoint-from'); require('../../modules/esnext.set.is-subset-of'); require('../../modules/esnext.set.is-superset-of'); require('../../modules/esnext.set.join'); require('../../modules/esnext.set.map'); require('../../modules/esnext.set.reduce'); require('../../modules/esnext.set.some'); require('../../modules/esnext.set.symmetric-difference'); require('../../modules/esnext.set.union'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/set/add-all.js 0000644 0001750 0000144 00000000271 03560116604 024237 0 ustar andreh users require('../../modules/es.set'); require('../../modules/esnext.set.add-all'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Set', 'addAll'); apollo-server-demo/node_modules/core-js/features/set/map.js 0000644 0001750 0000144 00000000262 03560116604 023516 0 ustar andreh users require('../../modules/es.set'); require('../../modules/esnext.set.map'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Set', 'map'); apollo-server-demo/node_modules/core-js/features/set/intersection.js 0000644 0001750 0000144 00000000304 03560116604 025444 0 ustar andreh users require('../../modules/es.set'); require('../../modules/esnext.set.intersection'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Set', 'intersection'); apollo-server-demo/node_modules/core-js/features/set/is-subset-of.js 0000644 0001750 0000144 00000000446 03560116604 025265 0 ustar andreh users require('../../modules/es.set'); require('../../modules/es.string.iterator'); require('../../modules/esnext.set.is-subset-of'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Set', 'isSubsetOf'); apollo-server-demo/node_modules/core-js/features/set/delete-all.js 0000644 0001750 0000144 00000000277 03560116604 024757 0 ustar andreh users require('../../modules/es.set'); require('../../modules/esnext.set.delete-all'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Set', 'deleteAll'); apollo-server-demo/node_modules/core-js/features/set/is-disjoint-from.js 0000644 0001750 0000144 00000000312 03560116604 026132 0 ustar andreh users require('../../modules/es.set'); require('../../modules/esnext.set.is-disjoint-from'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Set', 'isDisjointFrom'); apollo-server-demo/node_modules/core-js/features/set/every.js 0000644 0001750 0000144 00000000266 03560116604 024077 0 ustar andreh users require('../../modules/es.set'); require('../../modules/esnext.set.every'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Set', 'every'); apollo-server-demo/node_modules/core-js/features/set/symmetric-difference.js 0000644 0001750 0000144 00000000467 03560116604 027054 0 ustar andreh users require('../../modules/es.set'); require('../../modules/es.string.iterator'); require('../../modules/esnext.set.symmetric-difference'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Set', 'symmetricDifference'); apollo-server-demo/node_modules/core-js/features/set/difference.js 0000644 0001750 0000144 00000000444 03560116604 025035 0 ustar andreh users require('../../modules/es.set'); require('../../modules/es.string.iterator'); require('../../modules/esnext.set.difference'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Set', 'difference'); apollo-server-demo/node_modules/core-js/features/set/union.js 0000644 0001750 0000144 00000000432 03560116604 024070 0 ustar andreh users require('../../modules/es.set'); require('../../modules/es.string.iterator'); require('../../modules/esnext.set.union'); require('../../modules/web.dom-collections.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Set', 'union'); apollo-server-demo/node_modules/core-js/features/set/join.js 0000644 0001750 0000144 00000000264 03560116604 023702 0 ustar andreh users require('../../modules/es.set'); require('../../modules/esnext.set.join'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Set', 'join'); apollo-server-demo/node_modules/core-js/features/set/from.js 0000644 0001750 0000144 00000000653 03560116604 023710 0 ustar andreh users 'use strict'; require('../../modules/es.set'); require('../../modules/es.string.iterator'); require('../../modules/esnext.set.from'); require('../../modules/web.dom-collections.iterator'); var path = require('../../internals/path'); var Set = path.Set; var setFrom = Set.from; module.exports = function from(source, mapFn, thisArg) { return setFrom.call(typeof this === 'function' ? this : Set, source, mapFn, thisArg); }; apollo-server-demo/node_modules/core-js/features/set/is-superset-of.js 0000644 0001750 0000144 00000000306 03560116604 025625 0 ustar andreh users require('../../modules/es.set'); require('../../modules/esnext.set.is-superset-of'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Set', 'isSupersetOf'); apollo-server-demo/node_modules/core-js/features/set/filter.js 0000644 0001750 0000144 00000000270 03560116604 024225 0 ustar andreh users require('../../modules/es.set'); require('../../modules/esnext.set.filter'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Set', 'filter'); apollo-server-demo/node_modules/core-js/features/set/find.js 0000644 0001750 0000144 00000000264 03560116604 023663 0 ustar andreh users require('../../modules/es.set'); require('../../modules/esnext.set.find'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Set', 'find'); apollo-server-demo/node_modules/core-js/features/set/of.js 0000644 0001750 0000144 00000000577 03560116604 023356 0 ustar andreh users 'use strict'; require('../../modules/es.set'); require('../../modules/es.string.iterator'); require('../../modules/esnext.set.of'); require('../../modules/web.dom-collections.iterator'); var path = require('../../internals/path'); var Set = path.Set; var setOf = Set.of; module.exports = function of() { return setOf.apply(typeof this === 'function' ? this : Set, arguments); }; apollo-server-demo/node_modules/core-js/features/url-search-params/ 0000755 0001750 0000144 00000000000 14067647701 025151 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/url-search-params/index.js 0000644 0001750 0000144 00000000122 03560116604 026576 0 ustar andreh users var parent = require('../../stable/url-search-params'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/data-view/ 0000755 0001750 0000144 00000000000 14067647701 023504 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/data-view/index.js 0000644 0001750 0000144 00000000106 03560116604 025133 0 ustar andreh users var parent = require('../../es/data-view'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/json/ 0000755 0001750 0000144 00000000000 14067647701 022574 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/json/index.js 0000644 0001750 0000144 00000000101 03560116604 024216 0 ustar andreh users var parent = require('../../es/json'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/json/to-string-tag.js 0000644 0001750 0000144 00000000117 03560116604 025615 0 ustar andreh users var parent = require('../../es/json/to-string-tag'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/json/stringify.js 0000644 0001750 0000144 00000000113 03560116604 025130 0 ustar andreh users var parent = require('../../es/json/stringify'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/math/ 0000755 0001750 0000144 00000000000 14067647701 022554 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/math/rad-per-deg.js 0000644 0001750 0000144 00000000123 03560116604 025162 0 ustar andreh users require('../../modules/esnext.math.rad-per-deg'); module.exports = 180 / Math.PI; apollo-server-demo/node_modules/core-js/features/math/index.js 0000644 0001750 0000144 00000001267 03560116604 024214 0 ustar andreh users var parent = require('../../es/math'); require('../../modules/esnext.math.clamp'); require('../../modules/esnext.math.deg-per-rad'); require('../../modules/esnext.math.degrees'); require('../../modules/esnext.math.fscale'); require('../../modules/esnext.math.rad-per-deg'); require('../../modules/esnext.math.radians'); require('../../modules/esnext.math.scale'); require('../../modules/esnext.math.seeded-prng'); require('../../modules/esnext.math.signbit'); // TODO: Remove from `core-js@4` require('../../modules/esnext.math.iaddh'); require('../../modules/esnext.math.isubh'); require('../../modules/esnext.math.imulh'); require('../../modules/esnext.math.umulh'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/math/imul.js 0000644 0001750 0000144 00000000106 03560116604 024042 0 ustar andreh users var parent = require('../../es/math/imul'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/math/iaddh.js 0000644 0001750 0000144 00000000173 03560116604 024151 0 ustar andreh users require('../../modules/esnext.math.iaddh'); var path = require('../../internals/path'); module.exports = path.Math.iaddh; apollo-server-demo/node_modules/core-js/features/math/cosh.js 0000644 0001750 0000144 00000000106 03560116604 024030 0 ustar andreh users var parent = require('../../es/math/cosh'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/math/fround.js 0000644 0001750 0000144 00000000110 03560116604 024364 0 ustar andreh users var parent = require('../../es/math/fround'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/math/trunc.js 0000644 0001750 0000144 00000000107 03560116604 024230 0 ustar andreh users var parent = require('../../es/math/trunc'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/math/to-string-tag.js 0000644 0001750 0000144 00000000117 03560116604 025575 0 ustar andreh users var parent = require('../../es/math/to-string-tag'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/math/acosh.js 0000644 0001750 0000144 00000000107 03560116604 024172 0 ustar andreh users var parent = require('../../es/math/acosh'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/math/clz32.js 0000644 0001750 0000144 00000000107 03560116604 024032 0 ustar andreh users var parent = require('../../es/math/clz32'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/math/scale.js 0000644 0001750 0000144 00000000173 03560116604 024167 0 ustar andreh users require('../../modules/esnext.math.scale'); var path = require('../../internals/path'); module.exports = path.Math.scale; apollo-server-demo/node_modules/core-js/features/math/deg-per-rad.js 0000644 0001750 0000144 00000000123 03560116604 025162 0 ustar andreh users require('../../modules/esnext.math.deg-per-rad'); module.exports = Math.PI / 180; apollo-server-demo/node_modules/core-js/features/math/signbit.js 0000644 0001750 0000144 00000000177 03560116604 024543 0 ustar andreh users require('../../modules/esnext.math.signbit'); var path = require('../../internals/path'); module.exports = path.Math.signbit; apollo-server-demo/node_modules/core-js/features/math/sign.js 0000644 0001750 0000144 00000000106 03560116604 024034 0 ustar andreh users var parent = require('../../es/math/sign'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/math/radians.js 0000644 0001750 0000144 00000000177 03560116604 024525 0 ustar andreh users require('../../modules/esnext.math.radians'); var path = require('../../internals/path'); module.exports = path.Math.radians; apollo-server-demo/node_modules/core-js/features/math/umulh.js 0000644 0001750 0000144 00000000173 03560116604 024232 0 ustar andreh users require('../../modules/esnext.math.umulh'); var path = require('../../internals/path'); module.exports = path.Math.umulh; apollo-server-demo/node_modules/core-js/features/math/expm1.js 0000644 0001750 0000144 00000000107 03560116604 024127 0 ustar andreh users var parent = require('../../es/math/expm1'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/math/atanh.js 0000644 0001750 0000144 00000000107 03560116604 024170 0 ustar andreh users var parent = require('../../es/math/atanh'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/math/sinh.js 0000644 0001750 0000144 00000000106 03560116604 024035 0 ustar andreh users var parent = require('../../es/math/sinh'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/math/degrees.js 0000644 0001750 0000144 00000000177 03560116604 024522 0 ustar andreh users require('../../modules/esnext.math.degrees'); var path = require('../../internals/path'); module.exports = path.Math.degrees; apollo-server-demo/node_modules/core-js/features/math/log1p.js 0000644 0001750 0000144 00000000107 03560116604 024117 0 ustar andreh users var parent = require('../../es/math/log1p'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/math/log10.js 0000644 0001750 0000144 00000000107 03560116604 024017 0 ustar andreh users var parent = require('../../es/math/log10'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/math/asinh.js 0000644 0001750 0000144 00000000107 03560116604 024177 0 ustar andreh users var parent = require('../../es/math/asinh'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/math/log2.js 0000644 0001750 0000144 00000000106 03560116604 023737 0 ustar andreh users var parent = require('../../es/math/log2'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/math/isubh.js 0000644 0001750 0000144 00000000173 03560116604 024212 0 ustar andreh users require('../../modules/esnext.math.isubh'); var path = require('../../internals/path'); module.exports = path.Math.isubh; apollo-server-demo/node_modules/core-js/features/math/hypot.js 0000644 0001750 0000144 00000000107 03560116604 024240 0 ustar andreh users var parent = require('../../es/math/hypot'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/math/imulh.js 0000644 0001750 0000144 00000000173 03560116604 024216 0 ustar andreh users require('../../modules/esnext.math.imulh'); var path = require('../../internals/path'); module.exports = path.Math.imulh; apollo-server-demo/node_modules/core-js/features/math/clamp.js 0000644 0001750 0000144 00000000173 03560116604 024174 0 ustar andreh users require('../../modules/esnext.math.clamp'); var path = require('../../internals/path'); module.exports = path.Math.clamp; apollo-server-demo/node_modules/core-js/features/math/fscale.js 0000644 0001750 0000144 00000000175 03560116604 024337 0 ustar andreh users require('../../modules/esnext.math.fscale'); var path = require('../../internals/path'); module.exports = path.Math.fscale; apollo-server-demo/node_modules/core-js/features/math/seeded-prng.js 0000644 0001750 0000144 00000000206 03560116604 025272 0 ustar andreh users require('../../modules/esnext.math.seeded-prng'); var path = require('../../internals/path'); module.exports = path.Math.seededPRNG; apollo-server-demo/node_modules/core-js/features/math/tanh.js 0000644 0001750 0000144 00000000106 03560116604 024026 0 ustar andreh users var parent = require('../../es/math/tanh'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/math/cbrt.js 0000644 0001750 0000144 00000000106 03560116604 024026 0 ustar andreh users var parent = require('../../es/math/cbrt'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/url/ 0000755 0001750 0000144 00000000000 14067647701 022425 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/url/index.js 0000644 0001750 0000144 00000000104 03560116604 024052 0 ustar andreh users var parent = require('../../stable/url'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/url/to-json.js 0000644 0001750 0000144 00000000114 03560116604 024335 0 ustar andreh users var parent = require('../../stable/url/to-json'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/typed-array/ 0000755 0001750 0000144 00000000000 14067647701 024064 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/typed-array/int32-array.js 0000644 0001750 0000144 00000000124 03560116604 026457 0 ustar andreh users var parent = require('../../es/typed-array/int32-array'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/typed-array/uint8-array.js 0000644 0001750 0000144 00000000124 03560116604 026567 0 ustar andreh users var parent = require('../../es/typed-array/uint8-array'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/typed-array/sort.js 0000644 0001750 0000144 00000000056 03560116604 025377 0 ustar andreh users require('../../modules/es.typed-array.sort'); apollo-server-demo/node_modules/core-js/features/typed-array/to-locale-string.js 0000644 0001750 0000144 00000000072 03560116604 027571 0 ustar andreh users require('../../modules/es.typed-array.to-locale-string'); apollo-server-demo/node_modules/core-js/features/typed-array/reduce.js 0000644 0001750 0000144 00000000060 03560116604 025652 0 ustar andreh users require('../../modules/es.typed-array.reduce'); apollo-server-demo/node_modules/core-js/features/typed-array/set.js 0000644 0001750 0000144 00000000055 03560116604 025202 0 ustar andreh users require('../../modules/es.typed-array.set'); apollo-server-demo/node_modules/core-js/features/typed-array/some.js 0000644 0001750 0000144 00000000056 03560116604 025353 0 ustar andreh users require('../../modules/es.typed-array.some'); apollo-server-demo/node_modules/core-js/features/typed-array/index.js 0000644 0001750 0000144 00000000260 03560116604 025514 0 ustar andreh users var parent = require('../../es/typed-array'); require('../../modules/esnext.typed-array.at'); require('../../modules/esnext.typed-array.filter-out'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/typed-array/iterator.js 0000644 0001750 0000144 00000000062 03560116604 026236 0 ustar andreh users require('../../modules/es.typed-array.iterator'); apollo-server-demo/node_modules/core-js/features/typed-array/float32-array.js 0000644 0001750 0000144 00000000126 03560116604 026774 0 ustar andreh users var parent = require('../../es/typed-array/float32-array'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/typed-array/copy-within.js 0000644 0001750 0000144 00000000065 03560116604 026662 0 ustar andreh users require('../../modules/es.typed-array.copy-within'); apollo-server-demo/node_modules/core-js/features/typed-array/map.js 0000644 0001750 0000144 00000000055 03560116604 025164 0 ustar andreh users require('../../modules/es.typed-array.map'); apollo-server-demo/node_modules/core-js/features/typed-array/uint16-array.js 0000644 0001750 0000144 00000000125 03560116604 026647 0 ustar andreh users var parent = require('../../es/typed-array/uint16-array'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/typed-array/includes.js 0000644 0001750 0000144 00000000062 03560116604 026213 0 ustar andreh users require('../../modules/es.typed-array.includes'); apollo-server-demo/node_modules/core-js/features/typed-array/reduce-right.js 0000644 0001750 0000144 00000000066 03560116604 026773 0 ustar andreh users require('../../modules/es.typed-array.reduce-right'); apollo-server-demo/node_modules/core-js/features/typed-array/every.js 0000644 0001750 0000144 00000000057 03560116604 025543 0 ustar andreh users require('../../modules/es.typed-array.every'); apollo-server-demo/node_modules/core-js/features/typed-array/fill.js 0000644 0001750 0000144 00000000056 03560116604 025336 0 ustar andreh users require('../../modules/es.typed-array.fill'); apollo-server-demo/node_modules/core-js/features/typed-array/index-of.js 0000644 0001750 0000144 00000000062 03560116604 026116 0 ustar andreh users require('../../modules/es.typed-array.index-of'); apollo-server-demo/node_modules/core-js/features/typed-array/last-index-of.js 0000644 0001750 0000144 00000000067 03560116604 027064 0 ustar andreh users require('../../modules/es.typed-array.last-index-of'); apollo-server-demo/node_modules/core-js/features/typed-array/uint32-array.js 0000644 0001750 0000144 00000000125 03560116604 026645 0 ustar andreh users var parent = require('../../es/typed-array/uint32-array'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/typed-array/values.js 0000644 0001750 0000144 00000000062 03560116604 025704 0 ustar andreh users require('../../modules/es.typed-array.iterator'); apollo-server-demo/node_modules/core-js/features/typed-array/int8-array.js 0000644 0001750 0000144 00000000123 03560116604 026401 0 ustar andreh users var parent = require('../../es/typed-array/int8-array'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/typed-array/join.js 0000644 0001750 0000144 00000000056 03560116604 025347 0 ustar andreh users require('../../modules/es.typed-array.join'); apollo-server-demo/node_modules/core-js/features/typed-array/from.js 0000644 0001750 0000144 00000000056 03560116604 025353 0 ustar andreh users require('../../modules/es.typed-array.from'); apollo-server-demo/node_modules/core-js/features/typed-array/entries.js 0000644 0001750 0000144 00000000062 03560116604 026056 0 ustar andreh users require('../../modules/es.typed-array.iterator'); apollo-server-demo/node_modules/core-js/features/typed-array/filter-out.js 0000644 0001750 0000144 00000000070 03560116604 026476 0 ustar andreh users require('../../modules/esnext.typed-array.filter-out'); apollo-server-demo/node_modules/core-js/features/typed-array/filter.js 0000644 0001750 0000144 00000000060 03560116604 025670 0 ustar andreh users require('../../modules/es.typed-array.filter'); apollo-server-demo/node_modules/core-js/features/typed-array/at.js 0000644 0001750 0000144 00000000060 03560116604 025007 0 ustar andreh users require('../../modules/esnext.typed-array.at'); apollo-server-demo/node_modules/core-js/features/typed-array/for-each.js 0000644 0001750 0000144 00000000062 03560116604 026071 0 ustar andreh users require('../../modules/es.typed-array.for-each'); apollo-server-demo/node_modules/core-js/features/typed-array/find-index.js 0000644 0001750 0000144 00000000064 03560116604 026434 0 ustar andreh users require('../../modules/es.typed-array.find-index'); apollo-server-demo/node_modules/core-js/features/typed-array/keys.js 0000644 0001750 0000144 00000000062 03560116604 025360 0 ustar andreh users require('../../modules/es.typed-array.iterator'); apollo-server-demo/node_modules/core-js/features/typed-array/find.js 0000644 0001750 0000144 00000000056 03560116604 025330 0 ustar andreh users require('../../modules/es.typed-array.find'); apollo-server-demo/node_modules/core-js/features/typed-array/to-string.js 0000644 0001750 0000144 00000000063 03560116604 026334 0 ustar andreh users require('../../modules/es.typed-array.to-string'); apollo-server-demo/node_modules/core-js/features/typed-array/int16-array.js 0000644 0001750 0000144 00000000124 03560116604 026461 0 ustar andreh users var parent = require('../../es/typed-array/int16-array'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/typed-array/of.js 0000644 0001750 0000144 00000000054 03560116604 025012 0 ustar andreh users require('../../modules/es.typed-array.of'); apollo-server-demo/node_modules/core-js/features/typed-array/reverse.js 0000644 0001750 0000144 00000000061 03560116604 026057 0 ustar andreh users require('../../modules/es.typed-array.reverse'); apollo-server-demo/node_modules/core-js/features/typed-array/subarray.js 0000644 0001750 0000144 00000000062 03560116604 026235 0 ustar andreh users require('../../modules/es.typed-array.subarray'); apollo-server-demo/node_modules/core-js/features/typed-array/uint8-clamped-array.js 0000644 0001750 0000144 00000000134 03560116604 030173 0 ustar andreh users var parent = require('../../es/typed-array/uint8-clamped-array'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/typed-array/float64-array.js 0000644 0001750 0000144 00000000126 03560116604 027001 0 ustar andreh users var parent = require('../../es/typed-array/float64-array'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/typed-array/slice.js 0000644 0001750 0000144 00000000057 03560116604 025510 0 ustar andreh users require('../../modules/es.typed-array.slice'); apollo-server-demo/node_modules/core-js/features/observable/ 0000755 0001750 0000144 00000000000 14067647701 023747 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/observable/index.js 0000644 0001750 0000144 00000000500 03560116604 025374 0 ustar andreh users require('../../modules/esnext.observable'); require('../../modules/esnext.symbol.observable'); require('../../modules/es.object.to-string'); require('../../modules/es.string.iterator'); require('../../modules/web.dom-collections.iterator'); var path = require('../../internals/path'); module.exports = path.Observable; apollo-server-demo/node_modules/core-js/features/weak-set/ 0000755 0001750 0000144 00000000000 14067647701 023343 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/weak-set/index.js 0000644 0001750 0000144 00000000410 03560116604 024770 0 ustar andreh users var parent = require('../../es/weak-set'); require('../../modules/esnext.weak-set.add-all'); require('../../modules/esnext.weak-set.delete-all'); require('../../modules/esnext.weak-set.from'); require('../../modules/esnext.weak-set.of'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/weak-set/add-all.js 0000644 0001750 0000144 00000000307 03560116604 025164 0 ustar andreh users require('../../modules/es.weak-set'); require('../../modules/esnext.weak-set.add-all'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('WeakSet', 'addAll'); apollo-server-demo/node_modules/core-js/features/weak-set/delete-all.js 0000644 0001750 0000144 00000000315 03560116604 025675 0 ustar andreh users require('../../modules/es.weak-set'); require('../../modules/esnext.weak-set.delete-all'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('WeakSet', 'deleteAll'); apollo-server-demo/node_modules/core-js/features/weak-set/from.js 0000644 0001750 0000144 00000000715 03560116604 024634 0 ustar andreh users 'use strict'; require('../../modules/es.string.iterator'); require('../../modules/es.weak-set'); require('../../modules/esnext.weak-set.from'); require('../../modules/web.dom-collections.iterator'); var path = require('../../internals/path'); var WeakSet = path.WeakSet; var weakSetfrom = WeakSet.from; module.exports = function from(source, mapFn, thisArg) { return weakSetfrom.call(typeof this === 'function' ? this : WeakSet, source, mapFn, thisArg); }; apollo-server-demo/node_modules/core-js/features/weak-set/of.js 0000644 0001750 0000144 00000000641 03560116604 024273 0 ustar andreh users 'use strict'; require('../../modules/es.string.iterator'); require('../../modules/es.weak-set'); require('../../modules/esnext.weak-set.of'); require('../../modules/web.dom-collections.iterator'); var path = require('../../internals/path'); var WeakSet = path.WeakSet; var weakSetOf = WeakSet.of; module.exports = function of() { return weakSetOf.apply(typeof this === 'function' ? this : WeakSet, arguments); }; apollo-server-demo/node_modules/core-js/features/reflect/ 0000755 0001750 0000144 00000000000 14067647701 023247 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/reflect/get-own-metadata.js 0000644 0001750 0000144 00000000225 03560116604 026727 0 ustar andreh users require('../../modules/esnext.reflect.get-own-metadata'); var path = require('../../internals/path'); module.exports = path.Reflect.getOwnMetadata; apollo-server-demo/node_modules/core-js/features/reflect/define-metadata.js 0000644 0001750 0000144 00000000224 03560116604 026600 0 ustar andreh users require('../../modules/esnext.reflect.define-metadata'); var path = require('../../internals/path'); module.exports = path.Reflect.defineMetadata; apollo-server-demo/node_modules/core-js/features/reflect/set.js 0000644 0001750 0000144 00000000110 03560116604 024355 0 ustar andreh users var parent = require('../../es/reflect/set'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/reflect/index.js 0000644 0001750 0000144 00000001102 03560116604 024673 0 ustar andreh users var parent = require('../../es/reflect'); require('../../modules/esnext.reflect.define-metadata'); require('../../modules/esnext.reflect.delete-metadata'); require('../../modules/esnext.reflect.get-metadata'); require('../../modules/esnext.reflect.get-metadata-keys'); require('../../modules/esnext.reflect.get-own-metadata'); require('../../modules/esnext.reflect.get-own-metadata-keys'); require('../../modules/esnext.reflect.has-metadata'); require('../../modules/esnext.reflect.has-own-metadata'); require('../../modules/esnext.reflect.metadata'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/reflect/prevent-extensions.js 0000644 0001750 0000144 00000000127 03560116604 027452 0 ustar andreh users var parent = require('../../es/reflect/prevent-extensions'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/reflect/get-own-property-descriptor.js 0000644 0001750 0000144 00000000140 03560116604 031203 0 ustar andreh users var parent = require('../../es/reflect/get-own-property-descriptor'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/reflect/delete-property.js 0000644 0001750 0000144 00000000124 03560116604 026713 0 ustar andreh users var parent = require('../../es/reflect/delete-property'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/reflect/get-prototype-of.js 0000644 0001750 0000144 00000000125 03560116604 027014 0 ustar andreh users var parent = require('../../es/reflect/get-prototype-of'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/reflect/get-own-metadata-keys.js 0000644 0001750 0000144 00000000236 03560116604 027702 0 ustar andreh users require('../../modules/esnext.reflect.get-own-metadata-keys'); var path = require('../../internals/path'); module.exports = path.Reflect.getOwnMetadataKeys; apollo-server-demo/node_modules/core-js/features/reflect/get-metadata.js 0000644 0001750 0000144 00000000216 03560116604 026126 0 ustar andreh users require('../../modules/esnext.reflect.get-metadata'); var path = require('../../internals/path'); module.exports = path.Reflect.getMetadata; apollo-server-demo/node_modules/core-js/features/reflect/to-string-tag.js 0000644 0001750 0000144 00000000120 03560116604 026262 0 ustar andreh users require('../../modules/es.reflect.to-string-tag'); module.exports = 'Reflect'; apollo-server-demo/node_modules/core-js/features/reflect/get-metadata-keys.js 0000644 0001750 0000144 00000000227 03560116604 027101 0 ustar andreh users require('../../modules/esnext.reflect.get-metadata-keys'); var path = require('../../internals/path'); module.exports = path.Reflect.getMetadataKeys; apollo-server-demo/node_modules/core-js/features/reflect/define-property.js 0000644 0001750 0000144 00000000124 03560116604 026703 0 ustar andreh users var parent = require('../../es/reflect/define-property'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/reflect/own-keys.js 0000644 0001750 0000144 00000000115 03560116604 025343 0 ustar andreh users var parent = require('../../es/reflect/own-keys'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/reflect/metadata.js 0000644 0001750 0000144 00000000207 03560116604 025351 0 ustar andreh users require('../../modules/esnext.reflect.metadata'); var path = require('../../internals/path'); module.exports = path.Reflect.metadata; apollo-server-demo/node_modules/core-js/features/reflect/apply.js 0000644 0001750 0000144 00000000112 03560116604 024711 0 ustar andreh users var parent = require('../../es/reflect/apply'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/reflect/has-own-metadata.js 0000644 0001750 0000144 00000000225 03560116604 026723 0 ustar andreh users require('../../modules/esnext.reflect.has-own-metadata'); var path = require('../../internals/path'); module.exports = path.Reflect.hasOwnMetadata; apollo-server-demo/node_modules/core-js/features/reflect/get.js 0000644 0001750 0000144 00000000110 03560116604 024341 0 ustar andreh users var parent = require('../../es/reflect/get'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/reflect/delete-metadata.js 0000644 0001750 0000144 00000000224 03560116604 026610 0 ustar andreh users require('../../modules/esnext.reflect.delete-metadata'); var path = require('../../internals/path'); module.exports = path.Reflect.deleteMetadata; apollo-server-demo/node_modules/core-js/features/reflect/has-metadata.js 0000644 0001750 0000144 00000000216 03560116604 026122 0 ustar andreh users require('../../modules/esnext.reflect.has-metadata'); var path = require('../../internals/path'); module.exports = path.Reflect.hasMetadata; apollo-server-demo/node_modules/core-js/features/reflect/set-prototype-of.js 0000644 0001750 0000144 00000000125 03560116604 027030 0 ustar andreh users var parent = require('../../es/reflect/set-prototype-of'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/reflect/construct.js 0000644 0001750 0000144 00000000116 03560116604 025614 0 ustar andreh users var parent = require('../../es/reflect/construct'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/reflect/is-extensible.js 0000644 0001750 0000144 00000000122 03560116604 026340 0 ustar andreh users var parent = require('../../es/reflect/is-extensible'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/reflect/has.js 0000644 0001750 0000144 00000000110 03560116604 024335 0 ustar andreh users var parent = require('../../es/reflect/has'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/regexp/ 0000755 0001750 0000144 00000000000 14067647701 023115 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/regexp/index.js 0000644 0001750 0000144 00000000103 03560116604 024541 0 ustar andreh users var parent = require('../../es/regexp'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/regexp/match.js 0000644 0001750 0000144 00000000111 03560116604 024525 0 ustar andreh users var parent = require('../../es/regexp/match'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/regexp/sticky.js 0000644 0001750 0000144 00000000112 03560116604 024740 0 ustar andreh users var parent = require('../../es/regexp/sticky'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/regexp/flags.js 0000644 0001750 0000144 00000000111 03560116604 024525 0 ustar andreh users var parent = require('../../es/regexp/flags'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/regexp/constructor.js 0000644 0001750 0000144 00000000117 03560116604 026024 0 ustar andreh users var parent = require('../../es/regexp/constructor'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/regexp/test.js 0000644 0001750 0000144 00000000110 03560116604 024407 0 ustar andreh users var parent = require('../../es/regexp/test'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/regexp/split.js 0000644 0001750 0000144 00000000111 03560116604 024564 0 ustar andreh users var parent = require('../../es/regexp/split'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/regexp/search.js 0000644 0001750 0000144 00000000112 03560116604 024677 0 ustar andreh users var parent = require('../../es/regexp/search'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/regexp/replace.js 0000644 0001750 0000144 00000000113 03560116604 025046 0 ustar andreh users var parent = require('../../es/regexp/replace'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/regexp/to-string.js 0000644 0001750 0000144 00000000115 03560116604 025363 0 ustar andreh users var parent = require('../../es/regexp/to-string'); module.exports = parent; apollo-server-demo/node_modules/core-js/features/bigint/ 0000755 0001750 0000144 00000000000 14067647701 023077 5 ustar andreh users apollo-server-demo/node_modules/core-js/features/bigint/index.js 0000644 0001750 0000144 00000000175 03560116604 024534 0 ustar andreh users require('../../modules/esnext.bigint.range'); var BigInt = require('../../internals/path').BigInt; module.exports = BigInt; apollo-server-demo/node_modules/core-js/features/bigint/range.js 0000644 0001750 0000144 00000000215 03560116604 024514 0 ustar andreh users require('../../modules/esnext.bigint.range'); var BigInt = require('../../internals/path').BigInt; module.exports = BigInt && BigInt.range; apollo-server-demo/node_modules/core-js/features/get-iterator-method.js 0000644 0001750 0000144 00000000310 03560116604 026024 0 ustar andreh users require('../modules/web.dom-collections.iterator'); require('../modules/es.string.iterator'); var getIteratorMethod = require('../internals/get-iterator-method'); module.exports = getIteratorMethod; apollo-server-demo/node_modules/core-js/internals/ 0000755 0001750 0000144 00000000000 14067647701 022004 5 ustar andreh users apollo-server-demo/node_modules/core-js/internals/set-global.js 0000644 0001750 0000144 00000000460 03560116604 024360 0 ustar andreh users var global = require('../internals/global'); var createNonEnumerableProperty = require('../internals/create-non-enumerable-property'); module.exports = function (key, value) { try { createNonEnumerableProperty(global, key, value); } catch (error) { global[key] = value; } return value; }; apollo-server-demo/node_modules/core-js/internals/create-property.js 0000644 0001750 0000144 00000000721 03560116604 025454 0 ustar andreh users 'use strict'; var toPrimitive = require('../internals/to-primitive'); var definePropertyModule = require('../internals/object-define-property'); var createPropertyDescriptor = require('../internals/create-property-descriptor'); module.exports = function (object, key, value) { var propertyKey = toPrimitive(key); if (propertyKey in object) definePropertyModule.f(object, propertyKey, createPropertyDescriptor(0, value)); else object[propertyKey] = value; }; apollo-server-demo/node_modules/core-js/internals/number-parse-float.js 0000644 0001750 0000144 00000001060 03560116604 026027 0 ustar andreh users var global = require('../internals/global'); var trim = require('../internals/string-trim').trim; var whitespaces = require('../internals/whitespaces'); var $parseFloat = global.parseFloat; var FORCED = 1 / $parseFloat(whitespaces + '-0') !== -Infinity; // `parseFloat` method // https://tc39.es/ecma262/#sec-parsefloat-string module.exports = FORCED ? function parseFloat(string) { var trimmedString = trim(String(string)); var result = $parseFloat(trimmedString); return result === 0 && trimmedString.charAt(0) == '-' ? -0 : result; } : $parseFloat; apollo-server-demo/node_modules/core-js/internals/regexp-sticky-helpers.js 0000644 0001750 0000144 00000001134 03560116604 026564 0 ustar andreh users 'use strict'; var fails = require('./fails'); // babel-minify transpiles RegExp('a', 'y') -> /a/y and it causes SyntaxError, // so we use an intermediate function. function RE(s, f) { return RegExp(s, f); } exports.UNSUPPORTED_Y = fails(function () { // babel-minify transpiles RegExp('a', 'y') -> /a/y and it causes SyntaxError var re = RE('a', 'y'); re.lastIndex = 2; return re.exec('abcd') != null; }); exports.BROKEN_CARET = fails(function () { // https://bugzilla.mozilla.org/show_bug.cgi?id=773687 var re = RE('^r', 'gy'); re.lastIndex = 2; return re.exec('str') != null; }); apollo-server-demo/node_modules/core-js/internals/collection-of.js 0000644 0001750 0000144 00000000352 03560116604 025064 0 ustar andreh users 'use strict'; // https://tc39.github.io/proposal-setmap-offrom/ module.exports = function of() { var length = arguments.length; var A = new Array(length); while (length--) A[length] = arguments[length]; return new this(A); }; apollo-server-demo/node_modules/core-js/internals/species-constructor.js 0000644 0001750 0000144 00000000775 03560116604 026356 0 ustar andreh users var anObject = require('../internals/an-object'); var aFunction = require('../internals/a-function'); var wellKnownSymbol = require('../internals/well-known-symbol'); var SPECIES = wellKnownSymbol('species'); // `SpeciesConstructor` abstract operation // https://tc39.es/ecma262/#sec-speciesconstructor module.exports = function (O, defaultConstructor) { var C = anObject(O).constructor; var S; return C === undefined || (S = anObject(C)[SPECIES]) == undefined ? defaultConstructor : aFunction(S); }; apollo-server-demo/node_modules/core-js/internals/object-keys-internal.js 0000644 0001750 0000144 00000001106 03560116604 026356 0 ustar andreh users var has = require('../internals/has'); var toIndexedObject = require('../internals/to-indexed-object'); var indexOf = require('../internals/array-includes').indexOf; var hiddenKeys = require('../internals/hidden-keys'); module.exports = function (object, names) { var O = toIndexedObject(object); var i = 0; var result = []; var key; for (key in O) !has(hiddenKeys, key) && has(O, key) && result.push(key); // Don't enum bug & hidden keys while (names.length > i) if (has(O, key = names[i++])) { ~indexOf(result, key) || result.push(key); } return result; }; apollo-server-demo/node_modules/core-js/internals/object-get-own-property-names.js 0000644 0001750 0000144 00000000633 03560116604 030140 0 ustar andreh users var internalObjectKeys = require('../internals/object-keys-internal'); var enumBugKeys = require('../internals/enum-bug-keys'); var hiddenKeys = enumBugKeys.concat('length', 'prototype'); // `Object.getOwnPropertyNames` method // https://tc39.es/ecma262/#sec-object.getownpropertynames exports.f = Object.getOwnPropertyNames || function getOwnPropertyNames(O) { return internalObjectKeys(O, hiddenKeys); }; apollo-server-demo/node_modules/core-js/internals/array-from.js 0000644 0001750 0000144 00000003454 03560116604 024414 0 ustar andreh users 'use strict'; var bind = require('../internals/function-bind-context'); var toObject = require('../internals/to-object'); var callWithSafeIterationClosing = require('../internals/call-with-safe-iteration-closing'); var isArrayIteratorMethod = require('../internals/is-array-iterator-method'); var toLength = require('../internals/to-length'); var createProperty = require('../internals/create-property'); var getIteratorMethod = require('../internals/get-iterator-method'); // `Array.from` method implementation // https://tc39.es/ecma262/#sec-array.from module.exports = function from(arrayLike /* , mapfn = undefined, thisArg = undefined */) { var O = toObject(arrayLike); var C = typeof this == 'function' ? this : Array; var argumentsLength = arguments.length; var mapfn = argumentsLength > 1 ? arguments[1] : undefined; var mapping = mapfn !== undefined; var iteratorMethod = getIteratorMethod(O); var index = 0; var length, result, step, iterator, next, value; if (mapping) mapfn = bind(mapfn, argumentsLength > 2 ? arguments[2] : undefined, 2); // if the target is not iterable or it's an array with the default iterator - use a simple case if (iteratorMethod != undefined && !(C == Array && isArrayIteratorMethod(iteratorMethod))) { iterator = iteratorMethod.call(O); next = iterator.next; result = new C(); for (;!(step = next.call(iterator)).done; index++) { value = mapping ? callWithSafeIterationClosing(iterator, mapfn, [step.value, index], true) : step.value; createProperty(result, index, value); } } else { length = toLength(O.length); result = new C(length); for (;length > index; index++) { value = mapping ? mapfn(O[index], index) : O[index]; createProperty(result, index, value); } } result.length = index; return result; }; apollo-server-demo/node_modules/core-js/internals/descriptors.js 0000644 0001750 0000144 00000000342 03560116604 024667 0 ustar andreh users var fails = require('../internals/fails'); // Detect IE8's incomplete defineProperty implementation module.exports = !fails(function () { return Object.defineProperty({}, 1, { get: function () { return 7; } })[1] != 7; }); apollo-server-demo/node_modules/core-js/internals/collection-weak.js 0000644 0001750 0000144 00000007473 03560116604 025422 0 ustar andreh users 'use strict'; var redefineAll = require('../internals/redefine-all'); var getWeakData = require('../internals/internal-metadata').getWeakData; var anObject = require('../internals/an-object'); var isObject = require('../internals/is-object'); var anInstance = require('../internals/an-instance'); var iterate = require('../internals/iterate'); var ArrayIterationModule = require('../internals/array-iteration'); var $has = require('../internals/has'); var InternalStateModule = require('../internals/internal-state'); var setInternalState = InternalStateModule.set; var internalStateGetterFor = InternalStateModule.getterFor; var find = ArrayIterationModule.find; var findIndex = ArrayIterationModule.findIndex; var id = 0; // fallback for uncaught frozen keys var uncaughtFrozenStore = function (store) { return store.frozen || (store.frozen = new UncaughtFrozenStore()); }; var UncaughtFrozenStore = function () { this.entries = []; }; var findUncaughtFrozen = function (store, key) { return find(store.entries, function (it) { return it[0] === key; }); }; UncaughtFrozenStore.prototype = { get: function (key) { var entry = findUncaughtFrozen(this, key); if (entry) return entry[1]; }, has: function (key) { return !!findUncaughtFrozen(this, key); }, set: function (key, value) { var entry = findUncaughtFrozen(this, key); if (entry) entry[1] = value; else this.entries.push([key, value]); }, 'delete': function (key) { var index = findIndex(this.entries, function (it) { return it[0] === key; }); if (~index) this.entries.splice(index, 1); return !!~index; } }; module.exports = { getConstructor: function (wrapper, CONSTRUCTOR_NAME, IS_MAP, ADDER) { var C = wrapper(function (that, iterable) { anInstance(that, C, CONSTRUCTOR_NAME); setInternalState(that, { type: CONSTRUCTOR_NAME, id: id++, frozen: undefined }); if (iterable != undefined) iterate(iterable, that[ADDER], { that: that, AS_ENTRIES: IS_MAP }); }); var getInternalState = internalStateGetterFor(CONSTRUCTOR_NAME); var define = function (that, key, value) { var state = getInternalState(that); var data = getWeakData(anObject(key), true); if (data === true) uncaughtFrozenStore(state).set(key, value); else data[state.id] = value; return that; }; redefineAll(C.prototype, { // 23.3.3.2 WeakMap.prototype.delete(key) // 23.4.3.3 WeakSet.prototype.delete(value) 'delete': function (key) { var state = getInternalState(this); if (!isObject(key)) return false; var data = getWeakData(key); if (data === true) return uncaughtFrozenStore(state)['delete'](key); return data && $has(data, state.id) && delete data[state.id]; }, // 23.3.3.4 WeakMap.prototype.has(key) // 23.4.3.4 WeakSet.prototype.has(value) has: function has(key) { var state = getInternalState(this); if (!isObject(key)) return false; var data = getWeakData(key); if (data === true) return uncaughtFrozenStore(state).has(key); return data && $has(data, state.id); } }); redefineAll(C.prototype, IS_MAP ? { // 23.3.3.3 WeakMap.prototype.get(key) get: function get(key) { var state = getInternalState(this); if (isObject(key)) { var data = getWeakData(key); if (data === true) return uncaughtFrozenStore(state).get(key); return data ? data[state.id] : undefined; } }, // 23.3.3.5 WeakMap.prototype.set(key, value) set: function set(key, value) { return define(this, key, value); } } : { // 23.4.3.1 WeakSet.prototype.add(value) add: function add(value) { return define(this, value, true); } }); return C; } }; apollo-server-demo/node_modules/core-js/internals/composite-key.js 0000644 0001750 0000144 00000002576 03560116604 025131 0 ustar andreh users // TODO: in core-js@4, move /modules/ dependencies to public entries for better optimization by tools like `preset-env` var Map = require('../modules/es.map'); var WeakMap = require('../modules/es.weak-map'); var create = require('../internals/object-create'); var isObject = require('../internals/is-object'); var Node = function () { // keys this.object = null; this.symbol = null; // child nodes this.primitives = null; this.objectsByIndex = create(null); }; Node.prototype.get = function (key, initializer) { return this[key] || (this[key] = initializer()); }; Node.prototype.next = function (i, it, IS_OBJECT) { var store = IS_OBJECT ? this.objectsByIndex[i] || (this.objectsByIndex[i] = new WeakMap()) : this.primitives || (this.primitives = new Map()); var entry = store.get(it); if (!entry) store.set(it, entry = new Node()); return entry; }; var root = new Node(); module.exports = function () { var active = root; var length = arguments.length; var i, it; // for prevent leaking, start from objects for (i = 0; i < length; i++) { if (isObject(it = arguments[i])) active = active.next(i, it, true); } if (this === Object && active === root) throw TypeError('Composite keys must contain a non-primitive component'); for (i = 0; i < length; i++) { if (!isObject(it = arguments[i])) active = active.next(i, it, false); } return active; }; apollo-server-demo/node_modules/core-js/internals/async-iterator-iteration.js 0000644 0001750 0000144 00000004631 03560116604 027273 0 ustar andreh users 'use strict'; // https://github.com/tc39/proposal-iterator-helpers var aFunction = require('../internals/a-function'); var anObject = require('../internals/an-object'); var getBuiltIn = require('../internals/get-built-in'); var Promise = getBuiltIn('Promise'); var push = [].push; var createMethod = function (TYPE) { var IS_TO_ARRAY = TYPE == 0; var IS_FOR_EACH = TYPE == 1; var IS_EVERY = TYPE == 2; var IS_SOME = TYPE == 3; return function (iterator, fn) { anObject(iterator); var next = aFunction(iterator.next); var array = IS_TO_ARRAY ? [] : undefined; if (!IS_TO_ARRAY) aFunction(fn); return new Promise(function (resolve, reject) { var closeIteration = function (method, argument) { try { var returnMethod = iterator['return']; if (returnMethod !== undefined) { return Promise.resolve(returnMethod.call(iterator)).then(function () { method(argument); }, function (error) { reject(error); }); } } catch (error2) { return reject(error2); } method(argument); }; var onError = function (error) { closeIteration(reject, error); }; var loop = function () { try { Promise.resolve(anObject(next.call(iterator))).then(function (step) { try { if (anObject(step).done) { resolve(IS_TO_ARRAY ? array : IS_SOME ? false : IS_EVERY || undefined); } else { var value = step.value; if (IS_TO_ARRAY) { push.call(array, value); loop(); } else { Promise.resolve(fn(value)).then(function (result) { if (IS_FOR_EACH) { loop(); } else if (IS_EVERY) { result ? loop() : closeIteration(resolve, false); } else { result ? closeIteration(resolve, IS_SOME || value) : loop(); } }, onError); } } } catch (error) { onError(error); } }, onError); } catch (error2) { onError(error2); } }; loop(); }); }; }; module.exports = { toArray: createMethod(0), forEach: createMethod(1), every: createMethod(2), some: createMethod(3), find: createMethod(4) }; apollo-server-demo/node_modules/core-js/internals/not-a-regexp.js 0000644 0001750 0000144 00000000302 03560116604 024630 0 ustar andreh users var isRegExp = require('../internals/is-regexp'); module.exports = function (it) { if (isRegExp(it)) { throw TypeError("The method doesn't accept regular expressions"); } return it; }; apollo-server-demo/node_modules/core-js/internals/object-prototype-accessors-forced.js 0000644 0001750 0000144 00000000724 03560116604 031066 0 ustar andreh users 'use strict'; var IS_PURE = require('../internals/is-pure'); var global = require('../internals/global'); var fails = require('../internals/fails'); // Forced replacement object prototype accessors methods module.exports = IS_PURE || !fails(function () { var key = Math.random(); // In FF throws only define methods // eslint-disable-next-line no-undef, no-useless-call __defineSetter__.call(null, key, function () { /* empty */ }); delete global[key]; }); apollo-server-demo/node_modules/core-js/internals/new-promise-capability.js 0000644 0001750 0000144 00000001012 03560116604 026705 0 ustar andreh users 'use strict'; var aFunction = require('../internals/a-function'); var PromiseCapability = function (C) { var resolve, reject; this.promise = new C(function ($$resolve, $$reject) { if (resolve !== undefined || reject !== undefined) throw TypeError('Bad Promise constructor'); resolve = $$resolve; reject = $$reject; }); this.resolve = aFunction(resolve); this.reject = aFunction(reject); }; // 25.4.1.5 NewPromiseCapability(C) module.exports.f = function (C) { return new PromiseCapability(C); }; apollo-server-demo/node_modules/core-js/internals/add-to-unscopables.js 0000644 0001750 0000144 00000001232 03560116604 026011 0 ustar andreh users var wellKnownSymbol = require('../internals/well-known-symbol'); var create = require('../internals/object-create'); var definePropertyModule = require('../internals/object-define-property'); var UNSCOPABLES = wellKnownSymbol('unscopables'); var ArrayPrototype = Array.prototype; // Array.prototype[@@unscopables] // https://tc39.es/ecma262/#sec-array.prototype-@@unscopables if (ArrayPrototype[UNSCOPABLES] == undefined) { definePropertyModule.f(ArrayPrototype, UNSCOPABLES, { configurable: true, value: create(null) }); } // add a key to Array.prototype[@@unscopables] module.exports = function (key) { ArrayPrototype[UNSCOPABLES][key] = true; }; apollo-server-demo/node_modules/core-js/internals/ie8-dom-define.js 0000644 0001750 0000144 00000000572 03560116604 025025 0 ustar andreh users var DESCRIPTORS = require('../internals/descriptors'); var fails = require('../internals/fails'); var createElement = require('../internals/document-create-element'); // Thank's IE8 for his funny defineProperty module.exports = !DESCRIPTORS && !fails(function () { return Object.defineProperty(createElement('div'), 'a', { get: function () { return 7; } }).a != 7; }); apollo-server-demo/node_modules/core-js/internals/collection-strong.js 0000644 0001750 0000144 00000015136 03560116604 026002 0 ustar andreh users 'use strict'; var defineProperty = require('../internals/object-define-property').f; var create = require('../internals/object-create'); var redefineAll = require('../internals/redefine-all'); var bind = require('../internals/function-bind-context'); var anInstance = require('../internals/an-instance'); var iterate = require('../internals/iterate'); var defineIterator = require('../internals/define-iterator'); var setSpecies = require('../internals/set-species'); var DESCRIPTORS = require('../internals/descriptors'); var fastKey = require('../internals/internal-metadata').fastKey; var InternalStateModule = require('../internals/internal-state'); var setInternalState = InternalStateModule.set; var internalStateGetterFor = InternalStateModule.getterFor; module.exports = { getConstructor: function (wrapper, CONSTRUCTOR_NAME, IS_MAP, ADDER) { var C = wrapper(function (that, iterable) { anInstance(that, C, CONSTRUCTOR_NAME); setInternalState(that, { type: CONSTRUCTOR_NAME, index: create(null), first: undefined, last: undefined, size: 0 }); if (!DESCRIPTORS) that.size = 0; if (iterable != undefined) iterate(iterable, that[ADDER], { that: that, AS_ENTRIES: IS_MAP }); }); var getInternalState = internalStateGetterFor(CONSTRUCTOR_NAME); var define = function (that, key, value) { var state = getInternalState(that); var entry = getEntry(that, key); var previous, index; // change existing entry if (entry) { entry.value = value; // create new entry } else { state.last = entry = { index: index = fastKey(key, true), key: key, value: value, previous: previous = state.last, next: undefined, removed: false }; if (!state.first) state.first = entry; if (previous) previous.next = entry; if (DESCRIPTORS) state.size++; else that.size++; // add to index if (index !== 'F') state.index[index] = entry; } return that; }; var getEntry = function (that, key) { var state = getInternalState(that); // fast case var index = fastKey(key); var entry; if (index !== 'F') return state.index[index]; // frozen object case for (entry = state.first; entry; entry = entry.next) { if (entry.key == key) return entry; } }; redefineAll(C.prototype, { // 23.1.3.1 Map.prototype.clear() // 23.2.3.2 Set.prototype.clear() clear: function clear() { var that = this; var state = getInternalState(that); var data = state.index; var entry = state.first; while (entry) { entry.removed = true; if (entry.previous) entry.previous = entry.previous.next = undefined; delete data[entry.index]; entry = entry.next; } state.first = state.last = undefined; if (DESCRIPTORS) state.size = 0; else that.size = 0; }, // 23.1.3.3 Map.prototype.delete(key) // 23.2.3.4 Set.prototype.delete(value) 'delete': function (key) { var that = this; var state = getInternalState(that); var entry = getEntry(that, key); if (entry) { var next = entry.next; var prev = entry.previous; delete state.index[entry.index]; entry.removed = true; if (prev) prev.next = next; if (next) next.previous = prev; if (state.first == entry) state.first = next; if (state.last == entry) state.last = prev; if (DESCRIPTORS) state.size--; else that.size--; } return !!entry; }, // 23.2.3.6 Set.prototype.forEach(callbackfn, thisArg = undefined) // 23.1.3.5 Map.prototype.forEach(callbackfn, thisArg = undefined) forEach: function forEach(callbackfn /* , that = undefined */) { var state = getInternalState(this); var boundFunction = bind(callbackfn, arguments.length > 1 ? arguments[1] : undefined, 3); var entry; while (entry = entry ? entry.next : state.first) { boundFunction(entry.value, entry.key, this); // revert to the last existing entry while (entry && entry.removed) entry = entry.previous; } }, // 23.1.3.7 Map.prototype.has(key) // 23.2.3.7 Set.prototype.has(value) has: function has(key) { return !!getEntry(this, key); } }); redefineAll(C.prototype, IS_MAP ? { // 23.1.3.6 Map.prototype.get(key) get: function get(key) { var entry = getEntry(this, key); return entry && entry.value; }, // 23.1.3.9 Map.prototype.set(key, value) set: function set(key, value) { return define(this, key === 0 ? 0 : key, value); } } : { // 23.2.3.1 Set.prototype.add(value) add: function add(value) { return define(this, value = value === 0 ? 0 : value, value); } }); if (DESCRIPTORS) defineProperty(C.prototype, 'size', { get: function () { return getInternalState(this).size; } }); return C; }, setStrong: function (C, CONSTRUCTOR_NAME, IS_MAP) { var ITERATOR_NAME = CONSTRUCTOR_NAME + ' Iterator'; var getInternalCollectionState = internalStateGetterFor(CONSTRUCTOR_NAME); var getInternalIteratorState = internalStateGetterFor(ITERATOR_NAME); // add .keys, .values, .entries, [@@iterator] // 23.1.3.4, 23.1.3.8, 23.1.3.11, 23.1.3.12, 23.2.3.5, 23.2.3.8, 23.2.3.10, 23.2.3.11 defineIterator(C, CONSTRUCTOR_NAME, function (iterated, kind) { setInternalState(this, { type: ITERATOR_NAME, target: iterated, state: getInternalCollectionState(iterated), kind: kind, last: undefined }); }, function () { var state = getInternalIteratorState(this); var kind = state.kind; var entry = state.last; // revert to the last existing entry while (entry && entry.removed) entry = entry.previous; // get next entry if (!state.target || !(state.last = entry = entry ? entry.next : state.state.first)) { // or finish the iteration state.target = undefined; return { value: undefined, done: true }; } // return step by kind if (kind == 'keys') return { value: entry.key, done: false }; if (kind == 'values') return { value: entry.value, done: false }; return { value: [entry.key, entry.value], done: false }; }, IS_MAP ? 'entries' : 'values', !IS_MAP, true); // add [@@species], 23.1.2.2, 23.2.2.2 setSpecies(CONSTRUCTOR_NAME); } }; apollo-server-demo/node_modules/core-js/internals/same-value-zero.js 0000644 0001750 0000144 00000000316 03560116604 025343 0 ustar andreh users // `SameValueZero` abstract operation // https://tc39.es/ecma262/#sec-samevaluezero module.exports = function (x, y) { // eslint-disable-next-line no-self-compare return x === y || x != x && y != y; }; apollo-server-demo/node_modules/core-js/internals/entry-virtual.js 0000644 0001750 0000144 00000000203 03560116604 025147 0 ustar andreh users var global = require('../internals/global'); module.exports = function (CONSTRUCTOR) { return global[CONSTRUCTOR].prototype; }; apollo-server-demo/node_modules/core-js/internals/set-to-string-tag.js 0000644 0001750 0000144 00000000651 03560116604 025621 0 ustar andreh users var defineProperty = require('../internals/object-define-property').f; var has = require('../internals/has'); var wellKnownSymbol = require('../internals/well-known-symbol'); var TO_STRING_TAG = wellKnownSymbol('toStringTag'); module.exports = function (it, TAG, STATIC) { if (it && !has(it = STATIC ? it : it.prototype, TO_STRING_TAG)) { defineProperty(it, TO_STRING_TAG, { configurable: true, value: TAG }); } }; apollo-server-demo/node_modules/core-js/internals/get-map-iterator.js 0000644 0001750 0000144 00000000364 03560116604 025513 0 ustar andreh users var IS_PURE = require('../internals/is-pure'); var getIterator = require('../internals/get-iterator'); module.exports = IS_PURE ? getIterator : function (it) { // eslint-disable-next-line no-undef return Map.prototype.entries.call(it); }; apollo-server-demo/node_modules/core-js/internals/html.js 0000644 0001750 0000144 00000000164 03560116604 023274 0 ustar andreh users var getBuiltIn = require('../internals/get-built-in'); module.exports = getBuiltIn('document', 'documentElement'); apollo-server-demo/node_modules/core-js/internals/check-correctness-of-iteration.js 0000644 0001750 0000144 00000001650 03560116604 030334 0 ustar andreh users var wellKnownSymbol = require('../internals/well-known-symbol'); var ITERATOR = wellKnownSymbol('iterator'); var SAFE_CLOSING = false; try { var called = 0; var iteratorWithReturn = { next: function () { return { done: !!called++ }; }, 'return': function () { SAFE_CLOSING = true; } }; iteratorWithReturn[ITERATOR] = function () { return this; }; // eslint-disable-next-line no-throw-literal Array.from(iteratorWithReturn, function () { throw 2; }); } catch (error) { /* empty */ } module.exports = function (exec, SKIP_CLOSING) { if (!SKIP_CLOSING && !SAFE_CLOSING) return false; var ITERATION_SUPPORT = false; try { var object = {}; object[ITERATOR] = function () { return { next: function () { return { done: ITERATION_SUPPORT = true }; } }; }; exec(object); } catch (error) { /* empty */ } return ITERATION_SUPPORT; }; apollo-server-demo/node_modules/core-js/internals/math-log1p.js 0000644 0001750 0000144 00000000340 03560116604 024275 0 ustar andreh users var log = Math.log; // `Math.log1p` method implementation // https://tc39.es/ecma262/#sec-math.log1p module.exports = Math.log1p || function log1p(x) { return (x = +x) > -1e-8 && x < 1e-8 ? x - x * x / 2 : log(1 + x); }; apollo-server-demo/node_modules/core-js/internals/object-set-prototype-of.js 0000644 0001750 0000144 00000001524 03560116604 027035 0 ustar andreh users var anObject = require('../internals/an-object'); var aPossiblePrototype = require('../internals/a-possible-prototype'); // `Object.setPrototypeOf` method // https://tc39.es/ecma262/#sec-object.setprototypeof // Works with __proto__ only. Old v8 can't work with null proto objects. /* eslint-disable no-proto */ module.exports = Object.setPrototypeOf || ('__proto__' in {} ? function () { var CORRECT_SETTER = false; var test = {}; var setter; try { setter = Object.getOwnPropertyDescriptor(Object.prototype, '__proto__').set; setter.call(test, []); CORRECT_SETTER = test instanceof Array; } catch (error) { /* empty */ } return function setPrototypeOf(O, proto) { anObject(O); aPossiblePrototype(proto); if (CORRECT_SETTER) setter.call(O, proto); else O.__proto__ = proto; return O; }; }() : undefined); apollo-server-demo/node_modules/core-js/internals/correct-prototype-getter.js 0000644 0001750 0000144 00000000320 03560116604 027316 0 ustar andreh users var fails = require('../internals/fails'); module.exports = !fails(function () { function F() { /* empty */ } F.prototype.constructor = null; return Object.getPrototypeOf(new F()) !== F.prototype; }); apollo-server-demo/node_modules/core-js/internals/iterate.js 0000644 0001750 0000144 00000004011 03560116604 023760 0 ustar andreh users var anObject = require('../internals/an-object'); var isArrayIteratorMethod = require('../internals/is-array-iterator-method'); var toLength = require('../internals/to-length'); var bind = require('../internals/function-bind-context'); var getIteratorMethod = require('../internals/get-iterator-method'); var iteratorClose = require('../internals/iterator-close'); var Result = function (stopped, result) { this.stopped = stopped; this.result = result; }; module.exports = function (iterable, unboundFunction, options) { var that = options && options.that; var AS_ENTRIES = !!(options && options.AS_ENTRIES); var IS_ITERATOR = !!(options && options.IS_ITERATOR); var INTERRUPTED = !!(options && options.INTERRUPTED); var fn = bind(unboundFunction, that, 1 + AS_ENTRIES + INTERRUPTED); var iterator, iterFn, index, length, result, next, step; var stop = function (condition) { if (iterator) iteratorClose(iterator); return new Result(true, condition); }; var callFn = function (value) { if (AS_ENTRIES) { anObject(value); return INTERRUPTED ? fn(value[0], value[1], stop) : fn(value[0], value[1]); } return INTERRUPTED ? fn(value, stop) : fn(value); }; if (IS_ITERATOR) { iterator = iterable; } else { iterFn = getIteratorMethod(iterable); if (typeof iterFn != 'function') throw TypeError('Target is not iterable'); // optimisation for array iterators if (isArrayIteratorMethod(iterFn)) { for (index = 0, length = toLength(iterable.length); length > index; index++) { result = callFn(iterable[index]); if (result && result instanceof Result) return result; } return new Result(false); } iterator = iterFn.call(iterable); } next = iterator.next; while (!(step = next.call(iterator)).done) { try { result = callFn(step.value); } catch (error) { iteratorClose(iterator); throw error; } if (typeof result == 'object' && result && result instanceof Result) return result; } return new Result(false); }; apollo-server-demo/node_modules/core-js/internals/object-property-is-enumerable.js 0000644 0001750 0000144 00000001107 03560116604 030204 0 ustar andreh users 'use strict'; var nativePropertyIsEnumerable = {}.propertyIsEnumerable; var getOwnPropertyDescriptor = Object.getOwnPropertyDescriptor; // Nashorn ~ JDK8 bug var NASHORN_BUG = getOwnPropertyDescriptor && !nativePropertyIsEnumerable.call({ 1: 2 }, 1); // `Object.prototype.propertyIsEnumerable` method implementation // https://tc39.es/ecma262/#sec-object.prototype.propertyisenumerable exports.f = NASHORN_BUG ? function propertyIsEnumerable(V) { var descriptor = getOwnPropertyDescriptor(this, V); return !!descriptor && descriptor.enumerable; } : nativePropertyIsEnumerable; apollo-server-demo/node_modules/core-js/internals/hidden-keys.js 0000644 0001750 0000144 00000000025 03560116604 024530 0 ustar andreh users module.exports = {}; apollo-server-demo/node_modules/core-js/internals/map-upsert.js 0000644 0001750 0000144 00000001321 03560116604 024421 0 ustar andreh users 'use strict'; var anObject = require('../internals/an-object'); // `Map.prototype.upsert` method // https://github.com/thumbsupep/proposal-upsert module.exports = function upsert(key, updateFn /* , insertFn */) { var map = anObject(this); var insertFn = arguments.length > 2 ? arguments[2] : undefined; var value; if (typeof updateFn != 'function' && typeof insertFn != 'function') { throw TypeError('At least one callback required'); } if (map.has(key)) { value = map.get(key); if (typeof updateFn == 'function') { value = updateFn(value); map.set(key, value); } } else if (typeof insertFn == 'function') { value = insertFn(); map.set(key, value); } return value; }; apollo-server-demo/node_modules/core-js/internals/correct-is-regexp-logic.js 0000644 0001750 0000144 00000000556 03560116604 026772 0 ustar andreh users var wellKnownSymbol = require('../internals/well-known-symbol'); var MATCH = wellKnownSymbol('match'); module.exports = function (METHOD_NAME) { var regexp = /./; try { '/./'[METHOD_NAME](regexp); } catch (error1) { try { regexp[MATCH] = false; return '/./'[METHOD_NAME](regexp); } catch (error2) { /* empty */ } } return false; }; apollo-server-demo/node_modules/core-js/internals/get-substitution.js 0000644 0001750 0000144 00000002504 03560116604 025661 0 ustar andreh users var toObject = require('../internals/to-object'); var floor = Math.floor; var replace = ''.replace; var SUBSTITUTION_SYMBOLS = /\$([$&'`]|\d\d?|<[^>]*>)/g; var SUBSTITUTION_SYMBOLS_NO_NAMED = /\$([$&'`]|\d\d?)/g; // https://tc39.es/ecma262/#sec-getsubstitution module.exports = function (matched, str, position, captures, namedCaptures, replacement) { var tailPos = position + matched.length; var m = captures.length; var symbols = SUBSTITUTION_SYMBOLS_NO_NAMED; if (namedCaptures !== undefined) { namedCaptures = toObject(namedCaptures); symbols = SUBSTITUTION_SYMBOLS; } return replace.call(replacement, symbols, function (match, ch) { var capture; switch (ch.charAt(0)) { case '$': return '$'; case '&': return matched; case '`': return str.slice(0, position); case "'": return str.slice(tailPos); case '<': capture = namedCaptures[ch.slice(1, -1)]; break; default: // \d\d? var n = +ch; if (n === 0) return match; if (n > m) { var f = floor(n / 10); if (f === 0) return match; if (f <= m) return captures[f - 1] === undefined ? ch.charAt(1) : captures[f - 1] + ch.charAt(1); return match; } capture = captures[n - 1]; } return capture === undefined ? '' : capture; }); }; apollo-server-demo/node_modules/core-js/internals/define-iterator.js 0000644 0001750 0000144 00000007712 03560116604 025417 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var createIteratorConstructor = require('../internals/create-iterator-constructor'); var getPrototypeOf = require('../internals/object-get-prototype-of'); var setPrototypeOf = require('../internals/object-set-prototype-of'); var setToStringTag = require('../internals/set-to-string-tag'); var createNonEnumerableProperty = require('../internals/create-non-enumerable-property'); var redefine = require('../internals/redefine'); var wellKnownSymbol = require('../internals/well-known-symbol'); var IS_PURE = require('../internals/is-pure'); var Iterators = require('../internals/iterators'); var IteratorsCore = require('../internals/iterators-core'); var IteratorPrototype = IteratorsCore.IteratorPrototype; var BUGGY_SAFARI_ITERATORS = IteratorsCore.BUGGY_SAFARI_ITERATORS; var ITERATOR = wellKnownSymbol('iterator'); var KEYS = 'keys'; var VALUES = 'values'; var ENTRIES = 'entries'; var returnThis = function () { return this; }; module.exports = function (Iterable, NAME, IteratorConstructor, next, DEFAULT, IS_SET, FORCED) { createIteratorConstructor(IteratorConstructor, NAME, next); var getIterationMethod = function (KIND) { if (KIND === DEFAULT && defaultIterator) return defaultIterator; if (!BUGGY_SAFARI_ITERATORS && KIND in IterablePrototype) return IterablePrototype[KIND]; switch (KIND) { case KEYS: return function keys() { return new IteratorConstructor(this, KIND); }; case VALUES: return function values() { return new IteratorConstructor(this, KIND); }; case ENTRIES: return function entries() { return new IteratorConstructor(this, KIND); }; } return function () { return new IteratorConstructor(this); }; }; var TO_STRING_TAG = NAME + ' Iterator'; var INCORRECT_VALUES_NAME = false; var IterablePrototype = Iterable.prototype; var nativeIterator = IterablePrototype[ITERATOR] || IterablePrototype['@@iterator'] || DEFAULT && IterablePrototype[DEFAULT]; var defaultIterator = !BUGGY_SAFARI_ITERATORS && nativeIterator || getIterationMethod(DEFAULT); var anyNativeIterator = NAME == 'Array' ? IterablePrototype.entries || nativeIterator : nativeIterator; var CurrentIteratorPrototype, methods, KEY; // fix native if (anyNativeIterator) { CurrentIteratorPrototype = getPrototypeOf(anyNativeIterator.call(new Iterable())); if (IteratorPrototype !== Object.prototype && CurrentIteratorPrototype.next) { if (!IS_PURE && getPrototypeOf(CurrentIteratorPrototype) !== IteratorPrototype) { if (setPrototypeOf) { setPrototypeOf(CurrentIteratorPrototype, IteratorPrototype); } else if (typeof CurrentIteratorPrototype[ITERATOR] != 'function') { createNonEnumerableProperty(CurrentIteratorPrototype, ITERATOR, returnThis); } } // Set @@toStringTag to native iterators setToStringTag(CurrentIteratorPrototype, TO_STRING_TAG, true, true); if (IS_PURE) Iterators[TO_STRING_TAG] = returnThis; } } // fix Array#{values, @@iterator}.name in V8 / FF if (DEFAULT == VALUES && nativeIterator && nativeIterator.name !== VALUES) { INCORRECT_VALUES_NAME = true; defaultIterator = function values() { return nativeIterator.call(this); }; } // define iterator if ((!IS_PURE || FORCED) && IterablePrototype[ITERATOR] !== defaultIterator) { createNonEnumerableProperty(IterablePrototype, ITERATOR, defaultIterator); } Iterators[NAME] = defaultIterator; // export additional methods if (DEFAULT) { methods = { values: getIterationMethod(VALUES), keys: IS_SET ? defaultIterator : getIterationMethod(KEYS), entries: getIterationMethod(ENTRIES) }; if (FORCED) for (KEY in methods) { if (BUGGY_SAFARI_ITERATORS || INCORRECT_VALUES_NAME || !(KEY in IterablePrototype)) { redefine(IterablePrototype, KEY, methods[KEY]); } } else $({ target: NAME, proto: true, forced: BUGGY_SAFARI_ITERATORS || INCORRECT_VALUES_NAME }, methods); } return methods; }; apollo-server-demo/node_modules/core-js/internals/collection-from.js 0000644 0001750 0000144 00000001524 03560116604 025425 0 ustar andreh users 'use strict'; // https://tc39.github.io/proposal-setmap-offrom/ var aFunction = require('../internals/a-function'); var bind = require('../internals/function-bind-context'); var iterate = require('../internals/iterate'); module.exports = function from(source /* , mapFn, thisArg */) { var length = arguments.length; var mapFn = length > 1 ? arguments[1] : undefined; var mapping, array, n, boundFunction; aFunction(this); mapping = mapFn !== undefined; if (mapping) aFunction(mapFn); if (source == undefined) return new this(); array = []; if (mapping) { n = 0; boundFunction = bind(mapFn, length > 2 ? arguments[2] : undefined, 2); iterate(source, function (nextItem) { array.push(boundFunction(nextItem, n++)); }); } else { iterate(source, array.push, { that: array }); } return new this(array); }; apollo-server-demo/node_modules/core-js/internals/to-length.js 0000644 0001750 0000144 00000000451 03560116604 024230 0 ustar andreh users var toInteger = require('../internals/to-integer'); var min = Math.min; // `ToLength` abstract operation // https://tc39.es/ecma262/#sec-tolength module.exports = function (argument) { return argument > 0 ? min(toInteger(argument), 0x1FFFFFFFFFFFFF) : 0; // 2 ** 53 - 1 == 9007199254740991 }; apollo-server-demo/node_modules/core-js/internals/array-buffer-native.js 0000644 0001750 0000144 00000000130 03560116604 026172 0 ustar andreh users module.exports = typeof ArrayBuffer !== 'undefined' && typeof DataView !== 'undefined'; apollo-server-demo/node_modules/core-js/internals/ieee754.js 0000644 0001750 0000144 00000005420 03560116604 023477 0 ustar andreh users // IEEE754 conversions based on https://github.com/feross/ieee754 // eslint-disable-next-line no-shadow-restricted-names var Infinity = 1 / 0; var abs = Math.abs; var pow = Math.pow; var floor = Math.floor; var log = Math.log; var LN2 = Math.LN2; var pack = function (number, mantissaLength, bytes) { var buffer = new Array(bytes); var exponentLength = bytes * 8 - mantissaLength - 1; var eMax = (1 << exponentLength) - 1; var eBias = eMax >> 1; var rt = mantissaLength === 23 ? pow(2, -24) - pow(2, -77) : 0; var sign = number < 0 || number === 0 && 1 / number < 0 ? 1 : 0; var index = 0; var exponent, mantissa, c; number = abs(number); // eslint-disable-next-line no-self-compare if (number != number || number === Infinity) { // eslint-disable-next-line no-self-compare mantissa = number != number ? 1 : 0; exponent = eMax; } else { exponent = floor(log(number) / LN2); if (number * (c = pow(2, -exponent)) < 1) { exponent--; c *= 2; } if (exponent + eBias >= 1) { number += rt / c; } else { number += rt * pow(2, 1 - eBias); } if (number * c >= 2) { exponent++; c /= 2; } if (exponent + eBias >= eMax) { mantissa = 0; exponent = eMax; } else if (exponent + eBias >= 1) { mantissa = (number * c - 1) * pow(2, mantissaLength); exponent = exponent + eBias; } else { mantissa = number * pow(2, eBias - 1) * pow(2, mantissaLength); exponent = 0; } } for (; mantissaLength >= 8; buffer[index++] = mantissa & 255, mantissa /= 256, mantissaLength -= 8); exponent = exponent << mantissaLength | mantissa; exponentLength += mantissaLength; for (; exponentLength > 0; buffer[index++] = exponent & 255, exponent /= 256, exponentLength -= 8); buffer[--index] |= sign * 128; return buffer; }; var unpack = function (buffer, mantissaLength) { var bytes = buffer.length; var exponentLength = bytes * 8 - mantissaLength - 1; var eMax = (1 << exponentLength) - 1; var eBias = eMax >> 1; var nBits = exponentLength - 7; var index = bytes - 1; var sign = buffer[index--]; var exponent = sign & 127; var mantissa; sign >>= 7; for (; nBits > 0; exponent = exponent * 256 + buffer[index], index--, nBits -= 8); mantissa = exponent & (1 << -nBits) - 1; exponent >>= -nBits; nBits += mantissaLength; for (; nBits > 0; mantissa = mantissa * 256 + buffer[index], index--, nBits -= 8); if (exponent === 0) { exponent = 1 - eBias; } else if (exponent === eMax) { return mantissa ? NaN : sign ? -Infinity : Infinity; } else { mantissa = mantissa + pow(2, mantissaLength); exponent = exponent - eBias; } return (sign ? -1 : 1) * mantissa * pow(2, exponent - mantissaLength); }; module.exports = { pack: pack, unpack: unpack }; apollo-server-demo/node_modules/core-js/internals/is-forced.js 0000644 0001750 0000144 00000001075 03560116604 024205 0 ustar andreh users var fails = require('../internals/fails'); var replacement = /#|\.prototype\./; var isForced = function (feature, detection) { var value = data[normalize(feature)]; return value == POLYFILL ? true : value == NATIVE ? false : typeof detection == 'function' ? fails(detection) : !!detection; }; var normalize = isForced.normalize = function (string) { return String(string).replace(replacement, '.').toLowerCase(); }; var data = isForced.data = {}; var NATIVE = isForced.NATIVE = 'N'; var POLYFILL = isForced.POLYFILL = 'P'; module.exports = isForced; apollo-server-demo/node_modules/core-js/internals/redefine.js 0000644 0001750 0000144 00000003065 03560116604 024114 0 ustar andreh users var global = require('../internals/global'); var createNonEnumerableProperty = require('../internals/create-non-enumerable-property'); var has = require('../internals/has'); var setGlobal = require('../internals/set-global'); var inspectSource = require('../internals/inspect-source'); var InternalStateModule = require('../internals/internal-state'); var getInternalState = InternalStateModule.get; var enforceInternalState = InternalStateModule.enforce; var TEMPLATE = String(String).split('String'); (module.exports = function (O, key, value, options) { var unsafe = options ? !!options.unsafe : false; var simple = options ? !!options.enumerable : false; var noTargetGet = options ? !!options.noTargetGet : false; var state; if (typeof value == 'function') { if (typeof key == 'string' && !has(value, 'name')) { createNonEnumerableProperty(value, 'name', key); } state = enforceInternalState(value); if (!state.source) { state.source = TEMPLATE.join(typeof key == 'string' ? key : ''); } } if (O === global) { if (simple) O[key] = value; else setGlobal(key, value); return; } else if (!unsafe) { delete O[key]; } else if (!noTargetGet && O[key]) { simple = true; } if (simple) O[key] = value; else createNonEnumerableProperty(O, key, value); // add fake Function#toString for correct work wrapped methods / constructors with methods like LoDash isNative })(Function.prototype, 'toString', function toString() { return typeof this == 'function' && getInternalState(this).source || inspectSource(this); }); apollo-server-demo/node_modules/core-js/internals/map-emplace.js 0000644 0001750 0000144 00000000615 03560116604 024512 0 ustar andreh users 'use strict'; var anObject = require('../internals/an-object'); // `Map.prototype.emplace` method // https://github.com/thumbsupep/proposal-upsert module.exports = function emplace(key, handler) { var map = anObject(this); var value = (map.has(key) && 'update' in handler) ? handler.update(map.get(key), key, map) : handler.insert(key, map); map.set(key, value); return value; }; apollo-server-demo/node_modules/core-js/internals/array-last-index-of.js 0000644 0001750 0000144 00000002641 03560116604 026120 0 ustar andreh users 'use strict'; var toIndexedObject = require('../internals/to-indexed-object'); var toInteger = require('../internals/to-integer'); var toLength = require('../internals/to-length'); var arrayMethodIsStrict = require('../internals/array-method-is-strict'); var arrayMethodUsesToLength = require('../internals/array-method-uses-to-length'); var min = Math.min; var nativeLastIndexOf = [].lastIndexOf; var NEGATIVE_ZERO = !!nativeLastIndexOf && 1 / [1].lastIndexOf(1, -0) < 0; var STRICT_METHOD = arrayMethodIsStrict('lastIndexOf'); // For preventing possible almost infinite loop in non-standard implementations, test the forward version of the method var USES_TO_LENGTH = arrayMethodUsesToLength('indexOf', { ACCESSORS: true, 1: 0 }); var FORCED = NEGATIVE_ZERO || !STRICT_METHOD || !USES_TO_LENGTH; // `Array.prototype.lastIndexOf` method implementation // https://tc39.es/ecma262/#sec-array.prototype.lastindexof module.exports = FORCED ? function lastIndexOf(searchElement /* , fromIndex = @[*-1] */) { // convert -0 to +0 if (NEGATIVE_ZERO) return nativeLastIndexOf.apply(this, arguments) || 0; var O = toIndexedObject(this); var length = toLength(O.length); var index = length - 1; if (arguments.length > 1) index = min(index, toInteger(arguments[1])); if (index < 0) index = length + index; for (;index >= 0; index--) if (index in O && O[index] === searchElement) return index || 0; return -1; } : nativeLastIndexOf; apollo-server-demo/node_modules/core-js/internals/enum-bug-keys.js 0000644 0001750 0000144 00000000262 03560116604 025017 0 ustar andreh users // IE8- don't enum bug keys module.exports = [ 'constructor', 'hasOwnProperty', 'isPrototypeOf', 'propertyIsEnumerable', 'toLocaleString', 'toString', 'valueOf' ]; apollo-server-demo/node_modules/core-js/internals/string-punycode-to-ascii.js 0000644 0001750 0000144 00000012153 03560116604 027171 0 ustar andreh users 'use strict'; // based on https://github.com/bestiejs/punycode.js/blob/master/punycode.js var maxInt = 2147483647; // aka. 0x7FFFFFFF or 2^31-1 var base = 36; var tMin = 1; var tMax = 26; var skew = 38; var damp = 700; var initialBias = 72; var initialN = 128; // 0x80 var delimiter = '-'; // '\x2D' var regexNonASCII = /[^\0-\u007E]/; // non-ASCII chars var regexSeparators = /[.\u3002\uFF0E\uFF61]/g; // RFC 3490 separators var OVERFLOW_ERROR = 'Overflow: input needs wider integers to process'; var baseMinusTMin = base - tMin; var floor = Math.floor; var stringFromCharCode = String.fromCharCode; /** * Creates an array containing the numeric code points of each Unicode * character in the string. While JavaScript uses UCS-2 internally, * this function will convert a pair of surrogate halves (each of which * UCS-2 exposes as separate characters) into a single code point, * matching UTF-16. */ var ucs2decode = function (string) { var output = []; var counter = 0; var length = string.length; while (counter < length) { var value = string.charCodeAt(counter++); if (value >= 0xD800 && value <= 0xDBFF && counter < length) { // It's a high surrogate, and there is a next character. var extra = string.charCodeAt(counter++); if ((extra & 0xFC00) == 0xDC00) { // Low surrogate. output.push(((value & 0x3FF) << 10) + (extra & 0x3FF) + 0x10000); } else { // It's an unmatched surrogate; only append this code unit, in case the // next code unit is the high surrogate of a surrogate pair. output.push(value); counter--; } } else { output.push(value); } } return output; }; /** * Converts a digit/integer into a basic code point. */ var digitToBasic = function (digit) { // 0..25 map to ASCII a..z or A..Z // 26..35 map to ASCII 0..9 return digit + 22 + 75 * (digit < 26); }; /** * Bias adaptation function as per section 3.4 of RFC 3492. * https://tools.ietf.org/html/rfc3492#section-3.4 */ var adapt = function (delta, numPoints, firstTime) { var k = 0; delta = firstTime ? floor(delta / damp) : delta >> 1; delta += floor(delta / numPoints); for (; delta > baseMinusTMin * tMax >> 1; k += base) { delta = floor(delta / baseMinusTMin); } return floor(k + (baseMinusTMin + 1) * delta / (delta + skew)); }; /** * Converts a string of Unicode symbols (e.g. a domain name label) to a * Punycode string of ASCII-only symbols. */ // eslint-disable-next-line max-statements var encode = function (input) { var output = []; // Convert the input in UCS-2 to an array of Unicode code points. input = ucs2decode(input); // Cache the length. var inputLength = input.length; // Initialize the state. var n = initialN; var delta = 0; var bias = initialBias; var i, currentValue; // Handle the basic code points. for (i = 0; i < input.length; i++) { currentValue = input[i]; if (currentValue < 0x80) { output.push(stringFromCharCode(currentValue)); } } var basicLength = output.length; // number of basic code points. var handledCPCount = basicLength; // number of code points that have been handled; // Finish the basic string with a delimiter unless it's empty. if (basicLength) { output.push(delimiter); } // Main encoding loop: while (handledCPCount < inputLength) { // All non-basic code points < n have been handled already. Find the next larger one: var m = maxInt; for (i = 0; i < input.length; i++) { currentValue = input[i]; if (currentValue >= n && currentValue < m) { m = currentValue; } } // Increase `delta` enough to advance the decoder's <n,i> state to <m,0>, but guard against overflow. var handledCPCountPlusOne = handledCPCount + 1; if (m - n > floor((maxInt - delta) / handledCPCountPlusOne)) { throw RangeError(OVERFLOW_ERROR); } delta += (m - n) * handledCPCountPlusOne; n = m; for (i = 0; i < input.length; i++) { currentValue = input[i]; if (currentValue < n && ++delta > maxInt) { throw RangeError(OVERFLOW_ERROR); } if (currentValue == n) { // Represent delta as a generalized variable-length integer. var q = delta; for (var k = base; /* no condition */; k += base) { var t = k <= bias ? tMin : (k >= bias + tMax ? tMax : k - bias); if (q < t) break; var qMinusT = q - t; var baseMinusT = base - t; output.push(stringFromCharCode(digitToBasic(t + qMinusT % baseMinusT))); q = floor(qMinusT / baseMinusT); } output.push(stringFromCharCode(digitToBasic(q))); bias = adapt(delta, handledCPCountPlusOne, handledCPCount == basicLength); delta = 0; ++handledCPCount; } } ++delta; ++n; } return output.join(''); }; module.exports = function (input) { var encoded = []; var labels = input.toLowerCase().replace(regexSeparators, '\u002E').split('.'); var i, label; for (i = 0; i < labels.length; i++) { label = labels[i]; encoded.push(regexNonASCII.test(label) ? 'xn--' + encode(label) : label); } return encoded.join('.'); }; apollo-server-demo/node_modules/core-js/internals/to-positive-integer.js 0000644 0001750 0000144 00000000321 03560116604 026240 0 ustar andreh users var toInteger = require('../internals/to-integer'); module.exports = function (it) { var result = toInteger(it); if (result < 0) throw RangeError("The argument can't be less than 0"); return result; }; apollo-server-demo/node_modules/core-js/internals/array-includes.js 0000644 0001750 0000144 00000002365 03560116604 025257 0 ustar andreh users var toIndexedObject = require('../internals/to-indexed-object'); var toLength = require('../internals/to-length'); var toAbsoluteIndex = require('../internals/to-absolute-index'); // `Array.prototype.{ indexOf, includes }` methods implementation var createMethod = function (IS_INCLUDES) { return function ($this, el, fromIndex) { var O = toIndexedObject($this); var length = toLength(O.length); var index = toAbsoluteIndex(fromIndex, length); var value; // Array#includes uses SameValueZero equality algorithm // eslint-disable-next-line no-self-compare if (IS_INCLUDES && el != el) while (length > index) { value = O[index++]; // eslint-disable-next-line no-self-compare if (value != value) return true; // Array#indexOf ignores holes, Array#includes - not } else for (;length > index; index++) { if ((IS_INCLUDES || index in O) && O[index] === el) return IS_INCLUDES || index || 0; } return !IS_INCLUDES && -1; }; }; module.exports = { // `Array.prototype.includes` method // https://tc39.es/ecma262/#sec-array.prototype.includes includes: createMethod(true), // `Array.prototype.indexOf` method // https://tc39.es/ecma262/#sec-array.prototype.indexof indexOf: createMethod(false) }; apollo-server-demo/node_modules/core-js/internals/set-species.js 0000644 0001750 0000144 00000001176 03560116604 024560 0 ustar andreh users 'use strict'; var getBuiltIn = require('../internals/get-built-in'); var definePropertyModule = require('../internals/object-define-property'); var wellKnownSymbol = require('../internals/well-known-symbol'); var DESCRIPTORS = require('../internals/descriptors'); var SPECIES = wellKnownSymbol('species'); module.exports = function (CONSTRUCTOR_NAME) { var Constructor = getBuiltIn(CONSTRUCTOR_NAME); var defineProperty = definePropertyModule.f; if (DESCRIPTORS && Constructor && !Constructor[SPECIES]) { defineProperty(Constructor, SPECIES, { configurable: true, get: function () { return this; } }); } }; apollo-server-demo/node_modules/core-js/internals/function-bind.js 0000644 0001750 0000144 00000002044 03560116604 025066 0 ustar andreh users 'use strict'; var aFunction = require('../internals/a-function'); var isObject = require('../internals/is-object'); var slice = [].slice; var factories = {}; var construct = function (C, argsLength, args) { if (!(argsLength in factories)) { for (var list = [], i = 0; i < argsLength; i++) list[i] = 'a[' + i + ']'; // eslint-disable-next-line no-new-func factories[argsLength] = Function('C,a', 'return new C(' + list.join(',') + ')'); } return factories[argsLength](C, args); }; // `Function.prototype.bind` method implementation // https://tc39.es/ecma262/#sec-function.prototype.bind module.exports = Function.bind || function bind(that /* , ...args */) { var fn = aFunction(this); var partArgs = slice.call(arguments, 1); var boundFunction = function bound(/* args... */) { var args = partArgs.concat(slice.call(arguments)); return this instanceof boundFunction ? construct(fn, args.length, args) : fn.apply(that, args); }; if (isObject(fn.prototype)) boundFunction.prototype = fn.prototype; return boundFunction; }; apollo-server-demo/node_modules/core-js/internals/reflect-metadata.js 0000644 0001750 0000144 00000003534 03560116604 025536 0 ustar andreh users // TODO: in core-js@4, move /modules/ dependencies to public entries for better optimization by tools like `preset-env` var Map = require('../modules/es.map'); var WeakMap = require('../modules/es.weak-map'); var shared = require('../internals/shared'); var metadata = shared('metadata'); var store = metadata.store || (metadata.store = new WeakMap()); var getOrCreateMetadataMap = function (target, targetKey, create) { var targetMetadata = store.get(target); if (!targetMetadata) { if (!create) return; store.set(target, targetMetadata = new Map()); } var keyMetadata = targetMetadata.get(targetKey); if (!keyMetadata) { if (!create) return; targetMetadata.set(targetKey, keyMetadata = new Map()); } return keyMetadata; }; var ordinaryHasOwnMetadata = function (MetadataKey, O, P) { var metadataMap = getOrCreateMetadataMap(O, P, false); return metadataMap === undefined ? false : metadataMap.has(MetadataKey); }; var ordinaryGetOwnMetadata = function (MetadataKey, O, P) { var metadataMap = getOrCreateMetadataMap(O, P, false); return metadataMap === undefined ? undefined : metadataMap.get(MetadataKey); }; var ordinaryDefineOwnMetadata = function (MetadataKey, MetadataValue, O, P) { getOrCreateMetadataMap(O, P, true).set(MetadataKey, MetadataValue); }; var ordinaryOwnMetadataKeys = function (target, targetKey) { var metadataMap = getOrCreateMetadataMap(target, targetKey, false); var keys = []; if (metadataMap) metadataMap.forEach(function (_, key) { keys.push(key); }); return keys; }; var toMetadataKey = function (it) { return it === undefined || typeof it == 'symbol' ? it : String(it); }; module.exports = { store: store, getMap: getOrCreateMetadataMap, has: ordinaryHasOwnMetadata, get: ordinaryGetOwnMetadata, set: ordinaryDefineOwnMetadata, keys: ordinaryOwnMetadataKeys, toKey: toMetadataKey }; apollo-server-demo/node_modules/core-js/internals/an-instance.js 0000644 0001750 0000144 00000000271 03560116604 024527 0 ustar andreh users module.exports = function (it, Constructor, name) { if (!(it instanceof Constructor)) { throw TypeError('Incorrect ' + (name ? name + ' ' : '') + 'invocation'); } return it; }; apollo-server-demo/node_modules/core-js/internals/string-html-forced.js 0000644 0001750 0000144 00000000502 03560116604 026034 0 ustar andreh users var fails = require('../internals/fails'); // check the existence of a method, lowercase // of a tag and escaping quotes in arguments module.exports = function (METHOD_NAME) { return fails(function () { var test = ''[METHOD_NAME]('"'); return test !== test.toLowerCase() || test.split('"').length > 3; }); }; apollo-server-demo/node_modules/core-js/internals/object-assign.js 0000644 0001750 0000144 00000004023 03560116604 025056 0 ustar andreh users 'use strict'; var DESCRIPTORS = require('../internals/descriptors'); var fails = require('../internals/fails'); var objectKeys = require('../internals/object-keys'); var getOwnPropertySymbolsModule = require('../internals/object-get-own-property-symbols'); var propertyIsEnumerableModule = require('../internals/object-property-is-enumerable'); var toObject = require('../internals/to-object'); var IndexedObject = require('../internals/indexed-object'); var nativeAssign = Object.assign; var defineProperty = Object.defineProperty; // `Object.assign` method // https://tc39.es/ecma262/#sec-object.assign module.exports = !nativeAssign || fails(function () { // should have correct order of operations (Edge bug) if (DESCRIPTORS && nativeAssign({ b: 1 }, nativeAssign(defineProperty({}, 'a', { enumerable: true, get: function () { defineProperty(this, 'b', { value: 3, enumerable: false }); } }), { b: 2 })).b !== 1) return true; // should work with symbols and should have deterministic property order (V8 bug) var A = {}; var B = {}; // eslint-disable-next-line no-undef var symbol = Symbol(); var alphabet = 'abcdefghijklmnopqrst'; A[symbol] = 7; alphabet.split('').forEach(function (chr) { B[chr] = chr; }); return nativeAssign({}, A)[symbol] != 7 || objectKeys(nativeAssign({}, B)).join('') != alphabet; }) ? function assign(target, source) { // eslint-disable-line no-unused-vars var T = toObject(target); var argumentsLength = arguments.length; var index = 1; var getOwnPropertySymbols = getOwnPropertySymbolsModule.f; var propertyIsEnumerable = propertyIsEnumerableModule.f; while (argumentsLength > index) { var S = IndexedObject(arguments[index++]); var keys = getOwnPropertySymbols ? objectKeys(S).concat(getOwnPropertySymbols(S)) : objectKeys(S); var length = keys.length; var j = 0; var key; while (length > j) { key = keys[j++]; if (!DESCRIPTORS || propertyIsEnumerable.call(S, key)) T[key] = S[key]; } } return T; } : nativeAssign; apollo-server-demo/node_modules/core-js/internals/array-copy-within.js 0000644 0001750 0000144 00000001702 03560116604 025715 0 ustar andreh users 'use strict'; var toObject = require('../internals/to-object'); var toAbsoluteIndex = require('../internals/to-absolute-index'); var toLength = require('../internals/to-length'); var min = Math.min; // `Array.prototype.copyWithin` method implementation // https://tc39.es/ecma262/#sec-array.prototype.copywithin module.exports = [].copyWithin || function copyWithin(target /* = 0 */, start /* = 0, end = @length */) { var O = toObject(this); var len = toLength(O.length); var to = toAbsoluteIndex(target, len); var from = toAbsoluteIndex(start, len); var end = arguments.length > 2 ? arguments[2] : undefined; var count = min((end === undefined ? len : toAbsoluteIndex(end, len)) - from, len - to); var inc = 1; if (from < to && to < from + count) { inc = -1; from += count - 1; to += count - 1; } while (count-- > 0) { if (from in O) O[to] = O[from]; else delete O[to]; to += inc; from += inc; } return O; }; apollo-server-demo/node_modules/core-js/internals/document-create-element.js 0000644 0001750 0000144 00000000524 03560116604 027036 0 ustar andreh users var global = require('../internals/global'); var isObject = require('../internals/is-object'); var document = global.document; // typeof document.createElement is 'object' in old IE var EXISTS = isObject(document) && isObject(document.createElement); module.exports = function (it) { return EXISTS ? document.createElement(it) : {}; }; apollo-server-demo/node_modules/core-js/internals/well-known-symbol-wrapped.js 0000644 0001750 0000144 00000000137 03560116604 027370 0 ustar andreh users var wellKnownSymbol = require('../internals/well-known-symbol'); exports.f = wellKnownSymbol; apollo-server-demo/node_modules/core-js/internals/object-define-properties.js 0000644 0001750 0000144 00000001200 03560116604 027210 0 ustar andreh users var DESCRIPTORS = require('../internals/descriptors'); var definePropertyModule = require('../internals/object-define-property'); var anObject = require('../internals/an-object'); var objectKeys = require('../internals/object-keys'); // `Object.defineProperties` method // https://tc39.es/ecma262/#sec-object.defineproperties module.exports = DESCRIPTORS ? Object.defineProperties : function defineProperties(O, Properties) { anObject(O); var keys = objectKeys(Properties); var length = keys.length; var index = 0; var key; while (length > index) definePropertyModule.f(O, key = keys[index++], Properties[key]); return O; }; apollo-server-demo/node_modules/core-js/internals/create-property-descriptor.js 0000644 0001750 0000144 00000000255 03560116604 027632 0 ustar andreh users module.exports = function (bitmap, value) { return { enumerable: !(bitmap & 1), configurable: !(bitmap & 2), writable: !(bitmap & 4), value: value }; }; apollo-server-demo/node_modules/core-js/internals/date-to-iso-string.js 0000644 0001750 0000144 00000002344 03560116604 025763 0 ustar andreh users 'use strict'; var fails = require('../internals/fails'); var padStart = require('../internals/string-pad').start; var abs = Math.abs; var DatePrototype = Date.prototype; var getTime = DatePrototype.getTime; var nativeDateToISOString = DatePrototype.toISOString; // `Date.prototype.toISOString` method implementation // https://tc39.es/ecma262/#sec-date.prototype.toisostring // PhantomJS / old WebKit fails here: module.exports = (fails(function () { return nativeDateToISOString.call(new Date(-5e13 - 1)) != '0385-07-25T07:06:39.999Z'; }) || !fails(function () { nativeDateToISOString.call(new Date(NaN)); })) ? function toISOString() { if (!isFinite(getTime.call(this))) throw RangeError('Invalid time value'); var date = this; var year = date.getUTCFullYear(); var milliseconds = date.getUTCMilliseconds(); var sign = year < 0 ? '-' : year > 9999 ? '+' : ''; return sign + padStart(abs(year), sign ? 6 : 4, 0) + '-' + padStart(date.getUTCMonth() + 1, 2, 0) + '-' + padStart(date.getUTCDate(), 2, 0) + 'T' + padStart(date.getUTCHours(), 2, 0) + ':' + padStart(date.getUTCMinutes(), 2, 0) + ':' + padStart(date.getUTCSeconds(), 2, 0) + '.' + padStart(milliseconds, 3, 0) + 'Z'; } : nativeDateToISOString; apollo-server-demo/node_modules/core-js/internals/array-reduce.js 0000644 0001750 0000144 00000002457 03560116604 024722 0 ustar andreh users var aFunction = require('../internals/a-function'); var toObject = require('../internals/to-object'); var IndexedObject = require('../internals/indexed-object'); var toLength = require('../internals/to-length'); // `Array.prototype.{ reduce, reduceRight }` methods implementation var createMethod = function (IS_RIGHT) { return function (that, callbackfn, argumentsLength, memo) { aFunction(callbackfn); var O = toObject(that); var self = IndexedObject(O); var length = toLength(O.length); var index = IS_RIGHT ? length - 1 : 0; var i = IS_RIGHT ? -1 : 1; if (argumentsLength < 2) while (true) { if (index in self) { memo = self[index]; index += i; break; } index += i; if (IS_RIGHT ? index < 0 : length <= index) { throw TypeError('Reduce of empty array with no initial value'); } } for (;IS_RIGHT ? index >= 0 : length > index; index += i) if (index in self) { memo = callbackfn(memo, self[index], index, O); } return memo; }; }; module.exports = { // `Array.prototype.reduce` method // https://tc39.es/ecma262/#sec-array.prototype.reduce left: createMethod(false), // `Array.prototype.reduceRight` method // https://tc39.es/ecma262/#sec-array.prototype.reduceright right: createMethod(true) }; apollo-server-demo/node_modules/core-js/internals/advance-string-index.js 0000644 0001750 0000144 00000000430 03560116604 026336 0 ustar andreh users 'use strict'; var charAt = require('../internals/string-multibyte').charAt; // `AdvanceStringIndex` abstract operation // https://tc39.es/ecma262/#sec-advancestringindex module.exports = function (S, index, unicode) { return index + (unicode ? charAt(S, index).length : 1); }; apollo-server-demo/node_modules/core-js/internals/engine-v8-version.js 0000644 0001750 0000144 00000001020 03560116604 025603 0 ustar andreh users var global = require('../internals/global'); var userAgent = require('../internals/engine-user-agent'); var process = global.process; var versions = process && process.versions; var v8 = versions && versions.v8; var match, version; if (v8) { match = v8.split('.'); version = match[0] + match[1]; } else if (userAgent) { match = userAgent.match(/Edge\/(\d+)/); if (!match || match[1] >= 74) { match = userAgent.match(/Chrome\/(\d+)/); if (match) version = match[1]; } } module.exports = version && +version; apollo-server-demo/node_modules/core-js/internals/to-absolute-index.js 0000644 0001750 0000144 00000000667 03560116604 025703 0 ustar andreh users var toInteger = require('../internals/to-integer'); var max = Math.max; var min = Math.min; // Helper for a popular repeating case of the spec: // Let integer be ? ToInteger(index). // If integer < 0, let result be max((length + integer), 0); else let result be min(integer, length). module.exports = function (index, length) { var integer = toInteger(index); return integer < 0 ? max(integer + length, 0) : min(integer, length); }; apollo-server-demo/node_modules/core-js/internals/README.md 0000644 0001750 0000144 00000000077 03560116604 023254 0 ustar andreh users This folder contains internal parts of `core-js` like helpers. apollo-server-demo/node_modules/core-js/internals/array-method-uses-to-length.js 0000644 0001750 0000144 00000001576 03560116604 027610 0 ustar andreh users var DESCRIPTORS = require('../internals/descriptors'); var fails = require('../internals/fails'); var has = require('../internals/has'); var defineProperty = Object.defineProperty; var cache = {}; var thrower = function (it) { throw it; }; module.exports = function (METHOD_NAME, options) { if (has(cache, METHOD_NAME)) return cache[METHOD_NAME]; if (!options) options = {}; var method = [][METHOD_NAME]; var ACCESSORS = has(options, 'ACCESSORS') ? options.ACCESSORS : false; var argument0 = has(options, 0) ? options[0] : thrower; var argument1 = has(options, 1) ? options[1] : undefined; return cache[METHOD_NAME] = !!method && !fails(function () { if (ACCESSORS && !DESCRIPTORS) return true; var O = { length: -1 }; if (ACCESSORS) defineProperty(O, 1, { enumerable: true, get: thrower }); else O[1] = 1; method.call(O, argument0, argument1); }); }; apollo-server-demo/node_modules/core-js/internals/is-iterable.js 0000644 0001750 0000144 00000000652 03560116604 024532 0 ustar andreh users var classof = require('../internals/classof'); var wellKnownSymbol = require('../internals/well-known-symbol'); var Iterators = require('../internals/iterators'); var ITERATOR = wellKnownSymbol('iterator'); module.exports = function (it) { var O = Object(it); return O[ITERATOR] !== undefined || '@@iterator' in O // eslint-disable-next-line no-prototype-builtins || Iterators.hasOwnProperty(classof(O)); }; apollo-server-demo/node_modules/core-js/internals/to-string-tag-support.js 0000644 0001750 0000144 00000000322 03560116604 026535 0 ustar andreh users var wellKnownSymbol = require('../internals/well-known-symbol'); var TO_STRING_TAG = wellKnownSymbol('toStringTag'); var test = {}; test[TO_STRING_TAG] = 'z'; module.exports = String(test) === '[object z]'; apollo-server-demo/node_modules/core-js/internals/engine-is-node.js 0000644 0001750 0000144 00000000230 03560116604 025123 0 ustar andreh users var classof = require('../internals/classof-raw'); var global = require('../internals/global'); module.exports = classof(global.process) == 'process'; apollo-server-demo/node_modules/core-js/internals/to-offset.js 0000644 0001750 0000144 00000000340 03560116604 024232 0 ustar andreh users var toPositiveInteger = require('../internals/to-positive-integer'); module.exports = function (it, BYTES) { var offset = toPositiveInteger(it); if (offset % BYTES) throw RangeError('Wrong offset'); return offset; }; apollo-server-demo/node_modules/core-js/internals/object-get-prototype-of.js 0000644 0001750 0000144 00000001302 03560116604 027013 0 ustar andreh users var has = require('../internals/has'); var toObject = require('../internals/to-object'); var sharedKey = require('../internals/shared-key'); var CORRECT_PROTOTYPE_GETTER = require('../internals/correct-prototype-getter'); var IE_PROTO = sharedKey('IE_PROTO'); var ObjectPrototype = Object.prototype; // `Object.getPrototypeOf` method // https://tc39.es/ecma262/#sec-object.getprototypeof module.exports = CORRECT_PROTOTYPE_GETTER ? Object.getPrototypeOf : function (O) { O = toObject(O); if (has(O, IE_PROTO)) return O[IE_PROTO]; if (typeof O.constructor == 'function' && O instanceof O.constructor) { return O.constructor.prototype; } return O instanceof Object ? ObjectPrototype : null; }; apollo-server-demo/node_modules/core-js/internals/array-iteration.js 0000644 0001750 0000144 00000005352 03560116604 025446 0 ustar andreh users var bind = require('../internals/function-bind-context'); var IndexedObject = require('../internals/indexed-object'); var toObject = require('../internals/to-object'); var toLength = require('../internals/to-length'); var arraySpeciesCreate = require('../internals/array-species-create'); var push = [].push; // `Array.prototype.{ forEach, map, filter, some, every, find, findIndex, filterOut }` methods implementation var createMethod = function (TYPE) { var IS_MAP = TYPE == 1; var IS_FILTER = TYPE == 2; var IS_SOME = TYPE == 3; var IS_EVERY = TYPE == 4; var IS_FIND_INDEX = TYPE == 6; var IS_FILTER_OUT = TYPE == 7; var NO_HOLES = TYPE == 5 || IS_FIND_INDEX; return function ($this, callbackfn, that, specificCreate) { var O = toObject($this); var self = IndexedObject(O); var boundFunction = bind(callbackfn, that, 3); var length = toLength(self.length); var index = 0; var create = specificCreate || arraySpeciesCreate; var target = IS_MAP ? create($this, length) : IS_FILTER || IS_FILTER_OUT ? create($this, 0) : undefined; var value, result; for (;length > index; index++) if (NO_HOLES || index in self) { value = self[index]; result = boundFunction(value, index, O); if (TYPE) { if (IS_MAP) target[index] = result; // map else if (result) switch (TYPE) { case 3: return true; // some case 5: return value; // find case 6: return index; // findIndex case 2: push.call(target, value); // filter } else switch (TYPE) { case 4: return false; // every case 7: push.call(target, value); // filterOut } } } return IS_FIND_INDEX ? -1 : IS_SOME || IS_EVERY ? IS_EVERY : target; }; }; module.exports = { // `Array.prototype.forEach` method // https://tc39.es/ecma262/#sec-array.prototype.foreach forEach: createMethod(0), // `Array.prototype.map` method // https://tc39.es/ecma262/#sec-array.prototype.map map: createMethod(1), // `Array.prototype.filter` method // https://tc39.es/ecma262/#sec-array.prototype.filter filter: createMethod(2), // `Array.prototype.some` method // https://tc39.es/ecma262/#sec-array.prototype.some some: createMethod(3), // `Array.prototype.every` method // https://tc39.es/ecma262/#sec-array.prototype.every every: createMethod(4), // `Array.prototype.find` method // https://tc39.es/ecma262/#sec-array.prototype.find find: createMethod(5), // `Array.prototype.findIndex` method // https://tc39.es/ecma262/#sec-array.prototype.findIndex findIndex: createMethod(6), // `Array.prototype.filterOut` method // https://github.com/tc39/proposal-array-filtering filterOut: createMethod(7) }; apollo-server-demo/node_modules/core-js/internals/global.js 0000644 0001750 0000144 00000000765 03560116604 023577 0 ustar andreh users var check = function (it) { return it && it.Math == Math && it; }; // https://github.com/zloirock/core-js/issues/86#issuecomment-115759028 module.exports = // eslint-disable-next-line no-undef check(typeof globalThis == 'object' && globalThis) || check(typeof window == 'object' && window) || check(typeof self == 'object' && self) || check(typeof global == 'object' && global) || // eslint-disable-next-line no-new-func (function () { return this; })() || Function('return this')(); apollo-server-demo/node_modules/core-js/internals/internal-state.js 0000644 0001750 0000144 00000003222 03560116604 025260 0 ustar andreh users var NATIVE_WEAK_MAP = require('../internals/native-weak-map'); var global = require('../internals/global'); var isObject = require('../internals/is-object'); var createNonEnumerableProperty = require('../internals/create-non-enumerable-property'); var objectHas = require('../internals/has'); var shared = require('../internals/shared-store'); var sharedKey = require('../internals/shared-key'); var hiddenKeys = require('../internals/hidden-keys'); var WeakMap = global.WeakMap; var set, get, has; var enforce = function (it) { return has(it) ? get(it) : set(it, {}); }; var getterFor = function (TYPE) { return function (it) { var state; if (!isObject(it) || (state = get(it)).type !== TYPE) { throw TypeError('Incompatible receiver, ' + TYPE + ' required'); } return state; }; }; if (NATIVE_WEAK_MAP) { var store = shared.state || (shared.state = new WeakMap()); var wmget = store.get; var wmhas = store.has; var wmset = store.set; set = function (it, metadata) { metadata.facade = it; wmset.call(store, it, metadata); return metadata; }; get = function (it) { return wmget.call(store, it) || {}; }; has = function (it) { return wmhas.call(store, it); }; } else { var STATE = sharedKey('state'); hiddenKeys[STATE] = true; set = function (it, metadata) { metadata.facade = it; createNonEnumerableProperty(it, STATE, metadata); return metadata; }; get = function (it) { return objectHas(it, STATE) ? it[STATE] : {}; }; has = function (it) { return objectHas(it, STATE); }; } module.exports = { set: set, get: get, has: has, enforce: enforce, getterFor: getterFor }; apollo-server-demo/node_modules/core-js/internals/create-non-enumerable-property.js 0000644 0001750 0000144 00000000666 03560116604 030371 0 ustar andreh users var DESCRIPTORS = require('../internals/descriptors'); var definePropertyModule = require('../internals/object-define-property'); var createPropertyDescriptor = require('../internals/create-property-descriptor'); module.exports = DESCRIPTORS ? function (object, key, value) { return definePropertyModule.f(object, key, createPropertyDescriptor(1, value)); } : function (object, key, value) { object[key] = value; return object; }; apollo-server-demo/node_modules/core-js/internals/array-for-each.js 0000644 0001750 0000144 00000001221 03560116604 025123 0 ustar andreh users 'use strict'; var $forEach = require('../internals/array-iteration').forEach; var arrayMethodIsStrict = require('../internals/array-method-is-strict'); var arrayMethodUsesToLength = require('../internals/array-method-uses-to-length'); var STRICT_METHOD = arrayMethodIsStrict('forEach'); var USES_TO_LENGTH = arrayMethodUsesToLength('forEach'); // `Array.prototype.forEach` method implementation // https://tc39.es/ecma262/#sec-array.prototype.foreach module.exports = (!STRICT_METHOD || !USES_TO_LENGTH) ? function forEach(callbackfn /* , thisArg */) { return $forEach(this, callbackfn, arguments.length > 1 ? arguments[1] : undefined); } : [].forEach; apollo-server-demo/node_modules/core-js/internals/shared-store.js 0000644 0001750 0000144 00000000323 03560116604 024725 0 ustar andreh users var global = require('../internals/global'); var setGlobal = require('../internals/set-global'); var SHARED = '__core-js_shared__'; var store = global[SHARED] || setGlobal(SHARED, {}); module.exports = store; apollo-server-demo/node_modules/core-js/internals/iterator-close.js 0000644 0001750 0000144 00000000346 03560116604 025266 0 ustar andreh users var anObject = require('../internals/an-object'); module.exports = function (iterator) { var returnMethod = iterator['return']; if (returnMethod !== undefined) { return anObject(returnMethod.call(iterator)).value; } }; apollo-server-demo/node_modules/core-js/internals/object-get-own-property-descriptor.js 0000644 0001750 0000144 00000001742 03560116604 031215 0 ustar andreh users var DESCRIPTORS = require('../internals/descriptors'); var propertyIsEnumerableModule = require('../internals/object-property-is-enumerable'); var createPropertyDescriptor = require('../internals/create-property-descriptor'); var toIndexedObject = require('../internals/to-indexed-object'); var toPrimitive = require('../internals/to-primitive'); var has = require('../internals/has'); var IE8_DOM_DEFINE = require('../internals/ie8-dom-define'); var nativeGetOwnPropertyDescriptor = Object.getOwnPropertyDescriptor; // `Object.getOwnPropertyDescriptor` method // https://tc39.es/ecma262/#sec-object.getownpropertydescriptor exports.f = DESCRIPTORS ? nativeGetOwnPropertyDescriptor : function getOwnPropertyDescriptor(O, P) { O = toIndexedObject(O); P = toPrimitive(P, true); if (IE8_DOM_DEFINE) try { return nativeGetOwnPropertyDescriptor(O, P); } catch (error) { /* empty */ } if (has(O, P)) return createPropertyDescriptor(!propertyIsEnumerableModule.f.call(O, P), O[P]); }; apollo-server-demo/node_modules/core-js/internals/is-pure.js 0000644 0001750 0000144 00000000030 03560116604 023704 0 ustar andreh users module.exports = false; apollo-server-demo/node_modules/core-js/internals/host-report-errors.js 0000644 0001750 0000144 00000000337 03560116604 026132 0 ustar andreh users var global = require('../internals/global'); module.exports = function (a, b) { var console = global.console; if (console && console.error) { arguments.length === 1 ? console.error(a) : console.error(a, b); } }; apollo-server-demo/node_modules/core-js/internals/typed-array-from.js 0000644 0001750 0000144 00000002342 03560116604 025532 0 ustar andreh users var toObject = require('../internals/to-object'); var toLength = require('../internals/to-length'); var getIteratorMethod = require('../internals/get-iterator-method'); var isArrayIteratorMethod = require('../internals/is-array-iterator-method'); var bind = require('../internals/function-bind-context'); var aTypedArrayConstructor = require('../internals/array-buffer-view-core').aTypedArrayConstructor; module.exports = function from(source /* , mapfn, thisArg */) { var O = toObject(source); var argumentsLength = arguments.length; var mapfn = argumentsLength > 1 ? arguments[1] : undefined; var mapping = mapfn !== undefined; var iteratorMethod = getIteratorMethod(O); var i, length, result, step, iterator, next; if (iteratorMethod != undefined && !isArrayIteratorMethod(iteratorMethod)) { iterator = iteratorMethod.call(O); next = iterator.next; O = []; while (!(step = next.call(iterator)).done) { O.push(step.value); } } if (mapping && argumentsLength > 2) { mapfn = bind(mapfn, arguments[2], 2); } length = toLength(O.length); result = new (aTypedArrayConstructor(this))(length); for (i = 0; length > i; i++) { result[i] = mapping ? mapfn(O[i], i) : O[i]; } return result; }; apollo-server-demo/node_modules/core-js/internals/to-primitive.js 0000644 0001750 0000144 00000001404 03560116604 024756 0 ustar andreh users var isObject = require('../internals/is-object'); // `ToPrimitive` abstract operation // https://tc39.es/ecma262/#sec-toprimitive // instead of the ES6 spec version, we didn't implement @@toPrimitive case // and the second argument - flag - preferred type is a string module.exports = function (input, PREFERRED_STRING) { if (!isObject(input)) return input; var fn, val; if (PREFERRED_STRING && typeof (fn = input.toString) == 'function' && !isObject(val = fn.call(input))) return val; if (typeof (fn = input.valueOf) == 'function' && !isObject(val = fn.call(input))) return val; if (!PREFERRED_STRING && typeof (fn = input.toString) == 'function' && !isObject(val = fn.call(input))) return val; throw TypeError("Can't convert object to primitive value"); }; apollo-server-demo/node_modules/core-js/internals/iterator-create-proxy.js 0000644 0001750 0000144 00000003342 03560116604 026602 0 ustar andreh users 'use strict'; var path = require('../internals/path'); var aFunction = require('../internals/a-function'); var anObject = require('../internals/an-object'); var create = require('../internals/object-create'); var createNonEnumerableProperty = require('../internals/create-non-enumerable-property'); var redefineAll = require('../internals/redefine-all'); var wellKnownSymbol = require('../internals/well-known-symbol'); var InternalStateModule = require('../internals/internal-state'); var setInternalState = InternalStateModule.set; var getInternalState = InternalStateModule.get; var TO_STRING_TAG = wellKnownSymbol('toStringTag'); var $return = function (value) { var iterator = getInternalState(this).iterator; var $$return = iterator['return']; return $$return === undefined ? { done: true, value: value } : anObject($$return.call(iterator, value)); }; var $throw = function (value) { var iterator = getInternalState(this).iterator; var $$throw = iterator['throw']; if ($$throw === undefined) throw value; return $$throw.call(iterator, value); }; module.exports = function (nextHandler, IS_ITERATOR) { var IteratorProxy = function Iterator(state) { state.next = aFunction(state.iterator.next); state.done = false; setInternalState(this, state); }; IteratorProxy.prototype = redefineAll(create(path.Iterator.prototype), { next: function next() { var state = getInternalState(this); var result = state.done ? undefined : nextHandler.apply(state, arguments); return { done: state.done, value: result }; }, 'return': $return, 'throw': $throw }); if (!IS_ITERATOR) { createNonEnumerableProperty(IteratorProxy.prototype, TO_STRING_TAG, 'Generator'); } return IteratorProxy; }; apollo-server-demo/node_modules/core-js/internals/array-buffer.js 0000644 0001750 0000144 00000021624 03560116604 024721 0 ustar andreh users 'use strict'; var global = require('../internals/global'); var DESCRIPTORS = require('../internals/descriptors'); var NATIVE_ARRAY_BUFFER = require('../internals/array-buffer-native'); var createNonEnumerableProperty = require('../internals/create-non-enumerable-property'); var redefineAll = require('../internals/redefine-all'); var fails = require('../internals/fails'); var anInstance = require('../internals/an-instance'); var toInteger = require('../internals/to-integer'); var toLength = require('../internals/to-length'); var toIndex = require('../internals/to-index'); var IEEE754 = require('../internals/ieee754'); var getPrototypeOf = require('../internals/object-get-prototype-of'); var setPrototypeOf = require('../internals/object-set-prototype-of'); var getOwnPropertyNames = require('../internals/object-get-own-property-names').f; var defineProperty = require('../internals/object-define-property').f; var arrayFill = require('../internals/array-fill'); var setToStringTag = require('../internals/set-to-string-tag'); var InternalStateModule = require('../internals/internal-state'); var getInternalState = InternalStateModule.get; var setInternalState = InternalStateModule.set; var ARRAY_BUFFER = 'ArrayBuffer'; var DATA_VIEW = 'DataView'; var PROTOTYPE = 'prototype'; var WRONG_LENGTH = 'Wrong length'; var WRONG_INDEX = 'Wrong index'; var NativeArrayBuffer = global[ARRAY_BUFFER]; var $ArrayBuffer = NativeArrayBuffer; var $DataView = global[DATA_VIEW]; var $DataViewPrototype = $DataView && $DataView[PROTOTYPE]; var ObjectPrototype = Object.prototype; var RangeError = global.RangeError; var packIEEE754 = IEEE754.pack; var unpackIEEE754 = IEEE754.unpack; var packInt8 = function (number) { return [number & 0xFF]; }; var packInt16 = function (number) { return [number & 0xFF, number >> 8 & 0xFF]; }; var packInt32 = function (number) { return [number & 0xFF, number >> 8 & 0xFF, number >> 16 & 0xFF, number >> 24 & 0xFF]; }; var unpackInt32 = function (buffer) { return buffer[3] << 24 | buffer[2] << 16 | buffer[1] << 8 | buffer[0]; }; var packFloat32 = function (number) { return packIEEE754(number, 23, 4); }; var packFloat64 = function (number) { return packIEEE754(number, 52, 8); }; var addGetter = function (Constructor, key) { defineProperty(Constructor[PROTOTYPE], key, { get: function () { return getInternalState(this)[key]; } }); }; var get = function (view, count, index, isLittleEndian) { var intIndex = toIndex(index); var store = getInternalState(view); if (intIndex + count > store.byteLength) throw RangeError(WRONG_INDEX); var bytes = getInternalState(store.buffer).bytes; var start = intIndex + store.byteOffset; var pack = bytes.slice(start, start + count); return isLittleEndian ? pack : pack.reverse(); }; var set = function (view, count, index, conversion, value, isLittleEndian) { var intIndex = toIndex(index); var store = getInternalState(view); if (intIndex + count > store.byteLength) throw RangeError(WRONG_INDEX); var bytes = getInternalState(store.buffer).bytes; var start = intIndex + store.byteOffset; var pack = conversion(+value); for (var i = 0; i < count; i++) bytes[start + i] = pack[isLittleEndian ? i : count - i - 1]; }; if (!NATIVE_ARRAY_BUFFER) { $ArrayBuffer = function ArrayBuffer(length) { anInstance(this, $ArrayBuffer, ARRAY_BUFFER); var byteLength = toIndex(length); setInternalState(this, { bytes: arrayFill.call(new Array(byteLength), 0), byteLength: byteLength }); if (!DESCRIPTORS) this.byteLength = byteLength; }; $DataView = function DataView(buffer, byteOffset, byteLength) { anInstance(this, $DataView, DATA_VIEW); anInstance(buffer, $ArrayBuffer, DATA_VIEW); var bufferLength = getInternalState(buffer).byteLength; var offset = toInteger(byteOffset); if (offset < 0 || offset > bufferLength) throw RangeError('Wrong offset'); byteLength = byteLength === undefined ? bufferLength - offset : toLength(byteLength); if (offset + byteLength > bufferLength) throw RangeError(WRONG_LENGTH); setInternalState(this, { buffer: buffer, byteLength: byteLength, byteOffset: offset }); if (!DESCRIPTORS) { this.buffer = buffer; this.byteLength = byteLength; this.byteOffset = offset; } }; if (DESCRIPTORS) { addGetter($ArrayBuffer, 'byteLength'); addGetter($DataView, 'buffer'); addGetter($DataView, 'byteLength'); addGetter($DataView, 'byteOffset'); } redefineAll($DataView[PROTOTYPE], { getInt8: function getInt8(byteOffset) { return get(this, 1, byteOffset)[0] << 24 >> 24; }, getUint8: function getUint8(byteOffset) { return get(this, 1, byteOffset)[0]; }, getInt16: function getInt16(byteOffset /* , littleEndian */) { var bytes = get(this, 2, byteOffset, arguments.length > 1 ? arguments[1] : undefined); return (bytes[1] << 8 | bytes[0]) << 16 >> 16; }, getUint16: function getUint16(byteOffset /* , littleEndian */) { var bytes = get(this, 2, byteOffset, arguments.length > 1 ? arguments[1] : undefined); return bytes[1] << 8 | bytes[0]; }, getInt32: function getInt32(byteOffset /* , littleEndian */) { return unpackInt32(get(this, 4, byteOffset, arguments.length > 1 ? arguments[1] : undefined)); }, getUint32: function getUint32(byteOffset /* , littleEndian */) { return unpackInt32(get(this, 4, byteOffset, arguments.length > 1 ? arguments[1] : undefined)) >>> 0; }, getFloat32: function getFloat32(byteOffset /* , littleEndian */) { return unpackIEEE754(get(this, 4, byteOffset, arguments.length > 1 ? arguments[1] : undefined), 23); }, getFloat64: function getFloat64(byteOffset /* , littleEndian */) { return unpackIEEE754(get(this, 8, byteOffset, arguments.length > 1 ? arguments[1] : undefined), 52); }, setInt8: function setInt8(byteOffset, value) { set(this, 1, byteOffset, packInt8, value); }, setUint8: function setUint8(byteOffset, value) { set(this, 1, byteOffset, packInt8, value); }, setInt16: function setInt16(byteOffset, value /* , littleEndian */) { set(this, 2, byteOffset, packInt16, value, arguments.length > 2 ? arguments[2] : undefined); }, setUint16: function setUint16(byteOffset, value /* , littleEndian */) { set(this, 2, byteOffset, packInt16, value, arguments.length > 2 ? arguments[2] : undefined); }, setInt32: function setInt32(byteOffset, value /* , littleEndian */) { set(this, 4, byteOffset, packInt32, value, arguments.length > 2 ? arguments[2] : undefined); }, setUint32: function setUint32(byteOffset, value /* , littleEndian */) { set(this, 4, byteOffset, packInt32, value, arguments.length > 2 ? arguments[2] : undefined); }, setFloat32: function setFloat32(byteOffset, value /* , littleEndian */) { set(this, 4, byteOffset, packFloat32, value, arguments.length > 2 ? arguments[2] : undefined); }, setFloat64: function setFloat64(byteOffset, value /* , littleEndian */) { set(this, 8, byteOffset, packFloat64, value, arguments.length > 2 ? arguments[2] : undefined); } }); } else { if (!fails(function () { NativeArrayBuffer(1); }) || !fails(function () { new NativeArrayBuffer(-1); // eslint-disable-line no-new }) || fails(function () { new NativeArrayBuffer(); // eslint-disable-line no-new new NativeArrayBuffer(1.5); // eslint-disable-line no-new new NativeArrayBuffer(NaN); // eslint-disable-line no-new return NativeArrayBuffer.name != ARRAY_BUFFER; })) { $ArrayBuffer = function ArrayBuffer(length) { anInstance(this, $ArrayBuffer); return new NativeArrayBuffer(toIndex(length)); }; var ArrayBufferPrototype = $ArrayBuffer[PROTOTYPE] = NativeArrayBuffer[PROTOTYPE]; for (var keys = getOwnPropertyNames(NativeArrayBuffer), j = 0, key; keys.length > j;) { if (!((key = keys[j++]) in $ArrayBuffer)) { createNonEnumerableProperty($ArrayBuffer, key, NativeArrayBuffer[key]); } } ArrayBufferPrototype.constructor = $ArrayBuffer; } // WebKit bug - the same parent prototype for typed arrays and data view if (setPrototypeOf && getPrototypeOf($DataViewPrototype) !== ObjectPrototype) { setPrototypeOf($DataViewPrototype, ObjectPrototype); } // iOS Safari 7.x bug var testView = new $DataView(new $ArrayBuffer(2)); var nativeSetInt8 = $DataViewPrototype.setInt8; testView.setInt8(0, 2147483648); testView.setInt8(1, 2147483649); if (testView.getInt8(0) || !testView.getInt8(1)) redefineAll($DataViewPrototype, { setInt8: function setInt8(byteOffset, value) { nativeSetInt8.call(this, byteOffset, value << 24 >> 24); }, setUint8: function setUint8(byteOffset, value) { nativeSetInt8.call(this, byteOffset, value << 24 >> 24); } }, { unsafe: true }); } setToStringTag($ArrayBuffer, ARRAY_BUFFER); setToStringTag($DataView, DATA_VIEW); module.exports = { ArrayBuffer: $ArrayBuffer, DataView: $DataView }; apollo-server-demo/node_modules/core-js/internals/inherit-if-required.js 0000644 0001750 0000144 00000001265 03560116604 026207 0 ustar andreh users var isObject = require('../internals/is-object'); var setPrototypeOf = require('../internals/object-set-prototype-of'); // makes subclassing work correct for wrapped built-ins module.exports = function ($this, dummy, Wrapper) { var NewTarget, NewTargetPrototype; if ( // it can work only with native `setPrototypeOf` setPrototypeOf && // we haven't completely correct pre-ES6 way for getting `new.target`, so use this typeof (NewTarget = dummy.constructor) == 'function' && NewTarget !== Wrapper && isObject(NewTargetPrototype = NewTarget.prototype) && NewTargetPrototype !== Wrapper.prototype ) setPrototypeOf($this, NewTargetPrototype); return $this; }; apollo-server-demo/node_modules/core-js/internals/own-keys.js 0000644 0001750 0000144 00000001150 03560116604 024100 0 ustar andreh users var getBuiltIn = require('../internals/get-built-in'); var getOwnPropertyNamesModule = require('../internals/object-get-own-property-names'); var getOwnPropertySymbolsModule = require('../internals/object-get-own-property-symbols'); var anObject = require('../internals/an-object'); // all object keys, includes non-enumerable and symbols module.exports = getBuiltIn('Reflect', 'ownKeys') || function ownKeys(it) { var keys = getOwnPropertyNamesModule.f(anObject(it)); var getOwnPropertySymbols = getOwnPropertySymbolsModule.f; return getOwnPropertySymbols ? keys.concat(getOwnPropertySymbols(it)) : keys; }; apollo-server-demo/node_modules/core-js/internals/promise-resolve.js 0000644 0001750 0000144 00000000653 03560116604 025466 0 ustar andreh users var anObject = require('../internals/an-object'); var isObject = require('../internals/is-object'); var newPromiseCapability = require('../internals/new-promise-capability'); module.exports = function (C, x) { anObject(C); if (isObject(x) && x.constructor === C) return x; var promiseCapability = newPromiseCapability.f(C); var resolve = promiseCapability.resolve; resolve(x); return promiseCapability.promise; }; apollo-server-demo/node_modules/core-js/internals/string-pad.js 0000644 0001750 0000144 00000002347 03560116604 024405 0 ustar andreh users // https://github.com/tc39/proposal-string-pad-start-end var toLength = require('../internals/to-length'); var repeat = require('../internals/string-repeat'); var requireObjectCoercible = require('../internals/require-object-coercible'); var ceil = Math.ceil; // `String.prototype.{ padStart, padEnd }` methods implementation var createMethod = function (IS_END) { return function ($this, maxLength, fillString) { var S = String(requireObjectCoercible($this)); var stringLength = S.length; var fillStr = fillString === undefined ? ' ' : String(fillString); var intMaxLength = toLength(maxLength); var fillLen, stringFiller; if (intMaxLength <= stringLength || fillStr == '') return S; fillLen = intMaxLength - stringLength; stringFiller = repeat.call(fillStr, ceil(fillLen / fillStr.length)); if (stringFiller.length > fillLen) stringFiller = stringFiller.slice(0, fillLen); return IS_END ? S + stringFiller : stringFiller + S; }; }; module.exports = { // `String.prototype.padStart` method // https://tc39.es/ecma262/#sec-string.prototype.padstart start: createMethod(false), // `String.prototype.padEnd` method // https://tc39.es/ecma262/#sec-string.prototype.padend end: createMethod(true) }; apollo-server-demo/node_modules/core-js/internals/dom-iterables.js 0000644 0001750 0000144 00000001361 03560116604 025057 0 ustar andreh users // iterable DOM collections // flag - `iterable` interface - 'entries', 'keys', 'values', 'forEach' methods module.exports = { CSSRuleList: 0, CSSStyleDeclaration: 0, CSSValueList: 0, ClientRectList: 0, DOMRectList: 0, DOMStringList: 0, DOMTokenList: 1, DataTransferItemList: 0, FileList: 0, HTMLAllCollection: 0, HTMLCollection: 0, HTMLFormElement: 0, HTMLSelectElement: 0, MediaList: 0, MimeTypeArray: 0, NamedNodeMap: 0, NodeList: 1, PaintRequestList: 0, Plugin: 0, PluginArray: 0, SVGLengthList: 0, SVGNumberList: 0, SVGPathSegList: 0, SVGPointList: 0, SVGStringList: 0, SVGTransformList: 0, SourceBufferList: 0, StyleSheetList: 0, TextTrackCueList: 0, TextTrackList: 0, TouchList: 0 }; apollo-server-demo/node_modules/core-js/internals/engine-is-ios.js 0000644 0001750 0000144 00000000201 03560116604 024766 0 ustar andreh users var userAgent = require('../internals/engine-user-agent'); module.exports = /(iphone|ipod|ipad).*applewebkit/i.test(userAgent); apollo-server-demo/node_modules/core-js/internals/is-regexp.js 0000644 0001750 0000144 00000000664 03560116604 024240 0 ustar andreh users var isObject = require('../internals/is-object'); var classof = require('../internals/classof-raw'); var wellKnownSymbol = require('../internals/well-known-symbol'); var MATCH = wellKnownSymbol('match'); // `IsRegExp` abstract operation // https://tc39.es/ecma262/#sec-isregexp module.exports = function (it) { var isRegExp; return isObject(it) && ((isRegExp = it[MATCH]) !== undefined ? !!isRegExp : classof(it) == 'RegExp'); }; apollo-server-demo/node_modules/core-js/internals/object-get-own-property-symbols.js 0000644 0001750 0000144 00000000052 03560116604 030520 0 ustar andreh users exports.f = Object.getOwnPropertySymbols; apollo-server-demo/node_modules/core-js/internals/iterators-core.js 0000644 0001750 0000144 00000003103 03560116604 025266 0 ustar andreh users 'use strict'; var fails = require('../internals/fails'); var getPrototypeOf = require('../internals/object-get-prototype-of'); var createNonEnumerableProperty = require('../internals/create-non-enumerable-property'); var has = require('../internals/has'); var wellKnownSymbol = require('../internals/well-known-symbol'); var IS_PURE = require('../internals/is-pure'); var ITERATOR = wellKnownSymbol('iterator'); var BUGGY_SAFARI_ITERATORS = false; var returnThis = function () { return this; }; // `%IteratorPrototype%` object // https://tc39.es/ecma262/#sec-%iteratorprototype%-object var IteratorPrototype, PrototypeOfArrayIteratorPrototype, arrayIterator; if ([].keys) { arrayIterator = [].keys(); // Safari 8 has buggy iterators w/o `next` if (!('next' in arrayIterator)) BUGGY_SAFARI_ITERATORS = true; else { PrototypeOfArrayIteratorPrototype = getPrototypeOf(getPrototypeOf(arrayIterator)); if (PrototypeOfArrayIteratorPrototype !== Object.prototype) IteratorPrototype = PrototypeOfArrayIteratorPrototype; } } var NEW_ITERATOR_PROTOTYPE = IteratorPrototype == undefined || fails(function () { var test = {}; // FF44- legacy iterators case return IteratorPrototype[ITERATOR].call(test) !== test; }); if (NEW_ITERATOR_PROTOTYPE) IteratorPrototype = {}; // 25.1.2.1.1 %IteratorPrototype%[@@iterator]() if ((!IS_PURE || NEW_ITERATOR_PROTOTYPE) && !has(IteratorPrototype, ITERATOR)) { createNonEnumerableProperty(IteratorPrototype, ITERATOR, returnThis); } module.exports = { IteratorPrototype: IteratorPrototype, BUGGY_SAFARI_ITERATORS: BUGGY_SAFARI_ITERATORS }; apollo-server-demo/node_modules/core-js/internals/to-indexed-object.js 0000644 0001750 0000144 00000000435 03560116604 025635 0 ustar andreh users // toObject with fallback for non-array-like ES3 strings var IndexedObject = require('../internals/indexed-object'); var requireObjectCoercible = require('../internals/require-object-coercible'); module.exports = function (it) { return IndexedObject(requireObjectCoercible(it)); }; apollo-server-demo/node_modules/core-js/internals/is-object.js 0000644 0001750 0000144 00000000156 03560116604 024210 0 ustar andreh users module.exports = function (it) { return typeof it === 'object' ? it !== null : typeof it === 'function'; }; apollo-server-demo/node_modules/core-js/internals/native-url.js 0000644 0001750 0000144 00000002200 03560116604 024407 0 ustar andreh users var fails = require('../internals/fails'); var wellKnownSymbol = require('../internals/well-known-symbol'); var IS_PURE = require('../internals/is-pure'); var ITERATOR = wellKnownSymbol('iterator'); module.exports = !fails(function () { var url = new URL('b?a=1&b=2&c=3', 'http://a'); var searchParams = url.searchParams; var result = ''; url.pathname = 'c%20d'; searchParams.forEach(function (value, key) { searchParams['delete']('b'); result += key + value; }); return (IS_PURE && !url.toJSON) || !searchParams.sort || url.href !== 'http://a/c%20d?a=1&c=3' || searchParams.get('c') !== '3' || String(new URLSearchParams('?a=1')) !== 'a=1' || !searchParams[ITERATOR] // throws in Edge || new URL('https://a@b').username !== 'a' || new URLSearchParams(new URLSearchParams('a=b')).get('a') !== 'b' // not punycoded in Edge || new URL('http://теÑÑ‚').host !== 'xn--e1aybc' // not escaped in Chrome 62- || new URL('http://a#б').hash !== '#%D0%B1' // fails in Chrome 66- || result !== 'a1c3' // throws in Safari || new URL('http://x', undefined).host !== 'x'; }); apollo-server-demo/node_modules/core-js/internals/get-set-iterator.js 0000644 0001750 0000144 00000000363 03560116604 025530 0 ustar andreh users var IS_PURE = require('../internals/is-pure'); var getIterator = require('../internals/get-iterator'); module.exports = IS_PURE ? getIterator : function (it) { // eslint-disable-next-line no-undef return Set.prototype.values.call(it); }; apollo-server-demo/node_modules/core-js/internals/native-weak-map.js 0000644 0001750 0000144 00000000347 03560116604 025321 0 ustar andreh users var global = require('../internals/global'); var inspectSource = require('../internals/inspect-source'); var WeakMap = global.WeakMap; module.exports = typeof WeakMap === 'function' && /native code/.test(inspectSource(WeakMap)); apollo-server-demo/node_modules/core-js/internals/define-well-known-symbol.js 0000644 0001750 0000144 00000000665 03560116604 027166 0 ustar andreh users var path = require('../internals/path'); var has = require('../internals/has'); var wrappedWellKnownSymbolModule = require('../internals/well-known-symbol-wrapped'); var defineProperty = require('../internals/object-define-property').f; module.exports = function (NAME) { var Symbol = path.Symbol || (path.Symbol = {}); if (!has(Symbol, NAME)) defineProperty(Symbol, NAME, { value: wrappedWellKnownSymbolModule.f(NAME) }); }; apollo-server-demo/node_modules/core-js/internals/string-multibyte.js 0000644 0001750 0000144 00000002167 03560116604 025657 0 ustar andreh users var toInteger = require('../internals/to-integer'); var requireObjectCoercible = require('../internals/require-object-coercible'); // `String.prototype.{ codePointAt, at }` methods implementation var createMethod = function (CONVERT_TO_STRING) { return function ($this, pos) { var S = String(requireObjectCoercible($this)); var position = toInteger(pos); var size = S.length; var first, second; if (position < 0 || position >= size) return CONVERT_TO_STRING ? '' : undefined; first = S.charCodeAt(position); return first < 0xD800 || first > 0xDBFF || position + 1 === size || (second = S.charCodeAt(position + 1)) < 0xDC00 || second > 0xDFFF ? CONVERT_TO_STRING ? S.charAt(position) : first : CONVERT_TO_STRING ? S.slice(position, position + 2) : (first - 0xD800 << 10) + (second - 0xDC00) + 0x10000; }; }; module.exports = { // `String.prototype.codePointAt` method // https://tc39.es/ecma262/#sec-string.prototype.codepointat codeAt: createMethod(false), // `String.prototype.at` method // https://github.com/mathiasbynens/String.prototype.at charAt: createMethod(true) }; apollo-server-demo/node_modules/core-js/internals/array-fill.js 0000644 0001750 0000144 00000001342 03560116604 024371 0 ustar andreh users 'use strict'; var toObject = require('../internals/to-object'); var toAbsoluteIndex = require('../internals/to-absolute-index'); var toLength = require('../internals/to-length'); // `Array.prototype.fill` method implementation // https://tc39.es/ecma262/#sec-array.prototype.fill module.exports = function fill(value /* , start = 0, end = @length */) { var O = toObject(this); var length = toLength(O.length); var argumentsLength = arguments.length; var index = toAbsoluteIndex(argumentsLength > 1 ? arguments[1] : undefined, length); var end = argumentsLength > 2 ? arguments[2] : undefined; var endPos = end === undefined ? length : toAbsoluteIndex(end, length); while (endPos > index) O[index++] = value; return O; }; apollo-server-demo/node_modules/core-js/internals/object-to-string.js 0000644 0001750 0000144 00000000563 03560116604 025525 0 ustar andreh users 'use strict'; var TO_STRING_TAG_SUPPORT = require('../internals/to-string-tag-support'); var classof = require('../internals/classof'); // `Object.prototype.toString` method implementation // https://tc39.es/ecma262/#sec-object.prototype.tostring module.exports = TO_STRING_TAG_SUPPORT ? {}.toString : function toString() { return '[object ' + classof(this) + ']'; }; apollo-server-demo/node_modules/core-js/internals/number-parse-int.js 0000644 0001750 0000144 00000001051 03560116604 025514 0 ustar andreh users var global = require('../internals/global'); var trim = require('../internals/string-trim').trim; var whitespaces = require('../internals/whitespaces'); var $parseInt = global.parseInt; var hex = /^[+-]?0[Xx]/; var FORCED = $parseInt(whitespaces + '08') !== 8 || $parseInt(whitespaces + '0x16') !== 22; // `parseInt` method // https://tc39.es/ecma262/#sec-parseint-string-radix module.exports = FORCED ? function parseInt(string, radix) { var S = trim(String(string)); return $parseInt(S, (radix >>> 0) || (hex.test(S) ? 16 : 10)); } : $parseInt; apollo-server-demo/node_modules/core-js/internals/call-with-safe-iteration-closing.js 0000644 0001750 0000144 00000000653 03560116604 030563 0 ustar andreh users var anObject = require('../internals/an-object'); var iteratorClose = require('../internals/iterator-close'); // call something on iterator step with safe closing on error module.exports = function (iterator, fn, value, ENTRIES) { try { return ENTRIES ? fn(anObject(value)[0], value[1]) : fn(value); // 7.4.6 IteratorClose(iterator, completion) } catch (error) { iteratorClose(iterator); throw error; } }; apollo-server-demo/node_modules/core-js/internals/object-iterator.js 0000644 0001750 0000144 00000002440 03560116604 025424 0 ustar andreh users 'use strict'; var InternalStateModule = require('../internals/internal-state'); var createIteratorConstructor = require('../internals/create-iterator-constructor'); var has = require('../internals/has'); var objectKeys = require('../internals/object-keys'); var toObject = require('../internals/to-object'); var OBJECT_ITERATOR = 'Object Iterator'; var setInternalState = InternalStateModule.set; var getInternalState = InternalStateModule.getterFor(OBJECT_ITERATOR); module.exports = createIteratorConstructor(function ObjectIterator(source, mode) { var object = toObject(source); setInternalState(this, { type: OBJECT_ITERATOR, mode: mode, object: object, keys: objectKeys(object), index: 0 }); }, 'Object', function next() { var state = getInternalState(this); var keys = state.keys; while (true) { if (keys === null || state.index >= keys.length) { state.object = state.keys = null; return { value: undefined, done: true }; } var key = keys[state.index++]; var object = state.object; if (!has(object, key)) continue; switch (state.mode) { case 'keys': return { value: key, done: false }; case 'values': return { value: object[key], done: false }; } /* entries */ return { value: [key, object[key]], done: false }; } }); apollo-server-demo/node_modules/core-js/internals/function-bind-context.js 0000644 0001750 0000144 00000001127 03560116604 026551 0 ustar andreh users var aFunction = require('../internals/a-function'); // optional / simple context binding module.exports = function (fn, that, length) { aFunction(fn); if (that === undefined) return fn; switch (length) { case 0: return function () { return fn.call(that); }; case 1: return function (a) { return fn.call(that, a); }; case 2: return function (a, b) { return fn.call(that, a, b); }; case 3: return function (a, b, c) { return fn.call(that, a, b, c); }; } return function (/* ...args */) { return fn.apply(that, arguments); }; }; apollo-server-demo/node_modules/core-js/internals/copy-constructor-properties.js 0000644 0001750 0000144 00000001150 03560116604 030053 0 ustar andreh users var has = require('../internals/has'); var ownKeys = require('../internals/own-keys'); var getOwnPropertyDescriptorModule = require('../internals/object-get-own-property-descriptor'); var definePropertyModule = require('../internals/object-define-property'); module.exports = function (target, source) { var keys = ownKeys(source); var defineProperty = definePropertyModule.f; var getOwnPropertyDescriptor = getOwnPropertyDescriptorModule.f; for (var i = 0; i < keys.length; i++) { var key = keys[i]; if (!has(target, key)) defineProperty(target, key, getOwnPropertyDescriptor(source, key)); } }; apollo-server-demo/node_modules/core-js/internals/math-fround.js 0000644 0001750 0000144 00000001435 03560116604 024556 0 ustar andreh users var sign = require('../internals/math-sign'); var abs = Math.abs; var pow = Math.pow; var EPSILON = pow(2, -52); var EPSILON32 = pow(2, -23); var MAX32 = pow(2, 127) * (2 - EPSILON32); var MIN32 = pow(2, -126); var roundTiesToEven = function (n) { return n + 1 / EPSILON - 1 / EPSILON; }; // `Math.fround` method implementation // https://tc39.es/ecma262/#sec-math.fround module.exports = Math.fround || function fround(x) { var $abs = abs(x); var $sign = sign(x); var a, result; if ($abs < MIN32) return $sign * roundTiesToEven($abs / MIN32 / EPSILON32) * MIN32 * EPSILON32; a = (1 + EPSILON32 / EPSILON) * $abs; result = a - (a - $abs); // eslint-disable-next-line no-self-compare if (result > MAX32 || result != result) return $sign * Infinity; return $sign * result; }; apollo-server-demo/node_modules/core-js/internals/indexed-object.js 0000644 0001750 0000144 00000000767 03560116604 025225 0 ustar andreh users var fails = require('../internals/fails'); var classof = require('../internals/classof-raw'); var split = ''.split; // fallback for non-array-like ES3 and non-enumerable old V8 strings module.exports = fails(function () { // throws an error in rhino, see https://github.com/mozilla/rhino/issues/346 // eslint-disable-next-line no-prototype-builtins return !Object('z').propertyIsEnumerable(0); }) ? function (it) { return classof(it) == 'String' ? split.call(it, '') : Object(it); } : Object; apollo-server-demo/node_modules/core-js/internals/classof.js 0000644 0001750 0000144 00000001761 03560116604 023766 0 ustar andreh users var TO_STRING_TAG_SUPPORT = require('../internals/to-string-tag-support'); var classofRaw = require('../internals/classof-raw'); var wellKnownSymbol = require('../internals/well-known-symbol'); var TO_STRING_TAG = wellKnownSymbol('toStringTag'); // ES3 wrong here var CORRECT_ARGUMENTS = classofRaw(function () { return arguments; }()) == 'Arguments'; // fallback for IE11 Script Access Denied error var tryGet = function (it, key) { try { return it[key]; } catch (error) { /* empty */ } }; // getting tag from ES6+ `Object.prototype.toString` module.exports = TO_STRING_TAG_SUPPORT ? classofRaw : function (it) { var O, tag, result; return it === undefined ? 'Undefined' : it === null ? 'Null' // @@toStringTag case : typeof (tag = tryGet(O = Object(it), TO_STRING_TAG)) == 'string' ? tag // builtinTag case : CORRECT_ARGUMENTS ? classofRaw(O) // ES3 arguments fallback : (result = classofRaw(O)) == 'Object' && typeof O.callee == 'function' ? 'Arguments' : result; }; apollo-server-demo/node_modules/core-js/internals/array-species-create.js 0000644 0001750 0000144 00000001312 03560116604 026334 0 ustar andreh users var isObject = require('../internals/is-object'); var isArray = require('../internals/is-array'); var wellKnownSymbol = require('../internals/well-known-symbol'); var SPECIES = wellKnownSymbol('species'); // `ArraySpeciesCreate` abstract operation // https://tc39.es/ecma262/#sec-arrayspeciescreate module.exports = function (originalArray, length) { var C; if (isArray(originalArray)) { C = originalArray.constructor; // cross-realm fallback if (typeof C == 'function' && (C === Array || isArray(C.prototype))) C = undefined; else if (isObject(C)) { C = C[SPECIES]; if (C === null) C = undefined; } } return new (C === undefined ? Array : C)(length === 0 ? 0 : length); }; apollo-server-demo/node_modules/core-js/internals/freezing.js 0000644 0001750 0000144 00000000222 03560116604 024134 0 ustar andreh users var fails = require('../internals/fails'); module.exports = !fails(function () { return Object.isExtensible(Object.preventExtensions({})); }); apollo-server-demo/node_modules/core-js/internals/is-integer.js 0000644 0001750 0000144 00000000422 03560116604 024373 0 ustar andreh users var isObject = require('../internals/is-object'); var floor = Math.floor; // `Number.isInteger` method implementation // https://tc39.es/ecma262/#sec-number.isinteger module.exports = function isInteger(it) { return !isObject(it) && isFinite(it) && floor(it) === it; }; apollo-server-demo/node_modules/core-js/internals/native-symbol.js 0000644 0001750 0000144 00000000363 03560116604 025122 0 ustar andreh users var fails = require('../internals/fails'); module.exports = !!Object.getOwnPropertySymbols && !fails(function () { // Chrome 38 Symbol has incorrect toString conversion // eslint-disable-next-line no-undef return !String(Symbol()); }); apollo-server-demo/node_modules/core-js/internals/require-object-coercible.js 0000644 0001750 0000144 00000000335 03560116604 027175 0 ustar andreh users // `RequireObjectCoercible` abstract operation // https://tc39.es/ecma262/#sec-requireobjectcoercible module.exports = function (it) { if (it == undefined) throw TypeError("Can't call method on " + it); return it; }; apollo-server-demo/node_modules/core-js/internals/classof-raw.js 0000644 0001750 0000144 00000000152 03560116604 024546 0 ustar andreh users var toString = {}.toString; module.exports = function (it) { return toString.call(it).slice(8, -1); }; apollo-server-demo/node_modules/core-js/internals/regexp-exec-abstract.js 0000644 0001750 0000144 00000001117 03560116604 026344 0 ustar andreh users var classof = require('./classof-raw'); var regexpExec = require('./regexp-exec'); // `RegExpExec` abstract operation // https://tc39.es/ecma262/#sec-regexpexec module.exports = function (R, S) { var exec = R.exec; if (typeof exec === 'function') { var result = exec.call(R, S); if (typeof result !== 'object') { throw TypeError('RegExp exec method returned something other than an Object or null'); } return result; } if (classof(R) !== 'RegExp') { throw TypeError('RegExp#exec called on incompatible receiver'); } return regexpExec.call(R, S); }; apollo-server-demo/node_modules/core-js/internals/internal-metadata.js 0000644 0001750 0000144 00000003346 03560116604 025727 0 ustar andreh users var hiddenKeys = require('../internals/hidden-keys'); var isObject = require('../internals/is-object'); var has = require('../internals/has'); var defineProperty = require('../internals/object-define-property').f; var uid = require('../internals/uid'); var FREEZING = require('../internals/freezing'); var METADATA = uid('meta'); var id = 0; var isExtensible = Object.isExtensible || function () { return true; }; var setMetadata = function (it) { defineProperty(it, METADATA, { value: { objectID: 'O' + ++id, // object ID weakData: {} // weak collections IDs } }); }; var fastKey = function (it, create) { // return a primitive with prefix if (!isObject(it)) return typeof it == 'symbol' ? it : (typeof it == 'string' ? 'S' : 'P') + it; if (!has(it, METADATA)) { // can't set metadata to uncaught frozen object if (!isExtensible(it)) return 'F'; // not necessary to add metadata if (!create) return 'E'; // add missing metadata setMetadata(it); // return object ID } return it[METADATA].objectID; }; var getWeakData = function (it, create) { if (!has(it, METADATA)) { // can't set metadata to uncaught frozen object if (!isExtensible(it)) return true; // not necessary to add metadata if (!create) return false; // add missing metadata setMetadata(it); // return the store of weak collections IDs } return it[METADATA].weakData; }; // add metadata on freeze-family methods calling var onFreeze = function (it) { if (FREEZING && meta.REQUIRED && isExtensible(it) && !has(it, METADATA)) setMetadata(it); return it; }; var meta = module.exports = { REQUIRED: false, fastKey: fastKey, getWeakData: getWeakData, onFreeze: onFreeze }; hiddenKeys[METADATA] = true; apollo-server-demo/node_modules/core-js/internals/math-scale.js 0000644 0001750 0000144 00000001055 03560116604 024346 0 ustar andreh users // `Math.scale` method implementation // https://rwaldron.github.io/proposal-math-extensions/ module.exports = Math.scale || function scale(x, inLow, inHigh, outLow, outHigh) { if ( arguments.length === 0 /* eslint-disable no-self-compare */ || x != x || inLow != inLow || inHigh != inHigh || outLow != outLow || outHigh != outHigh /* eslint-enable no-self-compare */ ) return NaN; if (x === Infinity || x === -Infinity) return x; return (x - inLow) * (outHigh - outLow) / (inHigh - inLow) + outLow; }; apollo-server-demo/node_modules/core-js/internals/typed-array-constructors-require-wrappers.js 0000644 0001750 0000144 00000001534 03560116604 032654 0 ustar andreh users /* eslint-disable no-new */ var global = require('../internals/global'); var fails = require('../internals/fails'); var checkCorrectnessOfIteration = require('../internals/check-correctness-of-iteration'); var NATIVE_ARRAY_BUFFER_VIEWS = require('../internals/array-buffer-view-core').NATIVE_ARRAY_BUFFER_VIEWS; var ArrayBuffer = global.ArrayBuffer; var Int8Array = global.Int8Array; module.exports = !NATIVE_ARRAY_BUFFER_VIEWS || !fails(function () { Int8Array(1); }) || !fails(function () { new Int8Array(-1); }) || !checkCorrectnessOfIteration(function (iterable) { new Int8Array(); new Int8Array(null); new Int8Array(1.5); new Int8Array(iterable); }, true) || fails(function () { // Safari (11+) bug - a reason why even Safari 13 should load a typed array polyfill return new Int8Array(new ArrayBuffer(2), 1, undefined).length !== 1; }); apollo-server-demo/node_modules/core-js/internals/number-is-finite.js 0000644 0001750 0000144 00000000426 03560116604 025506 0 ustar andreh users var global = require('../internals/global'); var globalIsFinite = global.isFinite; // `Number.isFinite` method // https://tc39.es/ecma262/#sec-number.isfinite module.exports = Number.isFinite || function isFinite(it) { return typeof it == 'number' && globalIsFinite(it); }; apollo-server-demo/node_modules/core-js/internals/string-repeat.js 0000644 0001750 0000144 00000001075 03560116604 025116 0 ustar andreh users 'use strict'; var toInteger = require('../internals/to-integer'); var requireObjectCoercible = require('../internals/require-object-coercible'); // `String.prototype.repeat` method implementation // https://tc39.es/ecma262/#sec-string.prototype.repeat module.exports = ''.repeat || function repeat(count) { var str = String(requireObjectCoercible(this)); var result = ''; var n = toInteger(count); if (n < 0 || n == Infinity) throw RangeError('Wrong number of repetitions'); for (;n > 0; (n >>>= 1) && (str += str)) if (n & 1) result += str; return result; }; apollo-server-demo/node_modules/core-js/internals/regexp-exec.js 0000644 0001750 0000144 00000005400 03560116604 024542 0 ustar andreh users 'use strict'; var regexpFlags = require('./regexp-flags'); var stickyHelpers = require('./regexp-sticky-helpers'); var nativeExec = RegExp.prototype.exec; // This always refers to the native implementation, because the // String#replace polyfill uses ./fix-regexp-well-known-symbol-logic.js, // which loads this file before patching the method. var nativeReplace = String.prototype.replace; var patchedExec = nativeExec; var UPDATES_LAST_INDEX_WRONG = (function () { var re1 = /a/; var re2 = /b*/g; nativeExec.call(re1, 'a'); nativeExec.call(re2, 'a'); return re1.lastIndex !== 0 || re2.lastIndex !== 0; })(); var UNSUPPORTED_Y = stickyHelpers.UNSUPPORTED_Y || stickyHelpers.BROKEN_CARET; // nonparticipating capturing group, copied from es5-shim's String#split patch. var NPCG_INCLUDED = /()??/.exec('')[1] !== undefined; var PATCH = UPDATES_LAST_INDEX_WRONG || NPCG_INCLUDED || UNSUPPORTED_Y; if (PATCH) { patchedExec = function exec(str) { var re = this; var lastIndex, reCopy, match, i; var sticky = UNSUPPORTED_Y && re.sticky; var flags = regexpFlags.call(re); var source = re.source; var charsAdded = 0; var strCopy = str; if (sticky) { flags = flags.replace('y', ''); if (flags.indexOf('g') === -1) { flags += 'g'; } strCopy = String(str).slice(re.lastIndex); // Support anchored sticky behavior. if (re.lastIndex > 0 && (!re.multiline || re.multiline && str[re.lastIndex - 1] !== '\n')) { source = '(?: ' + source + ')'; strCopy = ' ' + strCopy; charsAdded++; } // ^(? + rx + ) is needed, in combination with some str slicing, to // simulate the 'y' flag. reCopy = new RegExp('^(?:' + source + ')', flags); } if (NPCG_INCLUDED) { reCopy = new RegExp('^' + source + '$(?!\\s)', flags); } if (UPDATES_LAST_INDEX_WRONG) lastIndex = re.lastIndex; match = nativeExec.call(sticky ? reCopy : re, strCopy); if (sticky) { if (match) { match.input = match.input.slice(charsAdded); match[0] = match[0].slice(charsAdded); match.index = re.lastIndex; re.lastIndex += match[0].length; } else re.lastIndex = 0; } else if (UPDATES_LAST_INDEX_WRONG && match) { re.lastIndex = re.global ? match.index + match[0].length : lastIndex; } if (NPCG_INCLUDED && match && match.length > 1) { // Fix browsers whose `exec` methods don't consistently return `undefined` // for NPCG, like IE8. NOTE: This doesn' work for /(.?)?/ nativeReplace.call(match[0], reCopy, function () { for (i = 1; i < arguments.length - 2; i++) { if (arguments[i] === undefined) match[i] = undefined; } }); } return match; }; } module.exports = patchedExec; apollo-server-demo/node_modules/core-js/internals/array-buffer-view-core.js 0000644 0001750 0000144 00000014176 03560116604 026623 0 ustar andreh users 'use strict'; var NATIVE_ARRAY_BUFFER = require('../internals/array-buffer-native'); var DESCRIPTORS = require('../internals/descriptors'); var global = require('../internals/global'); var isObject = require('../internals/is-object'); var has = require('../internals/has'); var classof = require('../internals/classof'); var createNonEnumerableProperty = require('../internals/create-non-enumerable-property'); var redefine = require('../internals/redefine'); var defineProperty = require('../internals/object-define-property').f; var getPrototypeOf = require('../internals/object-get-prototype-of'); var setPrototypeOf = require('../internals/object-set-prototype-of'); var wellKnownSymbol = require('../internals/well-known-symbol'); var uid = require('../internals/uid'); var Int8Array = global.Int8Array; var Int8ArrayPrototype = Int8Array && Int8Array.prototype; var Uint8ClampedArray = global.Uint8ClampedArray; var Uint8ClampedArrayPrototype = Uint8ClampedArray && Uint8ClampedArray.prototype; var TypedArray = Int8Array && getPrototypeOf(Int8Array); var TypedArrayPrototype = Int8ArrayPrototype && getPrototypeOf(Int8ArrayPrototype); var ObjectPrototype = Object.prototype; var isPrototypeOf = ObjectPrototype.isPrototypeOf; var TO_STRING_TAG = wellKnownSymbol('toStringTag'); var TYPED_ARRAY_TAG = uid('TYPED_ARRAY_TAG'); // Fixing native typed arrays in Opera Presto crashes the browser, see #595 var NATIVE_ARRAY_BUFFER_VIEWS = NATIVE_ARRAY_BUFFER && !!setPrototypeOf && classof(global.opera) !== 'Opera'; var TYPED_ARRAY_TAG_REQIRED = false; var NAME; var TypedArrayConstructorsList = { Int8Array: 1, Uint8Array: 1, Uint8ClampedArray: 1, Int16Array: 2, Uint16Array: 2, Int32Array: 4, Uint32Array: 4, Float32Array: 4, Float64Array: 8 }; var BigIntArrayConstructorsList = { BigInt64Array: 8, BigUint64Array: 8 }; var isView = function isView(it) { if (!isObject(it)) return false; var klass = classof(it); return klass === 'DataView' || has(TypedArrayConstructorsList, klass) || has(BigIntArrayConstructorsList, klass); }; var isTypedArray = function (it) { if (!isObject(it)) return false; var klass = classof(it); return has(TypedArrayConstructorsList, klass) || has(BigIntArrayConstructorsList, klass); }; var aTypedArray = function (it) { if (isTypedArray(it)) return it; throw TypeError('Target is not a typed array'); }; var aTypedArrayConstructor = function (C) { if (setPrototypeOf) { if (isPrototypeOf.call(TypedArray, C)) return C; } else for (var ARRAY in TypedArrayConstructorsList) if (has(TypedArrayConstructorsList, NAME)) { var TypedArrayConstructor = global[ARRAY]; if (TypedArrayConstructor && (C === TypedArrayConstructor || isPrototypeOf.call(TypedArrayConstructor, C))) { return C; } } throw TypeError('Target is not a typed array constructor'); }; var exportTypedArrayMethod = function (KEY, property, forced) { if (!DESCRIPTORS) return; if (forced) for (var ARRAY in TypedArrayConstructorsList) { var TypedArrayConstructor = global[ARRAY]; if (TypedArrayConstructor && has(TypedArrayConstructor.prototype, KEY)) { delete TypedArrayConstructor.prototype[KEY]; } } if (!TypedArrayPrototype[KEY] || forced) { redefine(TypedArrayPrototype, KEY, forced ? property : NATIVE_ARRAY_BUFFER_VIEWS && Int8ArrayPrototype[KEY] || property); } }; var exportTypedArrayStaticMethod = function (KEY, property, forced) { var ARRAY, TypedArrayConstructor; if (!DESCRIPTORS) return; if (setPrototypeOf) { if (forced) for (ARRAY in TypedArrayConstructorsList) { TypedArrayConstructor = global[ARRAY]; if (TypedArrayConstructor && has(TypedArrayConstructor, KEY)) { delete TypedArrayConstructor[KEY]; } } if (!TypedArray[KEY] || forced) { // V8 ~ Chrome 49-50 `%TypedArray%` methods are non-writable non-configurable try { return redefine(TypedArray, KEY, forced ? property : NATIVE_ARRAY_BUFFER_VIEWS && Int8Array[KEY] || property); } catch (error) { /* empty */ } } else return; } for (ARRAY in TypedArrayConstructorsList) { TypedArrayConstructor = global[ARRAY]; if (TypedArrayConstructor && (!TypedArrayConstructor[KEY] || forced)) { redefine(TypedArrayConstructor, KEY, property); } } }; for (NAME in TypedArrayConstructorsList) { if (!global[NAME]) NATIVE_ARRAY_BUFFER_VIEWS = false; } // WebKit bug - typed arrays constructors prototype is Object.prototype if (!NATIVE_ARRAY_BUFFER_VIEWS || typeof TypedArray != 'function' || TypedArray === Function.prototype) { // eslint-disable-next-line no-shadow TypedArray = function TypedArray() { throw TypeError('Incorrect invocation'); }; if (NATIVE_ARRAY_BUFFER_VIEWS) for (NAME in TypedArrayConstructorsList) { if (global[NAME]) setPrototypeOf(global[NAME], TypedArray); } } if (!NATIVE_ARRAY_BUFFER_VIEWS || !TypedArrayPrototype || TypedArrayPrototype === ObjectPrototype) { TypedArrayPrototype = TypedArray.prototype; if (NATIVE_ARRAY_BUFFER_VIEWS) for (NAME in TypedArrayConstructorsList) { if (global[NAME]) setPrototypeOf(global[NAME].prototype, TypedArrayPrototype); } } // WebKit bug - one more object in Uint8ClampedArray prototype chain if (NATIVE_ARRAY_BUFFER_VIEWS && getPrototypeOf(Uint8ClampedArrayPrototype) !== TypedArrayPrototype) { setPrototypeOf(Uint8ClampedArrayPrototype, TypedArrayPrototype); } if (DESCRIPTORS && !has(TypedArrayPrototype, TO_STRING_TAG)) { TYPED_ARRAY_TAG_REQIRED = true; defineProperty(TypedArrayPrototype, TO_STRING_TAG, { get: function () { return isObject(this) ? this[TYPED_ARRAY_TAG] : undefined; } }); for (NAME in TypedArrayConstructorsList) if (global[NAME]) { createNonEnumerableProperty(global[NAME], TYPED_ARRAY_TAG, NAME); } } module.exports = { NATIVE_ARRAY_BUFFER_VIEWS: NATIVE_ARRAY_BUFFER_VIEWS, TYPED_ARRAY_TAG: TYPED_ARRAY_TAG_REQIRED && TYPED_ARRAY_TAG, aTypedArray: aTypedArray, aTypedArrayConstructor: aTypedArrayConstructor, exportTypedArrayMethod: exportTypedArrayMethod, exportTypedArrayStaticMethod: exportTypedArrayStaticMethod, isView: isView, isTypedArray: isTypedArray, TypedArray: TypedArray, TypedArrayPrototype: TypedArrayPrototype }; apollo-server-demo/node_modules/core-js/internals/shared-key.js 0000644 0001750 0000144 00000000304 03560116604 024360 0 ustar andreh users var shared = require('../internals/shared'); var uid = require('../internals/uid'); var keys = shared('keys'); module.exports = function (key) { return keys[key] || (keys[key] = uid(key)); }; apollo-server-demo/node_modules/core-js/internals/is-array-iterator-method.js 0000644 0001750 0000144 00000000551 03560116604 027164 0 ustar andreh users var wellKnownSymbol = require('../internals/well-known-symbol'); var Iterators = require('../internals/iterators'); var ITERATOR = wellKnownSymbol('iterator'); var ArrayPrototype = Array.prototype; // check on default Array iterator module.exports = function (it) { return it !== undefined && (Iterators.Array === it || ArrayPrototype[ITERATOR] === it); }; apollo-server-demo/node_modules/core-js/internals/microtask.js 0000644 0001750 0000144 00000005157 03560116604 024333 0 ustar andreh users var global = require('../internals/global'); var getOwnPropertyDescriptor = require('../internals/object-get-own-property-descriptor').f; var macrotask = require('../internals/task').set; var IS_IOS = require('../internals/engine-is-ios'); var IS_WEBOS_WEBKIT = require('../internals/engine-is-webos-webkit'); var IS_NODE = require('../internals/engine-is-node'); var MutationObserver = global.MutationObserver || global.WebKitMutationObserver; var document = global.document; var process = global.process; var Promise = global.Promise; // Node.js 11 shows ExperimentalWarning on getting `queueMicrotask` var queueMicrotaskDescriptor = getOwnPropertyDescriptor(global, 'queueMicrotask'); var queueMicrotask = queueMicrotaskDescriptor && queueMicrotaskDescriptor.value; var flush, head, last, notify, toggle, node, promise, then; // modern engines have queueMicrotask method if (!queueMicrotask) { flush = function () { var parent, fn; if (IS_NODE && (parent = process.domain)) parent.exit(); while (head) { fn = head.fn; head = head.next; try { fn(); } catch (error) { if (head) notify(); else last = undefined; throw error; } } last = undefined; if (parent) parent.enter(); }; // browsers with MutationObserver, except iOS - https://github.com/zloirock/core-js/issues/339 // also except WebOS Webkit https://github.com/zloirock/core-js/issues/898 if (!IS_IOS && !IS_NODE && !IS_WEBOS_WEBKIT && MutationObserver && document) { toggle = true; node = document.createTextNode(''); new MutationObserver(flush).observe(node, { characterData: true }); notify = function () { node.data = toggle = !toggle; }; // environments with maybe non-completely correct, but existent Promise } else if (Promise && Promise.resolve) { // Promise.resolve without an argument throws an error in LG WebOS 2 promise = Promise.resolve(undefined); then = promise.then; notify = function () { then.call(promise, flush); }; // Node.js without promises } else if (IS_NODE) { notify = function () { process.nextTick(flush); }; // for other environments - macrotask based on: // - setImmediate // - MessageChannel // - window.postMessag // - onreadystatechange // - setTimeout } else { notify = function () { // strange IE + webpack dev server bug - use .call(global) macrotask.call(global, flush); }; } } module.exports = queueMicrotask || function (fn) { var task = { fn: fn, next: undefined }; if (last) last.next = task; if (!head) { head = task; notify(); } last = task; }; apollo-server-demo/node_modules/core-js/internals/perform.js 0000644 0001750 0000144 00000000234 03560116604 024000 0 ustar andreh users module.exports = function (exec) { try { return { error: false, value: exec() }; } catch (error) { return { error: true, value: error }; } }; apollo-server-demo/node_modules/core-js/internals/task.js 0000644 0001750 0000144 00000005630 03560116604 023275 0 ustar andreh users var global = require('../internals/global'); var fails = require('../internals/fails'); var bind = require('../internals/function-bind-context'); var html = require('../internals/html'); var createElement = require('../internals/document-create-element'); var IS_IOS = require('../internals/engine-is-ios'); var IS_NODE = require('../internals/engine-is-node'); var location = global.location; var set = global.setImmediate; var clear = global.clearImmediate; var process = global.process; var MessageChannel = global.MessageChannel; var Dispatch = global.Dispatch; var counter = 0; var queue = {}; var ONREADYSTATECHANGE = 'onreadystatechange'; var defer, channel, port; var run = function (id) { // eslint-disable-next-line no-prototype-builtins if (queue.hasOwnProperty(id)) { var fn = queue[id]; delete queue[id]; fn(); } }; var runner = function (id) { return function () { run(id); }; }; var listener = function (event) { run(event.data); }; var post = function (id) { // old engines have not location.origin global.postMessage(id + '', location.protocol + '//' + location.host); }; // Node.js 0.9+ & IE10+ has setImmediate, otherwise: if (!set || !clear) { set = function setImmediate(fn) { var args = []; var i = 1; while (arguments.length > i) args.push(arguments[i++]); queue[++counter] = function () { // eslint-disable-next-line no-new-func (typeof fn == 'function' ? fn : Function(fn)).apply(undefined, args); }; defer(counter); return counter; }; clear = function clearImmediate(id) { delete queue[id]; }; // Node.js 0.8- if (IS_NODE) { defer = function (id) { process.nextTick(runner(id)); }; // Sphere (JS game engine) Dispatch API } else if (Dispatch && Dispatch.now) { defer = function (id) { Dispatch.now(runner(id)); }; // Browsers with MessageChannel, includes WebWorkers // except iOS - https://github.com/zloirock/core-js/issues/624 } else if (MessageChannel && !IS_IOS) { channel = new MessageChannel(); port = channel.port2; channel.port1.onmessage = listener; defer = bind(port.postMessage, port, 1); // Browsers with postMessage, skip WebWorkers // IE8 has postMessage, but it's sync & typeof its postMessage is 'object' } else if ( global.addEventListener && typeof postMessage == 'function' && !global.importScripts && location && location.protocol !== 'file:' && !fails(post) ) { defer = post; global.addEventListener('message', listener, false); // IE8- } else if (ONREADYSTATECHANGE in createElement('script')) { defer = function (id) { html.appendChild(createElement('script'))[ONREADYSTATECHANGE] = function () { html.removeChild(this); run(id); }; }; // Rest old browsers } else { defer = function (id) { setTimeout(runner(id), 0); }; } } module.exports = { set: set, clear: clear }; apollo-server-demo/node_modules/core-js/internals/math-expm1.js 0000644 0001750 0000144 00000000673 03560116604 024316 0 ustar andreh users var nativeExpm1 = Math.expm1; var exp = Math.exp; // `Math.expm1` method implementation // https://tc39.es/ecma262/#sec-math.expm1 module.exports = (!nativeExpm1 // Old FF bug || nativeExpm1(10) > 22025.465794806719 || nativeExpm1(10) < 22025.4657948067165168 // Tor Browser bug || nativeExpm1(-2e-17) != -2e-17 ) ? function expm1(x) { return (x = +x) == 0 ? x : x > -1e-6 && x < 1e-6 ? x + x * x / 2 : exp(x) - 1; } : nativeExpm1; apollo-server-demo/node_modules/core-js/internals/object-get-own-property-names-external.js 0000644 0001750 0000144 00000001341 03560116604 031755 0 ustar andreh users var toIndexedObject = require('../internals/to-indexed-object'); var nativeGetOwnPropertyNames = require('../internals/object-get-own-property-names').f; var toString = {}.toString; var windowNames = typeof window == 'object' && window && Object.getOwnPropertyNames ? Object.getOwnPropertyNames(window) : []; var getWindowNames = function (it) { try { return nativeGetOwnPropertyNames(it); } catch (error) { return windowNames.slice(); } }; // fallback for IE11 buggy Object.getOwnPropertyNames with iframe and window module.exports.f = function getOwnPropertyNames(it) { return windowNames && toString.call(it) == '[object Window]' ? getWindowNames(it) : nativeGetOwnPropertyNames(toIndexedObject(it)); }; apollo-server-demo/node_modules/core-js/internals/native-promise-constructor.js 0000644 0001750 0000144 00000000117 03560116604 027653 0 ustar andreh users var global = require('../internals/global'); module.exports = global.Promise; apollo-server-demo/node_modules/core-js/internals/to-index.js 0000644 0001750 0000144 00000000611 03560116604 024054 0 ustar andreh users var toInteger = require('../internals/to-integer'); var toLength = require('../internals/to-length'); // `ToIndex` abstract operation // https://tc39.es/ecma262/#sec-toindex module.exports = function (it) { if (it === undefined) return 0; var number = toInteger(it); var length = toLength(number); if (number !== length) throw RangeError('Wrong length or index'); return length; }; apollo-server-demo/node_modules/core-js/internals/whitespaces.js 0000644 0001750 0000144 00000000374 03560116604 024652 0 ustar andreh users // a string of all valid unicode whitespaces // eslint-disable-next-line max-len module.exports = '\u0009\u000A\u000B\u000C\u000D\u0020\u00A0\u1680\u2000\u2001\u2002\u2003\u2004\u2005\u2006\u2007\u2008\u2009\u200A\u202F\u205F\u3000\u2028\u2029\uFEFF'; apollo-server-demo/node_modules/core-js/internals/array-method-is-strict.js 0000644 0001750 0000144 00000000501 03560116604 026636 0 ustar andreh users 'use strict'; var fails = require('../internals/fails'); module.exports = function (METHOD_NAME, argument) { var method = [][METHOD_NAME]; return !!method && fails(function () { // eslint-disable-next-line no-useless-call,no-throw-literal method.call(null, argument || function () { throw 1; }, 1); }); }; apollo-server-demo/node_modules/core-js/internals/date-to-primitive.js 0000644 0001750 0000144 00000000507 03560116604 025674 0 ustar andreh users 'use strict'; var anObject = require('../internals/an-object'); var toPrimitive = require('../internals/to-primitive'); module.exports = function (hint) { if (hint !== 'string' && hint !== 'number' && hint !== 'default') { throw TypeError('Incorrect hint'); } return toPrimitive(anObject(this), hint !== 'number'); }; apollo-server-demo/node_modules/core-js/internals/math-sign.js 0000644 0001750 0000144 00000000350 03560116604 024214 0 ustar andreh users // `Math.sign` method implementation // https://tc39.es/ecma262/#sec-math.sign module.exports = Math.sign || function sign(x) { // eslint-disable-next-line no-self-compare return (x = +x) == 0 || x != x ? x : x < 0 ? -1 : 1; }; apollo-server-demo/node_modules/core-js/internals/flatten-into-array.js 0000644 0001750 0000144 00000002124 03560116604 026046 0 ustar andreh users 'use strict'; var isArray = require('../internals/is-array'); var toLength = require('../internals/to-length'); var bind = require('../internals/function-bind-context'); // `FlattenIntoArray` abstract operation // https://tc39.github.io/proposal-flatMap/#sec-FlattenIntoArray var flattenIntoArray = function (target, original, source, sourceLen, start, depth, mapper, thisArg) { var targetIndex = start; var sourceIndex = 0; var mapFn = mapper ? bind(mapper, thisArg, 3) : false; var element; while (sourceIndex < sourceLen) { if (sourceIndex in source) { element = mapFn ? mapFn(source[sourceIndex], sourceIndex, original) : source[sourceIndex]; if (depth > 0 && isArray(element)) { targetIndex = flattenIntoArray(target, original, element, toLength(element.length), targetIndex, depth - 1) - 1; } else { if (targetIndex >= 0x1FFFFFFFFFFFFF) throw TypeError('Exceed the acceptable array length'); target[targetIndex] = element; } targetIndex++; } sourceIndex++; } return targetIndex; }; module.exports = flattenIntoArray; apollo-server-demo/node_modules/core-js/internals/regexp-flags.js 0000644 0001750 0000144 00000000745 03560116604 024721 0 ustar andreh users 'use strict'; var anObject = require('../internals/an-object'); // `RegExp.prototype.flags` getter implementation // https://tc39.es/ecma262/#sec-get-regexp.prototype.flags module.exports = function () { var that = anObject(this); var result = ''; if (that.global) result += 'g'; if (that.ignoreCase) result += 'i'; if (that.multiline) result += 'm'; if (that.dotAll) result += 's'; if (that.unicode) result += 'u'; if (that.sticky) result += 'y'; return result; }; apollo-server-demo/node_modules/core-js/internals/string-trim.js 0000644 0001750 0000144 00000002042 03560116604 024604 0 ustar andreh users var requireObjectCoercible = require('../internals/require-object-coercible'); var whitespaces = require('../internals/whitespaces'); var whitespace = '[' + whitespaces + ']'; var ltrim = RegExp('^' + whitespace + whitespace + '*'); var rtrim = RegExp(whitespace + whitespace + '*$'); // `String.prototype.{ trim, trimStart, trimEnd, trimLeft, trimRight }` methods implementation var createMethod = function (TYPE) { return function ($this) { var string = String(requireObjectCoercible($this)); if (TYPE & 1) string = string.replace(ltrim, ''); if (TYPE & 2) string = string.replace(rtrim, ''); return string; }; }; module.exports = { // `String.prototype.{ trimLeft, trimStart }` methods // https://tc39.es/ecma262/#sec-string.prototype.trimstart start: createMethod(1), // `String.prototype.{ trimRight, trimEnd }` methods // https://tc39.es/ecma262/#sec-string.prototype.trimend end: createMethod(2), // `String.prototype.trim` method // https://tc39.es/ecma262/#sec-string.prototype.trim trim: createMethod(3) }; apollo-server-demo/node_modules/core-js/internals/iterators.js 0000644 0001750 0000144 00000000025 03560116604 024340 0 ustar andreh users module.exports = {}; apollo-server-demo/node_modules/core-js/internals/array-method-has-species-support.js 0000644 0001750 0000144 00000001241 03560116604 030635 0 ustar andreh users var fails = require('../internals/fails'); var wellKnownSymbol = require('../internals/well-known-symbol'); var V8_VERSION = require('../internals/engine-v8-version'); var SPECIES = wellKnownSymbol('species'); module.exports = function (METHOD_NAME) { // We can't use this feature detection in V8 since it causes // deoptimization and serious performance degradation // https://github.com/zloirock/core-js/issues/677 return V8_VERSION >= 51 || !fails(function () { var array = []; var constructor = array.constructor = {}; constructor[SPECIES] = function () { return { foo: 1 }; }; return array[METHOD_NAME](Boolean).foo !== 1; }); }; apollo-server-demo/node_modules/core-js/internals/is-array.js 0000644 0001750 0000144 00000000333 03560116604 024055 0 ustar andreh users var classof = require('../internals/classof-raw'); // `IsArray` abstract operation // https://tc39.es/ecma262/#sec-isarray module.exports = Array.isArray || function isArray(arg) { return classof(arg) == 'Array'; }; apollo-server-demo/node_modules/core-js/internals/a-function.js 0000644 0001750 0000144 00000000214 03560116604 024367 0 ustar andreh users module.exports = function (it) { if (typeof it != 'function') { throw TypeError(String(it) + ' is not a function'); } return it; }; apollo-server-demo/node_modules/core-js/internals/engine-user-agent.js 0000644 0001750 0000144 00000000165 03560116604 025646 0 ustar andreh users var getBuiltIn = require('../internals/get-built-in'); module.exports = getBuiltIn('navigator', 'userAgent') || ''; apollo-server-demo/node_modules/core-js/internals/string-trim-forced.js 0000644 0001750 0000144 00000000647 03560116604 026055 0 ustar andreh users var fails = require('../internals/fails'); var whitespaces = require('../internals/whitespaces'); var non = '\u200B\u0085\u180E'; // check that a method works with the correct list // of whitespaces and has a correct name module.exports = function (METHOD_NAME) { return fails(function () { return !!whitespaces[METHOD_NAME]() || non[METHOD_NAME]() != non || whitespaces[METHOD_NAME].name !== METHOD_NAME; }); }; apollo-server-demo/node_modules/core-js/internals/create-html.js 0000644 0001750 0000144 00000000731 03560116604 024535 0 ustar andreh users var requireObjectCoercible = require('../internals/require-object-coercible'); var quot = /"/g; // B.2.3.2.1 CreateHTML(string, tag, attribute, value) // https://tc39.es/ecma262/#sec-createhtml module.exports = function (string, tag, attribute, value) { var S = String(requireObjectCoercible(string)); var p1 = '<' + tag; if (attribute !== '') p1 += ' ' + attribute + '="' + String(value).replace(quot, '"') + '"'; return p1 + '>' + S + '</' + tag + '>'; }; apollo-server-demo/node_modules/core-js/internals/get-iterator.js 0000644 0001750 0000144 00000000533 03560116604 024736 0 ustar andreh users var anObject = require('../internals/an-object'); var getIteratorMethod = require('../internals/get-iterator-method'); module.exports = function (it) { var iteratorMethod = getIteratorMethod(it); if (typeof iteratorMethod != 'function') { throw TypeError(String(it) + ' is not iterable'); } return anObject(iteratorMethod.call(it)); }; apollo-server-demo/node_modules/core-js/internals/fails.js 0000644 0001750 0000144 00000000154 03560116604 023425 0 ustar andreh users module.exports = function (exec) { try { return !!exec(); } catch (error) { return true; } }; apollo-server-demo/node_modules/core-js/internals/fix-regexp-well-known-symbol-logic.js 0000644 0001750 0000144 00000011067 03560116604 031103 0 ustar andreh users 'use strict'; // TODO: Remove from `core-js@4` since it's moved to entry points require('../modules/es.regexp.exec'); var redefine = require('../internals/redefine'); var fails = require('../internals/fails'); var wellKnownSymbol = require('../internals/well-known-symbol'); var regexpExec = require('../internals/regexp-exec'); var createNonEnumerableProperty = require('../internals/create-non-enumerable-property'); var SPECIES = wellKnownSymbol('species'); var REPLACE_SUPPORTS_NAMED_GROUPS = !fails(function () { // #replace needs built-in support for named groups. // #match works fine because it just return the exec results, even if it has // a "grops" property. var re = /./; re.exec = function () { var result = []; result.groups = { a: '7' }; return result; }; return ''.replace(re, '$<a>') !== '7'; }); // IE <= 11 replaces $0 with the whole match, as if it was $& // https://stackoverflow.com/questions/6024666/getting-ie-to-replace-a-regex-with-the-literal-string-0 var REPLACE_KEEPS_$0 = (function () { return 'a'.replace(/./, '$0') === '$0'; })(); var REPLACE = wellKnownSymbol('replace'); // Safari <= 13.0.3(?) substitutes nth capture where n>m with an empty string var REGEXP_REPLACE_SUBSTITUTES_UNDEFINED_CAPTURE = (function () { if (/./[REPLACE]) { return /./[REPLACE]('a', '$0') === ''; } return false; })(); // Chrome 51 has a buggy "split" implementation when RegExp#exec !== nativeExec // Weex JS has frozen built-in prototypes, so use try / catch wrapper var SPLIT_WORKS_WITH_OVERWRITTEN_EXEC = !fails(function () { var re = /(?:)/; var originalExec = re.exec; re.exec = function () { return originalExec.apply(this, arguments); }; var result = 'ab'.split(re); return result.length !== 2 || result[0] !== 'a' || result[1] !== 'b'; }); module.exports = function (KEY, length, exec, sham) { var SYMBOL = wellKnownSymbol(KEY); var DELEGATES_TO_SYMBOL = !fails(function () { // String methods call symbol-named RegEp methods var O = {}; O[SYMBOL] = function () { return 7; }; return ''[KEY](O) != 7; }); var DELEGATES_TO_EXEC = DELEGATES_TO_SYMBOL && !fails(function () { // Symbol-named RegExp methods call .exec var execCalled = false; var re = /a/; if (KEY === 'split') { // We can't use real regex here since it causes deoptimization // and serious performance degradation in V8 // https://github.com/zloirock/core-js/issues/306 re = {}; // RegExp[@@split] doesn't call the regex's exec method, but first creates // a new one. We need to return the patched regex when creating the new one. re.constructor = {}; re.constructor[SPECIES] = function () { return re; }; re.flags = ''; re[SYMBOL] = /./[SYMBOL]; } re.exec = function () { execCalled = true; return null; }; re[SYMBOL](''); return !execCalled; }); if ( !DELEGATES_TO_SYMBOL || !DELEGATES_TO_EXEC || (KEY === 'replace' && !( REPLACE_SUPPORTS_NAMED_GROUPS && REPLACE_KEEPS_$0 && !REGEXP_REPLACE_SUBSTITUTES_UNDEFINED_CAPTURE )) || (KEY === 'split' && !SPLIT_WORKS_WITH_OVERWRITTEN_EXEC) ) { var nativeRegExpMethod = /./[SYMBOL]; var methods = exec(SYMBOL, ''[KEY], function (nativeMethod, regexp, str, arg2, forceStringMethod) { if (regexp.exec === regexpExec) { if (DELEGATES_TO_SYMBOL && !forceStringMethod) { // The native String method already delegates to @@method (this // polyfilled function), leasing to infinite recursion. // We avoid it by directly calling the native @@method method. return { done: true, value: nativeRegExpMethod.call(regexp, str, arg2) }; } return { done: true, value: nativeMethod.call(str, regexp, arg2) }; } return { done: false }; }, { REPLACE_KEEPS_$0: REPLACE_KEEPS_$0, REGEXP_REPLACE_SUBSTITUTES_UNDEFINED_CAPTURE: REGEXP_REPLACE_SUBSTITUTES_UNDEFINED_CAPTURE }); var stringMethod = methods[0]; var regexMethod = methods[1]; redefine(String.prototype, KEY, stringMethod); redefine(RegExp.prototype, SYMBOL, length == 2 // 21.2.5.8 RegExp.prototype[@@replace](string, replaceValue) // 21.2.5.11 RegExp.prototype[@@split](string, limit) ? function (string, arg) { return regexMethod.call(string, this, arg); } // 21.2.5.6 RegExp.prototype[@@match](string) // 21.2.5.9 RegExp.prototype[@@search](string) : function (string) { return regexMethod.call(string, this); } ); } if (sham) createNonEnumerableProperty(RegExp.prototype[SYMBOL], 'sham', true); }; apollo-server-demo/node_modules/core-js/internals/well-known-symbol.js 0000644 0001750 0000144 00000001360 03560116604 025727 0 ustar andreh users var global = require('../internals/global'); var shared = require('../internals/shared'); var has = require('../internals/has'); var uid = require('../internals/uid'); var NATIVE_SYMBOL = require('../internals/native-symbol'); var USE_SYMBOL_AS_UID = require('../internals/use-symbol-as-uid'); var WellKnownSymbolsStore = shared('wks'); var Symbol = global.Symbol; var createWellKnownSymbol = USE_SYMBOL_AS_UID ? Symbol : Symbol && Symbol.withoutSetter || uid; module.exports = function (name) { if (!has(WellKnownSymbolsStore, name)) { if (NATIVE_SYMBOL && has(Symbol, name)) WellKnownSymbolsStore[name] = Symbol[name]; else WellKnownSymbolsStore[name] = createWellKnownSymbol('Symbol.' + name); } return WellKnownSymbolsStore[name]; }; apollo-server-demo/node_modules/core-js/internals/get-built-in.js 0000644 0001750 0000144 00000000662 03560116604 024633 0 ustar andreh users var path = require('../internals/path'); var global = require('../internals/global'); var aFunction = function (variable) { return typeof variable == 'function' ? variable : undefined; }; module.exports = function (namespace, method) { return arguments.length < 2 ? aFunction(path[namespace]) || aFunction(global[namespace]) : path[namespace] && path[namespace][method] || global[namespace] && global[namespace][method]; }; apollo-server-demo/node_modules/core-js/internals/string-pad-webkit-bug.js 0000644 0001750 0000144 00000000367 03560116604 026443 0 ustar andreh users // https://github.com/zloirock/core-js/issues/280 var userAgent = require('../internals/engine-user-agent'); // eslint-disable-next-line unicorn/no-unsafe-regex module.exports = /Version\/10\.\d+(\.\d+)?( Mobile\/\w+)? Safari\//.test(userAgent); apollo-server-demo/node_modules/core-js/internals/get-async-iterator-method.js 0000644 0001750 0000144 00000000506 03560116604 027327 0 ustar andreh users var getIteratorMethod = require('../internals/get-iterator-method'); var wellKnownSymbol = require('../internals/well-known-symbol'); var ASYNC_ITERATOR = wellKnownSymbol('asyncIterator'); module.exports = function (it) { var method = it[ASYNC_ITERATOR]; return method === undefined ? getIteratorMethod(it) : method; }; apollo-server-demo/node_modules/core-js/internals/to-integer.js 0000644 0001750 0000144 00000000371 03560116604 024405 0 ustar andreh users var ceil = Math.ceil; var floor = Math.floor; // `ToInteger` abstract operation // https://tc39.es/ecma262/#sec-tointeger module.exports = function (argument) { return isNaN(argument = +argument) ? 0 : (argument > 0 ? floor : ceil)(argument); }; apollo-server-demo/node_modules/core-js/internals/collection-add-all.js 0000644 0001750 0000144 00000000603 03560116604 025755 0 ustar andreh users 'use strict'; var anObject = require('../internals/an-object'); var aFunction = require('../internals/a-function'); // https://github.com/tc39/collection-methods module.exports = function (/* ...elements */) { var set = anObject(this); var adder = aFunction(set.add); for (var k = 0, len = arguments.length; k < len; k++) { adder.call(set, arguments[k]); } return set; }; apollo-server-demo/node_modules/core-js/internals/a-possible-prototype.js 0000644 0001750 0000144 00000000320 03560116604 026423 0 ustar andreh users var isObject = require('../internals/is-object'); module.exports = function (it) { if (!isObject(it) && it !== null) { throw TypeError("Can't set " + String(it) + ' as a prototype'); } return it; }; apollo-server-demo/node_modules/core-js/internals/use-symbol-as-uid.js 0000644 0001750 0000144 00000000344 03560116604 025607 0 ustar andreh users var NATIVE_SYMBOL = require('../internals/native-symbol'); module.exports = NATIVE_SYMBOL // eslint-disable-next-line no-undef && !Symbol.sham // eslint-disable-next-line no-undef && typeof Symbol.iterator == 'symbol'; apollo-server-demo/node_modules/core-js/internals/engine-is-webos-webkit.js 0000644 0001750 0000144 00000000163 03560116604 026605 0 ustar andreh users var userAgent = require('../internals/engine-user-agent'); module.exports = /web0s(?!.*chrome)/i.test(userAgent); apollo-server-demo/node_modules/core-js/internals/object-to-array.js 0000644 0001750 0000144 00000001714 03560116604 025334 0 ustar andreh users var DESCRIPTORS = require('../internals/descriptors'); var objectKeys = require('../internals/object-keys'); var toIndexedObject = require('../internals/to-indexed-object'); var propertyIsEnumerable = require('../internals/object-property-is-enumerable').f; // `Object.{ entries, values }` methods implementation var createMethod = function (TO_ENTRIES) { return function (it) { var O = toIndexedObject(it); var keys = objectKeys(O); var length = keys.length; var i = 0; var result = []; var key; while (length > i) { key = keys[i++]; if (!DESCRIPTORS || propertyIsEnumerable.call(O, key)) { result.push(TO_ENTRIES ? [key, O[key]] : O[key]); } } return result; }; }; module.exports = { // `Object.entries` method // https://tc39.es/ecma262/#sec-object.entries entries: createMethod(true), // `Object.values` method // https://tc39.es/ecma262/#sec-object.values values: createMethod(false) }; apollo-server-demo/node_modules/core-js/internals/an-object.js 0000644 0001750 0000144 00000000264 03560116604 024173 0 ustar andreh users var isObject = require('../internals/is-object'); module.exports = function (it) { if (!isObject(it)) { throw TypeError(String(it) + ' is not an object'); } return it; }; apollo-server-demo/node_modules/core-js/internals/path.js 0000644 0001750 0000144 00000000107 03560116604 023261 0 ustar andreh users var global = require('../internals/global'); module.exports = global; apollo-server-demo/node_modules/core-js/internals/async-iterator-create-proxy.js 0000644 0001750 0000144 00000003767 03560116604 027730 0 ustar andreh users 'use strict'; var path = require('../internals/path'); var aFunction = require('../internals/a-function'); var anObject = require('../internals/an-object'); var create = require('../internals/object-create'); var createNonEnumerableProperty = require('../internals/create-non-enumerable-property'); var redefineAll = require('../internals/redefine-all'); var wellKnownSymbol = require('../internals/well-known-symbol'); var InternalStateModule = require('../internals/internal-state'); var getBuiltIn = require('../internals/get-built-in'); var Promise = getBuiltIn('Promise'); var setInternalState = InternalStateModule.set; var getInternalState = InternalStateModule.get; var TO_STRING_TAG = wellKnownSymbol('toStringTag'); var $return = function (value) { var iterator = getInternalState(this).iterator; var $$return = iterator['return']; return $$return === undefined ? Promise.resolve({ done: true, value: value }) : anObject($$return.call(iterator, value)); }; var $throw = function (value) { var iterator = getInternalState(this).iterator; var $$throw = iterator['throw']; return $$throw === undefined ? Promise.reject(value) : $$throw.call(iterator, value); }; module.exports = function (nextHandler, IS_ITERATOR) { var AsyncIteratorProxy = function AsyncIterator(state) { state.next = aFunction(state.iterator.next); state.done = false; setInternalState(this, state); }; AsyncIteratorProxy.prototype = redefineAll(create(path.AsyncIterator.prototype), { next: function next(arg) { var state = getInternalState(this); if (state.done) return Promise.resolve({ done: true, value: undefined }); try { return Promise.resolve(anObject(nextHandler.call(state, arg, Promise))); } catch (error) { return Promise.reject(error); } }, 'return': $return, 'throw': $throw }); if (!IS_ITERATOR) { createNonEnumerableProperty(AsyncIteratorProxy.prototype, TO_STRING_TAG, 'Generator'); } return AsyncIteratorProxy; }; apollo-server-demo/node_modules/core-js/internals/to-object.js 0000644 0001750 0000144 00000000367 03560116604 024223 0 ustar andreh users var requireObjectCoercible = require('../internals/require-object-coercible'); // `ToObject` abstract operation // https://tc39.es/ecma262/#sec-toobject module.exports = function (argument) { return Object(requireObjectCoercible(argument)); }; apollo-server-demo/node_modules/core-js/internals/collection-delete-all.js 0000644 0001750 0000144 00000001016 03560116604 026466 0 ustar andreh users 'use strict'; var anObject = require('../internals/an-object'); var aFunction = require('../internals/a-function'); // https://github.com/tc39/collection-methods module.exports = function (/* ...elements */) { var collection = anObject(this); var remover = aFunction(collection['delete']); var allDeleted = true; var wasDeleted; for (var k = 0, len = arguments.length; k < len; k++) { wasDeleted = remover.call(collection, arguments[k]); allDeleted = allDeleted && wasDeleted; } return !!allDeleted; }; apollo-server-demo/node_modules/core-js/internals/same-value.js 0000644 0001750 0000144 00000000361 03560116604 024366 0 ustar andreh users // `SameValue` abstract operation // https://tc39.es/ecma262/#sec-samevalue module.exports = Object.is || function is(x, y) { // eslint-disable-next-line no-self-compare return x === y ? x !== 0 || 1 / x === 1 / y : x != x && y != y; }; apollo-server-demo/node_modules/core-js/internals/collection.js 0000644 0001750 0000144 00000010323 03560116604 024461 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var global = require('../internals/global'); var isForced = require('../internals/is-forced'); var redefine = require('../internals/redefine'); var InternalMetadataModule = require('../internals/internal-metadata'); var iterate = require('../internals/iterate'); var anInstance = require('../internals/an-instance'); var isObject = require('../internals/is-object'); var fails = require('../internals/fails'); var checkCorrectnessOfIteration = require('../internals/check-correctness-of-iteration'); var setToStringTag = require('../internals/set-to-string-tag'); var inheritIfRequired = require('../internals/inherit-if-required'); module.exports = function (CONSTRUCTOR_NAME, wrapper, common) { var IS_MAP = CONSTRUCTOR_NAME.indexOf('Map') !== -1; var IS_WEAK = CONSTRUCTOR_NAME.indexOf('Weak') !== -1; var ADDER = IS_MAP ? 'set' : 'add'; var NativeConstructor = global[CONSTRUCTOR_NAME]; var NativePrototype = NativeConstructor && NativeConstructor.prototype; var Constructor = NativeConstructor; var exported = {}; var fixMethod = function (KEY) { var nativeMethod = NativePrototype[KEY]; redefine(NativePrototype, KEY, KEY == 'add' ? function add(value) { nativeMethod.call(this, value === 0 ? 0 : value); return this; } : KEY == 'delete' ? function (key) { return IS_WEAK && !isObject(key) ? false : nativeMethod.call(this, key === 0 ? 0 : key); } : KEY == 'get' ? function get(key) { return IS_WEAK && !isObject(key) ? undefined : nativeMethod.call(this, key === 0 ? 0 : key); } : KEY == 'has' ? function has(key) { return IS_WEAK && !isObject(key) ? false : nativeMethod.call(this, key === 0 ? 0 : key); } : function set(key, value) { nativeMethod.call(this, key === 0 ? 0 : key, value); return this; } ); }; // eslint-disable-next-line max-len if (isForced(CONSTRUCTOR_NAME, typeof NativeConstructor != 'function' || !(IS_WEAK || NativePrototype.forEach && !fails(function () { new NativeConstructor().entries().next(); })))) { // create collection constructor Constructor = common.getConstructor(wrapper, CONSTRUCTOR_NAME, IS_MAP, ADDER); InternalMetadataModule.REQUIRED = true; } else if (isForced(CONSTRUCTOR_NAME, true)) { var instance = new Constructor(); // early implementations not supports chaining var HASNT_CHAINING = instance[ADDER](IS_WEAK ? {} : -0, 1) != instance; // V8 ~ Chromium 40- weak-collections throws on primitives, but should return false var THROWS_ON_PRIMITIVES = fails(function () { instance.has(1); }); // most early implementations doesn't supports iterables, most modern - not close it correctly // eslint-disable-next-line no-new var ACCEPT_ITERABLES = checkCorrectnessOfIteration(function (iterable) { new NativeConstructor(iterable); }); // for early implementations -0 and +0 not the same var BUGGY_ZERO = !IS_WEAK && fails(function () { // V8 ~ Chromium 42- fails only with 5+ elements var $instance = new NativeConstructor(); var index = 5; while (index--) $instance[ADDER](index, index); return !$instance.has(-0); }); if (!ACCEPT_ITERABLES) { Constructor = wrapper(function (dummy, iterable) { anInstance(dummy, Constructor, CONSTRUCTOR_NAME); var that = inheritIfRequired(new NativeConstructor(), dummy, Constructor); if (iterable != undefined) iterate(iterable, that[ADDER], { that: that, AS_ENTRIES: IS_MAP }); return that; }); Constructor.prototype = NativePrototype; NativePrototype.constructor = Constructor; } if (THROWS_ON_PRIMITIVES || BUGGY_ZERO) { fixMethod('delete'); fixMethod('has'); IS_MAP && fixMethod('get'); } if (BUGGY_ZERO || HASNT_CHAINING) fixMethod(ADDER); // weak collections should not contains .clear method if (IS_WEAK && NativePrototype.clear) delete NativePrototype.clear; } exported[CONSTRUCTOR_NAME] = Constructor; $({ global: true, forced: Constructor != NativeConstructor }, exported); setToStringTag(Constructor, CONSTRUCTOR_NAME); if (!IS_WEAK) common.setStrong(Constructor, CONSTRUCTOR_NAME, IS_MAP); return Constructor; }; apollo-server-demo/node_modules/core-js/internals/has.js 0000644 0001750 0000144 00000000171 03560116604 023101 0 ustar andreh users var hasOwnProperty = {}.hasOwnProperty; module.exports = function (it, key) { return hasOwnProperty.call(it, key); }; apollo-server-demo/node_modules/core-js/internals/redefine-all.js 0000644 0001750 0000144 00000000272 03560116604 024657 0 ustar andreh users var redefine = require('../internals/redefine'); module.exports = function (target, src, options) { for (var key in src) redefine(target, key, src[key], options); return target; }; apollo-server-demo/node_modules/core-js/internals/object-keys.js 0000644 0001750 0000144 00000000450 03560116604 024545 0 ustar andreh users var internalObjectKeys = require('../internals/object-keys-internal'); var enumBugKeys = require('../internals/enum-bug-keys'); // `Object.keys` method // https://tc39.es/ecma262/#sec-object.keys module.exports = Object.keys || function keys(O) { return internalObjectKeys(O, enumBugKeys); }; apollo-server-demo/node_modules/core-js/internals/typed-array-constructor.js 0000644 0001750 0000144 00000022726 03560116604 027164 0 ustar andreh users 'use strict'; var $ = require('../internals/export'); var global = require('../internals/global'); var DESCRIPTORS = require('../internals/descriptors'); var TYPED_ARRAYS_CONSTRUCTORS_REQUIRES_WRAPPERS = require('../internals/typed-array-constructors-require-wrappers'); var ArrayBufferViewCore = require('../internals/array-buffer-view-core'); var ArrayBufferModule = require('../internals/array-buffer'); var anInstance = require('../internals/an-instance'); var createPropertyDescriptor = require('../internals/create-property-descriptor'); var createNonEnumerableProperty = require('../internals/create-non-enumerable-property'); var toLength = require('../internals/to-length'); var toIndex = require('../internals/to-index'); var toOffset = require('../internals/to-offset'); var toPrimitive = require('../internals/to-primitive'); var has = require('../internals/has'); var classof = require('../internals/classof'); var isObject = require('../internals/is-object'); var create = require('../internals/object-create'); var setPrototypeOf = require('../internals/object-set-prototype-of'); var getOwnPropertyNames = require('../internals/object-get-own-property-names').f; var typedArrayFrom = require('../internals/typed-array-from'); var forEach = require('../internals/array-iteration').forEach; var setSpecies = require('../internals/set-species'); var definePropertyModule = require('../internals/object-define-property'); var getOwnPropertyDescriptorModule = require('../internals/object-get-own-property-descriptor'); var InternalStateModule = require('../internals/internal-state'); var inheritIfRequired = require('../internals/inherit-if-required'); var getInternalState = InternalStateModule.get; var setInternalState = InternalStateModule.set; var nativeDefineProperty = definePropertyModule.f; var nativeGetOwnPropertyDescriptor = getOwnPropertyDescriptorModule.f; var round = Math.round; var RangeError = global.RangeError; var ArrayBuffer = ArrayBufferModule.ArrayBuffer; var DataView = ArrayBufferModule.DataView; var NATIVE_ARRAY_BUFFER_VIEWS = ArrayBufferViewCore.NATIVE_ARRAY_BUFFER_VIEWS; var TYPED_ARRAY_TAG = ArrayBufferViewCore.TYPED_ARRAY_TAG; var TypedArray = ArrayBufferViewCore.TypedArray; var TypedArrayPrototype = ArrayBufferViewCore.TypedArrayPrototype; var aTypedArrayConstructor = ArrayBufferViewCore.aTypedArrayConstructor; var isTypedArray = ArrayBufferViewCore.isTypedArray; var BYTES_PER_ELEMENT = 'BYTES_PER_ELEMENT'; var WRONG_LENGTH = 'Wrong length'; var fromList = function (C, list) { var index = 0; var length = list.length; var result = new (aTypedArrayConstructor(C))(length); while (length > index) result[index] = list[index++]; return result; }; var addGetter = function (it, key) { nativeDefineProperty(it, key, { get: function () { return getInternalState(this)[key]; } }); }; var isArrayBuffer = function (it) { var klass; return it instanceof ArrayBuffer || (klass = classof(it)) == 'ArrayBuffer' || klass == 'SharedArrayBuffer'; }; var isTypedArrayIndex = function (target, key) { return isTypedArray(target) && typeof key != 'symbol' && key in target && String(+key) == String(key); }; var wrappedGetOwnPropertyDescriptor = function getOwnPropertyDescriptor(target, key) { return isTypedArrayIndex(target, key = toPrimitive(key, true)) ? createPropertyDescriptor(2, target[key]) : nativeGetOwnPropertyDescriptor(target, key); }; var wrappedDefineProperty = function defineProperty(target, key, descriptor) { if (isTypedArrayIndex(target, key = toPrimitive(key, true)) && isObject(descriptor) && has(descriptor, 'value') && !has(descriptor, 'get') && !has(descriptor, 'set') // TODO: add validation descriptor w/o calling accessors && !descriptor.configurable && (!has(descriptor, 'writable') || descriptor.writable) && (!has(descriptor, 'enumerable') || descriptor.enumerable) ) { target[key] = descriptor.value; return target; } return nativeDefineProperty(target, key, descriptor); }; if (DESCRIPTORS) { if (!NATIVE_ARRAY_BUFFER_VIEWS) { getOwnPropertyDescriptorModule.f = wrappedGetOwnPropertyDescriptor; definePropertyModule.f = wrappedDefineProperty; addGetter(TypedArrayPrototype, 'buffer'); addGetter(TypedArrayPrototype, 'byteOffset'); addGetter(TypedArrayPrototype, 'byteLength'); addGetter(TypedArrayPrototype, 'length'); } $({ target: 'Object', stat: true, forced: !NATIVE_ARRAY_BUFFER_VIEWS }, { getOwnPropertyDescriptor: wrappedGetOwnPropertyDescriptor, defineProperty: wrappedDefineProperty }); module.exports = function (TYPE, wrapper, CLAMPED) { var BYTES = TYPE.match(/\d+$/)[0] / 8; var CONSTRUCTOR_NAME = TYPE + (CLAMPED ? 'Clamped' : '') + 'Array'; var GETTER = 'get' + TYPE; var SETTER = 'set' + TYPE; var NativeTypedArrayConstructor = global[CONSTRUCTOR_NAME]; var TypedArrayConstructor = NativeTypedArrayConstructor; var TypedArrayConstructorPrototype = TypedArrayConstructor && TypedArrayConstructor.prototype; var exported = {}; var getter = function (that, index) { var data = getInternalState(that); return data.view[GETTER](index * BYTES + data.byteOffset, true); }; var setter = function (that, index, value) { var data = getInternalState(that); if (CLAMPED) value = (value = round(value)) < 0 ? 0 : value > 0xFF ? 0xFF : value & 0xFF; data.view[SETTER](index * BYTES + data.byteOffset, value, true); }; var addElement = function (that, index) { nativeDefineProperty(that, index, { get: function () { return getter(this, index); }, set: function (value) { return setter(this, index, value); }, enumerable: true }); }; if (!NATIVE_ARRAY_BUFFER_VIEWS) { TypedArrayConstructor = wrapper(function (that, data, offset, $length) { anInstance(that, TypedArrayConstructor, CONSTRUCTOR_NAME); var index = 0; var byteOffset = 0; var buffer, byteLength, length; if (!isObject(data)) { length = toIndex(data); byteLength = length * BYTES; buffer = new ArrayBuffer(byteLength); } else if (isArrayBuffer(data)) { buffer = data; byteOffset = toOffset(offset, BYTES); var $len = data.byteLength; if ($length === undefined) { if ($len % BYTES) throw RangeError(WRONG_LENGTH); byteLength = $len - byteOffset; if (byteLength < 0) throw RangeError(WRONG_LENGTH); } else { byteLength = toLength($length) * BYTES; if (byteLength + byteOffset > $len) throw RangeError(WRONG_LENGTH); } length = byteLength / BYTES; } else if (isTypedArray(data)) { return fromList(TypedArrayConstructor, data); } else { return typedArrayFrom.call(TypedArrayConstructor, data); } setInternalState(that, { buffer: buffer, byteOffset: byteOffset, byteLength: byteLength, length: length, view: new DataView(buffer) }); while (index < length) addElement(that, index++); }); if (setPrototypeOf) setPrototypeOf(TypedArrayConstructor, TypedArray); TypedArrayConstructorPrototype = TypedArrayConstructor.prototype = create(TypedArrayPrototype); } else if (TYPED_ARRAYS_CONSTRUCTORS_REQUIRES_WRAPPERS) { TypedArrayConstructor = wrapper(function (dummy, data, typedArrayOffset, $length) { anInstance(dummy, TypedArrayConstructor, CONSTRUCTOR_NAME); return inheritIfRequired(function () { if (!isObject(data)) return new NativeTypedArrayConstructor(toIndex(data)); if (isArrayBuffer(data)) return $length !== undefined ? new NativeTypedArrayConstructor(data, toOffset(typedArrayOffset, BYTES), $length) : typedArrayOffset !== undefined ? new NativeTypedArrayConstructor(data, toOffset(typedArrayOffset, BYTES)) : new NativeTypedArrayConstructor(data); if (isTypedArray(data)) return fromList(TypedArrayConstructor, data); return typedArrayFrom.call(TypedArrayConstructor, data); }(), dummy, TypedArrayConstructor); }); if (setPrototypeOf) setPrototypeOf(TypedArrayConstructor, TypedArray); forEach(getOwnPropertyNames(NativeTypedArrayConstructor), function (key) { if (!(key in TypedArrayConstructor)) { createNonEnumerableProperty(TypedArrayConstructor, key, NativeTypedArrayConstructor[key]); } }); TypedArrayConstructor.prototype = TypedArrayConstructorPrototype; } if (TypedArrayConstructorPrototype.constructor !== TypedArrayConstructor) { createNonEnumerableProperty(TypedArrayConstructorPrototype, 'constructor', TypedArrayConstructor); } if (TYPED_ARRAY_TAG) { createNonEnumerableProperty(TypedArrayConstructorPrototype, TYPED_ARRAY_TAG, CONSTRUCTOR_NAME); } exported[CONSTRUCTOR_NAME] = TypedArrayConstructor; $({ global: true, forced: TypedArrayConstructor != NativeTypedArrayConstructor, sham: !NATIVE_ARRAY_BUFFER_VIEWS }, exported); if (!(BYTES_PER_ELEMENT in TypedArrayConstructor)) { createNonEnumerableProperty(TypedArrayConstructor, BYTES_PER_ELEMENT, BYTES); } if (!(BYTES_PER_ELEMENT in TypedArrayConstructorPrototype)) { createNonEnumerableProperty(TypedArrayConstructorPrototype, BYTES_PER_ELEMENT, BYTES); } setSpecies(CONSTRUCTOR_NAME); }; } else module.exports = function () { /* empty */ }; apollo-server-demo/node_modules/core-js/internals/inspect-source.js 0000644 0001750 0000144 00000000522 03560116604 025271 0 ustar andreh users var store = require('../internals/shared-store'); var functionToString = Function.toString; // this helper broken in `3.4.1-3.4.4`, so we can't use `shared` helper if (typeof store.inspectSource != 'function') { store.inspectSource = function (it) { return functionToString.call(it); }; } module.exports = store.inspectSource; apollo-server-demo/node_modules/core-js/internals/uid.js 0000644 0001750 0000144 00000000261 03560116604 023107 0 ustar andreh users var id = 0; var postfix = Math.random(); module.exports = function (key) { return 'Symbol(' + String(key === undefined ? '' : key) + ')_' + (++id + postfix).toString(36); }; apollo-server-demo/node_modules/core-js/internals/shared.js 0000644 0001750 0000144 00000000540 03560116604 023574 0 ustar andreh users var IS_PURE = require('../internals/is-pure'); var store = require('../internals/shared-store'); (module.exports = function (key, value) { return store[key] || (store[key] = value !== undefined ? value : {}); })('versions', []).push({ version: '3.8.3', mode: IS_PURE ? 'pure' : 'global', copyright: '© 2021 Denis Pushkarev (zloirock.ru)' }); apollo-server-demo/node_modules/core-js/internals/create-iterator-constructor.js 0000644 0001750 0000144 00000001352 03560116604 030005 0 ustar andreh users 'use strict'; var IteratorPrototype = require('../internals/iterators-core').IteratorPrototype; var create = require('../internals/object-create'); var createPropertyDescriptor = require('../internals/create-property-descriptor'); var setToStringTag = require('../internals/set-to-string-tag'); var Iterators = require('../internals/iterators'); var returnThis = function () { return this; }; module.exports = function (IteratorConstructor, NAME, next) { var TO_STRING_TAG = NAME + ' Iterator'; IteratorConstructor.prototype = create(IteratorPrototype, { next: createPropertyDescriptor(1, next) }); setToStringTag(IteratorConstructor, TO_STRING_TAG, false, true); Iterators[TO_STRING_TAG] = returnThis; return IteratorConstructor; }; apollo-server-demo/node_modules/core-js/internals/range-iterator.js 0000644 0001750 0000144 00000006365 03560116604 025264 0 ustar andreh users 'use strict'; var InternalStateModule = require('../internals/internal-state'); var createIteratorConstructor = require('../internals/create-iterator-constructor'); var isObject = require('../internals/is-object'); var defineProperties = require('../internals/object-define-properties'); var DESCRIPTORS = require('../internals/descriptors'); var INCORRECT_RANGE = 'Incorrect Number.range arguments'; var RANGE_ITERATOR = 'RangeIterator'; var setInternalState = InternalStateModule.set; var getInternalState = InternalStateModule.getterFor(RANGE_ITERATOR); var $RangeIterator = createIteratorConstructor(function RangeIterator(start, end, option, type, zero, one) { if (typeof start != type || (end !== Infinity && end !== -Infinity && typeof end != type)) { throw new TypeError(INCORRECT_RANGE); } if (start === Infinity || start === -Infinity) { throw new RangeError(INCORRECT_RANGE); } var ifIncrease = end > start; var inclusiveEnd = false; var step; if (option === undefined) { step = undefined; } else if (isObject(option)) { step = option.step; inclusiveEnd = !!option.inclusive; } else if (typeof option == type) { step = option; } else { throw new TypeError(INCORRECT_RANGE); } if (step == null) { step = ifIncrease ? one : -one; } if (typeof step != type) { throw new TypeError(INCORRECT_RANGE); } if (step === Infinity || step === -Infinity || (step === zero && start !== end)) { throw new RangeError(INCORRECT_RANGE); } // eslint-disable-next-line no-self-compare var hitsEnd = start != start || end != end || step != step || (end > start) !== (step > zero); setInternalState(this, { type: RANGE_ITERATOR, start: start, end: end, step: step, inclusiveEnd: inclusiveEnd, hitsEnd: hitsEnd, currentCount: zero, zero: zero }); if (!DESCRIPTORS) { this.start = start; this.end = end; this.step = step; this.inclusive = inclusiveEnd; } }, RANGE_ITERATOR, function next() { var state = getInternalState(this); if (state.hitsEnd) return { value: undefined, done: true }; var start = state.start; var end = state.end; var step = state.step; var currentYieldingValue = start + (step * state.currentCount++); if (currentYieldingValue === end) state.hitsEnd = true; var inclusiveEnd = state.inclusiveEnd; var endCondition; if (end > start) { endCondition = inclusiveEnd ? currentYieldingValue > end : currentYieldingValue >= end; } else { endCondition = inclusiveEnd ? end > currentYieldingValue : end >= currentYieldingValue; } if (endCondition) { return { value: undefined, done: state.hitsEnd = true }; } return { value: currentYieldingValue, done: false }; }); var getter = function (fn) { return { get: fn, set: function () { /* empty */ }, configurable: true, enumerable: false }; }; if (DESCRIPTORS) { defineProperties($RangeIterator.prototype, { start: getter(function () { return getInternalState(this).start; }), end: getter(function () { return getInternalState(this).end; }), inclusive: getter(function () { return getInternalState(this).inclusiveEnd; }), step: getter(function () { return getInternalState(this).step; }) }); } module.exports = $RangeIterator; apollo-server-demo/node_modules/core-js/internals/export.js 0000644 0001750 0000144 00000004727 03560116604 023662 0 ustar andreh users var global = require('../internals/global'); var getOwnPropertyDescriptor = require('../internals/object-get-own-property-descriptor').f; var createNonEnumerableProperty = require('../internals/create-non-enumerable-property'); var redefine = require('../internals/redefine'); var setGlobal = require('../internals/set-global'); var copyConstructorProperties = require('../internals/copy-constructor-properties'); var isForced = require('../internals/is-forced'); /* options.target - name of the target object options.global - target is the global object options.stat - export as static methods of target options.proto - export as prototype methods of target options.real - real prototype method for the `pure` version options.forced - export even if the native feature is available options.bind - bind methods to the target, required for the `pure` version options.wrap - wrap constructors to preventing global pollution, required for the `pure` version options.unsafe - use the simple assignment of property instead of delete + defineProperty options.sham - add a flag to not completely full polyfills options.enumerable - export as enumerable property options.noTargetGet - prevent calling a getter on target */ module.exports = function (options, source) { var TARGET = options.target; var GLOBAL = options.global; var STATIC = options.stat; var FORCED, target, key, targetProperty, sourceProperty, descriptor; if (GLOBAL) { target = global; } else if (STATIC) { target = global[TARGET] || setGlobal(TARGET, {}); } else { target = (global[TARGET] || {}).prototype; } if (target) for (key in source) { sourceProperty = source[key]; if (options.noTargetGet) { descriptor = getOwnPropertyDescriptor(target, key); targetProperty = descriptor && descriptor.value; } else targetProperty = target[key]; FORCED = isForced(GLOBAL ? key : TARGET + (STATIC ? '.' : '#') + key, options.forced); // contained in target if (!FORCED && targetProperty !== undefined) { if (typeof sourceProperty === typeof targetProperty) continue; copyConstructorProperties(sourceProperty, targetProperty); } // add a flag to not completely full polyfills if (options.sham || (targetProperty && targetProperty.sham)) { createNonEnumerableProperty(sourceProperty, 'sham', true); } // extend global redefine(target, key, sourceProperty, options); } }; apollo-server-demo/node_modules/core-js/internals/this-number-value.js 0000644 0001750 0000144 00000000465 03560116604 025703 0 ustar andreh users var classof = require('../internals/classof-raw'); // `thisNumberValue` abstract operation // https://tc39.es/ecma262/#sec-thisnumbervalue module.exports = function (value) { if (typeof value != 'number' && classof(value) != 'Number') { throw TypeError('Incorrect invocation'); } return +value; }; apollo-server-demo/node_modules/core-js/internals/entry-unbind.js 0000644 0001750 0000144 00000000404 03560116604 024743 0 ustar andreh users var global = require('../internals/global'); var bind = require('../internals/function-bind-context'); var call = Function.call; module.exports = function (CONSTRUCTOR, METHOD, length) { return bind(call, global[CONSTRUCTOR].prototype[METHOD], length); }; apollo-server-demo/node_modules/core-js/internals/object-define-property.js 0000644 0001750 0000144 00000001436 03560116604 026713 0 ustar andreh users var DESCRIPTORS = require('../internals/descriptors'); var IE8_DOM_DEFINE = require('../internals/ie8-dom-define'); var anObject = require('../internals/an-object'); var toPrimitive = require('../internals/to-primitive'); var nativeDefineProperty = Object.defineProperty; // `Object.defineProperty` method // https://tc39.es/ecma262/#sec-object.defineproperty exports.f = DESCRIPTORS ? nativeDefineProperty : function defineProperty(O, P, Attributes) { anObject(O); P = toPrimitive(P, true); anObject(Attributes); if (IE8_DOM_DEFINE) try { return nativeDefineProperty(O, P, Attributes); } catch (error) { /* empty */ } if ('get' in Attributes || 'set' in Attributes) throw TypeError('Accessors not supported'); if ('value' in Attributes) O[P] = Attributes.value; return O; }; apollo-server-demo/node_modules/core-js/internals/async-iterator-prototype.js 0000644 0001750 0000144 00000003002 03560116604 027331 0 ustar andreh users var global = require('../internals/global'); var shared = require('../internals/shared-store'); var getPrototypeOf = require('../internals/object-get-prototype-of'); var has = require('../internals/has'); var createNonEnumerableProperty = require('../internals/create-non-enumerable-property'); var wellKnownSymbol = require('../internals/well-known-symbol'); var IS_PURE = require('../internals/is-pure'); var USE_FUNCTION_CONSTRUCTOR = 'USE_FUNCTION_CONSTRUCTOR'; var ASYNC_ITERATOR = wellKnownSymbol('asyncIterator'); var AsyncIterator = global.AsyncIterator; var PassedAsyncIteratorPrototype = shared.AsyncIteratorPrototype; var AsyncIteratorPrototype, prototype; if (!IS_PURE) { if (PassedAsyncIteratorPrototype) { AsyncIteratorPrototype = PassedAsyncIteratorPrototype; } else if (typeof AsyncIterator == 'function') { AsyncIteratorPrototype = AsyncIterator.prototype; } else if (shared[USE_FUNCTION_CONSTRUCTOR] || global[USE_FUNCTION_CONSTRUCTOR]) { try { // eslint-disable-next-line no-new-func prototype = getPrototypeOf(getPrototypeOf(getPrototypeOf(Function('return async function*(){}()')()))); if (getPrototypeOf(prototype) === Object.prototype) AsyncIteratorPrototype = prototype; } catch (error) { /* empty */ } } } if (!AsyncIteratorPrototype) AsyncIteratorPrototype = {}; if (!has(AsyncIteratorPrototype, ASYNC_ITERATOR)) { createNonEnumerableProperty(AsyncIteratorPrototype, ASYNC_ITERATOR, function () { return this; }); } module.exports = AsyncIteratorPrototype; apollo-server-demo/node_modules/core-js/internals/get-iterator-method.js 0000644 0001750 0000144 00000000527 03560116604 026217 0 ustar andreh users var classof = require('../internals/classof'); var Iterators = require('../internals/iterators'); var wellKnownSymbol = require('../internals/well-known-symbol'); var ITERATOR = wellKnownSymbol('iterator'); module.exports = function (it) { if (it != undefined) return it[ITERATOR] || it['@@iterator'] || Iterators[classof(it)]; }; apollo-server-demo/node_modules/core-js/internals/object-create.js 0000644 0001750 0000144 00000005473 03560116604 025047 0 ustar andreh users var anObject = require('../internals/an-object'); var defineProperties = require('../internals/object-define-properties'); var enumBugKeys = require('../internals/enum-bug-keys'); var hiddenKeys = require('../internals/hidden-keys'); var html = require('../internals/html'); var documentCreateElement = require('../internals/document-create-element'); var sharedKey = require('../internals/shared-key'); var GT = '>'; var LT = '<'; var PROTOTYPE = 'prototype'; var SCRIPT = 'script'; var IE_PROTO = sharedKey('IE_PROTO'); var EmptyConstructor = function () { /* empty */ }; var scriptTag = function (content) { return LT + SCRIPT + GT + content + LT + '/' + SCRIPT + GT; }; // Create object with fake `null` prototype: use ActiveX Object with cleared prototype var NullProtoObjectViaActiveX = function (activeXDocument) { activeXDocument.write(scriptTag('')); activeXDocument.close(); var temp = activeXDocument.parentWindow.Object; activeXDocument = null; // avoid memory leak return temp; }; // Create object with fake `null` prototype: use iframe Object with cleared prototype var NullProtoObjectViaIFrame = function () { // Thrash, waste and sodomy: IE GC bug var iframe = documentCreateElement('iframe'); var JS = 'java' + SCRIPT + ':'; var iframeDocument; iframe.style.display = 'none'; html.appendChild(iframe); // https://github.com/zloirock/core-js/issues/475 iframe.src = String(JS); iframeDocument = iframe.contentWindow.document; iframeDocument.open(); iframeDocument.write(scriptTag('document.F=Object')); iframeDocument.close(); return iframeDocument.F; }; // Check for document.domain and active x support // No need to use active x approach when document.domain is not set // see https://github.com/es-shims/es5-shim/issues/150 // variation of https://github.com/kitcambridge/es5-shim/commit/4f738ac066346 // avoid IE GC bug var activeXDocument; var NullProtoObject = function () { try { /* global ActiveXObject */ activeXDocument = document.domain && new ActiveXObject('htmlfile'); } catch (error) { /* ignore */ } NullProtoObject = activeXDocument ? NullProtoObjectViaActiveX(activeXDocument) : NullProtoObjectViaIFrame(); var length = enumBugKeys.length; while (length--) delete NullProtoObject[PROTOTYPE][enumBugKeys[length]]; return NullProtoObject(); }; hiddenKeys[IE_PROTO] = true; // `Object.create` method // https://tc39.es/ecma262/#sec-object.create module.exports = Object.create || function create(O, Properties) { var result; if (O !== null) { EmptyConstructor[PROTOTYPE] = anObject(O); result = new EmptyConstructor(); EmptyConstructor[PROTOTYPE] = null; // add "__proto__" for Object.getPrototypeOf polyfill result[IE_PROTO] = O; } else result = NullProtoObject(); return Properties === undefined ? result : defineProperties(result, Properties); }; apollo-server-demo/node_modules/core-js/proposals/ 0000755 0001750 0000144 00000000000 14067647701 022027 5 ustar andreh users apollo-server-demo/node_modules/core-js/proposals/index.js 0000644 0001750 0000144 00000000025 03560116604 023456 0 ustar andreh users require('../stage'); apollo-server-demo/node_modules/core-js/proposals/string-match-all.js 0000644 0001750 0000144 00000000120 03560116604 025511 0 ustar andreh users // TODO: Remove from `core-js@4` require('../modules/esnext.string.match-all'); apollo-server-demo/node_modules/core-js/proposals/pattern-matching.js 0000644 0001750 0000144 00000000063 03560116604 025616 0 ustar andreh users require('../modules/esnext.symbol.pattern-match'); apollo-server-demo/node_modules/core-js/proposals/promise-try.js 0000644 0001750 0000144 00000000052 03560116604 024641 0 ustar andreh users require('../modules/esnext.promise.try'); apollo-server-demo/node_modules/core-js/proposals/array-unique.js 0000644 0001750 0000144 00000000175 03560116604 024777 0 ustar andreh users // https://github.com/tc39/proposal-array-unique require('../modules/es.map'); require('../modules/esnext.array.unique-by'); apollo-server-demo/node_modules/core-js/proposals/map-upsert.js 0000644 0001750 0000144 00000000567 03560116604 024457 0 ustar andreh users // https://github.com/thumbsupep/proposal-upsert require('../modules/esnext.map.emplace'); // TODO: remove from `core-js@4` require('../modules/esnext.map.update-or-insert'); // TODO: remove from `core-js@4` require('../modules/esnext.map.upsert'); require('../modules/esnext.weak-map.emplace'); // TODO: remove from `core-js@4` require('../modules/esnext.weak-map.upsert'); apollo-server-demo/node_modules/core-js/proposals/string-at.js 0000644 0001750 0000144 00000000050 03560116604 024255 0 ustar andreh users require('../modules/esnext.string.at'); apollo-server-demo/node_modules/core-js/proposals/number-from-string.js 0000644 0001750 0000144 00000000061 03560116604 026104 0 ustar andreh users require('../modules/esnext.number.from-string'); apollo-server-demo/node_modules/core-js/proposals/set-methods.js 0000644 0001750 0000144 00000000516 03560116604 024610 0 ustar andreh users require('../modules/esnext.set.difference'); require('../modules/esnext.set.intersection'); require('../modules/esnext.set.is-disjoint-from'); require('../modules/esnext.set.is-subset-of'); require('../modules/esnext.set.is-superset-of'); require('../modules/esnext.set.union'); require('../modules/esnext.set.symmetric-difference'); apollo-server-demo/node_modules/core-js/proposals/reflect-metadata.js 0000644 0001750 0000144 00000000743 03560116604 025560 0 ustar andreh users require('../modules/esnext.reflect.define-metadata'); require('../modules/esnext.reflect.delete-metadata'); require('../modules/esnext.reflect.get-metadata'); require('../modules/esnext.reflect.get-metadata-keys'); require('../modules/esnext.reflect.get-own-metadata'); require('../modules/esnext.reflect.get-own-metadata-keys'); require('../modules/esnext.reflect.has-metadata'); require('../modules/esnext.reflect.has-own-metadata'); require('../modules/esnext.reflect.metadata'); apollo-server-demo/node_modules/core-js/proposals/math-signbit.js 0000644 0001750 0000144 00000000053 03560116604 024736 0 ustar andreh users require('../modules/esnext.math.signbit'); apollo-server-demo/node_modules/core-js/proposals/efficient-64-bit-arithmetic.js 0000644 0001750 0000144 00000000322 03560116604 027435 0 ustar andreh users // TODO: remove from `core-js@4` as withdrawn require('../modules/esnext.math.iaddh'); require('../modules/esnext.math.isubh'); require('../modules/esnext.math.imulh'); require('../modules/esnext.math.umulh'); apollo-server-demo/node_modules/core-js/proposals/array-filtering.js 0000644 0001750 0000144 00000000230 03560116604 025444 0 ustar andreh users // https://github.com/tc39/proposal-array-filtering require('../modules/esnext.array.filter-out'); require('../modules/esnext.typed-array.filter-out'); apollo-server-demo/node_modules/core-js/proposals/map-update-or-insert.js 0000644 0001750 0000144 00000000072 03560116604 026326 0 ustar andreh users // TODO: remove from `core-js@4` require('./map-upsert'); apollo-server-demo/node_modules/core-js/proposals/relative-indexing-method.js 0000644 0001750 0000144 00000000423 03560116604 027245 0 ustar andreh users // https://github.com/tc39/proposal-relative-indexing-method require('../modules/esnext.array.at'); // TODO: disabled by default because of the conflict with another proposal // require('../modules/esnext.string.at-alternative'); require('../modules/esnext.typed-array.at'); apollo-server-demo/node_modules/core-js/proposals/math-extensions.js 0000644 0001750 0000144 00000000460 03560116604 025500 0 ustar andreh users require('../modules/esnext.math.clamp'); require('../modules/esnext.math.deg-per-rad'); require('../modules/esnext.math.degrees'); require('../modules/esnext.math.fscale'); require('../modules/esnext.math.rad-per-deg'); require('../modules/esnext.math.radians'); require('../modules/esnext.math.scale'); apollo-server-demo/node_modules/core-js/proposals/collection-methods.js 0000644 0001750 0000144 00000002160 03560116604 026145 0 ustar andreh users require('../modules/esnext.map.group-by'); require('../modules/esnext.map.key-by'); require('../modules/esnext.map.delete-all'); require('../modules/esnext.map.every'); require('../modules/esnext.map.filter'); require('../modules/esnext.map.find'); require('../modules/esnext.map.find-key'); require('../modules/esnext.map.includes'); require('../modules/esnext.map.key-of'); require('../modules/esnext.map.map-keys'); require('../modules/esnext.map.map-values'); require('../modules/esnext.map.merge'); require('../modules/esnext.map.reduce'); require('../modules/esnext.map.some'); require('../modules/esnext.map.update'); require('../modules/esnext.set.add-all'); require('../modules/esnext.set.delete-all'); require('../modules/esnext.set.every'); require('../modules/esnext.set.filter'); require('../modules/esnext.set.find'); require('../modules/esnext.set.join'); require('../modules/esnext.set.map'); require('../modules/esnext.set.reduce'); require('../modules/esnext.set.some'); require('../modules/esnext.weak-map.delete-all'); require('../modules/esnext.weak-set.add-all'); require('../modules/esnext.weak-set.delete-all'); apollo-server-demo/node_modules/core-js/proposals/seeded-random.js 0000644 0001750 0000144 00000000057 03560116604 025063 0 ustar andreh users require('../modules/esnext.math.seeded-prng'); apollo-server-demo/node_modules/core-js/proposals/iterator-helpers.js 0000644 0001750 0000144 00000002552 03560116604 025647 0 ustar andreh users require('../modules/esnext.async-iterator.constructor'); require('../modules/esnext.async-iterator.as-indexed-pairs'); require('../modules/esnext.async-iterator.drop'); require('../modules/esnext.async-iterator.every'); require('../modules/esnext.async-iterator.filter'); require('../modules/esnext.async-iterator.find'); require('../modules/esnext.async-iterator.flat-map'); require('../modules/esnext.async-iterator.for-each'); require('../modules/esnext.async-iterator.from'); require('../modules/esnext.async-iterator.map'); require('../modules/esnext.async-iterator.reduce'); require('../modules/esnext.async-iterator.some'); require('../modules/esnext.async-iterator.take'); require('../modules/esnext.async-iterator.to-array'); require('../modules/esnext.iterator.constructor'); require('../modules/esnext.iterator.as-indexed-pairs'); require('../modules/esnext.iterator.drop'); require('../modules/esnext.iterator.every'); require('../modules/esnext.iterator.filter'); require('../modules/esnext.iterator.find'); require('../modules/esnext.iterator.flat-map'); require('../modules/esnext.iterator.for-each'); require('../modules/esnext.iterator.from'); require('../modules/esnext.iterator.map'); require('../modules/esnext.iterator.reduce'); require('../modules/esnext.iterator.some'); require('../modules/esnext.iterator.take'); require('../modules/esnext.iterator.to-array'); apollo-server-demo/node_modules/core-js/proposals/array-last.js 0000644 0001750 0000144 00000000135 03560116604 024430 0 ustar andreh users require('../modules/esnext.array.last-index'); require('../modules/esnext.array.last-item'); apollo-server-demo/node_modules/core-js/proposals/promise-any.js 0000644 0001750 0000144 00000000130 03560116604 024607 0 ustar andreh users require('../modules/esnext.aggregate-error'); require('../modules/esnext.promise.any'); apollo-server-demo/node_modules/core-js/proposals/observable.js 0000644 0001750 0000144 00000000131 03560116604 024471 0 ustar andreh users require('../modules/esnext.observable'); require('../modules/esnext.symbol.observable'); apollo-server-demo/node_modules/core-js/proposals/using-statement.js 0000644 0001750 0000144 00000000224 03560116604 025477 0 ustar andreh users // https://github.com/tc39/proposal-using-statement require('../modules/esnext.symbol.async-dispose'); require('../modules/esnext.symbol.dispose'); apollo-server-demo/node_modules/core-js/proposals/global-this.js 0000644 0001750 0000144 00000000161 03560116604 024555 0 ustar andreh users require('../modules/esnext.global-this'); var global = require('../internals/global'); module.exports = global; apollo-server-demo/node_modules/core-js/proposals/object-iteration.js 0000644 0001750 0000144 00000000311 03560116604 025607 0 ustar andreh users // TODO: remove from `core-js@4` as withdrawn require('../modules/esnext.object.iterate-entries'); require('../modules/esnext.object.iterate-keys'); require('../modules/esnext.object.iterate-values'); apollo-server-demo/node_modules/core-js/proposals/number-range.js 0000644 0001750 0000144 00000000207 03560116604 024733 0 ustar andreh users // https://github.com/tc39/proposal-Number.range require('../modules/esnext.bigint.range'); require('../modules/esnext.number.range'); apollo-server-demo/node_modules/core-js/proposals/promise-all-settled.js 0000644 0001750 0000144 00000000123 03560116604 026234 0 ustar andreh users // TODO: Remove from `core-js@4` require('../modules/esnext.promise.all-settled'); apollo-server-demo/node_modules/core-js/proposals/collection-of-from.js 0000644 0001750 0000144 00000000504 03560116604 026047 0 ustar andreh users require('../modules/esnext.map.from'); require('../modules/esnext.map.of'); require('../modules/esnext.set.from'); require('../modules/esnext.set.of'); require('../modules/esnext.weak-map.from'); require('../modules/esnext.weak-map.of'); require('../modules/esnext.weak-set.from'); require('../modules/esnext.weak-set.of'); apollo-server-demo/node_modules/core-js/proposals/url.js 0000644 0001750 0000144 00000000163 03560116604 023154 0 ustar andreh users require('../modules/web.url'); require('../modules/web.url.to-json'); require('../modules/web.url-search-params'); apollo-server-demo/node_modules/core-js/proposals/array-is-template-object.js 0000644 0001750 0000144 00000000067 03560116604 027161 0 ustar andreh users require('../modules/esnext.array.is-template-object'); apollo-server-demo/node_modules/core-js/proposals/string-code-points.js 0000644 0001750 0000144 00000000061 03560116604 026077 0 ustar andreh users require('../modules/esnext.string.code-points'); apollo-server-demo/node_modules/core-js/proposals/string-replace-all.js 0000644 0001750 0000144 00000000142 03560116604 026034 0 ustar andreh users require('../modules/esnext.string.replace-all'); require('../modules/esnext.symbol.replace-all'); apollo-server-demo/node_modules/core-js/proposals/keys-composition.js 0000644 0001750 0000144 00000000133 03560116604 025663 0 ustar andreh users require('../modules/esnext.composite-key'); require('../modules/esnext.composite-symbol'); apollo-server-demo/node_modules/core-js/es/ 0000755 0001750 0000144 00000000000 14067647701 020414 5 ustar andreh users apollo-server-demo/node_modules/core-js/es/weak-map/ 0000755 0001750 0000144 00000000000 14067647701 022116 5 ustar andreh users apollo-server-demo/node_modules/core-js/es/weak-map/index.js 0000644 0001750 0000144 00000000327 03560116604 023552 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.weak-map'); require('../../modules/web.dom-collections.iterator'); var path = require('../../internals/path'); module.exports = path.WeakMap; apollo-server-demo/node_modules/core-js/es/index.js 0000644 0001750 0000144 00000021614 03560116604 022052 0 ustar andreh users require('../modules/es.symbol'); require('../modules/es.symbol.async-iterator'); require('../modules/es.symbol.description'); require('../modules/es.symbol.has-instance'); require('../modules/es.symbol.is-concat-spreadable'); require('../modules/es.symbol.iterator'); require('../modules/es.symbol.match'); require('../modules/es.symbol.match-all'); require('../modules/es.symbol.replace'); require('../modules/es.symbol.search'); require('../modules/es.symbol.species'); require('../modules/es.symbol.split'); require('../modules/es.symbol.to-primitive'); require('../modules/es.symbol.to-string-tag'); require('../modules/es.symbol.unscopables'); require('../modules/es.aggregate-error'); require('../modules/es.array.from'); require('../modules/es.array.is-array'); require('../modules/es.array.of'); require('../modules/es.array.concat'); require('../modules/es.array.copy-within'); require('../modules/es.array.every'); require('../modules/es.array.fill'); require('../modules/es.array.filter'); require('../modules/es.array.find'); require('../modules/es.array.find-index'); require('../modules/es.array.flat'); require('../modules/es.array.flat-map'); require('../modules/es.array.for-each'); require('../modules/es.array.includes'); require('../modules/es.array.index-of'); require('../modules/es.array.join'); require('../modules/es.array.last-index-of'); require('../modules/es.array.map'); require('../modules/es.array.reduce'); require('../modules/es.array.reduce-right'); require('../modules/es.array.reverse'); require('../modules/es.array.slice'); require('../modules/es.array.some'); require('../modules/es.array.sort'); require('../modules/es.array.splice'); require('../modules/es.array.species'); require('../modules/es.array.unscopables.flat'); require('../modules/es.array.unscopables.flat-map'); require('../modules/es.array.iterator'); require('../modules/es.function.bind'); require('../modules/es.function.name'); require('../modules/es.function.has-instance'); require('../modules/es.global-this'); require('../modules/es.object.assign'); require('../modules/es.object.create'); require('../modules/es.object.define-property'); require('../modules/es.object.define-properties'); require('../modules/es.object.entries'); require('../modules/es.object.freeze'); require('../modules/es.object.from-entries'); require('../modules/es.object.get-own-property-descriptor'); require('../modules/es.object.get-own-property-descriptors'); require('../modules/es.object.get-own-property-names'); require('../modules/es.object.get-prototype-of'); require('../modules/es.object.is'); require('../modules/es.object.is-extensible'); require('../modules/es.object.is-frozen'); require('../modules/es.object.is-sealed'); require('../modules/es.object.keys'); require('../modules/es.object.prevent-extensions'); require('../modules/es.object.seal'); require('../modules/es.object.set-prototype-of'); require('../modules/es.object.values'); require('../modules/es.object.to-string'); require('../modules/es.object.define-getter'); require('../modules/es.object.define-setter'); require('../modules/es.object.lookup-getter'); require('../modules/es.object.lookup-setter'); require('../modules/es.string.from-code-point'); require('../modules/es.string.raw'); require('../modules/es.string.code-point-at'); require('../modules/es.string.ends-with'); require('../modules/es.string.includes'); require('../modules/es.string.match'); require('../modules/es.string.match-all'); require('../modules/es.string.pad-end'); require('../modules/es.string.pad-start'); require('../modules/es.string.repeat'); require('../modules/es.string.replace'); require('../modules/es.string.search'); require('../modules/es.string.split'); require('../modules/es.string.starts-with'); require('../modules/es.string.trim'); require('../modules/es.string.trim-start'); require('../modules/es.string.trim-end'); require('../modules/es.string.iterator'); require('../modules/es.string.anchor'); require('../modules/es.string.big'); require('../modules/es.string.blink'); require('../modules/es.string.bold'); require('../modules/es.string.fixed'); require('../modules/es.string.fontcolor'); require('../modules/es.string.fontsize'); require('../modules/es.string.italics'); require('../modules/es.string.link'); require('../modules/es.string.small'); require('../modules/es.string.strike'); require('../modules/es.string.sub'); require('../modules/es.string.sup'); require('../modules/es.string.replace-all'); require('../modules/es.regexp.constructor'); require('../modules/es.regexp.exec'); require('../modules/es.regexp.flags'); require('../modules/es.regexp.sticky'); require('../modules/es.regexp.test'); require('../modules/es.regexp.to-string'); require('../modules/es.parse-int'); require('../modules/es.parse-float'); require('../modules/es.number.constructor'); require('../modules/es.number.epsilon'); require('../modules/es.number.is-finite'); require('../modules/es.number.is-integer'); require('../modules/es.number.is-nan'); require('../modules/es.number.is-safe-integer'); require('../modules/es.number.max-safe-integer'); require('../modules/es.number.min-safe-integer'); require('../modules/es.number.parse-float'); require('../modules/es.number.parse-int'); require('../modules/es.number.to-fixed'); require('../modules/es.number.to-precision'); require('../modules/es.math.acosh'); require('../modules/es.math.asinh'); require('../modules/es.math.atanh'); require('../modules/es.math.cbrt'); require('../modules/es.math.clz32'); require('../modules/es.math.cosh'); require('../modules/es.math.expm1'); require('../modules/es.math.fround'); require('../modules/es.math.hypot'); require('../modules/es.math.imul'); require('../modules/es.math.log10'); require('../modules/es.math.log1p'); require('../modules/es.math.log2'); require('../modules/es.math.sign'); require('../modules/es.math.sinh'); require('../modules/es.math.tanh'); require('../modules/es.math.to-string-tag'); require('../modules/es.math.trunc'); require('../modules/es.date.now'); require('../modules/es.date.to-json'); require('../modules/es.date.to-iso-string'); require('../modules/es.date.to-string'); require('../modules/es.date.to-primitive'); require('../modules/es.json.stringify'); require('../modules/es.json.to-string-tag'); require('../modules/es.promise'); require('../modules/es.promise.all-settled'); require('../modules/es.promise.any'); require('../modules/es.promise.finally'); require('../modules/es.map'); require('../modules/es.set'); require('../modules/es.weak-map'); require('../modules/es.weak-set'); require('../modules/es.array-buffer.constructor'); require('../modules/es.array-buffer.is-view'); require('../modules/es.array-buffer.slice'); require('../modules/es.data-view'); require('../modules/es.typed-array.int8-array'); require('../modules/es.typed-array.uint8-array'); require('../modules/es.typed-array.uint8-clamped-array'); require('../modules/es.typed-array.int16-array'); require('../modules/es.typed-array.uint16-array'); require('../modules/es.typed-array.int32-array'); require('../modules/es.typed-array.uint32-array'); require('../modules/es.typed-array.float32-array'); require('../modules/es.typed-array.float64-array'); require('../modules/es.typed-array.from'); require('../modules/es.typed-array.of'); require('../modules/es.typed-array.copy-within'); require('../modules/es.typed-array.every'); require('../modules/es.typed-array.fill'); require('../modules/es.typed-array.filter'); require('../modules/es.typed-array.find'); require('../modules/es.typed-array.find-index'); require('../modules/es.typed-array.for-each'); require('../modules/es.typed-array.includes'); require('../modules/es.typed-array.index-of'); require('../modules/es.typed-array.iterator'); require('../modules/es.typed-array.join'); require('../modules/es.typed-array.last-index-of'); require('../modules/es.typed-array.map'); require('../modules/es.typed-array.reduce'); require('../modules/es.typed-array.reduce-right'); require('../modules/es.typed-array.reverse'); require('../modules/es.typed-array.set'); require('../modules/es.typed-array.slice'); require('../modules/es.typed-array.some'); require('../modules/es.typed-array.sort'); require('../modules/es.typed-array.subarray'); require('../modules/es.typed-array.to-locale-string'); require('../modules/es.typed-array.to-string'); require('../modules/es.reflect.apply'); require('../modules/es.reflect.construct'); require('../modules/es.reflect.define-property'); require('../modules/es.reflect.delete-property'); require('../modules/es.reflect.get'); require('../modules/es.reflect.get-own-property-descriptor'); require('../modules/es.reflect.get-prototype-of'); require('../modules/es.reflect.has'); require('../modules/es.reflect.is-extensible'); require('../modules/es.reflect.own-keys'); require('../modules/es.reflect.prevent-extensions'); require('../modules/es.reflect.set'); require('../modules/es.reflect.set-prototype-of'); require('../modules/es.reflect.to-string-tag'); var path = require('../internals/path'); module.exports = path; apollo-server-demo/node_modules/core-js/es/array/ 0000755 0001750 0000144 00000000000 14067647701 021532 5 ustar andreh users apollo-server-demo/node_modules/core-js/es/array/sort.js 0000644 0001750 0000144 00000000223 03560116604 023041 0 ustar andreh users require('../../modules/es.array.sort'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'sort'); apollo-server-demo/node_modules/core-js/es/array/reduce.js 0000644 0001750 0000144 00000000227 03560116604 023325 0 ustar andreh users require('../../modules/es.array.reduce'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'reduce'); apollo-server-demo/node_modules/core-js/es/array/some.js 0000644 0001750 0000144 00000000223 03560116604 023015 0 ustar andreh users require('../../modules/es.array.some'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'some'); apollo-server-demo/node_modules/core-js/es/array/index.js 0000644 0001750 0000144 00000002536 03560116604 023172 0 ustar andreh users require('../../modules/es.string.iterator'); require('../../modules/es.array.from'); require('../../modules/es.array.is-array'); require('../../modules/es.array.of'); require('../../modules/es.array.concat'); require('../../modules/es.array.copy-within'); require('../../modules/es.array.every'); require('../../modules/es.array.fill'); require('../../modules/es.array.filter'); require('../../modules/es.array.find'); require('../../modules/es.array.find-index'); require('../../modules/es.array.flat'); require('../../modules/es.array.flat-map'); require('../../modules/es.array.for-each'); require('../../modules/es.array.includes'); require('../../modules/es.array.index-of'); require('../../modules/es.array.iterator'); require('../../modules/es.array.join'); require('../../modules/es.array.last-index-of'); require('../../modules/es.array.map'); require('../../modules/es.array.reduce'); require('../../modules/es.array.reduce-right'); require('../../modules/es.array.reverse'); require('../../modules/es.array.slice'); require('../../modules/es.array.some'); require('../../modules/es.array.sort'); require('../../modules/es.array.species'); require('../../modules/es.array.splice'); require('../../modules/es.array.unscopables.flat'); require('../../modules/es.array.unscopables.flat-map'); var path = require('../../internals/path'); module.exports = path.Array; apollo-server-demo/node_modules/core-js/es/array/iterator.js 0000644 0001750 0000144 00000000231 03560116604 023702 0 ustar andreh users require('../../modules/es.array.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'values'); apollo-server-demo/node_modules/core-js/es/array/copy-within.js 0000644 0001750 0000144 00000000240 03560116604 024323 0 ustar andreh users require('../../modules/es.array.copy-within'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'copyWithin'); apollo-server-demo/node_modules/core-js/es/array/map.js 0000644 0001750 0000144 00000000221 03560116604 022625 0 ustar andreh users require('../../modules/es.array.map'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'map'); apollo-server-demo/node_modules/core-js/es/array/includes.js 0000644 0001750 0000144 00000000233 03560116604 023661 0 ustar andreh users require('../../modules/es.array.includes'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'includes'); apollo-server-demo/node_modules/core-js/es/array/reduce-right.js 0000644 0001750 0000144 00000000242 03560116604 024435 0 ustar andreh users require('../../modules/es.array.reduce-right'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'reduceRight'); apollo-server-demo/node_modules/core-js/es/array/every.js 0000644 0001750 0000144 00000000225 03560116604 023206 0 ustar andreh users require('../../modules/es.array.every'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'every'); apollo-server-demo/node_modules/core-js/es/array/fill.js 0000644 0001750 0000144 00000000223 03560116604 023000 0 ustar andreh users require('../../modules/es.array.fill'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'fill'); apollo-server-demo/node_modules/core-js/es/array/flat-map.js 0000644 0001750 0000144 00000000322 03560116604 023553 0 ustar andreh users require('../../modules/es.array.flat-map'); require('../../modules/es.array.unscopables.flat-map'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'flatMap'); apollo-server-demo/node_modules/core-js/es/array/index-of.js 0000644 0001750 0000144 00000000232 03560116604 023563 0 ustar andreh users require('../../modules/es.array.index-of'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'indexOf'); apollo-server-demo/node_modules/core-js/es/array/virtual/ 0000755 0001750 0000144 00000000000 14067647701 023220 5 ustar andreh users apollo-server-demo/node_modules/core-js/es/array/virtual/sort.js 0000644 0001750 0000144 00000000231 03560116604 024526 0 ustar andreh users require('../../../modules/es.array.sort'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').sort; apollo-server-demo/node_modules/core-js/es/array/virtual/reduce.js 0000644 0001750 0000144 00000000235 03560116604 025012 0 ustar andreh users require('../../../modules/es.array.reduce'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').reduce; apollo-server-demo/node_modules/core-js/es/array/virtual/some.js 0000644 0001750 0000144 00000000231 03560116604 024502 0 ustar andreh users require('../../../modules/es.array.some'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').some; apollo-server-demo/node_modules/core-js/es/array/virtual/index.js 0000644 0001750 0000144 00000002444 03560116604 024656 0 ustar andreh users require('../../../modules/es.array.concat'); require('../../../modules/es.array.copy-within'); require('../../../modules/es.array.every'); require('../../../modules/es.array.fill'); require('../../../modules/es.array.filter'); require('../../../modules/es.array.find'); require('../../../modules/es.array.find-index'); require('../../../modules/es.array.flat'); require('../../../modules/es.array.flat-map'); require('../../../modules/es.array.for-each'); require('../../../modules/es.array.includes'); require('../../../modules/es.array.index-of'); require('../../../modules/es.array.iterator'); require('../../../modules/es.array.join'); require('../../../modules/es.array.last-index-of'); require('../../../modules/es.array.map'); require('../../../modules/es.array.reduce'); require('../../../modules/es.array.reduce-right'); require('../../../modules/es.array.reverse'); require('../../../modules/es.array.slice'); require('../../../modules/es.array.some'); require('../../../modules/es.array.sort'); require('../../../modules/es.array.species'); require('../../../modules/es.array.splice'); require('../../../modules/es.array.unscopables.flat'); require('../../../modules/es.array.unscopables.flat-map'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array'); apollo-server-demo/node_modules/core-js/es/array/virtual/iterator.js 0000644 0001750 0000144 00000000237 03560116604 025376 0 ustar andreh users require('../../../modules/es.array.iterator'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').values; apollo-server-demo/node_modules/core-js/es/array/virtual/copy-within.js 0000644 0001750 0000144 00000000246 03560116604 026017 0 ustar andreh users require('../../../modules/es.array.copy-within'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').copyWithin; apollo-server-demo/node_modules/core-js/es/array/virtual/map.js 0000644 0001750 0000144 00000000227 03560116604 024321 0 ustar andreh users require('../../../modules/es.array.map'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').map; apollo-server-demo/node_modules/core-js/es/array/virtual/includes.js 0000644 0001750 0000144 00000000241 03560116604 025346 0 ustar andreh users require('../../../modules/es.array.includes'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').includes; apollo-server-demo/node_modules/core-js/es/array/virtual/reduce-right.js 0000644 0001750 0000144 00000000250 03560116604 026122 0 ustar andreh users require('../../../modules/es.array.reduce-right'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').reduceRight; apollo-server-demo/node_modules/core-js/es/array/virtual/every.js 0000644 0001750 0000144 00000000233 03560116604 024673 0 ustar andreh users require('../../../modules/es.array.every'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').every; apollo-server-demo/node_modules/core-js/es/array/virtual/fill.js 0000644 0001750 0000144 00000000231 03560116604 024465 0 ustar andreh users require('../../../modules/es.array.fill'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').fill; apollo-server-demo/node_modules/core-js/es/array/virtual/flat-map.js 0000644 0001750 0000144 00000000333 03560116604 025243 0 ustar andreh users require('../../../modules/es.array.flat-map'); require('../../../modules/es.array.unscopables.flat-map'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').flatMap; apollo-server-demo/node_modules/core-js/es/array/virtual/index-of.js 0000644 0001750 0000144 00000000240 03560116604 025250 0 ustar andreh users require('../../../modules/es.array.index-of'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').indexOf; apollo-server-demo/node_modules/core-js/es/array/virtual/splice.js 0000644 0001750 0000144 00000000235 03560116604 025022 0 ustar andreh users require('../../../modules/es.array.splice'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').splice; apollo-server-demo/node_modules/core-js/es/array/virtual/last-index-of.js 0000644 0001750 0000144 00000000251 03560116604 026213 0 ustar andreh users require('../../../modules/es.array.last-index-of'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').lastIndexOf; apollo-server-demo/node_modules/core-js/es/array/virtual/flat.js 0000644 0001750 0000144 00000000320 03560116604 024464 0 ustar andreh users require('../../../modules/es.array.flat'); require('../../../modules/es.array.unscopables.flat'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').flat; apollo-server-demo/node_modules/core-js/es/array/virtual/values.js 0000644 0001750 0000144 00000000237 03560116604 025044 0 ustar andreh users require('../../../modules/es.array.iterator'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').values; apollo-server-demo/node_modules/core-js/es/array/virtual/join.js 0000644 0001750 0000144 00000000231 03560116604 024476 0 ustar andreh users require('../../../modules/es.array.join'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').join; apollo-server-demo/node_modules/core-js/es/array/virtual/entries.js 0000644 0001750 0000144 00000000240 03560116604 025210 0 ustar andreh users require('../../../modules/es.array.iterator'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').entries; apollo-server-demo/node_modules/core-js/es/array/virtual/filter-out.js 0000644 0001750 0000144 00000000250 03560116604 025632 0 ustar andreh users require('../../../modules/esnext.array.filter-out'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').filterOut; apollo-server-demo/node_modules/core-js/es/array/virtual/filter.js 0000644 0001750 0000144 00000000235 03560116604 025030 0 ustar andreh users require('../../../modules/es.array.filter'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').filter; apollo-server-demo/node_modules/core-js/es/array/virtual/concat.js 0000644 0001750 0000144 00000000235 03560116604 025012 0 ustar andreh users require('../../../modules/es.array.concat'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').concat; apollo-server-demo/node_modules/core-js/es/array/virtual/for-each.js 0000644 0001750 0000144 00000000240 03560116604 025223 0 ustar andreh users require('../../../modules/es.array.for-each'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').forEach; apollo-server-demo/node_modules/core-js/es/array/virtual/find-index.js 0000644 0001750 0000144 00000000244 03560116604 025570 0 ustar andreh users require('../../../modules/es.array.find-index'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').findIndex; apollo-server-demo/node_modules/core-js/es/array/virtual/keys.js 0000644 0001750 0000144 00000000235 03560116604 024516 0 ustar andreh users require('../../../modules/es.array.iterator'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').keys; apollo-server-demo/node_modules/core-js/es/array/virtual/find.js 0000644 0001750 0000144 00000000231 03560116604 024457 0 ustar andreh users require('../../../modules/es.array.find'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').find; apollo-server-demo/node_modules/core-js/es/array/virtual/reverse.js 0000644 0001750 0000144 00000000237 03560116604 025220 0 ustar andreh users require('../../../modules/es.array.reverse'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').reverse; apollo-server-demo/node_modules/core-js/es/array/virtual/slice.js 0000644 0001750 0000144 00000000233 03560116604 024640 0 ustar andreh users require('../../../modules/es.array.slice'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Array').slice; apollo-server-demo/node_modules/core-js/es/array/splice.js 0000644 0001750 0000144 00000000227 03560116604 023335 0 ustar andreh users require('../../modules/es.array.splice'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'splice'); apollo-server-demo/node_modules/core-js/es/array/last-index-of.js 0000644 0001750 0000144 00000000243 03560116604 024526 0 ustar andreh users require('../../modules/es.array.last-index-of'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'lastIndexOf'); apollo-server-demo/node_modules/core-js/es/array/flat.js 0000644 0001750 0000144 00000000307 03560116604 023003 0 ustar andreh users require('../../modules/es.array.flat'); require('../../modules/es.array.unscopables.flat'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'flat'); apollo-server-demo/node_modules/core-js/es/array/values.js 0000644 0001750 0000144 00000000231 03560116604 023350 0 ustar andreh users require('../../modules/es.array.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'values'); apollo-server-demo/node_modules/core-js/es/array/join.js 0000644 0001750 0000144 00000000223 03560116604 023011 0 ustar andreh users require('../../modules/es.array.join'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'join'); apollo-server-demo/node_modules/core-js/es/array/from.js 0000644 0001750 0000144 00000000244 03560116604 023020 0 ustar andreh users require('../../modules/es.string.iterator'); require('../../modules/es.array.from'); var path = require('../../internals/path'); module.exports = path.Array.from; apollo-server-demo/node_modules/core-js/es/array/entries.js 0000644 0001750 0000144 00000000232 03560116604 023523 0 ustar andreh users require('../../modules/es.array.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'entries'); apollo-server-demo/node_modules/core-js/es/array/filter.js 0000644 0001750 0000144 00000000227 03560116604 023343 0 ustar andreh users require('../../modules/es.array.filter'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'filter'); apollo-server-demo/node_modules/core-js/es/array/concat.js 0000644 0001750 0000144 00000000227 03560116604 023325 0 ustar andreh users require('../../modules/es.array.concat'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'concat'); apollo-server-demo/node_modules/core-js/es/array/for-each.js 0000644 0001750 0000144 00000000232 03560116604 023536 0 ustar andreh users require('../../modules/es.array.for-each'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'forEach'); apollo-server-demo/node_modules/core-js/es/array/is-array.js 0000644 0001750 0000144 00000000176 03560116604 023610 0 ustar andreh users require('../../modules/es.array.is-array'); var path = require('../../internals/path'); module.exports = path.Array.isArray; apollo-server-demo/node_modules/core-js/es/array/find-index.js 0000644 0001750 0000144 00000000236 03560116604 024103 0 ustar andreh users require('../../modules/es.array.find-index'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'findIndex'); apollo-server-demo/node_modules/core-js/es/array/keys.js 0000644 0001750 0000144 00000000227 03560116604 023031 0 ustar andreh users require('../../modules/es.array.iterator'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'keys'); apollo-server-demo/node_modules/core-js/es/array/find.js 0000644 0001750 0000144 00000000223 03560116604 022772 0 ustar andreh users require('../../modules/es.array.find'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'find'); apollo-server-demo/node_modules/core-js/es/array/of.js 0000644 0001750 0000144 00000000163 03560116604 022461 0 ustar andreh users require('../../modules/es.array.of'); var path = require('../../internals/path'); module.exports = path.Array.of; apollo-server-demo/node_modules/core-js/es/array/reverse.js 0000644 0001750 0000144 00000000231 03560116604 023524 0 ustar andreh users require('../../modules/es.array.reverse'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'reverse'); apollo-server-demo/node_modules/core-js/es/array/slice.js 0000644 0001750 0000144 00000000225 03560116604 023153 0 ustar andreh users require('../../modules/es.array.slice'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Array', 'slice'); apollo-server-demo/node_modules/core-js/es/array-buffer/ 0000755 0001750 0000144 00000000000 14067647701 023001 5 ustar andreh users apollo-server-demo/node_modules/core-js/es/array-buffer/index.js 0000644 0001750 0000144 00000000426 03560116604 024435 0 ustar andreh users require('../../modules/es.array-buffer.constructor'); require('../../modules/es.array-buffer.is-view'); require('../../modules/es.array-buffer.slice'); require('../../modules/es.object.to-string'); var path = require('../../internals/path'); module.exports = path.ArrayBuffer; apollo-server-demo/node_modules/core-js/es/array-buffer/is-view.js 0000644 0001750 0000144 00000000211 03560116604 024701 0 ustar andreh users require('../../modules/es.array-buffer.is-view'); var path = require('../../internals/path'); module.exports = path.ArrayBuffer.isView; apollo-server-demo/node_modules/core-js/es/array-buffer/constructor.js 0000644 0001750 0000144 00000000264 03560116604 025713 0 ustar andreh users require('../../modules/es.array-buffer.constructor'); require('../../modules/es.object.to-string'); var path = require('../../internals/path'); module.exports = path.ArrayBuffer; apollo-server-demo/node_modules/core-js/es/array-buffer/slice.js 0000644 0001750 0000144 00000000060 03560116604 024417 0 ustar andreh users require('../../modules/es.array-buffer.slice'); apollo-server-demo/node_modules/core-js/es/README.md 0000644 0001750 0000144 00000000216 03560116604 021657 0 ustar andreh users This folder contains entry points for [stable ECMAScript features](https://github.com/zloirock/core-js/tree/v3#ecmascript) with dependencies. apollo-server-demo/node_modules/core-js/es/symbol/ 0000755 0001750 0000144 00000000000 14067647701 021721 5 ustar andreh users apollo-server-demo/node_modules/core-js/es/symbol/index.js 0000644 0001750 0000144 00000001754 03560116604 023362 0 ustar andreh users require('../../modules/es.array.concat'); require('../../modules/es.object.to-string'); require('../../modules/es.symbol'); require('../../modules/es.symbol.async-iterator'); require('../../modules/es.symbol.description'); require('../../modules/es.symbol.has-instance'); require('../../modules/es.symbol.is-concat-spreadable'); require('../../modules/es.symbol.iterator'); require('../../modules/es.symbol.match'); require('../../modules/es.symbol.match-all'); require('../../modules/es.symbol.replace'); require('../../modules/es.symbol.search'); require('../../modules/es.symbol.species'); require('../../modules/es.symbol.split'); require('../../modules/es.symbol.to-primitive'); require('../../modules/es.symbol.to-string-tag'); require('../../modules/es.symbol.unscopables'); require('../../modules/es.json.to-string-tag'); require('../../modules/es.math.to-string-tag'); require('../../modules/es.reflect.to-string-tag'); var path = require('../../internals/path'); module.exports = path.Symbol; apollo-server-demo/node_modules/core-js/es/symbol/iterator.js 0000644 0001750 0000144 00000000450 03560116604 024074 0 ustar andreh users require('../../modules/es.symbol.iterator'); require('../../modules/es.string.iterator'); require('../../modules/web.dom-collections.iterator'); var WrappedWellKnownSymbolModule = require('../../internals/well-known-symbol-wrapped'); module.exports = WrappedWellKnownSymbolModule.f('iterator'); apollo-server-demo/node_modules/core-js/es/symbol/to-string-tag.js 0000644 0001750 0000144 00000000615 03560116604 024745 0 ustar andreh users require('../../modules/es.symbol.to-string-tag'); require('../../modules/es.object.to-string'); require('../../modules/es.json.to-string-tag'); require('../../modules/es.math.to-string-tag'); require('../../modules/es.reflect.to-string-tag'); var WrappedWellKnownSymbolModule = require('../../internals/well-known-symbol-wrapped'); module.exports = WrappedWellKnownSymbolModule.f('toStringTag'); apollo-server-demo/node_modules/core-js/es/symbol/match.js 0000644 0001750 0000144 00000000350 03560116604 023336 0 ustar andreh users require('../../modules/es.symbol.match'); require('../../modules/es.string.match'); var WrappedWellKnownSymbolModule = require('../../internals/well-known-symbol-wrapped'); module.exports = WrappedWellKnownSymbolModule.f('match'); apollo-server-demo/node_modules/core-js/es/symbol/unscopables.js 0000644 0001750 0000144 00000000312 03560116604 024556 0 ustar andreh users require('../../modules/es.symbol.unscopables'); var WrappedWellKnownSymbolModule = require('../../internals/well-known-symbol-wrapped'); module.exports = WrappedWellKnownSymbolModule.f('unscopables'); apollo-server-demo/node_modules/core-js/es/symbol/is-concat-spreadable.js 0000644 0001750 0000144 00000000404 03560116604 026222 0 ustar andreh users require('../../modules/es.symbol.is-concat-spreadable'); require('../../modules/es.array.concat'); var WrappedWellKnownSymbolModule = require('../../internals/well-known-symbol-wrapped'); module.exports = WrappedWellKnownSymbolModule.f('isConcatSpreadable'); apollo-server-demo/node_modules/core-js/es/symbol/for.js 0000644 0001750 0000144 00000000166 03560116604 023035 0 ustar andreh users require('../../modules/es.symbol'); var path = require('../../internals/path'); module.exports = path.Symbol['for']; apollo-server-demo/node_modules/core-js/es/symbol/key-for.js 0000644 0001750 0000144 00000000166 03560116604 023623 0 ustar andreh users require('../../modules/es.symbol'); var path = require('../../internals/path'); module.exports = path.Symbol.keyFor; apollo-server-demo/node_modules/core-js/es/symbol/to-primitive.js 0000644 0001750 0000144 00000000313 03560116604 024671 0 ustar andreh users require('../../modules/es.symbol.to-primitive'); var WrappedWellKnownSymbolModule = require('../../internals/well-known-symbol-wrapped'); module.exports = WrappedWellKnownSymbolModule.f('toPrimitive'); apollo-server-demo/node_modules/core-js/es/symbol/async-iterator.js 0000644 0001750 0000144 00000000317 03560116604 025211 0 ustar andreh users require('../../modules/es.symbol.async-iterator'); var WrappedWellKnownSymbolModule = require('../../internals/well-known-symbol-wrapped'); module.exports = WrappedWellKnownSymbolModule.f('asyncIterator'); apollo-server-demo/node_modules/core-js/es/symbol/split.js 0000644 0001750 0000144 00000000350 03560116604 023375 0 ustar andreh users require('../../modules/es.symbol.split'); require('../../modules/es.string.split'); var WrappedWellKnownSymbolModule = require('../../internals/well-known-symbol-wrapped'); module.exports = WrappedWellKnownSymbolModule.f('split'); apollo-server-demo/node_modules/core-js/es/symbol/has-instance.js 0000644 0001750 0000144 00000000376 03560116604 024627 0 ustar andreh users require('../../modules/es.symbol.has-instance'); require('../../modules/es.function.has-instance'); var WrappedWellKnownSymbolModule = require('../../internals/well-known-symbol-wrapped'); module.exports = WrappedWellKnownSymbolModule.f('hasInstance'); apollo-server-demo/node_modules/core-js/es/symbol/species.js 0000644 0001750 0000144 00000000302 03560116604 023672 0 ustar andreh users require('../../modules/es.symbol.species'); var WrappedWellKnownSymbolModule = require('../../internals/well-known-symbol-wrapped'); module.exports = WrappedWellKnownSymbolModule.f('species'); apollo-server-demo/node_modules/core-js/es/symbol/search.js 0000644 0001750 0000144 00000000353 03560116604 023512 0 ustar andreh users require('../../modules/es.symbol.search'); require('../../modules/es.string.search'); var WrappedWellKnownSymbolModule = require('../../internals/well-known-symbol-wrapped'); module.exports = WrappedWellKnownSymbolModule.f('search'); apollo-server-demo/node_modules/core-js/es/symbol/replace.js 0000644 0001750 0000144 00000000356 03560116604 023663 0 ustar andreh users require('../../modules/es.symbol.replace'); require('../../modules/es.string.replace'); var WrappedWellKnownSymbolModule = require('../../internals/well-known-symbol-wrapped'); module.exports = WrappedWellKnownSymbolModule.f('replace'); apollo-server-demo/node_modules/core-js/es/symbol/description.js 0000644 0001750 0000144 00000000060 03560116604 024563 0 ustar andreh users require('../../modules/es.symbol.description'); apollo-server-demo/node_modules/core-js/es/symbol/match-all.js 0000644 0001750 0000144 00000000363 03560116604 024110 0 ustar andreh users require('../../modules/es.symbol.match-all'); require('../../modules/es.string.match-all'); var WrappedWellKnownSymbolModule = require('../../internals/well-known-symbol-wrapped'); module.exports = WrappedWellKnownSymbolModule.f('matchAll'); apollo-server-demo/node_modules/core-js/es/instance/ 0000755 0001750 0000144 00000000000 14067647701 022220 5 ustar andreh users apollo-server-demo/node_modules/core-js/es/instance/sort.js 0000644 0001750 0000144 00000000363 03560116604 023534 0 ustar andreh users var sort = require('../array/virtual/sort'); var ArrayPrototype = Array.prototype; module.exports = function (it) { var own = it.sort; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.sort) ? sort : own; }; apollo-server-demo/node_modules/core-js/es/instance/reduce.js 0000644 0001750 0000144 00000000375 03560116604 024017 0 ustar andreh users var reduce = require('../array/virtual/reduce'); var ArrayPrototype = Array.prototype; module.exports = function (it) { var own = it.reduce; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.reduce) ? reduce : own; }; apollo-server-demo/node_modules/core-js/es/instance/some.js 0000644 0001750 0000144 00000000363 03560116604 023510 0 ustar andreh users var some = require('../array/virtual/some'); var ArrayPrototype = Array.prototype; module.exports = function (it) { var own = it.some; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.some) ? some : own; }; apollo-server-demo/node_modules/core-js/es/instance/copy-within.js 0000644 0001750 0000144 00000000422 03560116604 025013 0 ustar andreh users var copyWithin = require('../array/virtual/copy-within'); var ArrayPrototype = Array.prototype; module.exports = function (it) { var own = it.copyWithin; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.copyWithin) ? copyWithin : own; }; apollo-server-demo/node_modules/core-js/es/instance/repeat.js 0000644 0001750 0000144 00000000441 03560116604 024022 0 ustar andreh users var repeat = require('../string/virtual/repeat'); var StringPrototype = String.prototype; module.exports = function (it) { var own = it.repeat; return typeof it === 'string' || it === StringPrototype || (it instanceof String && own === StringPrototype.repeat) ? repeat : own; }; apollo-server-demo/node_modules/core-js/es/instance/map.js 0000644 0001750 0000144 00000000356 03560116604 023324 0 ustar andreh users var map = require('../array/virtual/map'); var ArrayPrototype = Array.prototype; module.exports = function (it) { var own = it.map; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.map) ? map : own; }; apollo-server-demo/node_modules/core-js/es/instance/includes.js 0000644 0001750 0000144 00000001025 03560116604 024347 0 ustar andreh users var arrayIncludes = require('../array/virtual/includes'); var stringIncludes = require('../string/virtual/includes'); var ArrayPrototype = Array.prototype; var StringPrototype = String.prototype; module.exports = function (it) { var own = it.includes; if (it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.includes)) return arrayIncludes; if (typeof it === 'string' || it === StringPrototype || (it instanceof String && own === StringPrototype.includes)) { return stringIncludes; } return own; }; apollo-server-demo/node_modules/core-js/es/instance/reduce-right.js 0000644 0001750 0000144 00000000427 03560116604 025130 0 ustar andreh users var reduceRight = require('../array/virtual/reduce-right'); var ArrayPrototype = Array.prototype; module.exports = function (it) { var own = it.reduceRight; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.reduceRight) ? reduceRight : own; }; apollo-server-demo/node_modules/core-js/es/instance/code-point-at.js 0000644 0001750 0000144 00000000474 03560116604 025213 0 ustar andreh users var codePointAt = require('../string/virtual/code-point-at'); var StringPrototype = String.prototype; module.exports = function (it) { var own = it.codePointAt; return typeof it === 'string' || it === StringPrototype || (it instanceof String && own === StringPrototype.codePointAt) ? codePointAt : own; }; apollo-server-demo/node_modules/core-js/es/instance/every.js 0000644 0001750 0000144 00000000370 03560116604 023675 0 ustar andreh users var every = require('../array/virtual/every'); var ArrayPrototype = Array.prototype; module.exports = function (it) { var own = it.every; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.every) ? every : own; }; apollo-server-demo/node_modules/core-js/es/instance/fill.js 0000644 0001750 0000144 00000000363 03560116604 023473 0 ustar andreh users var fill = require('../array/virtual/fill'); var ArrayPrototype = Array.prototype; module.exports = function (it) { var own = it.fill; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.fill) ? fill : own; }; apollo-server-demo/node_modules/core-js/es/instance/flat-map.js 0000644 0001750 0000144 00000000403 03560116604 024241 0 ustar andreh users var flatMap = require('../array/virtual/flat-map'); var ArrayPrototype = Array.prototype; module.exports = function (it) { var own = it.flatMap; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.flatMap) ? flatMap : own; }; apollo-server-demo/node_modules/core-js/es/instance/index-of.js 0000644 0001750 0000144 00000000403 03560116604 024251 0 ustar andreh users var indexOf = require('../array/virtual/index-of'); var ArrayPrototype = Array.prototype; module.exports = function (it) { var own = it.indexOf; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.indexOf) ? indexOf : own; }; apollo-server-demo/node_modules/core-js/es/instance/trim-left.js 0000644 0001750 0000144 00000000454 03560116604 024451 0 ustar andreh users var trimLeft = require('../string/virtual/trim-left'); var StringPrototype = String.prototype; module.exports = function (it) { var own = it.trimLeft; return typeof it === 'string' || it === StringPrototype || (it instanceof String && own === StringPrototype.trimLeft) ? trimLeft : own; }; apollo-server-demo/node_modules/core-js/es/instance/pad-end.js 0000644 0001750 0000144 00000000442 03560116604 024053 0 ustar andreh users var padEnd = require('../string/virtual/pad-end'); var StringPrototype = String.prototype; module.exports = function (it) { var own = it.padEnd; return typeof it === 'string' || it === StringPrototype || (it instanceof String && own === StringPrototype.padEnd) ? padEnd : own; }; apollo-server-demo/node_modules/core-js/es/instance/bind.js 0000644 0001750 0000144 00000000405 03560116604 023456 0 ustar andreh users var bind = require('../function/virtual/bind'); var FunctionPrototype = Function.prototype; module.exports = function (it) { var own = it.bind; return it === FunctionPrototype || (it instanceof Function && own === FunctionPrototype.bind) ? bind : own; }; apollo-server-demo/node_modules/core-js/es/instance/splice.js 0000644 0001750 0000144 00000000375 03560116604 024027 0 ustar andreh users var splice = require('../array/virtual/splice'); var ArrayPrototype = Array.prototype; module.exports = function (it) { var own = it.splice; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.splice) ? splice : own; }; apollo-server-demo/node_modules/core-js/es/instance/last-index-of.js 0000644 0001750 0000144 00000000430 03560116604 025212 0 ustar andreh users var lastIndexOf = require('../array/virtual/last-index-of'); var ArrayPrototype = Array.prototype; module.exports = function (it) { var own = it.lastIndexOf; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.lastIndexOf) ? lastIndexOf : own; }; apollo-server-demo/node_modules/core-js/es/instance/flags.js 0000644 0001750 0000144 00000000334 03560116604 023637 0 ustar andreh users var flags = require('../regexp/flags'); var RegExpPrototype = RegExp.prototype; module.exports = function (it) { return (it === RegExpPrototype || it instanceof RegExp) && !('flags' in it) ? flags(it) : it.flags; }; apollo-server-demo/node_modules/core-js/es/instance/flat.js 0000644 0001750 0000144 00000000363 03560116604 023473 0 ustar andreh users var flat = require('../array/virtual/flat'); var ArrayPrototype = Array.prototype; module.exports = function (it) { var own = it.flat; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.flat) ? flat : own; }; apollo-server-demo/node_modules/core-js/es/instance/values.js 0000644 0001750 0000144 00000000375 03560116604 024047 0 ustar andreh users var values = require('../array/virtual/values'); var ArrayPrototype = Array.prototype; module.exports = function (it) { var own = it.values; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.values) ? values : own; }; apollo-server-demo/node_modules/core-js/es/instance/starts-with.js 0000644 0001750 0000144 00000000466 03560116604 025042 0 ustar andreh users var startsWith = require('../string/virtual/starts-with'); var StringPrototype = String.prototype; module.exports = function (it) { var own = it.startsWith; return typeof it === 'string' || it === StringPrototype || (it instanceof String && own === StringPrototype.startsWith) ? startsWith : own; }; apollo-server-demo/node_modules/core-js/es/instance/entries.js 0000644 0001750 0000144 00000000402 03560116604 024210 0 ustar andreh users var entries = require('../array/virtual/entries'); var ArrayPrototype = Array.prototype; module.exports = function (it) { var own = it.entries; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.entries) ? entries : own; }; apollo-server-demo/node_modules/core-js/es/instance/trim-end.js 0000644 0001750 0000144 00000000447 03560116604 024267 0 ustar andreh users var trimEnd = require('../string/virtual/trim-end'); var StringPrototype = String.prototype; module.exports = function (it) { var own = it.trimEnd; return typeof it === 'string' || it === StringPrototype || (it instanceof String && own === StringPrototype.trimEnd) ? trimEnd : own; }; apollo-server-demo/node_modules/core-js/es/instance/filter.js 0000644 0001750 0000144 00000000375 03560116604 024035 0 ustar andreh users var filter = require('../array/virtual/filter'); var ArrayPrototype = Array.prototype; module.exports = function (it) { var own = it.filter; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.filter) ? filter : own; }; apollo-server-demo/node_modules/core-js/es/instance/trim-start.js 0000644 0001750 0000144 00000000461 03560116604 024652 0 ustar andreh users var trimStart = require('../string/virtual/trim-start'); var StringPrototype = String.prototype; module.exports = function (it) { var own = it.trimStart; return typeof it === 'string' || it === StringPrototype || (it instanceof String && own === StringPrototype.trimStart) ? trimStart : own; }; apollo-server-demo/node_modules/core-js/es/instance/concat.js 0000644 0001750 0000144 00000000375 03560116604 024017 0 ustar andreh users var concat = require('../array/virtual/concat'); var ArrayPrototype = Array.prototype; module.exports = function (it) { var own = it.concat; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.concat) ? concat : own; }; apollo-server-demo/node_modules/core-js/es/instance/trim.js 0000644 0001750 0000144 00000000427 03560116604 023521 0 ustar andreh users var trim = require('../string/virtual/trim'); var StringPrototype = String.prototype; module.exports = function (it) { var own = it.trim; return typeof it === 'string' || it === StringPrototype || (it instanceof String && own === StringPrototype.trim) ? trim : own; }; apollo-server-demo/node_modules/core-js/es/instance/for-each.js 0000644 0001750 0000144 00000000403 03560116604 024224 0 ustar andreh users var forEach = require('../array/virtual/for-each'); var ArrayPrototype = Array.prototype; module.exports = function (it) { var own = it.forEach; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.forEach) ? forEach : own; }; apollo-server-demo/node_modules/core-js/es/instance/find-index.js 0000644 0001750 0000144 00000000415 03560116604 024570 0 ustar andreh users var findIndex = require('../array/virtual/find-index'); var ArrayPrototype = Array.prototype; module.exports = function (it) { var own = it.findIndex; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.findIndex) ? findIndex : own; }; apollo-server-demo/node_modules/core-js/es/instance/pad-start.js 0000644 0001750 0000144 00000000454 03560116604 024445 0 ustar andreh users var padStart = require('../string/virtual/pad-start'); var StringPrototype = String.prototype; module.exports = function (it) { var own = it.padStart; return typeof it === 'string' || it === StringPrototype || (it instanceof String && own === StringPrototype.padStart) ? padStart : own; }; apollo-server-demo/node_modules/core-js/es/instance/keys.js 0000644 0001750 0000144 00000000363 03560116604 023520 0 ustar andreh users var keys = require('../array/virtual/keys'); var ArrayPrototype = Array.prototype; module.exports = function (it) { var own = it.keys; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.keys) ? keys : own; }; apollo-server-demo/node_modules/core-js/es/instance/find.js 0000644 0001750 0000144 00000000363 03560116604 023465 0 ustar andreh users var find = require('../array/virtual/find'); var ArrayPrototype = Array.prototype; module.exports = function (it) { var own = it.find; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.find) ? find : own; }; apollo-server-demo/node_modules/core-js/es/instance/replace-all.js 0000644 0001750 0000144 00000000466 03560116604 024732 0 ustar andreh users var replaceAll = require('../string/virtual/replace-all'); var StringPrototype = String.prototype; module.exports = function (it) { var own = it.replaceAll; return typeof it === 'string' || it === StringPrototype || (it instanceof String && own === StringPrototype.replaceAll) ? replaceAll : own; }; apollo-server-demo/node_modules/core-js/es/instance/match-all.js 0000644 0001750 0000144 00000000454 03560116604 024410 0 ustar andreh users var matchAll = require('../string/virtual/match-all'); var StringPrototype = String.prototype; module.exports = function (it) { var own = it.matchAll; return typeof it === 'string' || it === StringPrototype || (it instanceof String && own === StringPrototype.matchAll) ? matchAll : own; }; apollo-server-demo/node_modules/core-js/es/instance/reverse.js 0000644 0001750 0000144 00000000402 03560116604 024212 0 ustar andreh users var reverse = require('../array/virtual/reverse'); var ArrayPrototype = Array.prototype; module.exports = function (it) { var own = it.reverse; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.reverse) ? reverse : own; }; apollo-server-demo/node_modules/core-js/es/instance/trim-right.js 0000644 0001750 0000144 00000000461 03560116604 024632 0 ustar andreh users var trimRight = require('../string/virtual/trim-right'); var StringPrototype = String.prototype; module.exports = function (it) { var own = it.trimRight; return typeof it === 'string' || it === StringPrototype || (it instanceof String && own === StringPrototype.trimRight) ? trimRight : own; }; apollo-server-demo/node_modules/core-js/es/instance/ends-with.js 0000644 0001750 0000144 00000000454 03560116604 024450 0 ustar andreh users var endsWith = require('../string/virtual/ends-with'); var StringPrototype = String.prototype; module.exports = function (it) { var own = it.endsWith; return typeof it === 'string' || it === StringPrototype || (it instanceof String && own === StringPrototype.endsWith) ? endsWith : own; }; apollo-server-demo/node_modules/core-js/es/instance/slice.js 0000644 0001750 0000144 00000000370 03560116604 023642 0 ustar andreh users var slice = require('../array/virtual/slice'); var ArrayPrototype = Array.prototype; module.exports = function (it) { var own = it.slice; return it === ArrayPrototype || (it instanceof Array && own === ArrayPrototype.slice) ? slice : own; }; apollo-server-demo/node_modules/core-js/es/map/ 0000755 0001750 0000144 00000000000 14067647701 021171 5 ustar andreh users apollo-server-demo/node_modules/core-js/es/map/index.js 0000644 0001750 0000144 00000000373 03560116604 022626 0 ustar andreh users require('../../modules/es.map'); require('../../modules/es.object.to-string'); require('../../modules/es.string.iterator'); require('../../modules/web.dom-collections.iterator'); var path = require('../../internals/path'); module.exports = path.Map; apollo-server-demo/node_modules/core-js/es/string/ 0000755 0001750 0000144 00000000000 14067647701 021722 5 ustar andreh users apollo-server-demo/node_modules/core-js/es/string/index.js 0000644 0001750 0000144 00000002762 03560116604 023363 0 ustar andreh users require('../../modules/es.regexp.exec'); require('../../modules/es.string.from-code-point'); require('../../modules/es.string.raw'); require('../../modules/es.string.code-point-at'); require('../../modules/es.string.ends-with'); require('../../modules/es.string.includes'); require('../../modules/es.string.match'); require('../../modules/es.string.match-all'); require('../../modules/es.string.pad-end'); require('../../modules/es.string.pad-start'); require('../../modules/es.string.repeat'); require('../../modules/es.string.replace'); require('../../modules/es.string.replace-all'); require('../../modules/es.string.search'); require('../../modules/es.string.split'); require('../../modules/es.string.starts-with'); require('../../modules/es.string.trim'); require('../../modules/es.string.trim-start'); require('../../modules/es.string.trim-end'); require('../../modules/es.string.iterator'); require('../../modules/es.string.anchor'); require('../../modules/es.string.big'); require('../../modules/es.string.blink'); require('../../modules/es.string.bold'); require('../../modules/es.string.fixed'); require('../../modules/es.string.fontcolor'); require('../../modules/es.string.fontsize'); require('../../modules/es.string.italics'); require('../../modules/es.string.link'); require('../../modules/es.string.small'); require('../../modules/es.string.strike'); require('../../modules/es.string.sub'); require('../../modules/es.string.sup'); var path = require('../../internals/path'); module.exports = path.String; apollo-server-demo/node_modules/core-js/es/string/iterator.js 0000644 0001750 0000144 00000000330 03560116604 024072 0 ustar andreh users require('../../modules/es.string.iterator'); var Iterators = require('../../internals/iterators'); var getStringIterator = Iterators.String; module.exports = function (it) { return getStringIterator.call(it); }; apollo-server-demo/node_modules/core-js/es/string/repeat.js 0000644 0001750 0000144 00000000231 03560116604 023521 0 ustar andreh users require('../../modules/es.string.repeat'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'repeat'); apollo-server-demo/node_modules/core-js/es/string/includes.js 0000644 0001750 0000144 00000000235 03560116604 024053 0 ustar andreh users require('../../modules/es.string.includes'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'includes'); apollo-server-demo/node_modules/core-js/es/string/italics.js 0000644 0001750 0000144 00000000233 03560116604 023673 0 ustar andreh users require('../../modules/es.string.italics'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'italics'); apollo-server-demo/node_modules/core-js/es/string/sub.js 0000644 0001750 0000144 00000000223 03560116604 023033 0 ustar andreh users require('../../modules/es.string.sub'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'sub'); apollo-server-demo/node_modules/core-js/es/string/fixed.js 0000644 0001750 0000144 00000000227 03560116604 023345 0 ustar andreh users require('../../modules/es.string.fixed'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'fixed'); apollo-server-demo/node_modules/core-js/es/string/code-point-at.js 0000644 0001750 0000144 00000000245 03560116604 024711 0 ustar andreh users require('../../modules/es.string.code-point-at'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'codePointAt'); apollo-server-demo/node_modules/core-js/es/string/match.js 0000644 0001750 0000144 00000000300 03560116604 023332 0 ustar andreh users require('../../modules/es.regexp.exec'); require('../../modules/es.string.match'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'match'); apollo-server-demo/node_modules/core-js/es/string/trim-left.js 0000644 0001750 0000144 00000000237 03560116604 024152 0 ustar andreh users require('../../modules/es.string.trim-start'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'trimLeft'); apollo-server-demo/node_modules/core-js/es/string/pad-end.js 0000644 0001750 0000144 00000000232 03560116604 023552 0 ustar andreh users require('../../modules/es.string.pad-end'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'padEnd'); apollo-server-demo/node_modules/core-js/es/string/virtual/ 0000755 0001750 0000144 00000000000 14067647701 023410 5 ustar andreh users apollo-server-demo/node_modules/core-js/es/string/virtual/index.js 0000644 0001750 0000144 00000002746 03560116604 025053 0 ustar andreh users require('../../../modules/es.string.code-point-at'); require('../../../modules/es.string.ends-with'); require('../../../modules/es.string.includes'); require('../../../modules/es.string.match'); require('../../../modules/es.string.match-all'); require('../../../modules/es.string.pad-end'); require('../../../modules/es.string.pad-start'); require('../../../modules/es.string.repeat'); require('../../../modules/es.string.replace'); require('../../../modules/es.string.replace-all'); require('../../../modules/es.string.search'); require('../../../modules/es.string.split'); require('../../../modules/es.string.starts-with'); require('../../../modules/es.string.trim'); require('../../../modules/es.string.trim-start'); require('../../../modules/es.string.trim-end'); require('../../../modules/es.string.iterator'); require('../../../modules/es.string.anchor'); require('../../../modules/es.string.big'); require('../../../modules/es.string.blink'); require('../../../modules/es.string.bold'); require('../../../modules/es.string.fixed'); require('../../../modules/es.string.fontcolor'); require('../../../modules/es.string.fontsize'); require('../../../modules/es.string.italics'); require('../../../modules/es.string.link'); require('../../../modules/es.string.small'); require('../../../modules/es.string.strike'); require('../../../modules/es.string.sub'); require('../../../modules/es.string.sup'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String'); apollo-server-demo/node_modules/core-js/es/string/virtual/iterator.js 0000644 0001750 0000144 00000000215 03560116604 025562 0 ustar andreh users require('../../../modules/es.string.iterator'); var Iterators = require('../../../internals/iterators'); module.exports = Iterators.String; apollo-server-demo/node_modules/core-js/es/string/virtual/repeat.js 0000644 0001750 0000144 00000000237 03560116604 025215 0 ustar andreh users require('../../../modules/es.string.repeat'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').repeat; apollo-server-demo/node_modules/core-js/es/string/virtual/includes.js 0000644 0001750 0000144 00000000243 03560116604 025540 0 ustar andreh users require('../../../modules/es.string.includes'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').includes; apollo-server-demo/node_modules/core-js/es/string/virtual/italics.js 0000644 0001750 0000144 00000000241 03560116604 025360 0 ustar andreh users require('../../../modules/es.string.italics'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').italics; apollo-server-demo/node_modules/core-js/es/string/virtual/sub.js 0000644 0001750 0000144 00000000231 03560116604 024520 0 ustar andreh users require('../../../modules/es.string.sub'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').sub; apollo-server-demo/node_modules/core-js/es/string/virtual/fixed.js 0000644 0001750 0000144 00000000235 03560116604 025032 0 ustar andreh users require('../../../modules/es.string.fixed'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').fixed; apollo-server-demo/node_modules/core-js/es/string/virtual/code-point-at.js 0000644 0001750 0000144 00000000253 03560116604 026376 0 ustar andreh users require('../../../modules/es.string.code-point-at'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').codePointAt; apollo-server-demo/node_modules/core-js/es/string/virtual/trim-left.js 0000644 0001750 0000144 00000000245 03560116604 025637 0 ustar andreh users require('../../../modules/es.string.trim-start'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').trimLeft; apollo-server-demo/node_modules/core-js/es/string/virtual/pad-end.js 0000644 0001750 0000144 00000000240 03560116604 025237 0 ustar andreh users require('../../../modules/es.string.pad-end'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').padEnd; apollo-server-demo/node_modules/core-js/es/string/virtual/small.js 0000644 0001750 0000144 00000000235 03560116604 025043 0 ustar andreh users require('../../../modules/es.string.small'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').small; apollo-server-demo/node_modules/core-js/es/string/virtual/strike.js 0000644 0001750 0000144 00000000237 03560116604 025236 0 ustar andreh users require('../../../modules/es.string.strike'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').strike; apollo-server-demo/node_modules/core-js/es/string/virtual/bold.js 0000644 0001750 0000144 00000000233 03560116604 024651 0 ustar andreh users require('../../../modules/es.string.bold'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').bold; apollo-server-demo/node_modules/core-js/es/string/virtual/link.js 0000644 0001750 0000144 00000000233 03560116604 024666 0 ustar andreh users require('../../../modules/es.string.link'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').link; apollo-server-demo/node_modules/core-js/es/string/virtual/big.js 0000644 0001750 0000144 00000000231 03560116604 024470 0 ustar andreh users require('../../../modules/es.string.big'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').big; apollo-server-demo/node_modules/core-js/es/string/virtual/fontcolor.js 0000644 0001750 0000144 00000000245 03560116604 025741 0 ustar andreh users require('../../../modules/es.string.fontcolor'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').fontcolor; apollo-server-demo/node_modules/core-js/es/string/virtual/fontsize.js 0000644 0001750 0000144 00000000243 03560116604 025573 0 ustar andreh users require('../../../modules/es.string.fontsize'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').fontsize; apollo-server-demo/node_modules/core-js/es/string/virtual/starts-with.js 0000644 0001750 0000144 00000000250 03560116604 026221 0 ustar andreh users require('../../../modules/es.string.starts-with'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').startsWith; apollo-server-demo/node_modules/core-js/es/string/virtual/trim-end.js 0000644 0001750 0000144 00000000244 03560116604 025452 0 ustar andreh users require('../../../modules/es.string.trim-end'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').trimRight; apollo-server-demo/node_modules/core-js/es/string/virtual/trim-start.js 0000644 0001750 0000144 00000000245 03560116604 026042 0 ustar andreh users require('../../../modules/es.string.trim-start'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').trimLeft; apollo-server-demo/node_modules/core-js/es/string/virtual/blink.js 0000644 0001750 0000144 00000000235 03560116604 025032 0 ustar andreh users require('../../../modules/es.string.blink'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').blink; apollo-server-demo/node_modules/core-js/es/string/virtual/sup.js 0000644 0001750 0000144 00000000231 03560116604 024536 0 ustar andreh users require('../../../modules/es.string.sup'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').sup; apollo-server-demo/node_modules/core-js/es/string/virtual/trim.js 0000644 0001750 0000144 00000000233 03560116604 024704 0 ustar andreh users require('../../../modules/es.string.trim'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').trim; apollo-server-demo/node_modules/core-js/es/string/virtual/pad-start.js 0000644 0001750 0000144 00000000244 03560116604 025632 0 ustar andreh users require('../../../modules/es.string.pad-start'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').padStart; apollo-server-demo/node_modules/core-js/es/string/virtual/anchor.js 0000644 0001750 0000144 00000000237 03560116604 025207 0 ustar andreh users require('../../../modules/es.string.anchor'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').anchor; apollo-server-demo/node_modules/core-js/es/string/virtual/replace-all.js 0000644 0001750 0000144 00000000250 03560116604 026111 0 ustar andreh users require('../../../modules/es.string.replace-all'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').replaceAll; apollo-server-demo/node_modules/core-js/es/string/virtual/match-all.js 0000644 0001750 0000144 00000000244 03560116604 025575 0 ustar andreh users require('../../../modules/es.string.match-all'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').matchAll; apollo-server-demo/node_modules/core-js/es/string/virtual/trim-right.js 0000644 0001750 0000144 00000000244 03560116604 026021 0 ustar andreh users require('../../../modules/es.string.trim-end'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').trimRight; apollo-server-demo/node_modules/core-js/es/string/virtual/ends-with.js 0000644 0001750 0000144 00000000244 03560116604 025635 0 ustar andreh users require('../../../modules/es.string.ends-with'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('String').endsWith; apollo-server-demo/node_modules/core-js/es/string/small.js 0000644 0001750 0000144 00000000227 03560116604 023356 0 ustar andreh users require('../../modules/es.string.small'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'small'); apollo-server-demo/node_modules/core-js/es/string/strike.js 0000644 0001750 0000144 00000000231 03560116604 023542 0 ustar andreh users require('../../modules/es.string.strike'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'strike'); apollo-server-demo/node_modules/core-js/es/string/bold.js 0000644 0001750 0000144 00000000225 03560116604 023164 0 ustar andreh users require('../../modules/es.string.bold'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'bold'); apollo-server-demo/node_modules/core-js/es/string/link.js 0000644 0001750 0000144 00000000225 03560116604 023201 0 ustar andreh users require('../../modules/es.string.link'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'link'); apollo-server-demo/node_modules/core-js/es/string/big.js 0000644 0001750 0000144 00000000223 03560116604 023003 0 ustar andreh users require('../../modules/es.string.big'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'big'); apollo-server-demo/node_modules/core-js/es/string/fontcolor.js 0000644 0001750 0000144 00000000237 03560116604 024254 0 ustar andreh users require('../../modules/es.string.fontcolor'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'fontcolor'); apollo-server-demo/node_modules/core-js/es/string/split.js 0000644 0001750 0000144 00000000300 03560116604 023371 0 ustar andreh users require('../../modules/es.regexp.exec'); require('../../modules/es.string.split'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'split'); apollo-server-demo/node_modules/core-js/es/string/fontsize.js 0000644 0001750 0000144 00000000235 03560116604 024106 0 ustar andreh users require('../../modules/es.string.fontsize'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'fontsize'); apollo-server-demo/node_modules/core-js/es/string/starts-with.js 0000644 0001750 0000144 00000000242 03560116604 024534 0 ustar andreh users require('../../modules/es.string.starts-with'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'startsWith'); apollo-server-demo/node_modules/core-js/es/string/trim-end.js 0000644 0001750 0000144 00000000236 03560116604 023765 0 ustar andreh users require('../../modules/es.string.trim-end'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'trimRight'); apollo-server-demo/node_modules/core-js/es/string/search.js 0000644 0001750 0000144 00000000302 03560116604 023505 0 ustar andreh users require('../../modules/es.regexp.exec'); require('../../modules/es.string.search'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'search'); apollo-server-demo/node_modules/core-js/es/string/replace.js 0000644 0001750 0000144 00000000304 03560116604 023655 0 ustar andreh users require('../../modules/es.regexp.exec'); require('../../modules/es.string.replace'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'replace'); apollo-server-demo/node_modules/core-js/es/string/trim-start.js 0000644 0001750 0000144 00000000237 03560116604 024355 0 ustar andreh users require('../../modules/es.string.trim-start'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'trimLeft'); apollo-server-demo/node_modules/core-js/es/string/blink.js 0000644 0001750 0000144 00000000227 03560116604 023345 0 ustar andreh users require('../../modules/es.string.blink'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'blink'); apollo-server-demo/node_modules/core-js/es/string/sup.js 0000644 0001750 0000144 00000000223 03560116604 023051 0 ustar andreh users require('../../modules/es.string.sup'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'sup'); apollo-server-demo/node_modules/core-js/es/string/trim.js 0000644 0001750 0000144 00000000225 03560116604 023217 0 ustar andreh users require('../../modules/es.string.trim'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'trim'); apollo-server-demo/node_modules/core-js/es/string/pad-start.js 0000644 0001750 0000144 00000000236 03560116604 024145 0 ustar andreh users require('../../modules/es.string.pad-start'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'padStart'); apollo-server-demo/node_modules/core-js/es/string/anchor.js 0000644 0001750 0000144 00000000231 03560116604 023513 0 ustar andreh users require('../../modules/es.string.anchor'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'anchor'); apollo-server-demo/node_modules/core-js/es/string/replace-all.js 0000644 0001750 0000144 00000000242 03560116604 024424 0 ustar andreh users require('../../modules/es.string.replace-all'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'replaceAll'); apollo-server-demo/node_modules/core-js/es/string/match-all.js 0000644 0001750 0000144 00000000236 03560116604 024110 0 ustar andreh users require('../../modules/es.string.match-all'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'matchAll'); apollo-server-demo/node_modules/core-js/es/string/trim-right.js 0000644 0001750 0000144 00000000236 03560116604 024334 0 ustar andreh users require('../../modules/es.string.trim-end'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'trimRight'); apollo-server-demo/node_modules/core-js/es/string/from-code-point.js 0000644 0001750 0000144 00000000215 03560116604 025245 0 ustar andreh users require('../../modules/es.string.from-code-point'); var path = require('../../internals/path'); module.exports = path.String.fromCodePoint; apollo-server-demo/node_modules/core-js/es/string/ends-with.js 0000644 0001750 0000144 00000000236 03560116604 024150 0 ustar andreh users require('../../modules/es.string.ends-with'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('String', 'endsWith'); apollo-server-demo/node_modules/core-js/es/string/raw.js 0000644 0001750 0000144 00000000167 03560116604 023042 0 ustar andreh users require('../../modules/es.string.raw'); var path = require('../../internals/path'); module.exports = path.String.raw; apollo-server-demo/node_modules/core-js/es/date/ 0000755 0001750 0000144 00000000000 14067647701 021331 5 ustar andreh users apollo-server-demo/node_modules/core-js/es/date/index.js 0000644 0001750 0000144 00000000444 03560116604 022765 0 ustar andreh users require('../../modules/es.date.now'); require('../../modules/es.date.to-json'); require('../../modules/es.date.to-iso-string'); require('../../modules/es.date.to-string'); require('../../modules/es.date.to-primitive'); var path = require('../../internals/path'); module.exports = path.Date; apollo-server-demo/node_modules/core-js/es/date/to-iso-string.js 0000644 0001750 0000144 00000000313 03560116604 024367 0 ustar andreh users require('../../modules/es.date.to-iso-string'); require('../../modules/es.date.to-json'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Date', 'toISOString'); apollo-server-demo/node_modules/core-js/es/date/now.js 0000644 0001750 0000144 00000000163 03560116604 022457 0 ustar andreh users require('../../modules/es.date.now'); var path = require('../../internals/path'); module.exports = path.Date.now; apollo-server-demo/node_modules/core-js/es/date/to-primitive.js 0000644 0001750 0000144 00000000277 03560116604 024312 0 ustar andreh users require('../../modules/es.date.to-primitive'); var toPrimitive = require('../../internals/date-to-primitive'); module.exports = function (it, hint) { return toPrimitive.call(it, hint); }; apollo-server-demo/node_modules/core-js/es/date/to-json.js 0000644 0001750 0000144 00000000226 03560116604 023245 0 ustar andreh users require('../../modules/es.date.to-json'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Date', 'toJSON'); apollo-server-demo/node_modules/core-js/es/date/to-string.js 0000644 0001750 0000144 00000000245 03560116604 023603 0 ustar andreh users require('../../modules/es.date.to-string'); var dateToString = Date.prototype.toString; module.exports = function toString(it) { return dateToString.call(it); }; apollo-server-demo/node_modules/core-js/es/function/ 0000755 0001750 0000144 00000000000 14067647701 022241 5 ustar andreh users apollo-server-demo/node_modules/core-js/es/function/index.js 0000644 0001750 0000144 00000000326 03560116604 023674 0 ustar andreh users require('../../modules/es.function.bind'); require('../../modules/es.function.name'); require('../../modules/es.function.has-instance'); var path = require('../../internals/path'); module.exports = path.Function; apollo-server-demo/node_modules/core-js/es/function/virtual/ 0000755 0001750 0000144 00000000000 14067647701 023727 5 ustar andreh users apollo-server-demo/node_modules/core-js/es/function/virtual/index.js 0000644 0001750 0000144 00000000232 03560116604 025356 0 ustar andreh users require('../../../modules/es.function.bind'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Function'); apollo-server-demo/node_modules/core-js/es/function/virtual/bind.js 0000644 0001750 0000144 00000000237 03560116604 025170 0 ustar andreh users require('../../../modules/es.function.bind'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Function').bind; apollo-server-demo/node_modules/core-js/es/function/bind.js 0000644 0001750 0000144 00000000231 03560116604 023474 0 ustar andreh users require('../../modules/es.function.bind'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Function', 'bind'); apollo-server-demo/node_modules/core-js/es/function/has-instance.js 0000644 0001750 0000144 00000000263 03560116604 025142 0 ustar andreh users require('../../modules/es.function.has-instance'); var wellKnownSymbol = require('../../internals/well-known-symbol'); module.exports = Function[wellKnownSymbol('hasInstance')]; apollo-server-demo/node_modules/core-js/es/function/name.js 0000644 0001750 0000144 00000000053 03560116604 023502 0 ustar andreh users require('../../modules/es.function.name'); apollo-server-demo/node_modules/core-js/es/global-this.js 0000644 0001750 0000144 00000000130 03560116604 023136 0 ustar andreh users require('../modules/es.global-this'); module.exports = require('../internals/global'); apollo-server-demo/node_modules/core-js/es/number/ 0000755 0001750 0000144 00000000000 14067647701 021704 5 ustar andreh users apollo-server-demo/node_modules/core-js/es/number/index.js 0000644 0001750 0000144 00000001211 03560116604 023331 0 ustar andreh users require('../../modules/es.number.constructor'); require('../../modules/es.number.epsilon'); require('../../modules/es.number.is-finite'); require('../../modules/es.number.is-integer'); require('../../modules/es.number.is-nan'); require('../../modules/es.number.is-safe-integer'); require('../../modules/es.number.max-safe-integer'); require('../../modules/es.number.min-safe-integer'); require('../../modules/es.number.parse-float'); require('../../modules/es.number.parse-int'); require('../../modules/es.number.to-fixed'); require('../../modules/es.number.to-precision'); var path = require('../../internals/path'); module.exports = path.Number; apollo-server-demo/node_modules/core-js/es/number/epsilon.js 0000644 0001750 0000144 00000000120 03560116604 023671 0 ustar andreh users require('../../modules/es.number.epsilon'); module.exports = Math.pow(2, -52); apollo-server-demo/node_modules/core-js/es/number/to-fixed.js 0000644 0001750 0000144 00000000234 03560116604 023745 0 ustar andreh users require('../../modules/es.number.to-fixed'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Number', 'toFixed'); apollo-server-demo/node_modules/core-js/es/number/virtual/ 0000755 0001750 0000144 00000000000 14067647701 023372 5 ustar andreh users apollo-server-demo/node_modules/core-js/es/number/virtual/index.js 0000644 0001750 0000144 00000000316 03560116604 025024 0 ustar andreh users require('../../../modules/es.number.to-fixed'); require('../../../modules/es.number.to-precision'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Number'); apollo-server-demo/node_modules/core-js/es/number/virtual/to-fixed.js 0000644 0001750 0000144 00000000242 03560116604 025432 0 ustar andreh users require('../../../modules/es.number.to-fixed'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Number').toFixed; apollo-server-demo/node_modules/core-js/es/number/virtual/to-precision.js 0000644 0001750 0000144 00000000252 03560116604 026327 0 ustar andreh users require('../../../modules/es.number.to-precision'); var entryVirtual = require('../../../internals/entry-virtual'); module.exports = entryVirtual('Number').toPrecision; apollo-server-demo/node_modules/core-js/es/number/constructor.js 0000644 0001750 0000144 00000000112 03560116604 024606 0 ustar andreh users require('../../modules/es.number.constructor'); module.exports = Number; apollo-server-demo/node_modules/core-js/es/number/min-safe-integer.js 0000644 0001750 0000144 00000000132 03560116604 025355 0 ustar andreh users require('../../modules/es.number.min-safe-integer'); module.exports = -0x1FFFFFFFFFFFFF; apollo-server-demo/node_modules/core-js/es/number/max-safe-integer.js 0000644 0001750 0000144 00000000131 03560116604 025356 0 ustar andreh users require('../../modules/es.number.max-safe-integer'); module.exports = 0x1FFFFFFFFFFFFF; apollo-server-demo/node_modules/core-js/es/number/is-integer.js 0000644 0001750 0000144 00000000204 03560116604 024271 0 ustar andreh users require('../../modules/es.number.is-integer'); var path = require('../../internals/path'); module.exports = path.Number.isInteger; apollo-server-demo/node_modules/core-js/es/number/to-precision.js 0000644 0001750 0000144 00000000244 03560116604 024642 0 ustar andreh users require('../../modules/es.number.to-precision'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Number', 'toPrecision'); apollo-server-demo/node_modules/core-js/es/number/parse-float.js 0000644 0001750 0000144 00000000206 03560116604 024442 0 ustar andreh users require('../../modules/es.number.parse-float'); var path = require('../../internals/path'); module.exports = path.Number.parseFloat; apollo-server-demo/node_modules/core-js/es/number/parse-int.js 0000644 0001750 0000144 00000000202 03560116604 024123 0 ustar andreh users require('../../modules/es.number.parse-int'); var path = require('../../internals/path'); module.exports = path.Number.parseInt; apollo-server-demo/node_modules/core-js/es/number/is-nan.js 0000644 0001750 0000144 00000000174 03560116604 023416 0 ustar andreh users require('../../modules/es.number.is-nan'); var path = require('../../internals/path'); module.exports = path.Number.isNaN; apollo-server-demo/node_modules/core-js/es/number/is-finite.js 0000644 0001750 0000144 00000000202 03560116604 024110 0 ustar andreh users require('../../modules/es.number.is-finite'); var path = require('../../internals/path'); module.exports = path.Number.isFinite; apollo-server-demo/node_modules/core-js/es/number/is-safe-integer.js 0000644 0001750 0000144 00000000215 03560116604 025207 0 ustar andreh users require('../../modules/es.number.is-safe-integer'); var path = require('../../internals/path'); module.exports = path.Number.isSafeInteger; apollo-server-demo/node_modules/core-js/es/parse-float.js 0000644 0001750 0000144 00000000162 03560116604 023153 0 ustar andreh users require('../modules/es.parse-float'); var path = require('../internals/path'); module.exports = path.parseFloat; apollo-server-demo/node_modules/core-js/es/promise/ 0000755 0001750 0000144 00000000000 14067647701 022072 5 ustar andreh users apollo-server-demo/node_modules/core-js/es/promise/index.js 0000644 0001750 0000144 00000000667 03560116604 023535 0 ustar andreh users require('../../modules/es.aggregate-error'); require('../../modules/es.object.to-string'); require('../../modules/es.promise'); require('../../modules/es.promise.all-settled'); require('../../modules/es.promise.any'); require('../../modules/es.promise.finally'); require('../../modules/es.string.iterator'); require('../../modules/web.dom-collections.iterator'); var path = require('../../internals/path'); module.exports = path.Promise; apollo-server-demo/node_modules/core-js/es/promise/finally.js 0000644 0001750 0000144 00000000302 03560116604 024046 0 ustar andreh users require('../../modules/es.promise'); require('../../modules/es.promise.finally'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Promise', 'finally'); apollo-server-demo/node_modules/core-js/es/promise/all-settled.js 0000644 0001750 0000144 00000000676 03560116604 024640 0 ustar andreh users 'use strict'; require('../../modules/es.promise'); require('../../modules/es.promise.all-settled'); require('../../modules/es.string.iterator'); require('../../modules/web.dom-collections.iterator'); var path = require('../../internals/path'); var Promise = path.Promise; var $allSettled = Promise.allSettled; module.exports = function allSettled(iterable) { return $allSettled.call(typeof this === 'function' ? this : Promise, iterable); }; apollo-server-demo/node_modules/core-js/es/promise/any.js 0000644 0001750 0000144 00000000707 03560116604 023210 0 ustar andreh users 'use strict'; require('../../modules/es.aggregate-error'); require('../../modules/es.promise'); require('../../modules/es.promise.any'); require('../../modules/es.string.iterator'); require('../../modules/web.dom-collections.iterator'); var path = require('../../internals/path'); var Promise = path.Promise; var $any = Promise.any; module.exports = function any(iterable) { return $any.call(typeof this === 'function' ? this : Promise, iterable); }; apollo-server-demo/node_modules/core-js/es/aggregate-error.js 0000644 0001750 0000144 00000000330 03560116604 024010 0 ustar andreh users require('../modules/es.aggregate-error'); require('../modules/es.string.iterator'); require('../modules/web.dom-collections.iterator'); var path = require('../internals/path'); module.exports = path.AggregateError; apollo-server-demo/node_modules/core-js/es/object/ 0000755 0001750 0000144 00000000000 14067647701 021662 5 ustar andreh users apollo-server-demo/node_modules/core-js/es/object/get-own-property-names.js 0000644 0001750 0000144 00000000343 03560116604 026550 0 ustar andreh users require('../../modules/es.object.get-own-property-names'); var path = require('../../internals/path'); var Object = path.Object; module.exports = function getOwnPropertyNames(it) { return Object.getOwnPropertyNames(it); }; apollo-server-demo/node_modules/core-js/es/object/index.js 0000644 0001750 0000144 00000002717 03560116604 023323 0 ustar andreh users require('../../modules/es.symbol'); require('../../modules/es.object.assign'); require('../../modules/es.object.create'); require('../../modules/es.object.define-property'); require('../../modules/es.object.define-properties'); require('../../modules/es.object.entries'); require('../../modules/es.object.freeze'); require('../../modules/es.object.from-entries'); require('../../modules/es.object.get-own-property-descriptor'); require('../../modules/es.object.get-own-property-descriptors'); require('../../modules/es.object.get-own-property-names'); require('../../modules/es.object.get-prototype-of'); require('../../modules/es.object.is'); require('../../modules/es.object.is-extensible'); require('../../modules/es.object.is-frozen'); require('../../modules/es.object.is-sealed'); require('../../modules/es.object.keys'); require('../../modules/es.object.prevent-extensions'); require('../../modules/es.object.seal'); require('../../modules/es.object.set-prototype-of'); require('../../modules/es.object.values'); require('../../modules/es.object.to-string'); require('../../modules/es.object.define-getter'); require('../../modules/es.object.define-setter'); require('../../modules/es.object.lookup-getter'); require('../../modules/es.object.lookup-setter'); require('../../modules/es.json.to-string-tag'); require('../../modules/es.math.to-string-tag'); require('../../modules/es.reflect.to-string-tag'); var path = require('../../internals/path'); module.exports = path.Object; apollo-server-demo/node_modules/core-js/es/object/lookup-getter.js 0000644 0001750 0000144 00000000252 03560116604 025005 0 ustar andreh users require('../../modules/es.object.lookup-setter'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Object', '__lookupGetter__'); apollo-server-demo/node_modules/core-js/es/object/prevent-extensions.js 0000644 0001750 0000144 00000000224 03560116604 026063 0 ustar andreh users require('../../modules/es.object.prevent-extensions'); var path = require('../../internals/path'); module.exports = path.Object.preventExtensions; apollo-server-demo/node_modules/core-js/es/object/get-own-property-descriptor.js 0000644 0001750 0000144 00000000554 03560116604 027627 0 ustar andreh users require('../../modules/es.object.get-own-property-descriptor'); var path = require('../../internals/path'); var Object = path.Object; var getOwnPropertyDescriptor = module.exports = function getOwnPropertyDescriptor(it, key) { return Object.getOwnPropertyDescriptor(it, key); }; if (Object.getOwnPropertyDescriptor.sham) getOwnPropertyDescriptor.sham = true; apollo-server-demo/node_modules/core-js/es/object/get-prototype-of.js 0000644 0001750 0000144 00000000217 03560116604 025431 0 ustar andreh users require('../../modules/es.object.get-prototype-of'); var path = require('../../internals/path'); module.exports = path.Object.getPrototypeOf; apollo-server-demo/node_modules/core-js/es/object/define-getter.js 0000644 0001750 0000144 00000000252 03560116604 024726 0 ustar andreh users require('../../modules/es.object.define-getter'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Object', '__defineGetter__'); apollo-server-demo/node_modules/core-js/es/object/is-sealed.js 0000644 0001750 0000144 00000000202 03560116604 024045 0 ustar andreh users require('../../modules/es.object.is-sealed'); var path = require('../../internals/path'); module.exports = path.Object.isSealed; apollo-server-demo/node_modules/core-js/es/object/assign.js 0000644 0001750 0000144 00000000175 03560116604 023474 0 ustar andreh users require('../../modules/es.object.assign'); var path = require('../../internals/path'); module.exports = path.Object.assign; apollo-server-demo/node_modules/core-js/es/object/define-property.js 0000644 0001750 0000144 00000000472 03560116604 025324 0 ustar andreh users require('../../modules/es.object.define-property'); var path = require('../../internals/path'); var Object = path.Object; var defineProperty = module.exports = function defineProperty(it, key, desc) { return Object.defineProperty(it, key, desc); }; if (Object.defineProperty.sham) defineProperty.sham = true; apollo-server-demo/node_modules/core-js/es/object/get-own-property-symbols.js 0000644 0001750 0000144 00000000205 03560116604 027132 0 ustar andreh users require('../../modules/es.symbol'); var path = require('../../internals/path'); module.exports = path.Object.getOwnPropertySymbols; apollo-server-demo/node_modules/core-js/es/object/create.js 0000644 0001750 0000144 00000000275 03560116604 023454 0 ustar andreh users require('../../modules/es.object.create'); var path = require('../../internals/path'); var Object = path.Object; module.exports = function create(P, D) { return Object.create(P, D); }; apollo-server-demo/node_modules/core-js/es/object/values.js 0000644 0001750 0000144 00000000175 03560116604 023507 0 ustar andreh users require('../../modules/es.object.values'); var path = require('../../internals/path'); module.exports = path.Object.values; apollo-server-demo/node_modules/core-js/es/object/define-setter.js 0000644 0001750 0000144 00000000252 03560116604 024742 0 ustar andreh users require('../../modules/es.object.define-setter'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Object', '__defineSetter__'); apollo-server-demo/node_modules/core-js/es/object/entries.js 0000644 0001750 0000144 00000000177 03560116604 023663 0 ustar andreh users require('../../modules/es.object.entries'); var path = require('../../internals/path'); module.exports = path.Object.entries; apollo-server-demo/node_modules/core-js/es/object/freeze.js 0000644 0001750 0000144 00000000175 03560116604 023470 0 ustar andreh users require('../../modules/es.object.freeze'); var path = require('../../internals/path'); module.exports = path.Object.freeze; apollo-server-demo/node_modules/core-js/es/object/get-own-property-descriptors.js 0000644 0001750 0000144 00000000246 03560116604 030010 0 ustar andreh users require('../../modules/es.object.get-own-property-descriptors'); var path = require('../../internals/path'); module.exports = path.Object.getOwnPropertyDescriptors; apollo-server-demo/node_modules/core-js/es/object/set-prototype-of.js 0000644 0001750 0000144 00000000217 03560116604 025445 0 ustar andreh users require('../../modules/es.object.set-prototype-of'); var path = require('../../internals/path'); module.exports = path.Object.setPrototypeOf; apollo-server-demo/node_modules/core-js/es/object/is.js 0000644 0001750 0000144 00000000165 03560116604 022622 0 ustar andreh users require('../../modules/es.object.is'); var path = require('../../internals/path'); module.exports = path.Object.is; apollo-server-demo/node_modules/core-js/es/object/seal.js 0000644 0001750 0000144 00000000171 03560116604 023130 0 ustar andreh users require('../../modules/es.object.seal'); var path = require('../../internals/path'); module.exports = path.Object.seal; apollo-server-demo/node_modules/core-js/es/object/keys.js 0000644 0001750 0000144 00000000171 03560116604 023157 0 ustar andreh users require('../../modules/es.object.keys'); var path = require('../../internals/path'); module.exports = path.Object.keys; apollo-server-demo/node_modules/core-js/es/object/from-entries.js 0000644 0001750 0000144 00000000264 03560116604 024621 0 ustar andreh users require('../../modules/es.array.iterator'); require('../../modules/es.object.from-entries'); var path = require('../../internals/path'); module.exports = path.Object.fromEntries; apollo-server-demo/node_modules/core-js/es/object/to-string.js 0000644 0001750 0000144 00000000501 03560116604 024127 0 ustar andreh users require('../../modules/es.json.to-string-tag'); require('../../modules/es.math.to-string-tag'); require('../../modules/es.object.to-string'); require('../../modules/es.reflect.to-string-tag'); var classof = require('../../internals/classof'); module.exports = function (it) { return '[object ' + classof(it) + ']'; }; apollo-server-demo/node_modules/core-js/es/object/is-extensible.js 0000644 0001750 0000144 00000000212 03560116604 024753 0 ustar andreh users require('../../modules/es.object.is-extensible'); var path = require('../../internals/path'); module.exports = path.Object.isExtensible; apollo-server-demo/node_modules/core-js/es/object/lookup-setter.js 0000644 0001750 0000144 00000000252 03560116604 025021 0 ustar andreh users require('../../modules/es.object.lookup-setter'); var entryUnbind = require('../../internals/entry-unbind'); module.exports = entryUnbind('Object', '__lookupSetter__'); apollo-server-demo/node_modules/core-js/es/object/is-frozen.js 0000644 0001750 0000144 00000000202 03560116604 024113 0 ustar andreh users require('../../modules/es.object.is-frozen'); var path = require('../../internals/path'); module.exports = path.Object.isFrozen; apollo-server-demo/node_modules/core-js/es/object/define-properties.js 0000644 0001750 0000144 00000000464 03560116604 025635 0 ustar andreh users require('../../modules/es.object.define-properties'); var path = require('../../internals/path'); var Object = path.Object; var defineProperties = module.exports = function defineProperties(T, D) { return Object.defineProperties(T, D); }; if (Object.defineProperties.sham) defineProperties.sham = true; apollo-server-demo/node_modules/core-js/es/parse-int.js 0000644 0001750 0000144 00000000156 03560116604 022643 0 ustar andreh users require('../modules/es.parse-int'); var path = require('../internals/path'); module.exports = path.parseInt; apollo-server-demo/node_modules/core-js/es/set/ 0000755 0001750 0000144 00000000000 14067647701 021207 5 ustar andreh users apollo-server-demo/node_modules/core-js/es/set/index.js 0000644 0001750 0000144 00000000373 03560116604 022644 0 ustar andreh users require('../../modules/es.set'); require('../../modules/es.object.to-string'); require('../../modules/es.string.iterator'); require('../../modules/web.dom-collections.iterator'); var path = require('../../internals/path'); module.exports = path.Set; apollo-server-demo/node_modules/core-js/es/data-view/ 0000755 0001750 0000144 00000000000 14067647701 022275 5 ustar andreh users apollo-server-demo/node_modules/core-js/es/data-view/index.js 0000644 0001750 0000144 00000000242 03560116604 023725 0 ustar andreh users require('../../modules/es.data-view'); require('../../modules/es.object.to-string'); var path = require('../../internals/path'); module.exports = path.DataView; apollo-server-demo/node_modules/core-js/es/json/ 0000755 0001750 0000144 00000000000 14067647701 021365 5 ustar andreh users apollo-server-demo/node_modules/core-js/es/json/index.js 0000644 0001750 0000144 00000000324 03560116604 023016 0 ustar andreh users require('../../modules/es.json.stringify'); require('../../modules/es.json.to-string-tag'); var path = require('../../internals/path'); module.exports = path.JSON || (path.JSON = { stringify: JSON.stringify }); apollo-server-demo/node_modules/core-js/es/json/to-string-tag.js 0000644 0001750 0000144 00000000112 03560116604 024401 0 ustar andreh users require('../../modules/es.json.to-string-tag'); module.exports = 'JSON'; apollo-server-demo/node_modules/core-js/es/json/stringify.js 0000644 0001750 0000144 00000000463 03560116604 023731 0 ustar andreh users require('../../modules/es.json.stringify'); var core = require('../../internals/path'); if (!core.JSON) core.JSON = { stringify: JSON.stringify }; // eslint-disable-next-line no-unused-vars module.exports = function stringify(it, replacer, space) { return core.JSON.stringify.apply(null, arguments); }; apollo-server-demo/node_modules/core-js/es/math/ 0000755 0001750 0000144 00000000000 14067647701 021345 5 ustar andreh users apollo-server-demo/node_modules/core-js/es/math/index.js 0000644 0001750 0000144 00000001433 03560116604 023000 0 ustar andreh users require('../../modules/es.math.acosh'); require('../../modules/es.math.asinh'); require('../../modules/es.math.atanh'); require('../../modules/es.math.cbrt'); require('../../modules/es.math.clz32'); require('../../modules/es.math.cosh'); require('../../modules/es.math.expm1'); require('../../modules/es.math.fround'); require('../../modules/es.math.hypot'); require('../../modules/es.math.imul'); require('../../modules/es.math.log10'); require('../../modules/es.math.log1p'); require('../../modules/es.math.log2'); require('../../modules/es.math.sign'); require('../../modules/es.math.sinh'); require('../../modules/es.math.tanh'); require('../../modules/es.math.to-string-tag'); require('../../modules/es.math.trunc'); var path = require('../../internals/path'); module.exports = path.Math; apollo-server-demo/node_modules/core-js/es/math/imul.js 0000644 0001750 0000144 00000000165 03560116604 022640 0 ustar andreh users require('../../modules/es.math.imul'); var path = require('../../internals/path'); module.exports = path.Math.imul; apollo-server-demo/node_modules/core-js/es/math/cosh.js 0000644 0001750 0000144 00000000165 03560116604 022626 0 ustar andreh users require('../../modules/es.math.cosh'); var path = require('../../internals/path'); module.exports = path.Math.cosh; apollo-server-demo/node_modules/core-js/es/math/fround.js 0000644 0001750 0000144 00000000171 03560116604 023164 0 ustar andreh users require('../../modules/es.math.fround'); var path = require('../../internals/path'); module.exports = path.Math.fround; apollo-server-demo/node_modules/core-js/es/math/trunc.js 0000644 0001750 0000144 00000000167 03560116604 023027 0 ustar andreh users require('../../modules/es.math.trunc'); var path = require('../../internals/path'); module.exports = path.Math.trunc; apollo-server-demo/node_modules/core-js/es/math/to-string-tag.js 0000644 0001750 0000144 00000000112 03560116604 024361 0 ustar andreh users require('../../modules/es.math.to-string-tag'); module.exports = 'Math'; apollo-server-demo/node_modules/core-js/es/math/acosh.js 0000644 0001750 0000144 00000000167 03560116604 022771 0 ustar andreh users require('../../modules/es.math.acosh'); var path = require('../../internals/path'); module.exports = path.Math.acosh; apollo-server-demo/node_modules/core-js/es/math/clz32.js 0000644 0001750 0000144 00000000167 03560116604 022631 0 ustar andreh users require('../../modules/es.math.clz32'); var path = require('../../internals/path'); module.exports = path.Math.clz32; apollo-server-demo/node_modules/core-js/es/math/sign.js 0000644 0001750 0000144 00000000165 03560116604 022632 0 ustar andreh users require('../../modules/es.math.sign'); var path = require('../../internals/path'); module.exports = path.Math.sign; apollo-server-demo/node_modules/core-js/es/math/expm1.js 0000644 0001750 0000144 00000000167 03560116604 022726 0 ustar andreh users require('../../modules/es.math.expm1'); var path = require('../../internals/path'); module.exports = path.Math.expm1; apollo-server-demo/node_modules/core-js/es/math/atanh.js 0000644 0001750 0000144 00000000167 03560116604 022767 0 ustar andreh users require('../../modules/es.math.atanh'); var path = require('../../internals/path'); module.exports = path.Math.atanh; apollo-server-demo/node_modules/core-js/es/math/sinh.js 0000644 0001750 0000144 00000000165 03560116604 022633 0 ustar andreh users require('../../modules/es.math.sinh'); var path = require('../../internals/path'); module.exports = path.Math.sinh; apollo-server-demo/node_modules/core-js/es/math/log1p.js 0000644 0001750 0000144 00000000167 03560116604 022716 0 ustar andreh users require('../../modules/es.math.log1p'); var path = require('../../internals/path'); module.exports = path.Math.log1p; apollo-server-demo/node_modules/core-js/es/math/log10.js 0000644 0001750 0000144 00000000167 03560116604 022616 0 ustar andreh users require('../../modules/es.math.log10'); var path = require('../../internals/path'); module.exports = path.Math.log10; apollo-server-demo/node_modules/core-js/es/math/asinh.js 0000644 0001750 0000144 00000000167 03560116604 022776 0 ustar andreh users require('../../modules/es.math.asinh'); var path = require('../../internals/path'); module.exports = path.Math.asinh; apollo-server-demo/node_modules/core-js/es/math/log2.js 0000644 0001750 0000144 00000000165 03560116604 022535 0 ustar andreh users require('../../modules/es.math.log2'); var path = require('../../internals/path'); module.exports = path.Math.log2; apollo-server-demo/node_modules/core-js/es/math/hypot.js 0000644 0001750 0000144 00000000167 03560116604 023037 0 ustar andreh users require('../../modules/es.math.hypot'); var path = require('../../internals/path'); module.exports = path.Math.hypot; apollo-server-demo/node_modules/core-js/es/math/tanh.js 0000644 0001750 0000144 00000000165 03560116604 022624 0 ustar andreh users require('../../modules/es.math.tanh'); var path = require('../../internals/path'); module.exports = path.Math.tanh; apollo-server-demo/node_modules/core-js/es/math/cbrt.js 0000644 0001750 0000144 00000000165 03560116604 022624 0 ustar andreh users require('../../modules/es.math.cbrt'); var path = require('../../internals/path'); module.exports = path.Math.cbrt; apollo-server-demo/node_modules/core-js/es/typed-array/ 0000755 0001750 0000144 00000000000 14067647701 022655 5 ustar andreh users apollo-server-demo/node_modules/core-js/es/typed-array/int32-array.js 0000644 0001750 0000144 00000000240 03560116604 025247 0 ustar andreh users require('../../modules/es.typed-array.int32-array'); require('./methods'); var global = require('../../internals/global'); module.exports = global.Int32Array; apollo-server-demo/node_modules/core-js/es/typed-array/uint8-array.js 0000644 0001750 0000144 00000000240 03560116604 025357 0 ustar andreh users require('../../modules/es.typed-array.uint8-array'); require('./methods'); var global = require('../../internals/global'); module.exports = global.Uint8Array; apollo-server-demo/node_modules/core-js/es/typed-array/sort.js 0000644 0001750 0000144 00000000056 03560116604 024170 0 ustar andreh users require('../../modules/es.typed-array.sort'); apollo-server-demo/node_modules/core-js/es/typed-array/to-locale-string.js 0000644 0001750 0000144 00000000072 03560116604 026362 0 ustar andreh users require('../../modules/es.typed-array.to-locale-string'); apollo-server-demo/node_modules/core-js/es/typed-array/reduce.js 0000644 0001750 0000144 00000000060 03560116604 024443 0 ustar andreh users require('../../modules/es.typed-array.reduce'); apollo-server-demo/node_modules/core-js/es/typed-array/set.js 0000644 0001750 0000144 00000000055 03560116604 023773 0 ustar andreh users require('../../modules/es.typed-array.set'); apollo-server-demo/node_modules/core-js/es/typed-array/some.js 0000644 0001750 0000144 00000000056 03560116604 024144 0 ustar andreh users require('../../modules/es.typed-array.some'); apollo-server-demo/node_modules/core-js/es/typed-array/index.js 0000644 0001750 0000144 00000001065 03560116604 024311 0 ustar andreh users require('../../modules/es.typed-array.int8-array'); require('../../modules/es.typed-array.uint8-array'); require('../../modules/es.typed-array.uint8-clamped-array'); require('../../modules/es.typed-array.int16-array'); require('../../modules/es.typed-array.uint16-array'); require('../../modules/es.typed-array.int32-array'); require('../../modules/es.typed-array.uint32-array'); require('../../modules/es.typed-array.float32-array'); require('../../modules/es.typed-array.float64-array'); require('./methods'); module.exports = require('../../internals/global'); apollo-server-demo/node_modules/core-js/es/typed-array/iterator.js 0000644 0001750 0000144 00000000062 03560116604 025027 0 ustar andreh users require('../../modules/es.typed-array.iterator'); apollo-server-demo/node_modules/core-js/es/typed-array/float32-array.js 0000644 0001750 0000144 00000000244 03560116604 025566 0 ustar andreh users require('../../modules/es.typed-array.float32-array'); require('./methods'); var global = require('../../internals/global'); module.exports = global.Float32Array; apollo-server-demo/node_modules/core-js/es/typed-array/copy-within.js 0000644 0001750 0000144 00000000065 03560116604 025453 0 ustar andreh users require('../../modules/es.typed-array.copy-within'); apollo-server-demo/node_modules/core-js/es/typed-array/map.js 0000644 0001750 0000144 00000000055 03560116604 023755 0 ustar andreh users require('../../modules/es.typed-array.map'); apollo-server-demo/node_modules/core-js/es/typed-array/uint16-array.js 0000644 0001750 0000144 00000000242 03560116604 025440 0 ustar andreh users require('../../modules/es.typed-array.uint16-array'); require('./methods'); var global = require('../../internals/global'); module.exports = global.Uint16Array; apollo-server-demo/node_modules/core-js/es/typed-array/includes.js 0000644 0001750 0000144 00000000062 03560116604 025004 0 ustar andreh users require('../../modules/es.typed-array.includes'); apollo-server-demo/node_modules/core-js/es/typed-array/reduce-right.js 0000644 0001750 0000144 00000000066 03560116604 025564 0 ustar andreh users require('../../modules/es.typed-array.reduce-right'); apollo-server-demo/node_modules/core-js/es/typed-array/every.js 0000644 0001750 0000144 00000000057 03560116604 024334 0 ustar andreh users require('../../modules/es.typed-array.every'); apollo-server-demo/node_modules/core-js/es/typed-array/fill.js 0000644 0001750 0000144 00000000056 03560116604 024127 0 ustar andreh users require('../../modules/es.typed-array.fill'); apollo-server-demo/node_modules/core-js/es/typed-array/index-of.js 0000644 0001750 0000144 00000000062 03560116604 024707 0 ustar andreh users require('../../modules/es.typed-array.index-of'); apollo-server-demo/node_modules/core-js/es/typed-array/last-index-of.js 0000644 0001750 0000144 00000000067 03560116604 025655 0 ustar andreh users require('../../modules/es.typed-array.last-index-of'); apollo-server-demo/node_modules/core-js/es/typed-array/uint32-array.js 0000644 0001750 0000144 00000000242 03560116604 025436 0 ustar andreh users require('../../modules/es.typed-array.uint32-array'); require('./methods'); var global = require('../../internals/global'); module.exports = global.Uint32Array; apollo-server-demo/node_modules/core-js/es/typed-array/values.js 0000644 0001750 0000144 00000000062 03560116604 024475 0 ustar andreh users require('../../modules/es.typed-array.iterator'); apollo-server-demo/node_modules/core-js/es/typed-array/int8-array.js 0000644 0001750 0000144 00000000236 03560116604 025177 0 ustar andreh users require('../../modules/es.typed-array.int8-array'); require('./methods'); var global = require('../../internals/global'); module.exports = global.Int8Array; apollo-server-demo/node_modules/core-js/es/typed-array/join.js 0000644 0001750 0000144 00000000056 03560116604 024140 0 ustar andreh users require('../../modules/es.typed-array.join'); apollo-server-demo/node_modules/core-js/es/typed-array/from.js 0000644 0001750 0000144 00000000056 03560116604 024144 0 ustar andreh users require('../../modules/es.typed-array.from'); apollo-server-demo/node_modules/core-js/es/typed-array/entries.js 0000644 0001750 0000144 00000000062 03560116604 024647 0 ustar andreh users require('../../modules/es.typed-array.iterator'); apollo-server-demo/node_modules/core-js/es/typed-array/filter.js 0000644 0001750 0000144 00000000060 03560116604 024461 0 ustar andreh users require('../../modules/es.typed-array.filter'); apollo-server-demo/node_modules/core-js/es/typed-array/for-each.js 0000644 0001750 0000144 00000000062 03560116604 024662 0 ustar andreh users require('../../modules/es.typed-array.for-each'); apollo-server-demo/node_modules/core-js/es/typed-array/methods.js 0000644 0001750 0000144 00000002364 03560116604 024650 0 ustar andreh users require('../../modules/es.typed-array.from'); require('../../modules/es.typed-array.of'); require('../../modules/es.typed-array.copy-within'); require('../../modules/es.typed-array.every'); require('../../modules/es.typed-array.fill'); require('../../modules/es.typed-array.filter'); require('../../modules/es.typed-array.find'); require('../../modules/es.typed-array.find-index'); require('../../modules/es.typed-array.for-each'); require('../../modules/es.typed-array.includes'); require('../../modules/es.typed-array.index-of'); require('../../modules/es.typed-array.join'); require('../../modules/es.typed-array.last-index-of'); require('../../modules/es.typed-array.map'); require('../../modules/es.typed-array.reduce'); require('../../modules/es.typed-array.reduce-right'); require('../../modules/es.typed-array.reverse'); require('../../modules/es.typed-array.set'); require('../../modules/es.typed-array.slice'); require('../../modules/es.typed-array.some'); require('../../modules/es.typed-array.sort'); require('../../modules/es.typed-array.subarray'); require('../../modules/es.typed-array.to-locale-string'); require('../../modules/es.typed-array.to-string'); require('../../modules/es.typed-array.iterator'); require('../../modules/es.object.to-string'); apollo-server-demo/node_modules/core-js/es/typed-array/find-index.js 0000644 0001750 0000144 00000000064 03560116604 025225 0 ustar andreh users require('../../modules/es.typed-array.find-index'); apollo-server-demo/node_modules/core-js/es/typed-array/keys.js 0000644 0001750 0000144 00000000062 03560116604 024151 0 ustar andreh users require('../../modules/es.typed-array.iterator'); apollo-server-demo/node_modules/core-js/es/typed-array/find.js 0000644 0001750 0000144 00000000056 03560116604 024121 0 ustar andreh users require('../../modules/es.typed-array.find'); apollo-server-demo/node_modules/core-js/es/typed-array/to-string.js 0000644 0001750 0000144 00000000063 03560116604 025125 0 ustar andreh users require('../../modules/es.typed-array.to-string'); apollo-server-demo/node_modules/core-js/es/typed-array/int16-array.js 0000644 0001750 0000144 00000000240 03560116604 025251 0 ustar andreh users require('../../modules/es.typed-array.int16-array'); require('./methods'); var global = require('../../internals/global'); module.exports = global.Int16Array; apollo-server-demo/node_modules/core-js/es/typed-array/of.js 0000644 0001750 0000144 00000000054 03560116604 023603 0 ustar andreh users require('../../modules/es.typed-array.of'); apollo-server-demo/node_modules/core-js/es/typed-array/reverse.js 0000644 0001750 0000144 00000000061 03560116604 024650 0 ustar andreh users require('../../modules/es.typed-array.reverse'); apollo-server-demo/node_modules/core-js/es/typed-array/subarray.js 0000644 0001750 0000144 00000000062 03560116604 025026 0 ustar andreh users require('../../modules/es.typed-array.subarray'); apollo-server-demo/node_modules/core-js/es/typed-array/uint8-clamped-array.js 0000644 0001750 0000144 00000000257 03560116604 026772 0 ustar andreh users require('../../modules/es.typed-array.uint8-clamped-array'); require('./methods'); var global = require('../../internals/global'); module.exports = global.Uint8ClampedArray; apollo-server-demo/node_modules/core-js/es/typed-array/float64-array.js 0000644 0001750 0000144 00000000244 03560116604 025573 0 ustar andreh users require('../../modules/es.typed-array.float64-array'); require('./methods'); var global = require('../../internals/global'); module.exports = global.Float64Array; apollo-server-demo/node_modules/core-js/es/typed-array/slice.js 0000644 0001750 0000144 00000000057 03560116604 024301 0 ustar andreh users require('../../modules/es.typed-array.slice'); apollo-server-demo/node_modules/core-js/es/weak-set/ 0000755 0001750 0000144 00000000000 14067647701 022134 5 ustar andreh users apollo-server-demo/node_modules/core-js/es/weak-set/index.js 0000644 0001750 0000144 00000000327 03560116604 023570 0 ustar andreh users require('../../modules/es.object.to-string'); require('../../modules/es.weak-set'); require('../../modules/web.dom-collections.iterator'); var path = require('../../internals/path'); module.exports = path.WeakSet; apollo-server-demo/node_modules/core-js/es/reflect/ 0000755 0001750 0000144 00000000000 14067647701 022040 5 ustar andreh users apollo-server-demo/node_modules/core-js/es/reflect/set.js 0000644 0001750 0000144 00000000171 03560116604 023155 0 ustar andreh users require('../../modules/es.reflect.set'); var path = require('../../internals/path'); module.exports = path.Reflect.set; apollo-server-demo/node_modules/core-js/es/reflect/index.js 0000644 0001750 0000144 00000001404 03560116604 023471 0 ustar andreh users require('../../modules/es.reflect.apply'); require('../../modules/es.reflect.construct'); require('../../modules/es.reflect.define-property'); require('../../modules/es.reflect.delete-property'); require('../../modules/es.reflect.get'); require('../../modules/es.reflect.get-own-property-descriptor'); require('../../modules/es.reflect.get-prototype-of'); require('../../modules/es.reflect.has'); require('../../modules/es.reflect.is-extensible'); require('../../modules/es.reflect.own-keys'); require('../../modules/es.reflect.prevent-extensions'); require('../../modules/es.reflect.set'); require('../../modules/es.reflect.set-prototype-of'); require('../../modules/es.reflect.to-string-tag'); var path = require('../../internals/path'); module.exports = path.Reflect; apollo-server-demo/node_modules/core-js/es/reflect/prevent-extensions.js 0000644 0001750 0000144 00000000226 03560116604 026243 0 ustar andreh users require('../../modules/es.reflect.prevent-extensions'); var path = require('../../internals/path'); module.exports = path.Reflect.preventExtensions; apollo-server-demo/node_modules/core-js/es/reflect/get-own-property-descriptor.js 0000644 0001750 0000144 00000000246 03560116604 030003 0 ustar andreh users require('../../modules/es.reflect.get-own-property-descriptor'); var path = require('../../internals/path'); module.exports = path.Reflect.getOwnPropertyDescriptor; apollo-server-demo/node_modules/core-js/es/reflect/delete-property.js 0000644 0001750 0000144 00000000220 03560116604 025501 0 ustar andreh users require('../../modules/es.reflect.delete-property'); var path = require('../../internals/path'); module.exports = path.Reflect.deleteProperty; apollo-server-demo/node_modules/core-js/es/reflect/get-prototype-of.js 0000644 0001750 0000144 00000000221 03560116604 025602 0 ustar andreh users require('../../modules/es.reflect.get-prototype-of'); var path = require('../../internals/path'); module.exports = path.Reflect.getPrototypeOf; apollo-server-demo/node_modules/core-js/es/reflect/to-string-tag.js 0000644 0001750 0000144 00000000120 03560116604 025053 0 ustar andreh users require('../../modules/es.reflect.to-string-tag'); module.exports = 'Reflect'; apollo-server-demo/node_modules/core-js/es/reflect/define-property.js 0000644 0001750 0000144 00000000220 03560116604 025471 0 ustar andreh users require('../../modules/es.reflect.define-property'); var path = require('../../internals/path'); module.exports = path.Reflect.defineProperty; apollo-server-demo/node_modules/core-js/es/reflect/own-keys.js 0000644 0001750 0000144 00000000202 03560116604 024131 0 ustar andreh users require('../../modules/es.reflect.own-keys'); var path = require('../../internals/path'); module.exports = path.Reflect.ownKeys; apollo-server-demo/node_modules/core-js/es/reflect/apply.js 0000644 0001750 0000144 00000000175 03560116604 023513 0 ustar andreh users require('../../modules/es.reflect.apply'); var path = require('../../internals/path'); module.exports = path.Reflect.apply; apollo-server-demo/node_modules/core-js/es/reflect/get.js 0000644 0001750 0000144 00000000171 03560116604 023141 0 ustar andreh users require('../../modules/es.reflect.get'); var path = require('../../internals/path'); module.exports = path.Reflect.get; apollo-server-demo/node_modules/core-js/es/reflect/set-prototype-of.js 0000644 0001750 0000144 00000000221 03560116604 025616 0 ustar andreh users require('../../modules/es.reflect.set-prototype-of'); var path = require('../../internals/path'); module.exports = path.Reflect.setPrototypeOf; apollo-server-demo/node_modules/core-js/es/reflect/construct.js 0000644 0001750 0000144 00000000205 03560116604 024404 0 ustar andreh users require('../../modules/es.reflect.construct'); var path = require('../../internals/path'); module.exports = path.Reflect.construct; apollo-server-demo/node_modules/core-js/es/reflect/is-extensible.js 0000644 0001750 0000144 00000000214 03560116604 025133 0 ustar andreh users require('../../modules/es.reflect.is-extensible'); var path = require('../../internals/path'); module.exports = path.Reflect.isExtensible; apollo-server-demo/node_modules/core-js/es/reflect/has.js 0000644 0001750 0000144 00000000171 03560116604 023135 0 ustar andreh users require('../../modules/es.reflect.has'); var path = require('../../internals/path'); module.exports = path.Reflect.has; apollo-server-demo/node_modules/core-js/es/regexp/ 0000755 0001750 0000144 00000000000 14067647701 021706 5 ustar andreh users apollo-server-demo/node_modules/core-js/es/regexp/index.js 0000644 0001750 0000144 00000000660 03560116604 023342 0 ustar andreh users require('../../modules/es.regexp.constructor'); require('../../modules/es.regexp.to-string'); require('../../modules/es.regexp.exec'); require('../../modules/es.regexp.flags'); require('../../modules/es.regexp.sticky'); require('../../modules/es.regexp.test'); require('../../modules/es.string.match'); require('../../modules/es.string.replace'); require('../../modules/es.string.search'); require('../../modules/es.string.split'); apollo-server-demo/node_modules/core-js/es/regexp/match.js 0000644 0001750 0000144 00000000357 03560116604 023332 0 ustar andreh users require('../../modules/es.string.match'); var wellKnownSymbol = require('../../internals/well-known-symbol'); var MATCH = wellKnownSymbol('match'); module.exports = function (it, str) { return RegExp.prototype[MATCH].call(it, str); }; apollo-server-demo/node_modules/core-js/es/regexp/sticky.js 0000644 0001750 0000144 00000000144 03560116604 023536 0 ustar andreh users require('../../modules/es.regexp.sticky'); module.exports = function (it) { return it.sticky; }; apollo-server-demo/node_modules/core-js/es/regexp/flags.js 0000644 0001750 0000144 00000000235 03560116604 023325 0 ustar andreh users require('../../modules/es.regexp.flags'); var flags = require('../../internals/regexp-flags'); module.exports = function (it) { return flags.call(it); }; apollo-server-demo/node_modules/core-js/es/regexp/constructor.js 0000644 0001750 0000144 00000000112 03560116604 024610 0 ustar andreh users require('../../modules/es.regexp.constructor'); module.exports = RegExp; apollo-server-demo/node_modules/core-js/es/regexp/test.js 0000644 0001750 0000144 00000000260 03560116604 023206 0 ustar andreh users require('../../modules/es.regexp.exec'); require('../../modules/es.regexp.test'); module.exports = function (re, string) { return RegExp.prototype.test.call(re, string); }; apollo-server-demo/node_modules/core-js/es/regexp/split.js 0000644 0001750 0000144 00000000375 03560116604 023371 0 ustar andreh users require('../../modules/es.string.split'); var wellKnownSymbol = require('../../internals/well-known-symbol'); var SPLIT = wellKnownSymbol('split'); module.exports = function (it, str, limit) { return RegExp.prototype[SPLIT].call(it, str, limit); }; apollo-server-demo/node_modules/core-js/es/regexp/search.js 0000644 0001750 0000144 00000000363 03560116604 023500 0 ustar andreh users require('../../modules/es.string.search'); var wellKnownSymbol = require('../../internals/well-known-symbol'); var SEARCH = wellKnownSymbol('search'); module.exports = function (it, str) { return RegExp.prototype[SEARCH].call(it, str); }; apollo-server-demo/node_modules/core-js/es/regexp/replace.js 0000644 0001750 0000144 00000000413 03560116604 023642 0 ustar andreh users require('../../modules/es.string.replace'); var wellKnownSymbol = require('../../internals/well-known-symbol'); var REPLACE = wellKnownSymbol('replace'); module.exports = function (it, str, replacer) { return RegExp.prototype[REPLACE].call(it, str, replacer); }; apollo-server-demo/node_modules/core-js/es/regexp/to-string.js 0000644 0001750 0000144 00000000210 03560116604 024150 0 ustar andreh users require('../../modules/es.regexp.to-string'); module.exports = function toString(it) { return RegExp.prototype.toString.call(it); }; apollo-server-demo/node_modules/core-js/stage/ 0000755 0001750 0000144 00000000000 14067647701 021110 5 ustar andreh users apollo-server-demo/node_modules/core-js/stage/3.js 0000644 0001750 0000144 00000000151 03560116604 021572 0 ustar andreh users require('../proposals/relative-indexing-method'); var parent = require('./4'); module.exports = parent; apollo-server-demo/node_modules/core-js/stage/index.js 0000644 0001750 0000144 00000000077 03560116604 022546 0 ustar andreh users var proposals = require('./pre'); module.exports = proposals; apollo-server-demo/node_modules/core-js/stage/1.js 0000644 0001750 0000144 00000001275 03560116604 021600 0 ustar andreh users require('../proposals/array-filtering'); require('../proposals/array-last'); require('../proposals/array-unique'); require('../proposals/collection-methods'); require('../proposals/collection-of-from'); require('../proposals/keys-composition'); require('../proposals/math-extensions'); require('../proposals/math-signbit'); require('../proposals/number-from-string'); require('../proposals/number-range'); require('../proposals/object-iteration'); require('../proposals/observable'); require('../proposals/pattern-matching'); require('../proposals/promise-try'); require('../proposals/seeded-random'); require('../proposals/string-code-points'); var parent = require('./2'); module.exports = parent; apollo-server-demo/node_modules/core-js/stage/pre.js 0000644 0001750 0000144 00000000141 03560116604 022215 0 ustar andreh users require('../proposals/reflect-metadata'); var parent = require('./0'); module.exports = parent; apollo-server-demo/node_modules/core-js/stage/4.js 0000644 0001750 0000144 00000000416 03560116604 021577 0 ustar andreh users require('../proposals/global-this'); require('../proposals/promise-all-settled'); require('../proposals/promise-any'); require('../proposals/string-match-all'); require('../proposals/string-replace-all'); var path = require('../internals/path'); module.exports = path; apollo-server-demo/node_modules/core-js/stage/README.md 0000644 0001750 0000144 00000000222 03560116604 022350 0 ustar andreh users This folder contains entry points for [ECMAScript proposals](https://github.com/zloirock/core-js/tree/v3#ecmascript-proposals) with dependencies. apollo-server-demo/node_modules/core-js/stage/0.js 0000644 0001750 0000144 00000000254 03560116604 021573 0 ustar andreh users require('../proposals/efficient-64-bit-arithmetic'); require('../proposals/string-at'); require('../proposals/url'); var parent = require('./1'); module.exports = parent; apollo-server-demo/node_modules/core-js/stage/2.js 0000644 0001750 0000144 00000000405 03560116604 021573 0 ustar andreh users require('../proposals/array-is-template-object'); require('../proposals/iterator-helpers'); require('../proposals/map-upsert'); require('../proposals/set-methods'); require('../proposals/using-statement'); var parent = require('./3'); module.exports = parent; apollo-server-demo/node_modules/core-js/configurator.js 0000644 0001750 0000144 00000002023 03560116604 023027 0 ustar andreh users var has = require('./internals/has'); var isArray = require('./internals/is-array'); var isForced = require('./internals/is-forced'); var shared = require('./internals/shared-store'); var data = isForced.data; var normalize = isForced.normalize; var USE_FUNCTION_CONSTRUCTOR = 'USE_FUNCTION_CONSTRUCTOR'; var ASYNC_ITERATOR_PROTOTYPE = 'AsyncIteratorPrototype'; var setAggressivenessLevel = function (object, constant) { if (isArray(object)) for (var i = 0; i < object.length; i++) data[normalize(object[i])] = constant; }; module.exports = function (options) { if (typeof options == 'object') { setAggressivenessLevel(options.useNative, isForced.NATIVE); setAggressivenessLevel(options.usePolyfill, isForced.POLYFILL); setAggressivenessLevel(options.useFeatureDetection, null); if (has(options, USE_FUNCTION_CONSTRUCTOR)) shared[USE_FUNCTION_CONSTRUCTOR] = !!options[USE_FUNCTION_CONSTRUCTOR]; if (has(options, ASYNC_ITERATOR_PROTOTYPE)) shared[USE_FUNCTION_CONSTRUCTOR] = options[ASYNC_ITERATOR_PROTOTYPE]; } }; apollo-server-demo/node_modules/ee-first/ 0000755 0001750 0000144 00000000000 14067647700 020160 5 ustar andreh users apollo-server-demo/node_modules/ee-first/index.js 0000644 0001750 0000144 00000003224 12530671667 021630 0 ustar andreh users /*! * ee-first * Copyright(c) 2014 Jonathan Ong * MIT Licensed */ 'use strict' /** * Module exports. * @public */ module.exports = first /** * Get the first event in a set of event emitters and event pairs. * * @param {array} stuff * @param {function} done * @public */ function first(stuff, done) { if (!Array.isArray(stuff)) throw new TypeError('arg must be an array of [ee, events...] arrays') var cleanups = [] for (var i = 0; i < stuff.length; i++) { var arr = stuff[i] if (!Array.isArray(arr) || arr.length < 2) throw new TypeError('each array member must be [ee, events...]') var ee = arr[0] for (var j = 1; j < arr.length; j++) { var event = arr[j] var fn = listener(event, callback) // listen to the event ee.on(event, fn) // push this listener to the list of cleanups cleanups.push({ ee: ee, event: event, fn: fn, }) } } function callback() { cleanup() done.apply(null, arguments) } function cleanup() { var x for (var i = 0; i < cleanups.length; i++) { x = cleanups[i] x.ee.removeListener(x.event, x.fn) } } function thunk(fn) { done = fn } thunk.cancel = cleanup return thunk } /** * Create the event listener. * @private */ function listener(event, done) { return function onevent(arg1) { var args = new Array(arguments.length) var ee = this var err = event === 'error' ? arg1 : null // copy args to prevent arguments escaping scope for (var i = 0; i < args.length; i++) { args[i] = arguments[i] } done(err, ee, event, args) } } apollo-server-demo/node_modules/ee-first/LICENSE 0000644 0001750 0000144 00000002113 12373443356 021161 0 ustar andreh users The MIT License (MIT) Copyright (c) 2014 Jonathan Ong me@jongleberry.com Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/ee-first/README.md 0000644 0001750 0000144 00000005071 12530663437 021440 0 ustar andreh users # EE First [![NPM version][npm-image]][npm-url] [![Build status][travis-image]][travis-url] [![Test coverage][coveralls-image]][coveralls-url] [![License][license-image]][license-url] [![Downloads][downloads-image]][downloads-url] [![Gittip][gittip-image]][gittip-url] Get the first event in a set of event emitters and event pairs, then clean up after itself. ## Install ```sh $ npm install ee-first ``` ## API ```js var first = require('ee-first') ``` ### first(arr, listener) Invoke `listener` on the first event from the list specified in `arr`. `arr` is an array of arrays, with each array in the format `[ee, ...event]`. `listener` will be called only once, the first time any of the given events are emitted. If `error` is one of the listened events, then if that fires first, the `listener` will be given the `err` argument. The `listener` is invoked as `listener(err, ee, event, args)`, where `err` is the first argument emitted from an `error` event, if applicable; `ee` is the event emitter that fired; `event` is the string event name that fired; and `args` is an array of the arguments that were emitted on the event. ```js var ee1 = new EventEmitter() var ee2 = new EventEmitter() first([ [ee1, 'close', 'end', 'error'], [ee2, 'error'] ], function (err, ee, event, args) { // listener invoked }) ``` #### .cancel() The group of listeners can be cancelled before being invoked and have all the event listeners removed from the underlying event emitters. ```js var thunk = first([ [ee1, 'close', 'end', 'error'], [ee2, 'error'] ], function (err, ee, event, args) { // listener invoked }) // cancel and clean up thunk.cancel() ``` [npm-image]: https://img.shields.io/npm/v/ee-first.svg?style=flat-square [npm-url]: https://npmjs.org/package/ee-first [github-tag]: http://img.shields.io/github/tag/jonathanong/ee-first.svg?style=flat-square [github-url]: https://github.com/jonathanong/ee-first/tags [travis-image]: https://img.shields.io/travis/jonathanong/ee-first.svg?style=flat-square [travis-url]: https://travis-ci.org/jonathanong/ee-first [coveralls-image]: https://img.shields.io/coveralls/jonathanong/ee-first.svg?style=flat-square [coveralls-url]: https://coveralls.io/r/jonathanong/ee-first?branch=master [license-image]: http://img.shields.io/npm/l/ee-first.svg?style=flat-square [license-url]: LICENSE.md [downloads-image]: http://img.shields.io/npm/dm/ee-first.svg?style=flat-square [downloads-url]: https://npmjs.org/package/ee-first [gittip-image]: https://img.shields.io/gittip/jonathanong.svg?style=flat-square [gittip-url]: https://www.gittip.com/jonathanong/ apollo-server-demo/node_modules/ee-first/package.json 0000644 0001750 0000144 00000001762 12530672075 022450 0 ustar andreh users { "name": "ee-first", "description": "return the first event in a set of ee/event pairs", "version": "1.1.1", "author": { "name": "Jonathan Ong", "email": "me@jongleberry.com", "url": "http://jongleberry.com", "twitter": "https://twitter.com/jongleberry" }, "contributors": [ "Douglas Christopher Wilson <doug@somethingdoug.com>" ], "license": "MIT", "repository": "jonathanong/ee-first", "devDependencies": { "istanbul": "0.3.9", "mocha": "2.2.5" }, "files": [ "index.js", "LICENSE" ], "scripts": { "test": "mocha --reporter spec --bail --check-leaks test/", "test-cov": "istanbul cover node_modules/mocha/bin/_mocha -- --reporter dot --check-leaks test/", "test-travis": "istanbul cover node_modules/mocha/bin/_mocha --report lcovonly -- --reporter spec --check-leaks test/" } ,"_resolved": "https://registry.npmjs.org/ee-first/-/ee-first-1.1.1.tgz" ,"_integrity": "sha1-WQxhFWsK4vTwJVcyoViyZrxWsh0=" ,"_from": "ee-first@1.1.1" } apollo-server-demo/node_modules/symbol-observable/ 0000755 0001750 0000144 00000000000 14067647700 022071 5 ustar andreh users apollo-server-demo/node_modules/symbol-observable/index.js 0000644 0001750 0000144 00000000051 13207326727 023530 0 ustar andreh users module.exports = require('./lib/index'); apollo-server-demo/node_modules/symbol-observable/readme.md 0000644 0001750 0000144 00000001436 13232664014 023642 0 ustar andreh users # symbol-observable [](https://travis-ci.org/benlesh/symbol-observable) > [`Symbol.observable`](https://github.com/zenparsing/es-observable) [ponyfill](https://ponyfill.com) ## Install ``` $ npm install --save symbol-observable ``` ## Usage ```js const symbolObservable = require('symbol-observable').default; console.log(symbolObservable); //=> Symbol(observable) ``` ## Related - [is-observable](https://github.com/sindresorhus/is-observable) - Check if a value is an Observable - [observable-to-promise](https://github.com/sindresorhus/observable-to-promise) - Convert an Observable to a Promise ## License MIT © [Sindre Sorhus](https://sindresorhus.com) and [Ben Lesh](https://github.com/benlesh) apollo-server-demo/node_modules/symbol-observable/index.d.ts 0000644 0001750 0000144 00000000356 13232663752 023774 0 ustar andreh users declare const observableSymbol: symbol; export default observableSymbol; declare global { export interface SymbolConstructor { readonly observable: symbol; } } export interface Symbol { readonly [Symbol.observable]: symbol; } apollo-server-demo/node_modules/symbol-observable/package.json 0000644 0001750 0000144 00000002466 13232664040 024354 0 ustar andreh users { "name": "symbol-observable", "version": "1.2.0", "description": "Symbol.observable ponyfill", "license": "MIT", "repository": "blesh/symbol-observable", "author": { "name": "Ben Lesh", "email": "ben@benlesh.com" }, "engines": { "node": ">=0.10.0" }, "scripts": { "test": "npm run build && mocha && tsc && node ./ts-test/test.js && check-es3-syntax -p lib/ --kill", "build": "babel es --out-dir lib", "prepublish": "npm test" }, "files": [ "index.js", "ponyfill.js", "index.d.ts", "es/index.js", "es/ponyfill/js", "lib/index.js", "lib/ponyfill.js" ], "main": "lib/index.js", "module": "es/index.js", "jsnext:main": "es/index.js", "typings": "index.d.ts", "keywords": [ "symbol", "observable", "observables", "ponyfill", "polyfill", "shim" ], "devDependencies": { "babel-cli": "^6.9.0", "babel-preset-es2015": "^6.9.0", "babel-preset-es3": "^1.0.0", "chai": "^3.5.0", "check-es3-syntax-cli": "^0.1.0", "mocha": "^2.4.5", "typescript": "^2.1.4" } ,"_resolved": "https://registry.npmjs.org/symbol-observable/-/symbol-observable-1.2.0.tgz" ,"_integrity": "sha512-e900nM8RRtGhlV36KGEU9k65K3mPb1WV70OdjfxlG2EAuM1noi/E/BaW/uMhL7bPEssK8QV57vN3esixjUvcXQ==" ,"_from": "symbol-observable@1.2.0" } apollo-server-demo/node_modules/symbol-observable/license 0000644 0001750 0000144 00000002210 13207326727 023427 0 ustar andreh users The MIT License (MIT) Copyright (c) Sindre Sorhus <sindresorhus@gmail.com> (sindresorhus.com) Copyright (c) Ben Lesh <ben@benlesh.com> Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/symbol-observable/es/ 0000755 0001750 0000144 00000000000 14067647700 022500 5 ustar andreh users apollo-server-demo/node_modules/symbol-observable/es/index.js 0000644 0001750 0000144 00000000623 13207326727 024144 0 ustar andreh users /* global window */ import ponyfill from './ponyfill.js'; var root; if (typeof self !== 'undefined') { root = self; } else if (typeof window !== 'undefined') { root = window; } else if (typeof global !== 'undefined') { root = global; } else if (typeof module !== 'undefined') { root = module; } else { root = Function('return this')(); } var result = ponyfill(root); export default result; apollo-server-demo/node_modules/symbol-observable/es/ponyfill.js 0000644 0001750 0000144 00000000515 13207326727 024671 0 ustar andreh users export default function symbolObservablePonyfill(root) { var result; var Symbol = root.Symbol; if (typeof Symbol === 'function') { if (Symbol.observable) { result = Symbol.observable; } else { result = Symbol('observable'); Symbol.observable = result; } } else { result = '@@observable'; } return result; }; apollo-server-demo/node_modules/symbol-observable/CHANGELOG.md 0000644 0001750 0000144 00000011640 13232664063 023676 0 ustar andreh users <a name="1.2.0"></a> # [1.2.0](https://github.com/blesh/symbol-observable/compare/1.1.0...v1.2.0) (2018-01-26) ### Bug Fixes * **TypeScript:** Remove global Symbol declaration ([427c3d7](https://github.com/blesh/symbol-observable/commit/427c3d7)) * common js usage example (#30) ([42c2ffa](https://github.com/blesh/symbol-observable/commit/42c2ffa)) ### Features * **bundlers:** Add module and main entries in package.json (#33) ([97673e1](https://github.com/blesh/symbol-observable/commit/97673e1)) <a name="1.1.0"></a> # [1.1.0](https://github.com/blesh/symbol-observable/compare/1.0.4...v1.1.0) (2017-11-28) ### Bug Fixes * **TypeScript:** update TS to 2.0, fix typings ([e08474e](https://github.com/blesh/symbol-observable/commit/e08474e)), closes [#27](https://github.com/blesh/symbol-observable/issues/27) ### Features * **browser:** Fully qualified import for native esm browser support (#31) ([8ae5f8e](https://github.com/blesh/symbol-observable/commit/8ae5f8e)) * **index.d.ts:** add type info to Symbol.observable ([e4be157](https://github.com/blesh/symbol-observable/commit/e4be157)) <a name="1.0.4"></a> ## [1.0.4](https://github.com/blesh/symbol-observable/compare/1.0.3...v1.0.4) (2016-10-13) ### Bug Fixes * **global:** global variable location no longer assumes `module` exists ([4f85ede](https://github.com/blesh/symbol-observable/commit/4f85ede)), closes [#24](https://github.com/blesh/symbol-observable/issues/24) <a name="1.0.3"></a> ## [1.0.3](https://github.com/blesh/symbol-observable/compare/1.0.2...v1.0.3) (2016-10-11) ### Bug Fixes * **mozilla addons support:** fix obtaining global object (#23) ([38da34d](https://github.com/blesh/symbol-observable/commit/38da34d)), closes [#23](https://github.com/blesh/symbol-observable/issues/23) <a name="1.0.2"></a> ## [1.0.2](https://github.com/blesh/symbol-observable/compare/1.0.1...v1.0.2) (2016-08-09) ### Bug Fixes * **ECMAScript 3**: ensure output is ES3 compatible ([3f37af3](https://github.com/blesh/symbol-observable/commit/3f37af3)) <a name="1.0.1"></a> ## [1.0.1](https://github.com/blesh/symbol-observable/compare/1.0.0...v1.0.1) (2016-06-15) ### Bug Fixes * **bundlers:** fix issue that caused some bundlers not to be able to locate `/lib` (#19) ([dd8fdfe](https://github.com/blesh/symbol-observable/commit/dd8fdfe)), closes [(#19](https://github.com/(/issues/19) [#17](https://github.com/blesh/symbol-observable/issues/17) <a name="1.0.0"></a> # [1.0.0](https://github.com/blesh/symbol-observable/compare/0.2.4...v1.0.0) (2016-06-13) ### Bug Fixes * **index.js:** use typeof to check for global or window definitions (#8) ([5f4c2c6](https://github.com/blesh/symbol-observable/commit/5f4c2c6)) * **types:** use default syntax for typedef ([240e3a6](https://github.com/blesh/symbol-observable/commit/240e3a6)) * **TypeScript:** exported ponyfill now works with TypeScript ([c0b894e](https://github.com/blesh/symbol-observable/commit/c0b894e)) ### Features * **es2015:** add es2015 implementation to support rollup (#10) ([7a41de9](https://github.com/blesh/symbol-observable/commit/7a41de9)) ### BREAKING CHANGES * TypeScript: CJS users will now have to `require('symbol-observable').default` rather than just `require('symbol-observable')` this was done to better support ES6 module bundlers. <a name="0.2.4"></a> ## [0.2.4](https://github.com/blesh/symbol-observable/compare/0.2.2...v0.2.4) (2016-04-25) ### Bug Fixes * **IE8 support:** Ensure ES3 support so IE8 is happy ([9aaa7c3](https://github.com/blesh/symbol-observable/commit/9aaa7c3)) * **Symbol.observable:** should NOT equal `Symbol.for('observable')`. ([3b0fdee](https://github.com/blesh/symbol-observable/commit/3b0fdee)), closes [#7](https://github.com/blesh/symbol-observable/issues/7) <a name="0.2.3"></a> ## [0.2.3](https://github.com/blesh/symbol-observable/compare/0.2.3...v0.2.3) (2016-04-24) ### Bug Fixes - **IE8/ECMAScript 3**: Make sure legacy browsers don't choke on a property named `for`. ([9aaa7c](https://github.com/blesh/symbol-observable/9aaa7c)) <a name="0.2.2"></a> ## [0.2.2](https://github.com/sindresorhus/symbol-observable/compare/0.2.1...v0.2.2) (2016-04-19) ### Features * **TypeScript:** add TypeScript typings file ([befd7a](https://github.com/sindresorhus/symbol-observable/commit/befd7a)) <a name="0.2.1"></a> ## [0.2.1](https://github.com/sindresorhus/symbol-observable/compare/0.2.0...v0.2.1) (2016-04-19) ### Bug Fixes * **publish:** publish all required files ([5f26c3a](https://github.com/sindresorhus/symbol-observable/commit/5f26c3a)) <a name="0.2.0"></a> # [0.2.0](https://github.com/sindresorhus/symbol-observable/compare/v0.1.0...v0.2.0) (2016-04-19) ### Bug Fixes * **Symbol.observable:** ensure Symbol.for(\'observable\') matches Symbol.observable ([ada343f](https://github.com/sindresorhus/symbol-observable/commit/ada343f)), closes [#1](https://github.com/sindresorhus/symbol-observable/issues/1) [#2](https://github.com/sindresorhus/symbol-observable/issues/2) apollo-server-demo/node_modules/symbol-observable/lib/ 0000755 0001750 0000144 00000000000 14067647700 022637 5 ustar andreh users apollo-server-demo/node_modules/symbol-observable/lib/index.js 0000644 0001750 0000144 00000001230 13232664133 024270 0 ustar andreh users 'use strict'; Object.defineProperty(exports, "__esModule", { value: true }); var _ponyfill = require('./ponyfill.js'); var _ponyfill2 = _interopRequireDefault(_ponyfill); function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { 'default': obj }; } var root; /* global window */ if (typeof self !== 'undefined') { root = self; } else if (typeof window !== 'undefined') { root = window; } else if (typeof global !== 'undefined') { root = global; } else if (typeof module !== 'undefined') { root = module; } else { root = Function('return this')(); } var result = (0, _ponyfill2['default'])(root); exports['default'] = result; apollo-server-demo/node_modules/symbol-observable/lib/ponyfill.js 0000644 0001750 0000144 00000000701 13232664133 025017 0 ustar andreh users 'use strict'; Object.defineProperty(exports, "__esModule", { value: true }); exports['default'] = symbolObservablePonyfill; function symbolObservablePonyfill(root) { var result; var _Symbol = root.Symbol; if (typeof _Symbol === 'function') { if (_Symbol.observable) { result = _Symbol.observable; } else { result = _Symbol('observable'); _Symbol.observable = result; } } else { result = '@@observable'; } return result; }; apollo-server-demo/node_modules/commander/ 0000755 0001750 0000144 00000000000 14067647700 020407 5 ustar andreh users apollo-server-demo/node_modules/commander/index.js 0000644 0001750 0000144 00000066311 03560116604 022051 0 ustar andreh users /** * Module dependencies. */ var EventEmitter = require('events').EventEmitter; var spawn = require('child_process').spawn; var path = require('path'); var dirname = path.dirname; var basename = path.basename; var fs = require('fs'); /** * Inherit `Command` from `EventEmitter.prototype`. */ require('util').inherits(Command, EventEmitter); /** * Expose the root command. */ exports = module.exports = new Command(); /** * Expose `Command`. */ exports.Command = Command; /** * Expose `Option`. */ exports.Option = Option; /** * Initialize a new `Option` with the given `flags` and `description`. * * @param {String} flags * @param {String} description * @api public */ function Option(flags, description) { this.flags = flags; this.required = flags.indexOf('<') >= 0; this.optional = flags.indexOf('[') >= 0; this.bool = flags.indexOf('-no-') === -1; flags = flags.split(/[ ,|]+/); if (flags.length > 1 && !/^[[<]/.test(flags[1])) this.short = flags.shift(); this.long = flags.shift(); this.description = description || ''; } /** * Return option name. * * @return {String} * @api private */ Option.prototype.name = function() { return this.long .replace('--', '') .replace('no-', ''); }; /** * Return option name, in a camelcase format that can be used * as a object attribute key. * * @return {String} * @api private */ Option.prototype.attributeName = function() { return camelcase(this.name()); }; /** * Check if `arg` matches the short or long flag. * * @param {String} arg * @return {Boolean} * @api private */ Option.prototype.is = function(arg) { return this.short === arg || this.long === arg; }; /** * Initialize a new `Command`. * * @param {String} name * @api public */ function Command(name) { this.commands = []; this.options = []; this._execs = {}; this._allowUnknownOption = false; this._args = []; this._name = name || ''; } /** * Add command `name`. * * The `.action()` callback is invoked when the * command `name` is specified via __ARGV__, * and the remaining arguments are applied to the * function for access. * * When the `name` is "*" an un-matched command * will be passed as the first arg, followed by * the rest of __ARGV__ remaining. * * Examples: * * program * .version('0.0.1') * .option('-C, --chdir <path>', 'change the working directory') * .option('-c, --config <path>', 'set config path. defaults to ./deploy.conf') * .option('-T, --no-tests', 'ignore test hook') * * program * .command('setup') * .description('run remote setup commands') * .action(function() { * console.log('setup'); * }); * * program * .command('exec <cmd>') * .description('run the given remote command') * .action(function(cmd) { * console.log('exec "%s"', cmd); * }); * * program * .command('teardown <dir> [otherDirs...]') * .description('run teardown commands') * .action(function(dir, otherDirs) { * console.log('dir "%s"', dir); * if (otherDirs) { * otherDirs.forEach(function (oDir) { * console.log('dir "%s"', oDir); * }); * } * }); * * program * .command('*') * .description('deploy the given env') * .action(function(env) { * console.log('deploying "%s"', env); * }); * * program.parse(process.argv); * * @param {String} name * @param {String} [desc] for git-style sub-commands * @return {Command} the new command * @api public */ Command.prototype.command = function(name, desc, opts) { if (typeof desc === 'object' && desc !== null) { opts = desc; desc = null; } opts = opts || {}; var args = name.split(/ +/); var cmd = new Command(args.shift()); if (desc) { cmd.description(desc); this.executables = true; this._execs[cmd._name] = true; if (opts.isDefault) this.defaultExecutable = cmd._name; } cmd._noHelp = !!opts.noHelp; this.commands.push(cmd); cmd.parseExpectedArgs(args); cmd.parent = this; if (desc) return this; return cmd; }; /** * Define argument syntax for the top-level command. * * @api public */ Command.prototype.arguments = function(desc) { return this.parseExpectedArgs(desc.split(/ +/)); }; /** * Add an implicit `help [cmd]` subcommand * which invokes `--help` for the given command. * * @api private */ Command.prototype.addImplicitHelpCommand = function() { this.command('help [cmd]', 'display help for [cmd]'); }; /** * Parse expected `args`. * * For example `["[type]"]` becomes `[{ required: false, name: 'type' }]`. * * @param {Array} args * @return {Command} for chaining * @api public */ Command.prototype.parseExpectedArgs = function(args) { if (!args.length) return; var self = this; args.forEach(function(arg) { var argDetails = { required: false, name: '', variadic: false }; switch (arg[0]) { case '<': argDetails.required = true; argDetails.name = arg.slice(1, -1); break; case '[': argDetails.name = arg.slice(1, -1); break; } if (argDetails.name.length > 3 && argDetails.name.slice(-3) === '...') { argDetails.variadic = true; argDetails.name = argDetails.name.slice(0, -3); } if (argDetails.name) { self._args.push(argDetails); } }); return this; }; /** * Register callback `fn` for the command. * * Examples: * * program * .command('help') * .description('display verbose help') * .action(function() { * // output help here * }); * * @param {Function} fn * @return {Command} for chaining * @api public */ Command.prototype.action = function(fn) { var self = this; var listener = function(args, unknown) { // Parse any so-far unknown options args = args || []; unknown = unknown || []; var parsed = self.parseOptions(unknown); // Output help if necessary outputHelpIfNecessary(self, parsed.unknown); // If there are still any unknown options, then we simply // die, unless someone asked for help, in which case we give it // to them, and then we die. if (parsed.unknown.length > 0) { self.unknownOption(parsed.unknown[0]); } // Leftover arguments need to be pushed back. Fixes issue #56 if (parsed.args.length) args = parsed.args.concat(args); self._args.forEach(function(arg, i) { if (arg.required && args[i] == null) { self.missingArgument(arg.name); } else if (arg.variadic) { if (i !== self._args.length - 1) { self.variadicArgNotLast(arg.name); } args[i] = args.splice(i); } }); // Always append ourselves to the end of the arguments, // to make sure we match the number of arguments the user // expects if (self._args.length) { args[self._args.length] = self; } else { args.push(self); } fn.apply(self, args); }; var parent = this.parent || this; var name = parent === this ? '*' : this._name; parent.on('command:' + name, listener); if (this._alias) parent.on('command:' + this._alias, listener); return this; }; /** * Define option with `flags`, `description` and optional * coercion `fn`. * * The `flags` string should contain both the short and long flags, * separated by comma, a pipe or space. The following are all valid * all will output this way when `--help` is used. * * "-p, --pepper" * "-p|--pepper" * "-p --pepper" * * Examples: * * // simple boolean defaulting to false * program.option('-p, --pepper', 'add pepper'); * * --pepper * program.pepper * // => Boolean * * // simple boolean defaulting to true * program.option('-C, --no-cheese', 'remove cheese'); * * program.cheese * // => true * * --no-cheese * program.cheese * // => false * * // required argument * program.option('-C, --chdir <path>', 'change the working directory'); * * --chdir /tmp * program.chdir * // => "/tmp" * * // optional argument * program.option('-c, --cheese [type]', 'add cheese [marble]'); * * @param {String} flags * @param {String} description * @param {Function|*} [fn] or default * @param {*} [defaultValue] * @return {Command} for chaining * @api public */ Command.prototype.option = function(flags, description, fn, defaultValue) { var self = this, option = new Option(flags, description), oname = option.name(), name = option.attributeName(); // default as 3rd arg if (typeof fn !== 'function') { if (fn instanceof RegExp) { var regex = fn; fn = function(val, def) { var m = regex.exec(val); return m ? m[0] : def; }; } else { defaultValue = fn; fn = null; } } // preassign default value only for --no-*, [optional], or <required> if (!option.bool || option.optional || option.required) { // when --no-* we make sure default is true if (!option.bool) defaultValue = true; // preassign only if we have a default if (defaultValue !== undefined) { self[name] = defaultValue; option.defaultValue = defaultValue; } } // register the option this.options.push(option); // when it's passed assign the value // and conditionally invoke the callback this.on('option:' + oname, function(val) { // coercion if (val !== null && fn) { val = fn(val, self[name] === undefined ? defaultValue : self[name]); } // unassigned or bool if (typeof self[name] === 'boolean' || typeof self[name] === 'undefined') { // if no value, bool true, and we have a default, then use it! if (val == null) { self[name] = option.bool ? defaultValue || true : false; } else { self[name] = val; } } else if (val !== null) { // reassign self[name] = val; } }); return this; }; /** * Allow unknown options on the command line. * * @param {Boolean} arg if `true` or omitted, no error will be thrown * for unknown options. * @api public */ Command.prototype.allowUnknownOption = function(arg) { this._allowUnknownOption = arguments.length === 0 || arg; return this; }; /** * Parse `argv`, settings options and invoking commands when defined. * * @param {Array} argv * @return {Command} for chaining * @api public */ Command.prototype.parse = function(argv) { // implicit help if (this.executables) this.addImplicitHelpCommand(); // store raw args this.rawArgs = argv; // guess name this._name = this._name || basename(argv[1], '.js'); // github-style sub-commands with no sub-command if (this.executables && argv.length < 3 && !this.defaultExecutable) { // this user needs help argv.push('--help'); } // process argv var parsed = this.parseOptions(this.normalize(argv.slice(2))); var args = this.args = parsed.args; var result = this.parseArgs(this.args, parsed.unknown); // executable sub-commands var name = result.args[0]; var aliasCommand = null; // check alias of sub commands if (name) { aliasCommand = this.commands.filter(function(command) { return command.alias() === name; })[0]; } if (this._execs[name] === true) { return this.executeSubCommand(argv, args, parsed.unknown); } else if (aliasCommand) { // is alias of a subCommand args[0] = aliasCommand._name; return this.executeSubCommand(argv, args, parsed.unknown); } else if (this.defaultExecutable) { // use the default subcommand args.unshift(this.defaultExecutable); return this.executeSubCommand(argv, args, parsed.unknown); } return result; }; /** * Execute a sub-command executable. * * @param {Array} argv * @param {Array} args * @param {Array} unknown * @api private */ Command.prototype.executeSubCommand = function(argv, args, unknown) { args = args.concat(unknown); if (!args.length) this.help(); if (args[0] === 'help' && args.length === 1) this.help(); // <cmd> --help if (args[0] === 'help') { args[0] = args[1]; args[1] = '--help'; } // executable var f = argv[1]; // name of the subcommand, link `pm-install` var bin = basename(f, path.extname(f)) + '-' + args[0]; // In case of globally installed, get the base dir where executable // subcommand file should be located at var baseDir; var resolvedLink = fs.realpathSync(f); baseDir = dirname(resolvedLink); // prefer local `./<bin>` to bin in the $PATH var localBin = path.join(baseDir, bin); // whether bin file is a js script with explicit `.js` or `.ts` extension var isExplicitJS = false; if (exists(localBin + '.js')) { bin = localBin + '.js'; isExplicitJS = true; } else if (exists(localBin + '.ts')) { bin = localBin + '.ts'; isExplicitJS = true; } else if (exists(localBin)) { bin = localBin; } args = args.slice(1); var proc; if (process.platform !== 'win32') { if (isExplicitJS) { args.unshift(bin); // add executable arguments to spawn args = (process.execArgv || []).concat(args); proc = spawn(process.argv[0], args, { stdio: 'inherit', customFds: [0, 1, 2] }); } else { proc = spawn(bin, args, { stdio: 'inherit', customFds: [0, 1, 2] }); } } else { args.unshift(bin); proc = spawn(process.execPath, args, { stdio: 'inherit' }); } var signals = ['SIGUSR1', 'SIGUSR2', 'SIGTERM', 'SIGINT', 'SIGHUP']; signals.forEach(function(signal) { process.on(signal, function() { if (proc.killed === false && proc.exitCode === null) { proc.kill(signal); } }); }); proc.on('close', process.exit.bind(process)); proc.on('error', function(err) { if (err.code === 'ENOENT') { console.error('error: %s(1) does not exist, try --help', bin); } else if (err.code === 'EACCES') { console.error('error: %s(1) not executable. try chmod or run with root', bin); } process.exit(1); }); // Store the reference to the child process this.runningCommand = proc; }; /** * Normalize `args`, splitting joined short flags. For example * the arg "-abc" is equivalent to "-a -b -c". * This also normalizes equal sign and splits "--abc=def" into "--abc def". * * @param {Array} args * @return {Array} * @api private */ Command.prototype.normalize = function(args) { var ret = [], arg, lastOpt, index; for (var i = 0, len = args.length; i < len; ++i) { arg = args[i]; if (i > 0) { lastOpt = this.optionFor(args[i - 1]); } if (arg === '--') { // Honor option terminator ret = ret.concat(args.slice(i)); break; } else if (lastOpt && lastOpt.required) { ret.push(arg); } else if (arg.length > 1 && arg[0] === '-' && arg[1] !== '-') { arg.slice(1).split('').forEach(function(c) { ret.push('-' + c); }); } else if (/^--/.test(arg) && ~(index = arg.indexOf('='))) { ret.push(arg.slice(0, index), arg.slice(index + 1)); } else { ret.push(arg); } } return ret; }; /** * Parse command `args`. * * When listener(s) are available those * callbacks are invoked, otherwise the "*" * event is emitted and those actions are invoked. * * @param {Array} args * @return {Command} for chaining * @api private */ Command.prototype.parseArgs = function(args, unknown) { var name; if (args.length) { name = args[0]; if (this.listeners('command:' + name).length) { this.emit('command:' + args.shift(), args, unknown); } else { this.emit('command:*', args); } } else { outputHelpIfNecessary(this, unknown); // If there were no args and we have unknown options, // then they are extraneous and we need to error. if (unknown.length > 0) { this.unknownOption(unknown[0]); } if (this.commands.length === 0 && this._args.filter(function(a) { return a.required; }).length === 0) { this.emit('command:*'); } } return this; }; /** * Return an option matching `arg` if any. * * @param {String} arg * @return {Option} * @api private */ Command.prototype.optionFor = function(arg) { for (var i = 0, len = this.options.length; i < len; ++i) { if (this.options[i].is(arg)) { return this.options[i]; } } }; /** * Parse options from `argv` returning `argv` * void of these options. * * @param {Array} argv * @return {Array} * @api public */ Command.prototype.parseOptions = function(argv) { var args = [], len = argv.length, literal, option, arg; var unknownOptions = []; // parse options for (var i = 0; i < len; ++i) { arg = argv[i]; // literal args after -- if (literal) { args.push(arg); continue; } if (arg === '--') { literal = true; continue; } // find matching Option option = this.optionFor(arg); // option is defined if (option) { // requires arg if (option.required) { arg = argv[++i]; if (arg == null) return this.optionMissingArgument(option); this.emit('option:' + option.name(), arg); // optional arg } else if (option.optional) { arg = argv[i + 1]; if (arg == null || (arg[0] === '-' && arg !== '-')) { arg = null; } else { ++i; } this.emit('option:' + option.name(), arg); // bool } else { this.emit('option:' + option.name()); } continue; } // looks like an option if (arg.length > 1 && arg[0] === '-') { unknownOptions.push(arg); // If the next argument looks like it might be // an argument for this option, we pass it on. // If it isn't, then it'll simply be ignored if ((i + 1) < argv.length && argv[i + 1][0] !== '-') { unknownOptions.push(argv[++i]); } continue; } // arg args.push(arg); } return { args: args, unknown: unknownOptions }; }; /** * Return an object containing options as key-value pairs * * @return {Object} * @api public */ Command.prototype.opts = function() { var result = {}, len = this.options.length; for (var i = 0; i < len; i++) { var key = this.options[i].attributeName(); result[key] = key === this._versionOptionName ? this._version : this[key]; } return result; }; /** * Argument `name` is missing. * * @param {String} name * @api private */ Command.prototype.missingArgument = function(name) { console.error("error: missing required argument `%s'", name); process.exit(1); }; /** * `Option` is missing an argument, but received `flag` or nothing. * * @param {String} option * @param {String} flag * @api private */ Command.prototype.optionMissingArgument = function(option, flag) { if (flag) { console.error("error: option `%s' argument missing, got `%s'", option.flags, flag); } else { console.error("error: option `%s' argument missing", option.flags); } process.exit(1); }; /** * Unknown option `flag`. * * @param {String} flag * @api private */ Command.prototype.unknownOption = function(flag) { if (this._allowUnknownOption) return; console.error("error: unknown option `%s'", flag); process.exit(1); }; /** * Variadic argument with `name` is not the last argument as required. * * @param {String} name * @api private */ Command.prototype.variadicArgNotLast = function(name) { console.error("error: variadic arguments must be last `%s'", name); process.exit(1); }; /** * Set the program version to `str`. * * This method auto-registers the "-V, --version" flag * which will print the version number when passed. * * @param {String} str * @param {String} [flags] * @return {Command} for chaining * @api public */ Command.prototype.version = function(str, flags) { if (arguments.length === 0) return this._version; this._version = str; flags = flags || '-V, --version'; var versionOption = new Option(flags, 'output the version number'); this._versionOptionName = versionOption.long.substr(2) || 'version'; this.options.push(versionOption); this.on('option:' + this._versionOptionName, function() { process.stdout.write(str + '\n'); process.exit(0); }); return this; }; /** * Set the description to `str`. * * @param {String} str * @param {Object} argsDescription * @return {String|Command} * @api public */ Command.prototype.description = function(str, argsDescription) { if (arguments.length === 0) return this._description; this._description = str; this._argsDescription = argsDescription; return this; }; /** * Set an alias for the command * * @param {String} alias * @return {String|Command} * @api public */ Command.prototype.alias = function(alias) { var command = this; if (this.commands.length !== 0) { command = this.commands[this.commands.length - 1]; } if (arguments.length === 0) return command._alias; if (alias === command._name) throw new Error('Command alias can\'t be the same as its name'); command._alias = alias; return this; }; /** * Set / get the command usage `str`. * * @param {String} str * @return {String|Command} * @api public */ Command.prototype.usage = function(str) { var args = this._args.map(function(arg) { return humanReadableArgName(arg); }); var usage = '[options]' + (this.commands.length ? ' [command]' : '') + (this._args.length ? ' ' + args.join(' ') : ''); if (arguments.length === 0) return this._usage || usage; this._usage = str; return this; }; /** * Get or set the name of the command * * @param {String} str * @return {String|Command} * @api public */ Command.prototype.name = function(str) { if (arguments.length === 0) return this._name; this._name = str; return this; }; /** * Return prepared commands. * * @return {Array} * @api private */ Command.prototype.prepareCommands = function() { return this.commands.filter(function(cmd) { return !cmd._noHelp; }).map(function(cmd) { var args = cmd._args.map(function(arg) { return humanReadableArgName(arg); }).join(' '); return [ cmd._name + (cmd._alias ? '|' + cmd._alias : '') + (cmd.options.length ? ' [options]' : '') + (args ? ' ' + args : ''), cmd._description ]; }); }; /** * Return the largest command length. * * @return {Number} * @api private */ Command.prototype.largestCommandLength = function() { var commands = this.prepareCommands(); return commands.reduce(function(max, command) { return Math.max(max, command[0].length); }, 0); }; /** * Return the largest option length. * * @return {Number} * @api private */ Command.prototype.largestOptionLength = function() { var options = [].slice.call(this.options); options.push({ flags: '-h, --help' }); return options.reduce(function(max, option) { return Math.max(max, option.flags.length); }, 0); }; /** * Return the largest arg length. * * @return {Number} * @api private */ Command.prototype.largestArgLength = function() { return this._args.reduce(function(max, arg) { return Math.max(max, arg.name.length); }, 0); }; /** * Return the pad width. * * @return {Number} * @api private */ Command.prototype.padWidth = function() { var width = this.largestOptionLength(); if (this._argsDescription && this._args.length) { if (this.largestArgLength() > width) { width = this.largestArgLength(); } } if (this.commands && this.commands.length) { if (this.largestCommandLength() > width) { width = this.largestCommandLength(); } } return width; }; /** * Return help for options. * * @return {String} * @api private */ Command.prototype.optionHelp = function() { var width = this.padWidth(); // Append the help information return this.options.map(function(option) { return pad(option.flags, width) + ' ' + option.description + ((option.bool && option.defaultValue !== undefined) ? ' (default: ' + JSON.stringify(option.defaultValue) + ')' : ''); }).concat([pad('-h, --help', width) + ' ' + 'output usage information']) .join('\n'); }; /** * Return command help documentation. * * @return {String} * @api private */ Command.prototype.commandHelp = function() { if (!this.commands.length) return ''; var commands = this.prepareCommands(); var width = this.padWidth(); return [ 'Commands:', commands.map(function(cmd) { var desc = cmd[1] ? ' ' + cmd[1] : ''; return (desc ? pad(cmd[0], width) : cmd[0]) + desc; }).join('\n').replace(/^/gm, ' '), '' ].join('\n'); }; /** * Return program help documentation. * * @return {String} * @api private */ Command.prototype.helpInformation = function() { var desc = []; if (this._description) { desc = [ this._description, '' ]; var argsDescription = this._argsDescription; if (argsDescription && this._args.length) { var width = this.padWidth(); desc.push('Arguments:'); desc.push(''); this._args.forEach(function(arg) { desc.push(' ' + pad(arg.name, width) + ' ' + argsDescription[arg.name]); }); desc.push(''); } } var cmdName = this._name; if (this._alias) { cmdName = cmdName + '|' + this._alias; } var usage = [ 'Usage: ' + cmdName + ' ' + this.usage(), '' ]; var cmds = []; var commandHelp = this.commandHelp(); if (commandHelp) cmds = [commandHelp]; var options = [ 'Options:', '' + this.optionHelp().replace(/^/gm, ' '), '' ]; return usage .concat(desc) .concat(options) .concat(cmds) .join('\n'); }; /** * Output help information for this command * * @api public */ Command.prototype.outputHelp = function(cb) { if (!cb) { cb = function(passthru) { return passthru; }; } process.stdout.write(cb(this.helpInformation())); this.emit('--help'); }; /** * Output help information and exit. * * @api public */ Command.prototype.help = function(cb) { this.outputHelp(cb); process.exit(); }; /** * Camel-case the given `flag` * * @param {String} flag * @return {String} * @api private */ function camelcase(flag) { return flag.split('-').reduce(function(str, word) { return str + word[0].toUpperCase() + word.slice(1); }); } /** * Pad `str` to `width`. * * @param {String} str * @param {Number} width * @return {String} * @api private */ function pad(str, width) { var len = Math.max(0, width - str.length); return str + Array(len + 1).join(' '); } /** * Output help information if necessary * * @param {Command} command to output help for * @param {Array} array of options to search for -h or --help * @api private */ function outputHelpIfNecessary(cmd, options) { options = options || []; for (var i = 0; i < options.length; i++) { if (options[i] === '--help' || options[i] === '-h') { cmd.outputHelp(); process.exit(0); } } } /** * Takes an argument an returns its human readable equivalent for help usage. * * @param {Object} arg * @return {String} * @api private */ function humanReadableArgName(arg) { var nameOutput = arg.name + (arg.variadic === true ? '...' : ''); return arg.required ? '<' + nameOutput + '>' : '[' + nameOutput + ']'; } // for versions before node v0.8 when there weren't `fs.existsSync` function exists(file) { try { if (fs.statSync(file).isFile()) { return true; } } catch (e) { return false; } } apollo-server-demo/node_modules/commander/LICENSE 0000644 0001750 0000144 00000002112 03560116604 021376 0 ustar andreh users (The MIT License) Copyright (c) 2011 TJ Holowaychuk <tj@vision-media.ca> Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the 'Software'), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/commander/Readme.md 0000644 0001750 0000144 00000030757 03560116604 022130 0 ustar andreh users # Commander.js [](http://travis-ci.org/tj/commander.js) [](https://www.npmjs.org/package/commander) [](https://npmcharts.com/compare/commander?minimal=true) [](https://packagephobia.now.sh/result?p=commander) [](https://gitter.im/tj/commander.js?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge) The complete solution for [node.js](http://nodejs.org) command-line interfaces, inspired by Ruby's [commander](https://github.com/commander-rb/commander). [API documentation](http://tj.github.com/commander.js/) ## Installation $ npm install commander ## Option parsing Options with commander are defined with the `.option()` method, also serving as documentation for the options. The example below parses args and options from `process.argv`, leaving remaining args as the `program.args` array which were not consumed by options. ```js #!/usr/bin/env node /** * Module dependencies. */ var program = require('commander'); program .version('0.1.0') .option('-p, --peppers', 'Add peppers') .option('-P, --pineapple', 'Add pineapple') .option('-b, --bbq-sauce', 'Add bbq sauce') .option('-c, --cheese [type]', 'Add the specified type of cheese [marble]', 'marble') .parse(process.argv); console.log('you ordered a pizza with:'); if (program.peppers) console.log(' - peppers'); if (program.pineapple) console.log(' - pineapple'); if (program.bbqSauce) console.log(' - bbq'); console.log(' - %s cheese', program.cheese); ``` Short flags may be passed as a single arg, for example `-abc` is equivalent to `-a -b -c`. Multi-word options such as "--template-engine" are camel-cased, becoming `program.templateEngine` etc. Note that multi-word options starting with `--no` prefix negate the boolean value of the following word. For example, `--no-sauce` sets the value of `program.sauce` to false. ```js #!/usr/bin/env node /** * Module dependencies. */ var program = require('commander'); program .option('--no-sauce', 'Remove sauce') .parse(process.argv); console.log('you ordered a pizza'); if (program.sauce) console.log(' with sauce'); else console.log(' without sauce'); ``` To get string arguments from options you will need to use angle brackets <> for required inputs or square brackets [] for optional inputs. e.g. ```.option('-m --myarg [myVar]', 'my super cool description')``` Then to access the input if it was passed in. e.g. ```var myInput = program.myarg``` **NOTE**: If you pass a argument without using brackets the example above will return true and not the value passed in. ## Version option Calling the `version` implicitly adds the `-V` and `--version` options to the command. When either of these options is present, the command prints the version number and exits. $ ./examples/pizza -V 0.0.1 If you want your program to respond to the `-v` option instead of the `-V` option, simply pass custom flags to the `version` method using the same syntax as the `option` method. ```js program .version('0.0.1', '-v, --version') ``` The version flags can be named anything, but the long option is required. ## Command-specific options You can attach options to a command. ```js #!/usr/bin/env node var program = require('commander'); program .command('rm <dir>') .option('-r, --recursive', 'Remove recursively') .action(function (dir, cmd) { console.log('remove ' + dir + (cmd.recursive ? ' recursively' : '')) }) program.parse(process.argv) ``` A command's options are validated when the command is used. Any unknown options will be reported as an error. However, if an action-based command does not define an action, then the options are not validated. ## Coercion ```js function range(val) { return val.split('..').map(Number); } function list(val) { return val.split(','); } function collect(val, memo) { memo.push(val); return memo; } function increaseVerbosity(v, total) { return total + 1; } program .version('0.1.0') .usage('[options] <file ...>') .option('-i, --integer <n>', 'An integer argument', parseInt) .option('-f, --float <n>', 'A float argument', parseFloat) .option('-r, --range <a>..<b>', 'A range', range) .option('-l, --list <items>', 'A list', list) .option('-o, --optional [value]', 'An optional value') .option('-c, --collect [value]', 'A repeatable value', collect, []) .option('-v, --verbose', 'A value that can be increased', increaseVerbosity, 0) .parse(process.argv); console.log(' int: %j', program.integer); console.log(' float: %j', program.float); console.log(' optional: %j', program.optional); program.range = program.range || []; console.log(' range: %j..%j', program.range[0], program.range[1]); console.log(' list: %j', program.list); console.log(' collect: %j', program.collect); console.log(' verbosity: %j', program.verbose); console.log(' args: %j', program.args); ``` ## Regular Expression ```js program .version('0.1.0') .option('-s --size <size>', 'Pizza size', /^(large|medium|small)$/i, 'medium') .option('-d --drink [drink]', 'Drink', /^(coke|pepsi|izze)$/i) .parse(process.argv); console.log(' size: %j', program.size); console.log(' drink: %j', program.drink); ``` ## Variadic arguments The last argument of a command can be variadic, and only the last argument. To make an argument variadic you have to append `...` to the argument name. Here is an example: ```js #!/usr/bin/env node /** * Module dependencies. */ var program = require('commander'); program .version('0.1.0') .command('rmdir <dir> [otherDirs...]') .action(function (dir, otherDirs) { console.log('rmdir %s', dir); if (otherDirs) { otherDirs.forEach(function (oDir) { console.log('rmdir %s', oDir); }); } }); program.parse(process.argv); ``` An `Array` is used for the value of a variadic argument. This applies to `program.args` as well as the argument passed to your action as demonstrated above. ## Specify the argument syntax ```js #!/usr/bin/env node var program = require('commander'); program .version('0.1.0') .arguments('<cmd> [env]') .action(function (cmd, env) { cmdValue = cmd; envValue = env; }); program.parse(process.argv); if (typeof cmdValue === 'undefined') { console.error('no command given!'); process.exit(1); } console.log('command:', cmdValue); console.log('environment:', envValue || "no environment given"); ``` Angled brackets (e.g. `<cmd>`) indicate required input. Square brackets (e.g. `[env]`) indicate optional input. ## Git-style sub-commands ```js // file: ./examples/pm var program = require('commander'); program .version('0.1.0') .command('install [name]', 'install one or more packages') .command('search [query]', 'search with optional query') .command('list', 'list packages installed', {isDefault: true}) .parse(process.argv); ``` When `.command()` is invoked with a description argument, no `.action(callback)` should be called to handle sub-commands, otherwise there will be an error. This tells commander that you're going to use separate executables for sub-commands, much like `git(1)` and other popular tools. The commander will try to search the executables in the directory of the entry script (like `./examples/pm`) with the name `program-command`, like `pm-install`, `pm-search`. Options can be passed with the call to `.command()`. Specifying `true` for `opts.noHelp` will remove the subcommand from the generated help output. Specifying `true` for `opts.isDefault` will run the subcommand if no other subcommand is specified. If the program is designed to be installed globally, make sure the executables have proper modes, like `755`. ### `--harmony` You can enable `--harmony` option in two ways: * Use `#! /usr/bin/env node --harmony` in the sub-commands scripts. Note some os version don’t support this pattern. * Use the `--harmony` option when call the command, like `node --harmony examples/pm publish`. The `--harmony` option will be preserved when spawning sub-command process. ## Automated --help The help information is auto-generated based on the information commander already knows about your program, so the following `--help` info is for free: ``` $ ./examples/pizza --help Usage: pizza [options] An application for pizzas ordering Options: -h, --help output usage information -V, --version output the version number -p, --peppers Add peppers -P, --pineapple Add pineapple -b, --bbq Add bbq sauce -c, --cheese <type> Add the specified type of cheese [marble] -C, --no-cheese You do not want any cheese ``` ## Custom help You can display arbitrary `-h, --help` information by listening for "--help". Commander will automatically exit once you are done so that the remainder of your program does not execute causing undesired behaviors, for example in the following executable "stuff" will not output when `--help` is used. ```js #!/usr/bin/env node /** * Module dependencies. */ var program = require('commander'); program .version('0.1.0') .option('-f, --foo', 'enable some foo') .option('-b, --bar', 'enable some bar') .option('-B, --baz', 'enable some baz'); // must be before .parse() since // node's emit() is immediate program.on('--help', function(){ console.log('') console.log('Examples:'); console.log(' $ custom-help --help'); console.log(' $ custom-help -h'); }); program.parse(process.argv); console.log('stuff'); ``` Yields the following help output when `node script-name.js -h` or `node script-name.js --help` are run: ``` Usage: custom-help [options] Options: -h, --help output usage information -V, --version output the version number -f, --foo enable some foo -b, --bar enable some bar -B, --baz enable some baz Examples: $ custom-help --help $ custom-help -h ``` ## .outputHelp(cb) Output help information without exiting. Optional callback cb allows post-processing of help text before it is displayed. If you want to display help by default (e.g. if no command was provided), you can use something like: ```js var program = require('commander'); var colors = require('colors'); program .version('0.1.0') .command('getstream [url]', 'get stream URL') .parse(process.argv); if (!process.argv.slice(2).length) { program.outputHelp(make_red); } function make_red(txt) { return colors.red(txt); //display the help text in red on the console } ``` ## .help(cb) Output help information and exit immediately. Optional callback cb allows post-processing of help text before it is displayed. ## Custom event listeners You can execute custom actions by listening to command and option events. ```js program.on('option:verbose', function () { process.env.VERBOSE = this.verbose; }); // error on unknown commands program.on('command:*', function () { console.error('Invalid command: %s\nSee --help for a list of available commands.', program.args.join(' ')); process.exit(1); }); ``` ## Examples ```js var program = require('commander'); program .version('0.1.0') .option('-C, --chdir <path>', 'change the working directory') .option('-c, --config <path>', 'set config path. defaults to ./deploy.conf') .option('-T, --no-tests', 'ignore test hook'); program .command('setup [env]') .description('run setup commands for all envs') .option("-s, --setup_mode [mode]", "Which setup mode to use") .action(function(env, options){ var mode = options.setup_mode || "normal"; env = env || 'all'; console.log('setup for %s env(s) with %s mode', env, mode); }); program .command('exec <cmd>') .alias('ex') .description('execute the given remote cmd') .option("-e, --exec_mode <mode>", "Which exec mode to use") .action(function(cmd, options){ console.log('exec "%s" using %s mode', cmd, options.exec_mode); }).on('--help', function() { console.log(''); console.log('Examples:'); console.log(''); console.log(' $ deploy exec sequential'); console.log(' $ deploy exec async'); }); program .command('*') .action(function(env){ console.log('deploying "%s"', env); }); program.parse(process.argv); ``` More Demos can be found in the [examples](https://github.com/tj/commander.js/tree/master/examples) directory. ## License [MIT](https://github.com/tj/commander.js/blob/master/LICENSE) apollo-server-demo/node_modules/commander/package.json 0000644 0001750 0000144 00000002072 03560116604 022664 0 ustar andreh users { "name": "commander", "version": "2.20.3", "description": "the complete solution for node.js command-line programs", "keywords": [ "commander", "command", "option", "parser" ], "author": "TJ Holowaychuk <tj@vision-media.ca>", "license": "MIT", "repository": { "type": "git", "url": "https://github.com/tj/commander.js.git" }, "scripts": { "lint": "eslint index.js", "test": "node test/run.js && npm run test-typings", "test-typings": "tsc -p tsconfig.json" }, "main": "index", "files": [ "index.js", "typings/index.d.ts" ], "dependencies": {}, "devDependencies": { "@types/node": "^12.7.8", "eslint": "^6.4.0", "should": "^13.2.3", "sinon": "^7.5.0", "standard": "^14.3.1", "ts-node": "^8.4.1", "typescript": "^3.6.3" }, "typings": "typings/index.d.ts" ,"_resolved": "https://registry.npmjs.org/commander/-/commander-2.20.3.tgz" ,"_integrity": "sha512-GpVkmM8vF2vQUkj2LvZmD35JxeJOLCwJ9cUkugyk2nuhbv3+mJvpLYYt+0+USMxE+oj+ey/lJEnhZw75x/OMcQ==" ,"_from": "commander@2.20.3" } apollo-server-demo/node_modules/commander/CHANGELOG.md 0000644 0001750 0000144 00000026114 03560116604 022212 0 ustar andreh users 2.20.3 / 2019-10-11 ================== * Support Node.js 0.10 (Revert #1059) * Ran "npm unpublish commander@2.20.2". There is no 2.20.2. 2.20.1 / 2019-09-29 ================== * Improve executable subcommand tracking * Update dev dependencies 2.20.0 / 2019-04-02 ================== * fix: resolve symbolic links completely when hunting for subcommands (#935) * Update index.d.ts (#930) * Update Readme.md (#924) * Remove --save option as it isn't required anymore (#918) * Add link to the license file (#900) * Added example of receiving args from options (#858) * Added missing semicolon (#882) * Add extension to .eslintrc (#876) 2.19.0 / 2018-10-02 ================== * Removed newline after Options and Commands headers (#864) * Bugfix - Error output (#862) * Fix to change default value to string (#856) 2.18.0 / 2018-09-07 ================== * Standardize help output (#853) * chmod 644 travis.yml (#851) * add support for execute typescript subcommand via ts-node (#849) 2.17.1 / 2018-08-07 ================== * Fix bug in command emit (#844) 2.17.0 / 2018-08-03 ================== * fixed newline output after help information (#833) * Fix to emit the action even without command (#778) * npm update (#823) 2.16.0 / 2018-06-29 ================== * Remove Makefile and `test/run` (#821) * Make 'npm test' run on Windows (#820) * Add badge to display install size (#807) * chore: cache node_modules (#814) * chore: remove Node.js 4 (EOL), add Node.js 10 (#813) * fixed typo in readme (#812) * Fix types (#804) * Update eslint to resolve vulnerabilities in lodash (#799) * updated readme with custom event listeners. (#791) * fix tests (#794) 2.15.0 / 2018-03-07 ================== * Update downloads badge to point to graph of downloads over time instead of duplicating link to npm * Arguments description 2.14.1 / 2018-02-07 ================== * Fix typing of help function 2.14.0 / 2018-02-05 ================== * only register the option:version event once * Fixes issue #727: Passing empty string for option on command is set to undefined * enable eqeqeq rule * resolves #754 add linter configuration to project * resolves #560 respect custom name for version option * document how to override the version flag * document using options per command 2.13.0 / 2018-01-09 ================== * Do not print default for --no- * remove trailing spaces in command help * Update CI's Node.js to LTS and latest version * typedefs: Command and Option types added to commander namespace 2.12.2 / 2017-11-28 ================== * fix: typings are not shipped 2.12.1 / 2017-11-23 ================== * Move @types/node to dev dependency 2.12.0 / 2017-11-22 ================== * add attributeName() method to Option objects * Documentation updated for options with --no prefix * typings: `outputHelp` takes a string as the first parameter * typings: use overloads * feat(typings): update to match js api * Print default value in option help * Fix translation error * Fail when using same command and alias (#491) * feat(typings): add help callback * fix bug when description is add after command with options (#662) * Format js code * Rename History.md to CHANGELOG.md (#668) * feat(typings): add typings to support TypeScript (#646) * use current node 2.11.0 / 2017-07-03 ================== * Fix help section order and padding (#652) * feature: support for signals to subcommands (#632) * Fixed #37, --help should not display first (#447) * Fix translation errors. (#570) * Add package-lock.json * Remove engines * Upgrade package version * Prefix events to prevent conflicts between commands and options (#494) * Removing dependency on graceful-readlink * Support setting name in #name function and make it chainable * Add .vscode directory to .gitignore (Visual Studio Code metadata) * Updated link to ruby commander in readme files 2.10.0 / 2017-06-19 ================== * Update .travis.yml. drop support for older node.js versions. * Fix require arguments in README.md * On SemVer you do not start from 0.0.1 * Add missing semi colon in readme * Add save param to npm install * node v6 travis test * Update Readme_zh-CN.md * Allow literal '--' to be passed-through as an argument * Test subcommand alias help * link build badge to master branch * Support the alias of Git style sub-command * added keyword commander for better search result on npm * Fix Sub-Subcommands * test node.js stable * Fixes TypeError when a command has an option called `--description` * Update README.md to make it beginner friendly and elaborate on the difference between angled and square brackets. * Add chinese Readme file 2.9.0 / 2015-10-13 ================== * Add option `isDefault` to set default subcommand #415 @Qix- * Add callback to allow filtering or post-processing of help text #434 @djulien * Fix `undefined` text in help information close #414 #416 @zhiyelee 2.8.1 / 2015-04-22 ================== * Back out `support multiline description` Close #396 #397 2.8.0 / 2015-04-07 ================== * Add `process.execArg` support, execution args like `--harmony` will be passed to sub-commands #387 @DigitalIO @zhiyelee * Fix bug in Git-style sub-commands #372 @zhiyelee * Allow commands to be hidden from help #383 @tonylukasavage * When git-style sub-commands are in use, yet none are called, display help #382 @claylo * Add ability to specify arguments syntax for top-level command #258 @rrthomas * Support multiline descriptions #208 @zxqfox 2.7.1 / 2015-03-11 ================== * Revert #347 (fix collisions when option and first arg have same name) which causes a bug in #367. 2.7.0 / 2015-03-09 ================== * Fix git-style bug when installed globally. Close #335 #349 @zhiyelee * Fix collisions when option and first arg have same name. Close #346 #347 @tonylukasavage * Add support for camelCase on `opts()`. Close #353 @nkzawa * Add node.js 0.12 and io.js to travis.yml * Allow RegEx options. #337 @palanik * Fixes exit code when sub-command failing. Close #260 #332 @pirelenito * git-style `bin` files in $PATH make sense. Close #196 #327 @zhiyelee 2.6.0 / 2014-12-30 ================== * added `Command#allowUnknownOption` method. Close #138 #318 @doozr @zhiyelee * Add application description to the help msg. Close #112 @dalssoft 2.5.1 / 2014-12-15 ================== * fixed two bugs incurred by variadic arguments. Close #291 @Quentin01 #302 @zhiyelee 2.5.0 / 2014-10-24 ================== * add support for variadic arguments. Closes #277 @whitlockjc 2.4.0 / 2014-10-17 ================== * fixed a bug on executing the coercion function of subcommands option. Closes #270 * added `Command.prototype.name` to retrieve command name. Closes #264 #266 @tonylukasavage * added `Command.prototype.opts` to retrieve all the options as a simple object of key-value pairs. Closes #262 @tonylukasavage * fixed a bug on subcommand name. Closes #248 @jonathandelgado * fixed function normalize doesn’t honor option terminator. Closes #216 @abbr 2.3.0 / 2014-07-16 ================== * add command alias'. Closes PR #210 * fix: Typos. Closes #99 * fix: Unused fs module. Closes #217 2.2.0 / 2014-03-29 ================== * add passing of previous option value * fix: support subcommands on windows. Closes #142 * Now the defaultValue passed as the second argument of the coercion function. 2.1.0 / 2013-11-21 ================== * add: allow cflag style option params, unit test, fixes #174 2.0.0 / 2013-07-18 ================== * remove input methods (.prompt, .confirm, etc) 1.3.2 / 2013-07-18 ================== * add support for sub-commands to co-exist with the original command 1.3.1 / 2013-07-18 ================== * add quick .runningCommand hack so you can opt-out of other logic when running a sub command 1.3.0 / 2013-07-09 ================== * add EACCES error handling * fix sub-command --help 1.2.0 / 2013-06-13 ================== * allow "-" hyphen as an option argument * support for RegExp coercion 1.1.1 / 2012-11-20 ================== * add more sub-command padding * fix .usage() when args are present. Closes #106 1.1.0 / 2012-11-16 ================== * add git-style executable subcommand support. Closes #94 1.0.5 / 2012-10-09 ================== * fix `--name` clobbering. Closes #92 * fix examples/help. Closes #89 1.0.4 / 2012-09-03 ================== * add `outputHelp()` method. 1.0.3 / 2012-08-30 ================== * remove invalid .version() defaulting 1.0.2 / 2012-08-24 ================== * add `--foo=bar` support [arv] * fix password on node 0.8.8. Make backward compatible with 0.6 [focusaurus] 1.0.1 / 2012-08-03 ================== * fix issue #56 * fix tty.setRawMode(mode) was moved to tty.ReadStream#setRawMode() (i.e. process.stdin.setRawMode()) 1.0.0 / 2012-07-05 ================== * add support for optional option descriptions * add defaulting of `.version()` to package.json's version 0.6.1 / 2012-06-01 ================== * Added: append (yes or no) on confirmation * Added: allow node.js v0.7.x 0.6.0 / 2012-04-10 ================== * Added `.prompt(obj, callback)` support. Closes #49 * Added default support to .choose(). Closes #41 * Fixed the choice example 0.5.1 / 2011-12-20 ================== * Fixed `password()` for recent nodes. Closes #36 0.5.0 / 2011-12-04 ================== * Added sub-command option support [itay] 0.4.3 / 2011-12-04 ================== * Fixed custom help ordering. Closes #32 0.4.2 / 2011-11-24 ================== * Added travis support * Fixed: line-buffered input automatically trimmed. Closes #31 0.4.1 / 2011-11-18 ================== * Removed listening for "close" on --help 0.4.0 / 2011-11-15 ================== * Added support for `--`. Closes #24 0.3.3 / 2011-11-14 ================== * Fixed: wait for close event when writing help info [Jerry Hamlet] 0.3.2 / 2011-11-01 ================== * Fixed long flag definitions with values [felixge] 0.3.1 / 2011-10-31 ================== * Changed `--version` short flag to `-V` from `-v` * Changed `.version()` so it's configurable [felixge] 0.3.0 / 2011-10-31 ================== * Added support for long flags only. Closes #18 0.2.1 / 2011-10-24 ================== * "node": ">= 0.4.x < 0.7.0". Closes #20 0.2.0 / 2011-09-26 ================== * Allow for defaults that are not just boolean. Default peassignment only occurs for --no-*, optional, and required arguments. [Jim Isaacs] 0.1.0 / 2011-08-24 ================== * Added support for custom `--help` output 0.0.5 / 2011-08-18 ================== * Changed: when the user enters nothing prompt for password again * Fixed issue with passwords beginning with numbers [NuckChorris] 0.0.4 / 2011-08-15 ================== * Fixed `Commander#args` 0.0.3 / 2011-08-15 ================== * Added default option value support 0.0.2 / 2011-08-15 ================== * Added mask support to `Command#password(str[, mask], fn)` * Added `Command#password(str, fn)` 0.0.1 / 2010-01-03 ================== * Initial release apollo-server-demo/node_modules/commander/typings/ 0000755 0001750 0000144 00000000000 14067647700 022104 5 ustar andreh users apollo-server-demo/node_modules/commander/typings/index.d.ts 0000644 0001750 0000144 00000020474 03560116604 024002 0 ustar andreh users // Type definitions for commander 2.11 // Project: https://github.com/visionmedia/commander.js // Definitions by: Alan Agius <https://github.com/alan-agius4>, Marcelo Dezem <https://github.com/mdezem>, vvakame <https://github.com/vvakame>, Jules Randolph <https://github.com/sveinburne> // Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped declare namespace local { class Option { flags: string; required: boolean; optional: boolean; bool: boolean; short?: string; long: string; description: string; /** * Initialize a new `Option` with the given `flags` and `description`. * * @param {string} flags * @param {string} [description] */ constructor(flags: string, description?: string); } class Command extends NodeJS.EventEmitter { [key: string]: any; args: string[]; /** * Initialize a new `Command`. * * @param {string} [name] */ constructor(name?: string); /** * Set the program version to `str`. * * This method auto-registers the "-V, --version" flag * which will print the version number when passed. * * @param {string} str * @param {string} [flags] * @returns {Command} for chaining */ version(str: string, flags?: string): Command; /** * Add command `name`. * * The `.action()` callback is invoked when the * command `name` is specified via __ARGV__, * and the remaining arguments are applied to the * function for access. * * When the `name` is "*" an un-matched command * will be passed as the first arg, followed by * the rest of __ARGV__ remaining. * * @example * program * .version('0.0.1') * .option('-C, --chdir <path>', 'change the working directory') * .option('-c, --config <path>', 'set config path. defaults to ./deploy.conf') * .option('-T, --no-tests', 'ignore test hook') * * program * .command('setup') * .description('run remote setup commands') * .action(function() { * console.log('setup'); * }); * * program * .command('exec <cmd>') * .description('run the given remote command') * .action(function(cmd) { * console.log('exec "%s"', cmd); * }); * * program * .command('teardown <dir> [otherDirs...]') * .description('run teardown commands') * .action(function(dir, otherDirs) { * console.log('dir "%s"', dir); * if (otherDirs) { * otherDirs.forEach(function (oDir) { * console.log('dir "%s"', oDir); * }); * } * }); * * program * .command('*') * .description('deploy the given env') * .action(function(env) { * console.log('deploying "%s"', env); * }); * * program.parse(process.argv); * * @param {string} name * @param {string} [desc] for git-style sub-commands * @param {CommandOptions} [opts] command options * @returns {Command} the new command */ command(name: string, desc?: string, opts?: commander.CommandOptions): Command; /** * Define argument syntax for the top-level command. * * @param {string} desc * @returns {Command} for chaining */ arguments(desc: string): Command; /** * Parse expected `args`. * * For example `["[type]"]` becomes `[{ required: false, name: 'type' }]`. * * @param {string[]} args * @returns {Command} for chaining */ parseExpectedArgs(args: string[]): Command; /** * Register callback `fn` for the command. * * @example * program * .command('help') * .description('display verbose help') * .action(function() { * // output help here * }); * * @param {(...args: any[]) => void} fn * @returns {Command} for chaining */ action(fn: (...args: any[]) => void): Command; /** * Define option with `flags`, `description` and optional * coercion `fn`. * * The `flags` string should contain both the short and long flags, * separated by comma, a pipe or space. The following are all valid * all will output this way when `--help` is used. * * "-p, --pepper" * "-p|--pepper" * "-p --pepper" * * @example * // simple boolean defaulting to false * program.option('-p, --pepper', 'add pepper'); * * --pepper * program.pepper * // => Boolean * * // simple boolean defaulting to true * program.option('-C, --no-cheese', 'remove cheese'); * * program.cheese * // => true * * --no-cheese * program.cheese * // => false * * // required argument * program.option('-C, --chdir <path>', 'change the working directory'); * * --chdir /tmp * program.chdir * // => "/tmp" * * // optional argument * program.option('-c, --cheese [type]', 'add cheese [marble]'); * * @param {string} flags * @param {string} [description] * @param {((arg1: any, arg2: any) => void) | RegExp} [fn] function or default * @param {*} [defaultValue] * @returns {Command} for chaining */ option(flags: string, description?: string, fn?: ((arg1: any, arg2: any) => void) | RegExp, defaultValue?: any): Command; option(flags: string, description?: string, defaultValue?: any): Command; /** * Allow unknown options on the command line. * * @param {boolean} [arg] if `true` or omitted, no error will be thrown for unknown options. * @returns {Command} for chaining */ allowUnknownOption(arg?: boolean): Command; /** * Parse `argv`, settings options and invoking commands when defined. * * @param {string[]} argv * @returns {Command} for chaining */ parse(argv: string[]): Command; /** * Parse options from `argv` returning `argv` void of these options. * * @param {string[]} argv * @returns {ParseOptionsResult} */ parseOptions(argv: string[]): commander.ParseOptionsResult; /** * Return an object containing options as key-value pairs * * @returns {{[key: string]: any}} */ opts(): { [key: string]: any }; /** * Set the description to `str`. * * @param {string} str * @param {{[argName: string]: string}} argsDescription * @return {(Command | string)} */ description(str: string, argsDescription?: {[argName: string]: string}): Command; description(): string; /** * Set an alias for the command. * * @param {string} alias * @return {(Command | string)} */ alias(alias: string): Command; alias(): string; /** * Set or get the command usage. * * @param {string} str * @return {(Command | string)} */ usage(str: string): Command; usage(): string; /** * Set the name of the command. * * @param {string} str * @return {Command} */ name(str: string): Command; /** * Get the name of the command. * * @return {string} */ name(): string; /** * Output help information for this command. * * @param {(str: string) => string} [cb] */ outputHelp(cb?: (str: string) => string): void; /** Output help information and exit. * * @param {(str: string) => string} [cb] */ help(cb?: (str: string) => string): never; } } declare namespace commander { type Command = local.Command type Option = local.Option interface CommandOptions { noHelp?: boolean; isDefault?: boolean; } interface ParseOptionsResult { args: string[]; unknown: string[]; } interface CommanderStatic extends Command { Command: typeof local.Command; Option: typeof local.Option; CommandOptions: CommandOptions; ParseOptionsResult: ParseOptionsResult; } } declare const commander: commander.CommanderStatic; export = commander; apollo-server-demo/node_modules/apollo-server-caching/ 0000755 0001750 0000144 00000000000 14067647700 022626 5 ustar andreh users apollo-server-demo/node_modules/apollo-server-caching/dist/ 0000755 0001750 0000144 00000000000 14067647700 023571 5 ustar andreh users apollo-server-demo/node_modules/apollo-server-caching/dist/index.js 0000644 0001750 0000144 00000001024 03560116604 025221 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); var InMemoryLRUCache_1 = require("./InMemoryLRUCache"); Object.defineProperty(exports, "InMemoryLRUCache", { enumerable: true, get: function () { return InMemoryLRUCache_1.InMemoryLRUCache; } }); var PrefixingKeyValueCache_1 = require("./PrefixingKeyValueCache"); Object.defineProperty(exports, "PrefixingKeyValueCache", { enumerable: true, get: function () { return PrefixingKeyValueCache_1.PrefixingKeyValueCache; } }); //# sourceMappingURL=index.js.map apollo-server-demo/node_modules/apollo-server-caching/dist/PrefixingKeyValueCache.js 0000644 0001750 0000144 00000001152 03560116604 030441 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); exports.PrefixingKeyValueCache = void 0; class PrefixingKeyValueCache { constructor(wrapped, prefix) { this.wrapped = wrapped; this.prefix = prefix; } get(key) { return this.wrapped.get(this.prefix + key); } set(key, value, options) { return this.wrapped.set(this.prefix + key, value, options); } delete(key) { return this.wrapped.delete(this.prefix + key); } } exports.PrefixingKeyValueCache = PrefixingKeyValueCache; //# sourceMappingURL=PrefixingKeyValueCache.js.map apollo-server-demo/node_modules/apollo-server-caching/dist/KeyValueCache.js 0000644 0001750 0000144 00000000170 03560116604 026564 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); ; //# sourceMappingURL=KeyValueCache.js.map apollo-server-demo/node_modules/apollo-server-caching/dist/InMemoryLRUCache.d.ts.map 0000644 0001750 0000144 00000001325 03560116604 030174 0 ustar andreh users {"version":3,"file":"InMemoryLRUCache.d.ts","sourceRoot":"","sources":["../src/InMemoryLRUCache.ts"],"names":[],"mappings":"AACA,OAAO,EAAE,qBAAqB,EAAE,MAAM,iBAAiB,CAAC;AAYxD,qBAAa,gBAAgB,CAAC,CAAC,GAAG,MAAM,CAAE,YAAW,qBAAqB,CAAC,CAAC,CAAC;IAC3E,OAAO,CAAC,KAAK,CAAsB;gBAGvB,EACV,OAAkB,EAClB,cAAyC,EACzC,SAAS,GACV,GAAE;QACD,OAAO,CAAC,EAAE,MAAM,CAAC;QACjB,cAAc,CAAC,EAAE,CAAC,KAAK,EAAE,CAAC,EAAE,GAAG,EAAE,MAAM,KAAK,MAAM,CAAC;QACnD,SAAS,CAAC,EAAE,CAAC,GAAG,EAAE,MAAM,EAAE,KAAK,EAAE,CAAC,KAAK,IAAI,CAAC;KACxC;IAQA,GAAG,CAAC,GAAG,EAAE,MAAM;IAGf,GAAG,CAAC,GAAG,EAAE,MAAM,EAAE,KAAK,EAAE,CAAC,EAAE,OAAO,CAAC,EAAE;QAAE,GAAG,CAAC,EAAE,MAAM,CAAA;KAAE;IAIrD,MAAM,CAAC,GAAG,EAAE,MAAM;IAMlB,KAAK,IAAI,OAAO,CAAC,IAAI,CAAC;IAGtB,YAAY;CAGnB"} apollo-server-demo/node_modules/apollo-server-caching/dist/InMemoryLRUCache.d.ts 0000644 0001750 0000144 00000001222 03560116604 027414 0 ustar andreh users import { TestableKeyValueCache } from './KeyValueCache'; export declare class InMemoryLRUCache<V = string> implements TestableKeyValueCache<V> { private store; constructor({ maxSize, sizeCalculator, onDispose, }?: { maxSize?: number; sizeCalculator?: (value: V, key: string) => number; onDispose?: (key: string, value: V) => void; }); get(key: string): Promise<V | undefined>; set(key: string, value: V, options?: { ttl?: number; }): Promise<void>; delete(key: string): Promise<void>; flush(): Promise<void>; getTotalSize(): Promise<number>; } //# sourceMappingURL=InMemoryLRUCache.d.ts.map apollo-server-demo/node_modules/apollo-server-caching/dist/index.d.ts 0000644 0001750 0000144 00000000377 03560116604 025467 0 ustar andreh users export { KeyValueCache, TestableKeyValueCache, KeyValueCacheSetOptions, } from './KeyValueCache'; export { InMemoryLRUCache } from './InMemoryLRUCache'; export { PrefixingKeyValueCache } from './PrefixingKeyValueCache'; //# sourceMappingURL=index.d.ts.map apollo-server-demo/node_modules/apollo-server-caching/dist/PrefixingKeyValueCache.d.ts.map 0000644 0001750 0000144 00000000770 03560116604 031456 0 ustar andreh users {"version":3,"file":"PrefixingKeyValueCache.d.ts","sourceRoot":"","sources":["../src/PrefixingKeyValueCache.ts"],"names":[],"mappings":"AAAA,OAAO,EAAE,aAAa,EAAE,uBAAuB,EAAE,MAAM,iBAAiB,CAAC;AAYzE,qBAAa,sBAAsB,CAAC,CAAC,GAAG,MAAM,CAAE,YAAW,aAAa,CAAC,CAAC,CAAC;IAC7D,OAAO,CAAC,OAAO;IAAoB,OAAO,CAAC,MAAM;gBAAzC,OAAO,EAAE,aAAa,CAAC,CAAC,CAAC,EAAU,MAAM,EAAE,MAAM;IAErE,GAAG,CAAC,GAAG,EAAE,MAAM;IAGf,GAAG,CAAC,GAAG,EAAE,MAAM,EAAE,KAAK,EAAE,CAAC,EAAE,OAAO,CAAC,EAAE,uBAAuB;IAG5D,MAAM,CAAC,GAAG,EAAE,MAAM;CAGnB"} apollo-server-demo/node_modules/apollo-server-caching/dist/index.d.ts.map 0000644 0001750 0000144 00000000405 03560116604 026233 0 ustar andreh users {"version":3,"file":"index.d.ts","sourceRoot":"","sources":["../src/index.ts"],"names":[],"mappings":"AAAA,OAAO,EACL,aAAa,EACb,qBAAqB,EACrB,uBAAuB,GACxB,MAAM,iBAAiB,CAAC;AACzB,OAAO,EAAE,gBAAgB,EAAE,MAAM,oBAAoB,CAAC;AACtD,OAAO,EAAE,sBAAsB,EAAE,MAAM,0BAA0B,CAAC"} apollo-server-demo/node_modules/apollo-server-caching/dist/InMemoryLRUCache.js.map 0000644 0001750 0000144 00000002071 03560116604 027737 0 ustar andreh users {"version":3,"file":"InMemoryLRUCache.js","sourceRoot":"","sources":["../src/InMemoryLRUCache.ts"],"names":[],"mappings":";;;;;;;;;;;;;;;AAAA,0DAAiC;AAGjC,SAAS,wBAAwB,CAAC,IAAS;IACzC,IAAI,KAAK,CAAC,OAAO,CAAC,IAAI,CAAC,IAAI,OAAO,IAAI,KAAK,QAAQ,EAAE;QACnD,OAAO,IAAI,CAAC,MAAM,CAAC;KACpB;IAID,OAAO,CAAC,CAAC;AACX,CAAC;AAED,MAAa,gBAAgB;IAI3B,YAAY,EACV,OAAO,GAAG,QAAQ,EAClB,cAAc,GAAG,wBAAwB,EACzC,SAAS,MAKP,EAAE;QACJ,IAAI,CAAC,KAAK,GAAG,IAAI,mBAAQ,CAAC;YACxB,GAAG,EAAE,OAAO;YACZ,MAAM,EAAE,cAAc;YACtB,OAAO,EAAE,SAAS;SACnB,CAAC,CAAC;IACL,CAAC;IAEK,GAAG,CAAC,GAAW;;YACnB,OAAO,IAAI,CAAC,KAAK,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC;QAC7B,CAAC;KAAA;IACK,GAAG,CAAC,GAAW,EAAE,KAAQ,EAAE,OAA0B;;YACzD,MAAM,MAAM,GAAG,OAAO,IAAI,OAAO,CAAC,GAAG,IAAI,OAAO,CAAC,GAAG,GAAG,IAAI,CAAC;YAC5D,IAAI,CAAC,KAAK,CAAC,GAAG,CAAC,GAAG,EAAE,KAAK,EAAE,MAAM,CAAC,CAAC;QACrC,CAAC;KAAA;IACK,MAAM,CAAC,GAAW;;YACtB,IAAI,CAAC,KAAK,CAAC,GAAG,CAAC,GAAG,CAAC,CAAC;QACtB,CAAC;KAAA;IAIK,KAAK;;YACT,IAAI,CAAC,KAAK,CAAC,KAAK,EAAE,CAAC;QACrB,CAAC;KAAA;IACK,YAAY;;YAChB,OAAO,IAAI,CAAC,KAAK,CAAC,MAAM,CAAC;QAC3B,CAAC;KAAA;CACF;AAvCD,4CAuCC"} apollo-server-demo/node_modules/apollo-server-caching/dist/KeyValueCache.d.ts 0000644 0001750 0000144 00000000734 03560116604 027026 0 ustar andreh users export interface KeyValueCacheSetOptions { ttl?: number | null; } export interface KeyValueCache<V = string> { get(key: string): Promise<V | undefined>; set(key: string, value: V, options?: KeyValueCacheSetOptions): Promise<void>; delete(key: string): Promise<boolean | void>; } export interface TestableKeyValueCache<V = string> extends KeyValueCache<V> { flush?(): Promise<void>; close?(): Promise<void>; } //# sourceMappingURL=KeyValueCache.d.ts.map apollo-server-demo/node_modules/apollo-server-caching/dist/InMemoryLRUCache.js 0000644 0001750 0000144 00000004352 03560116604 027167 0 ustar andreh users "use strict"; var __awaiter = (this && this.__awaiter) || function (thisArg, _arguments, P, generator) { function adopt(value) { return value instanceof P ? value : new P(function (resolve) { resolve(value); }); } return new (P || (P = Promise))(function (resolve, reject) { function fulfilled(value) { try { step(generator.next(value)); } catch (e) { reject(e); } } function rejected(value) { try { step(generator["throw"](value)); } catch (e) { reject(e); } } function step(result) { result.done ? resolve(result.value) : adopt(result.value).then(fulfilled, rejected); } step((generator = generator.apply(thisArg, _arguments || [])).next()); }); }; var __importDefault = (this && this.__importDefault) || function (mod) { return (mod && mod.__esModule) ? mod : { "default": mod }; }; Object.defineProperty(exports, "__esModule", { value: true }); exports.InMemoryLRUCache = void 0; const lru_cache_1 = __importDefault(require("lru-cache")); function defaultLengthCalculation(item) { if (Array.isArray(item) || typeof item === 'string') { return item.length; } return 1; } class InMemoryLRUCache { constructor({ maxSize = Infinity, sizeCalculator = defaultLengthCalculation, onDispose, } = {}) { this.store = new lru_cache_1.default({ max: maxSize, length: sizeCalculator, dispose: onDispose, }); } get(key) { return __awaiter(this, void 0, void 0, function* () { return this.store.get(key); }); } set(key, value, options) { return __awaiter(this, void 0, void 0, function* () { const maxAge = options && options.ttl && options.ttl * 1000; this.store.set(key, value, maxAge); }); } delete(key) { return __awaiter(this, void 0, void 0, function* () { this.store.del(key); }); } flush() { return __awaiter(this, void 0, void 0, function* () { this.store.reset(); }); } getTotalSize() { return __awaiter(this, void 0, void 0, function* () { return this.store.length; }); } } exports.InMemoryLRUCache = InMemoryLRUCache; //# sourceMappingURL=InMemoryLRUCache.js.map apollo-server-demo/node_modules/apollo-server-caching/dist/KeyValueCache.d.ts.map 0000644 0001750 0000144 00000001216 03560116604 027576 0 ustar andreh users {"version":3,"file":"KeyValueCache.d.ts","sourceRoot":"","sources":["../src/KeyValueCache.ts"],"names":[],"mappings":"AACA,MAAM,WAAW,uBAAuB;IAKtC,GAAG,CAAC,EAAE,MAAM,GAAG,IAAI,CAAA;CACpB;AAED,MAAM,WAAW,aAAa,CAAC,CAAC,GAAG,MAAM;IACvC,GAAG,CAAC,GAAG,EAAE,MAAM,GAAG,OAAO,CAAC,CAAC,GAAG,SAAS,CAAC,CAAC;IACzC,GAAG,CAAC,GAAG,EAAE,MAAM,EAAE,KAAK,EAAE,CAAC,EAAE,OAAO,CAAC,EAAE,uBAAuB,GAAG,OAAO,CAAC,IAAI,CAAC,CAAC;IAC7E,MAAM,CAAC,GAAG,EAAE,MAAM,GAAG,OAAO,CAAC,OAAO,GAAG,IAAI,CAAC,CAAC;CAC9C;AAED,MAAM,WAAW,qBAAqB,CAAC,CAAC,GAAG,MAAM,CAAE,SAAQ,aAAa,CAAC,CAAC,CAAC;IAIzE,KAAK,CAAC,IAAI,OAAO,CAAC,IAAI,CAAC,CAAC;IAExB,KAAK,CAAC,IAAI,OAAO,CAAC,IAAI,CAAC,CAAC;CACzB"} apollo-server-demo/node_modules/apollo-server-caching/dist/KeyValueCache.js.map 0000644 0001750 0000144 00000000201 03560116604 027333 0 ustar andreh users {"version":3,"file":"KeyValueCache.js","sourceRoot":"","sources":["../src/KeyValueCache.ts"],"names":[],"mappings":";;AAOC,CAAC"} apollo-server-demo/node_modules/apollo-server-caching/dist/PrefixingKeyValueCache.js.map 0000644 0001750 0000144 00000001157 03560116604 031222 0 ustar andreh users {"version":3,"file":"PrefixingKeyValueCache.js","sourceRoot":"","sources":["../src/PrefixingKeyValueCache.ts"],"names":[],"mappings":";;;AAYA,MAAa,sBAAsB;IACjC,YAAoB,OAAyB,EAAU,MAAc;QAAjD,YAAO,GAAP,OAAO,CAAkB;QAAU,WAAM,GAAN,MAAM,CAAQ;IAAG,CAAC;IAEzE,GAAG,CAAC,GAAW;QACb,OAAO,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,IAAI,CAAC,MAAM,GAAG,GAAG,CAAC,CAAC;IAC7C,CAAC;IACD,GAAG,CAAC,GAAW,EAAE,KAAQ,EAAE,OAAiC;QAC1D,OAAO,IAAI,CAAC,OAAO,CAAC,GAAG,CAAC,IAAI,CAAC,MAAM,GAAG,GAAG,EAAE,KAAK,EAAE,OAAO,CAAC,CAAC;IAC7D,CAAC;IACD,MAAM,CAAC,GAAW;QAChB,OAAO,IAAI,CAAC,OAAO,CAAC,MAAM,CAAC,IAAI,CAAC,MAAM,GAAG,GAAG,CAAC,CAAC;IAChD,CAAC;CACF;AAZD,wDAYC"} apollo-server-demo/node_modules/apollo-server-caching/dist/index.js.map 0000644 0001750 0000144 00000000260 03560116604 025776 0 ustar andreh users {"version":3,"file":"index.js","sourceRoot":"","sources":["../src/index.ts"],"names":[],"mappings":";;AAKA,uDAAsD;AAA7C,oHAAA,gBAAgB,OAAA;AACzB,mEAAkE;AAAzD,gIAAA,sBAAsB,OAAA"} apollo-server-demo/node_modules/apollo-server-caching/dist/PrefixingKeyValueCache.d.ts 0000644 0001750 0000144 00000000755 03560116604 030705 0 ustar andreh users import { KeyValueCache, KeyValueCacheSetOptions } from './KeyValueCache'; export declare class PrefixingKeyValueCache<V = string> implements KeyValueCache<V> { private wrapped; private prefix; constructor(wrapped: KeyValueCache<V>, prefix: string); get(key: string): Promise<V | undefined>; set(key: string, value: V, options?: KeyValueCacheSetOptions): Promise<void>; delete(key: string): Promise<boolean | void>; } //# sourceMappingURL=PrefixingKeyValueCache.d.ts.map apollo-server-demo/node_modules/apollo-server-caching/LICENSE 0000644 0001750 0000144 00000002154 03560116604 023623 0 ustar andreh users The MIT License (MIT) Copyright (c) 2016-2020 Apollo Graph, Inc. (Formerly Meteor Development Group, Inc.) Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/apollo-server-caching/src/ 0000755 0001750 0000144 00000000000 14067647700 023415 5 ustar andreh users apollo-server-demo/node_modules/apollo-server-caching/src/InMemoryLRUCache.ts 0000644 0001750 0000144 00000002744 03560116604 027030 0 ustar andreh users import LRUCache from 'lru-cache'; import { TestableKeyValueCache } from './KeyValueCache'; function defaultLengthCalculation(item: any) { if (Array.isArray(item) || typeof item === 'string') { return item.length; } // Go with the lru-cache default "naive" size, in lieu anything better: // https://github.com/isaacs/node-lru-cache/blob/a71be6cd/index.js#L17 return 1; } export class InMemoryLRUCache<V = string> implements TestableKeyValueCache<V> { private store: LRUCache<string, V>; // FIXME: Define reasonable default max size of the cache constructor({ maxSize = Infinity, sizeCalculator = defaultLengthCalculation, onDispose, }: { maxSize?: number; sizeCalculator?: (value: V, key: string) => number; onDispose?: (key: string, value: V) => void; } = {}) { this.store = new LRUCache({ max: maxSize, length: sizeCalculator, dispose: onDispose, }); } async get(key: string) { return this.store.get(key); } async set(key: string, value: V, options?: { ttl?: number }) { const maxAge = options && options.ttl && options.ttl * 1000; this.store.set(key, value, maxAge); } async delete(key: string) { this.store.del(key); } // Drops all data from the cache. This should only be used by test suites --- // production code should never drop all data from an end user cache. async flush(): Promise<void> { this.store.reset(); } async getTotalSize() { return this.store.length; } } apollo-server-demo/node_modules/apollo-server-caching/src/__tests__/ 0000755 0001750 0000144 00000000000 14067647700 025353 5 ustar andreh users apollo-server-demo/node_modules/apollo-server-caching/src/__tests__/PrefixingKeyValueCache.test.ts 0000644 0001750 0000144 00000001054 03560116604 033214 0 ustar andreh users import { InMemoryLRUCache } from '../InMemoryLRUCache'; import { PrefixingKeyValueCache } from '../PrefixingKeyValueCache'; describe('PrefixingKeyValueCache', () => { it('prefixes', async () => { const inner = new InMemoryLRUCache(); const prefixing = new PrefixingKeyValueCache(inner, 'prefix:'); await prefixing.set('foo', 'bar'); expect(await prefixing.get('foo')).toBe('bar'); expect(await inner.get('prefix:foo')).toBe('bar'); await prefixing.delete('foo'); expect(await prefixing.get('foo')).toBe(undefined); }); }); apollo-server-demo/node_modules/apollo-server-caching/src/__tests__/tsconfig.json 0000644 0001750 0000144 00000000223 03560116604 030045 0 ustar andreh users { "extends": "../../../../tsconfig.test.base", "include": ["**/*", "../../../../__mocks__"], "references": [ { "path": "../../" }, ] } apollo-server-demo/node_modules/apollo-server-caching/src/__tests__/testsuite.ts 0000644 0001750 0000144 00000005071 03560116604 027745 0 ustar andreh users import { advanceTimeBy, mockDate, unmockDate } from '__mocks__/date'; import { TestableKeyValueCache } from '../'; export function testKeyValueCache_Basics(keyValueCache: TestableKeyValueCache) { describe('basic cache functionality', () => { beforeEach(() => { keyValueCache.flush && keyValueCache.flush(); }); it('can do a basic get and set', async () => { await keyValueCache.set('hello', 'world'); expect(await keyValueCache.get('hello')).toBe('world'); expect(await keyValueCache.get('missing')).toBeUndefined(); }); it('can do a basic set and delete', async () => { await keyValueCache.set('hello', 'world'); expect(await keyValueCache.get('hello')).toBe('world'); await keyValueCache.delete('hello'); expect(await keyValueCache.get('hello')).toBeUndefined(); }); }); } export function testKeyValueCache_Expiration( keyValueCache: TestableKeyValueCache, ) { describe('time-based cache expunging', () => { beforeAll(() => { mockDate(); jest.useFakeTimers(); }); beforeEach(() => { keyValueCache.flush && keyValueCache.flush(); }); afterAll(() => { unmockDate(); keyValueCache.close && keyValueCache.close(); }); it('is able to expire keys based on ttl', async () => { await keyValueCache.set('short', 's', { ttl: 1 }); await keyValueCache.set('long', 'l', { ttl: 5 }); expect(await keyValueCache.get('short')).toBe('s'); expect(await keyValueCache.get('long')).toBe('l'); advanceTimeBy(1500); jest.advanceTimersByTime(1500); expect(await keyValueCache.get('short')).toBeUndefined(); expect(await keyValueCache.get('long')).toBe('l'); advanceTimeBy(4000); jest.advanceTimersByTime(4000); expect(await keyValueCache.get('short')).toBeUndefined(); expect(await keyValueCache.get('long')).toBeUndefined(); }); it('does not expire when ttl is null', async () => { await keyValueCache.set('forever', 'yours', { ttl: null }); expect(await keyValueCache.get('forever')).toBe('yours'); advanceTimeBy(1500); jest.advanceTimersByTime(1500); expect(await keyValueCache.get('forever')).toBe('yours'); advanceTimeBy(4000); jest.advanceTimersByTime(4000); expect(await keyValueCache.get('forever')).toBe('yours'); }); }); } export function testKeyValueCache(keyValueCache: TestableKeyValueCache) { describe('KeyValueCache Test Suite', () => { testKeyValueCache_Basics(keyValueCache); testKeyValueCache_Expiration(keyValueCache); }); } apollo-server-demo/node_modules/apollo-server-caching/src/__tests__/InMemoryLRUCache.test.ts 0000644 0001750 0000144 00000000532 03560116604 031735 0 ustar andreh users import { testKeyValueCache_Basics, testKeyValueCache_Expiration, } from '../../../apollo-server-caching/src/__tests__/testsuite'; import { InMemoryLRUCache } from '../InMemoryLRUCache'; describe('InMemoryLRUCache', () => { const cache = new InMemoryLRUCache(); testKeyValueCache_Basics(cache); testKeyValueCache_Expiration(cache); }); apollo-server-demo/node_modules/apollo-server-caching/src/PrefixingKeyValueCache.ts 0000644 0001750 0000144 00000002161 03560116604 030300 0 ustar andreh users import { KeyValueCache, KeyValueCacheSetOptions } from './KeyValueCache'; // PrefixingKeyValueCache wraps another cache and adds a prefix to all keys used // by all operations. This allows multiple features to share the same // underlying cache without conflicts. // // Note that PrefixingKeyValueCache explicitly does not implement // TestableKeyValueCache, and notably does not implement the flush() // method. Most implementations of TestableKeyValueCache.flush() send a simple // command that wipes the entire backend cache system, which wouldn't support // "only wipe the part of the cache with this prefix", so trying to provide a // flush() method here could be confusingly dangerous. export class PrefixingKeyValueCache<V = string> implements KeyValueCache<V> { constructor(private wrapped: KeyValueCache<V>, private prefix: string) {} get(key: string) { return this.wrapped.get(this.prefix + key); } set(key: string, value: V, options?: KeyValueCacheSetOptions) { return this.wrapped.set(this.prefix + key, value, options); } delete(key: string) { return this.wrapped.delete(this.prefix + key); } } apollo-server-demo/node_modules/apollo-server-caching/src/KeyValueCache.ts 0000644 0001750 0000144 00000001567 03560116604 026435 0 ustar andreh users /** Options for {@link KeyValueCache.set} */ export interface KeyValueCacheSetOptions { /** * Specified in **seconds**, the time-to-live (TTL) value limits the lifespan * of the data being stored in the cache. */ ttl?: number | null }; export interface KeyValueCache<V = string> { get(key: string): Promise<V | undefined>; set(key: string, value: V, options?: KeyValueCacheSetOptions): Promise<void>; delete(key: string): Promise<boolean | void>; } export interface TestableKeyValueCache<V = string> extends KeyValueCache<V> { // Drops all data from the cache. This should only be used by test suites --- // production code should never drop all data from an end user cache (and // notably, PrefixingKeyValueCache intentionally doesn't implement this). flush?(): Promise<void>; // Close connections associated with this cache. close?(): Promise<void>; } apollo-server-demo/node_modules/apollo-server-caching/src/index.ts 0000644 0001750 0000144 00000000342 03560116604 025061 0 ustar andreh users export { KeyValueCache, TestableKeyValueCache, KeyValueCacheSetOptions, } from './KeyValueCache'; export { InMemoryLRUCache } from './InMemoryLRUCache'; export { PrefixingKeyValueCache } from './PrefixingKeyValueCache'; apollo-server-demo/node_modules/apollo-server-caching/README.md 0000644 0001750 0000144 00000004114 03560116604 024073 0 ustar andreh users # apollo-server-caching [](https://badge.fury.io/js/apollo-server-caching) [](https://circleci.com/gh/apollographql/apollo-server) ## Implementing your own Cache Internally, Apollo Server uses the `KeyValueCache` interface to provide a caching store for the Data Sources. An in-memory LRU cache is used by default, and we provide connectors for [Memcached](../apollo-server-cache-memcached)/[Redis](../apollo-server-cache-redis) backends. Built with extensibility in mind, you can also implement your own cache to use with Apollo Server, in a way that best suits your application needs. It needs to implement the following interface that can be exported from `apollo-server-caching`: ```typescript export interface KeyValueCache { get(key: string): Promise<string | undefined>; set(key: string, value: string, options?: { ttl?: number }): Promise<void>; } ``` > The `ttl` value for the `set` method's `options` is specified in __seconds__. ## Testing cache implementations ### Test helpers You can export and run a jest test suite from `apollo-server-caching` to test your implementation: ```typescript // ../__tests__/YourKeyValueCache.test.ts import YourKeyValueCache from '../src/YourKeyValueCache'; import { testKeyValueCache } from 'apollo-server-caching'; testKeyValueCache(new MemcachedCache('localhost')); ``` The default `testKeyValueCache` helper will run all key-value store tests on the specified store, including basic `get` and `set` functionality, along with time-based expunging rules. Some key-value cache implementations may not be able to support the full suite of tests (for example, some tests might not be able to expire based on time). For those cases, there are more granular implementations which can be used: * `testKeyValueCache_Basic` * `testKeyValueCache_Expiration` For more details, consult the [source for `apollo-server-caching`](./src/__tests__/testsuite.ts). ### Running tests Run tests with `jest --verbose` apollo-server-demo/node_modules/apollo-server-caching/package.json 0000644 0001750 0000144 00000001600 03560116604 025077 0 ustar andreh users { "name": "apollo-server-caching", "version": "0.5.3", "author": "Apollo <opensource@apollographql.com>", "license": "MIT", "repository": { "type": "git", "url": "https://github.com/apollographql/apollo-server", "directory": "packages/apollo-server-caching" }, "homepage": "https://github.com/apollographql/apollo-server#readme", "bugs": { "url": "https://github.com/apollographql/apollo-server/issues" }, "main": "dist/index.js", "types": "dist/index.d.ts", "engines": { "node": ">=6" }, "dependencies": { "lru-cache": "^6.0.0" }, "gitHead": "c212627be591cd2c2469321b58b15f1b7909778d" ,"_resolved": "https://registry.npmjs.org/apollo-server-caching/-/apollo-server-caching-0.5.3.tgz" ,"_integrity": "sha512-iMi3087iphDAI0U2iSBE9qtx9kQoMMEWr6w+LwXruBD95ek9DWyj7OeC2U/ngLjRsXM43DoBDXlu7R+uMjahrQ==" ,"_from": "apollo-server-caching@0.5.3" } apollo-server-demo/node_modules/fast-json-stable-stringify/ 0000755 0001750 0000144 00000000000 14067647700 023632 5 ustar andreh users apollo-server-demo/node_modules/fast-json-stable-stringify/index.js 0000644 0001750 0000144 00000003465 03560116604 025275 0 ustar andreh users 'use strict'; module.exports = function (data, opts) { if (!opts) opts = {}; if (typeof opts === 'function') opts = { cmp: opts }; var cycles = (typeof opts.cycles === 'boolean') ? opts.cycles : false; var cmp = opts.cmp && (function (f) { return function (node) { return function (a, b) { var aobj = { key: a, value: node[a] }; var bobj = { key: b, value: node[b] }; return f(aobj, bobj); }; }; })(opts.cmp); var seen = []; return (function stringify (node) { if (node && node.toJSON && typeof node.toJSON === 'function') { node = node.toJSON(); } if (node === undefined) return; if (typeof node == 'number') return isFinite(node) ? '' + node : 'null'; if (typeof node !== 'object') return JSON.stringify(node); var i, out; if (Array.isArray(node)) { out = '['; for (i = 0; i < node.length; i++) { if (i) out += ','; out += stringify(node[i]) || 'null'; } return out + ']'; } if (node === null) return 'null'; if (seen.indexOf(node) !== -1) { if (cycles) return JSON.stringify('__cycle__'); throw new TypeError('Converting circular structure to JSON'); } var seenIndex = seen.push(node) - 1; var keys = Object.keys(node).sort(cmp && cmp(node)); out = ''; for (i = 0; i < keys.length; i++) { var key = keys[i]; var value = stringify(node[key]); if (!value) continue; if (out) out += ','; out += JSON.stringify(key) + ':' + value; } seen.splice(seenIndex, 1); return '{' + out + '}'; })(data); }; apollo-server-demo/node_modules/fast-json-stable-stringify/LICENSE 0000644 0001750 0000144 00000002171 03560116604 024626 0 ustar andreh users This software is released under the MIT license: Copyright (c) 2017 Evgeny Poberezkin Copyright (c) 2013 James Halliday Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/fast-json-stable-stringify/index.d.ts 0000644 0001750 0000144 00000000156 03560116604 025523 0 ustar andreh users declare module 'fast-json-stable-stringify' { function stringify(obj: any): string; export = stringify; } apollo-server-demo/node_modules/fast-json-stable-stringify/benchmark/ 0000755 0001750 0000144 00000000000 14067647700 025564 5 ustar andreh users apollo-server-demo/node_modules/fast-json-stable-stringify/benchmark/index.js 0000644 0001750 0000144 00000001344 03560116604 027221 0 ustar andreh users 'use strict'; const Benchmark = require('benchmark'); const suite = new Benchmark.Suite; const testData = require('./test.json'); const stringifyPackages = { // 'JSON.stringify': JSON.stringify, 'fast-json-stable-stringify': require('../index'), 'json-stable-stringify': true, 'fast-stable-stringify': true, 'faster-stable-stringify': true }; for (const name in stringifyPackages) { let func = stringifyPackages[name]; if (func === true) func = require(name); suite.add(name, function() { func(testData); }); } suite .on('cycle', (event) => console.log(String(event.target))) .on('complete', function () { console.log('The fastest is ' + this.filter('fastest').map('name')); }) .run({async: true}); apollo-server-demo/node_modules/fast-json-stable-stringify/benchmark/test.json 0000644 0001750 0000144 00000007364 03560116604 027436 0 ustar andreh users [ { "_id": "59ef4a83ee8364808d761beb", "index": 0, "guid": "e50ffae9-7128-4148-9ee5-40c3fc523c5d", "isActive": false, "balance": "$2,341.81", "picture": "http://placehold.it/32x32", "age": 28, "eyeColor": "brown", "name": "Carey Savage", "gender": "female", "company": "VERAQ", "email": "careysavage@veraq.com", "phone": "+1 (897) 574-3014", "address": "458 Willow Street, Henrietta, California, 7234", "about": "Nisi reprehenderit nulla ad officia pariatur non dolore laboris irure cupidatat laborum. Minim eu ex Lorem adipisicing exercitation irure minim sunt est enim mollit incididunt voluptate nulla. Ut mollit anim reprehenderit et aliqua ex esse aliquip. Aute sit duis deserunt do incididunt consequat minim qui dolor commodo deserunt et voluptate.\r\n", "registered": "2014-05-21T01:56:51 -01:00", "latitude": 63.89502, "longitude": 62.369807, "tags": [ "nostrud", "nisi", "consectetur", "ullamco", "cupidatat", "culpa", "commodo" ], "friends": [ { "id": 0, "name": "Henry Walls" }, { "id": 1, "name": "Janice Baker" }, { "id": 2, "name": "Russell Bush" } ], "greeting": "Hello, Carey Savage! You have 4 unread messages.", "favoriteFruit": "banana" }, { "_id": "59ef4a83ff5774a691454e89", "index": 1, "guid": "2bee9efc-4095-4c2e-87ef-d08c8054c89d", "isActive": true, "balance": "$1,618.15", "picture": "http://placehold.it/32x32", "age": 35, "eyeColor": "blue", "name": "Elinor Pearson", "gender": "female", "company": "FLEXIGEN", "email": "elinorpearson@flexigen.com", "phone": "+1 (923) 548-3751", "address": "600 Bayview Avenue, Draper, Montana, 3088", "about": "Mollit commodo ea sit Lorem velit. Irure anim esse Lorem sint quis officia ut. Aliqua nisi dolore in aute deserunt mollit ex ea in mollit.\r\n", "registered": "2017-04-22T07:58:41 -01:00", "latitude": -87.824919, "longitude": 69.538927, "tags": [ "fugiat", "labore", "proident", "quis", "eiusmod", "qui", "est" ], "friends": [ { "id": 0, "name": "Massey Wagner" }, { "id": 1, "name": "Marcella Ferrell" }, { "id": 2, "name": "Evans Mckee" } ], "greeting": "Hello, Elinor Pearson! You have 3 unread messages.", "favoriteFruit": "strawberry" }, { "_id": "59ef4a839ec8a4be4430b36b", "index": 2, "guid": "ddd6e8c0-95bd-416d-8b46-a768d6363809", "isActive": false, "balance": "$2,046.95", "picture": "http://placehold.it/32x32", "age": 40, "eyeColor": "green", "name": "Irwin Davidson", "gender": "male", "company": "DANJA", "email": "irwindavidson@danja.com", "phone": "+1 (883) 537-2041", "address": "439 Cook Street, Chapin, Kentucky, 7398", "about": "Irure velit non commodo aliqua exercitation ut nostrud minim magna. Dolor ad ad ut irure eu. Non pariatur dolor eiusmod ipsum do et exercitation cillum. Et amet laboris minim eiusmod ullamco magna ea reprehenderit proident sunt.\r\n", "registered": "2016-09-01T07:49:08 -01:00", "latitude": -49.803812, "longitude": 104.93279, "tags": [ "consequat", "enim", "quis", "magna", "est", "culpa", "tempor" ], "friends": [ { "id": 0, "name": "Ruth Hansen" }, { "id": 1, "name": "Kathrine Austin" }, { "id": 2, "name": "Rivera Munoz" } ], "greeting": "Hello, Irwin Davidson! You have 2 unread messages.", "favoriteFruit": "banana" } ] apollo-server-demo/node_modules/fast-json-stable-stringify/.github/ 0000755 0001750 0000144 00000000000 14067647700 025172 5 ustar andreh users apollo-server-demo/node_modules/fast-json-stable-stringify/.github/FUNDING.yml 0000644 0001750 0000144 00000000053 03560116604 026773 0 ustar andreh users tidelift: "npm/fast-json-stable-stringify" apollo-server-demo/node_modules/fast-json-stable-stringify/README.md 0000644 0001750 0000144 00000006675 03560116604 025115 0 ustar andreh users # fast-json-stable-stringify Deterministic `JSON.stringify()` - a faster version of [@substack](https://github.com/substack)'s json-stable-strigify without [jsonify](https://github.com/substack/jsonify). You can also pass in a custom comparison function. [](https://travis-ci.org/epoberezkin/fast-json-stable-stringify) [](https://coveralls.io/github/epoberezkin/fast-json-stable-stringify?branch=master) # example ``` js var stringify = require('fast-json-stable-stringify'); var obj = { c: 8, b: [{z:6,y:5,x:4},7], a: 3 }; console.log(stringify(obj)); ``` output: ``` {"a":3,"b":[{"x":4,"y":5,"z":6},7],"c":8} ``` # methods ``` js var stringify = require('fast-json-stable-stringify') ``` ## var str = stringify(obj, opts) Return a deterministic stringified string `str` from the object `obj`. ## options ### cmp If `opts` is given, you can supply an `opts.cmp` to have a custom comparison function for object keys. Your function `opts.cmp` is called with these parameters: ``` js opts.cmp({ key: akey, value: avalue }, { key: bkey, value: bvalue }) ``` For example, to sort on the object key names in reverse order you could write: ``` js var stringify = require('fast-json-stable-stringify'); var obj = { c: 8, b: [{z:6,y:5,x:4},7], a: 3 }; var s = stringify(obj, function (a, b) { return a.key < b.key ? 1 : -1; }); console.log(s); ``` which results in the output string: ``` {"c":8,"b":[{"z":6,"y":5,"x":4},7],"a":3} ``` Or if you wanted to sort on the object values in reverse order, you could write: ``` var stringify = require('fast-json-stable-stringify'); var obj = { d: 6, c: 5, b: [{z:3,y:2,x:1},9], a: 10 }; var s = stringify(obj, function (a, b) { return a.value < b.value ? 1 : -1; }); console.log(s); ``` which outputs: ``` {"d":6,"c":5,"b":[{"z":3,"y":2,"x":1},9],"a":10} ``` ### cycles Pass `true` in `opts.cycles` to stringify circular property as `__cycle__` - the result will not be a valid JSON string in this case. TypeError will be thrown in case of circular object without this option. # install With [npm](https://npmjs.org) do: ``` npm install fast-json-stable-stringify ``` # benchmark To run benchmark (requires Node.js 6+): ``` node benchmark ``` Results: ``` fast-json-stable-stringify x 17,189 ops/sec ±1.43% (83 runs sampled) json-stable-stringify x 13,634 ops/sec ±1.39% (85 runs sampled) fast-stable-stringify x 20,212 ops/sec ±1.20% (84 runs sampled) faster-stable-stringify x 15,549 ops/sec ±1.12% (84 runs sampled) The fastest is fast-stable-stringify ``` ## Enterprise support fast-json-stable-stringify package is a part of [Tidelift enterprise subscription](https://tidelift.com/subscription/pkg/npm-fast-json-stable-stringify?utm_source=npm-fast-json-stable-stringify&utm_medium=referral&utm_campaign=enterprise&utm_term=repo) - it provides a centralised commercial support to open-source software users, in addition to the support provided by software maintainers. ## Security contact To report a security vulnerability, please use the [Tidelift security contact](https://tidelift.com/security). Tidelift will coordinate the fix and disclosure. Please do NOT report security vulnerability via GitHub issues. # license [MIT](https://github.com/epoberezkin/fast-json-stable-stringify/blob/master/LICENSE) apollo-server-demo/node_modules/fast-json-stable-stringify/package.json 0000644 0001750 0000144 00000002764 03560116604 026117 0 ustar andreh users { "name": "fast-json-stable-stringify", "version": "2.1.0", "description": "deterministic `JSON.stringify()` - a faster version of substack's json-stable-strigify without jsonify", "main": "index.js", "types": "index.d.ts", "dependencies": {}, "devDependencies": { "benchmark": "^2.1.4", "coveralls": "^3.0.0", "eslint": "^6.7.0", "fast-stable-stringify": "latest", "faster-stable-stringify": "latest", "json-stable-stringify": "latest", "nyc": "^14.1.0", "pre-commit": "^1.2.2", "tape": "^4.11.0" }, "scripts": { "eslint": "eslint index.js test", "test-spec": "tape test/*.js", "test": "npm run eslint && nyc npm run test-spec" }, "repository": { "type": "git", "url": "git://github.com/epoberezkin/fast-json-stable-stringify.git" }, "homepage": "https://github.com/epoberezkin/fast-json-stable-stringify", "keywords": [ "json", "stringify", "deterministic", "hash", "stable" ], "author": { "name": "James Halliday", "email": "mail@substack.net", "url": "http://substack.net" }, "license": "MIT", "nyc": { "exclude": [ "test", "node_modules" ], "reporter": [ "lcov", "text-summary" ] } ,"_resolved": "https://registry.npmjs.org/fast-json-stable-stringify/-/fast-json-stable-stringify-2.1.0.tgz" ,"_integrity": "sha512-lhd/wF+Lk98HZoTCtlVraHtfh5XYijIjalXck7saUtuanSDyLMxnHhSXEDJqHxD7msR8D0uCmqlkwjCV8xvwHw==" ,"_from": "fast-json-stable-stringify@2.1.0" } apollo-server-demo/node_modules/fast-json-stable-stringify/example/ 0000755 0001750 0000144 00000000000 14067647700 025265 5 ustar andreh users apollo-server-demo/node_modules/fast-json-stable-stringify/example/str.js 0000644 0001750 0000144 00000000141 03560116604 026415 0 ustar andreh users var stringify = require('../'); var obj = { c: 6, b: [4,5], a: 3 }; console.log(stringify(obj)); apollo-server-demo/node_modules/fast-json-stable-stringify/example/value_cmp.js 0000644 0001750 0000144 00000000274 03560116604 027567 0 ustar andreh users var stringify = require('../'); var obj = { d: 6, c: 5, b: [{z:3,y:2,x:1},9], a: 10 }; var s = stringify(obj, function (a, b) { return a.value < b.value ? 1 : -1; }); console.log(s); apollo-server-demo/node_modules/fast-json-stable-stringify/example/nested.js 0000644 0001750 0000144 00000000155 03560116604 027074 0 ustar andreh users var stringify = require('../'); var obj = { c: 8, b: [{z:6,y:5,x:4},7], a: 3 }; console.log(stringify(obj)); apollo-server-demo/node_modules/fast-json-stable-stringify/example/key_cmp.js 0000644 0001750 0000144 00000000261 03560116604 027237 0 ustar andreh users var stringify = require('../'); var obj = { c: 8, b: [{z:6,y:5,x:4},7], a: 3 }; var s = stringify(obj, function (a, b) { return a.key < b.key ? 1 : -1; }); console.log(s); apollo-server-demo/node_modules/fast-json-stable-stringify/test/ 0000755 0001750 0000144 00000000000 14067647700 024611 5 ustar andreh users apollo-server-demo/node_modules/fast-json-stable-stringify/test/str.js 0000644 0001750 0000144 00000002146 03560116604 025750 0 ustar andreh users 'use strict'; var test = require('tape'); var stringify = require('../'); test('simple object', function (t) { t.plan(1); var obj = { c: 6, b: [4,5], a: 3, z: null }; t.equal(stringify(obj), '{"a":3,"b":[4,5],"c":6,"z":null}'); }); test('object with undefined', function (t) { t.plan(1); var obj = { a: 3, z: undefined }; t.equal(stringify(obj), '{"a":3}'); }); test('object with null', function (t) { t.plan(1); var obj = { a: 3, z: null }; t.equal(stringify(obj), '{"a":3,"z":null}'); }); test('object with NaN and Infinity', function (t) { t.plan(1); var obj = { a: 3, b: NaN, c: Infinity }; t.equal(stringify(obj), '{"a":3,"b":null,"c":null}'); }); test('array with undefined', function (t) { t.plan(1); var obj = [4, undefined, 6]; t.equal(stringify(obj), '[4,null,6]'); }); test('object with empty string', function (t) { t.plan(1); var obj = { a: 3, z: '' }; t.equal(stringify(obj), '{"a":3,"z":""}'); }); test('array with empty string', function (t) { t.plan(1); var obj = [4, '', 6]; t.equal(stringify(obj), '[4,"",6]'); }); apollo-server-demo/node_modules/fast-json-stable-stringify/test/cmp.js 0000644 0001750 0000144 00000000536 03560116604 025720 0 ustar andreh users 'use strict'; var test = require('tape'); var stringify = require('../'); test('custom comparison function', function (t) { t.plan(1); var obj = { c: 8, b: [{z:6,y:5,x:4},7], a: 3 }; var s = stringify(obj, function (a, b) { return a.key < b.key ? 1 : -1; }); t.equal(s, '{"c":8,"b":[{"z":6,"y":5,"x":4},7],"a":3}'); }); apollo-server-demo/node_modules/fast-json-stable-stringify/test/to-json.js 0000644 0001750 0000144 00000001137 03560116604 026530 0 ustar andreh users 'use strict'; var test = require('tape'); var stringify = require('../'); test('toJSON function', function (t) { t.plan(1); var obj = { one: 1, two: 2, toJSON: function() { return { one: 1 }; } }; t.equal(stringify(obj), '{"one":1}' ); }); test('toJSON returns string', function (t) { t.plan(1); var obj = { one: 1, two: 2, toJSON: function() { return 'one'; } }; t.equal(stringify(obj), '"one"'); }); test('toJSON returns array', function (t) { t.plan(1); var obj = { one: 1, two: 2, toJSON: function() { return ['one']; } }; t.equal(stringify(obj), '["one"]'); }); apollo-server-demo/node_modules/fast-json-stable-stringify/test/nested.js 0000644 0001750 0000144 00000002173 03560116604 026422 0 ustar andreh users 'use strict'; var test = require('tape'); var stringify = require('../'); test('nested', function (t) { t.plan(1); var obj = { c: 8, b: [{z:6,y:5,x:4},7], a: 3 }; t.equal(stringify(obj), '{"a":3,"b":[{"x":4,"y":5,"z":6},7],"c":8}'); }); test('cyclic (default)', function (t) { t.plan(1); var one = { a: 1 }; var two = { a: 2, one: one }; one.two = two; try { stringify(one); } catch (ex) { t.equal(ex.toString(), 'TypeError: Converting circular structure to JSON'); } }); test('cyclic (specifically allowed)', function (t) { t.plan(1); var one = { a: 1 }; var two = { a: 2, one: one }; one.two = two; t.equal(stringify(one, {cycles:true}), '{"a":1,"two":{"a":2,"one":"__cycle__"}}'); }); test('repeated non-cyclic value', function(t) { t.plan(1); var one = { x: 1 }; var two = { a: one, b: one }; t.equal(stringify(two), '{"a":{"x":1},"b":{"x":1}}'); }); test('acyclic but with reused obj-property pointers', function (t) { t.plan(1); var x = { a: 1 }; var y = { b: x, c: x }; t.equal(stringify(y), '{"b":{"a":1},"c":{"a":1}}'); }); apollo-server-demo/node_modules/fast-json-stable-stringify/.eslintrc.yml 0000644 0001750 0000144 00000001062 03560116604 026243 0 ustar andreh users extends: eslint:recommended env: node: true browser: true rules: block-scoped-var: 2 callback-return: 2 dot-notation: 2 indent: 2 linebreak-style: [2, unix] new-cap: 2 no-console: [2, allow: [warn, error]] no-else-return: 2 no-eq-null: 2 no-fallthrough: 2 no-invalid-this: 2 no-return-assign: 2 no-shadow: 1 no-trailing-spaces: 2 no-use-before-define: [2, nofunc] quotes: [2, single, avoid-escape] semi: [2, always] strict: [2, global] valid-jsdoc: [2, requireReturn: false] no-control-regex: 0 no-useless-escape: 2 apollo-server-demo/node_modules/fast-json-stable-stringify/.travis.yml 0000644 0001750 0000144 00000000157 03560116604 025734 0 ustar andreh users language: node_js node_js: - "8" - "10" - "12" - "13" after_script: - coveralls < coverage/lcov.info apollo-server-demo/node_modules/apollo-server/ 0000755 0001750 0000144 00000000000 14067647700 021234 5 ustar andreh users apollo-server-demo/node_modules/apollo-server/dist/ 0000755 0001750 0000144 00000000000 14067647700 022177 5 ustar andreh users apollo-server-demo/node_modules/apollo-server/dist/index.js 0000644 0001750 0000144 00000010665 03560116604 023642 0 ustar andreh users "use strict"; var __createBinding = (this && this.__createBinding) || (Object.create ? (function(o, m, k, k2) { if (k2 === undefined) k2 = k; Object.defineProperty(o, k2, { enumerable: true, get: function() { return m[k]; } }); }) : (function(o, m, k, k2) { if (k2 === undefined) k2 = k; o[k2] = m[k]; })); var __exportStar = (this && this.__exportStar) || function(m, exports) { for (var p in m) if (p !== "default" && !exports.hasOwnProperty(p)) __createBinding(exports, m, p); }; var __awaiter = (this && this.__awaiter) || function (thisArg, _arguments, P, generator) { function adopt(value) { return value instanceof P ? value : new P(function (resolve) { resolve(value); }); } return new (P || (P = Promise))(function (resolve, reject) { function fulfilled(value) { try { step(generator.next(value)); } catch (e) { reject(e); } } function rejected(value) { try { step(generator["throw"](value)); } catch (e) { reject(e); } } function step(result) { result.done ? resolve(result.value) : adopt(result.value).then(fulfilled, rejected); } step((generator = generator.apply(thisArg, _arguments || [])).next()); }); }; var __importDefault = (this && this.__importDefault) || function (mod) { return (mod && mod.__esModule) ? mod : { "default": mod }; }; Object.defineProperty(exports, "__esModule", { value: true }); exports.ApolloServer = void 0; const express_1 = __importDefault(require("express")); const http_1 = __importDefault(require("http")); const apollo_server_express_1 = require("apollo-server-express"); __exportStar(require("./exports"), exports); class ApolloServer extends apollo_server_express_1.ApolloServer { constructor(config) { super(config); this.cors = config && config.cors; this.onHealthCheck = config && config.onHealthCheck; } createServerInfo(server, subscriptionsPath) { const serverInfo = Object.assign(Object.assign({}, server.address()), { server, subscriptionsPath }); let hostForUrl = serverInfo.address; if (serverInfo.address === '' || serverInfo.address === '::') hostForUrl = 'localhost'; serverInfo.url = require('url').format({ protocol: 'http', hostname: hostForUrl, port: serverInfo.port, pathname: this.graphqlPath, }); serverInfo.subscriptionsUrl = require('url').format({ protocol: 'ws', hostname: hostForUrl, port: serverInfo.port, slashes: true, pathname: subscriptionsPath, }); return serverInfo; } applyMiddleware() { throw new Error('To use Apollo Server with an existing express application, please use apollo-server-express'); } listen(...opts) { const _super = Object.create(null, { applyMiddleware: { get: () => super.applyMiddleware } }); return __awaiter(this, void 0, void 0, function* () { const app = express_1.default(); app.disable('x-powered-by'); _super.applyMiddleware.call(this, { app, path: '/', bodyParserConfig: { limit: '50mb' }, onHealthCheck: this.onHealthCheck, cors: typeof this.cors !== 'undefined' ? this.cors : { origin: '*', }, }); const httpServer = http_1.default.createServer(app); this.httpServer = httpServer; if (this.subscriptionServerOptions) { this.installSubscriptionHandlers(httpServer); } yield new Promise(resolve => { httpServer.once('listening', resolve); httpServer.listen(...(opts.length ? opts : [{ port: 4000 }])); }); return this.createServerInfo(httpServer, this.subscriptionsPath); }); } stop() { const _super = Object.create(null, { stop: { get: () => super.stop } }); return __awaiter(this, void 0, void 0, function* () { if (this.httpServer) { const httpServer = this.httpServer; yield new Promise(resolve => httpServer.close(resolve)); this.httpServer = undefined; } yield _super.stop.call(this); }); } } exports.ApolloServer = ApolloServer; //# sourceMappingURL=index.js.map apollo-server-demo/node_modules/apollo-server/dist/exports.d.ts 0000644 0001750 0000144 00000000721 03560116604 024463 0 ustar andreh users export * from 'graphql-tools'; export * from 'graphql-subscriptions'; export { gql, GraphQLUpload, GraphQLOptions, GraphQLExtension, Config, GraphQLSchemaModule, ApolloError, toApolloError, SyntaxError, ValidationError, AuthenticationError, ForbiddenError, UserInputError, defaultPlaygroundOptions, PlaygroundConfig, PlaygroundRenderPageOptions, } from 'apollo-server-core'; export { CorsOptions } from 'apollo-server-express'; //# sourceMappingURL=exports.d.ts.map apollo-server-demo/node_modules/apollo-server/dist/index.d.ts 0000644 0001750 0000144 00000001611 03560116604 024065 0 ustar andreh users /// <reference types="node" /> import express from 'express'; import http from 'http'; import { ApolloServer as ApolloServerBase, CorsOptions, ApolloServerExpressConfig } from 'apollo-server-express'; export * from './exports'; export interface ServerInfo { address: string; family: string; url: string; subscriptionsUrl: string; port: number | string; subscriptionsPath: string; server: http.Server; } export declare class ApolloServer extends ApolloServerBase { private httpServer?; private cors?; private onHealthCheck?; constructor(config: ApolloServerExpressConfig & { cors?: CorsOptions | boolean; onHealthCheck?: (req: express.Request) => Promise<any>; }); private createServerInfo; applyMiddleware(): void; listen(...opts: Array<any>): Promise<ServerInfo>; stop(): Promise<void>; } //# sourceMappingURL=index.d.ts.map apollo-server-demo/node_modules/apollo-server/dist/exports.js.map 0000644 0001750 0000144 00000000615 03560116604 025005 0 ustar andreh users {"version":3,"file":"exports.js","sourceRoot":"","sources":["../src/exports.ts"],"names":[],"mappings":";;;;;;;;;;;;AAAA,gDAA8B;AAC9B,wDAAsC;AAEtC,yDAmB4B;AAlB1B,yGAAA,GAAG,OAAA;AACH,mHAAA,aAAa,OAAA;AAEb,sHAAA,gBAAgB,OAAA;AAIhB,iHAAA,WAAW,OAAA;AACX,mHAAA,aAAa,OAAA;AACb,iHAAA,WAAW,OAAA;AACX,qHAAA,eAAe,OAAA;AACf,yHAAA,mBAAmB,OAAA;AACnB,oHAAA,cAAc,OAAA;AACd,oHAAA,cAAc,OAAA;AAEd,8HAAA,wBAAwB,OAAA"} apollo-server-demo/node_modules/apollo-server/dist/index.d.ts.map 0000644 0001750 0000144 00000001607 03560116604 024646 0 ustar andreh users {"version":3,"file":"index.d.ts","sourceRoot":"","sources":["../src/index.ts"],"names":[],"mappings":";AAIA,OAAO,OAAO,MAAM,SAAS,CAAC;AAC9B,OAAO,IAAI,MAAM,MAAM,CAAC;AACxB,OAAO,EACL,YAAY,IAAI,gBAAgB,EAChC,WAAW,EACX,yBAAyB,EAC1B,MAAM,uBAAuB,CAAC;AAE/B,cAAc,WAAW,CAAC;AAE1B,MAAM,WAAW,UAAU;IACzB,OAAO,EAAE,MAAM,CAAC;IAChB,MAAM,EAAE,MAAM,CAAC;IACf,GAAG,EAAE,MAAM,CAAC;IACZ,gBAAgB,EAAE,MAAM,CAAC;IACzB,IAAI,EAAE,MAAM,GAAG,MAAM,CAAC;IACtB,iBAAiB,EAAE,MAAM,CAAC;IAC1B,MAAM,EAAE,IAAI,CAAC,MAAM,CAAC;CACrB;AAED,qBAAa,YAAa,SAAQ,gBAAgB;IAChD,OAAO,CAAC,UAAU,CAAC,CAAc;IACjC,OAAO,CAAC,IAAI,CAAC,CAAwB;IACrC,OAAO,CAAC,aAAa,CAAC,CAAyC;gBAG7D,MAAM,EAAE,yBAAyB,GAAG;QAClC,IAAI,CAAC,EAAE,WAAW,GAAG,OAAO,CAAC;QAC7B,aAAa,CAAC,EAAE,CAAC,GAAG,EAAE,OAAO,CAAC,OAAO,KAAK,OAAO,CAAC,GAAG,CAAC,CAAC;KACxD;IAOH,OAAO,CAAC,gBAAgB;IA8CjB,eAAe;IAOT,MAAM,CAAC,GAAG,IAAI,EAAE,KAAK,CAAC,GAAG,CAAC,GAAG,OAAO,CAAC,UAAU,CAAC;IAuChD,IAAI;CAQlB"} apollo-server-demo/node_modules/apollo-server/dist/exports.js 0000644 0001750 0000144 00000004351 03560116604 024232 0 ustar andreh users "use strict"; var __createBinding = (this && this.__createBinding) || (Object.create ? (function(o, m, k, k2) { if (k2 === undefined) k2 = k; Object.defineProperty(o, k2, { enumerable: true, get: function() { return m[k]; } }); }) : (function(o, m, k, k2) { if (k2 === undefined) k2 = k; o[k2] = m[k]; })); var __exportStar = (this && this.__exportStar) || function(m, exports) { for (var p in m) if (p !== "default" && !exports.hasOwnProperty(p)) __createBinding(exports, m, p); }; Object.defineProperty(exports, "__esModule", { value: true }); __exportStar(require("graphql-tools"), exports); __exportStar(require("graphql-subscriptions"), exports); var apollo_server_core_1 = require("apollo-server-core"); Object.defineProperty(exports, "gql", { enumerable: true, get: function () { return apollo_server_core_1.gql; } }); Object.defineProperty(exports, "GraphQLUpload", { enumerable: true, get: function () { return apollo_server_core_1.GraphQLUpload; } }); Object.defineProperty(exports, "GraphQLExtension", { enumerable: true, get: function () { return apollo_server_core_1.GraphQLExtension; } }); Object.defineProperty(exports, "ApolloError", { enumerable: true, get: function () { return apollo_server_core_1.ApolloError; } }); Object.defineProperty(exports, "toApolloError", { enumerable: true, get: function () { return apollo_server_core_1.toApolloError; } }); Object.defineProperty(exports, "SyntaxError", { enumerable: true, get: function () { return apollo_server_core_1.SyntaxError; } }); Object.defineProperty(exports, "ValidationError", { enumerable: true, get: function () { return apollo_server_core_1.ValidationError; } }); Object.defineProperty(exports, "AuthenticationError", { enumerable: true, get: function () { return apollo_server_core_1.AuthenticationError; } }); Object.defineProperty(exports, "ForbiddenError", { enumerable: true, get: function () { return apollo_server_core_1.ForbiddenError; } }); Object.defineProperty(exports, "UserInputError", { enumerable: true, get: function () { return apollo_server_core_1.UserInputError; } }); Object.defineProperty(exports, "defaultPlaygroundOptions", { enumerable: true, get: function () { return apollo_server_core_1.defaultPlaygroundOptions; } }); //# sourceMappingURL=exports.js.map apollo-server-demo/node_modules/apollo-server/dist/index.js.map 0000644 0001750 0000144 00000004305 03560116604 024410 0 ustar andreh users {"version":3,"file":"index.js","sourceRoot":"","sources":["../src/index.ts"],"names":[],"mappings":";;;;;;;;;;;;;;;;;;;;;;;;;AAIA,sDAA8B;AAC9B,gDAAwB;AACxB,iEAI+B;AAE/B,4CAA0B;AAY1B,MAAa,YAAa,SAAQ,oCAAgB;IAKhD,YACE,MAGC;QAED,KAAK,CAAC,MAAM,CAAC,CAAC;QACd,IAAI,CAAC,IAAI,GAAG,MAAM,IAAI,MAAM,CAAC,IAAI,CAAC;QAClC,IAAI,CAAC,aAAa,GAAG,MAAM,IAAI,MAAM,CAAC,aAAa,CAAC;IACtD,CAAC;IAEO,gBAAgB,CACtB,MAAmB,EACnB,iBAA0B;QAE1B,MAAM,UAAU,mCAMV,MAAM,CAAC,OAAO,EAIhB,KACF,MAAM;YACN,iBAAiB,GAClB,CAAC;QAOF,IAAI,UAAU,GAAG,UAAU,CAAC,OAAO,CAAC;QACpC,IAAI,UAAU,CAAC,OAAO,KAAK,EAAE,IAAI,UAAU,CAAC,OAAO,KAAK,IAAI;YAC1D,UAAU,GAAG,WAAW,CAAC;QAE3B,UAAU,CAAC,GAAG,GAAG,OAAO,CAAC,KAAK,CAAC,CAAC,MAAM,CAAC;YACrC,QAAQ,EAAE,MAAM;YAChB,QAAQ,EAAE,UAAU;YACpB,IAAI,EAAE,UAAU,CAAC,IAAI;YACrB,QAAQ,EAAE,IAAI,CAAC,WAAW;SAC3B,CAAC,CAAC;QAEH,UAAU,CAAC,gBAAgB,GAAG,OAAO,CAAC,KAAK,CAAC,CAAC,MAAM,CAAC;YAClD,QAAQ,EAAE,IAAI;YACd,QAAQ,EAAE,UAAU;YACpB,IAAI,EAAE,UAAU,CAAC,IAAI;YACrB,OAAO,EAAE,IAAI;YACb,QAAQ,EAAE,iBAAiB;SAC5B,CAAC,CAAC;QAEH,OAAO,UAAU,CAAC;IACpB,CAAC;IAEM,eAAe;QACpB,MAAM,IAAI,KAAK,CACb,6FAA6F,CAC9F,CAAC;IACJ,CAAC;IAGY,MAAM,CAAC,GAAG,IAAgB;;;;;YAGrC,MAAM,GAAG,GAAG,iBAAO,EAAE,CAAC;YAEtB,GAAG,CAAC,OAAO,CAAC,cAAc,CAAC,CAAC;YAG5B,OAAM,eAAe,YAAC;gBACpB,GAAG;gBACH,IAAI,EAAE,GAAG;gBACT,gBAAgB,EAAE,EAAE,KAAK,EAAE,MAAM,EAAE;gBACnC,aAAa,EAAE,IAAI,CAAC,aAAa;gBACjC,IAAI,EACF,OAAO,IAAI,CAAC,IAAI,KAAK,WAAW;oBAC9B,CAAC,CAAC,IAAI,CAAC,IAAI;oBACX,CAAC,CAAC;wBACE,MAAM,EAAE,GAAG;qBACZ;aACR,EAAE;YAEH,MAAM,UAAU,GAAG,cAAI,CAAC,YAAY,CAAC,GAAG,CAAC,CAAC;YAC1C,IAAI,CAAC,UAAU,GAAG,UAAU,CAAC;YAE7B,IAAI,IAAI,CAAC,yBAAyB,EAAE;gBAClC,IAAI,CAAC,2BAA2B,CAAC,UAAU,CAAC,CAAC;aAC9C;YAED,MAAM,IAAI,OAAO,CAAC,OAAO,CAAC,EAAE;gBAC1B,UAAU,CAAC,IAAI,CAAC,WAAW,EAAE,OAAO,CAAC,CAAC;gBAItC,UAAU,CAAC,MAAM,CAAC,GAAG,CAAC,IAAI,CAAC,MAAM,CAAC,CAAC,CAAC,IAAI,CAAC,CAAC,CAAC,CAAC,EAAE,IAAI,EAAE,IAAI,EAAE,CAAC,CAAC,CAAC,CAAC;YAChE,CAAC,CAAC,CAAC;YAEH,OAAO,IAAI,CAAC,gBAAgB,CAAC,UAAU,EAAE,IAAI,CAAC,iBAAiB,CAAC,CAAC;QACnE,CAAC;KAAA;IAEY,IAAI;;;;;YACf,IAAI,IAAI,CAAC,UAAU,EAAE;gBACnB,MAAM,UAAU,GAAG,IAAI,CAAC,UAAU,CAAC;gBACnC,MAAM,IAAI,OAAO,CAAC,OAAO,CAAC,EAAE,CAAC,UAAU,CAAC,KAAK,CAAC,OAAO,CAAC,CAAC,CAAC;gBACxD,IAAI,CAAC,UAAU,GAAG,SAAS,CAAC;aAC7B;YACD,MAAM,OAAM,IAAI,WAAE,CAAC;QACrB,CAAC;KAAA;CACF;AApHD,oCAoHC"} apollo-server-demo/node_modules/apollo-server/dist/exports.d.ts.map 0000644 0001750 0000144 00000000624 03560116604 025241 0 ustar andreh users {"version":3,"file":"exports.d.ts","sourceRoot":"","sources":["../src/exports.ts"],"names":[],"mappings":"AAAA,cAAc,eAAe,CAAC;AAC9B,cAAc,uBAAuB,CAAC;AAEtC,OAAO,EACL,GAAG,EACH,aAAa,EACb,cAAc,EACd,gBAAgB,EAChB,MAAM,EACN,mBAAmB,EAEnB,WAAW,EACX,aAAa,EACb,WAAW,EACX,eAAe,EACf,mBAAmB,EACnB,cAAc,EACd,cAAc,EAEd,wBAAwB,EACxB,gBAAgB,EAChB,2BAA2B,GAC5B,MAAM,oBAAoB,CAAC;AAE5B,OAAO,EAAE,WAAW,EAAE,MAAM,uBAAuB,CAAC"} apollo-server-demo/node_modules/apollo-server/LICENSE 0000644 0001750 0000144 00000002154 03560116604 022231 0 ustar andreh users The MIT License (MIT) Copyright (c) 2016-2020 Apollo Graph, Inc. (Formerly Meteor Development Group, Inc.) Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/apollo-server/src/ 0000755 0001750 0000144 00000000000 14067647700 022023 5 ustar andreh users apollo-server-demo/node_modules/apollo-server/src/__tests__/ 0000755 0001750 0000144 00000000000 14067647700 023761 5 ustar andreh users apollo-server-demo/node_modules/apollo-server/src/__tests__/tsconfig.json 0000644 0001750 0000144 00000000172 03560116604 026456 0 ustar andreh users { "extends": "../../../../tsconfig.test.base", "include": ["**/*"], "references": [ { "path": "../../" }, ] } apollo-server-demo/node_modules/apollo-server/src/__tests__/index.test.ts 0000644 0001750 0000144 00000013570 03560116604 026412 0 ustar andreh users import request from 'request'; import { createApolloFetch } from 'apollo-fetch'; import { gql, ApolloServer } from '../index'; const typeDefs = gql` type Query { hello: String } `; const resolvers = { Query: { hello: () => 'hi', }, }; describe('apollo-server', () => { describe('constructor', () => { it('accepts typeDefs and resolvers', () => { expect(() => new ApolloServer({ typeDefs, resolvers })).not.toThrow; }); it('accepts typeDefs and mocks', () => { expect(() => new ApolloServer({ typeDefs, mocks: true })).not.toThrow; }); it('runs serverWillStart and serverWillStop', async () => { const fn = jest.fn(); const beAsync = () => new Promise((res) => res()); const server = new ApolloServer({ typeDefs, resolvers, plugins: [ { async serverWillStart() { fn('a'); await beAsync(); fn('b'); return { async serverWillStop() { fn('c'); await beAsync(); fn('d'); }, }; }, }, ], }); await server.listen(); expect(fn.mock.calls).toEqual([['a'], ['b']]); await server.stop(); expect(fn.mock.calls).toEqual([['a'], ['b'], ['c'], ['d']]); }); // These tests are duplicates of ones in apollo-server-integration-testsuite // We don't actually expect Jest to do much here, the purpose of these // tests is to make sure our typings are correct, and to trigger a // compile error if they aren't describe('context field', () => { describe('as a function', () => { it('can accept and return `req`', () => { expect( new ApolloServer({ typeDefs, resolvers, context: ({ req }) => ({ req }), }), ).not.toThrow; }); it('can accept nothing and return an empty object', () => { expect( new ApolloServer({ typeDefs, resolvers, context: () => ({}), }), ).not.toThrow; }); }); }); describe('as an object', () => { it('can be an empty object', () => { expect( new ApolloServer({ typeDefs, resolvers, context: {}, }), ).not.toThrow; }); it('can contain arbitrary values', () => { expect( new ApolloServer({ typeDefs, resolvers, context: { value: 'arbitrary' }, }), ).not.toThrow; }); }); }); describe('without registerServer', () => { let server: ApolloServer; afterEach(async () => { await server.stop(); }); it('can be queried', async () => { server = new ApolloServer({ typeDefs, resolvers, }); const { url: uri } = await server.listen({ port: 0 }); const apolloFetch = createApolloFetch({ uri }); const result = await apolloFetch({ query: '{hello}' }); expect(result.data).toEqual({ hello: 'hi' }); expect(result.errors).toBeUndefined(); }); it('renders GraphQL playground when browser requests', async () => { const nodeEnv = process.env.NODE_ENV; delete process.env.NODE_ENV; server = new ApolloServer({ typeDefs, resolvers, stopOnTerminationSignals: false, }); const { url } = await server.listen({ port: 0 }); return new Promise((resolve, reject) => { request( { url, method: 'GET', headers: { accept: 'text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,image/apng,*/*;q=0.8', }, }, (error, response, body) => { process.env.NODE_ENV = nodeEnv; if (error) { reject(error); } else { expect(body).toMatch('GraphQLPlayground'); expect(response.statusCode).toEqual(200); resolve(); } }, ); }); }); it('configures cors', async () => { server = new ApolloServer({ typeDefs, resolvers, }); const { url: uri } = await server.listen({ port: 0 }); const apolloFetch = createApolloFetch({ uri }).useAfter( (response, next) => { expect( response.response.headers.get('access-control-allow-origin'), ).toEqual('*'); next(); }, ); await apolloFetch({ query: '{hello}' }); }); it('configures cors', async () => { server = new ApolloServer({ typeDefs, resolvers, cors: { origin: 'localhost' }, }); const { url: uri } = await server.listen({ port: 0 }); const apolloFetch = createApolloFetch({ uri }).useAfter( (response, next) => { expect( response.response.headers.get('access-control-allow-origin'), ).toEqual('localhost'); next(); }, ); await apolloFetch({ query: '{hello}' }); }); it('creates a healthcheck endpoint', async () => { server = new ApolloServer({ typeDefs, resolvers, }); const { port } = await server.listen({ port: 0 }); return new Promise((resolve, reject) => { request( { url: `http://localhost:${port}/.well-known/apollo/server-health`, method: 'GET', }, (error, response, body) => { if (error) { reject(error); } else { expect(body).toEqual(JSON.stringify({ status: 'pass' })); expect(response.statusCode).toEqual(200); resolve(); } }, ); }); }); }); }); apollo-server-demo/node_modules/apollo-server/src/index.ts 0000644 0001750 0000144 00000010424 03560116604 023471 0 ustar andreh users // Note: express is only used if you use the ApolloServer.listen API to create // an express app for you instead of applyMiddleware (which you might not even // use with express). The dependency is unused otherwise, so don't worry if // you're not using express or your version doesn't quite match up. import express from 'express'; import http from 'http'; import { ApolloServer as ApolloServerBase, CorsOptions, ApolloServerExpressConfig, } from 'apollo-server-express'; export * from './exports'; export interface ServerInfo { address: string; family: string; url: string; subscriptionsUrl: string; port: number | string; subscriptionsPath: string; server: http.Server; } export class ApolloServer extends ApolloServerBase { private httpServer?: http.Server; private cors?: CorsOptions | boolean; private onHealthCheck?: (req: express.Request) => Promise<any>; constructor( config: ApolloServerExpressConfig & { cors?: CorsOptions | boolean; onHealthCheck?: (req: express.Request) => Promise<any>; }, ) { super(config); this.cors = config && config.cors; this.onHealthCheck = config && config.onHealthCheck; } private createServerInfo( server: http.Server, subscriptionsPath?: string, ): ServerInfo { const serverInfo: any = { // TODO: Once we bump to `@types/node@10` or higher, we can replace cast // with the `net.AddressInfo` type, rather than this custom interface. // Unfortunately, prior to the 10.x types, this type existed on `dgram`, // but not on `net`, and in later types, the `server.address()` signature // can also be a string. ...(server.address() as { address: string; family: string; port: number; }), server, subscriptionsPath, }; // Convert IPs which mean "any address" (IPv4 or IPv6) into localhost // corresponding loopback ip. Note that the url field we're setting is // primarily for consumption by our test suite. If this heuristic is wrong // for your use case, explicitly specify a frontend host (in the `host` // option to ApolloServer.listen). let hostForUrl = serverInfo.address; if (serverInfo.address === '' || serverInfo.address === '::') hostForUrl = 'localhost'; serverInfo.url = require('url').format({ protocol: 'http', hostname: hostForUrl, port: serverInfo.port, pathname: this.graphqlPath, }); serverInfo.subscriptionsUrl = require('url').format({ protocol: 'ws', hostname: hostForUrl, port: serverInfo.port, slashes: true, pathname: subscriptionsPath, }); return serverInfo; } public applyMiddleware() { throw new Error( 'To use Apollo Server with an existing express application, please use apollo-server-express', ); } // Listen takes the same arguments as http.Server.listen. public async listen(...opts: Array<any>): Promise<ServerInfo> { // This class is the easy mode for people who don't create their own express // object, so we have to create it. const app = express(); app.disable('x-powered-by'); // provide generous values for the getting started experience super.applyMiddleware({ app, path: '/', bodyParserConfig: { limit: '50mb' }, onHealthCheck: this.onHealthCheck, cors: typeof this.cors !== 'undefined' ? this.cors : { origin: '*', }, }); const httpServer = http.createServer(app); this.httpServer = httpServer; if (this.subscriptionServerOptions) { this.installSubscriptionHandlers(httpServer); } await new Promise(resolve => { httpServer.once('listening', resolve); // If the user passed a callback to listen, it'll get called in addition // to our resolver. They won't have the ability to get the ServerInfo // object unless they use our Promise, though. httpServer.listen(...(opts.length ? opts : [{ port: 4000 }])); }); return this.createServerInfo(httpServer, this.subscriptionsPath); } public async stop() { if (this.httpServer) { const httpServer = this.httpServer; await new Promise(resolve => httpServer.close(resolve)); this.httpServer = undefined; } await super.stop(); } } apollo-server-demo/node_modules/apollo-server/src/exports.ts 0000644 0001750 0000144 00000000752 03560116604 024071 0 ustar andreh users export * from 'graphql-tools'; export * from 'graphql-subscriptions'; export { gql, GraphQLUpload, GraphQLOptions, GraphQLExtension, Config, GraphQLSchemaModule, // Errors ApolloError, toApolloError, SyntaxError, ValidationError, AuthenticationError, ForbiddenError, UserInputError, // playground defaultPlaygroundOptions, PlaygroundConfig, PlaygroundRenderPageOptions, } from 'apollo-server-core'; export { CorsOptions } from 'apollo-server-express'; apollo-server-demo/node_modules/apollo-server/README.md 0000644 0001750 0000144 00000016103 03560116604 022502 0 ustar andreh users # <a href='https://www.apollographql.com/'><img src='https://user-images.githubusercontent.com/841294/53402609-b97a2180-39ba-11e9-8100-812bab86357c.png' height='100' alt='Apollo Server'></a> ## GraphQL Server for Express, Koa, Hapi, Lambda, and more. [](https://badge.fury.io/js/apollo-server-core) [](https://circleci.com/gh/apollographql/apollo-server/tree/main) [](https://spectrum.chat/apollo) [](https://github.com/apollographql/apollo-server/blob/HEAD/CHANGELOG.md) Apollo Server is a community-maintained open-source GraphQL server. It works with pretty much all Node.js HTTP server frameworks, and we're happy to take PRs to add more! Apollo Server works with any GraphQL schema built with [GraphQL.js](https://github.com/graphql/graphql-js)--or define a schema's type definitions using schema definition language (SDL). [Read the documentation](https://www.apollographql.com/docs/apollo-server/) for information on getting started and many other use cases and [follow the CHANGELOG](https://github.com/apollographql/apollo-server/blob/HEAD/CHANGELOG.md) for updates. ## Principles Apollo Server is built with the following principles in mind: - **By the community, for the community**: Its development is driven by the needs of developers. - **Simplicity**: By keeping things simple, it is more secure and easier to implement and contribute. - **Performance**: It is well-tested and production-ready. Anyone is welcome to contribute to Apollo Server, just read [CONTRIBUTING.md](./CONTRIBUTING.md), take a look at the [roadmap](./ROADMAP.md) and make your first PR! ## Getting started To get started with Apollo Server: * Install with `npm install apollo-server-<integration>` * Write a GraphQL schema * Use one of the following snippets There are two ways to install Apollo Server: * **[Standalone](#installation-standalone)**: For applications that do not require an existing web framework, use the `apollo-server` package. * **[Integrations](#installation-integrations)**: For applications with a web framework (e.g. `express`, `koa`, `hapi`, etc.), use the appropriate Apollo Server integration package. For more info, please refer to the [Apollo Server docs](https://www.apollographql.com/docs/apollo-server/v2). ### Installation: Standalone In a new project, install the `apollo-server` and `graphql` dependencies using: npm install apollo-server graphql Then, create an `index.js` which defines the schema and its functionality (i.e. resolvers): ```js const { ApolloServer, gql } = require('apollo-server'); // The GraphQL schema const typeDefs = gql` type Query { "A simple type for getting started!" hello: String } `; // A map of functions which return data for the schema. const resolvers = { Query: { hello: () => 'world', }, }; const server = new ApolloServer({ typeDefs, resolvers, }); server.listen().then(({ url }) => { console.log(`🚀 Server ready at ${url}`); }); ``` > Due to its human-readability, we recommend using [schema-definition language (SDL)](https://www.apollographql.com/docs/apollo-server/essentials/schema/#schema-definition-language) to define a GraphQL schema--[a `GraphQLSchema` object from `graphql-js`](https://github.com/graphql/graphql-js/#using-graphqljs) can also be specified instead of `typeDefs` and `resolvers` using the `schema` property: > > ```js > const server = new ApolloServer({ > schema: ... > }); > ``` Finally, start the server using `node index.js` and go to the URL returned on the console. For more details, check out the Apollo Server [Getting Started guide](https://www.apollographql.com/docs/apollo-server/getting-started.html) and the [fullstack tutorial](https://www.apollographql.com/docs/tutorial/introduction.html). For questions, the [Apollo community on Spectrum.chat](https://spectrum.chat) is a great place to get help. ## Installation: Integrations While the standalone installation above can be used without making a decision about which web framework to use, the Apollo Server integration packages are paired with specific web frameworks (e.g. Express, Koa, hapi). The following web frameworks have Apollo Server integrations, and each of these linked integrations has its own installation instructions and examples on its package `README.md`: - [Express](https://github.com/apollographql/apollo-server/tree/main/packages/apollo-server-express) _(Most popular)_ - [Koa](https://github.com/apollographql/apollo-server/tree/main/packages/apollo-server-koa) - [Hapi](https://github.com/apollographql/apollo-server/tree/main/packages/apollo-server-hapi) - [Fastify](https://github.com/apollographql/apollo-server/tree/main/packages/apollo-server-fastify) - [Amazon Lambda](https://github.com/apollographql/apollo-server/tree/main/packages/apollo-server-lambda) - [Micro](https://github.com/apollographql/apollo-server/tree/main/packages/apollo-server-micro) - [Azure Functions](https://github.com/apollographql/apollo-server/tree/main/packages/apollo-server-azure-functions) - [Google Cloud Functions](https://github.com/apollographql/apollo-server/tree/main/packages/apollo-server-cloud-functions) - [Cloudflare](https://github.com/apollographql/apollo-server/tree/main/packages/apollo-server-cloudflare) _(Experimental)_ ## Context A request context is available for each request. When `context` is defined as a function, it will be called on each request and will receive an object containing a `req` property, which represents the request itself. By returning an object from the `context` function, it will be available as the third positional parameter of the resolvers: ```js new ApolloServer({ typeDefs, resolvers: { Query: { books: (parent, args, context, info) => { console.log(context.myProperty); // Will be `true`! return books; }, } }, context: async ({ req }) => { return { myProperty: true }; }, }) ``` ## Documentation The [Apollo Server documentation](https://apollographql.com/docs/apollo-server/) contains additional details on how to get started with GraphQL and Apollo Server. The raw Markdown source of the documentation is available within the `docs/` directory of this monorepo--to contribute, please use the _Edit on GitHub_ buttons at the bottom of each page. ## Development If you wish to develop or contribute to Apollo Server, we suggest the following: - Fork this repository - Install the Apollo Server project on your computer ``` git clone https://github.com/[your-user]/apollo-server cd apollo-server npm install cd packages/apollo-server-<integration>/ npm link ``` - Install your local Apollo Server in the other App ``` cd ~/myApp npm link apollo-server-<integration> ``` ## Community Are you stuck? Want to contribute? Come visit us in the [Apollo community on Spectrum.chat!](https://spectrum.chat/apollo) ## Maintainers - [@abernix](https://github.com/abernix) (Apollo) apollo-server-demo/node_modules/apollo-server/package.json 0000644 0001750 0000144 00000002242 03560116604 023510 0 ustar andreh users { "name": "apollo-server", "version": "2.19.2", "description": "Production ready GraphQL Server", "author": "Apollo <opensource@apollographql.com>", "main": "dist/index.js", "types": "dist/index.d.ts", "repository": { "type": "git", "url": "https://github.com/apollographql/apollo-server", "directory": "packages/apollo-server" }, "keywords": [ "GraphQL", "Apollo", "Server", "Javascript" ], "license": "MIT", "bugs": { "url": "https://github.com/apollographql/apollo-server/issues" }, "homepage": "https://github.com/apollographql/apollo-server#readme", "dependencies": { "apollo-server-core": "^2.19.2", "apollo-server-express": "^2.19.2", "express": "^4.0.0", "graphql-subscriptions": "^1.0.0", "graphql-tools": "^4.0.0" }, "peerDependencies": { "graphql": "^0.12.0 || ^0.13.0 || ^14.0.0 || ^15.0.0" }, "gitHead": "c212627be591cd2c2469321b58b15f1b7909778d" ,"_resolved": "https://registry.npmjs.org/apollo-server/-/apollo-server-2.19.2.tgz" ,"_integrity": "sha512-fyl8U2O1haBOvaF3Z4+ZNj2Z9KXtw0Hb13NG2+J7vyHTdDL/hEwX9bp9AnWlfXOYL8s/VeankAUNqw8kggBeZw==" ,"_from": "apollo-server@2.19.2" } apollo-server-demo/node_modules/apollo-server/CHANGELOG.md 0000644 0001750 0000144 00000001167 03560116604 023040 0 ustar andreh users # Changelog ### vNEXT * `apollo-server`: move non-schema related options into listen [PR#1059](https://github.com/apollographql/apollo-server/pull/1059) * `apollo-server`: add `bodyParserConfig` options [PR#1059](https://github.com/apollographql/apollo-server/pull/1059) * `apollo-server`: add `/.well-known/apollo/server-health` endpoint with async callback for additional checks, ie database poke [PR#992](https://github.com/apollographql/apollo-server/pull/992) * `apollo-server`: collocate graphql gui with endpoint and provide gui when accessed from browser [PR#987](https://github.com/apollographql/apollo-server/pull/987) apollo-server-demo/node_modules/type-is/ 0000755 0001750 0000144 00000000000 14067647700 020034 5 ustar andreh users apollo-server-demo/node_modules/type-is/index.js 0000644 0001750 0000144 00000012672 03560116604 021477 0 ustar andreh users /*! * type-is * Copyright(c) 2014 Jonathan Ong * Copyright(c) 2014-2015 Douglas Christopher Wilson * MIT Licensed */ 'use strict' /** * Module dependencies. * @private */ var typer = require('media-typer') var mime = require('mime-types') /** * Module exports. * @public */ module.exports = typeofrequest module.exports.is = typeis module.exports.hasBody = hasbody module.exports.normalize = normalize module.exports.match = mimeMatch /** * Compare a `value` content-type with `types`. * Each `type` can be an extension like `html`, * a special shortcut like `multipart` or `urlencoded`, * or a mime type. * * If no types match, `false` is returned. * Otherwise, the first `type` that matches is returned. * * @param {String} value * @param {Array} types * @public */ function typeis (value, types_) { var i var types = types_ // remove parameters and normalize var val = tryNormalizeType(value) // no type or invalid if (!val) { return false } // support flattened arguments if (types && !Array.isArray(types)) { types = new Array(arguments.length - 1) for (i = 0; i < types.length; i++) { types[i] = arguments[i + 1] } } // no types, return the content type if (!types || !types.length) { return val } var type for (i = 0; i < types.length; i++) { if (mimeMatch(normalize(type = types[i]), val)) { return type[0] === '+' || type.indexOf('*') !== -1 ? val : type } } // no matches return false } /** * Check if a request has a request body. * A request with a body __must__ either have `transfer-encoding` * or `content-length` headers set. * http://www.w3.org/Protocols/rfc2616/rfc2616-sec4.html#sec4.3 * * @param {Object} request * @return {Boolean} * @public */ function hasbody (req) { return req.headers['transfer-encoding'] !== undefined || !isNaN(req.headers['content-length']) } /** * Check if the incoming request contains the "Content-Type" * header field, and it contains any of the give mime `type`s. * If there is no request body, `null` is returned. * If there is no content type, `false` is returned. * Otherwise, it returns the first `type` that matches. * * Examples: * * // With Content-Type: text/html; charset=utf-8 * this.is('html'); // => 'html' * this.is('text/html'); // => 'text/html' * this.is('text/*', 'application/json'); // => 'text/html' * * // When Content-Type is application/json * this.is('json', 'urlencoded'); // => 'json' * this.is('application/json'); // => 'application/json' * this.is('html', 'application/*'); // => 'application/json' * * this.is('html'); // => false * * @param {String|Array} types... * @return {String|false|null} * @public */ function typeofrequest (req, types_) { var types = types_ // no body if (!hasbody(req)) { return null } // support flattened arguments if (arguments.length > 2) { types = new Array(arguments.length - 1) for (var i = 0; i < types.length; i++) { types[i] = arguments[i + 1] } } // request content type var value = req.headers['content-type'] return typeis(value, types) } /** * Normalize a mime type. * If it's a shorthand, expand it to a valid mime type. * * In general, you probably want: * * var type = is(req, ['urlencoded', 'json', 'multipart']); * * Then use the appropriate body parsers. * These three are the most common request body types * and are thus ensured to work. * * @param {String} type * @private */ function normalize (type) { if (typeof type !== 'string') { // invalid type return false } switch (type) { case 'urlencoded': return 'application/x-www-form-urlencoded' case 'multipart': return 'multipart/*' } if (type[0] === '+') { // "+json" -> "*/*+json" expando return '*/*' + type } return type.indexOf('/') === -1 ? mime.lookup(type) : type } /** * Check if `expected` mime type * matches `actual` mime type with * wildcard and +suffix support. * * @param {String} expected * @param {String} actual * @return {Boolean} * @private */ function mimeMatch (expected, actual) { // invalid type if (expected === false) { return false } // split types var actualParts = actual.split('/') var expectedParts = expected.split('/') // invalid format if (actualParts.length !== 2 || expectedParts.length !== 2) { return false } // validate type if (expectedParts[0] !== '*' && expectedParts[0] !== actualParts[0]) { return false } // validate suffix wildcard if (expectedParts[1].substr(0, 2) === '*+') { return expectedParts[1].length <= actualParts[1].length + 1 && expectedParts[1].substr(1) === actualParts[1].substr(1 - expectedParts[1].length) } // validate subtype if (expectedParts[1] !== '*' && expectedParts[1] !== actualParts[1]) { return false } return true } /** * Normalize a type and remove parameters. * * @param {string} value * @return {string} * @private */ function normalizeType (value) { // parse the type var type = typer.parse(value) // remove the parameters type.parameters = undefined // reformat it return typer.format(type) } /** * Try to normalize a type and remove parameters. * * @param {string} value * @return {string} * @private */ function tryNormalizeType (value) { if (!value) { return null } try { return normalizeType(value) } catch (err) { return null } } apollo-server-demo/node_modules/type-is/LICENSE 0000644 0001750 0000144 00000002224 03560116604 021027 0 ustar andreh users (The MIT License) Copyright (c) 2014 Jonathan Ong <me@jongleberry.com> Copyright (c) 2014-2015 Douglas Christopher Wilson <doug@somethingdoug.com> Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the 'Software'), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/type-is/HISTORY.md 0000644 0001750 0000144 00000012507 03560116604 021512 0 ustar andreh users 1.6.18 / 2019-04-26 =================== * Fix regression passing request object to `typeis.is` 1.6.17 / 2019-04-25 =================== * deps: mime-types@~2.1.24 - Add Apple file extensions from IANA - Add extension `.csl` to `application/vnd.citationstyles.style+xml` - Add extension `.es` to `application/ecmascript` - Add extension `.nq` to `application/n-quads` - Add extension `.nt` to `application/n-triples` - Add extension `.owl` to `application/rdf+xml` - Add extensions `.siv` and `.sieve` to `application/sieve` - Add extensions from IANA for `image/*` types - Add extensions from IANA for `model/*` types - Add extensions to HEIC image types - Add new mime types - Add `text/mdx` with extension `.mdx` * perf: prevent internal `throw` on invalid type 1.6.16 / 2018-02-16 =================== * deps: mime-types@~2.1.18 - Add `application/raml+yaml` with extension `.raml` - Add `application/wasm` with extension `.wasm` - Add `text/shex` with extension `.shex` - Add extensions for JPEG-2000 images - Add extensions from IANA for `message/*` types - Add extension `.mjs` to `application/javascript` - Add extension `.wadl` to `application/vnd.sun.wadl+xml` - Add extension `.gz` to `application/gzip` - Add glTF types and extensions - Add new mime types - Update extensions `.md` and `.markdown` to be `text/markdown` - Update font MIME types - Update `text/hjson` to registered `application/hjson` 1.6.15 / 2017-03-31 =================== * deps: mime-types@~2.1.15 - Add new mime types 1.6.14 / 2016-11-18 =================== * deps: mime-types@~2.1.13 - Add new mime types 1.6.13 / 2016-05-18 =================== * deps: mime-types@~2.1.11 - Add new mime types 1.6.12 / 2016-02-28 =================== * deps: mime-types@~2.1.10 - Add new mime types - Fix extension of `application/dash+xml` - Update primary extension for `audio/mp4` 1.6.11 / 2016-01-29 =================== * deps: mime-types@~2.1.9 - Add new mime types 1.6.10 / 2015-12-01 =================== * deps: mime-types@~2.1.8 - Add new mime types 1.6.9 / 2015-09-27 ================== * deps: mime-types@~2.1.7 - Add new mime types 1.6.8 / 2015-09-04 ================== * deps: mime-types@~2.1.6 - Add new mime types 1.6.7 / 2015-08-20 ================== * Fix type error when given invalid type to match against * deps: mime-types@~2.1.5 - Add new mime types 1.6.6 / 2015-07-31 ================== * deps: mime-types@~2.1.4 - Add new mime types 1.6.5 / 2015-07-16 ================== * deps: mime-types@~2.1.3 - Add new mime types 1.6.4 / 2015-07-01 ================== * deps: mime-types@~2.1.2 - Add new mime types * perf: enable strict mode * perf: remove argument reassignment 1.6.3 / 2015-06-08 ================== * deps: mime-types@~2.1.1 - Add new mime types * perf: reduce try block size * perf: remove bitwise operations 1.6.2 / 2015-05-10 ================== * deps: mime-types@~2.0.11 - Add new mime types 1.6.1 / 2015-03-13 ================== * deps: mime-types@~2.0.10 - Add new mime types 1.6.0 / 2015-02-12 ================== * fix false-positives in `hasBody` `Transfer-Encoding` check * support wildcard for both type and subtype (`*/*`) 1.5.7 / 2015-02-09 ================== * fix argument reassignment * deps: mime-types@~2.0.9 - Add new mime types 1.5.6 / 2015-01-29 ================== * deps: mime-types@~2.0.8 - Add new mime types 1.5.5 / 2014-12-30 ================== * deps: mime-types@~2.0.7 - Add new mime types - Fix missing extensions - Fix various invalid MIME type entries - Remove example template MIME types - deps: mime-db@~1.5.0 1.5.4 / 2014-12-10 ================== * deps: mime-types@~2.0.4 - Add new mime types - deps: mime-db@~1.3.0 1.5.3 / 2014-11-09 ================== * deps: mime-types@~2.0.3 - Add new mime types - deps: mime-db@~1.2.0 1.5.2 / 2014-09-28 ================== * deps: mime-types@~2.0.2 - Add new mime types - deps: mime-db@~1.1.0 1.5.1 / 2014-09-07 ================== * Support Node.js 0.6 * deps: media-typer@0.3.0 * deps: mime-types@~2.0.1 - Support Node.js 0.6 1.5.0 / 2014-09-05 ================== * fix `hasbody` to be true for `content-length: 0` 1.4.0 / 2014-09-02 ================== * update mime-types 1.3.2 / 2014-06-24 ================== * use `~` range on mime-types 1.3.1 / 2014-06-19 ================== * fix global variable leak 1.3.0 / 2014-06-19 ================== * improve type parsing - invalid media type never matches - media type not case-sensitive - extra LWS does not affect results 1.2.2 / 2014-06-19 ================== * fix behavior on unknown type argument 1.2.1 / 2014-06-03 ================== * switch dependency from `mime` to `mime-types@1.0.0` 1.2.0 / 2014-05-11 ================== * support suffix matching: - `+json` matches `application/vnd+json` - `*/vnd+json` matches `application/vnd+json` - `application/*+json` matches `application/vnd+json` 1.1.0 / 2014-04-12 ================== * add non-array values support * expose internal utilities: - `.is()` - `.hasBody()` - `.normalize()` - `.match()` 1.0.1 / 2014-03-30 ================== * add `multipart` as a shorthand apollo-server-demo/node_modules/type-is/README.md 0000644 0001750 0000144 00000012077 03560116604 021310 0 ustar andreh users # type-is [![NPM Version][npm-version-image]][npm-url] [![NPM Downloads][npm-downloads-image]][npm-url] [![Node.js Version][node-version-image]][node-version-url] [![Build Status][travis-image]][travis-url] [![Test Coverage][coveralls-image]][coveralls-url] Infer the content-type of a request. ### Install This is a [Node.js](https://nodejs.org/en/) module available through the [npm registry](https://www.npmjs.com/). Installation is done using the [`npm install` command](https://docs.npmjs.com/getting-started/installing-npm-packages-locally): ```sh $ npm install type-is ``` ## API ```js var http = require('http') var typeis = require('type-is') http.createServer(function (req, res) { var istext = typeis(req, ['text/*']) res.end('you ' + (istext ? 'sent' : 'did not send') + ' me text') }) ``` ### typeis(request, types) Checks if the `request` is one of the `types`. If the request has no body, even if there is a `Content-Type` header, then `null` is returned. If the `Content-Type` header is invalid or does not matches any of the `types`, then `false` is returned. Otherwise, a string of the type that matched is returned. The `request` argument is expected to be a Node.js HTTP request. The `types` argument is an array of type strings. Each type in the `types` array can be one of the following: - A file extension name such as `json`. This name will be returned if matched. - A mime type such as `application/json`. - A mime type with a wildcard such as `*/*` or `*/json` or `application/*`. The full mime type will be returned if matched. - A suffix such as `+json`. This can be combined with a wildcard such as `*/vnd+json` or `application/*+json`. The full mime type will be returned if matched. Some examples to illustrate the inputs and returned value: <!-- eslint-disable no-undef --> ```js // req.headers.content-type = 'application/json' typeis(req, ['json']) // => 'json' typeis(req, ['html', 'json']) // => 'json' typeis(req, ['application/*']) // => 'application/json' typeis(req, ['application/json']) // => 'application/json' typeis(req, ['html']) // => false ``` ### typeis.hasBody(request) Returns a Boolean if the given `request` has a body, regardless of the `Content-Type` header. Having a body has no relation to how large the body is (it may be 0 bytes). This is similar to how file existence works. If a body does exist, then this indicates that there is data to read from the Node.js request stream. <!-- eslint-disable no-undef --> ```js if (typeis.hasBody(req)) { // read the body, since there is one req.on('data', function (chunk) { // ... }) } ``` ### typeis.is(mediaType, types) Checks if the `mediaType` is one of the `types`. If the `mediaType` is invalid or does not matches any of the `types`, then `false` is returned. Otherwise, a string of the type that matched is returned. The `mediaType` argument is expected to be a [media type](https://tools.ietf.org/html/rfc6838) string. The `types` argument is an array of type strings. Each type in the `types` array can be one of the following: - A file extension name such as `json`. This name will be returned if matched. - A mime type such as `application/json`. - A mime type with a wildcard such as `*/*` or `*/json` or `application/*`. The full mime type will be returned if matched. - A suffix such as `+json`. This can be combined with a wildcard such as `*/vnd+json` or `application/*+json`. The full mime type will be returned if matched. Some examples to illustrate the inputs and returned value: <!-- eslint-disable no-undef --> ```js var mediaType = 'application/json' typeis.is(mediaType, ['json']) // => 'json' typeis.is(mediaType, ['html', 'json']) // => 'json' typeis.is(mediaType, ['application/*']) // => 'application/json' typeis.is(mediaType, ['application/json']) // => 'application/json' typeis.is(mediaType, ['html']) // => false ``` ## Examples ### Example body parser ```js var express = require('express') var typeis = require('type-is') var app = express() app.use(function bodyParser (req, res, next) { if (!typeis.hasBody(req)) { return next() } switch (typeis(req, ['urlencoded', 'json', 'multipart'])) { case 'urlencoded': // parse urlencoded body throw new Error('implement urlencoded body parsing') case 'json': // parse json body throw new Error('implement json body parsing') case 'multipart': // parse multipart body throw new Error('implement multipart body parsing') default: // 415 error code res.statusCode = 415 res.end() break } }) ``` ## License [MIT](LICENSE) [coveralls-image]: https://badgen.net/coveralls/c/github/jshttp/type-is/master [coveralls-url]: https://coveralls.io/r/jshttp/type-is?branch=master [node-version-image]: https://badgen.net/npm/node/type-is [node-version-url]: https://nodejs.org/en/download [npm-downloads-image]: https://badgen.net/npm/dm/type-is [npm-url]: https://npmjs.org/package/type-is [npm-version-image]: https://badgen.net/npm/v/type-is [travis-image]: https://badgen.net/travis/jshttp/type-is/master [travis-url]: https://travis-ci.org/jshttp/type-is apollo-server-demo/node_modules/type-is/package.json 0000644 0001750 0000144 00000002501 03560116604 022306 0 ustar andreh users { "name": "type-is", "description": "Infer the content-type of a request.", "version": "1.6.18", "contributors": [ "Douglas Christopher Wilson <doug@somethingdoug.com>", "Jonathan Ong <me@jongleberry.com> (http://jongleberry.com)" ], "license": "MIT", "repository": "jshttp/type-is", "dependencies": { "media-typer": "0.3.0", "mime-types": "~2.1.24" }, "devDependencies": { "eslint": "5.16.0", "eslint-config-standard": "12.0.0", "eslint-plugin-import": "2.17.2", "eslint-plugin-markdown": "1.0.0", "eslint-plugin-node": "8.0.1", "eslint-plugin-promise": "4.1.1", "eslint-plugin-standard": "4.0.0", "mocha": "6.1.4", "nyc": "14.0.0" }, "engines": { "node": ">= 0.6" }, "files": [ "LICENSE", "HISTORY.md", "index.js" ], "scripts": { "lint": "eslint --plugin markdown --ext js,md .", "test": "mocha --reporter spec --check-leaks --bail test/", "test-cov": "nyc --reporter=html --reporter=text npm test", "test-travis": "nyc --reporter=text npm test" }, "keywords": [ "content", "type", "checking" ] ,"_resolved": "https://registry.npmjs.org/type-is/-/type-is-1.6.18.tgz" ,"_integrity": "sha512-TkRKr9sUTxEH8MdfuCSP7VizJyzRNMjj2J2do2Jr3Kym598JVdEksuzPQCnlFPW4ky9Q+iA+ma9BGm06XQBy8g==" ,"_from": "type-is@1.6.18" } apollo-server-demo/node_modules/encodeurl/ 0000755 0001750 0000144 00000000000 14067647700 020422 5 ustar andreh users apollo-server-demo/node_modules/encodeurl/index.js 0000644 0001750 0000144 00000003062 13231252251 022051 0 ustar andreh users /*! * encodeurl * Copyright(c) 2016 Douglas Christopher Wilson * MIT Licensed */ 'use strict' /** * Module exports. * @public */ module.exports = encodeUrl /** * RegExp to match non-URL code points, *after* encoding (i.e. not including "%") * and including invalid escape sequences. * @private */ var ENCODE_CHARS_REGEXP = /(?:[^\x21\x25\x26-\x3B\x3D\x3F-\x5B\x5D\x5F\x61-\x7A\x7E]|%(?:[^0-9A-Fa-f]|[0-9A-Fa-f][^0-9A-Fa-f]|$))+/g /** * RegExp to match unmatched surrogate pair. * @private */ var UNMATCHED_SURROGATE_PAIR_REGEXP = /(^|[^\uD800-\uDBFF])[\uDC00-\uDFFF]|[\uD800-\uDBFF]([^\uDC00-\uDFFF]|$)/g /** * String to replace unmatched surrogate pair with. * @private */ var UNMATCHED_SURROGATE_PAIR_REPLACE = '$1\uFFFD$2' /** * Encode a URL to a percent-encoded form, excluding already-encoded sequences. * * This function will take an already-encoded URL and encode all the non-URL * code points. This function will not encode the "%" character unless it is * not part of a valid sequence (`%20` will be left as-is, but `%foo` will * be encoded as `%25foo`). * * This encode is meant to be "safe" and does not throw errors. It will try as * hard as it can to properly encode the given URL, including replacing any raw, * unpaired surrogate pairs with the Unicode replacement character prior to * encoding. * * @param {string} url * @return {string} * @public */ function encodeUrl (url) { return String(url) .replace(UNMATCHED_SURROGATE_PAIR_REGEXP, UNMATCHED_SURROGATE_PAIR_REPLACE) .replace(ENCODE_CHARS_REGEXP, encodeURI) } apollo-server-demo/node_modules/encodeurl/LICENSE 0000644 0001750 0000144 00000002101 13231247563 021414 0 ustar andreh users (The MIT License) Copyright (c) 2016 Douglas Christopher Wilson Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the 'Software'), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/encodeurl/HISTORY.md 0000644 0001750 0000144 00000000356 13231253165 022077 0 ustar andreh users 1.0.2 / 2018-01-21 ================== * Fix encoding `%` as last character 1.0.1 / 2016-06-09 ================== * Fix encoding unpaired surrogates at start/end of string 1.0.0 / 2016-06-08 ================== * Initial release apollo-server-demo/node_modules/encodeurl/README.md 0000644 0001750 0000144 00000007417 13231247563 021705 0 ustar andreh users # encodeurl [![NPM Version][npm-image]][npm-url] [![NPM Downloads][downloads-image]][downloads-url] [![Node.js Version][node-version-image]][node-version-url] [![Build Status][travis-image]][travis-url] [![Test Coverage][coveralls-image]][coveralls-url] Encode a URL to a percent-encoded form, excluding already-encoded sequences ## Installation This is a [Node.js](https://nodejs.org/en/) module available through the [npm registry](https://www.npmjs.com/). Installation is done using the [`npm install` command](https://docs.npmjs.com/getting-started/installing-npm-packages-locally): ```sh $ npm install encodeurl ``` ## API ```js var encodeUrl = require('encodeurl') ``` ### encodeUrl(url) Encode a URL to a percent-encoded form, excluding already-encoded sequences. This function will take an already-encoded URL and encode all the non-URL code points (as UTF-8 byte sequences). This function will not encode the "%" character unless it is not part of a valid sequence (`%20` will be left as-is, but `%foo` will be encoded as `%25foo`). This encode is meant to be "safe" and does not throw errors. It will try as hard as it can to properly encode the given URL, including replacing any raw, unpaired surrogate pairs with the Unicode replacement character prior to encoding. This function is _similar_ to the intrinsic function `encodeURI`, except it will not encode the `%` character if that is part of a valid sequence, will not encode `[` and `]` (for IPv6 hostnames) and will replace raw, unpaired surrogate pairs with the Unicode replacement character (instead of throwing). ## Examples ### Encode a URL containing user-controled data ```js var encodeUrl = require('encodeurl') var escapeHtml = require('escape-html') http.createServer(function onRequest (req, res) { // get encoded form of inbound url var url = encodeUrl(req.url) // create html message var body = '<p>Location ' + escapeHtml(url) + ' not found</p>' // send a 404 res.statusCode = 404 res.setHeader('Content-Type', 'text/html; charset=UTF-8') res.setHeader('Content-Length', String(Buffer.byteLength(body, 'utf-8'))) res.end(body, 'utf-8') }) ``` ### Encode a URL for use in a header field ```js var encodeUrl = require('encodeurl') var escapeHtml = require('escape-html') var url = require('url') http.createServer(function onRequest (req, res) { // parse inbound url var href = url.parse(req) // set new host for redirect href.host = 'localhost' href.protocol = 'https:' href.slashes = true // create location header var location = encodeUrl(url.format(href)) // create html message var body = '<p>Redirecting to new site: ' + escapeHtml(location) + '</p>' // send a 301 res.statusCode = 301 res.setHeader('Content-Type', 'text/html; charset=UTF-8') res.setHeader('Content-Length', String(Buffer.byteLength(body, 'utf-8'))) res.setHeader('Location', location) res.end(body, 'utf-8') }) ``` ## Testing ```sh $ npm test $ npm run lint ``` ## References - [RFC 3986: Uniform Resource Identifier (URI): Generic Syntax][rfc-3986] - [WHATWG URL Living Standard][whatwg-url] [rfc-3986]: https://tools.ietf.org/html/rfc3986 [whatwg-url]: https://url.spec.whatwg.org/ ## License [MIT](LICENSE) [npm-image]: https://img.shields.io/npm/v/encodeurl.svg [npm-url]: https://npmjs.org/package/encodeurl [node-version-image]: https://img.shields.io/node/v/encodeurl.svg [node-version-url]: https://nodejs.org/en/download [travis-image]: https://img.shields.io/travis/pillarjs/encodeurl.svg [travis-url]: https://travis-ci.org/pillarjs/encodeurl [coveralls-image]: https://img.shields.io/coveralls/pillarjs/encodeurl.svg [coveralls-url]: https://coveralls.io/r/pillarjs/encodeurl?branch=master [downloads-image]: https://img.shields.io/npm/dm/encodeurl.svg [downloads-url]: https://npmjs.org/package/encodeurl apollo-server-demo/node_modules/encodeurl/package.json 0000644 0001750 0000144 00000002335 13231253150 022673 0 ustar andreh users { "name": "encodeurl", "description": "Encode a URL to a percent-encoded form, excluding already-encoded sequences", "version": "1.0.2", "contributors": [ "Douglas Christopher Wilson <doug@somethingdoug.com>" ], "license": "MIT", "keywords": [ "encode", "encodeurl", "url" ], "repository": "pillarjs/encodeurl", "devDependencies": { "eslint": "3.19.0", "eslint-config-standard": "10.2.1", "eslint-plugin-import": "2.8.0", "eslint-plugin-node": "5.2.1", "eslint-plugin-promise": "3.6.0", "eslint-plugin-standard": "3.0.1", "istanbul": "0.4.5", "mocha": "2.5.3" }, "files": [ "LICENSE", "HISTORY.md", "README.md", "index.js" ], "engines": { "node": ">= 0.8" }, "scripts": { "lint": "eslint .", "test": "mocha --reporter spec --bail --check-leaks test/", "test-cov": "istanbul cover node_modules/mocha/bin/_mocha -- --reporter dot --check-leaks test/", "test-travis": "istanbul cover node_modules/mocha/bin/_mocha --report lcovonly -- --reporter spec --check-leaks test/" } ,"_resolved": "https://registry.npmjs.org/encodeurl/-/encodeurl-1.0.2.tgz" ,"_integrity": "sha1-rT/0yG7C0CkyL1oCw6mmBslbP1k=" ,"_from": "encodeurl@1.0.2" } apollo-server-demo/node_modules/object-inspect/ 0000755 0001750 0000144 00000000000 14067647701 021354 5 ustar andreh users apollo-server-demo/node_modules/object-inspect/index.js 0000644 0001750 0000144 00000031463 03560116604 023015 0 ustar andreh users var hasMap = typeof Map === 'function' && Map.prototype; var mapSizeDescriptor = Object.getOwnPropertyDescriptor && hasMap ? Object.getOwnPropertyDescriptor(Map.prototype, 'size') : null; var mapSize = hasMap && mapSizeDescriptor && typeof mapSizeDescriptor.get === 'function' ? mapSizeDescriptor.get : null; var mapForEach = hasMap && Map.prototype.forEach; var hasSet = typeof Set === 'function' && Set.prototype; var setSizeDescriptor = Object.getOwnPropertyDescriptor && hasSet ? Object.getOwnPropertyDescriptor(Set.prototype, 'size') : null; var setSize = hasSet && setSizeDescriptor && typeof setSizeDescriptor.get === 'function' ? setSizeDescriptor.get : null; var setForEach = hasSet && Set.prototype.forEach; var hasWeakMap = typeof WeakMap === 'function' && WeakMap.prototype; var weakMapHas = hasWeakMap ? WeakMap.prototype.has : null; var hasWeakSet = typeof WeakSet === 'function' && WeakSet.prototype; var weakSetHas = hasWeakSet ? WeakSet.prototype.has : null; var booleanValueOf = Boolean.prototype.valueOf; var objectToString = Object.prototype.toString; var functionToString = Function.prototype.toString; var match = String.prototype.match; var bigIntValueOf = typeof BigInt === 'function' ? BigInt.prototype.valueOf : null; var gOPS = Object.getOwnPropertySymbols; var symToString = typeof Symbol === 'function' ? Symbol.prototype.toString : null; var isEnumerable = Object.prototype.propertyIsEnumerable; var inspectCustom = require('./util.inspect').custom; var inspectSymbol = inspectCustom && isSymbol(inspectCustom) ? inspectCustom : null; module.exports = function inspect_(obj, options, depth, seen) { var opts = options || {}; if (has(opts, 'quoteStyle') && (opts.quoteStyle !== 'single' && opts.quoteStyle !== 'double')) { throw new TypeError('option "quoteStyle" must be "single" or "double"'); } if ( has(opts, 'maxStringLength') && (typeof opts.maxStringLength === 'number' ? opts.maxStringLength < 0 && opts.maxStringLength !== Infinity : opts.maxStringLength !== null ) ) { throw new TypeError('option "maxStringLength", if provided, must be a positive integer, Infinity, or `null`'); } var customInspect = has(opts, 'customInspect') ? opts.customInspect : true; if (typeof customInspect !== 'boolean') { throw new TypeError('option "customInspect", if provided, must be `true` or `false`'); } if ( has(opts, 'indent') && opts.indent !== null && opts.indent !== '\t' && !(parseInt(opts.indent, 10) === opts.indent && opts.indent > 0) ) { throw new TypeError('options "indent" must be "\\t", an integer > 0, or `null`'); } if (typeof obj === 'undefined') { return 'undefined'; } if (obj === null) { return 'null'; } if (typeof obj === 'boolean') { return obj ? 'true' : 'false'; } if (typeof obj === 'string') { return inspectString(obj, opts); } if (typeof obj === 'number') { if (obj === 0) { return Infinity / obj > 0 ? '0' : '-0'; } return String(obj); } if (typeof obj === 'bigint') { return String(obj) + 'n'; } var maxDepth = typeof opts.depth === 'undefined' ? 5 : opts.depth; if (typeof depth === 'undefined') { depth = 0; } if (depth >= maxDepth && maxDepth > 0 && typeof obj === 'object') { return isArray(obj) ? '[Array]' : '[Object]'; } var indent = getIndent(opts, depth); if (typeof seen === 'undefined') { seen = []; } else if (indexOf(seen, obj) >= 0) { return '[Circular]'; } function inspect(value, from, noIndent) { if (from) { seen = seen.slice(); seen.push(from); } if (noIndent) { var newOpts = { depth: opts.depth }; if (has(opts, 'quoteStyle')) { newOpts.quoteStyle = opts.quoteStyle; } return inspect_(value, newOpts, depth + 1, seen); } return inspect_(value, opts, depth + 1, seen); } if (typeof obj === 'function') { var name = nameOf(obj); var keys = arrObjKeys(obj, inspect); return '[Function' + (name ? ': ' + name : ' (anonymous)') + ']' + (keys.length > 0 ? ' { ' + keys.join(', ') + ' }' : ''); } if (isSymbol(obj)) { var symString = symToString.call(obj); return typeof obj === 'object' ? markBoxed(symString) : symString; } if (isElement(obj)) { var s = '<' + String(obj.nodeName).toLowerCase(); var attrs = obj.attributes || []; for (var i = 0; i < attrs.length; i++) { s += ' ' + attrs[i].name + '=' + wrapQuotes(quote(attrs[i].value), 'double', opts); } s += '>'; if (obj.childNodes && obj.childNodes.length) { s += '...'; } s += '</' + String(obj.nodeName).toLowerCase() + '>'; return s; } if (isArray(obj)) { if (obj.length === 0) { return '[]'; } var xs = arrObjKeys(obj, inspect); if (indent && !singleLineValues(xs)) { return '[' + indentedJoin(xs, indent) + ']'; } return '[ ' + xs.join(', ') + ' ]'; } if (isError(obj)) { var parts = arrObjKeys(obj, inspect); if (parts.length === 0) { return '[' + String(obj) + ']'; } return '{ [' + String(obj) + '] ' + parts.join(', ') + ' }'; } if (typeof obj === 'object' && customInspect) { if (inspectSymbol && typeof obj[inspectSymbol] === 'function') { return obj[inspectSymbol](); } else if (typeof obj.inspect === 'function') { return obj.inspect(); } } if (isMap(obj)) { var mapParts = []; mapForEach.call(obj, function (value, key) { mapParts.push(inspect(key, obj, true) + ' => ' + inspect(value, obj)); }); return collectionOf('Map', mapSize.call(obj), mapParts, indent); } if (isSet(obj)) { var setParts = []; setForEach.call(obj, function (value) { setParts.push(inspect(value, obj)); }); return collectionOf('Set', setSize.call(obj), setParts, indent); } if (isWeakMap(obj)) { return weakCollectionOf('WeakMap'); } if (isWeakSet(obj)) { return weakCollectionOf('WeakSet'); } if (isNumber(obj)) { return markBoxed(inspect(Number(obj))); } if (isBigInt(obj)) { return markBoxed(inspect(bigIntValueOf.call(obj))); } if (isBoolean(obj)) { return markBoxed(booleanValueOf.call(obj)); } if (isString(obj)) { return markBoxed(inspect(String(obj))); } if (!isDate(obj) && !isRegExp(obj)) { var ys = arrObjKeys(obj, inspect); if (ys.length === 0) { return '{}'; } if (indent) { return '{' + indentedJoin(ys, indent) + '}'; } return '{ ' + ys.join(', ') + ' }'; } return String(obj); }; function wrapQuotes(s, defaultStyle, opts) { var quoteChar = (opts.quoteStyle || defaultStyle) === 'double' ? '"' : "'"; return quoteChar + s + quoteChar; } function quote(s) { return String(s).replace(/"/g, '"'); } function isArray(obj) { return toStr(obj) === '[object Array]'; } function isDate(obj) { return toStr(obj) === '[object Date]'; } function isRegExp(obj) { return toStr(obj) === '[object RegExp]'; } function isError(obj) { return toStr(obj) === '[object Error]'; } function isSymbol(obj) { return toStr(obj) === '[object Symbol]'; } function isString(obj) { return toStr(obj) === '[object String]'; } function isNumber(obj) { return toStr(obj) === '[object Number]'; } function isBigInt(obj) { return toStr(obj) === '[object BigInt]'; } function isBoolean(obj) { return toStr(obj) === '[object Boolean]'; } var hasOwn = Object.prototype.hasOwnProperty || function (key) { return key in this; }; function has(obj, key) { return hasOwn.call(obj, key); } function toStr(obj) { return objectToString.call(obj); } function nameOf(f) { if (f.name) { return f.name; } var m = match.call(functionToString.call(f), /^function\s*([\w$]+)/); if (m) { return m[1]; } return null; } function indexOf(xs, x) { if (xs.indexOf) { return xs.indexOf(x); } for (var i = 0, l = xs.length; i < l; i++) { if (xs[i] === x) { return i; } } return -1; } function isMap(x) { if (!mapSize || !x || typeof x !== 'object') { return false; } try { mapSize.call(x); try { setSize.call(x); } catch (s) { return true; } return x instanceof Map; // core-js workaround, pre-v2.5.0 } catch (e) {} return false; } function isWeakMap(x) { if (!weakMapHas || !x || typeof x !== 'object') { return false; } try { weakMapHas.call(x, weakMapHas); try { weakSetHas.call(x, weakSetHas); } catch (s) { return true; } return x instanceof WeakMap; // core-js workaround, pre-v2.5.0 } catch (e) {} return false; } function isSet(x) { if (!setSize || !x || typeof x !== 'object') { return false; } try { setSize.call(x); try { mapSize.call(x); } catch (m) { return true; } return x instanceof Set; // core-js workaround, pre-v2.5.0 } catch (e) {} return false; } function isWeakSet(x) { if (!weakSetHas || !x || typeof x !== 'object') { return false; } try { weakSetHas.call(x, weakSetHas); try { weakMapHas.call(x, weakMapHas); } catch (s) { return true; } return x instanceof WeakSet; // core-js workaround, pre-v2.5.0 } catch (e) {} return false; } function isElement(x) { if (!x || typeof x !== 'object') { return false; } if (typeof HTMLElement !== 'undefined' && x instanceof HTMLElement) { return true; } return typeof x.nodeName === 'string' && typeof x.getAttribute === 'function'; } function inspectString(str, opts) { if (str.length > opts.maxStringLength) { var remaining = str.length - opts.maxStringLength; var trailer = '... ' + remaining + ' more character' + (remaining > 1 ? 's' : ''); return inspectString(str.slice(0, opts.maxStringLength), opts) + trailer; } // eslint-disable-next-line no-control-regex var s = str.replace(/(['\\])/g, '\\$1').replace(/[\x00-\x1f]/g, lowbyte); return wrapQuotes(s, 'single', opts); } function lowbyte(c) { var n = c.charCodeAt(0); var x = { 8: 'b', 9: 't', 10: 'n', 12: 'f', 13: 'r' }[n]; if (x) { return '\\' + x; } return '\\x' + (n < 0x10 ? '0' : '') + n.toString(16).toUpperCase(); } function markBoxed(str) { return 'Object(' + str + ')'; } function weakCollectionOf(type) { return type + ' { ? }'; } function collectionOf(type, size, entries, indent) { var joinedEntries = indent ? indentedJoin(entries, indent) : entries.join(', '); return type + ' (' + size + ') {' + joinedEntries + '}'; } function singleLineValues(xs) { for (var i = 0; i < xs.length; i++) { if (indexOf(xs[i], '\n') >= 0) { return false; } } return true; } function getIndent(opts, depth) { var baseIndent; if (opts.indent === '\t') { baseIndent = '\t'; } else if (typeof opts.indent === 'number' && opts.indent > 0) { baseIndent = Array(opts.indent + 1).join(' '); } else { return null; } return { base: baseIndent, prev: Array(depth + 1).join(baseIndent) }; } function indentedJoin(xs, indent) { if (xs.length === 0) { return ''; } var lineJoiner = '\n' + indent.prev + indent.base; return lineJoiner + xs.join(',' + lineJoiner) + '\n' + indent.prev; } function arrObjKeys(obj, inspect) { var isArr = isArray(obj); var xs = []; if (isArr) { xs.length = obj.length; for (var i = 0; i < obj.length; i++) { xs[i] = has(obj, i) ? inspect(obj[i], obj) : ''; } } for (var key in obj) { // eslint-disable-line no-restricted-syntax if (!has(obj, key)) { continue; } // eslint-disable-line no-restricted-syntax, no-continue if (isArr && String(Number(key)) === key && key < obj.length) { continue; } // eslint-disable-line no-restricted-syntax, no-continue if ((/[^\w$]/).test(key)) { xs.push(inspect(key, obj) + ': ' + inspect(obj[key], obj)); } else { xs.push(key + ': ' + inspect(obj[key], obj)); } } if (typeof gOPS === 'function') { var syms = gOPS(obj); for (var j = 0; j < syms.length; j++) { if (isEnumerable.call(obj, syms[j])) { xs.push('[' + inspect(syms[j]) + ']: ' + inspect(obj[syms[j]], obj)); } } } return xs; } apollo-server-demo/node_modules/object-inspect/LICENSE 0000644 0001750 0000144 00000002057 03560116604 022352 0 ustar andreh users MIT License Copyright (c) 2013 James Halliday Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/object-inspect/.eslintrc 0000644 0001750 0000144 00000003124 03560116604 023165 0 ustar andreh users { "root": true, "extends": "@ljharb", "rules": { "complexity": 0, "func-style": [2, "declaration"], "indent": [2, 4], "max-lines": 1, "max-lines-per-function": 1, "max-params": [2, 4], "max-statements": [2, 100], "max-statements-per-line": [2, { "max": 2 }], "no-magic-numbers": 0, "no-param-reassign": 1, "operator-linebreak": [2, "before"], "strict": 0, // TODO }, "globals": { "BigInt": false, "WeakSet": false, "WeakMap": false, }, "overrides": [ { "files": ["test/**", "test-*", "example/**"], "rules": { "array-bracket-newline": 0, "id-length": 0, "max-params": 0, "max-statements": 0, "max-statements-per-line": 0, "object-curly-newline": 0, "sort-keys": 0, }, }, { "files": ["example/**"], "rules": { "no-console": 0, }, }, { "files": ["test/browser/**"], "env": { "browser": true, }, }, { "files": ["test/bigint*"], "rules": { "new-cap": [2, { "capIsNewExceptions": ["BigInt"] }], }, }, { "files": "index.js", "globals": { "HTMLElement": false, }, "rules": { "no-use-before-define": 1, }, }, ], } apollo-server-demo/node_modules/object-inspect/.github/ 0000755 0001750 0000144 00000000000 14067647701 022714 5 ustar andreh users apollo-server-demo/node_modules/object-inspect/.github/workflows/ 0000755 0001750 0000144 00000000000 14067647701 024751 5 ustar andreh users apollo-server-demo/node_modules/object-inspect/.github/workflows/node-zero.yml 0000644 0001750 0000144 00000003204 03560116604 027362 0 ustar andreh users name: 'Tests: node.js (0.x)' on: [pull_request, push] jobs: matrix: runs-on: ubuntu-latest outputs: stable: ${{ steps.set-matrix.outputs.requireds }} unstable: ${{ steps.set-matrix.outputs.optionals }} steps: - uses: ljharb/actions/node/matrix@main id: set-matrix with: preset: '0.x' stable: needs: [matrix] name: 'stable minors' runs-on: ubuntu-latest strategy: matrix: ${{ fromJson(needs.matrix.outputs.stable) }} steps: - uses: actions/checkout@v2 - uses: ljharb/actions/node/run@main name: 'npm install && npm run tests-only' with: node-version: ${{ matrix.node-version }} command: 'tests-only' cache-node-modules-key: node_modules-${{ github.workflow }}-${{ github.action }}-${{ github.run_id }} skip-ls-check: true unstable: needs: [matrix, stable] name: 'unstable minors' continue-on-error: true if: ${{ !github.head_ref || !startsWith(github.head_ref, 'renovate') }} runs-on: ubuntu-latest strategy: matrix: ${{ fromJson(needs.matrix.outputs.unstable) }} steps: - uses: actions/checkout@v2 - uses: ljharb/actions/node/run@main name: 'npm install && npm run tests-only' with: node-version: ${{ matrix.node-version }} command: 'tests-only' cache-node-modules-key: node_modules-${{ github.workflow }}-${{ github.action }}-${{ github.run_id }} skip-ls-check: true node: name: 'node 0.x' needs: [stable, unstable] runs-on: ubuntu-latest steps: - run: 'echo tests completed' apollo-server-demo/node_modules/object-inspect/.github/workflows/require-allow-edits.yml 0000644 0001750 0000144 00000000301 03560116604 031351 0 ustar andreh users name: Require “Allow Edits†on: [pull_request_target] jobs: _: name: "Require “Allow Editsâ€" runs-on: ubuntu-latest steps: - uses: ljharb/require-allow-edits@main apollo-server-demo/node_modules/object-inspect/.github/workflows/rebase.yml 0000644 0001750 0000144 00000000401 03560116604 026715 0 ustar andreh users name: Automatic Rebase on: [pull_request_target] jobs: _: name: "Automatic Rebase" runs-on: ubuntu-latest steps: - uses: actions/checkout@v2 - uses: ljharb/rebase@master env: GITHUB_TOKEN: ${{ secrets.GITHUB_TOKEN }} apollo-server-demo/node_modules/object-inspect/.github/workflows/node-4+.yml 0000644 0001750 0000144 00000002532 03560116604 026624 0 ustar andreh users name: 'Tests: node.js' on: [pull_request, push] jobs: matrix: runs-on: ubuntu-latest outputs: latest: ${{ steps.set-matrix.outputs.requireds }} minors: ${{ steps.set-matrix.outputs.optionals }} steps: - uses: ljharb/actions/node/matrix@main id: set-matrix with: preset: '>=4' latest: needs: [matrix] name: 'latest minors' runs-on: ubuntu-latest strategy: matrix: ${{ fromJson(needs.matrix.outputs.latest) }} steps: - uses: actions/checkout@v2 - uses: ljharb/actions/node/run@main name: 'npm install && npm run tests-only' with: node-version: ${{ matrix.node-version }} command: 'tests-only' minors: needs: [matrix, latest] name: 'non-latest minors' continue-on-error: true if: ${{ !github.head_ref || !startsWith(github.head_ref, 'renovate') }} runs-on: ubuntu-latest strategy: matrix: ${{ fromJson(needs.matrix.outputs.minors) }} steps: - uses: actions/checkout@v2 - uses: ljharb/actions/node/run@main name: 'npm install && npm run tests-only' with: node-version: ${{ matrix.node-version }} command: 'tests-only' node: name: 'node 4+' needs: [latest, minors] runs-on: ubuntu-latest steps: - run: 'echo tests completed' apollo-server-demo/node_modules/object-inspect/.github/workflows/node-iojs.yml 0000644 0001750 0000144 00000002636 03560116604 027357 0 ustar andreh users name: 'Tests: node.js (io.js)' on: [pull_request, push] jobs: matrix: runs-on: ubuntu-latest outputs: latest: ${{ steps.set-matrix.outputs.requireds }} minors: ${{ steps.set-matrix.outputs.optionals }} steps: - uses: ljharb/actions/node/matrix@main id: set-matrix with: preset: 'iojs' latest: needs: [matrix] name: 'latest minors' runs-on: ubuntu-latest strategy: matrix: ${{ fromJson(needs.matrix.outputs.latest) }} steps: - uses: actions/checkout@v2 - uses: ljharb/actions/node/run@main name: 'npm install && npm run tests-only' with: node-version: ${{ matrix.node-version }} command: 'tests-only' skip-ls-check: true minors: needs: [matrix, latest] name: 'non-latest minors' continue-on-error: true if: ${{ !github.head_ref || !startsWith(github.head_ref, 'renovate') }} runs-on: ubuntu-latest strategy: matrix: ${{ fromJson(needs.matrix.outputs.minors) }} steps: - uses: actions/checkout@v2 - uses: ljharb/actions/node/run@main name: 'npm install && npm run tests-only' with: node-version: ${{ matrix.node-version }} command: 'tests-only' skip-ls-check: true node: name: 'io.js' needs: [latest, minors] runs-on: ubuntu-latest steps: - run: 'echo tests completed' apollo-server-demo/node_modules/object-inspect/.github/workflows/node-pretest.yml 0000644 0001750 0000144 00000001067 03560116604 030076 0 ustar andreh users name: 'Tests: pretest/posttest' on: [pull_request, push] jobs: pretest: runs-on: ubuntu-latest steps: - uses: actions/checkout@v2 - uses: ljharb/actions/node/run@main name: 'npm install && npm run pretest' with: node-version: 'lts/*' command: 'pretest' posttest: runs-on: ubuntu-latest steps: - uses: actions/checkout@v2 - uses: ljharb/actions/node/run@main name: 'npm install && npm run posttest' with: node-version: 'lts/*' command: 'posttest' apollo-server-demo/node_modules/object-inspect/test-core-js.js 0000644 0001750 0000144 00000001026 03560116604 024215 0 ustar andreh users 'use strict'; require('core-js'); var inspect = require('./'); var test = require('tape'); test('Maps', function (t) { t.equal(inspect(new Map([[1, 2]])), 'Map (1) {1 => 2}'); t.end(); }); test('WeakMaps', function (t) { t.equal(inspect(new WeakMap([[{}, 2]])), 'WeakMap { ? }'); t.end(); }); test('Sets', function (t) { t.equal(inspect(new Set([[1, 2]])), 'Set (1) {[ 1, 2 ]}'); t.end(); }); test('WeakSets', function (t) { t.equal(inspect(new WeakSet([[1, 2]])), 'WeakSet { ? }'); t.end(); }); apollo-server-demo/node_modules/object-inspect/.editorconfig 0000644 0001750 0000144 00000000436 03560116604 024021 0 ustar andreh users root = true [*] indent_style = tab indent_size = 4 end_of_line = lf charset = utf-8 trim_trailing_whitespace = true insert_final_newline = true max_line_length = 150 [CHANGELOG.md] indent_style = space indent_size = 2 [*.json] max_line_length = off [Makefile] max_line_length = off apollo-server-demo/node_modules/object-inspect/package.json 0000644 0001750 0000144 00000003536 03560116604 023636 0 ustar andreh users { "name": "object-inspect", "version": "1.9.0", "description": "string representations of objects in node and the browser", "main": "index.js", "devDependencies": { "@ljharb/eslint-config": "^17.3.0", "aud": "^1.1.3", "core-js": "^2.6.12", "eslint": "^7.14.0", "for-each": "^0.3.3", "nyc": "^10.3.2", "safe-publish-latest": "^1.1.4", "string.prototype.repeat": "^1.0.0", "tape": "^5.0.1" }, "scripts": { "prepublish": "safe-publish-latest", "pretest": "npm run lint", "lint": "eslint .", "test": "npm run tests-only", "tests-only": "nyc npm run tests-only:tape", "pretests-only:tape": "node test-core-js", "tests-only:tape": "tape 'test/*.js'", "posttest": "npx aud --production" }, "testling": { "files": [ "test/*.js", "test/browser/*.js" ], "browsers": [ "ie/6..latest", "chrome/latest", "firefox/latest", "safari/latest", "opera/latest", "iphone/latest", "ipad/latest", "android/latest" ] }, "repository": { "type": "git", "url": "git://github.com/inspect-js/object-inspect.git" }, "homepage": "https://github.com/inspect-js/object-inspect", "keywords": [ "inspect", "util.inspect", "object", "stringify", "pretty" ], "author": { "name": "James Halliday", "email": "mail@substack.net", "url": "http://substack.net" }, "funding": { "url": "https://github.com/sponsors/ljharb" }, "license": "MIT", "browser": { "./util.inspect.js": false }, "greenkeeper": { "ignore": [ "nyc", "core-js" ] } ,"_resolved": "https://registry.npmjs.org/object-inspect/-/object-inspect-1.9.0.tgz" ,"_integrity": "sha512-i3Bp9iTqwhaLZBxGkRfo5ZbE07BQRT7MGu8+nNgwW9ItGp1TzCTw2DLEoWwjClxBjOFI/hWljTAmYGCEwmtnOw==" ,"_from": "object-inspect@1.9.0" } apollo-server-demo/node_modules/object-inspect/example/ 0000755 0001750 0000144 00000000000 14067647701 023007 5 ustar andreh users apollo-server-demo/node_modules/object-inspect/example/inspect.js 0000644 0001750 0000144 00000000373 03560116604 025002 0 ustar andreh users 'use strict'; /* eslint-env browser */ var inspect = require('../'); var d = document.createElement('div'); d.setAttribute('id', 'beep'); d.innerHTML = '<b>wooo</b><i>iiiii</i>'; console.log(inspect([d, { a: 3, b: 4, c: [5, 6, [7, [8, [9]]]] }])); apollo-server-demo/node_modules/object-inspect/example/circular.js 0000644 0001750 0000144 00000000164 03560116604 025137 0 ustar andreh users 'use strict'; var inspect = require('../'); var obj = { a: 1, b: [3, 4] }; obj.c = obj; console.log(inspect(obj)); apollo-server-demo/node_modules/object-inspect/example/all.js 0000644 0001750 0000144 00000000607 03560116604 024105 0 ustar andreh users 'use strict'; var inspect = require('../'); var Buffer = require('safer-buffer').Buffer; var holes = ['a', 'b']; holes[4] = 'e'; holes[6] = 'g'; var obj = { a: 1, b: [3, 4, undefined, null], c: undefined, d: null, e: { regex: /^x/i, buf: Buffer.from('abc'), holes: holes }, now: new Date() }; obj.self = obj; console.log(inspect(obj)); apollo-server-demo/node_modules/object-inspect/example/fn.js 0000644 0001750 0000144 00000000176 03560116604 023741 0 ustar andreh users 'use strict'; var inspect = require('../'); var obj = [1, 2, function f(n) { return n + 5; }, 4]; console.log(inspect(obj)); apollo-server-demo/node_modules/object-inspect/.nycrc 0000644 0001750 0000144 00000000464 03560116604 022464 0 ustar andreh users { "all": true, "check-coverage": false, "instrumentation": false, "sourceMap": false, "reporter": ["text-summary", "text", "html", "json"], "lines": 93, "statements": 93, "functions": 96, "branches": 89, "exclude": [ "coverage", "example", "test", "test-core-js.js" ] } apollo-server-demo/node_modules/object-inspect/test/ 0000755 0001750 0000144 00000000000 14067647701 022333 5 ustar andreh users apollo-server-demo/node_modules/object-inspect/test/lowbyte.js 0000644 0001750 0000144 00000000414 03560116604 024342 0 ustar andreh users var test = require('tape'); var inspect = require('../'); var obj = { x: 'a\r\nb', y: '\x05! \x1f \x12' }; test('interpolate low bytes', function (t) { t.plan(1); t.equal( inspect(obj), "{ x: 'a\\r\\nb', y: '\\x05! \\x1F \\x12' }" ); }); apollo-server-demo/node_modules/object-inspect/test/deep.js 0000644 0001750 0000144 00000000503 03560116604 023571 0 ustar andreh users var inspect = require('../'); var test = require('tape'); test('deep', function (t) { t.plan(3); var obj = [[[[[[500]]]]]]; t.equal(inspect(obj), '[ [ [ [ [ [Array] ] ] ] ] ]'); t.equal(inspect(obj, { depth: 4 }), '[ [ [ [ [Array] ] ] ] ]'); t.equal(inspect(obj, { depth: 2 }), '[ [ [Array] ] ]'); }); apollo-server-demo/node_modules/object-inspect/test/holes.js 0000644 0001750 0000144 00000000377 03560116604 023777 0 ustar andreh users var test = require('tape'); var inspect = require('../'); var xs = ['a', 'b']; xs[5] = 'f'; xs[7] = 'j'; xs[8] = 'k'; test('holes', function (t) { t.plan(1); t.equal( inspect(xs), "[ 'a', 'b', , , , 'f', , 'j', 'k' ]" ); }); apollo-server-demo/node_modules/object-inspect/test/inspect.js 0000644 0001750 0000144 00000003720 03560116604 024325 0 ustar andreh users var test = require('tape'); var hasSymbols = require('has-symbols')(); var utilInspect = require('../util.inspect'); var repeat = require('string.prototype.repeat'); var inspect = require('..'); test('inspect', function (t) { t.plan(3); var obj = [{ inspect: function xyzInspect() { return '!XYZ¡'; } }, []]; t.equal(inspect(obj), '[ !XYZ¡, [] ]'); t.equal(inspect(obj, { customInspect: true }), '[ !XYZ¡, [] ]'); t.equal(inspect(obj, { customInspect: false }), '[ { inspect: [Function: xyzInspect] }, [] ]'); }); test('inspect custom symbol', { skip: !hasSymbols || !utilInspect || !utilInspect.custom }, function (t) { t.plan(3); var obj = { inspect: function stringInspect() { return 'string'; } }; obj[utilInspect.custom] = function custom() { return 'symbol'; }; t.equal(inspect([obj, []]), '[ ' + (utilInspect.custom ? 'symbol' : 'string') + ', [] ]'); t.equal(inspect([obj, []], { customInspect: true }), '[ ' + (utilInspect.custom ? 'symbol' : 'string') + ', [] ]'); t.equal( inspect([obj, []], { customInspect: false }), '[ { inspect: [Function: stringInspect]' + (utilInspect.custom ? ', [' + inspect(utilInspect.custom) + ']: [Function: custom]' : '') + ' }, [] ]' ); }); test('symbols', { skip: !hasSymbols }, function (t) { t.plan(2); var obj = { a: 1 }; obj[Symbol('test')] = 2; obj[Symbol.iterator] = 3; Object.defineProperty(obj, Symbol('non-enum'), { enumerable: false, value: 4 }); t.equal(inspect(obj), '{ a: 1, [Symbol(test)]: 2, [Symbol(Symbol.iterator)]: 3 }', 'object with symbols'); t.equal(inspect([obj, []]), '[ { a: 1, [Symbol(test)]: 2, [Symbol(Symbol.iterator)]: 3 }, [] ]', 'object with symbols'); }); test('maxStringLength', function (t) { t.equal( inspect([repeat('a', 1e8)], { maxStringLength: 10 }), '[ \'aaaaaaaaaa\'... 99999990 more characters ]', 'maxStringLength option limits output' ); t.end(); }); apollo-server-demo/node_modules/object-inspect/test/undef.js 0000644 0001750 0000144 00000000456 03560116604 023764 0 ustar andreh users var test = require('tape'); var inspect = require('../'); var obj = { a: 1, b: [3, 4, undefined, null], c: undefined, d: null }; test('undef and null', function (t) { t.plan(1); t.equal( inspect(obj), '{ a: 1, b: [ 3, 4, undefined, null ], c: undefined, d: null }' ); }); apollo-server-demo/node_modules/object-inspect/test/element.js 0000644 0001750 0000144 00000003047 03560116604 024313 0 ustar andreh users var inspect = require('../'); var test = require('tape'); test('element', function (t) { t.plan(3); var elem = { nodeName: 'div', attributes: [{ name: 'class', value: 'row' }], getAttribute: function (key) { return key; }, childNodes: [] }; var obj = [1, elem, 3]; t.deepEqual(inspect(obj), '[ 1, <div class="row"></div>, 3 ]'); t.deepEqual(inspect(obj, { quoteStyle: 'single' }), "[ 1, <div class='row'></div>, 3 ]"); t.deepEqual(inspect(obj, { quoteStyle: 'double' }), '[ 1, <div class="row"></div>, 3 ]'); }); test('element no attr', function (t) { t.plan(1); var elem = { nodeName: 'div', getAttribute: function (key) { return key; }, childNodes: [] }; var obj = [1, elem, 3]; t.deepEqual(inspect(obj), '[ 1, <div></div>, 3 ]'); }); test('element with contents', function (t) { t.plan(1); var elem = { nodeName: 'div', getAttribute: function (key) { return key; }, childNodes: [{ nodeName: 'b' }] }; var obj = [1, elem, 3]; t.deepEqual(inspect(obj), '[ 1, <div>...</div>, 3 ]'); }); test('element instance', function (t) { t.plan(1); var h = global.HTMLElement; global.HTMLElement = function (name, attr) { this.nodeName = name; this.attributes = attr; }; global.HTMLElement.prototype.getAttribute = function () {}; var elem = new global.HTMLElement('div', []); var obj = [1, elem, 3]; t.deepEqual(inspect(obj), '[ 1, <div></div>, 3 ]'); global.HTMLElement = h; }); apollo-server-demo/node_modules/object-inspect/test/circular.js 0000644 0001750 0000144 00000000703 03560116604 024462 0 ustar andreh users var inspect = require('../'); var test = require('tape'); test('circular', function (t) { t.plan(2); var obj = { a: 1, b: [3, 4] }; obj.c = obj; t.equal(inspect(obj), '{ a: 1, b: [ 3, 4 ], c: [Circular] }'); var double = {}; double.a = [double]; double.b = {}; double.b.inner = double.b; double.b.obj = double; t.equal(inspect(double), '{ a: [ [Circular] ], b: { inner: [Circular], obj: [Circular] } }'); }); apollo-server-demo/node_modules/object-inspect/test/bigint.js 0000644 0001750 0000144 00000001634 03560116604 024136 0 ustar andreh users var inspect = require('../'); var test = require('tape'); test('bigint', { skip: typeof BigInt === 'undefined' }, function (t) { t.test('primitives', function (st) { st.plan(3); st.equal(inspect(BigInt(-256)), '-256n'); st.equal(inspect(BigInt(0)), '0n'); st.equal(inspect(BigInt(256)), '256n'); }); t.test('objects', function (st) { st.plan(3); st.equal(inspect(Object(BigInt(-256))), 'Object(-256n)'); st.equal(inspect(Object(BigInt(0))), 'Object(0n)'); st.equal(inspect(Object(BigInt(256))), 'Object(256n)'); }); t.test('syntactic primitives', function (st) { st.plan(3); /* eslint-disable no-new-func */ st.equal(inspect(Function('return -256n')()), '-256n'); st.equal(inspect(Function('return 0n')()), '0n'); st.equal(inspect(Function('return 256n')()), '256n'); }); t.end(); }); apollo-server-demo/node_modules/object-inspect/test/values.js 0000644 0001750 0000144 00000013340 03560116604 024156 0 ustar andreh users var inspect = require('../'); var test = require('tape'); test('values', function (t) { t.plan(1); var obj = [{}, [], { 'a-b': 5 }]; t.equal(inspect(obj), '[ {}, [], { \'a-b\': 5 } ]'); }); test('arrays with properties', function (t) { t.plan(1); var arr = [3]; arr.foo = 'bar'; var obj = [1, 2, arr]; obj.baz = 'quux'; obj.index = -1; t.equal(inspect(obj), '[ 1, 2, [ 3, foo: \'bar\' ], baz: \'quux\', index: -1 ]'); }); test('has', function (t) { t.plan(1); var has = Object.prototype.hasOwnProperty; delete Object.prototype.hasOwnProperty; t.equal(inspect({ a: 1, b: 2 }), '{ a: 1, b: 2 }'); Object.prototype.hasOwnProperty = has; // eslint-disable-line no-extend-native }); test('indexOf seen', function (t) { t.plan(1); var xs = [1, 2, 3, {}]; xs.push(xs); var seen = []; seen.indexOf = undefined; t.equal( inspect(xs, {}, 0, seen), '[ 1, 2, 3, {}, [Circular] ]' ); }); test('seen seen', function (t) { t.plan(1); var xs = [1, 2, 3]; var seen = [xs]; seen.indexOf = undefined; t.equal( inspect(xs, {}, 0, seen), '[Circular]' ); }); test('seen seen seen', function (t) { t.plan(1); var xs = [1, 2, 3]; var seen = [5, xs]; seen.indexOf = undefined; t.equal( inspect(xs, {}, 0, seen), '[Circular]' ); }); test('symbols', { skip: typeof Symbol !== 'function' }, function (t) { var sym = Symbol('foo'); t.equal(inspect(sym), 'Symbol(foo)', 'Symbol("foo") should be "Symbol(foo)"'); t.equal(inspect(Object(sym)), 'Object(Symbol(foo))', 'Object(Symbol("foo")) should be "Object(Symbol(foo))"'); t.end(); }); test('Map', { skip: typeof Map !== 'function' }, function (t) { var map = new Map(); map.set({ a: 1 }, ['b']); map.set(3, NaN); var expectedString = 'Map (2) {' + inspect({ a: 1 }) + ' => ' + inspect(['b']) + ', 3 => NaN}'; t.equal(inspect(map), expectedString, 'new Map([[{ a: 1 }, ["b"]], [3, NaN]]) should show size and contents'); t.equal(inspect(new Map()), 'Map (0) {}', 'empty Map should show as empty'); var nestedMap = new Map(); nestedMap.set(nestedMap, map); t.equal(inspect(nestedMap), 'Map (1) {[Circular] => ' + expectedString + '}', 'Map containing a Map should work'); t.end(); }); test('WeakMap', { skip: typeof WeakMap !== 'function' }, function (t) { var map = new WeakMap(); map.set({ a: 1 }, ['b']); var expectedString = 'WeakMap { ? }'; t.equal(inspect(map), expectedString, 'new WeakMap([[{ a: 1 }, ["b"]]]) should not show size or contents'); t.equal(inspect(new WeakMap()), 'WeakMap { ? }', 'empty WeakMap should not show as empty'); t.end(); }); test('Set', { skip: typeof Set !== 'function' }, function (t) { var set = new Set(); set.add({ a: 1 }); set.add(['b']); var expectedString = 'Set (2) {' + inspect({ a: 1 }) + ', ' + inspect(['b']) + '}'; t.equal(inspect(set), expectedString, 'new Set([{ a: 1 }, ["b"]]) should show size and contents'); t.equal(inspect(new Set()), 'Set (0) {}', 'empty Set should show as empty'); var nestedSet = new Set(); nestedSet.add(set); nestedSet.add(nestedSet); t.equal(inspect(nestedSet), 'Set (2) {' + expectedString + ', [Circular]}', 'Set containing a Set should work'); t.end(); }); test('WeakSet', { skip: typeof WeakSet !== 'function' }, function (t) { var map = new WeakSet(); map.add({ a: 1 }); var expectedString = 'WeakSet { ? }'; t.equal(inspect(map), expectedString, 'new WeakSet([{ a: 1 }]) should not show size or contents'); t.equal(inspect(new WeakSet()), 'WeakSet { ? }', 'empty WeakSet should not show as empty'); t.end(); }); test('Strings', function (t) { var str = 'abc'; t.equal(inspect(str), "'" + str + "'", 'primitive string shows as such'); t.equal(inspect(str, { quoteStyle: 'single' }), "'" + str + "'", 'primitive string shows as such, single quoted'); t.equal(inspect(str, { quoteStyle: 'double' }), '"' + str + '"', 'primitive string shows as such, double quoted'); t.equal(inspect(Object(str)), 'Object(' + inspect(str) + ')', 'String object shows as such'); t.equal(inspect(Object(str), { quoteStyle: 'single' }), 'Object(' + inspect(str, { quoteStyle: 'single' }) + ')', 'String object shows as such, single quoted'); t.equal(inspect(Object(str), { quoteStyle: 'double' }), 'Object(' + inspect(str, { quoteStyle: 'double' }) + ')', 'String object shows as such, double quoted'); t.end(); }); test('Numbers', function (t) { var num = 42; t.equal(inspect(num), String(num), 'primitive number shows as such'); t.equal(inspect(Object(num)), 'Object(' + inspect(num) + ')', 'Number object shows as such'); t.end(); }); test('Booleans', function (t) { t.equal(inspect(true), String(true), 'primitive true shows as such'); t.equal(inspect(Object(true)), 'Object(' + inspect(true) + ')', 'Boolean object true shows as such'); t.equal(inspect(false), String(false), 'primitive false shows as such'); t.equal(inspect(Object(false)), 'Object(' + inspect(false) + ')', 'Boolean false object shows as such'); t.end(); }); test('Date', function (t) { var now = new Date(); t.equal(inspect(now), String(now), 'Date shows properly'); t.equal(inspect(new Date(NaN)), 'Invalid Date', 'Invalid Date shows properly'); t.end(); }); test('RegExps', function (t) { t.equal(inspect(/a/g), '/a/g', 'regex shows properly'); t.equal(inspect(new RegExp('abc', 'i')), '/abc/i', 'new RegExp shows properly'); var match = 'abc abc'.match(/[ab]+/); delete match.groups; // for node < 10 t.equal(inspect(match), '[ \'ab\', index: 0, input: \'abc abc\' ]', 'RegExp match object shows properly'); t.end(); }); apollo-server-demo/node_modules/object-inspect/test/quoteStyle.js 0000644 0001750 0000144 00000001645 03560116604 025042 0 ustar andreh users 'use strict'; var inspect = require('../'); var test = require('tape'); test('quoteStyle option', function (t) { t['throws'](function () { inspect(null, { quoteStyle: false }); }, 'false is not a valid value'); t['throws'](function () { inspect(null, { quoteStyle: true }); }, 'true is not a valid value'); t['throws'](function () { inspect(null, { quoteStyle: '' }); }, '"" is not a valid value'); t['throws'](function () { inspect(null, { quoteStyle: {} }); }, '{} is not a valid value'); t['throws'](function () { inspect(null, { quoteStyle: [] }); }, '[] is not a valid value'); t['throws'](function () { inspect(null, { quoteStyle: 42 }); }, '42 is not a valid value'); t['throws'](function () { inspect(null, { quoteStyle: NaN }); }, 'NaN is not a valid value'); t['throws'](function () { inspect(null, { quoteStyle: function () {} }); }, 'a function is not a valid value'); t.end(); }); apollo-server-demo/node_modules/object-inspect/test/err.js 0000644 0001750 0000144 00000001322 03560116604 023444 0 ustar andreh users var inspect = require('../'); var test = require('tape'); test('type error', function (t) { t.plan(1); var aerr = new TypeError(); aerr.foo = 555; aerr.bar = [1, 2, 3]; var berr = new TypeError('tuv'); berr.baz = 555; var cerr = new SyntaxError(); cerr.message = 'whoa'; cerr['a-b'] = 5; var obj = [ new TypeError(), new TypeError('xxx'), aerr, berr, cerr ]; t.equal(inspect(obj), '[ ' + [ '[TypeError]', '[TypeError: xxx]', '{ [TypeError] foo: 555, bar: [ 1, 2, 3 ] }', '{ [TypeError: tuv] baz: 555 }', '{ [SyntaxError: whoa] message: \'whoa\', \'a-b\': 5 }' ].join(', ') + ' ]'); }); apollo-server-demo/node_modules/object-inspect/test/fn.js 0000644 0001750 0000144 00000001366 03560116604 023267 0 ustar andreh users var inspect = require('../'); var test = require('tape'); test('function', function (t) { t.plan(1); var obj = [1, 2, function f(n) { return n; }, 4]; t.equal(inspect(obj), '[ 1, 2, [Function: f], 4 ]'); }); test('function name', function (t) { t.plan(1); var f = (function () { return function () {}; }()); f.toString = function toStr() { return 'function xxx () {}'; }; var obj = [1, 2, f, 4]; t.equal(inspect(obj), '[ 1, 2, [Function (anonymous)] { toString: [Function: toStr] }, 4 ]'); }); test('anon function', function (t) { var f = (function () { return function () {}; }()); var obj = [1, 2, f, 4]; t.equal(inspect(obj), '[ 1, 2, [Function (anonymous)], 4 ]'); t.end(); }); apollo-server-demo/node_modules/object-inspect/test/indent-option.js 0000644 0001750 0000144 00000014751 03560116604 025455 0 ustar andreh users var test = require('tape'); var forEach = require('for-each'); var inspect = require('../'); test('bad indent options', function (t) { forEach([ undefined, true, false, -1, 1.2, Infinity, -Infinity, NaN ], function (indent) { t['throws']( function () { inspect('', { indent: indent }); }, TypeError, inspect(indent) + ' is invalid' ); }); t.end(); }); test('simple object with indent', function (t) { t.plan(2); var obj = { a: 1, b: 2 }; var expectedSpaces = [ '{', ' a: 1,', ' b: 2', '}' ].join('\n'); var expectedTabs = [ '{', ' a: 1,', ' b: 2', '}' ].join('\n'); t.equal(inspect(obj, { indent: 2 }), expectedSpaces, 'two'); t.equal(inspect(obj, { indent: '\t' }), expectedTabs, 'tabs'); }); test('two deep object with indent', function (t) { t.plan(2); var obj = { a: 1, b: { c: 3, d: 4 } }; var expectedSpaces = [ '{', ' a: 1,', ' b: {', ' c: 3,', ' d: 4', ' }', '}' ].join('\n'); var expectedTabs = [ '{', ' a: 1,', ' b: {', ' c: 3,', ' d: 4', ' }', '}' ].join('\n'); t.equal(inspect(obj, { indent: 2 }), expectedSpaces, 'two'); t.equal(inspect(obj, { indent: '\t' }), expectedTabs, 'tabs'); }); test('simple array with all single line elements', function (t) { t.plan(2); var obj = [1, 2, 3, 'asdf\nsdf']; var expected = '[ 1, 2, 3, \'asdf\\nsdf\' ]'; t.equal(inspect(obj, { indent: 2 }), expected, 'two'); t.equal(inspect(obj, { indent: '\t' }), expected, 'tabs'); }); test('array with complex elements', function (t) { t.plan(2); var obj = [1, { a: 1, b: { c: 1 } }, 'asdf\nsdf']; var expectedSpaces = [ '[', ' 1,', ' {', ' a: 1,', ' b: {', ' c: 1', ' }', ' },', ' \'asdf\\nsdf\'', ']' ].join('\n'); var expectedTabs = [ '[', ' 1,', ' {', ' a: 1,', ' b: {', ' c: 1', ' }', ' },', ' \'asdf\\nsdf\'', ']' ].join('\n'); t.equal(inspect(obj, { indent: 2 }), expectedSpaces, 'two'); t.equal(inspect(obj, { indent: '\t' }), expectedTabs, 'tabs'); }); test('values', function (t) { t.plan(2); var obj = [{}, [], { 'a-b': 5 }]; var expectedSpaces = [ '[', ' {},', ' [],', ' {', ' \'a-b\': 5', ' }', ']' ].join('\n'); var expectedTabs = [ '[', ' {},', ' [],', ' {', ' \'a-b\': 5', ' }', ']' ].join('\n'); t.equal(inspect(obj, { indent: 2 }), expectedSpaces, 'two'); t.equal(inspect(obj, { indent: '\t' }), expectedTabs, 'tabs'); }); test('Map', { skip: typeof Map !== 'function' }, function (t) { var map = new Map(); map.set({ a: 1 }, ['b']); map.set(3, NaN); var expectedStringSpaces = [ 'Map (2) {', ' { a: 1 } => [ \'b\' ],', ' 3 => NaN', '}' ].join('\n'); var expectedStringTabs = [ 'Map (2) {', ' { a: 1 } => [ \'b\' ],', ' 3 => NaN', '}' ].join('\n'); var expectedStringTabsDoubleQuotes = [ 'Map (2) {', ' { a: 1 } => [ "b" ],', ' 3 => NaN', '}' ].join('\n'); t.equal( inspect(map, { indent: 2 }), expectedStringSpaces, 'Map keys are not indented (two)' ); t.equal( inspect(map, { indent: '\t' }), expectedStringTabs, 'Map keys are not indented (tabs)' ); t.equal( inspect(map, { indent: '\t', quoteStyle: 'double' }), expectedStringTabsDoubleQuotes, 'Map keys are not indented (tabs + double quotes)' ); t.equal(inspect(new Map(), { indent: 2 }), 'Map (0) {}', 'empty Map should show as empty (two)'); t.equal(inspect(new Map(), { indent: '\t' }), 'Map (0) {}', 'empty Map should show as empty (tabs)'); var nestedMap = new Map(); nestedMap.set(nestedMap, map); var expectedNestedSpaces = [ 'Map (1) {', ' [Circular] => Map (2) {', ' { a: 1 } => [ \'b\' ],', ' 3 => NaN', ' }', '}' ].join('\n'); var expectedNestedTabs = [ 'Map (1) {', ' [Circular] => Map (2) {', ' { a: 1 } => [ \'b\' ],', ' 3 => NaN', ' }', '}' ].join('\n'); t.equal(inspect(nestedMap, { indent: 2 }), expectedNestedSpaces, 'Map containing a Map should work (two)'); t.equal(inspect(nestedMap, { indent: '\t' }), expectedNestedTabs, 'Map containing a Map should work (tabs)'); t.end(); }); test('Set', { skip: typeof Set !== 'function' }, function (t) { var set = new Set(); set.add({ a: 1 }); set.add(['b']); var expectedStringSpaces = [ 'Set (2) {', ' {', ' a: 1', ' },', ' [ \'b\' ]', '}' ].join('\n'); var expectedStringTabs = [ 'Set (2) {', ' {', ' a: 1', ' },', ' [ \'b\' ]', '}' ].join('\n'); t.equal(inspect(set, { indent: 2 }), expectedStringSpaces, 'new Set([{ a: 1 }, ["b"]]) should show size and contents (two)'); t.equal(inspect(set, { indent: '\t' }), expectedStringTabs, 'new Set([{ a: 1 }, ["b"]]) should show size and contents (tabs)'); t.equal(inspect(new Set(), { indent: 2 }), 'Set (0) {}', 'empty Set should show as empty (two)'); t.equal(inspect(new Set(), { indent: '\t' }), 'Set (0) {}', 'empty Set should show as empty (tabs)'); var nestedSet = new Set(); nestedSet.add(set); nestedSet.add(nestedSet); var expectedNestedSpaces = [ 'Set (2) {', ' Set (2) {', ' {', ' a: 1', ' },', ' [ \'b\' ]', ' },', ' [Circular]', '}' ].join('\n'); var expectedNestedTabs = [ 'Set (2) {', ' Set (2) {', ' {', ' a: 1', ' },', ' [ \'b\' ]', ' },', ' [Circular]', '}' ].join('\n'); t.equal(inspect(nestedSet, { indent: 2 }), expectedNestedSpaces, 'Set containing a Set should work (two)'); t.equal(inspect(nestedSet, { indent: '\t' }), expectedNestedTabs, 'Set containing a Set should work (tabs)'); t.end(); }); apollo-server-demo/node_modules/object-inspect/test/number.js 0000644 0001750 0000144 00000000613 03560116604 024146 0 ustar andreh users var inspect = require('../'); var test = require('tape'); test('negative zero', function (t) { t.equal(inspect(0), '0', 'inspect(0) === "0"'); t.equal(inspect(Object(0)), 'Object(0)', 'inspect(Object(0)) === "Object(0)"'); t.equal(inspect(-0), '-0', 'inspect(-0) === "-0"'); t.equal(inspect(Object(-0)), 'Object(-0)', 'inspect(Object(-0)) === "Object(-0)"'); t.end(); }); apollo-server-demo/node_modules/object-inspect/test/has.js 0000644 0001750 0000144 00000001732 03560116604 023434 0 ustar andreh users var inspect = require('../'); var test = require('tape'); function withoutProperty(object, property, fn) { var original; if (Object.getOwnPropertyDescriptor) { original = Object.getOwnPropertyDescriptor(object, property); } else { original = object[property]; } delete object[property]; try { fn(); } finally { if (Object.getOwnPropertyDescriptor) { Object.defineProperty(object, property, original); } else { object[property] = original; } } } test('when Object#hasOwnProperty is deleted', function (t) { t.plan(1); var arr = [1, , 3]; // eslint-disable-line no-sparse-arrays // eslint-disable-next-line no-extend-native Array.prototype[1] = 2; // this is needed to account for "in" vs "hasOwnProperty" withoutProperty(Object.prototype, 'hasOwnProperty', function () { t.equal(inspect(arr), '[ 1, , 3 ]'); }); delete Array.prototype[1]; }); apollo-server-demo/node_modules/object-inspect/test/browser/ 0000755 0001750 0000144 00000000000 14067647701 024016 5 ustar andreh users apollo-server-demo/node_modules/object-inspect/test/browser/dom.js 0000644 0001750 0000144 00000000640 03560116604 025120 0 ustar andreh users var inspect = require('../../'); var test = require('tape'); test('dom element', function (t) { t.plan(1); var d = document.createElement('div'); d.setAttribute('id', 'beep'); d.innerHTML = '<b>wooo</b><i>iiiii</i>'; t.equal( inspect([d, { a: 3, b: 4, c: [5, 6, [7, [8, [9]]]] }]), '[ <div id="beep">...</div>, { a: 3, b: 4, c: [ 5, 6, [ 7, [ 8, [Object] ] ] ] } ]' ); }); apollo-server-demo/node_modules/object-inspect/.eslintignore 0000644 0001750 0000144 00000000012 03560116604 024035 0 ustar andreh users coverage/ apollo-server-demo/node_modules/object-inspect/util.inspect.js 0000644 0001750 0000144 00000000052 03560116604 024315 0 ustar andreh users module.exports = require('util').inspect; apollo-server-demo/node_modules/object-inspect/readme.markdown 0000644 0001750 0000144 00000002607 03560116604 024347 0 ustar andreh users # object-inspect string representations of objects in node and the browser [](https://travis-ci.com/inspect-js/object-inspect) # example ## circular ``` js var inspect = require('object-inspect'); var obj = { a: 1, b: [3,4] }; obj.c = obj; console.log(inspect(obj)); ``` ## dom element ``` js var inspect = require('object-inspect'); var d = document.createElement('div'); d.setAttribute('id', 'beep'); d.innerHTML = '<b>wooo</b><i>iiiii</i>'; console.log(inspect([ d, { a: 3, b : 4, c: [5,6,[7,[8,[9]]]] } ])); ``` output: ``` [ <div id="beep">...</div>, { a: 3, b: 4, c: [ 5, 6, [ 7, [ 8, [ ... ] ] ] ] } ] ``` # methods ``` js var inspect = require('object-inspect') ``` ## var s = inspect(obj, opts={}) Return a string `s` with the string representation of `obj` up to a depth of `opts.depth`. Additional options: - `quoteStyle`: must be "single" or "double", if present. Default `'single'` for strings, `'double'` for HTML elements. - `maxStringLength`: must be `0`, a positive integer, `Infinity`, or `null`, if present. Default `Infinity`. - `customInspect`: When `true`, a custom inspect method function will be invoked. Default `true`. - `indent`: must be "\t", `null`, or a positive integer. Default `null`. # install With [npm](https://npmjs.org) do: ``` npm install object-inspect ``` # license MIT apollo-server-demo/node_modules/negotiator/ 0000755 0001750 0000144 00000000000 14067647700 020615 5 ustar andreh users apollo-server-demo/node_modules/negotiator/index.js 0000644 0001750 0000144 00000006420 03560116604 022252 0 ustar andreh users /*! * negotiator * Copyright(c) 2012 Federico Romero * Copyright(c) 2012-2014 Isaac Z. Schlueter * Copyright(c) 2015 Douglas Christopher Wilson * MIT Licensed */ 'use strict'; /** * Cached loaded submodules. * @private */ var modules = Object.create(null); /** * Module exports. * @public */ module.exports = Negotiator; module.exports.Negotiator = Negotiator; /** * Create a Negotiator instance from a request. * @param {object} request * @public */ function Negotiator(request) { if (!(this instanceof Negotiator)) { return new Negotiator(request); } this.request = request; } Negotiator.prototype.charset = function charset(available) { var set = this.charsets(available); return set && set[0]; }; Negotiator.prototype.charsets = function charsets(available) { var preferredCharsets = loadModule('charset').preferredCharsets; return preferredCharsets(this.request.headers['accept-charset'], available); }; Negotiator.prototype.encoding = function encoding(available) { var set = this.encodings(available); return set && set[0]; }; Negotiator.prototype.encodings = function encodings(available) { var preferredEncodings = loadModule('encoding').preferredEncodings; return preferredEncodings(this.request.headers['accept-encoding'], available); }; Negotiator.prototype.language = function language(available) { var set = this.languages(available); return set && set[0]; }; Negotiator.prototype.languages = function languages(available) { var preferredLanguages = loadModule('language').preferredLanguages; return preferredLanguages(this.request.headers['accept-language'], available); }; Negotiator.prototype.mediaType = function mediaType(available) { var set = this.mediaTypes(available); return set && set[0]; }; Negotiator.prototype.mediaTypes = function mediaTypes(available) { var preferredMediaTypes = loadModule('mediaType').preferredMediaTypes; return preferredMediaTypes(this.request.headers.accept, available); }; // Backwards compatibility Negotiator.prototype.preferredCharset = Negotiator.prototype.charset; Negotiator.prototype.preferredCharsets = Negotiator.prototype.charsets; Negotiator.prototype.preferredEncoding = Negotiator.prototype.encoding; Negotiator.prototype.preferredEncodings = Negotiator.prototype.encodings; Negotiator.prototype.preferredLanguage = Negotiator.prototype.language; Negotiator.prototype.preferredLanguages = Negotiator.prototype.languages; Negotiator.prototype.preferredMediaType = Negotiator.prototype.mediaType; Negotiator.prototype.preferredMediaTypes = Negotiator.prototype.mediaTypes; /** * Load the given module. * @private */ function loadModule(moduleName) { var module = modules[moduleName]; if (module !== undefined) { return module; } // This uses a switch for static require analysis switch (moduleName) { case 'charset': module = require('./lib/charset'); break; case 'encoding': module = require('./lib/encoding'); break; case 'language': module = require('./lib/language'); break; case 'mediaType': module = require('./lib/mediaType'); break; default: throw new Error('Cannot find module \'' + moduleName + '\''); } // Store to prevent invoking require() modules[moduleName] = module; return module; } apollo-server-demo/node_modules/negotiator/LICENSE 0000644 0001750 0000144 00000002231 03560116604 021606 0 ustar andreh users (The MIT License) Copyright (c) 2012-2014 Federico Romero Copyright (c) 2012-2014 Isaac Z. Schlueter Copyright (c) 2014-2015 Douglas Christopher Wilson Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the 'Software'), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/negotiator/HISTORY.md 0000644 0001750 0000144 00000004546 03560116604 022277 0 ustar andreh users 0.6.2 / 2019-04-29 ================== * Fix sorting charset, encoding, and language with extra parameters 0.6.1 / 2016-05-02 ================== * perf: improve `Accept` parsing speed * perf: improve `Accept-Charset` parsing speed * perf: improve `Accept-Encoding` parsing speed * perf: improve `Accept-Language` parsing speed 0.6.0 / 2015-09-29 ================== * Fix including type extensions in parameters in `Accept` parsing * Fix parsing `Accept` parameters with quoted equals * Fix parsing `Accept` parameters with quoted semicolons * Lazy-load modules from main entry point * perf: delay type concatenation until needed * perf: enable strict mode * perf: hoist regular expressions * perf: remove closures getting spec properties * perf: remove a closure from media type parsing * perf: remove property delete from media type parsing 0.5.3 / 2015-05-10 ================== * Fix media type parameter matching to be case-insensitive 0.5.2 / 2015-05-06 ================== * Fix comparing media types with quoted values * Fix splitting media types with quoted commas 0.5.1 / 2015-02-14 ================== * Fix preference sorting to be stable for long acceptable lists 0.5.0 / 2014-12-18 ================== * Fix list return order when large accepted list * Fix missing identity encoding when q=0 exists * Remove dynamic building of Negotiator class 0.4.9 / 2014-10-14 ================== * Fix error when media type has invalid parameter 0.4.8 / 2014-09-28 ================== * Fix all negotiations to be case-insensitive * Stable sort preferences of same quality according to client order * Support Node.js 0.6 0.4.7 / 2014-06-24 ================== * Handle invalid provided languages * Handle invalid provided media types 0.4.6 / 2014-06-11 ================== * Order by specificity when quality is the same 0.4.5 / 2014-05-29 ================== * Fix regression in empty header handling 0.4.4 / 2014-05-29 ================== * Fix behaviors when headers are not present 0.4.3 / 2014-04-16 ================== * Handle slashes on media params correctly 0.4.2 / 2014-02-28 ================== * Fix media type sorting * Handle media types params strictly 0.4.1 / 2014-01-16 ================== * Use most specific matches 0.4.0 / 2014-01-09 ================== * Remove preferred prefix from methods apollo-server-demo/node_modules/negotiator/README.md 0000644 0001750 0000144 00000011313 03560116604 022061 0 ustar andreh users # negotiator [![NPM Version][npm-image]][npm-url] [![NPM Downloads][downloads-image]][downloads-url] [![Node.js Version][node-version-image]][node-version-url] [![Build Status][travis-image]][travis-url] [![Test Coverage][coveralls-image]][coveralls-url] An HTTP content negotiator for Node.js ## Installation ```sh $ npm install negotiator ``` ## API ```js var Negotiator = require('negotiator') ``` ### Accept Negotiation ```js availableMediaTypes = ['text/html', 'text/plain', 'application/json'] // The negotiator constructor receives a request object negotiator = new Negotiator(request) // Let's say Accept header is 'text/html, application/*;q=0.2, image/jpeg;q=0.8' negotiator.mediaTypes() // -> ['text/html', 'image/jpeg', 'application/*'] negotiator.mediaTypes(availableMediaTypes) // -> ['text/html', 'application/json'] negotiator.mediaType(availableMediaTypes) // -> 'text/html' ``` You can check a working example at `examples/accept.js`. #### Methods ##### mediaType() Returns the most preferred media type from the client. ##### mediaType(availableMediaType) Returns the most preferred media type from a list of available media types. ##### mediaTypes() Returns an array of preferred media types ordered by the client preference. ##### mediaTypes(availableMediaTypes) Returns an array of preferred media types ordered by priority from a list of available media types. ### Accept-Language Negotiation ```js negotiator = new Negotiator(request) availableLanguages = ['en', 'es', 'fr'] // Let's say Accept-Language header is 'en;q=0.8, es, pt' negotiator.languages() // -> ['es', 'pt', 'en'] negotiator.languages(availableLanguages) // -> ['es', 'en'] language = negotiator.language(availableLanguages) // -> 'es' ``` You can check a working example at `examples/language.js`. #### Methods ##### language() Returns the most preferred language from the client. ##### language(availableLanguages) Returns the most preferred language from a list of available languages. ##### languages() Returns an array of preferred languages ordered by the client preference. ##### languages(availableLanguages) Returns an array of preferred languages ordered by priority from a list of available languages. ### Accept-Charset Negotiation ```js availableCharsets = ['utf-8', 'iso-8859-1', 'iso-8859-5'] negotiator = new Negotiator(request) // Let's say Accept-Charset header is 'utf-8, iso-8859-1;q=0.8, utf-7;q=0.2' negotiator.charsets() // -> ['utf-8', 'iso-8859-1', 'utf-7'] negotiator.charsets(availableCharsets) // -> ['utf-8', 'iso-8859-1'] negotiator.charset(availableCharsets) // -> 'utf-8' ``` You can check a working example at `examples/charset.js`. #### Methods ##### charset() Returns the most preferred charset from the client. ##### charset(availableCharsets) Returns the most preferred charset from a list of available charsets. ##### charsets() Returns an array of preferred charsets ordered by the client preference. ##### charsets(availableCharsets) Returns an array of preferred charsets ordered by priority from a list of available charsets. ### Accept-Encoding Negotiation ```js availableEncodings = ['identity', 'gzip'] negotiator = new Negotiator(request) // Let's say Accept-Encoding header is 'gzip, compress;q=0.2, identity;q=0.5' negotiator.encodings() // -> ['gzip', 'identity', 'compress'] negotiator.encodings(availableEncodings) // -> ['gzip', 'identity'] negotiator.encoding(availableEncodings) // -> 'gzip' ``` You can check a working example at `examples/encoding.js`. #### Methods ##### encoding() Returns the most preferred encoding from the client. ##### encoding(availableEncodings) Returns the most preferred encoding from a list of available encodings. ##### encodings() Returns an array of preferred encodings ordered by the client preference. ##### encodings(availableEncodings) Returns an array of preferred encodings ordered by priority from a list of available encodings. ## See Also The [accepts](https://npmjs.org/package/accepts#readme) module builds on this module and provides an alternative interface, mime type validation, and more. ## License [MIT](LICENSE) [npm-image]: https://img.shields.io/npm/v/negotiator.svg [npm-url]: https://npmjs.org/package/negotiator [node-version-image]: https://img.shields.io/node/v/negotiator.svg [node-version-url]: https://nodejs.org/en/download/ [travis-image]: https://img.shields.io/travis/jshttp/negotiator/master.svg [travis-url]: https://travis-ci.org/jshttp/negotiator [coveralls-image]: https://img.shields.io/coveralls/jshttp/negotiator/master.svg [coveralls-url]: https://coveralls.io/r/jshttp/negotiator?branch=master [downloads-image]: https://img.shields.io/npm/dm/negotiator.svg [downloads-url]: https://npmjs.org/package/negotiator apollo-server-demo/node_modules/negotiator/package.json 0000644 0001750 0000144 00000002316 03560116604 023073 0 ustar andreh users { "name": "negotiator", "description": "HTTP content negotiation", "version": "0.6.2", "contributors": [ "Douglas Christopher Wilson <doug@somethingdoug.com>", "Federico Romero <federico.romero@outboxlabs.com>", "Isaac Z. Schlueter <i@izs.me> (http://blog.izs.me/)" ], "license": "MIT", "keywords": [ "http", "content negotiation", "accept", "accept-language", "accept-encoding", "accept-charset" ], "repository": "jshttp/negotiator", "devDependencies": { "eslint": "5.16.0", "eslint-plugin-markdown": "1.0.0", "mocha": "6.1.4", "nyc": "14.0.0" }, "files": [ "lib/", "HISTORY.md", "LICENSE", "index.js", "README.md" ], "engines": { "node": ">= 0.6" }, "scripts": { "lint": "eslint --plugin markdown --ext js,md .", "test": "mocha --reporter spec --check-leaks --bail test/", "test-cov": "nyc --reporter=html --reporter=text npm test", "test-travis": "nyc --reporter=text npm test" } ,"_resolved": "https://registry.npmjs.org/negotiator/-/negotiator-0.6.2.tgz" ,"_integrity": "sha512-hZXc7K2e+PgeI1eDBe/10Ard4ekbfrrqG8Ep+8Jmf4JID2bNg7NvCPOZN+kfF574pFQI7mum2AUqDidoKqcTOw==" ,"_from": "negotiator@0.6.2" } apollo-server-demo/node_modules/negotiator/lib/ 0000755 0001750 0000144 00000000000 14067647700 021363 5 ustar andreh users apollo-server-demo/node_modules/negotiator/lib/language.js 0000644 0001750 0000144 00000006520 03560116604 023475 0 ustar andreh users /** * negotiator * Copyright(c) 2012 Isaac Z. Schlueter * Copyright(c) 2014 Federico Romero * Copyright(c) 2014-2015 Douglas Christopher Wilson * MIT Licensed */ 'use strict'; /** * Module exports. * @public */ module.exports = preferredLanguages; module.exports.preferredLanguages = preferredLanguages; /** * Module variables. * @private */ var simpleLanguageRegExp = /^\s*([^\s\-;]+)(?:-([^\s;]+))?\s*(?:;(.*))?$/; /** * Parse the Accept-Language header. * @private */ function parseAcceptLanguage(accept) { var accepts = accept.split(','); for (var i = 0, j = 0; i < accepts.length; i++) { var language = parseLanguage(accepts[i].trim(), i); if (language) { accepts[j++] = language; } } // trim accepts accepts.length = j; return accepts; } /** * Parse a language from the Accept-Language header. * @private */ function parseLanguage(str, i) { var match = simpleLanguageRegExp.exec(str); if (!match) return null; var prefix = match[1], suffix = match[2], full = prefix; if (suffix) full += "-" + suffix; var q = 1; if (match[3]) { var params = match[3].split(';') for (var j = 0; j < params.length; j++) { var p = params[j].split('='); if (p[0] === 'q') q = parseFloat(p[1]); } } return { prefix: prefix, suffix: suffix, q: q, i: i, full: full }; } /** * Get the priority of a language. * @private */ function getLanguagePriority(language, accepted, index) { var priority = {o: -1, q: 0, s: 0}; for (var i = 0; i < accepted.length; i++) { var spec = specify(language, accepted[i], index); if (spec && (priority.s - spec.s || priority.q - spec.q || priority.o - spec.o) < 0) { priority = spec; } } return priority; } /** * Get the specificity of the language. * @private */ function specify(language, spec, index) { var p = parseLanguage(language) if (!p) return null; var s = 0; if(spec.full.toLowerCase() === p.full.toLowerCase()){ s |= 4; } else if (spec.prefix.toLowerCase() === p.full.toLowerCase()) { s |= 2; } else if (spec.full.toLowerCase() === p.prefix.toLowerCase()) { s |= 1; } else if (spec.full !== '*' ) { return null } return { i: index, o: spec.i, q: spec.q, s: s } }; /** * Get the preferred languages from an Accept-Language header. * @public */ function preferredLanguages(accept, provided) { // RFC 2616 sec 14.4: no header = * var accepts = parseAcceptLanguage(accept === undefined ? '*' : accept || ''); if (!provided) { // sorted list of all languages return accepts .filter(isQuality) .sort(compareSpecs) .map(getFullLanguage); } var priorities = provided.map(function getPriority(type, index) { return getLanguagePriority(type, accepts, index); }); // sorted list of accepted languages return priorities.filter(isQuality).sort(compareSpecs).map(function getLanguage(priority) { return provided[priorities.indexOf(priority)]; }); } /** * Compare two specs. * @private */ function compareSpecs(a, b) { return (b.q - a.q) || (b.s - a.s) || (a.o - b.o) || (a.i - b.i) || 0; } /** * Get full language string. * @private */ function getFullLanguage(spec) { return spec.full; } /** * Check if a spec has any quality. * @private */ function isQuality(spec) { return spec.q > 0; } apollo-server-demo/node_modules/negotiator/lib/charset.js 0000644 0001750 0000144 00000006011 03560116604 023336 0 ustar andreh users /** * negotiator * Copyright(c) 2012 Isaac Z. Schlueter * Copyright(c) 2014 Federico Romero * Copyright(c) 2014-2015 Douglas Christopher Wilson * MIT Licensed */ 'use strict'; /** * Module exports. * @public */ module.exports = preferredCharsets; module.exports.preferredCharsets = preferredCharsets; /** * Module variables. * @private */ var simpleCharsetRegExp = /^\s*([^\s;]+)\s*(?:;(.*))?$/; /** * Parse the Accept-Charset header. * @private */ function parseAcceptCharset(accept) { var accepts = accept.split(','); for (var i = 0, j = 0; i < accepts.length; i++) { var charset = parseCharset(accepts[i].trim(), i); if (charset) { accepts[j++] = charset; } } // trim accepts accepts.length = j; return accepts; } /** * Parse a charset from the Accept-Charset header. * @private */ function parseCharset(str, i) { var match = simpleCharsetRegExp.exec(str); if (!match) return null; var charset = match[1]; var q = 1; if (match[2]) { var params = match[2].split(';') for (var j = 0; j < params.length; j++) { var p = params[j].trim().split('='); if (p[0] === 'q') { q = parseFloat(p[1]); break; } } } return { charset: charset, q: q, i: i }; } /** * Get the priority of a charset. * @private */ function getCharsetPriority(charset, accepted, index) { var priority = {o: -1, q: 0, s: 0}; for (var i = 0; i < accepted.length; i++) { var spec = specify(charset, accepted[i], index); if (spec && (priority.s - spec.s || priority.q - spec.q || priority.o - spec.o) < 0) { priority = spec; } } return priority; } /** * Get the specificity of the charset. * @private */ function specify(charset, spec, index) { var s = 0; if(spec.charset.toLowerCase() === charset.toLowerCase()){ s |= 1; } else if (spec.charset !== '*' ) { return null } return { i: index, o: spec.i, q: spec.q, s: s } } /** * Get the preferred charsets from an Accept-Charset header. * @public */ function preferredCharsets(accept, provided) { // RFC 2616 sec 14.2: no header = * var accepts = parseAcceptCharset(accept === undefined ? '*' : accept || ''); if (!provided) { // sorted list of all charsets return accepts .filter(isQuality) .sort(compareSpecs) .map(getFullCharset); } var priorities = provided.map(function getPriority(type, index) { return getCharsetPriority(type, accepts, index); }); // sorted list of accepted charsets return priorities.filter(isQuality).sort(compareSpecs).map(function getCharset(priority) { return provided[priorities.indexOf(priority)]; }); } /** * Compare two specs. * @private */ function compareSpecs(a, b) { return (b.q - a.q) || (b.s - a.s) || (a.o - b.o) || (a.i - b.i) || 0; } /** * Get full charset string. * @private */ function getFullCharset(spec) { return spec.charset; } /** * Check if a spec has any quality. * @private */ function isQuality(spec) { return spec.q > 0; } apollo-server-demo/node_modules/negotiator/lib/encoding.js 0000644 0001750 0000144 00000006662 03560116604 023507 0 ustar andreh users /** * negotiator * Copyright(c) 2012 Isaac Z. Schlueter * Copyright(c) 2014 Federico Romero * Copyright(c) 2014-2015 Douglas Christopher Wilson * MIT Licensed */ 'use strict'; /** * Module exports. * @public */ module.exports = preferredEncodings; module.exports.preferredEncodings = preferredEncodings; /** * Module variables. * @private */ var simpleEncodingRegExp = /^\s*([^\s;]+)\s*(?:;(.*))?$/; /** * Parse the Accept-Encoding header. * @private */ function parseAcceptEncoding(accept) { var accepts = accept.split(','); var hasIdentity = false; var minQuality = 1; for (var i = 0, j = 0; i < accepts.length; i++) { var encoding = parseEncoding(accepts[i].trim(), i); if (encoding) { accepts[j++] = encoding; hasIdentity = hasIdentity || specify('identity', encoding); minQuality = Math.min(minQuality, encoding.q || 1); } } if (!hasIdentity) { /* * If identity doesn't explicitly appear in the accept-encoding header, * it's added to the list of acceptable encoding with the lowest q */ accepts[j++] = { encoding: 'identity', q: minQuality, i: i }; } // trim accepts accepts.length = j; return accepts; } /** * Parse an encoding from the Accept-Encoding header. * @private */ function parseEncoding(str, i) { var match = simpleEncodingRegExp.exec(str); if (!match) return null; var encoding = match[1]; var q = 1; if (match[2]) { var params = match[2].split(';'); for (var j = 0; j < params.length; j++) { var p = params[j].trim().split('='); if (p[0] === 'q') { q = parseFloat(p[1]); break; } } } return { encoding: encoding, q: q, i: i }; } /** * Get the priority of an encoding. * @private */ function getEncodingPriority(encoding, accepted, index) { var priority = {o: -1, q: 0, s: 0}; for (var i = 0; i < accepted.length; i++) { var spec = specify(encoding, accepted[i], index); if (spec && (priority.s - spec.s || priority.q - spec.q || priority.o - spec.o) < 0) { priority = spec; } } return priority; } /** * Get the specificity of the encoding. * @private */ function specify(encoding, spec, index) { var s = 0; if(spec.encoding.toLowerCase() === encoding.toLowerCase()){ s |= 1; } else if (spec.encoding !== '*' ) { return null } return { i: index, o: spec.i, q: spec.q, s: s } }; /** * Get the preferred encodings from an Accept-Encoding header. * @public */ function preferredEncodings(accept, provided) { var accepts = parseAcceptEncoding(accept || ''); if (!provided) { // sorted list of all encodings return accepts .filter(isQuality) .sort(compareSpecs) .map(getFullEncoding); } var priorities = provided.map(function getPriority(type, index) { return getEncodingPriority(type, accepts, index); }); // sorted list of accepted encodings return priorities.filter(isQuality).sort(compareSpecs).map(function getEncoding(priority) { return provided[priorities.indexOf(priority)]; }); } /** * Compare two specs. * @private */ function compareSpecs(a, b) { return (b.q - a.q) || (b.s - a.s) || (a.o - b.o) || (a.i - b.i) || 0; } /** * Get full encoding string. * @private */ function getFullEncoding(spec) { return spec.encoding; } /** * Check if a spec has any quality. * @private */ function isQuality(spec) { return spec.q > 0; } apollo-server-demo/node_modules/negotiator/lib/mediaType.js 0000644 0001750 0000144 00000012356 03560116604 023637 0 ustar andreh users /** * negotiator * Copyright(c) 2012 Isaac Z. Schlueter * Copyright(c) 2014 Federico Romero * Copyright(c) 2014-2015 Douglas Christopher Wilson * MIT Licensed */ 'use strict'; /** * Module exports. * @public */ module.exports = preferredMediaTypes; module.exports.preferredMediaTypes = preferredMediaTypes; /** * Module variables. * @private */ var simpleMediaTypeRegExp = /^\s*([^\s\/;]+)\/([^;\s]+)\s*(?:;(.*))?$/; /** * Parse the Accept header. * @private */ function parseAccept(accept) { var accepts = splitMediaTypes(accept); for (var i = 0, j = 0; i < accepts.length; i++) { var mediaType = parseMediaType(accepts[i].trim(), i); if (mediaType) { accepts[j++] = mediaType; } } // trim accepts accepts.length = j; return accepts; } /** * Parse a media type from the Accept header. * @private */ function parseMediaType(str, i) { var match = simpleMediaTypeRegExp.exec(str); if (!match) return null; var params = Object.create(null); var q = 1; var subtype = match[2]; var type = match[1]; if (match[3]) { var kvps = splitParameters(match[3]).map(splitKeyValuePair); for (var j = 0; j < kvps.length; j++) { var pair = kvps[j]; var key = pair[0].toLowerCase(); var val = pair[1]; // get the value, unwrapping quotes var value = val && val[0] === '"' && val[val.length - 1] === '"' ? val.substr(1, val.length - 2) : val; if (key === 'q') { q = parseFloat(value); break; } // store parameter params[key] = value; } } return { type: type, subtype: subtype, params: params, q: q, i: i }; } /** * Get the priority of a media type. * @private */ function getMediaTypePriority(type, accepted, index) { var priority = {o: -1, q: 0, s: 0}; for (var i = 0; i < accepted.length; i++) { var spec = specify(type, accepted[i], index); if (spec && (priority.s - spec.s || priority.q - spec.q || priority.o - spec.o) < 0) { priority = spec; } } return priority; } /** * Get the specificity of the media type. * @private */ function specify(type, spec, index) { var p = parseMediaType(type); var s = 0; if (!p) { return null; } if(spec.type.toLowerCase() == p.type.toLowerCase()) { s |= 4 } else if(spec.type != '*') { return null; } if(spec.subtype.toLowerCase() == p.subtype.toLowerCase()) { s |= 2 } else if(spec.subtype != '*') { return null; } var keys = Object.keys(spec.params); if (keys.length > 0) { if (keys.every(function (k) { return spec.params[k] == '*' || (spec.params[k] || '').toLowerCase() == (p.params[k] || '').toLowerCase(); })) { s |= 1 } else { return null } } return { i: index, o: spec.i, q: spec.q, s: s, } } /** * Get the preferred media types from an Accept header. * @public */ function preferredMediaTypes(accept, provided) { // RFC 2616 sec 14.2: no header = */* var accepts = parseAccept(accept === undefined ? '*/*' : accept || ''); if (!provided) { // sorted list of all types return accepts .filter(isQuality) .sort(compareSpecs) .map(getFullType); } var priorities = provided.map(function getPriority(type, index) { return getMediaTypePriority(type, accepts, index); }); // sorted list of accepted types return priorities.filter(isQuality).sort(compareSpecs).map(function getType(priority) { return provided[priorities.indexOf(priority)]; }); } /** * Compare two specs. * @private */ function compareSpecs(a, b) { return (b.q - a.q) || (b.s - a.s) || (a.o - b.o) || (a.i - b.i) || 0; } /** * Get full type string. * @private */ function getFullType(spec) { return spec.type + '/' + spec.subtype; } /** * Check if a spec has any quality. * @private */ function isQuality(spec) { return spec.q > 0; } /** * Count the number of quotes in a string. * @private */ function quoteCount(string) { var count = 0; var index = 0; while ((index = string.indexOf('"', index)) !== -1) { count++; index++; } return count; } /** * Split a key value pair. * @private */ function splitKeyValuePair(str) { var index = str.indexOf('='); var key; var val; if (index === -1) { key = str; } else { key = str.substr(0, index); val = str.substr(index + 1); } return [key, val]; } /** * Split an Accept header into media types. * @private */ function splitMediaTypes(accept) { var accepts = accept.split(','); for (var i = 1, j = 0; i < accepts.length; i++) { if (quoteCount(accepts[j]) % 2 == 0) { accepts[++j] = accepts[i]; } else { accepts[j] += ',' + accepts[i]; } } // trim accepts accepts.length = j + 1; return accepts; } /** * Split a string of parameters. * @private */ function splitParameters(str) { var parameters = str.split(';'); for (var i = 1, j = 0; i < parameters.length; i++) { if (quoteCount(parameters[j]) % 2 == 0) { parameters[++j] = parameters[i]; } else { parameters[j] += ';' + parameters[i]; } } // trim parameters parameters.length = j + 1; for (var i = 0; i < parameters.length; i++) { parameters[i] = parameters[i].trim(); } return parameters; } apollo-server-demo/node_modules/debug/ 0000755 0001750 0000144 00000000000 14067647700 017530 5 ustar andreh users apollo-server-demo/node_modules/debug/karma.conf.js 0000644 0001750 0000144 00000003310 13120350727 022067 0 ustar andreh users // Karma configuration // Generated on Fri Dec 16 2016 13:09:51 GMT+0000 (UTC) module.exports = function(config) { config.set({ // base path that will be used to resolve all patterns (eg. files, exclude) basePath: '', // frameworks to use // available frameworks: https://npmjs.org/browse/keyword/karma-adapter frameworks: ['mocha', 'chai', 'sinon'], // list of files / patterns to load in the browser files: [ 'dist/debug.js', 'test/*spec.js' ], // list of files to exclude exclude: [ 'src/node.js' ], // preprocess matching files before serving them to the browser // available preprocessors: https://npmjs.org/browse/keyword/karma-preprocessor preprocessors: { }, // test results reporter to use // possible values: 'dots', 'progress' // available reporters: https://npmjs.org/browse/keyword/karma-reporter reporters: ['progress'], // web server port port: 9876, // enable / disable colors in the output (reporters and logs) colors: true, // level of logging // possible values: config.LOG_DISABLE || config.LOG_ERROR || config.LOG_WARN || config.LOG_INFO || config.LOG_DEBUG logLevel: config.LOG_INFO, // enable / disable watching file and executing tests whenever any file changes autoWatch: true, // start these browsers // available browser launchers: https://npmjs.org/browse/keyword/karma-launcher browsers: ['PhantomJS'], // Continuous Integration mode // if true, Karma captures browsers, runs the tests and exits singleRun: false, // Concurrency level // how many browser should be started simultaneous concurrency: Infinity }) } apollo-server-demo/node_modules/debug/LICENSE 0000644 0001750 0000144 00000002123 13120350727 020520 0 ustar andreh users (The MIT License) Copyright (c) 2014 TJ Holowaychuk <tj@vision-media.ca> Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the 'Software'), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/debug/src/ 0000755 0001750 0000144 00000000000 14067647700 020317 5 ustar andreh users apollo-server-demo/node_modules/debug/src/browser.js 0000644 0001750 0000144 00000011176 13161210066 022327 0 ustar andreh users /** * This is the web browser implementation of `debug()`. * * Expose `debug()` as the module. */ exports = module.exports = require('./debug'); exports.log = log; exports.formatArgs = formatArgs; exports.save = save; exports.load = load; exports.useColors = useColors; exports.storage = 'undefined' != typeof chrome && 'undefined' != typeof chrome.storage ? chrome.storage.local : localstorage(); /** * Colors. */ exports.colors = [ 'lightseagreen', 'forestgreen', 'goldenrod', 'dodgerblue', 'darkorchid', 'crimson' ]; /** * Currently only WebKit-based Web Inspectors, Firefox >= v31, * and the Firebug extension (any Firefox version) are known * to support "%c" CSS customizations. * * TODO: add a `localStorage` variable to explicitly enable/disable colors */ function useColors() { // NB: In an Electron preload script, document will be defined but not fully // initialized. Since we know we're in Chrome, we'll just detect this case // explicitly if (typeof window !== 'undefined' && window.process && window.process.type === 'renderer') { return true; } // is webkit? http://stackoverflow.com/a/16459606/376773 // document is undefined in react-native: https://github.com/facebook/react-native/pull/1632 return (typeof document !== 'undefined' && document.documentElement && document.documentElement.style && document.documentElement.style.WebkitAppearance) || // is firebug? http://stackoverflow.com/a/398120/376773 (typeof window !== 'undefined' && window.console && (window.console.firebug || (window.console.exception && window.console.table))) || // is firefox >= v31? // https://developer.mozilla.org/en-US/docs/Tools/Web_Console#Styling_messages (typeof navigator !== 'undefined' && navigator.userAgent && navigator.userAgent.toLowerCase().match(/firefox\/(\d+)/) && parseInt(RegExp.$1, 10) >= 31) || // double check webkit in userAgent just in case we are in a worker (typeof navigator !== 'undefined' && navigator.userAgent && navigator.userAgent.toLowerCase().match(/applewebkit\/(\d+)/)); } /** * Map %j to `JSON.stringify()`, since no Web Inspectors do that by default. */ exports.formatters.j = function(v) { try { return JSON.stringify(v); } catch (err) { return '[UnexpectedJSONParseError]: ' + err.message; } }; /** * Colorize log arguments if enabled. * * @api public */ function formatArgs(args) { var useColors = this.useColors; args[0] = (useColors ? '%c' : '') + this.namespace + (useColors ? ' %c' : ' ') + args[0] + (useColors ? '%c ' : ' ') + '+' + exports.humanize(this.diff); if (!useColors) return; var c = 'color: ' + this.color; args.splice(1, 0, c, 'color: inherit') // the final "%c" is somewhat tricky, because there could be other // arguments passed either before or after the %c, so we need to // figure out the correct index to insert the CSS into var index = 0; var lastC = 0; args[0].replace(/%[a-zA-Z%]/g, function(match) { if ('%%' === match) return; index++; if ('%c' === match) { // we only are interested in the *last* %c // (the user may have provided their own) lastC = index; } }); args.splice(lastC, 0, c); } /** * Invokes `console.log()` when available. * No-op when `console.log` is not a "function". * * @api public */ function log() { // this hackery is required for IE8/9, where // the `console.log` function doesn't have 'apply' return 'object' === typeof console && console.log && Function.prototype.apply.call(console.log, console, arguments); } /** * Save `namespaces`. * * @param {String} namespaces * @api private */ function save(namespaces) { try { if (null == namespaces) { exports.storage.removeItem('debug'); } else { exports.storage.debug = namespaces; } } catch(e) {} } /** * Load `namespaces`. * * @return {String} returns the previously persisted debug modes * @api private */ function load() { var r; try { r = exports.storage.debug; } catch(e) {} // If debug isn't set in LS, and we're in Electron, try to load $DEBUG if (!r && typeof process !== 'undefined' && 'env' in process) { r = process.env.DEBUG; } return r; } /** * Enable namespaces listed in `localStorage.debug` initially. */ exports.enable(load()); /** * Localstorage attempts to return the localstorage. * * This is necessary because safari throws * when a user disables cookies/localstorage * and you attempt to access it. * * @return {LocalStorage} * @api private */ function localstorage() { try { return window.localStorage; } catch (e) {} } apollo-server-demo/node_modules/debug/src/index.js 0000644 0001750 0000144 00000000407 13161210066 021746 0 ustar andreh users /** * Detect Electron renderer process, which is node, but we should * treat as a browser. */ if (typeof process !== 'undefined' && process.type === 'renderer') { module.exports = require('./browser.js'); } else { module.exports = require('./node.js'); } apollo-server-demo/node_modules/debug/src/debug.js 0000644 0001750 0000144 00000010452 13161210066 021726 0 ustar andreh users /** * This is the common logic for both the Node.js and web browser * implementations of `debug()`. * * Expose `debug()` as the module. */ exports = module.exports = createDebug.debug = createDebug['default'] = createDebug; exports.coerce = coerce; exports.disable = disable; exports.enable = enable; exports.enabled = enabled; exports.humanize = require('ms'); /** * The currently active debug mode names, and names to skip. */ exports.names = []; exports.skips = []; /** * Map of special "%n" handling functions, for the debug "format" argument. * * Valid key names are a single, lower or upper-case letter, i.e. "n" and "N". */ exports.formatters = {}; /** * Previous log timestamp. */ var prevTime; /** * Select a color. * @param {String} namespace * @return {Number} * @api private */ function selectColor(namespace) { var hash = 0, i; for (i in namespace) { hash = ((hash << 5) - hash) + namespace.charCodeAt(i); hash |= 0; // Convert to 32bit integer } return exports.colors[Math.abs(hash) % exports.colors.length]; } /** * Create a debugger with the given `namespace`. * * @param {String} namespace * @return {Function} * @api public */ function createDebug(namespace) { function debug() { // disabled? if (!debug.enabled) return; var self = debug; // set `diff` timestamp var curr = +new Date(); var ms = curr - (prevTime || curr); self.diff = ms; self.prev = prevTime; self.curr = curr; prevTime = curr; // turn the `arguments` into a proper Array var args = new Array(arguments.length); for (var i = 0; i < args.length; i++) { args[i] = arguments[i]; } args[0] = exports.coerce(args[0]); if ('string' !== typeof args[0]) { // anything else let's inspect with %O args.unshift('%O'); } // apply any `formatters` transformations var index = 0; args[0] = args[0].replace(/%([a-zA-Z%])/g, function(match, format) { // if we encounter an escaped % then don't increase the array index if (match === '%%') return match; index++; var formatter = exports.formatters[format]; if ('function' === typeof formatter) { var val = args[index]; match = formatter.call(self, val); // now we need to remove `args[index]` since it's inlined in the `format` args.splice(index, 1); index--; } return match; }); // apply env-specific formatting (colors, etc.) exports.formatArgs.call(self, args); var logFn = debug.log || exports.log || console.log.bind(console); logFn.apply(self, args); } debug.namespace = namespace; debug.enabled = exports.enabled(namespace); debug.useColors = exports.useColors(); debug.color = selectColor(namespace); // env-specific initialization logic for debug instances if ('function' === typeof exports.init) { exports.init(debug); } return debug; } /** * Enables a debug mode by namespaces. This can include modes * separated by a colon and wildcards. * * @param {String} namespaces * @api public */ function enable(namespaces) { exports.save(namespaces); exports.names = []; exports.skips = []; var split = (typeof namespaces === 'string' ? namespaces : '').split(/[\s,]+/); var len = split.length; for (var i = 0; i < len; i++) { if (!split[i]) continue; // ignore empty strings namespaces = split[i].replace(/\*/g, '.*?'); if (namespaces[0] === '-') { exports.skips.push(new RegExp('^' + namespaces.substr(1) + '$')); } else { exports.names.push(new RegExp('^' + namespaces + '$')); } } } /** * Disable debug output. * * @api public */ function disable() { exports.enable(''); } /** * Returns true if the given mode name is enabled, false otherwise. * * @param {String} name * @return {Boolean} * @api public */ function enabled(name) { var i, len; for (i = 0, len = exports.skips.length; i < len; i++) { if (exports.skips[i].test(name)) { return false; } } for (i = 0, len = exports.names.length; i < len; i++) { if (exports.names[i].test(name)) { return true; } } return false; } /** * Coerce `val`. * * @param {Mixed} val * @return {Mixed} * @api private */ function coerce(val) { if (val instanceof Error) return val.stack || val.message; return val; } apollo-server-demo/node_modules/debug/src/node.js 0000644 0001750 0000144 00000013577 13161210274 021601 0 ustar andreh users /** * Module dependencies. */ var tty = require('tty'); var util = require('util'); /** * This is the Node.js implementation of `debug()`. * * Expose `debug()` as the module. */ exports = module.exports = require('./debug'); exports.init = init; exports.log = log; exports.formatArgs = formatArgs; exports.save = save; exports.load = load; exports.useColors = useColors; /** * Colors. */ exports.colors = [6, 2, 3, 4, 5, 1]; /** * Build up the default `inspectOpts` object from the environment variables. * * $ DEBUG_COLORS=no DEBUG_DEPTH=10 DEBUG_SHOW_HIDDEN=enabled node script.js */ exports.inspectOpts = Object.keys(process.env).filter(function (key) { return /^debug_/i.test(key); }).reduce(function (obj, key) { // camel-case var prop = key .substring(6) .toLowerCase() .replace(/_([a-z])/g, function (_, k) { return k.toUpperCase() }); // coerce string value into JS value var val = process.env[key]; if (/^(yes|on|true|enabled)$/i.test(val)) val = true; else if (/^(no|off|false|disabled)$/i.test(val)) val = false; else if (val === 'null') val = null; else val = Number(val); obj[prop] = val; return obj; }, {}); /** * The file descriptor to write the `debug()` calls to. * Set the `DEBUG_FD` env variable to override with another value. i.e.: * * $ DEBUG_FD=3 node script.js 3>debug.log */ var fd = parseInt(process.env.DEBUG_FD, 10) || 2; if (1 !== fd && 2 !== fd) { util.deprecate(function(){}, 'except for stderr(2) and stdout(1), any other usage of DEBUG_FD is deprecated. Override debug.log if you want to use a different log function (https://git.io/debug_fd)')() } var stream = 1 === fd ? process.stdout : 2 === fd ? process.stderr : createWritableStdioStream(fd); /** * Is stdout a TTY? Colored output is enabled when `true`. */ function useColors() { return 'colors' in exports.inspectOpts ? Boolean(exports.inspectOpts.colors) : tty.isatty(fd); } /** * Map %o to `util.inspect()`, all on a single line. */ exports.formatters.o = function(v) { this.inspectOpts.colors = this.useColors; return util.inspect(v, this.inspectOpts) .split('\n').map(function(str) { return str.trim() }).join(' '); }; /** * Map %o to `util.inspect()`, allowing multiple lines if needed. */ exports.formatters.O = function(v) { this.inspectOpts.colors = this.useColors; return util.inspect(v, this.inspectOpts); }; /** * Adds ANSI color escape codes if enabled. * * @api public */ function formatArgs(args) { var name = this.namespace; var useColors = this.useColors; if (useColors) { var c = this.color; var prefix = ' \u001b[3' + c + ';1m' + name + ' ' + '\u001b[0m'; args[0] = prefix + args[0].split('\n').join('\n' + prefix); args.push('\u001b[3' + c + 'm+' + exports.humanize(this.diff) + '\u001b[0m'); } else { args[0] = new Date().toUTCString() + ' ' + name + ' ' + args[0]; } } /** * Invokes `util.format()` with the specified arguments and writes to `stream`. */ function log() { return stream.write(util.format.apply(util, arguments) + '\n'); } /** * Save `namespaces`. * * @param {String} namespaces * @api private */ function save(namespaces) { if (null == namespaces) { // If you set a process.env field to null or undefined, it gets cast to the // string 'null' or 'undefined'. Just delete instead. delete process.env.DEBUG; } else { process.env.DEBUG = namespaces; } } /** * Load `namespaces`. * * @return {String} returns the previously persisted debug modes * @api private */ function load() { return process.env.DEBUG; } /** * Copied from `node/src/node.js`. * * XXX: It's lame that node doesn't expose this API out-of-the-box. It also * relies on the undocumented `tty_wrap.guessHandleType()` which is also lame. */ function createWritableStdioStream (fd) { var stream; var tty_wrap = process.binding('tty_wrap'); // Note stream._type is used for test-module-load-list.js switch (tty_wrap.guessHandleType(fd)) { case 'TTY': stream = new tty.WriteStream(fd); stream._type = 'tty'; // Hack to have stream not keep the event loop alive. // See https://github.com/joyent/node/issues/1726 if (stream._handle && stream._handle.unref) { stream._handle.unref(); } break; case 'FILE': var fs = require('fs'); stream = new fs.SyncWriteStream(fd, { autoClose: false }); stream._type = 'fs'; break; case 'PIPE': case 'TCP': var net = require('net'); stream = new net.Socket({ fd: fd, readable: false, writable: true }); // FIXME Should probably have an option in net.Socket to create a // stream from an existing fd which is writable only. But for now // we'll just add this hack and set the `readable` member to false. // Test: ./node test/fixtures/echo.js < /etc/passwd stream.readable = false; stream.read = null; stream._type = 'pipe'; // FIXME Hack to have stream not keep the event loop alive. // See https://github.com/joyent/node/issues/1726 if (stream._handle && stream._handle.unref) { stream._handle.unref(); } break; default: // Probably an error on in uv_guess_handle() throw new Error('Implement me. Unknown stream file type!'); } // For supporting legacy API we put the FD here. stream.fd = fd; stream._isStdio = true; return stream; } /** * Init logic for `debug` instances. * * Create a new `inspectOpts` object in case `useColors` is set * differently for a particular `debug` instance. */ function init (debug) { debug.inspectOpts = {}; var keys = Object.keys(exports.inspectOpts); for (var i = 0; i < keys.length; i++) { debug.inspectOpts[keys[i]] = exports.inspectOpts[keys[i]]; } } /** * Enable namespaces listed in `process.env.DEBUG` initially. */ exports.enable(load()); apollo-server-demo/node_modules/debug/src/inspector-log.js 0000644 0001750 0000144 00000000565 13160777500 023443 0 ustar andreh users module.exports = inspectorLog; // black hole const nullStream = new (require('stream').Writable)(); nullStream._write = () => {}; /** * Outputs a `console.log()` to the Node.js Inspector console *only*. */ function inspectorLog() { const stdout = console._stdout; console._stdout = nullStream; console.log.apply(console, arguments); console._stdout = stdout; } apollo-server-demo/node_modules/debug/.coveralls.yml 0000644 0001750 0000144 00000000056 13120350727 022311 0 ustar andreh users repo_token: SIAeZjKYlHK74rbcFvNHMUzjRiMpflxve apollo-server-demo/node_modules/debug/.eslintrc 0000644 0001750 0000144 00000000264 13161210066 021337 0 ustar andreh users { "env": { "browser": true, "node": true }, "rules": { "no-console": 0, "no-empty": [1, { "allowEmptyCatch": true }] }, "extends": "eslint:recommended" } apollo-server-demo/node_modules/debug/README.md 0000644 0001750 0000144 00000042776 13161210066 021010 0 ustar andreh users # debug [](https://travis-ci.org/visionmedia/debug) [](https://coveralls.io/github/visionmedia/debug?branch=master) [](https://visionmedia-community-slackin.now.sh/) [](#backers) [](#sponsors) A tiny node.js debugging utility modelled after node core's debugging technique. **Discussion around the V3 API is under way [here](https://github.com/visionmedia/debug/issues/370)** ## Installation ```bash $ npm install debug ``` ## Usage `debug` exposes a function; simply pass this function the name of your module, and it will return a decorated version of `console.error` for you to pass debug statements to. This will allow you to toggle the debug output for different parts of your module as well as the module as a whole. Example _app.js_: ```js var debug = require('debug')('http') , http = require('http') , name = 'My App'; // fake app debug('booting %s', name); http.createServer(function(req, res){ debug(req.method + ' ' + req.url); res.end('hello\n'); }).listen(3000, function(){ debug('listening'); }); // fake worker of some kind require('./worker'); ``` Example _worker.js_: ```js var debug = require('debug')('worker'); setInterval(function(){ debug('doing some work'); }, 1000); ``` The __DEBUG__ environment variable is then used to enable these based on space or comma-delimited names. Here are some examples:   #### Windows note On Windows the environment variable is set using the `set` command. ```cmd set DEBUG=*,-not_this ``` Note that PowerShell uses different syntax to set environment variables. ```cmd $env:DEBUG = "*,-not_this" ``` Then, run the program to be debugged as usual. ## Millisecond diff When actively developing an application it can be useful to see when the time spent between one `debug()` call and the next. Suppose for example you invoke `debug()` before requesting a resource, and after as well, the "+NNNms" will show you how much time was spent between calls.  When stdout is not a TTY, `Date#toUTCString()` is used, making it more useful for logging the debug information as shown below:  ## Conventions If you're using this in one or more of your libraries, you _should_ use the name of your library so that developers may toggle debugging as desired without guessing names. If you have more than one debuggers you _should_ prefix them with your library name and use ":" to separate features. For example "bodyParser" from Connect would then be "connect:bodyParser". ## Wildcards The `*` character may be used as a wildcard. Suppose for example your library has debuggers named "connect:bodyParser", "connect:compress", "connect:session", instead of listing all three with `DEBUG=connect:bodyParser,connect:compress,connect:session`, you may simply do `DEBUG=connect:*`, or to run everything using this module simply use `DEBUG=*`. You can also exclude specific debuggers by prefixing them with a "-" character. For example, `DEBUG=*,-connect:*` would include all debuggers except those starting with "connect:". ## Environment Variables When running through Node.js, you can set a few environment variables that will change the behavior of the debug logging: | Name | Purpose | |-----------|-------------------------------------------------| | `DEBUG` | Enables/disables specific debugging namespaces. | | `DEBUG_COLORS`| Whether or not to use colors in the debug output. | | `DEBUG_DEPTH` | Object inspection depth. | | `DEBUG_SHOW_HIDDEN` | Shows hidden properties on inspected objects. | __Note:__ The environment variables beginning with `DEBUG_` end up being converted into an Options object that gets used with `%o`/`%O` formatters. See the Node.js documentation for [`util.inspect()`](https://nodejs.org/api/util.html#util_util_inspect_object_options) for the complete list. ## Formatters Debug uses [printf-style](https://wikipedia.org/wiki/Printf_format_string) formatting. Below are the officially supported formatters: | Formatter | Representation | |-----------|----------------| | `%O` | Pretty-print an Object on multiple lines. | | `%o` | Pretty-print an Object all on a single line. | | `%s` | String. | | `%d` | Number (both integer and float). | | `%j` | JSON. Replaced with the string '[Circular]' if the argument contains circular references. | | `%%` | Single percent sign ('%'). This does not consume an argument. | ### Custom formatters You can add custom formatters by extending the `debug.formatters` object. For example, if you wanted to add support for rendering a Buffer as hex with `%h`, you could do something like: ```js const createDebug = require('debug') createDebug.formatters.h = (v) => { return v.toString('hex') } // …elsewhere const debug = createDebug('foo') debug('this is hex: %h', new Buffer('hello world')) // foo this is hex: 68656c6c6f20776f726c6421 +0ms ``` ## Browser support You can build a browser-ready script using [browserify](https://github.com/substack/node-browserify), or just use the [browserify-as-a-service](https://wzrd.in/) [build](https://wzrd.in/standalone/debug@latest), if you don't want to build it yourself. Debug's enable state is currently persisted by `localStorage`. Consider the situation shown below where you have `worker:a` and `worker:b`, and wish to debug both. You can enable this using `localStorage.debug`: ```js localStorage.debug = 'worker:*' ``` And then refresh the page. ```js a = debug('worker:a'); b = debug('worker:b'); setInterval(function(){ a('doing some work'); }, 1000); setInterval(function(){ b('doing some work'); }, 1200); ``` #### Web Inspector Colors Colors are also enabled on "Web Inspectors" that understand the `%c` formatting option. These are WebKit web inspectors, Firefox ([since version 31](https://hacks.mozilla.org/2014/05/editable-box-model-multiple-selection-sublime-text-keys-much-more-firefox-developer-tools-episode-31/)) and the Firebug plugin for Firefox (any version). Colored output looks something like:  ## Output streams By default `debug` will log to stderr, however this can be configured per-namespace by overriding the `log` method: Example _stdout.js_: ```js var debug = require('debug'); var error = debug('app:error'); // by default stderr is used error('goes to stderr!'); var log = debug('app:log'); // set this namespace to log via console.log log.log = console.log.bind(console); // don't forget to bind to console! log('goes to stdout'); error('still goes to stderr!'); // set all output to go via console.info // overrides all per-namespace log settings debug.log = console.info.bind(console); error('now goes to stdout via console.info'); log('still goes to stdout, but via console.info now'); ``` ## Authors - TJ Holowaychuk - Nathan Rajlich - Andrew Rhyne ## Backers Support us with a monthly donation and help us continue our activities. [[Become a backer](https://opencollective.com/debug#backer)] <a href="https://opencollective.com/debug/backer/0/website" target="_blank"><img src="https://opencollective.com/debug/backer/0/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/1/website" target="_blank"><img src="https://opencollective.com/debug/backer/1/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/2/website" target="_blank"><img src="https://opencollective.com/debug/backer/2/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/3/website" target="_blank"><img src="https://opencollective.com/debug/backer/3/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/4/website" target="_blank"><img src="https://opencollective.com/debug/backer/4/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/5/website" target="_blank"><img src="https://opencollective.com/debug/backer/5/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/6/website" target="_blank"><img src="https://opencollective.com/debug/backer/6/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/7/website" target="_blank"><img src="https://opencollective.com/debug/backer/7/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/8/website" target="_blank"><img src="https://opencollective.com/debug/backer/8/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/9/website" target="_blank"><img src="https://opencollective.com/debug/backer/9/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/10/website" target="_blank"><img src="https://opencollective.com/debug/backer/10/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/11/website" target="_blank"><img src="https://opencollective.com/debug/backer/11/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/12/website" target="_blank"><img src="https://opencollective.com/debug/backer/12/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/13/website" target="_blank"><img src="https://opencollective.com/debug/backer/13/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/14/website" target="_blank"><img src="https://opencollective.com/debug/backer/14/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/15/website" target="_blank"><img src="https://opencollective.com/debug/backer/15/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/16/website" target="_blank"><img src="https://opencollective.com/debug/backer/16/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/17/website" target="_blank"><img src="https://opencollective.com/debug/backer/17/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/18/website" target="_blank"><img src="https://opencollective.com/debug/backer/18/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/19/website" target="_blank"><img src="https://opencollective.com/debug/backer/19/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/20/website" target="_blank"><img src="https://opencollective.com/debug/backer/20/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/21/website" target="_blank"><img src="https://opencollective.com/debug/backer/21/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/22/website" target="_blank"><img src="https://opencollective.com/debug/backer/22/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/23/website" target="_blank"><img src="https://opencollective.com/debug/backer/23/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/24/website" target="_blank"><img src="https://opencollective.com/debug/backer/24/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/25/website" target="_blank"><img src="https://opencollective.com/debug/backer/25/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/26/website" target="_blank"><img src="https://opencollective.com/debug/backer/26/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/27/website" target="_blank"><img src="https://opencollective.com/debug/backer/27/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/28/website" target="_blank"><img src="https://opencollective.com/debug/backer/28/avatar.svg"></a> <a href="https://opencollective.com/debug/backer/29/website" target="_blank"><img src="https://opencollective.com/debug/backer/29/avatar.svg"></a> ## Sponsors Become a sponsor and get your logo on our README on Github with a link to your site. [[Become a sponsor](https://opencollective.com/debug#sponsor)] <a href="https://opencollective.com/debug/sponsor/0/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/0/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/1/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/1/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/2/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/2/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/3/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/3/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/4/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/4/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/5/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/5/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/6/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/6/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/7/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/7/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/8/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/8/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/9/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/9/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/10/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/10/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/11/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/11/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/12/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/12/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/13/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/13/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/14/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/14/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/15/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/15/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/16/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/16/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/17/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/17/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/18/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/18/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/19/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/19/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/20/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/20/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/21/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/21/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/22/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/22/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/23/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/23/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/24/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/24/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/25/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/25/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/26/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/26/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/27/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/27/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/28/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/28/avatar.svg"></a> <a href="https://opencollective.com/debug/sponsor/29/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/29/avatar.svg"></a> ## License (The MIT License) Copyright (c) 2014-2016 TJ Holowaychuk <tj@vision-media.ca> Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the 'Software'), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/debug/node.js 0000644 0001750 0000144 00000000050 13120350727 020773 0 ustar andreh users module.exports = require('./src/node'); apollo-server-demo/node_modules/debug/package.json 0000644 0001750 0000144 00000002476 13161210331 022003 0 ustar andreh users { "name": "debug", "version": "2.6.9", "repository": { "type": "git", "url": "git://github.com/visionmedia/debug.git" }, "description": "small debugging utility", "keywords": [ "debug", "log", "debugger" ], "author": "TJ Holowaychuk <tj@vision-media.ca>", "contributors": [ "Nathan Rajlich <nathan@tootallnate.net> (http://n8.io)", "Andrew Rhyne <rhyneandrew@gmail.com>" ], "license": "MIT", "dependencies": { "ms": "2.0.0" }, "devDependencies": { "browserify": "9.0.3", "chai": "^3.5.0", "concurrently": "^3.1.0", "coveralls": "^2.11.15", "eslint": "^3.12.1", "istanbul": "^0.4.5", "karma": "^1.3.0", "karma-chai": "^0.1.0", "karma-mocha": "^1.3.0", "karma-phantomjs-launcher": "^1.0.2", "karma-sinon": "^1.0.5", "mocha": "^3.2.0", "mocha-lcov-reporter": "^1.2.0", "rimraf": "^2.5.4", "sinon": "^1.17.6", "sinon-chai": "^2.8.0" }, "main": "./src/index.js", "browser": "./src/browser.js", "component": { "scripts": { "debug/index.js": "browser.js", "debug/debug.js": "debug.js" } } ,"_resolved": "https://registry.npmjs.org/debug/-/debug-2.6.9.tgz" ,"_integrity": "sha512-bC7ElrdJaJnPbAP+1EotYvqZsb3ecl5wi6Bfi6BJTUcNowp6cvspg0jXznRTKDjm/E7AdgFBVeAPVMNcKGsHMA==" ,"_from": "debug@2.6.9" } apollo-server-demo/node_modules/debug/Makefile 0000644 0001750 0000144 00000002043 13161210066 021150 0 ustar andreh users # get Makefile directory name: http://stackoverflow.com/a/5982798/376773 THIS_MAKEFILE_PATH:=$(word $(words $(MAKEFILE_LIST)),$(MAKEFILE_LIST)) THIS_DIR:=$(shell cd $(dir $(THIS_MAKEFILE_PATH));pwd) # BIN directory BIN := $(THIS_DIR)/node_modules/.bin # Path PATH := node_modules/.bin:$(PATH) SHELL := /bin/bash # applications NODE ?= $(shell which node) YARN ?= $(shell which yarn) PKG ?= $(if $(YARN),$(YARN),$(NODE) $(shell which npm)) BROWSERIFY ?= $(NODE) $(BIN)/browserify .FORCE: install: node_modules node_modules: package.json @NODE_ENV= $(PKG) install @touch node_modules lint: .FORCE eslint browser.js debug.js index.js node.js test-node: .FORCE istanbul cover node_modules/mocha/bin/_mocha -- test/**.js test-browser: .FORCE mkdir -p dist @$(BROWSERIFY) \ --standalone debug \ . > dist/debug.js karma start --single-run rimraf dist test: .FORCE concurrently \ "make test-node" \ "make test-browser" coveralls: cat ./coverage/lcov.info | ./node_modules/coveralls/bin/coveralls.js .PHONY: all install clean distclean apollo-server-demo/node_modules/debug/component.json 0000644 0001750 0000144 00000000501 13161210331 022375 0 ustar andreh users { "name": "debug", "repo": "visionmedia/debug", "description": "small debugging utility", "version": "2.6.9", "keywords": [ "debug", "log", "debugger" ], "main": "src/browser.js", "scripts": [ "src/browser.js", "src/debug.js" ], "dependencies": { "rauchg/ms.js": "0.7.1" } } apollo-server-demo/node_modules/debug/.npmignore 0000644 0001750 0000144 00000000110 13120350727 021504 0 ustar andreh users support test examples example *.sock dist yarn.lock coverage bower.json apollo-server-demo/node_modules/debug/CHANGELOG.md 0000644 0001750 0000144 00000026673 13161210326 021337 0 ustar andreh users 2.6.9 / 2017-09-22 ================== * remove ReDoS regexp in %o formatter (#504) 2.6.8 / 2017-05-18 ================== * Fix: Check for undefined on browser globals (#462, @marbemac) 2.6.7 / 2017-05-16 ================== * Fix: Update ms to 2.0.0 to fix regular expression denial of service vulnerability (#458, @hubdotcom) * Fix: Inline extend function in node implementation (#452, @dougwilson) * Docs: Fix typo (#455, @msasad) 2.6.5 / 2017-04-27 ================== * Fix: null reference check on window.documentElement.style.WebkitAppearance (#447, @thebigredgeek) * Misc: clean up browser reference checks (#447, @thebigredgeek) * Misc: add npm-debug.log to .gitignore (@thebigredgeek) 2.6.4 / 2017-04-20 ================== * Fix: bug that would occure if process.env.DEBUG is a non-string value. (#444, @LucianBuzzo) * Chore: ignore bower.json in npm installations. (#437, @joaovieira) * Misc: update "ms" to v0.7.3 (@tootallnate) 2.6.3 / 2017-03-13 ================== * Fix: Electron reference to `process.env.DEBUG` (#431, @paulcbetts) * Docs: Changelog fix (@thebigredgeek) 2.6.2 / 2017-03-10 ================== * Fix: DEBUG_MAX_ARRAY_LENGTH (#420, @slavaGanzin) * Docs: Add backers and sponsors from Open Collective (#422, @piamancini) * Docs: Add Slackin invite badge (@tootallnate) 2.6.1 / 2017-02-10 ================== * Fix: Module's `export default` syntax fix for IE8 `Expected identifier` error * Fix: Whitelist DEBUG_FD for values 1 and 2 only (#415, @pi0) * Fix: IE8 "Expected identifier" error (#414, @vgoma) * Fix: Namespaces would not disable once enabled (#409, @musikov) 2.6.0 / 2016-12-28 ================== * Fix: added better null pointer checks for browser useColors (@thebigredgeek) * Improvement: removed explicit `window.debug` export (#404, @tootallnate) * Improvement: deprecated `DEBUG_FD` environment variable (#405, @tootallnate) 2.5.2 / 2016-12-25 ================== * Fix: reference error on window within webworkers (#393, @KlausTrainer) * Docs: fixed README typo (#391, @lurch) * Docs: added notice about v3 api discussion (@thebigredgeek) 2.5.1 / 2016-12-20 ================== * Fix: babel-core compatibility 2.5.0 / 2016-12-20 ================== * Fix: wrong reference in bower file (@thebigredgeek) * Fix: webworker compatibility (@thebigredgeek) * Fix: output formatting issue (#388, @kribblo) * Fix: babel-loader compatibility (#383, @escwald) * Misc: removed built asset from repo and publications (@thebigredgeek) * Misc: moved source files to /src (#378, @yamikuronue) * Test: added karma integration and replaced babel with browserify for browser tests (#378, @yamikuronue) * Test: coveralls integration (#378, @yamikuronue) * Docs: simplified language in the opening paragraph (#373, @yamikuronue) 2.4.5 / 2016-12-17 ================== * Fix: `navigator` undefined in Rhino (#376, @jochenberger) * Fix: custom log function (#379, @hsiliev) * Improvement: bit of cleanup + linting fixes (@thebigredgeek) * Improvement: rm non-maintainted `dist/` dir (#375, @freewil) * Docs: simplified language in the opening paragraph. (#373, @yamikuronue) 2.4.4 / 2016-12-14 ================== * Fix: work around debug being loaded in preload scripts for electron (#368, @paulcbetts) 2.4.3 / 2016-12-14 ================== * Fix: navigation.userAgent error for react native (#364, @escwald) 2.4.2 / 2016-12-14 ================== * Fix: browser colors (#367, @tootallnate) * Misc: travis ci integration (@thebigredgeek) * Misc: added linting and testing boilerplate with sanity check (@thebigredgeek) 2.4.1 / 2016-12-13 ================== * Fix: typo that broke the package (#356) 2.4.0 / 2016-12-13 ================== * Fix: bower.json references unbuilt src entry point (#342, @justmatt) * Fix: revert "handle regex special characters" (@tootallnate) * Feature: configurable util.inspect()`options for NodeJS (#327, @tootallnate) * Feature: %O`(big O) pretty-prints objects (#322, @tootallnate) * Improvement: allow colors in workers (#335, @botverse) * Improvement: use same color for same namespace. (#338, @lchenay) 2.3.3 / 2016-11-09 ================== * Fix: Catch `JSON.stringify()` errors (#195, Jovan Alleyne) * Fix: Returning `localStorage` saved values (#331, Levi Thomason) * Improvement: Don't create an empty object when no `process` (Nathan Rajlich) 2.3.2 / 2016-11-09 ================== * Fix: be super-safe in index.js as well (@TooTallNate) * Fix: should check whether process exists (Tom Newby) 2.3.1 / 2016-11-09 ================== * Fix: Added electron compatibility (#324, @paulcbetts) * Improvement: Added performance optimizations (@tootallnate) * Readme: Corrected PowerShell environment variable example (#252, @gimre) * Misc: Removed yarn lock file from source control (#321, @fengmk2) 2.3.0 / 2016-11-07 ================== * Fix: Consistent placement of ms diff at end of output (#215, @gorangajic) * Fix: Escaping of regex special characters in namespace strings (#250, @zacronos) * Fix: Fixed bug causing crash on react-native (#282, @vkarpov15) * Feature: Enabled ES6+ compatible import via default export (#212 @bucaran) * Feature: Added %O formatter to reflect Chrome's console.log capability (#279, @oncletom) * Package: Update "ms" to 0.7.2 (#315, @DevSide) * Package: removed superfluous version property from bower.json (#207 @kkirsche) * Readme: fix USE_COLORS to DEBUG_COLORS * Readme: Doc fixes for format string sugar (#269, @mlucool) * Readme: Updated docs for DEBUG_FD and DEBUG_COLORS environment variables (#232, @mattlyons0) * Readme: doc fixes for PowerShell (#271 #243, @exoticknight @unreadable) * Readme: better docs for browser support (#224, @matthewmueller) * Tooling: Added yarn integration for development (#317, @thebigredgeek) * Misc: Renamed History.md to CHANGELOG.md (@thebigredgeek) * Misc: Added license file (#226 #274, @CantemoInternal @sdaitzman) * Misc: Updated contributors (@thebigredgeek) 2.2.0 / 2015-05-09 ================== * package: update "ms" to v0.7.1 (#202, @dougwilson) * README: add logging to file example (#193, @DanielOchoa) * README: fixed a typo (#191, @amir-s) * browser: expose `storage` (#190, @stephenmathieson) * Makefile: add a `distclean` target (#189, @stephenmathieson) 2.1.3 / 2015-03-13 ================== * Updated stdout/stderr example (#186) * Updated example/stdout.js to match debug current behaviour * Renamed example/stderr.js to stdout.js * Update Readme.md (#184) * replace high intensity foreground color for bold (#182, #183) 2.1.2 / 2015-03-01 ================== * dist: recompile * update "ms" to v0.7.0 * package: update "browserify" to v9.0.3 * component: fix "ms.js" repo location * changed bower package name * updated documentation about using debug in a browser * fix: security error on safari (#167, #168, @yields) 2.1.1 / 2014-12-29 ================== * browser: use `typeof` to check for `console` existence * browser: check for `console.log` truthiness (fix IE 8/9) * browser: add support for Chrome apps * Readme: added Windows usage remarks * Add `bower.json` to properly support bower install 2.1.0 / 2014-10-15 ================== * node: implement `DEBUG_FD` env variable support * package: update "browserify" to v6.1.0 * package: add "license" field to package.json (#135, @panuhorsmalahti) 2.0.0 / 2014-09-01 ================== * package: update "browserify" to v5.11.0 * node: use stderr rather than stdout for logging (#29, @stephenmathieson) 1.0.4 / 2014-07-15 ================== * dist: recompile * example: remove `console.info()` log usage * example: add "Content-Type" UTF-8 header to browser example * browser: place %c marker after the space character * browser: reset the "content" color via `color: inherit` * browser: add colors support for Firefox >= v31 * debug: prefer an instance `log()` function over the global one (#119) * Readme: update documentation about styled console logs for FF v31 (#116, @wryk) 1.0.3 / 2014-07-09 ================== * Add support for multiple wildcards in namespaces (#122, @seegno) * browser: fix lint 1.0.2 / 2014-06-10 ================== * browser: update color palette (#113, @gscottolson) * common: make console logging function configurable (#108, @timoxley) * node: fix %o colors on old node <= 0.8.x * Makefile: find node path using shell/which (#109, @timoxley) 1.0.1 / 2014-06-06 ================== * browser: use `removeItem()` to clear localStorage * browser, node: don't set DEBUG if namespaces is undefined (#107, @leedm777) * package: add "contributors" section * node: fix comment typo * README: list authors 1.0.0 / 2014-06-04 ================== * make ms diff be global, not be scope * debug: ignore empty strings in enable() * node: make DEBUG_COLORS able to disable coloring * *: export the `colors` array * npmignore: don't publish the `dist` dir * Makefile: refactor to use browserify * package: add "browserify" as a dev dependency * Readme: add Web Inspector Colors section * node: reset terminal color for the debug content * node: map "%o" to `util.inspect()` * browser: map "%j" to `JSON.stringify()` * debug: add custom "formatters" * debug: use "ms" module for humanizing the diff * Readme: add "bash" syntax highlighting * browser: add Firebug color support * browser: add colors for WebKit browsers * node: apply log to `console` * rewrite: abstract common logic for Node & browsers * add .jshintrc file 0.8.1 / 2014-04-14 ================== * package: re-add the "component" section 0.8.0 / 2014-03-30 ================== * add `enable()` method for nodejs. Closes #27 * change from stderr to stdout * remove unnecessary index.js file 0.7.4 / 2013-11-13 ================== * remove "browserify" key from package.json (fixes something in browserify) 0.7.3 / 2013-10-30 ================== * fix: catch localStorage security error when cookies are blocked (Chrome) * add debug(err) support. Closes #46 * add .browser prop to package.json. Closes #42 0.7.2 / 2013-02-06 ================== * fix package.json * fix: Mobile Safari (private mode) is broken with debug * fix: Use unicode to send escape character to shell instead of octal to work with strict mode javascript 0.7.1 / 2013-02-05 ================== * add repository URL to package.json * add DEBUG_COLORED to force colored output * add browserify support * fix component. Closes #24 0.7.0 / 2012-05-04 ================== * Added .component to package.json * Added debug.component.js build 0.6.0 / 2012-03-16 ================== * Added support for "-" prefix in DEBUG [Vinay Pulim] * Added `.enabled` flag to the node version [TooTallNate] 0.5.0 / 2012-02-02 ================== * Added: humanize diffs. Closes #8 * Added `debug.disable()` to the CS variant * Removed padding. Closes #10 * Fixed: persist client-side variant again. Closes #9 0.4.0 / 2012-02-01 ================== * Added browser variant support for older browsers [TooTallNate] * Added `debug.enable('project:*')` to browser variant [TooTallNate] * Added padding to diff (moved it to the right) 0.3.0 / 2012-01-26 ================== * Added millisecond diff when isatty, otherwise UTC string 0.2.0 / 2012-01-22 ================== * Added wildcard support 0.1.0 / 2011-12-02 ================== * Added: remove colors unless stderr isatty [TooTallNate] 0.0.1 / 2010-01-03 ================== * Initial release apollo-server-demo/node_modules/debug/.travis.yml 0000644 0001750 0000144 00000000214 13161210066 021617 0 ustar andreh users language: node_js node_js: - "6" - "5" - "4" install: - make node_modules script: - make lint - make test - make coveralls apollo-server-demo/node_modules/apollo-link/ 0000755 0001750 0000144 00000000000 14067647700 020663 5 ustar andreh users apollo-server-demo/node_modules/apollo-link/LICENSE 0000644 0001750 0000144 00000002120 03560116604 021651 0 ustar andreh users The MIT License (MIT) Copyright (c) 2016 - 2017 Meteor Development Group, Inc. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/apollo-link/README.md 0000644 0001750 0000144 00000004503 03560116604 022132 0 ustar andreh users # apollo-link ## Purpose `apollo-link` is a standard interface for modifying control flow of GraphQL requests and fetching GraphQL results, designed to provide a simple GraphQL client that is capable of extensions. The targeted use cases of `apollo-link` are highlighted below: * fetch queries directly without normalized cache * network interface for Apollo Client * network interface for Relay Modern * fetcher for Apollo Link is the interface for creating new links in your application. The client sends a request as a method call to a link and can recieve one or more (in the case of subscriptions) responses from the server. The responses are returned using the Observer pattern. 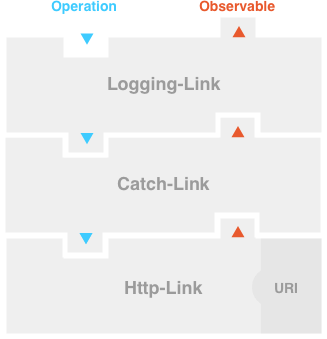 Results from the server can be provided by calling `next(result)` on the observer. In the case of a network/transport error (not a GraphQL Error) the `error(err)` method can be used to indicate a response will not be recieved. If multiple responses are not supported by the link, `complete()` should be called to inform the client no further data will be provided. In the case of an intermediate link, a second argument to `request(operation, forward)` is the link to `forward(operation)` to. `forward` returns an observable and it can be returned directly or subscribed to. ```js forward(operation).subscribe({ next: result => { handleTheResult(result) }, error: error => { handleTheNetworkError(error) }, }); ``` ## Implementing a basic custom transport ```js import { ApolloLink, Observable } from 'apollo-link'; export class CustomApolloLink extends ApolloLink { request(operation /*, forward*/) { //Whether no one is listening anymore let unsubscribed = false; return new Observable(observer => { somehowGetOperationToServer(operation, (error, result) => { if (unsubscribed) return; if (error) { //Network error observer.error(error); } else { observer.next(result); observer.complete(); //If subscriptions not supported } }); function unsubscribe() { unsubscribed = true; } return unsubscribe; }); } } ``` ## Installation `npm install apollo-link --save` apollo-server-demo/node_modules/apollo-link/package.json 0000644 0001750 0000144 00000004333 03560116604 023142 0 ustar andreh users { "name": "apollo-link", "version": "1.2.14", "description": "Flexible, lightweight transport layer for GraphQL", "author": "Evans Hauser <evanshauser@gmail.com>", "contributors": [ "James Baxley <james@meteor.com>", "Jonas Helfer <jonas@helfer.email>", "jon wong <j@jnwng.com>", "Sashko Stubailo <sashko@stubailo.com>" ], "license": "MIT", "main": "./lib/index.js", "module": "./lib/bundle.esm.js", "typings": "./lib/index.d.ts", "sideEffects": false, "repository": { "type": "git", "url": "git+https://github.com/apollographql/apollo-link.git" }, "bugs": { "url": "https://github.com/apollographql/apollo-link/issues" }, "homepage": "https://github.com/apollographql/apollo-link#readme", "scripts": { "build": "tsc && rollup -c", "clean": "rimraf lib/* && rimraf coverage/*", "coverage": "jest --coverage", "filesize": "../../scripts/minify", "lint": "tslint -c \"../../tslint.json\" -p tsconfig.json -c ../../tslint.json src/*.ts", "prebuild": "npm run clean", "prepare": "npm run build", "test": "npm run lint && jest", "watch": "tsc -w -p . & rollup -c -w" }, "dependencies": { "apollo-utilities": "^1.3.0", "ts-invariant": "^0.4.0", "tslib": "^1.9.3", "zen-observable-ts": "^0.8.21" }, "peerDependencies": { "graphql": "^0.11.3 || ^0.12.3 || ^0.13.0 || ^14.0.0 || ^15.0.0" }, "devDependencies": { "@types/graphql": "14.2.3", "@types/jest": "24.9.0", "@types/node": "9.6.55", "graphql": "15.0.0", "graphql-tag": "2.10.1", "jest": "24.9.0", "rimraf": "2.7.1", "rollup": "1.29.1", "ts-jest": "22.4.6", "tslint": "5.20.1", "typescript": "3.0.3" }, "jest": { "transform": { ".(ts|tsx)": "ts-jest" }, "testRegex": "(/__tests__/.*|\\.(test|spec))\\.(ts|tsx|js)$", "moduleFileExtensions": [ "ts", "tsx", "js", "json" ], "testURL": "http://localhost" }, "gitHead": "1012934b4fd9ab436c4fdcd5e9b1bb1e4c1b0d98" ,"_resolved": "https://registry.npmjs.org/apollo-link/-/apollo-link-1.2.14.tgz" ,"_integrity": "sha512-p67CMEFP7kOG1JZ0ZkYZwRDa369w5PIjtMjvrQd/HnIV8FRsHRqLqK+oAZQnFa1DDdZtOtHTi+aMIW6EatC2jg==" ,"_from": "apollo-link@1.2.14" } apollo-server-demo/node_modules/apollo-link/CHANGELOG.md 0000644 0001750 0000144 00000003072 03560116604 022464 0 ustar andreh users # Change log ---- **NOTE:** This changelog is no longer maintained. Changes are now tracked in the top level [`CHANGELOG.md`](https://github.com/apollographql/apollo-link/blob/master/CHANGELOG.md). ---- ### 1.2.4 - No changes ### 1.2.3 - Added `graphql` 14 to peer and dev deps; Updated `@types/graphql` to 14 <br/> [@hwillson](http://github.com/hwillson) in [#789](https://github.com/apollographql/apollo-link/pull/789) ### 1.2.2 - Update apollo-link [#559](https://github.com/apollographql/apollo-link/pull/559) - export graphql types and add @types/graphql as a regular dependency [PR#576](https://github.com/apollographql/apollo-link/pull/576) - moved @types/node to dev dependencies in package.josn to avoid collisions with other projects. [PR#540](https://github.com/apollographql/apollo-link/pull/540) ### 1.2.1 - update apollo link with zen-observable-ts to remove import issues [PR#515](https://github.com/apollographql/apollo-link/pull/515) ### 1.2.0 - Add `fromError` Observable helper - change import method of zen-observable for rollup compat ### 1.1.0 - Expose `#execute` on ApolloLink as static ### 1.0.7 - Update to graphql@0.12 ### 1.0.6 - update rollup ### 1.0.5 - fix bug where context wasn't merged when setting it ### 1.0.4 - export link util helpers ### 1.0.3 - removed requiring query on initial execution check - moved to move efficent rollup build ### 1.0.1, 1.0.2 <!-- never published as latest --> - preleases for dev tool integation ### 0.8.0 - added support for `extensions` on an operation ### 0.7.0 - new operation API and start of changelog apollo-server-demo/node_modules/apollo-link/lib/ 0000755 0001750 0000144 00000000000 14067647700 021431 5 ustar andreh users apollo-server-demo/node_modules/apollo-link/lib/linkUtils.d.ts 0000644 0001750 0000144 00000002061 03560116604 024166 0 ustar andreh users import Observable from 'zen-observable-ts'; import { GraphQLRequest, Operation } from './types'; import { ApolloLink } from './link'; import { getOperationName } from 'apollo-utilities'; export { getOperationName }; export declare function validateOperation(operation: GraphQLRequest): GraphQLRequest; export declare class LinkError extends Error { link: ApolloLink; constructor(message?: string, link?: ApolloLink); } export declare function isTerminating(link: ApolloLink): boolean; export declare function toPromise<R>(observable: Observable<R>): Promise<R>; export declare const makePromise: typeof toPromise; export declare function fromPromise<T>(promise: Promise<T>): Observable<T>; export declare function fromError<T>(errorValue: any): Observable<T>; export declare function transformOperation(operation: GraphQLRequest): GraphQLRequest; export declare function createOperation(starting: any, operation: GraphQLRequest): Operation; export declare function getKey(operation: GraphQLRequest): string; //# sourceMappingURL=linkUtils.d.ts.map apollo-server-demo/node_modules/apollo-link/lib/index.js 0000644 0001750 0000144 00000001221 03560116604 023060 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); var tslib_1 = require("tslib"); tslib_1.__exportStar(require("./link"), exports); var linkUtils_1 = require("./linkUtils"); exports.createOperation = linkUtils_1.createOperation; exports.makePromise = linkUtils_1.makePromise; exports.toPromise = linkUtils_1.toPromise; exports.fromPromise = linkUtils_1.fromPromise; exports.fromError = linkUtils_1.fromError; exports.getOperationName = linkUtils_1.getOperationName; var zen_observable_ts_1 = tslib_1.__importDefault(require("zen-observable-ts")); exports.Observable = zen_observable_ts_1.default; //# sourceMappingURL=index.js.map apollo-server-demo/node_modules/apollo-link/lib/test-utils.d.ts.map 0000644 0001750 0000144 00000000357 03560116604 025107 0 ustar andreh users {"version":3,"file":"test-utils.d.ts","sourceRoot":"","sources":["src/test-utils.ts"],"names":[],"mappings":"AAAA,OAAO,QAAQ,MAAM,uBAAuB,CAAC;AAC7C,OAAO,cAAc,MAAM,yBAAyB,CAAC;AACrD,cAAc,2BAA2B,CAAC;AAE1C,OAAO,EAAE,QAAQ,EAAE,cAAc,EAAE,CAAC"} apollo-server-demo/node_modules/apollo-link/lib/bundle.cjs.js.map 0000644 0001750 0000144 00000033622 03560116604 024566 0 ustar andreh users {"version":3,"file":"bundle.cjs.js","sources":["bundle.esm.js"],"sourcesContent":["import Observable from 'zen-observable-ts';\nexport { default as Observable } from 'zen-observable-ts';\nimport { invariant, InvariantError } from 'ts-invariant';\nimport { __extends, __assign } from 'tslib';\nimport { getOperationName } from 'apollo-utilities';\nexport { getOperationName } from 'apollo-utilities';\n\nfunction validateOperation(operation) {\n var OPERATION_FIELDS = [\n 'query',\n 'operationName',\n 'variables',\n 'extensions',\n 'context',\n ];\n for (var _i = 0, _a = Object.keys(operation); _i < _a.length; _i++) {\n var key = _a[_i];\n if (OPERATION_FIELDS.indexOf(key) < 0) {\n throw process.env.NODE_ENV === \"production\" ? new InvariantError(2) : new InvariantError(\"illegal argument: \" + key);\n }\n }\n return operation;\n}\nvar LinkError = (function (_super) {\n __extends(LinkError, _super);\n function LinkError(message, link) {\n var _this = _super.call(this, message) || this;\n _this.link = link;\n return _this;\n }\n return LinkError;\n}(Error));\nfunction isTerminating(link) {\n return link.request.length <= 1;\n}\nfunction toPromise(observable) {\n var completed = false;\n return new Promise(function (resolve, reject) {\n observable.subscribe({\n next: function (data) {\n if (completed) {\n process.env.NODE_ENV === \"production\" || invariant.warn(\"Promise Wrapper does not support multiple results from Observable\");\n }\n else {\n completed = true;\n resolve(data);\n }\n },\n error: reject,\n });\n });\n}\nvar makePromise = toPromise;\nfunction fromPromise(promise) {\n return new Observable(function (observer) {\n promise\n .then(function (value) {\n observer.next(value);\n observer.complete();\n })\n .catch(observer.error.bind(observer));\n });\n}\nfunction fromError(errorValue) {\n return new Observable(function (observer) {\n observer.error(errorValue);\n });\n}\nfunction transformOperation(operation) {\n var transformedOperation = {\n variables: operation.variables || {},\n extensions: operation.extensions || {},\n operationName: operation.operationName,\n query: operation.query,\n };\n if (!transformedOperation.operationName) {\n transformedOperation.operationName =\n typeof transformedOperation.query !== 'string'\n ? getOperationName(transformedOperation.query)\n : '';\n }\n return transformedOperation;\n}\nfunction createOperation(starting, operation) {\n var context = __assign({}, starting);\n var setContext = function (next) {\n if (typeof next === 'function') {\n context = __assign({}, context, next(context));\n }\n else {\n context = __assign({}, context, next);\n }\n };\n var getContext = function () { return (__assign({}, context)); };\n Object.defineProperty(operation, 'setContext', {\n enumerable: false,\n value: setContext,\n });\n Object.defineProperty(operation, 'getContext', {\n enumerable: false,\n value: getContext,\n });\n Object.defineProperty(operation, 'toKey', {\n enumerable: false,\n value: function () { return getKey(operation); },\n });\n return operation;\n}\nfunction getKey(operation) {\n var query = operation.query, variables = operation.variables, operationName = operation.operationName;\n return JSON.stringify([operationName, query, variables]);\n}\n\nfunction passthrough(op, forward) {\n return forward ? forward(op) : Observable.of();\n}\nfunction toLink(handler) {\n return typeof handler === 'function' ? new ApolloLink(handler) : handler;\n}\nfunction empty() {\n return new ApolloLink(function () { return Observable.of(); });\n}\nfunction from(links) {\n if (links.length === 0)\n return empty();\n return links.map(toLink).reduce(function (x, y) { return x.concat(y); });\n}\nfunction split(test, left, right) {\n var leftLink = toLink(left);\n var rightLink = toLink(right || new ApolloLink(passthrough));\n if (isTerminating(leftLink) && isTerminating(rightLink)) {\n return new ApolloLink(function (operation) {\n return test(operation)\n ? leftLink.request(operation) || Observable.of()\n : rightLink.request(operation) || Observable.of();\n });\n }\n else {\n return new ApolloLink(function (operation, forward) {\n return test(operation)\n ? leftLink.request(operation, forward) || Observable.of()\n : rightLink.request(operation, forward) || Observable.of();\n });\n }\n}\nvar concat = function (first, second) {\n var firstLink = toLink(first);\n if (isTerminating(firstLink)) {\n process.env.NODE_ENV === \"production\" || invariant.warn(new LinkError(\"You are calling concat on a terminating link, which will have no effect\", firstLink));\n return firstLink;\n }\n var nextLink = toLink(second);\n if (isTerminating(nextLink)) {\n return new ApolloLink(function (operation) {\n return firstLink.request(operation, function (op) { return nextLink.request(op) || Observable.of(); }) || Observable.of();\n });\n }\n else {\n return new ApolloLink(function (operation, forward) {\n return (firstLink.request(operation, function (op) {\n return nextLink.request(op, forward) || Observable.of();\n }) || Observable.of());\n });\n }\n};\nvar ApolloLink = (function () {\n function ApolloLink(request) {\n if (request)\n this.request = request;\n }\n ApolloLink.prototype.split = function (test, left, right) {\n return this.concat(split(test, left, right || new ApolloLink(passthrough)));\n };\n ApolloLink.prototype.concat = function (next) {\n return concat(this, next);\n };\n ApolloLink.prototype.request = function (operation, forward) {\n throw process.env.NODE_ENV === \"production\" ? new InvariantError(1) : new InvariantError('request is not implemented');\n };\n ApolloLink.empty = empty;\n ApolloLink.from = from;\n ApolloLink.split = split;\n ApolloLink.execute = execute;\n return ApolloLink;\n}());\nfunction execute(link, operation) {\n return (link.request(createOperation(operation.context, transformOperation(validateOperation(operation)))) || Observable.of());\n}\n\nexport { ApolloLink, concat, createOperation, empty, execute, from, fromError, fromPromise, makePromise, split, toPromise };\n//# sourceMappingURL=bundle.esm.js.map\n"],"names":["InvariantError","__extends","invariant","getOperationName","__assign"],"mappings":";;;;;;;;;;;AAOA,SAAS,iBAAiB,CAAC,SAAS,EAAE;AACtC,IAAI,IAAI,gBAAgB,GAAG;AAC3B,QAAQ,OAAO;AACf,QAAQ,eAAe;AACvB,QAAQ,WAAW;AACnB,QAAQ,YAAY;AACpB,QAAQ,SAAS;AACjB,KAAK,CAAC;AACN,IAAI,KAAK,IAAI,EAAE,GAAG,CAAC,EAAE,EAAE,GAAG,MAAM,CAAC,IAAI,CAAC,SAAS,CAAC,EAAE,EAAE,GAAG,EAAE,CAAC,MAAM,EAAE,EAAE,EAAE,EAAE;AACxE,QAAQ,IAAI,GAAG,GAAG,EAAE,CAAC,EAAE,CAAC,CAAC;AACzB,QAAQ,IAAI,gBAAgB,CAAC,OAAO,CAAC,GAAG,CAAC,GAAG,CAAC,EAAE;AAC/C,YAAY,MAAM,OAAO,CAAC,GAAG,CAAC,QAAQ,KAAK,YAAY,GAAG,IAAIA,0BAAc,CAAC,CAAC,CAAC,GAAG,IAAIA,0BAAc,CAAC,oBAAoB,GAAG,GAAG,CAAC,CAAC;AACjI,SAAS;AACT,KAAK;AACL,IAAI,OAAO,SAAS,CAAC;AACrB,CAAC;AACD,IAAI,SAAS,IAAI,UAAU,MAAM,EAAE;AACnC,IAAIC,eAAS,CAAC,SAAS,EAAE,MAAM,CAAC,CAAC;AACjC,IAAI,SAAS,SAAS,CAAC,OAAO,EAAE,IAAI,EAAE;AACtC,QAAQ,IAAI,KAAK,GAAG,MAAM,CAAC,IAAI,CAAC,IAAI,EAAE,OAAO,CAAC,IAAI,IAAI,CAAC;AACvD,QAAQ,KAAK,CAAC,IAAI,GAAG,IAAI,CAAC;AAC1B,QAAQ,OAAO,KAAK,CAAC;AACrB,KAAK;AACL,IAAI,OAAO,SAAS,CAAC;AACrB,CAAC,CAAC,KAAK,CAAC,CAAC,CAAC;AACV,SAAS,aAAa,CAAC,IAAI,EAAE;AAC7B,IAAI,OAAO,IAAI,CAAC,OAAO,CAAC,MAAM,IAAI,CAAC,CAAC;AACpC,CAAC;AACD,SAAS,SAAS,CAAC,UAAU,EAAE;AAC/B,IAAI,IAAI,SAAS,GAAG,KAAK,CAAC;AAC1B,IAAI,OAAO,IAAI,OAAO,CAAC,UAAU,OAAO,EAAE,MAAM,EAAE;AAClD,QAAQ,UAAU,CAAC,SAAS,CAAC;AAC7B,YAAY,IAAI,EAAE,UAAU,IAAI,EAAE;AAClC,gBAAgB,IAAI,SAAS,EAAE;AAC/B,oBAAoB,OAAO,CAAC,GAAG,CAAC,QAAQ,KAAK,YAAY,IAAIC,qBAAS,CAAC,IAAI,CAAC,mEAAmE,CAAC,CAAC;AACjJ,iBAAiB;AACjB,qBAAqB;AACrB,oBAAoB,SAAS,GAAG,IAAI,CAAC;AACrC,oBAAoB,OAAO,CAAC,IAAI,CAAC,CAAC;AAClC,iBAAiB;AACjB,aAAa;AACb,YAAY,KAAK,EAAE,MAAM;AACzB,SAAS,CAAC,CAAC;AACX,KAAK,CAAC,CAAC;AACP,CAAC;AACE,IAAC,WAAW,GAAG,UAAU;AAC5B,SAAS,WAAW,CAAC,OAAO,EAAE;AAC9B,IAAI,OAAO,IAAI,UAAU,CAAC,UAAU,QAAQ,EAAE;AAC9C,QAAQ,OAAO;AACf,aAAa,IAAI,CAAC,UAAU,KAAK,EAAE;AACnC,YAAY,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC;AACjC,YAAY,QAAQ,CAAC,QAAQ,EAAE,CAAC;AAChC,SAAS,CAAC;AACV,aAAa,KAAK,CAAC,QAAQ,CAAC,KAAK,CAAC,IAAI,CAAC,QAAQ,CAAC,CAAC,CAAC;AAClD,KAAK,CAAC,CAAC;AACP,CAAC;AACD,SAAS,SAAS,CAAC,UAAU,EAAE;AAC/B,IAAI,OAAO,IAAI,UAAU,CAAC,UAAU,QAAQ,EAAE;AAC9C,QAAQ,QAAQ,CAAC,KAAK,CAAC,UAAU,CAAC,CAAC;AACnC,KAAK,CAAC,CAAC;AACP,CAAC;AACD,SAAS,kBAAkB,CAAC,SAAS,EAAE;AACvC,IAAI,IAAI,oBAAoB,GAAG;AAC/B,QAAQ,SAAS,EAAE,SAAS,CAAC,SAAS,IAAI,EAAE;AAC5C,QAAQ,UAAU,EAAE,SAAS,CAAC,UAAU,IAAI,EAAE;AAC9C,QAAQ,aAAa,EAAE,SAAS,CAAC,aAAa;AAC9C,QAAQ,KAAK,EAAE,SAAS,CAAC,KAAK;AAC9B,KAAK,CAAC;AACN,IAAI,IAAI,CAAC,oBAAoB,CAAC,aAAa,EAAE;AAC7C,QAAQ,oBAAoB,CAAC,aAAa;AAC1C,YAAY,OAAO,oBAAoB,CAAC,KAAK,KAAK,QAAQ;AAC1D,kBAAkBC,gCAAgB,CAAC,oBAAoB,CAAC,KAAK,CAAC;AAC9D,kBAAkB,EAAE,CAAC;AACrB,KAAK;AACL,IAAI,OAAO,oBAAoB,CAAC;AAChC,CAAC;AACD,SAAS,eAAe,CAAC,QAAQ,EAAE,SAAS,EAAE;AAC9C,IAAI,IAAI,OAAO,GAAGC,cAAQ,CAAC,EAAE,EAAE,QAAQ,CAAC,CAAC;AACzC,IAAI,IAAI,UAAU,GAAG,UAAU,IAAI,EAAE;AACrC,QAAQ,IAAI,OAAO,IAAI,KAAK,UAAU,EAAE;AACxC,YAAY,OAAO,GAAGA,cAAQ,CAAC,EAAE,EAAE,OAAO,EAAE,IAAI,CAAC,OAAO,CAAC,CAAC,CAAC;AAC3D,SAAS;AACT,aAAa;AACb,YAAY,OAAO,GAAGA,cAAQ,CAAC,EAAE,EAAE,OAAO,EAAE,IAAI,CAAC,CAAC;AAClD,SAAS;AACT,KAAK,CAAC;AACN,IAAI,IAAI,UAAU,GAAG,YAAY,EAAE,QAAQA,cAAQ,CAAC,EAAE,EAAE,OAAO,CAAC,EAAE,EAAE,CAAC;AACrE,IAAI,MAAM,CAAC,cAAc,CAAC,SAAS,EAAE,YAAY,EAAE;AACnD,QAAQ,UAAU,EAAE,KAAK;AACzB,QAAQ,KAAK,EAAE,UAAU;AACzB,KAAK,CAAC,CAAC;AACP,IAAI,MAAM,CAAC,cAAc,CAAC,SAAS,EAAE,YAAY,EAAE;AACnD,QAAQ,UAAU,EAAE,KAAK;AACzB,QAAQ,KAAK,EAAE,UAAU;AACzB,KAAK,CAAC,CAAC;AACP,IAAI,MAAM,CAAC,cAAc,CAAC,SAAS,EAAE,OAAO,EAAE;AAC9C,QAAQ,UAAU,EAAE,KAAK;AACzB,QAAQ,KAAK,EAAE,YAAY,EAAE,OAAO,MAAM,CAAC,SAAS,CAAC,CAAC,EAAE;AACxD,KAAK,CAAC,CAAC;AACP,IAAI,OAAO,SAAS,CAAC;AACrB,CAAC;AACD,SAAS,MAAM,CAAC,SAAS,EAAE;AAC3B,IAAI,IAAI,KAAK,GAAG,SAAS,CAAC,KAAK,EAAE,SAAS,GAAG,SAAS,CAAC,SAAS,EAAE,aAAa,GAAG,SAAS,CAAC,aAAa,CAAC;AAC1G,IAAI,OAAO,IAAI,CAAC,SAAS,CAAC,CAAC,aAAa,EAAE,KAAK,EAAE,SAAS,CAAC,CAAC,CAAC;AAC7D,CAAC;AACD;AACA,SAAS,WAAW,CAAC,EAAE,EAAE,OAAO,EAAE;AAClC,IAAI,OAAO,OAAO,GAAG,OAAO,CAAC,EAAE,CAAC,GAAG,UAAU,CAAC,EAAE,EAAE,CAAC;AACnD,CAAC;AACD,SAAS,MAAM,CAAC,OAAO,EAAE;AACzB,IAAI,OAAO,OAAO,OAAO,KAAK,UAAU,GAAG,IAAI,UAAU,CAAC,OAAO,CAAC,GAAG,OAAO,CAAC;AAC7E,CAAC;AACD,SAAS,KAAK,GAAG;AACjB,IAAI,OAAO,IAAI,UAAU,CAAC,YAAY,EAAE,OAAO,UAAU,CAAC,EAAE,EAAE,CAAC,EAAE,CAAC,CAAC;AACnE,CAAC;AACD,SAAS,IAAI,CAAC,KAAK,EAAE;AACrB,IAAI,IAAI,KAAK,CAAC,MAAM,KAAK,CAAC;AAC1B,QAAQ,OAAO,KAAK,EAAE,CAAC;AACvB,IAAI,OAAO,KAAK,CAAC,GAAG,CAAC,MAAM,CAAC,CAAC,MAAM,CAAC,UAAU,CAAC,EAAE,CAAC,EAAE,EAAE,OAAO,CAAC,CAAC,MAAM,CAAC,CAAC,CAAC,CAAC,EAAE,CAAC,CAAC;AAC7E,CAAC;AACD,SAAS,KAAK,CAAC,IAAI,EAAE,IAAI,EAAE,KAAK,EAAE;AAClC,IAAI,IAAI,QAAQ,GAAG,MAAM,CAAC,IAAI,CAAC,CAAC;AAChC,IAAI,IAAI,SAAS,GAAG,MAAM,CAAC,KAAK,IAAI,IAAI,UAAU,CAAC,WAAW,CAAC,CAAC,CAAC;AACjE,IAAI,IAAI,aAAa,CAAC,QAAQ,CAAC,IAAI,aAAa,CAAC,SAAS,CAAC,EAAE;AAC7D,QAAQ,OAAO,IAAI,UAAU,CAAC,UAAU,SAAS,EAAE;AACnD,YAAY,OAAO,IAAI,CAAC,SAAS,CAAC;AAClC,kBAAkB,QAAQ,CAAC,OAAO,CAAC,SAAS,CAAC,IAAI,UAAU,CAAC,EAAE,EAAE;AAChE,kBAAkB,SAAS,CAAC,OAAO,CAAC,SAAS,CAAC,IAAI,UAAU,CAAC,EAAE,EAAE,CAAC;AAClE,SAAS,CAAC,CAAC;AACX,KAAK;AACL,SAAS;AACT,QAAQ,OAAO,IAAI,UAAU,CAAC,UAAU,SAAS,EAAE,OAAO,EAAE;AAC5D,YAAY,OAAO,IAAI,CAAC,SAAS,CAAC;AAClC,kBAAkB,QAAQ,CAAC,OAAO,CAAC,SAAS,EAAE,OAAO,CAAC,IAAI,UAAU,CAAC,EAAE,EAAE;AACzE,kBAAkB,SAAS,CAAC,OAAO,CAAC,SAAS,EAAE,OAAO,CAAC,IAAI,UAAU,CAAC,EAAE,EAAE,CAAC;AAC3E,SAAS,CAAC,CAAC;AACX,KAAK;AACL,CAAC;AACE,IAAC,MAAM,GAAG,UAAU,KAAK,EAAE,MAAM,EAAE;AACtC,IAAI,IAAI,SAAS,GAAG,MAAM,CAAC,KAAK,CAAC,CAAC;AAClC,IAAI,IAAI,aAAa,CAAC,SAAS,CAAC,EAAE;AAClC,QAAQ,OAAO,CAAC,GAAG,CAAC,QAAQ,KAAK,YAAY,IAAIF,qBAAS,CAAC,IAAI,CAAC,IAAI,SAAS,CAAC,yEAAyE,EAAE,SAAS,CAAC,CAAC,CAAC;AACrK,QAAQ,OAAO,SAAS,CAAC;AACzB,KAAK;AACL,IAAI,IAAI,QAAQ,GAAG,MAAM,CAAC,MAAM,CAAC,CAAC;AAClC,IAAI,IAAI,aAAa,CAAC,QAAQ,CAAC,EAAE;AACjC,QAAQ,OAAO,IAAI,UAAU,CAAC,UAAU,SAAS,EAAE;AACnD,YAAY,OAAO,SAAS,CAAC,OAAO,CAAC,SAAS,EAAE,UAAU,EAAE,EAAE,EAAE,OAAO,QAAQ,CAAC,OAAO,CAAC,EAAE,CAAC,IAAI,UAAU,CAAC,EAAE,EAAE,CAAC,EAAE,CAAC,IAAI,UAAU,CAAC,EAAE,EAAE,CAAC;AACtI,SAAS,CAAC,CAAC;AACX,KAAK;AACL,SAAS;AACT,QAAQ,OAAO,IAAI,UAAU,CAAC,UAAU,SAAS,EAAE,OAAO,EAAE;AAC5D,YAAY,QAAQ,SAAS,CAAC,OAAO,CAAC,SAAS,EAAE,UAAU,EAAE,EAAE;AAC/D,gBAAgB,OAAO,QAAQ,CAAC,OAAO,CAAC,EAAE,EAAE,OAAO,CAAC,IAAI,UAAU,CAAC,EAAE,EAAE,CAAC;AACxE,aAAa,CAAC,IAAI,UAAU,CAAC,EAAE,EAAE,EAAE;AACnC,SAAS,CAAC,CAAC;AACX,KAAK;AACL,EAAE;AACC,IAAC,UAAU,IAAI,YAAY;AAC9B,IAAI,SAAS,UAAU,CAAC,OAAO,EAAE;AACjC,QAAQ,IAAI,OAAO;AACnB,YAAY,IAAI,CAAC,OAAO,GAAG,OAAO,CAAC;AACnC,KAAK;AACL,IAAI,UAAU,CAAC,SAAS,CAAC,KAAK,GAAG,UAAU,IAAI,EAAE,IAAI,EAAE,KAAK,EAAE;AAC9D,QAAQ,OAAO,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,IAAI,EAAE,IAAI,EAAE,KAAK,IAAI,IAAI,UAAU,CAAC,WAAW,CAAC,CAAC,CAAC,CAAC;AACpF,KAAK,CAAC;AACN,IAAI,UAAU,CAAC,SAAS,CAAC,MAAM,GAAG,UAAU,IAAI,EAAE;AAClD,QAAQ,OAAO,MAAM,CAAC,IAAI,EAAE,IAAI,CAAC,CAAC;AAClC,KAAK,CAAC;AACN,IAAI,UAAU,CAAC,SAAS,CAAC,OAAO,GAAG,UAAU,SAAS,EAAE,OAAO,EAAE;AACjE,QAAQ,MAAM,OAAO,CAAC,GAAG,CAAC,QAAQ,KAAK,YAAY,GAAG,IAAIF,0BAAc,CAAC,CAAC,CAAC,GAAG,IAAIA,0BAAc,CAAC,4BAA4B,CAAC,CAAC;AAC/H,KAAK,CAAC;AACN,IAAI,UAAU,CAAC,KAAK,GAAG,KAAK,CAAC;AAC7B,IAAI,UAAU,CAAC,IAAI,GAAG,IAAI,CAAC;AAC3B,IAAI,UAAU,CAAC,KAAK,GAAG,KAAK,CAAC;AAC7B,IAAI,UAAU,CAAC,OAAO,GAAG,OAAO,CAAC;AACjC,IAAI,OAAO,UAAU,CAAC;AACtB,CAAC,EAAE,EAAE;AACL,SAAS,OAAO,CAAC,IAAI,EAAE,SAAS,EAAE;AAClC,IAAI,QAAQ,IAAI,CAAC,OAAO,CAAC,eAAe,CAAC,SAAS,CAAC,OAAO,EAAE,kBAAkB,CAAC,iBAAiB,CAAC,SAAS,CAAC,CAAC,CAAC,CAAC,IAAI,UAAU,CAAC,EAAE,EAAE,EAAE;AACnI;;;;;;;;;;;;;;;;;;;;;"} apollo-server-demo/node_modules/apollo-link/lib/test-utils.js 0000644 0001750 0000144 00000000712 03560116604 024072 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); var tslib_1 = require("tslib"); var mockLink_1 = tslib_1.__importDefault(require("./test-utils/mockLink")); exports.MockLink = mockLink_1.default; var setContext_1 = tslib_1.__importDefault(require("./test-utils/setContext")); exports.SetContextLink = setContext_1.default; tslib_1.__exportStar(require("./test-utils/testingUtils"), exports); //# sourceMappingURL=test-utils.js.map apollo-server-demo/node_modules/apollo-link/lib/link.js.map 0000644 0001750 0000144 00000006144 03560116604 023473 0 ustar andreh users {"version":3,"file":"link.js","sourceRoot":"","sources":["../src/link.ts"],"names":[],"mappings":";;;AAAA,gFAA2C;AAC3C,6CAAyD;AAUzD,yCAMqB;AAErB,SAAS,WAAW,CAAC,EAAE,EAAE,OAAO;IAC9B,OAAO,OAAO,CAAC,CAAC,CAAC,OAAO,CAAC,EAAE,CAAC,CAAC,CAAC,CAAC,2BAAU,CAAC,EAAE,EAAE,CAAC;AACjD,CAAC;AAED,SAAS,MAAM,CAAC,OAAoC;IAClD,OAAO,OAAO,OAAO,KAAK,UAAU,CAAC,CAAC,CAAC,IAAI,UAAU,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC,OAAO,CAAC;AAC3E,CAAC;AAED,SAAgB,KAAK;IACnB,OAAO,IAAI,UAAU,CAAC,cAAM,OAAA,2BAAU,CAAC,EAAE,EAAE,EAAf,CAAe,CAAC,CAAC;AAC/C,CAAC;AAFD,sBAEC;AAED,SAAgB,IAAI,CAAC,KAAmB;IACtC,IAAI,KAAK,CAAC,MAAM,KAAK,CAAC;QAAE,OAAO,KAAK,EAAE,CAAC;IACvC,OAAO,KAAK,CAAC,GAAG,CAAC,MAAM,CAAC,CAAC,MAAM,CAAC,UAAC,CAAC,EAAE,CAAC,IAAK,OAAA,CAAC,CAAC,MAAM,CAAC,CAAC,CAAC,EAAX,CAAW,CAAC,CAAC;AACzD,CAAC;AAHD,oBAGC;AAED,SAAgB,KAAK,CACnB,IAAgC,EAChC,IAAiC,EACjC,KAAmC;IAEnC,IAAM,QAAQ,GAAG,MAAM,CAAC,IAAI,CAAC,CAAC;IAC9B,IAAM,SAAS,GAAG,MAAM,CAAC,KAAK,IAAI,IAAI,UAAU,CAAC,WAAW,CAAC,CAAC,CAAC;IAE/D,IAAI,yBAAa,CAAC,QAAQ,CAAC,IAAI,yBAAa,CAAC,SAAS,CAAC,EAAE;QACvD,OAAO,IAAI,UAAU,CAAC,UAAA,SAAS;YAC7B,OAAO,IAAI,CAAC,SAAS,CAAC;gBACpB,CAAC,CAAC,QAAQ,CAAC,OAAO,CAAC,SAAS,CAAC,IAAI,2BAAU,CAAC,EAAE,EAAE;gBAChD,CAAC,CAAC,SAAS,CAAC,OAAO,CAAC,SAAS,CAAC,IAAI,2BAAU,CAAC,EAAE,EAAE,CAAC;QACtD,CAAC,CAAC,CAAC;KACJ;SAAM;QACL,OAAO,IAAI,UAAU,CAAC,UAAC,SAAS,EAAE,OAAO;YACvC,OAAO,IAAI,CAAC,SAAS,CAAC;gBACpB,CAAC,CAAC,QAAQ,CAAC,OAAO,CAAC,SAAS,EAAE,OAAO,CAAC,IAAI,2BAAU,CAAC,EAAE,EAAE;gBACzD,CAAC,CAAC,SAAS,CAAC,OAAO,CAAC,SAAS,EAAE,OAAO,CAAC,IAAI,2BAAU,CAAC,EAAE,EAAE,CAAC;QAC/D,CAAC,CAAC,CAAC;KACJ;AACH,CAAC;AArBD,sBAqBC;AAGY,QAAA,MAAM,GAAG,UACpB,KAAkC,EAClC,MAAmC;IAEnC,IAAM,SAAS,GAAG,MAAM,CAAC,KAAK,CAAC,CAAC;IAChC,IAAI,yBAAa,CAAC,SAAS,CAAC,EAAE;QAC5B,wBAAS,CAAC,IAAI,CACZ,IAAI,qBAAS,CACX,yEAAyE,EACzE,SAAS,CACV,CACF,CAAC;QACF,OAAO,SAAS,CAAC;KAClB;IACD,IAAM,QAAQ,GAAG,MAAM,CAAC,MAAM,CAAC,CAAC;IAEhC,IAAI,yBAAa,CAAC,QAAQ,CAAC,EAAE;QAC3B,OAAO,IAAI,UAAU,CACnB,UAAA,SAAS;YACP,OAAA,SAAS,CAAC,OAAO,CACf,SAAS,EACT,UAAA,EAAE,IAAI,OAAA,QAAQ,CAAC,OAAO,CAAC,EAAE,CAAC,IAAI,2BAAU,CAAC,EAAE,EAAE,EAAvC,CAAuC,CAC9C,IAAI,2BAAU,CAAC,EAAE,EAAE;QAHpB,CAGoB,CACvB,CAAC;KACH;SAAM;QACL,OAAO,IAAI,UAAU,CAAC,UAAC,SAAS,EAAE,OAAO;YACvC,OAAO,CACL,SAAS,CAAC,OAAO,CAAC,SAAS,EAAE,UAAA,EAAE;gBAC7B,OAAO,QAAQ,CAAC,OAAO,CAAC,EAAE,EAAE,OAAO,CAAC,IAAI,2BAAU,CAAC,EAAE,EAAE,CAAC;YAC1D,CAAC,CAAC,IAAI,2BAAU,CAAC,EAAE,EAAE,CACtB,CAAC;QACJ,CAAC,CAAC,CAAC;KACJ;AACH,CAAC,CAAC;AAEF;IAME,oBAAY,OAAwB;QAClC,IAAI,OAAO;YAAE,IAAI,CAAC,OAAO,GAAG,OAAO,CAAC;IACtC,CAAC;IAEM,0BAAK,GAAZ,UACE,IAAgC,EAChC,IAAiC,EACjC,KAAmC;QAEnC,OAAO,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,IAAI,EAAE,IAAI,EAAE,KAAK,IAAI,IAAI,UAAU,CAAC,WAAW,CAAC,CAAC,CAAC,CAAC;IAC9E,CAAC;IAEM,2BAAM,GAAb,UAAc,IAAiC;QAC7C,OAAO,cAAM,CAAC,IAAI,EAAE,IAAI,CAAC,CAAC;IAC5B,CAAC;IAEM,4BAAO,GAAd,UACE,SAAoB,EACpB,OAAkB;QAElB,MAAM,IAAI,6BAAc,CAAC,4BAA4B,CAAC,CAAC;IACzD,CAAC;IA1Ba,gBAAK,GAAG,KAAK,CAAC;IACd,eAAI,GAAG,IAAI,CAAC;IACZ,gBAAK,GAAG,KAAK,CAAC;IACd,kBAAO,GAAG,OAAO,CAAC;IAwBlC,iBAAC;CAAA,AA5BD,IA4BC;AA5BY,gCAAU;AA8BvB,SAAgB,OAAO,CACrB,IAAgB,EAChB,SAAyB;IAEzB,OAAO,CACL,IAAI,CAAC,OAAO,CACV,2BAAe,CACb,SAAS,CAAC,OAAO,EACjB,8BAAkB,CAAC,6BAAiB,CAAC,SAAS,CAAC,CAAC,CACjD,CACF,IAAI,2BAAU,CAAC,EAAE,EAAE,CACrB,CAAC;AACJ,CAAC;AAZD,0BAYC"} apollo-server-demo/node_modules/apollo-link/lib/linkUtils.js 0000644 0001750 0000144 00000007747 03560116604 023752 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); var tslib_1 = require("tslib"); var zen_observable_ts_1 = tslib_1.__importDefault(require("zen-observable-ts")); var apollo_utilities_1 = require("apollo-utilities"); exports.getOperationName = apollo_utilities_1.getOperationName; var ts_invariant_1 = require("ts-invariant"); function validateOperation(operation) { var OPERATION_FIELDS = [ 'query', 'operationName', 'variables', 'extensions', 'context', ]; for (var _i = 0, _a = Object.keys(operation); _i < _a.length; _i++) { var key = _a[_i]; if (OPERATION_FIELDS.indexOf(key) < 0) { throw new ts_invariant_1.InvariantError("illegal argument: " + key); } } return operation; } exports.validateOperation = validateOperation; var LinkError = (function (_super) { tslib_1.__extends(LinkError, _super); function LinkError(message, link) { var _this = _super.call(this, message) || this; _this.link = link; return _this; } return LinkError; }(Error)); exports.LinkError = LinkError; function isTerminating(link) { return link.request.length <= 1; } exports.isTerminating = isTerminating; function toPromise(observable) { var completed = false; return new Promise(function (resolve, reject) { observable.subscribe({ next: function (data) { if (completed) { ts_invariant_1.invariant.warn("Promise Wrapper does not support multiple results from Observable"); } else { completed = true; resolve(data); } }, error: reject, }); }); } exports.toPromise = toPromise; exports.makePromise = toPromise; function fromPromise(promise) { return new zen_observable_ts_1.default(function (observer) { promise .then(function (value) { observer.next(value); observer.complete(); }) .catch(observer.error.bind(observer)); }); } exports.fromPromise = fromPromise; function fromError(errorValue) { return new zen_observable_ts_1.default(function (observer) { observer.error(errorValue); }); } exports.fromError = fromError; function transformOperation(operation) { var transformedOperation = { variables: operation.variables || {}, extensions: operation.extensions || {}, operationName: operation.operationName, query: operation.query, }; if (!transformedOperation.operationName) { transformedOperation.operationName = typeof transformedOperation.query !== 'string' ? apollo_utilities_1.getOperationName(transformedOperation.query) : ''; } return transformedOperation; } exports.transformOperation = transformOperation; function createOperation(starting, operation) { var context = tslib_1.__assign({}, starting); var setContext = function (next) { if (typeof next === 'function') { context = tslib_1.__assign({}, context, next(context)); } else { context = tslib_1.__assign({}, context, next); } }; var getContext = function () { return (tslib_1.__assign({}, context)); }; Object.defineProperty(operation, 'setContext', { enumerable: false, value: setContext, }); Object.defineProperty(operation, 'getContext', { enumerable: false, value: getContext, }); Object.defineProperty(operation, 'toKey', { enumerable: false, value: function () { return getKey(operation); }, }); return operation; } exports.createOperation = createOperation; function getKey(operation) { var query = operation.query, variables = operation.variables, operationName = operation.operationName; return JSON.stringify([operationName, query, variables]); } exports.getKey = getKey; //# sourceMappingURL=linkUtils.js.map apollo-server-demo/node_modules/apollo-link/lib/index.d.ts 0000644 0001750 0000144 00000000416 03560116604 023321 0 ustar andreh users export * from './link'; export { createOperation, makePromise, toPromise, fromPromise, fromError, getOperationName, } from './linkUtils'; export * from './types'; import Observable from 'zen-observable-ts'; export { Observable }; //# sourceMappingURL=index.d.ts.map apollo-server-demo/node_modules/apollo-link/lib/link.d.ts.map 0000644 0001750 0000144 00000001732 03560116604 023725 0 ustar andreh users {"version":3,"file":"link.d.ts","sourceRoot":"","sources":["src/link.ts"],"names":[],"mappings":"AAAA,OAAO,UAAU,MAAM,mBAAmB,CAAC;AAG3C,OAAO,EACL,cAAc,EACd,QAAQ,EACR,SAAS,EACT,cAAc,EACd,WAAW,EACZ,MAAM,SAAS,CAAC;AAkBjB,wBAAgB,KAAK,IAAI,UAAU,CAElC;AAED,wBAAgB,IAAI,CAAC,KAAK,EAAE,UAAU,EAAE,GAAG,UAAU,CAGpD;AAED,wBAAgB,KAAK,CACnB,IAAI,EAAE,CAAC,EAAE,EAAE,SAAS,KAAK,OAAO,EAChC,IAAI,EAAE,UAAU,GAAG,cAAc,EACjC,KAAK,CAAC,EAAE,UAAU,GAAG,cAAc,GAClC,UAAU,CAiBZ;AAGD,eAAO,MAAM,MAAM,yFAiClB,CAAC;AAEF,qBAAa,UAAU;IACrB,OAAc,KAAK,eAAS;IAC5B,OAAc,IAAI,cAAQ;IAC1B,OAAc,KAAK,eAAS;IAC5B,OAAc,OAAO,iBAAW;gBAEpB,OAAO,CAAC,EAAE,cAAc;IAI7B,KAAK,CACV,IAAI,EAAE,CAAC,EAAE,EAAE,SAAS,KAAK,OAAO,EAChC,IAAI,EAAE,UAAU,GAAG,cAAc,EACjC,KAAK,CAAC,EAAE,UAAU,GAAG,cAAc,GAClC,UAAU;IAIN,MAAM,CAAC,IAAI,EAAE,UAAU,GAAG,cAAc,GAAG,UAAU;IAIrD,OAAO,CACZ,SAAS,EAAE,SAAS,EACpB,OAAO,CAAC,EAAE,QAAQ,GACjB,UAAU,CAAC,WAAW,CAAC,GAAG,IAAI;CAGlC;AAED,wBAAgB,OAAO,CACrB,IAAI,EAAE,UAAU,EAChB,SAAS,EAAE,cAAc,GACxB,UAAU,CAAC,WAAW,CAAC,CASzB"} apollo-server-demo/node_modules/apollo-link/lib/index.d.ts.map 0000644 0001750 0000144 00000000453 03560116604 024076 0 ustar andreh users {"version":3,"file":"index.d.ts","sourceRoot":"","sources":["src/index.ts"],"names":[],"mappings":"AAAA,cAAc,QAAQ,CAAC;AACvB,OAAO,EACL,eAAe,EACf,WAAW,EACX,SAAS,EACT,WAAW,EACX,SAAS,EACT,gBAAgB,GACjB,MAAM,aAAa,CAAC;AACrB,cAAc,SAAS,CAAC;AAExB,OAAO,UAAU,MAAM,mBAAmB,CAAC;AAC3C,OAAO,EAAE,UAAU,EAAE,CAAC"} apollo-server-demo/node_modules/apollo-link/lib/link.js 0000644 0001750 0000144 00000006760 03560116604 022723 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); var tslib_1 = require("tslib"); var zen_observable_ts_1 = tslib_1.__importDefault(require("zen-observable-ts")); var ts_invariant_1 = require("ts-invariant"); var linkUtils_1 = require("./linkUtils"); function passthrough(op, forward) { return forward ? forward(op) : zen_observable_ts_1.default.of(); } function toLink(handler) { return typeof handler === 'function' ? new ApolloLink(handler) : handler; } function empty() { return new ApolloLink(function () { return zen_observable_ts_1.default.of(); }); } exports.empty = empty; function from(links) { if (links.length === 0) return empty(); return links.map(toLink).reduce(function (x, y) { return x.concat(y); }); } exports.from = from; function split(test, left, right) { var leftLink = toLink(left); var rightLink = toLink(right || new ApolloLink(passthrough)); if (linkUtils_1.isTerminating(leftLink) && linkUtils_1.isTerminating(rightLink)) { return new ApolloLink(function (operation) { return test(operation) ? leftLink.request(operation) || zen_observable_ts_1.default.of() : rightLink.request(operation) || zen_observable_ts_1.default.of(); }); } else { return new ApolloLink(function (operation, forward) { return test(operation) ? leftLink.request(operation, forward) || zen_observable_ts_1.default.of() : rightLink.request(operation, forward) || zen_observable_ts_1.default.of(); }); } } exports.split = split; exports.concat = function (first, second) { var firstLink = toLink(first); if (linkUtils_1.isTerminating(firstLink)) { ts_invariant_1.invariant.warn(new linkUtils_1.LinkError("You are calling concat on a terminating link, which will have no effect", firstLink)); return firstLink; } var nextLink = toLink(second); if (linkUtils_1.isTerminating(nextLink)) { return new ApolloLink(function (operation) { return firstLink.request(operation, function (op) { return nextLink.request(op) || zen_observable_ts_1.default.of(); }) || zen_observable_ts_1.default.of(); }); } else { return new ApolloLink(function (operation, forward) { return (firstLink.request(operation, function (op) { return nextLink.request(op, forward) || zen_observable_ts_1.default.of(); }) || zen_observable_ts_1.default.of()); }); } }; var ApolloLink = (function () { function ApolloLink(request) { if (request) this.request = request; } ApolloLink.prototype.split = function (test, left, right) { return this.concat(split(test, left, right || new ApolloLink(passthrough))); }; ApolloLink.prototype.concat = function (next) { return exports.concat(this, next); }; ApolloLink.prototype.request = function (operation, forward) { throw new ts_invariant_1.InvariantError('request is not implemented'); }; ApolloLink.empty = empty; ApolloLink.from = from; ApolloLink.split = split; ApolloLink.execute = execute; return ApolloLink; }()); exports.ApolloLink = ApolloLink; function execute(link, operation) { return (link.request(linkUtils_1.createOperation(operation.context, linkUtils_1.transformOperation(linkUtils_1.validateOperation(operation)))) || zen_observable_ts_1.default.of()); } exports.execute = execute; //# sourceMappingURL=link.js.map apollo-server-demo/node_modules/apollo-link/lib/bundle.umd.js 0000644 0001750 0000144 00000017723 03560116604 024024 0 ustar andreh users (function (global, factory) { typeof exports === 'object' && typeof module !== 'undefined' ? factory(exports, require('zen-observable-ts'), require('ts-invariant'), require('tslib'), require('apollo-utilities')) : typeof define === 'function' && define.amd ? define(['exports', 'zen-observable-ts', 'ts-invariant', 'tslib', 'apollo-utilities'], factory) : (global = global || self, factory((global.apolloLink = global.apolloLink || {}, global.apolloLink.core = {}), global.apolloLink.zenObservable, global.invariant, global.tslib, global.apolloUtilities)); }(this, (function (exports, Observable, tsInvariant, tslib_1, apolloUtilities) { 'use strict'; Observable = Observable && Observable.hasOwnProperty('default') ? Observable['default'] : Observable; function validateOperation(operation) { var OPERATION_FIELDS = [ 'query', 'operationName', 'variables', 'extensions', 'context', ]; for (var _i = 0, _a = Object.keys(operation); _i < _a.length; _i++) { var key = _a[_i]; if (OPERATION_FIELDS.indexOf(key) < 0) { throw process.env.NODE_ENV === "production" ? new tsInvariant.InvariantError(2) : new tsInvariant.InvariantError("illegal argument: " + key); } } return operation; } var LinkError = (function (_super) { tslib_1.__extends(LinkError, _super); function LinkError(message, link) { var _this = _super.call(this, message) || this; _this.link = link; return _this; } return LinkError; }(Error)); function isTerminating(link) { return link.request.length <= 1; } function toPromise(observable) { var completed = false; return new Promise(function (resolve, reject) { observable.subscribe({ next: function (data) { if (completed) { process.env.NODE_ENV === "production" || tsInvariant.invariant.warn("Promise Wrapper does not support multiple results from Observable"); } else { completed = true; resolve(data); } }, error: reject, }); }); } var makePromise = toPromise; function fromPromise(promise) { return new Observable(function (observer) { promise .then(function (value) { observer.next(value); observer.complete(); }) .catch(observer.error.bind(observer)); }); } function fromError(errorValue) { return new Observable(function (observer) { observer.error(errorValue); }); } function transformOperation(operation) { var transformedOperation = { variables: operation.variables || {}, extensions: operation.extensions || {}, operationName: operation.operationName, query: operation.query, }; if (!transformedOperation.operationName) { transformedOperation.operationName = typeof transformedOperation.query !== 'string' ? apolloUtilities.getOperationName(transformedOperation.query) : ''; } return transformedOperation; } function createOperation(starting, operation) { var context = tslib_1.__assign({}, starting); var setContext = function (next) { if (typeof next === 'function') { context = tslib_1.__assign({}, context, next(context)); } else { context = tslib_1.__assign({}, context, next); } }; var getContext = function () { return (tslib_1.__assign({}, context)); }; Object.defineProperty(operation, 'setContext', { enumerable: false, value: setContext, }); Object.defineProperty(operation, 'getContext', { enumerable: false, value: getContext, }); Object.defineProperty(operation, 'toKey', { enumerable: false, value: function () { return getKey(operation); }, }); return operation; } function getKey(operation) { var query = operation.query, variables = operation.variables, operationName = operation.operationName; return JSON.stringify([operationName, query, variables]); } function passthrough(op, forward) { return forward ? forward(op) : Observable.of(); } function toLink(handler) { return typeof handler === 'function' ? new ApolloLink(handler) : handler; } function empty() { return new ApolloLink(function () { return Observable.of(); }); } function from(links) { if (links.length === 0) return empty(); return links.map(toLink).reduce(function (x, y) { return x.concat(y); }); } function split(test, left, right) { var leftLink = toLink(left); var rightLink = toLink(right || new ApolloLink(passthrough)); if (isTerminating(leftLink) && isTerminating(rightLink)) { return new ApolloLink(function (operation) { return test(operation) ? leftLink.request(operation) || Observable.of() : rightLink.request(operation) || Observable.of(); }); } else { return new ApolloLink(function (operation, forward) { return test(operation) ? leftLink.request(operation, forward) || Observable.of() : rightLink.request(operation, forward) || Observable.of(); }); } } var concat = function (first, second) { var firstLink = toLink(first); if (isTerminating(firstLink)) { process.env.NODE_ENV === "production" || tsInvariant.invariant.warn(new LinkError("You are calling concat on a terminating link, which will have no effect", firstLink)); return firstLink; } var nextLink = toLink(second); if (isTerminating(nextLink)) { return new ApolloLink(function (operation) { return firstLink.request(operation, function (op) { return nextLink.request(op) || Observable.of(); }) || Observable.of(); }); } else { return new ApolloLink(function (operation, forward) { return (firstLink.request(operation, function (op) { return nextLink.request(op, forward) || Observable.of(); }) || Observable.of()); }); } }; var ApolloLink = (function () { function ApolloLink(request) { if (request) this.request = request; } ApolloLink.prototype.split = function (test, left, right) { return this.concat(split(test, left, right || new ApolloLink(passthrough))); }; ApolloLink.prototype.concat = function (next) { return concat(this, next); }; ApolloLink.prototype.request = function (operation, forward) { throw process.env.NODE_ENV === "production" ? new tsInvariant.InvariantError(1) : new tsInvariant.InvariantError('request is not implemented'); }; ApolloLink.empty = empty; ApolloLink.from = from; ApolloLink.split = split; ApolloLink.execute = execute; return ApolloLink; }()); function execute(link, operation) { return (link.request(createOperation(operation.context, transformOperation(validateOperation(operation)))) || Observable.of()); } exports.Observable = Observable; Object.defineProperty(exports, 'getOperationName', { enumerable: true, get: function () { return apolloUtilities.getOperationName; } }); exports.ApolloLink = ApolloLink; exports.concat = concat; exports.createOperation = createOperation; exports.empty = empty; exports.execute = execute; exports.from = from; exports.fromError = fromError; exports.fromPromise = fromPromise; exports.makePromise = makePromise; exports.split = split; exports.toPromise = toPromise; Object.defineProperty(exports, '__esModule', { value: true }); }))); //# sourceMappingURL=bundle.umd.js.map apollo-server-demo/node_modules/apollo-link/lib/linkUtils.d.ts.map 0000644 0001750 0000144 00000001747 03560116604 024754 0 ustar andreh users {"version":3,"file":"linkUtils.d.ts","sourceRoot":"","sources":["src/linkUtils.ts"],"names":[],"mappings":"AAAA,OAAO,UAAU,MAAM,mBAAmB,CAAC;AAE3C,OAAO,EAAE,cAAc,EAAE,SAAS,EAAE,MAAM,SAAS,CAAC;AACpD,OAAO,EAAE,UAAU,EAAE,MAAM,QAAQ,CAAC;AAEpC,OAAO,EAAE,gBAAgB,EAAE,MAAM,kBAAkB,CAAC;AAEpD,OAAO,EAAE,gBAAgB,EAAE,CAAC;AAE5B,wBAAgB,iBAAiB,CAAC,SAAS,EAAE,cAAc,GAAG,cAAc,CAe3E;AAED,qBAAa,SAAU,SAAQ,KAAK;IAC3B,IAAI,EAAE,UAAU,CAAC;gBACZ,OAAO,CAAC,EAAE,MAAM,EAAE,IAAI,CAAC,EAAE,UAAU;CAIhD;AAED,wBAAgB,aAAa,CAAC,IAAI,EAAE,UAAU,GAAG,OAAO,CAEvD;AAED,wBAAgB,SAAS,CAAC,CAAC,EAAE,UAAU,EAAE,UAAU,CAAC,CAAC,CAAC,GAAG,OAAO,CAAC,CAAC,CAAC,CAiBlE;AAGD,eAAO,MAAM,WAAW,kBAAY,CAAC;AAErC,wBAAgB,WAAW,CAAC,CAAC,EAAE,OAAO,EAAE,OAAO,CAAC,CAAC,CAAC,GAAG,UAAU,CAAC,CAAC,CAAC,CASjE;AAED,wBAAgB,SAAS,CAAC,CAAC,EAAE,UAAU,EAAE,GAAG,GAAG,UAAU,CAAC,CAAC,CAAC,CAI3D;AAED,wBAAgB,kBAAkB,CAAC,SAAS,EAAE,cAAc,GAAG,cAAc,CAiB5E;AAED,wBAAgB,eAAe,CAC7B,QAAQ,EAAE,GAAG,EACb,SAAS,EAAE,cAAc,GACxB,SAAS,CA2BX;AAED,wBAAgB,MAAM,CAAC,SAAS,EAAE,cAAc,UAK/C"} apollo-server-demo/node_modules/apollo-link/lib/link.d.ts 0000644 0001750 0000144 00000002175 03560116604 023153 0 ustar andreh users import Observable from 'zen-observable-ts'; import { GraphQLRequest, NextLink, Operation, RequestHandler, FetchResult } from './types'; export declare function empty(): ApolloLink; export declare function from(links: ApolloLink[]): ApolloLink; export declare function split(test: (op: Operation) => boolean, left: ApolloLink | RequestHandler, right?: ApolloLink | RequestHandler): ApolloLink; export declare const concat: (first: RequestHandler | ApolloLink, second: RequestHandler | ApolloLink) => ApolloLink; export declare class ApolloLink { static empty: typeof empty; static from: typeof from; static split: typeof split; static execute: typeof execute; constructor(request?: RequestHandler); split(test: (op: Operation) => boolean, left: ApolloLink | RequestHandler, right?: ApolloLink | RequestHandler): ApolloLink; concat(next: ApolloLink | RequestHandler): ApolloLink; request(operation: Operation, forward?: NextLink): Observable<FetchResult> | null; } export declare function execute(link: ApolloLink, operation: GraphQLRequest): Observable<FetchResult>; //# sourceMappingURL=link.d.ts.map apollo-server-demo/node_modules/apollo-link/lib/types.js.map 0000644 0001750 0000144 00000000146 03560116604 023676 0 ustar andreh users {"version":3,"file":"types.js","sourceRoot":"","sources":["../src/types.ts"],"names":[],"mappings":""} apollo-server-demo/node_modules/apollo-link/lib/types.d.ts 0000644 0001750 0000144 00000002363 03560116604 023361 0 ustar andreh users import Observable from 'zen-observable-ts'; import { DocumentNode } from 'graphql/language/ast'; import { ExecutionResult as GraphQLExecutionResult } from 'graphql'; export { DocumentNode }; export interface ExecutionResult<TData = { [key: string]: any; }> extends GraphQLExecutionResult { data?: TData | null; } export interface GraphQLRequest { query: DocumentNode; variables?: Record<string, any>; operationName?: string; context?: Record<string, any>; extensions?: Record<string, any>; } export interface Operation { query: DocumentNode; variables: Record<string, any>; operationName: string; extensions: Record<string, any>; setContext: (context: Record<string, any>) => Record<string, any>; getContext: () => Record<string, any>; toKey: () => string; } export declare type FetchResult<TData = { [key: string]: any; }, C = Record<string, any>, E = Record<string, any>> = ExecutionResult<TData> & { extensions?: E; context?: C; }; export declare type NextLink = (operation: Operation) => Observable<FetchResult>; export declare type RequestHandler = (operation: Operation, forward: NextLink) => Observable<FetchResult> | null; //# sourceMappingURL=types.d.ts.map apollo-server-demo/node_modules/apollo-link/lib/test-utils.d.ts 0000644 0001750 0000144 00000000340 03560116604 024323 0 ustar andreh users import MockLink from './test-utils/mockLink'; import SetContextLink from './test-utils/setContext'; export * from './test-utils/testingUtils'; export { MockLink, SetContextLink }; //# sourceMappingURL=test-utils.d.ts.map apollo-server-demo/node_modules/apollo-link/lib/test-utils/ 0000755 0001750 0000144 00000000000 14067647700 023546 5 ustar andreh users apollo-server-demo/node_modules/apollo-link/lib/test-utils/mockLink.d.ts 0000644 0001750 0000144 00000000611 03560116604 026073 0 ustar andreh users import { Operation, RequestHandler, NextLink, FetchResult } from '../types'; import Observable from 'zen-observable-ts'; import { ApolloLink } from '../link'; export default class MockLink extends ApolloLink { constructor(handleRequest?: RequestHandler); request(operation: Operation, forward?: NextLink): Observable<FetchResult> | null; } //# sourceMappingURL=mockLink.d.ts.map apollo-server-demo/node_modules/apollo-link/lib/test-utils/setContext.js 0000644 0001750 0000144 00000001405 03560116604 026232 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); var tslib_1 = require("tslib"); var link_1 = require("../link"); var SetContextLink = (function (_super) { tslib_1.__extends(SetContextLink, _super); function SetContextLink(setContext) { if (setContext === void 0) { setContext = function (c) { return c; }; } var _this = _super.call(this) || this; _this.setContext = setContext; return _this; } SetContextLink.prototype.request = function (operation, forward) { operation.setContext(this.setContext(operation.getContext())); return forward(operation); }; return SetContextLink; }(link_1.ApolloLink)); exports.default = SetContextLink; //# sourceMappingURL=setContext.js.map apollo-server-demo/node_modules/apollo-link/lib/test-utils/setContext.js.map 0000644 0001750 0000144 00000000767 03560116604 027020 0 ustar andreh users {"version":3,"file":"setContext.js","sourceRoot":"","sources":["../../src/test-utils/setContext.ts"],"names":[],"mappings":";;;AAIA,gCAAqC;AAErC;IAA4C,0CAAU;IACpD,wBACU,UAEyB;QAFzB,2BAAA,EAAA,uBAEmB,CAAC,IAAI,OAAA,CAAC,EAAD,CAAC;QAHnC,YAKE,iBAAO,SACR;QALS,gBAAU,GAAV,UAAU,CAEe;;IAGnC,CAAC;IAEM,gCAAO,GAAd,UACE,SAAoB,EACpB,OAAiB;QAEjB,SAAS,CAAC,UAAU,CAAC,IAAI,CAAC,UAAU,CAAC,SAAS,CAAC,UAAU,EAAE,CAAC,CAAC,CAAC;QAC9D,OAAO,OAAO,CAAC,SAAS,CAAC,CAAC;IAC5B,CAAC;IACH,qBAAC;AAAD,CAAC,AAhBD,CAA4C,iBAAU,GAgBrD"} apollo-server-demo/node_modules/apollo-link/lib/test-utils/testingUtils.d.ts 0000644 0001750 0000144 00000000622 03560116604 027024 0 ustar andreh users import { ApolloLink } from '../link'; export declare function checkCalls<T>(calls: any[], results: Array<T>): void; export interface TestResultType { link: ApolloLink; results?: any[]; query?: string; done?: () => void; context?: any; variables?: any; } export declare function testLinkResults(params: TestResultType): void; //# sourceMappingURL=testingUtils.d.ts.map apollo-server-demo/node_modules/apollo-link/lib/test-utils/mockLink.js 0000644 0001750 0000144 00000001255 03560116604 025644 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); var tslib_1 = require("tslib"); var link_1 = require("../link"); var MockLink = (function (_super) { tslib_1.__extends(MockLink, _super); function MockLink(handleRequest) { if (handleRequest === void 0) { handleRequest = function () { return null; }; } var _this = _super.call(this) || this; _this.request = handleRequest; return _this; } MockLink.prototype.request = function (operation, forward) { throw Error('should be overridden'); }; return MockLink; }(link_1.ApolloLink)); exports.default = MockLink; //# sourceMappingURL=mockLink.js.map apollo-server-demo/node_modules/apollo-link/lib/test-utils/testingUtils.js 0000644 0001750 0000144 00000003064 03560116604 026573 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); var tslib_1 = require("tslib"); var graphql_tag_1 = tslib_1.__importDefault(require("graphql-tag")); var link_1 = require("../link"); var sampleQuery = graphql_tag_1.default(templateObject_1 || (templateObject_1 = tslib_1.__makeTemplateObject(["\n query SampleQuery {\n stub {\n id\n }\n }\n"], ["\n query SampleQuery {\n stub {\n id\n }\n }\n"]))); function checkCalls(calls, results) { if (calls === void 0) { calls = []; } expect(calls.length).toBe(results.length); calls.map(function (call, i) { return expect(call.data).toEqual(results[i]); }); } exports.checkCalls = checkCalls; function testLinkResults(params) { var link = params.link, context = params.context, variables = params.variables; var results = params.results || []; var query = params.query || sampleQuery; var done = params.done || (function () { return void 0; }); var spy = jest.fn(); link_1.execute(link, { query: query, context: context, variables: variables }).subscribe({ next: spy, error: function (error) { expect(error).toEqual(results.pop()); checkCalls(spy.mock.calls[0], results); if (done) { done(); } }, complete: function () { checkCalls(spy.mock.calls[0], results); if (done) { done(); } }, }); } exports.testLinkResults = testLinkResults; var templateObject_1; //# sourceMappingURL=testingUtils.js.map apollo-server-demo/node_modules/apollo-link/lib/test-utils/setContext.d.ts.map 0000644 0001750 0000144 00000000766 03560116604 027253 0 ustar andreh users {"version":3,"file":"setContext.d.ts","sourceRoot":"","sources":["../src/test-utils/setContext.ts"],"names":[],"mappings":"AAAA,OAAO,EAAE,SAAS,EAAE,QAAQ,EAAE,WAAW,EAAE,MAAM,UAAU,CAAC;AAE5D,OAAO,UAAU,MAAM,mBAAmB,CAAC;AAE3C,OAAO,EAAE,UAAU,EAAE,MAAM,SAAS,CAAC;AAErC,MAAM,CAAC,OAAO,OAAO,cAAe,SAAQ,UAAU;IAElD,OAAO,CAAC,UAAU;gBAAV,UAAU,GAAE,CAClB,OAAO,EAAE,MAAM,CAAC,MAAM,EAAE,GAAG,CAAC,KACzB,MAAM,CAAC,MAAM,EAAE,GAAG,CAAU;IAK5B,OAAO,CACZ,SAAS,EAAE,SAAS,EACpB,OAAO,EAAE,QAAQ,GAChB,UAAU,CAAC,WAAW,CAAC;CAI3B"} apollo-server-demo/node_modules/apollo-link/lib/test-utils/testingUtils.d.ts.map 0000644 0001750 0000144 00000001011 03560116604 027571 0 ustar andreh users {"version":3,"file":"testingUtils.d.ts","sourceRoot":"","sources":["../src/test-utils/testingUtils.ts"],"names":[],"mappings":"AACA,OAAO,EAAW,UAAU,EAAE,MAAM,SAAS,CAAC;AAU9C,wBAAgB,UAAU,CAAC,CAAC,EAAE,KAAK,EAAE,GAAG,EAAO,EAAE,OAAO,EAAE,KAAK,CAAC,CAAC,CAAC,QAGjE;AAED,MAAM,WAAW,cAAc;IAC7B,IAAI,EAAE,UAAU,CAAC;IACjB,OAAO,CAAC,EAAE,GAAG,EAAE,CAAC;IAChB,KAAK,CAAC,EAAE,MAAM,CAAC;IACf,IAAI,CAAC,EAAE,MAAM,IAAI,CAAC;IAClB,OAAO,CAAC,EAAE,GAAG,CAAC;IACd,SAAS,CAAC,EAAE,GAAG,CAAC;CACjB;AAED,wBAAgB,eAAe,CAAC,MAAM,EAAE,cAAc,QAuBrD"} apollo-server-demo/node_modules/apollo-link/lib/test-utils/mockLink.js.map 0000644 0001750 0000144 00000000640 03560116604 026415 0 ustar andreh users {"version":3,"file":"mockLink.js","sourceRoot":"","sources":["../../src/test-utils/mockLink.ts"],"names":[],"mappings":";;;AAIA,gCAAqC;AAErC;IAAsC,oCAAU;IAC9C,kBAAY,aAA0C;QAA1C,8BAAA,EAAA,8BAAsC,OAAA,IAAI,EAAJ,CAAI;QAAtD,YACE,iBAAO,SAER;QADC,KAAI,CAAC,OAAO,GAAG,aAAa,CAAC;;IAC/B,CAAC;IAEM,0BAAO,GAAd,UACE,SAAoB,EACpB,OAAkB;QAElB,MAAM,KAAK,CAAC,sBAAsB,CAAC,CAAC;IACtC,CAAC;IACH,eAAC;AAAD,CAAC,AAZD,CAAsC,iBAAU,GAY/C"} apollo-server-demo/node_modules/apollo-link/lib/test-utils/setContext.d.ts 0000644 0001750 0000144 00000000666 03560116604 026476 0 ustar andreh users import { Operation, NextLink, FetchResult } from '../types'; import Observable from 'zen-observable-ts'; import { ApolloLink } from '../link'; export default class SetContextLink extends ApolloLink { private setContext; constructor(setContext?: (context: Record<string, any>) => Record<string, any>); request(operation: Operation, forward: NextLink): Observable<FetchResult>; } //# sourceMappingURL=setContext.d.ts.map apollo-server-demo/node_modules/apollo-link/lib/test-utils/mockLink.d.ts.map 0000644 0001750 0000144 00000000653 03560116604 026655 0 ustar andreh users {"version":3,"file":"mockLink.d.ts","sourceRoot":"","sources":["../src/test-utils/mockLink.ts"],"names":[],"mappings":"AAAA,OAAO,EAAE,SAAS,EAAE,cAAc,EAAE,QAAQ,EAAE,WAAW,EAAE,MAAM,UAAU,CAAC;AAE5E,OAAO,UAAU,MAAM,mBAAmB,CAAC;AAE3C,OAAO,EAAE,UAAU,EAAE,MAAM,SAAS,CAAC;AAErC,MAAM,CAAC,OAAO,OAAO,QAAS,SAAQ,UAAU;gBAClC,aAAa,GAAE,cAA2B;IAK/C,OAAO,CACZ,SAAS,EAAE,SAAS,EACpB,OAAO,CAAC,EAAE,QAAQ,GACjB,UAAU,CAAC,WAAW,CAAC,GAAG,IAAI;CAGlC"} apollo-server-demo/node_modules/apollo-link/lib/test-utils/testingUtils.js.map 0000644 0001750 0000144 00000002505 03560116604 027346 0 ustar andreh users {"version":3,"file":"testingUtils.js","sourceRoot":"","sources":["../../src/test-utils/testingUtils.ts"],"names":[],"mappings":";;;AAAA,oEAA8B;AAC9B,gCAA8C;AAE9C,IAAM,WAAW,GAAG,qBAAG,wIAAA,6DAMtB,IAAA,CAAC;AAEF,SAAgB,UAAU,CAAI,KAAiB,EAAE,OAAiB;IAApC,sBAAA,EAAA,UAAiB;IAC7C,MAAM,CAAC,KAAK,CAAC,MAAM,CAAC,CAAC,IAAI,CAAC,OAAO,CAAC,MAAM,CAAC,CAAC;IAC1C,KAAK,CAAC,GAAG,CAAC,UAAC,IAAI,EAAE,CAAC,IAAK,OAAA,MAAM,CAAC,IAAI,CAAC,IAAI,CAAC,CAAC,OAAO,CAAC,OAAO,CAAC,CAAC,CAAC,CAAC,EAArC,CAAqC,CAAC,CAAC;AAChE,CAAC;AAHD,gCAGC;AAWD,SAAgB,eAAe,CAAC,MAAsB;IAC5C,IAAA,kBAAI,EAAE,wBAAO,EAAE,4BAAS,CAAY;IAC5C,IAAM,OAAO,GAAG,MAAM,CAAC,OAAO,IAAI,EAAE,CAAC;IACrC,IAAM,KAAK,GAAG,MAAM,CAAC,KAAK,IAAI,WAAW,CAAC;IAC1C,IAAM,IAAI,GAAG,MAAM,CAAC,IAAI,IAAI,CAAC,cAAM,OAAA,KAAK,CAAC,EAAN,CAAM,CAAC,CAAC;IAE3C,IAAM,GAAG,GAAG,IAAI,CAAC,EAAE,EAAE,CAAC;IACtB,cAAO,CAAC,IAAI,EAAE,EAAE,KAAK,OAAA,EAAE,OAAO,SAAA,EAAE,SAAS,WAAA,EAAE,CAAC,CAAC,SAAS,CAAC;QACrD,IAAI,EAAE,GAAG;QACT,KAAK,EAAE,UAAA,KAAK;YACV,MAAM,CAAC,KAAK,CAAC,CAAC,OAAO,CAAC,OAAO,CAAC,GAAG,EAAE,CAAC,CAAC;YACrC,UAAU,CAAC,GAAG,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC,EAAE,OAAO,CAAC,CAAC;YACvC,IAAI,IAAI,EAAE;gBACR,IAAI,EAAE,CAAC;aACR;QACH,CAAC;QACD,QAAQ,EAAE;YACR,UAAU,CAAC,GAAG,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC,CAAC,EAAE,OAAO,CAAC,CAAC;YACvC,IAAI,IAAI,EAAE;gBACR,IAAI,EAAE,CAAC;aACR;QACH,CAAC;KACF,CAAC,CAAC;AACL,CAAC;AAvBD,0CAuBC"} apollo-server-demo/node_modules/apollo-link/lib/index.js.map 0000644 0001750 0000144 00000000446 03560116604 023644 0 ustar andreh users {"version":3,"file":"index.js","sourceRoot":"","sources":["../src/index.ts"],"names":[],"mappings":";;;AAAA,iDAAuB;AACvB,yCAOqB;AANnB,sCAAA,eAAe,CAAA;AACf,kCAAA,WAAW,CAAA;AACX,gCAAA,SAAS,CAAA;AACT,kCAAA,WAAW,CAAA;AACX,gCAAA,SAAS,CAAA;AACT,uCAAA,gBAAgB,CAAA;AAIlB,gFAA2C;AAClC,qBADF,2BAAU,CACE"} apollo-server-demo/node_modules/apollo-link/lib/bundle.esm.js.map 0000644 0001750 0000144 00000030434 03560116604 024571 0 ustar andreh users {"version":3,"file":"bundle.esm.js","sources":["../src/linkUtils.ts","../src/link.ts"],"sourcesContent":["import Observable from 'zen-observable-ts';\n\nimport { GraphQLRequest, Operation } from './types';\nimport { ApolloLink } from './link';\n\nimport { getOperationName } from 'apollo-utilities';\nimport { invariant, InvariantError } from 'ts-invariant';\nexport { getOperationName };\n\nexport function validateOperation(operation: GraphQLRequest): GraphQLRequest {\n const OPERATION_FIELDS = [\n 'query',\n 'operationName',\n 'variables',\n 'extensions',\n 'context',\n ];\n for (let key of Object.keys(operation)) {\n if (OPERATION_FIELDS.indexOf(key) < 0) {\n throw new InvariantError(`illegal argument: ${key}`);\n }\n }\n\n return operation;\n}\n\nexport class LinkError extends Error {\n public link: ApolloLink;\n constructor(message?: string, link?: ApolloLink) {\n super(message);\n this.link = link;\n }\n}\n\nexport function isTerminating(link: ApolloLink): boolean {\n return link.request.length <= 1;\n}\n\nexport function toPromise<R>(observable: Observable<R>): Promise<R> {\n let completed = false;\n return new Promise<R>((resolve, reject) => {\n observable.subscribe({\n next: data => {\n if (completed) {\n invariant.warn(\n `Promise Wrapper does not support multiple results from Observable`,\n );\n } else {\n completed = true;\n resolve(data);\n }\n },\n error: reject,\n });\n });\n}\n\n// backwards compat\nexport const makePromise = toPromise;\n\nexport function fromPromise<T>(promise: Promise<T>): Observable<T> {\n return new Observable<T>(observer => {\n promise\n .then((value: T) => {\n observer.next(value);\n observer.complete();\n })\n .catch(observer.error.bind(observer));\n });\n}\n\nexport function fromError<T>(errorValue: any): Observable<T> {\n return new Observable<T>(observer => {\n observer.error(errorValue);\n });\n}\n\nexport function transformOperation(operation: GraphQLRequest): GraphQLRequest {\n const transformedOperation: GraphQLRequest = {\n variables: operation.variables || {},\n extensions: operation.extensions || {},\n operationName: operation.operationName,\n query: operation.query,\n };\n\n // best guess at an operation name\n if (!transformedOperation.operationName) {\n transformedOperation.operationName =\n typeof transformedOperation.query !== 'string'\n ? getOperationName(transformedOperation.query)\n : '';\n }\n\n return transformedOperation as Operation;\n}\n\nexport function createOperation(\n starting: any,\n operation: GraphQLRequest,\n): Operation {\n let context = { ...starting };\n const setContext = next => {\n if (typeof next === 'function') {\n context = { ...context, ...next(context) };\n } else {\n context = { ...context, ...next };\n }\n };\n const getContext = () => ({ ...context });\n\n Object.defineProperty(operation, 'setContext', {\n enumerable: false,\n value: setContext,\n });\n\n Object.defineProperty(operation, 'getContext', {\n enumerable: false,\n value: getContext,\n });\n\n Object.defineProperty(operation, 'toKey', {\n enumerable: false,\n value: () => getKey(operation),\n });\n\n return operation as Operation;\n}\n\nexport function getKey(operation: GraphQLRequest) {\n // XXX We're assuming here that query and variables will be serialized in\n // the same order, which might not always be true.\n const { query, variables, operationName } = operation;\n return JSON.stringify([operationName, query, variables]);\n}\n","import Observable from 'zen-observable-ts';\nimport { invariant, InvariantError } from 'ts-invariant';\n\nimport {\n GraphQLRequest,\n NextLink,\n Operation,\n RequestHandler,\n FetchResult,\n} from './types';\n\nimport {\n validateOperation,\n isTerminating,\n LinkError,\n transformOperation,\n createOperation,\n} from './linkUtils';\n\nfunction passthrough(op, forward) {\n return forward ? forward(op) : Observable.of();\n}\n\nfunction toLink(handler: RequestHandler | ApolloLink) {\n return typeof handler === 'function' ? new ApolloLink(handler) : handler;\n}\n\nexport function empty(): ApolloLink {\n return new ApolloLink(() => Observable.of());\n}\n\nexport function from(links: ApolloLink[]): ApolloLink {\n if (links.length === 0) return empty();\n return links.map(toLink).reduce((x, y) => x.concat(y));\n}\n\nexport function split(\n test: (op: Operation) => boolean,\n left: ApolloLink | RequestHandler,\n right?: ApolloLink | RequestHandler,\n): ApolloLink {\n const leftLink = toLink(left);\n const rightLink = toLink(right || new ApolloLink(passthrough));\n\n if (isTerminating(leftLink) && isTerminating(rightLink)) {\n return new ApolloLink(operation => {\n return test(operation)\n ? leftLink.request(operation) || Observable.of()\n : rightLink.request(operation) || Observable.of();\n });\n } else {\n return new ApolloLink((operation, forward) => {\n return test(operation)\n ? leftLink.request(operation, forward) || Observable.of()\n : rightLink.request(operation, forward) || Observable.of();\n });\n }\n}\n\n// join two Links together\nexport const concat = (\n first: ApolloLink | RequestHandler,\n second: ApolloLink | RequestHandler,\n) => {\n const firstLink = toLink(first);\n if (isTerminating(firstLink)) {\n invariant.warn(\n new LinkError(\n `You are calling concat on a terminating link, which will have no effect`,\n firstLink,\n ),\n );\n return firstLink;\n }\n const nextLink = toLink(second);\n\n if (isTerminating(nextLink)) {\n return new ApolloLink(\n operation =>\n firstLink.request(\n operation,\n op => nextLink.request(op) || Observable.of(),\n ) || Observable.of(),\n );\n } else {\n return new ApolloLink((operation, forward) => {\n return (\n firstLink.request(operation, op => {\n return nextLink.request(op, forward) || Observable.of();\n }) || Observable.of()\n );\n });\n }\n};\n\nexport class ApolloLink {\n public static empty = empty;\n public static from = from;\n public static split = split;\n public static execute = execute;\n\n constructor(request?: RequestHandler) {\n if (request) this.request = request;\n }\n\n public split(\n test: (op: Operation) => boolean,\n left: ApolloLink | RequestHandler,\n right?: ApolloLink | RequestHandler,\n ): ApolloLink {\n return this.concat(split(test, left, right || new ApolloLink(passthrough)));\n }\n\n public concat(next: ApolloLink | RequestHandler): ApolloLink {\n return concat(this, next);\n }\n\n public request(\n operation: Operation,\n forward?: NextLink,\n ): Observable<FetchResult> | null {\n throw new InvariantError('request is not implemented');\n }\n}\n\nexport function execute(\n link: ApolloLink,\n operation: GraphQLRequest,\n): Observable<FetchResult> {\n return (\n link.request(\n createOperation(\n operation.context,\n transformOperation(validateOperation(operation)),\n ),\n ) || Observable.of()\n );\n}\n"],"names":["tslib_1.__extends"],"mappings":";;;;;;;SASgB,iBAAiB,CAAC,SAAyB;IACzD,IAAM,gBAAgB,GAAG;QACvB,OAAO;QACP,eAAe;QACf,WAAW;QACX,YAAY;QACZ,SAAS;KACV,CAAC;IACF,KAAgB,UAAsB,EAAtB,KAAA,MAAM,CAAC,IAAI,CAAC,SAAS,CAAC,EAAtB,cAAsB,EAAtB,IAAsB,EAAE;QAAnC,IAAI,GAAG,SAAA;QACV,IAAI,gBAAgB,CAAC,OAAO,CAAC,GAAG,CAAC,GAAG,CAAC,EAAE;YACrC,MAAM;SACP;KACF;IAED,OAAO,SAAS,CAAC;AACnB,CAAC;AAED;IAA+BA,6BAAK;IAElC,mBAAY,OAAgB,EAAE,IAAiB;QAA/C,YACE,kBAAM,OAAO,CAAC,SAEf;QADC,KAAI,CAAC,IAAI,GAAG,IAAI,CAAC;;KAClB;IACH,gBAAC;AAAD,CANA,CAA+B,KAAK,GAMnC;SAEe,aAAa,CAAC,IAAgB;IAC5C,OAAO,IAAI,CAAC,OAAO,CAAC,MAAM,IAAI,CAAC,CAAC;AAClC,CAAC;SAEe,SAAS,CAAI,UAAyB;IACpD,IAAI,SAAS,GAAG,KAAK,CAAC;IACtB,OAAO,IAAI,OAAO,CAAI,UAAC,OAAO,EAAE,MAAM;QACpC,UAAU,CAAC,SAAS,CAAC;YACnB,IAAI,EAAE,UAAA,IAAI;gBACR,IAAI,SAAS,EAAE;oBACb;iBAGD;qBAAM;oBACL,SAAS,GAAG,IAAI,CAAC;oBACjB,OAAO,CAAC,IAAI,CAAC,CAAC;iBACf;aACF;YACD,KAAK,EAAE,MAAM;SACd,CAAC,CAAC;KACJ,CAAC,CAAC;AACL,CAAC;IAGY,WAAW,GAAG,UAAU;SAErB,WAAW,CAAI,OAAmB;IAChD,OAAO,IAAI,UAAU,CAAI,UAAA,QAAQ;QAC/B,OAAO;aACJ,IAAI,CAAC,UAAC,KAAQ;YACb,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC;YACrB,QAAQ,CAAC,QAAQ,EAAE,CAAC;SACrB,CAAC;aACD,KAAK,CAAC,QAAQ,CAAC,KAAK,CAAC,IAAI,CAAC,QAAQ,CAAC,CAAC,CAAC;KACzC,CAAC,CAAC;AACL,CAAC;SAEe,SAAS,CAAI,UAAe;IAC1C,OAAO,IAAI,UAAU,CAAI,UAAA,QAAQ;QAC/B,QAAQ,CAAC,KAAK,CAAC,UAAU,CAAC,CAAC;KAC5B,CAAC,CAAC;AACL,CAAC;SAEe,kBAAkB,CAAC,SAAyB;IAC1D,IAAM,oBAAoB,GAAmB;QAC3C,SAAS,EAAE,SAAS,CAAC,SAAS,IAAI,EAAE;QACpC,UAAU,EAAE,SAAS,CAAC,UAAU,IAAI,EAAE;QACtC,aAAa,EAAE,SAAS,CAAC,aAAa;QACtC,KAAK,EAAE,SAAS,CAAC,KAAK;KACvB,CAAC;IAGF,IAAI,CAAC,oBAAoB,CAAC,aAAa,EAAE;QACvC,oBAAoB,CAAC,aAAa;YAChC,OAAO,oBAAoB,CAAC,KAAK,KAAK,QAAQ;kBAC1C,gBAAgB,CAAC,oBAAoB,CAAC,KAAK,CAAC;kBAC5C,EAAE,CAAC;KACV;IAED,OAAO,oBAAiC,CAAC;AAC3C,CAAC;SAEe,eAAe,CAC7B,QAAa,EACb,SAAyB;IAEzB,IAAI,OAAO,gBAAQ,QAAQ,CAAE,CAAC;IAC9B,IAAM,UAAU,GAAG,UAAA,IAAI;QACrB,IAAI,OAAO,IAAI,KAAK,UAAU,EAAE;YAC9B,OAAO,gBAAQ,OAAO,EAAK,IAAI,CAAC,OAAO,CAAC,CAAE,CAAC;SAC5C;aAAM;YACL,OAAO,gBAAQ,OAAO,EAAK,IAAI,CAAE,CAAC;SACnC;KACF,CAAC;IACF,IAAM,UAAU,GAAG,cAAM,qBAAM,OAAO,KAAG,CAAC;IAE1C,MAAM,CAAC,cAAc,CAAC,SAAS,EAAE,YAAY,EAAE;QAC7C,UAAU,EAAE,KAAK;QACjB,KAAK,EAAE,UAAU;KAClB,CAAC,CAAC;IAEH,MAAM,CAAC,cAAc,CAAC,SAAS,EAAE,YAAY,EAAE;QAC7C,UAAU,EAAE,KAAK;QACjB,KAAK,EAAE,UAAU;KAClB,CAAC,CAAC;IAEH,MAAM,CAAC,cAAc,CAAC,SAAS,EAAE,OAAO,EAAE;QACxC,UAAU,EAAE,KAAK;QACjB,KAAK,EAAE,cAAM,OAAA,MAAM,CAAC,SAAS,CAAC,GAAA;KAC/B,CAAC,CAAC;IAEH,OAAO,SAAsB,CAAC;AAChC,CAAC;SAEe,MAAM,CAAC,SAAyB;IAGtC,IAAA,uBAAK,EAAE,+BAAS,EAAE,uCAAa,CAAe;IACtD,OAAO,IAAI,CAAC,SAAS,CAAC,CAAC,aAAa,EAAE,KAAK,EAAE,SAAS,CAAC,CAAC,CAAC;AAC3D;;AClHA,SAAS,WAAW,CAAC,EAAE,EAAE,OAAO;IAC9B,OAAO,OAAO,GAAG,OAAO,CAAC,EAAE,CAAC,GAAG,UAAU,CAAC,EAAE,EAAE,CAAC;AACjD,CAAC;AAED,SAAS,MAAM,CAAC,OAAoC;IAClD,OAAO,OAAO,OAAO,KAAK,UAAU,GAAG,IAAI,UAAU,CAAC,OAAO,CAAC,GAAG,OAAO,CAAC;AAC3E,CAAC;AAED,SAAgB,KAAK;IACnB,OAAO,IAAI,UAAU,CAAC,cAAM,OAAA,UAAU,CAAC,EAAE,EAAE,GAAA,CAAC,CAAC;AAC/C,CAAC;AAED,SAAgB,IAAI,CAAC,KAAmB;IACtC,IAAI,KAAK,CAAC,MAAM,KAAK,CAAC;QAAE,OAAO,KAAK,EAAE,CAAC;IACvC,OAAO,KAAK,CAAC,GAAG,CAAC,MAAM,CAAC,CAAC,MAAM,CAAC,UAAC,CAAC,EAAE,CAAC,IAAK,OAAA,CAAC,CAAC,MAAM,CAAC,CAAC,CAAC,GAAA,CAAC,CAAC;AACzD,CAAC;AAED,SAAgB,KAAK,CACnB,IAAgC,EAChC,IAAiC,EACjC,KAAmC;IAEnC,IAAM,QAAQ,GAAG,MAAM,CAAC,IAAI,CAAC,CAAC;IAC9B,IAAM,SAAS,GAAG,MAAM,CAAC,KAAK,IAAI,IAAI,UAAU,CAAC,WAAW,CAAC,CAAC,CAAC;IAE/D,IAAI,aAAa,CAAC,QAAQ,CAAC,IAAI,aAAa,CAAC,SAAS,CAAC,EAAE;QACvD,OAAO,IAAI,UAAU,CAAC,UAAA,SAAS;YAC7B,OAAO,IAAI,CAAC,SAAS,CAAC;kBAClB,QAAQ,CAAC,OAAO,CAAC,SAAS,CAAC,IAAI,UAAU,CAAC,EAAE,EAAE;kBAC9C,SAAS,CAAC,OAAO,CAAC,SAAS,CAAC,IAAI,UAAU,CAAC,EAAE,EAAE,CAAC;SACrD,CAAC,CAAC;KACJ;SAAM;QACL,OAAO,IAAI,UAAU,CAAC,UAAC,SAAS,EAAE,OAAO;YACvC,OAAO,IAAI,CAAC,SAAS,CAAC;kBAClB,QAAQ,CAAC,OAAO,CAAC,SAAS,EAAE,OAAO,CAAC,IAAI,UAAU,CAAC,EAAE,EAAE;kBACvD,SAAS,CAAC,OAAO,CAAC,SAAS,EAAE,OAAO,CAAC,IAAI,UAAU,CAAC,EAAE,EAAE,CAAC;SAC9D,CAAC,CAAC;KACJ;AACH,CAAC;AAGD,IAAa,MAAM,GAAG,UACpB,KAAkC,EAClC,MAAmC;IAEnC,IAAM,SAAS,GAAG,MAAM,CAAC,KAAK,CAAC,CAAC;IAChC,IAAI,aAAa,CAAC,SAAS,CAAC,EAAE;QAC5B;QAMA,OAAO,SAAS,CAAC;KAClB;IACD,IAAM,QAAQ,GAAG,MAAM,CAAC,MAAM,CAAC,CAAC;IAEhC,IAAI,aAAa,CAAC,QAAQ,CAAC,EAAE;QAC3B,OAAO,IAAI,UAAU,CACnB,UAAA,SAAS;YACP,OAAA,SAAS,CAAC,OAAO,CACf,SAAS,EACT,UAAA,EAAE,IAAI,OAAA,QAAQ,CAAC,OAAO,CAAC,EAAE,CAAC,IAAI,UAAU,CAAC,EAAE,EAAE,GAAA,CAC9C,IAAI,UAAU,CAAC,EAAE,EAAE;SAAA,CACvB,CAAC;KACH;SAAM;QACL,OAAO,IAAI,UAAU,CAAC,UAAC,SAAS,EAAE,OAAO;YACvC,QACE,SAAS,CAAC,OAAO,CAAC,SAAS,EAAE,UAAA,EAAE;gBAC7B,OAAO,QAAQ,CAAC,OAAO,CAAC,EAAE,EAAE,OAAO,CAAC,IAAI,UAAU,CAAC,EAAE,EAAE,CAAC;aACzD,CAAC,IAAI,UAAU,CAAC,EAAE,EAAE,EACrB;SACH,CAAC,CAAC;KACJ;AACH,CAAC,CAAC;AAEF;IAME,oBAAY,OAAwB;QAClC,IAAI,OAAO;YAAE,IAAI,CAAC,OAAO,GAAG,OAAO,CAAC;KACrC;IAEM,0BAAK,GAAZ,UACE,IAAgC,EAChC,IAAiC,EACjC,KAAmC;QAEnC,OAAO,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,IAAI,EAAE,IAAI,EAAE,KAAK,IAAI,IAAI,UAAU,CAAC,WAAW,CAAC,CAAC,CAAC,CAAC;KAC7E;IAEM,2BAAM,GAAb,UAAc,IAAiC;QAC7C,OAAO,MAAM,CAAC,IAAI,EAAE,IAAI,CAAC,CAAC;KAC3B;IAEM,4BAAO,GAAd,UACE,SAAoB,EACpB,OAAkB;QAElB,MAAM;KACP;IA1Ba,gBAAK,GAAG,KAAK,CAAC;IACd,eAAI,GAAG,IAAI,CAAC;IACZ,gBAAK,GAAG,KAAK,CAAC;IACd,kBAAO,GAAG,OAAO,CAAC;IAwBlC,iBAAC;CA5BD,IA4BC;SAEe,OAAO,CACrB,IAAgB,EAChB,SAAyB;IAEzB,QACE,IAAI,CAAC,OAAO,CACV,eAAe,CACb,SAAS,CAAC,OAAO,EACjB,kBAAkB,CAAC,iBAAiB,CAAC,SAAS,CAAC,CAAC,CACjD,CACF,IAAI,UAAU,CAAC,EAAE,EAAE,EACpB;AACJ,CAAC;;;;"} apollo-server-demo/node_modules/apollo-link/lib/bundle.umd.js.map 0000644 0001750 0000144 00000030512 03560116604 024567 0 ustar andreh users {"version":3,"file":"bundle.umd.js","sources":["../src/linkUtils.ts","../src/link.ts"],"sourcesContent":["import Observable from 'zen-observable-ts';\n\nimport { GraphQLRequest, Operation } from './types';\nimport { ApolloLink } from './link';\n\nimport { getOperationName } from 'apollo-utilities';\nimport { invariant, InvariantError } from 'ts-invariant';\nexport { getOperationName };\n\nexport function validateOperation(operation: GraphQLRequest): GraphQLRequest {\n const OPERATION_FIELDS = [\n 'query',\n 'operationName',\n 'variables',\n 'extensions',\n 'context',\n ];\n for (let key of Object.keys(operation)) {\n if (OPERATION_FIELDS.indexOf(key) < 0) {\n throw new InvariantError(`illegal argument: ${key}`);\n }\n }\n\n return operation;\n}\n\nexport class LinkError extends Error {\n public link: ApolloLink;\n constructor(message?: string, link?: ApolloLink) {\n super(message);\n this.link = link;\n }\n}\n\nexport function isTerminating(link: ApolloLink): boolean {\n return link.request.length <= 1;\n}\n\nexport function toPromise<R>(observable: Observable<R>): Promise<R> {\n let completed = false;\n return new Promise<R>((resolve, reject) => {\n observable.subscribe({\n next: data => {\n if (completed) {\n invariant.warn(\n `Promise Wrapper does not support multiple results from Observable`,\n );\n } else {\n completed = true;\n resolve(data);\n }\n },\n error: reject,\n });\n });\n}\n\n// backwards compat\nexport const makePromise = toPromise;\n\nexport function fromPromise<T>(promise: Promise<T>): Observable<T> {\n return new Observable<T>(observer => {\n promise\n .then((value: T) => {\n observer.next(value);\n observer.complete();\n })\n .catch(observer.error.bind(observer));\n });\n}\n\nexport function fromError<T>(errorValue: any): Observable<T> {\n return new Observable<T>(observer => {\n observer.error(errorValue);\n });\n}\n\nexport function transformOperation(operation: GraphQLRequest): GraphQLRequest {\n const transformedOperation: GraphQLRequest = {\n variables: operation.variables || {},\n extensions: operation.extensions || {},\n operationName: operation.operationName,\n query: operation.query,\n };\n\n // best guess at an operation name\n if (!transformedOperation.operationName) {\n transformedOperation.operationName =\n typeof transformedOperation.query !== 'string'\n ? getOperationName(transformedOperation.query)\n : '';\n }\n\n return transformedOperation as Operation;\n}\n\nexport function createOperation(\n starting: any,\n operation: GraphQLRequest,\n): Operation {\n let context = { ...starting };\n const setContext = next => {\n if (typeof next === 'function') {\n context = { ...context, ...next(context) };\n } else {\n context = { ...context, ...next };\n }\n };\n const getContext = () => ({ ...context });\n\n Object.defineProperty(operation, 'setContext', {\n enumerable: false,\n value: setContext,\n });\n\n Object.defineProperty(operation, 'getContext', {\n enumerable: false,\n value: getContext,\n });\n\n Object.defineProperty(operation, 'toKey', {\n enumerable: false,\n value: () => getKey(operation),\n });\n\n return operation as Operation;\n}\n\nexport function getKey(operation: GraphQLRequest) {\n // XXX We're assuming here that query and variables will be serialized in\n // the same order, which might not always be true.\n const { query, variables, operationName } = operation;\n return JSON.stringify([operationName, query, variables]);\n}\n","import Observable from 'zen-observable-ts';\nimport { invariant, InvariantError } from 'ts-invariant';\n\nimport {\n GraphQLRequest,\n NextLink,\n Operation,\n RequestHandler,\n FetchResult,\n} from './types';\n\nimport {\n validateOperation,\n isTerminating,\n LinkError,\n transformOperation,\n createOperation,\n} from './linkUtils';\n\nfunction passthrough(op, forward) {\n return forward ? forward(op) : Observable.of();\n}\n\nfunction toLink(handler: RequestHandler | ApolloLink) {\n return typeof handler === 'function' ? new ApolloLink(handler) : handler;\n}\n\nexport function empty(): ApolloLink {\n return new ApolloLink(() => Observable.of());\n}\n\nexport function from(links: ApolloLink[]): ApolloLink {\n if (links.length === 0) return empty();\n return links.map(toLink).reduce((x, y) => x.concat(y));\n}\n\nexport function split(\n test: (op: Operation) => boolean,\n left: ApolloLink | RequestHandler,\n right?: ApolloLink | RequestHandler,\n): ApolloLink {\n const leftLink = toLink(left);\n const rightLink = toLink(right || new ApolloLink(passthrough));\n\n if (isTerminating(leftLink) && isTerminating(rightLink)) {\n return new ApolloLink(operation => {\n return test(operation)\n ? leftLink.request(operation) || Observable.of()\n : rightLink.request(operation) || Observable.of();\n });\n } else {\n return new ApolloLink((operation, forward) => {\n return test(operation)\n ? leftLink.request(operation, forward) || Observable.of()\n : rightLink.request(operation, forward) || Observable.of();\n });\n }\n}\n\n// join two Links together\nexport const concat = (\n first: ApolloLink | RequestHandler,\n second: ApolloLink | RequestHandler,\n) => {\n const firstLink = toLink(first);\n if (isTerminating(firstLink)) {\n invariant.warn(\n new LinkError(\n `You are calling concat on a terminating link, which will have no effect`,\n firstLink,\n ),\n );\n return firstLink;\n }\n const nextLink = toLink(second);\n\n if (isTerminating(nextLink)) {\n return new ApolloLink(\n operation =>\n firstLink.request(\n operation,\n op => nextLink.request(op) || Observable.of(),\n ) || Observable.of(),\n );\n } else {\n return new ApolloLink((operation, forward) => {\n return (\n firstLink.request(operation, op => {\n return nextLink.request(op, forward) || Observable.of();\n }) || Observable.of()\n );\n });\n }\n};\n\nexport class ApolloLink {\n public static empty = empty;\n public static from = from;\n public static split = split;\n public static execute = execute;\n\n constructor(request?: RequestHandler) {\n if (request) this.request = request;\n }\n\n public split(\n test: (op: Operation) => boolean,\n left: ApolloLink | RequestHandler,\n right?: ApolloLink | RequestHandler,\n ): ApolloLink {\n return this.concat(split(test, left, right || new ApolloLink(passthrough)));\n }\n\n public concat(next: ApolloLink | RequestHandler): ApolloLink {\n return concat(this, next);\n }\n\n public request(\n operation: Operation,\n forward?: NextLink,\n ): Observable<FetchResult> | null {\n throw new InvariantError('request is not implemented');\n }\n}\n\nexport function execute(\n link: ApolloLink,\n operation: GraphQLRequest,\n): Observable<FetchResult> {\n return (\n link.request(\n createOperation(\n operation.context,\n transformOperation(validateOperation(operation)),\n ),\n ) || Observable.of()\n );\n}\n"],"names":["tslib_1.__extends","getOperationName"],"mappings":";;;;;;;;WASgB,iBAAiB,CAAC,SAAyB;MACzD,IAAM,gBAAgB,GAAG;UACvB,OAAO;UACP,eAAe;UACf,WAAW;UACX,YAAY;UACZ,SAAS;OACV,CAAC;MACF,KAAgB,UAAsB,EAAtB,KAAA,MAAM,CAAC,IAAI,CAAC,SAAS,CAAC,EAAtB,cAAsB,EAAtB,IAAsB,EAAE;UAAnC,IAAI,GAAG,SAAA;UACV,IAAI,gBAAgB,CAAC,OAAO,CAAC,GAAG,CAAC,GAAG,CAAC,EAAE;cACrC,MAAM;WACP;OACF;MAED,OAAO,SAAS,CAAC;EACnB,CAAC;EAED;MAA+BA,qCAAK;MAElC,mBAAY,OAAgB,EAAE,IAAiB;UAA/C,YACE,kBAAM,OAAO,CAAC,SAEf;UADC,KAAI,CAAC,IAAI,GAAG,IAAI,CAAC;;OAClB;MACH,gBAAC;EAAD,CANA,CAA+B,KAAK,GAMnC;WAEe,aAAa,CAAC,IAAgB;MAC5C,OAAO,IAAI,CAAC,OAAO,CAAC,MAAM,IAAI,CAAC,CAAC;EAClC,CAAC;WAEe,SAAS,CAAI,UAAyB;MACpD,IAAI,SAAS,GAAG,KAAK,CAAC;MACtB,OAAO,IAAI,OAAO,CAAI,UAAC,OAAO,EAAE,MAAM;UACpC,UAAU,CAAC,SAAS,CAAC;cACnB,IAAI,EAAE,UAAA,IAAI;kBACR,IAAI,SAAS,EAAE;sBACb;mBAGD;uBAAM;sBACL,SAAS,GAAG,IAAI,CAAC;sBACjB,OAAO,CAAC,IAAI,CAAC,CAAC;mBACf;eACF;cACD,KAAK,EAAE,MAAM;WACd,CAAC,CAAC;OACJ,CAAC,CAAC;EACL,CAAC;MAGY,WAAW,GAAG,UAAU;WAErB,WAAW,CAAI,OAAmB;MAChD,OAAO,IAAI,UAAU,CAAI,UAAA,QAAQ;UAC/B,OAAO;eACJ,IAAI,CAAC,UAAC,KAAQ;cACb,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC;cACrB,QAAQ,CAAC,QAAQ,EAAE,CAAC;WACrB,CAAC;eACD,KAAK,CAAC,QAAQ,CAAC,KAAK,CAAC,IAAI,CAAC,QAAQ,CAAC,CAAC,CAAC;OACzC,CAAC,CAAC;EACL,CAAC;WAEe,SAAS,CAAI,UAAe;MAC1C,OAAO,IAAI,UAAU,CAAI,UAAA,QAAQ;UAC/B,QAAQ,CAAC,KAAK,CAAC,UAAU,CAAC,CAAC;OAC5B,CAAC,CAAC;EACL,CAAC;WAEe,kBAAkB,CAAC,SAAyB;MAC1D,IAAM,oBAAoB,GAAmB;UAC3C,SAAS,EAAE,SAAS,CAAC,SAAS,IAAI,EAAE;UACpC,UAAU,EAAE,SAAS,CAAC,UAAU,IAAI,EAAE;UACtC,aAAa,EAAE,SAAS,CAAC,aAAa;UACtC,KAAK,EAAE,SAAS,CAAC,KAAK;OACvB,CAAC;MAGF,IAAI,CAAC,oBAAoB,CAAC,aAAa,EAAE;UACvC,oBAAoB,CAAC,aAAa;cAChC,OAAO,oBAAoB,CAAC,KAAK,KAAK,QAAQ;oBAC1CC,gCAAgB,CAAC,oBAAoB,CAAC,KAAK,CAAC;oBAC5C,EAAE,CAAC;OACV;MAED,OAAO,oBAAiC,CAAC;EAC3C,CAAC;WAEe,eAAe,CAC7B,QAAa,EACb,SAAyB;MAEzB,IAAI,OAAO,wBAAQ,QAAQ,CAAE,CAAC;MAC9B,IAAM,UAAU,GAAG,UAAA,IAAI;UACrB,IAAI,OAAO,IAAI,KAAK,UAAU,EAAE;cAC9B,OAAO,wBAAQ,OAAO,EAAK,IAAI,CAAC,OAAO,CAAC,CAAE,CAAC;WAC5C;eAAM;cACL,OAAO,wBAAQ,OAAO,EAAK,IAAI,CAAE,CAAC;WACnC;OACF,CAAC;MACF,IAAM,UAAU,GAAG,cAAM,6BAAM,OAAO,KAAG,CAAC;MAE1C,MAAM,CAAC,cAAc,CAAC,SAAS,EAAE,YAAY,EAAE;UAC7C,UAAU,EAAE,KAAK;UACjB,KAAK,EAAE,UAAU;OAClB,CAAC,CAAC;MAEH,MAAM,CAAC,cAAc,CAAC,SAAS,EAAE,YAAY,EAAE;UAC7C,UAAU,EAAE,KAAK;UACjB,KAAK,EAAE,UAAU;OAClB,CAAC,CAAC;MAEH,MAAM,CAAC,cAAc,CAAC,SAAS,EAAE,OAAO,EAAE;UACxC,UAAU,EAAE,KAAK;UACjB,KAAK,EAAE,cAAM,OAAA,MAAM,CAAC,SAAS,CAAC,GAAA;OAC/B,CAAC,CAAC;MAEH,OAAO,SAAsB,CAAC;EAChC,CAAC;WAEe,MAAM,CAAC,SAAyB;MAGtC,IAAA,uBAAK,EAAE,+BAAS,EAAE,uCAAa,CAAe;MACtD,OAAO,IAAI,CAAC,SAAS,CAAC,CAAC,aAAa,EAAE,KAAK,EAAE,SAAS,CAAC,CAAC,CAAC;EAC3D;;EClHA,SAAS,WAAW,CAAC,EAAE,EAAE,OAAO;MAC9B,OAAO,OAAO,GAAG,OAAO,CAAC,EAAE,CAAC,GAAG,UAAU,CAAC,EAAE,EAAE,CAAC;EACjD,CAAC;EAED,SAAS,MAAM,CAAC,OAAoC;MAClD,OAAO,OAAO,OAAO,KAAK,UAAU,GAAG,IAAI,UAAU,CAAC,OAAO,CAAC,GAAG,OAAO,CAAC;EAC3E,CAAC;AAED,WAAgB,KAAK;MACnB,OAAO,IAAI,UAAU,CAAC,cAAM,OAAA,UAAU,CAAC,EAAE,EAAE,GAAA,CAAC,CAAC;EAC/C,CAAC;AAED,WAAgB,IAAI,CAAC,KAAmB;MACtC,IAAI,KAAK,CAAC,MAAM,KAAK,CAAC;UAAE,OAAO,KAAK,EAAE,CAAC;MACvC,OAAO,KAAK,CAAC,GAAG,CAAC,MAAM,CAAC,CAAC,MAAM,CAAC,UAAC,CAAC,EAAE,CAAC,IAAK,OAAA,CAAC,CAAC,MAAM,CAAC,CAAC,CAAC,GAAA,CAAC,CAAC;EACzD,CAAC;AAED,WAAgB,KAAK,CACnB,IAAgC,EAChC,IAAiC,EACjC,KAAmC;MAEnC,IAAM,QAAQ,GAAG,MAAM,CAAC,IAAI,CAAC,CAAC;MAC9B,IAAM,SAAS,GAAG,MAAM,CAAC,KAAK,IAAI,IAAI,UAAU,CAAC,WAAW,CAAC,CAAC,CAAC;MAE/D,IAAI,aAAa,CAAC,QAAQ,CAAC,IAAI,aAAa,CAAC,SAAS,CAAC,EAAE;UACvD,OAAO,IAAI,UAAU,CAAC,UAAA,SAAS;cAC7B,OAAO,IAAI,CAAC,SAAS,CAAC;oBAClB,QAAQ,CAAC,OAAO,CAAC,SAAS,CAAC,IAAI,UAAU,CAAC,EAAE,EAAE;oBAC9C,SAAS,CAAC,OAAO,CAAC,SAAS,CAAC,IAAI,UAAU,CAAC,EAAE,EAAE,CAAC;WACrD,CAAC,CAAC;OACJ;WAAM;UACL,OAAO,IAAI,UAAU,CAAC,UAAC,SAAS,EAAE,OAAO;cACvC,OAAO,IAAI,CAAC,SAAS,CAAC;oBAClB,QAAQ,CAAC,OAAO,CAAC,SAAS,EAAE,OAAO,CAAC,IAAI,UAAU,CAAC,EAAE,EAAE;oBACvD,SAAS,CAAC,OAAO,CAAC,SAAS,EAAE,OAAO,CAAC,IAAI,UAAU,CAAC,EAAE,EAAE,CAAC;WAC9D,CAAC,CAAC;OACJ;EACH,CAAC;AAGD,MAAa,MAAM,GAAG,UACpB,KAAkC,EAClC,MAAmC;MAEnC,IAAM,SAAS,GAAG,MAAM,CAAC,KAAK,CAAC,CAAC;MAChC,IAAI,aAAa,CAAC,SAAS,CAAC,EAAE;UAC5B;UAMA,OAAO,SAAS,CAAC;OAClB;MACD,IAAM,QAAQ,GAAG,MAAM,CAAC,MAAM,CAAC,CAAC;MAEhC,IAAI,aAAa,CAAC,QAAQ,CAAC,EAAE;UAC3B,OAAO,IAAI,UAAU,CACnB,UAAA,SAAS;cACP,OAAA,SAAS,CAAC,OAAO,CACf,SAAS,EACT,UAAA,EAAE,IAAI,OAAA,QAAQ,CAAC,OAAO,CAAC,EAAE,CAAC,IAAI,UAAU,CAAC,EAAE,EAAE,GAAA,CAC9C,IAAI,UAAU,CAAC,EAAE,EAAE;WAAA,CACvB,CAAC;OACH;WAAM;UACL,OAAO,IAAI,UAAU,CAAC,UAAC,SAAS,EAAE,OAAO;cACvC,QACE,SAAS,CAAC,OAAO,CAAC,SAAS,EAAE,UAAA,EAAE;kBAC7B,OAAO,QAAQ,CAAC,OAAO,CAAC,EAAE,EAAE,OAAO,CAAC,IAAI,UAAU,CAAC,EAAE,EAAE,CAAC;eACzD,CAAC,IAAI,UAAU,CAAC,EAAE,EAAE,EACrB;WACH,CAAC,CAAC;OACJ;EACH,CAAC,CAAC;AAEF;MAME,oBAAY,OAAwB;UAClC,IAAI,OAAO;cAAE,IAAI,CAAC,OAAO,GAAG,OAAO,CAAC;OACrC;MAEM,0BAAK,GAAZ,UACE,IAAgC,EAChC,IAAiC,EACjC,KAAmC;UAEnC,OAAO,IAAI,CAAC,MAAM,CAAC,KAAK,CAAC,IAAI,EAAE,IAAI,EAAE,KAAK,IAAI,IAAI,UAAU,CAAC,WAAW,CAAC,CAAC,CAAC,CAAC;OAC7E;MAEM,2BAAM,GAAb,UAAc,IAAiC;UAC7C,OAAO,MAAM,CAAC,IAAI,EAAE,IAAI,CAAC,CAAC;OAC3B;MAEM,4BAAO,GAAd,UACE,SAAoB,EACpB,OAAkB;UAElB,MAAM;OACP;MA1Ba,gBAAK,GAAG,KAAK,CAAC;MACd,eAAI,GAAG,IAAI,CAAC;MACZ,gBAAK,GAAG,KAAK,CAAC;MACd,kBAAO,GAAG,OAAO,CAAC;MAwBlC,iBAAC;GA5BD,IA4BC;WAEe,OAAO,CACrB,IAAgB,EAChB,SAAyB;MAEzB,QACE,IAAI,CAAC,OAAO,CACV,eAAe,CACb,SAAS,CAAC,OAAO,EACjB,kBAAkB,CAAC,iBAAiB,CAAC,SAAS,CAAC,CAAC,CACjD,CACF,IAAI,UAAU,CAAC,EAAE,EAAE,EACpB;EACJ,CAAC;;;;;;;;;;;;;;;;;;;;;;;;;;;;;"} apollo-server-demo/node_modules/apollo-link/lib/linkUtils.js.map 0000644 0001750 0000144 00000005717 03560116604 024521 0 ustar andreh users {"version":3,"file":"linkUtils.js","sourceRoot":"","sources":["../src/linkUtils.ts"],"names":[],"mappings":";;;AAAA,gFAA2C;AAK3C,qDAAoD;AAE3C,2BAFA,mCAAgB,CAEA;AADzB,6CAAyD;AAGzD,SAAgB,iBAAiB,CAAC,SAAyB;IACzD,IAAM,gBAAgB,GAAG;QACvB,OAAO;QACP,eAAe;QACf,WAAW;QACX,YAAY;QACZ,SAAS;KACV,CAAC;IACF,KAAgB,UAAsB,EAAtB,KAAA,MAAM,CAAC,IAAI,CAAC,SAAS,CAAC,EAAtB,cAAsB,EAAtB,IAAsB,EAAE;QAAnC,IAAI,GAAG,SAAA;QACV,IAAI,gBAAgB,CAAC,OAAO,CAAC,GAAG,CAAC,GAAG,CAAC,EAAE;YACrC,MAAM,IAAI,6BAAc,CAAC,uBAAqB,GAAK,CAAC,CAAC;SACtD;KACF;IAED,OAAO,SAAS,CAAC;AACnB,CAAC;AAfD,8CAeC;AAED;IAA+B,qCAAK;IAElC,mBAAY,OAAgB,EAAE,IAAiB;QAA/C,YACE,kBAAM,OAAO,CAAC,SAEf;QADC,KAAI,CAAC,IAAI,GAAG,IAAI,CAAC;;IACnB,CAAC;IACH,gBAAC;AAAD,CAAC,AAND,CAA+B,KAAK,GAMnC;AANY,8BAAS;AAQtB,SAAgB,aAAa,CAAC,IAAgB;IAC5C,OAAO,IAAI,CAAC,OAAO,CAAC,MAAM,IAAI,CAAC,CAAC;AAClC,CAAC;AAFD,sCAEC;AAED,SAAgB,SAAS,CAAI,UAAyB;IACpD,IAAI,SAAS,GAAG,KAAK,CAAC;IACtB,OAAO,IAAI,OAAO,CAAI,UAAC,OAAO,EAAE,MAAM;QACpC,UAAU,CAAC,SAAS,CAAC;YACnB,IAAI,EAAE,UAAA,IAAI;gBACR,IAAI,SAAS,EAAE;oBACb,wBAAS,CAAC,IAAI,CACZ,mEAAmE,CACpE,CAAC;iBACH;qBAAM;oBACL,SAAS,GAAG,IAAI,CAAC;oBACjB,OAAO,CAAC,IAAI,CAAC,CAAC;iBACf;YACH,CAAC;YACD,KAAK,EAAE,MAAM;SACd,CAAC,CAAC;IACL,CAAC,CAAC,CAAC;AACL,CAAC;AAjBD,8BAiBC;AAGY,QAAA,WAAW,GAAG,SAAS,CAAC;AAErC,SAAgB,WAAW,CAAI,OAAmB;IAChD,OAAO,IAAI,2BAAU,CAAI,UAAA,QAAQ;QAC/B,OAAO;aACJ,IAAI,CAAC,UAAC,KAAQ;YACb,QAAQ,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC;YACrB,QAAQ,CAAC,QAAQ,EAAE,CAAC;QACtB,CAAC,CAAC;aACD,KAAK,CAAC,QAAQ,CAAC,KAAK,CAAC,IAAI,CAAC,QAAQ,CAAC,CAAC,CAAC;IAC1C,CAAC,CAAC,CAAC;AACL,CAAC;AATD,kCASC;AAED,SAAgB,SAAS,CAAI,UAAe;IAC1C,OAAO,IAAI,2BAAU,CAAI,UAAA,QAAQ;QAC/B,QAAQ,CAAC,KAAK,CAAC,UAAU,CAAC,CAAC;IAC7B,CAAC,CAAC,CAAC;AACL,CAAC;AAJD,8BAIC;AAED,SAAgB,kBAAkB,CAAC,SAAyB;IAC1D,IAAM,oBAAoB,GAAmB;QAC3C,SAAS,EAAE,SAAS,CAAC,SAAS,IAAI,EAAE;QACpC,UAAU,EAAE,SAAS,CAAC,UAAU,IAAI,EAAE;QACtC,aAAa,EAAE,SAAS,CAAC,aAAa;QACtC,KAAK,EAAE,SAAS,CAAC,KAAK;KACvB,CAAC;IAGF,IAAI,CAAC,oBAAoB,CAAC,aAAa,EAAE;QACvC,oBAAoB,CAAC,aAAa;YAChC,OAAO,oBAAoB,CAAC,KAAK,KAAK,QAAQ;gBAC5C,CAAC,CAAC,mCAAgB,CAAC,oBAAoB,CAAC,KAAK,CAAC;gBAC9C,CAAC,CAAC,EAAE,CAAC;KACV;IAED,OAAO,oBAAiC,CAAC;AAC3C,CAAC;AAjBD,gDAiBC;AAED,SAAgB,eAAe,CAC7B,QAAa,EACb,SAAyB;IAEzB,IAAI,OAAO,wBAAQ,QAAQ,CAAE,CAAC;IAC9B,IAAM,UAAU,GAAG,UAAA,IAAI;QACrB,IAAI,OAAO,IAAI,KAAK,UAAU,EAAE;YAC9B,OAAO,wBAAQ,OAAO,EAAK,IAAI,CAAC,OAAO,CAAC,CAAE,CAAC;SAC5C;aAAM;YACL,OAAO,wBAAQ,OAAO,EAAK,IAAI,CAAE,CAAC;SACnC;IACH,CAAC,CAAC;IACF,IAAM,UAAU,GAAG,cAAM,OAAA,sBAAM,OAAO,EAAG,EAAhB,CAAgB,CAAC;IAE1C,MAAM,CAAC,cAAc,CAAC,SAAS,EAAE,YAAY,EAAE;QAC7C,UAAU,EAAE,KAAK;QACjB,KAAK,EAAE,UAAU;KAClB,CAAC,CAAC;IAEH,MAAM,CAAC,cAAc,CAAC,SAAS,EAAE,YAAY,EAAE;QAC7C,UAAU,EAAE,KAAK;QACjB,KAAK,EAAE,UAAU;KAClB,CAAC,CAAC;IAEH,MAAM,CAAC,cAAc,CAAC,SAAS,EAAE,OAAO,EAAE;QACxC,UAAU,EAAE,KAAK;QACjB,KAAK,EAAE,cAAM,OAAA,MAAM,CAAC,SAAS,CAAC,EAAjB,CAAiB;KAC/B,CAAC,CAAC;IAEH,OAAO,SAAsB,CAAC;AAChC,CAAC;AA9BD,0CA8BC;AAED,SAAgB,MAAM,CAAC,SAAyB;IAGtC,IAAA,uBAAK,EAAE,+BAAS,EAAE,uCAAa,CAAe;IACtD,OAAO,IAAI,CAAC,SAAS,CAAC,CAAC,aAAa,EAAE,KAAK,EAAE,SAAS,CAAC,CAAC,CAAC;AAC3D,CAAC;AALD,wBAKC"} apollo-server-demo/node_modules/apollo-link/lib/bundle.cjs.js 0000644 0001750 0000144 00000016202 03560116604 024005 0 ustar andreh users 'use strict'; Object.defineProperty(exports, '__esModule', { value: true }); function _interopDefault (ex) { return (ex && (typeof ex === 'object') && 'default' in ex) ? ex['default'] : ex; } var Observable = _interopDefault(require('zen-observable-ts')); var tsInvariant = require('ts-invariant'); var tslib = require('tslib'); var apolloUtilities = require('apollo-utilities'); function validateOperation(operation) { var OPERATION_FIELDS = [ 'query', 'operationName', 'variables', 'extensions', 'context', ]; for (var _i = 0, _a = Object.keys(operation); _i < _a.length; _i++) { var key = _a[_i]; if (OPERATION_FIELDS.indexOf(key) < 0) { throw process.env.NODE_ENV === "production" ? new tsInvariant.InvariantError(2) : new tsInvariant.InvariantError("illegal argument: " + key); } } return operation; } var LinkError = (function (_super) { tslib.__extends(LinkError, _super); function LinkError(message, link) { var _this = _super.call(this, message) || this; _this.link = link; return _this; } return LinkError; }(Error)); function isTerminating(link) { return link.request.length <= 1; } function toPromise(observable) { var completed = false; return new Promise(function (resolve, reject) { observable.subscribe({ next: function (data) { if (completed) { process.env.NODE_ENV === "production" || tsInvariant.invariant.warn("Promise Wrapper does not support multiple results from Observable"); } else { completed = true; resolve(data); } }, error: reject, }); }); } var makePromise = toPromise; function fromPromise(promise) { return new Observable(function (observer) { promise .then(function (value) { observer.next(value); observer.complete(); }) .catch(observer.error.bind(observer)); }); } function fromError(errorValue) { return new Observable(function (observer) { observer.error(errorValue); }); } function transformOperation(operation) { var transformedOperation = { variables: operation.variables || {}, extensions: operation.extensions || {}, operationName: operation.operationName, query: operation.query, }; if (!transformedOperation.operationName) { transformedOperation.operationName = typeof transformedOperation.query !== 'string' ? apolloUtilities.getOperationName(transformedOperation.query) : ''; } return transformedOperation; } function createOperation(starting, operation) { var context = tslib.__assign({}, starting); var setContext = function (next) { if (typeof next === 'function') { context = tslib.__assign({}, context, next(context)); } else { context = tslib.__assign({}, context, next); } }; var getContext = function () { return (tslib.__assign({}, context)); }; Object.defineProperty(operation, 'setContext', { enumerable: false, value: setContext, }); Object.defineProperty(operation, 'getContext', { enumerable: false, value: getContext, }); Object.defineProperty(operation, 'toKey', { enumerable: false, value: function () { return getKey(operation); }, }); return operation; } function getKey(operation) { var query = operation.query, variables = operation.variables, operationName = operation.operationName; return JSON.stringify([operationName, query, variables]); } function passthrough(op, forward) { return forward ? forward(op) : Observable.of(); } function toLink(handler) { return typeof handler === 'function' ? new ApolloLink(handler) : handler; } function empty() { return new ApolloLink(function () { return Observable.of(); }); } function from(links) { if (links.length === 0) return empty(); return links.map(toLink).reduce(function (x, y) { return x.concat(y); }); } function split(test, left, right) { var leftLink = toLink(left); var rightLink = toLink(right || new ApolloLink(passthrough)); if (isTerminating(leftLink) && isTerminating(rightLink)) { return new ApolloLink(function (operation) { return test(operation) ? leftLink.request(operation) || Observable.of() : rightLink.request(operation) || Observable.of(); }); } else { return new ApolloLink(function (operation, forward) { return test(operation) ? leftLink.request(operation, forward) || Observable.of() : rightLink.request(operation, forward) || Observable.of(); }); } } var concat = function (first, second) { var firstLink = toLink(first); if (isTerminating(firstLink)) { process.env.NODE_ENV === "production" || tsInvariant.invariant.warn(new LinkError("You are calling concat on a terminating link, which will have no effect", firstLink)); return firstLink; } var nextLink = toLink(second); if (isTerminating(nextLink)) { return new ApolloLink(function (operation) { return firstLink.request(operation, function (op) { return nextLink.request(op) || Observable.of(); }) || Observable.of(); }); } else { return new ApolloLink(function (operation, forward) { return (firstLink.request(operation, function (op) { return nextLink.request(op, forward) || Observable.of(); }) || Observable.of()); }); } }; var ApolloLink = (function () { function ApolloLink(request) { if (request) this.request = request; } ApolloLink.prototype.split = function (test, left, right) { return this.concat(split(test, left, right || new ApolloLink(passthrough))); }; ApolloLink.prototype.concat = function (next) { return concat(this, next); }; ApolloLink.prototype.request = function (operation, forward) { throw process.env.NODE_ENV === "production" ? new tsInvariant.InvariantError(1) : new tsInvariant.InvariantError('request is not implemented'); }; ApolloLink.empty = empty; ApolloLink.from = from; ApolloLink.split = split; ApolloLink.execute = execute; return ApolloLink; }()); function execute(link, operation) { return (link.request(createOperation(operation.context, transformOperation(validateOperation(operation)))) || Observable.of()); } exports.Observable = Observable; Object.defineProperty(exports, 'getOperationName', { enumerable: true, get: function () { return apolloUtilities.getOperationName; } }); exports.ApolloLink = ApolloLink; exports.concat = concat; exports.createOperation = createOperation; exports.empty = empty; exports.execute = execute; exports.from = from; exports.fromError = fromError; exports.fromPromise = fromPromise; exports.makePromise = makePromise; exports.split = split; exports.toPromise = toPromise; //# sourceMappingURL=bundle.cjs.js.map apollo-server-demo/node_modules/apollo-link/lib/types.js 0000644 0001750 0000144 00000000156 03560116604 023123 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); //# sourceMappingURL=types.js.map apollo-server-demo/node_modules/apollo-link/lib/test-utils.js.map 0000644 0001750 0000144 00000000306 03560116604 024645 0 ustar andreh users {"version":3,"file":"test-utils.js","sourceRoot":"","sources":["../src/test-utils.ts"],"names":[],"mappings":";;;AAAA,2EAA6C;AAIpC,mBAJF,kBAAQ,CAIE;AAHjB,+EAAqD;AAGlC,yBAHZ,oBAAc,CAGY;AAFjC,oEAA0C"} apollo-server-demo/node_modules/apollo-link/lib/types.d.ts.map 0000644 0001750 0000144 00000002677 03560116604 024145 0 ustar andreh users {"version":3,"file":"types.d.ts","sourceRoot":"","sources":["src/types.ts"],"names":[],"mappings":"AAAA,OAAO,UAAU,MAAM,mBAAmB,CAAC;AAC3C,OAAO,EAAE,YAAY,EAAE,MAAM,sBAAsB,CAAC;AACpD,OAAO,EAAE,eAAe,IAAI,sBAAsB,EAAE,MAAM,SAAS,CAAC;AACpE,OAAO,EAAE,YAAY,EAAE,CAAC;AAExB,MAAM,WAAW,eAAe,CAC9B,KAAK,GAAG;IACN,CAAC,GAAG,EAAE,MAAM,GAAG,GAAG,CAAC;CACpB,CACD,SAAQ,sBAAsB;IAC9B,IAAI,CAAC,EAAE,KAAK,GAAG,IAAI,CAAC;CACrB;AAED,MAAM,WAAW,cAAc;IAC7B,KAAK,EAAE,YAAY,CAAC;IACpB,SAAS,CAAC,EAAE,MAAM,CAAC,MAAM,EAAE,GAAG,CAAC,CAAC;IAChC,aAAa,CAAC,EAAE,MAAM,CAAC;IACvB,OAAO,CAAC,EAAE,MAAM,CAAC,MAAM,EAAE,GAAG,CAAC,CAAC;IAC9B,UAAU,CAAC,EAAE,MAAM,CAAC,MAAM,EAAE,GAAG,CAAC,CAAC;CAClC;AAED,MAAM,WAAW,SAAS;IACxB,KAAK,EAAE,YAAY,CAAC;IACpB,SAAS,EAAE,MAAM,CAAC,MAAM,EAAE,GAAG,CAAC,CAAC;IAC/B,aAAa,EAAE,MAAM,CAAC;IACtB,UAAU,EAAE,MAAM,CAAC,MAAM,EAAE,GAAG,CAAC,CAAC;IAChC,UAAU,EAAE,CAAC,OAAO,EAAE,MAAM,CAAC,MAAM,EAAE,GAAG,CAAC,KAAK,MAAM,CAAC,MAAM,EAAE,GAAG,CAAC,CAAC;IAClE,UAAU,EAAE,MAAM,MAAM,CAAC,MAAM,EAAE,GAAG,CAAC,CAAC;IACtC,KAAK,EAAE,MAAM,MAAM,CAAC;CACrB;AAED,oBAAY,WAAW,CACrB,KAAK,GAAG;IAAE,CAAC,GAAG,EAAE,MAAM,GAAG,GAAG,CAAA;CAAE,EAC9B,CAAC,GAAG,MAAM,CAAC,MAAM,EAAE,GAAG,CAAC,EACvB,CAAC,GAAG,MAAM,CAAC,MAAM,EAAE,GAAG,CAAC,IACrB,eAAe,CAAC,KAAK,CAAC,GAAG;IAC3B,UAAU,CAAC,EAAE,CAAC,CAAC;IACf,OAAO,CAAC,EAAE,CAAC,CAAC;CACb,CAAC;AAEF,oBAAY,QAAQ,GAAG,CAAC,SAAS,EAAE,SAAS,KAAK,UAAU,CAAC,WAAW,CAAC,CAAC;AACzE,oBAAY,cAAc,GAAG,CAC3B,SAAS,EAAE,SAAS,EACpB,OAAO,EAAE,QAAQ,KACd,UAAU,CAAC,WAAW,CAAC,GAAG,IAAI,CAAC"} apollo-server-demo/node_modules/apollo-link/lib/bundle.esm.js 0000644 0001750 0000144 00000015075 03560116604 024021 0 ustar andreh users import Observable from 'zen-observable-ts'; export { default as Observable } from 'zen-observable-ts'; import { invariant, InvariantError } from 'ts-invariant'; import { __extends, __assign } from 'tslib'; import { getOperationName } from 'apollo-utilities'; export { getOperationName } from 'apollo-utilities'; function validateOperation(operation) { var OPERATION_FIELDS = [ 'query', 'operationName', 'variables', 'extensions', 'context', ]; for (var _i = 0, _a = Object.keys(operation); _i < _a.length; _i++) { var key = _a[_i]; if (OPERATION_FIELDS.indexOf(key) < 0) { throw process.env.NODE_ENV === "production" ? new InvariantError(2) : new InvariantError("illegal argument: " + key); } } return operation; } var LinkError = (function (_super) { __extends(LinkError, _super); function LinkError(message, link) { var _this = _super.call(this, message) || this; _this.link = link; return _this; } return LinkError; }(Error)); function isTerminating(link) { return link.request.length <= 1; } function toPromise(observable) { var completed = false; return new Promise(function (resolve, reject) { observable.subscribe({ next: function (data) { if (completed) { process.env.NODE_ENV === "production" || invariant.warn("Promise Wrapper does not support multiple results from Observable"); } else { completed = true; resolve(data); } }, error: reject, }); }); } var makePromise = toPromise; function fromPromise(promise) { return new Observable(function (observer) { promise .then(function (value) { observer.next(value); observer.complete(); }) .catch(observer.error.bind(observer)); }); } function fromError(errorValue) { return new Observable(function (observer) { observer.error(errorValue); }); } function transformOperation(operation) { var transformedOperation = { variables: operation.variables || {}, extensions: operation.extensions || {}, operationName: operation.operationName, query: operation.query, }; if (!transformedOperation.operationName) { transformedOperation.operationName = typeof transformedOperation.query !== 'string' ? getOperationName(transformedOperation.query) : ''; } return transformedOperation; } function createOperation(starting, operation) { var context = __assign({}, starting); var setContext = function (next) { if (typeof next === 'function') { context = __assign({}, context, next(context)); } else { context = __assign({}, context, next); } }; var getContext = function () { return (__assign({}, context)); }; Object.defineProperty(operation, 'setContext', { enumerable: false, value: setContext, }); Object.defineProperty(operation, 'getContext', { enumerable: false, value: getContext, }); Object.defineProperty(operation, 'toKey', { enumerable: false, value: function () { return getKey(operation); }, }); return operation; } function getKey(operation) { var query = operation.query, variables = operation.variables, operationName = operation.operationName; return JSON.stringify([operationName, query, variables]); } function passthrough(op, forward) { return forward ? forward(op) : Observable.of(); } function toLink(handler) { return typeof handler === 'function' ? new ApolloLink(handler) : handler; } function empty() { return new ApolloLink(function () { return Observable.of(); }); } function from(links) { if (links.length === 0) return empty(); return links.map(toLink).reduce(function (x, y) { return x.concat(y); }); } function split(test, left, right) { var leftLink = toLink(left); var rightLink = toLink(right || new ApolloLink(passthrough)); if (isTerminating(leftLink) && isTerminating(rightLink)) { return new ApolloLink(function (operation) { return test(operation) ? leftLink.request(operation) || Observable.of() : rightLink.request(operation) || Observable.of(); }); } else { return new ApolloLink(function (operation, forward) { return test(operation) ? leftLink.request(operation, forward) || Observable.of() : rightLink.request(operation, forward) || Observable.of(); }); } } var concat = function (first, second) { var firstLink = toLink(first); if (isTerminating(firstLink)) { process.env.NODE_ENV === "production" || invariant.warn(new LinkError("You are calling concat on a terminating link, which will have no effect", firstLink)); return firstLink; } var nextLink = toLink(second); if (isTerminating(nextLink)) { return new ApolloLink(function (operation) { return firstLink.request(operation, function (op) { return nextLink.request(op) || Observable.of(); }) || Observable.of(); }); } else { return new ApolloLink(function (operation, forward) { return (firstLink.request(operation, function (op) { return nextLink.request(op, forward) || Observable.of(); }) || Observable.of()); }); } }; var ApolloLink = (function () { function ApolloLink(request) { if (request) this.request = request; } ApolloLink.prototype.split = function (test, left, right) { return this.concat(split(test, left, right || new ApolloLink(passthrough))); }; ApolloLink.prototype.concat = function (next) { return concat(this, next); }; ApolloLink.prototype.request = function (operation, forward) { throw process.env.NODE_ENV === "production" ? new InvariantError(1) : new InvariantError('request is not implemented'); }; ApolloLink.empty = empty; ApolloLink.from = from; ApolloLink.split = split; ApolloLink.execute = execute; return ApolloLink; }()); function execute(link, operation) { return (link.request(createOperation(operation.context, transformOperation(validateOperation(operation)))) || Observable.of()); } export { ApolloLink, concat, createOperation, empty, execute, from, fromError, fromPromise, makePromise, split, toPromise }; //# sourceMappingURL=bundle.esm.js.map apollo-server-demo/node_modules/unpipe/ 0000755 0001750 0000144 00000000000 14067647700 017742 5 ustar andreh users apollo-server-demo/node_modules/unpipe/index.js 0000644 0001750 0000144 00000002136 12537362066 021410 0 ustar andreh users /*! * unpipe * Copyright(c) 2015 Douglas Christopher Wilson * MIT Licensed */ 'use strict' /** * Module exports. * @public */ module.exports = unpipe /** * Determine if there are Node.js pipe-like data listeners. * @private */ function hasPipeDataListeners(stream) { var listeners = stream.listeners('data') for (var i = 0; i < listeners.length; i++) { if (listeners[i].name === 'ondata') { return true } } return false } /** * Unpipe a stream from all destinations. * * @param {object} stream * @public */ function unpipe(stream) { if (!stream) { throw new TypeError('argument stream is required') } if (typeof stream.unpipe === 'function') { // new-style stream.unpipe() return } // Node.js 0.8 hack if (!hasPipeDataListeners(stream)) { return } var listener var listeners = stream.listeners('close') for (var i = 0; i < listeners.length; i++) { listener = listeners[i] if (listener.name !== 'cleanup' && listener.name !== 'onclose') { continue } // invoke the listener listener.call(stream) } } apollo-server-demo/node_modules/unpipe/LICENSE 0000644 0001750 0000144 00000002132 12537362066 020744 0 ustar andreh users (The MIT License) Copyright (c) 2015 Douglas Christopher Wilson <doug@somethingdoug.com> Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the 'Software'), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/unpipe/HISTORY.md 0000644 0001750 0000144 00000000073 12537362066 021424 0 ustar andreh users 1.0.0 / 2015-06-14 ================== * Initial release apollo-server-demo/node_modules/unpipe/README.md 0000644 0001750 0000144 00000002342 12537362066 021221 0 ustar andreh users # unpipe [![NPM Version][npm-image]][npm-url] [![NPM Downloads][downloads-image]][downloads-url] [![Node.js Version][node-image]][node-url] [![Build Status][travis-image]][travis-url] [![Test Coverage][coveralls-image]][coveralls-url] Unpipe a stream from all destinations. ## Installation ```sh $ npm install unpipe ``` ## API ```js var unpipe = require('unpipe') ``` ### unpipe(stream) Unpipes all destinations from a given stream. With stream 2+, this is equivalent to `stream.unpipe()`. When used with streams 1 style streams (typically Node.js 0.8 and below), this module attempts to undo the actions done in `stream.pipe(dest)`. ## License [MIT](LICENSE) [npm-image]: https://img.shields.io/npm/v/unpipe.svg [npm-url]: https://npmjs.org/package/unpipe [node-image]: https://img.shields.io/node/v/unpipe.svg [node-url]: http://nodejs.org/download/ [travis-image]: https://img.shields.io/travis/stream-utils/unpipe.svg [travis-url]: https://travis-ci.org/stream-utils/unpipe [coveralls-image]: https://img.shields.io/coveralls/stream-utils/unpipe.svg [coveralls-url]: https://coveralls.io/r/stream-utils/unpipe?branch=master [downloads-image]: https://img.shields.io/npm/dm/unpipe.svg [downloads-url]: https://npmjs.org/package/unpipe apollo-server-demo/node_modules/unpipe/package.json 0000644 0001750 0000144 00000001623 12537362066 022231 0 ustar andreh users { "name": "unpipe", "description": "Unpipe a stream from all destinations", "version": "1.0.0", "author": "Douglas Christopher Wilson <doug@somethingdoug.com>", "license": "MIT", "repository": "stream-utils/unpipe", "devDependencies": { "istanbul": "0.3.15", "mocha": "2.2.5", "readable-stream": "1.1.13" }, "files": [ "HISTORY.md", "LICENSE", "README.md", "index.js" ], "engines": { "node": ">= 0.8" }, "scripts": { "test": "mocha --reporter spec --bail --check-leaks test/", "test-cov": "istanbul cover node_modules/mocha/bin/_mocha -- --reporter dot --check-leaks test/", "test-travis": "istanbul cover node_modules/mocha/bin/_mocha --report lcovonly -- --reporter spec --check-leaks test/" } ,"_resolved": "https://registry.npmjs.org/unpipe/-/unpipe-1.0.0.tgz" ,"_integrity": "sha1-sr9O6FFKrmFltIF4KdIbLvSZBOw=" ,"_from": "unpipe@1.0.0" } apollo-server-demo/node_modules/content-disposition/ 0000755 0001750 0000144 00000000000 14067647700 022456 5 ustar andreh users apollo-server-demo/node_modules/content-disposition/index.js 0000644 0001750 0000144 00000024542 03560116604 024120 0 ustar andreh users /*! * content-disposition * Copyright(c) 2014-2017 Douglas Christopher Wilson * MIT Licensed */ 'use strict' /** * Module exports. * @public */ module.exports = contentDisposition module.exports.parse = parse /** * Module dependencies. * @private */ var basename = require('path').basename var Buffer = require('safe-buffer').Buffer /** * RegExp to match non attr-char, *after* encodeURIComponent (i.e. not including "%") * @private */ var ENCODE_URL_ATTR_CHAR_REGEXP = /[\x00-\x20"'()*,/:;<=>?@[\\\]{}\x7f]/g // eslint-disable-line no-control-regex /** * RegExp to match percent encoding escape. * @private */ var HEX_ESCAPE_REGEXP = /%[0-9A-Fa-f]{2}/ var HEX_ESCAPE_REPLACE_REGEXP = /%([0-9A-Fa-f]{2})/g /** * RegExp to match non-latin1 characters. * @private */ var NON_LATIN1_REGEXP = /[^\x20-\x7e\xa0-\xff]/g /** * RegExp to match quoted-pair in RFC 2616 * * quoted-pair = "\" CHAR * CHAR = <any US-ASCII character (octets 0 - 127)> * @private */ var QESC_REGEXP = /\\([\u0000-\u007f])/g // eslint-disable-line no-control-regex /** * RegExp to match chars that must be quoted-pair in RFC 2616 * @private */ var QUOTE_REGEXP = /([\\"])/g /** * RegExp for various RFC 2616 grammar * * parameter = token "=" ( token | quoted-string ) * token = 1*<any CHAR except CTLs or separators> * separators = "(" | ")" | "<" | ">" | "@" * | "," | ";" | ":" | "\" | <"> * | "/" | "[" | "]" | "?" | "=" * | "{" | "}" | SP | HT * quoted-string = ( <"> *(qdtext | quoted-pair ) <"> ) * qdtext = <any TEXT except <">> * quoted-pair = "\" CHAR * CHAR = <any US-ASCII character (octets 0 - 127)> * TEXT = <any OCTET except CTLs, but including LWS> * LWS = [CRLF] 1*( SP | HT ) * CRLF = CR LF * CR = <US-ASCII CR, carriage return (13)> * LF = <US-ASCII LF, linefeed (10)> * SP = <US-ASCII SP, space (32)> * HT = <US-ASCII HT, horizontal-tab (9)> * CTL = <any US-ASCII control character (octets 0 - 31) and DEL (127)> * OCTET = <any 8-bit sequence of data> * @private */ var PARAM_REGEXP = /;[\x09\x20]*([!#$%&'*+.0-9A-Z^_`a-z|~-]+)[\x09\x20]*=[\x09\x20]*("(?:[\x20!\x23-\x5b\x5d-\x7e\x80-\xff]|\\[\x20-\x7e])*"|[!#$%&'*+.0-9A-Z^_`a-z|~-]+)[\x09\x20]*/g // eslint-disable-line no-control-regex var TEXT_REGEXP = /^[\x20-\x7e\x80-\xff]+$/ var TOKEN_REGEXP = /^[!#$%&'*+.0-9A-Z^_`a-z|~-]+$/ /** * RegExp for various RFC 5987 grammar * * ext-value = charset "'" [ language ] "'" value-chars * charset = "UTF-8" / "ISO-8859-1" / mime-charset * mime-charset = 1*mime-charsetc * mime-charsetc = ALPHA / DIGIT * / "!" / "#" / "$" / "%" / "&" * / "+" / "-" / "^" / "_" / "`" * / "{" / "}" / "~" * language = ( 2*3ALPHA [ extlang ] ) * / 4ALPHA * / 5*8ALPHA * extlang = *3( "-" 3ALPHA ) * value-chars = *( pct-encoded / attr-char ) * pct-encoded = "%" HEXDIG HEXDIG * attr-char = ALPHA / DIGIT * / "!" / "#" / "$" / "&" / "+" / "-" / "." * / "^" / "_" / "`" / "|" / "~" * @private */ var EXT_VALUE_REGEXP = /^([A-Za-z0-9!#$%&+\-^_`{}~]+)'(?:[A-Za-z]{2,3}(?:-[A-Za-z]{3}){0,3}|[A-Za-z]{4,8}|)'((?:%[0-9A-Fa-f]{2}|[A-Za-z0-9!#$&+.^_`|~-])+)$/ /** * RegExp for various RFC 6266 grammar * * disposition-type = "inline" | "attachment" | disp-ext-type * disp-ext-type = token * disposition-parm = filename-parm | disp-ext-parm * filename-parm = "filename" "=" value * | "filename*" "=" ext-value * disp-ext-parm = token "=" value * | ext-token "=" ext-value * ext-token = <the characters in token, followed by "*"> * @private */ var DISPOSITION_TYPE_REGEXP = /^([!#$%&'*+.0-9A-Z^_`a-z|~-]+)[\x09\x20]*(?:$|;)/ // eslint-disable-line no-control-regex /** * Create an attachment Content-Disposition header. * * @param {string} [filename] * @param {object} [options] * @param {string} [options.type=attachment] * @param {string|boolean} [options.fallback=true] * @return {string} * @public */ function contentDisposition (filename, options) { var opts = options || {} // get type var type = opts.type || 'attachment' // get parameters var params = createparams(filename, opts.fallback) // format into string return format(new ContentDisposition(type, params)) } /** * Create parameters object from filename and fallback. * * @param {string} [filename] * @param {string|boolean} [fallback=true] * @return {object} * @private */ function createparams (filename, fallback) { if (filename === undefined) { return } var params = {} if (typeof filename !== 'string') { throw new TypeError('filename must be a string') } // fallback defaults to true if (fallback === undefined) { fallback = true } if (typeof fallback !== 'string' && typeof fallback !== 'boolean') { throw new TypeError('fallback must be a string or boolean') } if (typeof fallback === 'string' && NON_LATIN1_REGEXP.test(fallback)) { throw new TypeError('fallback must be ISO-8859-1 string') } // restrict to file base name var name = basename(filename) // determine if name is suitable for quoted string var isQuotedString = TEXT_REGEXP.test(name) // generate fallback name var fallbackName = typeof fallback !== 'string' ? fallback && getlatin1(name) : basename(fallback) var hasFallback = typeof fallbackName === 'string' && fallbackName !== name // set extended filename parameter if (hasFallback || !isQuotedString || HEX_ESCAPE_REGEXP.test(name)) { params['filename*'] = name } // set filename parameter if (isQuotedString || hasFallback) { params.filename = hasFallback ? fallbackName : name } return params } /** * Format object to Content-Disposition header. * * @param {object} obj * @param {string} obj.type * @param {object} [obj.parameters] * @return {string} * @private */ function format (obj) { var parameters = obj.parameters var type = obj.type if (!type || typeof type !== 'string' || !TOKEN_REGEXP.test(type)) { throw new TypeError('invalid type') } // start with normalized type var string = String(type).toLowerCase() // append parameters if (parameters && typeof parameters === 'object') { var param var params = Object.keys(parameters).sort() for (var i = 0; i < params.length; i++) { param = params[i] var val = param.substr(-1) === '*' ? ustring(parameters[param]) : qstring(parameters[param]) string += '; ' + param + '=' + val } } return string } /** * Decode a RFC 6987 field value (gracefully). * * @param {string} str * @return {string} * @private */ function decodefield (str) { var match = EXT_VALUE_REGEXP.exec(str) if (!match) { throw new TypeError('invalid extended field value') } var charset = match[1].toLowerCase() var encoded = match[2] var value // to binary string var binary = encoded.replace(HEX_ESCAPE_REPLACE_REGEXP, pdecode) switch (charset) { case 'iso-8859-1': value = getlatin1(binary) break case 'utf-8': value = Buffer.from(binary, 'binary').toString('utf8') break default: throw new TypeError('unsupported charset in extended field') } return value } /** * Get ISO-8859-1 version of string. * * @param {string} val * @return {string} * @private */ function getlatin1 (val) { // simple Unicode -> ISO-8859-1 transformation return String(val).replace(NON_LATIN1_REGEXP, '?') } /** * Parse Content-Disposition header string. * * @param {string} string * @return {object} * @public */ function parse (string) { if (!string || typeof string !== 'string') { throw new TypeError('argument string is required') } var match = DISPOSITION_TYPE_REGEXP.exec(string) if (!match) { throw new TypeError('invalid type format') } // normalize type var index = match[0].length var type = match[1].toLowerCase() var key var names = [] var params = {} var value // calculate index to start at index = PARAM_REGEXP.lastIndex = match[0].substr(-1) === ';' ? index - 1 : index // match parameters while ((match = PARAM_REGEXP.exec(string))) { if (match.index !== index) { throw new TypeError('invalid parameter format') } index += match[0].length key = match[1].toLowerCase() value = match[2] if (names.indexOf(key) !== -1) { throw new TypeError('invalid duplicate parameter') } names.push(key) if (key.indexOf('*') + 1 === key.length) { // decode extended value key = key.slice(0, -1) value = decodefield(value) // overwrite existing value params[key] = value continue } if (typeof params[key] === 'string') { continue } if (value[0] === '"') { // remove quotes and escapes value = value .substr(1, value.length - 2) .replace(QESC_REGEXP, '$1') } params[key] = value } if (index !== -1 && index !== string.length) { throw new TypeError('invalid parameter format') } return new ContentDisposition(type, params) } /** * Percent decode a single character. * * @param {string} str * @param {string} hex * @return {string} * @private */ function pdecode (str, hex) { return String.fromCharCode(parseInt(hex, 16)) } /** * Percent encode a single character. * * @param {string} char * @return {string} * @private */ function pencode (char) { return '%' + String(char) .charCodeAt(0) .toString(16) .toUpperCase() } /** * Quote a string for HTTP. * * @param {string} val * @return {string} * @private */ function qstring (val) { var str = String(val) return '"' + str.replace(QUOTE_REGEXP, '\\$1') + '"' } /** * Encode a Unicode string for HTTP (RFC 5987). * * @param {string} val * @return {string} * @private */ function ustring (val) { var str = String(val) // percent encode as UTF-8 var encoded = encodeURIComponent(str) .replace(ENCODE_URL_ATTR_CHAR_REGEXP, pencode) return 'UTF-8\'\'' + encoded } /** * Class for parsed Content-Disposition header for v8 optimization * * @public * @param {string} type * @param {object} parameters * @constructor */ function ContentDisposition (type, parameters) { this.type = type this.parameters = parameters } apollo-server-demo/node_modules/content-disposition/LICENSE 0000644 0001750 0000144 00000002106 03560116604 023450 0 ustar andreh users (The MIT License) Copyright (c) 2014-2017 Douglas Christopher Wilson Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the 'Software'), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/content-disposition/HISTORY.md 0000644 0001750 0000144 00000001670 03560116604 024133 0 ustar andreh users 0.5.3 / 2018-12-17 ================== * Use `safe-buffer` for improved Buffer API 0.5.2 / 2016-12-08 ================== * Fix `parse` to accept any linear whitespace character 0.5.1 / 2016-01-17 ================== * perf: enable strict mode 0.5.0 / 2014-10-11 ================== * Add `parse` function 0.4.0 / 2014-09-21 ================== * Expand non-Unicode `filename` to the full ISO-8859-1 charset 0.3.0 / 2014-09-20 ================== * Add `fallback` option * Add `type` option 0.2.0 / 2014-09-19 ================== * Reduce ambiguity of file names with hex escape in buggy browsers 0.1.2 / 2014-09-19 ================== * Fix periodic invalid Unicode filename header 0.1.1 / 2014-09-19 ================== * Fix invalid characters appearing in `filename*` parameter 0.1.0 / 2014-09-18 ================== * Make the `filename` argument optional 0.0.0 / 2014-09-18 ================== * Initial release apollo-server-demo/node_modules/content-disposition/README.md 0000644 0001750 0000144 00000012165 03560116604 023730 0 ustar andreh users # content-disposition [![NPM Version][npm-image]][npm-url] [![NPM Downloads][downloads-image]][downloads-url] [![Node.js Version][node-version-image]][node-version-url] [![Build Status][travis-image]][travis-url] [![Test Coverage][coveralls-image]][coveralls-url] Create and parse HTTP `Content-Disposition` header ## Installation ```sh $ npm install content-disposition ``` ## API <!-- eslint-disable no-unused-vars --> ```js var contentDisposition = require('content-disposition') ``` ### contentDisposition(filename, options) Create an attachment `Content-Disposition` header value using the given file name, if supplied. The `filename` is optional and if no file name is desired, but you want to specify `options`, set `filename` to `undefined`. <!-- eslint-disable no-undef --> ```js res.setHeader('Content-Disposition', contentDisposition('∫ maths.pdf')) ``` **note** HTTP headers are of the ISO-8859-1 character set. If you are writing this header through a means different from `setHeader` in Node.js, you'll want to specify the `'binary'` encoding in Node.js. #### Options `contentDisposition` accepts these properties in the options object. ##### fallback If the `filename` option is outside ISO-8859-1, then the file name is actually stored in a supplemental field for clients that support Unicode file names and a ISO-8859-1 version of the file name is automatically generated. This specifies the ISO-8859-1 file name to override the automatic generation or disables the generation all together, defaults to `true`. - A string will specify the ISO-8859-1 file name to use in place of automatic generation. - `false` will disable including a ISO-8859-1 file name and only include the Unicode version (unless the file name is already ISO-8859-1). - `true` will enable automatic generation if the file name is outside ISO-8859-1. If the `filename` option is ISO-8859-1 and this option is specified and has a different value, then the `filename` option is encoded in the extended field and this set as the fallback field, even though they are both ISO-8859-1. ##### type Specifies the disposition type, defaults to `"attachment"`. This can also be `"inline"`, or any other value (all values except inline are treated like `attachment`, but can convey additional information if both parties agree to it). The type is normalized to lower-case. ### contentDisposition.parse(string) <!-- eslint-disable no-undef, no-unused-vars --> ```js var disposition = contentDisposition.parse('attachment; filename="EURO rates.txt"; filename*=UTF-8\'\'%e2%82%ac%20rates.txt') ``` Parse a `Content-Disposition` header string. This automatically handles extended ("Unicode") parameters by decoding them and providing them under the standard parameter name. This will return an object with the following properties (examples are shown for the string `'attachment; filename="EURO rates.txt"; filename*=UTF-8\'\'%e2%82%ac%20rates.txt'`): - `type`: The disposition type (always lower case). Example: `'attachment'` - `parameters`: An object of the parameters in the disposition (name of parameter always lower case and extended versions replace non-extended versions). Example: `{filename: "€ rates.txt"}` ## Examples ### Send a file for download ```js var contentDisposition = require('content-disposition') var destroy = require('destroy') var fs = require('fs') var http = require('http') var onFinished = require('on-finished') var filePath = '/path/to/public/plans.pdf' http.createServer(function onRequest (req, res) { // set headers res.setHeader('Content-Type', 'application/pdf') res.setHeader('Content-Disposition', contentDisposition(filePath)) // send file var stream = fs.createReadStream(filePath) stream.pipe(res) onFinished(res, function () { destroy(stream) }) }) ``` ## Testing ```sh $ npm test ``` ## References - [RFC 2616: Hypertext Transfer Protocol -- HTTP/1.1][rfc-2616] - [RFC 5987: Character Set and Language Encoding for Hypertext Transfer Protocol (HTTP) Header Field Parameters][rfc-5987] - [RFC 6266: Use of the Content-Disposition Header Field in the Hypertext Transfer Protocol (HTTP)][rfc-6266] - [Test Cases for HTTP Content-Disposition header field (RFC 6266) and the Encodings defined in RFCs 2047, 2231 and 5987][tc-2231] [rfc-2616]: https://tools.ietf.org/html/rfc2616 [rfc-5987]: https://tools.ietf.org/html/rfc5987 [rfc-6266]: https://tools.ietf.org/html/rfc6266 [tc-2231]: http://greenbytes.de/tech/tc2231/ ## License [MIT](LICENSE) [npm-image]: https://img.shields.io/npm/v/content-disposition.svg [npm-url]: https://npmjs.org/package/content-disposition [node-version-image]: https://img.shields.io/node/v/content-disposition.svg [node-version-url]: https://nodejs.org/en/download [travis-image]: https://img.shields.io/travis/jshttp/content-disposition.svg [travis-url]: https://travis-ci.org/jshttp/content-disposition [coveralls-image]: https://img.shields.io/coveralls/jshttp/content-disposition.svg [coveralls-url]: https://coveralls.io/r/jshttp/content-disposition?branch=master [downloads-image]: https://img.shields.io/npm/dm/content-disposition.svg [downloads-url]: https://npmjs.org/package/content-disposition apollo-server-demo/node_modules/content-disposition/package.json 0000644 0001750 0000144 00000002714 03560116604 024736 0 ustar andreh users { "name": "content-disposition", "description": "Create and parse Content-Disposition header", "version": "0.5.3", "author": "Douglas Christopher Wilson <doug@somethingdoug.com>", "license": "MIT", "keywords": [ "content-disposition", "http", "rfc6266", "res" ], "repository": "jshttp/content-disposition", "dependencies": { "safe-buffer": "5.1.2" }, "devDependencies": { "deep-equal": "1.0.1", "eslint": "5.10.0", "eslint-config-standard": "12.0.0", "eslint-plugin-import": "2.14.0", "eslint-plugin-markdown": "1.0.0-rc.1", "eslint-plugin-node": "7.0.1", "eslint-plugin-promise": "4.0.1", "eslint-plugin-standard": "4.0.0", "istanbul": "0.4.5", "mocha": "5.2.0" }, "files": [ "LICENSE", "HISTORY.md", "README.md", "index.js" ], "engines": { "node": ">= 0.6" }, "scripts": { "lint": "eslint --plugin markdown --ext js,md .", "test": "mocha --reporter spec --bail --check-leaks test/", "test-cov": "istanbul cover node_modules/mocha/bin/_mocha -- --reporter dot --check-leaks test/", "test-travis": "istanbul cover node_modules/mocha/bin/_mocha --report lcovonly -- --reporter spec --check-leaks test/" } ,"_resolved": "https://registry.npmjs.org/content-disposition/-/content-disposition-0.5.3.tgz" ,"_integrity": "sha512-ExO0774ikEObIAEV9kDo50o+79VCUdEB6n6lzKgGwupcVeRlhrj3qGAfwq8G6uBJjkqLrhT0qEYFcWng8z1z0g==" ,"_from": "content-disposition@0.5.3" } apollo-server-demo/node_modules/apollo-tracing/ 0000755 0001750 0000144 00000000000 14067647700 021355 5 ustar andreh users apollo-server-demo/node_modules/apollo-tracing/dist/ 0000755 0001750 0000144 00000000000 14067647700 022320 5 ustar andreh users apollo-server-demo/node_modules/apollo-tracing/dist/index.js 0000644 0001750 0000144 00000006154 03560116604 023761 0 ustar andreh users "use strict"; Object.defineProperty(exports, "__esModule", { value: true }); exports.plugin = void 0; const graphql_1 = require("graphql"); const { name: PACKAGE_NAME } = require("../package.json"); exports.plugin = (_futureOptions = {}) => () => ({ requestDidStart() { const startWallTime = new Date(); let endWallTime; const startHrTime = process.hrtime(); let duration; const resolverCalls = []; return { executionDidStart: () => ({ executionDidEnd: () => { duration = process.hrtime(startHrTime); endWallTime = new Date(); }, willResolveField({ info }) { const resolverCall = { path: info.path, fieldName: info.fieldName, parentType: info.parentType, returnType: info.returnType, startOffset: process.hrtime(startHrTime), }; resolverCalls.push(resolverCall); return () => { resolverCall.endOffset = process.hrtime(startHrTime); }; }, }), willSendResponse({ response }) { if (typeof endWallTime === 'undefined' || typeof duration === 'undefined') { return; } const extensions = response.extensions || (response.extensions = Object.create(null)); if (typeof extensions.tracing !== 'undefined') { throw new Error(PACKAGE_NAME + ": Could not add `tracing` to " + "`extensions` since `tracing` was unexpectedly already present."); } extensions.tracing = { version: 1, startTime: startWallTime.toISOString(), endTime: endWallTime.toISOString(), duration: durationHrTimeToNanos(duration), execution: { resolvers: resolverCalls.map(resolverCall => { const startOffset = durationHrTimeToNanos(resolverCall.startOffset); const duration = resolverCall.endOffset ? durationHrTimeToNanos(resolverCall.endOffset) - startOffset : 0; return { path: [...graphql_1.responsePathAsArray(resolverCall.path)], parentType: resolverCall.parentType.toString(), fieldName: resolverCall.fieldName, returnType: resolverCall.returnType.toString(), startOffset, duration, }; }), }, }; }, }; }, }); function durationHrTimeToNanos(hrtime) { return hrtime[0] * 1e9 + hrtime[1]; } //# sourceMappingURL=index.js.map apollo-server-demo/node_modules/apollo-tracing/dist/index.d.ts 0000644 0001750 0000144 00000001047 03560116604 024211 0 ustar andreh users import { ApolloServerPlugin } from "apollo-server-plugin-base"; export interface TracingFormat { version: 1; startTime: string; endTime: string; duration: number; execution: { resolvers: { path: (string | number)[]; parentType: string; fieldName: string; returnType: string; startOffset: number; duration: number; }[]; }; } export declare const plugin: (_futureOptions?: {}) => () => ApolloServerPlugin; //# sourceMappingURL=index.d.ts.map apollo-server-demo/node_modules/apollo-tracing/dist/index.d.ts.map 0000644 0001750 0000144 00000001051 03560116604 024760 0 ustar andreh users {"version":3,"file":"index.d.ts","sourceRoot":"","sources":["../src/index.ts"],"names":[],"mappings":"AAKA,OAAO,EAAE,kBAAkB,EAAE,MAAM,2BAA2B,CAAC;AAI/D,MAAM,WAAW,aAAa;IAC5B,OAAO,EAAE,CAAC,CAAC;IACX,SAAS,EAAE,MAAM,CAAC;IAClB,OAAO,EAAE,MAAM,CAAC;IAChB,QAAQ,EAAE,MAAM,CAAC;IACjB,SAAS,EAAE;QACT,SAAS,EAAE;YACT,IAAI,EAAE,CAAC,MAAM,GAAG,MAAM,CAAC,EAAE,CAAC;YAC1B,UAAU,EAAE,MAAM,CAAC;YACnB,SAAS,EAAE,MAAM,CAAC;YAClB,UAAU,EAAE,MAAM,CAAC;YACnB,WAAW,EAAE,MAAM,CAAC;YACpB,QAAQ,EAAE,MAAM,CAAC;SAClB,EAAE,CAAC;KACL,CAAC;CACH;AAWD,eAAO,MAAM,MAAM,iCAAgC,kBA8FjD,CAAA"} apollo-server-demo/node_modules/apollo-tracing/dist/index.js.map 0000644 0001750 0000144 00000004217 03560116604 024533 0 ustar andreh users {"version":3,"file":"index.js","sourceRoot":"","sources":["../src/index.ts"],"names":[],"mappings":";;;AAAA,qCAIiB;AAGjB,MAAM,EAAE,IAAI,EAAE,YAAY,EAAE,GAAG,OAAO,CAAC,iBAAiB,CAAC,CAAC;AA4B7C,QAAA,MAAM,GAAG,CAAC,cAAc,GAAG,EAAE,EAAE,EAAE,CAAC,GAAuB,EAAE,CAAC,CAAC;IACxE,eAAe;QACb,MAAM,aAAa,GAAS,IAAI,IAAI,EAAE,CAAC;QACvC,IAAI,WAA6B,CAAC;QAClC,MAAM,WAAW,GAAuB,OAAO,CAAC,MAAM,EAAE,CAAC;QACzD,IAAI,QAAwC,CAAC;QAC7C,MAAM,aAAa,GAAmB,EAAE,CAAC;QAGzC,OAAO;YACL,iBAAiB,EAAE,GAAG,EAAE,CAAC,CAAC;gBAYxB,eAAe,EAAE,GAAG,EAAE;oBACpB,QAAQ,GAAG,OAAO,CAAC,MAAM,CAAC,WAAW,CAAC,CAAC;oBACvC,WAAW,GAAG,IAAI,IAAI,EAAE,CAAC;gBAC3B,CAAC;gBAED,gBAAgB,CAAC,EAAE,IAAI,EAAE;oBACvB,MAAM,YAAY,GAAiB;wBACjC,IAAI,EAAE,IAAI,CAAC,IAAI;wBACf,SAAS,EAAE,IAAI,CAAC,SAAS;wBACzB,UAAU,EAAE,IAAI,CAAC,UAAU;wBAC3B,UAAU,EAAE,IAAI,CAAC,UAAU;wBAC3B,WAAW,EAAE,OAAO,CAAC,MAAM,CAAC,WAAW,CAAC;qBACzC,CAAC;oBAEF,aAAa,CAAC,IAAI,CAAC,YAAY,CAAC,CAAC;oBAEjC,OAAO,GAAG,EAAE;wBACV,YAAY,CAAC,SAAS,GAAG,OAAO,CAAC,MAAM,CAAC,WAAW,CAAC,CAAC;oBACvD,CAAC,CAAC;gBACJ,CAAC;aACF,CAAC;YAEF,gBAAgB,CAAC,EAAE,QAAQ,EAAE;gBAK3B,IACE,OAAO,WAAW,KAAK,WAAW;oBAClC,OAAO,QAAQ,KAAK,WAAW,EAC/B;oBACA,OAAO;iBACR;gBAED,MAAM,UAAU,GACd,QAAQ,CAAC,UAAU,IAAI,CAAC,QAAQ,CAAC,UAAU,GAAG,MAAM,CAAC,MAAM,CAAC,IAAI,CAAC,CAAC,CAAC;gBAIrE,IAAI,OAAO,UAAU,CAAC,OAAO,KAAK,WAAW,EAAE;oBAC7C,MAAM,IAAI,KAAK,CAAC,YAAY,GAAG,+BAA+B;wBAC5D,gEAAgE,CAAC,CAAC;iBACrE;gBAGD,UAAU,CAAC,OAAO,GAAG;oBACnB,OAAO,EAAE,CAAC;oBACV,SAAS,EAAE,aAAa,CAAC,WAAW,EAAE;oBACtC,OAAO,EAAE,WAAW,CAAC,WAAW,EAAE;oBAClC,QAAQ,EAAE,qBAAqB,CAAC,QAAQ,CAAC;oBACzC,SAAS,EAAE;wBACT,SAAS,EAAE,aAAa,CAAC,GAAG,CAAC,YAAY,CAAC,EAAE;4BAC1C,MAAM,WAAW,GAAG,qBAAqB,CACvC,YAAY,CAAC,WAAW,CACzB,CAAC;4BACF,MAAM,QAAQ,GAAG,YAAY,CAAC,SAAS;gCACrC,CAAC,CAAC,qBAAqB,CAAC,YAAY,CAAC,SAAS,CAAC,GAAG,WAAW;gCAC7D,CAAC,CAAC,CAAC,CAAC;4BACN,OAAO;gCACL,IAAI,EAAE,CAAC,GAAG,6BAAmB,CAAC,YAAY,CAAC,IAAI,CAAC,CAAC;gCACjD,UAAU,EAAE,YAAY,CAAC,UAAU,CAAC,QAAQ,EAAE;gCAC9C,SAAS,EAAE,YAAY,CAAC,SAAS;gCACjC,UAAU,EAAE,YAAY,CAAC,UAAU,CAAC,QAAQ,EAAE;gCAC9C,WAAW;gCACX,QAAQ;6BACT,CAAC;wBACJ,CAAC,CAAC;qBACH;iBACF,CAAC;YACJ,CAAC;SACF,CAAC;IACJ,CAAC;CACF,CAAC,CAAA;AAeF,SAAS,qBAAqB,CAAC,MAA0B;IACvD,OAAO,MAAM,CAAC,CAAC,CAAC,GAAG,GAAG,GAAG,MAAM,CAAC,CAAC,CAAC,CAAC;AACrC,CAAC"} apollo-server-demo/node_modules/apollo-tracing/LICENSE 0000644 0001750 0000144 00000002154 03560116604 022352 0 ustar andreh users The MIT License (MIT) Copyright (c) 2016-2020 Apollo Graph, Inc. (Formerly Meteor Development Group, Inc.) Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. apollo-server-demo/node_modules/apollo-tracing/src/ 0000755 0001750 0000144 00000000000 14067647701 022145 5 ustar andreh users apollo-server-demo/node_modules/apollo-tracing/src/index.ts 0000644 0001750 0000144 00000011735 03560116604 023620 0 ustar andreh users import { ResponsePath, responsePathAsArray, GraphQLType, } from 'graphql'; import { ApolloServerPlugin } from "apollo-server-plugin-base"; const { name: PACKAGE_NAME } = require("../package.json"); export interface TracingFormat { version: 1; startTime: string; endTime: string; duration: number; execution: { resolvers: { path: (string | number)[]; parentType: string; fieldName: string; returnType: string; startOffset: number; duration: number; }[]; }; } interface ResolverCall { path: ResponsePath; fieldName: string; parentType: GraphQLType; returnType: GraphQLType; startOffset: HighResolutionTime; endOffset?: HighResolutionTime; } export const plugin = (_futureOptions = {}) => (): ApolloServerPlugin => ({ requestDidStart() { const startWallTime: Date = new Date(); let endWallTime: Date | undefined; const startHrTime: HighResolutionTime = process.hrtime(); let duration: HighResolutionTime | undefined; const resolverCalls: ResolverCall[] = []; return { executionDidStart: () => ({ // It's a little odd that we record the end time after execution rather // than at the end of the whole request, but because we need to include // our formatted trace in the request itself, we have to record it // before the request is over! // Historically speaking: It's WAS odd that we don't do traces for parse // or validation errors. Reason being: at the time that this was written // (now a plugin but originally an extension)). That was the case // because runQuery DIDN'T (again, at the time, when it was an // extension) support that since format() was only invoked after // execution. executionDidEnd: () => { duration = process.hrtime(startHrTime); endWallTime = new Date(); }, willResolveField({ info }) { const resolverCall: ResolverCall = { path: info.path, fieldName: info.fieldName, parentType: info.parentType, returnType: info.returnType, startOffset: process.hrtime(startHrTime), }; resolverCalls.push(resolverCall); return () => { resolverCall.endOffset = process.hrtime(startHrTime); }; }, }), willSendResponse({ response }) { // In the event that we are called prior to the initialization of // critical date metrics, we'll return undefined to signal that the // extension did not format properly. Any undefined extension // results are simply purged by the graphql-extensions module. if ( typeof endWallTime === 'undefined' || typeof duration === 'undefined' ) { return; } const extensions = response.extensions || (response.extensions = Object.create(null)); // Be defensive and make sure nothing else (other plugin, etc.) has // already used the `tracing` property on `extensions`. if (typeof extensions.tracing !== 'undefined') { throw new Error(PACKAGE_NAME + ": Could not add `tracing` to " + "`extensions` since `tracing` was unexpectedly already present."); } // Set the extensions. extensions.tracing = { version: 1, startTime: startWallTime.toISOString(), endTime: endWallTime.toISOString(), duration: durationHrTimeToNanos(duration), execution: { resolvers: resolverCalls.map(resolverCall => { const startOffset = durationHrTimeToNanos( resolverCall.startOffset, ); const duration = resolverCall.endOffset ? durationHrTimeToNanos(resolverCall.endOffset) - startOffset : 0; return { path: [...responsePathAsArray(resolverCall.path)], parentType: resolverCall.parentType.toString(), fieldName: resolverCall.fieldName, returnType: resolverCall.returnType.toString(), startOffset, duration, }; }), }, }; }, }; }, }) type HighResolutionTime = [number, number]; // Converts an hrtime array (as returned from process.hrtime) to nanoseconds. // // ONLY CALL THIS ON VALUES REPRESENTING DELTAS, NOT ON THE RAW RETURN VALUE // FROM process.hrtime() WITH NO ARGUMENTS. // // The entire point of the hrtime data structure is that the JavaScript Number // type can't represent all int64 values without loss of precision: // Number.MAX_SAFE_INTEGER nanoseconds is about 104 days. Calling this function // on a duration that represents a value less than 104 days is fine. Calling // this function on an absolute time (which is generally roughly time since // system boot) is not a good idea. function durationHrTimeToNanos(hrtime: HighResolutionTime) { return hrtime[0] * 1e9 + hrtime[1]; } apollo-server-demo/node_modules/apollo-tracing/README.md 0000644 0001750 0000144 00000002056 03560116604 022625 0 ustar andreh users # Apollo Tracing (for Node.js) This package is used to collect and expose trace data in the [Apollo Tracing](https://github.com/apollographql/apollo-tracing) format. It relies on instrumenting a GraphQL schema to collect resolver timings, and exposes trace data for an individual request under `extensions` as part of the GraphQL response. This data can be consumed by [Apollo Studio](https://www.apollographql.com/docs/studio/) (previously, Apollo Engine and Apollo Graph Manager) or any other tool to provide visualization and history of field-by-field execution performance. ## Usage ### Apollo Server Apollo Server includes built-in support for tracing from version 1.1.0 onwards. The only code change required is to add `tracing: true` to the options passed to the `ApolloServer` constructor options for your integration of choice. For example, for [`apollo-server-express`](https://npm.im/apollo-server-express): ```javascript const { ApolloServer } = require('apollo-server-express'); const server = new ApolloServer({ schema, tracing: true, }); ``` apollo-server-demo/node_modules/apollo-tracing/package.json 0000644 0001750 0000144 00000001476 03560116604 023641 0 ustar andreh users { "name": "apollo-tracing", "version": "0.12.2", "description": "Collect and expose trace data for GraphQL requests", "main": "./dist/index.js", "types": "./dist/index.d.ts", "license": "MIT", "repository": "apollographql/apollo-tracing-js", "author": "Apollo <opensource@apollographql.com>", "engines": { "node": ">=4.0" }, "dependencies": { "apollo-server-env": "^3.0.0", "apollo-server-plugin-base": "^0.10.4" }, "peerDependencies": { "graphql": "^0.12.0 || ^0.13.0 || ^14.0.0 || ^15.0.0" }, "gitHead": "c212627be591cd2c2469321b58b15f1b7909778d" ,"_resolved": "https://registry.npmjs.org/apollo-tracing/-/apollo-tracing-0.12.2.tgz" ,"_integrity": "sha512-SYN4o0C0wR1fyS3+P0FthyvsQVHFopdmN3IU64IaspR/RZScPxZ3Ae8uu++fTvkQflAkglnFM0aX6DkZERBp6w==" ,"_from": "apollo-tracing@0.12.2" } apollo-server-demo/node_modules/xss/ 0000755 0001750 0000144 00000000000 14067647700 017257 5 ustar andreh users apollo-server-demo/node_modules/xss/README.zh.md 0000644 0001750 0000144 00000033041 03560116604 021145 0 ustar andreh users [![NPM version][npm-image]][npm-url] [![build status][travis-image]][travis-url] [![Test coverage][coveralls-image]][coveralls-url] [![David deps][david-image]][david-url] [![node version][node-image]][node-url] [![npm download][download-image]][download-url] [![npm license][license-image]][download-url] [npm-image]: https://img.shields.io/npm/v/xss.svg?style=flat-square [npm-url]: https://npmjs.org/package/xss [travis-image]: https://img.shields.io/travis/leizongmin/js-xss.svg?style=flat-square [travis-url]: https://travis-ci.org/leizongmin/js-xss [coveralls-image]: https://img.shields.io/coveralls/leizongmin/js-xss.svg?style=flat-square [coveralls-url]: https://coveralls.io/r/leizongmin/js-xss?branch=master [david-image]: https://img.shields.io/david/leizongmin/js-xss.svg?style=flat-square [david-url]: https://david-dm.org/leizongmin/js-xss [node-image]: https://img.shields.io/badge/node.js-%3E=_0.10-green.svg?style=flat-square [node-url]: http://nodejs.org/download/ [download-image]: https://img.shields.io/npm/dm/xss.svg?style=flat-square [download-url]: https://npmjs.org/package/xss [license-image]: https://img.shields.io/npm/l/xss.svg # æ ¹æ®ç™½åå•过滤 HTML(é˜²æ¢ XSS 攻击) 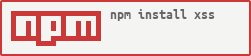 --- `xss`是一个用于对用户输入的内容进行过滤,以é¿å…éå— XSS 攻击的模å—([什么是 XSS 攻击?](http://baike.baidu.com/view/2161269.htm))。主è¦ç”¨äºŽè®ºå›ã€åšå®¢ã€ç½‘上商店ç‰ç‰ä¸€äº›å¯å…è®¸ç”¨æˆ·å½•å…¥é¡µé¢æŽ’ç‰ˆã€æ ¼å¼æŽ§åˆ¶ç›¸å…³çš„ HTML 的场景,`xss`模å—通过白å啿¥æŽ§åˆ¶å…è®¸çš„æ ‡ç¾åŠç›¸å…³çš„æ ‡ç¾å±žæ€§ï¼Œå¦å¤–还æä¾›äº†ä¸€ç³»åˆ—的接å£ä»¥ä¾¿ç”¨æˆ·æ‰©å±•,比其他åŒç±»æ¨¡å—æ›´ä¸ºçµæ´»ã€‚ **项目主页:** http://jsxss.com **在线测试:** http://jsxss.com/zh/try.html --- ## 特性 * 白åå•æŽ§åˆ¶å…许的 HTML æ ‡ç¾åŠå„æ ‡ç¾çš„属性 * 通过自定义处ç†å‡½æ•°ï¼Œå¯å¯¹ä»»æ„æ ‡ç¾åŠå…¶å±žæ€§è¿›è¡Œå¤„ç† ## å‚考资料 * [XSS 与å—符编ç 的那些事儿 ---科普文](http://drops.wooyun.org/tips/689) * [è…¾è®¯å®žä¾‹æ•™ç¨‹ï¼šé‚£äº›å¹´æˆ‘ä»¬ä¸€èµ·å¦ XSS](http://www.wooyun.org/whitehats/%E5%BF%83%E4%BC%A4%E7%9A%84%E7%98%A6%E5%AD%90) * [mXSS 攻击的æˆå› åŠå¸¸è§ç§ç±»](http://drops.wooyun.org/tips/956) * [XSS Filter Evasion Cheat Sheet](https://www.owasp.org/index.php/XSS_Filter_Evasion_Cheat_Sheet) * [Data URI scheme](http://en.wikipedia.org/wiki/Data_URI_scheme) * [XSS with Data URI Scheme](http://hi.baidu.com/badzzzz/item/bdbafe83144619c199255f7b) ## 性能(仅作å‚考) * xss 模å—:22.53 MB/s * validator@0.3.7 模å—çš„ xss()函数:6.9 MB/s 测试代ç å‚考 benchmark 目录 ## 安装 ### NPM ```bash npm install xss ``` ### Bower ```bash bower install xss ``` 或者 ```bash bower install https://github.com/leizongmin/js-xss.git ``` ## 使用方法 ### 在 Node.js ä¸ä½¿ç”¨ ```javascript var xss = require("xss"); var html = xss('<script>alert("xss");</script>'); console.log(html); ``` ### 在æµè§ˆå™¨ç«¯ä½¿ç”¨ Shim 模å¼ï¼ˆå‚考文件 `test/test.html`): ```html <script src="https://rawgit.com/leizongmin/js-xss/master/dist/xss.js"></script> <script> // 使用函数å filterXSSï¼Œç”¨æ³•ä¸€æ · var html = filterXSS('<script>alert("xss");</scr' + 'ipt>'); alert(html); </script> ``` AMD 模å¼ï¼ˆå‚考文件 `test/test_amd.html`): ```html <script> require.config({ baseUrl: './', paths: { xss: 'https://rawgit.com/leizongmin/js-xss/master/dist/xss.js' }, shim: { xss: {exports: 'filterXSS'} } }) require(['xss'], function (xss) { var html = xss('<script>alert("xss");</scr' + 'ipt>'); alert(html); }); </script> ``` **说明:请勿将 URL https://rawgit.com/leizongmin/js-xss/master/dist/xss.js 用于生产环境。** ### 使用命令行工具æ¥å¯¹æ–‡ä»¶è¿›è¡Œ XSS å¤„ç† ### å¤„ç†æ–‡ä»¶ å¯é€šè¿‡å†…置的 `xss` 命令æ¥å¯¹è¾“入的文件进行 XSS 处ç†ã€‚使用方法: ```bash xss -i <æºæ–‡ä»¶> -o <ç›®æ ‡æ–‡ä»¶> ``` 例: ```bash xss -i origin.html -o target.html ``` ### 在线测试 执行以下命令,å¯åœ¨å‘½ä»¤è¡Œä¸è¾“å…¥ HTML 代ç ,并看到过滤åŽçš„代ç : ```bash xss -t ``` è¯¦ç»†å‘½ä»¤è¡Œå‚æ•°è¯´æ˜Žï¼Œè¯·è¾“å…¥ `$ xss -h` æ¥æŸ¥çœ‹ã€‚ ## 自定义过滤规则 在调用 `xss()` 函数进行过滤时,å¯é€šè¿‡ç¬¬äºŒä¸ªå‚æ•°æ¥è®¾ç½®è‡ªå®šä¹‰è§„则: ```javascript options = {}; // 自定义规则 html = xss('<script>alert("xss");</script>', options); ``` å¦‚æžœä¸æƒ³æ¯æ¬¡éƒ½ä¼ 入一个 `options` 傿•°ï¼Œå¯ä»¥åˆ›å»ºä¸€ä¸ª `FilterXSS` å®žä¾‹ï¼ˆä½¿ç”¨è¿™ç§æ–¹æ³•速度更快): ``` options = {}; // 自定义规则 myxss = new xss.FilterXSS(options); // 以åŽç›´æŽ¥è°ƒç”¨ myxss.process() æ¥å¤„ç†å³å¯ html = myxss.process('<script>alert("xss");</script>'); ``` `options` 傿•°çš„详细说明è§ä¸‹æ–‡ã€‚ ### 白åå• é€šè¿‡ `whiteList` æ¥æŒ‡å®šï¼Œæ ¼å¼ä¸ºï¼š`{'æ ‡ç¾å': ['属性1', '属性2']}`。ä¸åœ¨ç™½åå•ä¸Šçš„æ ‡ç¾å°†è¢«è¿‡æ»¤ï¼Œä¸åœ¨ç™½åå•上的属性也会被过滤。以下是示例: ```javascript // åªå…许aæ ‡ç¾ï¼Œè¯¥æ ‡ç¾åªå…许href, title, target这三个属性 var options = { whiteList: { a: ["href", "title", "target"] } }; // 使用以上é…ç½®åŽï¼Œä¸‹é¢çš„HTML // <a href="#" onclick="hello()"><i>大家好</i></a> // 将被过滤为 // <a href="#">大家好</a> ``` 默认白åå•å‚考 `xss.whiteList`。 ### 自定义匹é…åˆ°æ ‡ç¾æ—¶çš„å¤„ç†æ–¹æ³• 通过 `onTag` æ¥æŒ‡å®šç›¸åº”的处ç†å‡½æ•°ã€‚以下是详细说明: ```javascript function onTag(tag, html, options) { // tag是当å‰çš„æ ‡ç¾å称,比如<a>æ ‡ç¾ï¼Œåˆ™tag的值是'a' // htmlæ˜¯è¯¥æ ‡ç¾çš„HTML,比如<a>æ ‡ç¾ï¼Œåˆ™html的值是'<a>' // optionsæ˜¯ä¸€äº›é™„åŠ çš„ä¿¡æ¯ï¼Œå…·ä½“如下: // isWhite booleanç±»åž‹ï¼Œè¡¨ç¤ºè¯¥æ ‡ç¾æ˜¯å¦åœ¨ç™½åå•上 // isClosing booleanç±»åž‹ï¼Œè¡¨ç¤ºè¯¥æ ‡ç¾æ˜¯å¦ä¸ºé—åˆæ ‡ç¾ï¼Œæ¯”如</a>时为true // position integerç±»åž‹ï¼Œè¡¨ç¤ºå½“å‰æ ‡ç¾åœ¨è¾“出的结果ä¸çš„èµ·å§‹ä½ç½® // sourcePosition integerç±»åž‹ï¼Œè¡¨ç¤ºå½“å‰æ ‡ç¾åœ¨åŽŸHTMLä¸çš„èµ·å§‹ä½ç½® // 如果返回一个å—ç¬¦ä¸²ï¼Œåˆ™å½“å‰æ ‡ç¾å°†è¢«æ›¿æ¢ä¸ºè¯¥å—符串 // 如果ä¸è¿”å›žä»»ä½•å€¼ï¼Œåˆ™ä½¿ç”¨é»˜è®¤çš„å¤„ç†æ–¹æ³•: // 在白åå•上: 通过onTagAttræ¥è¿‡æ»¤å±žæ€§ï¼Œè¯¦è§ä¸‹æ–‡ // ä¸åœ¨ç™½åå•上:通过onIgnoreTag指定,详è§ä¸‹æ–‡ } ``` ### 自定义匹é…åˆ°æ ‡ç¾çš„å±žæ€§æ—¶çš„å¤„ç†æ–¹æ³• 通过 `onTagAttr` æ¥æŒ‡å®šç›¸åº”的处ç†å‡½æ•°ã€‚以下是详细说明: ```javascript function onTagAttr(tag, name, value, isWhiteAttr) { // tag是当å‰çš„æ ‡ç¾å称,比如<a>æ ‡ç¾ï¼Œåˆ™tag的值是'a' // name是当å‰å±žæ€§çš„å称,比如href="#",则name的值是'href' // value是当å‰å±žæ€§çš„值,比如href="#",则value的值是'#' // isWhiteAttr是å¦ä¸ºç™½åå•上的属性 // 如果返回一个å—符串,则当å‰å±žæ€§å€¼å°†è¢«æ›¿æ¢ä¸ºè¯¥å—符串 // 如果ä¸è¿”å›žä»»ä½•å€¼ï¼Œåˆ™ä½¿ç”¨é»˜è®¤çš„å¤„ç†æ–¹æ³• // 在白åå•上: 调用safeAttrValueæ¥è¿‡æ»¤å±žæ€§å€¼ï¼Œå¹¶è¾“出该属性,详è§ä¸‹æ–‡ // ä¸åœ¨ç™½åå•上:通过onIgnoreTagAttr指定,详è§ä¸‹æ–‡ } ``` ### 自定义匹é…到ä¸åœ¨ç™½åå•ä¸Šçš„æ ‡ç¾æ—¶çš„å¤„ç†æ–¹æ³• 通过 `onIgnoreTag` æ¥æŒ‡å®šç›¸åº”的处ç†å‡½æ•°ã€‚以下是详细说明: ```javascript function onIgnoreTag(tag, html, options) { // 傿•°è¯´æ˜Žä¸ŽonTagç›¸åŒ // 如果返回一个å—ç¬¦ä¸²ï¼Œåˆ™å½“å‰æ ‡ç¾å°†è¢«æ›¿æ¢ä¸ºè¯¥å—符串 // 如果ä¸è¿”å›žä»»ä½•å€¼ï¼Œåˆ™ä½¿ç”¨é»˜è®¤çš„å¤„ç†æ–¹æ³•(通过escape指定,详è§ä¸‹æ–‡ï¼‰ } ``` ### 自定义匹é…到ä¸åœ¨ç™½åå•ä¸Šçš„å±žæ€§æ—¶çš„å¤„ç†æ–¹æ³• 通过 `onIgnoreTagAttr` æ¥æŒ‡å®šç›¸åº”的处ç†å‡½æ•°ã€‚以下是详细说明: ```javascript function onIgnoreTagAttr(tag, name, value, isWhiteAttr) { // 傿•°è¯´æ˜Žä¸ŽonTagAttrç›¸åŒ // 如果返回一个å—符串,则当å‰å±žæ€§å€¼å°†è¢«æ›¿æ¢ä¸ºè¯¥å—符串 // 如果ä¸è¿”å›žä»»ä½•å€¼ï¼Œåˆ™ä½¿ç”¨é»˜è®¤çš„å¤„ç†æ–¹æ³•ï¼ˆåˆ é™¤è¯¥å±žï¼‰ } ``` ### 自定义 HTML 转义函数 通过 `escapeHtml` æ¥æŒ‡å®šç›¸åº”的处ç†å‡½æ•°ã€‚以下是默认代ç **(ä¸å»ºè®®ä¿®æ”¹ï¼‰** : ```javascript function escapeHtml(html) { return html.replace(/</g, "<").replace(/>/g, ">"); } ``` ### è‡ªå®šä¹‰æ ‡ç¾å±žæ€§å€¼çš„转义函数 通过 `safeAttrValue` æ¥æŒ‡å®šç›¸åº”的处ç†å‡½æ•°ã€‚以下是详细说明: ```javascript function safeAttrValue(tag, name, value) { // 傿•°è¯´æ˜Žä¸ŽonTagAttr相åŒï¼ˆæ²¡æœ‰options傿•°ï¼‰ // 返回一个å—符串表示该属性值 } ``` ### 自定义 CSS 过滤器 如果é…ç½®ä¸å…è®¸äº†æ ‡ç¾çš„ `style` 属性,则它的值会通过[cssfilter](https://github.com/leizongmin/js-css-filter) 模å—处ç†ã€‚ `cssfilter` 模å—包å«äº†ä¸€ä¸ªé»˜è®¤çš„ CSS 白åå•ï¼Œä½ å¯ä»¥é€šè¿‡ä»¥ä¸‹çš„æ–¹å¼é…置: ```javascript myxss = new xss.FilterXSS({ css: { whiteList: { position: /^fixed|relative$/, top: true, left: true } } }); html = myxss.process('<script>alert("xss");</script>'); ``` å¦‚æžœä¸æƒ³ä½¿ç”¨ CSS 过滤器æ¥å¤„ç† `style` å±žæ€§çš„å†…å®¹ï¼Œå¯æŒ‡å®š `css` 选项的值为 `false`: ```javascript myxss = new xss.FilterXSS({ css: false }); ``` è¦èŽ·å–æ›´å¤šçš„帮助信æ¯å¯çœ‹è¿™é‡Œï¼šhttps://github.com/leizongmin/js-css-filter ### å¿«æ·é…ç½® #### 去掉ä¸åœ¨ç™½åå•ä¸Šçš„æ ‡ç¾ é€šè¿‡ `stripIgnoreTag` æ¥è®¾ç½®ï¼š * `true`:去掉ä¸åœ¨ç™½åå•ä¸Šçš„æ ‡ç¾ * `false`:(默认),使用é…置的`escape`å‡½æ•°å¯¹è¯¥æ ‡ç¾è¿›è¡Œè½¬ä¹‰ 示例: 当设置 `stripIgnoreTag = true`时,以下代ç ```html code:<script>alert(/xss/);</script> ``` 过滤åŽå°†è¾“出 ```html code:alert(/xss/); ``` #### 去掉ä¸åœ¨ç™½åå•ä¸Šçš„æ ‡ç¾åŠæ ‡ç¾ä½“ 通过 `stripIgnoreTagBody` æ¥è®¾ç½®ï¼š * `false|null|undefined`:(默认),ä¸ç‰¹æ®Šå¤„ç† * `'*'|true`:去掉所有ä¸åœ¨ç™½åå•ä¸Šçš„æ ‡ç¾ * `['tag1', 'tag2']`:仅去掉指定的ä¸åœ¨ç™½åå•ä¸Šçš„æ ‡ç¾ ç¤ºä¾‹ï¼š 当设置 `stripIgnoreTagBody = ['script']`时,以下代ç ```html code:<script>alert(/xss/);</script> ``` 过滤åŽå°†è¾“出 ```html code: ``` #### 去掉 HTML 备注 通过 `allowCommentTag` æ¥è®¾ç½®ï¼š * `true`:ä¸å¤„ç† * `false`:(默认),自动去掉 HTML ä¸çš„备注 示例: 当设置 `allowCommentTag = false` 时,以下代ç ```html code:<!-- something --> END ``` 过滤åŽå°†è¾“出 ```html code: END ``` ## 应用实例 ### å…è®¸æ ‡ç¾ä»¥ data-开头的属性 ```javascript var source = '<div a="1" b="2" data-a="3" data-b="4">hello</div>'; var html = xss(source, { onIgnoreTagAttr: function(tag, name, value, isWhiteAttr) { if (name.substr(0, 5) === "data-") { // 通过内置的escapeAttrValue函数æ¥å¯¹å±žæ€§å€¼è¿›è¡Œè½¬ä¹‰ return name + '="' + xss.escapeAttrValue(value) + '"'; } } }); console.log("%s\nconvert to:\n%s", source, html); ``` è¿è¡Œç»“果: ```html <div a="1" b="2" data-a="3" data-b="4">hello</div> convert to: <div data-a="3" data-b="4">hello</div> ``` ### å…许å称以 x-å¼€å¤´çš„æ ‡ç¾ ```javascript var source = "<x><x-1>he<x-2 checked></x-2>wwww</x-1><a>"; var html = xss(source, { onIgnoreTag: function(tag, html, options) { if (tag.substr(0, 2) === "x-") { // ä¸å¯¹å…¶å±žæ€§åˆ—表进行过滤 return html; } } }); console.log("%s\nconvert to:\n%s", source, html); ``` è¿è¡Œç»“果: ```html <x><x-1>he<x-2 checked></x-2>wwww</x-1><a> convert to: <x><x-1>he<x-2 checked></x-2>wwww</x-1><a> ``` ### åˆ†æž HTML 代ç ä¸çš„图片列表 ```javascript var source = '<img src="img1">a<img src="img2">b<img src="img3">c<img src="img4">d'; var list = []; var html = xss(source, { onTagAttr: function(tag, name, value, isWhiteAttr) { if (tag === "img" && name === "src") { // 使用内置的friendlyAttrValue函数æ¥å¯¹å±žæ€§å€¼è¿›è¡Œè½¬ä¹‰ï¼Œå¯å°†<è¿™ç±»çš„å®žä½“æ ‡è®°è½¬æ¢æˆæ‰“å°å—符< list.push(xss.friendlyAttrValue(value)); } // ä¸è¿”å›žä»»ä½•å€¼ï¼Œè¡¨ç¤ºè¿˜æ˜¯æŒ‰ç…§é»˜è®¤çš„æ–¹æ³•å¤„ç† } }); console.log("image list:\n%s", list.join(", ")); ``` è¿è¡Œç»“果: ```html image list: img1, img2, img3, img4 ``` ### 去除 HTML æ ‡ç¾ï¼ˆåªä¿ç•™æ–‡æœ¬å†…容) ```javascript var source = "<strong>hello</strong><script>alert(/xss/);</script>end"; var html = xss(source, { whiteList: [], // 白åå•ä¸ºç©ºï¼Œè¡¨ç¤ºè¿‡æ»¤æ‰€æœ‰æ ‡ç¾ stripIgnoreTag: true, // 过滤所有éžç™½å啿 ‡ç¾çš„HTML stripIgnoreTagBody: ["script"] // scriptæ ‡ç¾è¾ƒç‰¹æ®Šï¼Œéœ€è¦è¿‡æ»¤æ ‡ç¾ä¸é—´çš„内容 }); console.log("text: %s", html); ``` è¿è¡Œç»“果: ```html text: helloend ``` ## 授æƒåè®® ```text Copyright (c) 2012-2018 Zongmin Lei(é›·å®—æ°‘) <leizongmin@gmail.com> http://ucdok.com The MIT License Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. ``` apollo-server-demo/node_modules/xss/dist/ 0000755 0001750 0000144 00000000000 14067647700 020222 5 ustar andreh users apollo-server-demo/node_modules/xss/dist/xss.js 0000644 0001750 0000144 00000142457 03560116604 021400 0 ustar andreh users (function(){function r(e,n,t){function o(i,f){if(!n[i]){if(!e[i]){var c="function"==typeof require&&require;if(!f&&c)return c(i,!0);if(u)return u(i,!0);var a=new Error("Cannot find module '"+i+"'");throw a.code="MODULE_NOT_FOUND",a}var p=n[i]={exports:{}};e[i][0].call(p.exports,function(r){var n=e[i][1][r];return o(n||r)},p,p.exports,r,e,n,t)}return n[i].exports}for(var u="function"==typeof require&&require,i=0;i<t.length;i++)o(t[i]);return o}return r})()({1:[function(require,module,exports){ /** * default settings * * @author Zongmin Lei<leizongmin@gmail.com> */ var FilterCSS = require("cssfilter").FilterCSS; var getDefaultCSSWhiteList = require("cssfilter").getDefaultWhiteList; var _ = require("./util"); function getDefaultWhiteList() { return { a: ["target", "href", "title"], abbr: ["title"], address: [], area: ["shape", "coords", "href", "alt"], article: [], aside: [], audio: ["autoplay", "controls", "loop", "preload", "src"], b: [], bdi: ["dir"], bdo: ["dir"], big: [], blockquote: ["cite"], br: [], caption: [], center: [], cite: [], code: [], col: ["align", "valign", "span", "width"], colgroup: ["align", "valign", "span", "width"], dd: [], del: ["datetime"], details: ["open"], div: [], dl: [], dt: [], em: [], font: ["color", "size", "face"], footer: [], h1: [], h2: [], h3: [], h4: [], h5: [], h6: [], header: [], hr: [], i: [], img: ["src", "alt", "title", "width", "height"], ins: ["datetime"], li: [], mark: [], nav: [], ol: [], p: [], pre: [], s: [], section: [], small: [], span: [], sub: [], sup: [], strong: [], table: ["width", "border", "align", "valign"], tbody: ["align", "valign"], td: ["width", "rowspan", "colspan", "align", "valign"], tfoot: ["align", "valign"], th: ["width", "rowspan", "colspan", "align", "valign"], thead: ["align", "valign"], tr: ["rowspan", "align", "valign"], tt: [], u: [], ul: [], video: ["autoplay", "controls", "loop", "preload", "src", "height", "width"] }; } var defaultCSSFilter = new FilterCSS(); /** * default onTag function * * @param {String} tag * @param {String} html * @param {Object} options * @return {String} */ function onTag(tag, html, options) { // do nothing } /** * default onIgnoreTag function * * @param {String} tag * @param {String} html * @param {Object} options * @return {String} */ function onIgnoreTag(tag, html, options) { // do nothing } /** * default onTagAttr function * * @param {String} tag * @param {String} name * @param {String} value * @return {String} */ function onTagAttr(tag, name, value) { // do nothing } /** * default onIgnoreTagAttr function * * @param {String} tag * @param {String} name * @param {String} value * @return {String} */ function onIgnoreTagAttr(tag, name, value) { // do nothing } /** * default escapeHtml function * * @param {String} html */ function escapeHtml(html) { return html.replace(REGEXP_LT, "<").replace(REGEXP_GT, ">"); } /** * default safeAttrValue function * * @param {String} tag * @param {String} name * @param {String} value * @param {Object} cssFilter * @return {String} */ function safeAttrValue(tag, name, value, cssFilter) { // unescape attribute value firstly value = friendlyAttrValue(value); if (name === "href" || name === "src") { // filter `href` and `src` attribute // only allow the value that starts with `http://` | `https://` | `mailto:` | `/` | `#` value = _.trim(value); if (value === "#") return "#"; if ( !( value.substr(0, 7) === "http://" || value.substr(0, 8) === "https://" || value.substr(0, 7) === "mailto:" || value.substr(0, 4) === "tel:" || value.substr(0, 11) === "data:image/" || value.substr(0, 6) === "ftp://" || value.substr(0, 2) === "./" || value.substr(0, 3) === "../" || value[0] === "#" || value[0] === "/" ) ) { return ""; } } else if (name === "background") { // filter `background` attribute (maybe no use) // `javascript:` REGEXP_DEFAULT_ON_TAG_ATTR_4.lastIndex = 0; if (REGEXP_DEFAULT_ON_TAG_ATTR_4.test(value)) { return ""; } } else if (name === "style") { // `expression()` REGEXP_DEFAULT_ON_TAG_ATTR_7.lastIndex = 0; if (REGEXP_DEFAULT_ON_TAG_ATTR_7.test(value)) { return ""; } // `url()` REGEXP_DEFAULT_ON_TAG_ATTR_8.lastIndex = 0; if (REGEXP_DEFAULT_ON_TAG_ATTR_8.test(value)) { REGEXP_DEFAULT_ON_TAG_ATTR_4.lastIndex = 0; if (REGEXP_DEFAULT_ON_TAG_ATTR_4.test(value)) { return ""; } } if (cssFilter !== false) { cssFilter = cssFilter || defaultCSSFilter; value = cssFilter.process(value); } } // escape `<>"` before returns value = escapeAttrValue(value); return value; } // RegExp list var REGEXP_LT = /</g; var REGEXP_GT = />/g; var REGEXP_QUOTE = /"/g; var REGEXP_QUOTE_2 = /"/g; var REGEXP_ATTR_VALUE_1 = /&#([a-zA-Z0-9]*);?/gim; var REGEXP_ATTR_VALUE_COLON = /:?/gim; var REGEXP_ATTR_VALUE_NEWLINE = /&newline;?/gim; var REGEXP_DEFAULT_ON_TAG_ATTR_3 = /\/\*|\*\//gm; var REGEXP_DEFAULT_ON_TAG_ATTR_4 = /((j\s*a\s*v\s*a|v\s*b|l\s*i\s*v\s*e)\s*s\s*c\s*r\s*i\s*p\s*t\s*|m\s*o\s*c\s*h\s*a)\:/gi; var REGEXP_DEFAULT_ON_TAG_ATTR_5 = /^[\s"'`]*(d\s*a\s*t\s*a\s*)\:/gi; var REGEXP_DEFAULT_ON_TAG_ATTR_6 = /^[\s"'`]*(d\s*a\s*t\s*a\s*)\:\s*image\//gi; var REGEXP_DEFAULT_ON_TAG_ATTR_7 = /e\s*x\s*p\s*r\s*e\s*s\s*s\s*i\s*o\s*n\s*\(.*/gi; var REGEXP_DEFAULT_ON_TAG_ATTR_8 = /u\s*r\s*l\s*\(.*/gi; /** * escape doube quote * * @param {String} str * @return {String} str */ function escapeQuote(str) { return str.replace(REGEXP_QUOTE, """); } /** * unescape double quote * * @param {String} str * @return {String} str */ function unescapeQuote(str) { return str.replace(REGEXP_QUOTE_2, '"'); } /** * escape html entities * * @param {String} str * @return {String} */ function escapeHtmlEntities(str) { return str.replace(REGEXP_ATTR_VALUE_1, function replaceUnicode(str, code) { return code[0] === "x" || code[0] === "X" ? String.fromCharCode(parseInt(code.substr(1), 16)) : String.fromCharCode(parseInt(code, 10)); }); } /** * escape html5 new danger entities * * @param {String} str * @return {String} */ function escapeDangerHtml5Entities(str) { return str .replace(REGEXP_ATTR_VALUE_COLON, ":") .replace(REGEXP_ATTR_VALUE_NEWLINE, " "); } /** * clear nonprintable characters * * @param {String} str * @return {String} */ function clearNonPrintableCharacter(str) { var str2 = ""; for (var i = 0, len = str.length; i < len; i++) { str2 += str.charCodeAt(i) < 32 ? " " : str.charAt(i); } return _.trim(str2); } /** * get friendly attribute value * * @param {String} str * @return {String} */ function friendlyAttrValue(str) { str = unescapeQuote(str); str = escapeHtmlEntities(str); str = escapeDangerHtml5Entities(str); str = clearNonPrintableCharacter(str); return str; } /** * unescape attribute value * * @param {String} str * @return {String} */ function escapeAttrValue(str) { str = escapeQuote(str); str = escapeHtml(str); return str; } /** * `onIgnoreTag` function for removing all the tags that are not in whitelist */ function onIgnoreTagStripAll() { return ""; } /** * remove tag body * specify a `tags` list, if the tag is not in the `tags` list then process by the specify function (optional) * * @param {array} tags * @param {function} next */ function StripTagBody(tags, next) { if (typeof next !== "function") { next = function() {}; } var isRemoveAllTag = !Array.isArray(tags); function isRemoveTag(tag) { if (isRemoveAllTag) return true; return _.indexOf(tags, tag) !== -1; } var removeList = []; var posStart = false; return { onIgnoreTag: function(tag, html, options) { if (isRemoveTag(tag)) { if (options.isClosing) { var ret = "[/removed]"; var end = options.position + ret.length; removeList.push([ posStart !== false ? posStart : options.position, end ]); posStart = false; return ret; } else { if (!posStart) { posStart = options.position; } return "[removed]"; } } else { return next(tag, html, options); } }, remove: function(html) { var rethtml = ""; var lastPos = 0; _.forEach(removeList, function(pos) { rethtml += html.slice(lastPos, pos[0]); lastPos = pos[1]; }); rethtml += html.slice(lastPos); return rethtml; } }; } /** * remove html comments * * @param {String} html * @return {String} */ function stripCommentTag(html) { return html.replace(STRIP_COMMENT_TAG_REGEXP, ""); } var STRIP_COMMENT_TAG_REGEXP = /<!--[\s\S]*?-->/g; /** * remove invisible characters * * @param {String} html * @return {String} */ function stripBlankChar(html) { var chars = html.split(""); chars = chars.filter(function(char) { var c = char.charCodeAt(0); if (c === 127) return false; if (c <= 31) { if (c === 10 || c === 13) return true; return false; } return true; }); return chars.join(""); } exports.whiteList = getDefaultWhiteList(); exports.getDefaultWhiteList = getDefaultWhiteList; exports.onTag = onTag; exports.onIgnoreTag = onIgnoreTag; exports.onTagAttr = onTagAttr; exports.onIgnoreTagAttr = onIgnoreTagAttr; exports.safeAttrValue = safeAttrValue; exports.escapeHtml = escapeHtml; exports.escapeQuote = escapeQuote; exports.unescapeQuote = unescapeQuote; exports.escapeHtmlEntities = escapeHtmlEntities; exports.escapeDangerHtml5Entities = escapeDangerHtml5Entities; exports.clearNonPrintableCharacter = clearNonPrintableCharacter; exports.friendlyAttrValue = friendlyAttrValue; exports.escapeAttrValue = escapeAttrValue; exports.onIgnoreTagStripAll = onIgnoreTagStripAll; exports.StripTagBody = StripTagBody; exports.stripCommentTag = stripCommentTag; exports.stripBlankChar = stripBlankChar; exports.cssFilter = defaultCSSFilter; exports.getDefaultCSSWhiteList = getDefaultCSSWhiteList; },{"./util":4,"cssfilter":8}],2:[function(require,module,exports){ /** * xss * * @author Zongmin Lei<leizongmin@gmail.com> */ var DEFAULT = require("./default"); var parser = require("./parser"); var FilterXSS = require("./xss"); /** * filter xss function * * @param {String} html * @param {Object} options { whiteList, onTag, onTagAttr, onIgnoreTag, onIgnoreTagAttr, safeAttrValue, escapeHtml } * @return {String} */ function filterXSS(html, options) { var xss = new FilterXSS(options); return xss.process(html); } exports = module.exports = filterXSS; exports.filterXSS = filterXSS; exports.FilterXSS = FilterXSS; for (var i in DEFAULT) exports[i] = DEFAULT[i]; for (var i in parser) exports[i] = parser[i]; // using `xss` on the browser, output `filterXSS` to the globals if (typeof window !== "undefined") { window.filterXSS = module.exports; } // using `xss` on the WebWorker, output `filterXSS` to the globals function isWorkerEnv() { return typeof self !== 'undefined' && typeof DedicatedWorkerGlobalScope !== 'undefined' && self instanceof DedicatedWorkerGlobalScope; } if (isWorkerEnv()) { self.filterXSS = module.exports; } },{"./default":1,"./parser":3,"./xss":5}],3:[function(require,module,exports){ /** * Simple HTML Parser * * @author Zongmin Lei<leizongmin@gmail.com> */ var _ = require("./util"); /** * get tag name * * @param {String} html e.g. '<a hef="#">' * @return {String} */ function getTagName(html) { var i = _.spaceIndex(html); if (i === -1) { var tagName = html.slice(1, -1); } else { var tagName = html.slice(1, i + 1); } tagName = _.trim(tagName).toLowerCase(); if (tagName.slice(0, 1) === "/") tagName = tagName.slice(1); if (tagName.slice(-1) === "/") tagName = tagName.slice(0, -1); return tagName; } /** * is close tag? * * @param {String} html 如:'<a hef="#">' * @return {Boolean} */ function isClosing(html) { return html.slice(0, 2) === "</"; } /** * parse input html and returns processed html * * @param {String} html * @param {Function} onTag e.g. function (sourcePosition, position, tag, html, isClosing) * @param {Function} escapeHtml * @return {String} */ function parseTag(html, onTag, escapeHtml) { "use strict"; var rethtml = ""; var lastPos = 0; var tagStart = false; var quoteStart = false; var currentPos = 0; var len = html.length; var currentTagName = ""; var currentHtml = ""; chariterator: for (currentPos = 0; currentPos < len; currentPos++) { var c = html.charAt(currentPos); if (tagStart === false) { if (c === "<") { tagStart = currentPos; continue; } } else { if (quoteStart === false) { if (c === "<") { rethtml += escapeHtml(html.slice(lastPos, currentPos)); tagStart = currentPos; lastPos = currentPos; continue; } if (c === ">") { rethtml += escapeHtml(html.slice(lastPos, tagStart)); currentHtml = html.slice(tagStart, currentPos + 1); currentTagName = getTagName(currentHtml); rethtml += onTag( tagStart, rethtml.length, currentTagName, currentHtml, isClosing(currentHtml) ); lastPos = currentPos + 1; tagStart = false; continue; } if ((c === '"' || c === "'")) { var i = 1; var ic = html.charAt(currentPos - i); while ((ic === " ") || (ic === "=")) { if (ic === "=") { quoteStart = c; continue chariterator; } ic = html.charAt(currentPos - ++i); } } } else { if (c === quoteStart) { quoteStart = false; continue; } } } } if (lastPos < html.length) { rethtml += escapeHtml(html.substr(lastPos)); } return rethtml; } var REGEXP_ILLEGAL_ATTR_NAME = /[^a-zA-Z0-9_:\.\-]/gim; /** * parse input attributes and returns processed attributes * * @param {String} html e.g. `href="#" target="_blank"` * @param {Function} onAttr e.g. `function (name, value)` * @return {String} */ function parseAttr(html, onAttr) { "use strict"; var lastPos = 0; var retAttrs = []; var tmpName = false; var len = html.length; function addAttr(name, value) { name = _.trim(name); name = name.replace(REGEXP_ILLEGAL_ATTR_NAME, "").toLowerCase(); if (name.length < 1) return; var ret = onAttr(name, value || ""); if (ret) retAttrs.push(ret); } // é€ä¸ªåˆ†æžå—符 for (var i = 0; i < len; i++) { var c = html.charAt(i); var v, j; if (tmpName === false && c === "=") { tmpName = html.slice(lastPos, i); lastPos = i + 1; continue; } if (tmpName !== false) { if ( i === lastPos && (c === '"' || c === "'") && html.charAt(i - 1) === "=" ) { j = html.indexOf(c, i + 1); if (j === -1) { break; } else { v = _.trim(html.slice(lastPos + 1, j)); addAttr(tmpName, v); tmpName = false; i = j; lastPos = i + 1; continue; } } } if (/\s|\n|\t/.test(c)) { html = html.replace(/\s|\n|\t/g, " "); if (tmpName === false) { j = findNextEqual(html, i); if (j === -1) { v = _.trim(html.slice(lastPos, i)); addAttr(v); tmpName = false; lastPos = i + 1; continue; } else { i = j - 1; continue; } } else { j = findBeforeEqual(html, i - 1); if (j === -1) { v = _.trim(html.slice(lastPos, i)); v = stripQuoteWrap(v); addAttr(tmpName, v); tmpName = false; lastPos = i + 1; continue; } else { continue; } } } } if (lastPos < html.length) { if (tmpName === false) { addAttr(html.slice(lastPos)); } else { addAttr(tmpName, stripQuoteWrap(_.trim(html.slice(lastPos)))); } } return _.trim(retAttrs.join(" ")); } function findNextEqual(str, i) { for (; i < str.length; i++) { var c = str[i]; if (c === " ") continue; if (c === "=") return i; return -1; } } function findBeforeEqual(str, i) { for (; i > 0; i--) { var c = str[i]; if (c === " ") continue; if (c === "=") return i; return -1; } } function isQuoteWrapString(text) { if ( (text[0] === '"' && text[text.length - 1] === '"') || (text[0] === "'" && text[text.length - 1] === "'") ) { return true; } else { return false; } } function stripQuoteWrap(text) { if (isQuoteWrapString(text)) { return text.substr(1, text.length - 2); } else { return text; } } exports.parseTag = parseTag; exports.parseAttr = parseAttr; },{"./util":4}],4:[function(require,module,exports){ module.exports = { indexOf: function(arr, item) { var i, j; if (Array.prototype.indexOf) { return arr.indexOf(item); } for (i = 0, j = arr.length; i < j; i++) { if (arr[i] === item) { return i; } } return -1; }, forEach: function(arr, fn, scope) { var i, j; if (Array.prototype.forEach) { return arr.forEach(fn, scope); } for (i = 0, j = arr.length; i < j; i++) { fn.call(scope, arr[i], i, arr); } }, trim: function(str) { if (String.prototype.trim) { return str.trim(); } return str.replace(/(^\s*)|(\s*$)/g, ""); }, spaceIndex: function(str) { var reg = /\s|\n|\t/; var match = reg.exec(str); return match ? match.index : -1; } }; },{}],5:[function(require,module,exports){ /** * filter xss * * @author Zongmin Lei<leizongmin@gmail.com> */ var FilterCSS = require("cssfilter").FilterCSS; var DEFAULT = require("./default"); var parser = require("./parser"); var parseTag = parser.parseTag; var parseAttr = parser.parseAttr; var _ = require("./util"); /** * returns `true` if the input value is `undefined` or `null` * * @param {Object} obj * @return {Boolean} */ function isNull(obj) { return obj === undefined || obj === null; } /** * get attributes for a tag * * @param {String} html * @return {Object} * - {String} html * - {Boolean} closing */ function getAttrs(html) { var i = _.spaceIndex(html); if (i === -1) { return { html: "", closing: html[html.length - 2] === "/" }; } html = _.trim(html.slice(i + 1, -1)); var isClosing = html[html.length - 1] === "/"; if (isClosing) html = _.trim(html.slice(0, -1)); return { html: html, closing: isClosing }; } /** * shallow copy * * @param {Object} obj * @return {Object} */ function shallowCopyObject(obj) { var ret = {}; for (var i in obj) { ret[i] = obj[i]; } return ret; } /** * FilterXSS class * * @param {Object} options * whiteList, onTag, onTagAttr, onIgnoreTag, * onIgnoreTagAttr, safeAttrValue, escapeHtml * stripIgnoreTagBody, allowCommentTag, stripBlankChar * css{whiteList, onAttr, onIgnoreAttr} `css=false` means don't use `cssfilter` */ function FilterXSS(options) { options = shallowCopyObject(options || {}); if (options.stripIgnoreTag) { if (options.onIgnoreTag) { console.error( 'Notes: cannot use these two options "stripIgnoreTag" and "onIgnoreTag" at the same time' ); } options.onIgnoreTag = DEFAULT.onIgnoreTagStripAll; } options.whiteList = options.whiteList || DEFAULT.whiteList; options.onTag = options.onTag || DEFAULT.onTag; options.onTagAttr = options.onTagAttr || DEFAULT.onTagAttr; options.onIgnoreTag = options.onIgnoreTag || DEFAULT.onIgnoreTag; options.onIgnoreTagAttr = options.onIgnoreTagAttr || DEFAULT.onIgnoreTagAttr; options.safeAttrValue = options.safeAttrValue || DEFAULT.safeAttrValue; options.escapeHtml = options.escapeHtml || DEFAULT.escapeHtml; this.options = options; if (options.css === false) { this.cssFilter = false; } else { options.css = options.css || {}; this.cssFilter = new FilterCSS(options.css); } } /** * start process and returns result * * @param {String} html * @return {String} */ FilterXSS.prototype.process = function(html) { // compatible with the input html = html || ""; html = html.toString(); if (!html) return ""; var me = this; var options = me.options; var whiteList = options.whiteList; var onTag = options.onTag; var onIgnoreTag = options.onIgnoreTag; var onTagAttr = options.onTagAttr; var onIgnoreTagAttr = options.onIgnoreTagAttr; var safeAttrValue = options.safeAttrValue; var escapeHtml = options.escapeHtml; var cssFilter = me.cssFilter; // remove invisible characters if (options.stripBlankChar) { html = DEFAULT.stripBlankChar(html); } // remove html comments if (!options.allowCommentTag) { html = DEFAULT.stripCommentTag(html); } // if enable stripIgnoreTagBody var stripIgnoreTagBody = false; if (options.stripIgnoreTagBody) { var stripIgnoreTagBody = DEFAULT.StripTagBody( options.stripIgnoreTagBody, onIgnoreTag ); onIgnoreTag = stripIgnoreTagBody.onIgnoreTag; } var retHtml = parseTag( html, function(sourcePosition, position, tag, html, isClosing) { var info = { sourcePosition: sourcePosition, position: position, isClosing: isClosing, isWhite: whiteList.hasOwnProperty(tag) }; // call `onTag()` var ret = onTag(tag, html, info); if (!isNull(ret)) return ret; if (info.isWhite) { if (info.isClosing) { return "</" + tag + ">"; } var attrs = getAttrs(html); var whiteAttrList = whiteList[tag]; var attrsHtml = parseAttr(attrs.html, function(name, value) { // call `onTagAttr()` var isWhiteAttr = _.indexOf(whiteAttrList, name) !== -1; var ret = onTagAttr(tag, name, value, isWhiteAttr); if (!isNull(ret)) return ret; if (isWhiteAttr) { // call `safeAttrValue()` value = safeAttrValue(tag, name, value, cssFilter); if (value) { return name + '="' + value + '"'; } else { return name; } } else { // call `onIgnoreTagAttr()` var ret = onIgnoreTagAttr(tag, name, value, isWhiteAttr); if (!isNull(ret)) return ret; return; } }); // build new tag html var html = "<" + tag; if (attrsHtml) html += " " + attrsHtml; if (attrs.closing) html += " /"; html += ">"; return html; } else { // call `onIgnoreTag()` var ret = onIgnoreTag(tag, html, info); if (!isNull(ret)) return ret; return escapeHtml(html); } }, escapeHtml ); // if enable stripIgnoreTagBody if (stripIgnoreTagBody) { retHtml = stripIgnoreTagBody.remove(retHtml); } return retHtml; }; module.exports = FilterXSS; },{"./default":1,"./parser":3,"./util":4,"cssfilter":8}],6:[function(require,module,exports){ /** * cssfilter * * @author è€é›·<leizongmin@gmail.com> */ var DEFAULT = require('./default'); var parseStyle = require('./parser'); var _ = require('./util'); /** * 返回值是å¦ä¸ºç©º * * @param {Object} obj * @return {Boolean} */ function isNull (obj) { return (obj === undefined || obj === null); } /** * æµ…æ‹·è´å¯¹è±¡ * * @param {Object} obj * @return {Object} */ function shallowCopyObject (obj) { var ret = {}; for (var i in obj) { ret[i] = obj[i]; } return ret; } /** * 创建CSS过滤器 * * @param {Object} options * - {Object} whiteList * - {Function} onAttr * - {Function} onIgnoreAttr * - {Function} safeAttrValue */ function FilterCSS (options) { options = shallowCopyObject(options || {}); options.whiteList = options.whiteList || DEFAULT.whiteList; options.onAttr = options.onAttr || DEFAULT.onAttr; options.onIgnoreAttr = options.onIgnoreAttr || DEFAULT.onIgnoreAttr; options.safeAttrValue = options.safeAttrValue || DEFAULT.safeAttrValue; this.options = options; } FilterCSS.prototype.process = function (css) { // 兼容å„ç§å¥‡è‘©è¾“å…¥ css = css || ''; css = css.toString(); if (!css) return ''; var me = this; var options = me.options; var whiteList = options.whiteList; var onAttr = options.onAttr; var onIgnoreAttr = options.onIgnoreAttr; var safeAttrValue = options.safeAttrValue; var retCSS = parseStyle(css, function (sourcePosition, position, name, value, source) { var check = whiteList[name]; var isWhite = false; if (check === true) isWhite = check; else if (typeof check === 'function') isWhite = check(value); else if (check instanceof RegExp) isWhite = check.test(value); if (isWhite !== true) isWhite = false; // å¦‚æžœè¿‡æ»¤åŽ value 为空则直接忽略 value = safeAttrValue(name, value); if (!value) return; var opts = { position: position, sourcePosition: sourcePosition, source: source, isWhite: isWhite }; if (isWhite) { var ret = onAttr(name, value, opts); if (isNull(ret)) { return name + ':' + value; } else { return ret; } } else { var ret = onIgnoreAttr(name, value, opts); if (!isNull(ret)) { return ret; } } }); return retCSS; }; module.exports = FilterCSS; },{"./default":7,"./parser":9,"./util":10}],7:[function(require,module,exports){ /** * cssfilter * * @author è€é›·<leizongmin@gmail.com> */ function getDefaultWhiteList () { // 白åå•值说明: // true: å…许该属性 // Function: function (val) { } 返回true表示å…许该属性,其他值å‡è¡¨ç¤ºä¸å…许 // RegExp: regexp.test(val) 返回true表示å…许该属性,其他值å‡è¡¨ç¤ºä¸å…许 // 除上é¢åˆ—出的值外å‡è¡¨ç¤ºä¸å…许 var whiteList = {}; whiteList['align-content'] = false; // default: auto whiteList['align-items'] = false; // default: auto whiteList['align-self'] = false; // default: auto whiteList['alignment-adjust'] = false; // default: auto whiteList['alignment-baseline'] = false; // default: baseline whiteList['all'] = false; // default: depending on individual properties whiteList['anchor-point'] = false; // default: none whiteList['animation'] = false; // default: depending on individual properties whiteList['animation-delay'] = false; // default: 0 whiteList['animation-direction'] = false; // default: normal whiteList['animation-duration'] = false; // default: 0 whiteList['animation-fill-mode'] = false; // default: none whiteList['animation-iteration-count'] = false; // default: 1 whiteList['animation-name'] = false; // default: none whiteList['animation-play-state'] = false; // default: running whiteList['animation-timing-function'] = false; // default: ease whiteList['azimuth'] = false; // default: center whiteList['backface-visibility'] = false; // default: visible whiteList['background'] = true; // default: depending on individual properties whiteList['background-attachment'] = true; // default: scroll whiteList['background-clip'] = true; // default: border-box whiteList['background-color'] = true; // default: transparent whiteList['background-image'] = true; // default: none whiteList['background-origin'] = true; // default: padding-box whiteList['background-position'] = true; // default: 0% 0% whiteList['background-repeat'] = true; // default: repeat whiteList['background-size'] = true; // default: auto whiteList['baseline-shift'] = false; // default: baseline whiteList['binding'] = false; // default: none whiteList['bleed'] = false; // default: 6pt whiteList['bookmark-label'] = false; // default: content() whiteList['bookmark-level'] = false; // default: none whiteList['bookmark-state'] = false; // default: open whiteList['border'] = true; // default: depending on individual properties whiteList['border-bottom'] = true; // default: depending on individual properties whiteList['border-bottom-color'] = true; // default: current color whiteList['border-bottom-left-radius'] = true; // default: 0 whiteList['border-bottom-right-radius'] = true; // default: 0 whiteList['border-bottom-style'] = true; // default: none whiteList['border-bottom-width'] = true; // default: medium whiteList['border-collapse'] = true; // default: separate whiteList['border-color'] = true; // default: depending on individual properties whiteList['border-image'] = true; // default: none whiteList['border-image-outset'] = true; // default: 0 whiteList['border-image-repeat'] = true; // default: stretch whiteList['border-image-slice'] = true; // default: 100% whiteList['border-image-source'] = true; // default: none whiteList['border-image-width'] = true; // default: 1 whiteList['border-left'] = true; // default: depending on individual properties whiteList['border-left-color'] = true; // default: current color whiteList['border-left-style'] = true; // default: none whiteList['border-left-width'] = true; // default: medium whiteList['border-radius'] = true; // default: 0 whiteList['border-right'] = true; // default: depending on individual properties whiteList['border-right-color'] = true; // default: current color whiteList['border-right-style'] = true; // default: none whiteList['border-right-width'] = true; // default: medium whiteList['border-spacing'] = true; // default: 0 whiteList['border-style'] = true; // default: depending on individual properties whiteList['border-top'] = true; // default: depending on individual properties whiteList['border-top-color'] = true; // default: current color whiteList['border-top-left-radius'] = true; // default: 0 whiteList['border-top-right-radius'] = true; // default: 0 whiteList['border-top-style'] = true; // default: none whiteList['border-top-width'] = true; // default: medium whiteList['border-width'] = true; // default: depending on individual properties whiteList['bottom'] = false; // default: auto whiteList['box-decoration-break'] = true; // default: slice whiteList['box-shadow'] = true; // default: none whiteList['box-sizing'] = true; // default: content-box whiteList['box-snap'] = true; // default: none whiteList['box-suppress'] = true; // default: show whiteList['break-after'] = true; // default: auto whiteList['break-before'] = true; // default: auto whiteList['break-inside'] = true; // default: auto whiteList['caption-side'] = false; // default: top whiteList['chains'] = false; // default: none whiteList['clear'] = true; // default: none whiteList['clip'] = false; // default: auto whiteList['clip-path'] = false; // default: none whiteList['clip-rule'] = false; // default: nonzero whiteList['color'] = true; // default: implementation dependent whiteList['color-interpolation-filters'] = true; // default: auto whiteList['column-count'] = false; // default: auto whiteList['column-fill'] = false; // default: balance whiteList['column-gap'] = false; // default: normal whiteList['column-rule'] = false; // default: depending on individual properties whiteList['column-rule-color'] = false; // default: current color whiteList['column-rule-style'] = false; // default: medium whiteList['column-rule-width'] = false; // default: medium whiteList['column-span'] = false; // default: none whiteList['column-width'] = false; // default: auto whiteList['columns'] = false; // default: depending on individual properties whiteList['contain'] = false; // default: none whiteList['content'] = false; // default: normal whiteList['counter-increment'] = false; // default: none whiteList['counter-reset'] = false; // default: none whiteList['counter-set'] = false; // default: none whiteList['crop'] = false; // default: auto whiteList['cue'] = false; // default: depending on individual properties whiteList['cue-after'] = false; // default: none whiteList['cue-before'] = false; // default: none whiteList['cursor'] = false; // default: auto whiteList['direction'] = false; // default: ltr whiteList['display'] = true; // default: depending on individual properties whiteList['display-inside'] = true; // default: auto whiteList['display-list'] = true; // default: none whiteList['display-outside'] = true; // default: inline-level whiteList['dominant-baseline'] = false; // default: auto whiteList['elevation'] = false; // default: level whiteList['empty-cells'] = false; // default: show whiteList['filter'] = false; // default: none whiteList['flex'] = false; // default: depending on individual properties whiteList['flex-basis'] = false; // default: auto whiteList['flex-direction'] = false; // default: row whiteList['flex-flow'] = false; // default: depending on individual properties whiteList['flex-grow'] = false; // default: 0 whiteList['flex-shrink'] = false; // default: 1 whiteList['flex-wrap'] = false; // default: nowrap whiteList['float'] = false; // default: none whiteList['float-offset'] = false; // default: 0 0 whiteList['flood-color'] = false; // default: black whiteList['flood-opacity'] = false; // default: 1 whiteList['flow-from'] = false; // default: none whiteList['flow-into'] = false; // default: none whiteList['font'] = true; // default: depending on individual properties whiteList['font-family'] = true; // default: implementation dependent whiteList['font-feature-settings'] = true; // default: normal whiteList['font-kerning'] = true; // default: auto whiteList['font-language-override'] = true; // default: normal whiteList['font-size'] = true; // default: medium whiteList['font-size-adjust'] = true; // default: none whiteList['font-stretch'] = true; // default: normal whiteList['font-style'] = true; // default: normal whiteList['font-synthesis'] = true; // default: weight style whiteList['font-variant'] = true; // default: normal whiteList['font-variant-alternates'] = true; // default: normal whiteList['font-variant-caps'] = true; // default: normal whiteList['font-variant-east-asian'] = true; // default: normal whiteList['font-variant-ligatures'] = true; // default: normal whiteList['font-variant-numeric'] = true; // default: normal whiteList['font-variant-position'] = true; // default: normal whiteList['font-weight'] = true; // default: normal whiteList['grid'] = false; // default: depending on individual properties whiteList['grid-area'] = false; // default: depending on individual properties whiteList['grid-auto-columns'] = false; // default: auto whiteList['grid-auto-flow'] = false; // default: none whiteList['grid-auto-rows'] = false; // default: auto whiteList['grid-column'] = false; // default: depending on individual properties whiteList['grid-column-end'] = false; // default: auto whiteList['grid-column-start'] = false; // default: auto whiteList['grid-row'] = false; // default: depending on individual properties whiteList['grid-row-end'] = false; // default: auto whiteList['grid-row-start'] = false; // default: auto whiteList['grid-template'] = false; // default: depending on individual properties whiteList['grid-template-areas'] = false; // default: none whiteList['grid-template-columns'] = false; // default: none whiteList['grid-template-rows'] = false; // default: none whiteList['hanging-punctuation'] = false; // default: none whiteList['height'] = true; // default: auto whiteList['hyphens'] = false; // default: manual whiteList['icon'] = false; // default: auto whiteList['image-orientation'] = false; // default: auto whiteList['image-resolution'] = false; // default: normal whiteList['ime-mode'] = false; // default: auto whiteList['initial-letters'] = false; // default: normal whiteList['inline-box-align'] = false; // default: last whiteList['justify-content'] = false; // default: auto whiteList['justify-items'] = false; // default: auto whiteList['justify-self'] = false; // default: auto whiteList['left'] = false; // default: auto whiteList['letter-spacing'] = true; // default: normal whiteList['lighting-color'] = true; // default: white whiteList['line-box-contain'] = false; // default: block inline replaced whiteList['line-break'] = false; // default: auto whiteList['line-grid'] = false; // default: match-parent whiteList['line-height'] = false; // default: normal whiteList['line-snap'] = false; // default: none whiteList['line-stacking'] = false; // default: depending on individual properties whiteList['line-stacking-ruby'] = false; // default: exclude-ruby whiteList['line-stacking-shift'] = false; // default: consider-shifts whiteList['line-stacking-strategy'] = false; // default: inline-line-height whiteList['list-style'] = true; // default: depending on individual properties whiteList['list-style-image'] = true; // default: none whiteList['list-style-position'] = true; // default: outside whiteList['list-style-type'] = true; // default: disc whiteList['margin'] = true; // default: depending on individual properties whiteList['margin-bottom'] = true; // default: 0 whiteList['margin-left'] = true; // default: 0 whiteList['margin-right'] = true; // default: 0 whiteList['margin-top'] = true; // default: 0 whiteList['marker-offset'] = false; // default: auto whiteList['marker-side'] = false; // default: list-item whiteList['marks'] = false; // default: none whiteList['mask'] = false; // default: border-box whiteList['mask-box'] = false; // default: see individual properties whiteList['mask-box-outset'] = false; // default: 0 whiteList['mask-box-repeat'] = false; // default: stretch whiteList['mask-box-slice'] = false; // default: 0 fill whiteList['mask-box-source'] = false; // default: none whiteList['mask-box-width'] = false; // default: auto whiteList['mask-clip'] = false; // default: border-box whiteList['mask-image'] = false; // default: none whiteList['mask-origin'] = false; // default: border-box whiteList['mask-position'] = false; // default: center whiteList['mask-repeat'] = false; // default: no-repeat whiteList['mask-size'] = false; // default: border-box whiteList['mask-source-type'] = false; // default: auto whiteList['mask-type'] = false; // default: luminance whiteList['max-height'] = true; // default: none whiteList['max-lines'] = false; // default: none whiteList['max-width'] = true; // default: none whiteList['min-height'] = true; // default: 0 whiteList['min-width'] = true; // default: 0 whiteList['move-to'] = false; // default: normal whiteList['nav-down'] = false; // default: auto whiteList['nav-index'] = false; // default: auto whiteList['nav-left'] = false; // default: auto whiteList['nav-right'] = false; // default: auto whiteList['nav-up'] = false; // default: auto whiteList['object-fit'] = false; // default: fill whiteList['object-position'] = false; // default: 50% 50% whiteList['opacity'] = false; // default: 1 whiteList['order'] = false; // default: 0 whiteList['orphans'] = false; // default: 2 whiteList['outline'] = false; // default: depending on individual properties whiteList['outline-color'] = false; // default: invert whiteList['outline-offset'] = false; // default: 0 whiteList['outline-style'] = false; // default: none whiteList['outline-width'] = false; // default: medium whiteList['overflow'] = false; // default: depending on individual properties whiteList['overflow-wrap'] = false; // default: normal whiteList['overflow-x'] = false; // default: visible whiteList['overflow-y'] = false; // default: visible whiteList['padding'] = true; // default: depending on individual properties whiteList['padding-bottom'] = true; // default: 0 whiteList['padding-left'] = true; // default: 0 whiteList['padding-right'] = true; // default: 0 whiteList['padding-top'] = true; // default: 0 whiteList['page'] = false; // default: auto whiteList['page-break-after'] = false; // default: auto whiteList['page-break-before'] = false; // default: auto whiteList['page-break-inside'] = false; // default: auto whiteList['page-policy'] = false; // default: start whiteList['pause'] = false; // default: implementation dependent whiteList['pause-after'] = false; // default: implementation dependent whiteList['pause-before'] = false; // default: implementation dependent whiteList['perspective'] = false; // default: none whiteList['perspective-origin'] = false; // default: 50% 50% whiteList['pitch'] = false; // default: medium whiteList['pitch-range'] = false; // default: 50 whiteList['play-during'] = false; // default: auto whiteList['position'] = false; // default: static whiteList['presentation-level'] = false; // default: 0 whiteList['quotes'] = false; // default: text whiteList['region-fragment'] = false; // default: auto whiteList['resize'] = false; // default: none whiteList['rest'] = false; // default: depending on individual properties whiteList['rest-after'] = false; // default: none whiteList['rest-before'] = false; // default: none whiteList['richness'] = false; // default: 50 whiteList['right'] = false; // default: auto whiteList['rotation'] = false; // default: 0 whiteList['rotation-point'] = false; // default: 50% 50% whiteList['ruby-align'] = false; // default: auto whiteList['ruby-merge'] = false; // default: separate whiteList['ruby-position'] = false; // default: before whiteList['shape-image-threshold'] = false; // default: 0.0 whiteList['shape-outside'] = false; // default: none whiteList['shape-margin'] = false; // default: 0 whiteList['size'] = false; // default: auto whiteList['speak'] = false; // default: auto whiteList['speak-as'] = false; // default: normal whiteList['speak-header'] = false; // default: once whiteList['speak-numeral'] = false; // default: continuous whiteList['speak-punctuation'] = false; // default: none whiteList['speech-rate'] = false; // default: medium whiteList['stress'] = false; // default: 50 whiteList['string-set'] = false; // default: none whiteList['tab-size'] = false; // default: 8 whiteList['table-layout'] = false; // default: auto whiteList['text-align'] = true; // default: start whiteList['text-align-last'] = true; // default: auto whiteList['text-combine-upright'] = true; // default: none whiteList['text-decoration'] = true; // default: none whiteList['text-decoration-color'] = true; // default: currentColor whiteList['text-decoration-line'] = true; // default: none whiteList['text-decoration-skip'] = true; // default: objects whiteList['text-decoration-style'] = true; // default: solid whiteList['text-emphasis'] = true; // default: depending on individual properties whiteList['text-emphasis-color'] = true; // default: currentColor whiteList['text-emphasis-position'] = true; // default: over right whiteList['text-emphasis-style'] = true; // default: none whiteList['text-height'] = true; // default: auto whiteList['text-indent'] = true; // default: 0 whiteList['text-justify'] = true; // default: auto whiteList['text-orientation'] = true; // default: mixed whiteList['text-overflow'] = true; // default: clip whiteList['text-shadow'] = true; // default: none whiteList['text-space-collapse'] = true; // default: collapse whiteList['text-transform'] = true; // default: none whiteList['text-underline-position'] = true; // default: auto whiteList['text-wrap'] = true; // default: normal whiteList['top'] = false; // default: auto whiteList['transform'] = false; // default: none whiteList['transform-origin'] = false; // default: 50% 50% 0 whiteList['transform-style'] = false; // default: flat whiteList['transition'] = false; // default: depending on individual properties whiteList['transition-delay'] = false; // default: 0s whiteList['transition-duration'] = false; // default: 0s whiteList['transition-property'] = false; // default: all whiteList['transition-timing-function'] = false; // default: ease whiteList['unicode-bidi'] = false; // default: normal whiteList['vertical-align'] = false; // default: baseline whiteList['visibility'] = false; // default: visible whiteList['voice-balance'] = false; // default: center whiteList['voice-duration'] = false; // default: auto whiteList['voice-family'] = false; // default: implementation dependent whiteList['voice-pitch'] = false; // default: medium whiteList['voice-range'] = false; // default: medium whiteList['voice-rate'] = false; // default: normal whiteList['voice-stress'] = false; // default: normal whiteList['voice-volume'] = false; // default: medium whiteList['volume'] = false; // default: medium whiteList['white-space'] = false; // default: normal whiteList['widows'] = false; // default: 2 whiteList['width'] = true; // default: auto whiteList['will-change'] = false; // default: auto whiteList['word-break'] = true; // default: normal whiteList['word-spacing'] = true; // default: normal whiteList['word-wrap'] = true; // default: normal whiteList['wrap-flow'] = false; // default: auto whiteList['wrap-through'] = false; // default: wrap whiteList['writing-mode'] = false; // default: horizontal-tb whiteList['z-index'] = false; // default: auto return whiteList; } /** * 匹é…到白åå•上的一个属性时 * * @param {String} name * @param {String} value * @param {Object} options * @return {String} */ function onAttr (name, value, options) { // do nothing } /** * 匹é…到ä¸åœ¨ç™½åå•上的一个属性时 * * @param {String} name * @param {String} value * @param {Object} options * @return {String} */ function onIgnoreAttr (name, value, options) { // do nothing } var REGEXP_URL_JAVASCRIPT = /javascript\s*\:/img; /** * 过滤属性值 * * @param {String} name * @param {String} value * @return {String} */ function safeAttrValue(name, value) { if (REGEXP_URL_JAVASCRIPT.test(value)) return ''; return value; } exports.whiteList = getDefaultWhiteList(); exports.getDefaultWhiteList = getDefaultWhiteList; exports.onAttr = onAttr; exports.onIgnoreAttr = onIgnoreAttr; exports.safeAttrValue = safeAttrValue; },{}],8:[function(require,module,exports){ /** * cssfilter * * @author è€é›·<leizongmin@gmail.com> */ var DEFAULT = require('./default'); var FilterCSS = require('./css'); /** * XSS过滤 * * @param {String} css è¦è¿‡æ»¤çš„CSS代ç * @param {Object} options 选项:whiteList, onAttr, onIgnoreAttr * @return {String} */ function filterCSS (html, options) { var xss = new FilterCSS(options); return xss.process(html); } // 输出 exports = module.exports = filterCSS; exports.FilterCSS = FilterCSS; for (var i in DEFAULT) exports[i] = DEFAULT[i]; // 在æµè§ˆå™¨ç«¯ä½¿ç”¨ if (typeof window !== 'undefined') { window.filterCSS = module.exports; } },{"./css":6,"./default":7}],9:[function(require,module,exports){ /** * cssfilter * * @author è€é›·<leizongmin@gmail.com> */ var _ = require('./util'); /** * è§£æžstyle * * @param {String} css * @param {Function} onAttr 处ç†å±žæ€§çš„函数 * 傿•°æ ¼å¼ï¼š function (sourcePosition, position, name, value, source) * @return {String} */ function parseStyle (css, onAttr) { css = _.trimRight(css); if (css[css.length - 1] !== ';') css += ';'; var cssLength = css.length; var isParenthesisOpen = false; var lastPos = 0; var i = 0; var retCSS = ''; function addNewAttr () { // 如果没有æ£å¸¸çš„é—åˆåœ†æ‹¬å·ï¼Œåˆ™ç›´æŽ¥å¿½ç•¥å½“å‰å±žæ€§ if (!isParenthesisOpen) { var source = _.trim(css.slice(lastPos, i)); var j = source.indexOf(':'); if (j !== -1) { var name = _.trim(source.slice(0, j)); var value = _.trim(source.slice(j + 1)); // 必须有属性åç§° if (name) { var ret = onAttr(lastPos, retCSS.length, name, value, source); if (ret) retCSS += ret + '; '; } } } lastPos = i + 1; } for (; i < cssLength; i++) { var c = css[i]; if (c === '/' && css[i + 1] === '*') { // 备注开始 var j = css.indexOf('*/', i + 2); // 如果没有æ£å¸¸çš„备注结æŸï¼Œåˆ™åŽé¢çš„部分全部跳过 if (j === -1) break; // 直接将当å‰ä½ç½®è°ƒåˆ°å¤‡æ³¨ç»“尾,并且åˆå§‹åŒ–çŠ¶æ€ i = j + 1; lastPos = i + 1; isParenthesisOpen = false; } else if (c === '(') { isParenthesisOpen = true; } else if (c === ')') { isParenthesisOpen = false; } else if (c === ';') { if (isParenthesisOpen) { // 在圆括å·é‡Œé¢ï¼Œå¿½ç•¥ } else { addNewAttr(); } } else if (c === '\n') { addNewAttr(); } } return _.trim(retCSS); } module.exports = parseStyle; },{"./util":10}],10:[function(require,module,exports){ module.exports = { indexOf: function (arr, item) { var i, j; if (Array.prototype.indexOf) { return arr.indexOf(item); } for (i = 0, j = arr.length; i < j; i++) { if (arr[i] === item) { return i; } } return -1; }, forEach: function (arr, fn, scope) { var i, j; if (Array.prototype.forEach) { return arr.forEach(fn, scope); } for (i = 0, j = arr.length; i < j; i++) { fn.call(scope, arr[i], i, arr); } }, trim: function (str) { if (String.prototype.trim) { return str.trim(); } return str.replace(/(^\s*)|(\s*$)/g, ''); }, trimRight: function (str) { if (String.prototype.trimRight) { return str.trimRight(); } return str.replace(/(\s*$)/g, ''); } }; },{}]},{},[2]); apollo-server-demo/node_modules/xss/dist/xss.min.js 0000644 0001750 0000144 00000070751 03560116604 022157 0 ustar andreh users (function(){function r(e,n,t){function o(i,f){if(!n[i]){if(!e[i]){var c="function"==typeof require&&require;if(!f&&c)return c(i,!0);if(u)return u(i,!0);var a=new Error("Cannot find module '"+i+"'");throw a.code="MODULE_NOT_FOUND",a}var p=n[i]={exports:{}};e[i][0].call(p.exports,function(r){var n=e[i][1][r];return o(n||r)},p,p.exports,r,e,n,t)}return n[i].exports}for(var u="function"==typeof require&&require,i=0;i<t.length;i++)o(t[i]);return o}return r})()({1:[function(require,module,exports){var FilterCSS=require("cssfilter").FilterCSS;var getDefaultCSSWhiteList=require("cssfilter").getDefaultWhiteList;var _=require("./util");function getDefaultWhiteList(){return{a:["target","href","title"],abbr:["title"],address:[],area:["shape","coords","href","alt"],article:[],aside:[],audio:["autoplay","controls","loop","preload","src"],b:[],bdi:["dir"],bdo:["dir"],big:[],blockquote:["cite"],br:[],caption:[],center:[],cite:[],code:[],col:["align","valign","span","width"],colgroup:["align","valign","span","width"],dd:[],del:["datetime"],details:["open"],div:[],dl:[],dt:[],em:[],font:["color","size","face"],footer:[],h1:[],h2:[],h3:[],h4:[],h5:[],h6:[],header:[],hr:[],i:[],img:["src","alt","title","width","height"],ins:["datetime"],li:[],mark:[],nav:[],ol:[],p:[],pre:[],s:[],section:[],small:[],span:[],sub:[],sup:[],strong:[],table:["width","border","align","valign"],tbody:["align","valign"],td:["width","rowspan","colspan","align","valign"],tfoot:["align","valign"],th:["width","rowspan","colspan","align","valign"],thead:["align","valign"],tr:["rowspan","align","valign"],tt:[],u:[],ul:[],video:["autoplay","controls","loop","preload","src","height","width"]}}var defaultCSSFilter=new FilterCSS;function onTag(tag,html,options){}function onIgnoreTag(tag,html,options){}function onTagAttr(tag,name,value){}function onIgnoreTagAttr(tag,name,value){}function escapeHtml(html){return html.replace(REGEXP_LT,"<").replace(REGEXP_GT,">")}function safeAttrValue(tag,name,value,cssFilter){value=friendlyAttrValue(value);if(name==="href"||name==="src"){value=_.trim(value);if(value==="#")return"#";if(!(value.substr(0,7)==="http://"||value.substr(0,8)==="https://"||value.substr(0,7)==="mailto:"||value.substr(0,4)==="tel:"||value.substr(0,11)==="data:image/"||value.substr(0,6)==="ftp://"||value.substr(0,2)==="./"||value.substr(0,3)==="../"||value[0]==="#"||value[0]==="/")){return""}}else if(name==="background"){REGEXP_DEFAULT_ON_TAG_ATTR_4.lastIndex=0;if(REGEXP_DEFAULT_ON_TAG_ATTR_4.test(value)){return""}}else if(name==="style"){REGEXP_DEFAULT_ON_TAG_ATTR_7.lastIndex=0;if(REGEXP_DEFAULT_ON_TAG_ATTR_7.test(value)){return""}REGEXP_DEFAULT_ON_TAG_ATTR_8.lastIndex=0;if(REGEXP_DEFAULT_ON_TAG_ATTR_8.test(value)){REGEXP_DEFAULT_ON_TAG_ATTR_4.lastIndex=0;if(REGEXP_DEFAULT_ON_TAG_ATTR_4.test(value)){return""}}if(cssFilter!==false){cssFilter=cssFilter||defaultCSSFilter;value=cssFilter.process(value)}}value=escapeAttrValue(value);return value}var REGEXP_LT=/</g;var REGEXP_GT=/>/g;var REGEXP_QUOTE=/"/g;var REGEXP_QUOTE_2=/"/g;var REGEXP_ATTR_VALUE_1=/&#([a-zA-Z0-9]*);?/gim;var REGEXP_ATTR_VALUE_COLON=/:?/gim;var REGEXP_ATTR_VALUE_NEWLINE=/&newline;?/gim;var REGEXP_DEFAULT_ON_TAG_ATTR_3=/\/\*|\*\//gm;var REGEXP_DEFAULT_ON_TAG_ATTR_4=/((j\s*a\s*v\s*a|v\s*b|l\s*i\s*v\s*e)\s*s\s*c\s*r\s*i\s*p\s*t\s*|m\s*o\s*c\s*h\s*a)\:/gi;var REGEXP_DEFAULT_ON_TAG_ATTR_5=/^[\s"'`]*(d\s*a\s*t\s*a\s*)\:/gi;var REGEXP_DEFAULT_ON_TAG_ATTR_6=/^[\s"'`]*(d\s*a\s*t\s*a\s*)\:\s*image\//gi;var REGEXP_DEFAULT_ON_TAG_ATTR_7=/e\s*x\s*p\s*r\s*e\s*s\s*s\s*i\s*o\s*n\s*\(.*/gi;var REGEXP_DEFAULT_ON_TAG_ATTR_8=/u\s*r\s*l\s*\(.*/gi;function escapeQuote(str){return str.replace(REGEXP_QUOTE,""")}function unescapeQuote(str){return str.replace(REGEXP_QUOTE_2,'"')}function escapeHtmlEntities(str){return str.replace(REGEXP_ATTR_VALUE_1,function replaceUnicode(str,code){return code[0]==="x"||code[0]==="X"?String.fromCharCode(parseInt(code.substr(1),16)):String.fromCharCode(parseInt(code,10))})}function escapeDangerHtml5Entities(str){return str.replace(REGEXP_ATTR_VALUE_COLON,":").replace(REGEXP_ATTR_VALUE_NEWLINE," ")}function clearNonPrintableCharacter(str){var str2="";for(var i=0,len=str.length;i<len;i++){str2+=str.charCodeAt(i)<32?" ":str.charAt(i)}return _.trim(str2)}function friendlyAttrValue(str){str=unescapeQuote(str);str=escapeHtmlEntities(str);str=escapeDangerHtml5Entities(str);str=clearNonPrintableCharacter(str);return str}function escapeAttrValue(str){str=escapeQuote(str);str=escapeHtml(str);return str}function onIgnoreTagStripAll(){return""}function StripTagBody(tags,next){if(typeof next!=="function"){next=function(){}}var isRemoveAllTag=!Array.isArray(tags);function isRemoveTag(tag){if(isRemoveAllTag)return true;return _.indexOf(tags,tag)!==-1}var removeList=[];var posStart=false;return{onIgnoreTag:function(tag,html,options){if(isRemoveTag(tag)){if(options.isClosing){var ret="[/removed]";var end=options.position+ret.length;removeList.push([posStart!==false?posStart:options.position,end]);posStart=false;return ret}else{if(!posStart){posStart=options.position}return"[removed]"}}else{return next(tag,html,options)}},remove:function(html){var rethtml="";var lastPos=0;_.forEach(removeList,function(pos){rethtml+=html.slice(lastPos,pos[0]);lastPos=pos[1]});rethtml+=html.slice(lastPos);return rethtml}}}function stripCommentTag(html){return html.replace(STRIP_COMMENT_TAG_REGEXP,"")}var STRIP_COMMENT_TAG_REGEXP=/<!--[\s\S]*?-->/g;function stripBlankChar(html){var chars=html.split("");chars=chars.filter(function(char){var c=char.charCodeAt(0);if(c===127)return false;if(c<=31){if(c===10||c===13)return true;return false}return true});return chars.join("")}exports.whiteList=getDefaultWhiteList();exports.getDefaultWhiteList=getDefaultWhiteList;exports.onTag=onTag;exports.onIgnoreTag=onIgnoreTag;exports.onTagAttr=onTagAttr;exports.onIgnoreTagAttr=onIgnoreTagAttr;exports.safeAttrValue=safeAttrValue;exports.escapeHtml=escapeHtml;exports.escapeQuote=escapeQuote;exports.unescapeQuote=unescapeQuote;exports.escapeHtmlEntities=escapeHtmlEntities;exports.escapeDangerHtml5Entities=escapeDangerHtml5Entities;exports.clearNonPrintableCharacter=clearNonPrintableCharacter;exports.friendlyAttrValue=friendlyAttrValue;exports.escapeAttrValue=escapeAttrValue;exports.onIgnoreTagStripAll=onIgnoreTagStripAll;exports.StripTagBody=StripTagBody;exports.stripCommentTag=stripCommentTag;exports.stripBlankChar=stripBlankChar;exports.cssFilter=defaultCSSFilter;exports.getDefaultCSSWhiteList=getDefaultCSSWhiteList},{"./util":4,cssfilter:8}],2:[function(require,module,exports){var DEFAULT=require("./default");var parser=require("./parser");var FilterXSS=require("./xss");function filterXSS(html,options){var xss=new FilterXSS(options);return xss.process(html)}exports=module.exports=filterXSS;exports.filterXSS=filterXSS;exports.FilterXSS=FilterXSS;for(var i in DEFAULT)exports[i]=DEFAULT[i];for(var i in parser)exports[i]=parser[i];if(typeof window!=="undefined"){window.filterXSS=module.exports}function isWorkerEnv(){return typeof self!=="undefined"&&typeof DedicatedWorkerGlobalScope!=="undefined"&&self instanceof DedicatedWorkerGlobalScope}if(isWorkerEnv()){self.filterXSS=module.exports}},{"./default":1,"./parser":3,"./xss":5}],3:[function(require,module,exports){var _=require("./util");function getTagName(html){var i=_.spaceIndex(html);if(i===-1){var tagName=html.slice(1,-1)}else{var tagName=html.slice(1,i+1)}tagName=_.trim(tagName).toLowerCase();if(tagName.slice(0,1)==="/")tagName=tagName.slice(1);if(tagName.slice(-1)==="/")tagName=tagName.slice(0,-1);return tagName}function isClosing(html){return html.slice(0,2)==="</"}function parseTag(html,onTag,escapeHtml){"use strict";var rethtml="";var lastPos=0;var tagStart=false;var quoteStart=false;var currentPos=0;var len=html.length;var currentTagName="";var currentHtml="";chariterator:for(currentPos=0;currentPos<len;currentPos++){var c=html.charAt(currentPos);if(tagStart===false){if(c==="<"){tagStart=currentPos;continue}}else{if(quoteStart===false){if(c==="<"){rethtml+=escapeHtml(html.slice(lastPos,currentPos));tagStart=currentPos;lastPos=currentPos;continue}if(c===">"){rethtml+=escapeHtml(html.slice(lastPos,tagStart));currentHtml=html.slice(tagStart,currentPos+1);currentTagName=getTagName(currentHtml);rethtml+=onTag(tagStart,rethtml.length,currentTagName,currentHtml,isClosing(currentHtml));lastPos=currentPos+1;tagStart=false;continue}if(c==='"'||c==="'"){var i=1;var ic=html.charAt(currentPos-i);while(ic===" "||ic==="="){if(ic==="="){quoteStart=c;continue chariterator}ic=html.charAt(currentPos-++i)}}}else{if(c===quoteStart){quoteStart=false;continue}}}}if(lastPos<html.length){rethtml+=escapeHtml(html.substr(lastPos))}return rethtml}var REGEXP_ILLEGAL_ATTR_NAME=/[^a-zA-Z0-9_:\.\-]/gim;function parseAttr(html,onAttr){"use strict";var lastPos=0;var retAttrs=[];var tmpName=false;var len=html.length;function addAttr(name,value){name=_.trim(name);name=name.replace(REGEXP_ILLEGAL_ATTR_NAME,"").toLowerCase();if(name.length<1)return;var ret=onAttr(name,value||"");if(ret)retAttrs.push(ret)}for(var i=0;i<len;i++){var c=html.charAt(i);var v,j;if(tmpName===false&&c==="="){tmpName=html.slice(lastPos,i);lastPos=i+1;continue}if(tmpName!==false){if(i===lastPos&&(c==='"'||c==="'")&&html.charAt(i-1)==="="){j=html.indexOf(c,i+1);if(j===-1){break}else{v=_.trim(html.slice(lastPos+1,j));addAttr(tmpName,v);tmpName=false;i=j;lastPos=i+1;continue}}}if(/\s|\n|\t/.test(c)){html=html.replace(/\s|\n|\t/g," ");if(tmpName===false){j=findNextEqual(html,i);if(j===-1){v=_.trim(html.slice(lastPos,i));addAttr(v);tmpName=false;lastPos=i+1;continue}else{i=j-1;continue}}else{j=findBeforeEqual(html,i-1);if(j===-1){v=_.trim(html.slice(lastPos,i));v=stripQuoteWrap(v);addAttr(tmpName,v);tmpName=false;lastPos=i+1;continue}else{continue}}}}if(lastPos<html.length){if(tmpName===false){addAttr(html.slice(lastPos))}else{addAttr(tmpName,stripQuoteWrap(_.trim(html.slice(lastPos))))}}return _.trim(retAttrs.join(" "))}function findNextEqual(str,i){for(;i<str.length;i++){var c=str[i];if(c===" ")continue;if(c==="=")return i;return-1}}function findBeforeEqual(str,i){for(;i>0;i--){var c=str[i];if(c===" ")continue;if(c==="=")return i;return-1}}function isQuoteWrapString(text){if(text[0]==='"'&&text[text.length-1]==='"'||text[0]==="'"&&text[text.length-1]==="'"){return true}else{return false}}function stripQuoteWrap(text){if(isQuoteWrapString(text)){return text.substr(1,text.length-2)}else{return text}}exports.parseTag=parseTag;exports.parseAttr=parseAttr},{"./util":4}],4:[function(require,module,exports){module.exports={indexOf:function(arr,item){var i,j;if(Array.prototype.indexOf){return arr.indexOf(item)}for(i=0,j=arr.length;i<j;i++){if(arr[i]===item){return i}}return-1},forEach:function(arr,fn,scope){var i,j;if(Array.prototype.forEach){return arr.forEach(fn,scope)}for(i=0,j=arr.length;i<j;i++){fn.call(scope,arr[i],i,arr)}},trim:function(str){if(String.prototype.trim){return str.trim()}return str.replace(/(^\s*)|(\s*$)/g,"")},spaceIndex:function(str){var reg=/\s|\n|\t/;var match=reg.exec(str);return match?match.index:-1}}},{}],5:[function(require,module,exports){var FilterCSS=require("cssfilter").FilterCSS;var DEFAULT=require("./default");var parser=require("./parser");var parseTag=parser.parseTag;var parseAttr=parser.parseAttr;var _=require("./util");function isNull(obj){return obj===undefined||obj===null}function getAttrs(html){var i=_.spaceIndex(html);if(i===-1){return{html:"",closing:html[html.length-2]==="/"}}html=_.trim(html.slice(i+1,-1));var isClosing=html[html.length-1]==="/";if(isClosing)html=_.trim(html.slice(0,-1));return{html:html,closing:isClosing}}function shallowCopyObject(obj){var ret={};for(var i in obj){ret[i]=obj[i]}return ret}function FilterXSS(options){options=shallowCopyObject(options||{});if(options.stripIgnoreTag){if(options.onIgnoreTag){console.error('Notes: cannot use these two options "stripIgnoreTag" and "onIgnoreTag" at the same time')}options.onIgnoreTag=DEFAULT.onIgnoreTagStripAll}options.whiteList=options.whiteList||DEFAULT.whiteList;options.onTag=options.onTag||DEFAULT.onTag;options.onTagAttr=options.onTagAttr||DEFAULT.onTagAttr;options.onIgnoreTag=options.onIgnoreTag||DEFAULT.onIgnoreTag;options.onIgnoreTagAttr=options.onIgnoreTagAttr||DEFAULT.onIgnoreTagAttr;options.safeAttrValue=options.safeAttrValue||DEFAULT.safeAttrValue;options.escapeHtml=options.escapeHtml||DEFAULT.escapeHtml;this.options=options;if(options.css===false){this.cssFilter=false}else{options.css=options.css||{};this.cssFilter=new FilterCSS(options.css)}}FilterXSS.prototype.process=function(html){html=html||"";html=html.toString();if(!html)return"";var me=this;var options=me.options;var whiteList=options.whiteList;var onTag=options.onTag;var onIgnoreTag=options.onIgnoreTag;var onTagAttr=options.onTagAttr;var onIgnoreTagAttr=options.onIgnoreTagAttr;var safeAttrValue=options.safeAttrValue;var escapeHtml=options.escapeHtml;var cssFilter=me.cssFilter;if(options.stripBlankChar){html=DEFAULT.stripBlankChar(html)}if(!options.allowCommentTag){html=DEFAULT.stripCommentTag(html)}var stripIgnoreTagBody=false;if(options.stripIgnoreTagBody){var stripIgnoreTagBody=DEFAULT.StripTagBody(options.stripIgnoreTagBody,onIgnoreTag);onIgnoreTag=stripIgnoreTagBody.onIgnoreTag}var retHtml=parseTag(html,function(sourcePosition,position,tag,html,isClosing){var info={sourcePosition:sourcePosition,position:position,isClosing:isClosing,isWhite:whiteList.hasOwnProperty(tag)};var ret=onTag(tag,html,info);if(!isNull(ret))return ret;if(info.isWhite){if(info.isClosing){return"</"+tag+">"}var attrs=getAttrs(html);var whiteAttrList=whiteList[tag];var attrsHtml=parseAttr(attrs.html,function(name,value){var isWhiteAttr=_.indexOf(whiteAttrList,name)!==-1;var ret=onTagAttr(tag,name,value,isWhiteAttr);if(!isNull(ret))return ret;if(isWhiteAttr){value=safeAttrValue(tag,name,value,cssFilter);if(value){return name+'="'+value+'"'}else{return name}}else{var ret=onIgnoreTagAttr(tag,name,value,isWhiteAttr);if(!isNull(ret))return ret;return}});var html="<"+tag;if(attrsHtml)html+=" "+attrsHtml;if(attrs.closing)html+=" /";html+=">";return html}else{var ret=onIgnoreTag(tag,html,info);if(!isNull(ret))return ret;return escapeHtml(html)}},escapeHtml);if(stripIgnoreTagBody){retHtml=stripIgnoreTagBody.remove(retHtml)}return retHtml};module.exports=FilterXSS},{"./default":1,"./parser":3,"./util":4,cssfilter:8}],6:[function(require,module,exports){var DEFAULT=require("./default");var parseStyle=require("./parser");var _=require("./util");function isNull(obj){return obj===undefined||obj===null}function shallowCopyObject(obj){var ret={};for(var i in obj){ret[i]=obj[i]}return ret}function FilterCSS(options){options=shallowCopyObject(options||{});options.whiteList=options.whiteList||DEFAULT.whiteList;options.onAttr=options.onAttr||DEFAULT.onAttr;options.onIgnoreAttr=options.onIgnoreAttr||DEFAULT.onIgnoreAttr;options.safeAttrValue=options.safeAttrValue||DEFAULT.safeAttrValue;this.options=options}FilterCSS.prototype.process=function(css){css=css||"";css=css.toString();if(!css)return"";var me=this;var options=me.options;var whiteList=options.whiteList;var onAttr=options.onAttr;var onIgnoreAttr=options.onIgnoreAttr;var safeAttrValue=options.safeAttrValue;var retCSS=parseStyle(css,function(sourcePosition,position,name,value,source){var check=whiteList[name];var isWhite=false;if(check===true)isWhite=check;else if(typeof check==="function")isWhite=check(value);else if(check instanceof RegExp)isWhite=check.test(value);if(isWhite!==true)isWhite=false;value=safeAttrValue(name,value);if(!value)return;var opts={position:position,sourcePosition:sourcePosition,source:source,isWhite:isWhite};if(isWhite){var ret=onAttr(name,value,opts);if(isNull(ret)){return name+":"+value}else{return ret}}else{var ret=onIgnoreAttr(name,value,opts);if(!isNull(ret)){return ret}}});return retCSS};module.exports=FilterCSS},{"./default":7,"./parser":9,"./util":10}],7:[function(require,module,exports){function getDefaultWhiteList(){var whiteList={};whiteList["align-content"]=false;whiteList["align-items"]=false;whiteList["align-self"]=false;whiteList["alignment-adjust"]=false;whiteList["alignment-baseline"]=false;whiteList["all"]=false;whiteList["anchor-point"]=false;whiteList["animation"]=false;whiteList["animation-delay"]=false;whiteList["animation-direction"]=false;whiteList["animation-duration"]=false;whiteList["animation-fill-mode"]=false;whiteList["animation-iteration-count"]=false;whiteList["animation-name"]=false;whiteList["animation-play-state"]=false;whiteList["animation-timing-function"]=false;whiteList["azimuth"]=false;whiteList["backface-visibility"]=false;whiteList["background"]=true;whiteList["background-attachment"]=true;whiteList["background-clip"]=true;whiteList["background-color"]=true;whiteList["background-image"]=true;whiteList["background-origin"]=true;whiteList["background-position"]=true;whiteList["background-repeat"]=true;whiteList["background-size"]=true;whiteList["baseline-shift"]=false;whiteList["binding"]=false;whiteList["bleed"]=false;whiteList["bookmark-label"]=false;whiteList["bookmark-level"]=false;whiteList["bookmark-state"]=false;whiteList["border"]=true;whiteList["border-bottom"]=true;whiteList["border-bottom-color"]=true;whiteList["border-bottom-left-radius"]=true;whiteList["border-bottom-right-radius"]=true;whiteList["border-bottom-style"]=true;whiteList["border-bottom-width"]=true;whiteList["border-collapse"]=true;whiteList["border-color"]=true;whiteList["border-image"]=true;whiteList["border-image-outset"]=true;whiteList["border-image-repeat"]=true;whiteList["border-image-slice"]=true;whiteList["border-image-source"]=true;whiteList["border-image-width"]=true;whiteList["border-left"]=true;whiteList["border-left-color"]=true;whiteList["border-left-style"]=true;whiteList["border-left-width"]=true;whiteList["border-radius"]=true;whiteList["border-right"]=true;whiteList["border-right-color"]=true;whiteList["border-right-style"]=true;whiteList["border-right-width"]=true;whiteList["border-spacing"]=true;whiteList["border-style"]=true;whiteList["border-top"]=true;whiteList["border-top-color"]=true;whiteList["border-top-left-radius"]=true;whiteList["border-top-right-radius"]=true;whiteList["border-top-style"]=true;whiteList["border-top-width"]=true;whiteList["border-width"]=true;whiteList["bottom"]=false;whiteList["box-decoration-break"]=true;whiteList["box-shadow"]=true;whiteList["box-sizing"]=true;whiteList["box-snap"]=true;whiteList["box-suppress"]=true;whiteList["break-after"]=true;whiteList["break-before"]=true;whiteList["break-inside"]=true;whiteList["caption-side"]=false;whiteList["chains"]=false;whiteList["clear"]=true;whiteList["clip"]=false;whiteList["clip-path"]=false;whiteList["clip-rule"]=false;whiteList["color"]=true;whiteList["color-interpolation-filters"]=true;whiteList["column-count"]=false;whiteList["column-fill"]=false;whiteList["column-gap"]=false;whiteList["column-rule"]=false;whiteList["column-rule-color"]=false;whiteList["column-rule-style"]=false;whiteList["column-rule-width"]=false;whiteList["column-span"]=false;whiteList["column-width"]=false;whiteList["columns"]=false;whiteList["contain"]=false;whiteList["content"]=false;whiteList["counter-increment"]=false;whiteList["counter-reset"]=false;whiteList["counter-set"]=false;whiteList["crop"]=false;whiteList["cue"]=false;whiteList["cue-after"]=false;whiteList["cue-before"]=false;whiteList["cursor"]=false;whiteList["direction"]=false;whiteList["display"]=true;whiteList["display-inside"]=true;whiteList["display-list"]=true;whiteList["display-outside"]=true;whiteList["dominant-baseline"]=false;whiteList["elevation"]=false;whiteList["empty-cells"]=false;whiteList["filter"]=false;whiteList["flex"]=false;whiteList["flex-basis"]=false;whiteList["flex-direction"]=false;whiteList["flex-flow"]=false;whiteList["flex-grow"]=false;whiteList["flex-shrink"]=false;whiteList["flex-wrap"]=false;whiteList["float"]=false;whiteList["float-offset"]=false;whiteList["flood-color"]=false;whiteList["flood-opacity"]=false;whiteList["flow-from"]=false;whiteList["flow-into"]=false;whiteList["font"]=true;whiteList["font-family"]=true;whiteList["font-feature-settings"]=true;whiteList["font-kerning"]=true;whiteList["font-language-override"]=true;whiteList["font-size"]=true;whiteList["font-size-adjust"]=true;whiteList["font-stretch"]=true;whiteList["font-style"]=true;whiteList["font-synthesis"]=true;whiteList["font-variant"]=true;whiteList["font-variant-alternates"]=true;whiteList["font-variant-caps"]=true;whiteList["font-variant-east-asian"]=true;whiteList["font-variant-ligatures"]=true;whiteList["font-variant-numeric"]=true;whiteList["font-variant-position"]=true;whiteList["font-weight"]=true;whiteList["grid"]=false;whiteList["grid-area"]=false;whiteList["grid-auto-columns"]=false;whiteList["grid-auto-flow"]=false;whiteList["grid-auto-rows"]=false;whiteList["grid-column"]=false;whiteList["grid-column-end"]=false;whiteList["grid-column-start"]=false;whiteList["grid-row"]=false;whiteList["grid-row-end"]=false;whiteList["grid-row-start"]=false;whiteList["grid-template"]=false;whiteList["grid-template-areas"]=false;whiteList["grid-template-columns"]=false;whiteList["grid-template-rows"]=false;whiteList["hanging-punctuation"]=false;whiteList["height"]=true;whiteList["hyphens"]=false;whiteList["icon"]=false;whiteList["image-orientation"]=false;whiteList["image-resolution"]=false;whiteList["ime-mode"]=false;whiteList["initial-letters"]=false;whiteList["inline-box-align"]=false;whiteList["justify-content"]=false;whiteList["justify-items"]=false;whiteList["justify-self"]=false;whiteList["left"]=false;whiteList["letter-spacing"]=true;whiteList["lighting-color"]=true;whiteList["line-box-contain"]=false;whiteList["line-break"]=false;whiteList["line-grid"]=false;whiteList["line-height"]=false;whiteList["line-snap"]=false;whiteList["line-stacking"]=false;whiteList["line-stacking-ruby"]=false;whiteList["line-stacking-shift"]=false;whiteList["line-stacking-strategy"]=false;whiteList["list-style"]=true;whiteList["list-style-image"]=true;whiteList["list-style-position"]=true;whiteList["list-style-type"]=true;whiteList["margin"]=true;whiteList["margin-bottom"]=true;whiteList["margin-left"]=true;whiteList["margin-right"]=true;whiteList["margin-top"]=true;whiteList["marker-offset"]=false;whiteList["marker-side"]=false;whiteList["marks"]=false;whiteList["mask"]=false;whiteList["mask-box"]=false;whiteList["mask-box-outset"]=false;whiteList["mask-box-repeat"]=false;whiteList["mask-box-slice"]=false;whiteList["mask-box-source"]=false;whiteList["mask-box-width"]=false;whiteList["mask-clip"]=false;whiteList["mask-image"]=false;whiteList["mask-origin"]=false;whiteList["mask-position"]=false;whiteList["mask-repeat"]=false;whiteList["mask-size"]=false;whiteList["mask-source-type"]=false;whiteList["mask-type"]=false;whiteList["max-height"]=true;whiteList["max-lines"]=false;whiteList["max-width"]=true;whiteList["min-height"]=true;whiteList["min-width"]=true;whiteList["move-to"]=false;whiteList["nav-down"]=false;whiteList["nav-index"]=false;whiteList["nav-left"]=false;whiteList["nav-right"]=false;whiteList["nav-up"]=false;whiteList["object-fit"]=false;whiteList["object-position"]=false;whiteList["opacity"]=false;whiteList["order"]=false;whiteList["orphans"]=false;whiteList["outline"]=false;whiteList["outline-color"]=false;whiteList["outline-offset"]=false;whiteList["outline-style"]=false;whiteList["outline-width"]=false;whiteList["overflow"]=false;whiteList["overflow-wrap"]=false;whiteList["overflow-x"]=false;whiteList["overflow-y"]=false;whiteList["padding"]=true;whiteList["padding-bottom"]=true;whiteList["padding-left"]=true;whiteList["padding-right"]=true;whiteList["padding-top"]=true;whiteList["page"]=false;whiteList["page-break-after"]=false;whiteList["page-break-before"]=false;whiteList["page-break-inside"]=false;whiteList["page-policy"]=false;whiteList["pause"]=false;whiteList["pause-after"]=false;whiteList["pause-before"]=false;whiteList["perspective"]=false;whiteList["perspective-origin"]=false;whiteList["pitch"]=false;whiteList["pitch-range"]=false;whiteList["play-during"]=false;whiteList["position"]=false;whiteList["presentation-level"]=false;whiteList["quotes"]=false;whiteList["region-fragment"]=false;whiteList["resize"]=false;whiteList["rest"]=false;whiteList["rest-after"]=false;whiteList["rest-before"]=false;whiteList["richness"]=false;whiteList["right"]=false;whiteList["rotation"]=false;whiteList["rotation-point"]=false;whiteList["ruby-align"]=false;whiteList["ruby-merge"]=false;whiteList["ruby-position"]=false;whiteList["shape-image-threshold"]=false;whiteList["shape-outside"]=false;whiteList["shape-margin"]=false;whiteList["size"]=false;whiteList["speak"]=false;whiteList["speak-as"]=false;whiteList["speak-header"]=false;whiteList["speak-numeral"]=false;whiteList["speak-punctuation"]=false;whiteList["speech-rate"]=false;whiteList["stress"]=false;whiteList["string-set"]=false;whiteList["tab-size"]=false;whiteList["table-layout"]=false;whiteList["text-align"]=true;whiteList["text-align-last"]=true;whiteList["text-combine-upright"]=true;whiteList["text-decoration"]=true;whiteList["text-decoration-color"]=true;whiteList["text-decoration-line"]=true;whiteList["text-decoration-skip"]=true;whiteList["text-decoration-style"]=true;whiteList["text-emphasis"]=true;whiteList["text-emphasis-color"]=true;whiteList["text-emphasis-position"]=true;whiteList["text-emphasis-style"]=true;whiteList["text-height"]=true;whiteList["text-indent"]=true;whiteList["text-justify"]=true;whiteList["text-orientation"]=true;whiteList["text-overflow"]=true;whiteList["text-shadow"]=true;whiteList["text-space-collapse"]=true;whiteList["text-transform"]=true;whiteList["text-underline-position"]=true;whiteList["text-wrap"]=true;whiteList["top"]=false;whiteList["transform"]=false;whiteList["transform-origin"]=false;whiteList["transform-style"]=false;whiteList["transition"]=false;whiteList["transition-delay"]=false;whiteList["transition-duration"]=false;whiteList["transition-property"]=false;whiteList["transition-timing-function"]=false;whiteList["unicode-bidi"]=false;whiteList["vertical-align"]=false;whiteList["visibility"]=false;whiteList["voice-balance"]=false;whiteList["voice-duration"]=false;whiteList["voice-family"]=false;whiteList["voice-pitch"]=false;whiteList["voice-range"]=false;whiteList["voice-rate"]=false;whiteList["voice-stress"]=false;whiteList["voice-volume"]=false;whiteList["volume"]=false;whiteList["white-space"]=false;whiteList["widows"]=false;whiteList["width"]=true;whiteList["will-change"]=false;whiteList["word-break"]=true;whiteList["word-spacing"]=true;whiteList["word-wrap"]=true;whiteList["wrap-flow"]=false;whiteList["wrap-through"]=false;whiteList["writing-mode"]=false;whiteList["z-index"]=false;return whiteList}function onAttr(name,value,options){}function onIgnoreAttr(name,value,options){}var REGEXP_URL_JAVASCRIPT=/javascript\s*\:/gim;function safeAttrValue(name,value){if(REGEXP_URL_JAVASCRIPT.test(value))return"";return value}exports.whiteList=getDefaultWhiteList();exports.getDefaultWhiteList=getDefaultWhiteList;exports.onAttr=onAttr;exports.onIgnoreAttr=onIgnoreAttr;exports.safeAttrValue=safeAttrValue},{}],8:[function(require,module,exports){var DEFAULT=require("./default");var FilterCSS=require("./css");function filterCSS(html,options){var xss=new FilterCSS(options);return xss.process(html)}exports=module.exports=filterCSS;exports.FilterCSS=FilterCSS;for(var i in DEFAULT)exports[i]=DEFAULT[i];if(typeof window!=="undefined"){window.filterCSS=module.exports}},{"./css":6,"./default":7}],9:[function(require,module,exports){var _=require("./util");function parseStyle(css,onAttr){css=_.trimRight(css);if(css[css.length-1]!==";")css+=";";var cssLength=css.length;var isParenthesisOpen=false;var lastPos=0;var i=0;var retCSS="";function addNewAttr(){if(!isParenthesisOpen){var source=_.trim(css.slice(lastPos,i));var j=source.indexOf(":");if(j!==-1){var name=_.trim(source.slice(0,j));var value=_.trim(source.slice(j+1));if(name){var ret=onAttr(lastPos,retCSS.length,name,value,source);if(ret)retCSS+=ret+"; "}}}lastPos=i+1}for(;i<cssLength;i++){var c=css[i];if(c==="/"&&css[i+1]==="*"){var j=css.indexOf("*/",i+2);if(j===-1)break;i=j+1;lastPos=i+1;isParenthesisOpen=false}else if(c==="("){isParenthesisOpen=true}else if(c===")"){isParenthesisOpen=false}else if(c===";"){if(isParenthesisOpen){}else{addNewAttr()}}else if(c==="\n"){addNewAttr()}}return _.trim(retCSS)}module.exports=parseStyle},{"./util":10}],10:[function(require,module,exports){module.exports={indexOf:function(arr,item){var i,j;if(Array.prototype.indexOf){return arr.indexOf(item)}for(i=0,j=arr.length;i<j;i++){if(arr[i]===item){return i}}return-1},forEach:function(arr,fn,scope){var i,j;if(Array.prototype.forEach){return arr.forEach(fn,scope)}for(i=0,j=arr.length;i<j;i++){fn.call(scope,arr[i],i,arr)}},trim:function(str){if(String.prototype.trim){return str.trim()}return str.replace(/(^\s*)|(\s*$)/g,"")},trimRight:function(str){if(String.prototype.trimRight){return str.trimRight()}return str.replace(/(\s*$)/g,"")}}},{}]},{},[2]); apollo-server-demo/node_modules/xss/dist/test.html 0000644 0001750 0000144 00000000510 03560116604 022051 0 ustar andreh users <!doctype html> <html> <head> <title>测试</title> <meta charset="utf8"> </head> <body> <pre id="result"></pre> </body> </html> <script src="xss.js"></script> <script> var code = '<script>alert("xss");</' + 'script>'; document.querySelector('#result').innerText = code + '\nè¢«è½¬æ¢æˆäº†\n' + filterXSS(code); </script>